Computer Graphics Fall 2003 COMS 4160 Lecture 5
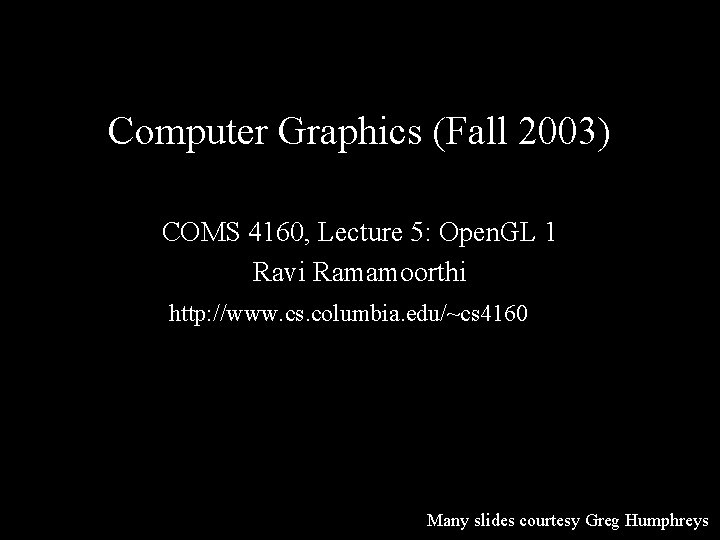
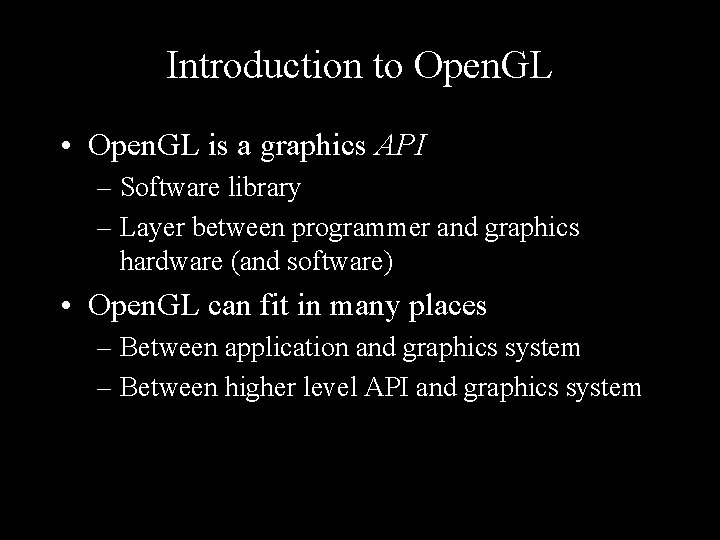
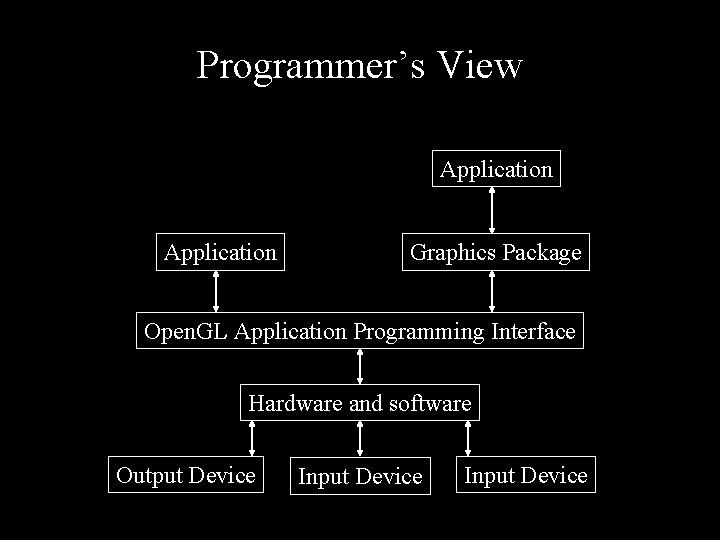
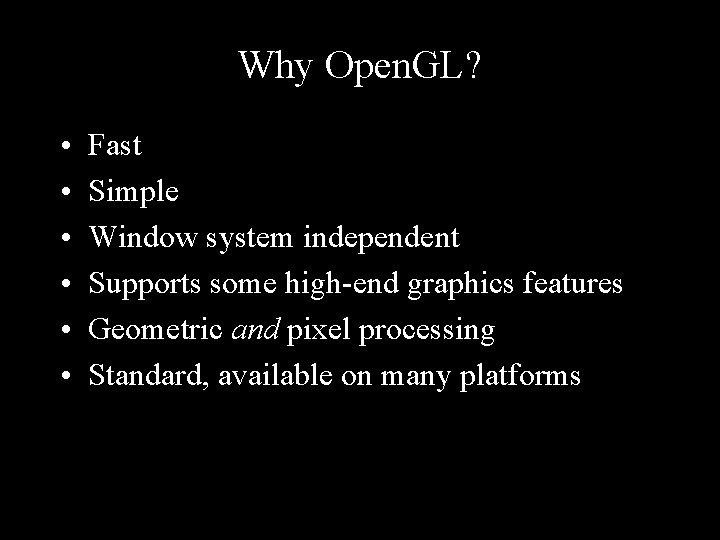
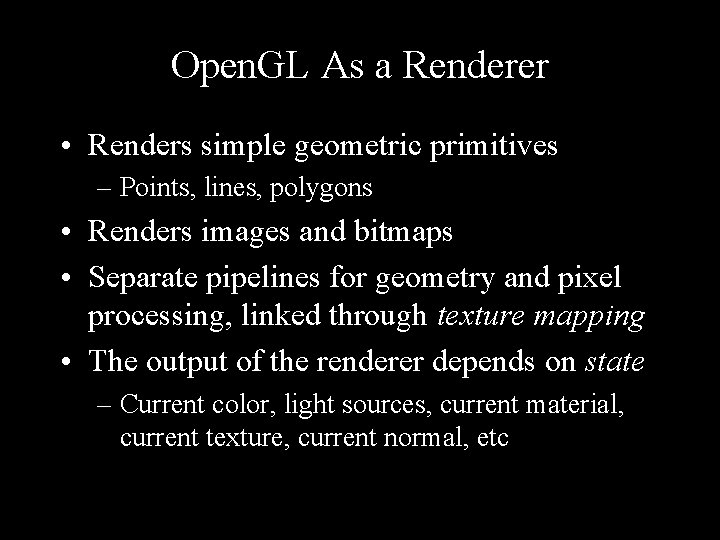
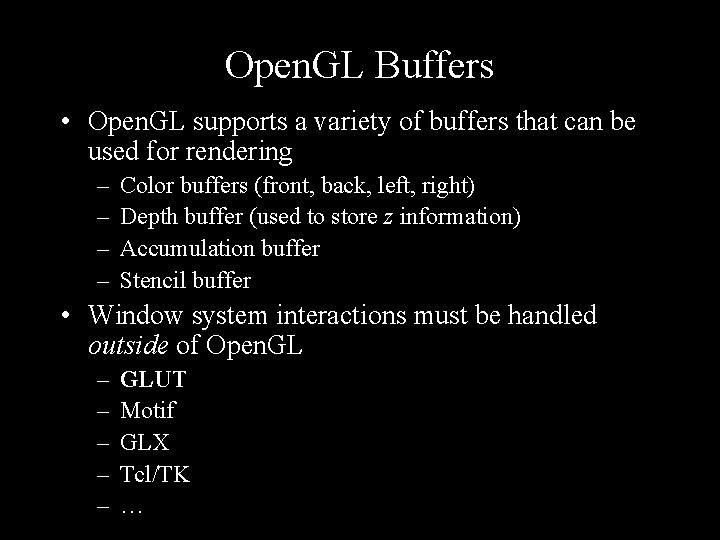
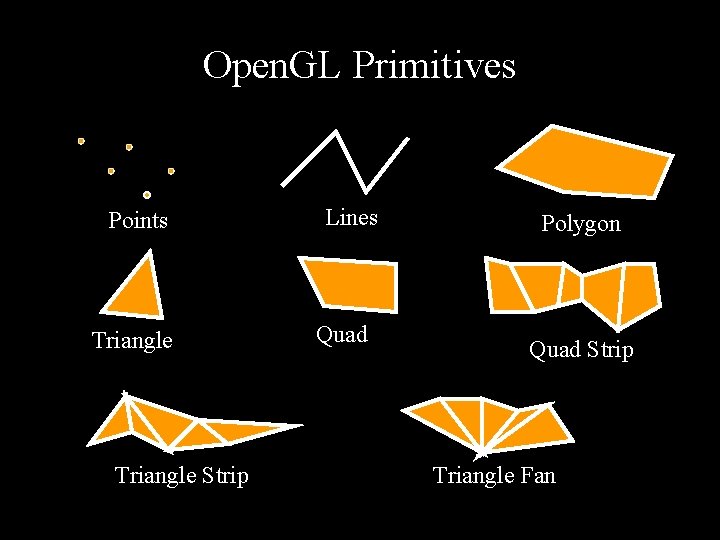
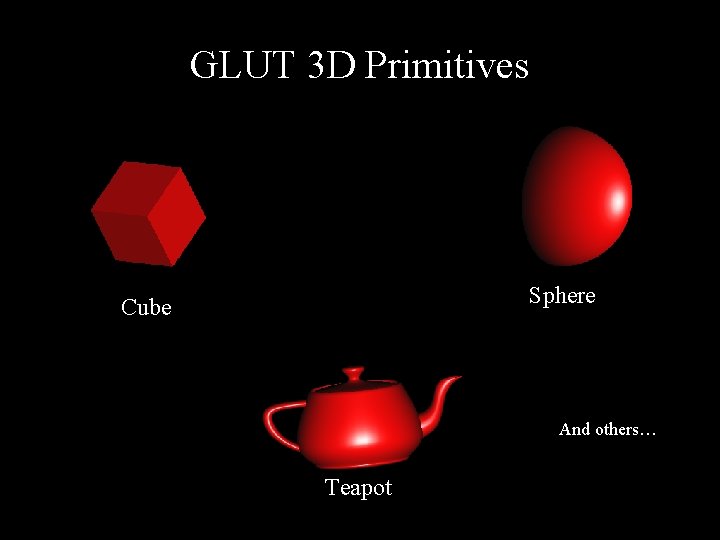
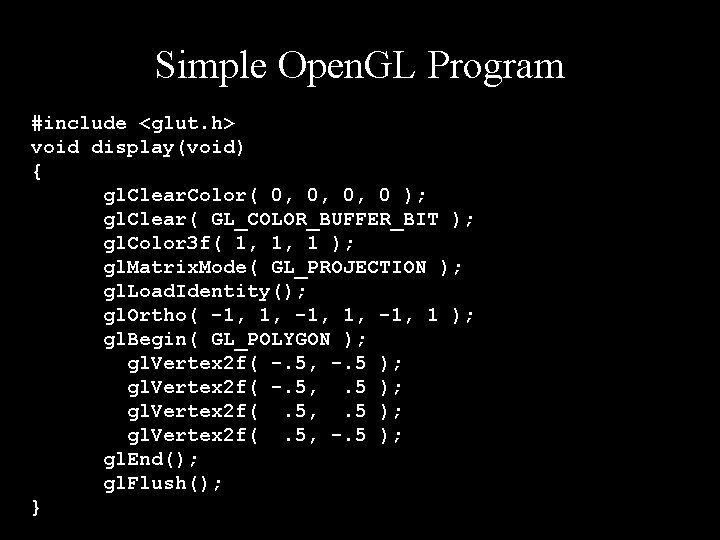
![Window System Glue int main( int argc, char *argv[] ) { glut. Init( &argc, Window System Glue int main( int argc, char *argv[] ) { glut. Init( &argc,](https://slidetodoc.com/presentation_image_h2/61765dcdaeb420c99c7db7927f64a1ee/image-10.jpg)
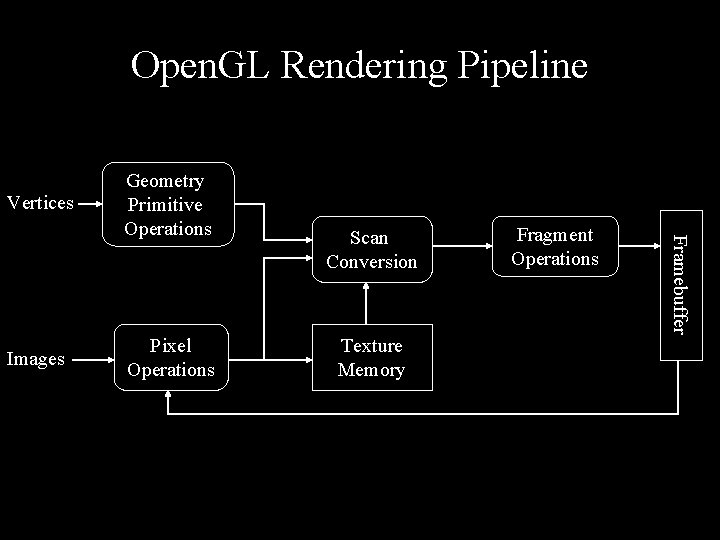
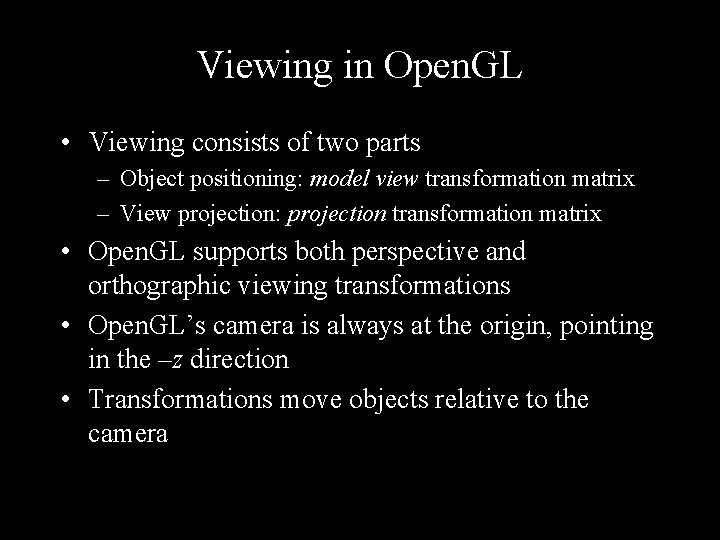
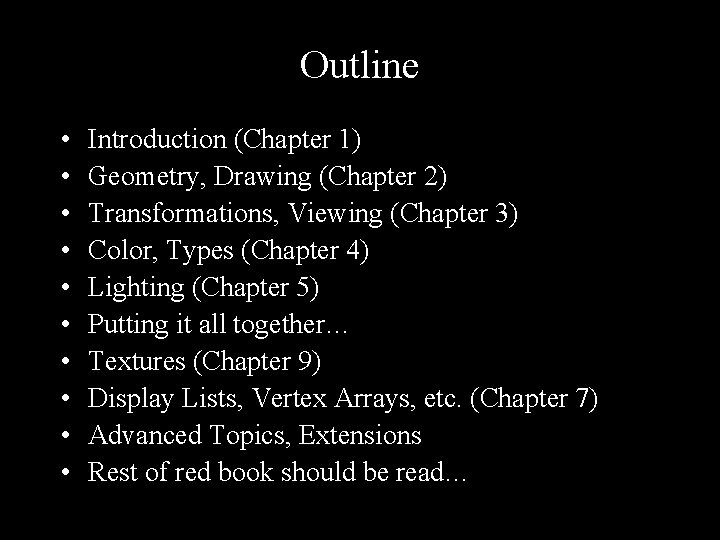
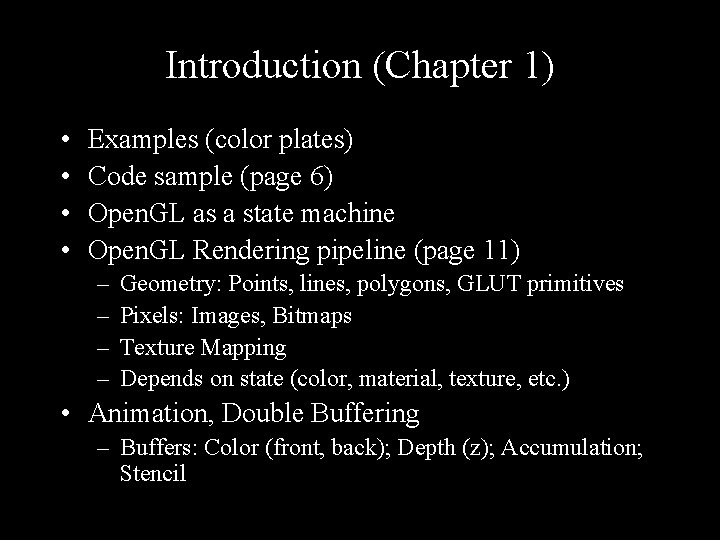
