The SinglyLinked Structure Rick Mercer Store elements stored
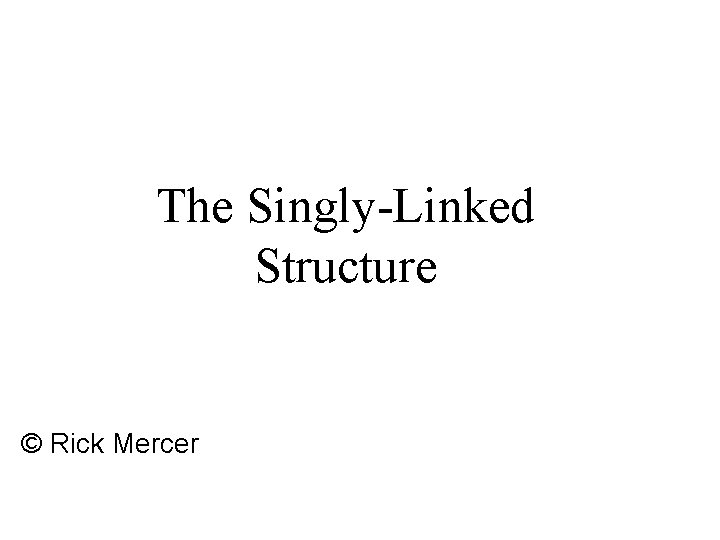
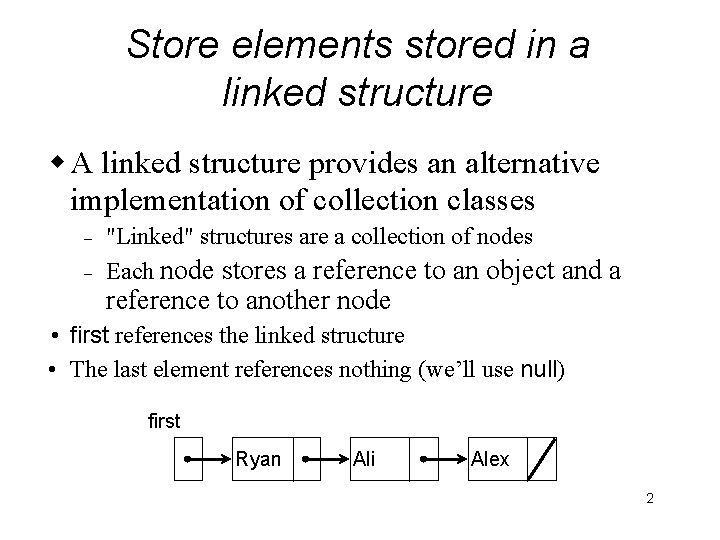
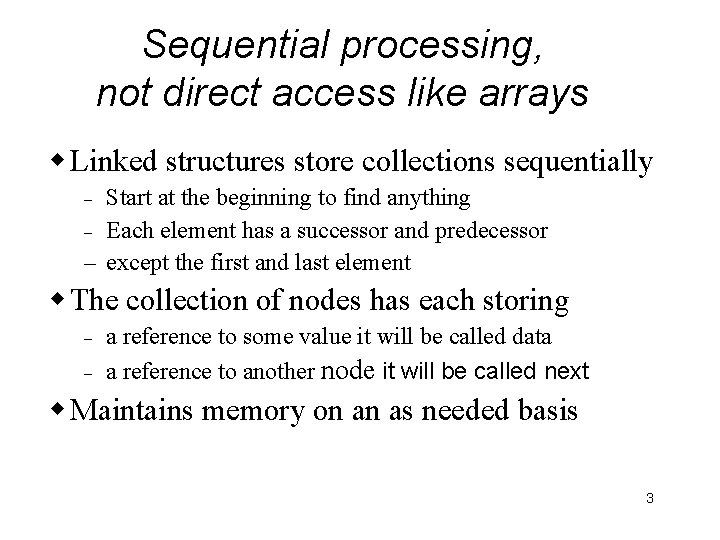
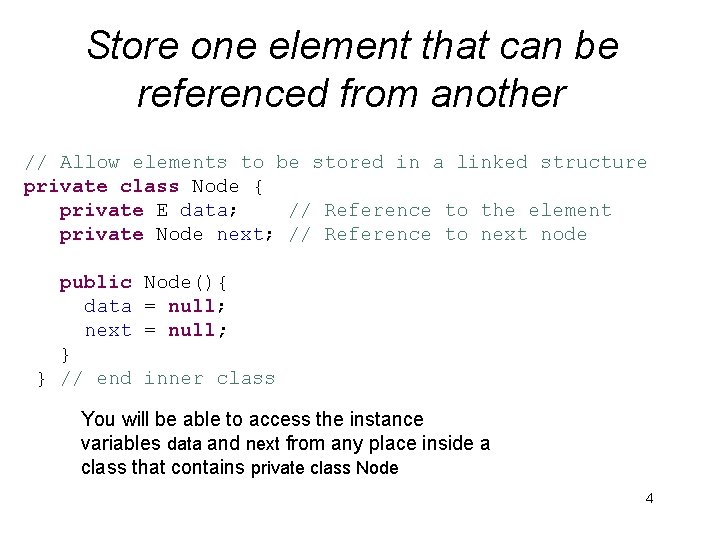
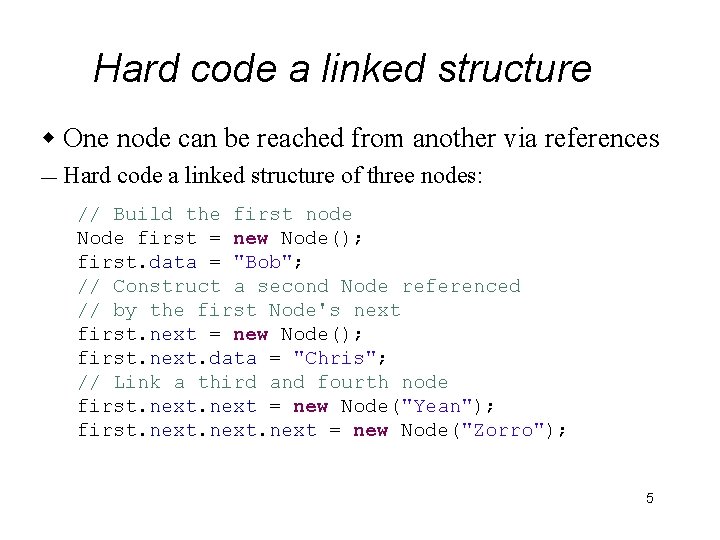
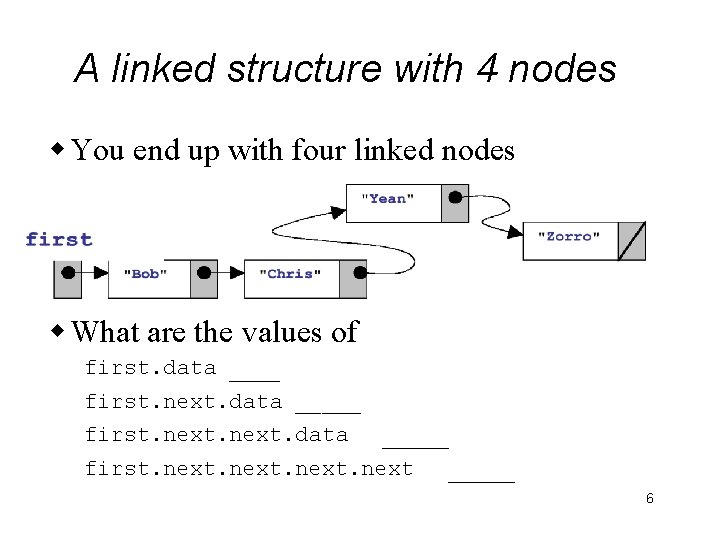
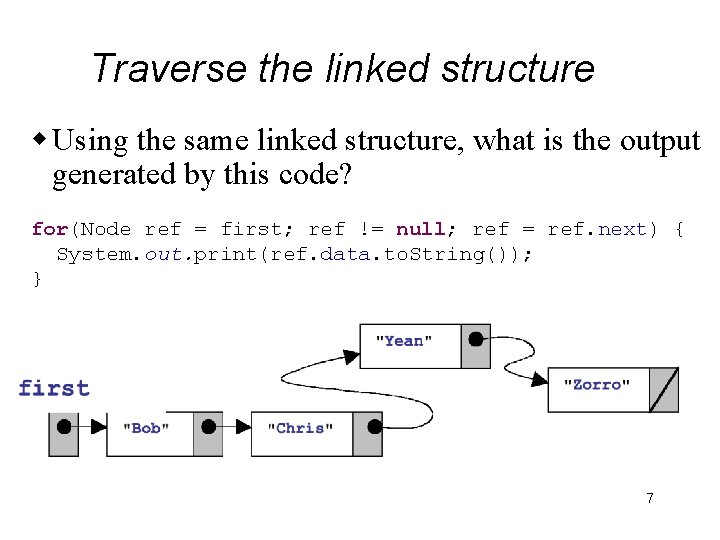
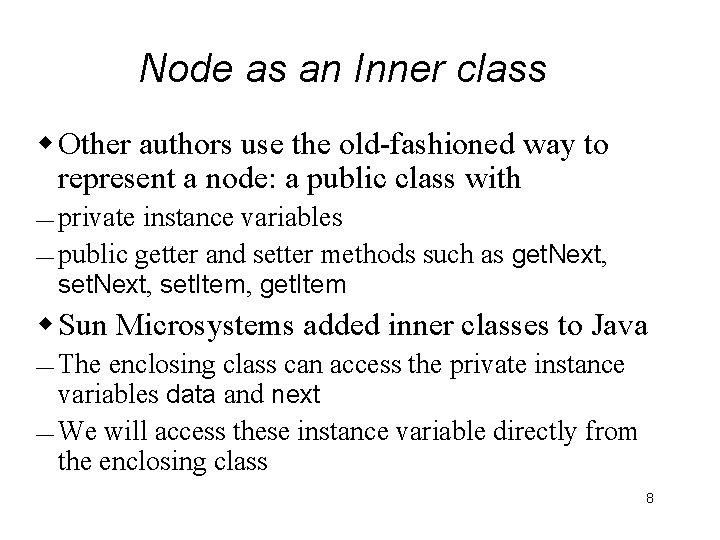
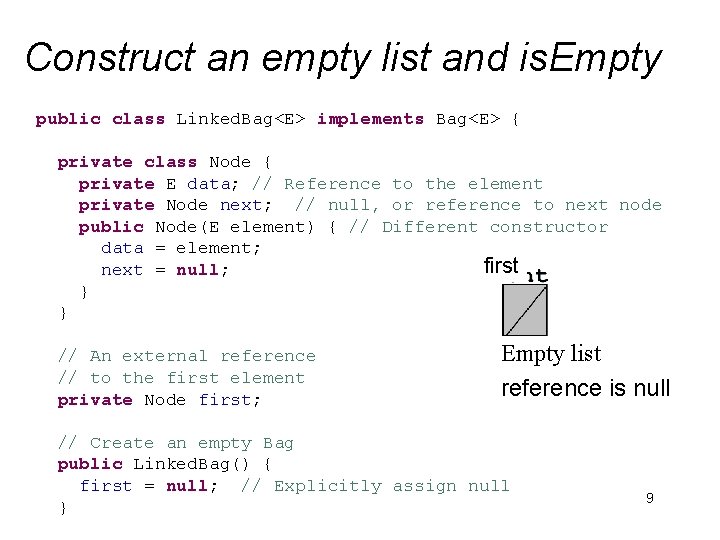
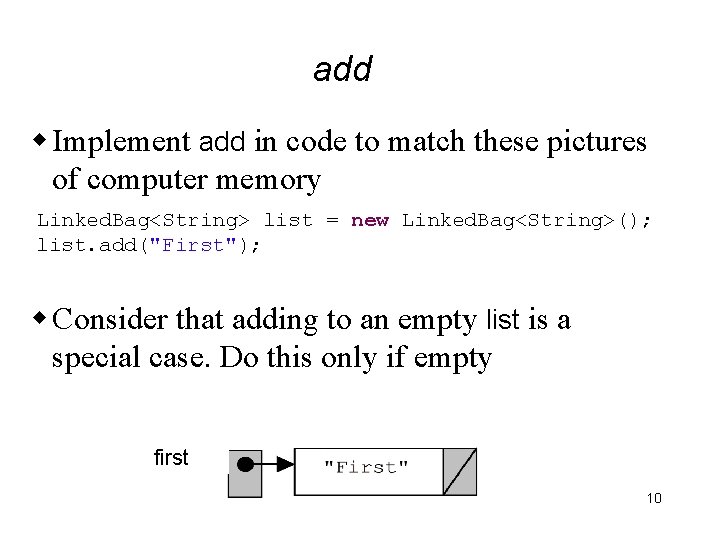
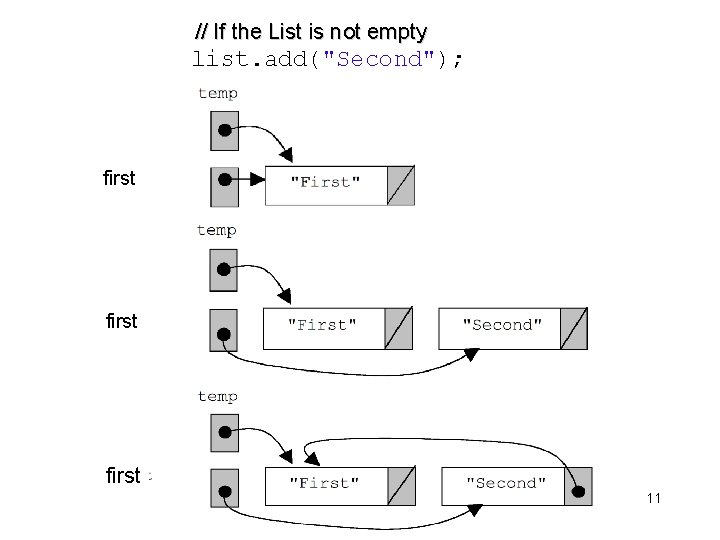
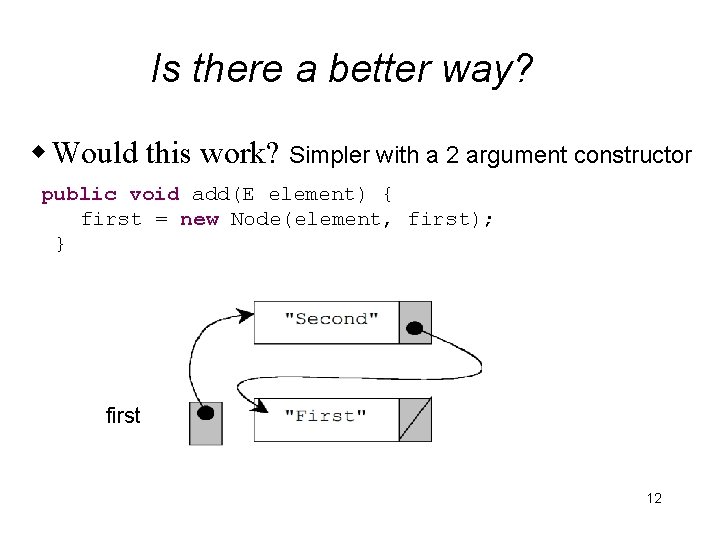
- Slides: 12
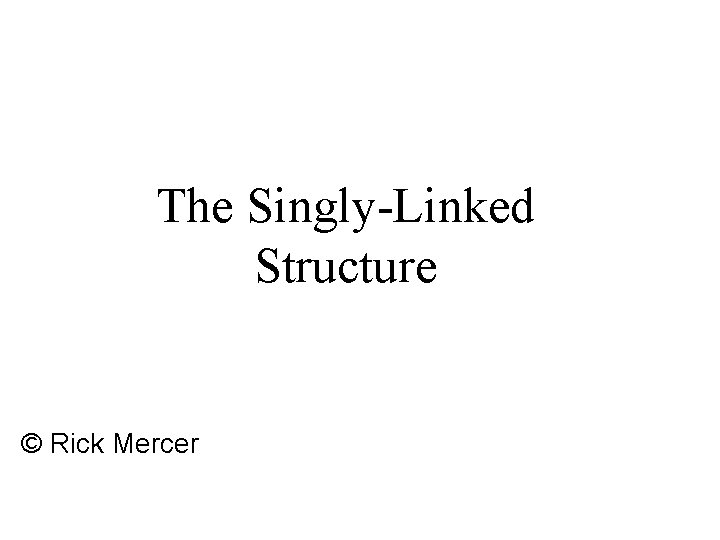
