Chapter 21 Generics 1 Why Do You Get
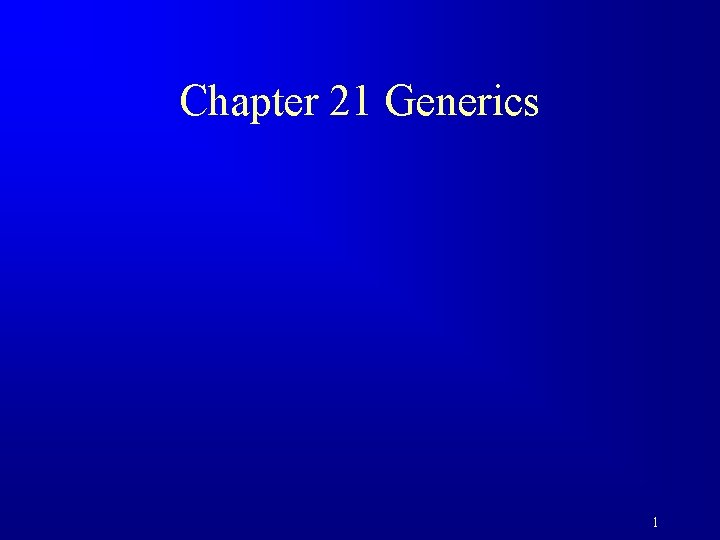
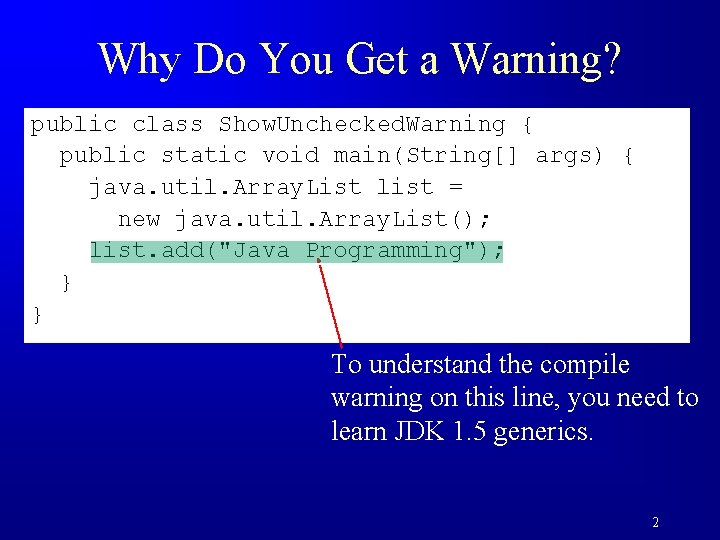
![Fix the Warning public class Show. Unchecked. Warning { public static void main(String[] args) Fix the Warning public class Show. Unchecked. Warning { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/94a27652cfa18ffbec0f171ea364a818/image-3.jpg)
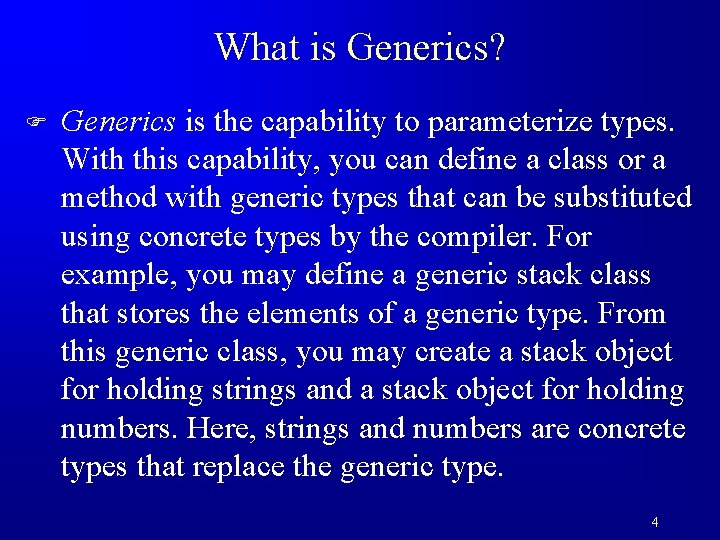
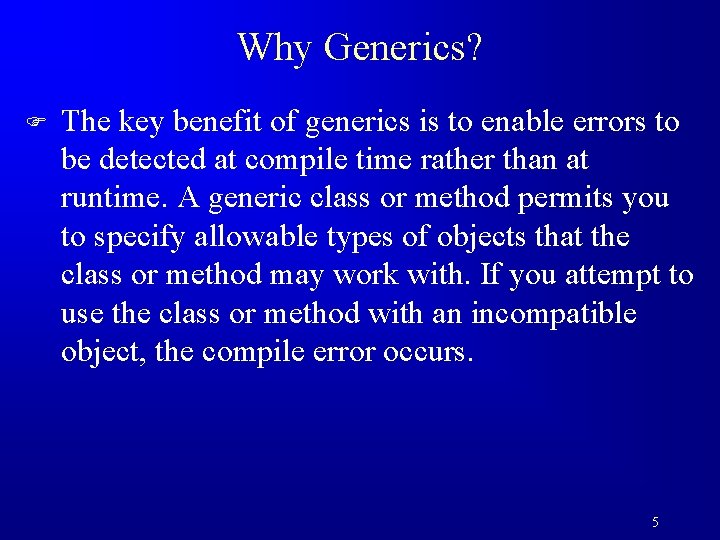
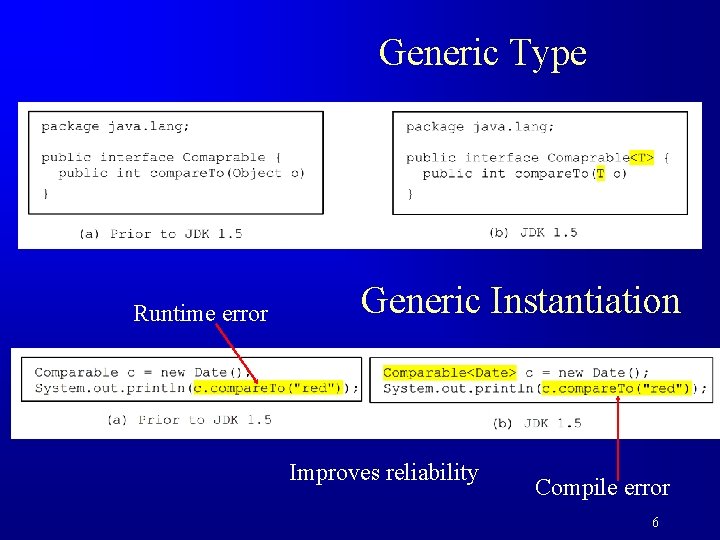
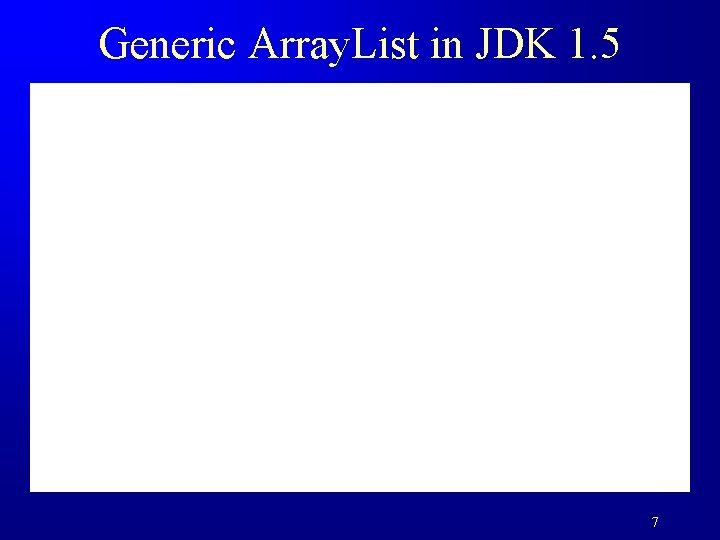
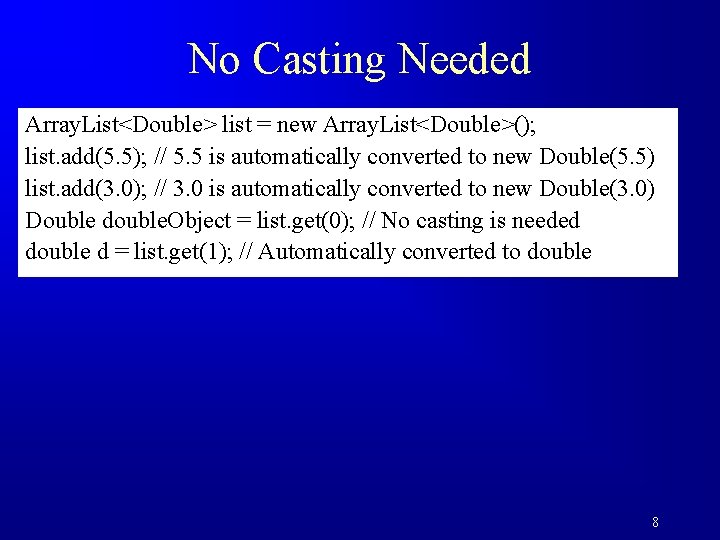
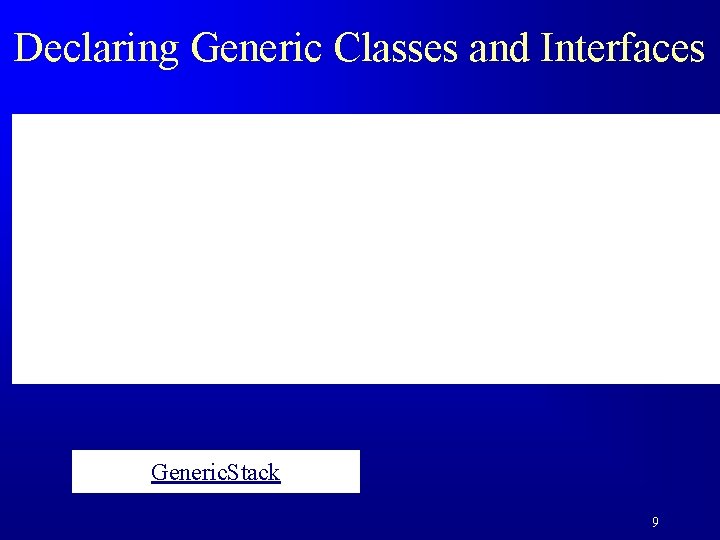
![Generic Methods public static <E> void print(E[] list) { for (int i = 0; Generic Methods public static <E> void print(E[] list) { for (int i = 0;](https://slidetodoc.com/presentation_image_h/94a27652cfa18ffbec0f171ea364a818/image-10.jpg)
![Bounded Generic Type public static void main(String[] args ) { Rectangle rectangle = new Bounded Generic Type public static void main(String[] args ) { Rectangle rectangle = new](https://slidetodoc.com/presentation_image_h/94a27652cfa18ffbec0f171ea364a818/image-11.jpg)
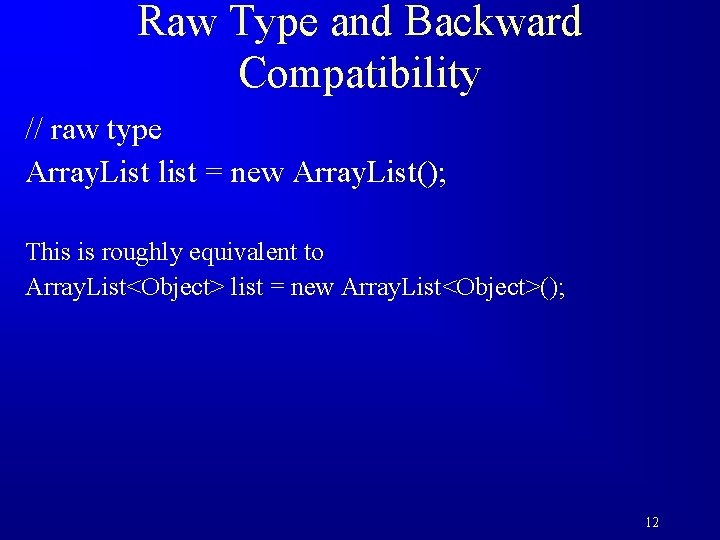
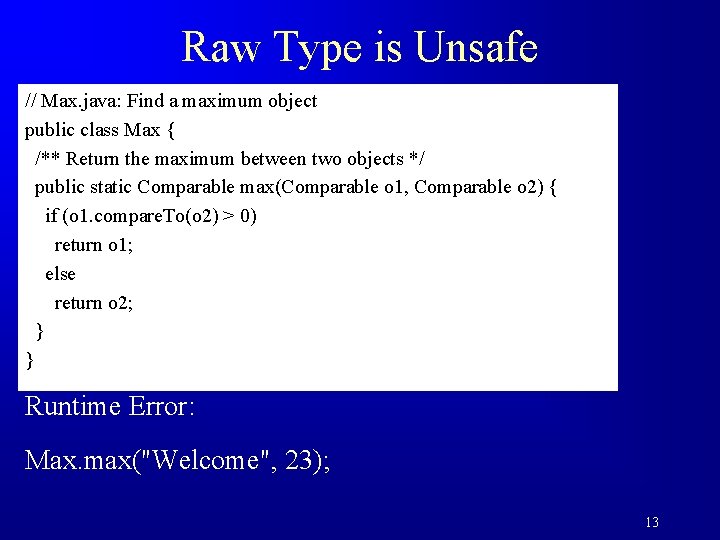
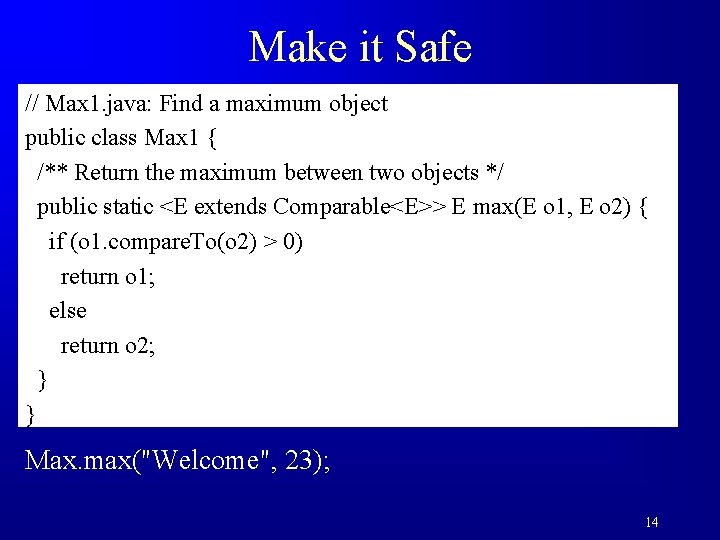
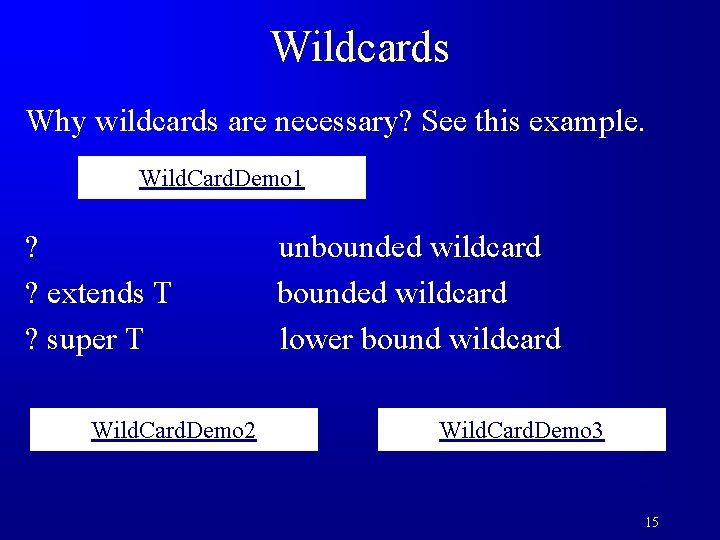
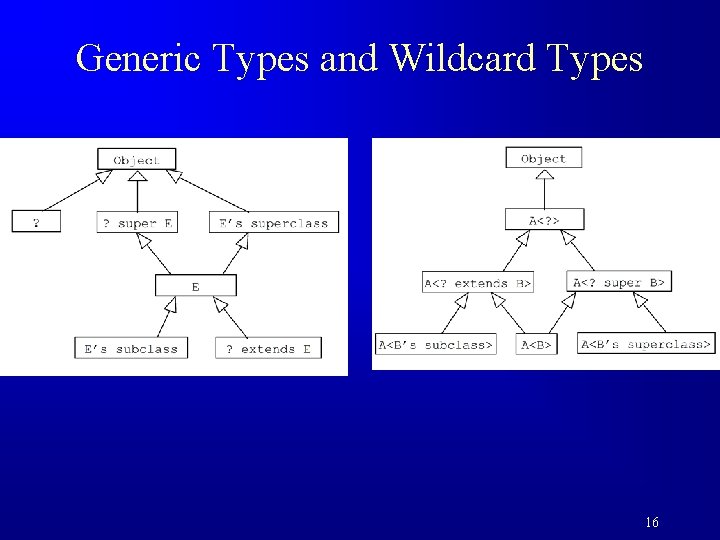
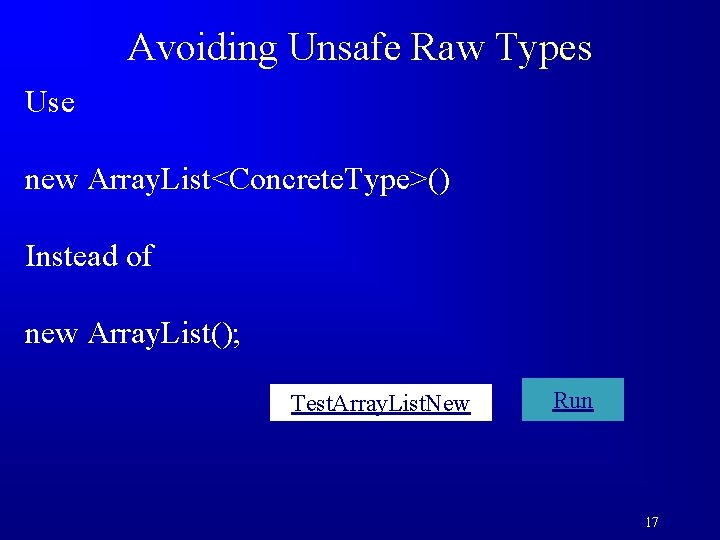
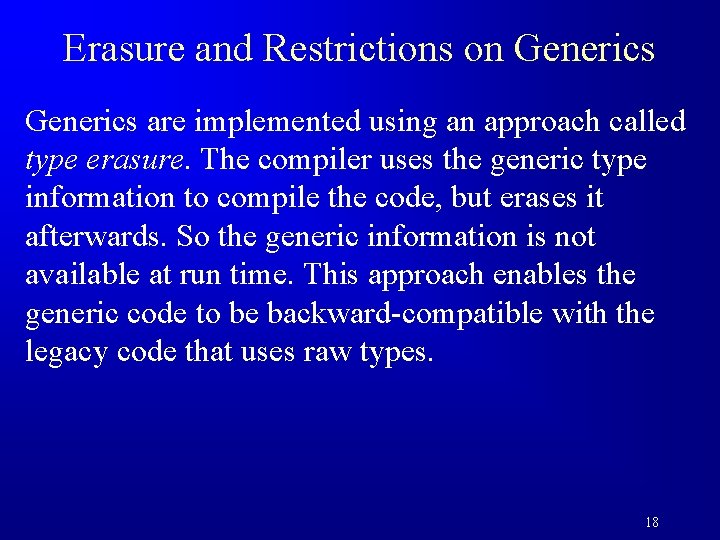
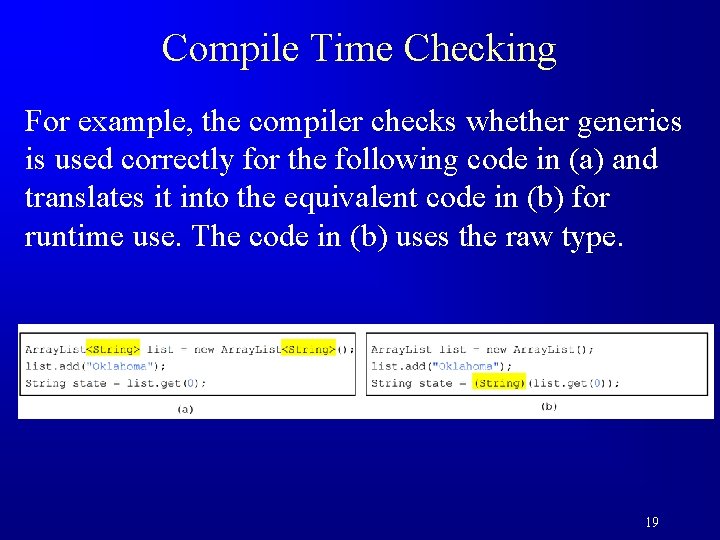
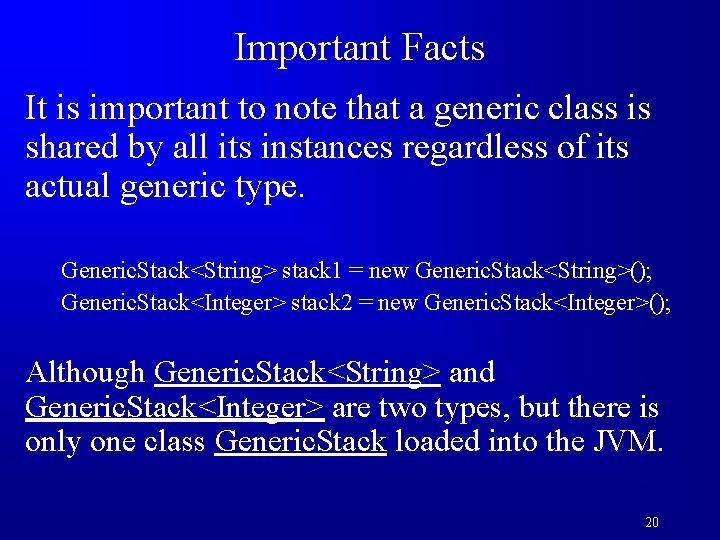
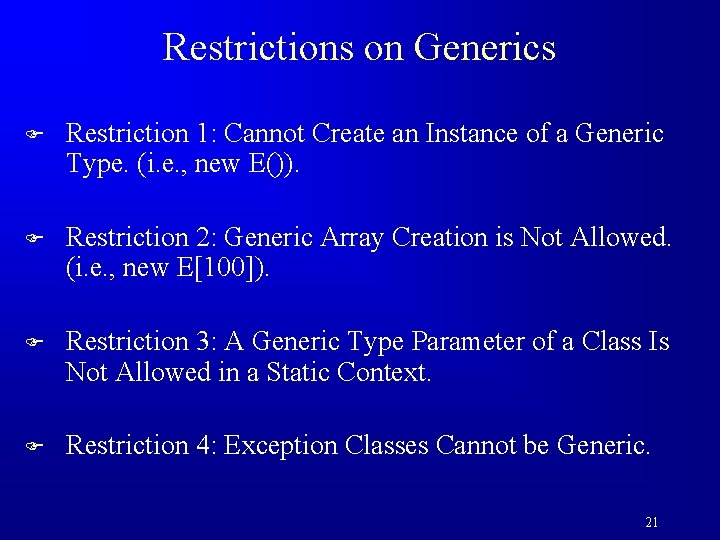
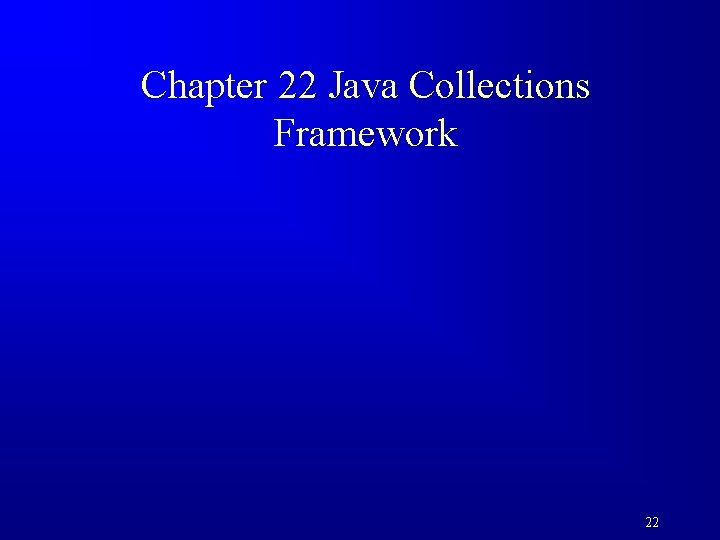
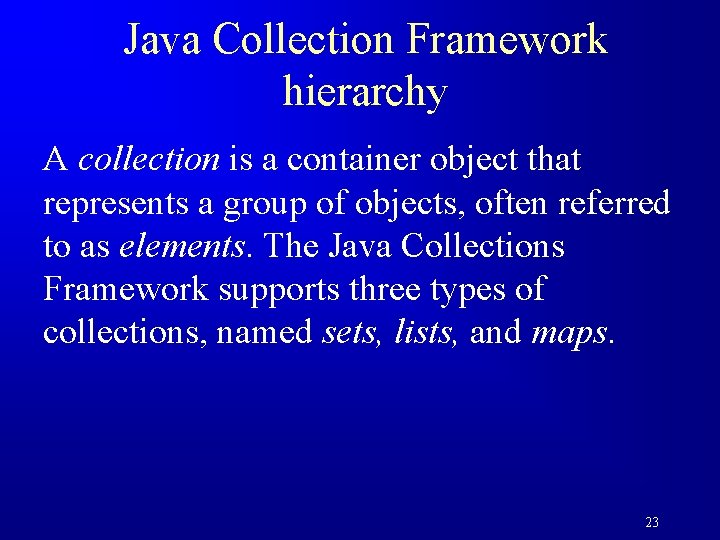
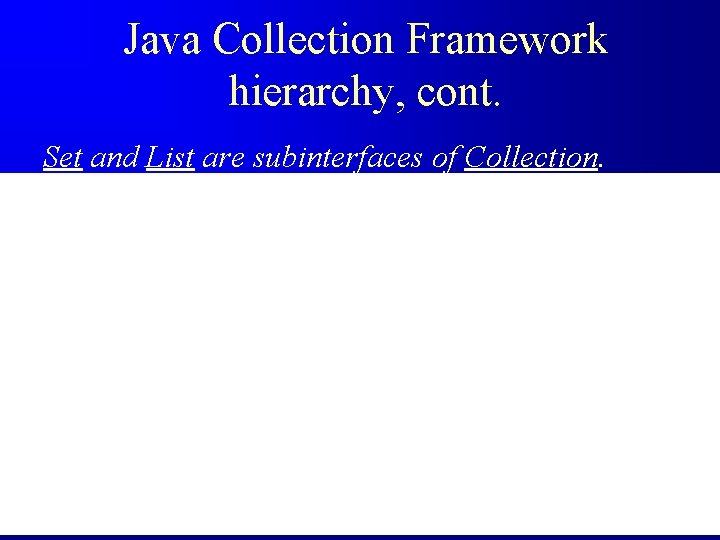
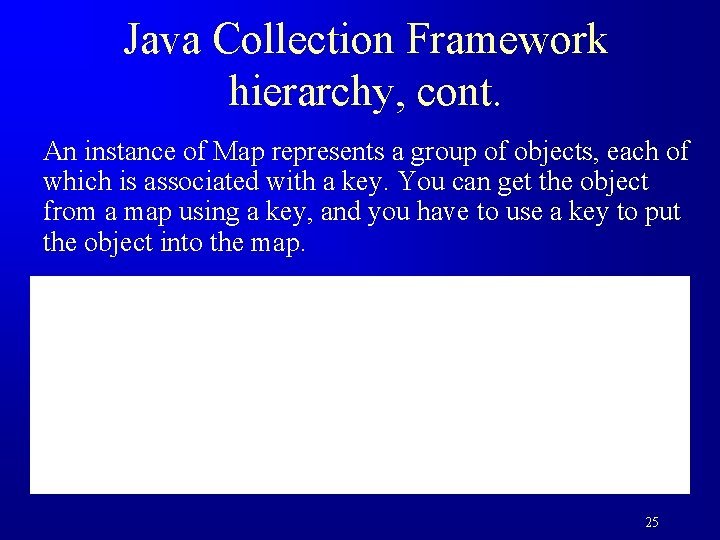
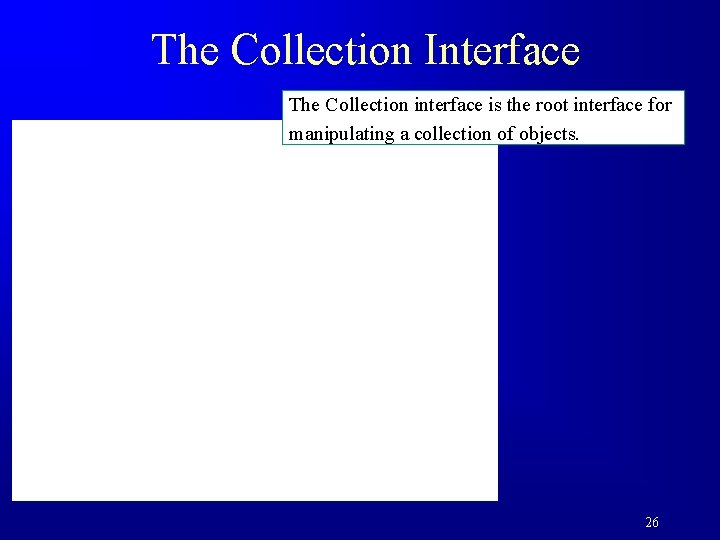
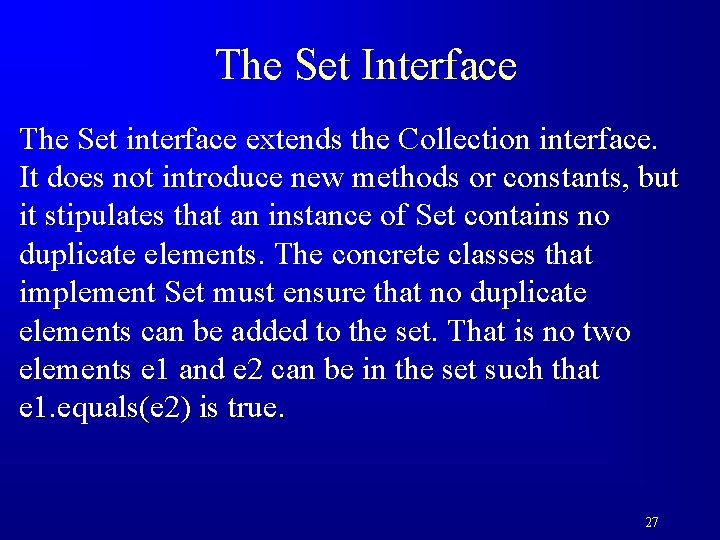
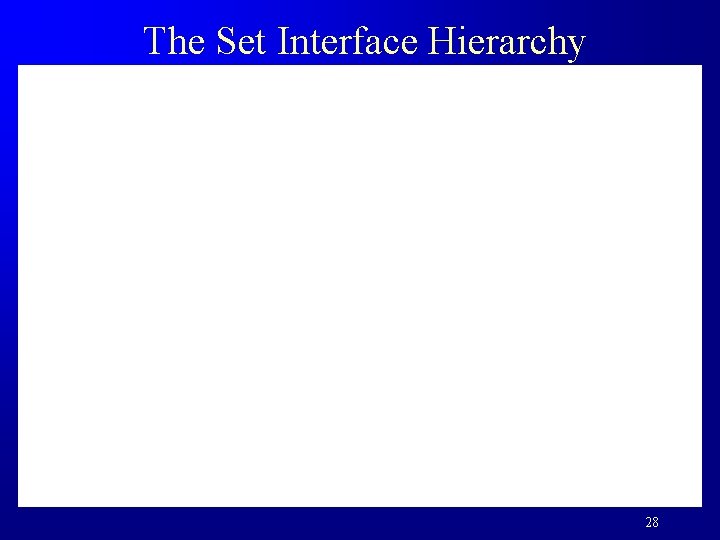
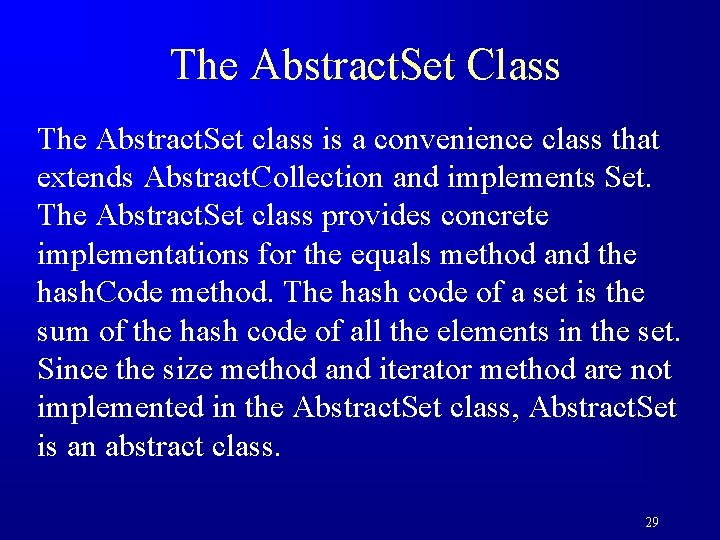
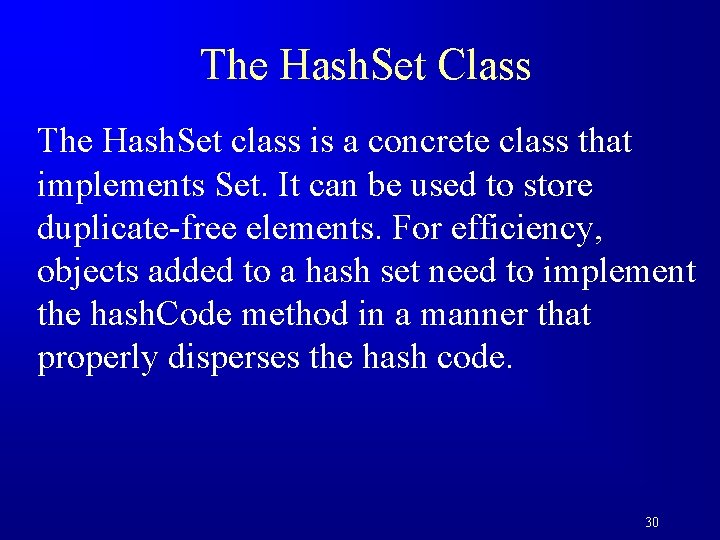
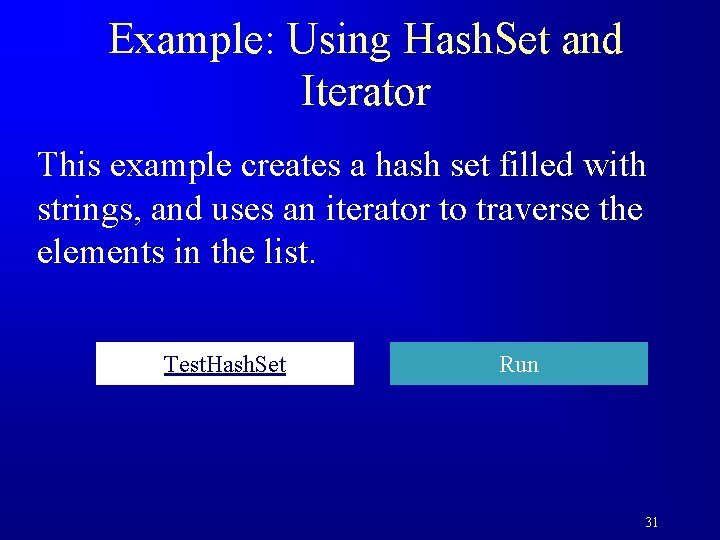
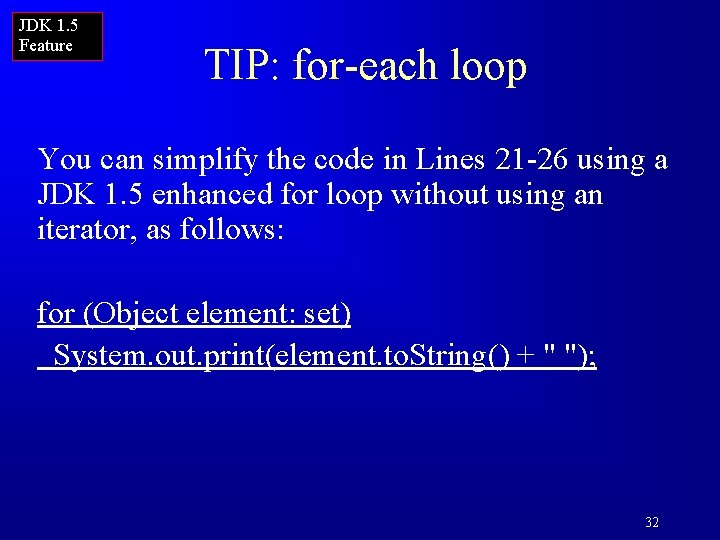
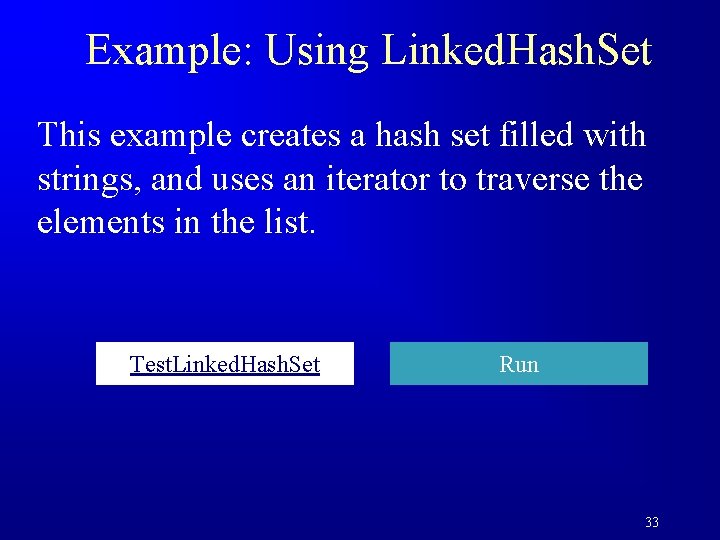
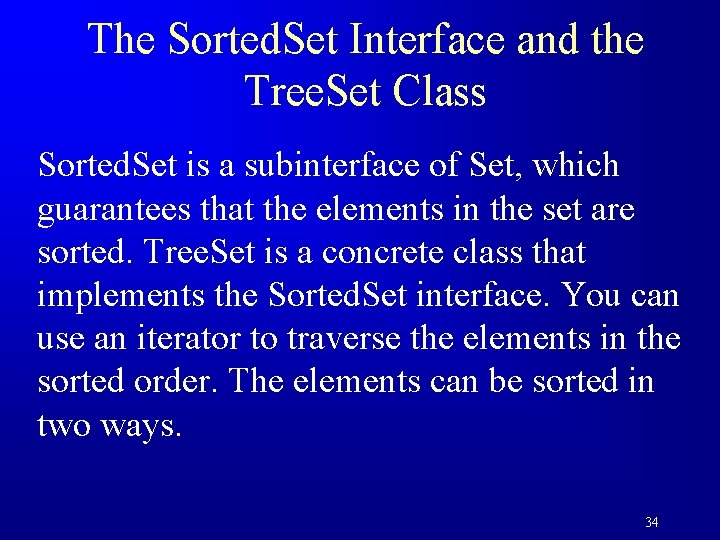
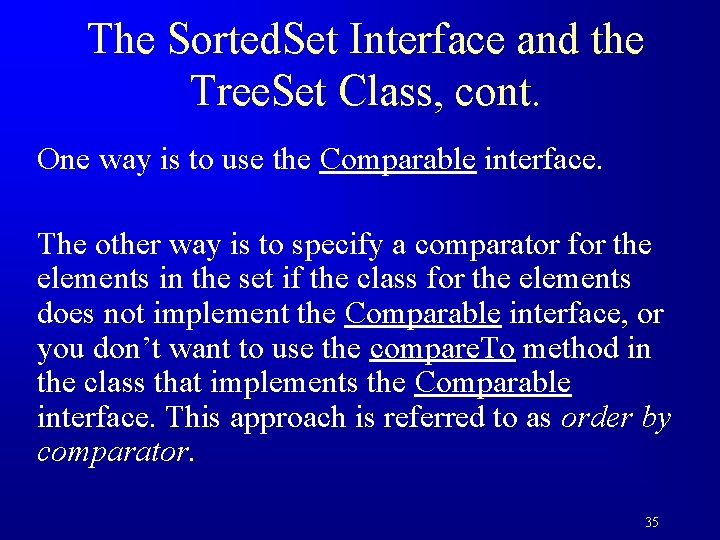
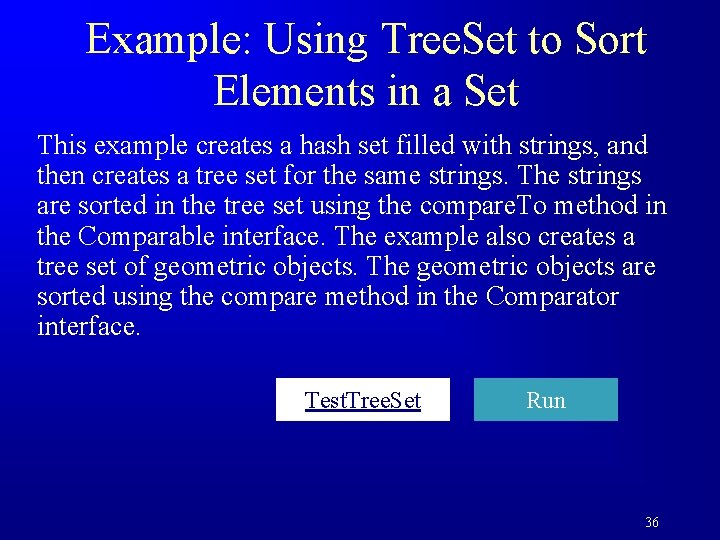
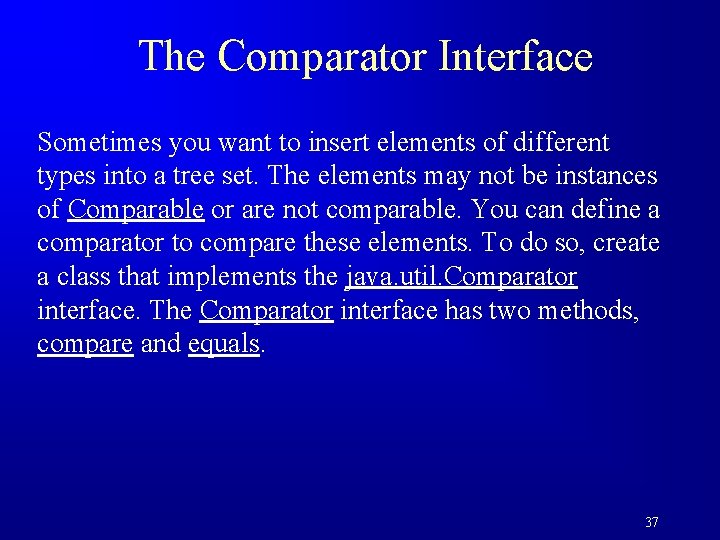
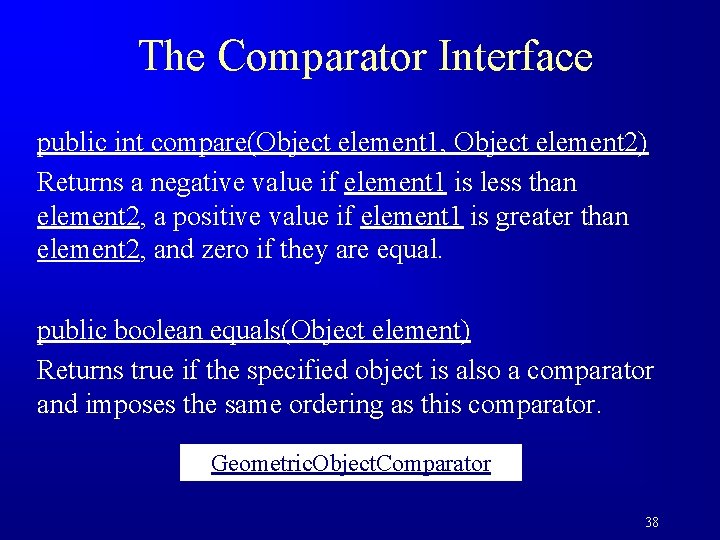
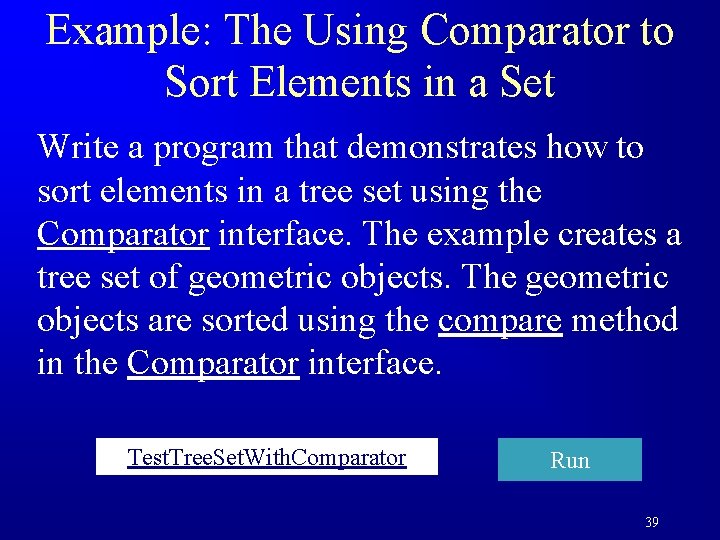
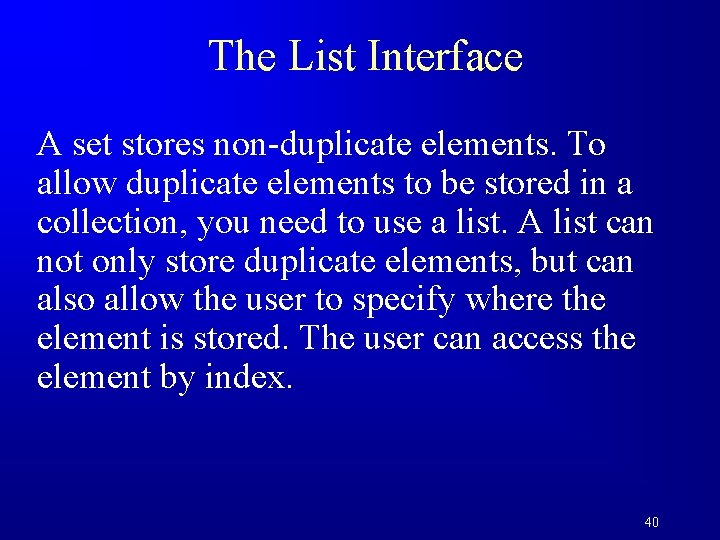
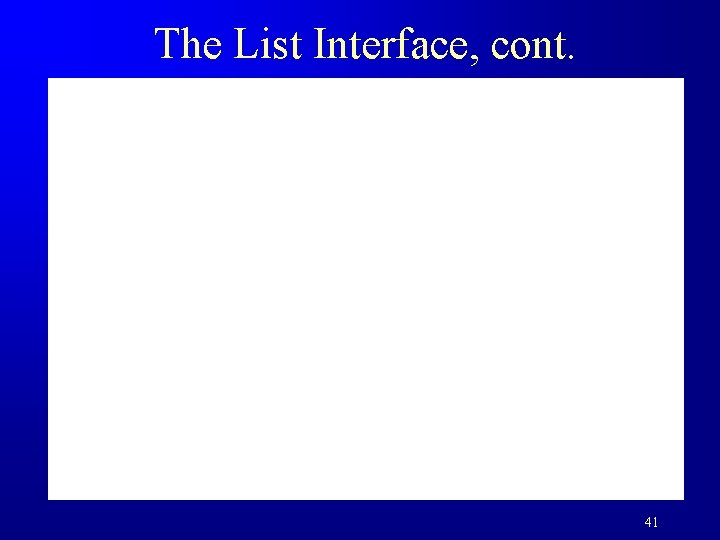
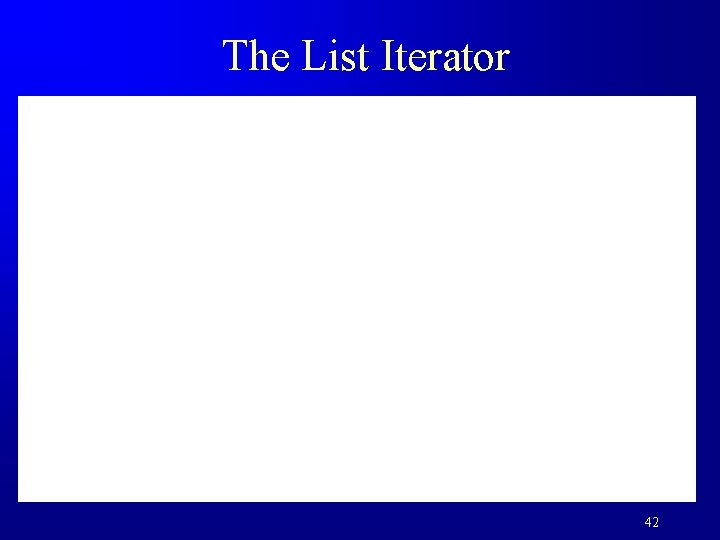
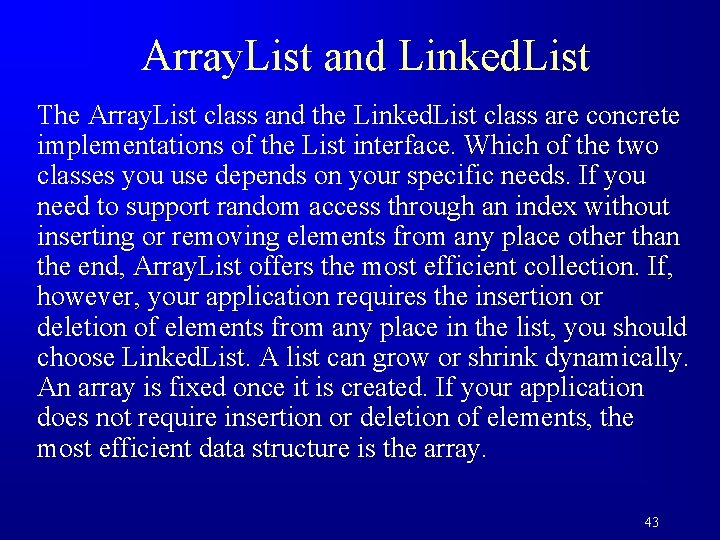
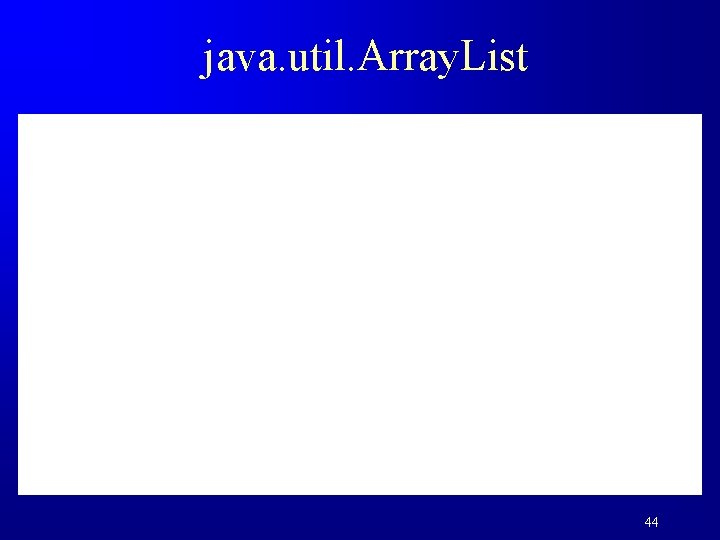
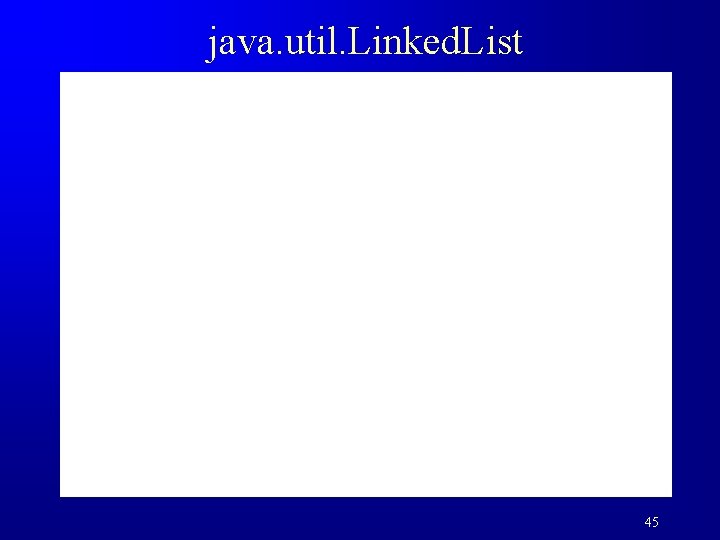
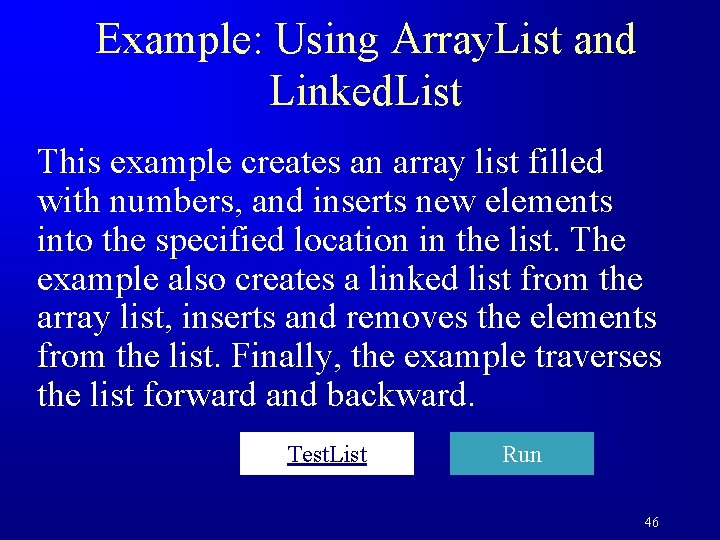
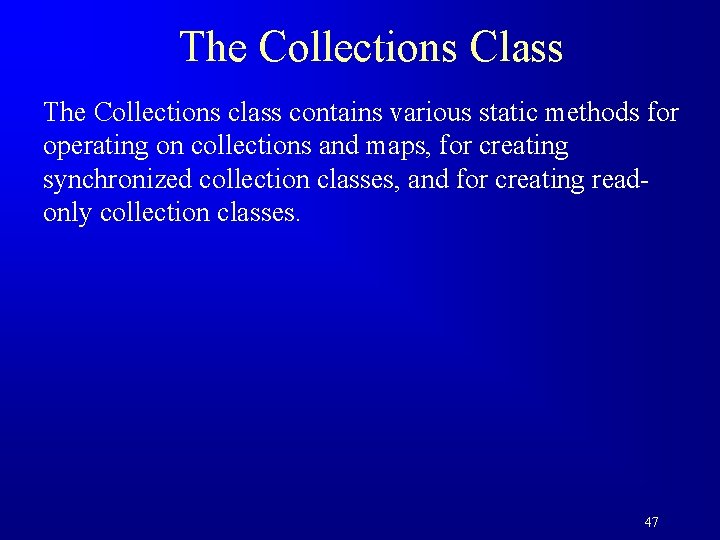
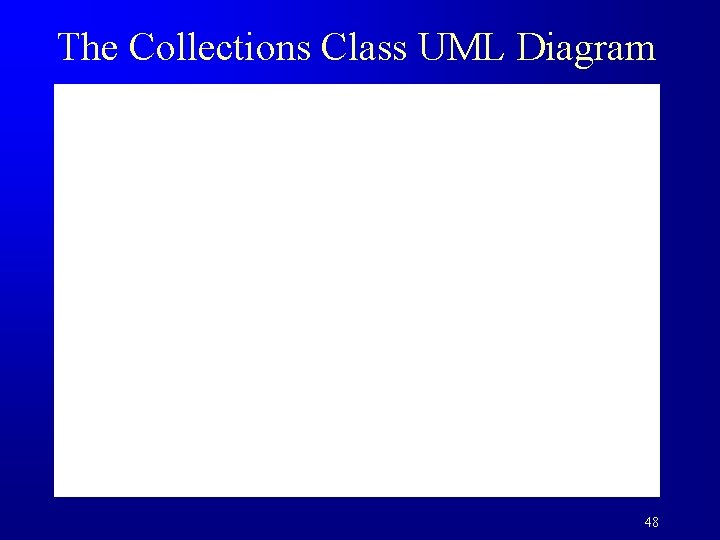
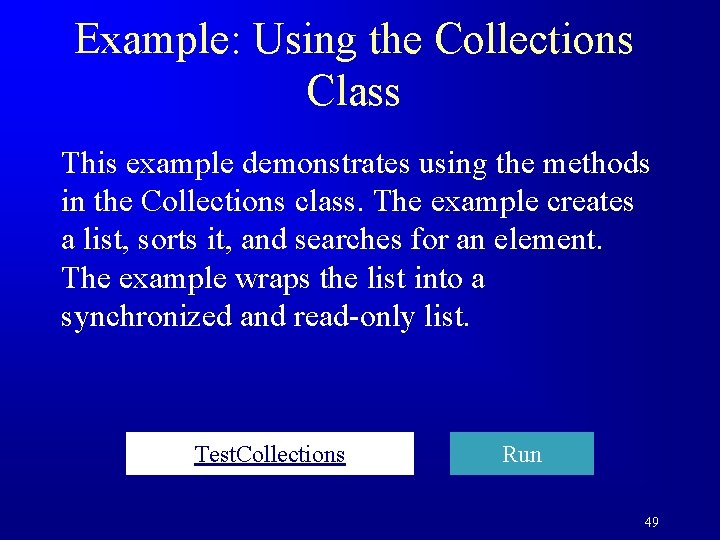
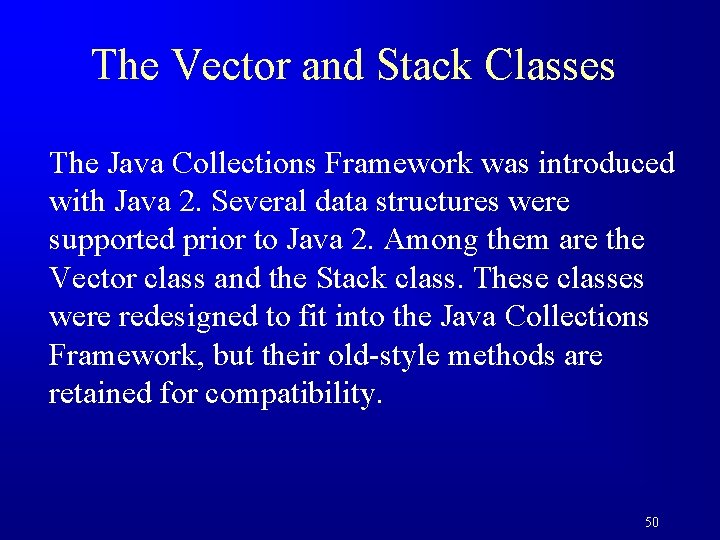
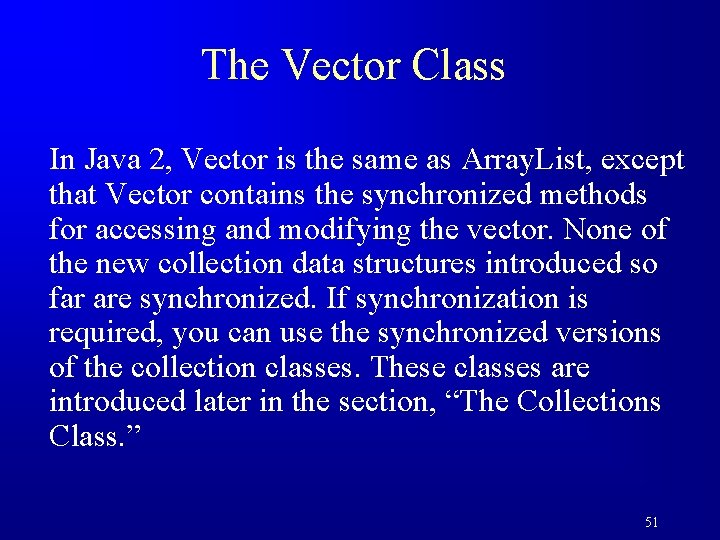
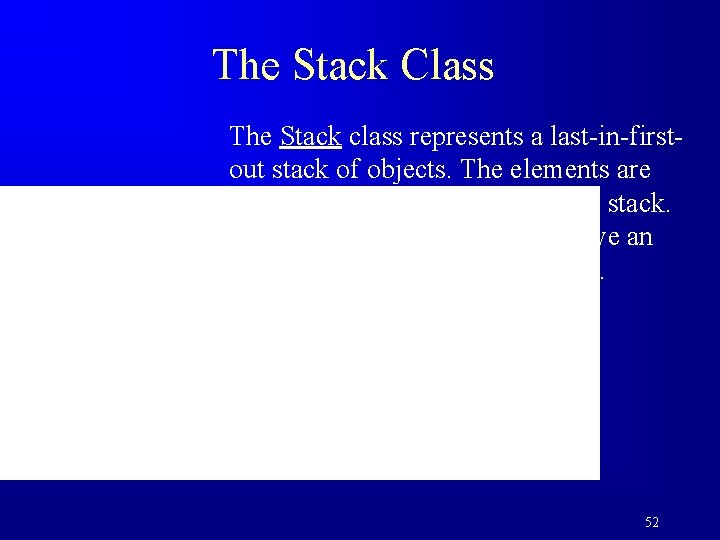
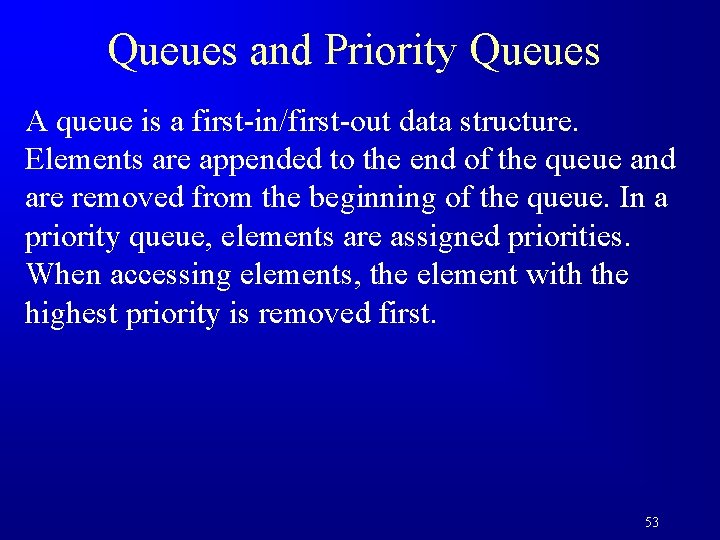
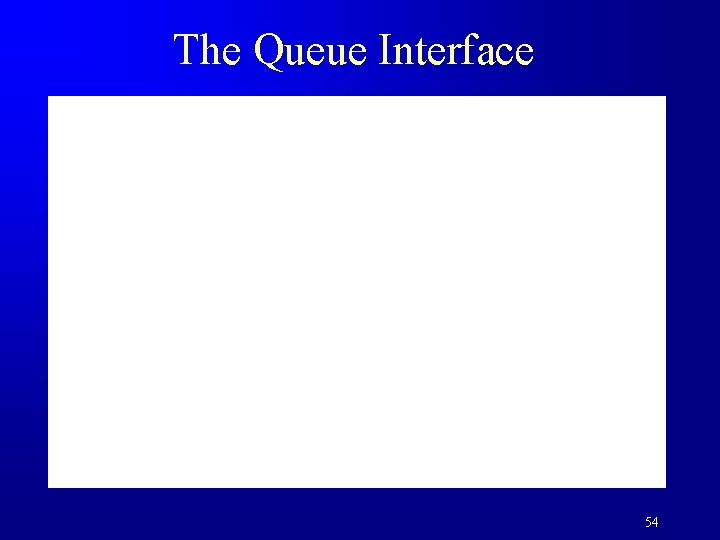
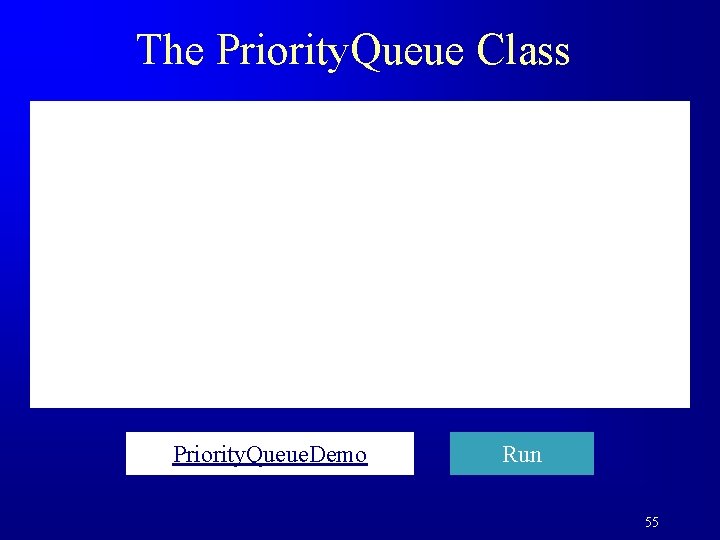
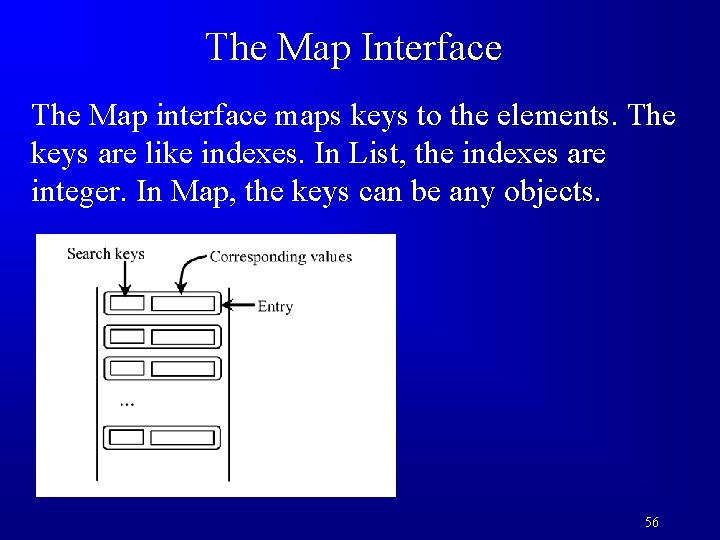
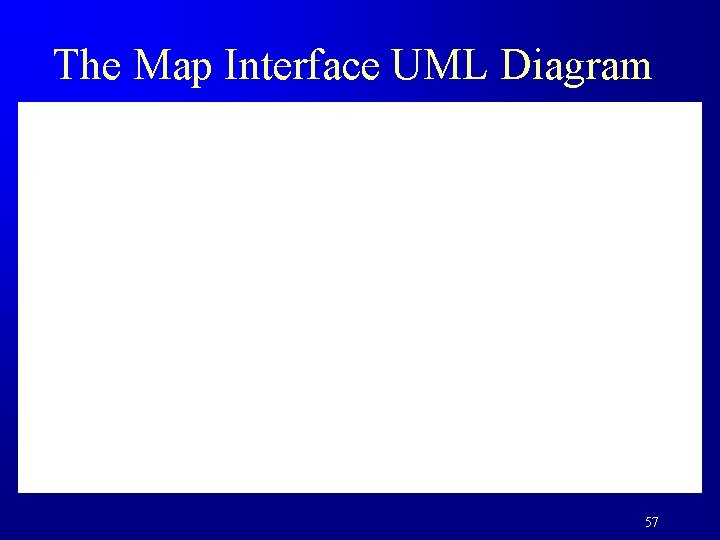
