Computer Graphics Spring 2003 COMS 4160 Lecture 13
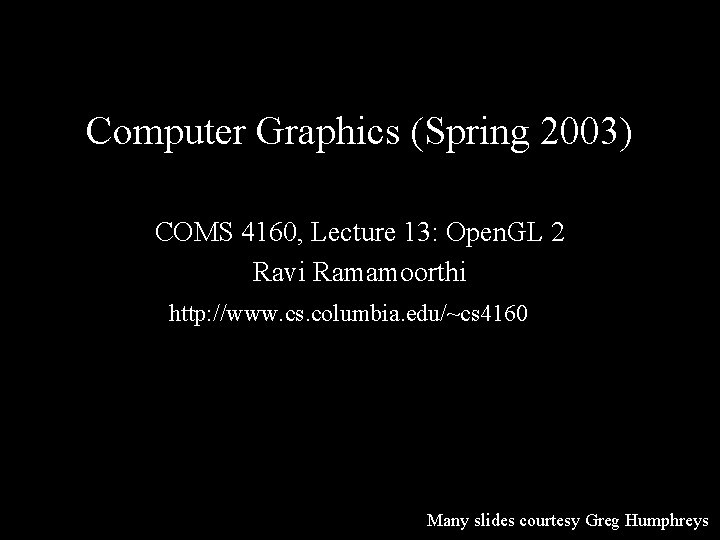
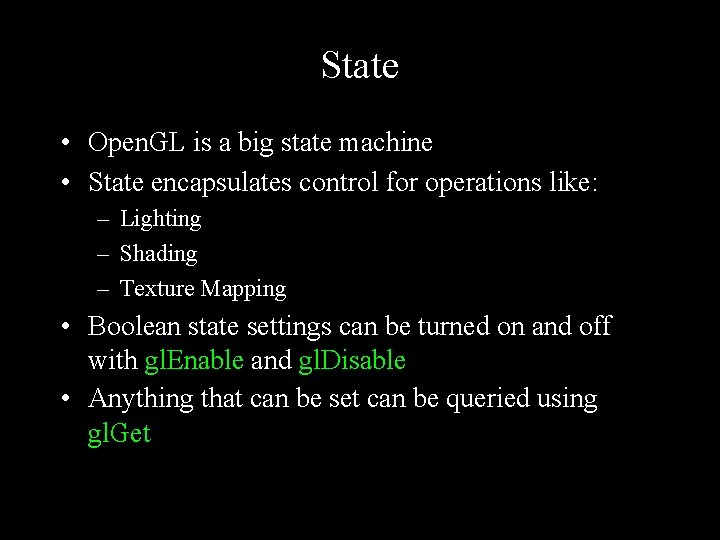
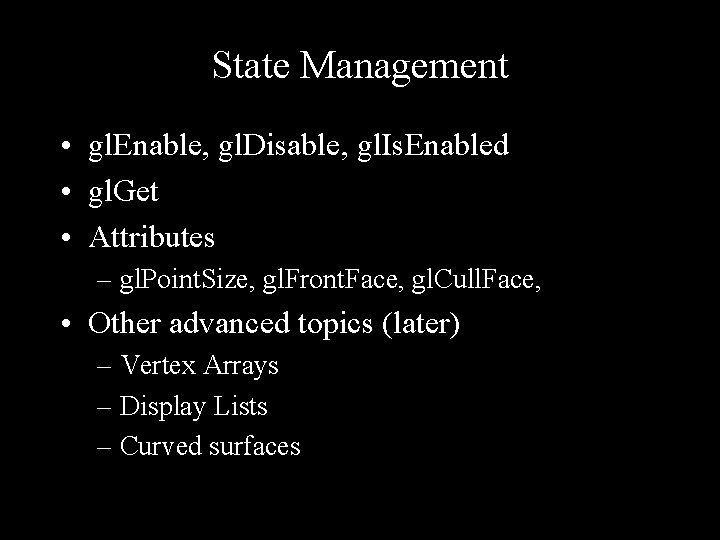
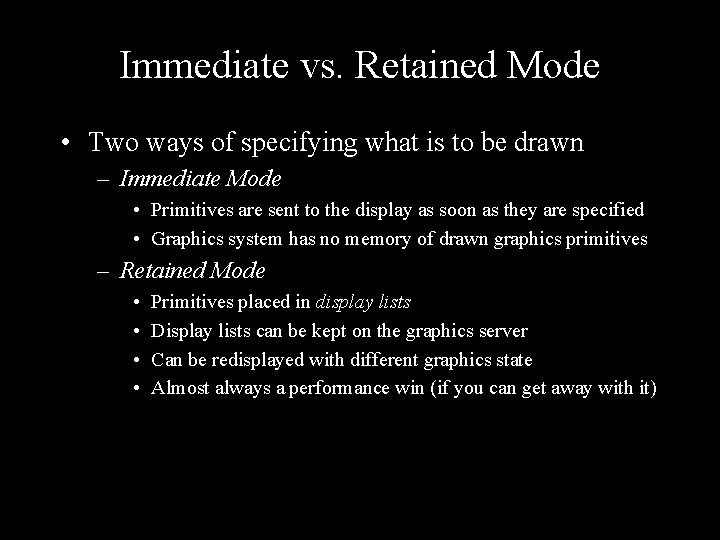
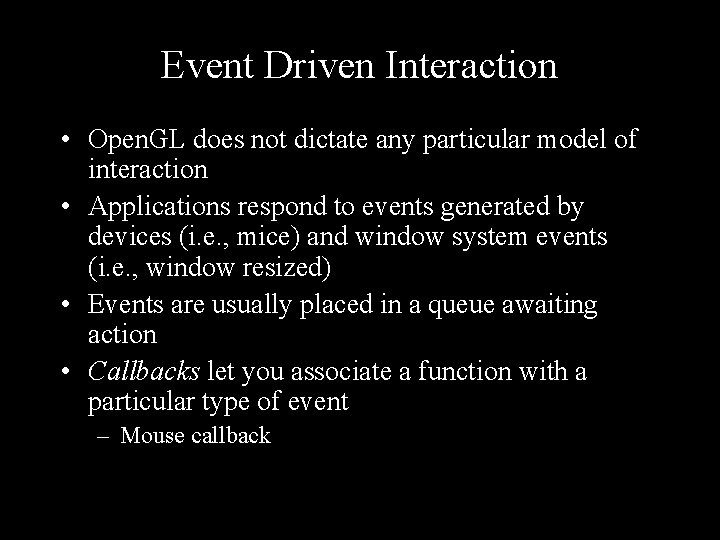
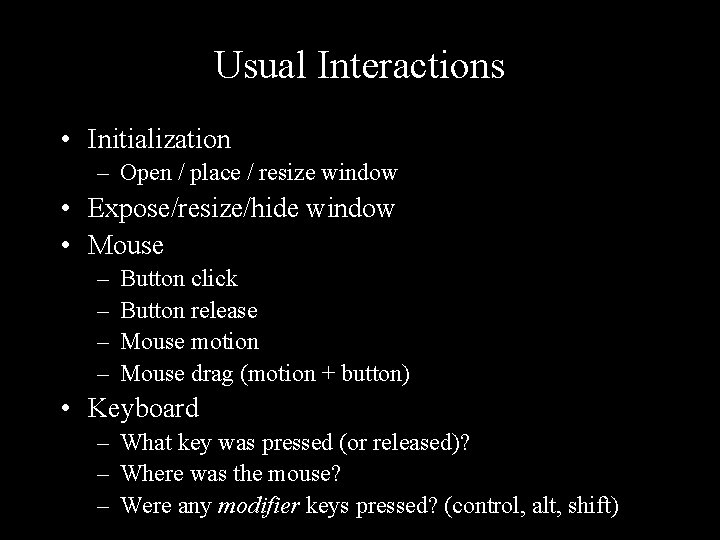
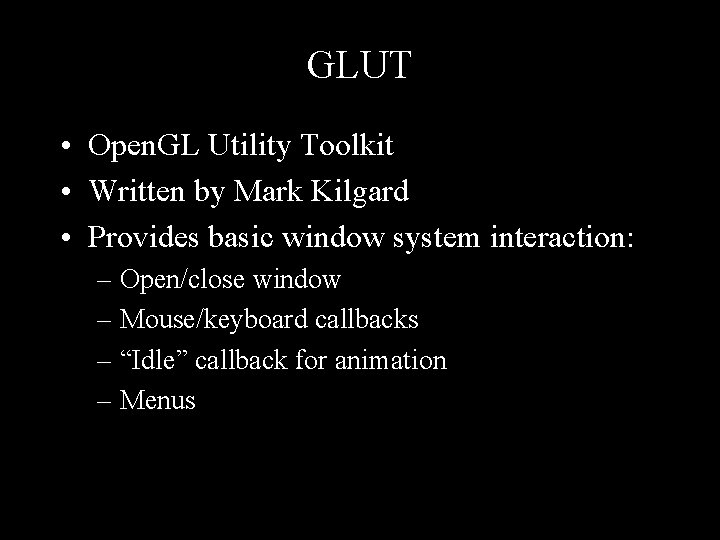
![Typical “main” Function int main( int argc, char *argv[] ) { glut. Init( &argc, Typical “main” Function int main( int argc, char *argv[] ) { glut. Init( &argc,](https://slidetodoc.com/presentation_image/aa610e65313d89f85fe2562d8e4507c3/image-8.jpg)
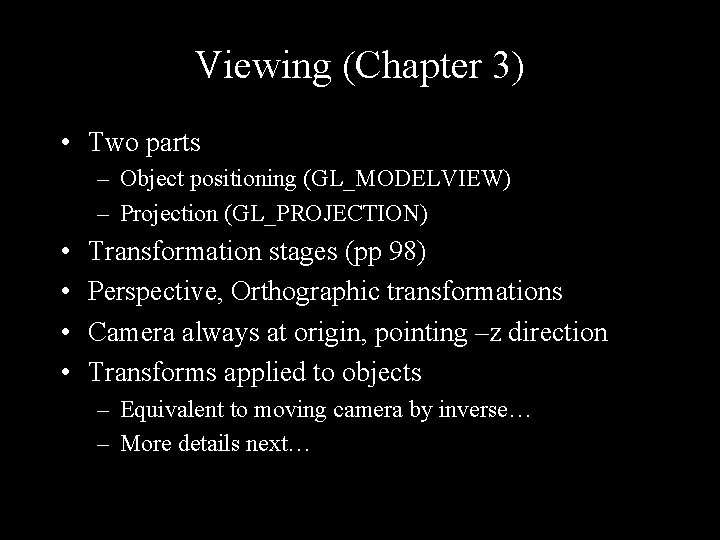
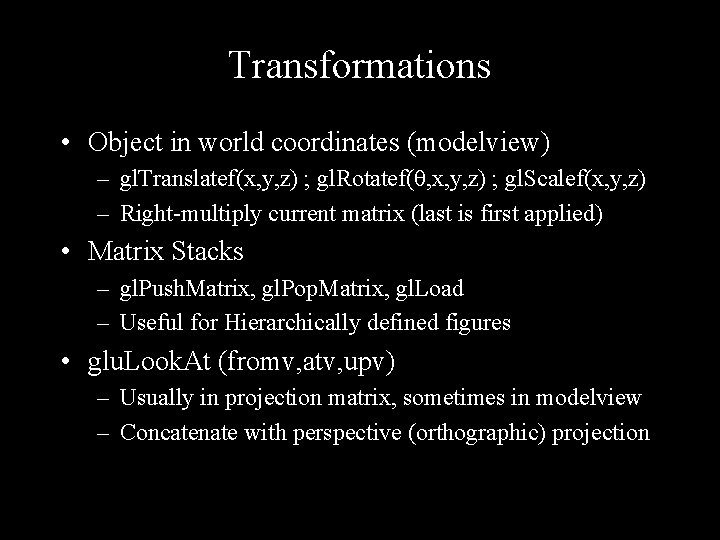
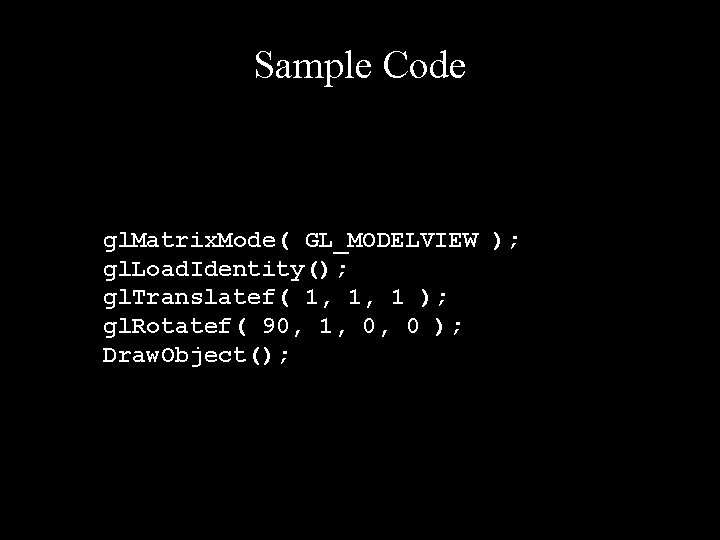
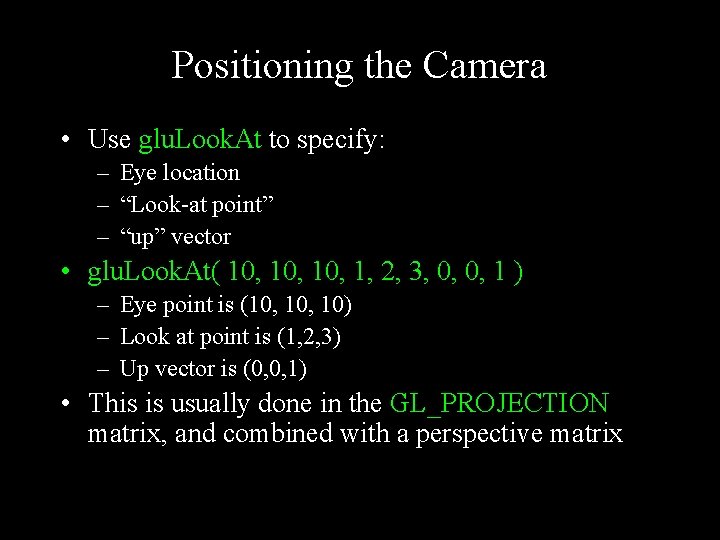
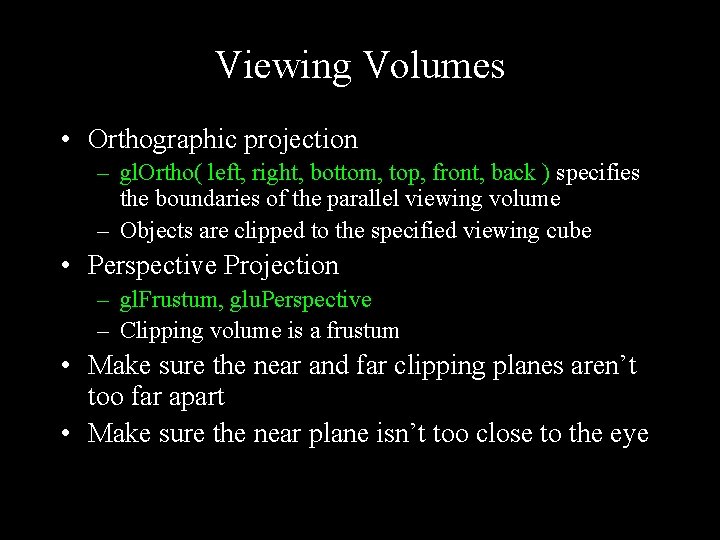
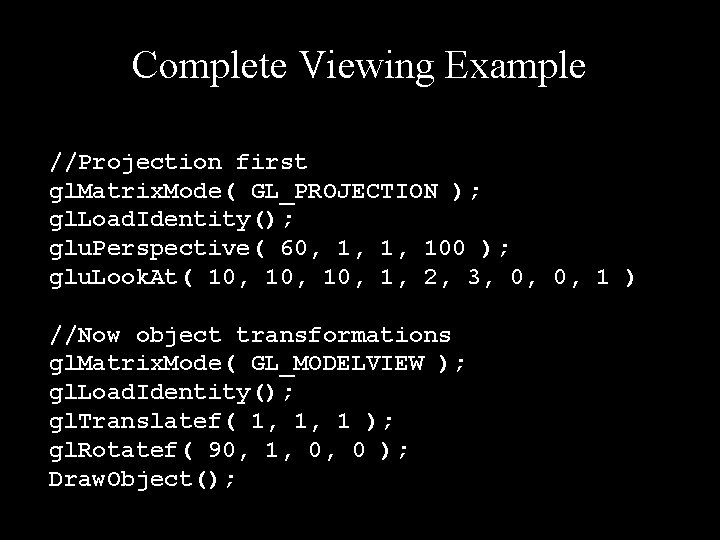
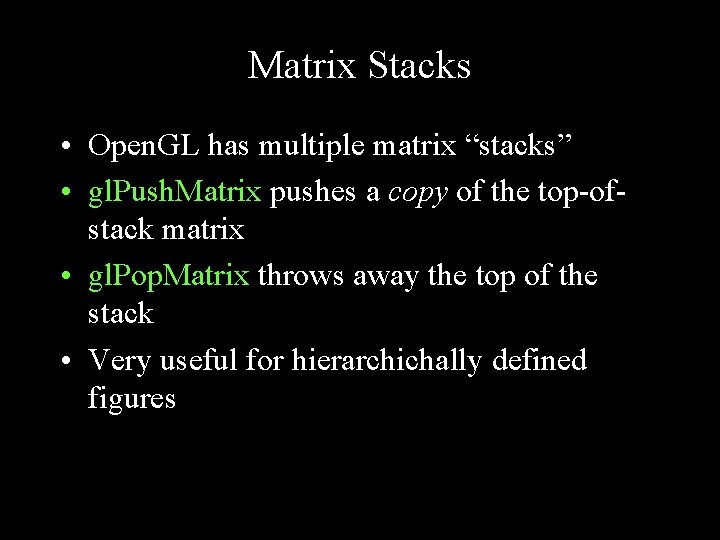
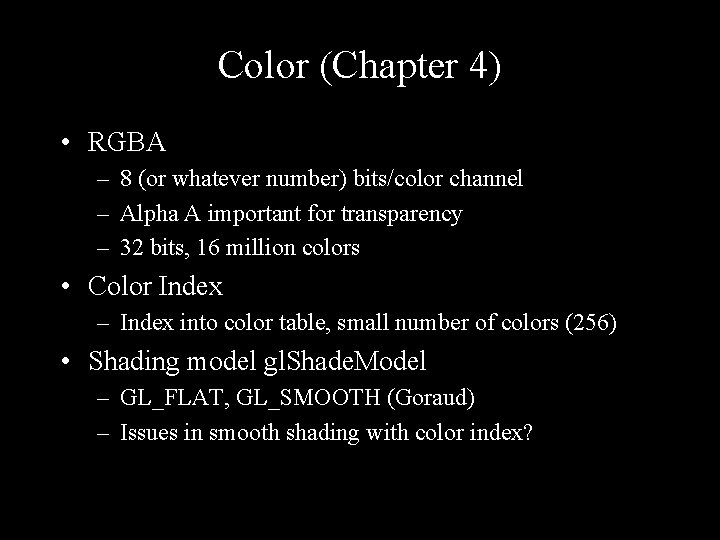
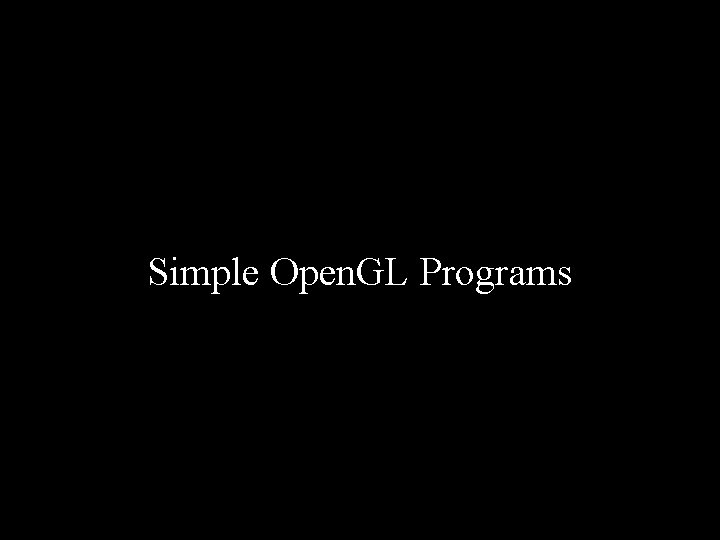
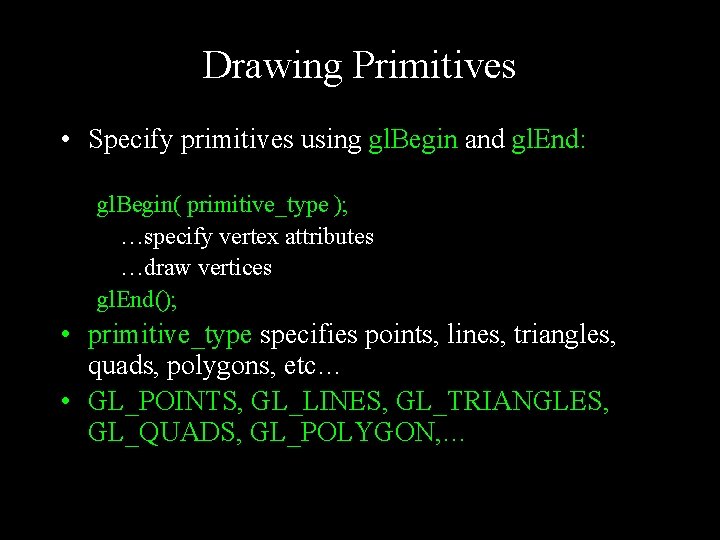
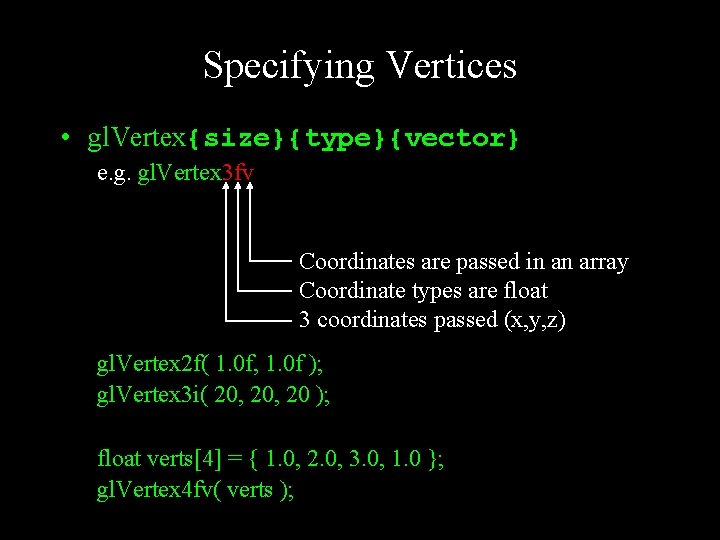
![Example: A Wireframe Cube GLfloat vertices[][3] = { {-1, -1}, {1, -1, 1}, {1, Example: A Wireframe Cube GLfloat vertices[][3] = { {-1, -1}, {1, -1, 1}, {1,](https://slidetodoc.com/presentation_image/aa610e65313d89f85fe2562d8e4507c3/image-20.jpg)
