Casts What are they Why do we need
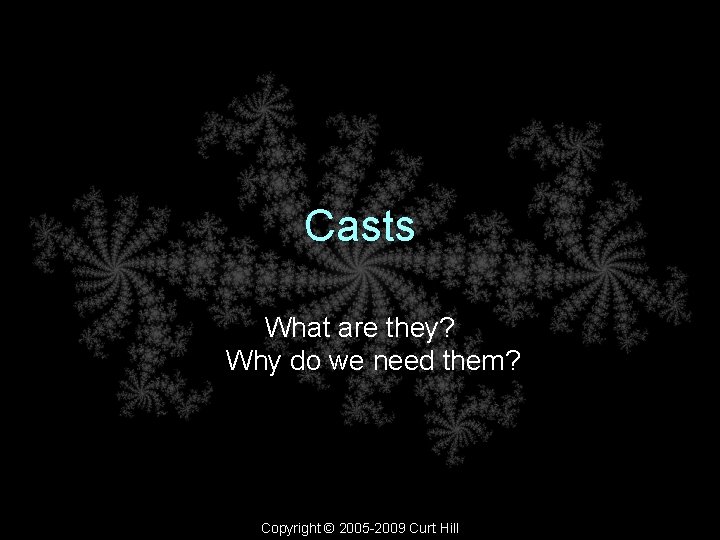
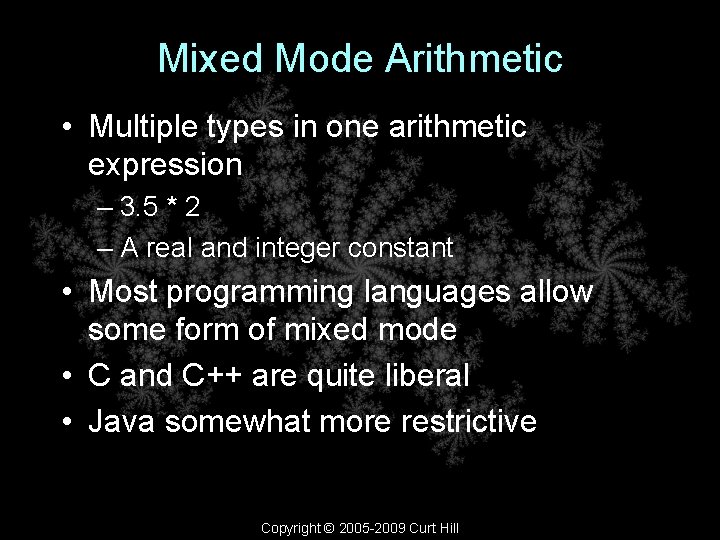
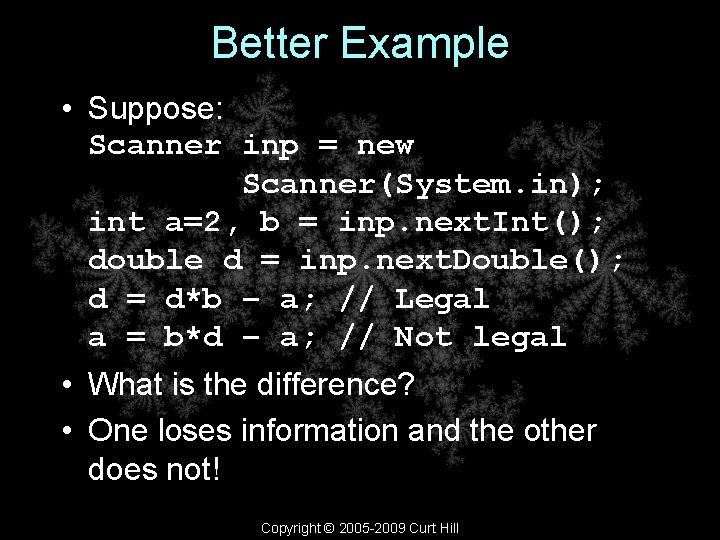
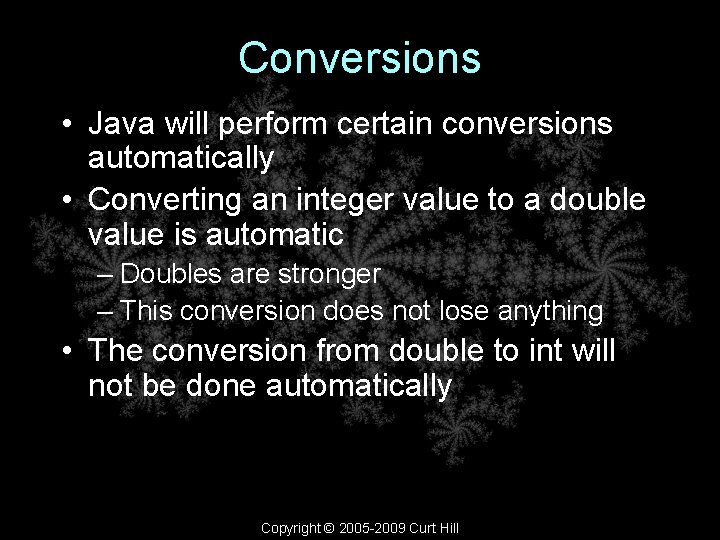
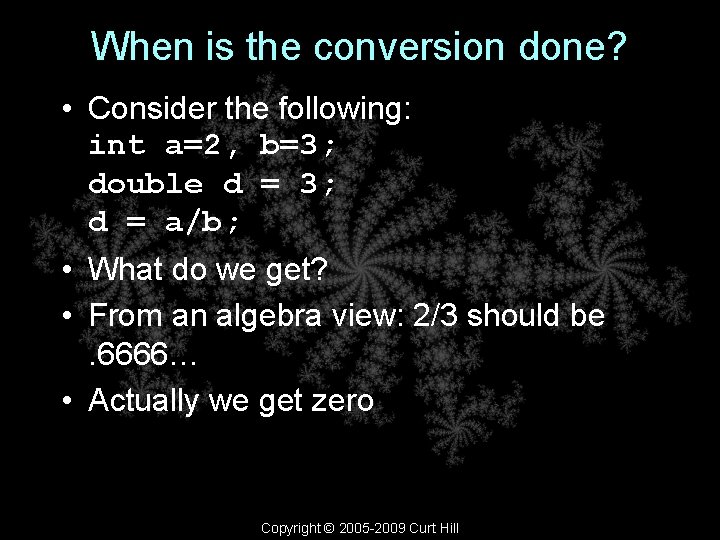
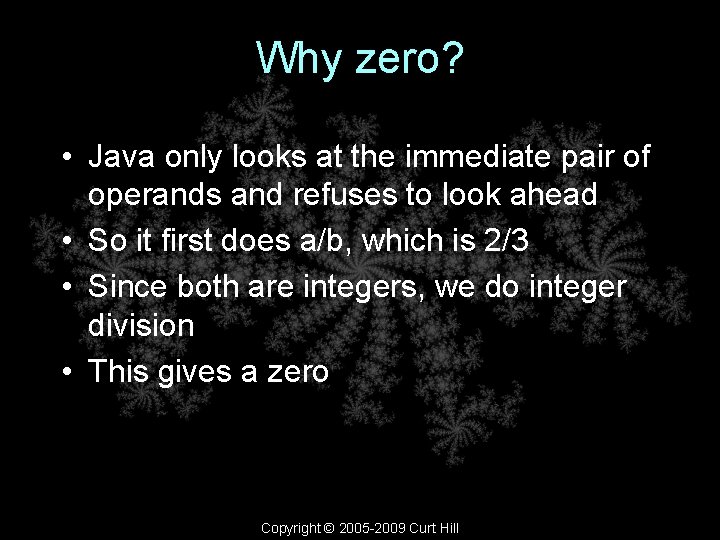
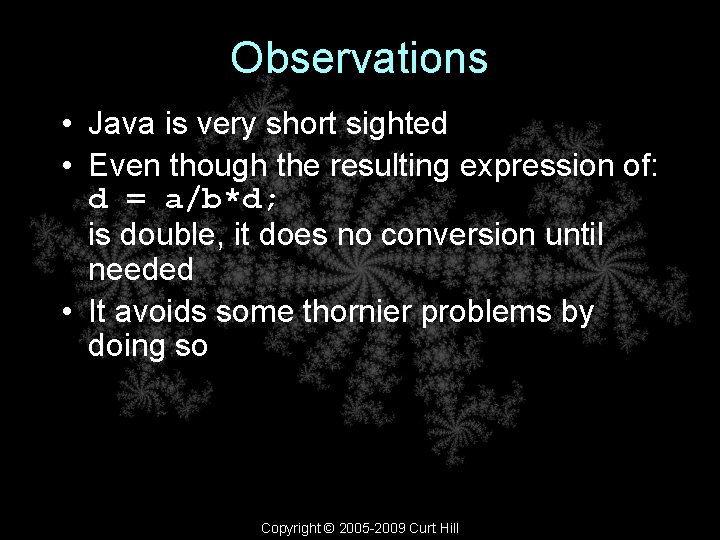
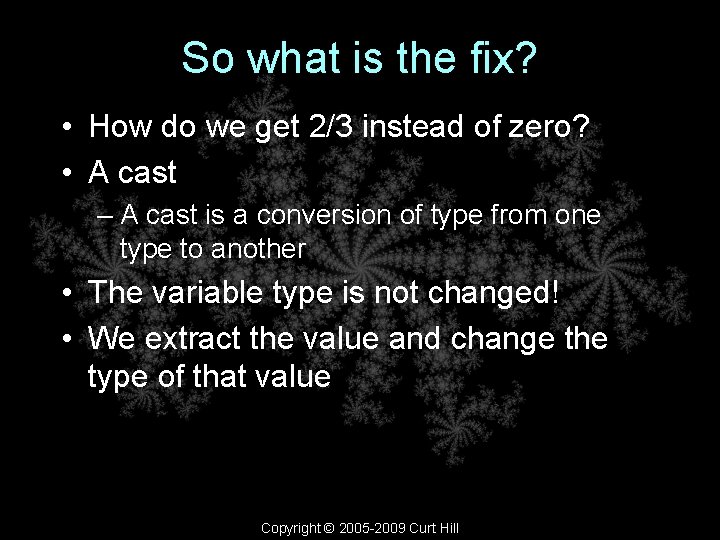
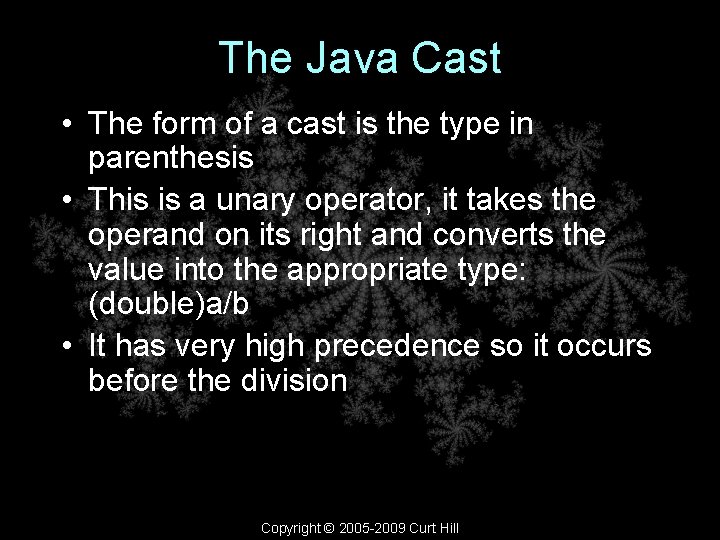
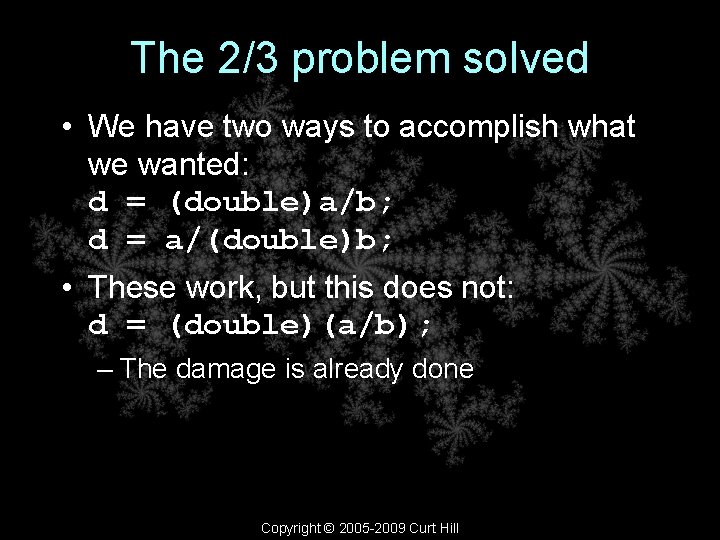
