Assignment Operators Topics Increment and Decrement Operators Assignment
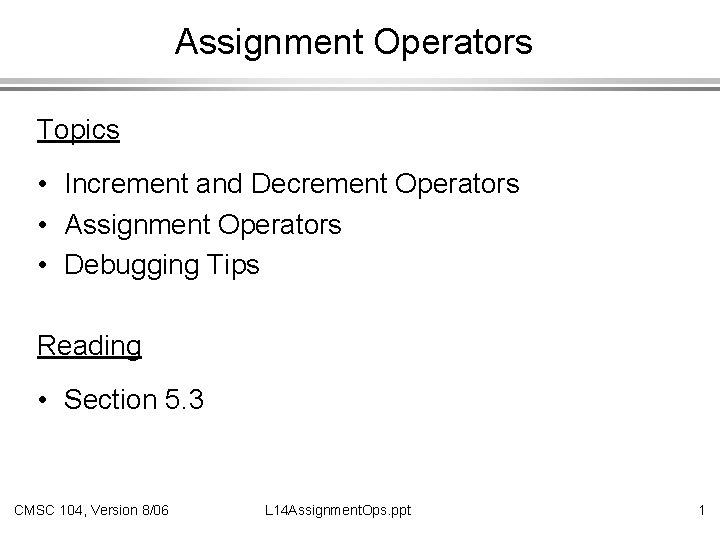
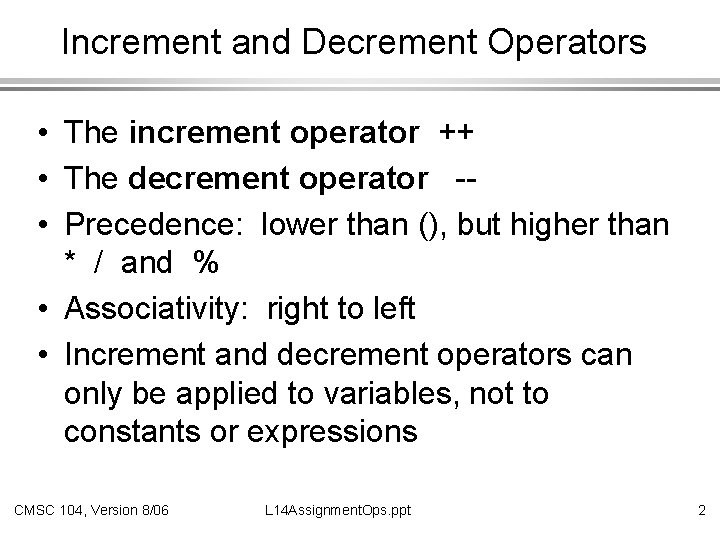
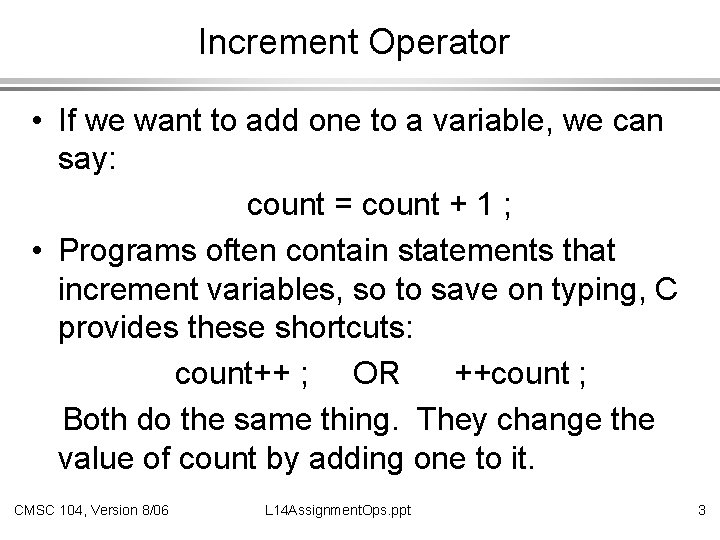
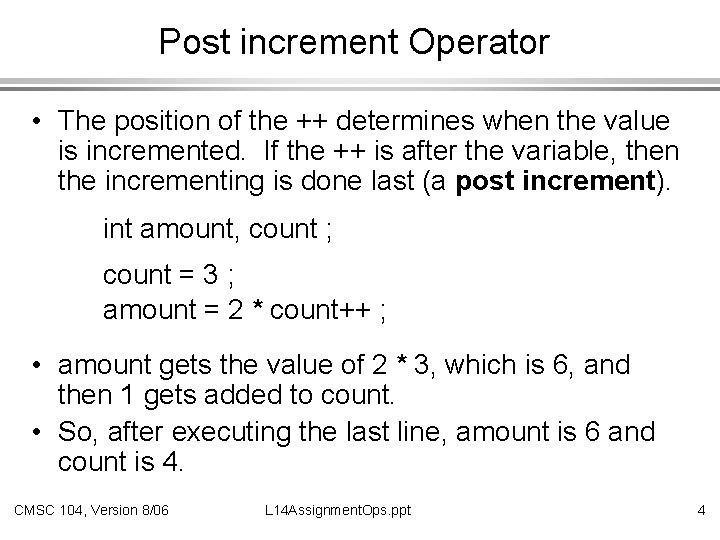
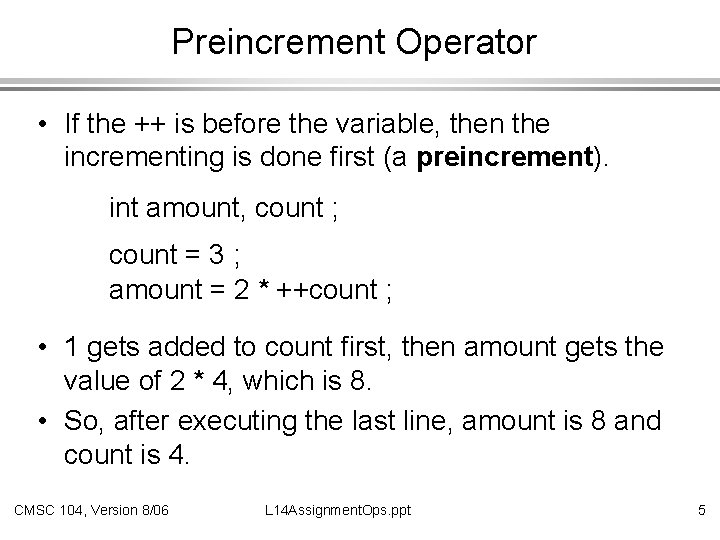
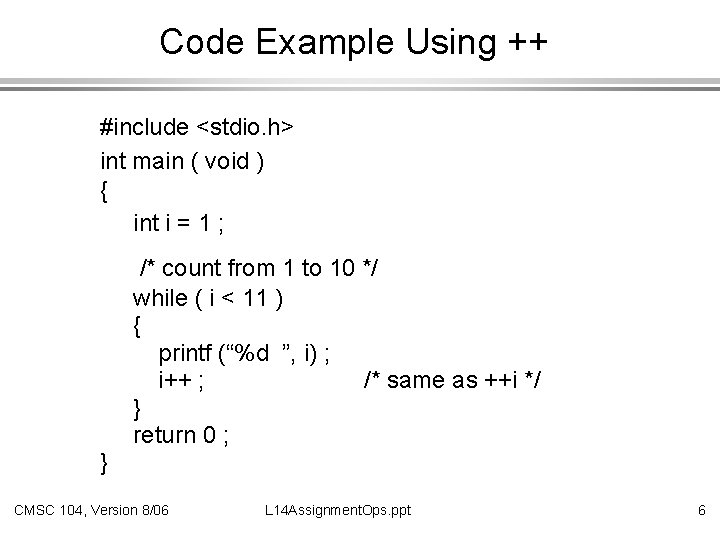
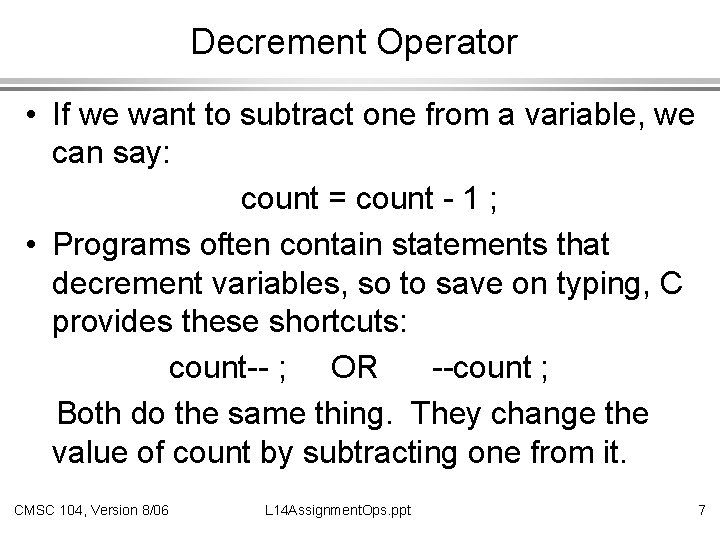
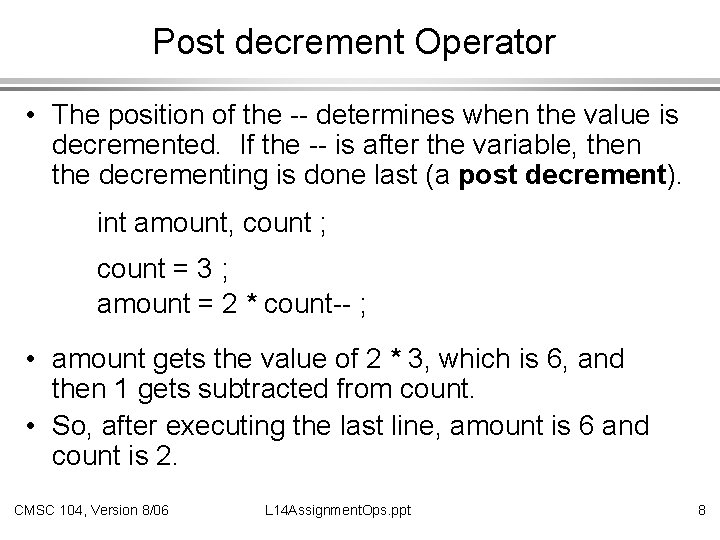
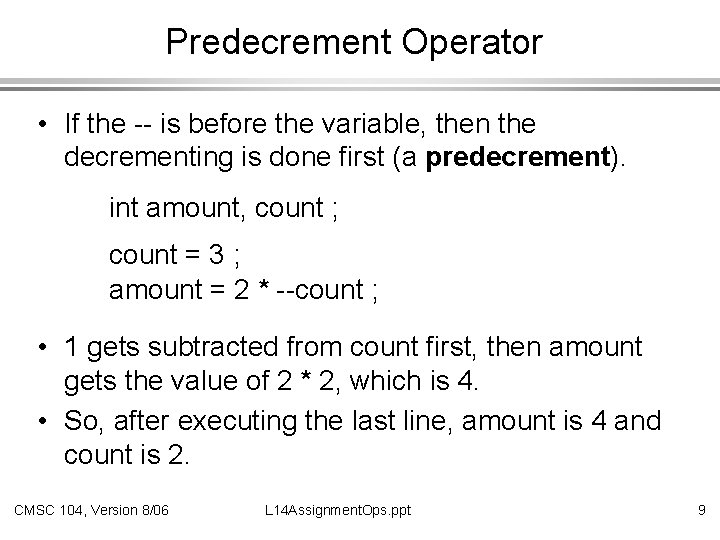
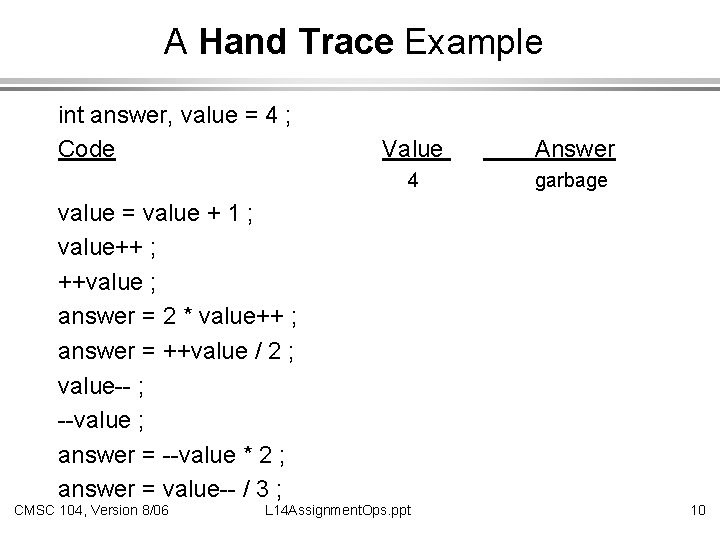
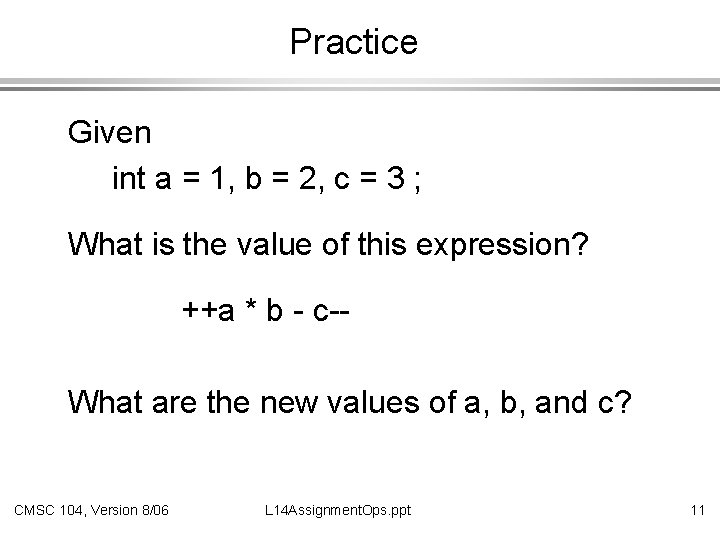
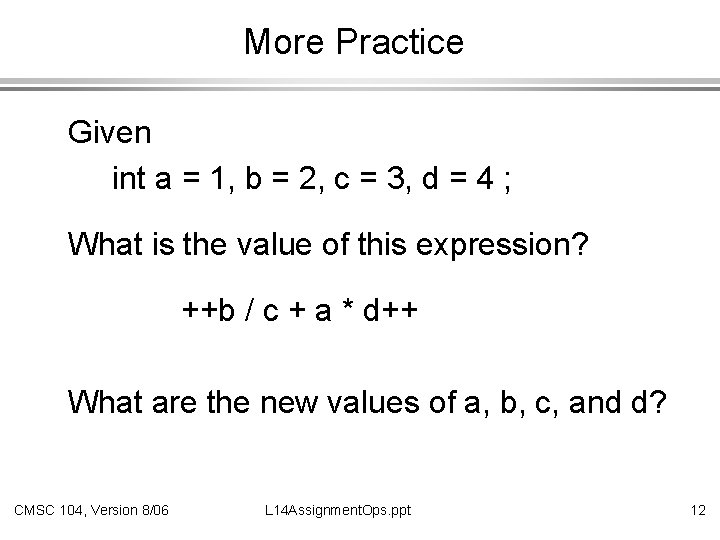
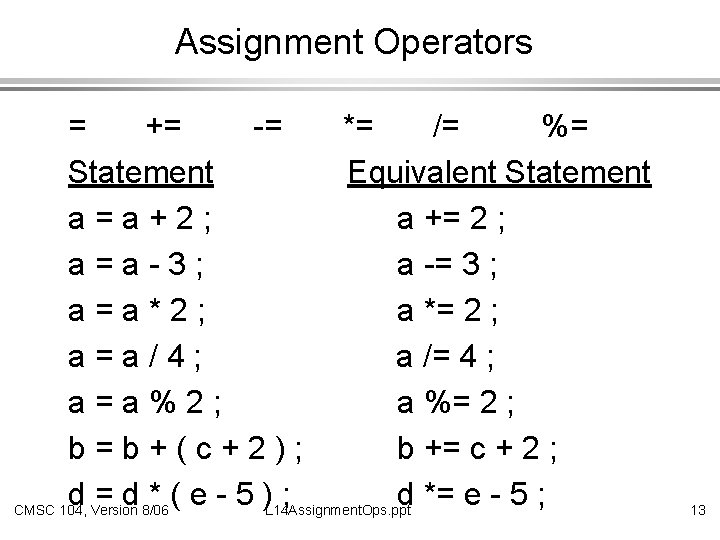
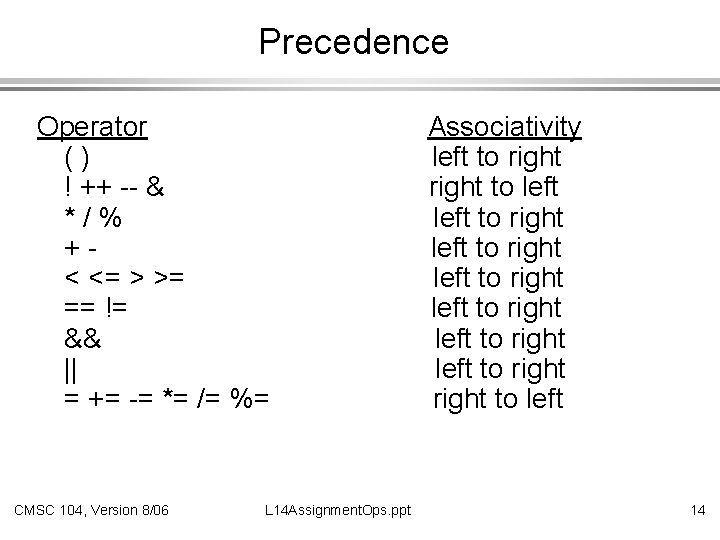
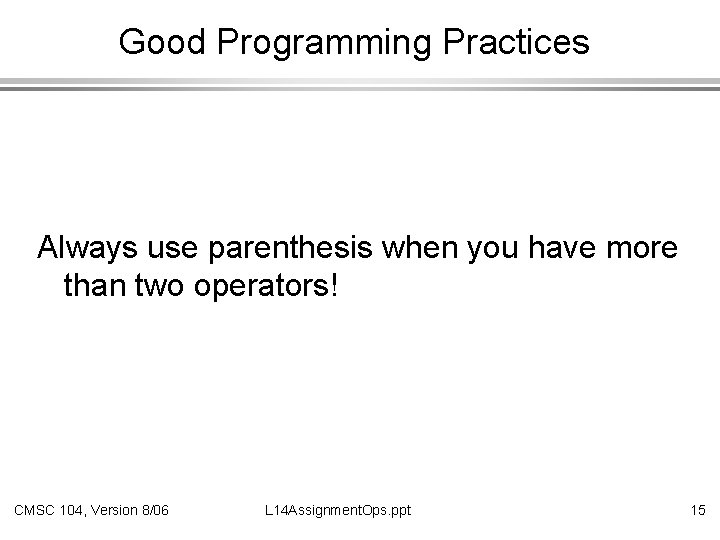
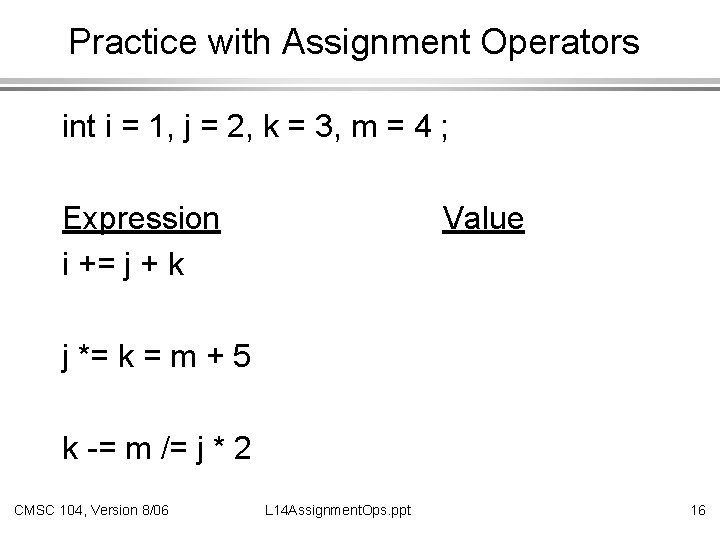
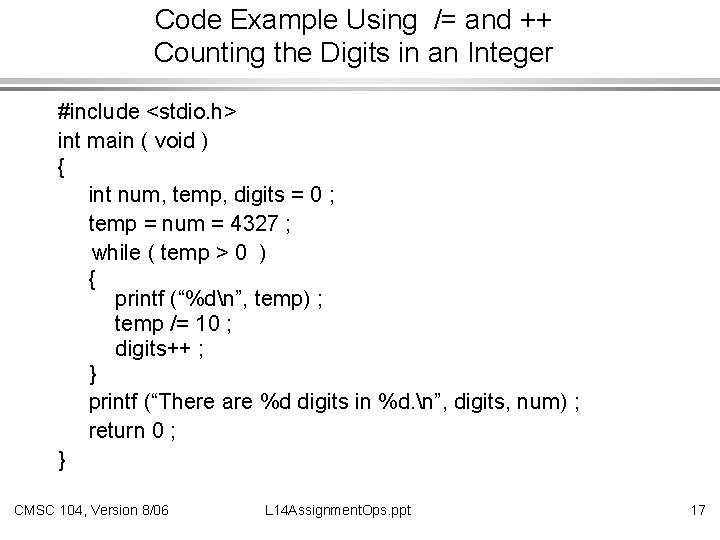
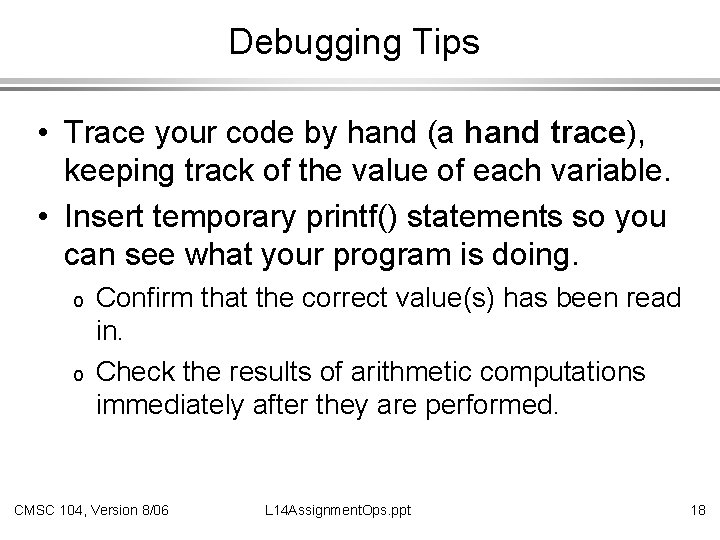
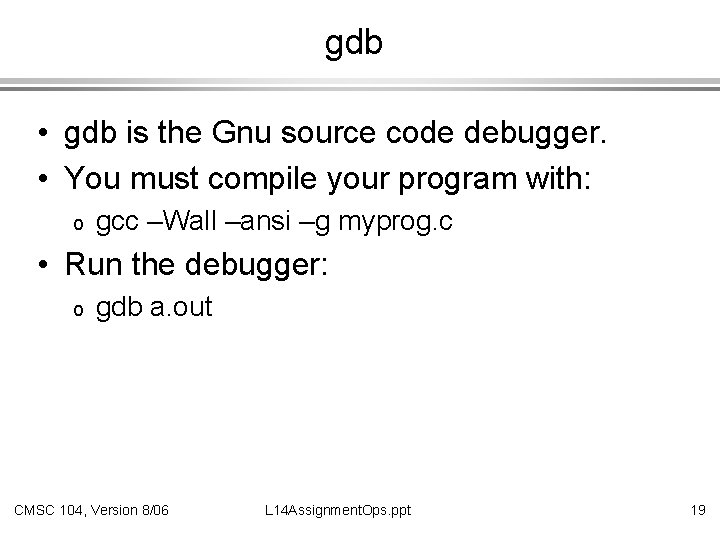
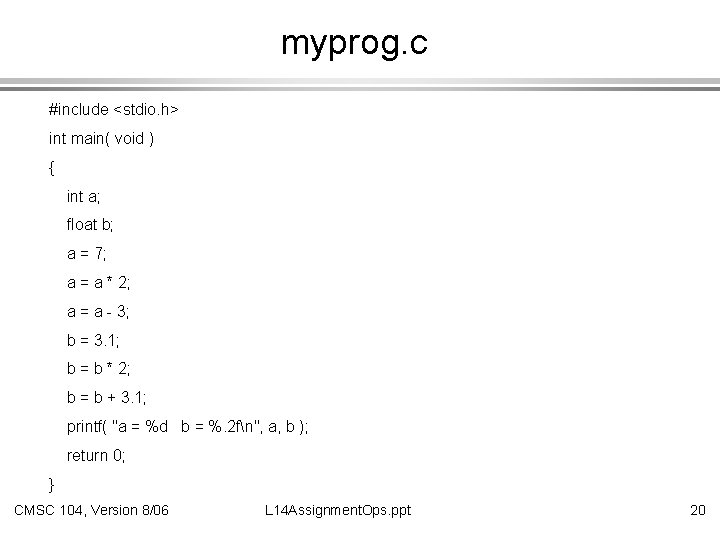
![gdb [burt@linux 2 ~/gdb]$ gcc -g -Wall -ansi myprog. c [burt@linux 2 ~/gdb]$ gdb gdb [burt@linux 2 ~/gdb]$ gcc -g -Wall -ansi myprog. c [burt@linux 2 ~/gdb]$ gdb](https://slidetodoc.com/presentation_image/b87a08f7a4fc2ab81fde8474de3871c4/image-21.jpg)
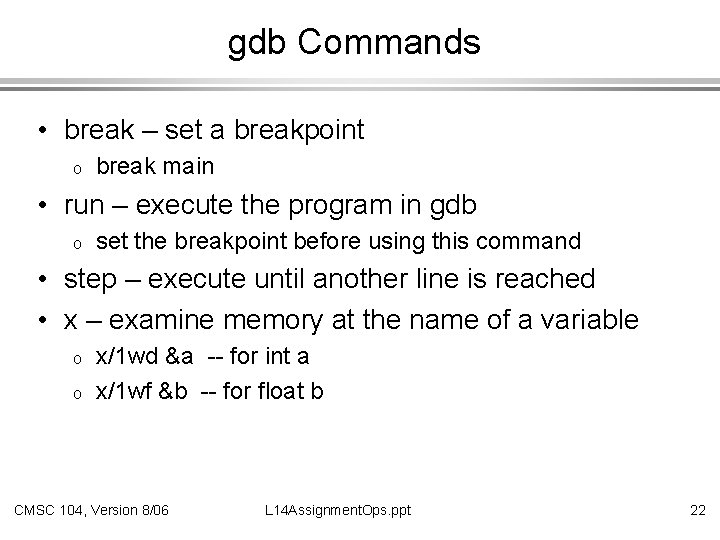
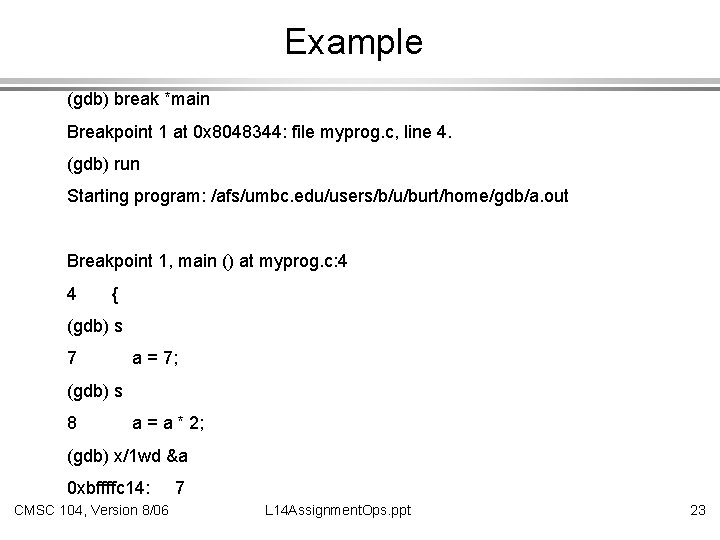
