Chapter 6 Repetition Asserting Java Rick Mercer Algorithmic
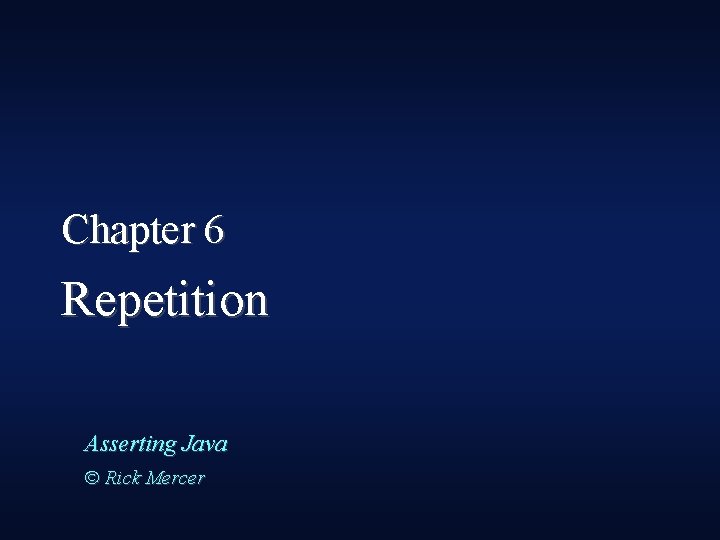
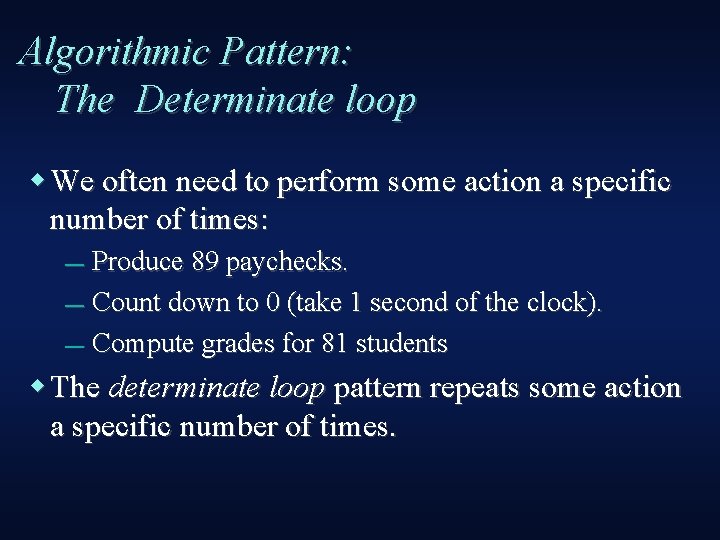
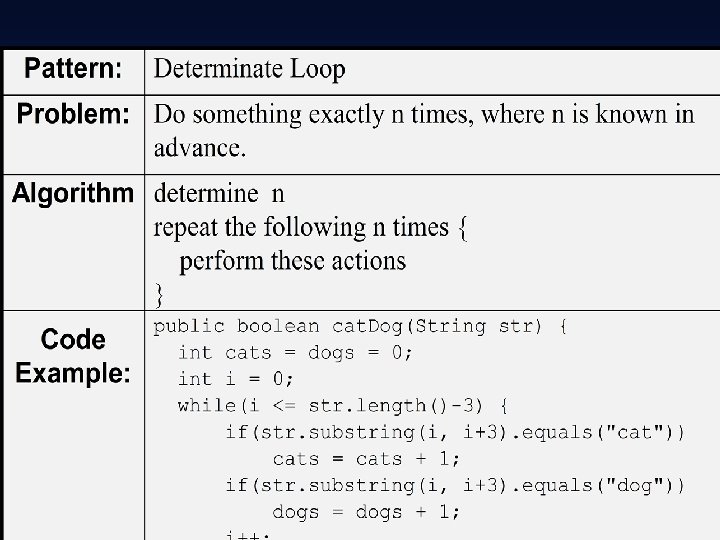
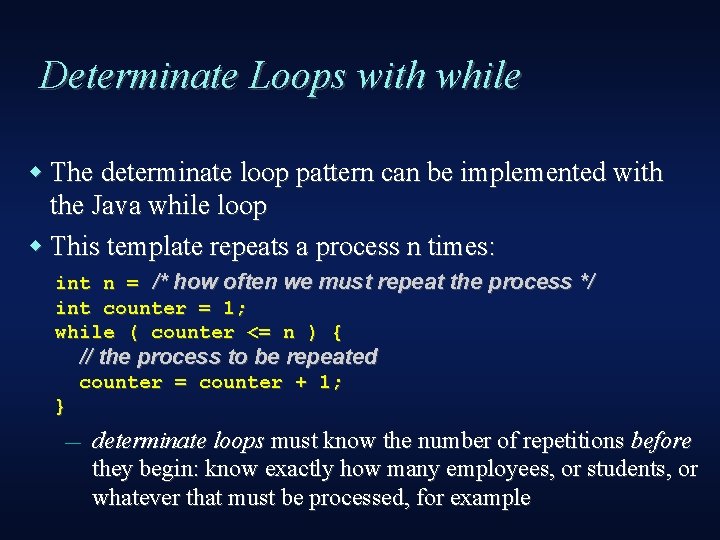
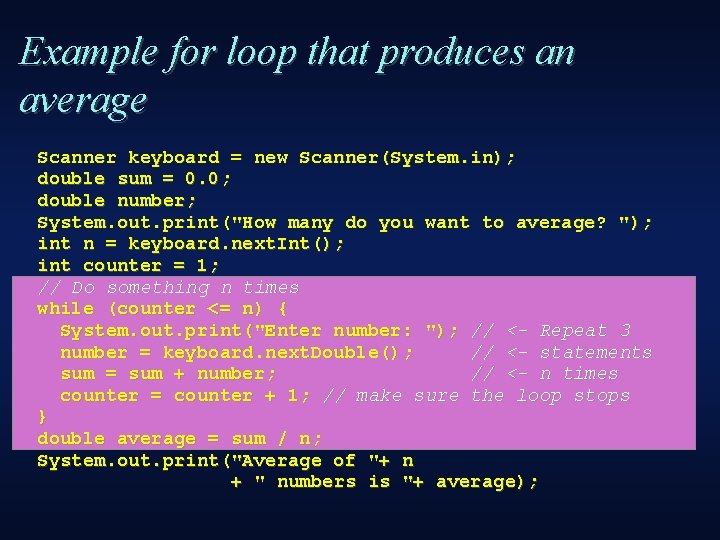
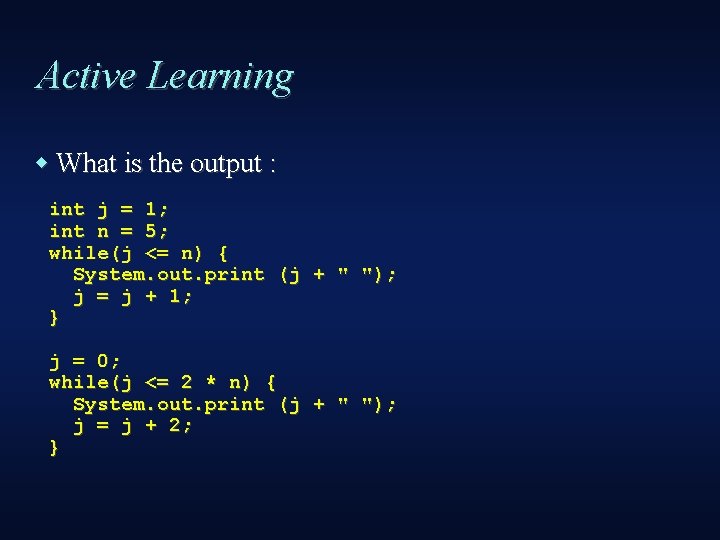
