CHAPTER 4 Repetition Structures Topics Augmented Assignment Operators
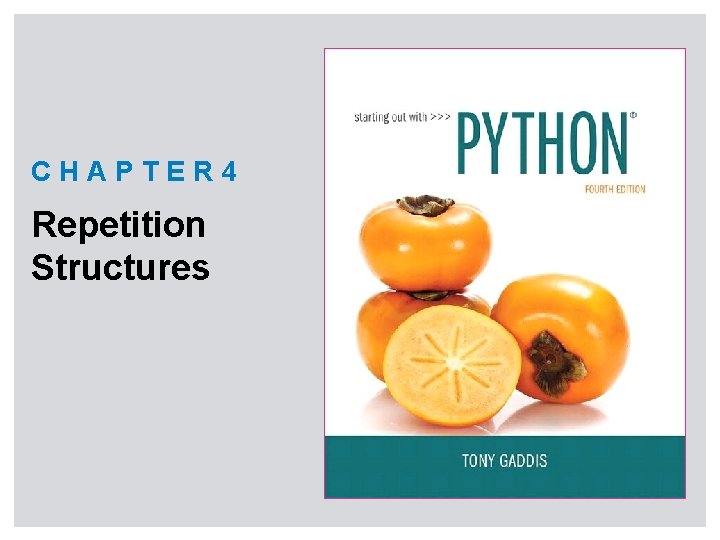
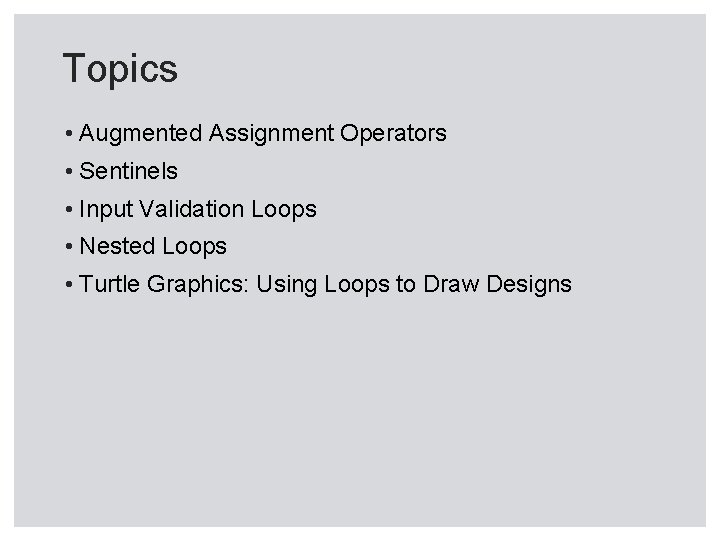
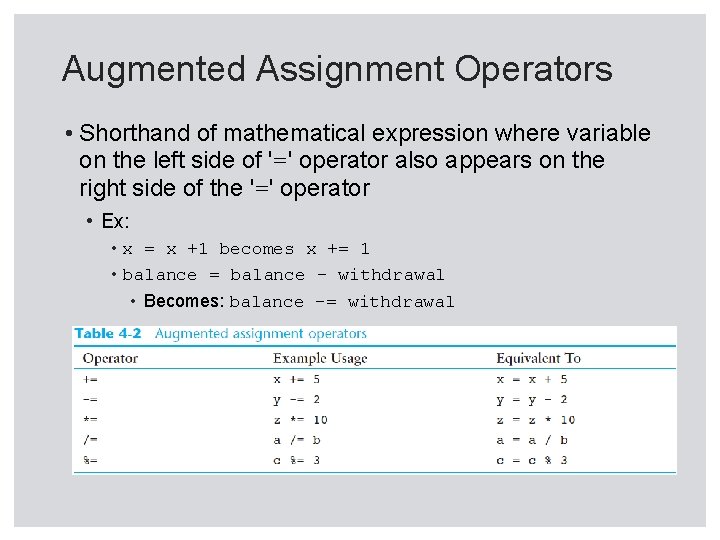
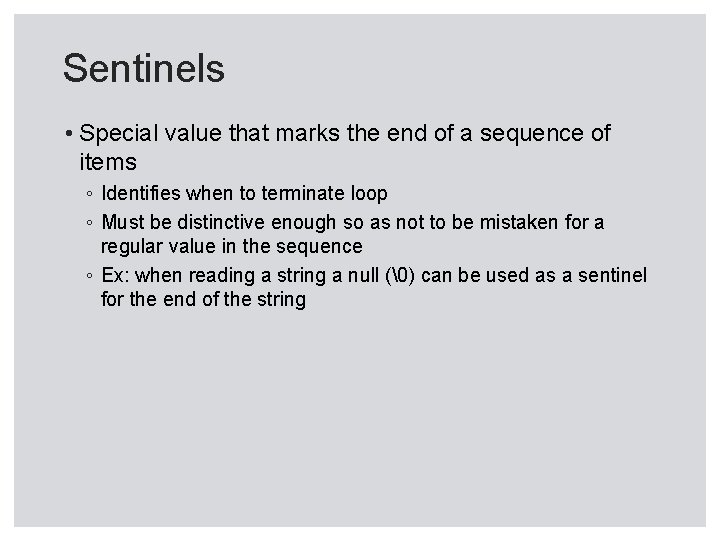
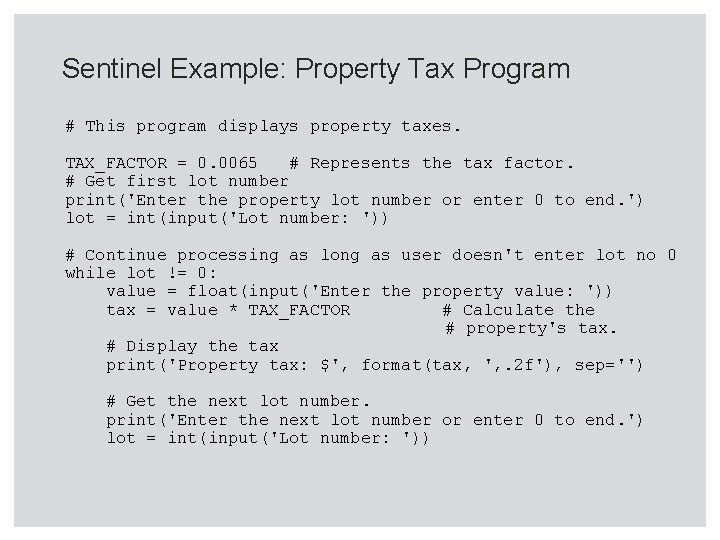
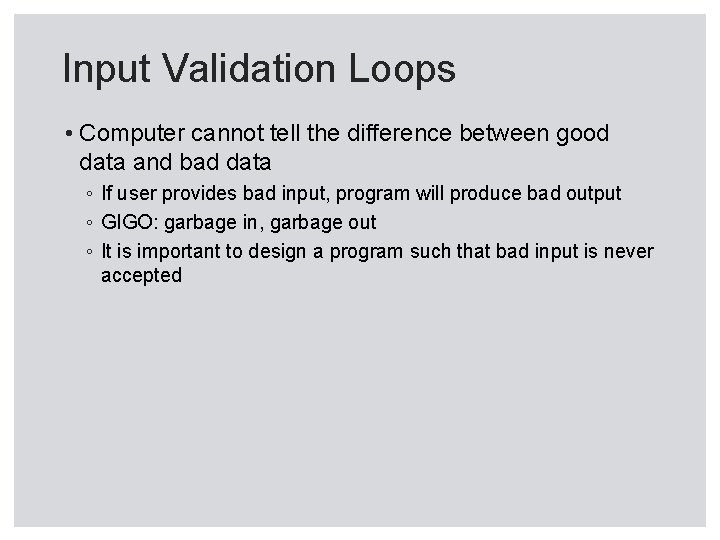
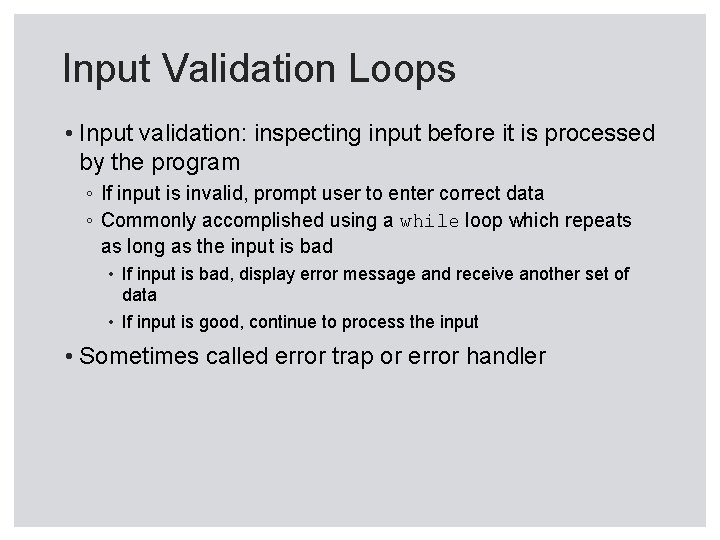
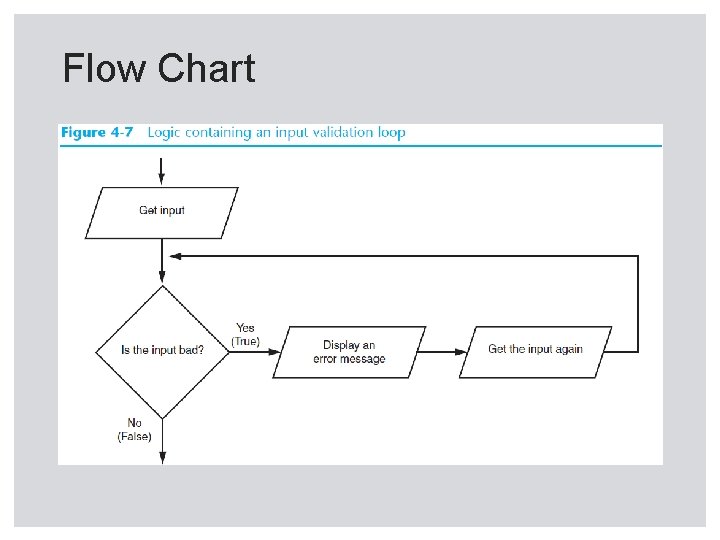
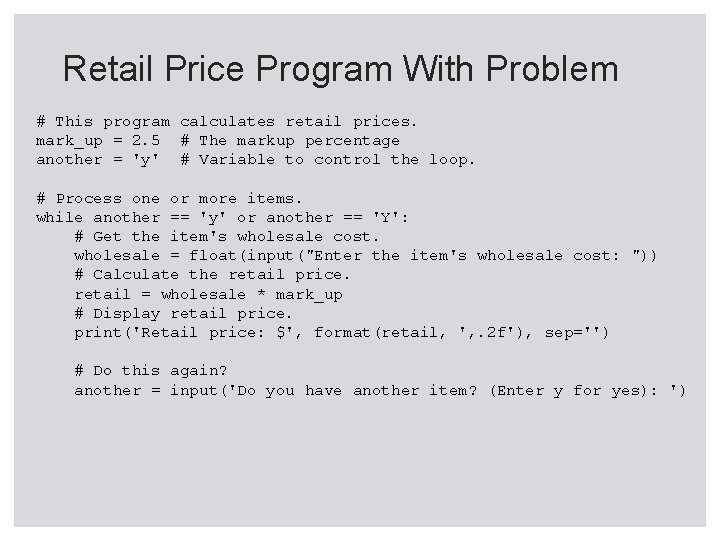
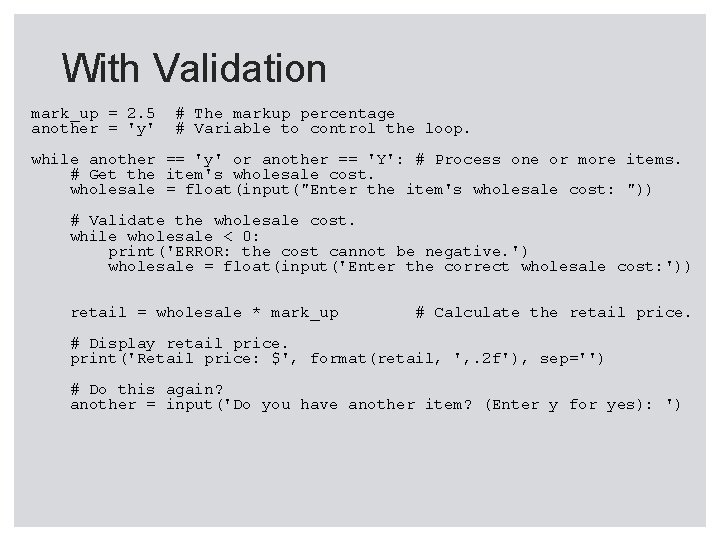
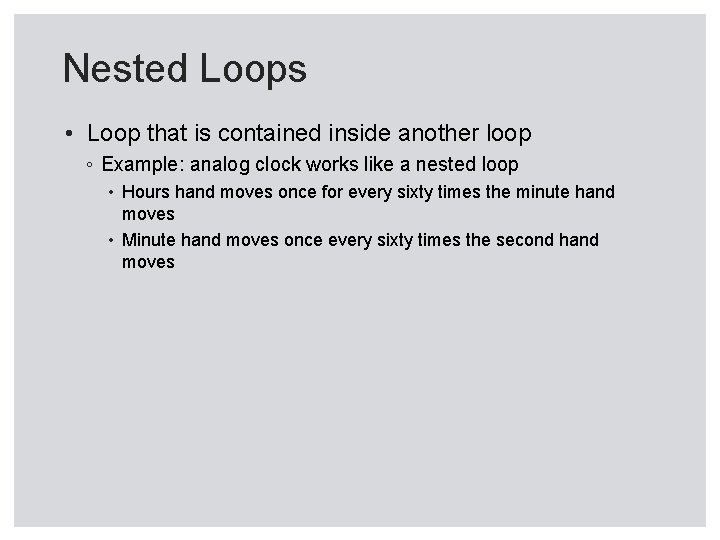
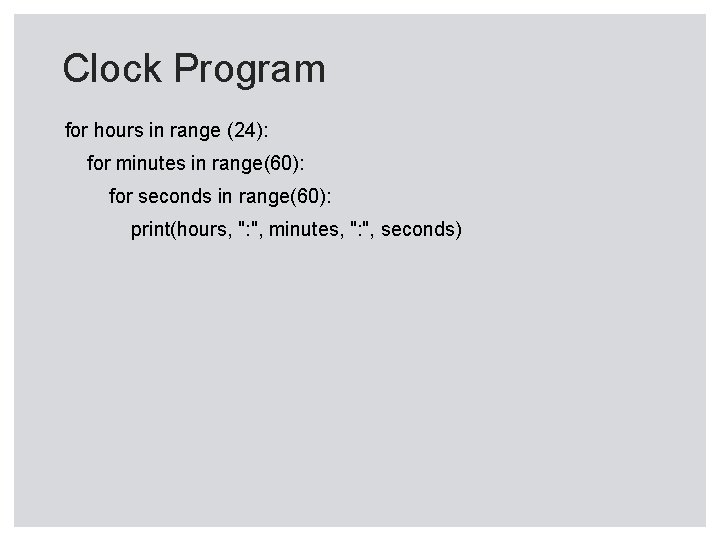
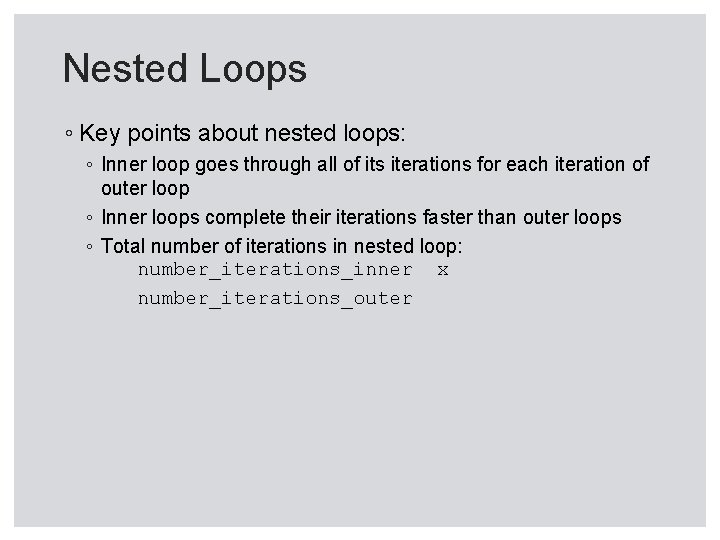
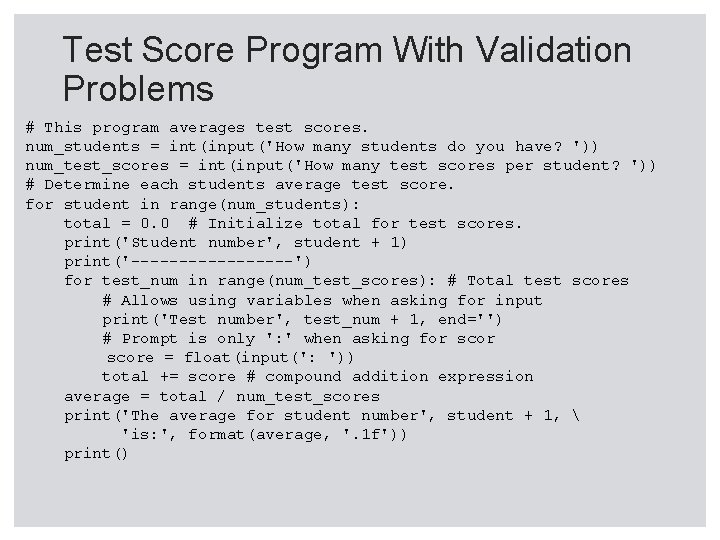
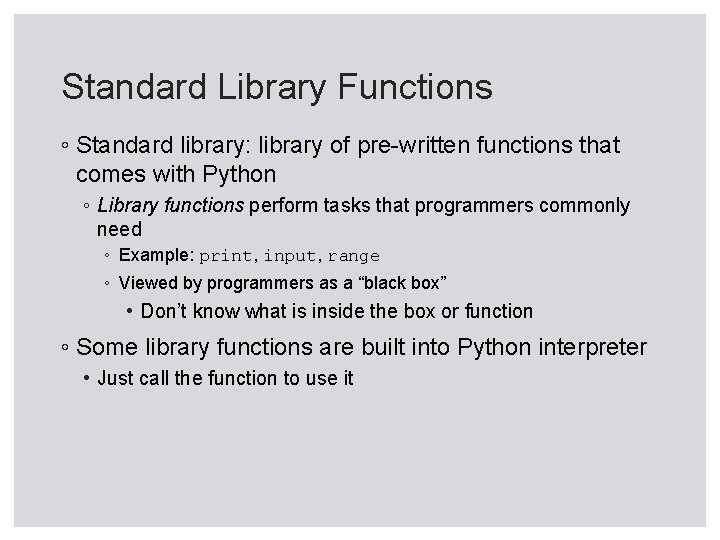
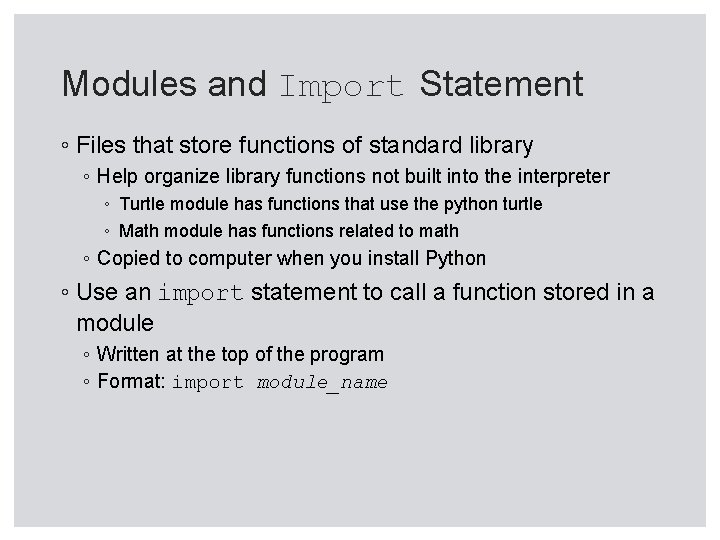
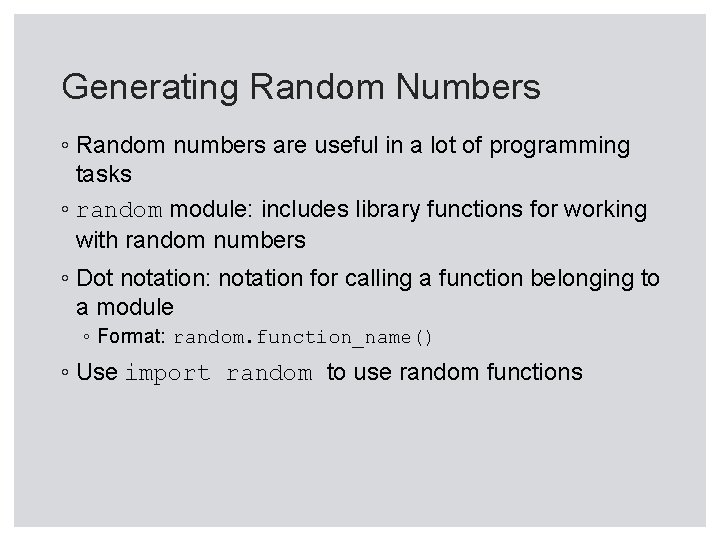
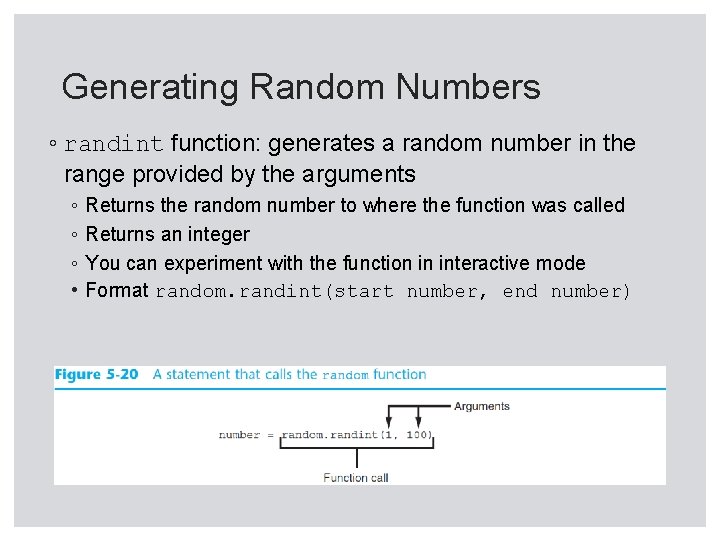
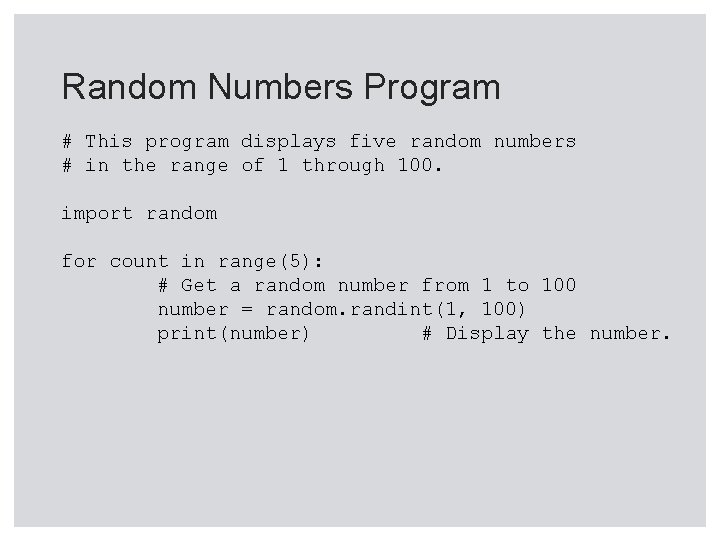
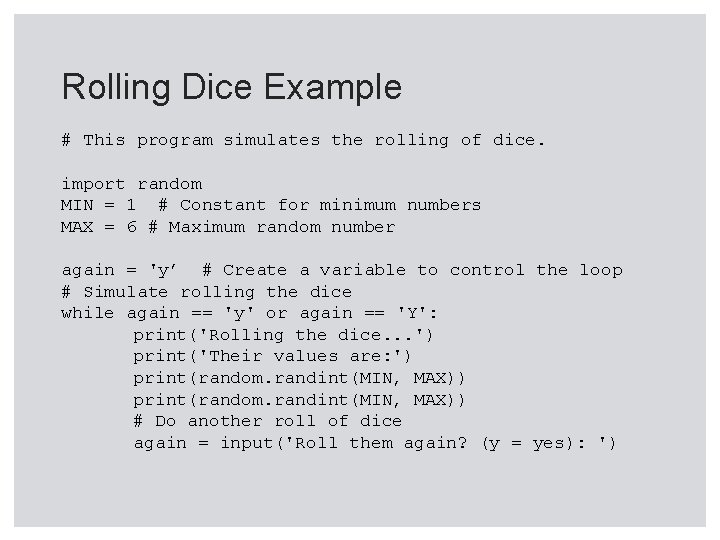
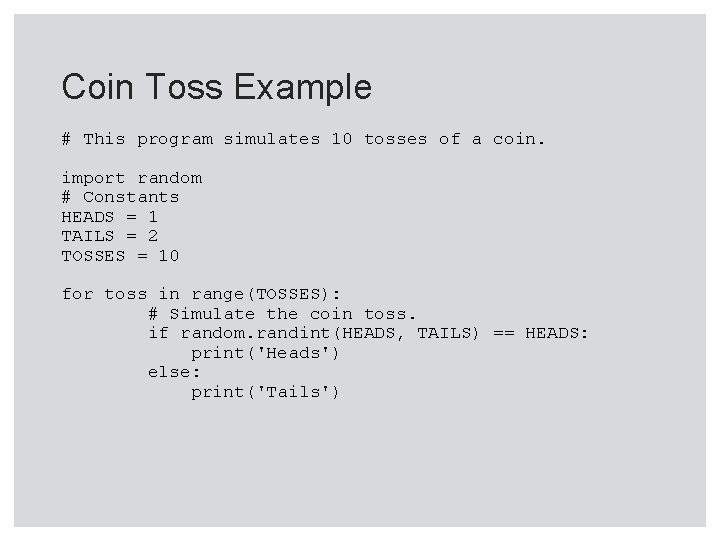
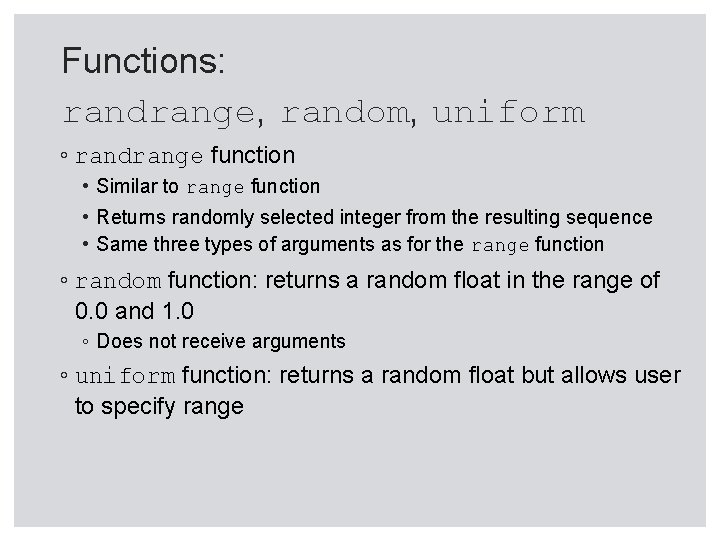
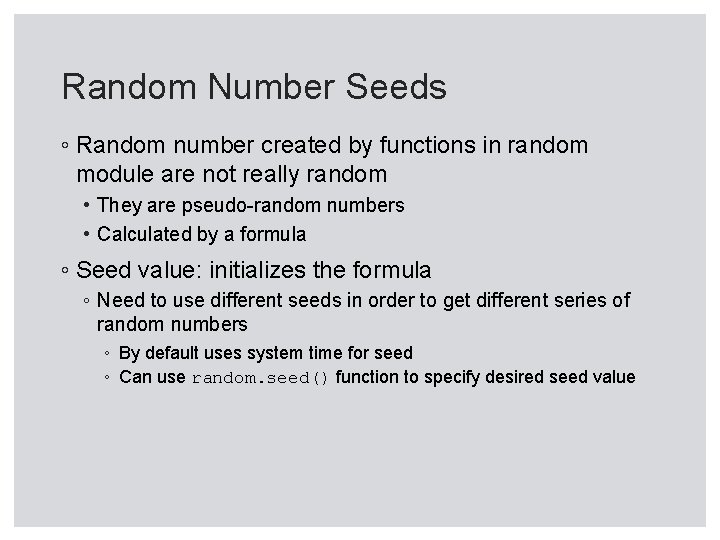
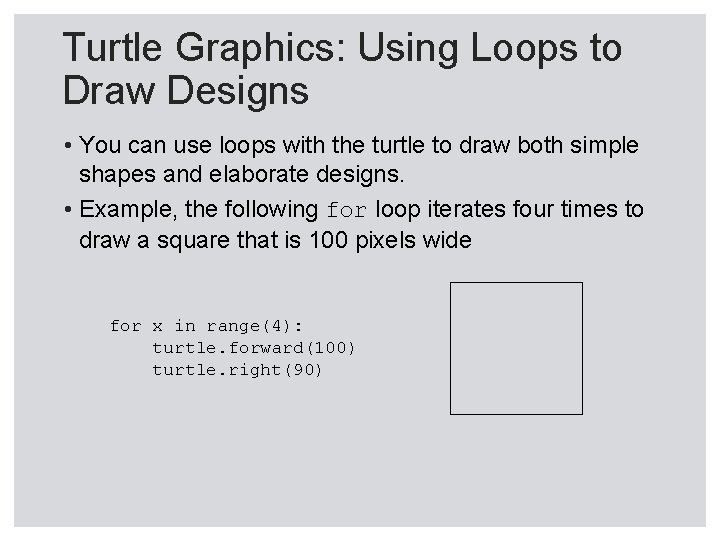
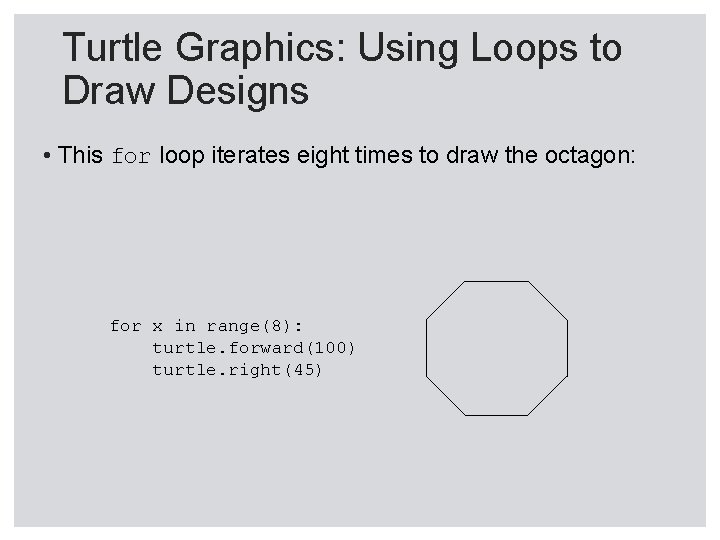
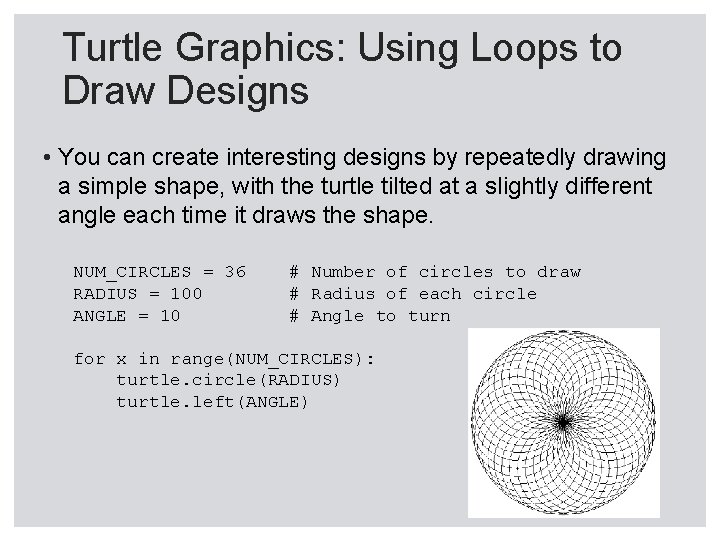
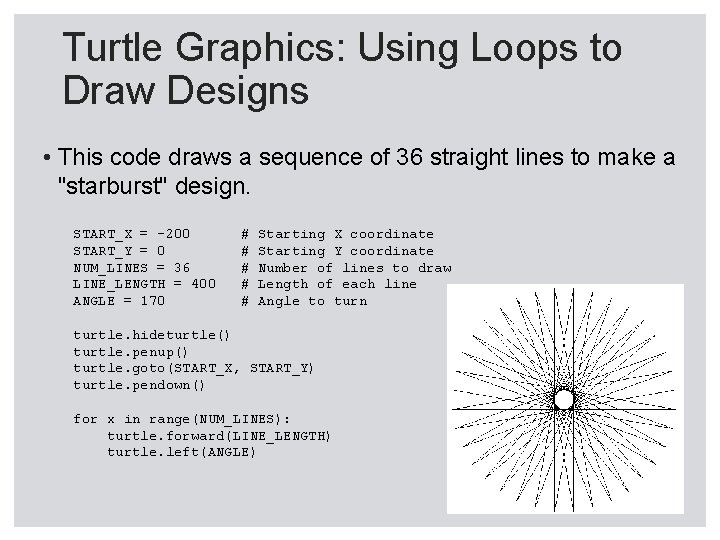
- Slides: 27
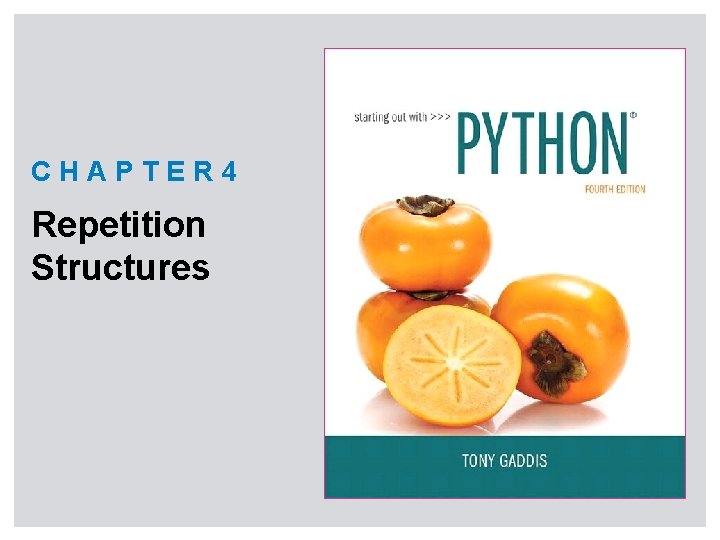
CHAPTER 4 Repetition Structures
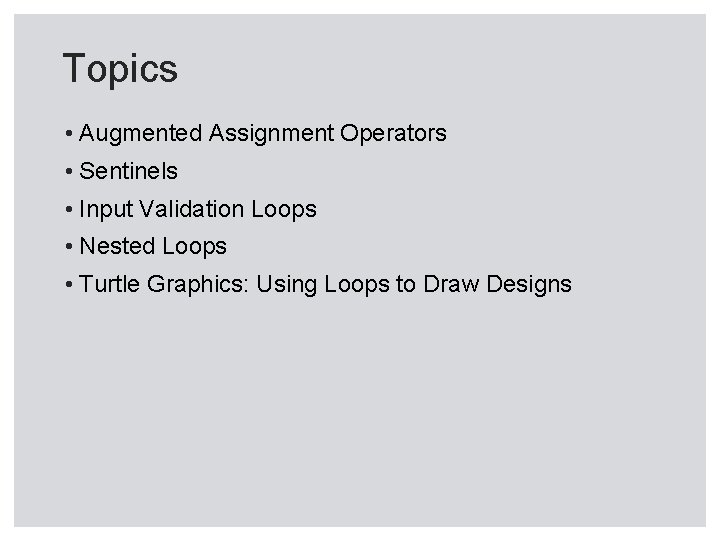
Topics • Augmented Assignment Operators • Sentinels • Input Validation Loops • Nested Loops • Turtle Graphics: Using Loops to Draw Designs
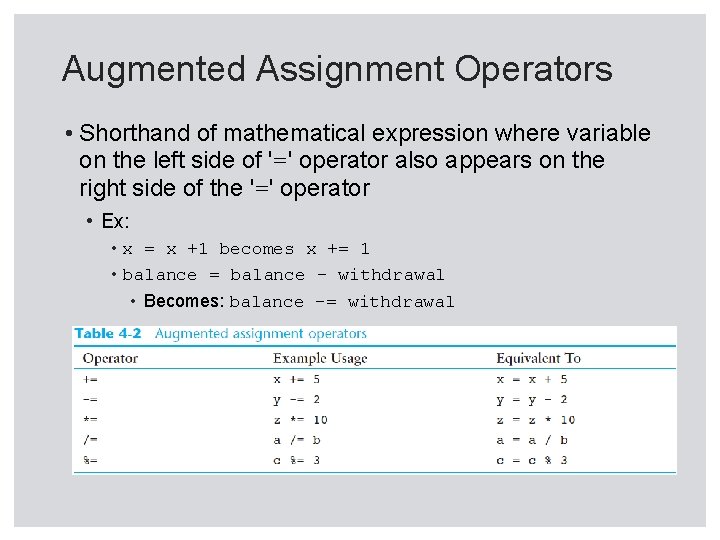