![Drawing (Chapter 2) • gl. Clear. Color(red, green, blue, alpha) [0, 0, 0, 0] Drawing (Chapter 2) • gl. Clear. Color(red, green, blue, alpha) [0, 0, 0, 0]](https://slidetodoc.com/presentation_image_h2/61765dcdaeb420c99c7db7927f64a1ee/image-15.jpg)
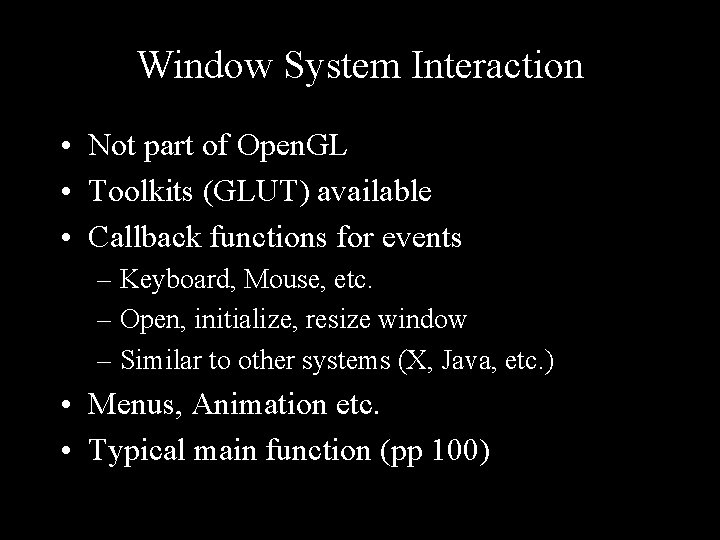
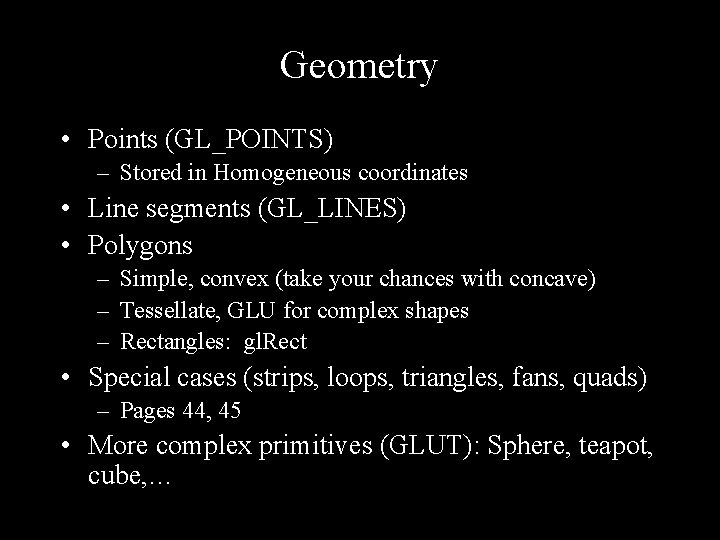
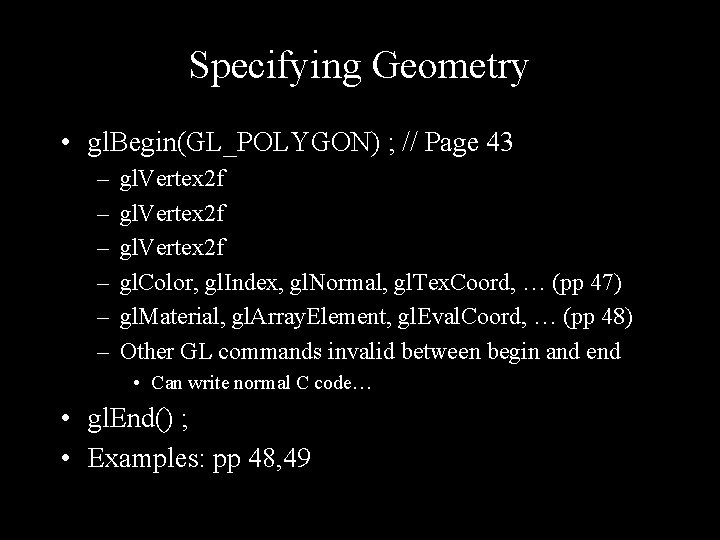
- Slides: 18
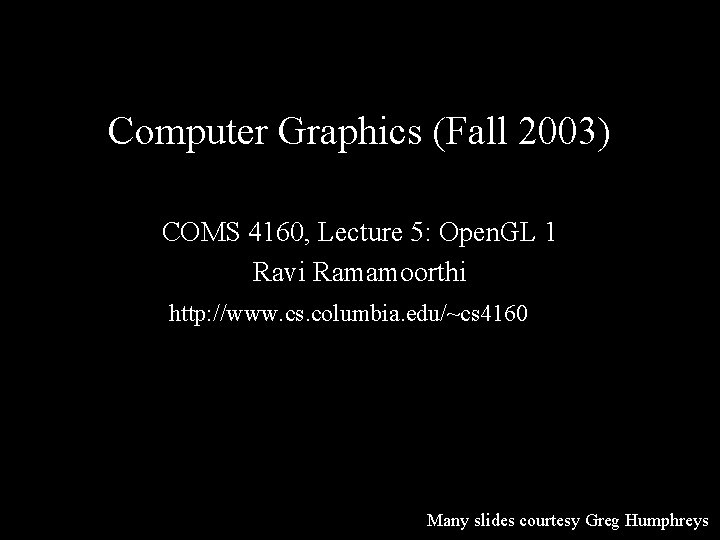
Computer Graphics (Fall 2003) COMS 4160, Lecture 5: Open. GL 1 Ravi Ramamoorthi http: //www. cs. columbia. edu/~cs 4160 Many slides courtesy Greg Humphreys
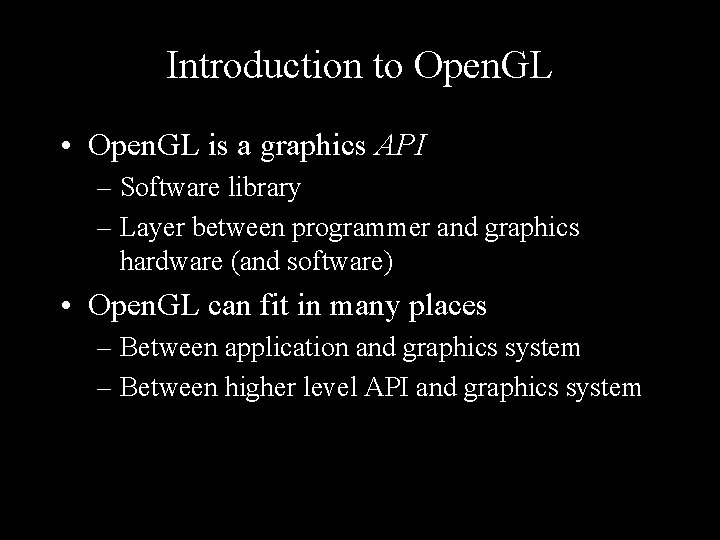
Introduction to Open. GL • Open. GL is a graphics API – Software library – Layer between programmer and graphics hardware (and software) • Open. GL can fit in many places – Between application and graphics system – Between higher level API and graphics system
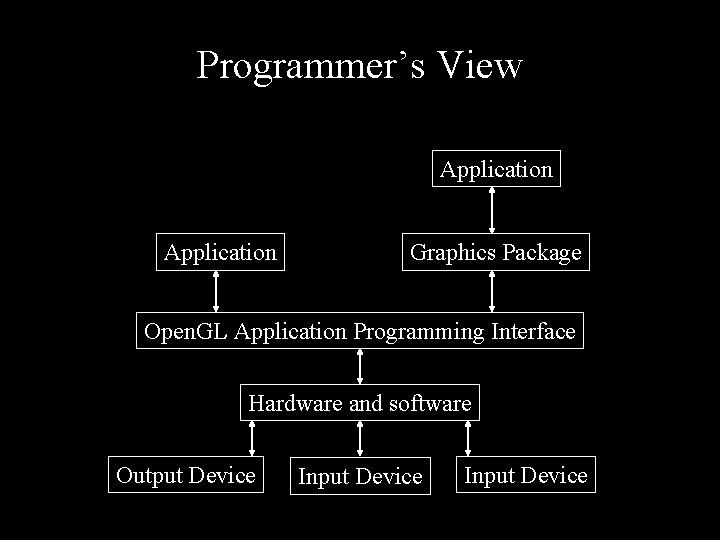