The Singly-Linked Structure © Rick Mercer
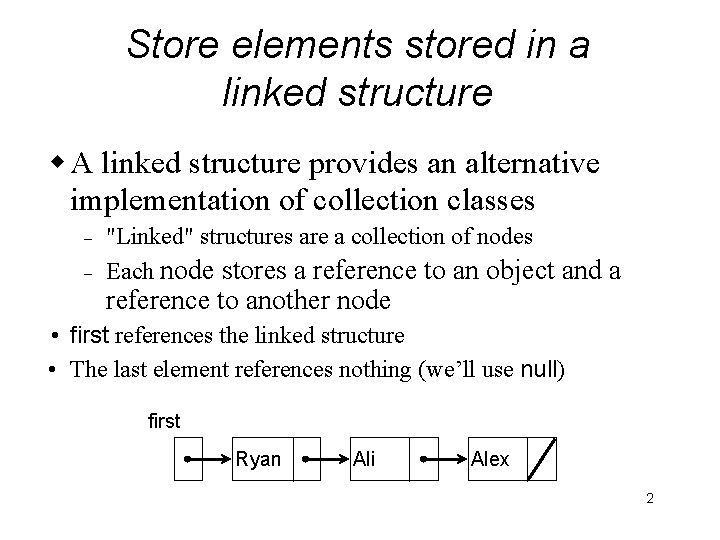
Store elements stored in a linked structure w A linked structure provides an alternative implementation of collection classes – – "Linked" structures are a collection of nodes Each node stores a reference to an object and a reference to another node • first references the linked structure • The last element references nothing (we’ll use null) first Ryan Ali Alex 2
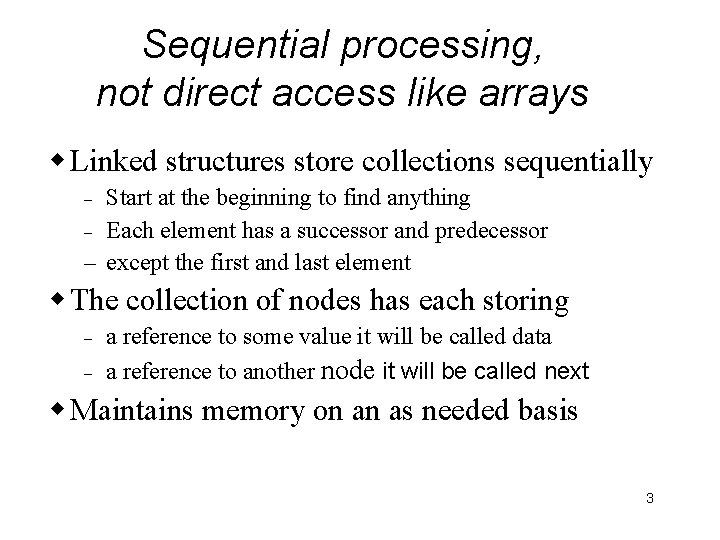