Augmented Assignment Operators • Shorthand of mathematical expression where variable on the left side of '=' operator also appears on the right side of the '=' operator • Ex: • x = x +1 becomes x += 1 • balance = balance – withdrawal • Becomes: balance -= withdrawal
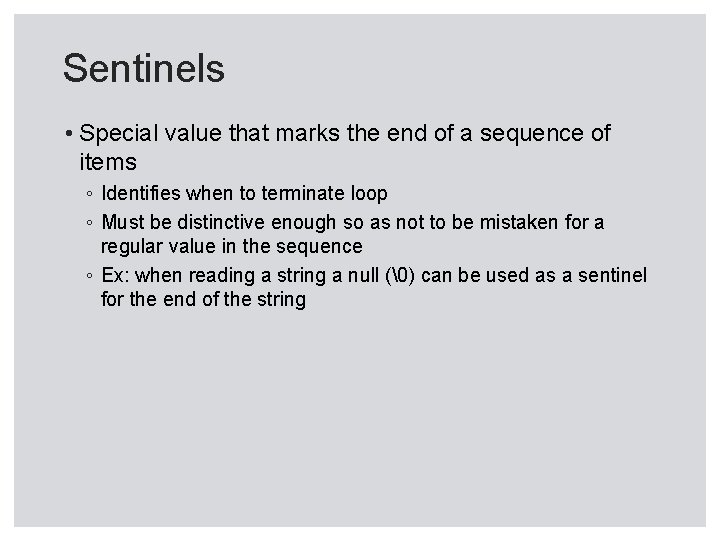
Sentinels • Special value that marks the end of a sequence of items ◦ Identifies when to terminate loop ◦ Must be distinctive enough so as not to be mistaken for a regular value in the sequence ◦ Ex: when reading a string a null ( ) can be used as a sentinel for the end of the string
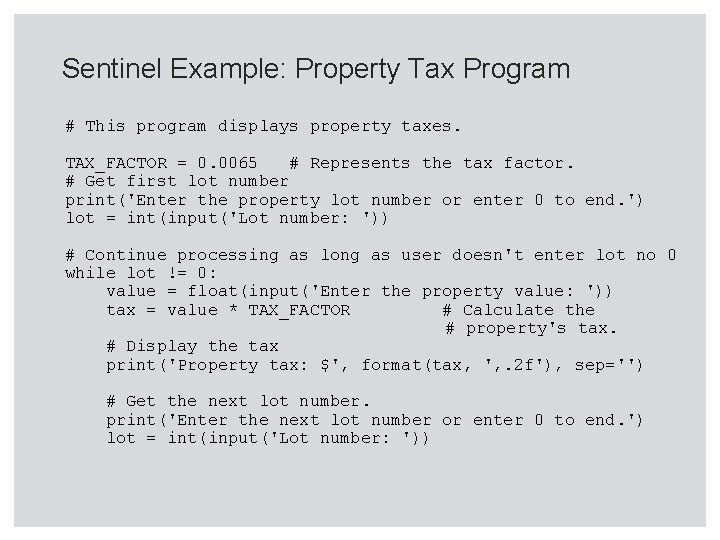
Sentinel Example: Property Tax Program # This program displays property taxes. TAX_FACTOR = 0. 0065 # Represents the tax factor. # Get first lot number print('Enter the property lot number or enter 0 to end. ') lot = int(input('Lot number: ')) # Continue processing as long as user doesn't enter lot no 0 while lot != 0: value = float(input('Enter the property value: ')) tax = value * TAX_FACTOR # Calculate the # property's tax. # Display the tax print('Property tax: $', format(tax, ', . 2 f'), sep='') # Get the next lot number. print('Enter the next lot number or enter 0 to end. ') lot = int(input('Lot number: '))
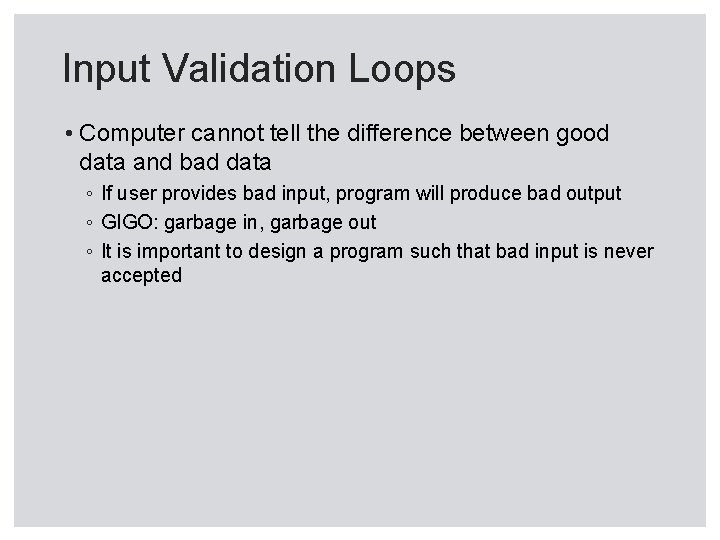
Input Validation Loops • Computer cannot tell the difference between good data and bad data ◦ If user provides bad input, program will produce bad output ◦ GIGO: garbage in, garbage out ◦ It is important to design a program such that bad input is never accepted