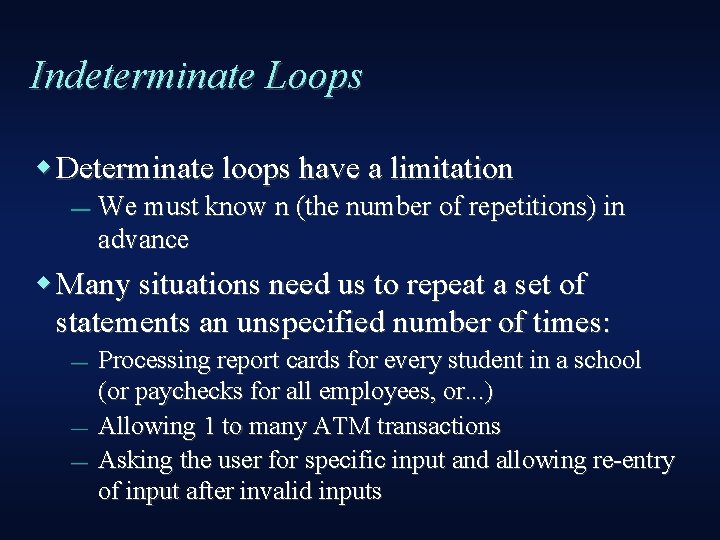
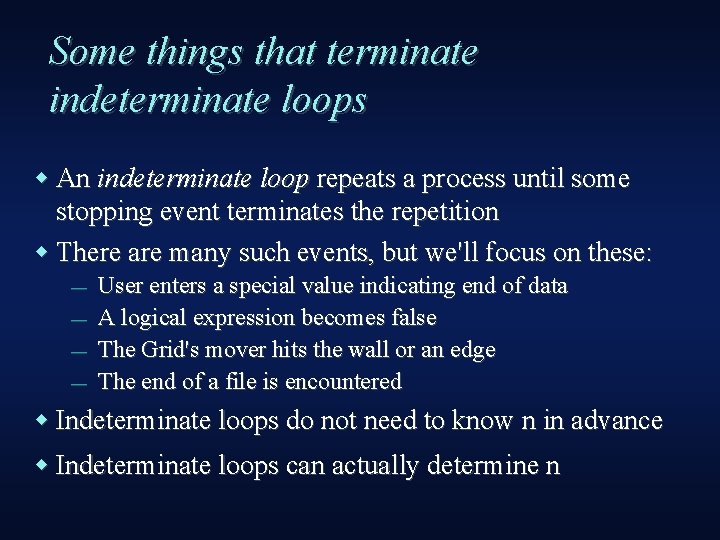
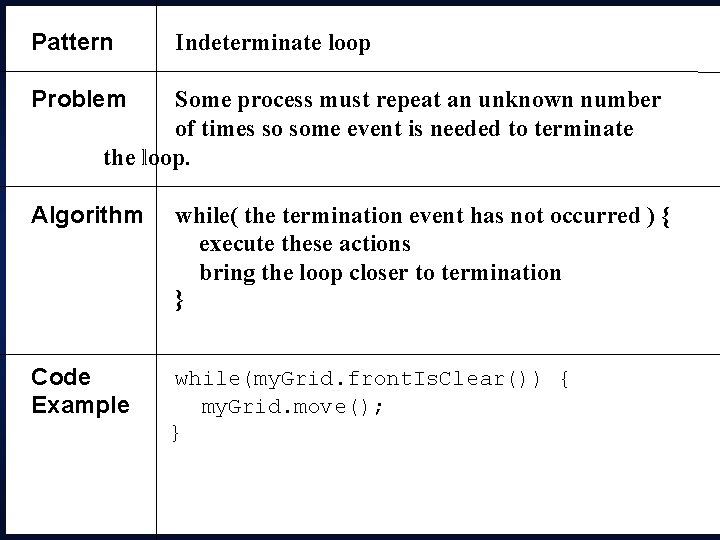
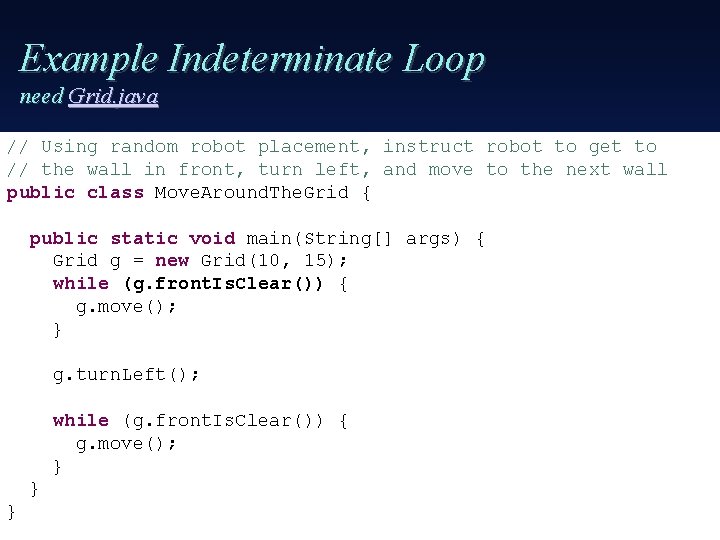
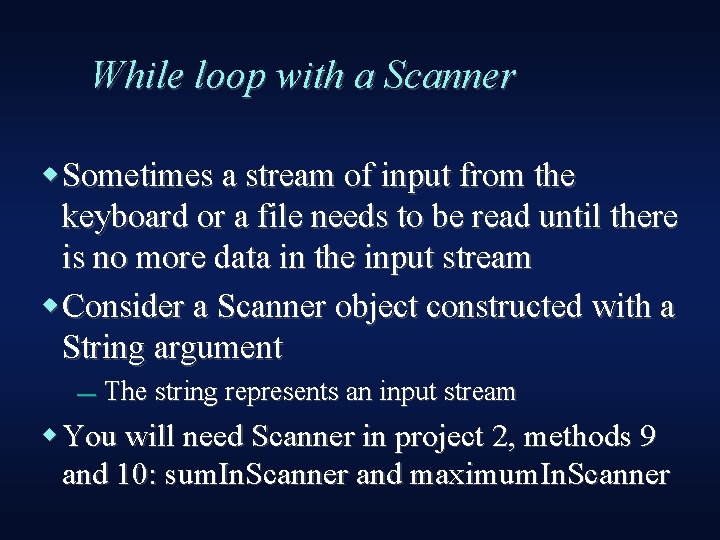
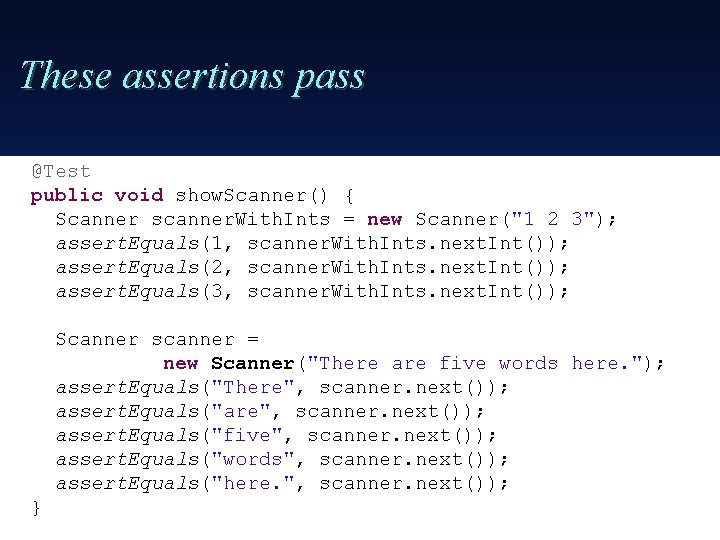
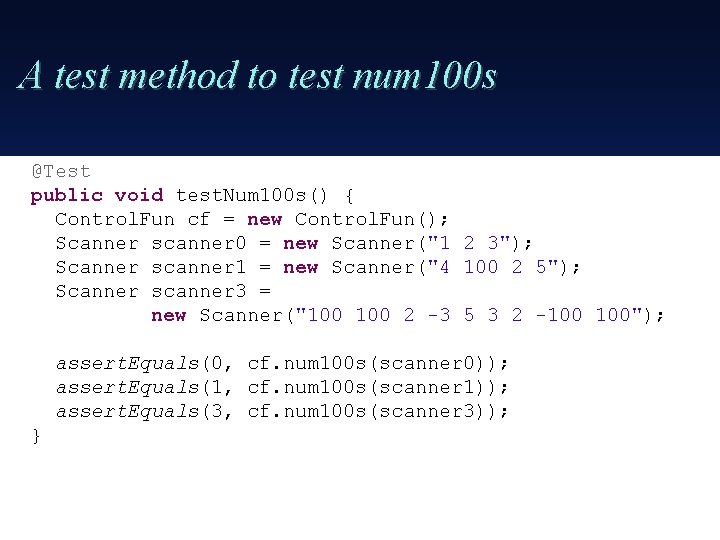