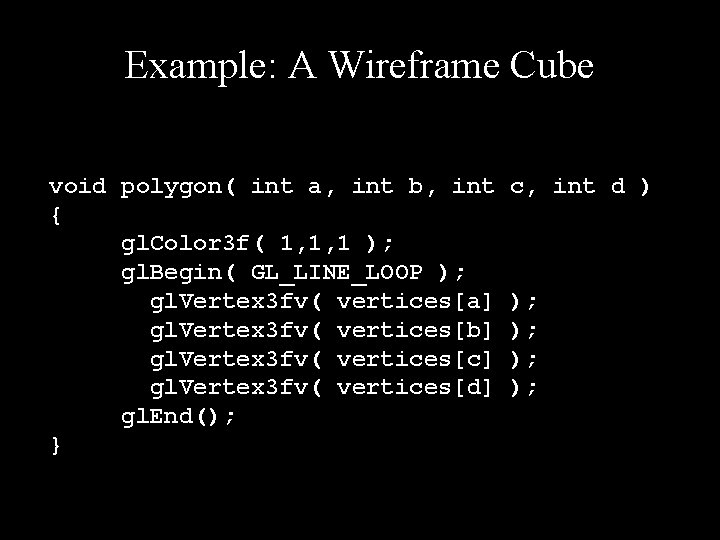
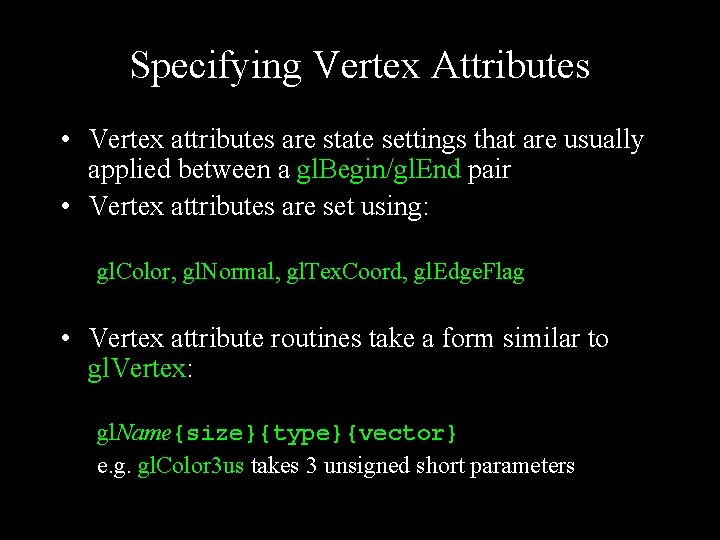
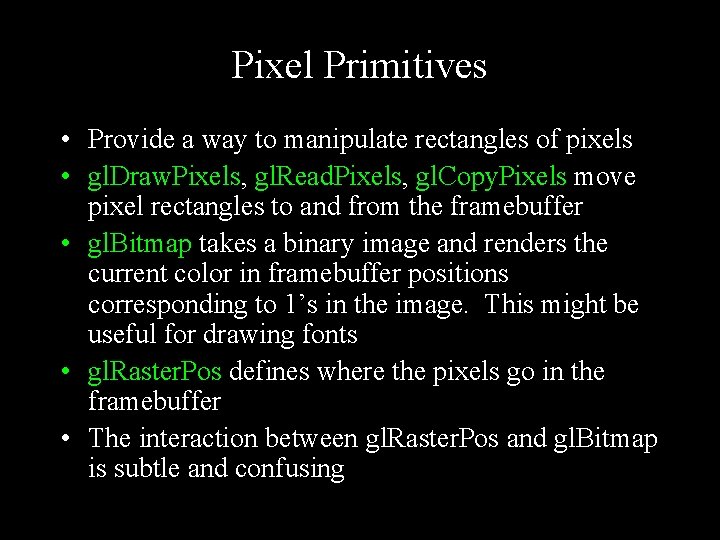
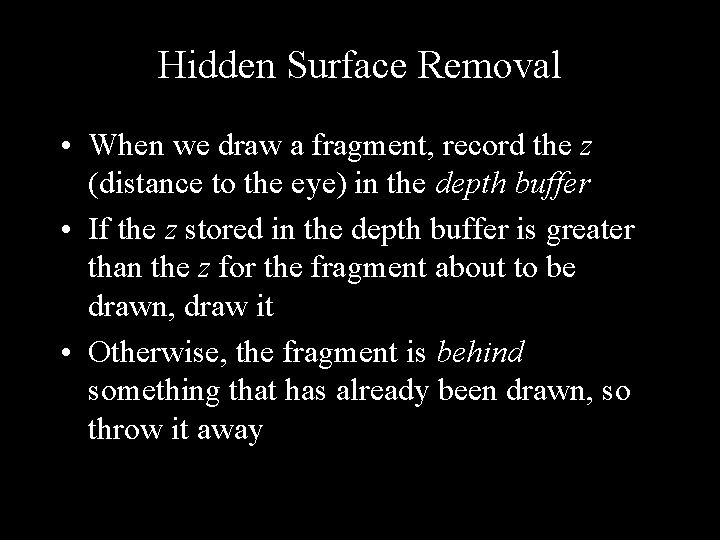
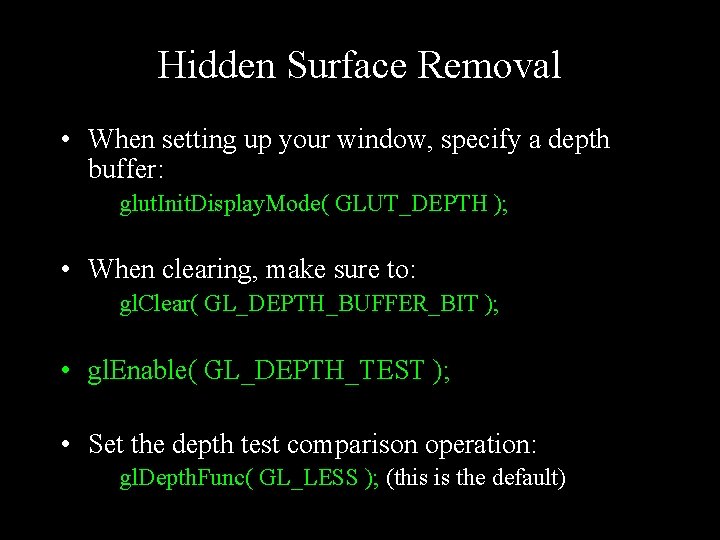
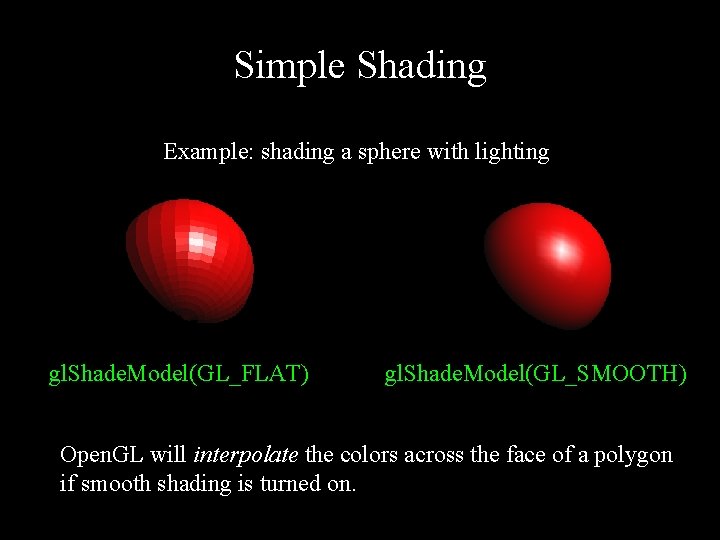
- Slides: 26
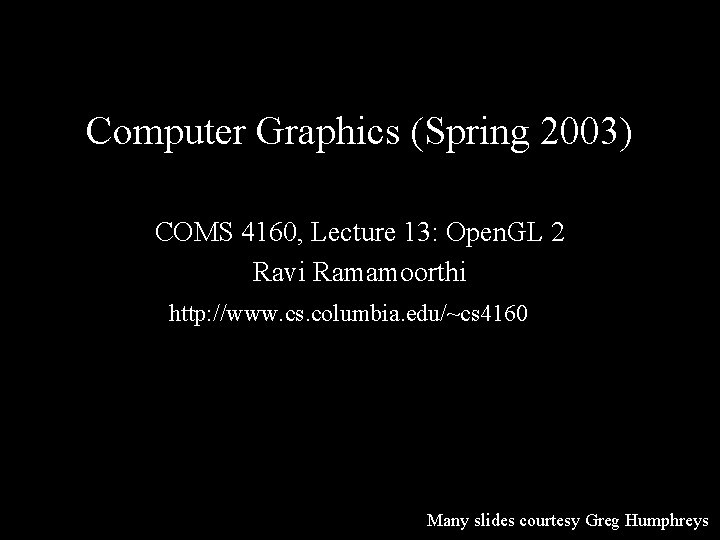
Computer Graphics (Spring 2003) COMS 4160, Lecture 13: Open. GL 2 Ravi Ramamoorthi http: //www. cs. columbia. edu/~cs 4160 Many slides courtesy Greg Humphreys
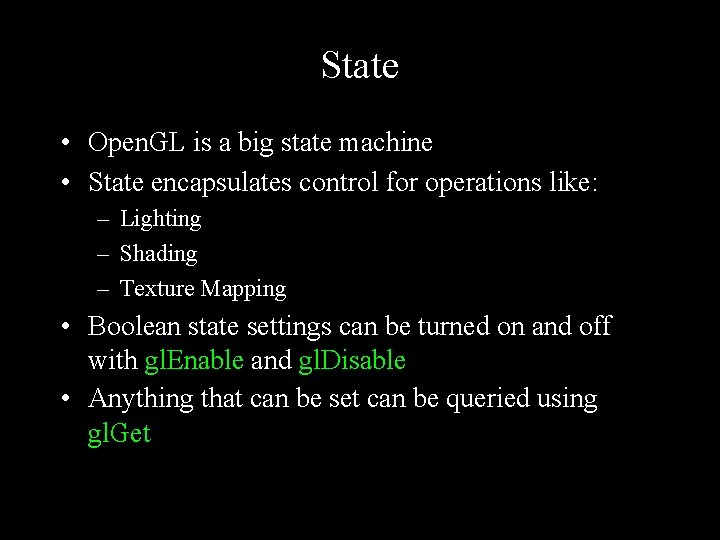
State • Open. GL is a big state machine • State encapsulates control for operations like: – Lighting – Shading – Texture Mapping • Boolean state settings can be turned on and off with gl. Enable and gl. Disable • Anything that can be set can be queried using gl. Get
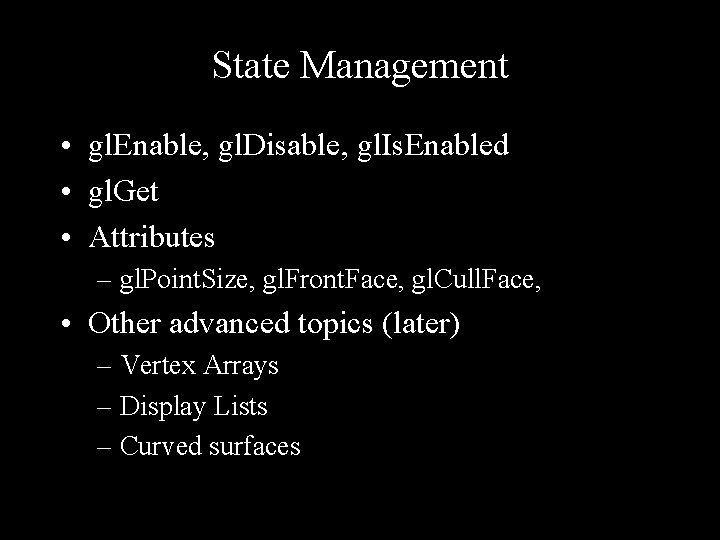
State Management • gl. Enable, gl. Disable, gl. Is. Enabled • gl. Get • Attributes – gl. Point. Size, gl. Front. Face, gl. Cull. Face, • Other advanced topics (later) – Vertex Arrays – Display Lists – Curved surfaces
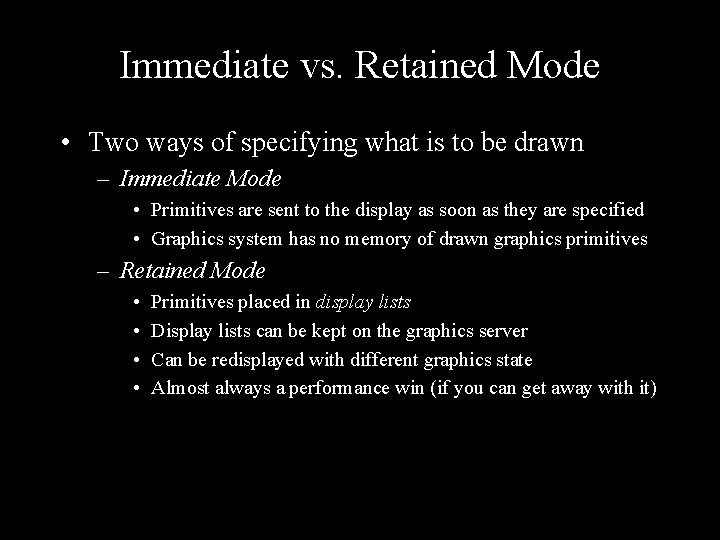
