Computer Science 312 Whats New in Java 8
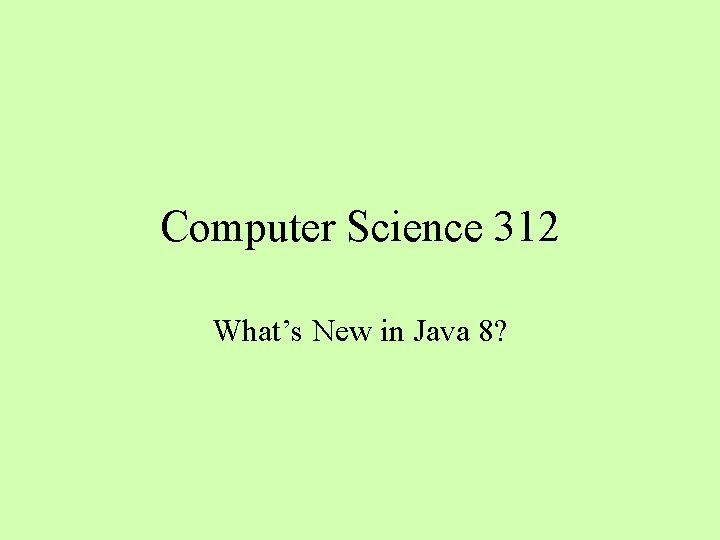
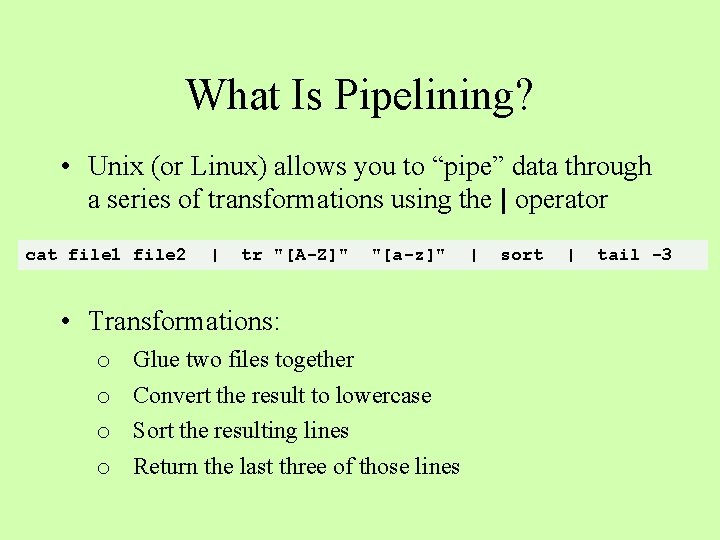
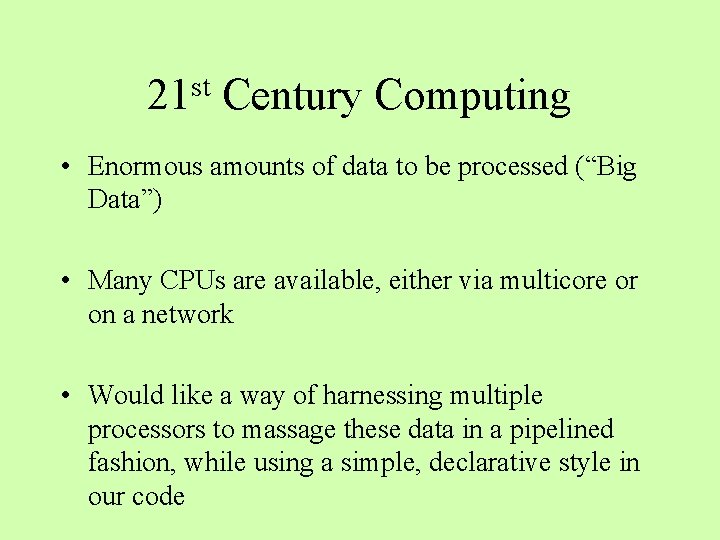
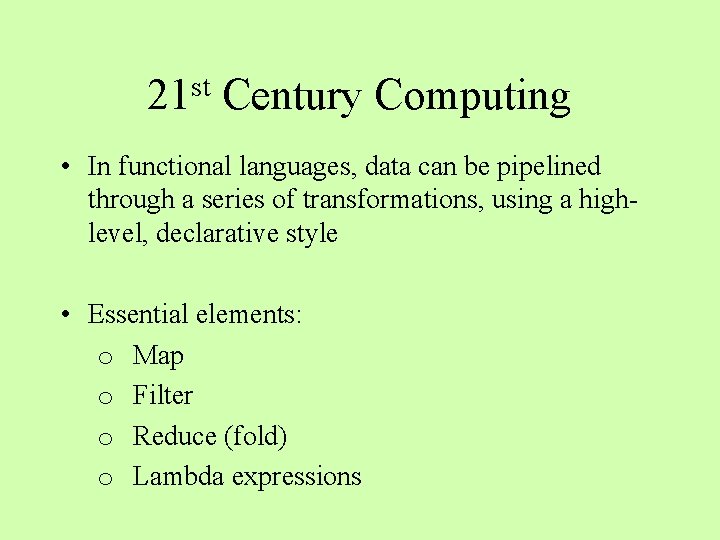
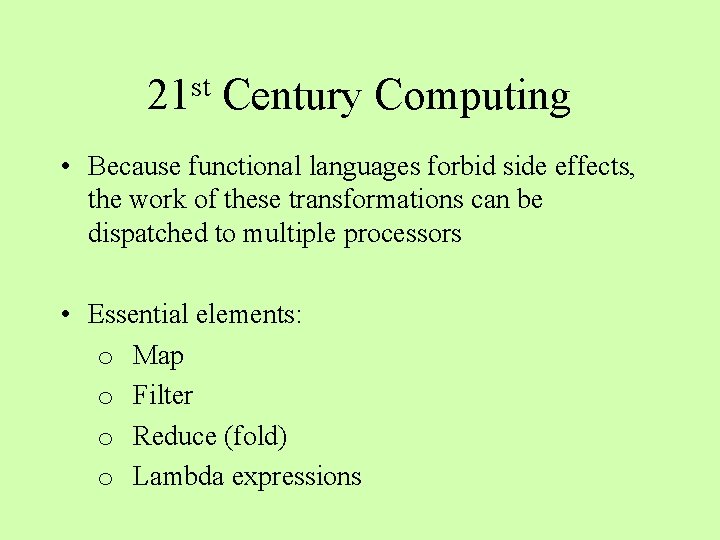
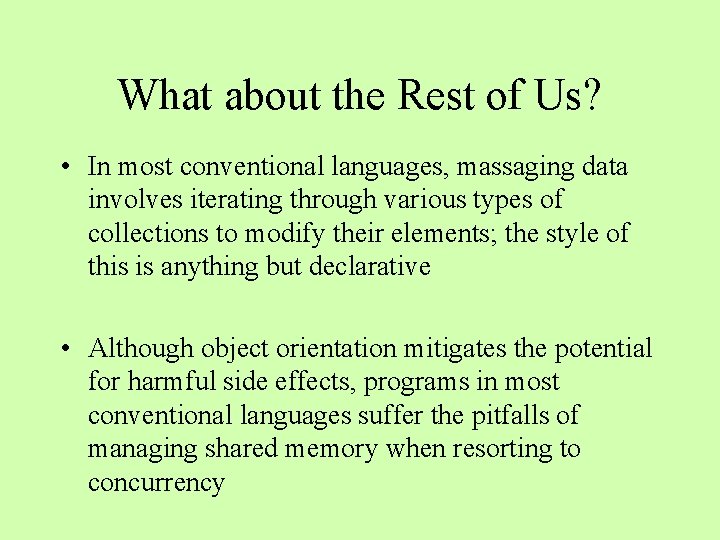
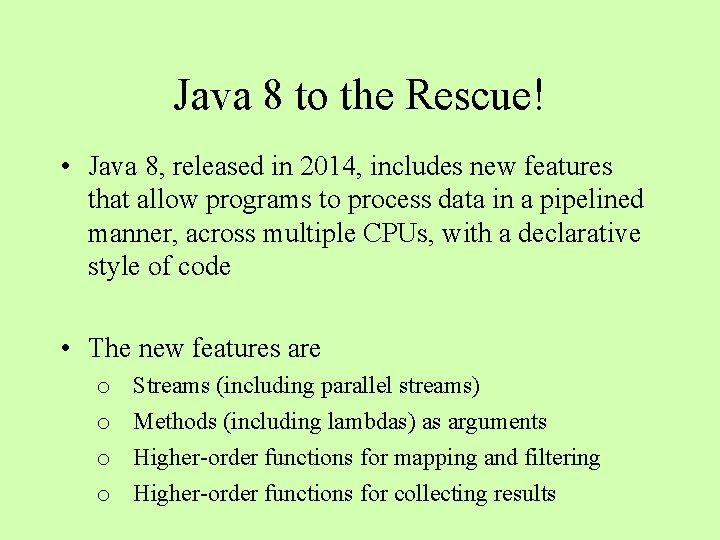