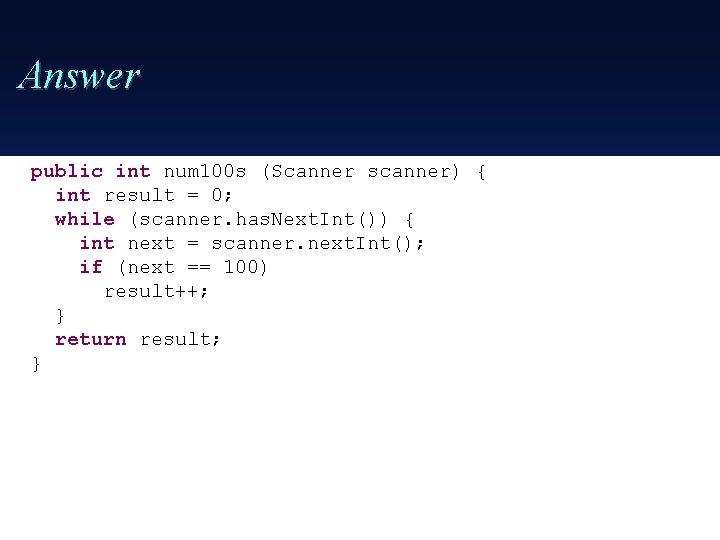
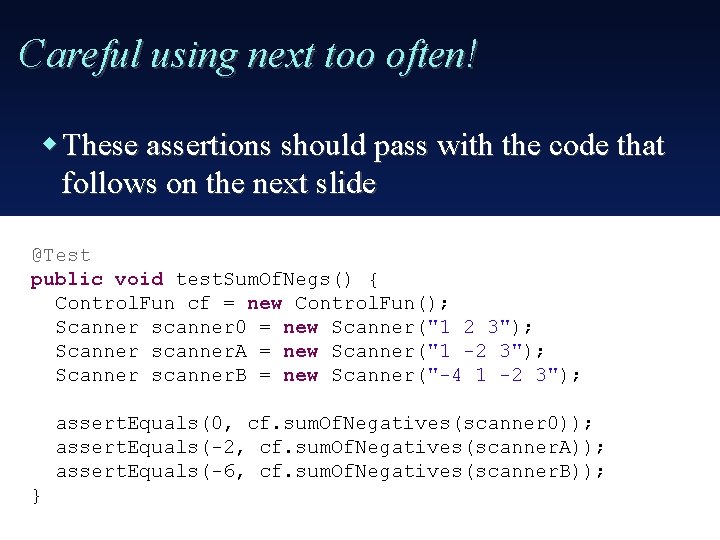
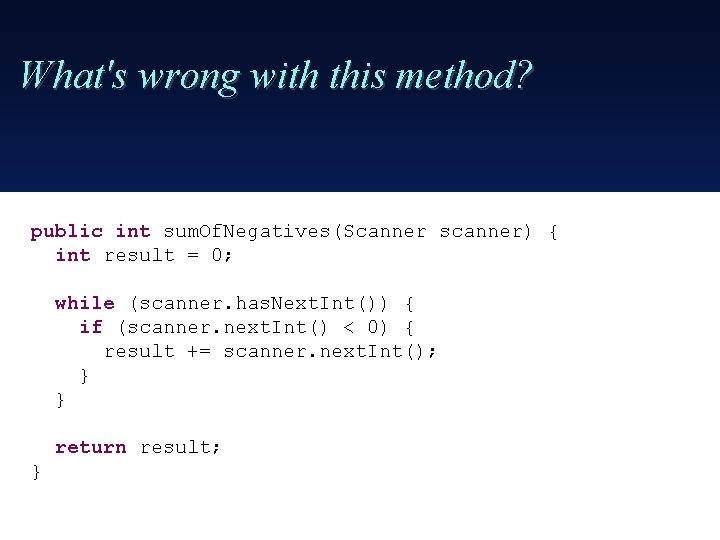
- Slides: 16
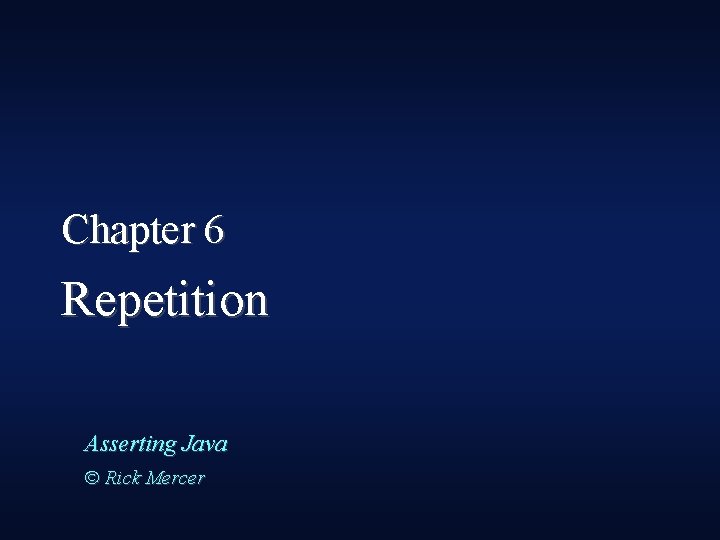
Chapter 6 Repetition Asserting Java © Rick Mercer
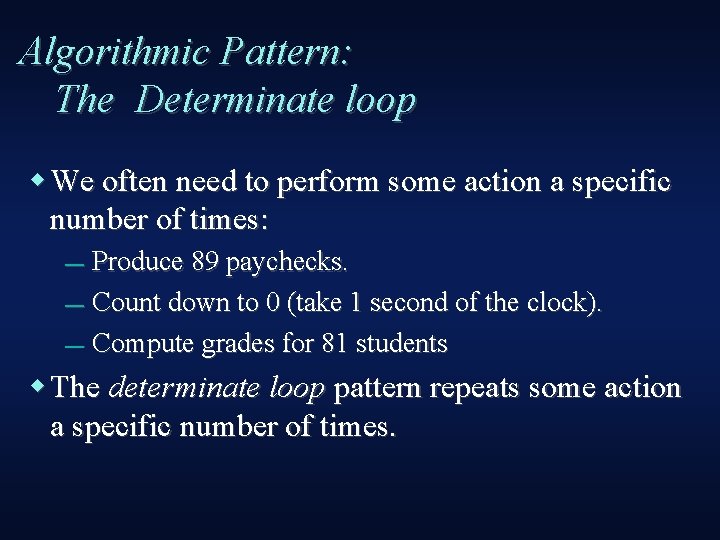
Algorithmic Pattern: The Determinate loop We often need to perform some action a specific number of times: Produce 89 paychecks. — Count down to 0 (take 1 second of the clock). — Compute grades for 81 students — The determinate loop pattern repeats some action a specific number of times.