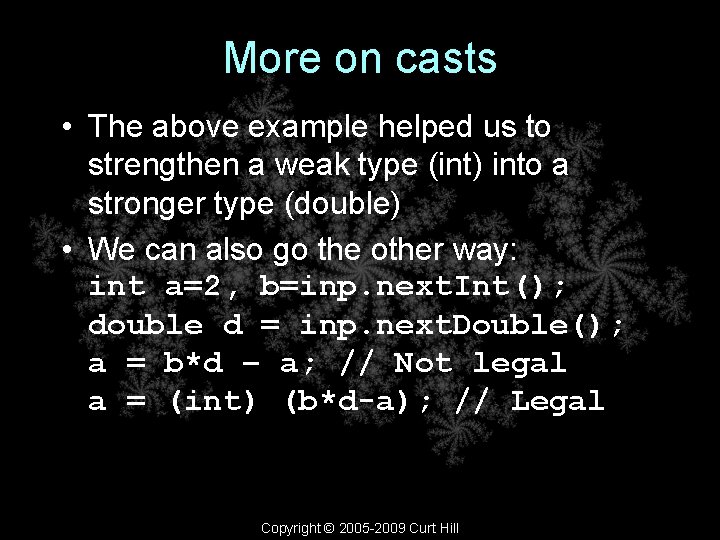
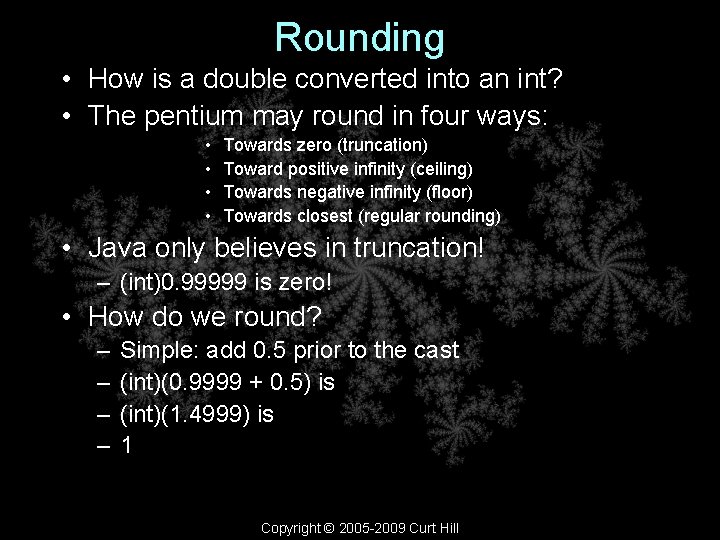
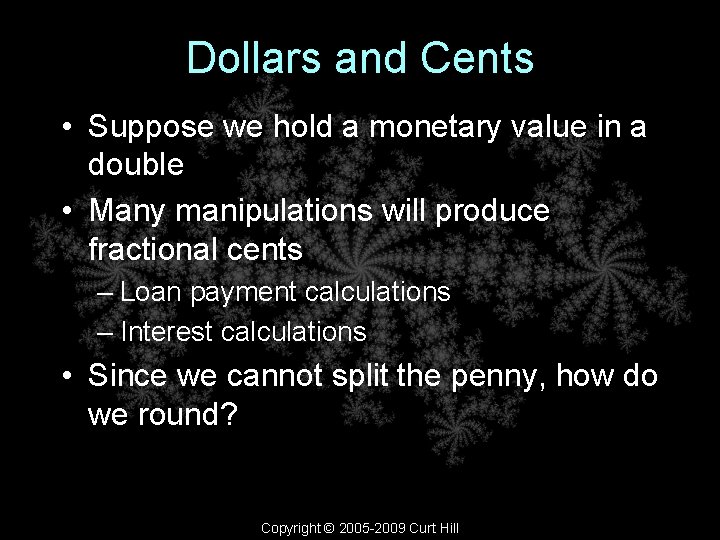
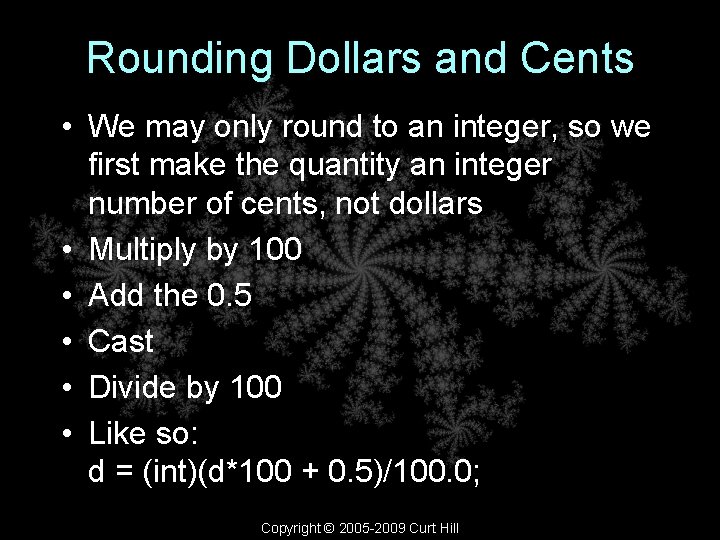
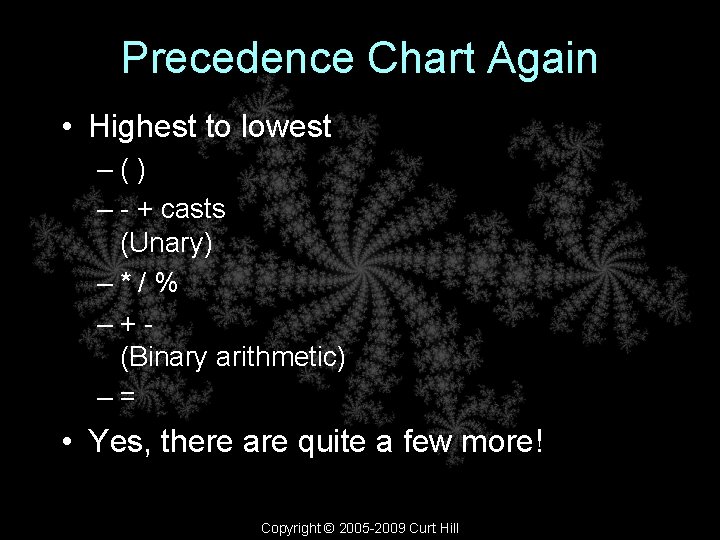
- Slides: 15
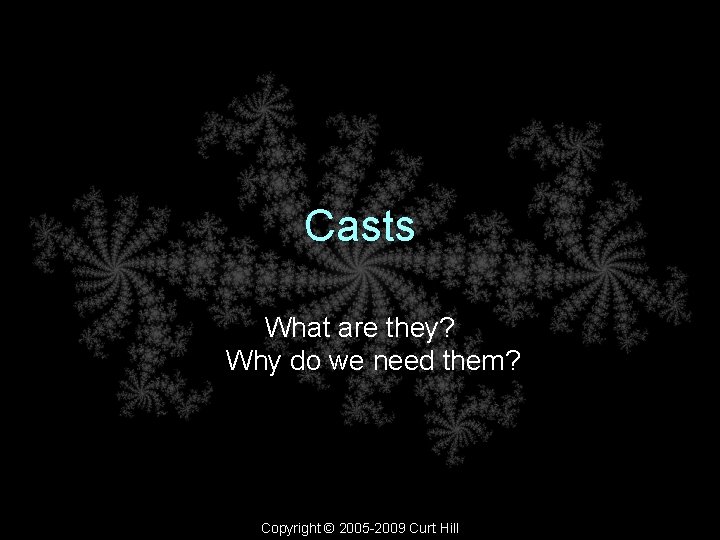
Casts What are they? Why do we need them? Copyright © 2005 -2009 Curt Hill
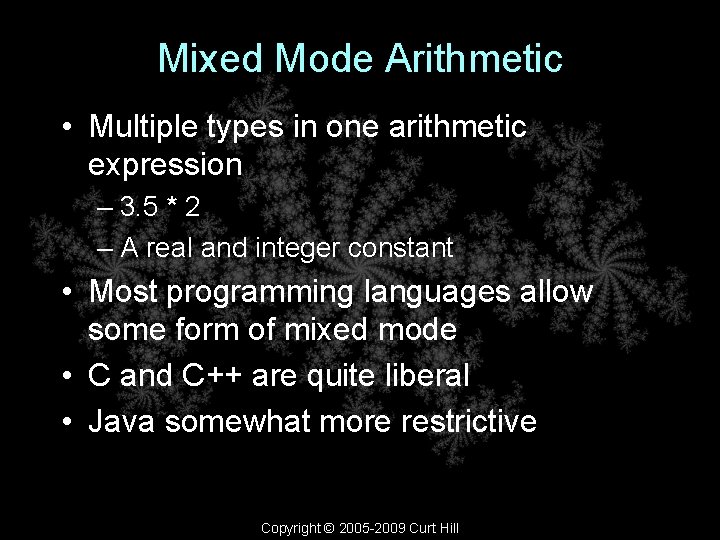
