Computing Fundamentals with Java 9 1 Rick Mercer
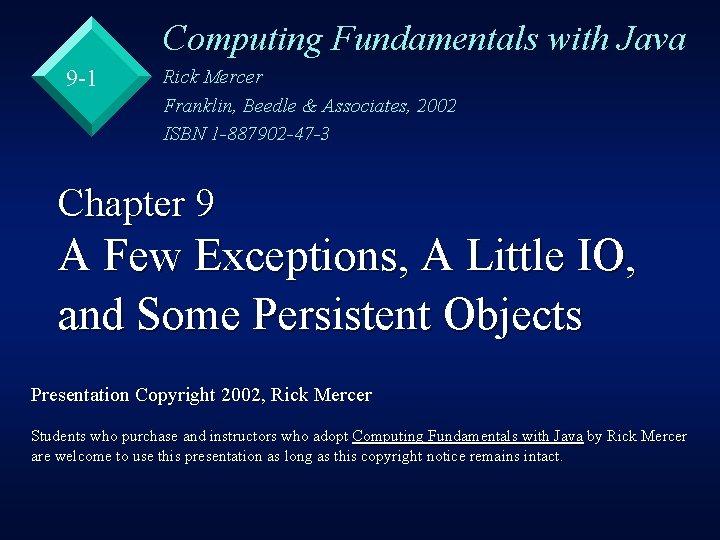
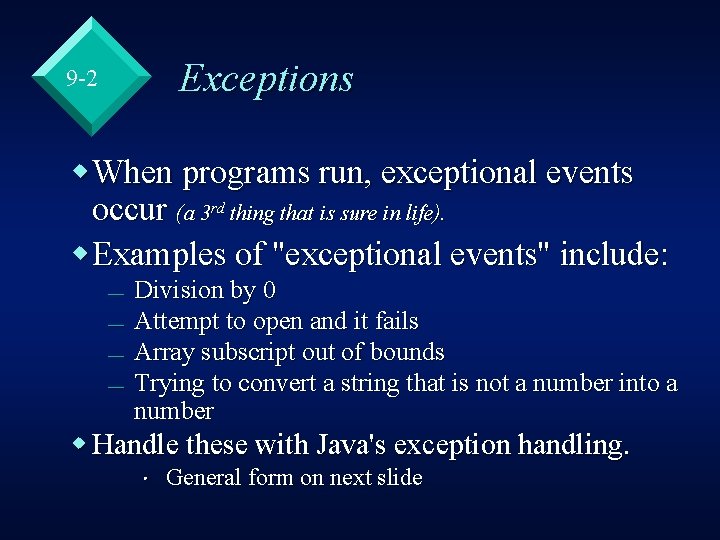
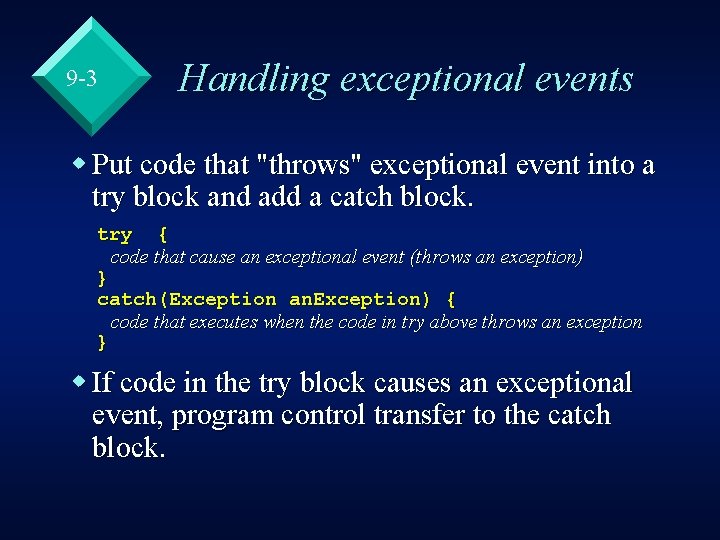
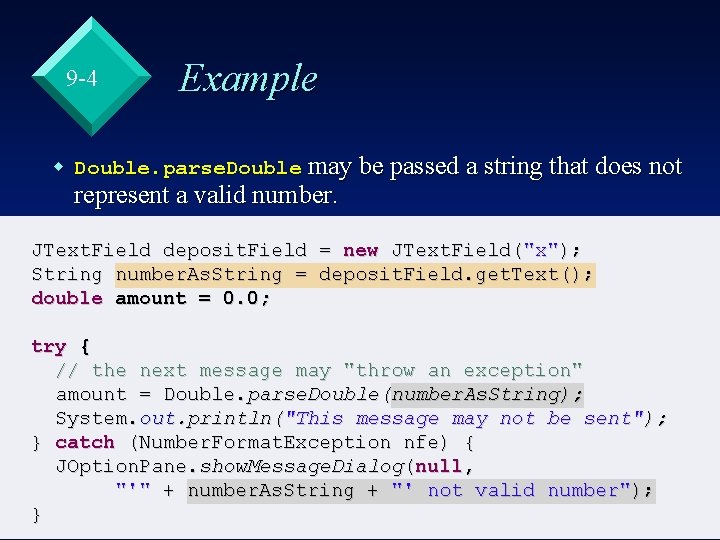
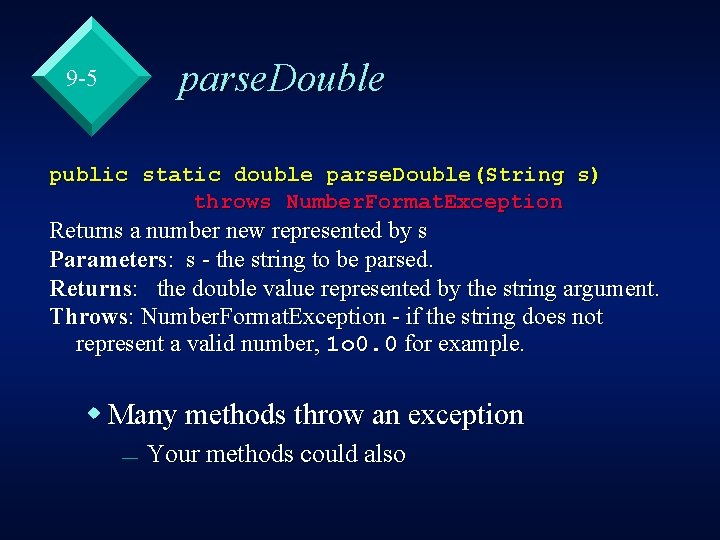
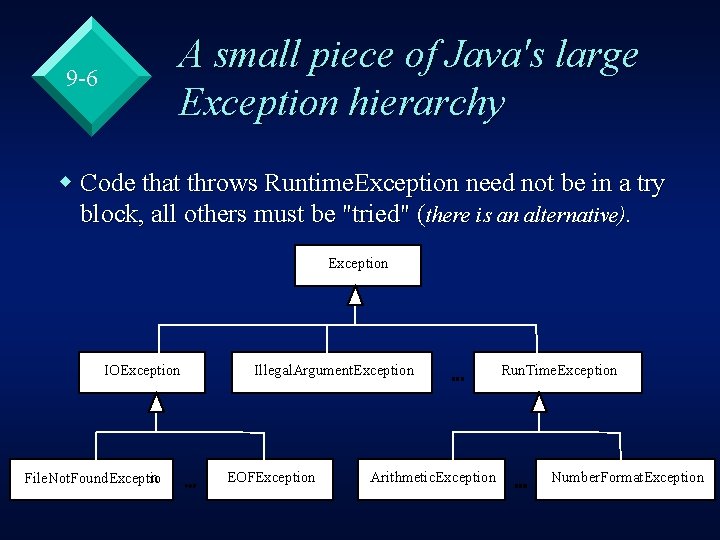
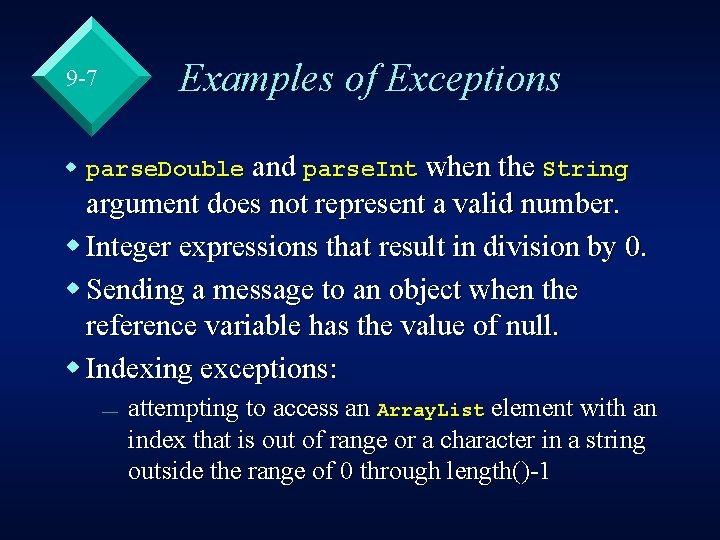
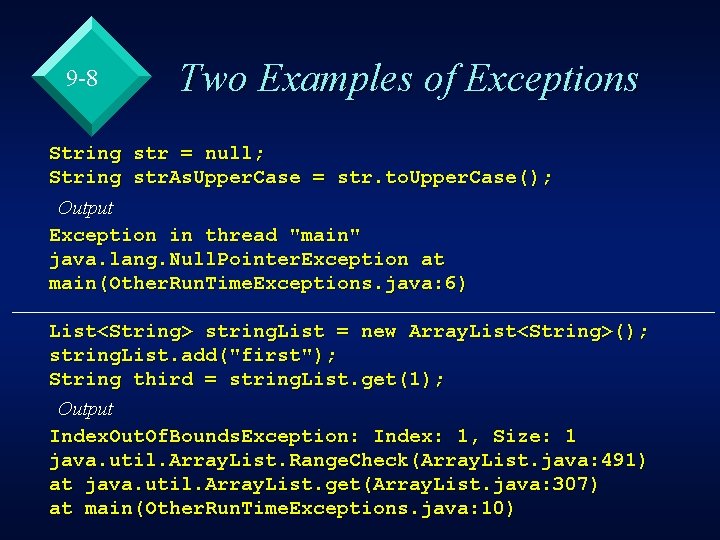
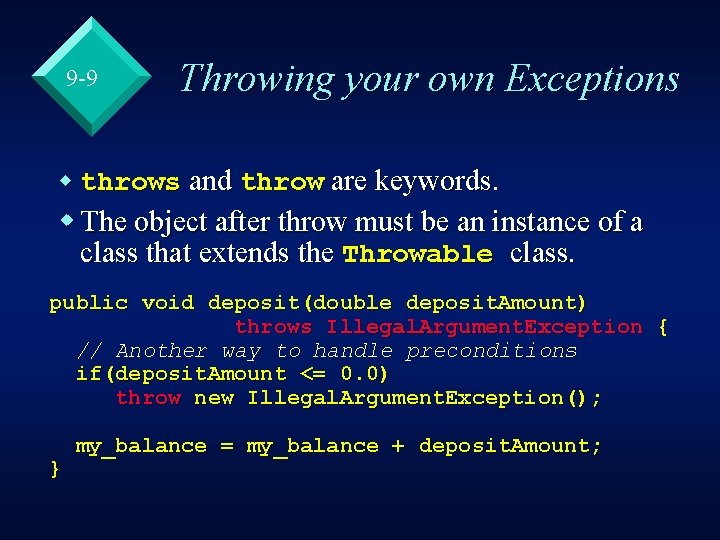
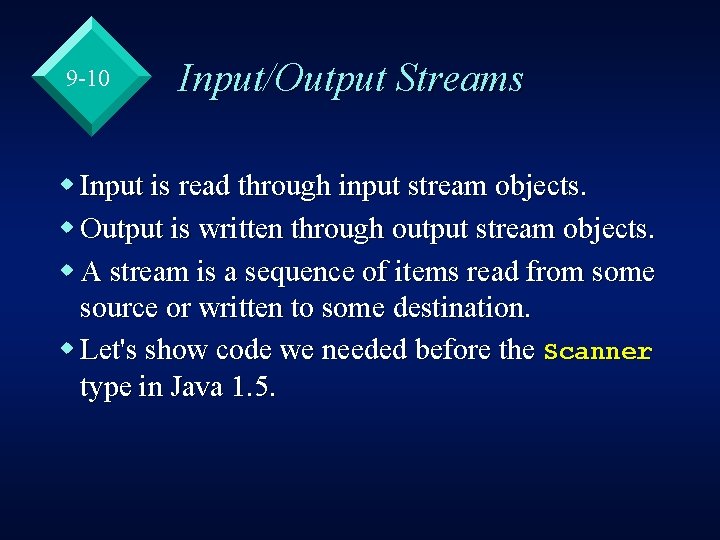
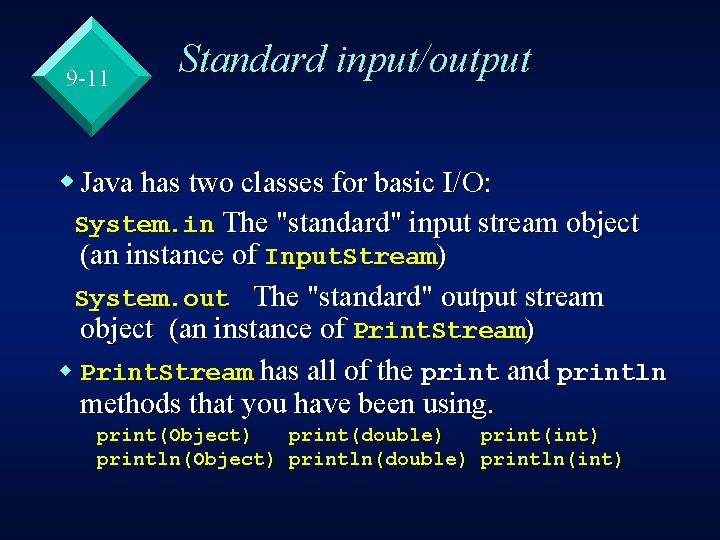
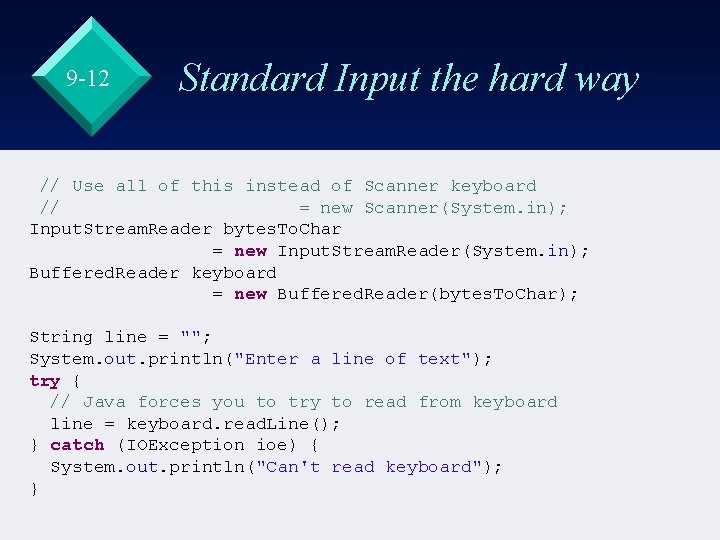
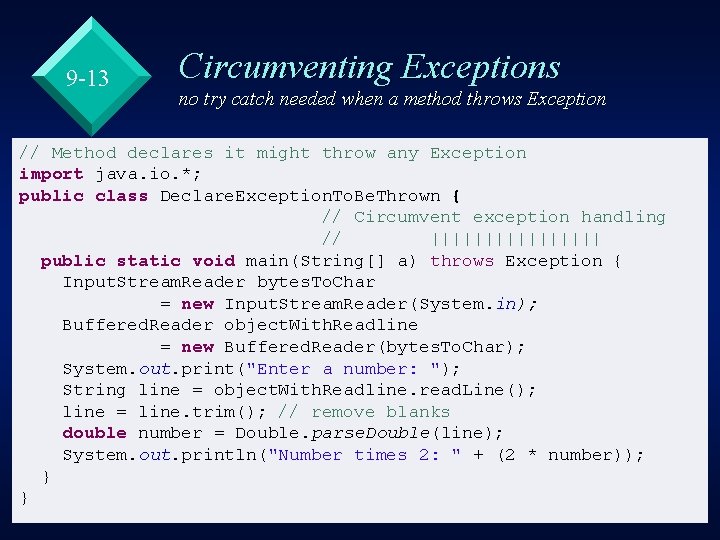