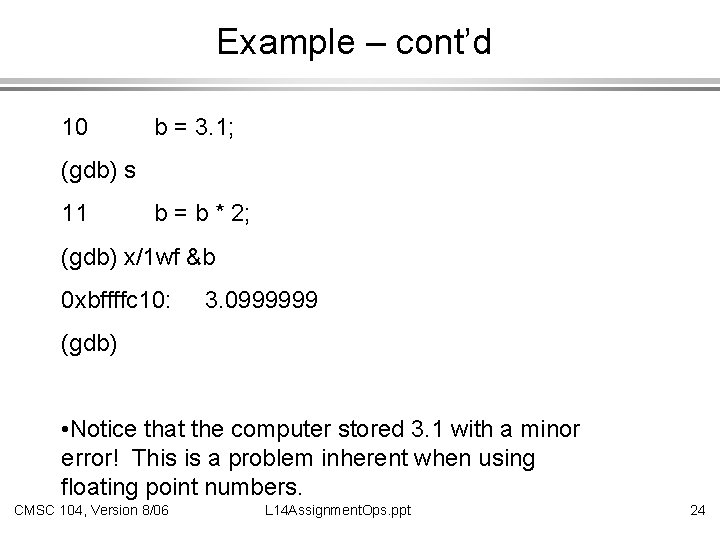
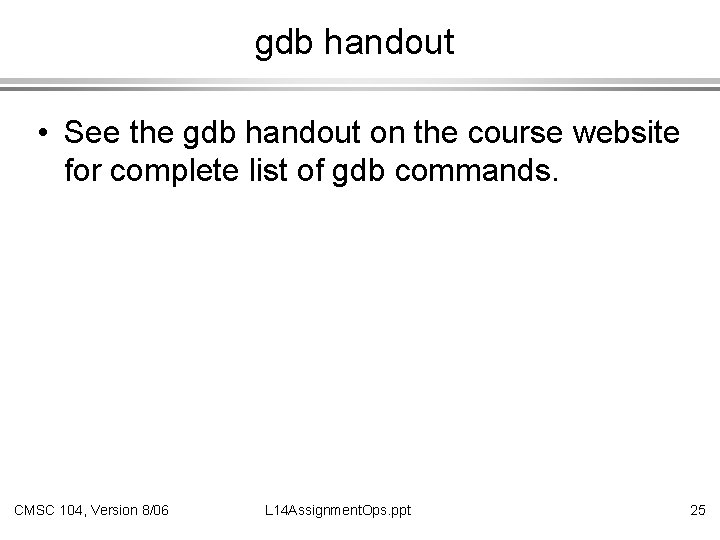
- Slides: 25
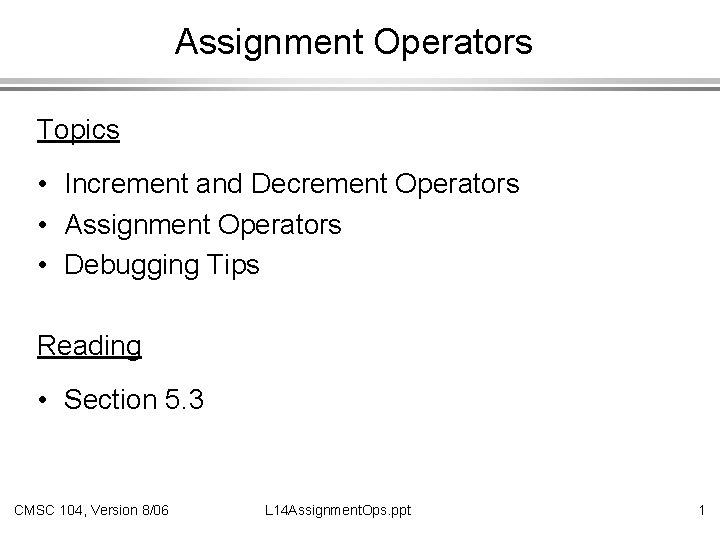
Assignment Operators Topics • Increment and Decrement Operators • Assignment Operators • Debugging Tips Reading • Section 5. 3 CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 1
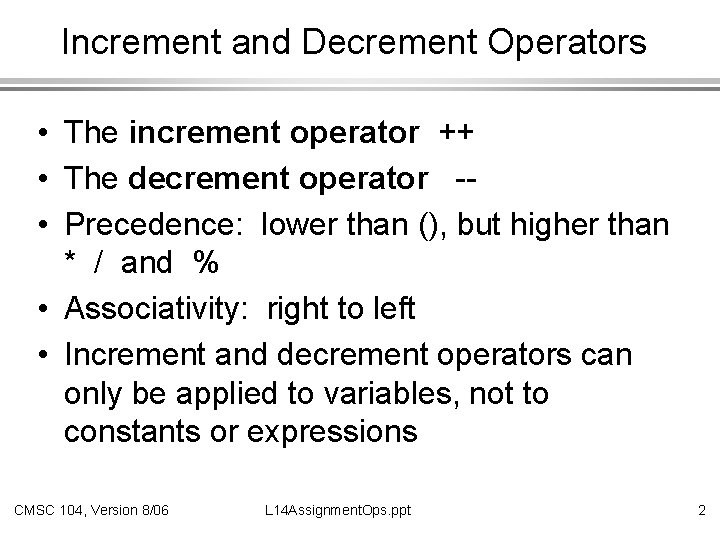
Increment and Decrement Operators • The increment operator ++ • The decrement operator - • Precedence: lower than (), but higher than * / and % • Associativity: right to left • Increment and decrement operators can only be applied to variables, not to constants or expressions CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 2
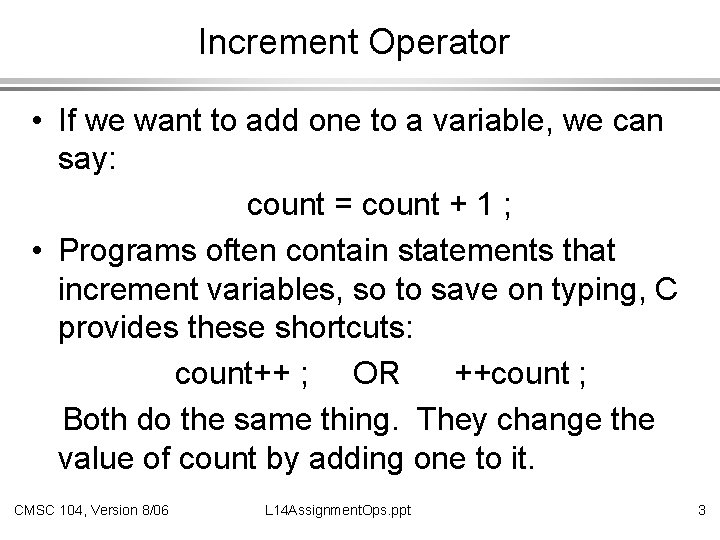
Increment Operator • If we want to add one to a variable, we can say: count = count + 1 ; • Programs often contain statements that increment variables, so to save on typing, C provides these shortcuts: count++ ; OR ++count ; Both do the same thing. They change the value of count by adding one to it. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 3
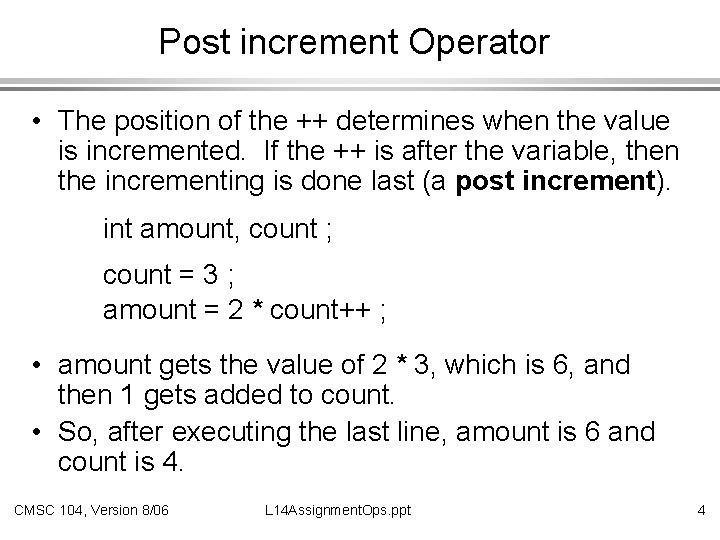