Mixed Mode Arithmetic • Multiple types in one arithmetic expression – 3. 5 * 2 – A real and integer constant • Most programming languages allow some form of mixed mode • C and C++ are quite liberal • Java somewhat more restrictive Copyright © 2005 -2009 Curt Hill
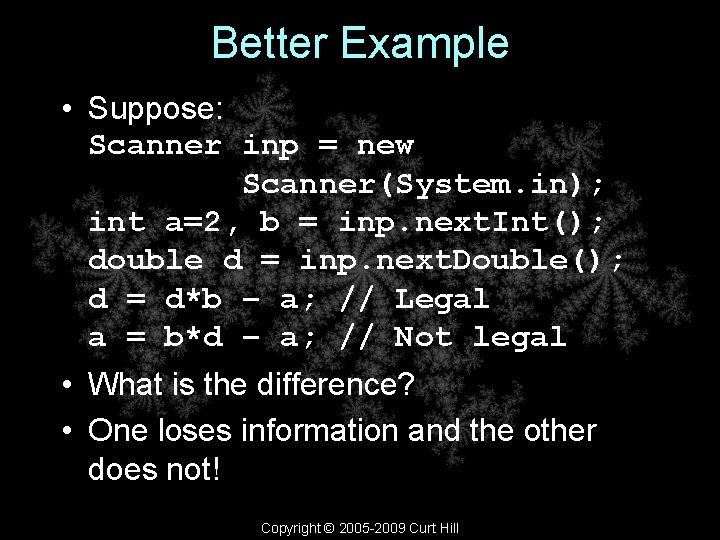
Better Example • Suppose: Scanner inp = new Scanner(System. in); int a=2, b = inp. next. Int(); double d = inp. next. Double(); d = d*b – a; // Legal