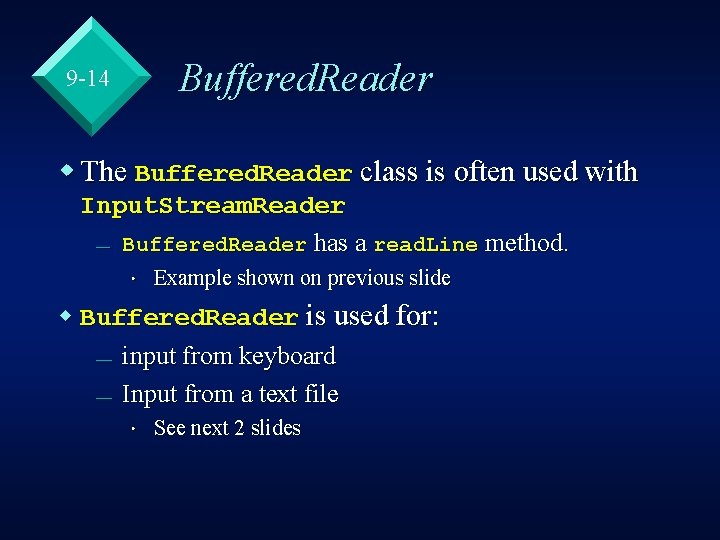
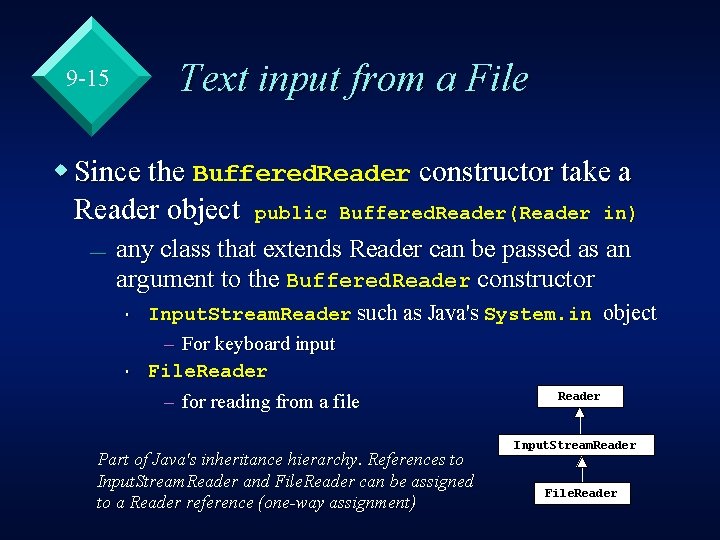
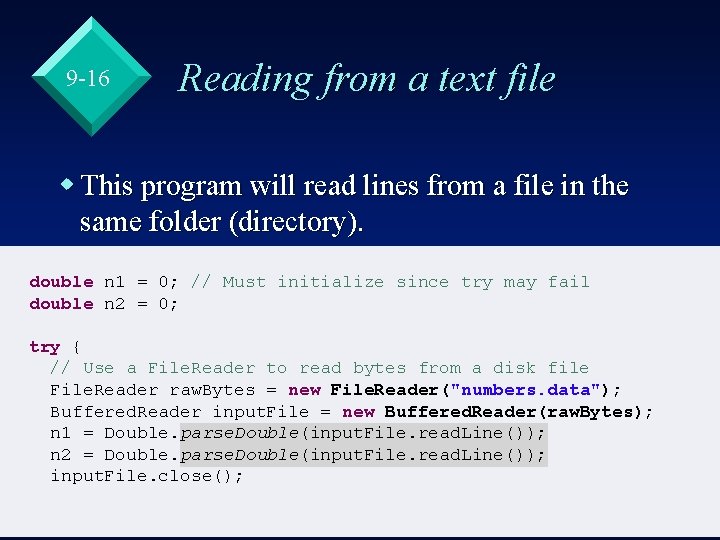
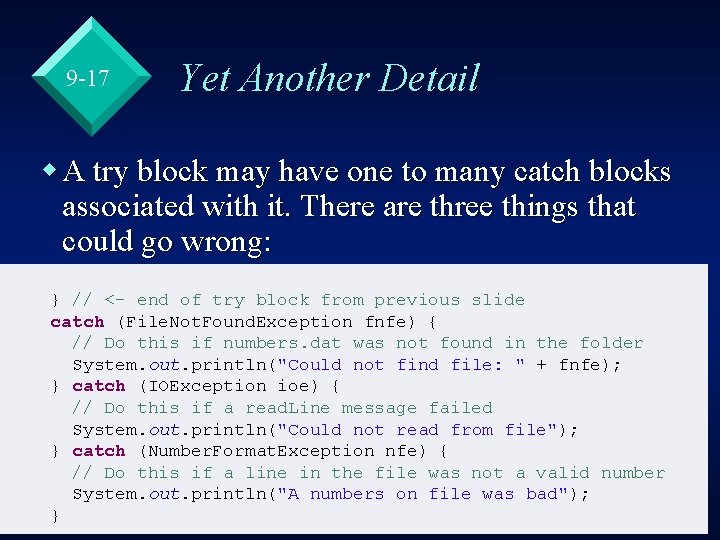
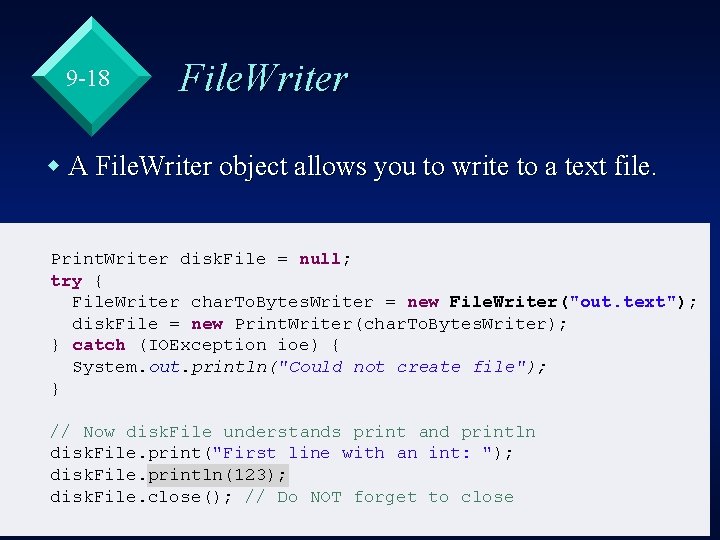
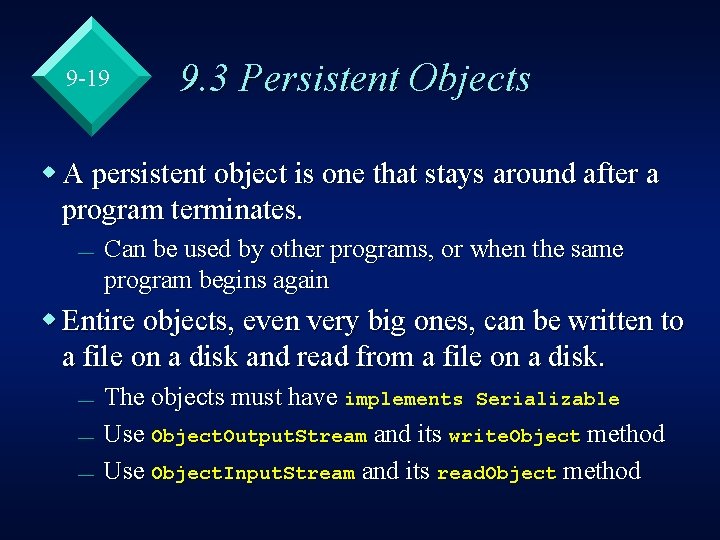
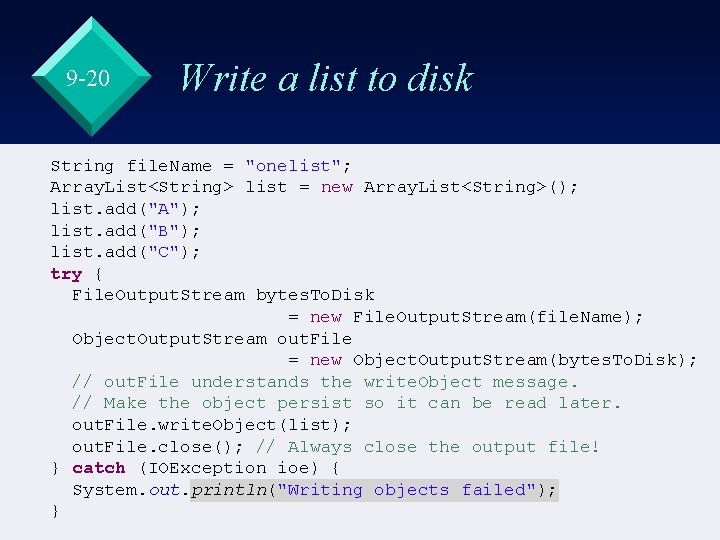
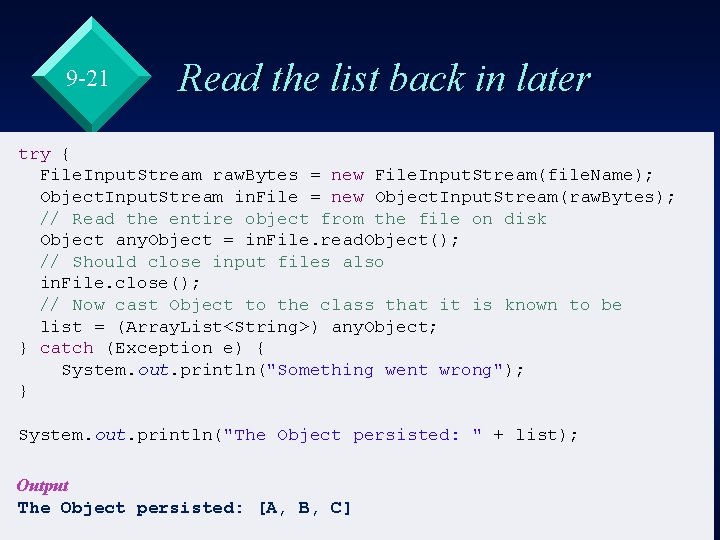
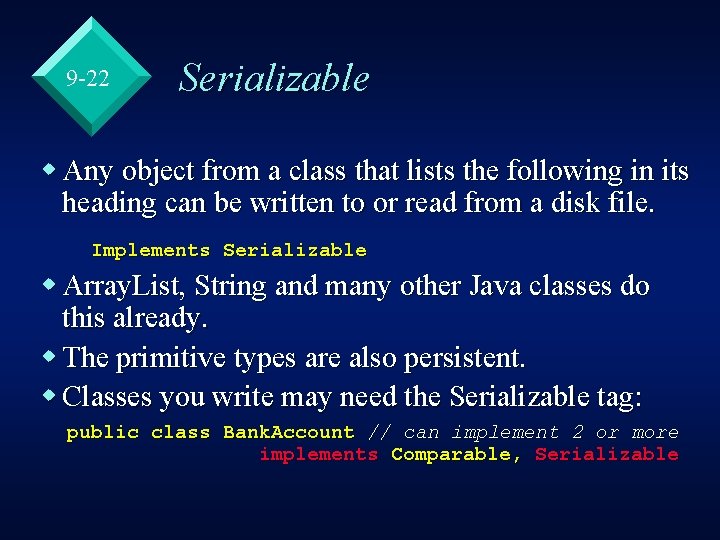
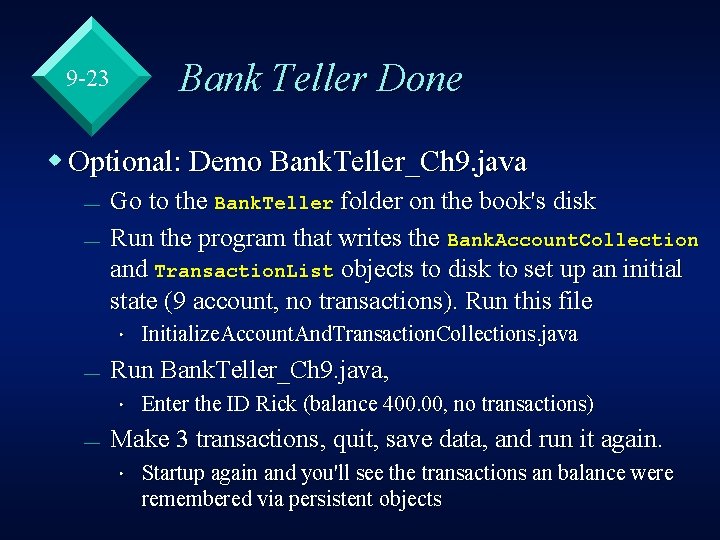
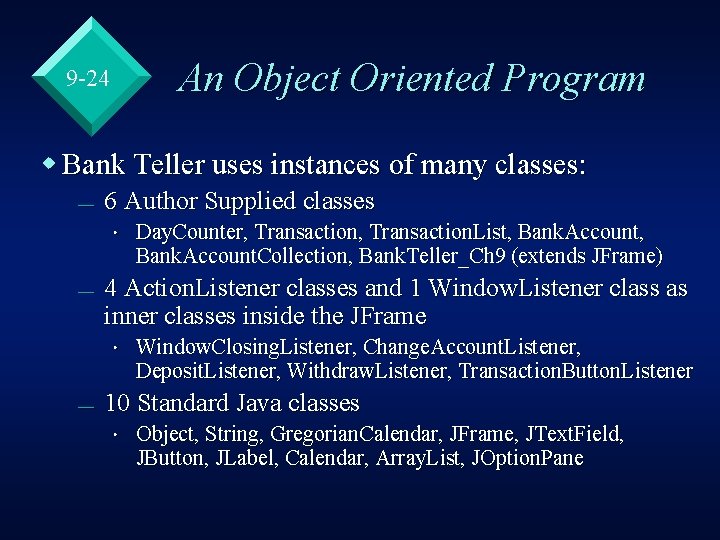
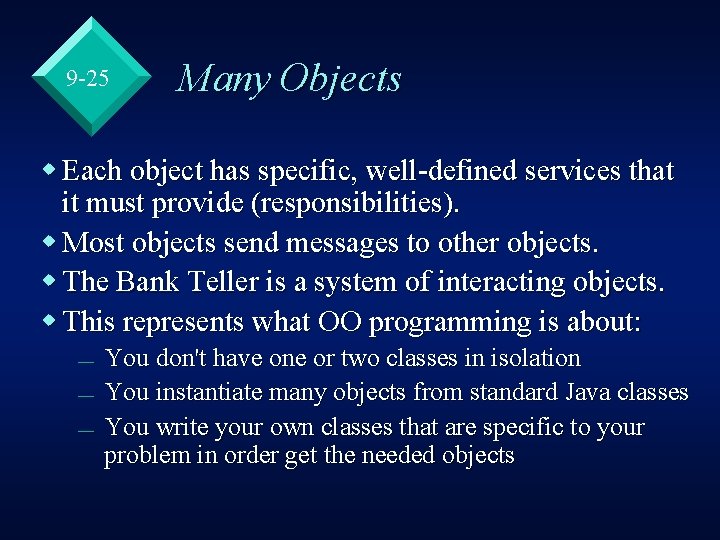
- Slides: 25
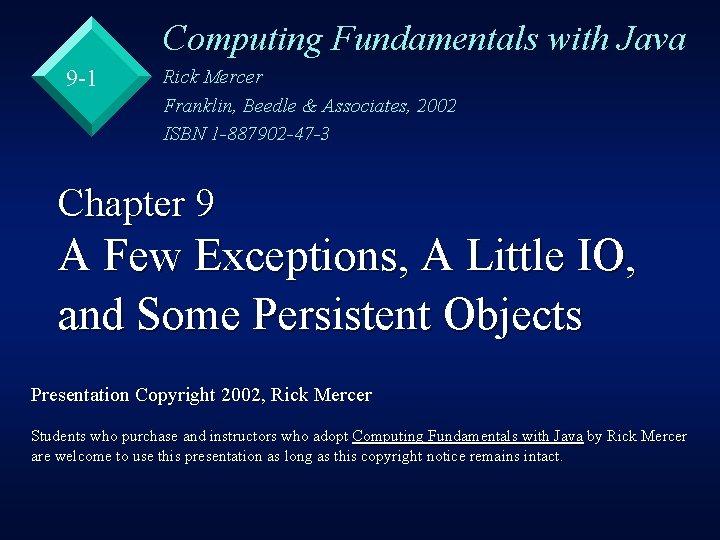