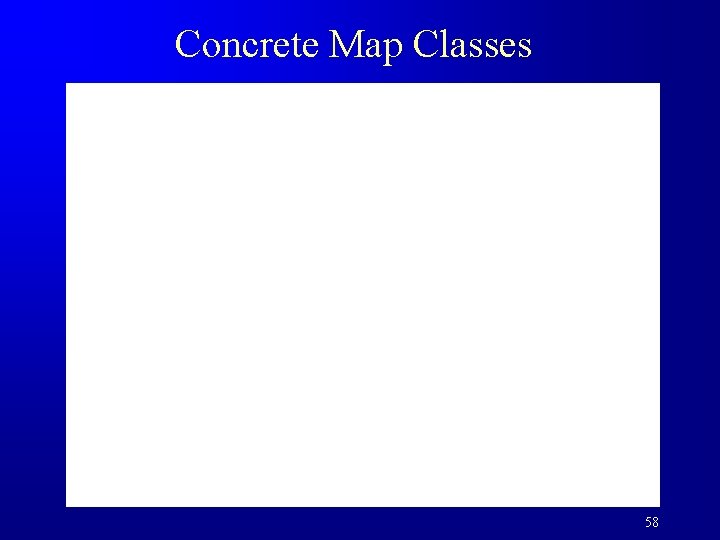
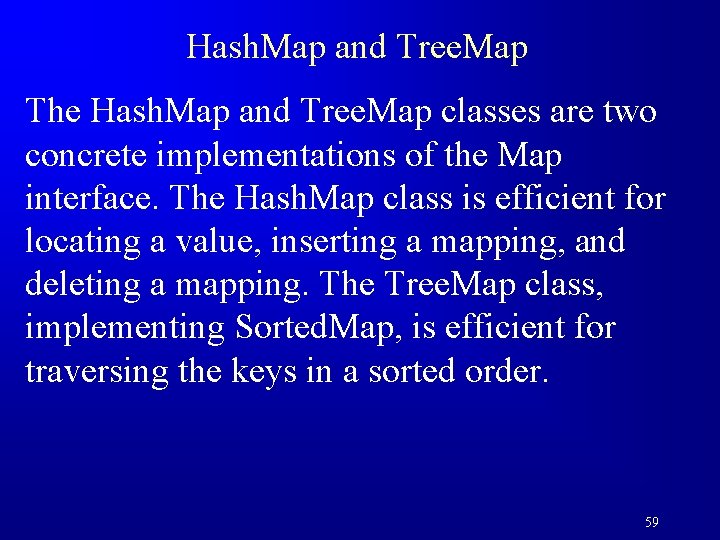
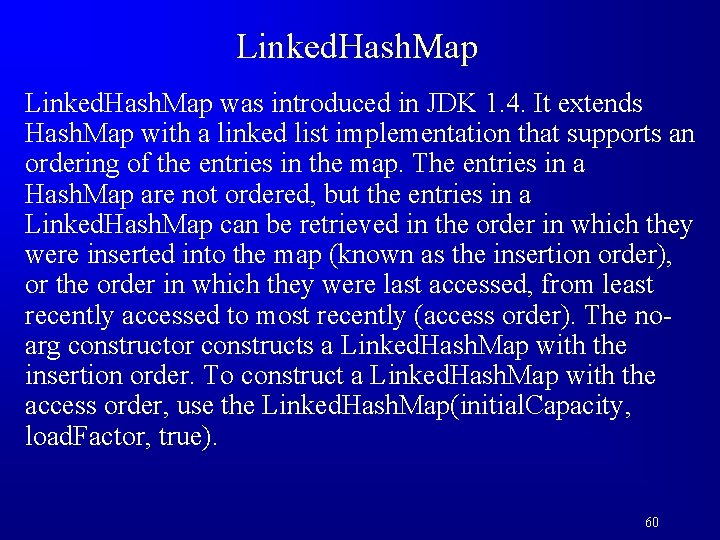
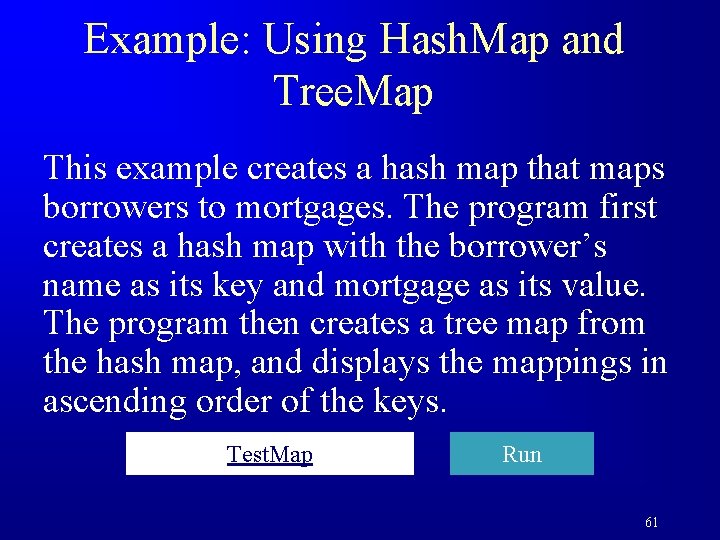
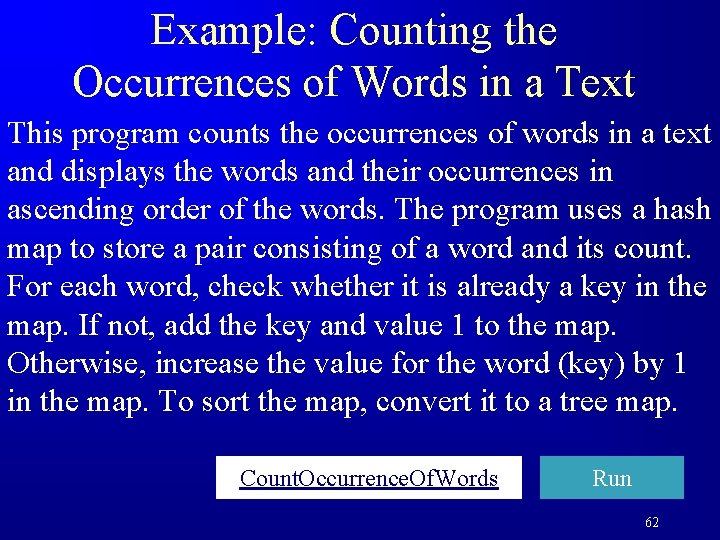
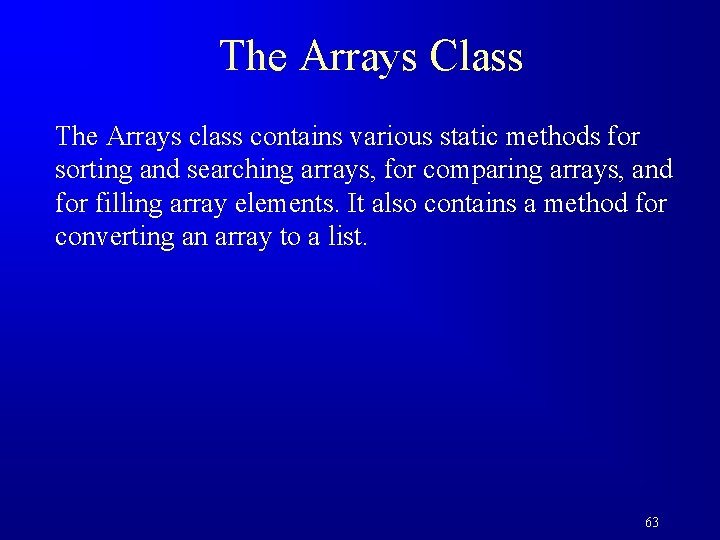
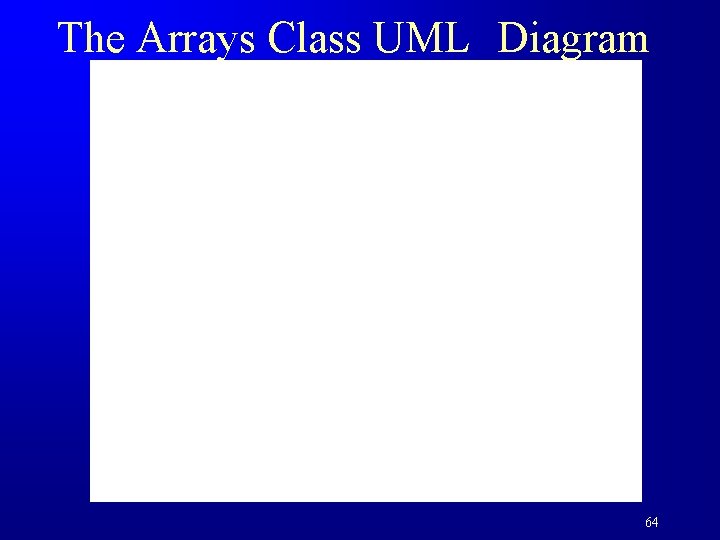
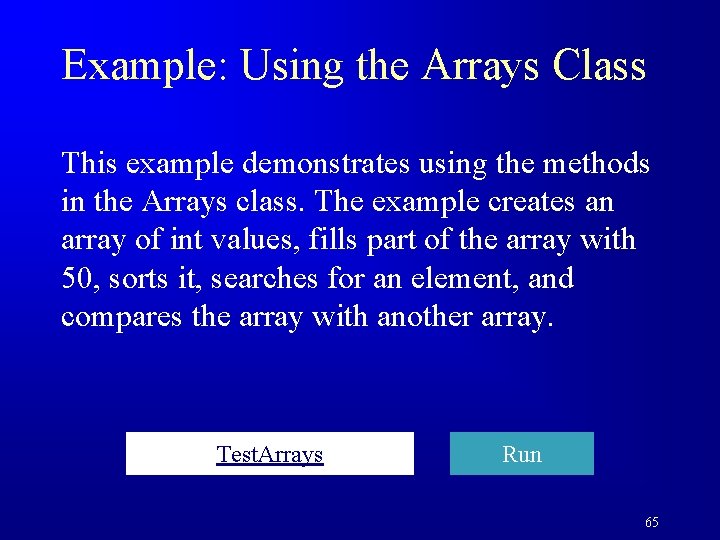
- Slides: 65
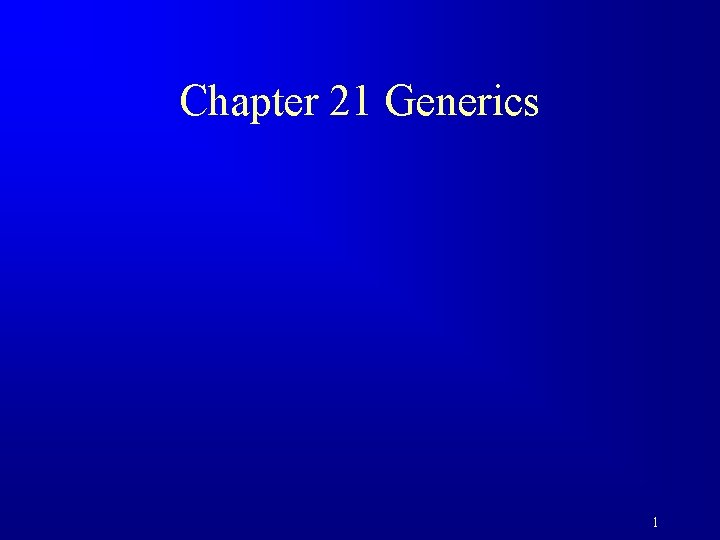
Chapter 21 Generics 1
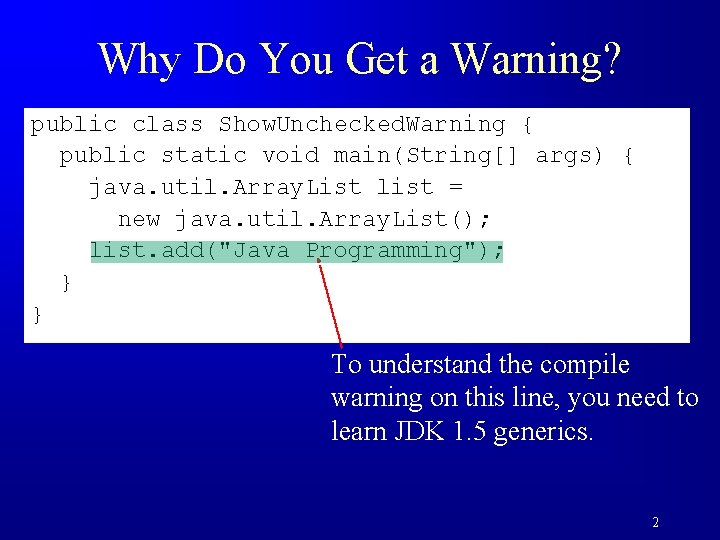
Why Do You Get a Warning? public class Show. Unchecked. Warning { public static void main(String[] args) { java. util. Array. List list = new java. util. Array. List(); list. add("Java Programming"); } } To understand the compile warning on this line, you need to learn JDK 1. 5 generics. 2
![Fix the Warning public class Show Unchecked Warning public static void mainString args Fix the Warning public class Show. Unchecked. Warning { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/94a27652cfa18ffbec0f171ea364a818/image-3.jpg)
Fix the Warning public class Show. Unchecked. Warning { public static void main(String[] args) { java. util. Array. List<String> list = new java. util. Array. List<String>(); ` list. add("Java Programming"); } } No compile warning on this line. 3
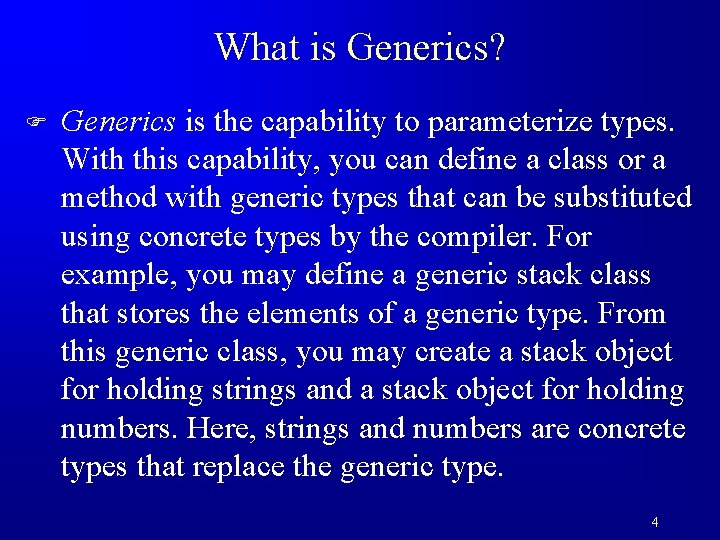
What is Generics? F Generics is the capability to parameterize types. With this capability, you can define a class or a method with generic types that can be substituted using concrete types by the compiler. For example, you may define a generic stack class that stores the elements of a generic type. From this generic class, you may create a stack object for holding strings and a stack object for holding numbers. Here, strings and numbers are concrete types that replace the generic type. 4
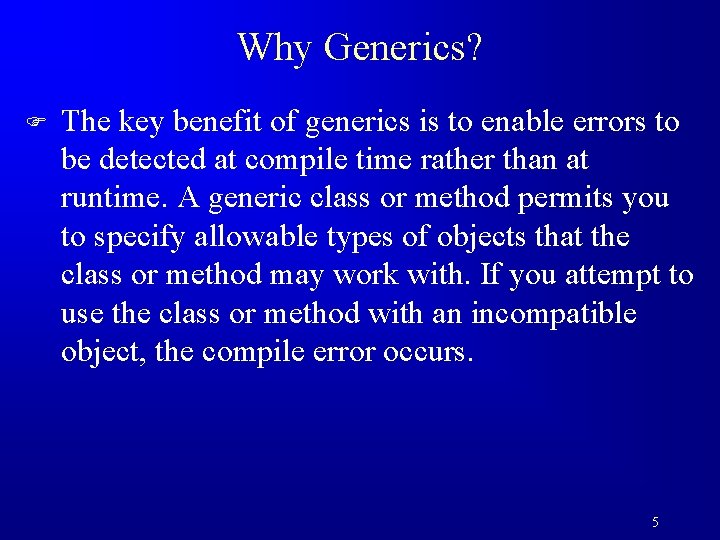
Why Generics? F The key benefit of generics is to enable errors to be detected at compile time rather than at runtime. A generic class or method permits you to specify allowable types of objects that the class or method may work with. If you attempt to use the class or method with an incompatible object, the compile error occurs. 5
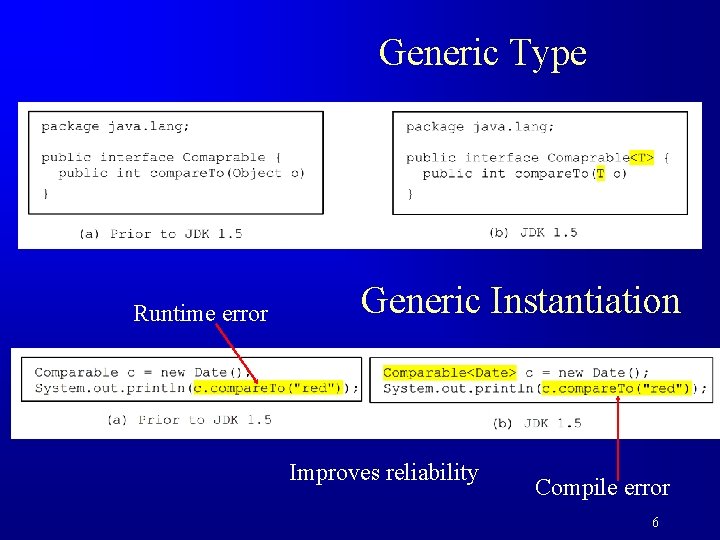
Generic Type Runtime error Generic Instantiation Improves reliability Compile error 6
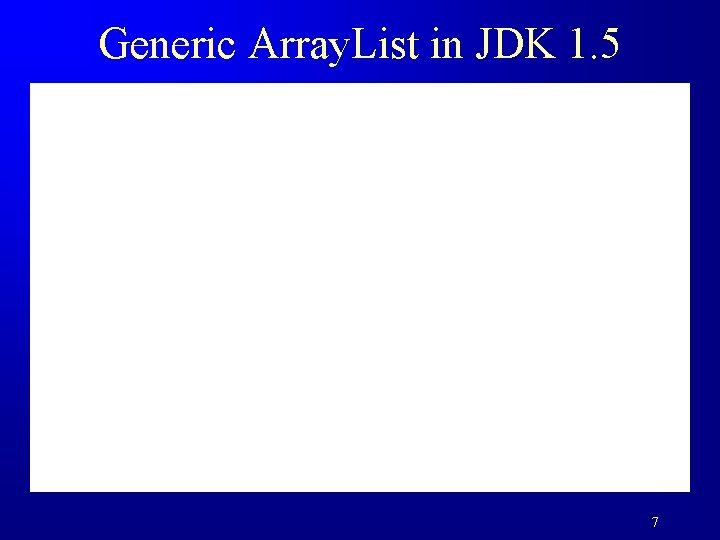
Generic Array. List in JDK 1. 5 7
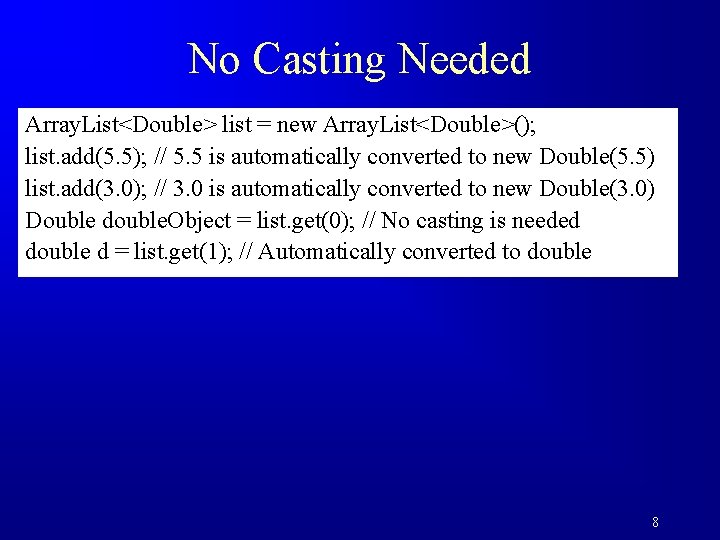
No Casting Needed Array. List<Double> list = new Array. List<Double>(); list. add(5. 5); // 5. 5 is automatically converted to new Double(5. 5) list. add(3. 0); // 3. 0 is automatically converted to new Double(3. 0) Double double. Object = list. get(0); // No casting is needed double d = list. get(1); // Automatically converted to double 8
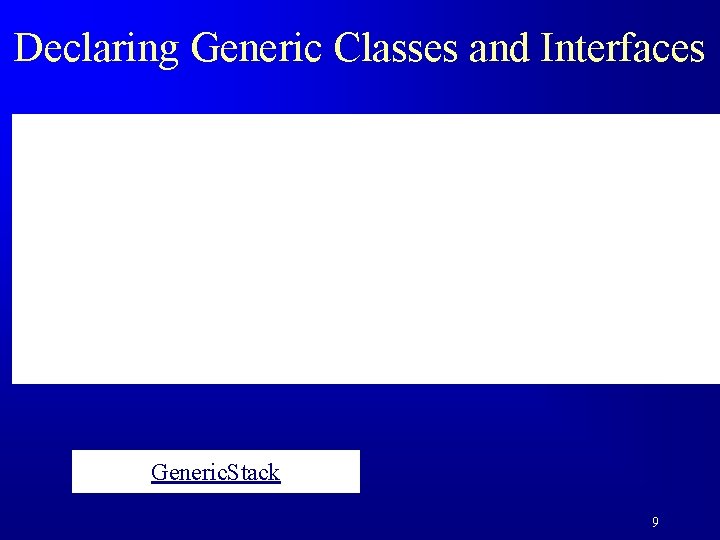
Declaring Generic Classes and Interfaces Generic. Stack 9
![Generic Methods public static E void printE list for int i 0 Generic Methods public static <E> void print(E[] list) { for (int i = 0;](https://slidetodoc.com/presentation_image_h/94a27652cfa18ffbec0f171ea364a818/image-10.jpg)