a = b*d – a; // Not legal • What is the difference? • One loses information and the other does not! Copyright © 2005 -2009 Curt Hill
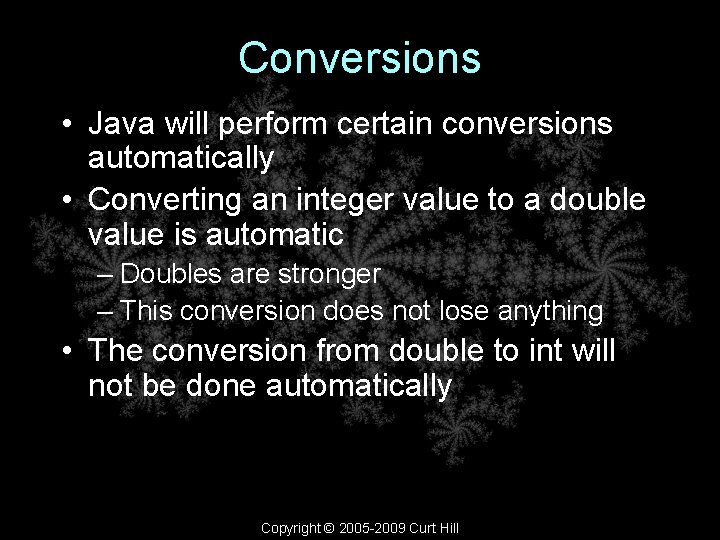
Conversions • Java will perform certain conversions automatically • Converting an integer value to a double value is automatic – Doubles are stronger – This conversion does not lose anything • The conversion from double to int will not be done automatically Copyright © 2005 -2009 Curt Hill