Post increment Operator • The position of the ++ determines when the value is incremented. If the ++ is after the variable, then the incrementing is done last (a post increment). int amount, count ; count = 3 ; amount = 2 * count++ ; • amount gets the value of 2 * 3, which is 6, and then 1 gets added to count. • So, after executing the last line, amount is 6 and count is 4. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 4
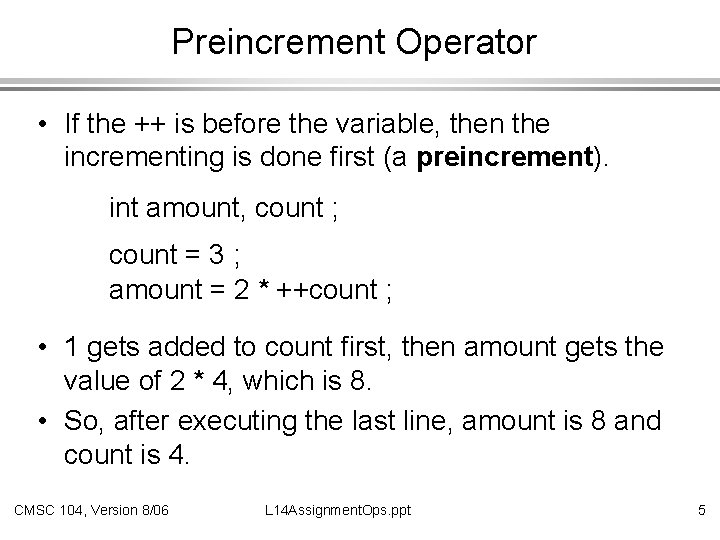
Preincrement Operator • If the ++ is before the variable, then the incrementing is done first (a preincrement). int amount, count ; count = 3 ; amount = 2 * ++count ; • 1 gets added to count first, then amount gets the value of 2 * 4, which is 8. • So, after executing the last line, amount is 8 and count is 4. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 5
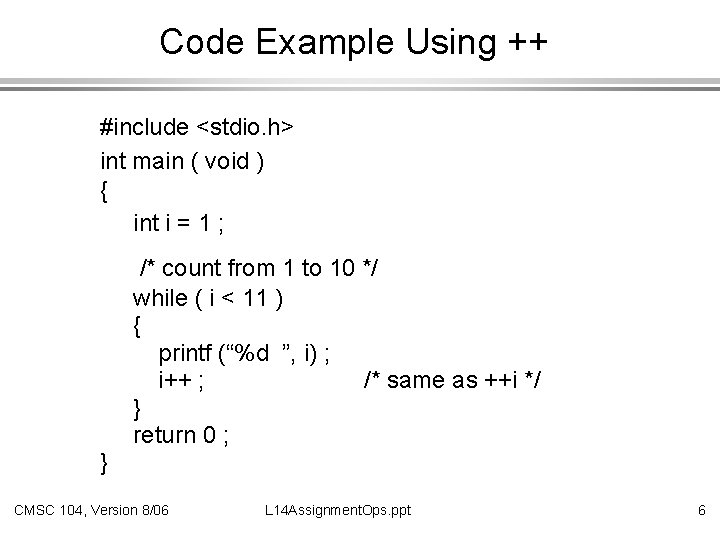
Code Example Using ++ #include <stdio. h> int main ( void ) { int i = 1 ; /* count from 1 to 10 */ while ( i < 11 ) { printf (“%d ”, i) ; i++ ; /* same as ++i */ } return 0 ; } CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 6
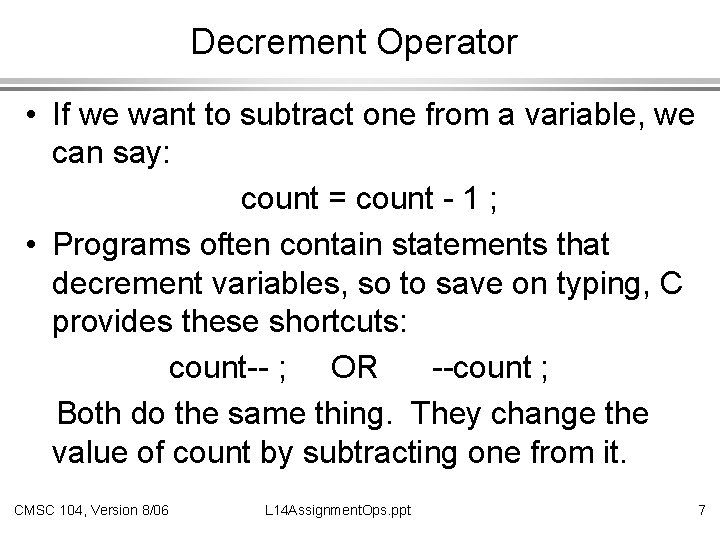
Decrement Operator • If we want to subtract one from a variable, we can say: count = count - 1 ; • Programs often contain statements that decrement variables, so to save on typing, C provides these shortcuts: count-- ; OR --count ; Both do the same thing. They change the value of count by subtracting one from it. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 7