Generic Methods public static <E> void print(E[] list) { for (int i = 0; i < list. length; i++) System. out. print(list[i] + " "); System. out. println(); } public static void print(Object[] list) { for (int i = 0; i < list. length; i++) System. out. print(list[i] + " "); System. out. println(); } 10
![Bounded Generic Type public static void mainString args Rectangle rectangle new Bounded Generic Type public static void main(String[] args ) { Rectangle rectangle = new](https://slidetodoc.com/presentation_image_h/94a27652cfa18ffbec0f171ea364a818/image-11.jpg)
Bounded Generic Type public static void main(String[] args ) { Rectangle rectangle = new Rectangle(2, 2); Circle 9 circle = new Circle 9(2); System. out. println("Same area? " + equal. Area(rectangle, circle)); } public static <E extends Geometric. Object> boolean equal. Area(E object 1, E object 2) { return object 1. get. Area() == object 2. get. Area(); } 11
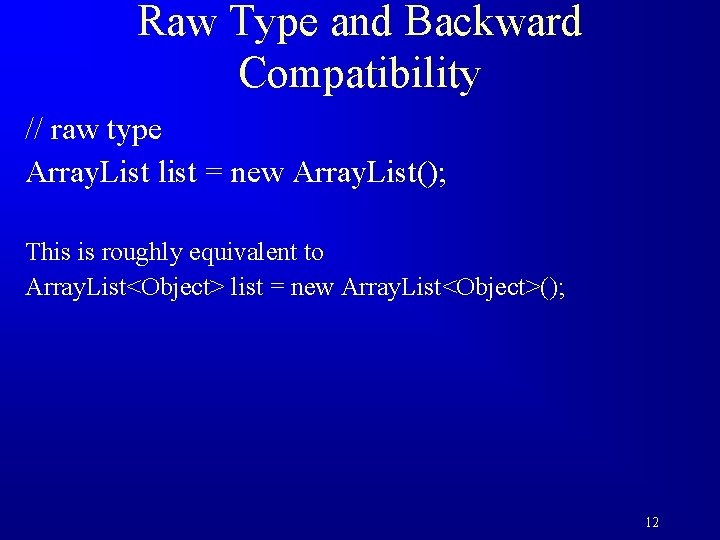
Raw Type and Backward Compatibility // raw type Array. List list = new Array. List(); This is roughly equivalent to Array. List<Object> list = new Array. List<Object>(); 12
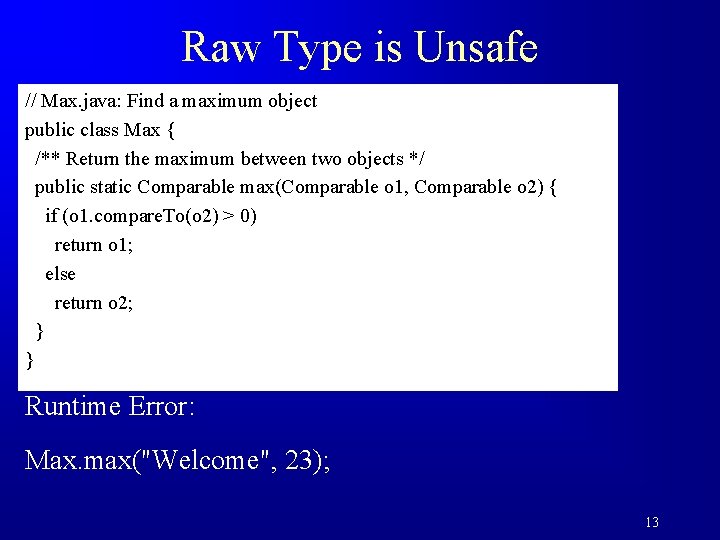
Raw Type is Unsafe // Max. java: Find a maximum object public class Max { /** Return the maximum between two objects */ public static Comparable max(Comparable o 1, Comparable o 2) { if (o 1. compare. To(o 2) > 0) return o 1; else return o 2; } } Runtime Error: Max. max("Welcome", 23); 13
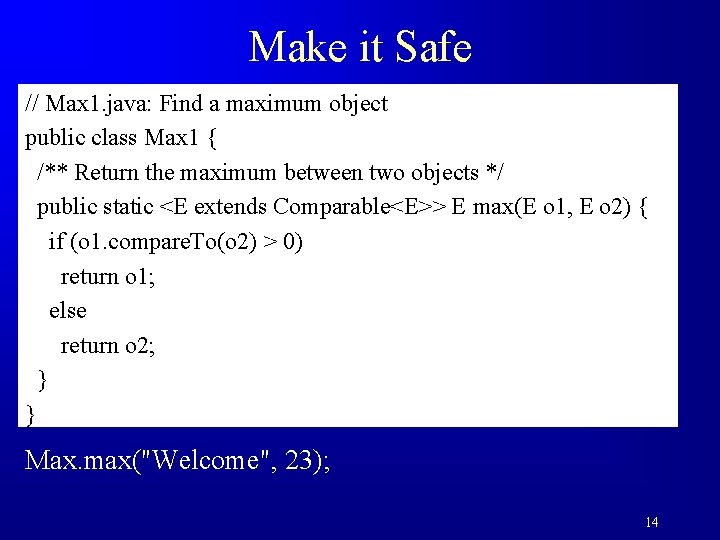
Make it Safe // Max 1. java: Find a maximum object public class Max 1 { /** Return the maximum between two objects */ public static <E extends Comparable<E>> E max(E o 1, E o 2) { if (o 1. compare. To(o 2) > 0) return o 1; else return o 2; } } Max. max("Welcome", 23); 14
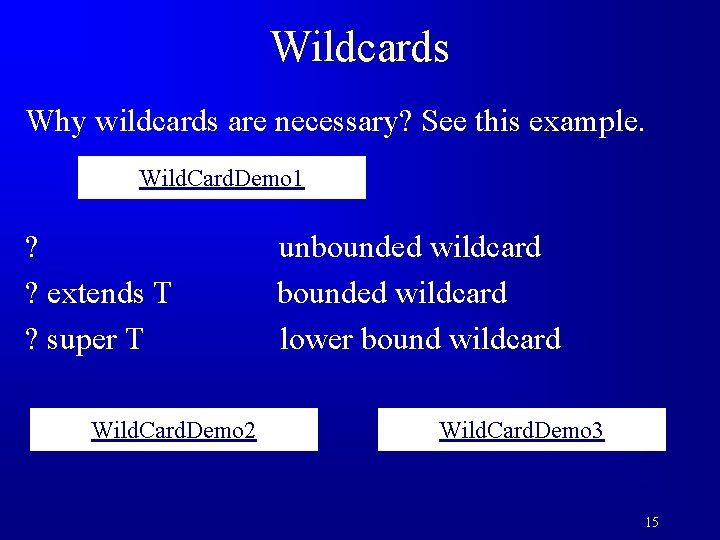
Wildcards Why wildcards are necessary? See this example. Wild. Card. Demo 1 ? unbounded wildcard ? extends T bounded wildcard ? super T lower bound wildcard Wild. Card. Demo 2 Wild. Card. Demo 3 15
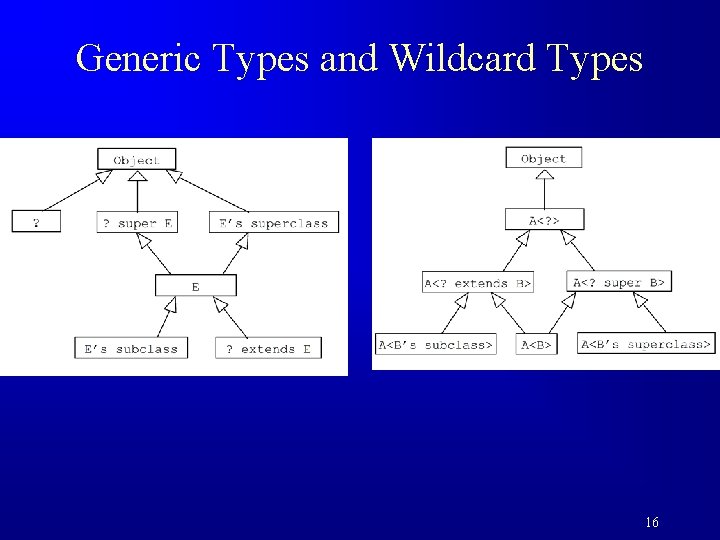
Generic Types and Wildcard Types 16
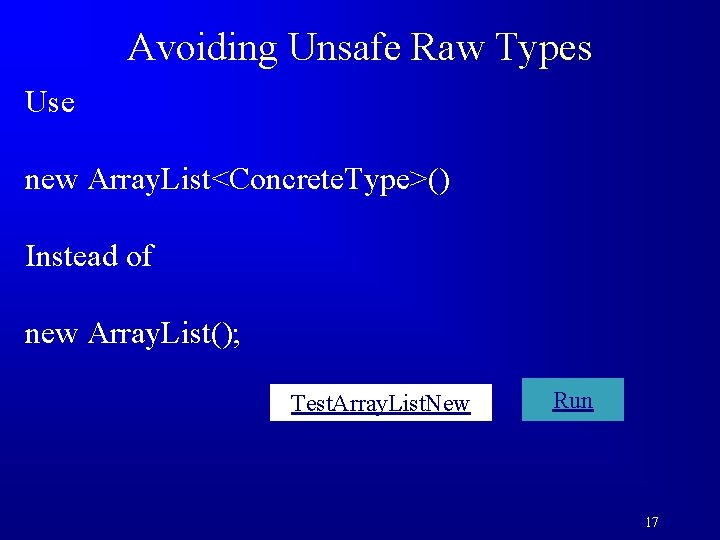
Avoiding Unsafe Raw Types Use new Array. List<Concrete. Type>() Instead of new Array. List(); Test. Array. List. New Run 17
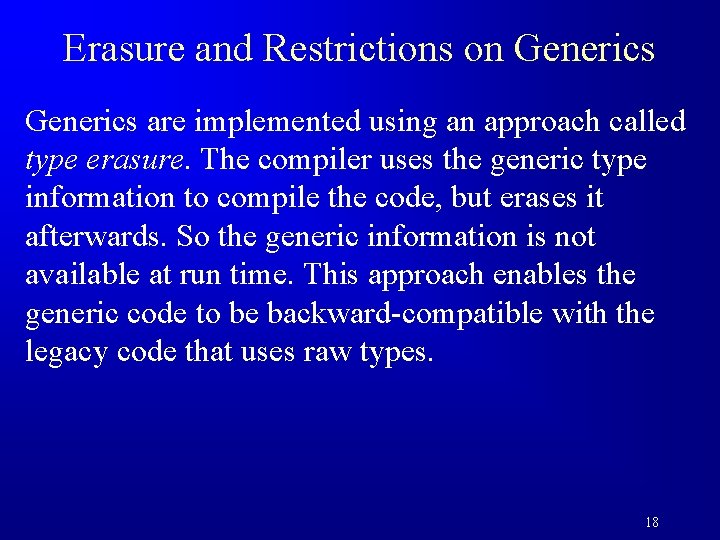
Erasure and Restrictions on Generics are implemented using an approach called type erasure. The compiler uses the generic type information to compile the code, but erases it afterwards. So the generic information is not available at run time. This approach enables the generic code to be backward-compatible with the legacy code that uses raw types. 18
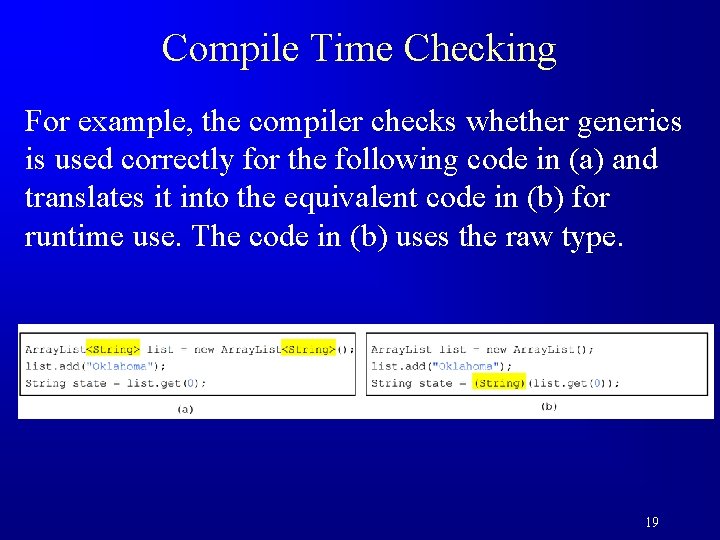
Compile Time Checking For example, the compiler checks whether generics is used correctly for the following code in (a) and translates it into the equivalent code in (b) for runtime use. The code in (b) uses the raw type. 19
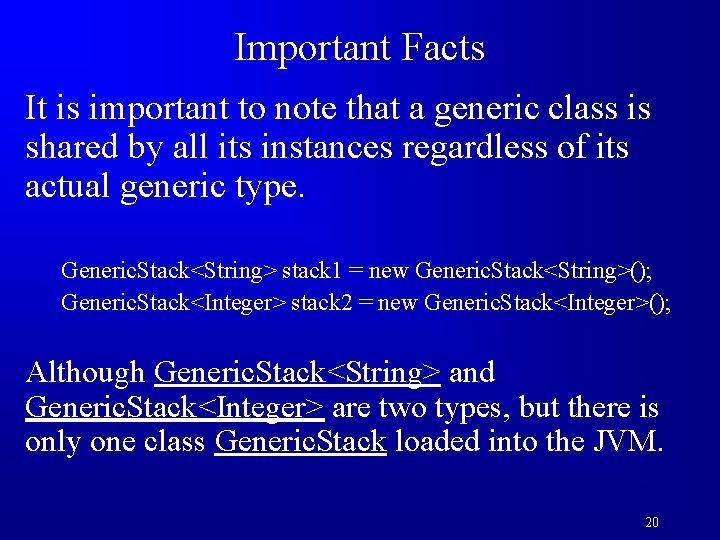
