CSCI 431 Programming Languages Fall 2002 Lecture 1
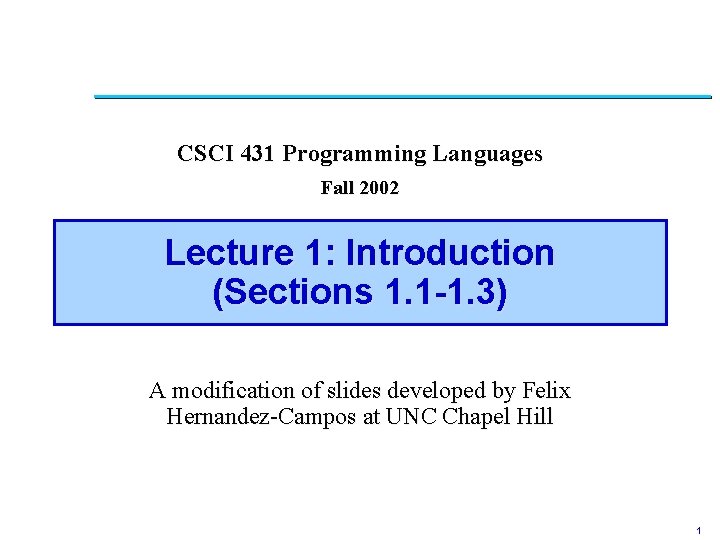
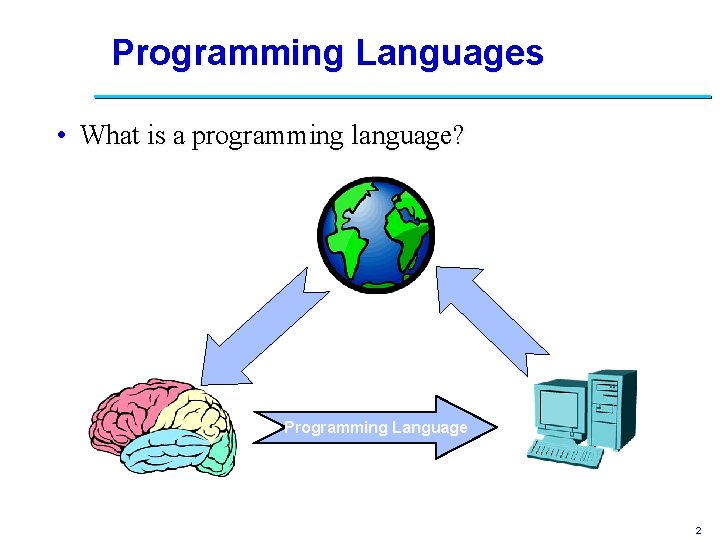
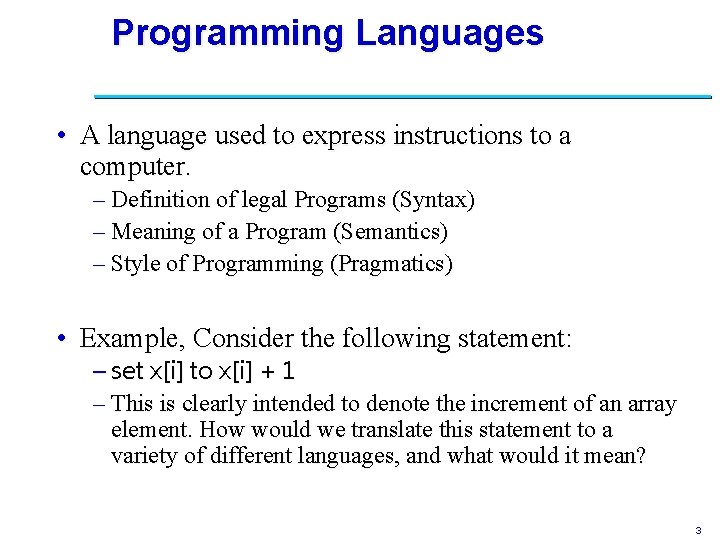
![In C (circa 1970), we would write this as x[i] = x[i] + 1; In C (circa 1970), we would write this as x[i] = x[i] + 1;](https://slidetodoc.com/presentation_image_h2/c0c77156547427c0b9e35c261be3a660/image-4.jpg)
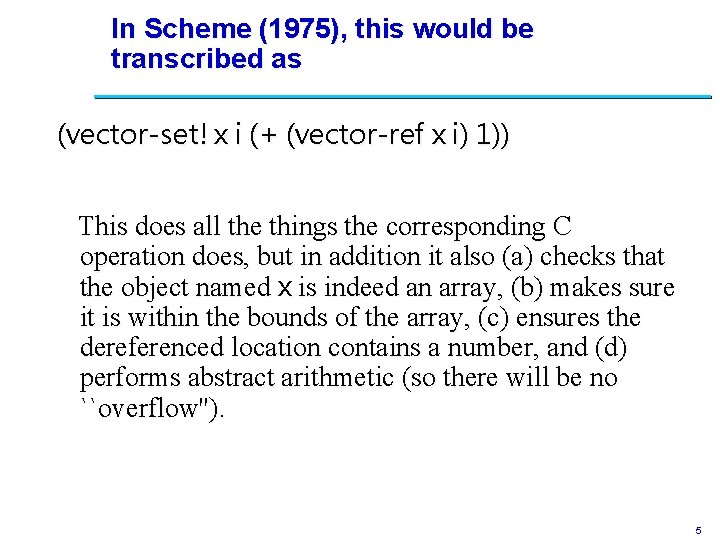
![Finally, in Java (circa 1991), one might write x[i] = x[i] + 1; which Finally, in Java (circa 1991), one might write x[i] = x[i] + 1; which](https://slidetodoc.com/presentation_image_h2/c0c77156547427c0b9e35c261be3a660/image-6.jpg)
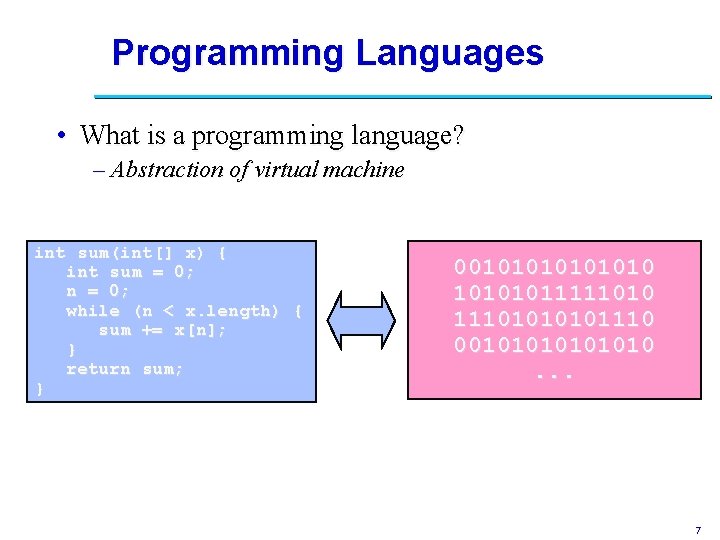
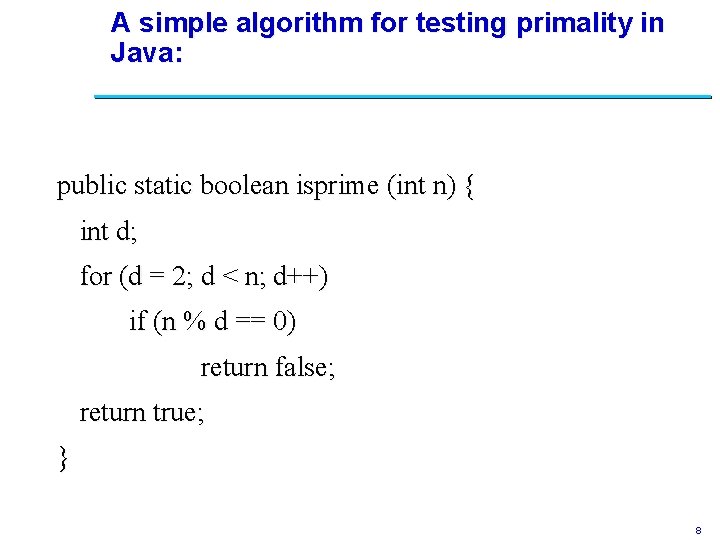
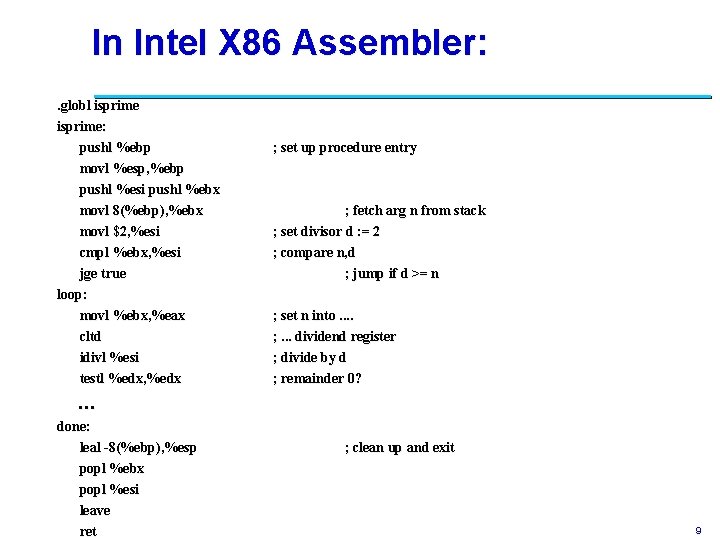
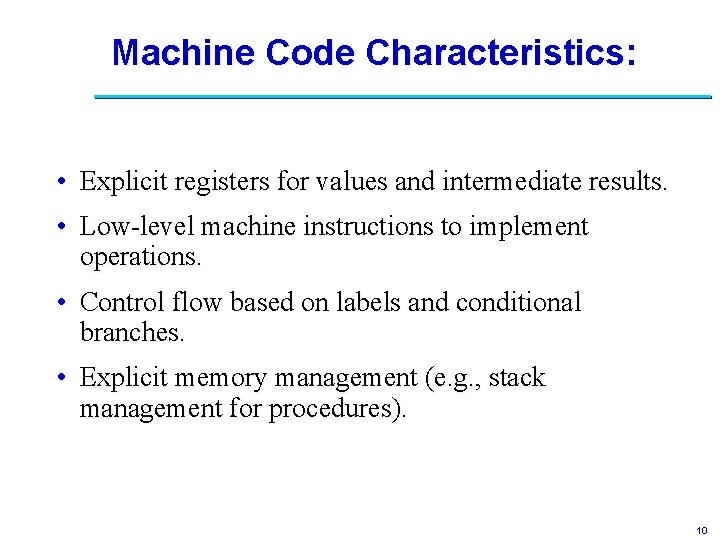
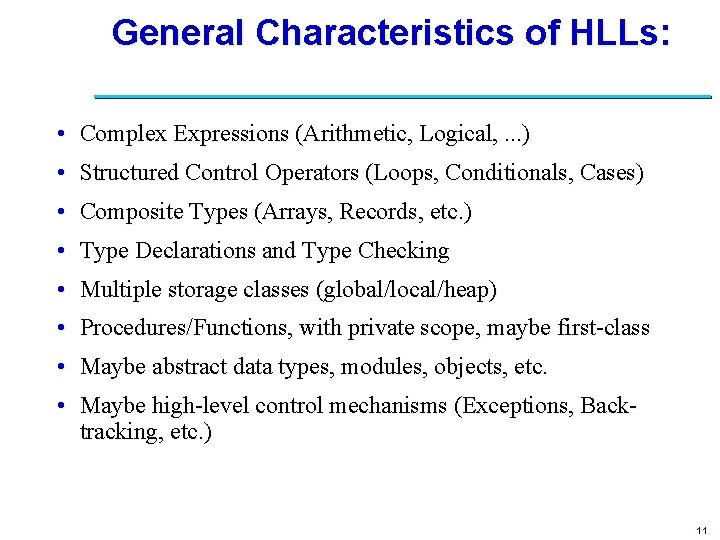
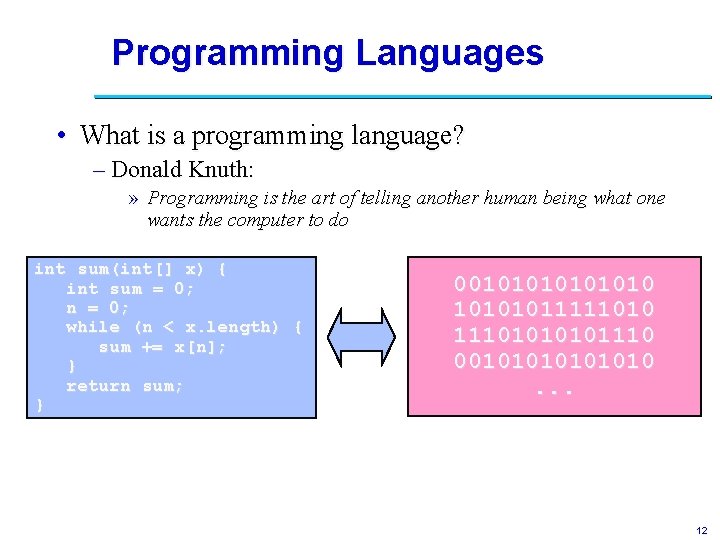
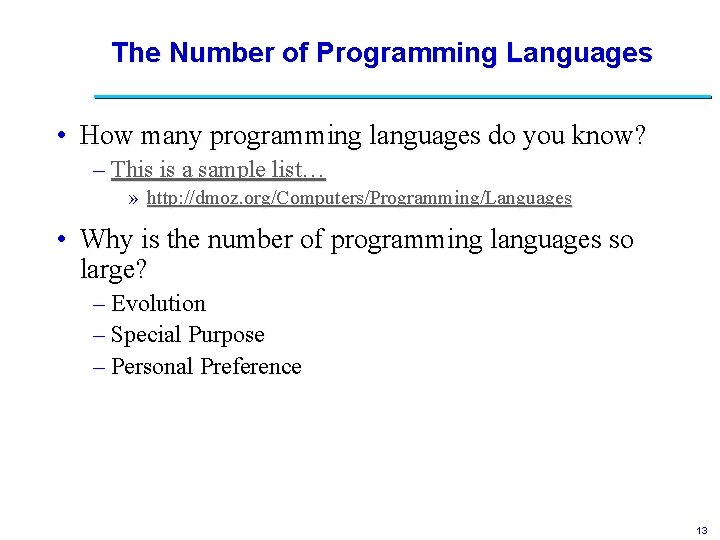
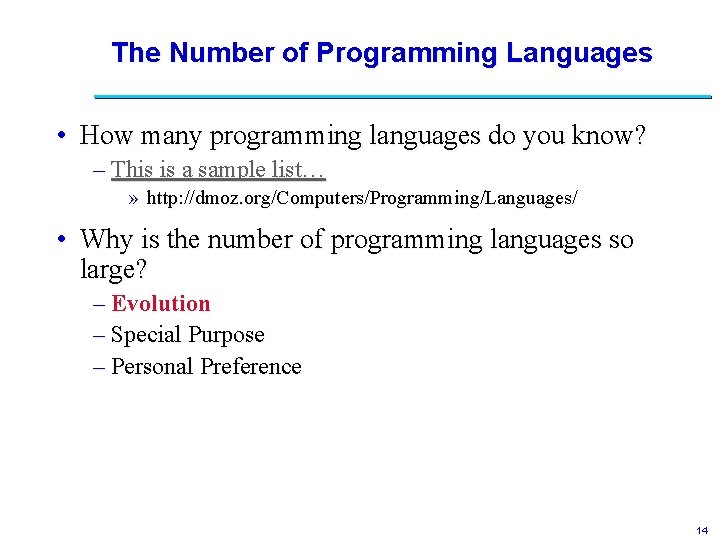
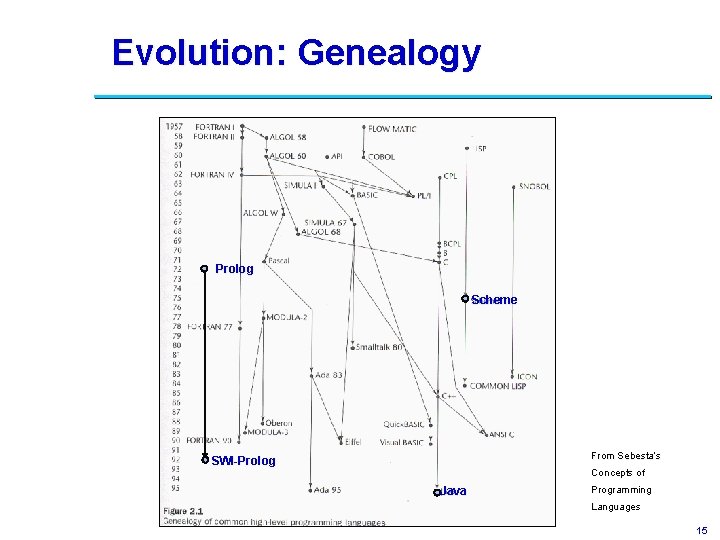
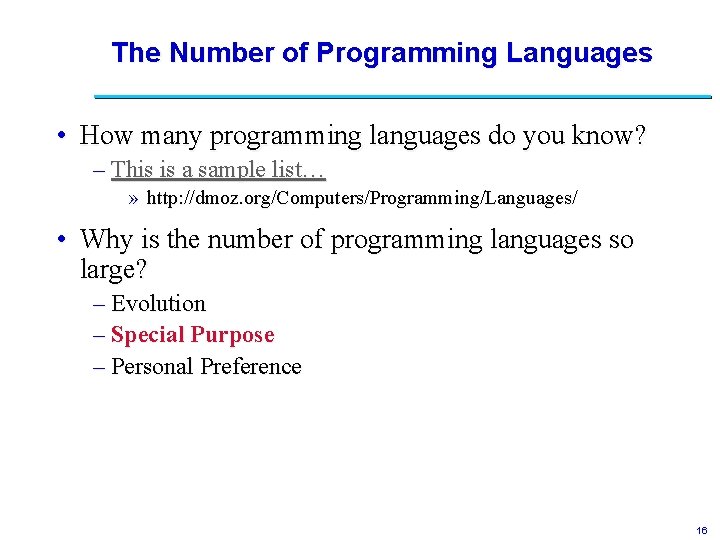
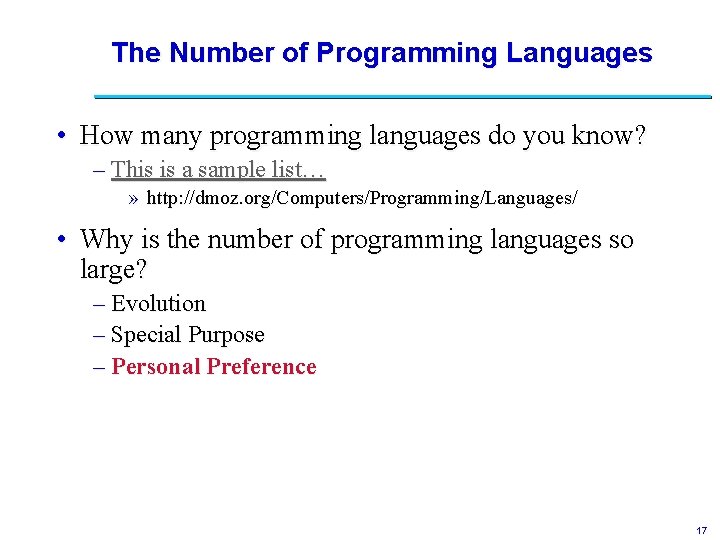
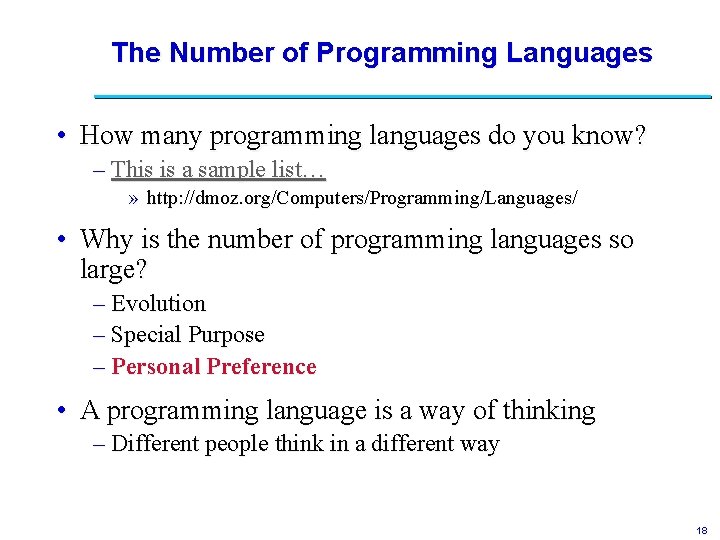
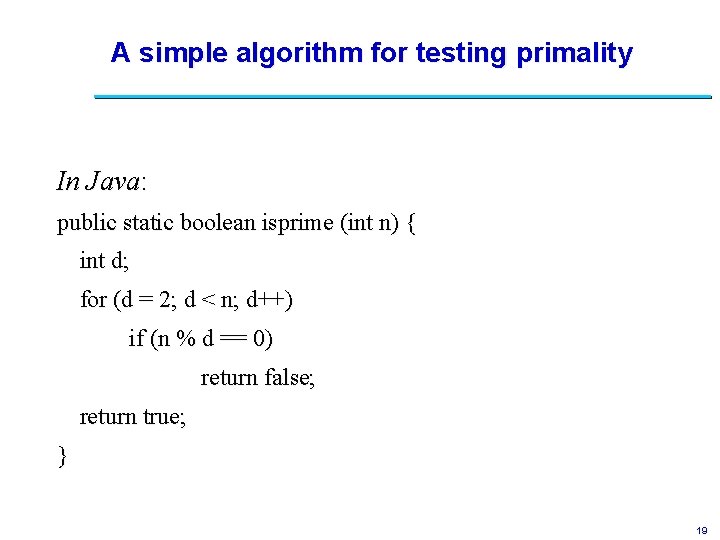
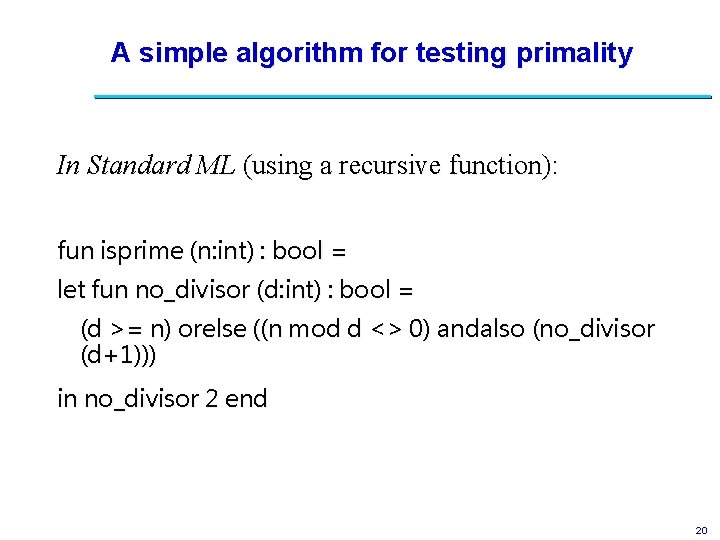
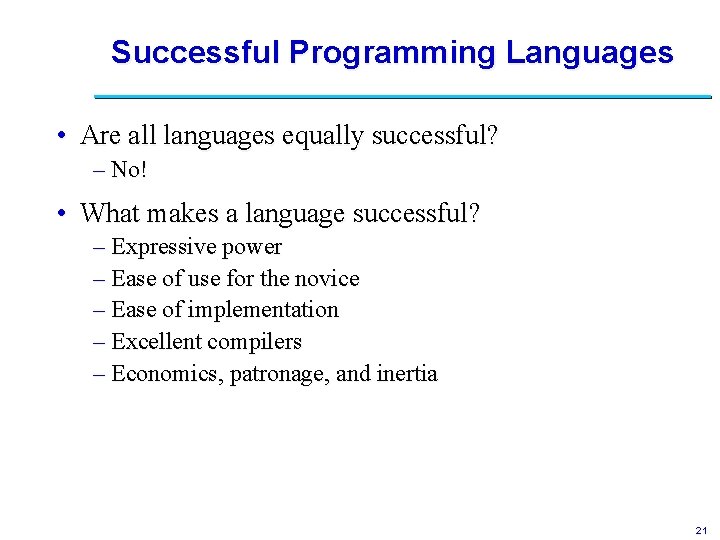
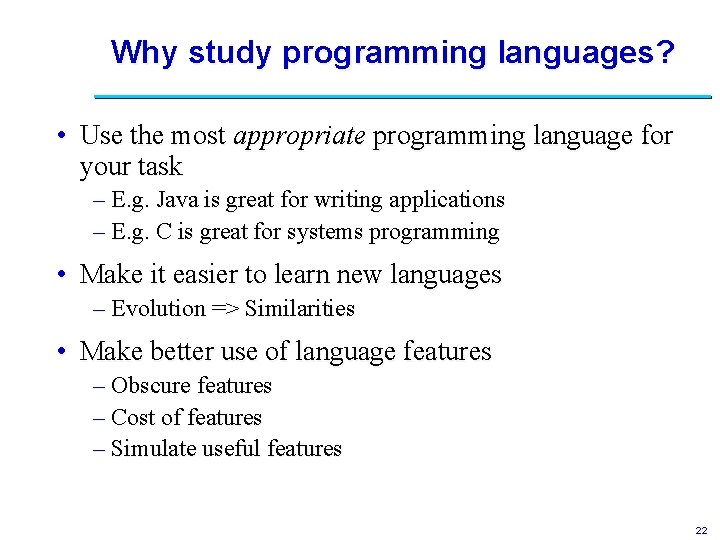
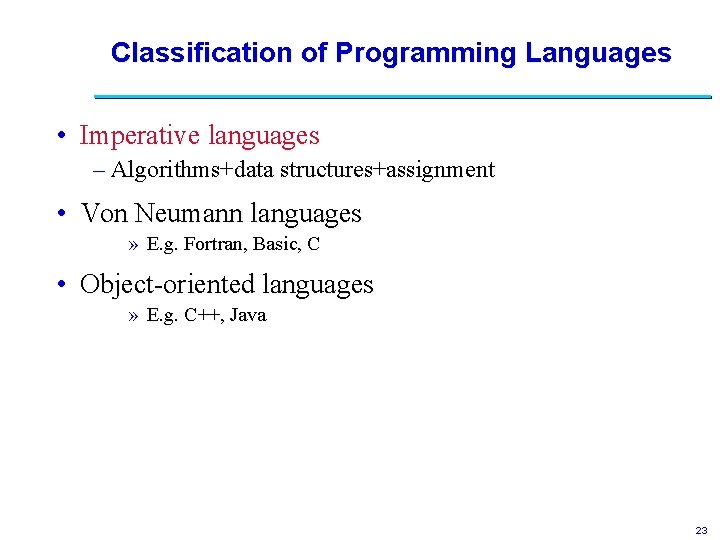