Sequential processing, not direct access like arrays w Linked structures store collections sequentially Start at the beginning to find anything – Each element has a successor and predecessor – except the first and last element – w The collection of nodes has each storing – – a reference to some value it will be called data a reference to another node it will be called next w Maintains memory on an as needed basis 3
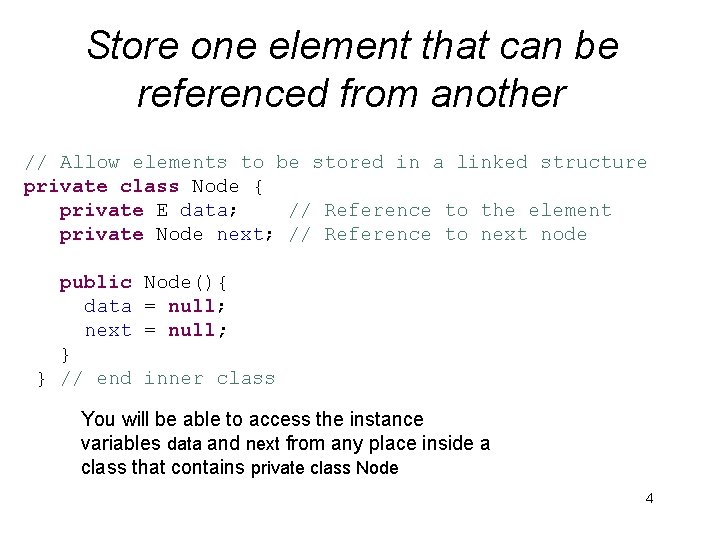
Store one element that can be referenced from another // Allow elements to be stored in a linked structure private class Node { private E data; // Reference to the element private Node next; // Reference to next node public