Immediate vs. Retained Mode • Two ways of specifying what is to be drawn – Immediate Mode • Primitives are sent to the display as soon as they are specified • Graphics system has no memory of drawn graphics primitives – Retained Mode • • Primitives placed in display lists Display lists can be kept on the graphics server Can be redisplayed with different graphics state Almost always a performance win (if you can get away with it)
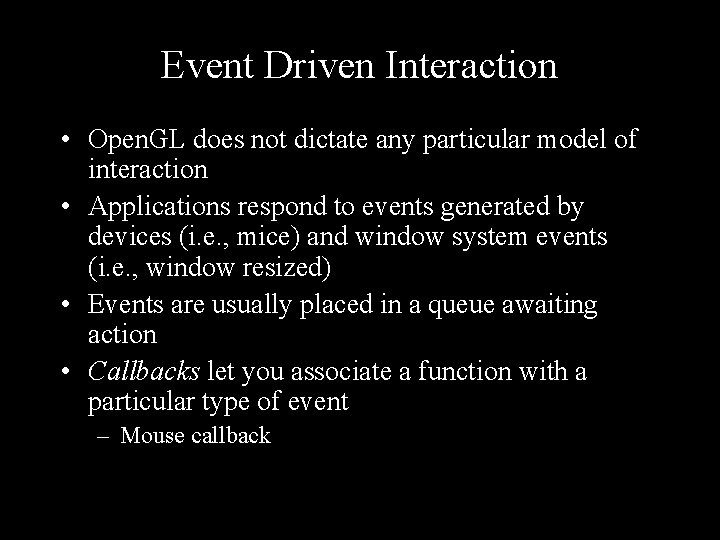
Event Driven Interaction • Open. GL does not dictate any particular model of interaction • Applications respond to events generated by devices (i. e. , mice) and window system events (i. e. , window resized) • Events are usually placed in a queue awaiting action • Callbacks let you associate a function with a particular type of event – Mouse callback
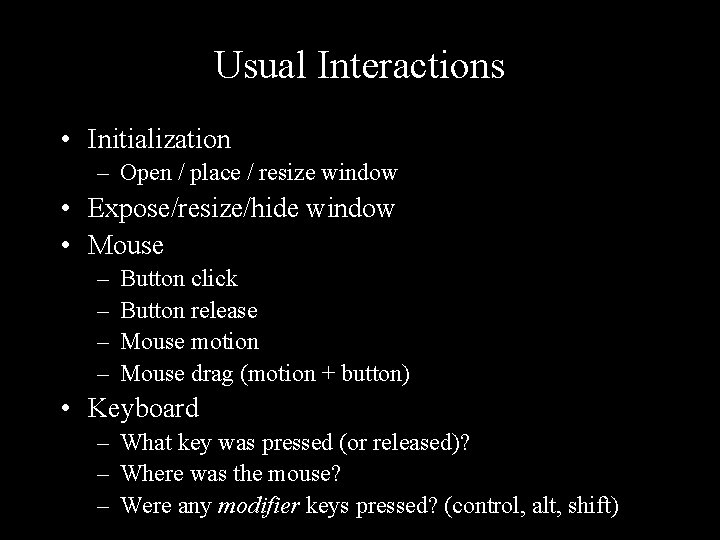
Usual Interactions • Initialization – Open / place / resize window • Expose/resize/hide window • Mouse – – Button click Button release Mouse motion Mouse drag (motion + button) • Keyboard – What key was pressed (or released)? – Where was the mouse? – Were any modifier keys pressed? (control, alt, shift)
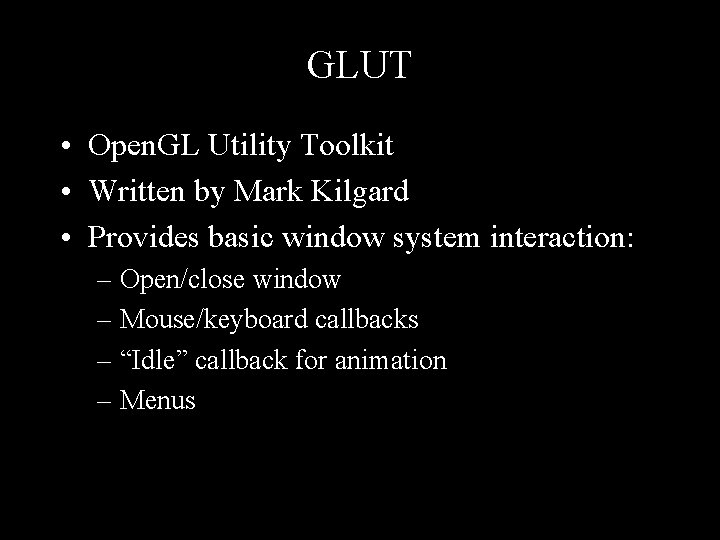