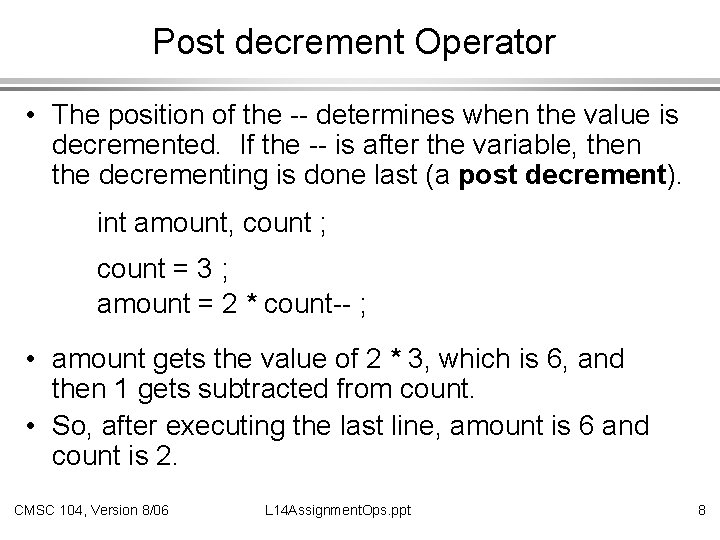
Post decrement Operator • The position of the -- determines when the value is decremented. If the -- is after the variable, then the decrementing is done last (a post decrement). int amount, count ; count = 3 ; amount = 2 * count-- ; • amount gets the value of 2 * 3, which is 6, and then 1 gets subtracted from count. • So, after executing the last line, amount is 6 and count is 2. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 8
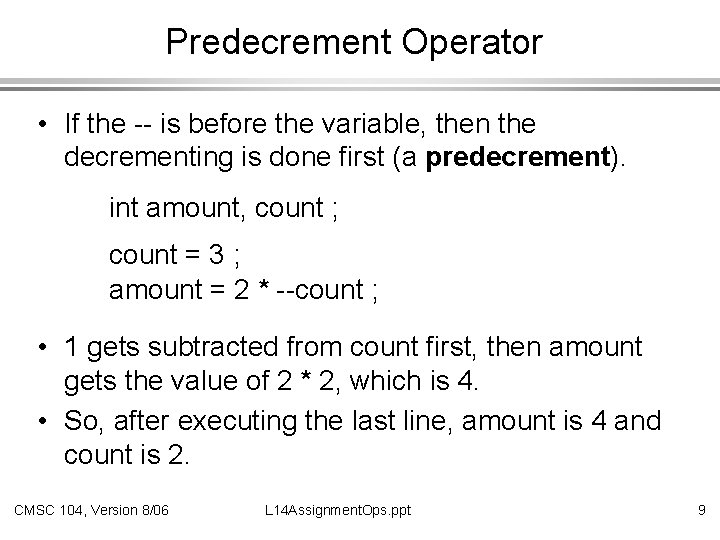
Predecrement Operator • If the -- is before the variable, then the decrementing is done first (a predecrement). int amount, count ; count = 3 ; amount = 2 * --count ; • 1 gets subtracted from count first, then amount gets the value of 2 * 2, which is 4. • So, after executing the last line, amount is 4 and count is 2. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 9
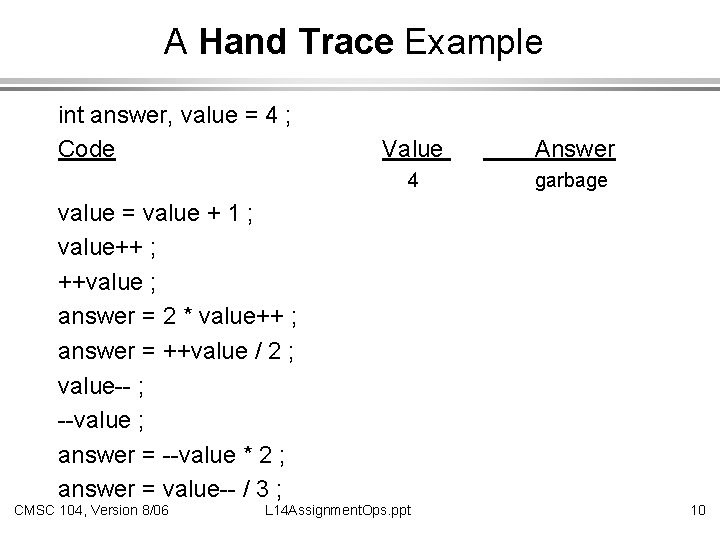
A Hand Trace Example int answer, value = 4 ; Code Value Answer 4 garbage value = value + 1 ; value++ ; ++value ; answer = 2 * value++ ; answer = ++value / 2 ; value-- ; --value ; answer = --value * 2 ; answer = value-- / 3 ; CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 10