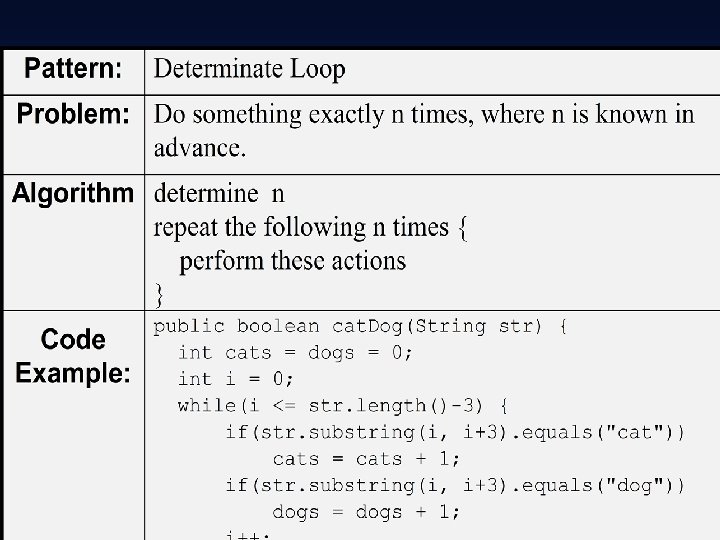
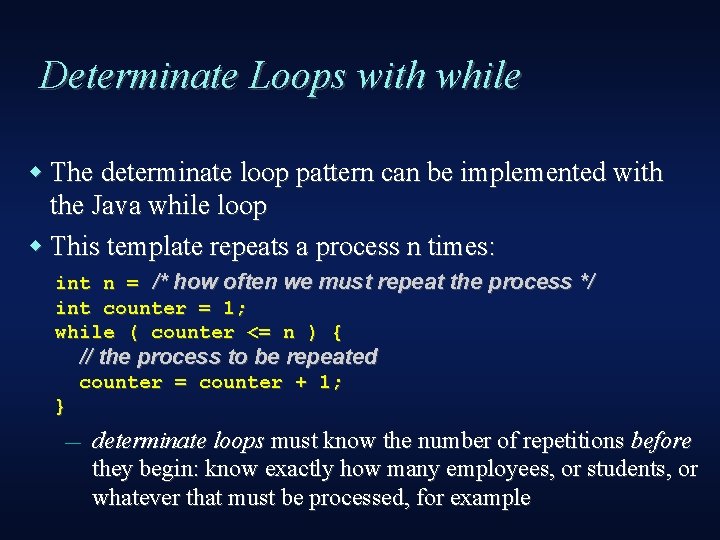
Determinate Loops with while The determinate loop pattern can be implemented with the Java while loop This template repeats a process n times: int n = /* how often we must repeat the process */ int counter = 1; while ( counter <= n ) { // the process to be repeated counter = counter + 1; } — determinate loops must know the number of repetitions before they begin: know exactly how many employees, or students, or whatever that must be processed, for example
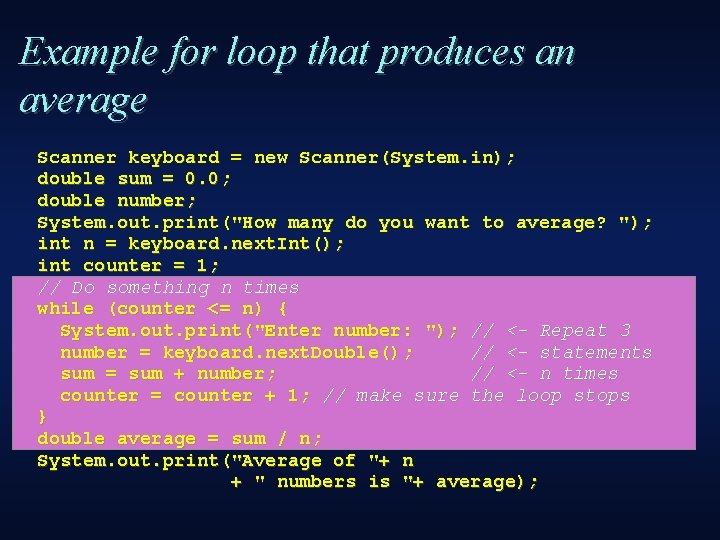
Example for loop that produces an average Scanner keyboard = new Scanner(System. in); double sum = 0. 0; double number; System. out. print("How many do you want to average? "); int n = keyboard. next. Int(); int counter = 1; // Do something n times while (counter <= n) { System. out. print("Enter number: "); // <- Repeat 3 number = keyboard. next. Double(); // <- statements sum = sum + number; // <- n times counter = counter + 1; // make sure the loop stops } double average = sum / n; System. out. print("Average of "+ n + " numbers is "+ average);
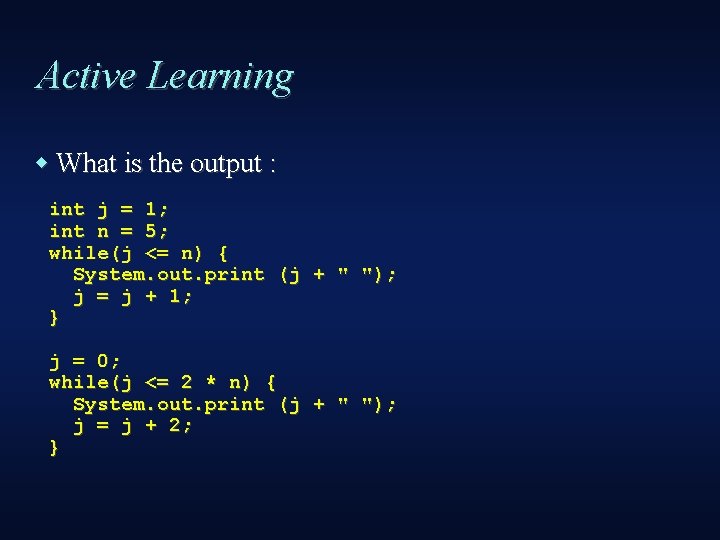
Active Learning What is the output : int j = 1; int n = 5; while(j <= n) { System. out. print (j + " "); j = j + 1; } j = 0; while(j <= 2 * n) { System. out. print (j + " "); j = j + 2; }
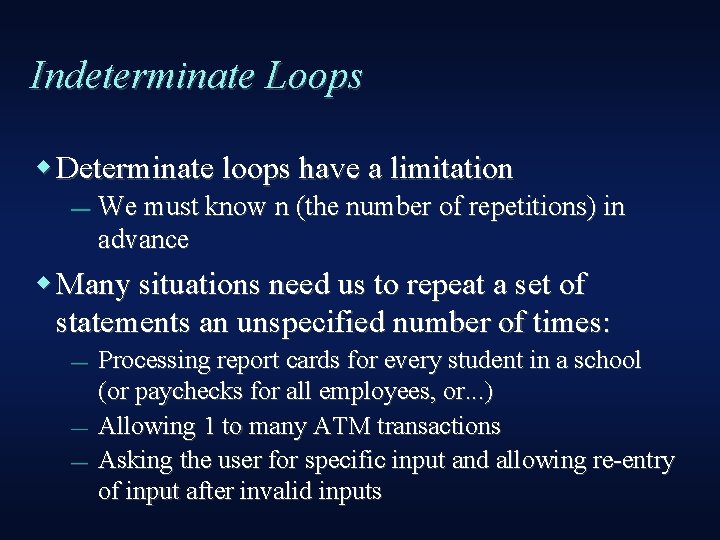