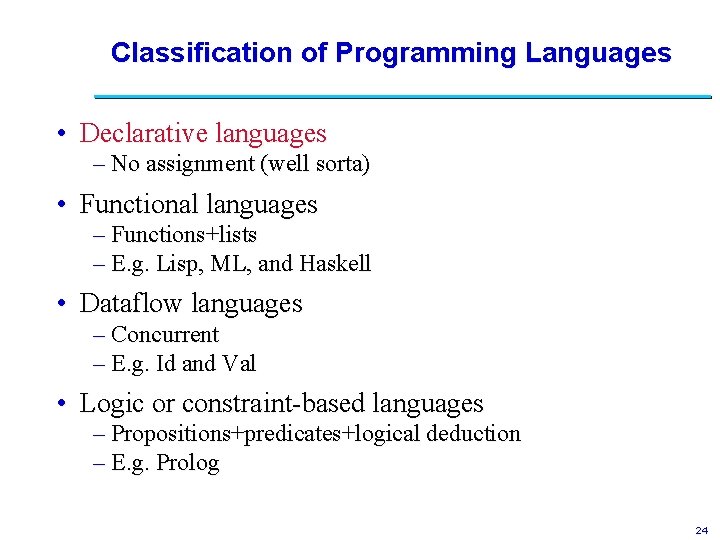
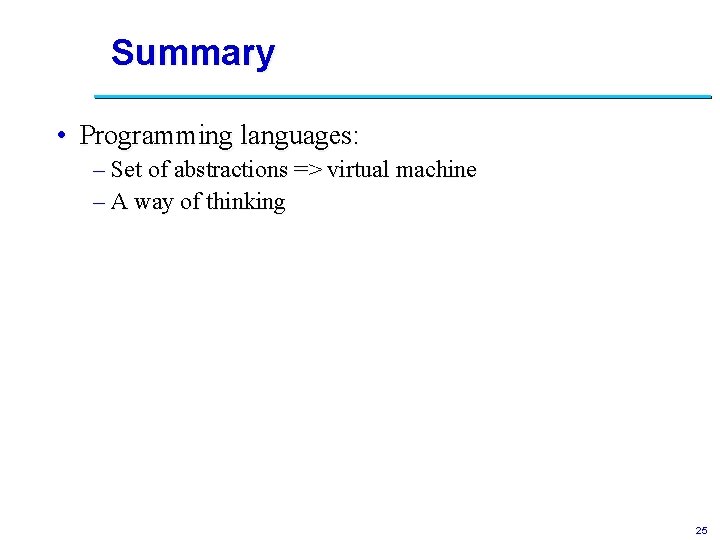
- Slides: 25
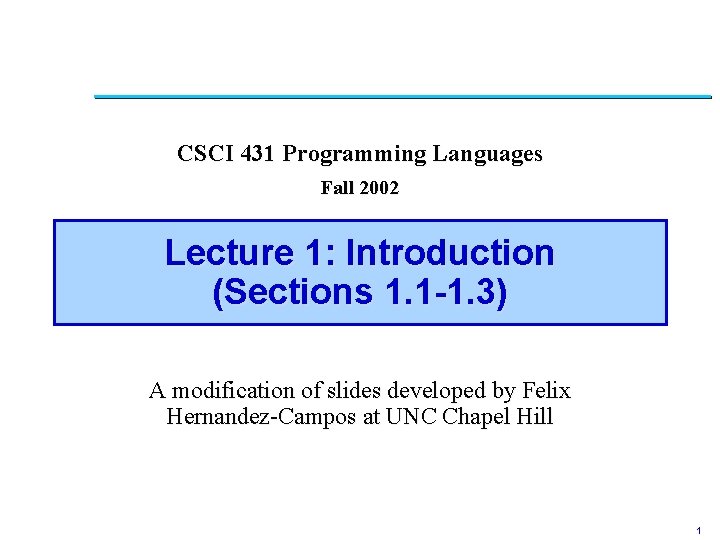
CSCI 431 Programming Languages Fall 2002 Lecture 1: Introduction (Sections 1. 1 -1. 3) A modification of slides developed by Felix Hernandez-Campos at UNC Chapel Hill 1
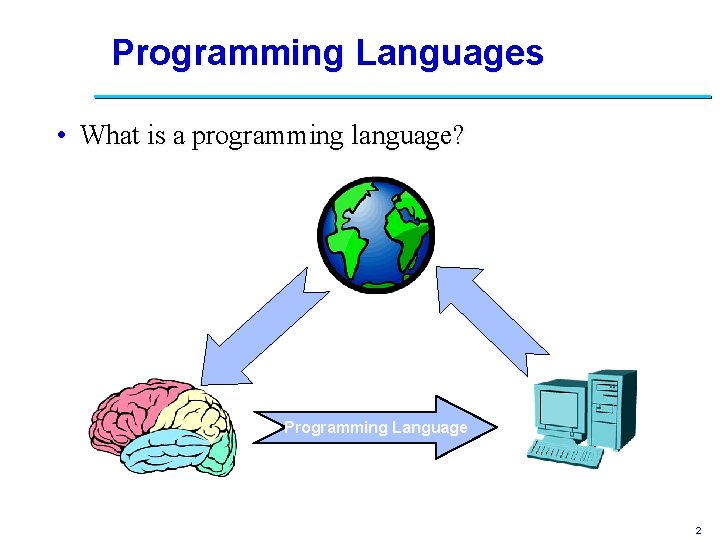
Programming Languages • What is a programming language? Programming Language 2
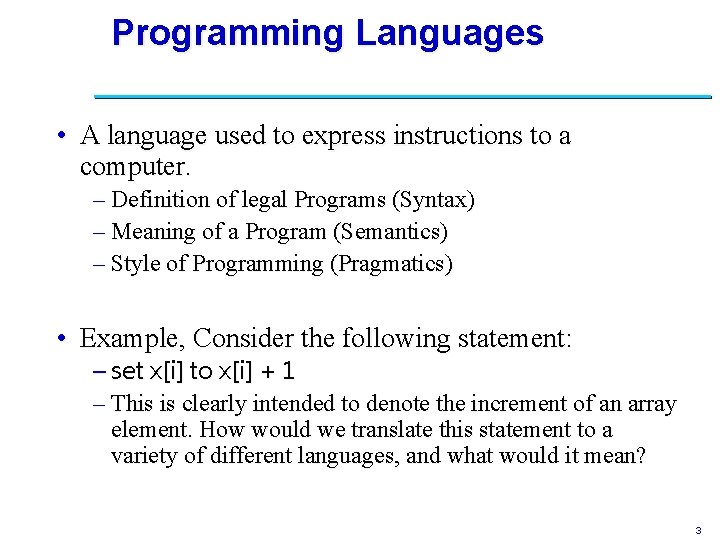
Programming Languages • A language used to express instructions to a computer. – Definition of legal Programs (Syntax) – Meaning of a Program (Semantics) – Style of Programming (Pragmatics) • Example, Consider the following statement: – set x[i] to x[i] + 1 – This is clearly intended to denote the increment of an array element. How would we translate this statement to a variety of different languages, and what would it mean? 3
![In C circa 1970 we would write this as xi xi 1 In C (circa 1970), we would write this as x[i] = x[i] + 1;](https://slidetodoc.com/presentation_image_h2/c0c77156547427c0b9e35c261be3a660/image-4.jpg)
In C (circa 1970), we would write this as x[i] = x[i] + 1; This performs a hardware lookup for the address of x and adds i to it. The addition is a hardware operation, so it is dependent upon the hardware in question. This resulting