Programmer’s View Application Graphics Package Open. GL Application Programming Interface Hardware and software Output Device Input Device
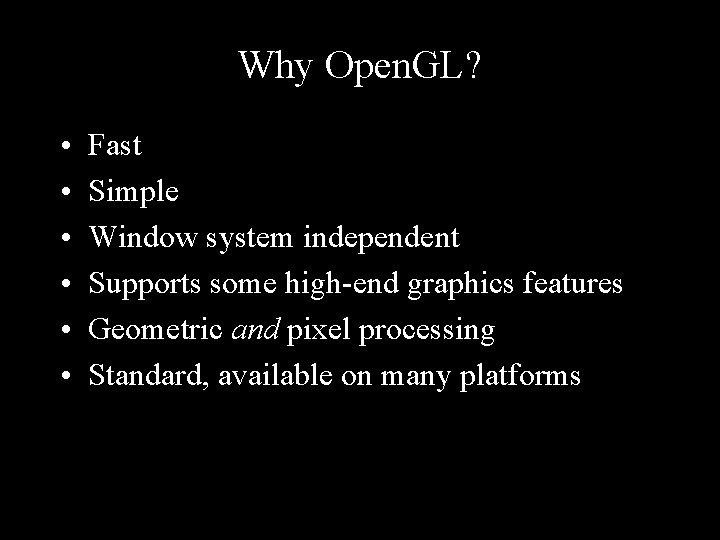
Why Open. GL? • • • Fast Simple Window system independent Supports some high-end graphics features Geometric and pixel processing Standard, available on many platforms
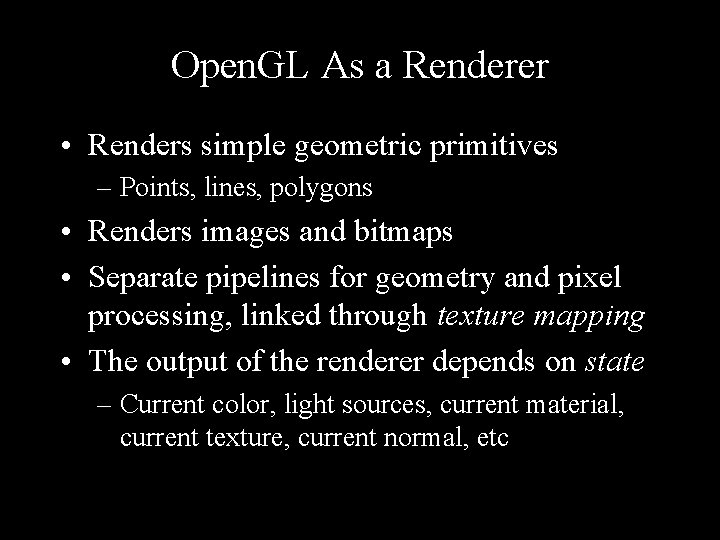
Open. GL As a Renderer • Renders simple geometric primitives – Points, lines, polygons • Renders images and bitmaps • Separate pipelines for geometry and pixel processing, linked through texture mapping • The output of the renderer depends on state – Current color, light sources, current material, current texture, current normal, etc
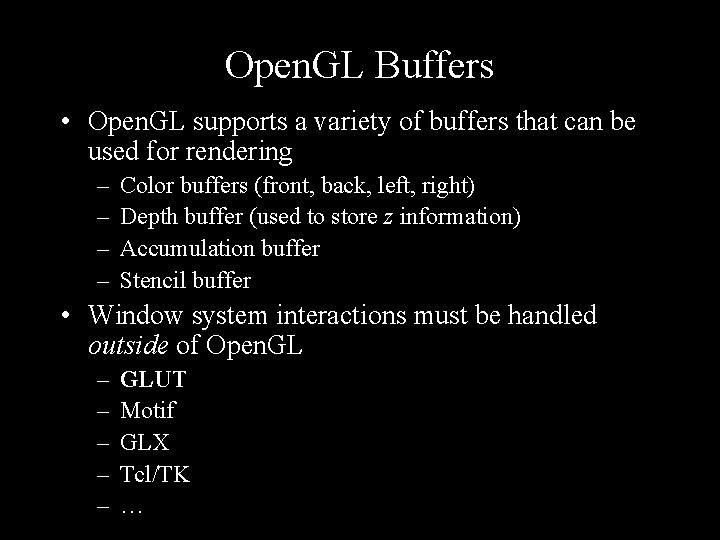
Open. GL Buffers • Open. GL supports a variety of buffers that can be used for rendering – – Color buffers (front, back, left, right) Depth