data next } } // end Node(){ = null; inner class You will be able to access the instance variables data and next from any place inside a class that contains private class Node 4
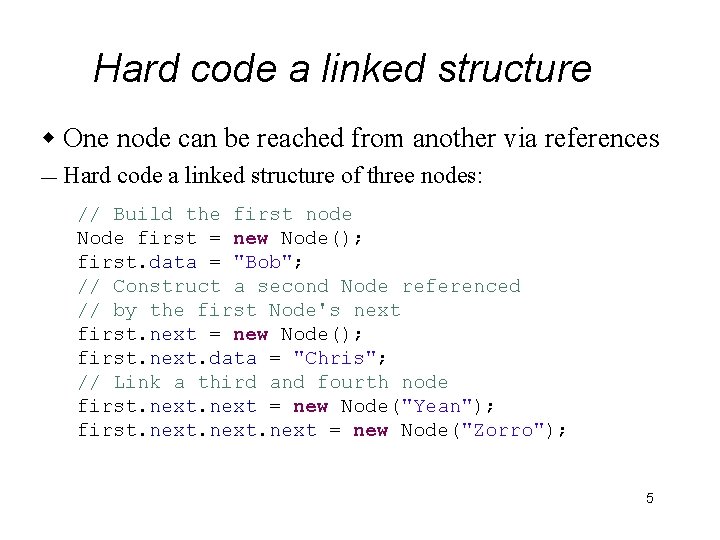
Hard code a linked structure w One node can be reached from another via references — Hard code a linked structure of three nodes: // Build the first node Node first = new Node(); first. data = "Bob"; // Construct a second Node referenced // by the first Node's next first. next = new Node(); first. next. data = "Chris"; // Link a third and fourth node first. next = new Node("Yean"); first. next = new Node("Zorro"); 5