address is then referenced (if it's legal which it might not be), 1 is added to the bit-string stored there (again, as a hardware addition, which can overflow), and the result is stored back to that location. However, no attempt has been made to determine that x is even a vector and that x[i] is a number. 4
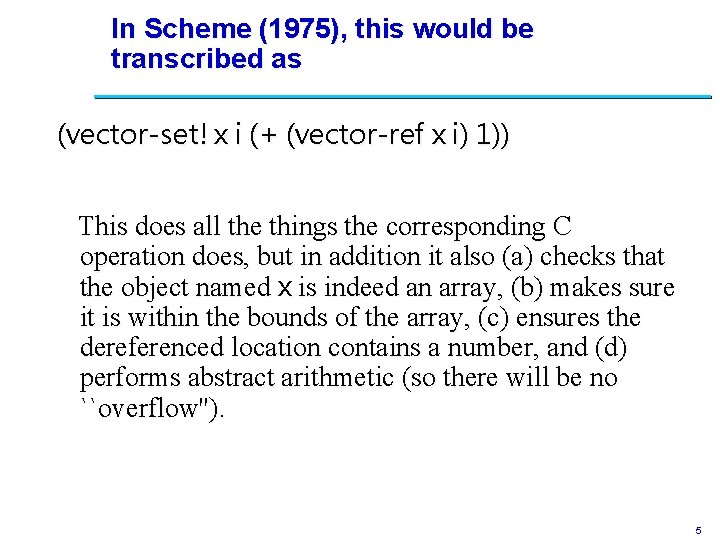
In Scheme (1975), this would be transcribed as (vector-set! x i (+ (vector-ref x i) 1)) This does all the things the corresponding C operation does, but in addition it also (a) checks that the object named x is indeed an array, (b) makes sure it is within the bounds of the array, (c) ensures the dereferenced location contains a number, and (d) performs abstract arithmetic (so there will be no ``overflow''). 5
![Finally in Java circa 1991 one might write xi xi 1 which Finally, in Java (circa 1991), one might write x[i] = x[i] + 1; which](https://slidetodoc.com/presentation_image_h2/c0c77156547427c0b9e35c261be3a660/image-6.jpg)
Finally, in Java (circa 1991), one might write x[i] = x[i] + 1; which looks identical to the C code. However, the actions performed are those performed by the Scheme code, with one major difference: the arithmetic is not as abstract. It is defined to be done as if the machine were a 32 -bit machine, which means we can always determine the result of an operation, no matter which machine we execute the program on, but we cannot have our numbers grow arbitrarily large. 6