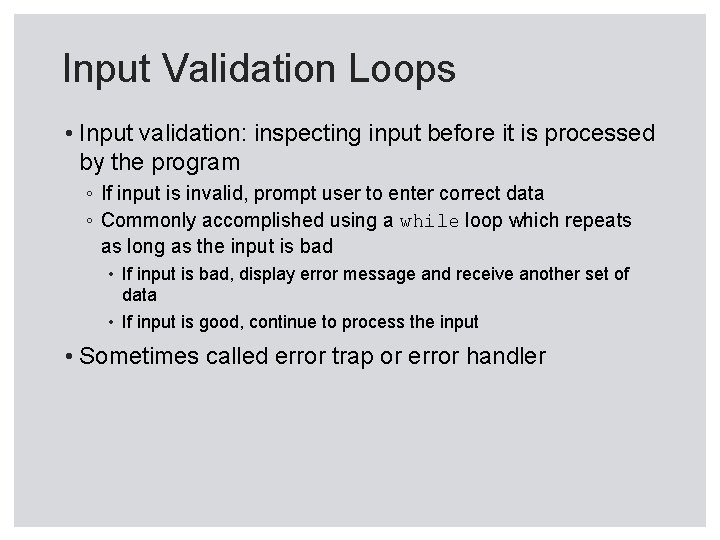
Input Validation Loops • Input validation: inspecting input before it is processed by the program ◦ If input is invalid, prompt user to enter correct data ◦ Commonly accomplished using a while loop which repeats as long as the input is bad • If input is bad, display error message and receive another set of data • If input is good, continue to process the input • Sometimes called error trap or error handler
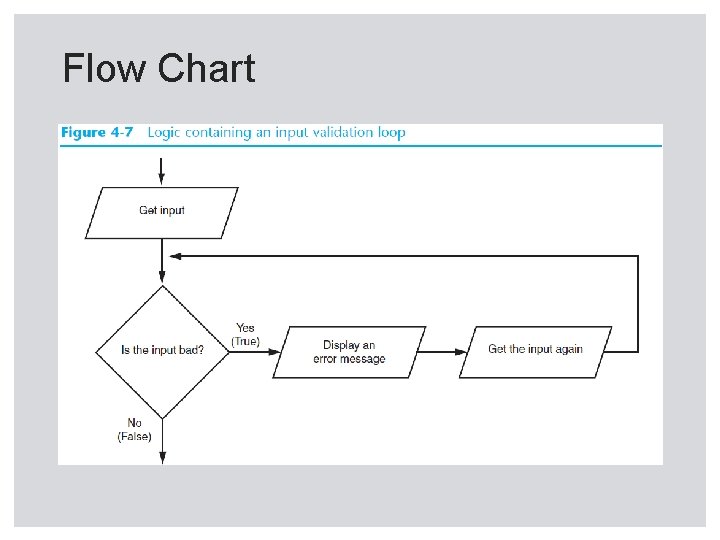
Flow Chart
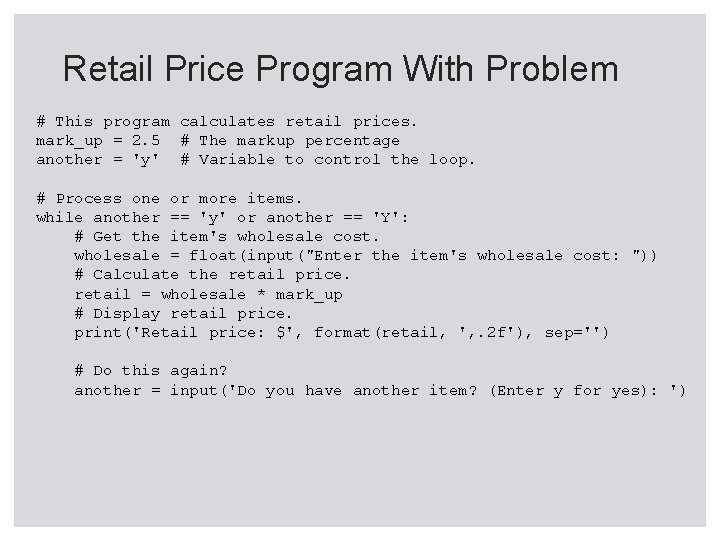
Retail Price Program With Problem # This program calculates retail prices. mark_up = 2. 5 # The markup percentage another = 'y' # Variable to control the loop. # Process one or more items. while another == 'y' or another == 'Y': # Get the item's wholesale cost. wholesale = float(input("Enter the item's wholesale cost: ")) # Calculate the retail price. retail = wholesale * mark_up # Display retail price. print('Retail price: $', format(retail, ', . 2 f'), sep='') # Do this again? another = input('Do you have another item? (Enter y for yes): ')
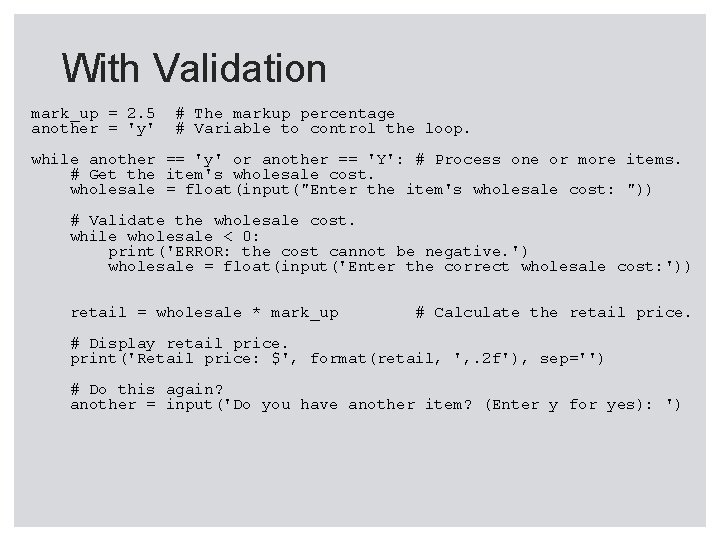
With Validation mark_up = 2. 5 another = 'y' # The markup percentage # Variable to control the loop. while another == 'y' or another == 'Y': # Process one or more items. # Get the item's wholesale cost. wholesale = float(input("Enter the item's wholesale cost: ")) # Validate the wholesale cost. while wholesale < 0: print('ERROR: the cost cannot be negative. ') wholesale = float(input('Enter the correct wholesale cost: ')) retail = wholesale * mark_up # Calculate the retail price. # Display retail price. print('Retail price: $', format(retail, ', . 