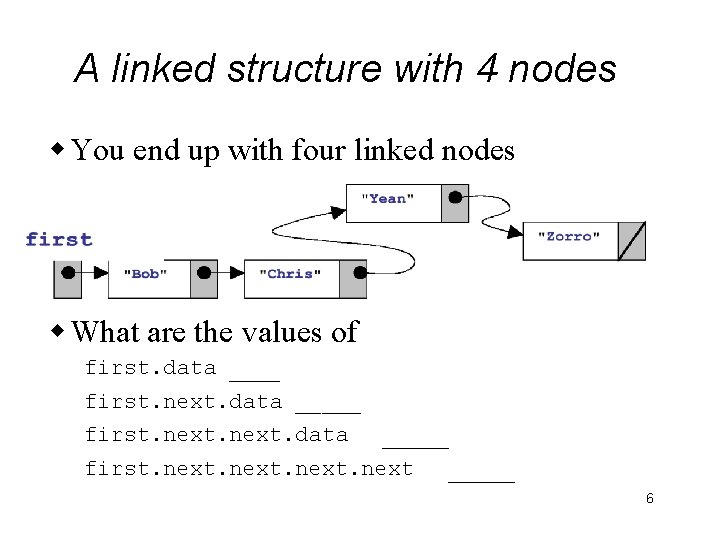
A linked structure with 4 nodes w You end up with four linked nodes w What are the values of first. data ___ first. next. data _____ first. next. data 6
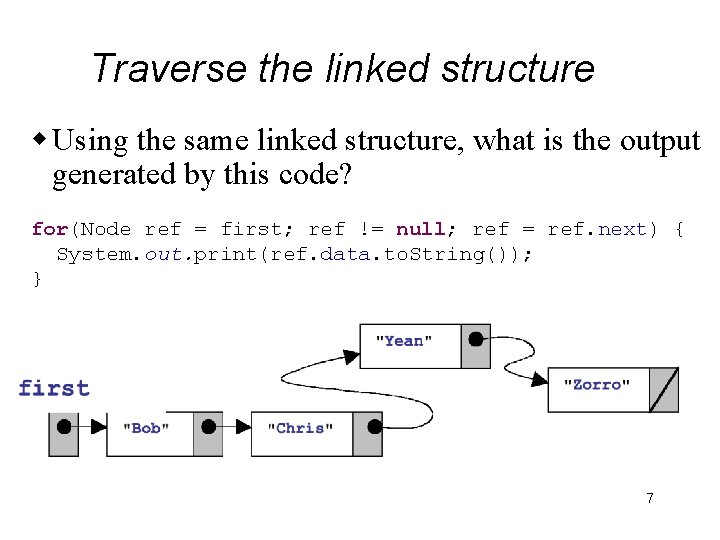
Traverse the linked structure w Using the same linked structure, what is the output generated by this code? for(Node ref = first; ref != null; ref = ref. next) { System. out. print(ref. data. to. String()); } 7
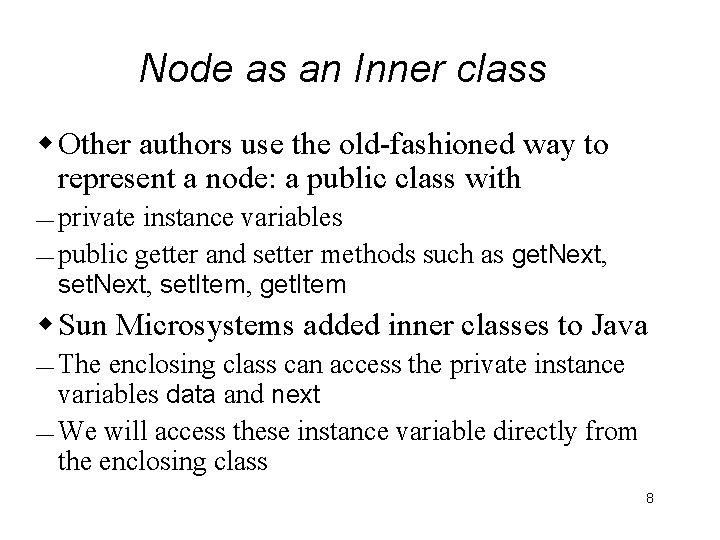