Important Facts It is important to note that a generic class is shared by all its instances regardless of its actual generic type. Generic. Stack<String> stack 1 = new Generic. Stack<String>(); Generic. Stack<Integer> stack 2 = new Generic. Stack<Integer>(); Although Generic. Stack<String> and Generic. Stack<Integer> are two types, but there is only one class Generic. Stack loaded into the JVM. 20
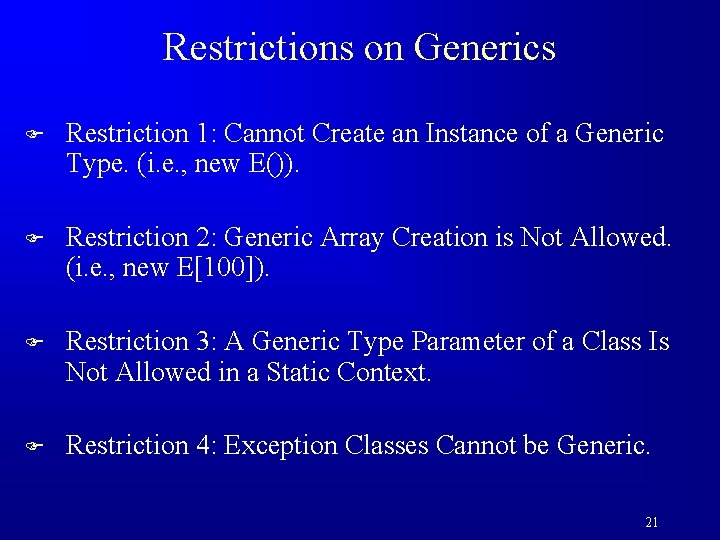
Restrictions on Generics F Restriction 1: Cannot Create an Instance of a Generic Type. (i. e. , new E()). F Restriction 2: Generic Array Creation is Not Allowed. (i. e. , new E[100]). F Restriction 3: A Generic Type Parameter of a Class Is Not Allowed in a Static Context. F Restriction 4: Exception Classes Cannot be Generic. 21
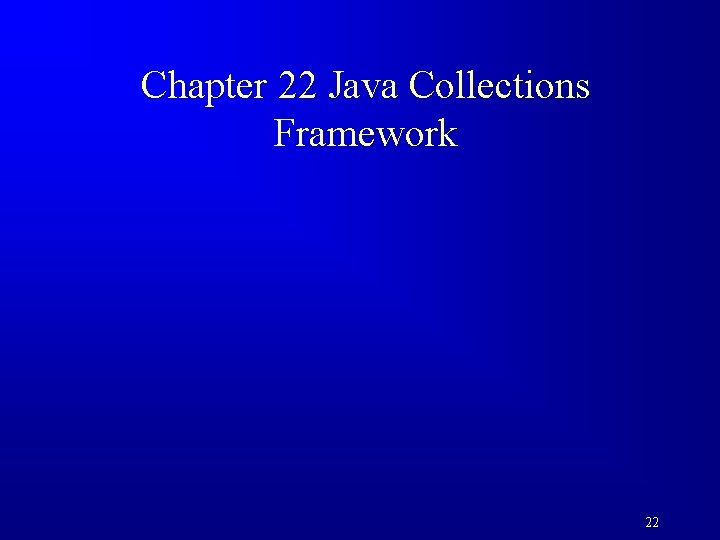
Chapter 22 Java Collections Framework 22
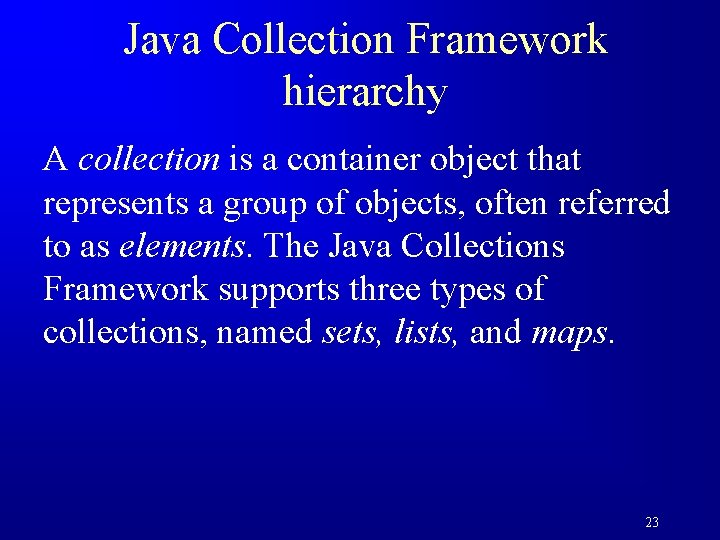
Java Collection Framework hierarchy A collection is a container object that represents a group of objects, often referred to as elements. The Java Collections Framework supports three types of collections, named sets, lists, and maps. 23
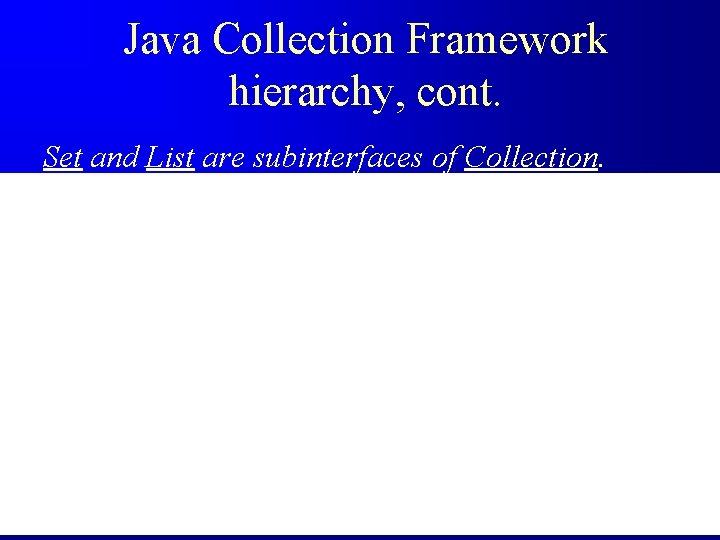
Java Collection Framework hierarchy, cont. Set and List are subinterfaces of Collection. 24
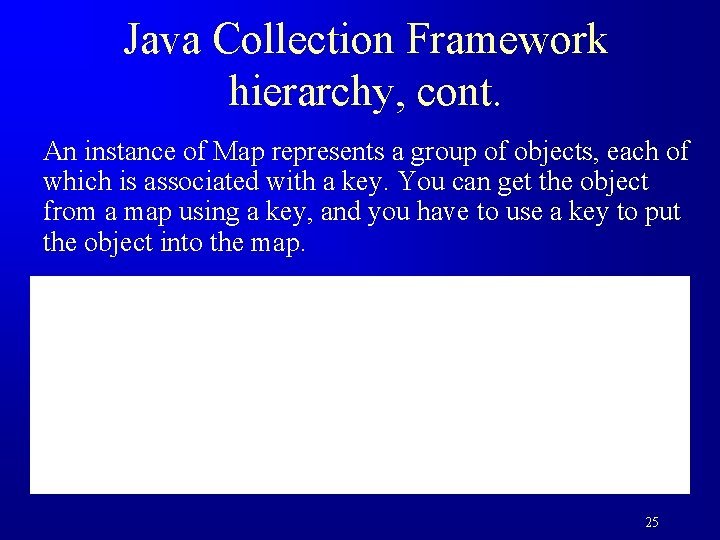
Java Collection Framework hierarchy, cont. An instance of Map represents a group of objects, each of which is associated with a key. You can get the object from a map using a key, and you have to use a key to put the object into the map. 25
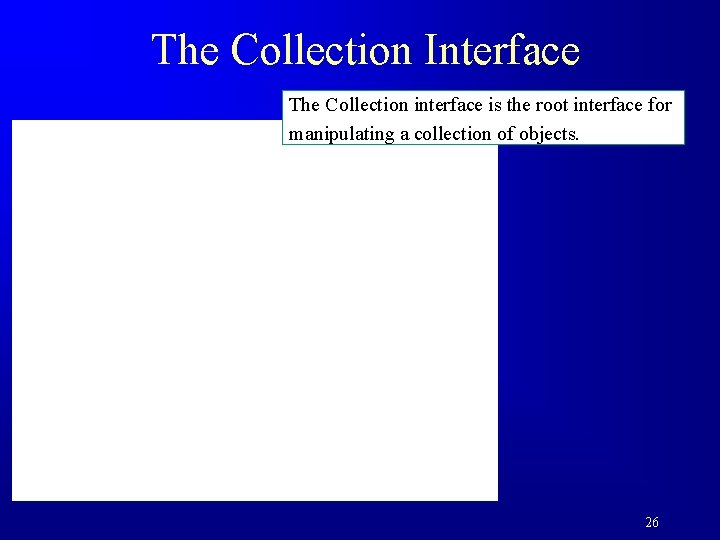
The Collection Interface The Collection interface is the root interface for manipulating a collection of objects. 26
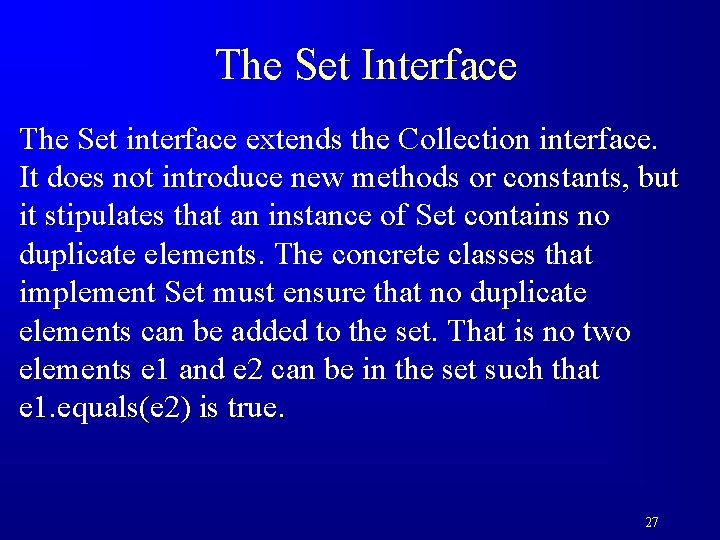
The Set Interface The Set interface extends the Collection interface. It does not introduce new methods or constants, but it stipulates that an instance of Set contains no duplicate elements. The concrete classes that implement Set must ensure that no duplicate elements can be added to the set. That is no two elements e 1 and e 2 can be in the set such that e 1. equals(e 2) is true. 27
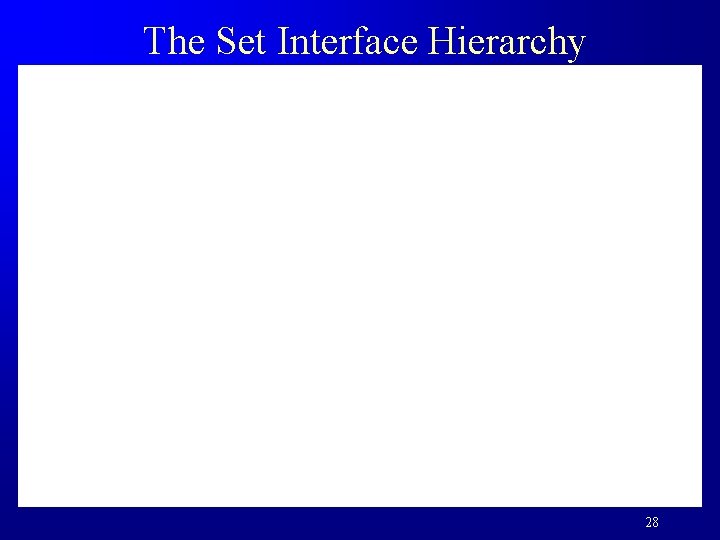
The Set Interface Hierarchy 28
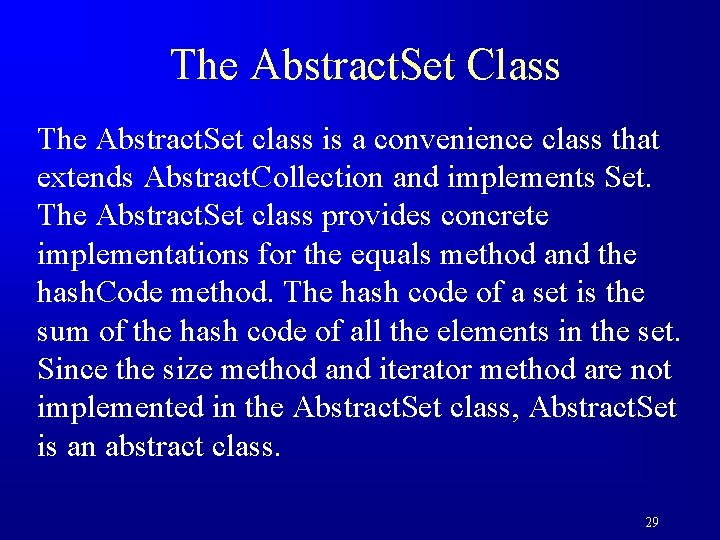
The Abstract. Set Class The Abstract. Set class is a convenience class that extends Abstract. Collection and implements Set. The Abstract. Set class provides concrete implementations for the equals method and the hash. Code method. The hash code of a set is the sum of the hash code of all the elements in the set. Since the size method and iterator method are not implemented in the Abstract. Set class, Abstract. Set is an abstract class. 29
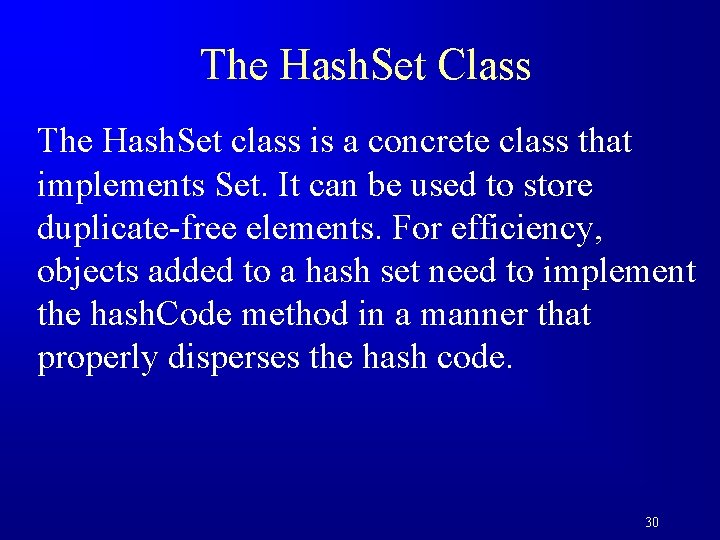
The Hash. Set Class The Hash. Set class is a concrete class that implements Set. It can be used to store duplicate-free elements. For efficiency, objects added to a hash set need to implement the hash. Code method in a manner that properly disperses the hash code. 30
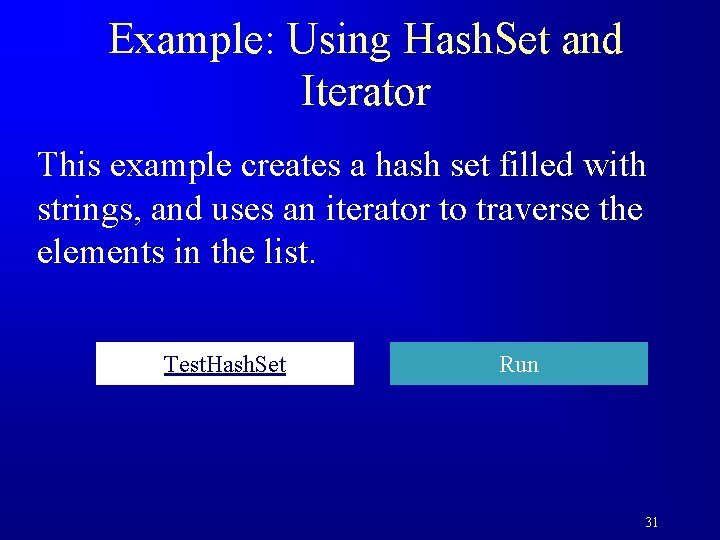