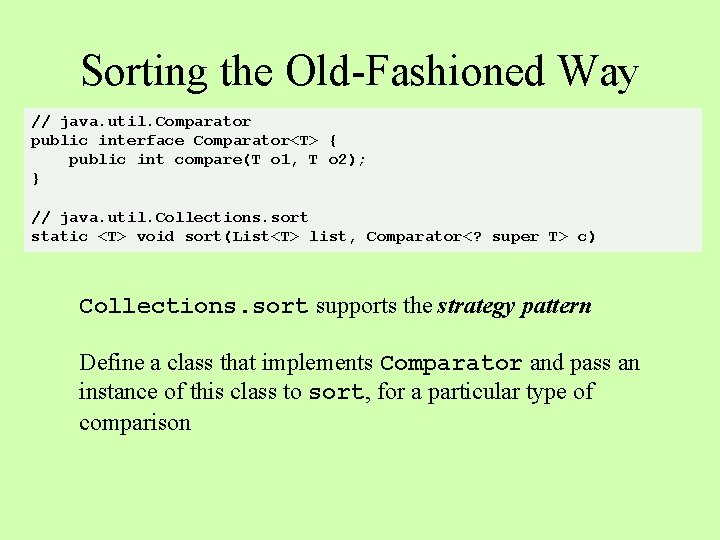
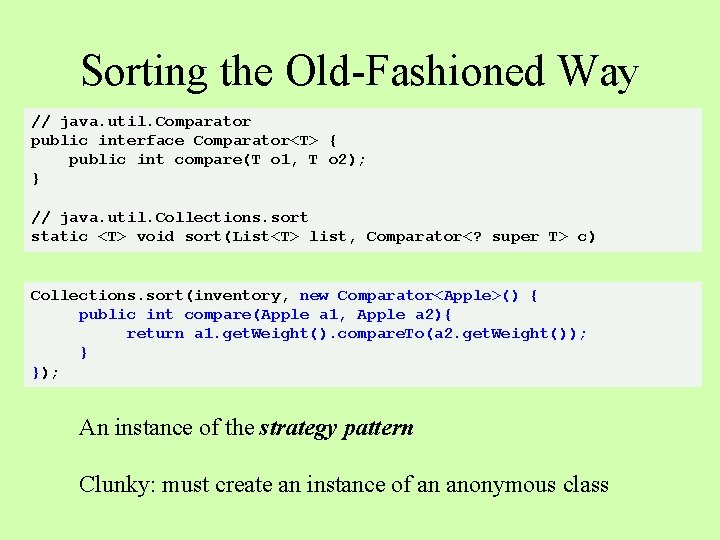
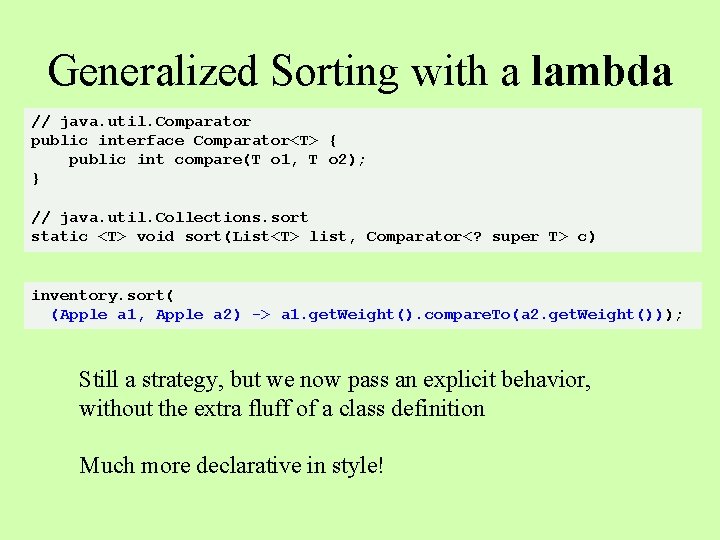
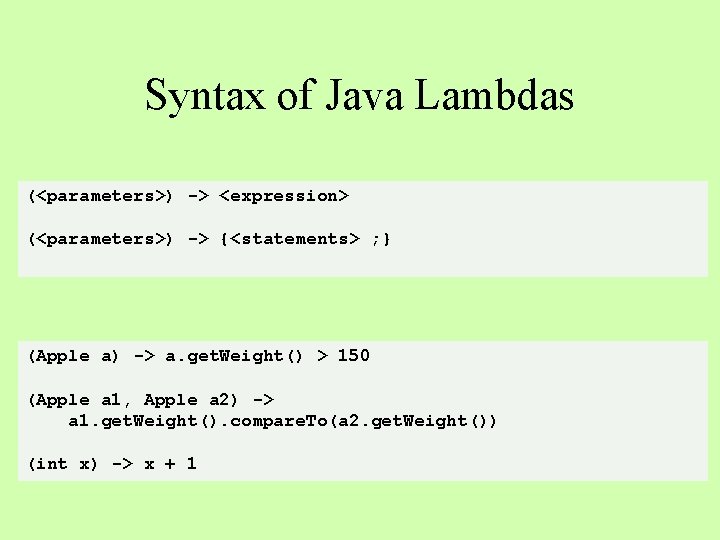
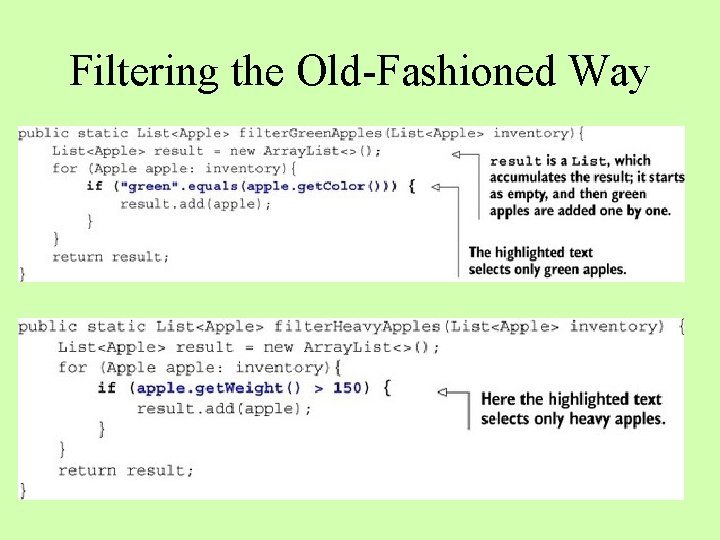
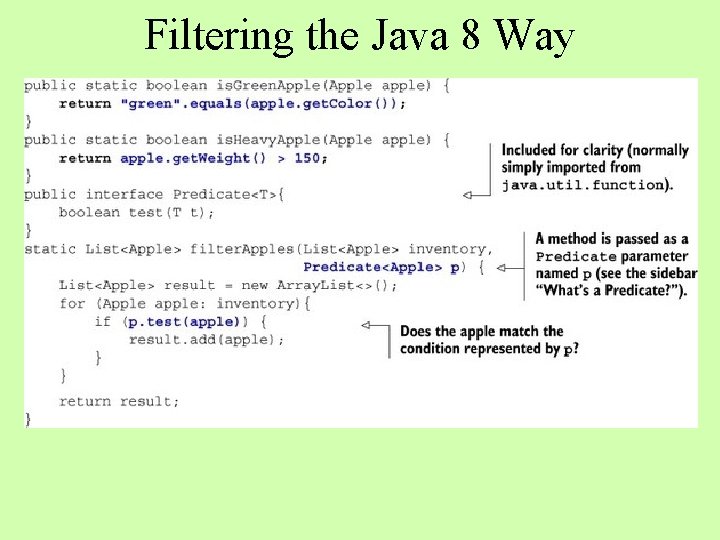
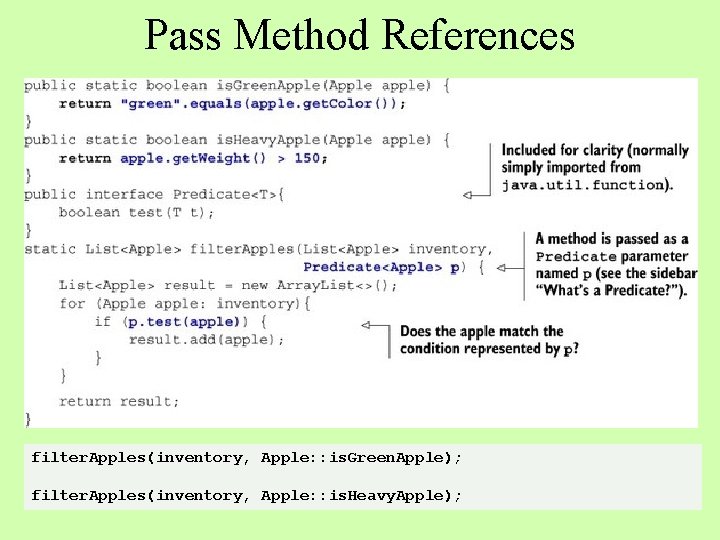