Indeterminate Loops Determinate loops have a limitation — We must know n (the number of repetitions) in advance Many situations need us to repeat a set of statements an unspecified number of times: — — — Processing report cards for every student in a school (or paychecks for all employees, or. . . ) Allowing 1 to many ATM transactions Asking the user for specific input and allowing re-entry of input after invalid inputs
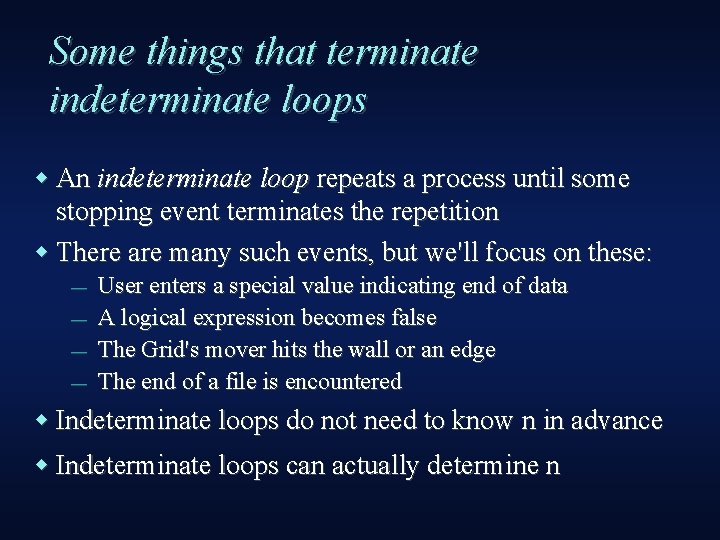
Some things that terminate indeterminate loops An indeterminate loop repeats a process until some stopping event terminates the repetition There are many such events, but we'll focus on these: — — User enters a special value indicating end of data A logical expression becomes false The Grid's mover hits the wall or an edge The end of a file is encountered Indeterminate loops do not need to know n in advance Indeterminate loops can actually determine n
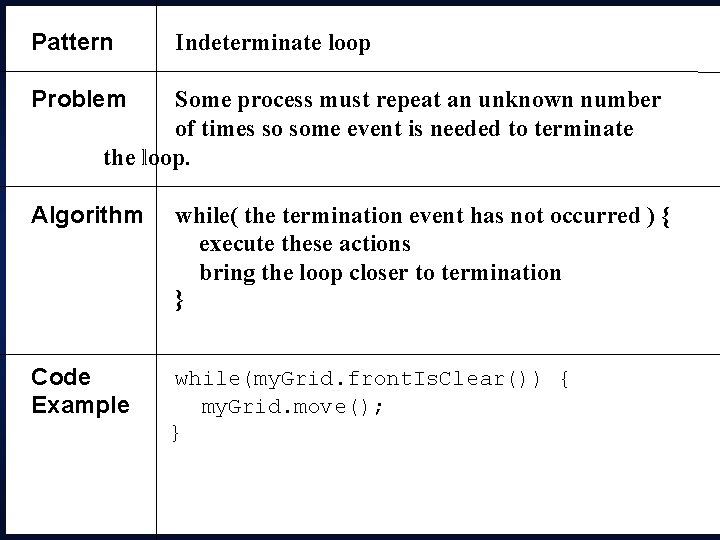
Pattern Indeterminate loop Problem Some process must repeat an unknown number of times so some event is needed to terminate the loop. Algorithm while( the termination event has not occurred ) { execute these actions bring the loop closer to termination } Code Example while(my. Grid. front. Is. Clear()) { my. Grid. move(); }
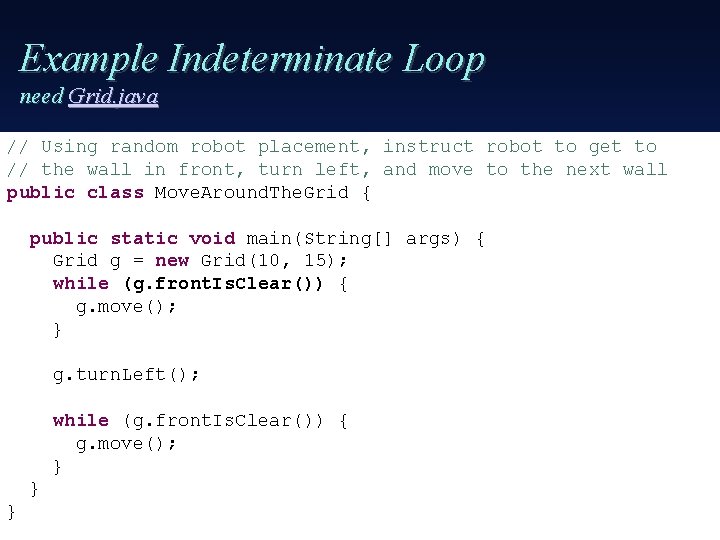
Example Indeterminate Loop need Grid. java // Using random robot placement, instruct robot to get to // the wall in front, turn left, and move to the next wall public class Move. Around. The. Grid { public static void main(String[] args) { Grid g = new Grid(10, 15); while (g. front. Is. Clear()) { g. move(); } g. turn. Left(); while (g. front. Is. Clear()) { g. move(); } } }