buffer (used to store z information) Accumulation buffer Stencil buffer • Window system interactions must be handled outside of Open. GL – – – GLUT Motif GLX Tcl/TK …
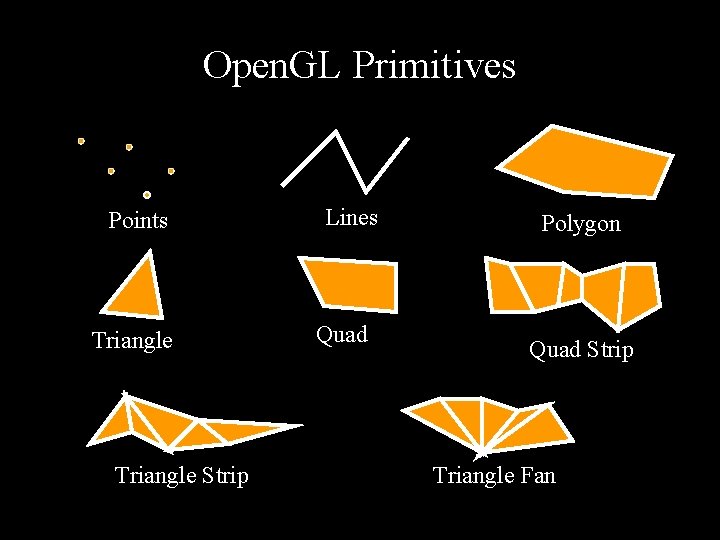
Open. GL Primitives Points Triangle Strip Lines Quad Polygon Quad Strip Triangle Fan
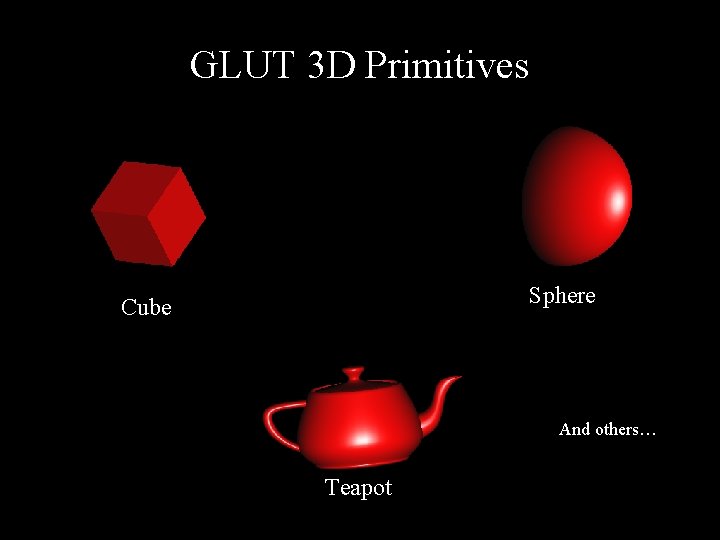
GLUT 3 D Primitives Sphere Cube And others… Teapot
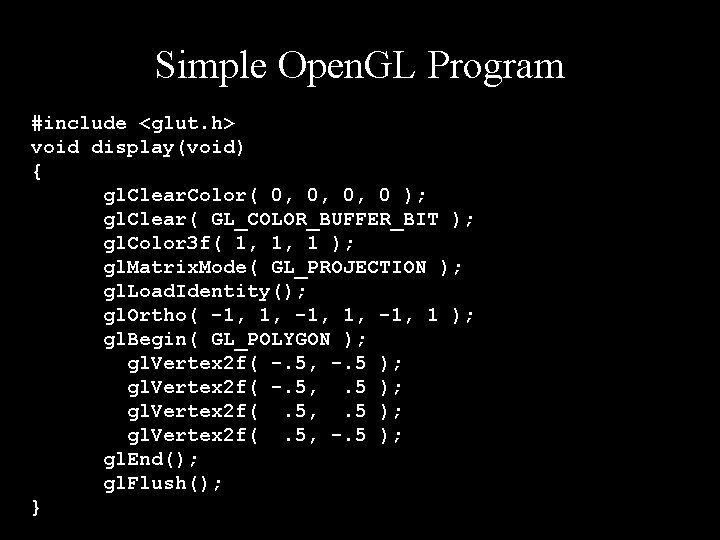
Simple Open. GL Program #include <glut. h> void display(void) { gl. Clear. Color( 0, 0, 0, 0 ); gl. Clear( GL_COLOR_BUFFER_BIT ); gl. Color 3 f( 1, 1, 1 ); gl. Matrix. Mode( GL_PROJECTION ); gl. Load. Identity(); gl. Ortho( -1, 1, -1, 1 ); gl. Begin( GL_POLYGON ); gl. Vertex 2 f( -. 5, -. 5 ); gl. Vertex 2 f( -. 5, . 5 ); gl. Vertex 2 f(. 5, -. 5 ); gl. End(); gl. Flush(); }