GLUT • Open. GL Utility Toolkit • Written by Mark Kilgard • Provides basic window system interaction: – Open/close window – Mouse/keyboard callbacks – “Idle” callback for animation – Menus
![Typical main Function int main int argc char argv glut Init argc Typical “main” Function int main( int argc, char *argv[] ) { glut. Init( &argc,](https://slidetodoc.com/presentation_image/aa610e65313d89f85fe2562d8e4507c3/image-8.jpg)
Typical “main” Function int main( int argc, char *argv[] ) { glut. Init( &argc, argv ); glut. Init. Display. Mode( GLUT_SINGLE | GLUT_RGB ); glut. Create. Window( “A window” ); glut. Display. Func( display ); glut. Reshape. Func( reshape ); glut. Mouse. Func( mouse ); glut. Idle. Func( idle ); glut. Main. Loop(); }
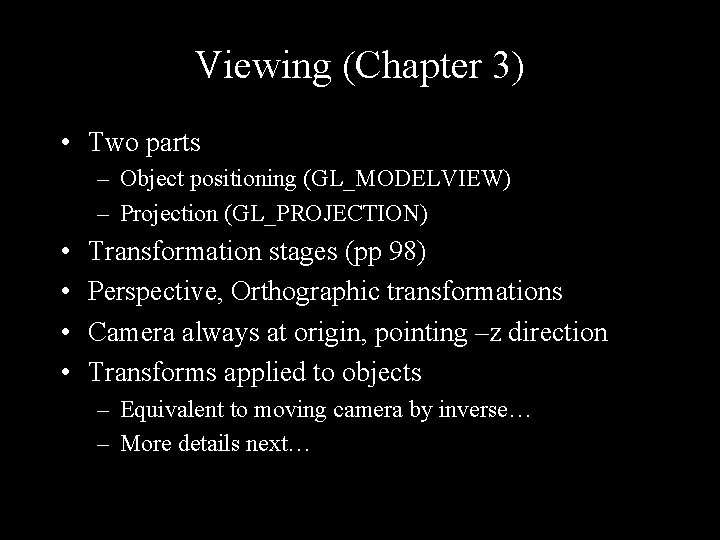
Viewing (Chapter 3) • Two parts – Object positioning (GL_MODELVIEW) – Projection (GL_PROJECTION) • • Transformation stages (pp 98) Perspective, Orthographic transformations Camera always at origin, pointing –z direction Transforms applied to objects – Equivalent to moving camera by inverse… – More details next…
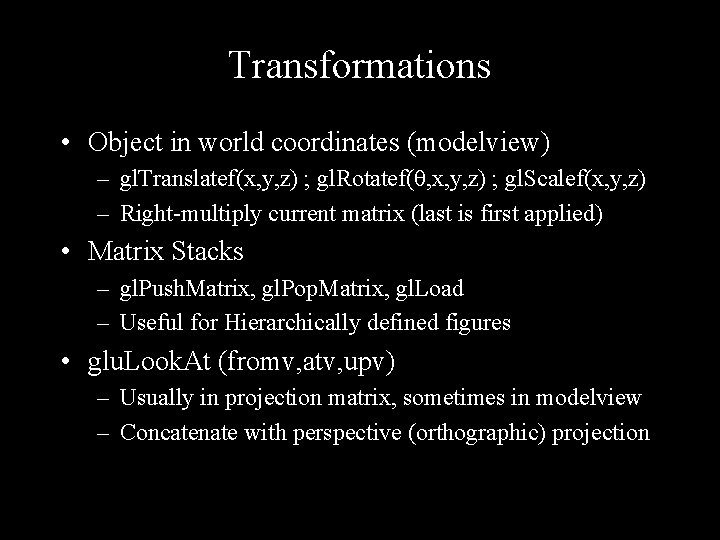