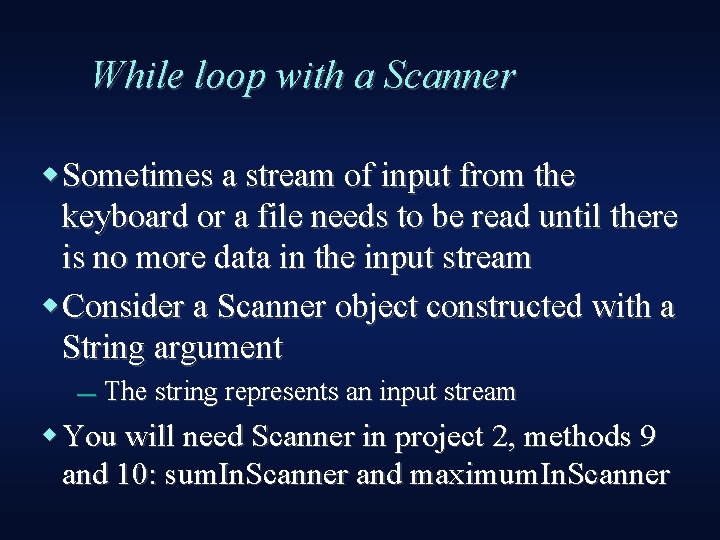
While loop with a Scanner Sometimes a stream of input from the keyboard or a file needs to be read until there is no more data in the input stream Consider a Scanner object constructed with a String argument — The string represents an input stream You will need Scanner in project 2, methods 9 and 10: sum. In. Scanner and maximum. In. Scanner
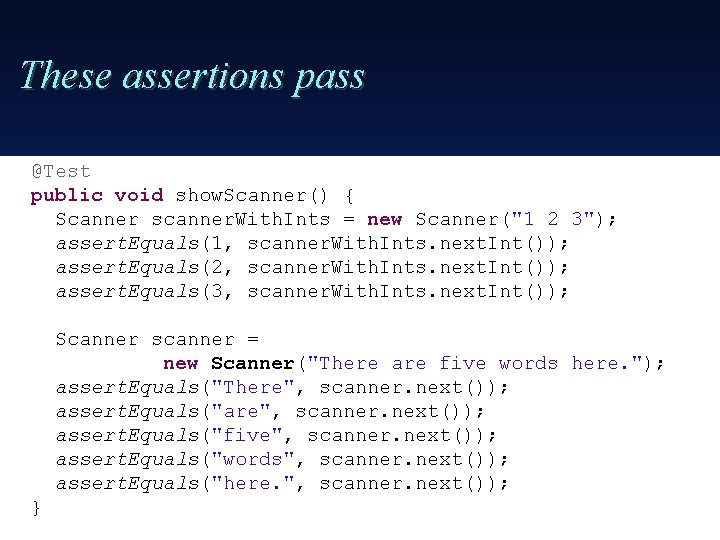
These assertions pass @Test public void show. Scanner() { Scanner scanner. With. Ints = new Scanner("1 2 3"); assert. Equals(1, scanner. With. Ints. next. Int()); assert. Equals(2, scanner. With. Ints. next. Int()); assert. Equals(3, scanner. With. Ints. next. Int()); Scanner scanner = new Scanner("There are five words here. "); assert. Equals("There", scanner. next()); assert. Equals("are", scanner. next()); assert. Equals("five", scanner. next()); assert. Equals("words", scanner. next()); assert. Equals("here. ", scanner. next()); }
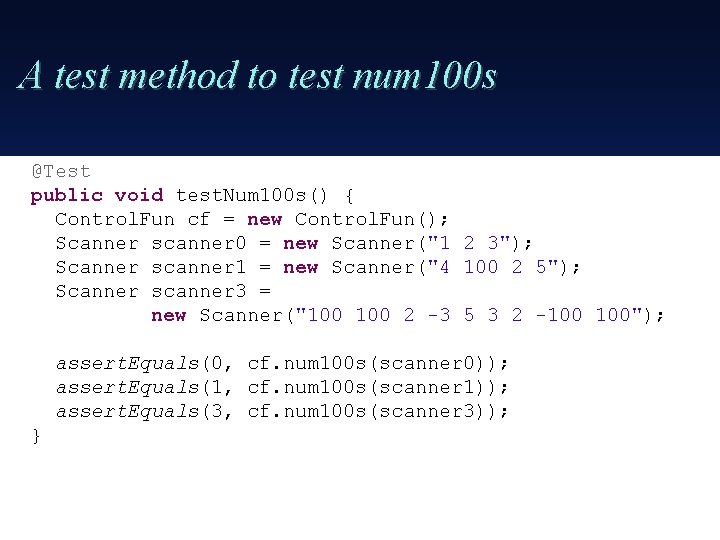
A test method to test num 100 s @Test public void test. Num 100 s() { Control. Fun cf = new Control. Fun(); Scanner scanner 0 = new Scanner("1 2 3"); Scanner scanner 1 = new Scanner("4 100 2 5"); Scanner scanner 3 = new Scanner("100 2 -3 5 3 2 -100 100"); assert. Equals(0, cf. num 100 s(scanner 0)); assert. Equals(1, cf. num 100 s(scanner 1)); assert. Equals(3, cf. num 100 s(scanner 3)); }
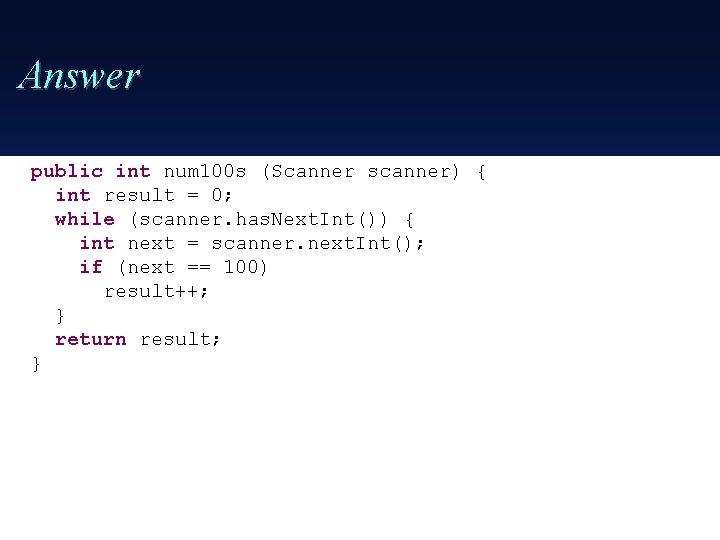