Example: Using Hash. Set and Iterator This example creates a hash set filled with strings, and uses an iterator to traverse the elements in the list. Test. Hash. Set Run 31
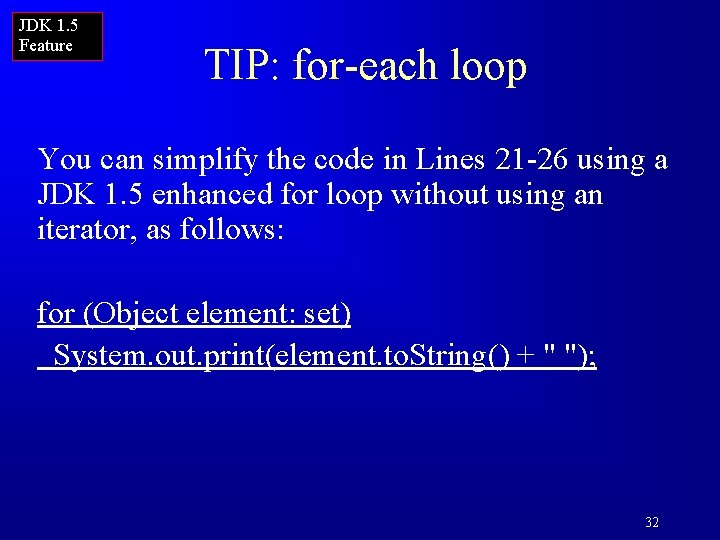
JDK 1. 5 Feature TIP: for-each loop You can simplify the code in Lines 21 -26 using a JDK 1. 5 enhanced for loop without using an iterator, as follows: for (Object element: set) System. out. print(element. to. String() + " "); 32
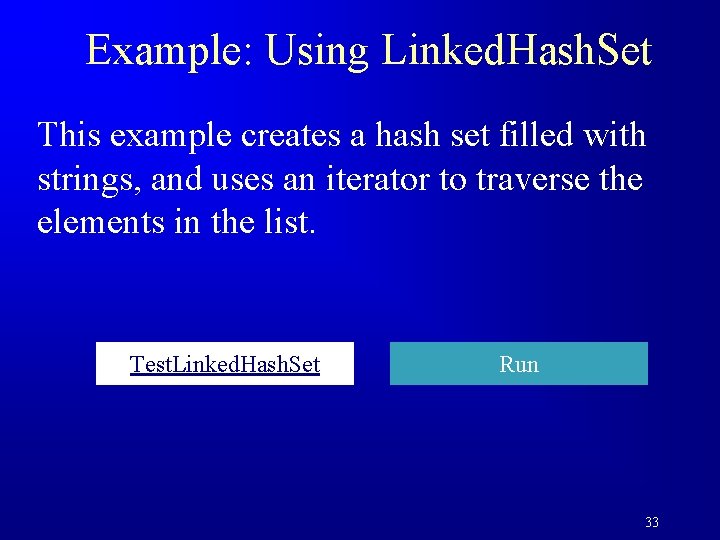
Example: Using Linked. Hash. Set This example creates a hash set filled with strings, and uses an iterator to traverse the elements in the list. Test. Linked. Hash. Set Run 33
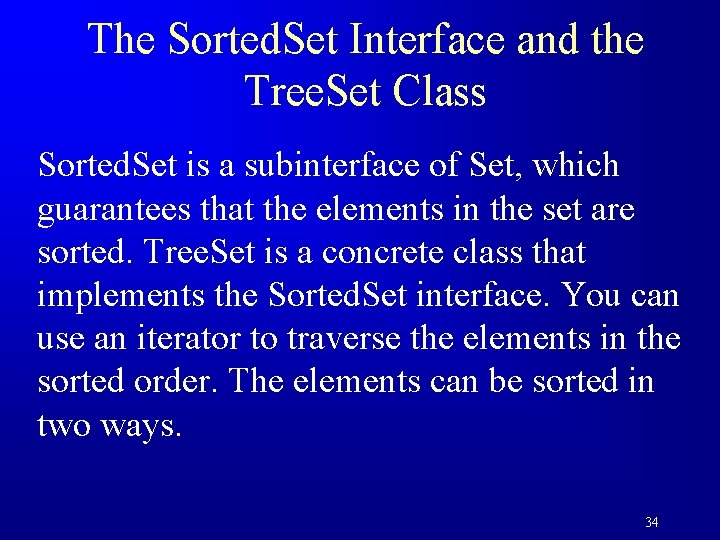
The Sorted. Set Interface and the Tree. Set Class Sorted. Set is a subinterface of Set, which guarantees that the elements in the set are sorted. Tree. Set is a concrete class that implements the Sorted. Set interface. You can use an iterator to traverse the elements in the sorted order. The elements can be sorted in two ways. 34
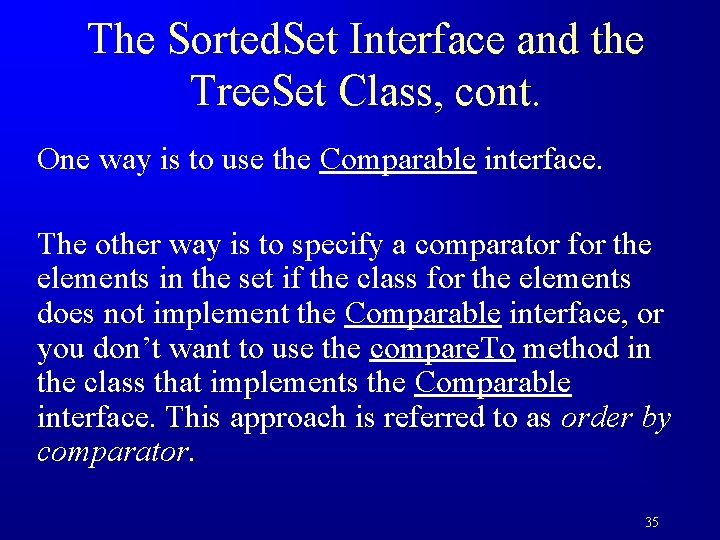
The Sorted. Set Interface and the Tree. Set Class, cont. One way is to use the Comparable interface. The other way is to specify a comparator for the elements in the set if the class for the elements does not implement the Comparable interface, or you don’t want to use the compare. To method in the class that implements the Comparable interface. This approach is referred to as order by comparator. 35
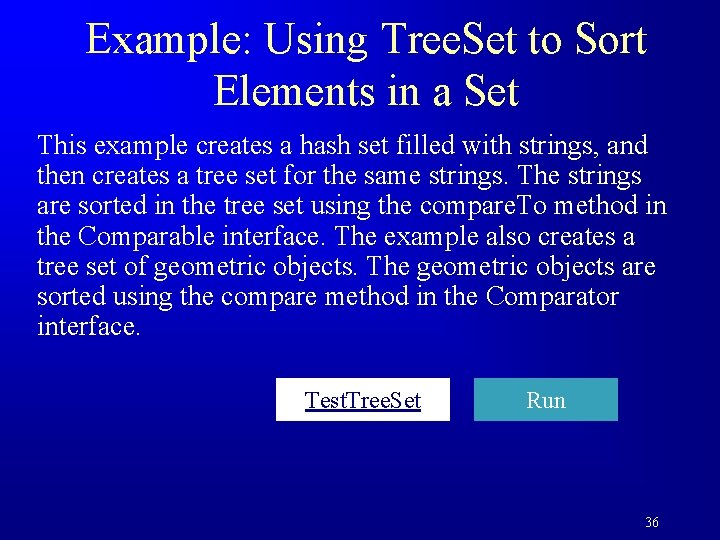
Example: Using Tree. Set to Sort Elements in a Set This example creates a hash set filled with strings, and then creates a tree set for the same strings. The strings are sorted in the tree set using the compare. To method in the Comparable interface. The example also creates a tree set of geometric objects. The geometric objects are sorted using the compare method in the Comparator interface. Test. Tree. Set Run 36
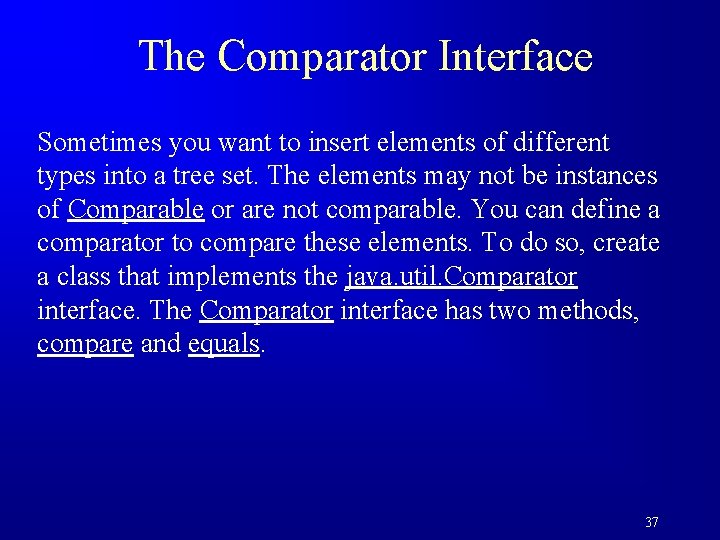
The Comparator Interface Sometimes you want to insert elements of different types into a tree set. The elements may not be instances of Comparable or are not comparable. You can define a comparator to compare these elements. To do so, create a class that implements the java. util. Comparator interface. The Comparator interface has two methods, compare and equals. 37
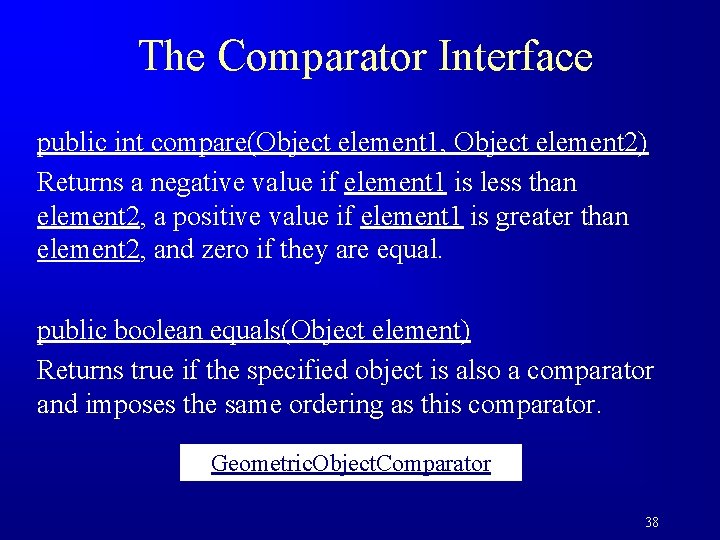
The Comparator Interface public int compare(Object element 1, Object element 2) Returns a negative value if element 1 is less than element 2, a positive value if element 1 is greater than element 2, and zero if they are equal. public boolean equals(Object element) Returns true if the specified object is also a comparator and imposes the same ordering as this comparator. Geometric. Object. Comparator 38
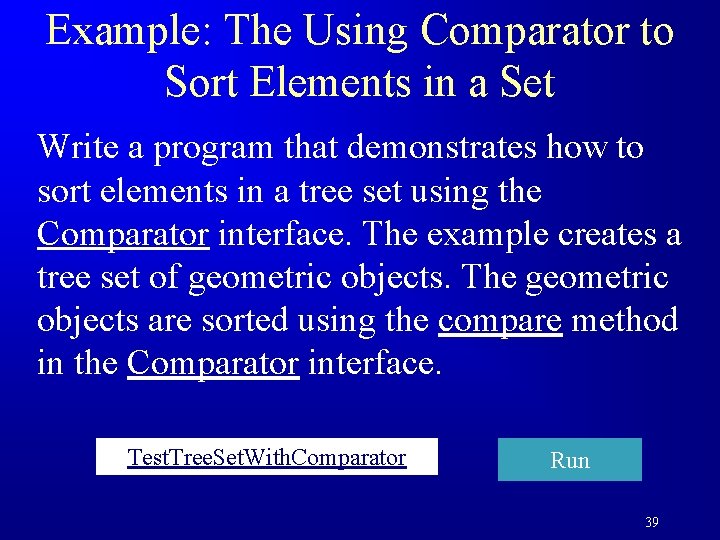
Example: The Using Comparator to Sort Elements in a Set Write a program that demonstrates how to sort elements in a tree set using the Comparator interface. The example creates a tree set of geometric objects. The geometric objects are sorted using the compare method in the Comparator interface. Test. Tree. Set. With. Comparator Run 39