2 f'), sep='') # Do this again? another = input('Do you have another item? (Enter y for yes): ')
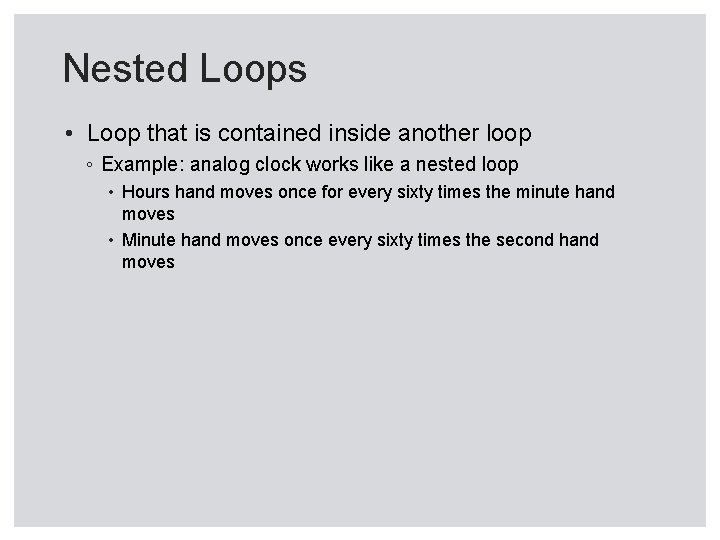
Nested Loops • Loop that is contained inside another loop ◦ Example: analog clock works like a nested loop • Hours hand moves once for every sixty times the minute hand moves • Minute hand moves once every sixty times the second hand moves
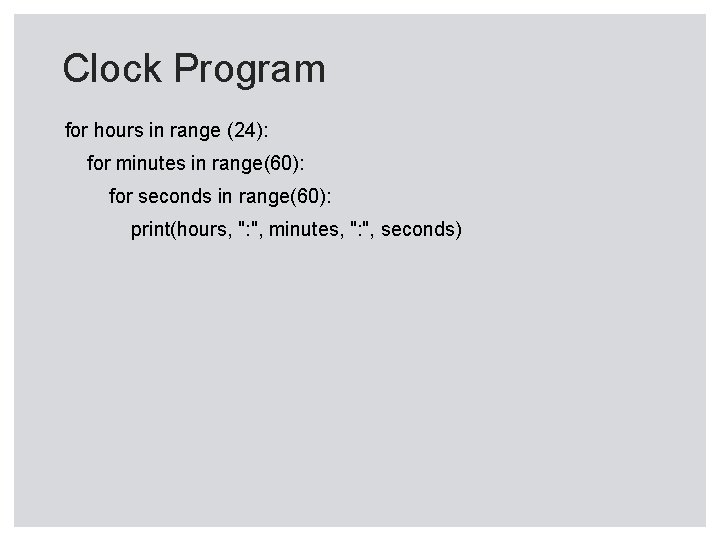
Clock Program for hours in range (24): for minutes in range(60): for seconds in range(60): print(hours, ": ", minutes, ": ", seconds)
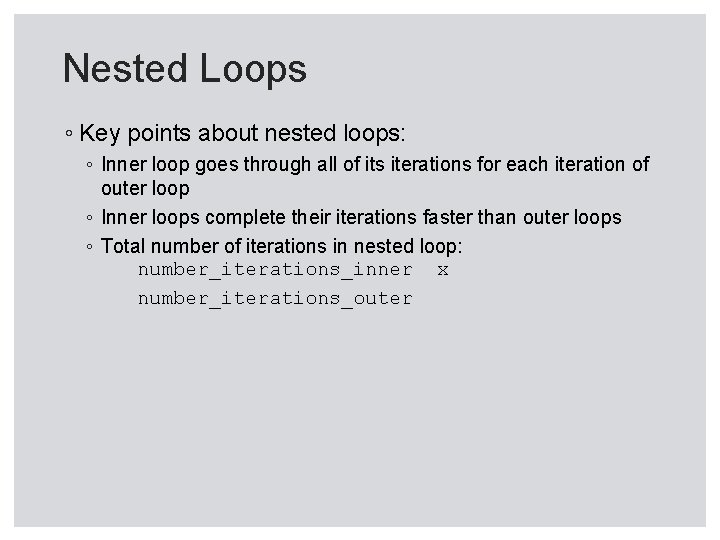
Nested Loops ◦ Key points about nested loops: ◦ Inner loop goes through all of its iterations for each iteration of outer loop ◦ Inner loops complete their iterations faster than outer loops ◦ Total number of iterations in nested loop: number_iterations_inner x number_iterations_outer
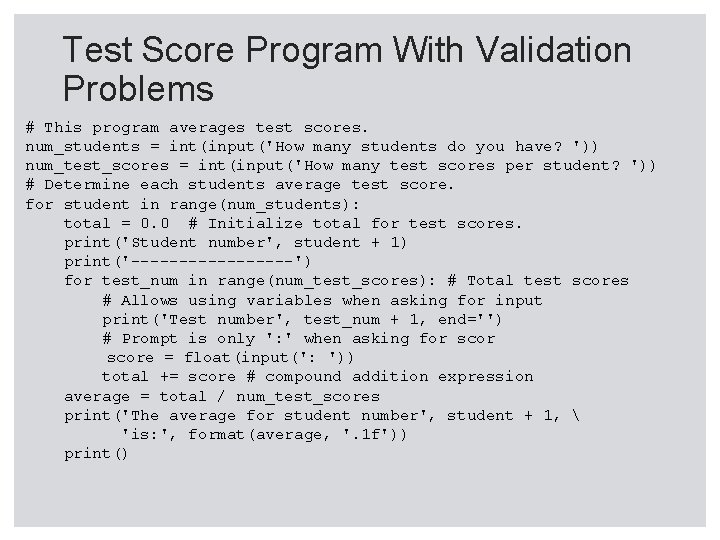
Test Score Program With Validation Problems # This program averages test scores. num_students = int(input('How many students do you have? ')) num_test_scores = int(input('How many test scores per student? ')) # Determine each students average test score. for student in range(num_students): total = 0. 