Transformations • Object in world coordinates (modelview) – gl. Translatef(x, y, z) ; gl. Rotatef(θ, x, y, z) ; gl. Scalef(x, y, z) – Right-multiply current matrix (last is first applied) • Matrix Stacks – gl. Push. Matrix, gl. Pop. Matrix, gl. Load – Useful for Hierarchically defined figures • glu. Look. At (fromv, atv, upv) – Usually in projection matrix, sometimes in modelview – Concatenate with perspective (orthographic) projection
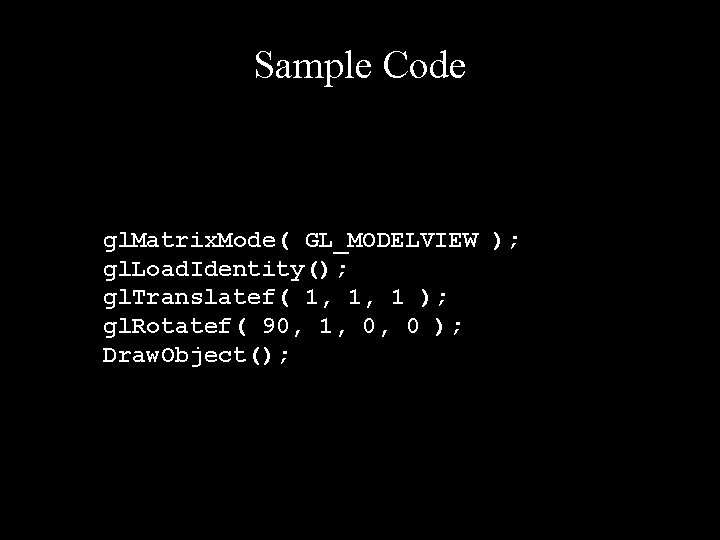
Sample Code gl. Matrix. Mode( GL_MODELVIEW ); gl. Load. Identity(); gl. Translatef( 1, 1, 1 ); gl. Rotatef( 90, 1, 0, 0 ); Draw. Object();
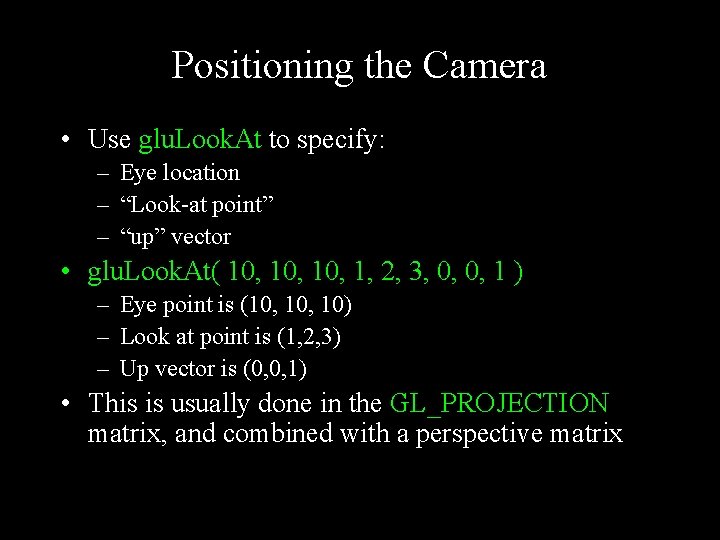
Positioning the Camera • Use glu. Look. At to specify: – Eye location – “Look-at point” – “up” vector • glu. Look. At( 10, 10, 1, 2, 3, 0, 0, 1 ) – Eye point is (10, 10) – Look at point is (1, 2, 3) – Up vector is (0, 0, 1) • This is usually done in the GL_PROJECTION matrix, and combined with a perspective matrix
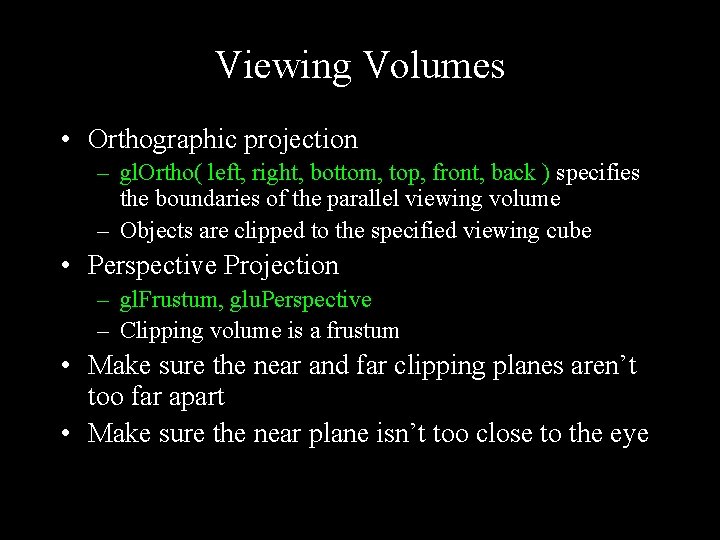
Viewing Volumes • Orthographic projection – gl. Ortho( left, right, bottom, top, front, back ) specifies the boundaries of the parallel viewing volume – Objects are clipped to the specified viewing cube • Perspective Projection – gl. Frustum, glu. Perspective – Clipping volume is a frustum • Make sure the near and far clipping planes aren’t too far apart • Make sure the near plane isn’t too close to the eye
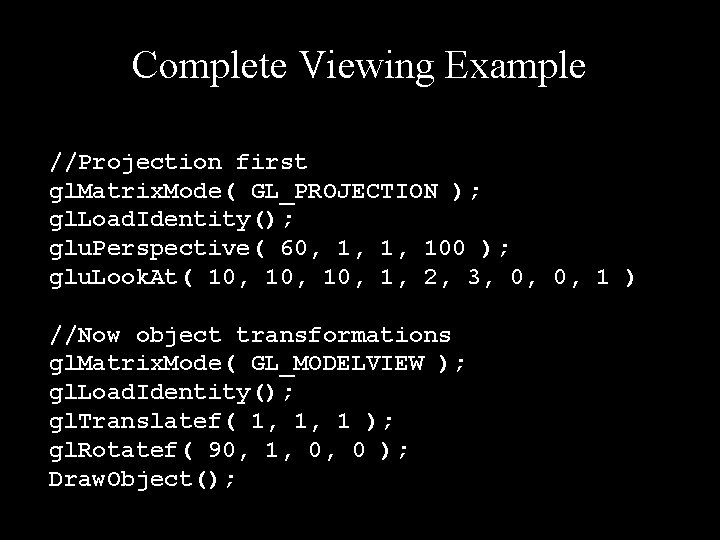
Complete Viewing Example //Projection first gl. Matrix. Mode( GL_PROJECTION ); gl. Load. Identity(); glu. Perspective( 60, 1, 1, 100 ); glu. Look. At( 10, 10, 1, 2, 3, 0, 0, 1 ) //Now object transformations gl. Matrix. Mode( GL_MODELVIEW ); gl. Load. Identity(); gl. Translatef( 1, 1, 1 ); gl. Rotatef( 90, 1, 0, 0 ); Draw. Object();
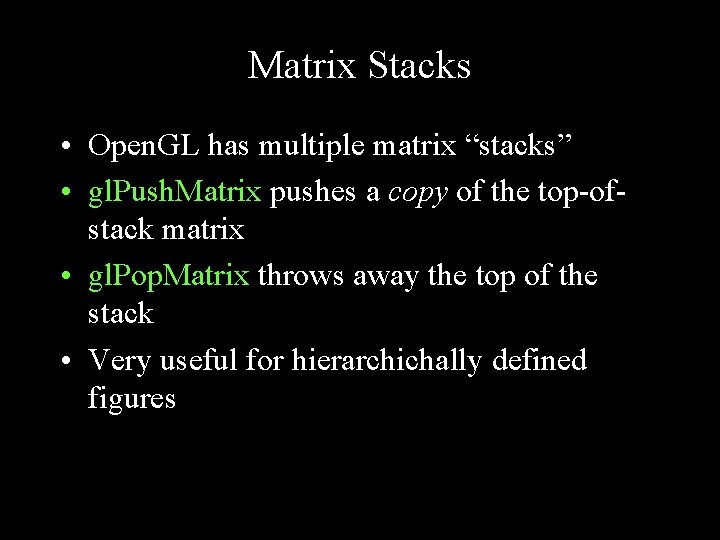
Matrix Stacks • Open. GL has multiple matrix “stacks” • gl. Push. Matrix pushes a copy of the top-ofstack matrix • gl. Pop. Matrix throws away the top of the stack • Very useful for hierarchichally defined figures
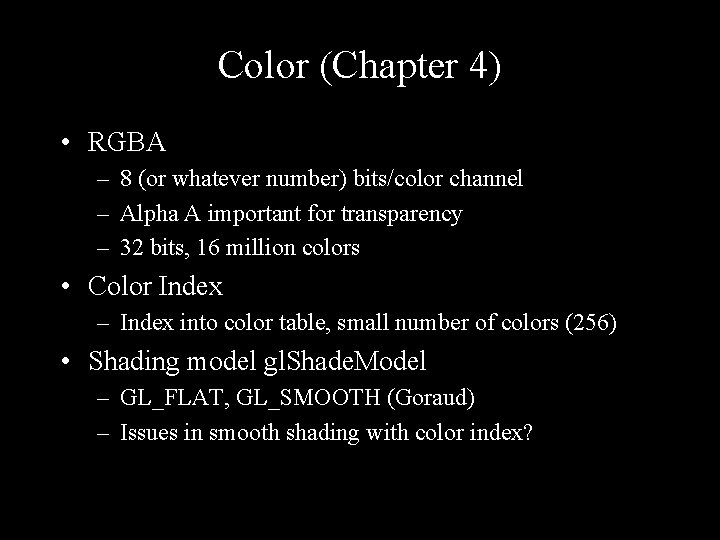
Color (Chapter 4) • RGBA – 8 (or whatever number) bits/color channel – Alpha A important for transparency – 32 bits, 16 million colors • Color Index – Index into color table, small number of colors (256) • Shading model gl. Shade. Model – GL_FLAT, GL_SMOOTH (Goraud) – Issues in smooth shading with color index?