Computing Fundamentals with Java 9 -1 Rick Mercer Franklin, Beedle & Associates, 2002 ISBN 1 -887902 -47 -3 Chapter 9 A Few Exceptions, A Little IO, and Some Persistent Objects Presentation Copyright 2002, Rick Mercer Students who purchase and instructors who adopt Computing Fundamentals with Java by Rick Mercer are welcome to use this presentation as long as this copyright notice remains intact.
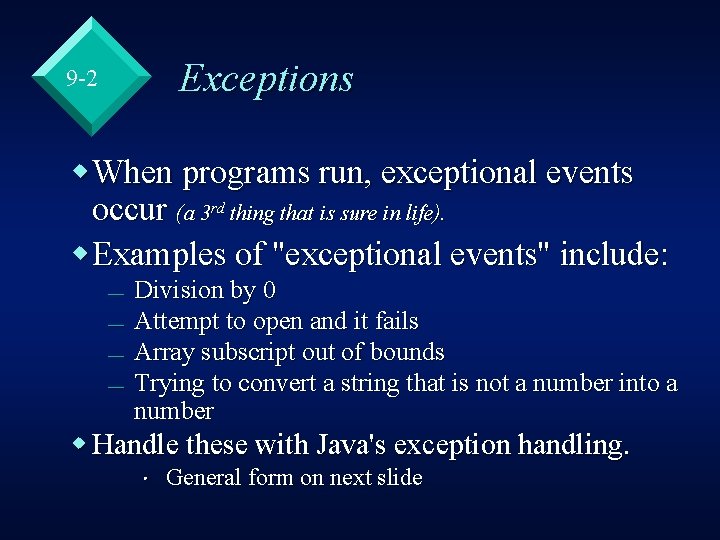
Exceptions 9 -2 w When programs run, exceptional events occur (a 3 rd thing that is sure in life). w Examples of "exceptional events" include: — — Division by 0 Attempt to open and it fails Array