0 # Initialize total for test scores. print('Student number', student + 1) print('---------') for test_num in range(num_test_scores): # Total test scores # Allows using variables when asking for input print('Test number', test_num + 1, end='') # Prompt is only ': ' when asking for score = float(input(': ')) total += score # compound addition expression average = total / num_test_scores print('The average for student number', student + 1, 'is: ', format(average, '. 1 f')) print()
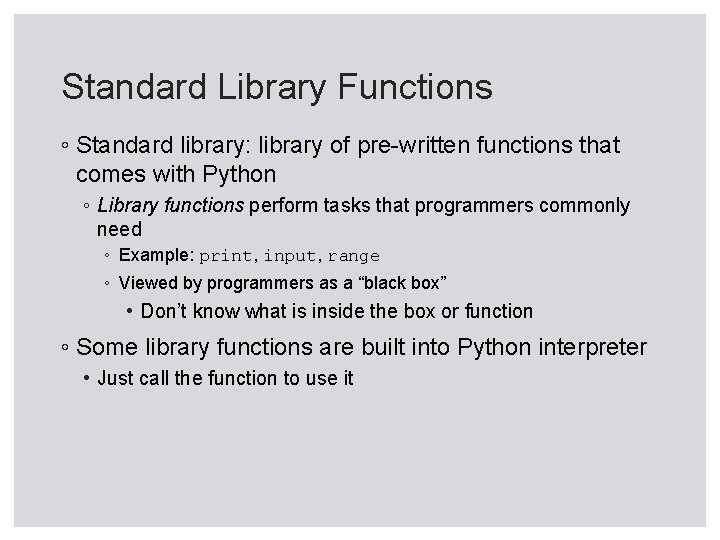
Standard Library Functions ◦ Standard library: library of pre-written functions that comes with Python ◦ Library functions perform tasks that programmers commonly need ◦ Example: print, input, range ◦ Viewed by programmers as a “black box” • Don’t know what is inside the box or function ◦ Some library functions are built into Python interpreter • Just call the function to use it
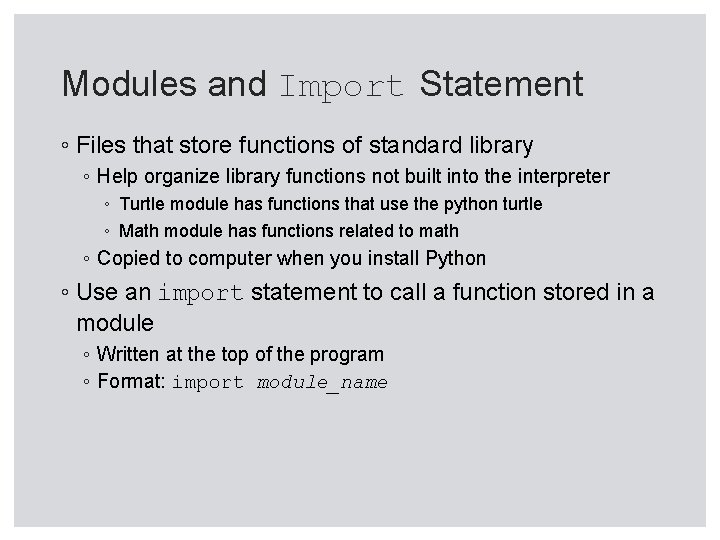
Modules and Import Statement ◦ Files that store functions of standard library ◦ Help organize library functions not built into the interpreter ◦ Turtle module has functions that use the python turtle ◦ Math module has functions related to math ◦ Copied to computer when you install Python ◦ Use an import statement to call a function stored in a module ◦ Written at the top of the program ◦ Format: import module_name
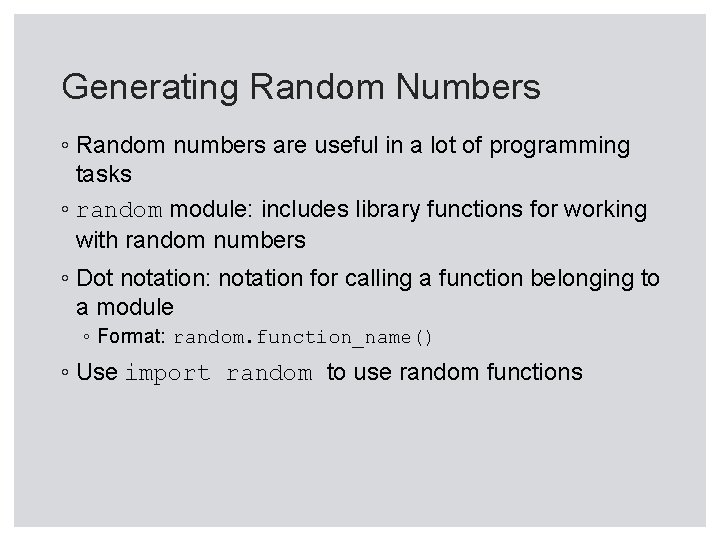
Generating Random Numbers ◦ Random numbers are useful in a lot of programming tasks ◦ random module: includes library functions for working with random numbers ◦ Dot notation: notation for calling a function belonging to a module ◦ Format: random. function_name() ◦ Use import random to use random functions