![Window System Glue int main int argc char argv glut Init argc Window System Glue int main( int argc, char *argv[] ) { glut. Init( &argc,](https://slidetodoc.com/presentation_image_h2/61765dcdaeb420c99c7db7927f64a1ee/image-10.jpg)
Window System Glue int main( int argc, char *argv[] ) { glut. Init( &argc, argv ); glut. Init. Display. Mode( GLUT_SINGLE | GLUT_RGB ); glut. Init. Window. Size( 250, 250 ); glut. Create. Window( “We love COMS 4160” ); glut. Display. Func( display ); glut. Main. Loop(); }
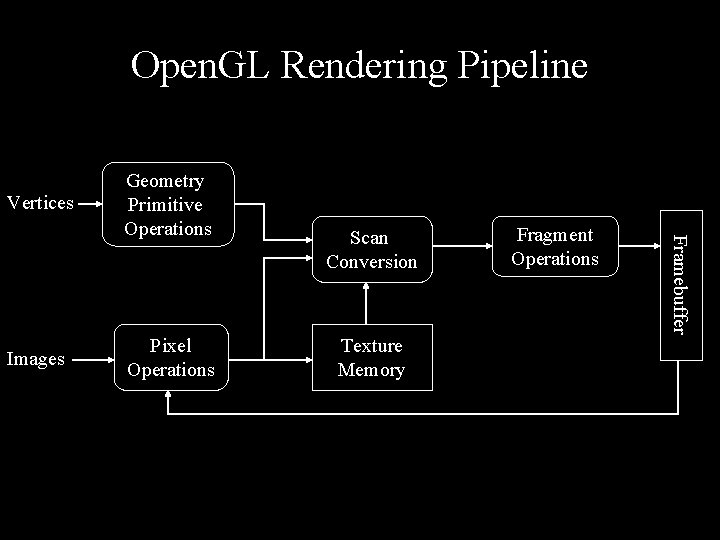
Open. GL Rendering Pipeline Vertices Pixel Operations Scan Conversion Texture Memory Fragment Operations Framebuffer Images Geometry Primitive Operations
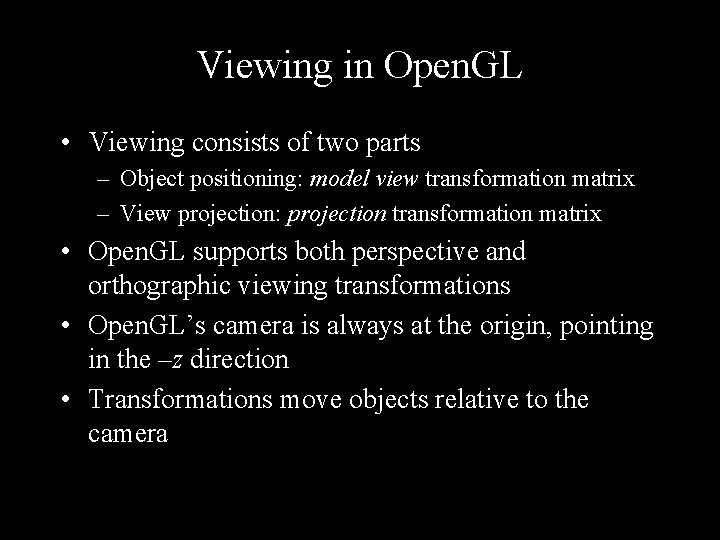
Viewing in Open. GL • Viewing consists of two parts – Object positioning: model view transformation matrix – View projection: projection transformation matrix • Open. GL supports both perspective and orthographic