subscript out of bounds Trying to convert a string that is not a number into a number w Handle these with Java's exception handling. • General form on next slide
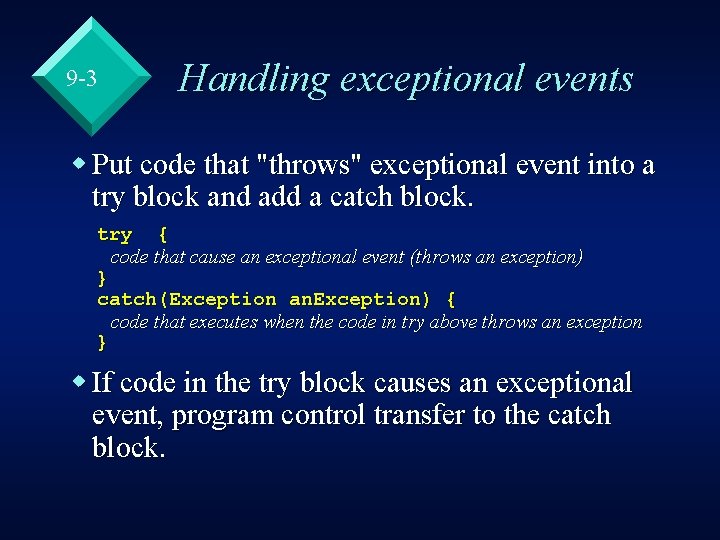
9 -3 Handling exceptional events w Put code that "throws" exceptional event into a try block and add a catch block. try { code that cause an exceptional event (throws an exception) } catch(Exception an. Exception) { code that executes when the code in try above throws an exception } w If code in the try block causes an exceptional event, program control transfer to the catch block.