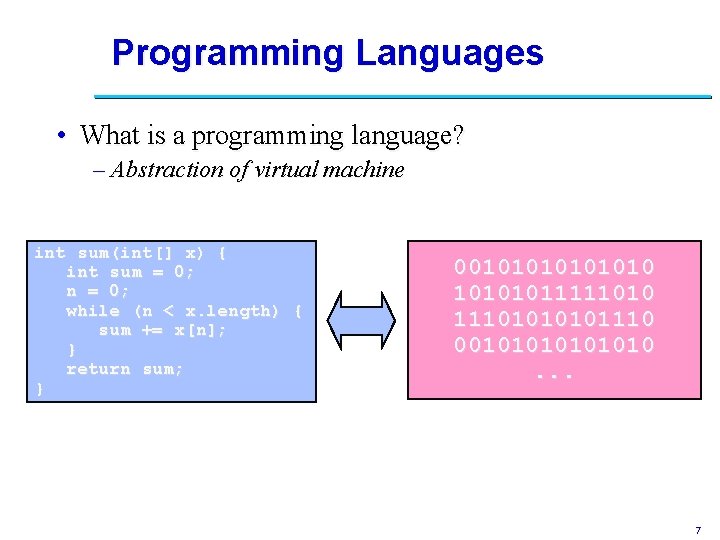
Programming Languages • What is a programming language? – Abstraction of virtual machine int sum(int[] x) { int sum = 0; n = 0; while (n < x. length) { sum += x[n]; } return sum; } 00101010111110101110 00101010. . . 7
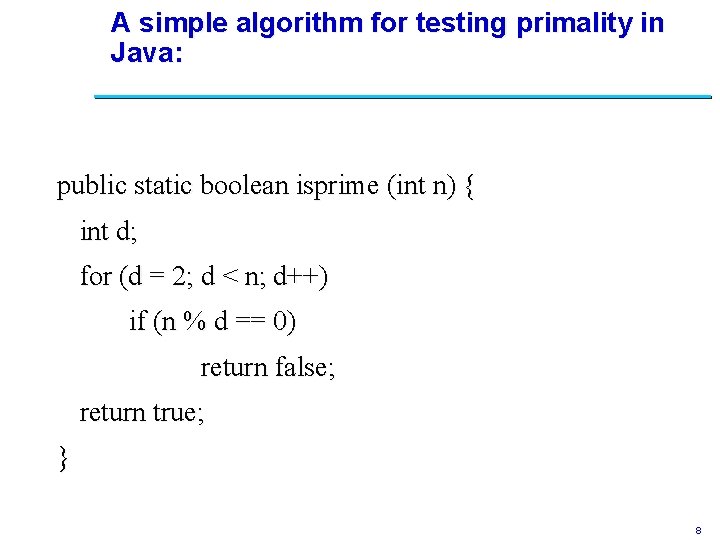
A simple algorithm for testing primality in Java: public static boolean isprime (int n) { int d; for (d = 2; d < n; d++) if (n % d == 0) return false; return true; } 8
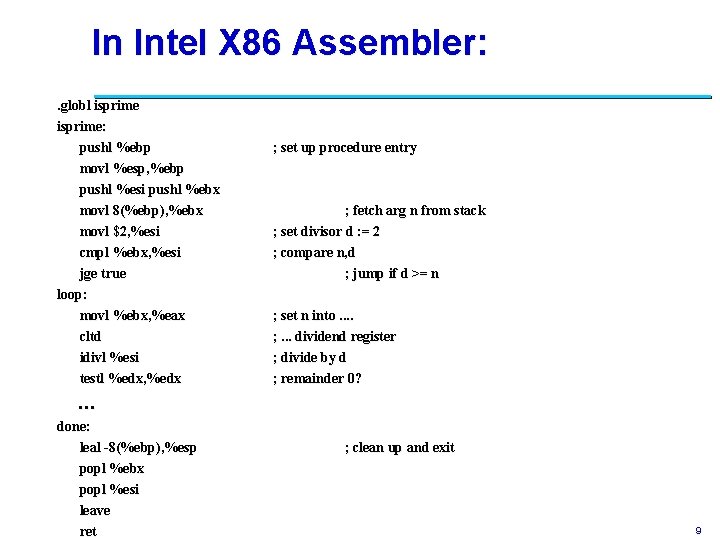
In Intel X 86 Assembler: . globl isprime: pushl %ebp movl %esp, %ebp pushl %esi pushl %ebx movl 8(%ebp), %ebx movl $2, %esi cmpl %ebx, %esi jge true loop: movl %ebx, %eax cltd idivl %esi testl %edx, %edx ; set up procedure entry ; fetch arg n from stack ; set divisor d : = 2 ; compare n, d ; jump if d >= n ; set n into. . ; . . . dividend register ; divide by d ; remainder 0? … done: leal -8(%ebp), %esp popl %ebx popl %esi leave ret ; clean up and exit 9
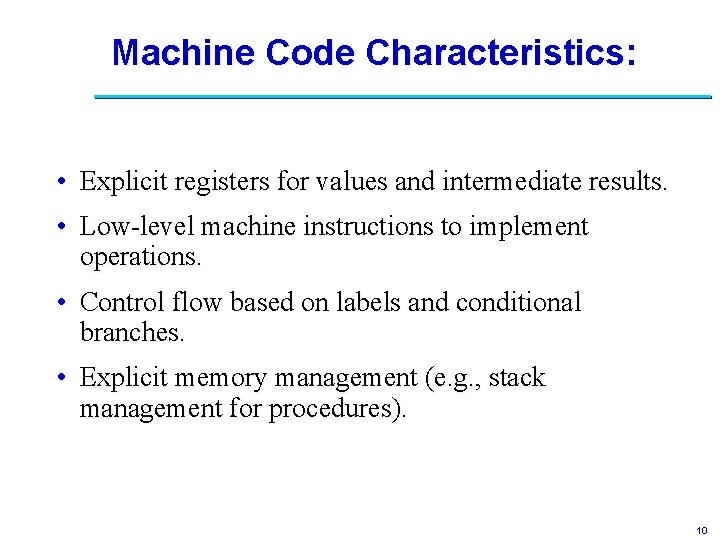
Machine Code Characteristics: • Explicit registers for values and intermediate results. • Low-level machine instructions to implement operations. • Control flow based on labels and conditional branches. • Explicit memory management (e. g. , stack management for procedures). 10