viewing transformations • Open. GL’s camera is always at the origin, pointing in the –z direction • Transformations move objects relative to the camera
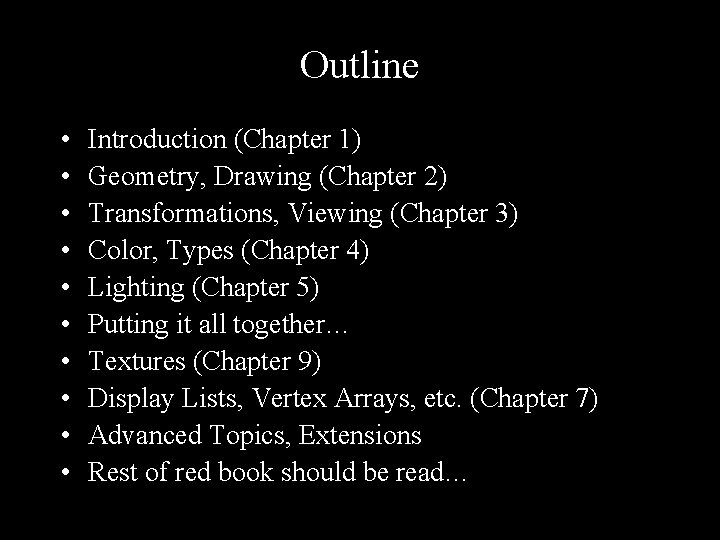
Outline • • • Introduction (Chapter 1) Geometry, Drawing (Chapter 2) Transformations, Viewing (Chapter 3) Color, Types (Chapter 4) Lighting (Chapter 5) Putting it all together… Textures (Chapter 9) Display Lists, Vertex Arrays, etc. (Chapter 7) Advanced Topics, Extensions Rest of red book should be read…
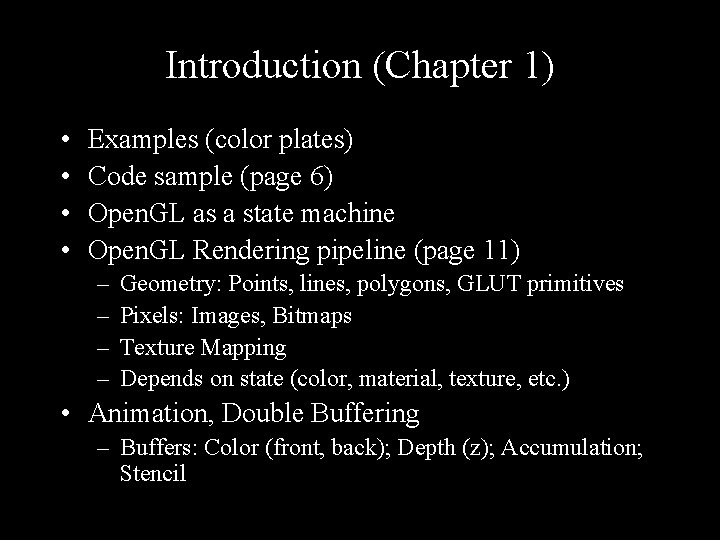
Introduction (Chapter 1) • • Examples (color plates) Code sample (page 6) Open. GL as a state machine Open. GL Rendering pipeline (page 11) – – Geometry: Points, lines, polygons, GLUT primitives Pixels: Images, Bitmaps Texture Mapping Depends on state (color, material, texture, etc. ) • Animation, Double Buffering – Buffers: Color (front, back); Depth (z); Accumulation; Stencil