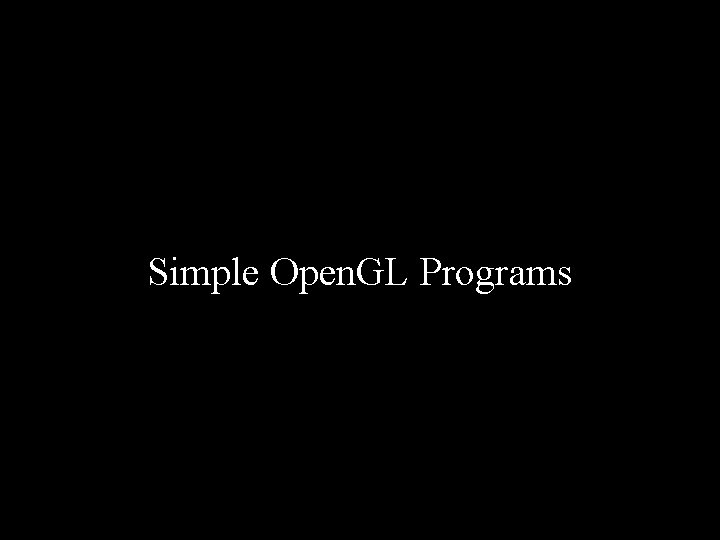
Simple Open. GL Programs
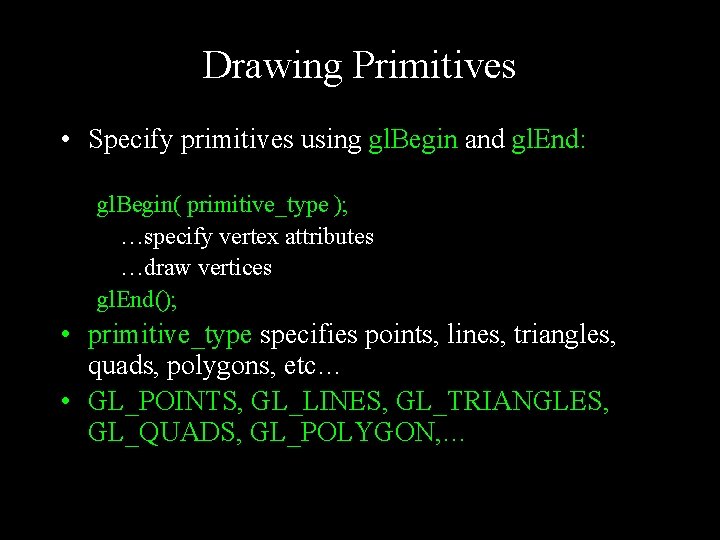
Drawing Primitives • Specify primitives using gl. Begin and gl. End: gl. Begin( primitive_type ); …specify vertex attributes …draw vertices gl. End(); • primitive_type specifies points, lines, triangles, quads, polygons, etc… • GL_POINTS, GL_LINES, GL_TRIANGLES, GL_QUADS, GL_POLYGON, …
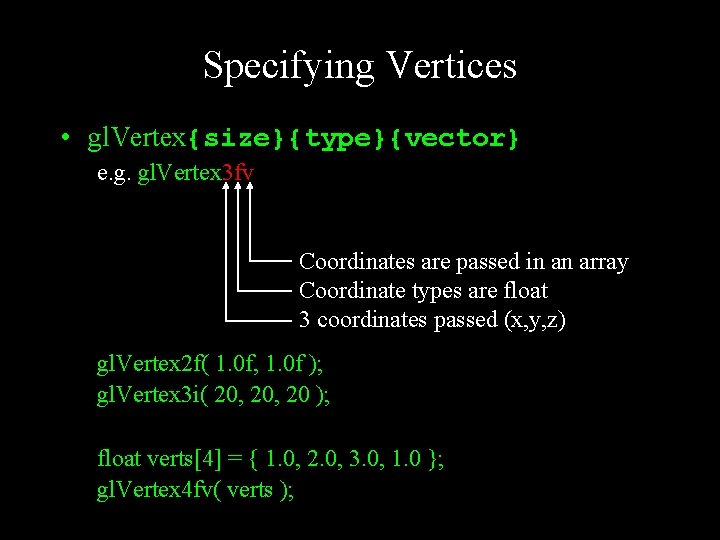
Specifying Vertices • gl. Vertex{size}{type}{vector} e. g. gl. Vertex 3 fv Coordinates are passed in an array Coordinate types are float 3 coordinates passed (x, y, z) gl. Vertex 2 f( 1. 0 f, 1. 0 f ); gl. Vertex 3 i( 20, 20 ); float verts[4] = { 1. 0, 2. 0, 3. 0, 1. 0 }; gl. Vertex 4 fv( verts );
![Example A Wireframe Cube GLfloat vertices3 1 1 1 1 1 1 Example: A Wireframe Cube GLfloat vertices[][3] = { {-1, -1}, {1, -1, 1}, {1,](https://slidetodoc.com/presentation_image/aa610e65313d89f85fe2562d8e4507c3/image-20.jpg)
Example: A Wireframe Cube GLfloat vertices[][3] = { {-1, -1}, {1, -1, 1}, {1, 1, -1}, {-1, -1, 1}, {1, 1, 1}, {-1, 1, 1} }; void cube(void) { polygon( 1, 0, 3, 2 ); polygon( 3, 7, 6, 2 ); polygon( 7, 3, 0, 4 ); polygon( 2, 6, 5, 1 ); polygon( 4, 5, 6, 7 ); polygon( 5, 4, 0, 1 ); }
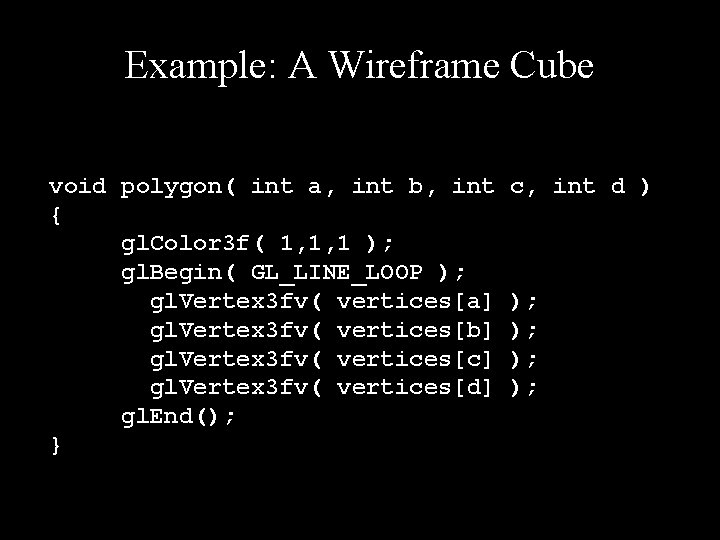
Example: A Wireframe Cube void polygon( int a, int b, int { gl. Color 3 f( 1, 1, 1 ); gl. Begin( GL_LINE_LOOP ); gl. Vertex 3 fv( vertices[a] gl. Vertex 3 fv( vertices[b] gl. Vertex 3 fv( vertices[c] gl. Vertex 3 fv( vertices[d] gl. End(); } c, int d ) ); );
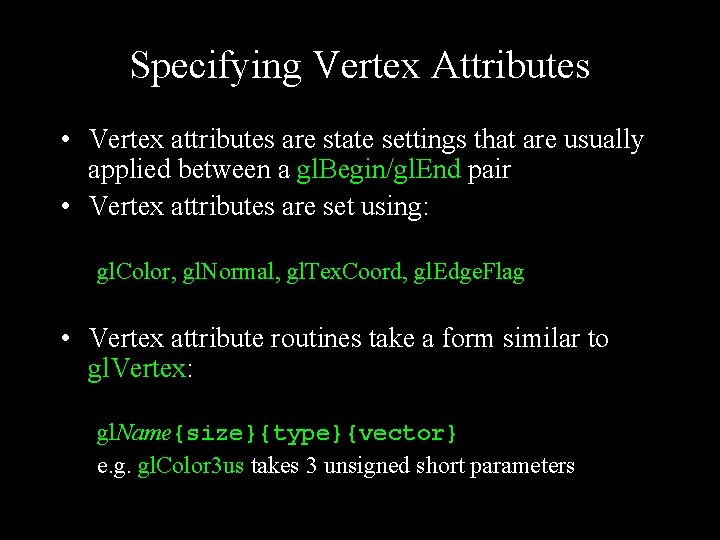
Specifying Vertex Attributes • Vertex attributes are state settings that are usually applied between a gl. Begin/gl. End pair • Vertex attributes are set using: gl. Color, gl. Normal, gl. Tex. Coord, gl. Edge. Flag • Vertex attribute routines take a form similar to gl. Vertex: gl. Name{size}{type}{vector} e. g. gl. Color 3 us takes 3 unsigned short parameters
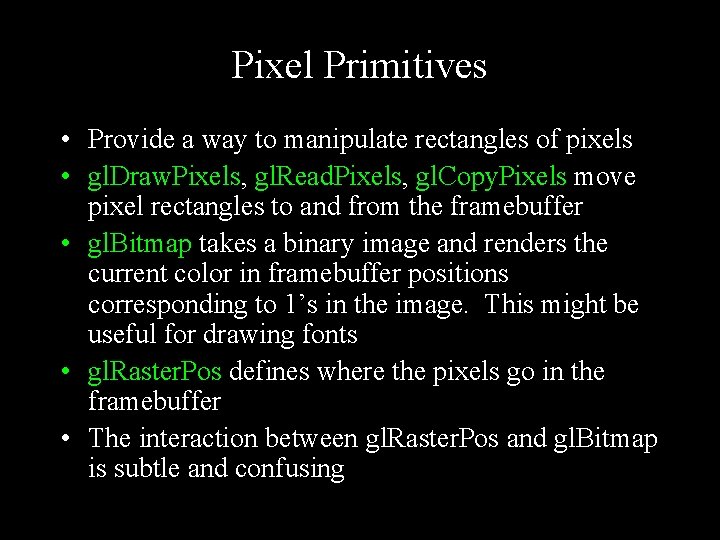