Node as an Inner class w Other authors use the old-fashioned way to represent a node: a public class with — private instance variables — public getter and setter methods such as get. Next, set. Item, get. Item w Sun Microsystems added inner classes to Java — The enclosing class can access the private instance variables data and next — We will access these instance variable directly from the enclosing class 8
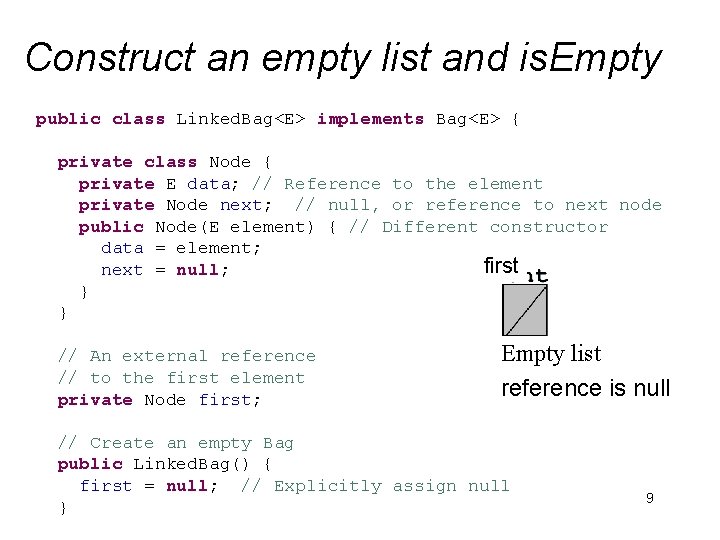
Construct an empty list and is. Empty public class Linked. Bag<E> implements Bag<E> { private class Node { private E data; // Reference to the element private Node next; // null, or reference to next