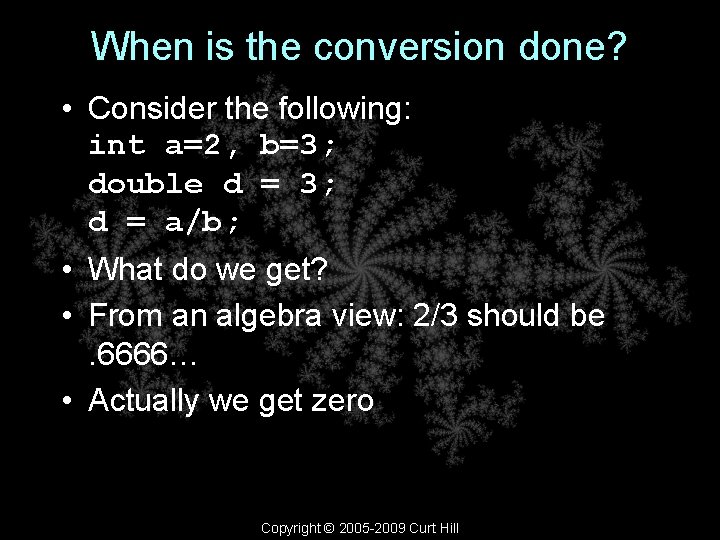
When is the conversion done? • Consider the following: int a=2, b=3; double d = 3; d = a/b; • What do we get? • From an algebra view: 2/3 should be. 6666… • Actually we get zero Copyright © 2005 -2009 Curt Hill
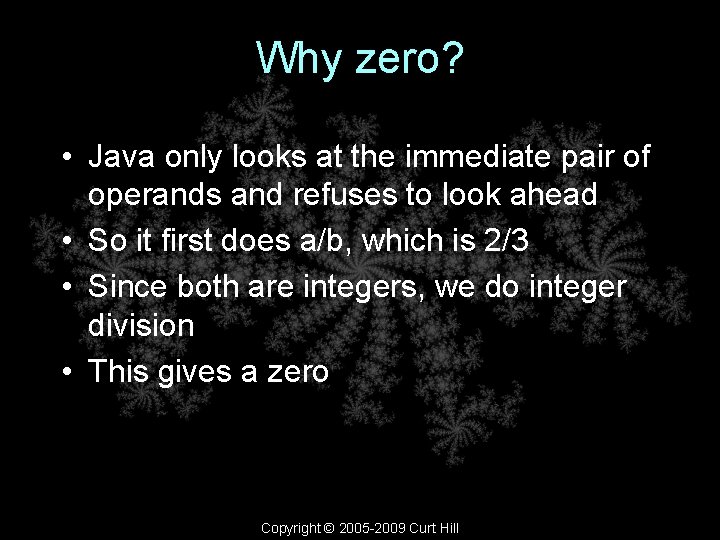
Why zero? • Java only looks at the immediate pair of operands and refuses to look ahead • So