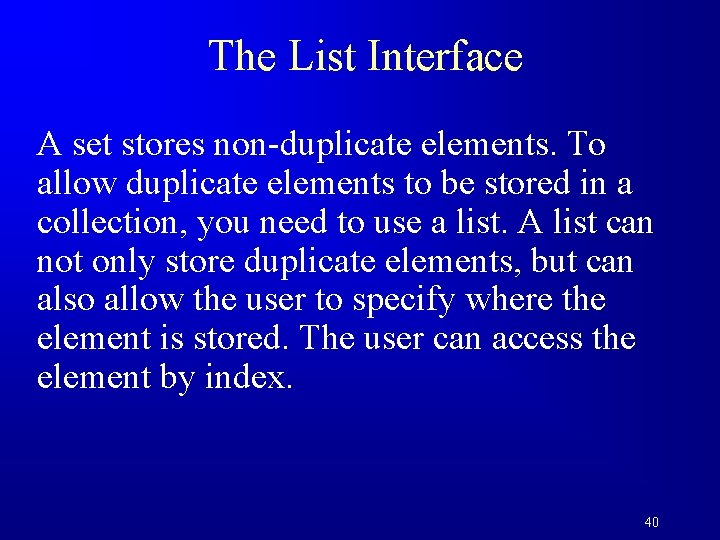
The List Interface A set stores non-duplicate elements. To allow duplicate elements to be stored in a collection, you need to use a list. A list can not only store duplicate elements, but can also allow the user to specify where the element is stored. The user can access the element by index. 40
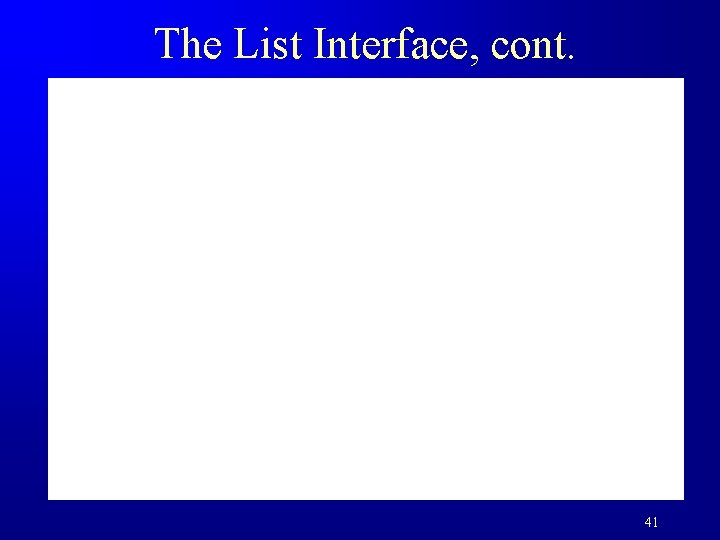
The List Interface, cont. 41
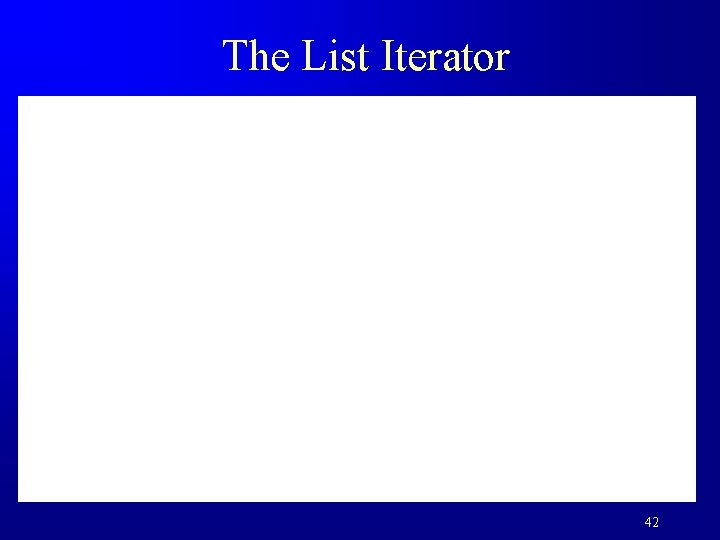
The List Iterator 42
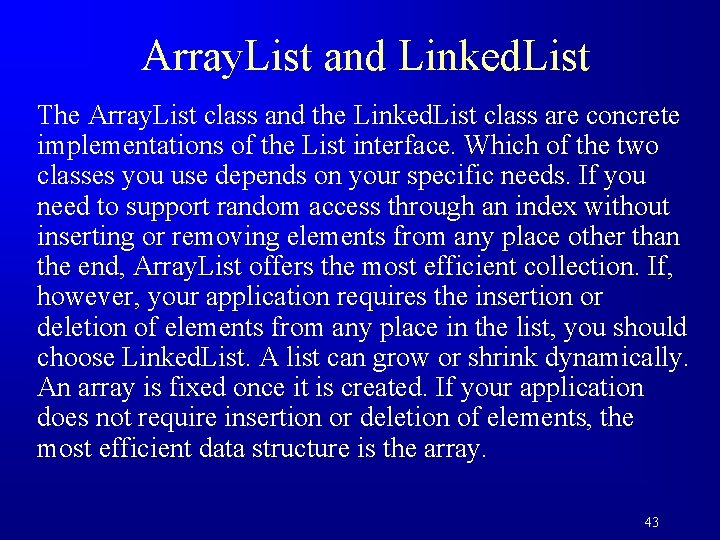
Array. List and Linked. List The Array. List class and the Linked. List class are concrete implementations of the List interface. Which of the two classes you use depends on your specific needs. If you need to support random access through an index without inserting or removing elements from any place other than the end, Array. List offers the most efficient collection. If, however, your application requires the insertion or deletion of elements from any place in the list, you should choose Linked. List. A list can grow or shrink dynamically. An array is fixed once it is created. If your application does not require insertion or deletion of elements, the most efficient data structure is the array. 43
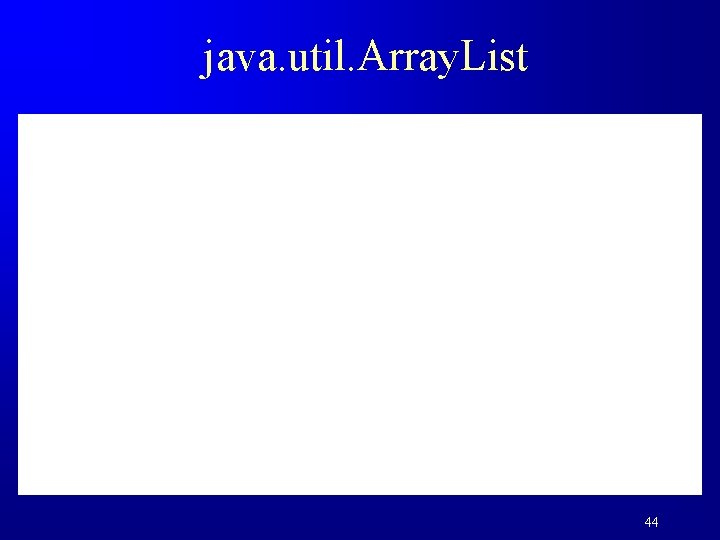
java. util. Array. List 44
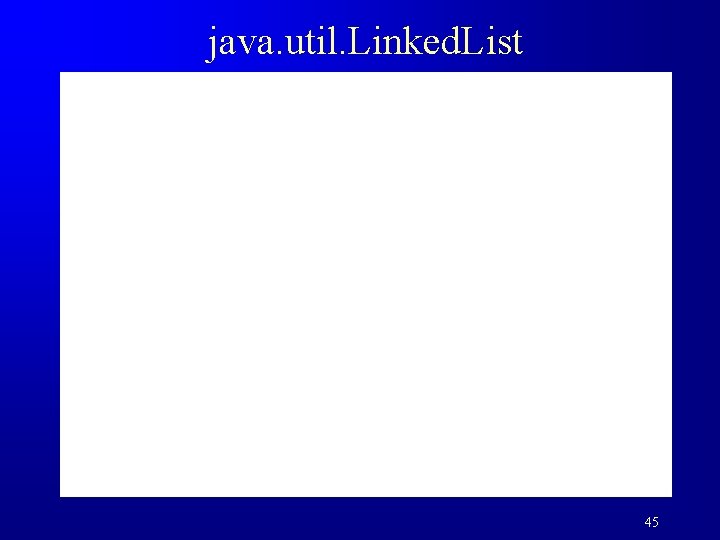
java. util. Linked. List 45
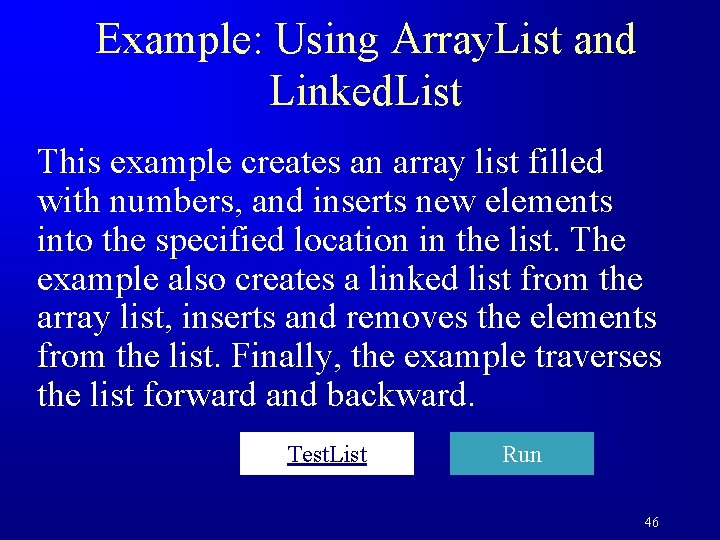
Example: Using Array. List and Linked. List This example creates an array list filled with numbers, and inserts new elements into the specified location in the list. The example also creates a linked list from the array list, inserts and removes the elements from the list. Finally, the example traverses the list forward and backward. Test. List Run 46
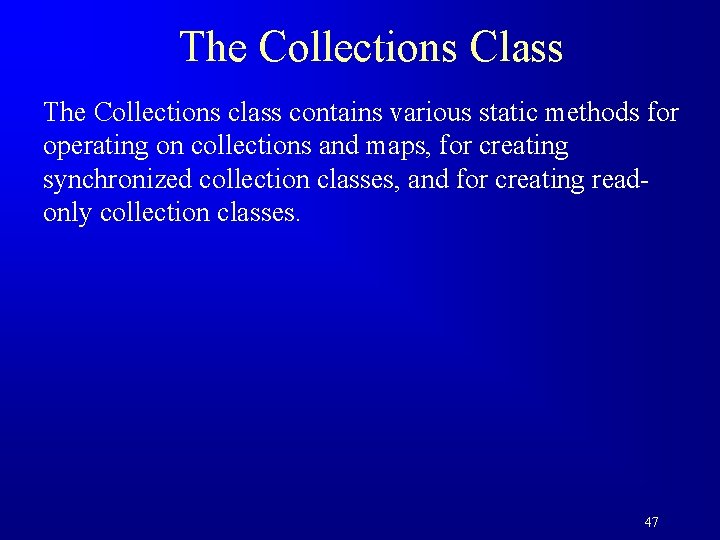
The Collections Class The Collections class contains various static methods for operating on collections and maps, for creating synchronized collection classes, and for creating readonly collection classes. 47
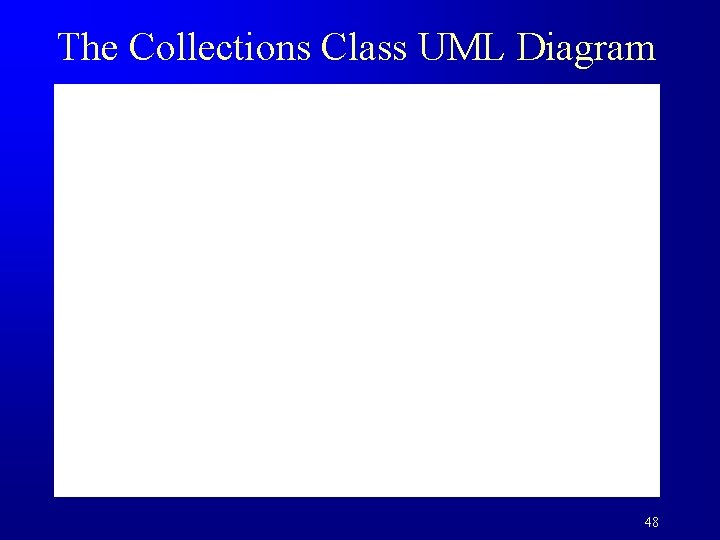
The Collections Class UML Diagram 48
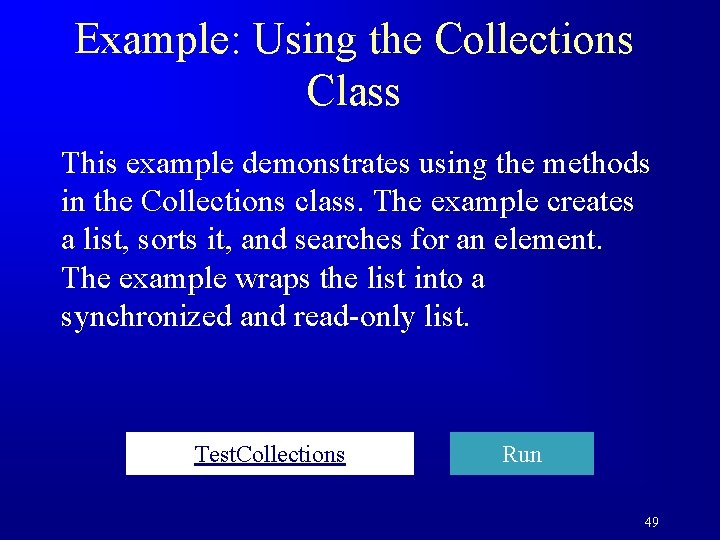
Example: Using the Collections Class This example demonstrates using the methods in the Collections class. The example creates a list, sorts it, and searches for an element. The example wraps the list into a synchronized and read-only list. Test. Collections Run 49
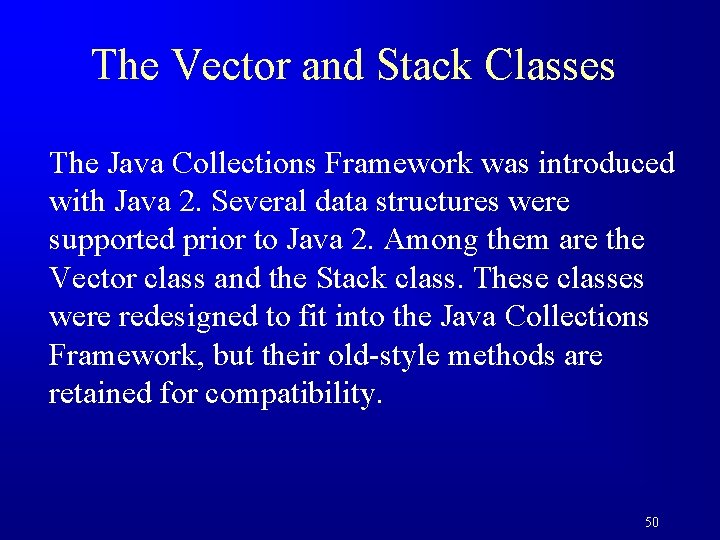
The Vector and Stack Classes The Java Collections Framework was introduced with Java 2. Several data structures were supported prior to Java 2. Among them are the Vector class and the Stack class. These classes were redesigned to fit into the Java Collections Framework, but their old-style methods are retained for compatibility. 50
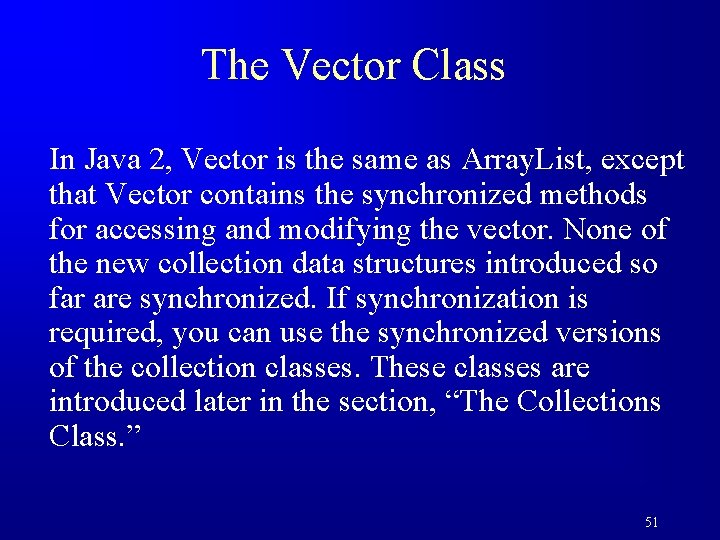