node public Node(E element) { // Different constructor data = element; first next = null; } } // An external reference // to the first element private Node first; Empty list reference is null // Create an empty Bag public Linked. Bag() { first = null; // Explicitly assign null } 9
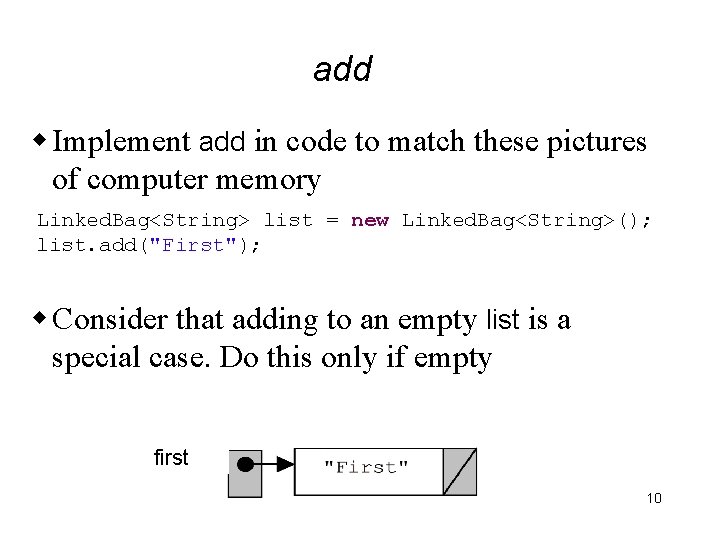
add w Implement add in code to match these pictures of computer memory Linked. Bag<String> list = new Linked. Bag<String>(); list. add("First"); w Consider that adding to an empty list is a special case. Do this only if empty first 10
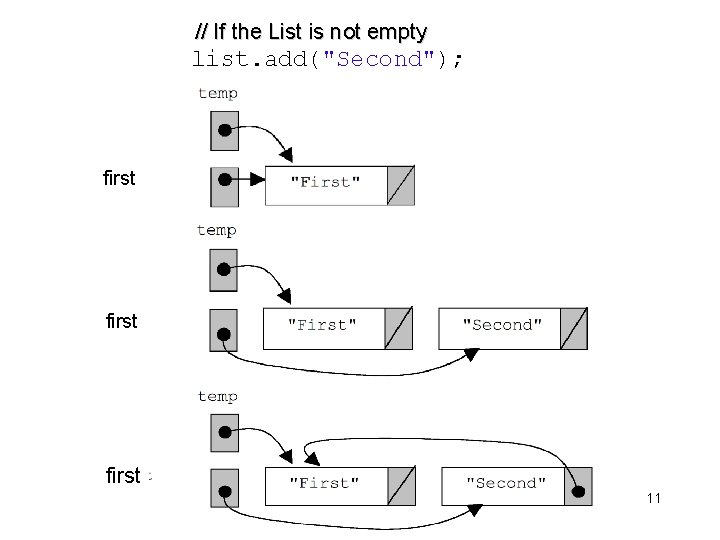