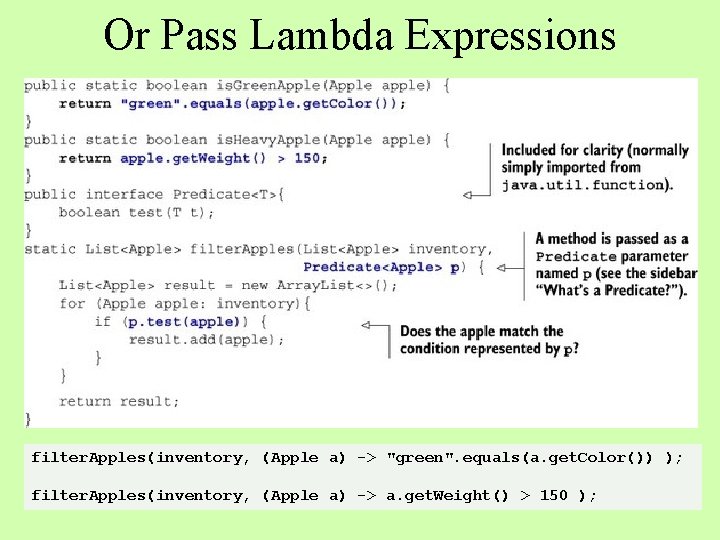
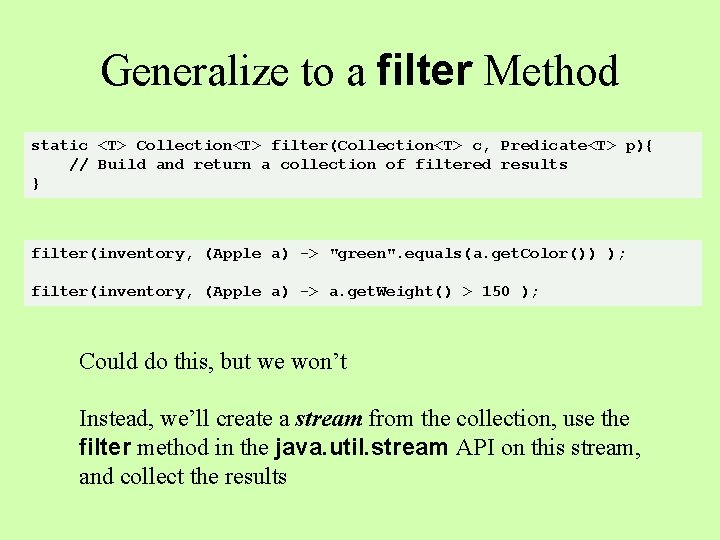
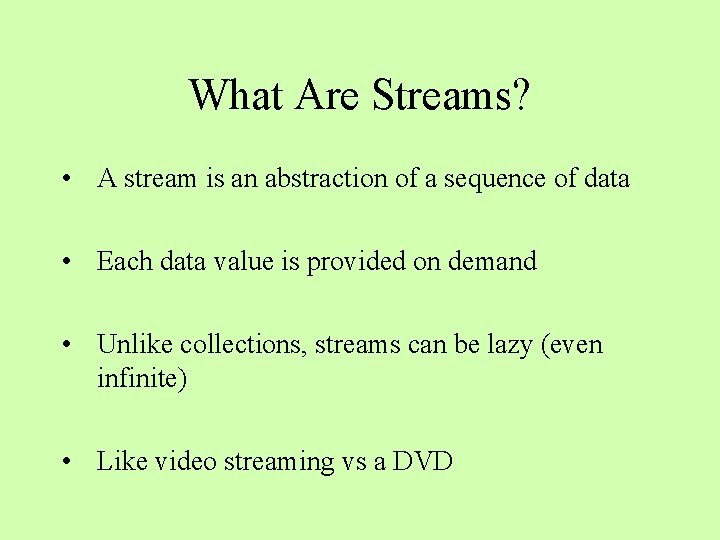
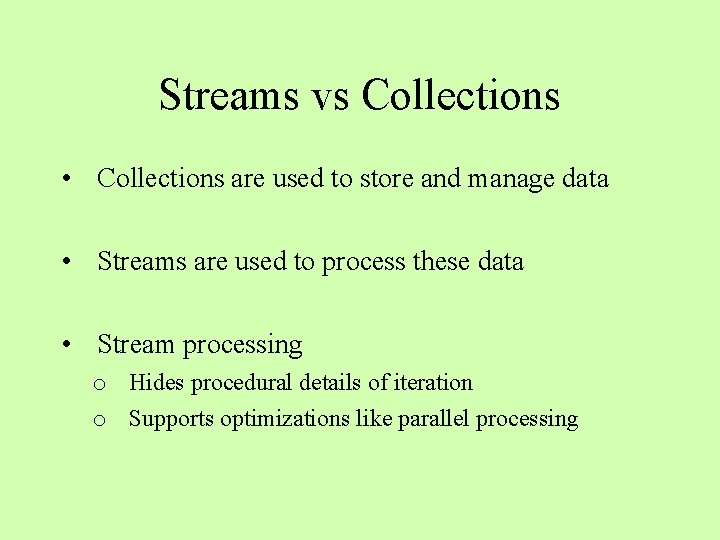
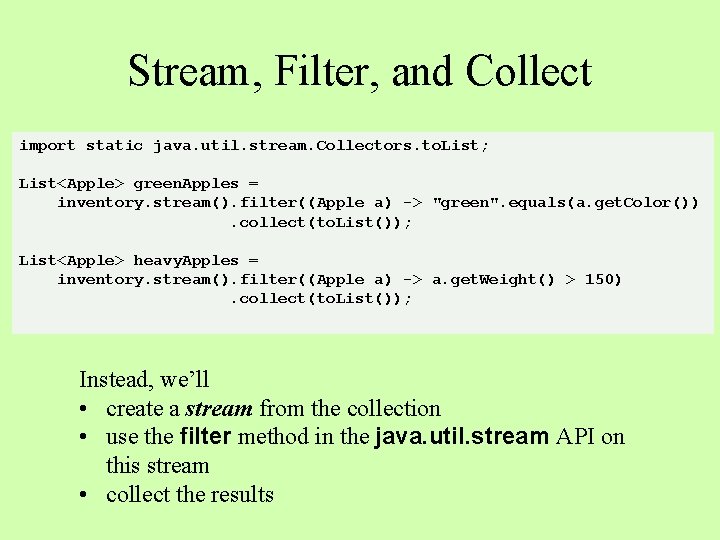
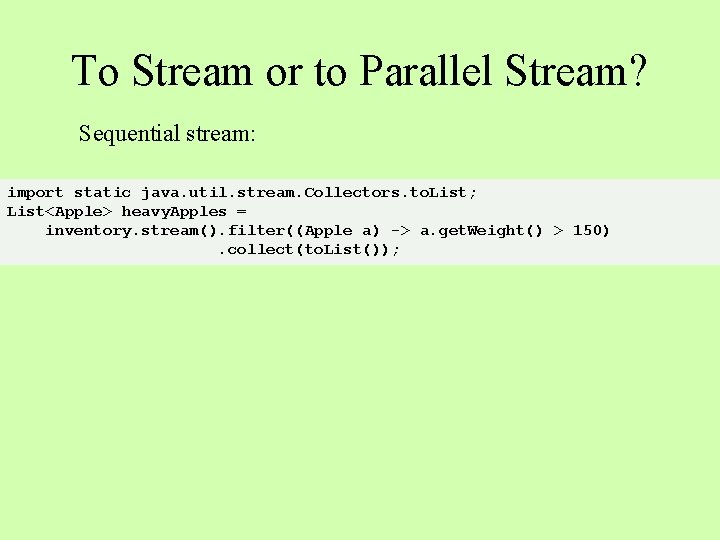
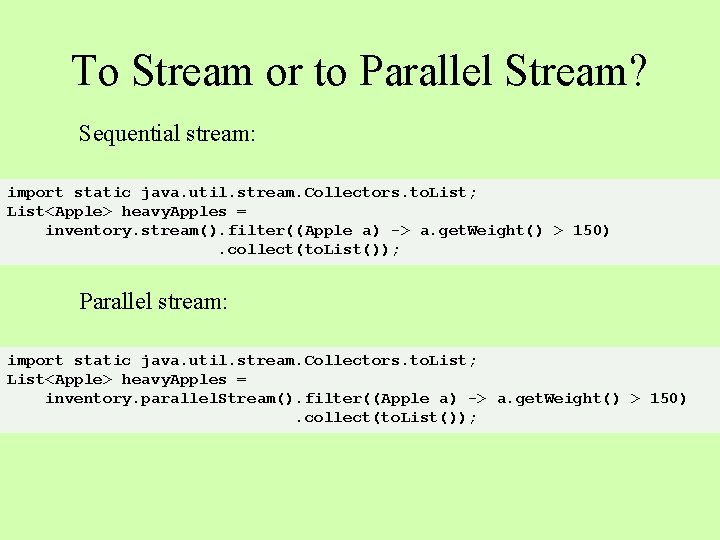
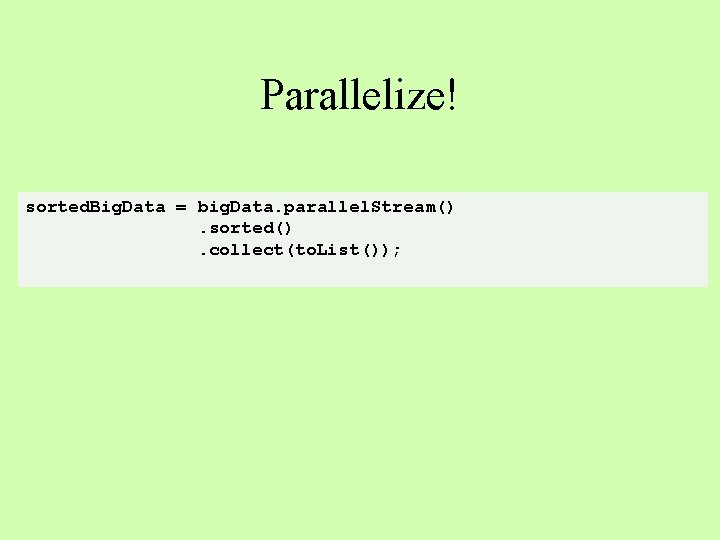