The Vector Class In Java 2, Vector is the same as Array. List, except that Vector contains the synchronized methods for accessing and modifying the vector. None of the new collection data structures introduced so far are synchronized. If synchronization is required, you can use the synchronized versions of the collection classes. These classes are introduced later in the section, “The Collections Class. ” 51
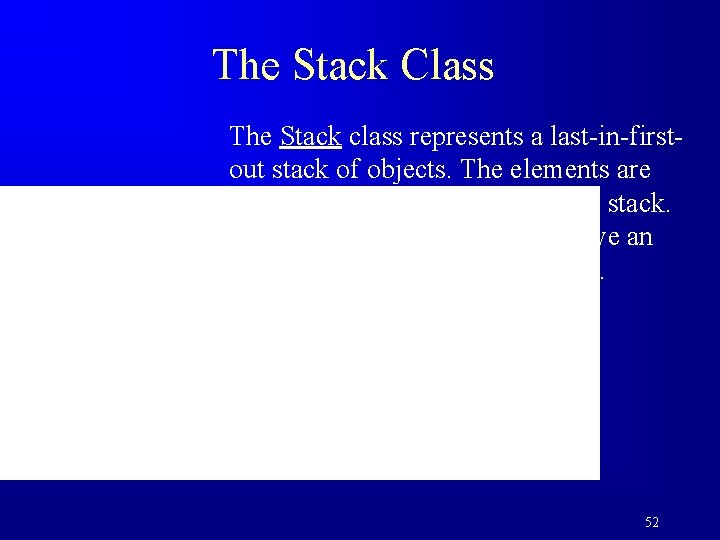
The Stack Class The Stack class represents a last-in-firstout stack of objects. The elements are accessed only from the top of the stack. You can retrieve, insert, or remove an element from the top of the stack. 52
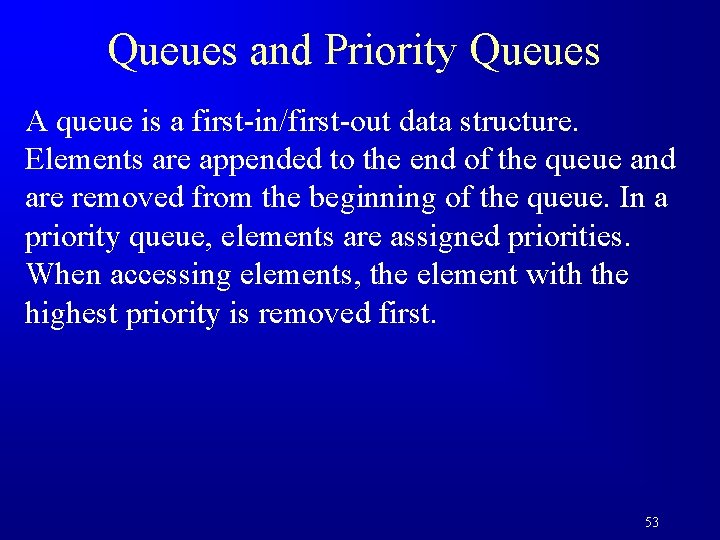
Queues and Priority Queues A queue is a first-in/first-out data structure. Elements are appended to the end of the queue and are removed from the beginning of the queue. In a priority queue, elements are assigned priorities. When accessing elements, the element with the highest priority is removed first. 53
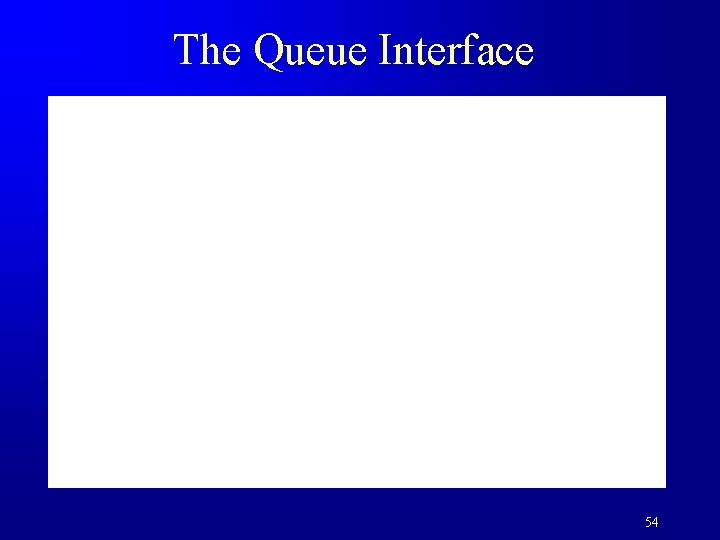
The Queue Interface 54
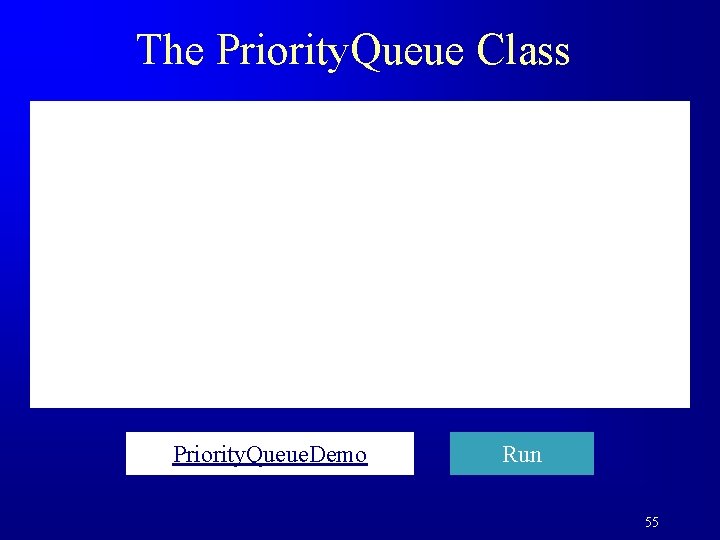
The Priority. Queue Class Priority. Queue. Demo Run 55
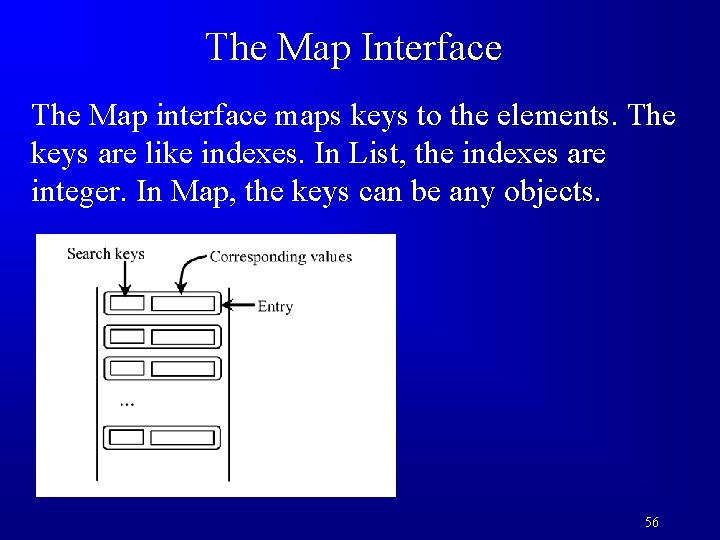
The Map Interface The Map interface maps keys to the elements. The keys are like indexes. In List, the indexes are integer. In Map, the keys can be any objects. 56
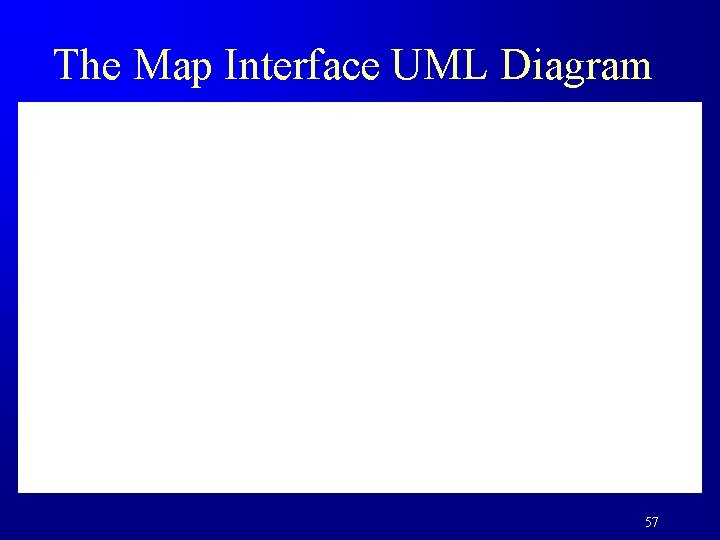
The Map Interface UML Diagram 57
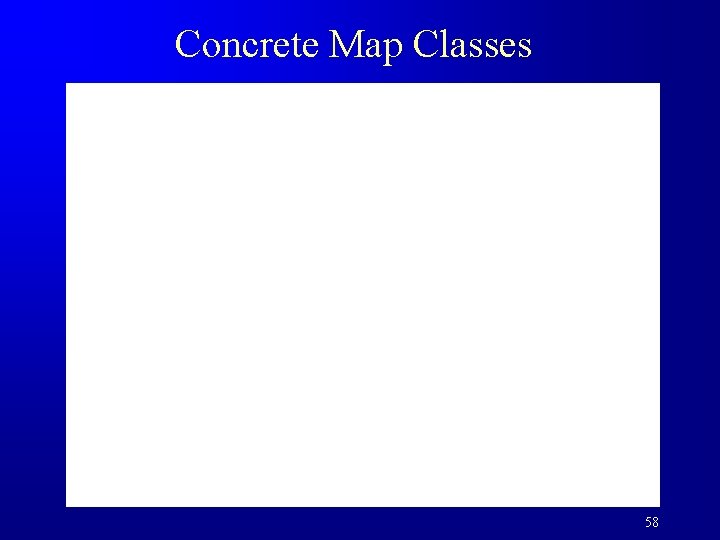
Concrete Map Classes 58
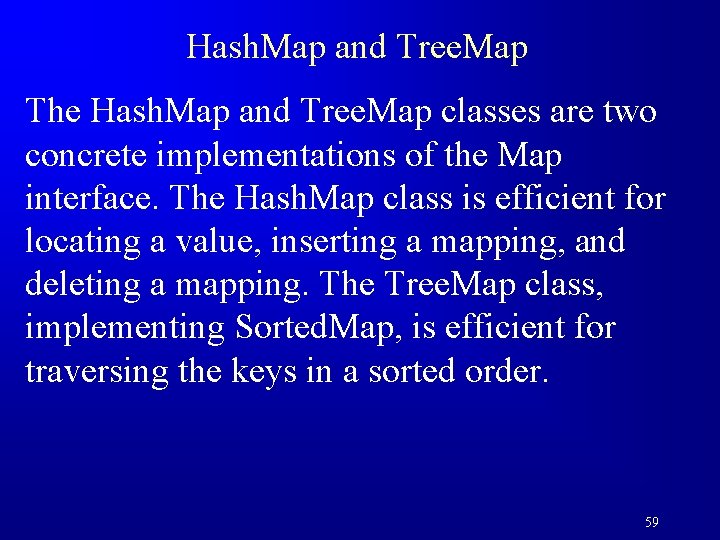
Hash. Map and Tree. Map The Hash. Map and Tree. Map classes are two concrete implementations of the Map interface. The Hash. Map class is efficient for locating a value, inserting a mapping, and deleting a mapping. The Tree. Map class, implementing Sorted. Map, is efficient for traversing the keys in a sorted order. 59
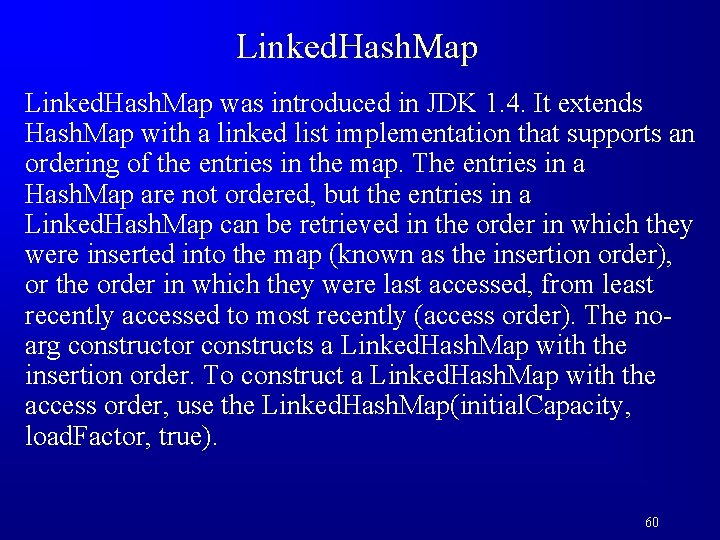
Linked. Hash. Map was introduced in JDK 1. 4. It extends Hash. Map with a linked list implementation that supports an ordering of the entries in the map. The entries in a Hash. Map are not ordered, but the entries in a Linked. Hash. Map can be retrieved in the order in which they were inserted into the map (known as the insertion order), or the order in which they were last accessed, from least recently accessed to most recently (access order). The noarg constructor constructs a Linked. Hash. Map with the insertion order. To construct a Linked. Hash. Map with the access order, use the Linked. Hash. Map(initial. Capacity, load. Factor, true). 60
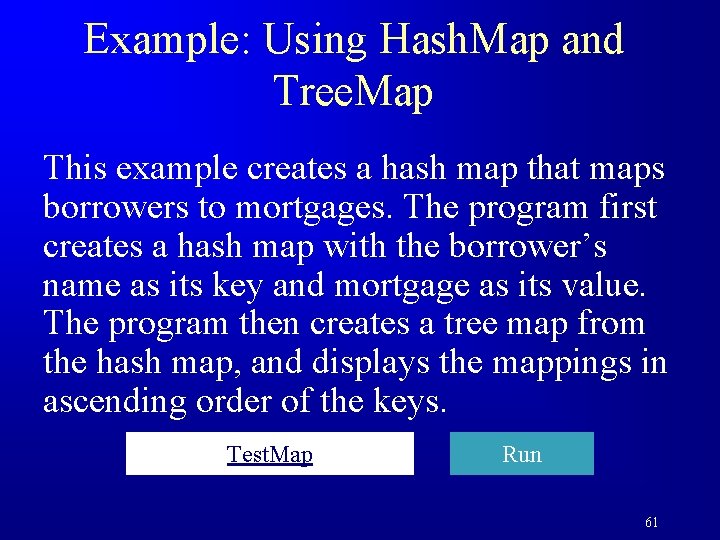
Example: Using Hash. Map and Tree. Map This example creates a hash map that maps borrowers to mortgages. The program first creates a hash map with the borrower’s name as its key and mortgage as its value. The program then creates a tree map from the hash map, and displays the mappings in ascending order of the keys. Test. Map Run 61
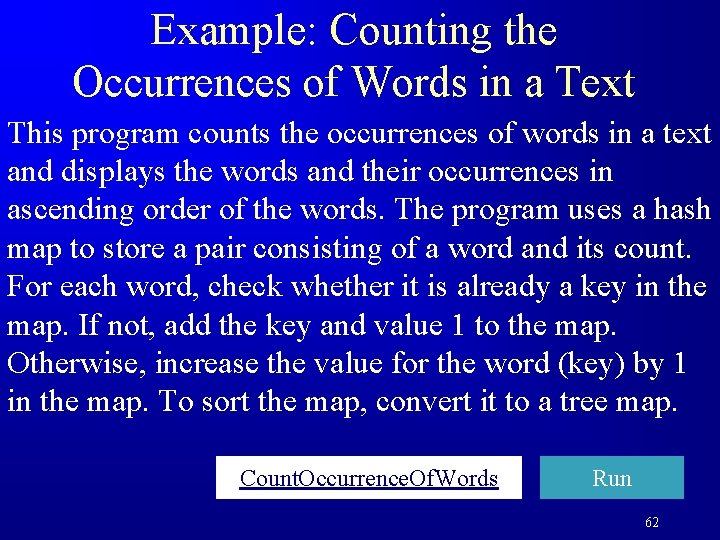