it first does a/b, which is 2/3 • Since both are integers, we do integer division • This gives a zero Copyright © 2005 -2009 Curt Hill
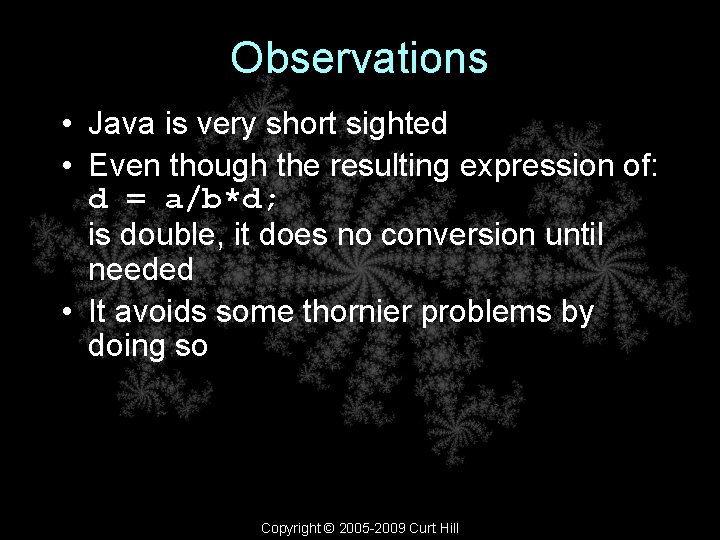
Observations • Java is very short sighted • Even though the resulting expression of: d = a/b*d; is double, it does no conversion until needed • It avoids some thornier problems by doing so Copyright © 2005 -2009 Curt Hill
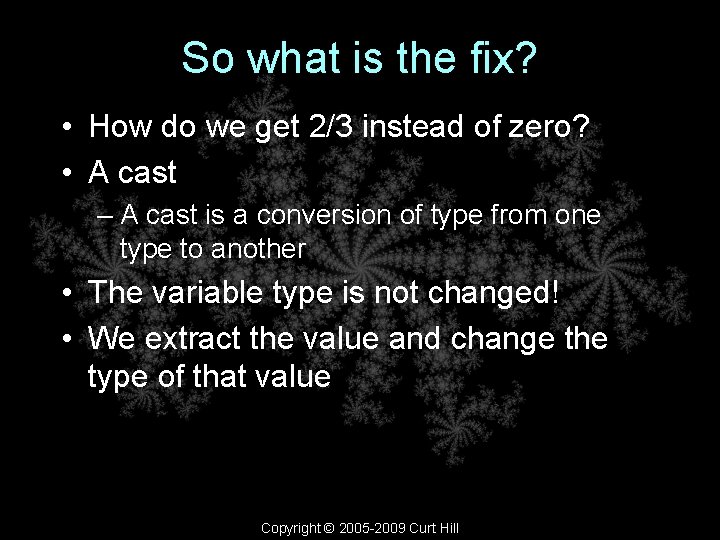