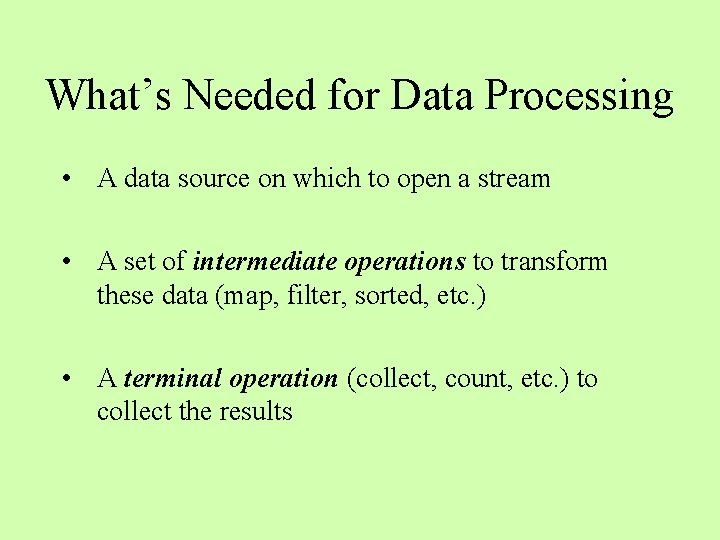
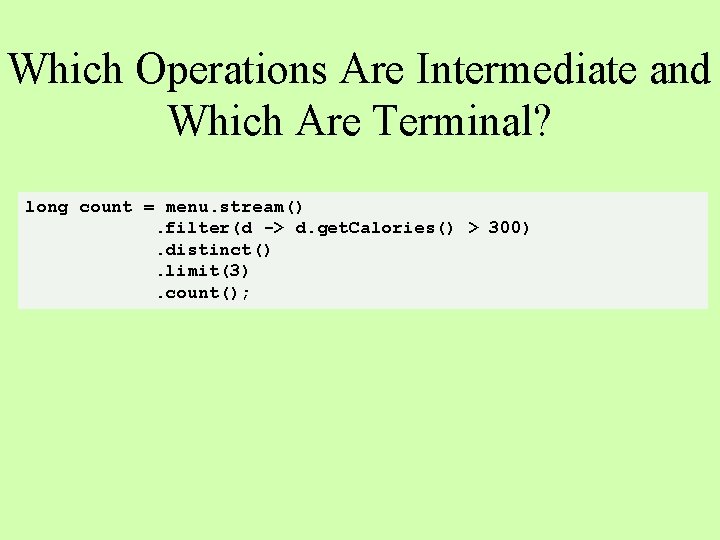
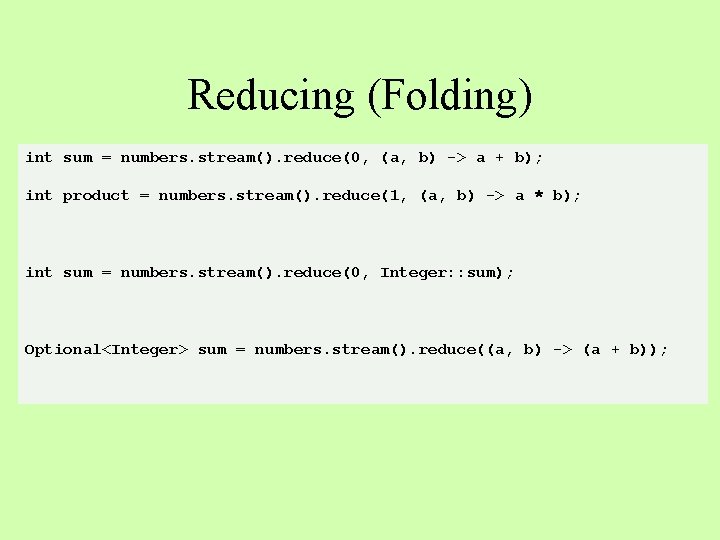
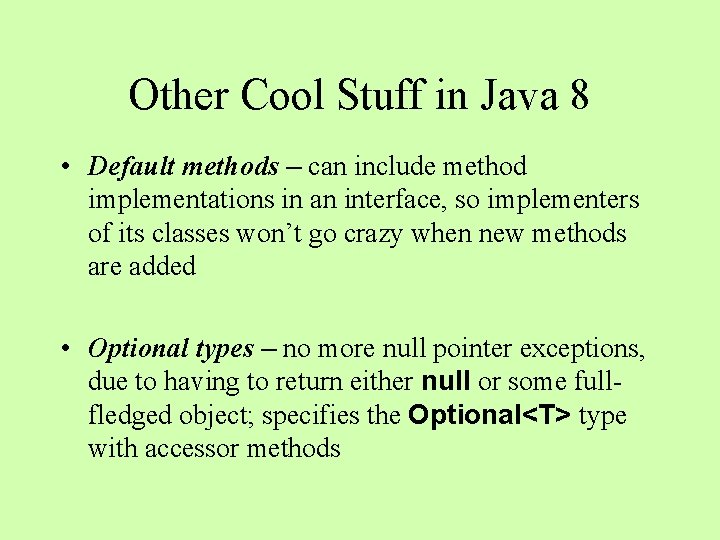
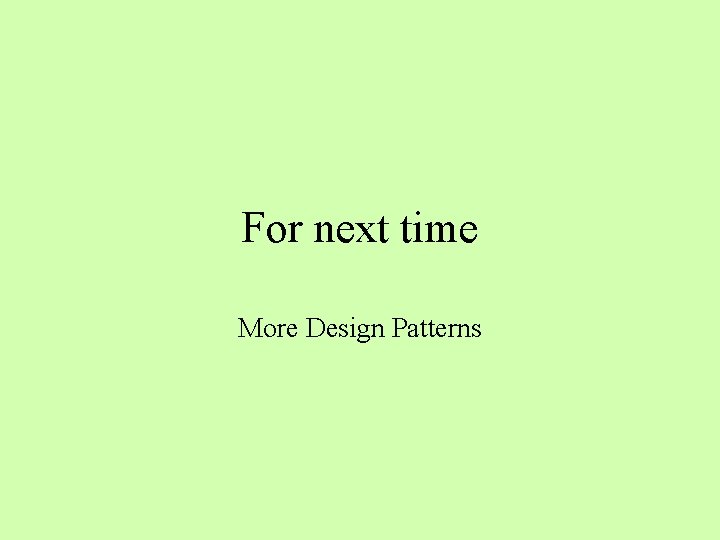
- Slides: 27
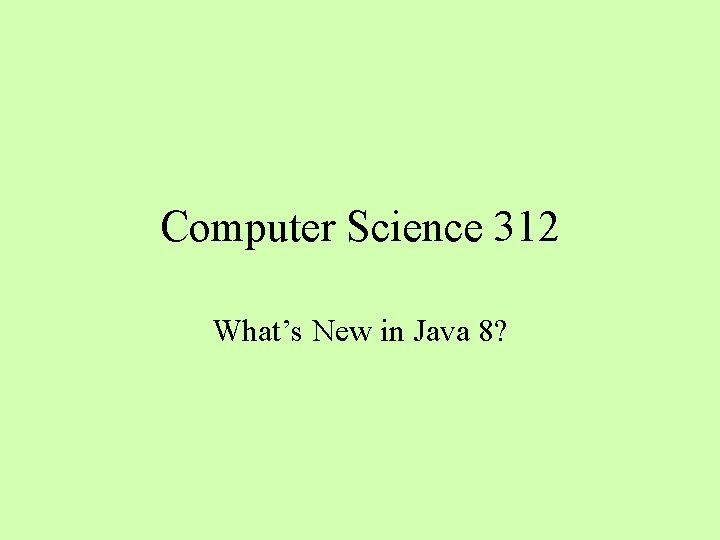
Computer Science 312 What’s New in Java 8?