Example: Counting the Occurrences of Words in a Text This program counts the occurrences of words in a text and displays the words and their occurrences in ascending order of the words. The program uses a hash map to store a pair consisting of a word and its count. For each word, check whether it is already a key in the map. If not, add the key and value 1 to the map. Otherwise, increase the value for the word (key) by 1 in the map. To sort the map, convert it to a tree map. Count. Occurrence. Of. Words Run 62
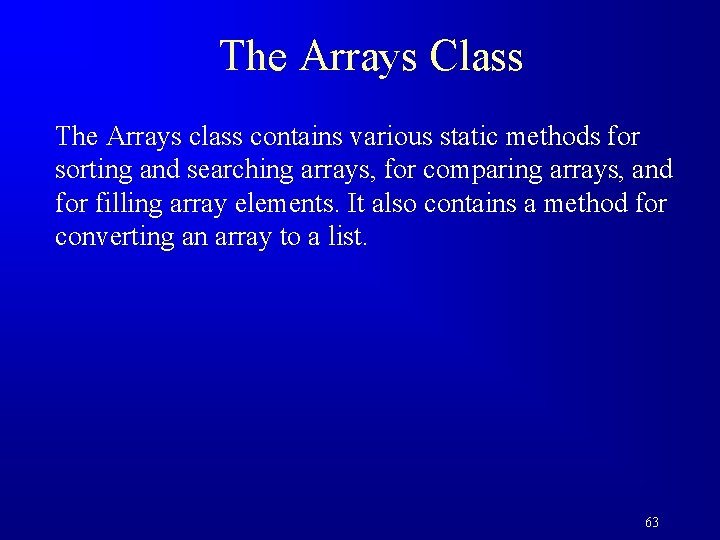
The Arrays Class The Arrays class contains various static methods for sorting and searching arrays, for comparing arrays, and for filling array elements. It also contains a method for converting an array to a list. 63
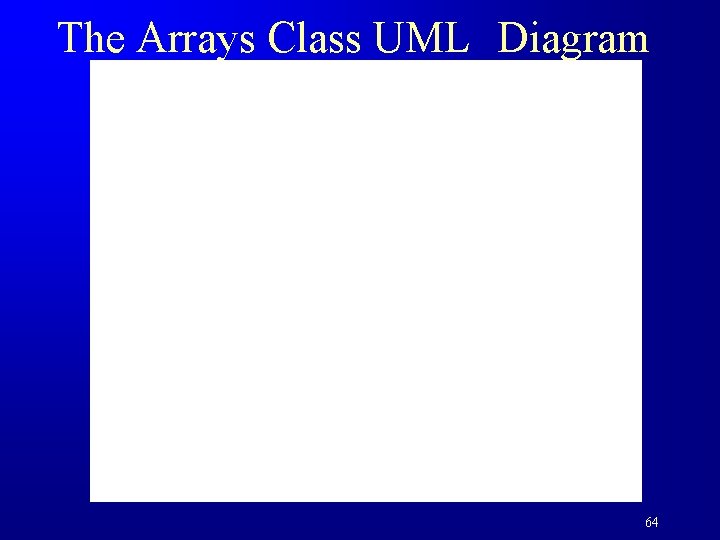
The Arrays Class UML Diagram 64
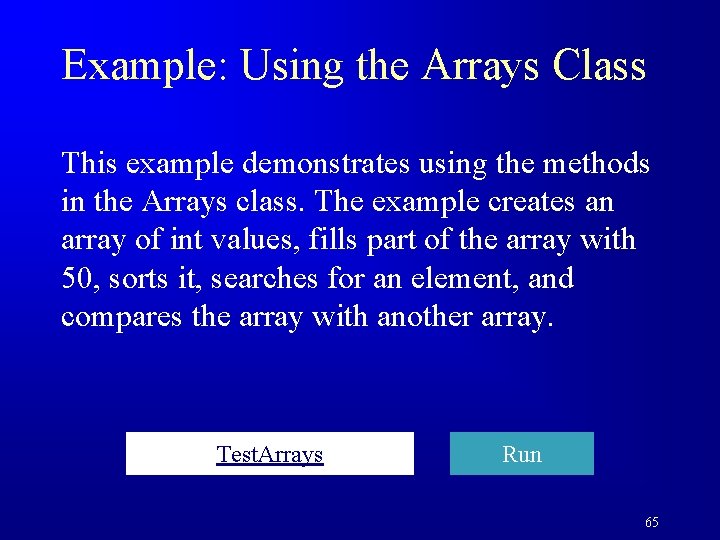
Example: Using the Arrays Class This example demonstrates using the methods in the Arrays class. The example creates an array of int values, fills part of the array with 50, sorts it, searches for an element, and compares the array with another array. Test. Arrays Run 65
Get in get on get off get out
Songs with literary devices
Why why why why
Java generics array
Generics java erklärung
Benefits of generics in java
Java generics array
System collections generic
Hospitality generics
Kotlinx serialization generics
C generics
Why willy why do you cry
Hooray cheered gilda. does that mean we can stay
Laura mulvey male gaze
Hey hey you you get off of my cloud
Conditionals 1 2
Rapporteren voorbeeld
What do you get when you cross darth vader with an elephant
Hey hey you you get off of my cloud
Get up get moving quiz
Get up get moving quiz
Get up get moving quiz
Sequence in pseudocode
Get focused get results
Get up get moving quiz
Dont ask why why why
If i could only teach you one thing
If you could be invisible what would you do and why?
Chapter 11 lightning thief
Knot dog
Why did jack want samneric to get him a coconut
Essential questions for the giver
Mercutio last words
Why does juliet kiss romeo’s lips?
Wnhen
You say that you love rain
Agree or disagree questions about life
If you think you can you can poem
Tell me what you eat and i shall tell you what you are
Will follow you wherever you ...........................
Theoretical yield
Who or what decides what you get?
William parry stuttering
Pervent yield
Stay ready so you don't have to get ready
Walmart protected pto 2021
In a pizza restaurant you get a basic pizza
Log(x+y)
What is the highest mark you can get on a foundation paper
How do you usually get to school
What is an error interval
When do you usually get up
Where did you get that hat
How to gain your parents trust back after lying
How do you get density
Mla format for google docs
If you get home the first
My son ___ be home by now. where can he be?
I'm excited to get to know you
You often get up at six thirty
Example of modal verbs
Did you get the note
Get to know you questions for adults
You measure the flame of a candle and get a reading of
What is sepsis disease
Bart simpson tin foil hat