Answer public int num 100 s (Scanner scanner) { int result = 0; while (scanner. has. Next. Int()) { int next = scanner. next. Int(); if (next == 100) result++; } return result; }
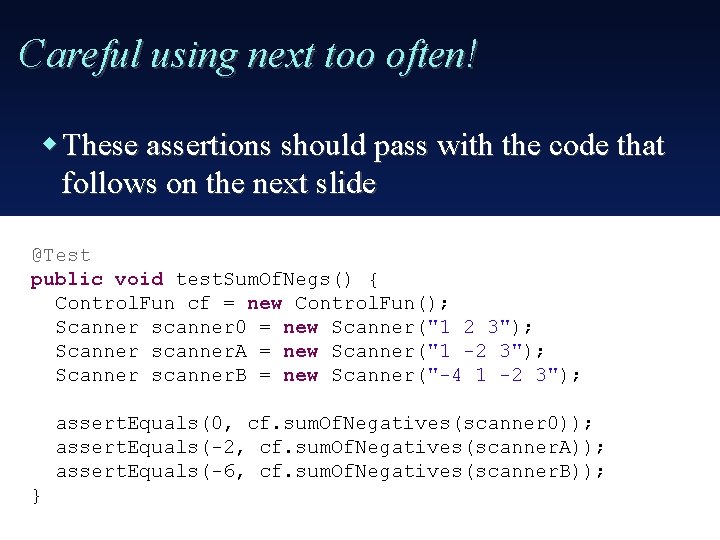
Careful using next too often! These assertions should pass with the code that follows on the next slide @Test public void test. Sum. Of. Negs() { Control. Fun cf = new Control. Fun(); Scanner scanner 0 = new Scanner("1 2 3"); Scanner scanner. A = new Scanner("1 -2 3"); Scanner scanner. B = new Scanner("-4 1 -2 3"); assert. Equals(0, cf. sum. Of. Negatives(scanner 0)); assert. Equals(-2, cf. sum. Of. Negatives(scanner. A)); assert. Equals(-6, cf. sum. Of. Negatives(scanner. B)); }
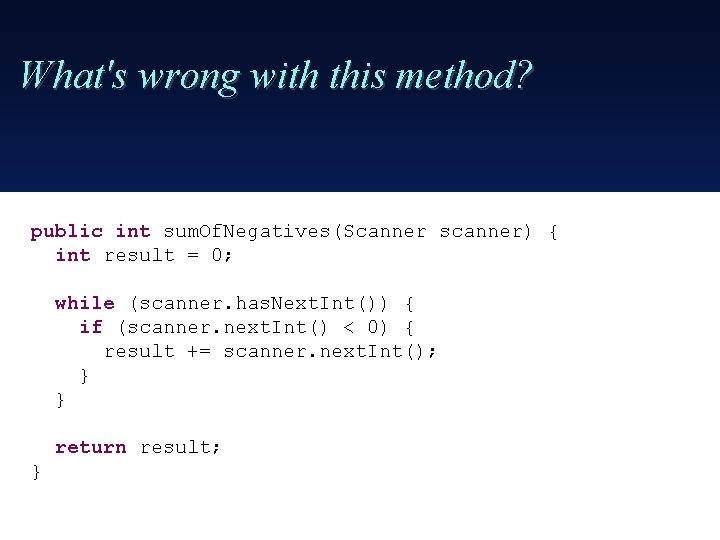
What's wrong with this method? public int sum. Of. Negatives(Scanner scanner) { int result = 0; while (scanner. has. Next. Int()) { if (scanner. next. Int() < 0) { result += scanner. next. Int(); } } return result; }
Algorithmic trading singapore
Asm chart for moore machine
Abstraction computer science gcse
Algorithmic nuggets in content delivery
Algorithmic cost modelling
Algorithmic graph theory and perfect graphs
Cara membuat algoritma yang baik dan benar
Introduction to algorithmic trading strategies
Introduction to algorithmic trading strategies
Algorithm analysis examples
Low frequency algorithmic trading
Introduction to algorithmic trading strategies
Algorithmic adc
Kalman filter trading strategy
Event log correlation
Asm chart examples
Gdpr algorithmic bias