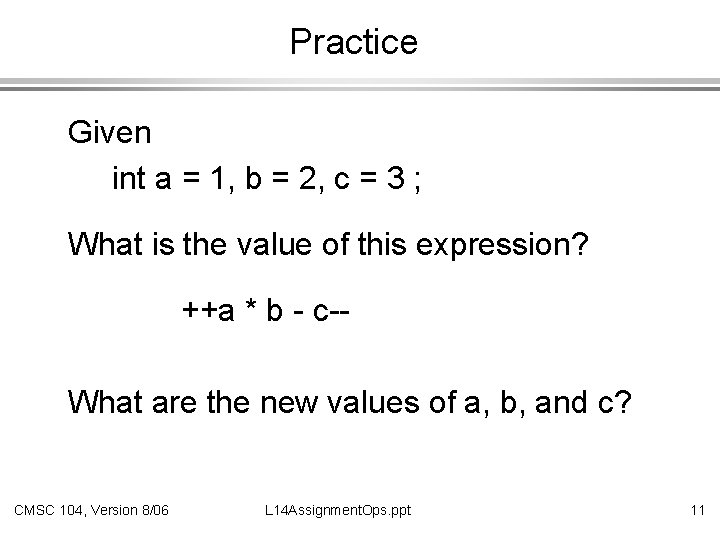
Practice Given int a = 1, b = 2, c = 3 ; What is the value of this expression? ++a * b - c-What are the new values of a, b, and c? CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 11
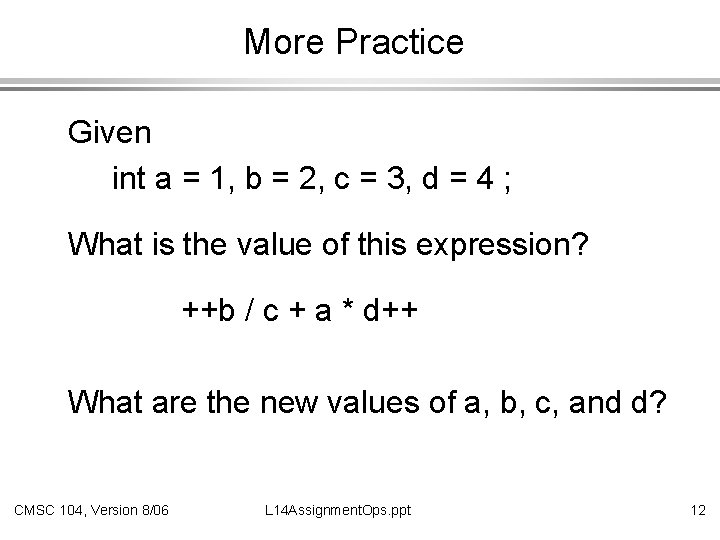
More Practice Given int a = 1, b = 2, c = 3, d = 4 ; What is the value of this expression? ++b / c + a * d++ What are the new values of a, b, c, and d? CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 12
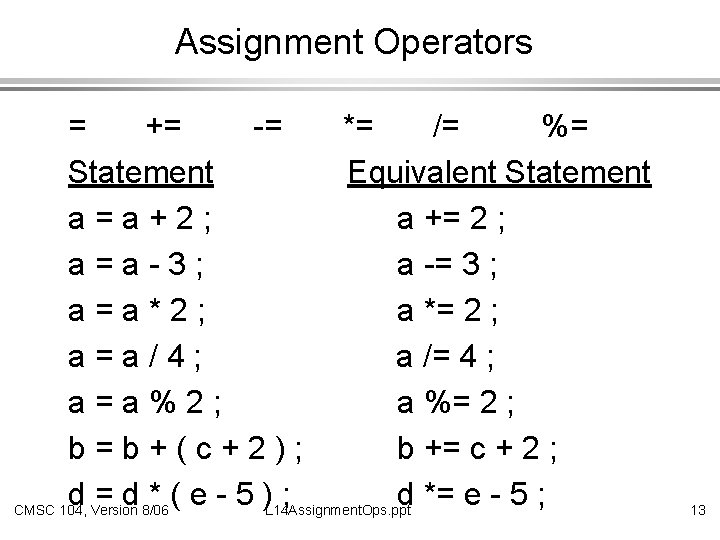
Assignment Operators = += -= *= /= %= Statement Equivalent Statement a=a+2; a += 2 ; a=a-3; a -= 3 ; a=a*2; a *= 2 ; a=a/4; a /= 4 ; a=a%2; a %= 2 ; b=b+(c+2); b += c + 2 ; d = d * ( e - 5 )L 14 Assignment. Ops. ppt ; d *= e - 5 ; CMSC 104, Version 8/06 13
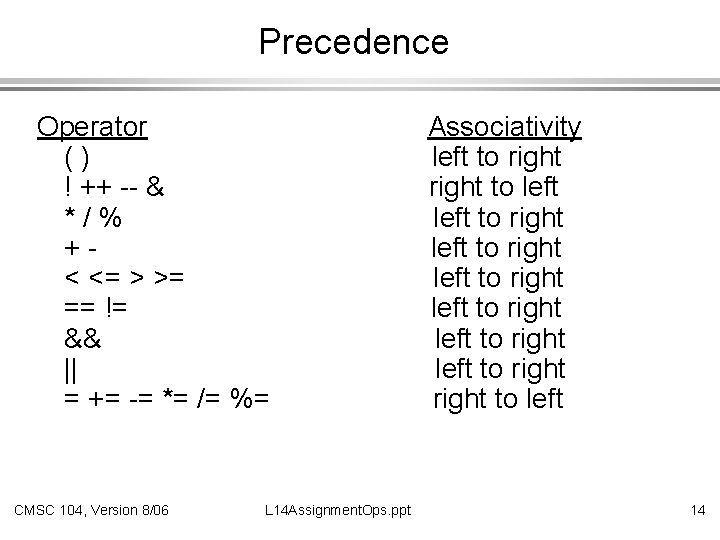
Precedence Operator () ! ++ -- & */% +< <= > >= == != && || = += -= *= /= %= CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt Associativity left to right to left to right left to right to left 14
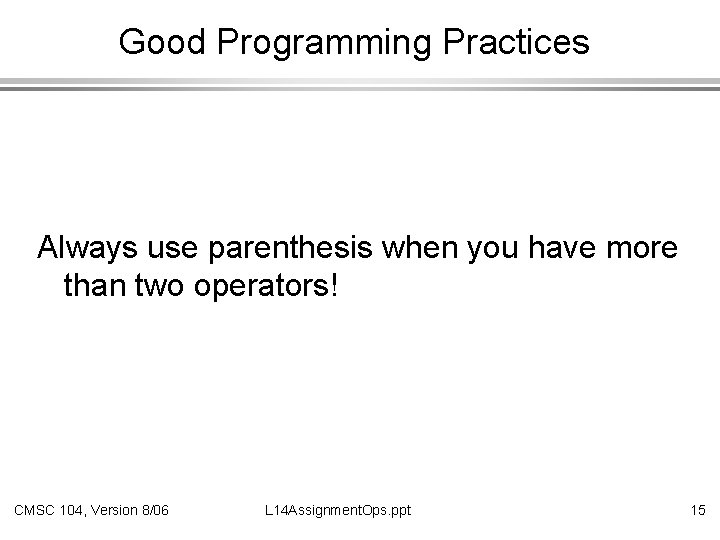