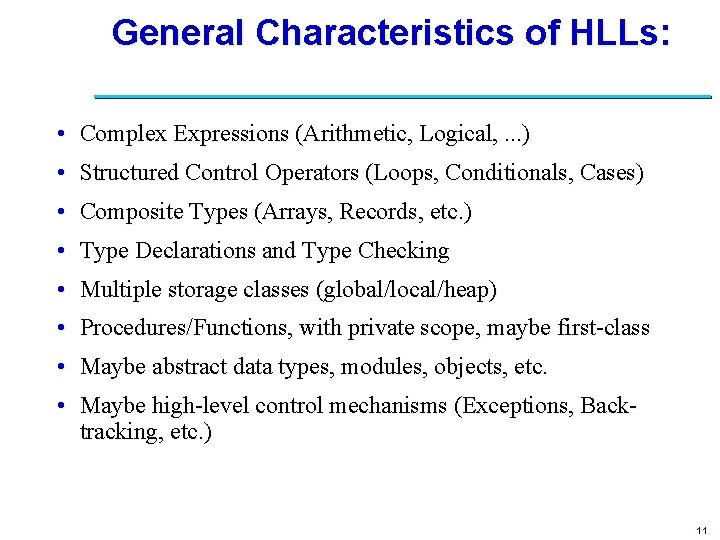
General Characteristics of HLLs: • Complex Expressions (Arithmetic, Logical, . . . ) • Structured Control Operators (Loops, Conditionals, Cases) • Composite Types (Arrays, Records, etc. ) • Type Declarations and Type Checking • Multiple storage classes (global/local/heap) • Procedures/Functions, with private scope, maybe first-class • Maybe abstract data types, modules, objects, etc. • Maybe high-level control mechanisms (Exceptions, Backtracking, etc. ) 11
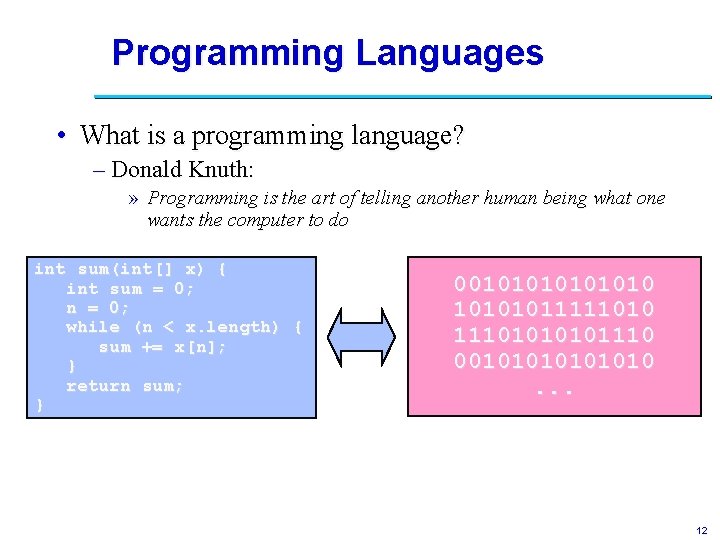
Programming Languages • What is a programming language? – Donald Knuth: » Programming is the art of telling another human being what one wants the computer to do int sum(int[] x) { int sum = 0; n = 0; while (n < x. length) { sum += x[n]; } return sum; } 00101010111110101110 00101010. . . 12
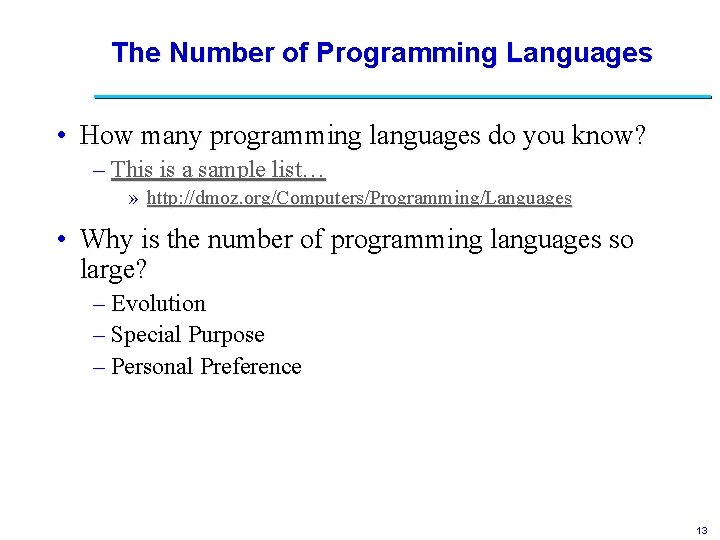
The Number of Programming Languages • How many programming languages do you know? – This is a sample list… » http: //dmoz. org/Computers/Programming/Languages • Why is the number of programming languages so large? – Evolution – Special Purpose – Personal Preference 13
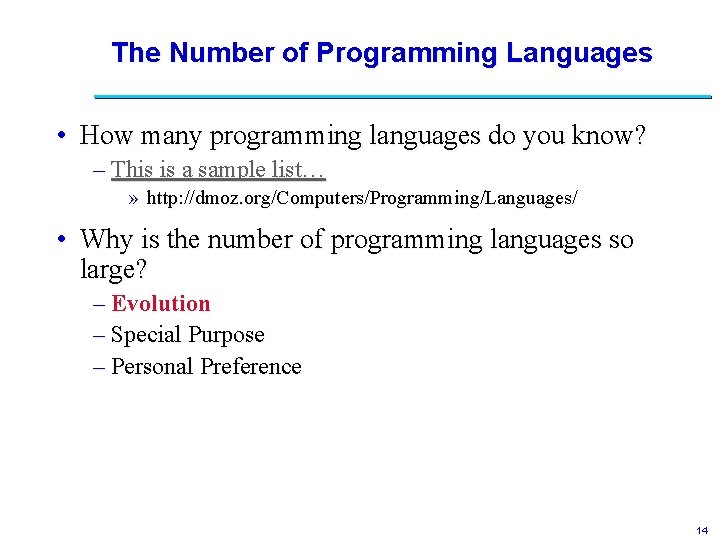