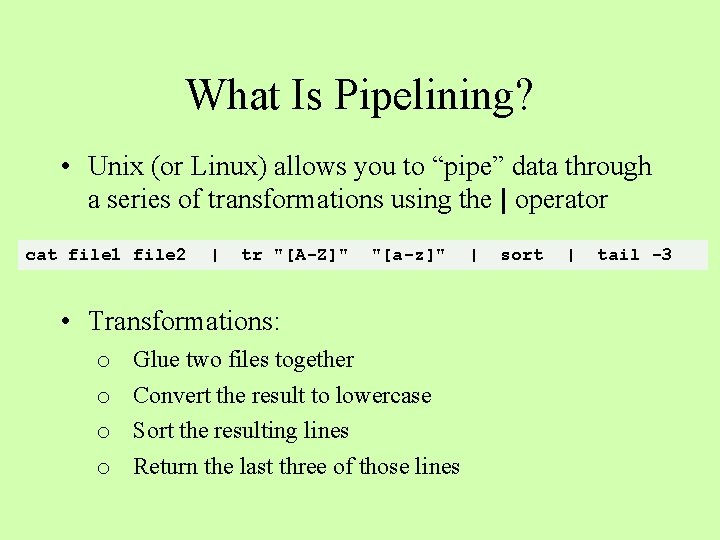
What Is Pipelining? • Unix (or Linux) allows you to “pipe” data through a series of transformations using the | operator cat file 1 file 2 | tr "[A-Z]" "[a-z]" • Transformations: o o Glue two files together Convert the result to lowercase Sort the resulting lines Return the last three of those lines | sort | tail -3
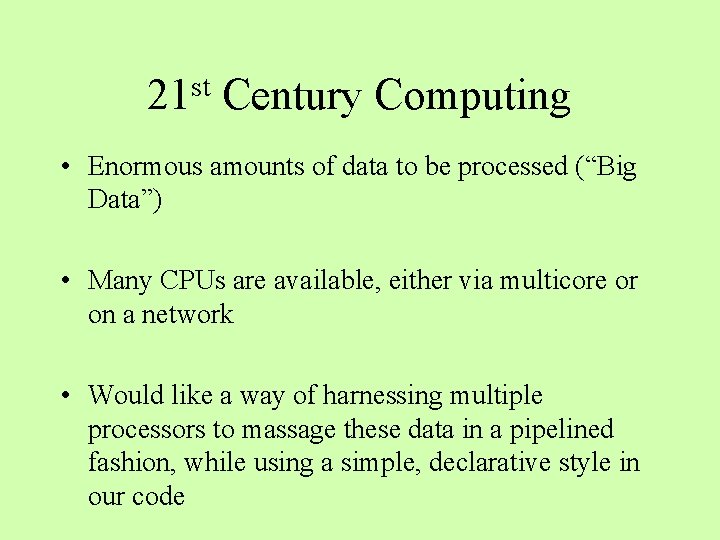
st 21 Century Computing • Enormous amounts of data to be processed (“Big Data”) • Many CPUs are available, either via multicore or on a network • Would like a way of harnessing multiple processors to massage these data in a pipelined fashion, while using a simple, declarative style in our code
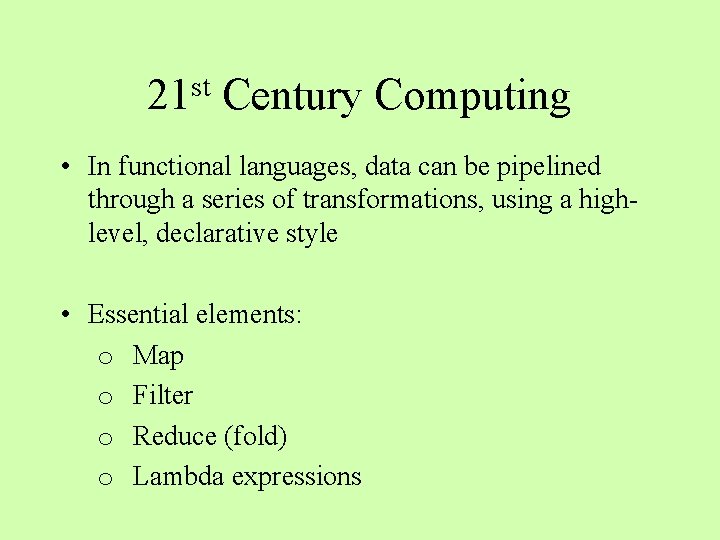
st 21 Century Computing • In functional languages, data can be pipelined through a series of transformations, using a highlevel, declarative style • Essential elements: o Map o Filter o Reduce (fold) o Lambda expressions
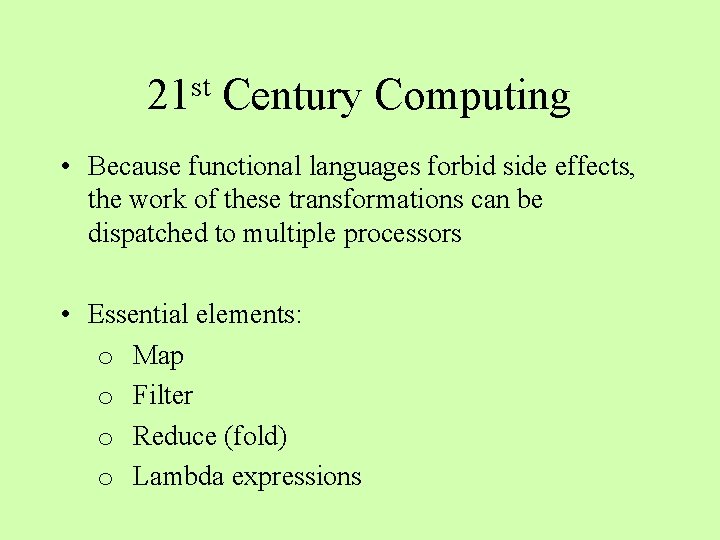
st 21 Century Computing • Because functional languages forbid side effects, the work of these transformations can be dispatched to multiple processors • Essential elements: o Map o Filter o Reduce (fold) o Lambda expressions
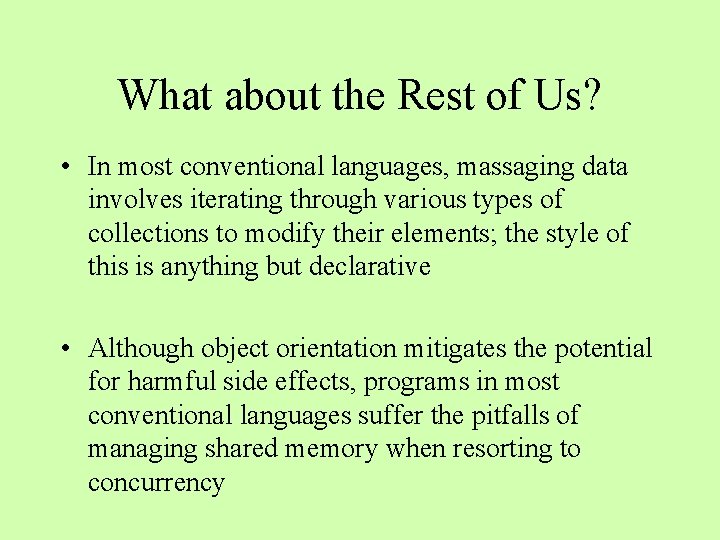
What about the Rest of Us? • In most conventional languages, massaging data involves iterating through various types of collections to modify their elements; the style of this is anything but declarative • Although object orientation mitigates the potential for harmful side effects, programs in most conventional languages suffer the pitfalls of managing shared memory when resorting to concurrency
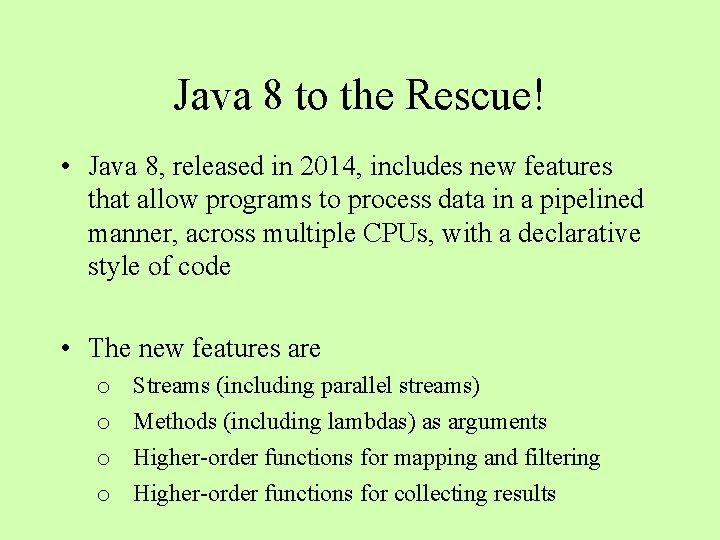
Java 8 to the Rescue! • Java 8, released in 2014, includes new features that allow programs to process data in a pipelined manner, across multiple CPUs, with a declarative style of code • The new features are o o Streams (including parallel streams) Methods (including lambdas) as arguments Higher-order functions for mapping and filtering Higher-order functions for collecting results
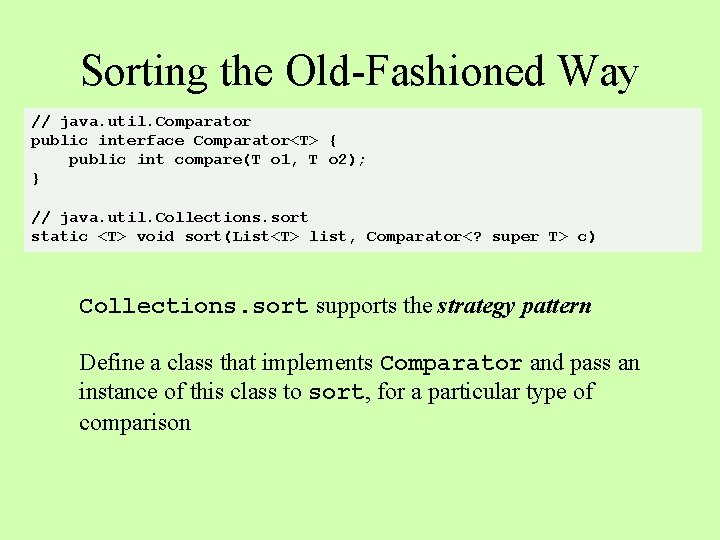
Sorting the Old-Fashioned Way // java. util. Comparator public interface Comparator<T> { public int compare(T o 1, T o 2); } // java. util. Collections. sort static <T> void sort(List<T> list, Comparator<? super T> c) Collections. sort supports the strategy pattern Define a class that implements Comparator and pass an instance of this class to sort, for a particular type of comparison
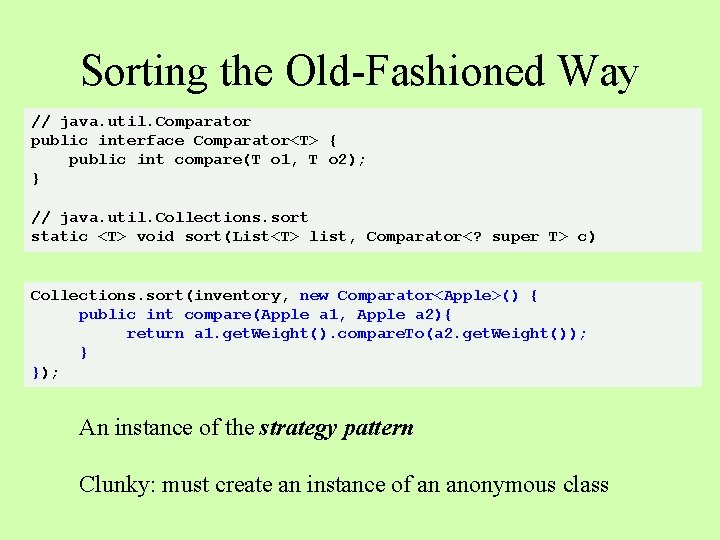
Sorting the Old-Fashioned Way // java. util. Comparator public interface Comparator<T> { public int compare(T o 1, T o 2); } // java. util. Collections. sort static <T> void sort(List<T> list, Comparator<? super T> c) Collections. sort(inventory, new Comparator<Apple>() { public int compare(Apple a 1, Apple a 2){ return a 1. get. Weight(). compare. To(a 2. get. Weight()); } }); An instance of the strategy pattern Clunky: must create an instance of an anonymous class
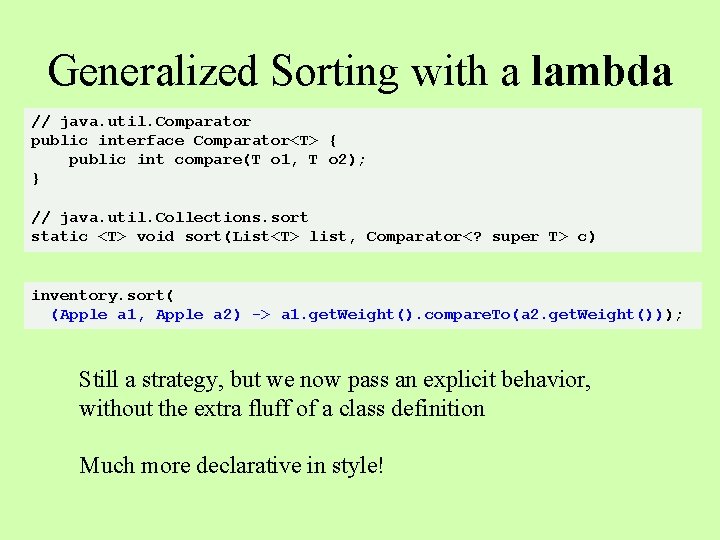
Generalized Sorting with a lambda // java. util. Comparator public interface Comparator<T> { public int compare(T o 1, T o 2); } // java. util. Collections. sort static <T> void sort(List<T> list, Comparator<? super T> c) inventory. sort( (Apple a 1, Apple a 2) -> a 1. get. Weight(). compare. To(a 2. get. Weight())); Still a strategy, but we now pass an explicit behavior, without the extra fluff of a class definition Much more declarative in style!