![Drawing Chapter 2 gl Clear Colorred green blue alpha 0 0 0 0 Drawing (Chapter 2) • gl. Clear. Color(red, green, blue, alpha) [0, 0, 0, 0]](https://slidetodoc.com/presentation_image_h2/61765dcdaeb420c99c7db7927f64a1ee/image-15.jpg)
Drawing (Chapter 2) • gl. Clear. Color(red, green, blue, alpha) [0, 0, 0, 0] • gl. Clear (GL_COLOR_BUFFER_BIT) – Other buffers… • gl. Color 3 f (r, g, b) • Draw (assembly line: Transform, clip, shade, …) – Client/Server • gl. Flush() (forces client to send network packet) – Doesn’t wait for completion; only begins execution – Previous commands execute in finite time • gl. Finish() (waits for ack that drawing complete) – Synchronization, sparing use
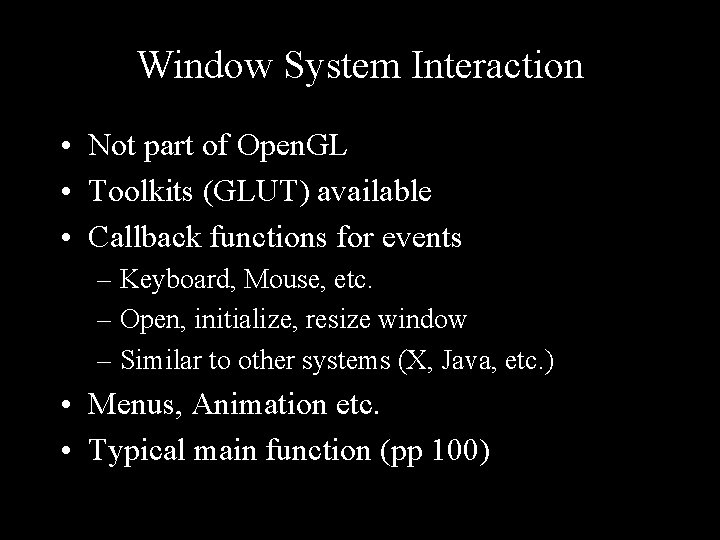