The Number of Programming Languages • How many programming languages do you know? – This is a sample list… » http: //dmoz. org/Computers/Programming/Languages/ • Why is the number of programming languages so large? – Evolution – Special Purpose – Personal Preference 14
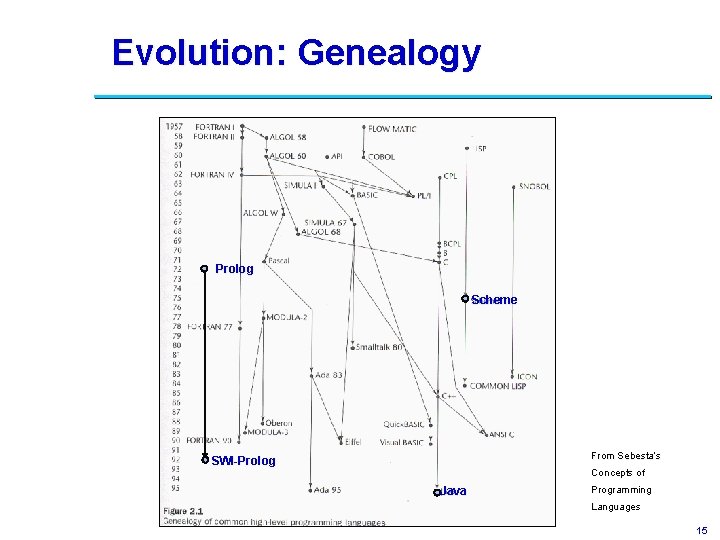
Evolution: Genealogy Prolog Scheme From Sebesta’s SWI-Prolog Concepts of Java Programming Languages 15
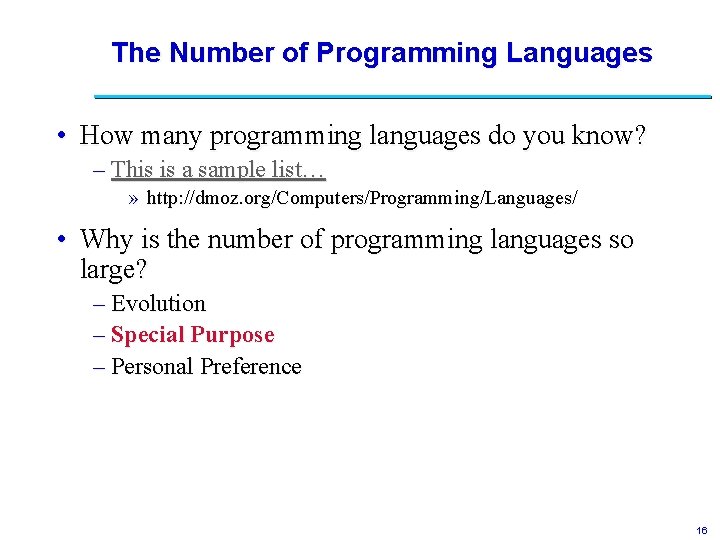
The Number of Programming Languages • How many programming languages do you know? – This is a sample list… » http: //dmoz. org/Computers/Programming/Languages/ • Why is the number of programming languages so large? – Evolution – Special Purpose – Personal Preference 16
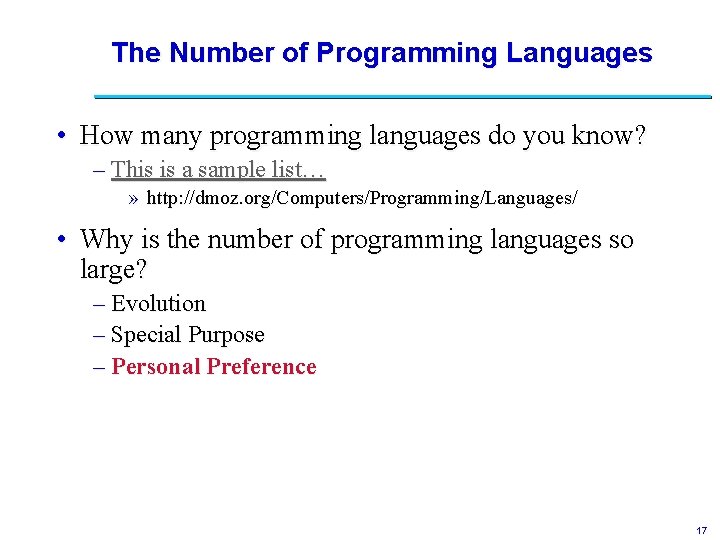
The Number of Programming Languages • How many programming languages do you know? – This is a sample list… » http: //dmoz. org/Computers/Programming/Languages/ • Why is the number of programming languages so large? – Evolution – Special Purpose – Personal Preference 17
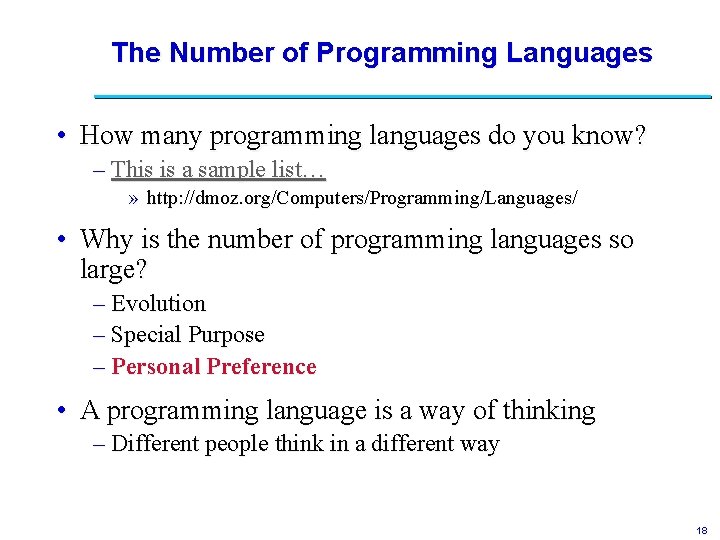
The Number of Programming Languages • How many programming languages do you know? – This is a sample list… » http: //dmoz. org/Computers/Programming/Languages/ • Why is the number of programming languages so large? – Evolution – Special Purpose – Personal Preference • A programming language is a way of thinking – Different people think in a different way 18
