Operators in Python Arithmetic operators Some operators in
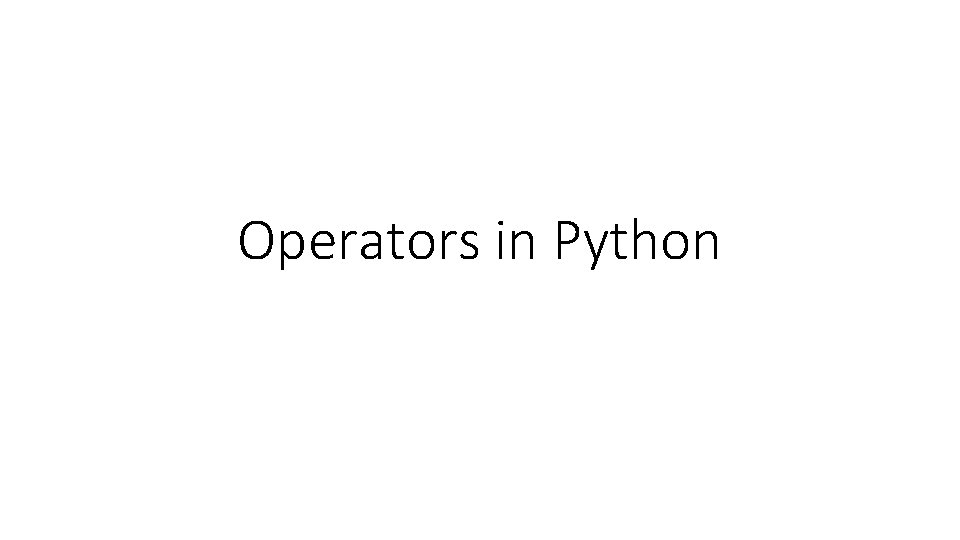
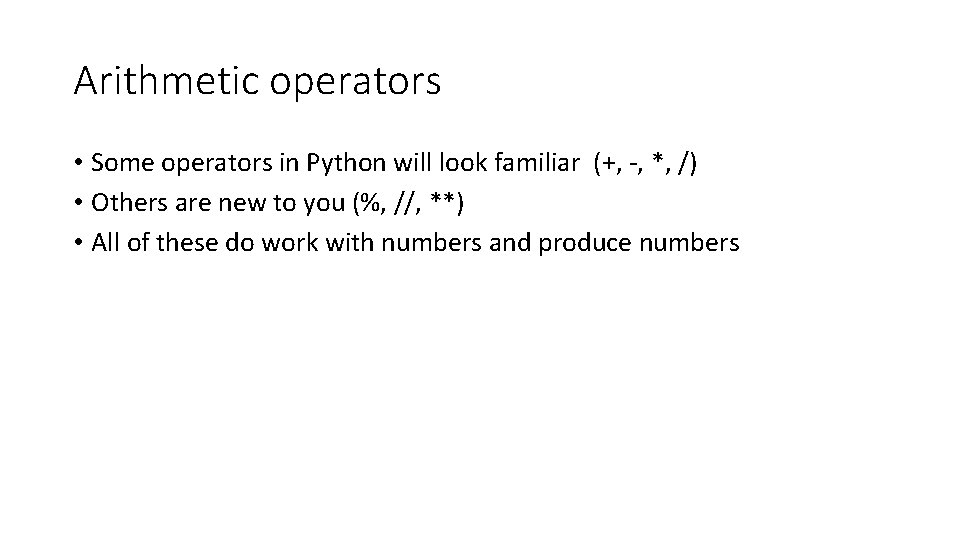
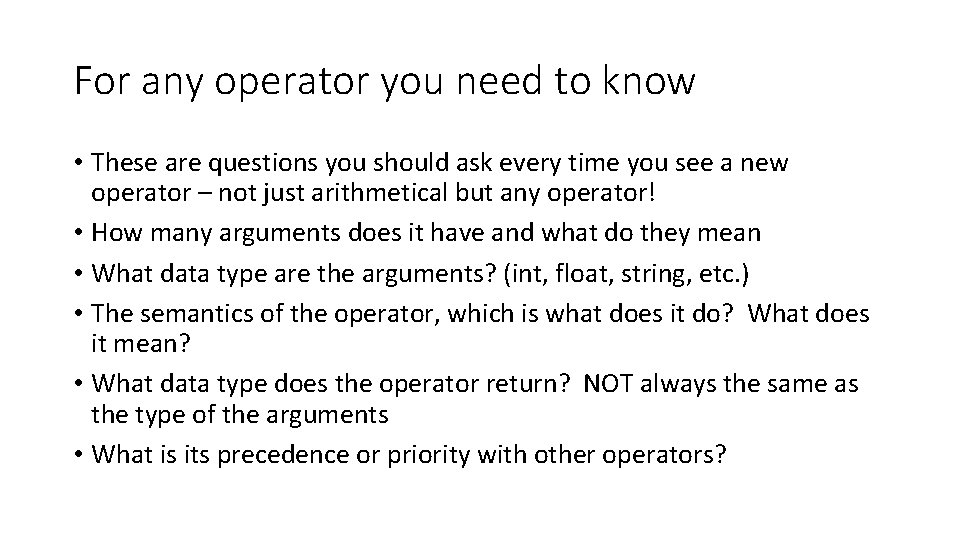
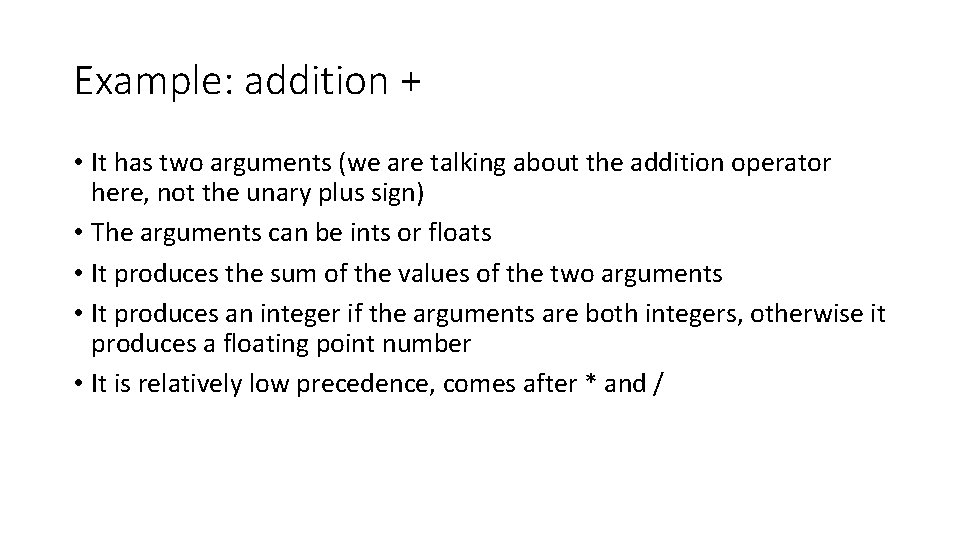
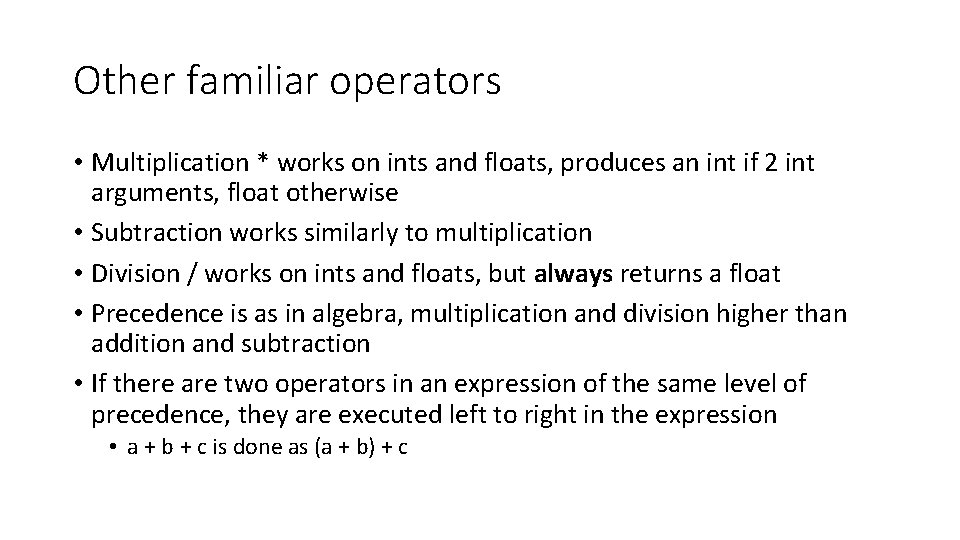
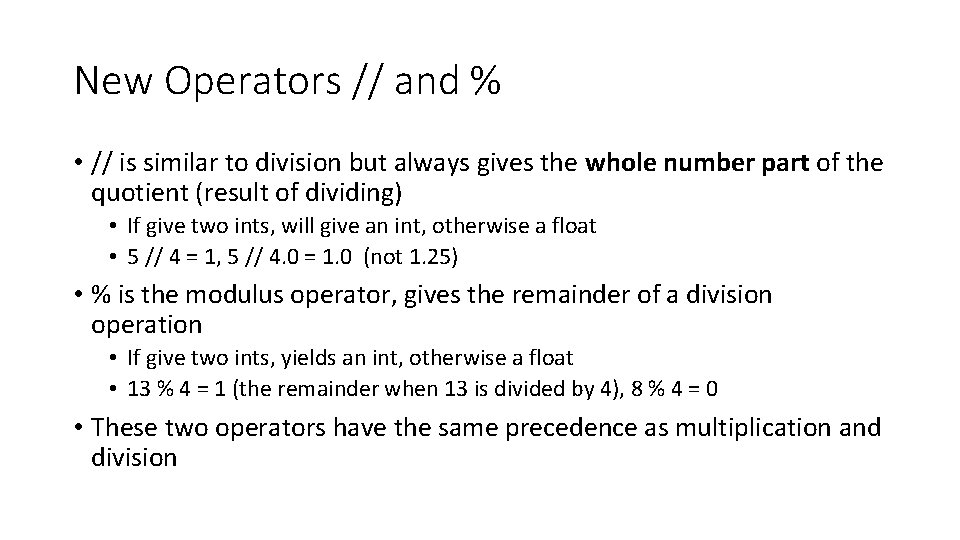