// If the List is not empty list. add("Second"); first 11
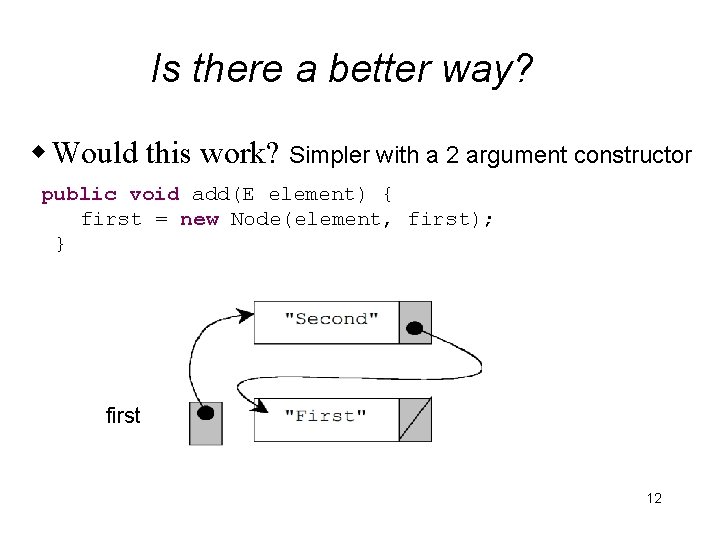
Is there a better way? w Would this work? Simpler with a 2 argument constructor public void add(E element) { first = new Node(element, first); } first 12
Pengertian stored procedure
Row store vs column store
Row store vs column store
Bradshaw mercer social needs
Introduction to unified modeling language
Jeff quackenbush newark
Hemans v coffie
Mercer job matching booklet 2020
Mercer canvs
Ramona t mercer nursing theory
Jelinek mercer smoothing
Mercer med library
Mercer human capital