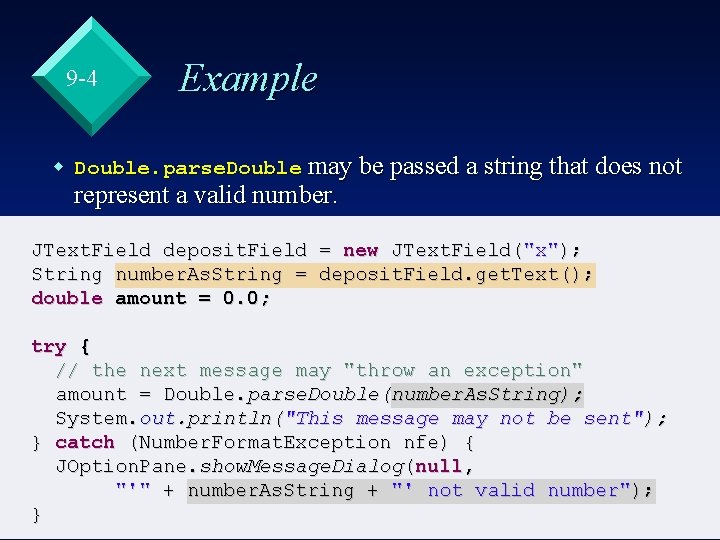
9 -4 Example w Double. parse. Double may be passed a string that does not represent a valid number. JText. Field deposit. Field = new JText. Field("x"); String number. As. String = deposit. Field. get. Text(); double amount = 0. 0; try { // the next message may "throw an exception" amount = Double. parse. Double(number. As. String); System. out. println("This message may not be sent"); } catch (Number. Format. Exception nfe) { JOption. Pane. show. Message. Dialog(null, "'" + number. As. String + "' not valid number"); }
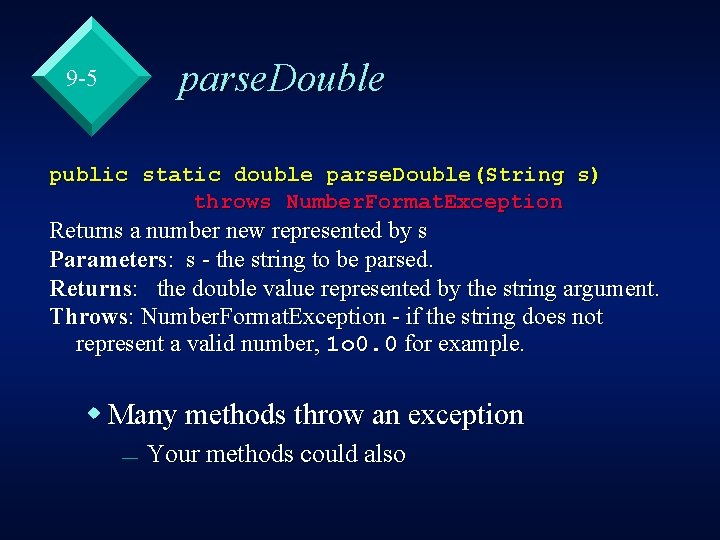
parse. Double 9 -5 public static double parse. Double(String s) throws Number. Format. Exception Returns a number new represented by s Parameters: s - the string to be parsed. Returns: the double value represented by the string argument. Throws: Number. Format. Exception - if the string does not represent a valid number, 1 o 0. 0 for example. w Many methods throw an exception — Your methods could also
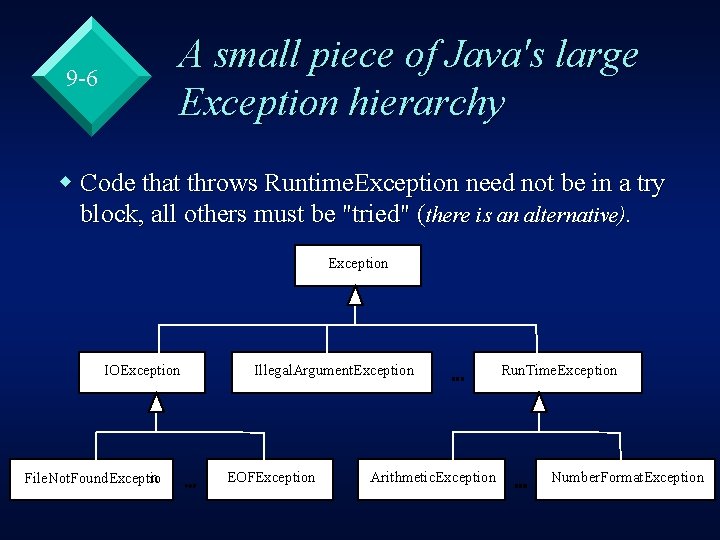
A small piece of Java's large Exception hierarchy 9 -6 w Code that throws Runtime. Exception need not be in a try block, all others must be "tried" (there is an alternative). Exception IOException n File. Not. Found. Exceptio Illegal. Argument. Exception EOFException Arithmetic. Exception Run. Time. Exception Number. Format. Exception
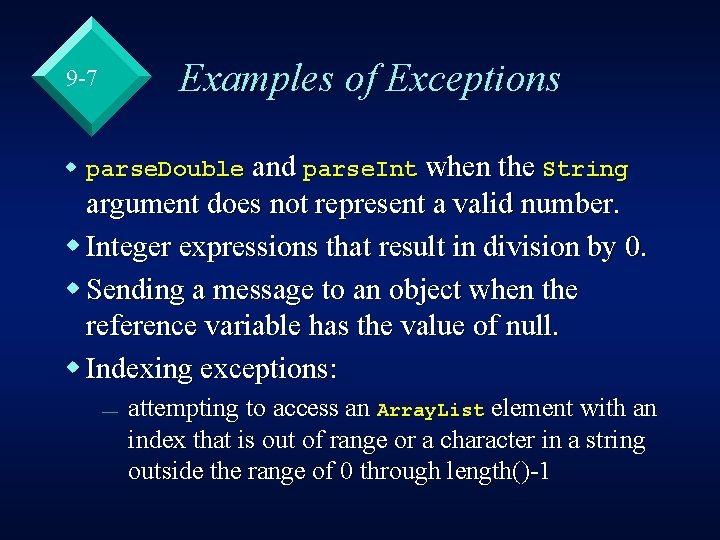
Examples of Exceptions 9 -7 w parse. Double and parse. Int when the String argument does not represent a valid number. w Integer expressions that result in division by 0. w Sending a message to an object when the reference variable has the value of null. w Indexing exceptions: — attempting to access an Array. List element with an index that is out of range or a character in a string outside the range of 0 through length()-1
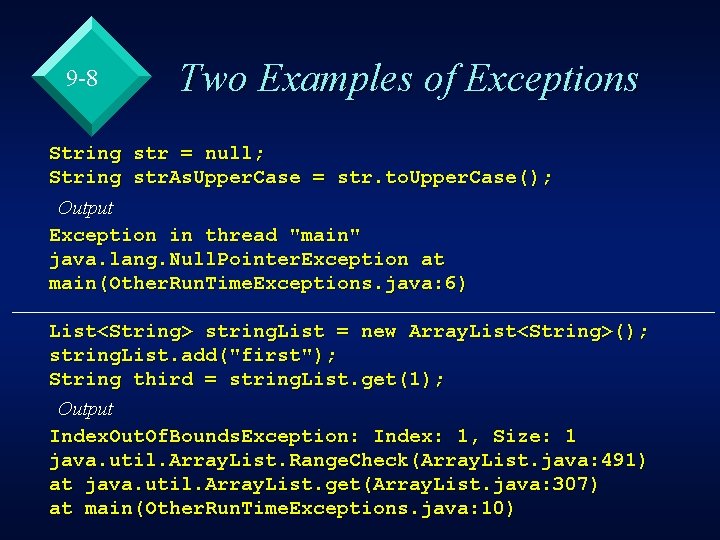
9 -8 Two Examples of Exceptions String str = null; String str. As. Upper. Case = str. to. Upper. Case(); Output Exception in thread "main" java. lang. Null. Pointer. Exception at main(Other. Run. Time. Exceptions. java: 6) List<String> string. List = new Array. List<String>(); string. List. add("first"); String third = string. List. get(1); Output Index. Out. Of. Bounds. Exception: Index: 1, Size: 1 java. util. Array. List. Range. Check(Array. List. java: 491) at java. util. Array. List. get(Array. List. java: 307) at main(Other. Run. Time. Exceptions. java: 10)
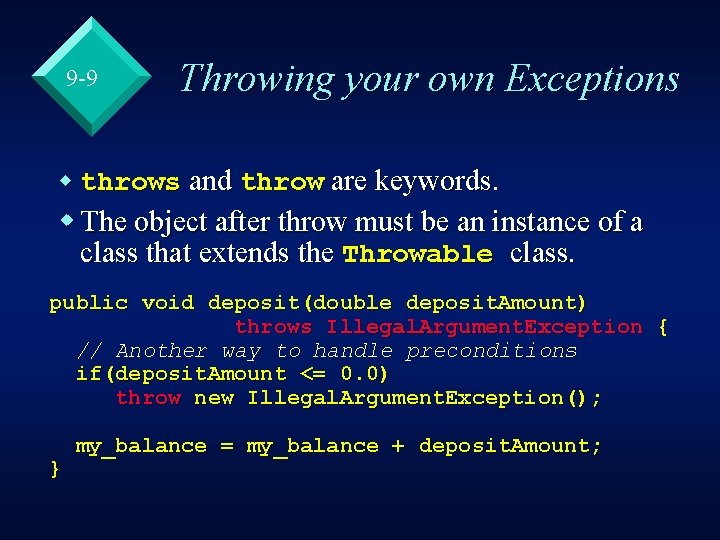
9 -9 Throwing your own Exceptions w throws and throw are keywords. w The object after throw must be an instance of a class that extends the Throwable class. public void deposit(double deposit. Amount) throws Illegal. Argument. Exception { // Another way to handle preconditions if(deposit. Amount <= 0. 0) throw new Illegal. Argument. Exception(); } my_balance = my_balance + deposit. Amount;
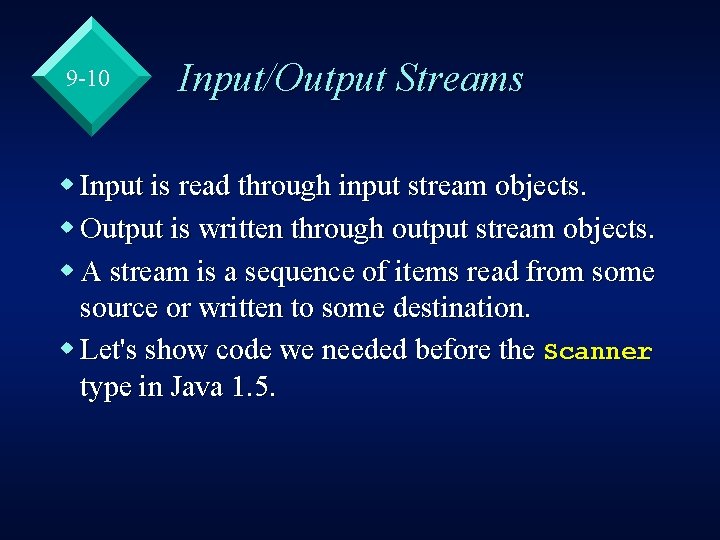
9 -10 Input/Output Streams w Input is read through input stream objects. w Output is written through output stream objects. w A stream is a sequence of items read from some source or written to some destination. w Let's show code we needed before the Scanner type in Java 1. 5.