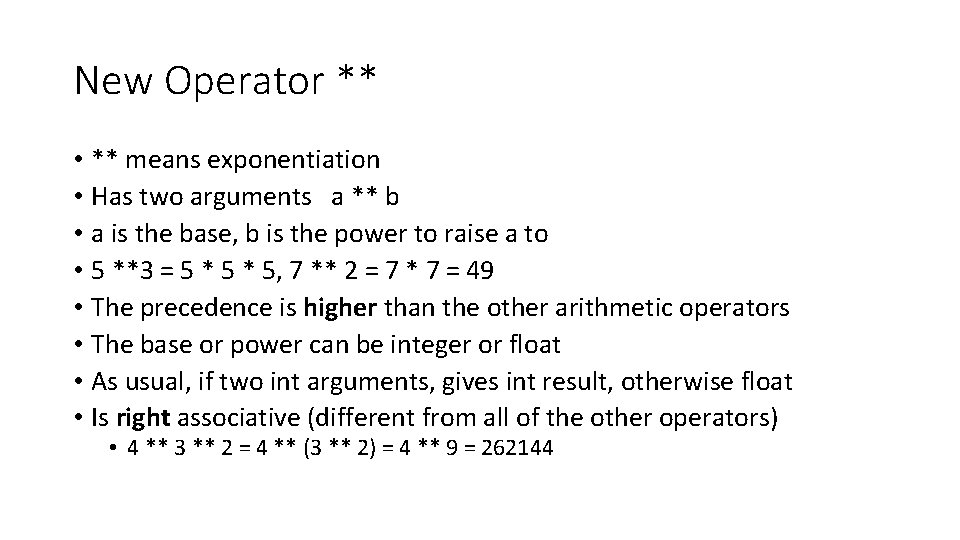
- Slides: 7
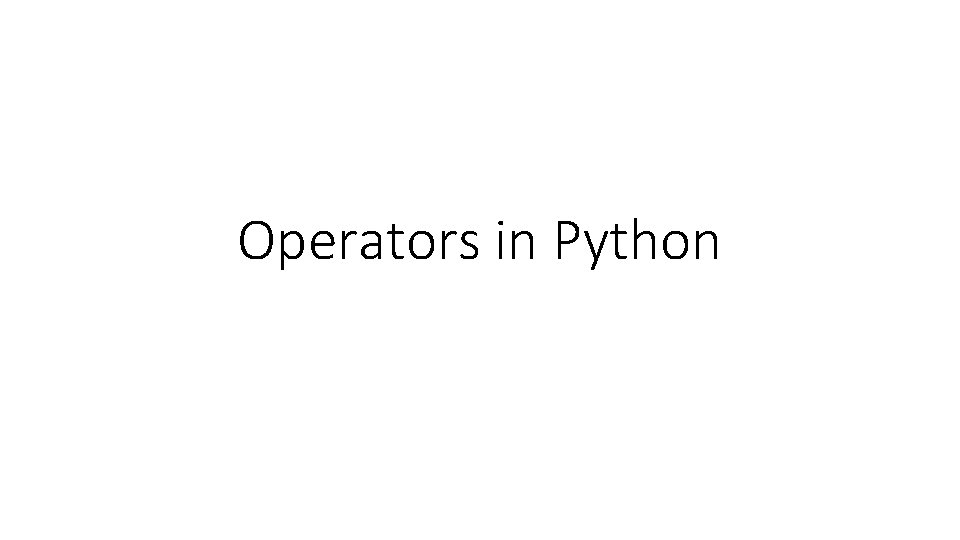
Operators in Python
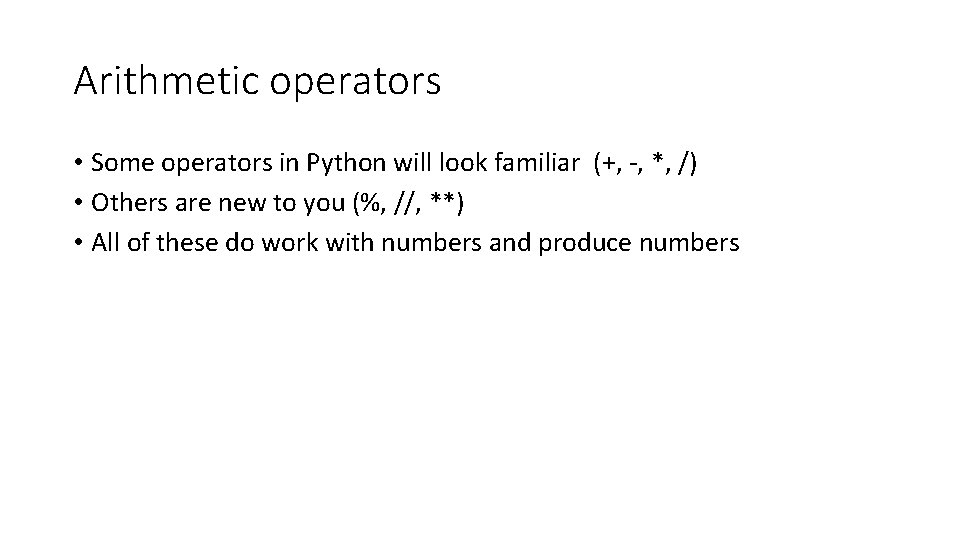
Arithmetic operators • Some operators in Python will look familiar (+, -, *, /) • Others are new to you (%, //, **) • All of