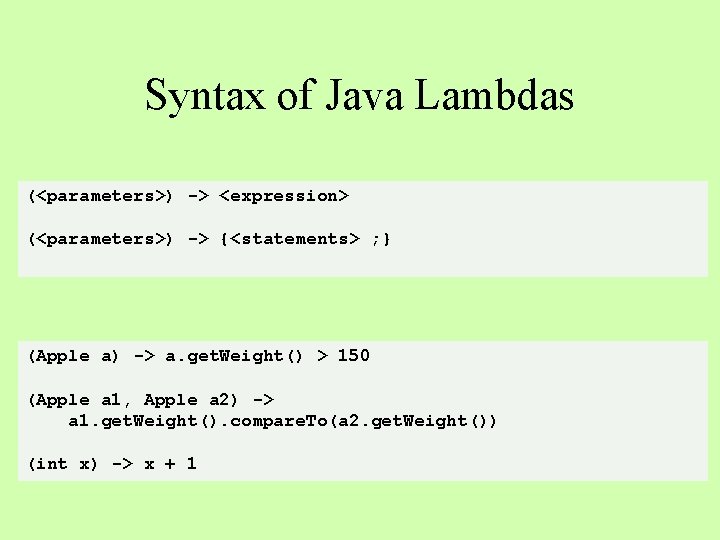
Syntax of Java Lambdas (<parameters>) -> <expression> (<parameters>) -> {<statements> ; } (Apple a) -> a. get. Weight() > 150 (Apple a 1, Apple a 2) -> a 1. get. Weight(). compare. To(a 2. get. Weight()) (int x) -> x + 1
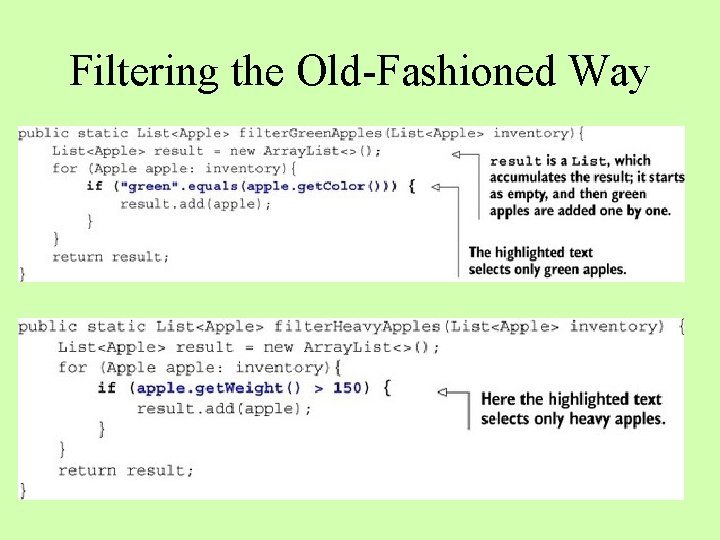
Filtering the Old-Fashioned Way
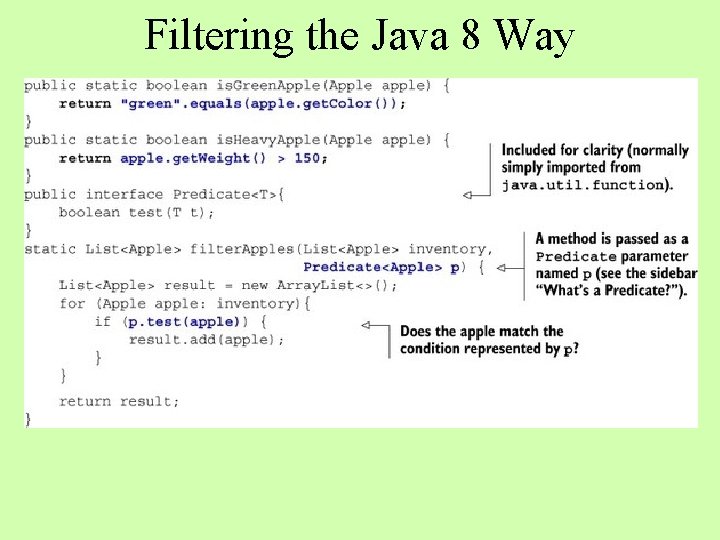
Filtering the Java 8 Way
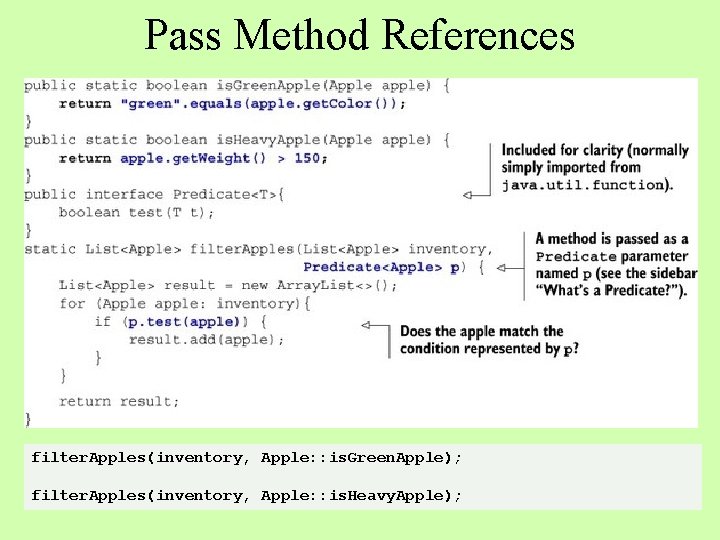
Pass Method References filter. Apples(inventory, Apple: : is. Green. Apple); filter. Apples(inventory, Apple: : is. Heavy. Apple);
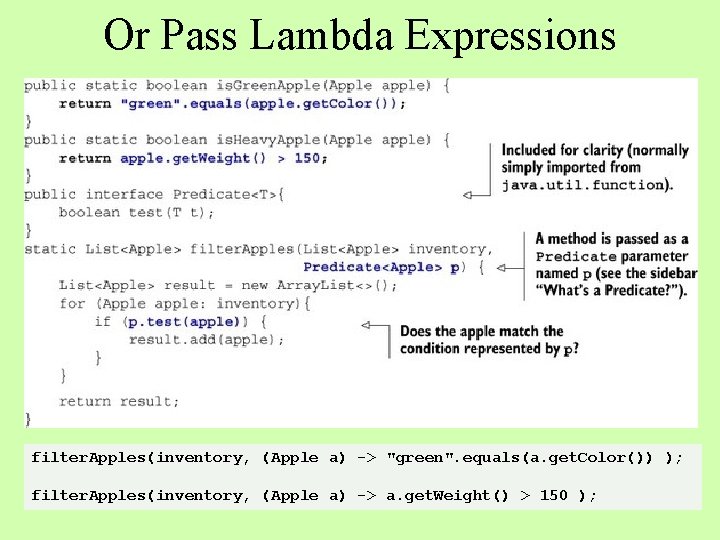
Or Pass Lambda Expressions filter. Apples(inventory, (Apple a) -> "green". equals(a. get. Color()) ); filter. Apples(inventory, (Apple a) -> a. get. Weight() > 150 );
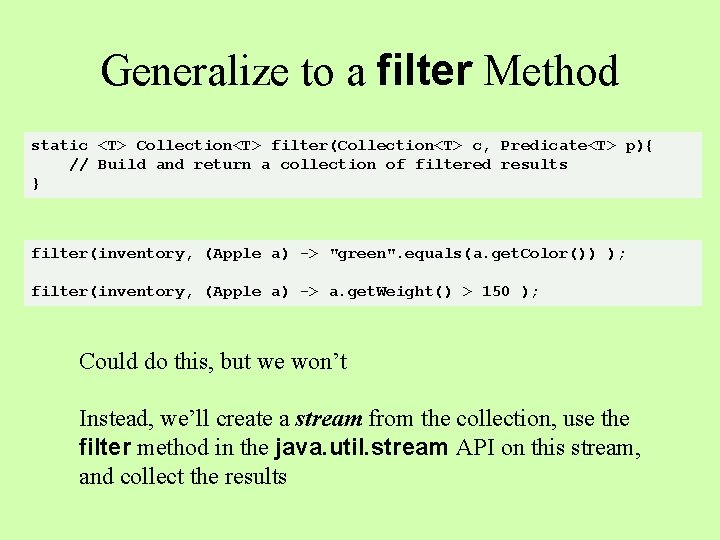
Generalize to a filter Method static <T> Collection<T> filter(Collection<T> c, Predicate<T> p){ // Build and return a collection of filtered results } filter(inventory, (Apple a) -> "green". equals(a. get. Color()) ); filter(inventory, (Apple a) -> a. get. Weight() > 150 ); Could do this, but we won’t Instead, we’ll create a stream from the collection, use the filter method in the java. util. stream API on this stream, and collect the results
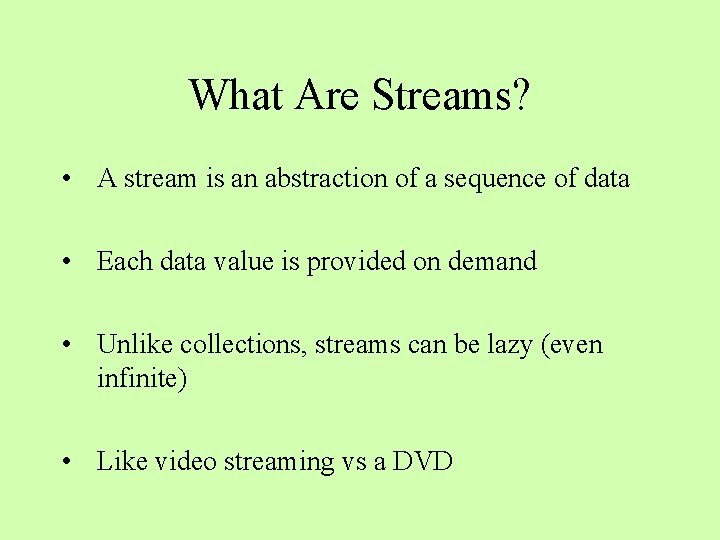
What Are Streams? • A stream is an abstraction of a sequence of data • Each data value is provided on demand • Unlike collections, streams can be lazy (even infinite) • Like video streaming vs a DVD
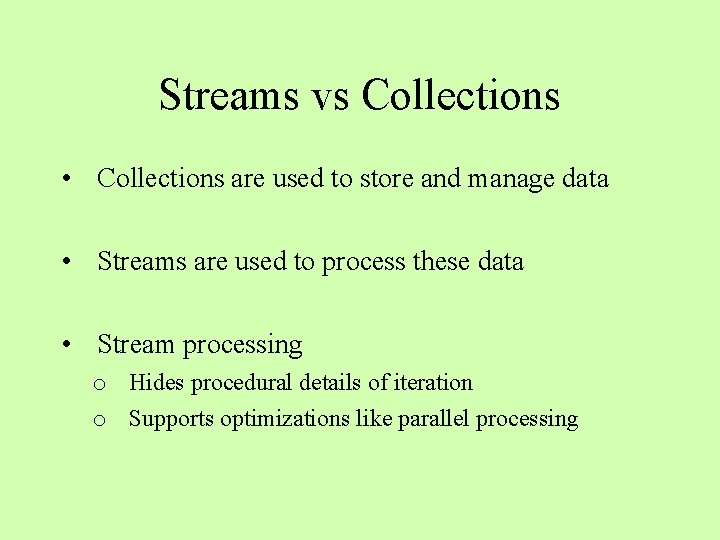