So what is the fix? • How do we get 2/3 instead of zero? • A cast – A cast is a conversion of type from one type to another • The variable type is not changed! • We extract the value and change the type of that value Copyright © 2005 -2009 Curt Hill
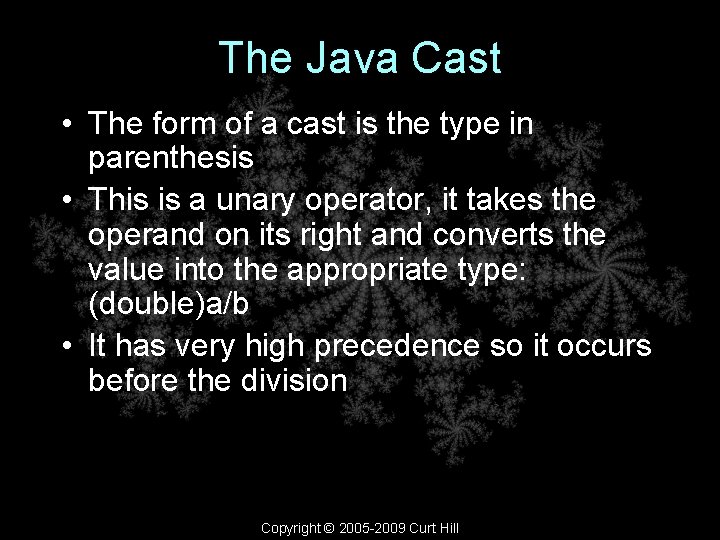
The Java Cast • The form of a cast is the type in parenthesis • This is a unary operator, it takes the operand on its