these do work with numbers and produce numbers
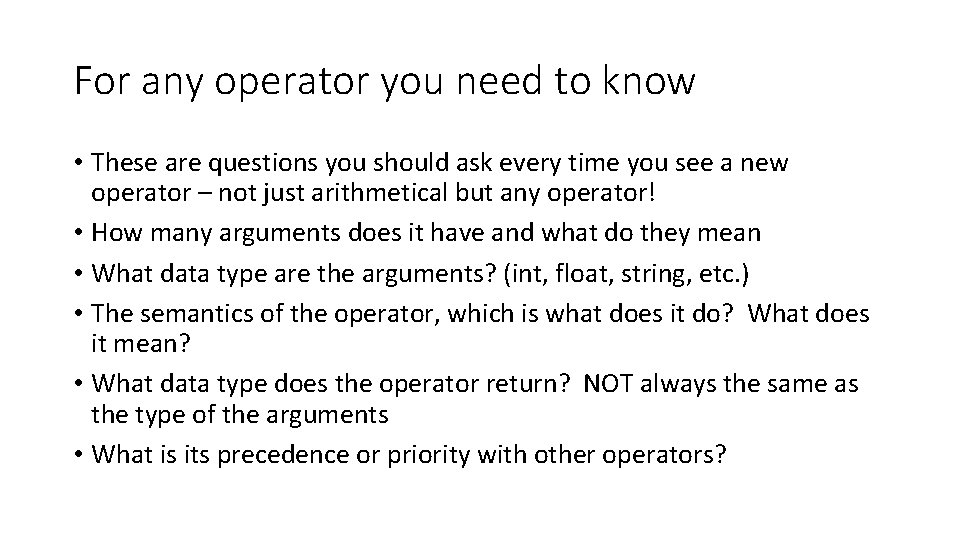
For any operator you need to know • These are questions you should ask every time you see a new operator – not just arithmetical but any operator! • How many arguments does it have and what do they mean • What data type are the arguments? (int, float, string, etc. ) • The semantics of the operator, which is what does it do? What does it mean? • What data type does the operator return? NOT always the same as the type of the arguments • What is its precedence or priority with other operators?