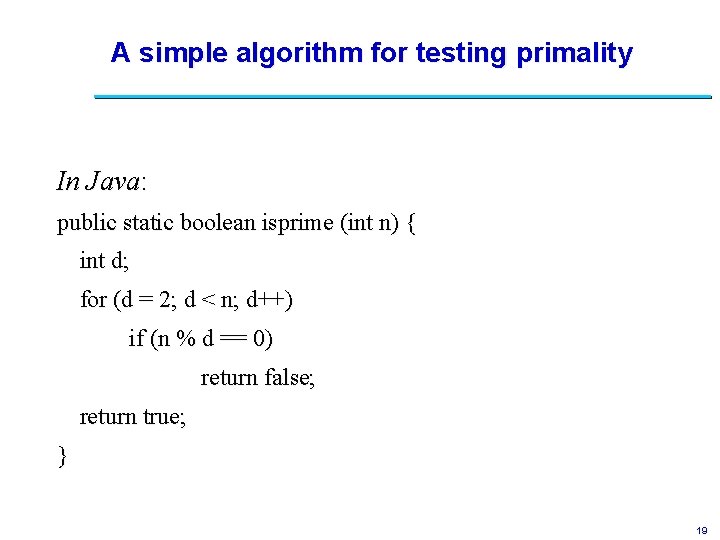
A simple algorithm for testing primality In Java: public static boolean isprime (int n) { int d; for (d = 2; d < n; d++) if (n % d == 0) return false; return true; } 19
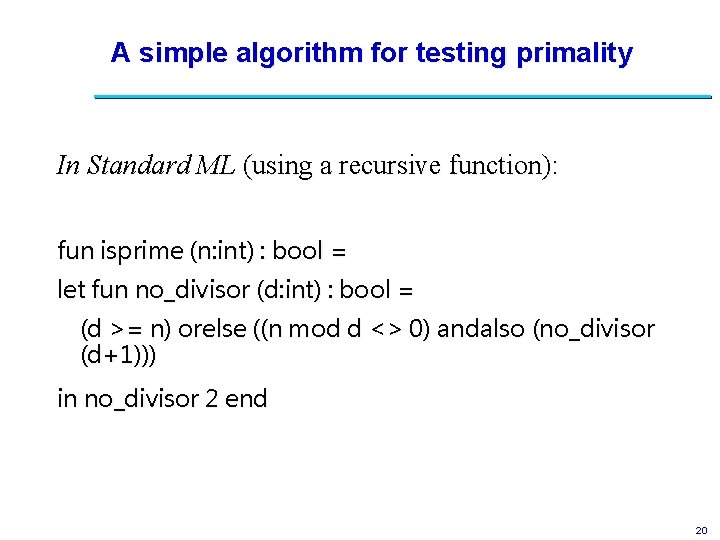
A simple algorithm for testing primality In Standard ML (using a recursive function): fun isprime (n: int) : bool = let fun no_divisor (d: int) : bool = (d >= n) orelse ((n mod d <> 0) andalso (no_divisor (d+1))) in no_divisor 2 end 20
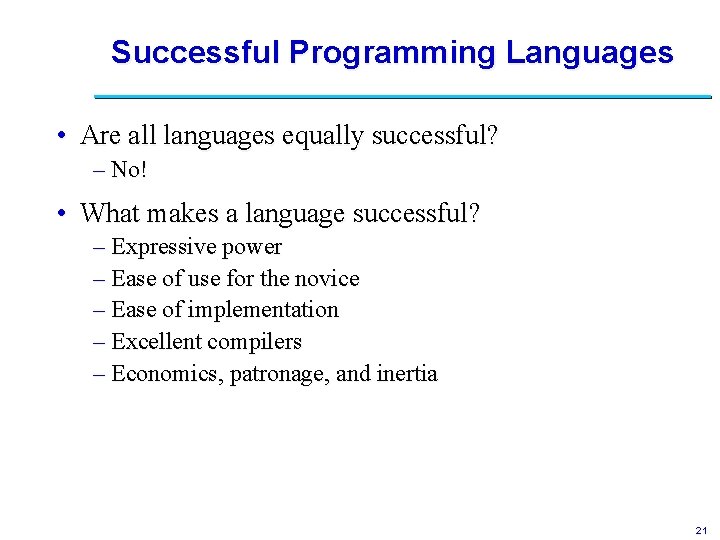
Successful Programming Languages • Are all languages equally successful? – No! • What makes a language successful? – Expressive power – Ease of use for the novice – Ease of implementation – Excellent compilers – Economics, patronage, and inertia 21
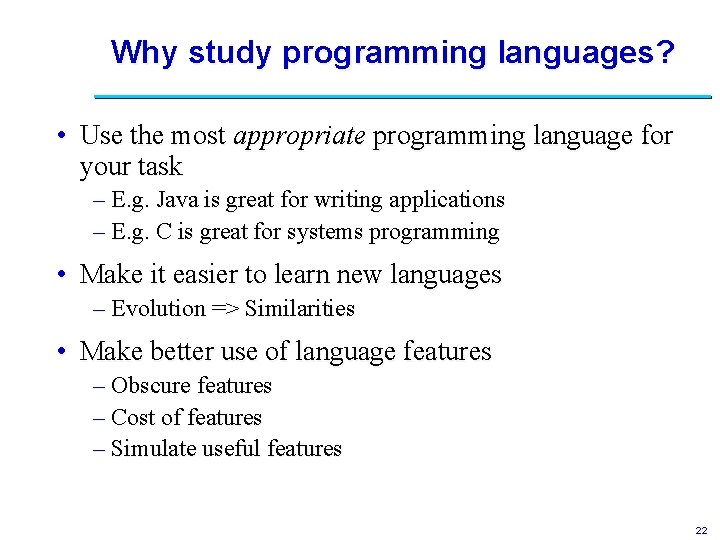
Why study programming languages? • Use the most appropriate programming language for your task – E. g. Java is great for writing applications – E. g. C is great for systems programming • Make it easier to learn new languages – Evolution => Similarities • Make better use of language features – Obscure features – Cost of features – Simulate useful features 22
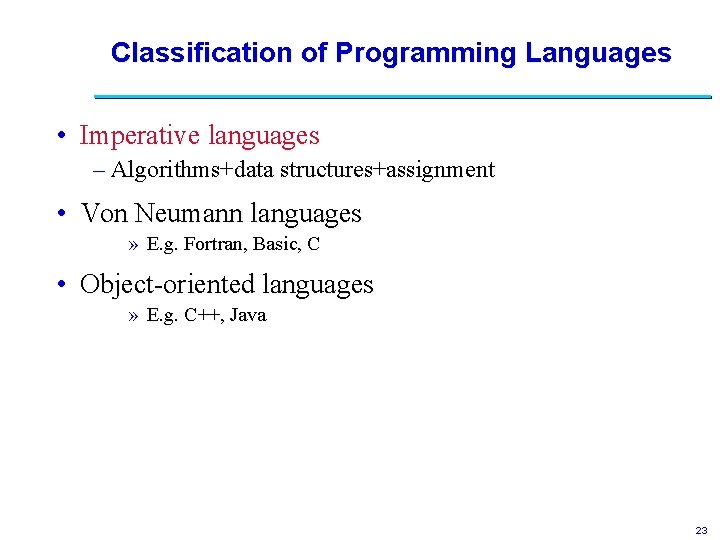
Classification of Programming Languages • Imperative languages – Algorithms+data structures+assignment • Von Neumann languages » E. g. Fortran, Basic, C • Object-oriented languages » E. g. C++, Java 23