Pixel Primitives • Provide a way to manipulate rectangles of pixels • gl. Draw. Pixels, gl. Read. Pixels, gl. Copy. Pixels move pixel rectangles to and from the framebuffer • gl. Bitmap takes a binary image and renders the current color in framebuffer positions corresponding to 1’s in the image. This might be useful for drawing fonts • gl. Raster. Pos defines where the pixels go in the framebuffer • The interaction between gl. Raster. Pos and gl. Bitmap is subtle and confusing
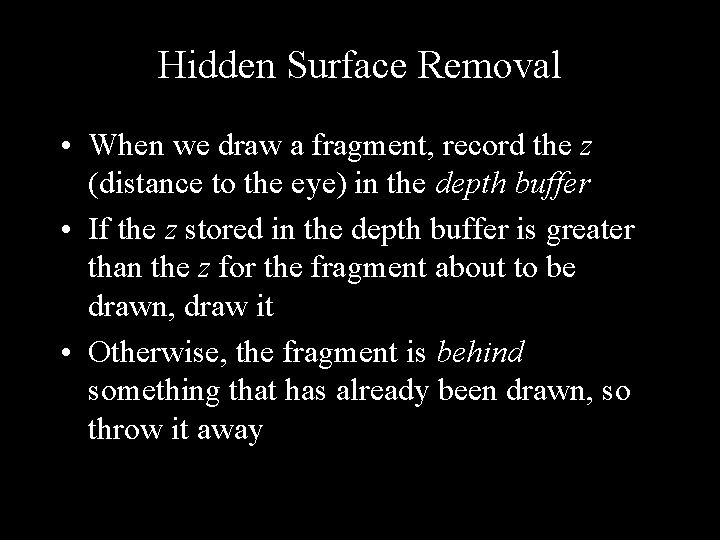
Hidden Surface Removal • When we draw a fragment, record the z (distance to the eye) in the depth buffer • If the z stored in the depth buffer is greater than the z for the fragment about to be drawn, draw it • Otherwise, the fragment is behind something that has already been drawn, so throw it away
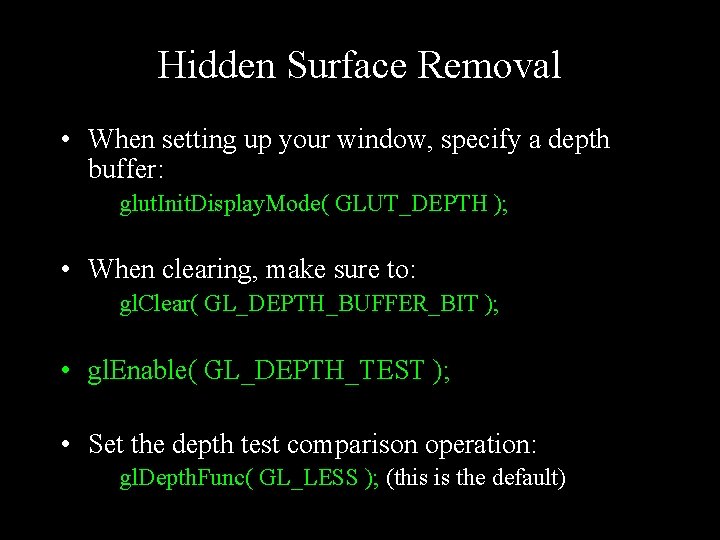
Hidden Surface Removal • When setting up your window, specify a depth buffer: glut. Init. Display. Mode( GLUT_DEPTH ); • When clearing, make sure to: gl. Clear( GL_DEPTH_BUFFER_BIT ); • gl. Enable( GL_DEPTH_TEST ); • Set the depth test comparison operation: gl. Depth. Func( GL_LESS ); (this is the default)
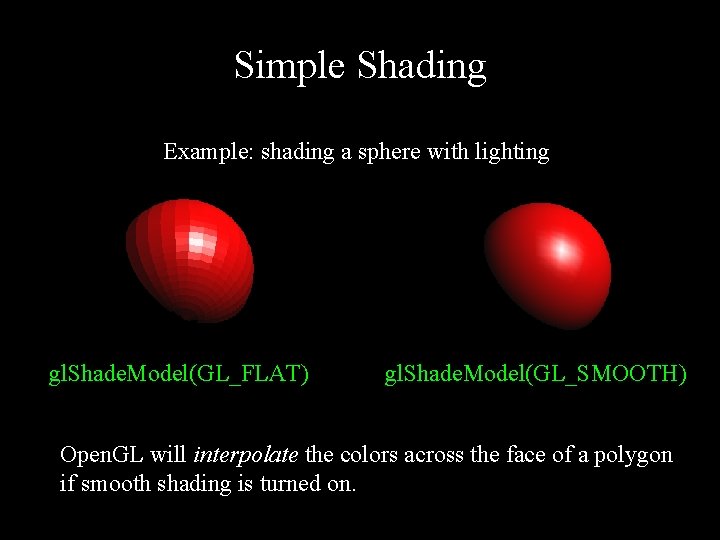
Simple Shading Example: shading a sphere with lighting gl. Shade. Model(GL_FLAT) gl. Shade. Model(GL_SMOOTH) Open. GL will interpolate the colors across the face of a polygon if smooth shading is turned on.
Spring summer fall winter and spring cast
Computer graphics
Computer graphics chapter 1 ppt
01:640:244 lecture notes - lecture 15: plat, idah, farad
Spring fall summer winter
Coms 6998
Sambit sahu columbia
Coms 4705
Halfcoms
Coms
Coms 6998
Coms 4118
Coms 4115
Coms 3261
Coms project
Coms 4118
Coms 6998
Coms 6998
Computer security 161 cryptocurrency lecture
Computer aided drug design lecture notes
Computer architecture lecture notes
Microarchitecture vs isa
Angel computer graphics
What is viewing in computer graphics
Video display devices in computer graphics
In two dimensional viewing we have?
Shear transformation in computer graphics