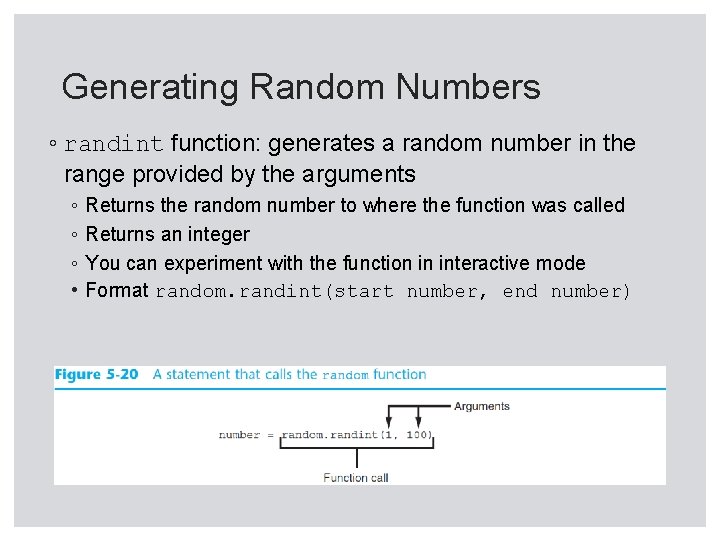
Generating Random Numbers ◦ randint function: generates a random number in the range provided by the arguments ◦ ◦ ◦ • Returns the random number to where the function was called Returns an integer You can experiment with the function in interactive mode Format random. randint(start number, end number)
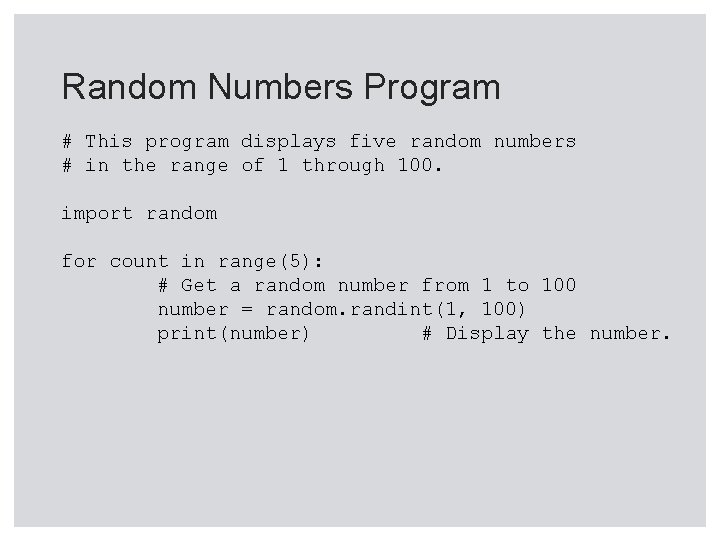
Random Numbers Program # This program displays five random numbers # in the range of 1 through 100. import random for count in range(5): # Get a random number from 1 to 100 number = random. randint(1, 100) print(number) # Display the number.
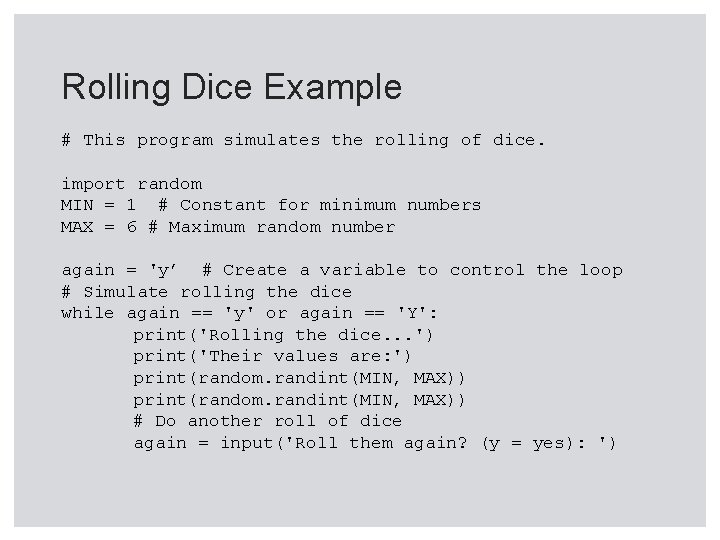
Rolling Dice Example # This program simulates the rolling of dice. import random MIN = 1 # Constant for minimum numbers MAX = 6 # Maximum random number again = 'y’ # Create a variable to control the loop # Simulate rolling the dice while again == 'y' or again == 'Y': print('Rolling the dice. . . ') print('Their values are: ') print(random. randint(MIN, MAX)) # Do another roll of dice again = input('Roll them again? (y = yes): ')
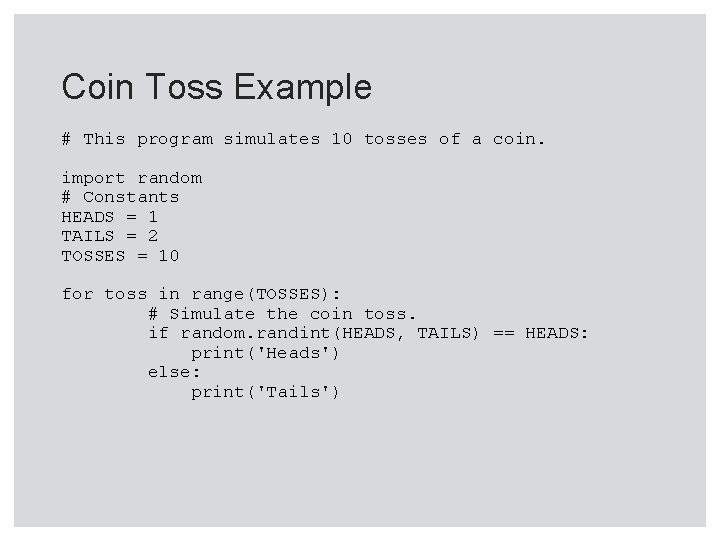
Coin Toss Example # This program simulates 10 tosses of a coin. import random # Constants HEADS = 1 TAILS = 2 TOSSES = 10 for toss in range(TOSSES): # Simulate the coin toss. if random. randint(HEADS, TAILS) == HEADS: print('Heads') else: print('Tails')
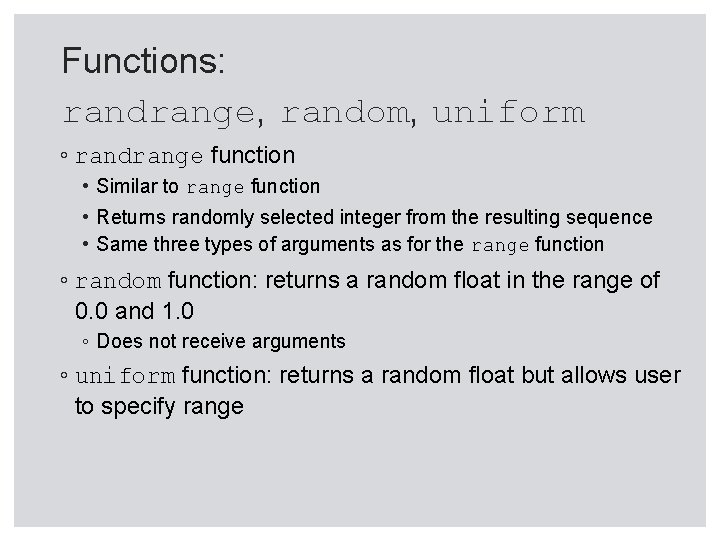