Good Programming Practices Always use parenthesis when you have more than two operators! CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 15
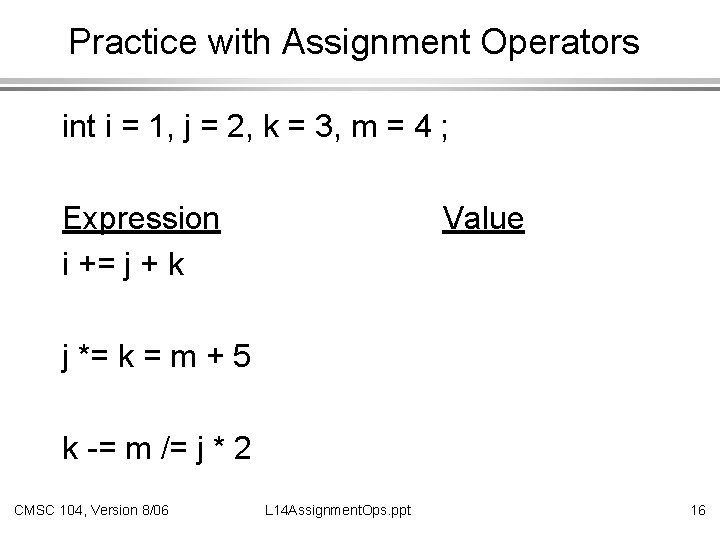
Practice with Assignment Operators int i = 1, j = 2, k = 3, m = 4 ; Expression i += j + k Value j *= k = m + 5 k -= m /= j * 2 CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 16
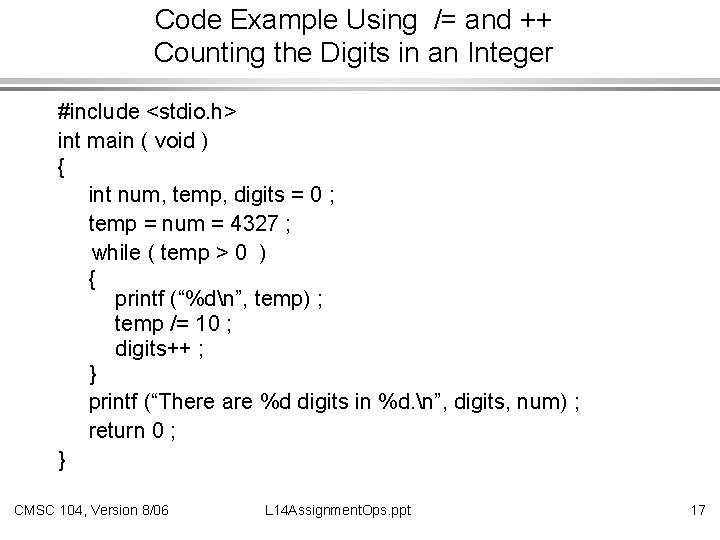
Code Example Using /= and ++ Counting the Digits in an Integer #include <stdio. h> int main ( void ) { int num, temp, digits = 0 ; temp = num = 4327 ; while ( temp > 0 ) { printf (“%dn”, temp) ; temp /= 10 ; digits++ ; } printf (“There are %d digits in %d. n”, digits, num) ; return 0 ; } CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 17
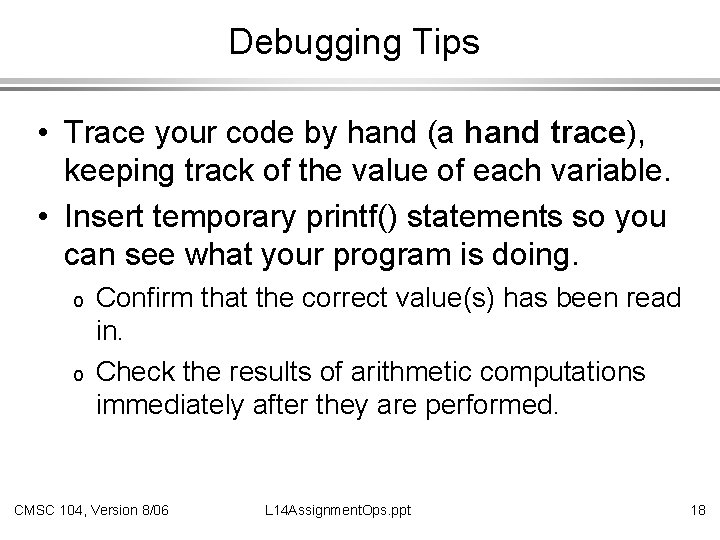
Debugging Tips • Trace your code by hand (a hand trace), keeping track of the value of each variable. • Insert temporary printf() statements so you can see what your program is doing. o o Confirm that the correct value(s) has been read in. Check the results of arithmetic computations immediately after they are performed. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 18
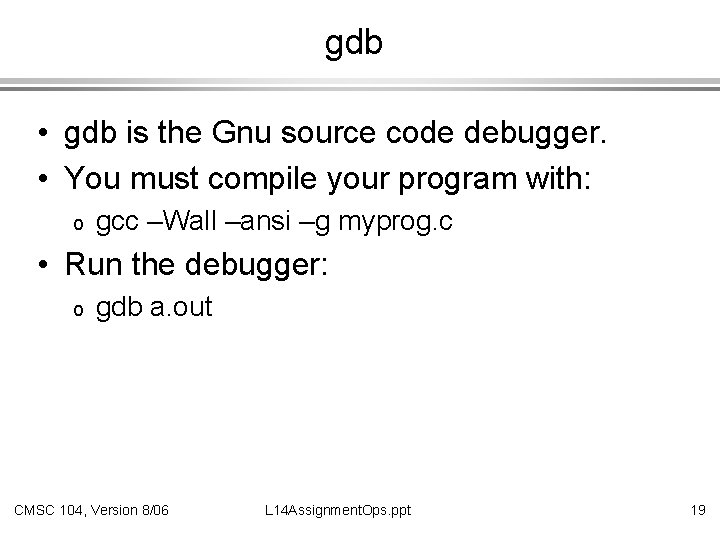