Window System Interaction • Not part of Open. GL • Toolkits (GLUT) available • Callback functions for events – Keyboard, Mouse, etc. – Open, initialize, resize window – Similar to other systems (X, Java, etc. ) • Menus, Animation etc. • Typical main function (pp 100)
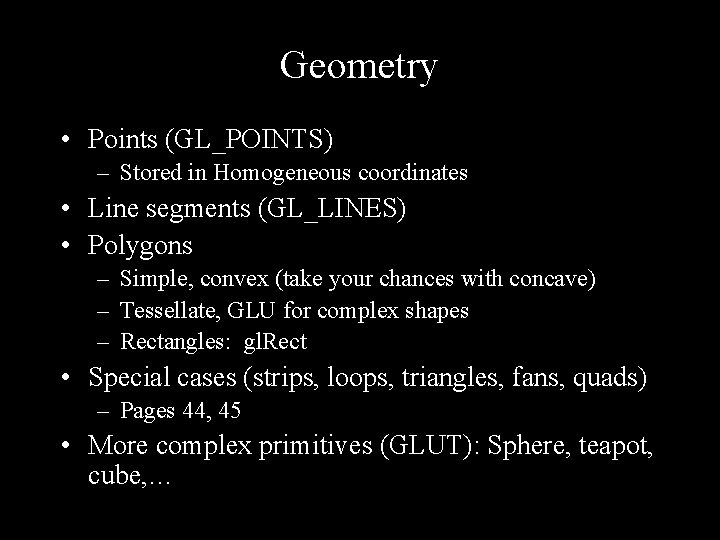
Geometry • Points (GL_POINTS) – Stored in Homogeneous coordinates • Line segments (GL_LINES) • Polygons – Simple, convex (take your chances with concave) – Tessellate, GLU for complex shapes – Rectangles: gl. Rect • Special cases (strips, loops, triangles, fans, quads) – Pages 44, 45 • More complex primitives (GLUT): Sphere, teapot, cube, …
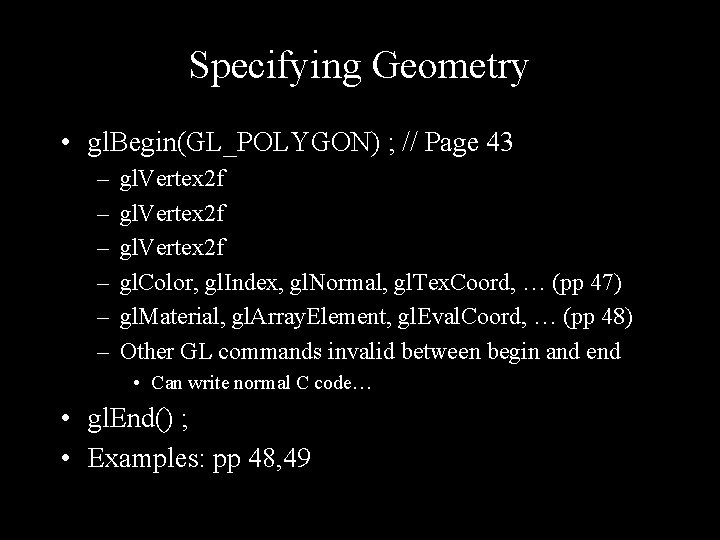
Specifying Geometry • gl. Begin(GL_POLYGON) ; // Page 43 – – – gl. Vertex 2 f gl. Color, gl. Index, gl. Normal, gl. Tex. Coord, … (pp 47) gl. Material, gl. Array. Element, gl. Eval. Coord, … (pp 48) Other GL commands invalid between begin and end • Can write normal C code… • gl. End() ; • Examples: pp 48, 49