Streams vs Collections • Collections are used to store and manage data • Streams are used to process these data • Stream processing o Hides procedural details of iteration o Supports optimizations like parallel processing
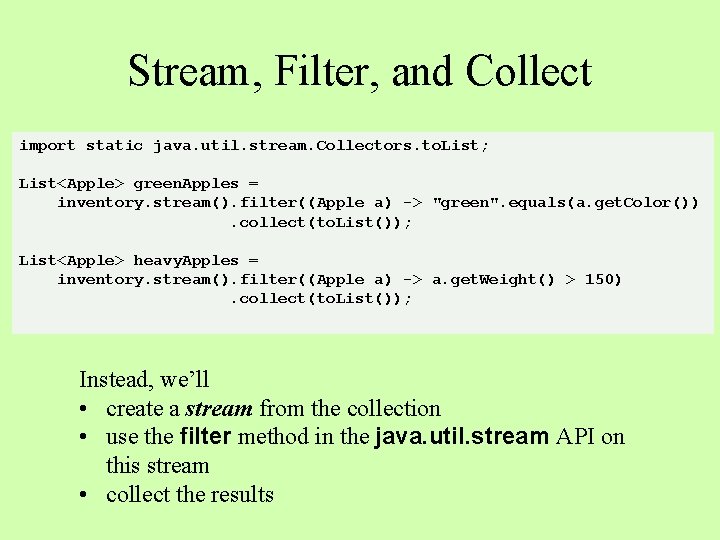
Stream, Filter, and Collect import static java. util. stream. Collectors. to. List; List<Apple> green. Apples = inventory. stream(). filter((Apple a) -> "green". equals(a. get. Color()). collect(to. List()); List<Apple> heavy. Apples = inventory. stream(). filter((Apple a) -> a. get. Weight() > 150). collect(to. List()); Instead, we’ll • create a stream from the collection • use the filter method in the java. util. stream API on this stream • collect the results
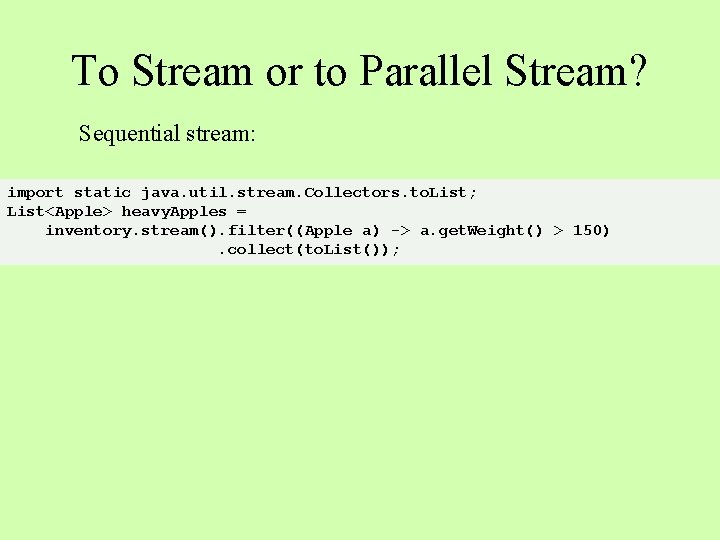
To Stream or to Parallel Stream? Sequential stream: import static java. util. stream. Collectors. to. List; List<Apple> heavy. Apples = inventory. stream(). filter((Apple a) -> a. get. Weight() > 150). collect(to. List());
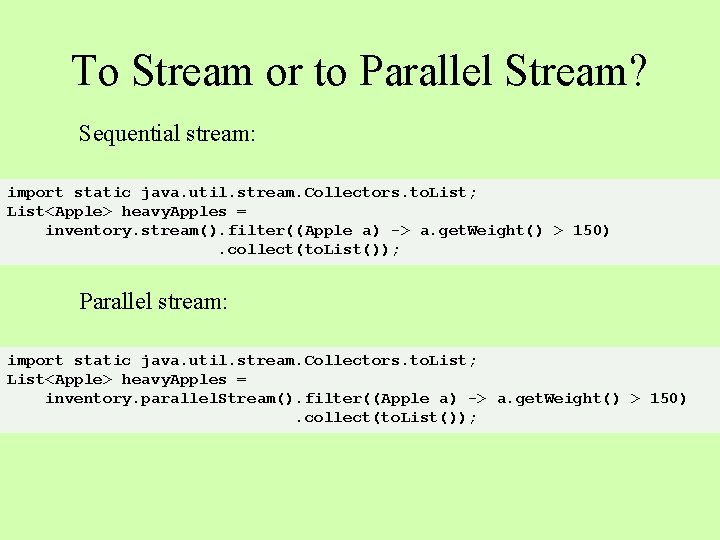
To Stream or to Parallel Stream? Sequential stream: import static java. util. stream. Collectors. to. List; List<Apple> heavy. Apples = inventory. stream(). filter((Apple a) -> a. get. Weight() > 150). collect(to. List()); Parallel stream: import static java. util. stream. Collectors. to. List; List<Apple> heavy. Apples = inventory. parallel. Stream(). filter((Apple a) -> a. get. Weight() > 150). collect(to. List());
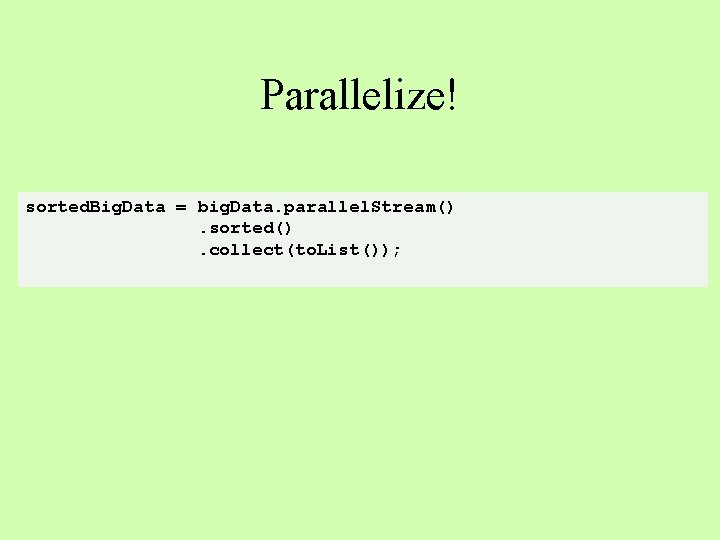
Parallelize! sorted. Big. Data = big. Data. parallel. Stream(). sorted(). collect(to. List());
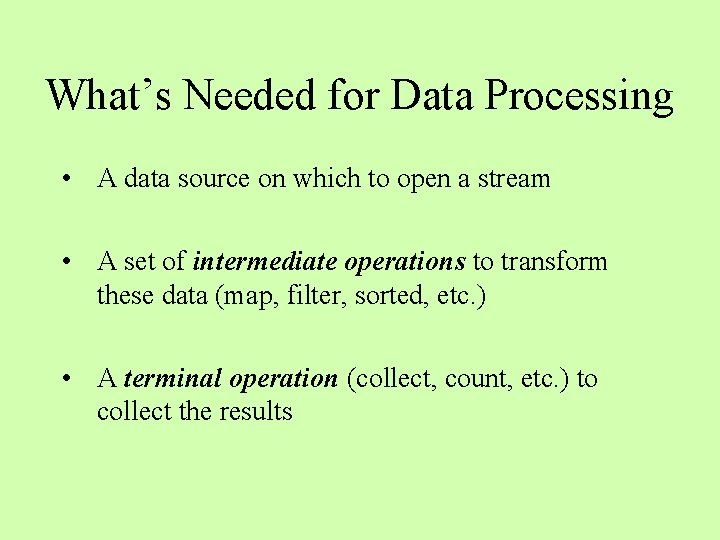