gdb • gdb is the Gnu source code debugger. • You must compile your program with: o gcc –Wall –ansi –g myprog. c • Run the debugger: o gdb a. out CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 19
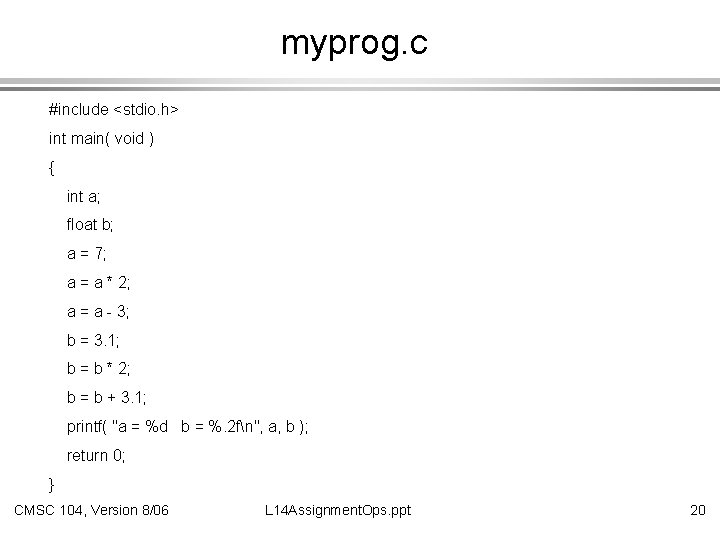
myprog. c #include <stdio. h> int main( void ) { int a; float b; a = 7; a = a * 2; a = a - 3; b = 3. 1; b = b * 2; b = b + 3. 1; printf( "a = %d b = %. 2 fn", a, b ); return 0; } CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 20
![gdb burtlinux 2 gdb gcc g Wall ansi myprog c burtlinux 2 gdb gdb gdb [burt@linux 2 ~/gdb]$ gcc -g -Wall -ansi myprog. c [burt@linux 2 ~/gdb]$ gdb](https://slidetodoc.com/presentation_image/b87a08f7a4fc2ab81fde8474de3871c4/image-21.jpg)
gdb [burt@linux 2 ~/gdb]$ gcc -g -Wall -ansi myprog. c [burt@linux 2 ~/gdb]$ gdb a. out GNU gdb Red Hat Linux (6. 1 post-1. 20040607. 52 rh) Copyright 2004 Free Software Foundation, Inc. GDB is free software, covered by the GNU General Public License, and you are welcome to change it and/or distribute copies of it under certain conditions. Type "show copying" to see the conditions. There is absolutely no warranty for GDB. Type "show warranty" for details. This GDB was configured as "i 386 -redhat-linux-gnu". . . Using host libthread_db library "/libthread_db. so. 1". (gdb) CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 21