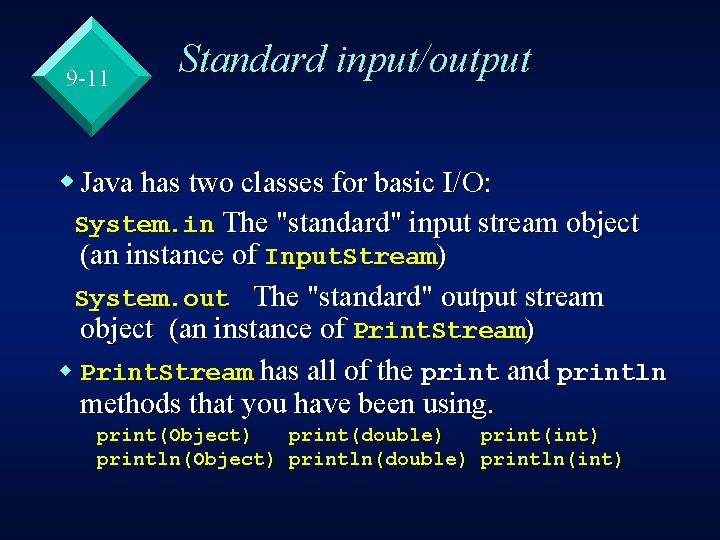
9 -11 Standard input/output w Java has two classes for basic I/O: System. in The "standard" input stream object (an instance of Input. Stream) System. out The "standard" output stream object (an instance of Print. Stream) w Print. Stream has all of the print and println methods that you have been using. print(Object) print(double) print(int) println(Object) println(double) println(int)
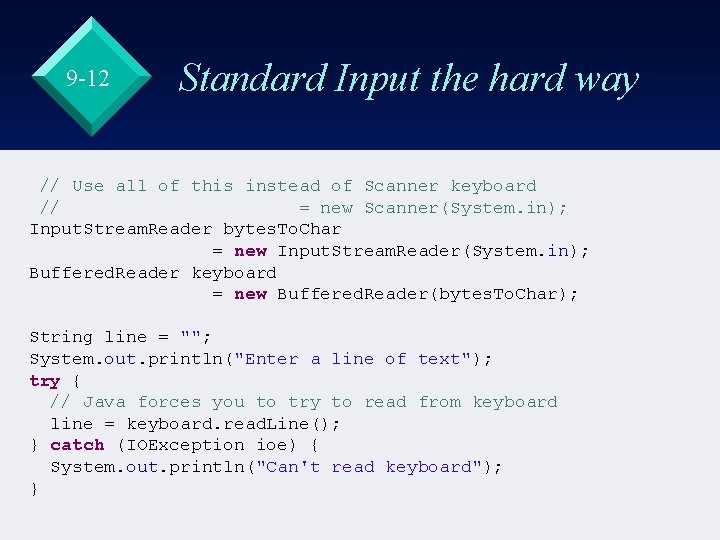
9 -12 Standard Input the hard way // Use all of this instead of Scanner keyboard // = new Scanner(System. in); Input. Stream. Reader bytes. To. Char = new Input. Stream. Reader(System. in); Buffered. Reader keyboard = new Buffered. Reader(bytes. To. Char); String line = ""; System. out. println("Enter a line of text"); try { // Java forces you to try to read from keyboard line = keyboard. read. Line(); } catch (IOException ioe) { System. out. println("Can't read keyboard"); }
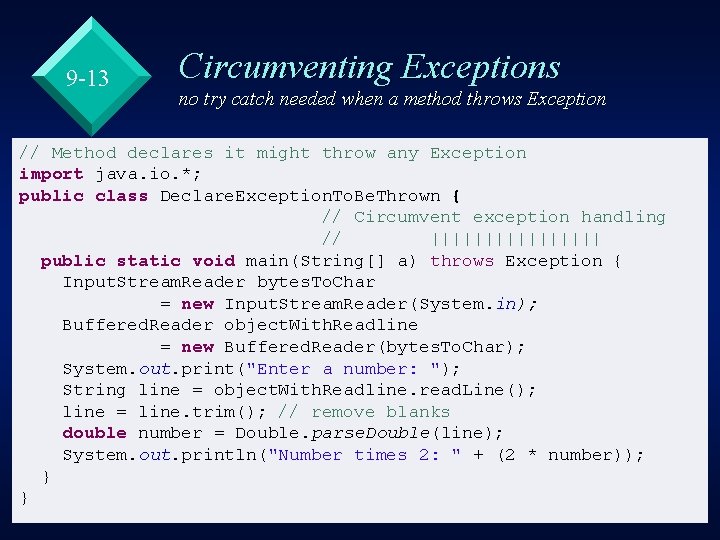
9 -13 Circumventing Exceptions no try catch needed when a method throws Exception // Method declares it might throw any Exception import java. io. *; public class Declare. Exception. To. Be. Thrown { // Circumvent exception handling // |||||||| public static void main(String[] a) throws Exception { Input. Stream. Reader bytes. To. Char = new Input. Stream. Reader(System. in); Buffered. Reader object. With. Readline = new Buffered. Reader(bytes. To. Char); System. out. print("Enter a number: "); String line = object. With. Readline. read. Line(); line = line. trim(); // remove blanks double number = Double. parse. Double(line); System. out. println("Number times 2: " + (2 * number)); } }
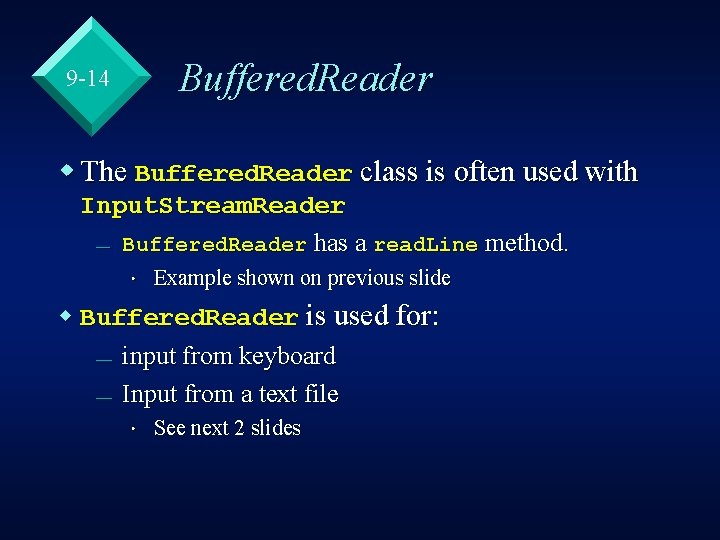
9 -14 Buffered. Reader w The Buffered. Reader class is often used with Input. Stream. Reader — Buffered. Reader has a read. Line method. • Example shown on previous slide w Buffered. Reader is used for: — input from keyboard — Input from a text file • See next 2 slides
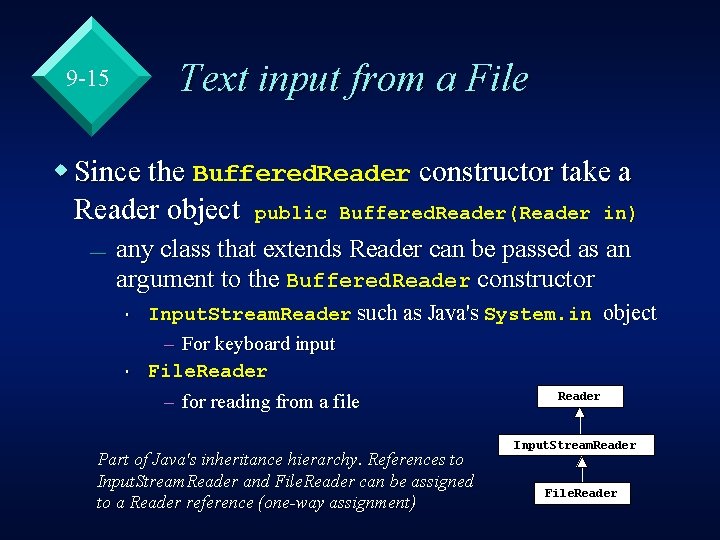
9 -15 Text input from a File w Since the Buffered. Reader constructor take a Reader object public Buffered. Reader(Reader in) — any class that extends Reader can be passed as an argument to the Buffered. Reader constructor • Input. Stream. Reader such as Java's System. in object – For keyboard input • File. Reader – for reading from a file Part of Java's inheritance hierarchy. References to Input. Stream. Reader and File. Reader can be assigned to a Reader reference (one-way assignment) Input. Stream. Reader File. Reader
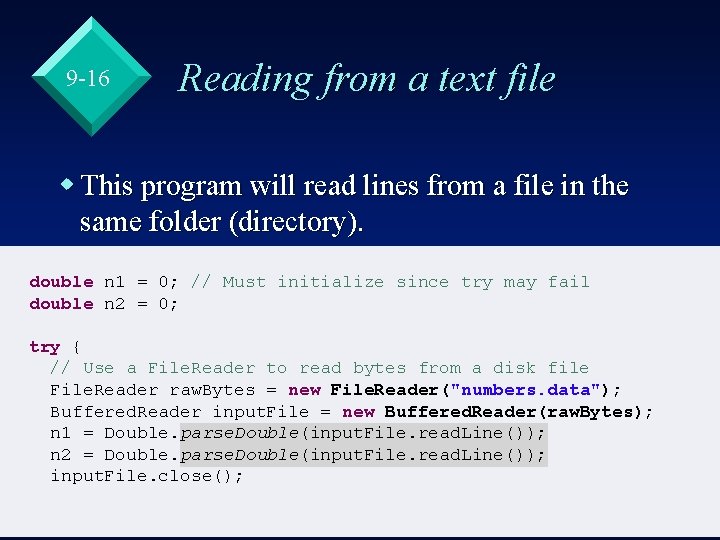
9 -16 Reading from a text file w This program will read lines from a file in the same folder (directory). double n 1 = 0; // Must initialize since try may fail double n 2 = 0; try { // Use a File. Reader to read bytes from a disk file File. Reader raw. Bytes = new File. Reader("numbers. data"); Buffered. Reader input. File = new Buffered. Reader(raw. Bytes); n 1 = Double. parse. Double(input. File. read. Line()); n 2 = Double. parse. Double(input. File. read. Line()); input. File. close();