Functions: randrange, random, uniform ◦ randrange function • Similar to range function • Returns randomly selected integer from the resulting sequence • Same three types of arguments as for the range function ◦ random function: returns a random float in the range of 0. 0 and 1. 0 ◦ Does not receive arguments ◦ uniform function: returns a random float but allows user to specify range
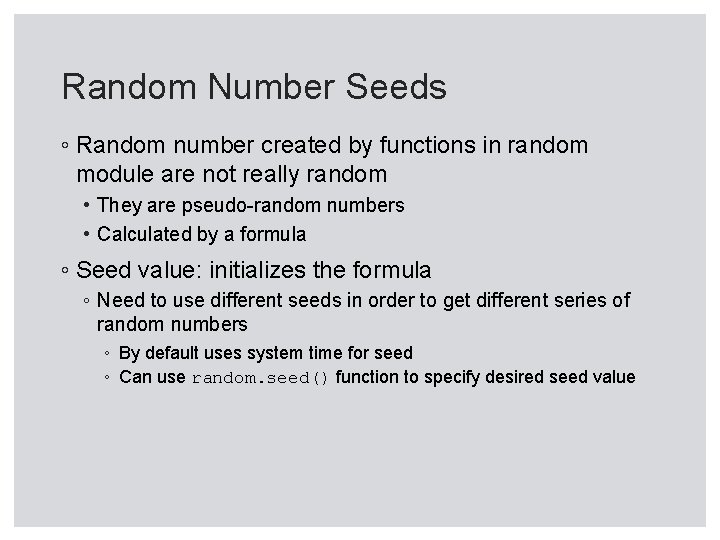
Random Number Seeds ◦ Random number created by functions in random module are not really random • They are pseudo-random numbers • Calculated by a formula ◦ Seed value: initializes the formula ◦ Need to use different seeds in order to get different series of random numbers ◦ By default uses system time for seed ◦ Can use random. seed() function to specify desired seed value
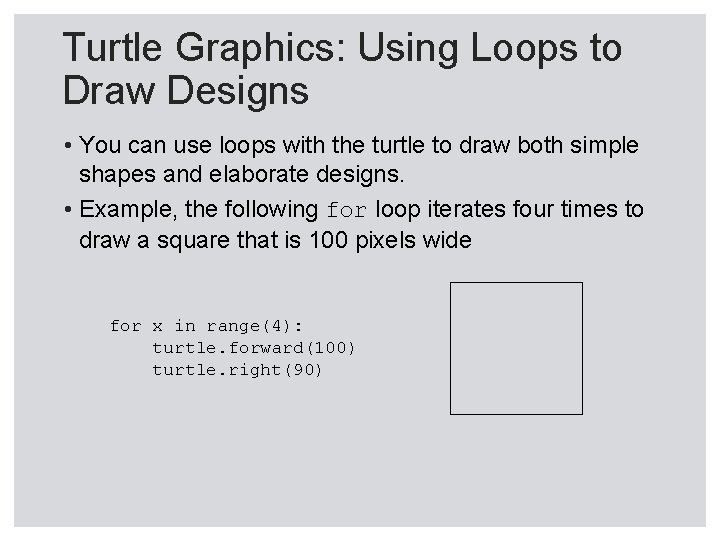
Turtle Graphics: Using Loops to Draw Designs • You can use loops with the turtle to draw both simple shapes and elaborate designs. • Example, the following for loop iterates four times to draw a square that is 100 pixels wide for x in range(4): turtle. forward(100) turtle. right(90)
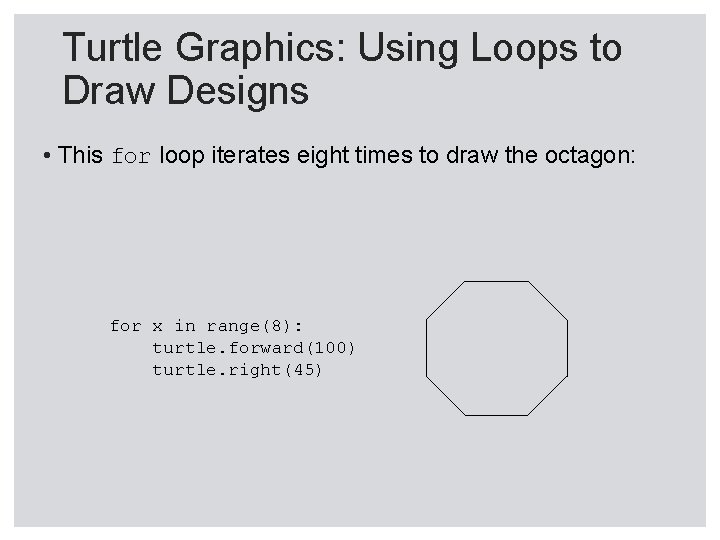
Turtle Graphics: Using Loops to Draw Designs • This for loop iterates eight times to draw the octagon: for x in range(8): turtle. forward(100) turtle. right(45)
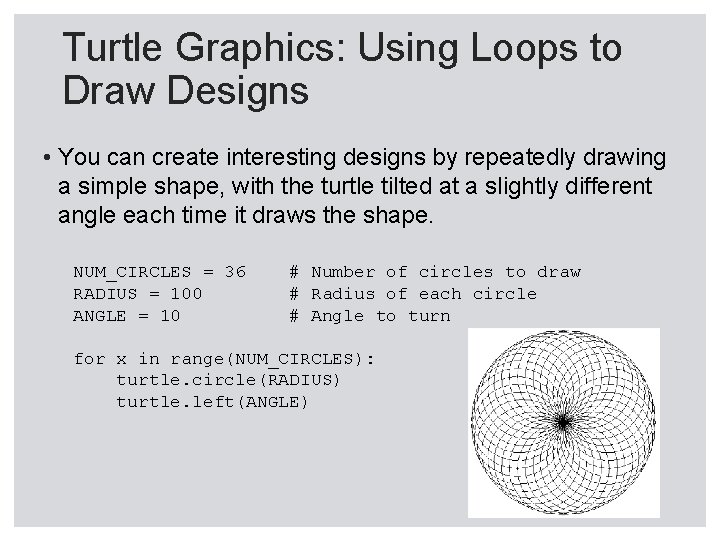
Turtle Graphics: Using Loops to Draw Designs • You can create interesting designs by repeatedly drawing a simple shape, with the turtle tilted at a slightly different angle each time it draws the shape. NUM_CIRCLES = 36 RADIUS = 100 ANGLE = 10 # Number of circles to draw # Radius of each circle # Angle to turn for x in range(NUM_CIRCLES): turtle. circle(RADIUS) turtle. left(ANGLE)