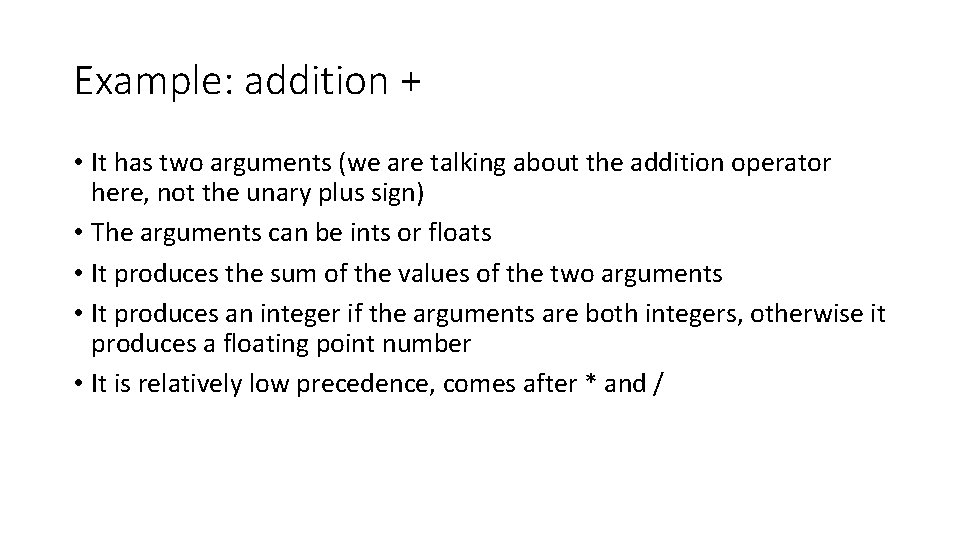
Example: addition + • It has two arguments (we are talking about the addition operator here, not the unary plus sign) • The arguments can be ints or floats • It produces the sum of the values of the two arguments • It produces an integer if the arguments are both integers, otherwise it produces a floating point number • It is relatively low precedence, comes after * and /
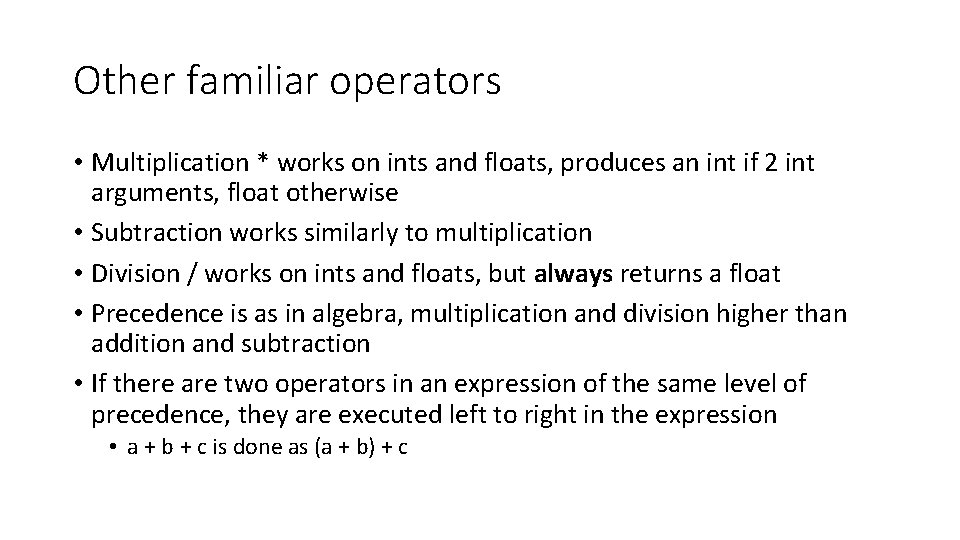
Other familiar operators • Multiplication * works on ints and floats, produces an int if 2 int arguments, float otherwise • Subtraction works similarly to multiplication • Division / works on ints and floats, but always returns a float • Precedence is as in algebra, multiplication and division higher than addition and subtraction • If there are two operators in an expression of the same level of precedence, they are executed left to right in the expression • a + b + c is done as (a + b) + c
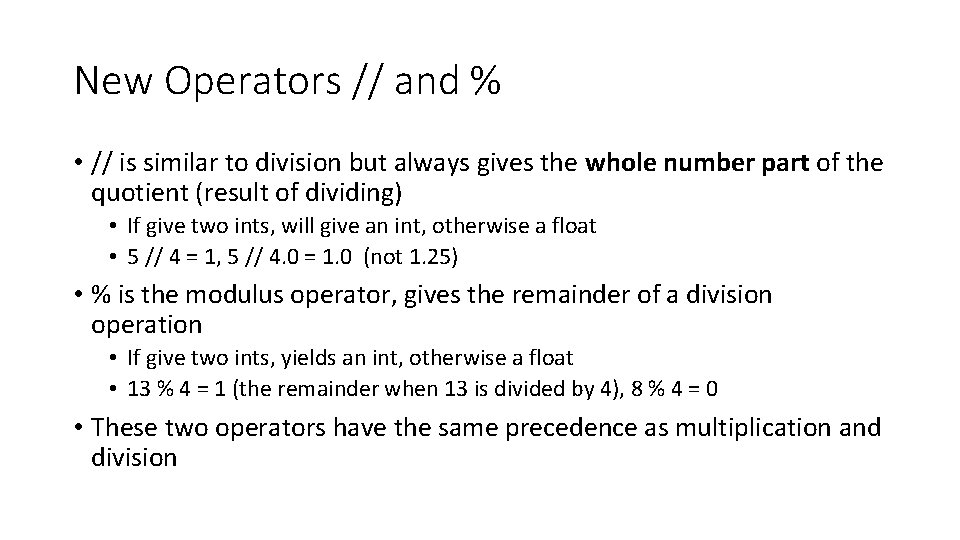