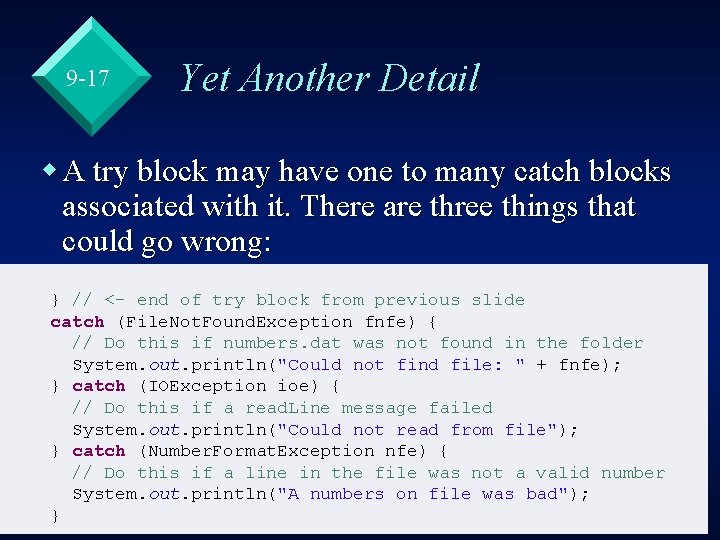
9 -17 Yet Another Detail w A try block may have one to many catch blocks associated with it. There are three things that could go wrong: } // <- end of try block from previous slide catch (File. Not. Found. Exception fnfe) { // Do this if numbers. dat was not found in the folder System. out. println("Could not find file: " + fnfe); } catch (IOException ioe) { // Do this if a read. Line message failed System. out. println("Could not read from file"); } catch (Number. Format. Exception nfe) { // Do this if a line in the file was not a valid number System. out. println("A numbers on file was bad"); }
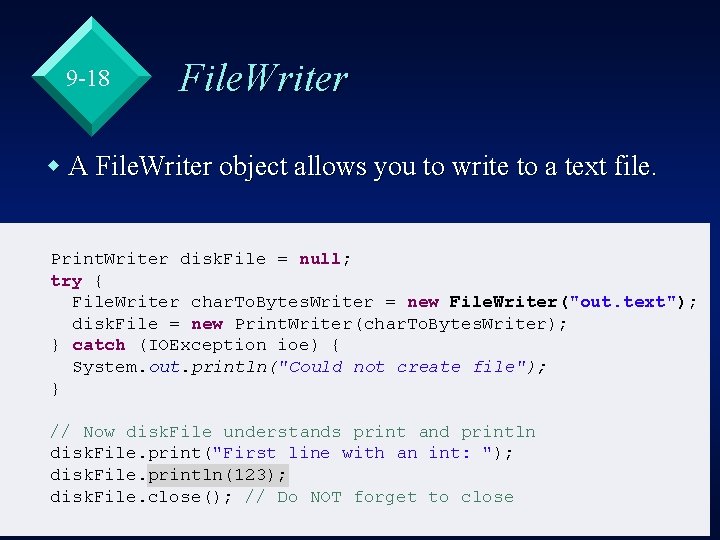
9 -18 File. Writer w A File. Writer object allows you to write to a text file. Print. Writer disk. File = null; try { File. Writer char. To. Bytes. Writer = new File. Writer("out. text"); disk. File = new Print. Writer(char. To. Bytes. Writer); } catch (IOException ioe) { System. out. println("Could not create file"); } // Now disk. File understands print and println disk. File. print("First line with an int: "); disk. File. println(123); disk. File. close(); // Do NOT forget to close
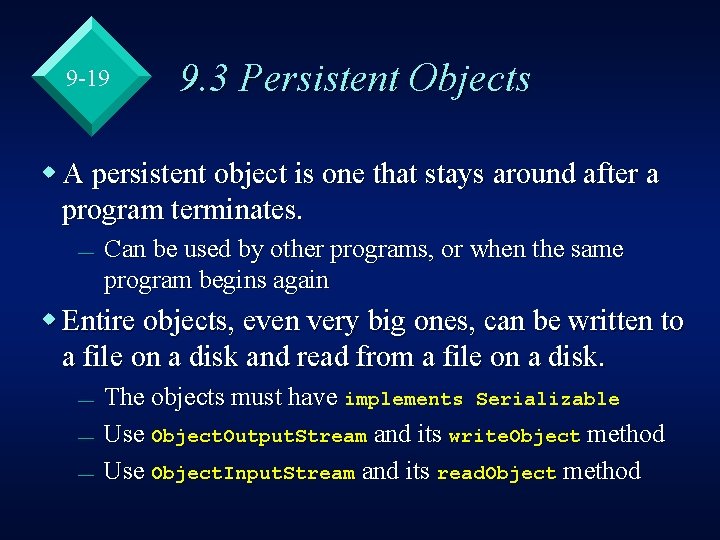
9 -19 9. 3 Persistent Objects w A persistent object is one that stays around after a program terminates. — Can be used by other programs, or when the same program begins again w Entire objects, even very big ones, can be written to a file on a disk and read from a file on a disk. — — — The objects must have implements Serializable Use Object. Output. Stream and its write. Object method Use Object. Input. Stream and its read. Object method
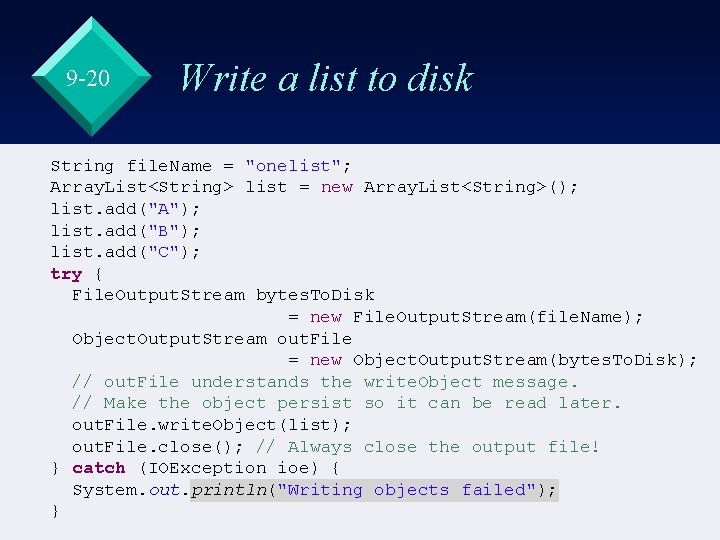
9 -20 Write a list to disk String file. Name = "onelist"; Array. List<String> list = new Array. List<String>(); list. add("A"); list. add("B"); list. add("C"); try { File. Output. Stream bytes. To. Disk = new File. Output. Stream(file. Name); Object. Output. Stream out. File = new Object. Output. Stream(bytes. To. Disk); // out. File understands the write. Object message. // Make the object persist so it can be read later. out. File. write. Object(list); out. File. close(); // Always close the output file! } catch (IOException ioe) { System. out. println("Writing objects failed"); }
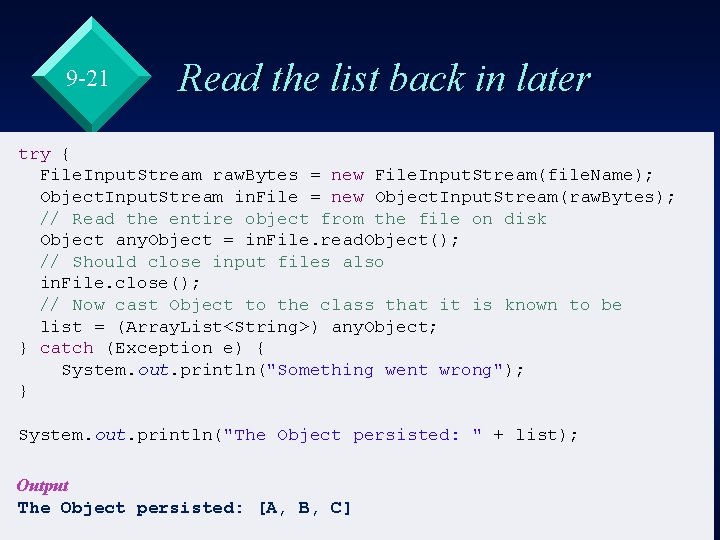
9 -21 Read the list back in later try { File. Input. Stream raw. Bytes = new File. Input. Stream(file. Name); Object. Input. Stream in. File = new Object. Input. Stream(raw. Bytes); // Read the entire object from the file on disk Object any. Object = in. File. read. Object(); // Should close input files also in. File. close(); // Now cast Object to the class that it is known to be list = (Array. List<String>) any. Object; } catch (Exception e) { System. out. println("Something went wrong"); } System. out. println("The Object persisted: " + list); Output The Object persisted: [A, B, C]