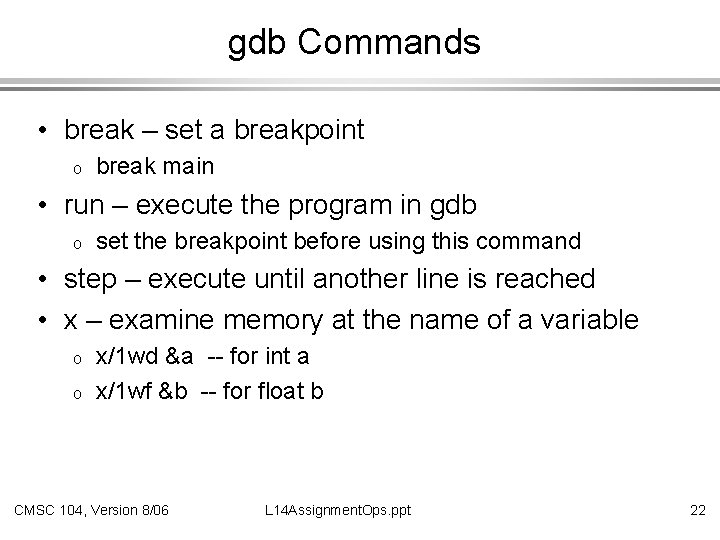
gdb Commands • break – set a breakpoint o break main • run – execute the program in gdb o set the breakpoint before using this command • step – execute until another line is reached • x – examine memory at the name of a variable o o x/1 wd &a -- for int a x/1 wf &b -- for float b CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 22
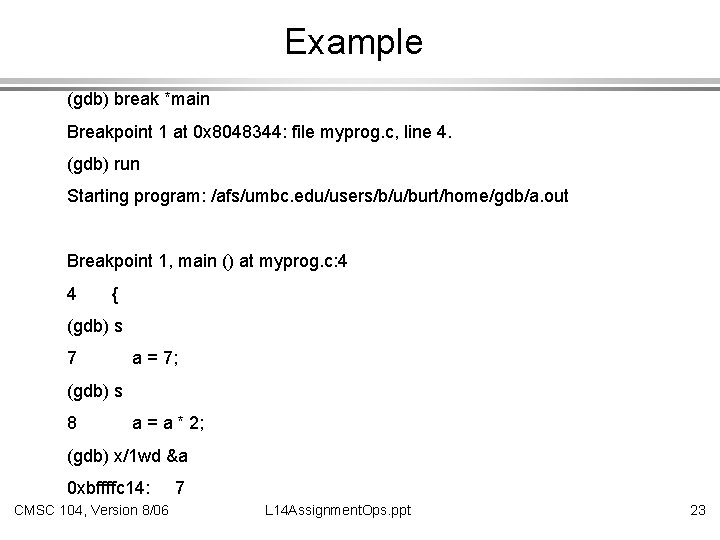
Example (gdb) break *main Breakpoint 1 at 0 x 8048344: file myprog. c, line 4. (gdb) run Starting program: /afs/umbc. edu/users/b/u/burt/home/gdb/a. out Breakpoint 1, main () at myprog. c: 4 4 { (gdb) s 7 a = 7; (gdb) s 8 a = a * 2; (gdb) x/1 wd &a 0 xbffffc 14: CMSC 104, Version 8/06 7 L 14 Assignment. Ops. ppt 23
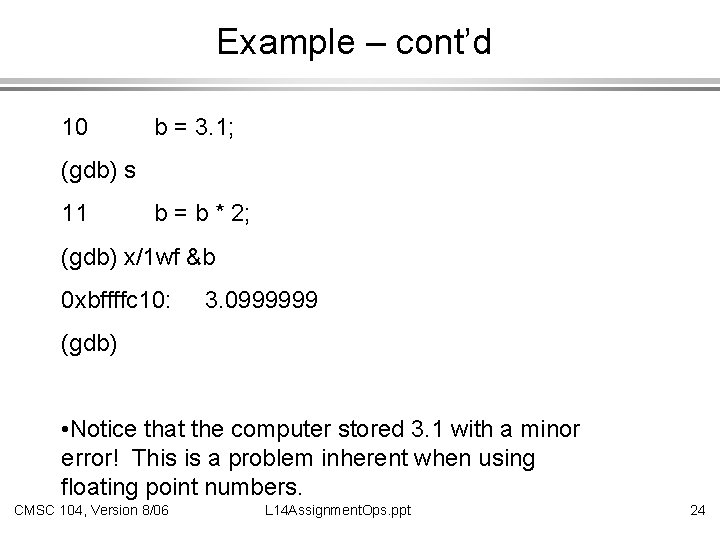
Example – cont’d 10 b = 3. 1; (gdb) s 11 b = b * 2; (gdb) x/1 wf &b 0 xbffffc 10: 3. 0999999 (gdb) • Notice that the computer stored 3. 1 with a minor error! This is a problem inherent when using floating point numbers. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 24
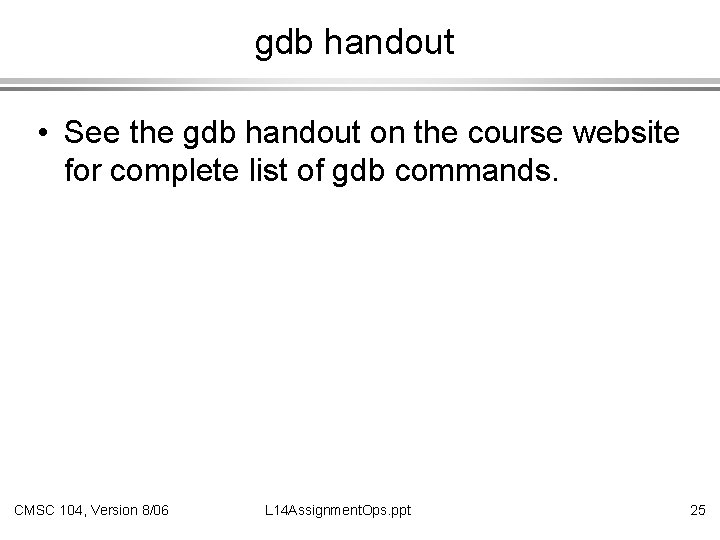
gdb handout • See the gdb handout on the course website for complete list of gdb commands. CMSC 104, Version 8/06 L 14 Assignment. Ops. ppt 25