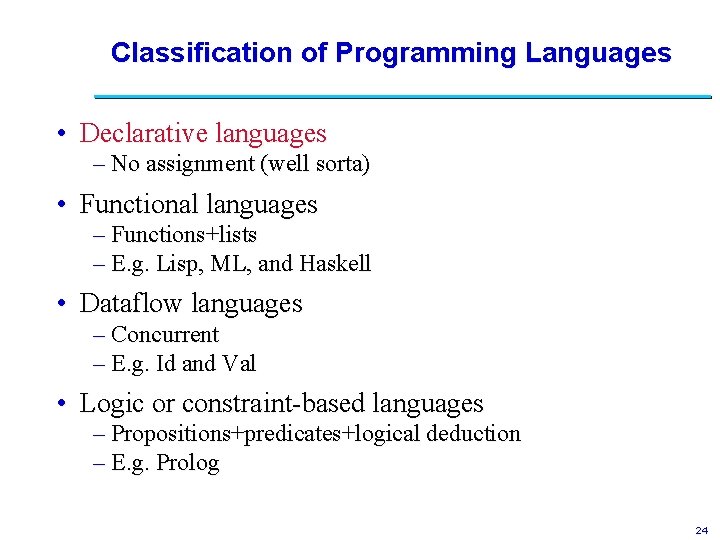
Classification of Programming Languages • Declarative languages – No assignment (well sorta) • Functional languages – Functions+lists – E. g. Lisp, ML, and Haskell • Dataflow languages – Concurrent – E. g. Id and Val • Logic or constraint-based languages – Propositions+predicates+logical deduction – E. g. Prolog 24
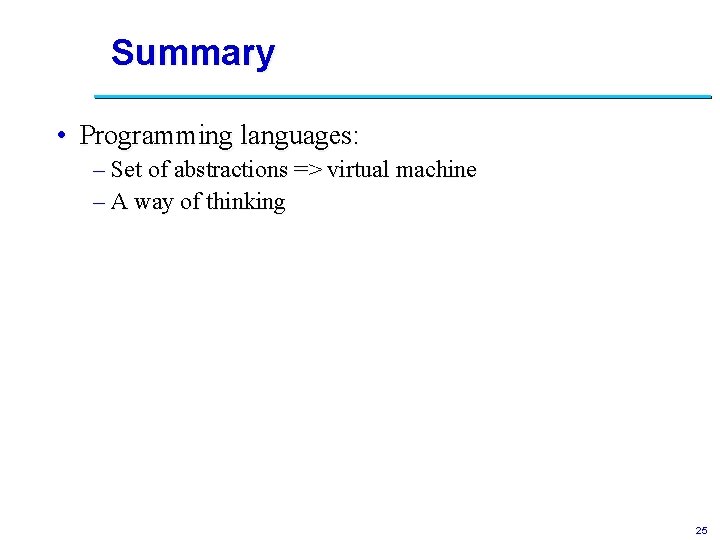
Summary • Programming languages: – Set of abstractions => virtual machine – A way of thinking 25
01:640:244 lecture notes - lecture 15: plat, idah, farad
Integral data types
Storage management in programming languages
Real-time systems and programming languages
Plc programming languages
Programing languages
The art of programming
Cornell programming languages
Types of programming languages
Language
Multithreading program in java
Advantages of application software
Xenia programming languages
Xkcd functional programming
Procedural programming languages
Programming languages
Transmission programming languages
Cs 421 uiuc
Cxc it
Brief history of programming languages
Mainstream programming languages
If programming languages were cars
Comparative programming languages
Low level linux programming
Programming languages
Real-time systems and programming languages