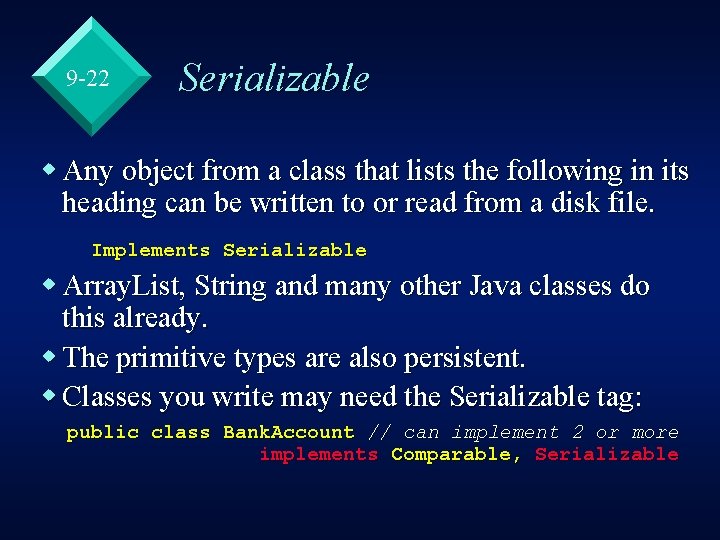
9 -22 Serializable w Any object from a class that lists the following in its heading can be written to or read from a disk file. Implements Serializable w Array. List, String and many other Java classes do this already. w The primitive types are also persistent. w Classes you write may need the Serializable tag: public class Bank. Account // can implement 2 or more implements Comparable, Serializable
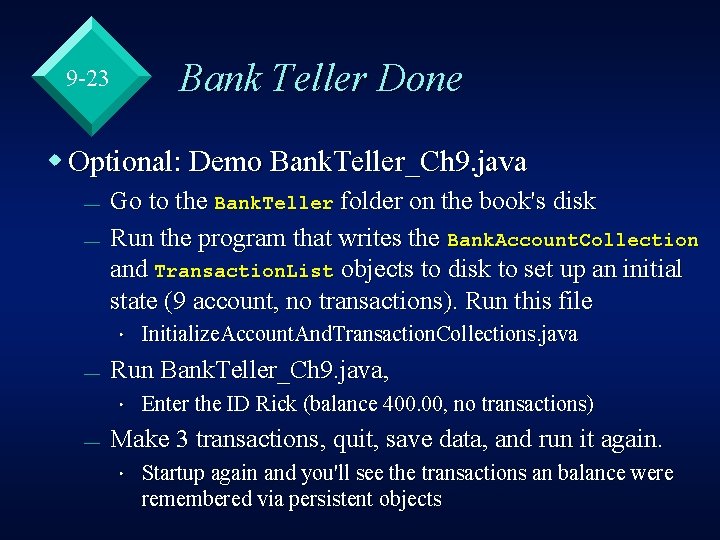
9 -23 Bank Teller Done w Optional: Demo Bank. Teller_Ch 9. java — — Go to the Bank. Teller folder on the book's disk Run the program that writes the Bank. Account. Collection and Transaction. List objects to disk to set up an initial state (9 account, no transactions). Run this file • Initialize. Account. And. Transaction. Collections. java — Run Bank. Teller_Ch 9. java, • Enter the ID Rick (balance 400. 00, no transactions) — Make 3 transactions, quit, save data, and run it again. • Startup again and you'll see the transactions an balance were remembered via persistent objects
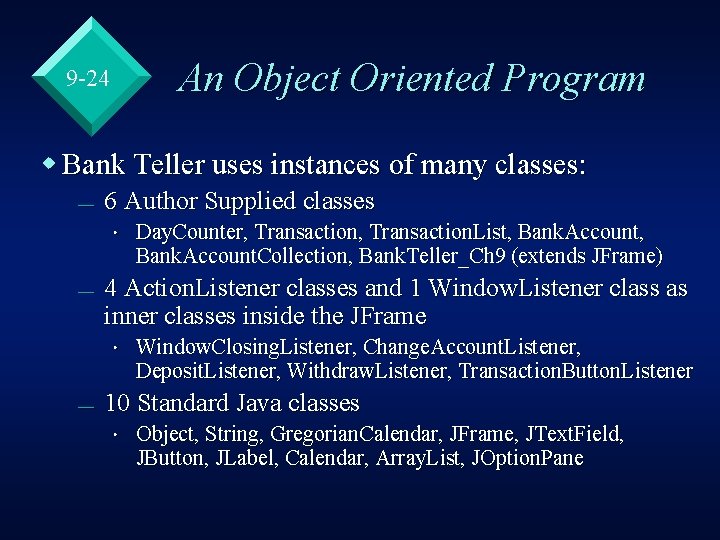
9 -24 An Object Oriented Program w Bank Teller uses instances of many classes: — 6 Author Supplied classes • Day. Counter, Transaction. List, Bank. Account. Collection, Bank. Teller_Ch 9 (extends JFrame) — 4 Action. Listener classes and 1 Window. Listener class as inner classes inside the JFrame • Window. Closing. Listener, Change. Account. Listener, Deposit. Listener, Withdraw. Listener, Transaction. Button. Listener — 10 Standard Java classes • Object, String, Gregorian. Calendar, JFrame, JText. Field, JButton, JLabel, Calendar, Array. List, JOption. Pane
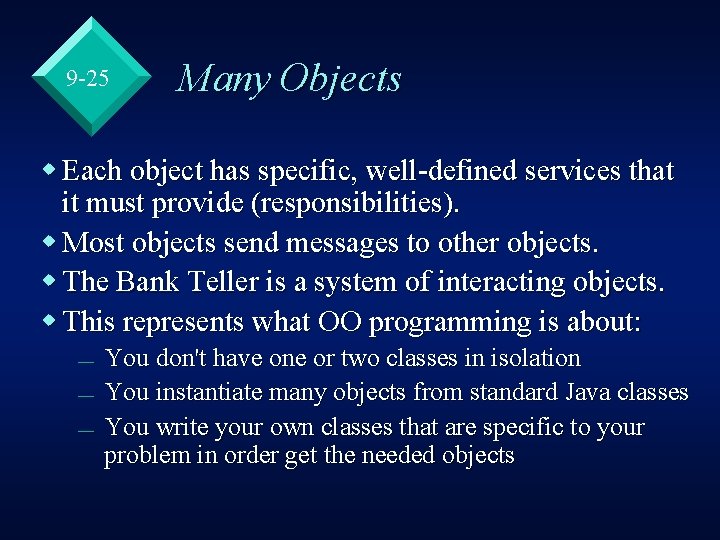
9 -25 Many Objects w Each object has specific, well-defined services that it must provide (responsibilities). w Most objects send messages to other objects. w The Bank Teller is a system of interacting objects. w This represents what OO programming is about: — — — You don't have one or two classes in isolation You instantiate many objects from standard Java classes You write your own classes that are specific to your problem in order get the needed objects
Logic and computer design fundamentals
Conventional computing and intelligent computing
[author] java message service - jms fundamentals course
Jms(java)
Program
Class fundamentals in java
Chapter 2 lab java fundamentals
Language fundamentals in java
Bradshaw mercer social needs
Introduction to unified modeling language
Tim mercer golf
Vitiating factors
Employee grade structure
Mercer canvs
Ramona t mercer theory
Language
Mercer medical library
Www.imercer.com
Mercer ipe framework
Non est factum rule
Child protect of mercer county
Ante stres
Mercer pay grades
Mercer medical library
Java import java.util.*
Java import java.util.*