New Operators // and % • // is similar to division but always gives the whole number part of the quotient (result of dividing) • If give two ints, will give an int, otherwise a float • 5 // 4 = 1, 5 // 4. 0 = 1. 0 (not 1. 25) • % is the modulus operator, gives the remainder of a division operation • If give two ints, yields an int, otherwise a float • 13 % 4 = 1 (the remainder when 13 is divided by 4), 8 % 4 = 0 • These two operators have the same precedence as multiplication and division
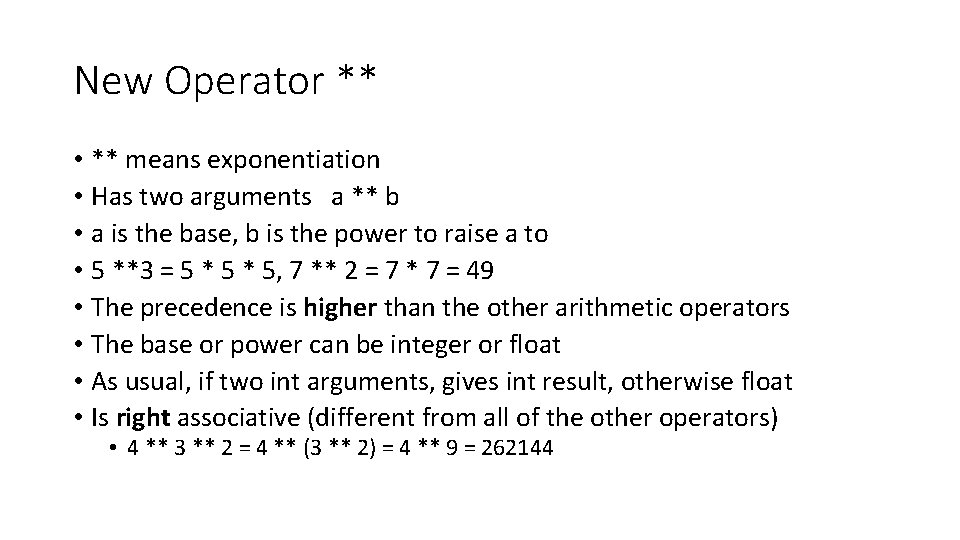
New Operator ** • ** means exponentiation • Has two arguments a ** b • a is the base, b is the power to raise a to • 5 **3 = 5 * 5, 7 ** 2 = 7 * 7 = 49 • The precedence is higher than the other arithmetic operators • The base or power can be integer or float • As usual, if two int arguments, gives int result, otherwise float • Is right associative (different from all of the other operators) • 4 ** 3 ** 2 = 4 ** (3 ** 2) = 4 ** 9 = 262144