What’s Needed for Data Processing • A data source on which to open a stream • A set of intermediate operations to transform these data (map, filter, sorted, etc. ) • A terminal operation (collect, count, etc. ) to collect the results
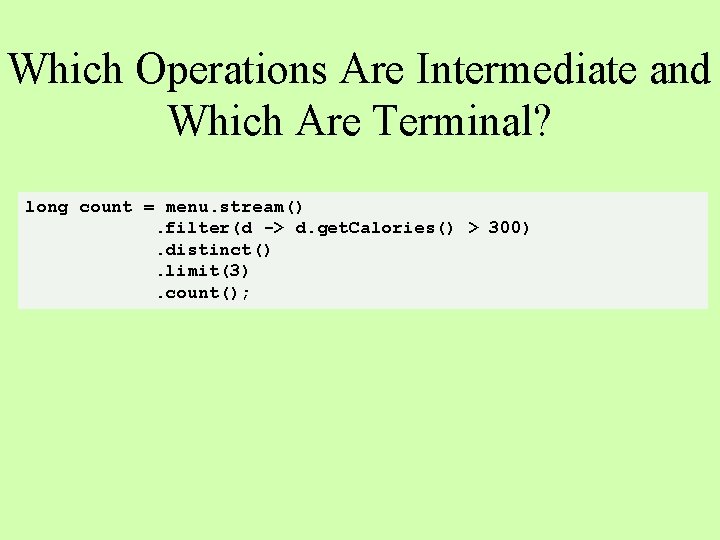
Which Operations Are Intermediate and Which Are Terminal? long count = menu. stream(). filter(d -> d. get. Calories() > 300). distinct(). limit(3). count();
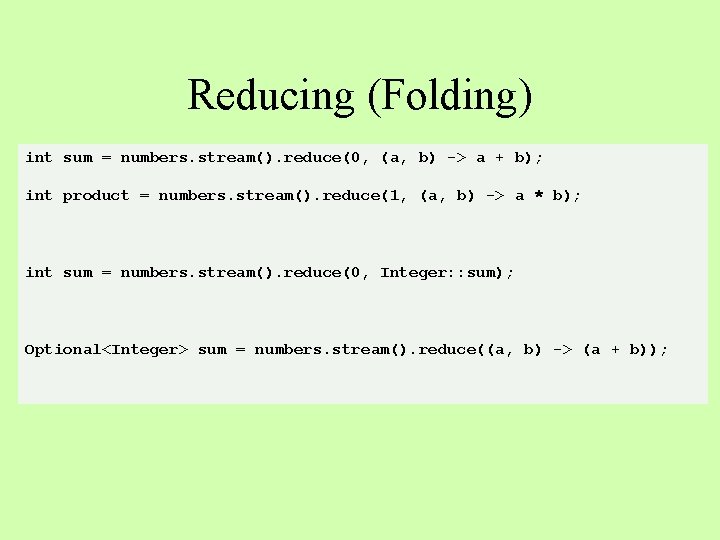
Reducing (Folding) int sum = numbers. stream(). reduce(0, (a, b) -> a + b); int product = numbers. stream(). reduce(1, (a, b) -> a * b); int sum = numbers. stream(). reduce(0, Integer: : sum); Optional<Integer> sum = numbers. stream(). reduce((a, b) -> (a + b));
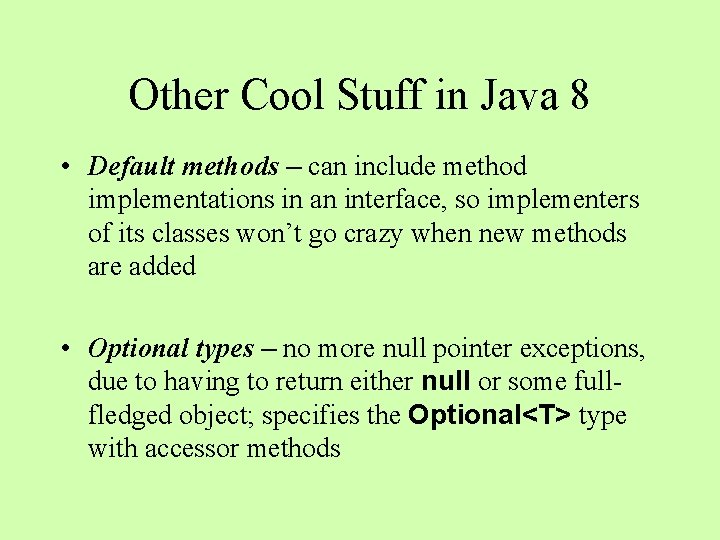
Other Cool Stuff in Java 8 • Default methods – can include method implementations in an interface, so implementers of its classes won’t go crazy when new methods are added • Optional types – no more null pointer exceptions, due to having to return either null or some fullfledged object; specifies the Optional<T> type with accessor methods
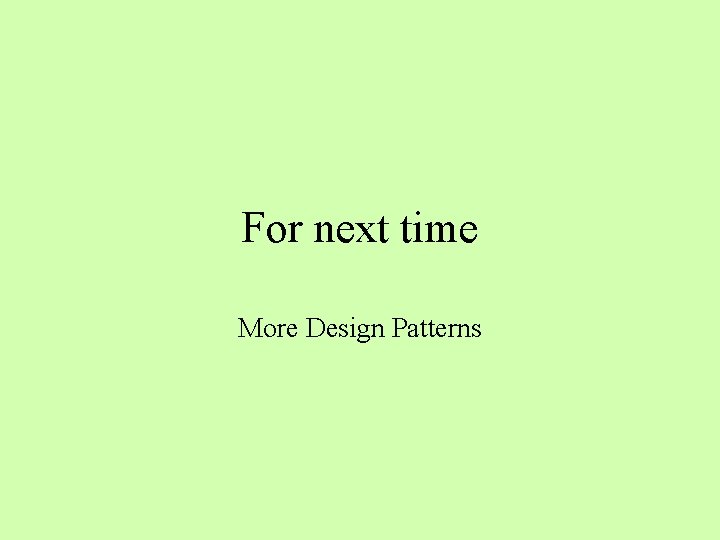
For next time More Design Patterns
Java 8 312
Hashim: what's your favorite subject
Cse 312
123+132+321+312
El 312 usps
Ics 312
Cse 312
Natural hazards definition ap human geography
Po box 30512 salt lake city
Uw cse 312
Bisc 312 usc
Altivar 312 solar
2-312
Ssis 312
21 cfr part 312
312 bus
1053 enteros 246 milésimos
What is the difference between 29 028 and 1 312
Katherine is very interested in cryogenics
Flow control instructions
Ics 312
Ics 312
Simplify. 312+18
Lebar balok
Mcs 312
Mcs 312
Ee 312
Mcs 312