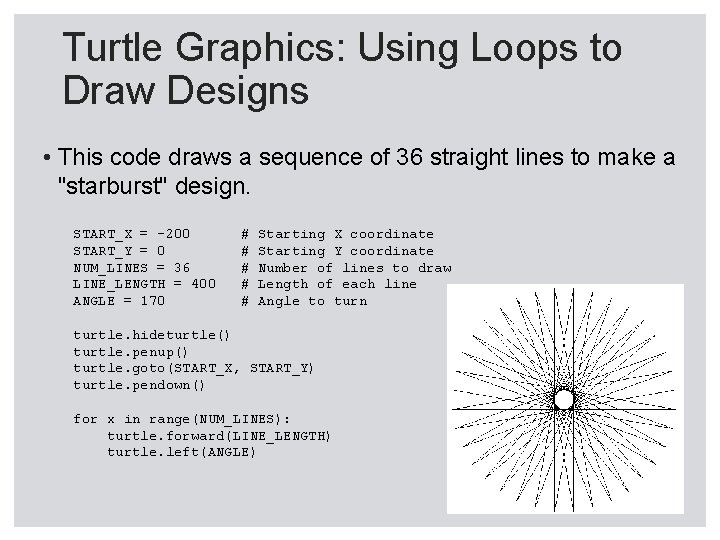
Turtle Graphics: Using Loops to Draw Designs • This code draws a sequence of 36 straight lines to make a "starburst" design. START_X = -200 START_Y = 0 NUM_LINES = 36 LINE_LENGTH = 400 ANGLE = 170 # # # Starting X coordinate Starting Y coordinate Number of lines to draw Length of each line Angle to turn turtle. hideturtle() turtle. penup() turtle. goto(START_X, START_Y) turtle. pendown() for x in range(NUM_LINES): turtle. forward(LINE_LENGTH) turtle. left(ANGLE)
Compound assignment operator overloading in c++ example
Examples of conditional operators
Perl operator precedence
Modern studies assignment topics
Difference between transfer and testimonial propaganda
Repetition structures
Human arm and whale flipper function
Augmented reality big data
Studio aurasma
Augmented product example
Core product augmented product
Augmented grammar
Augmented leads of ecg
The giver chapter 8 summary
Define augmented feedback
Virtual reality remote maintenance
Use case diagram augmented reality
Ciri ciri augmented reality
Augment matrix
Augmented grammar in ai
Augmented reality table
Microsoft augmented reality for students
Augmented vector left
Write augmented matrix for systems of equations calculator
Consider the augmented grammar given below
Task intrinsic feedback
Augmented reality: principles and practice
Multi user augmented reality