right and converts the value into the appropriate type: (double)a/b • It has very high precedence so it occurs before the division Copyright © 2005 -2009 Curt Hill
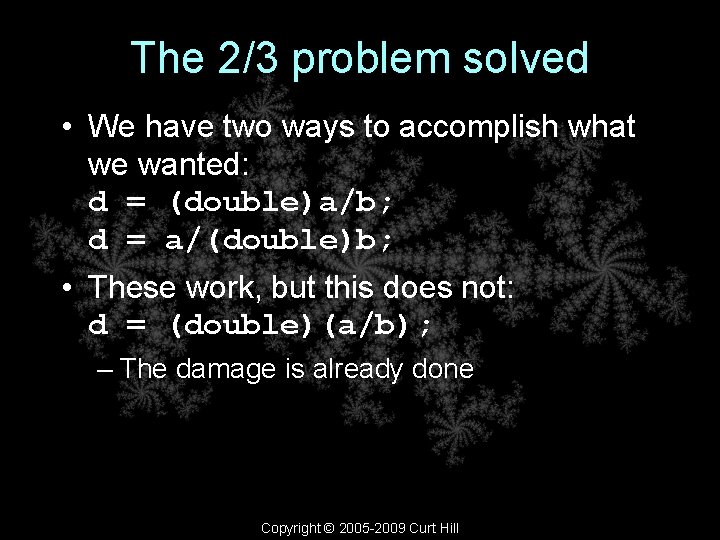
The 2/3 problem solved • We have two ways to accomplish what we wanted: d = (double)a/b; d = a/(double)b; • These work, but this does not: d = (double)(a/b); – The damage is already done Copyright © 2005 -2009 Curt Hill
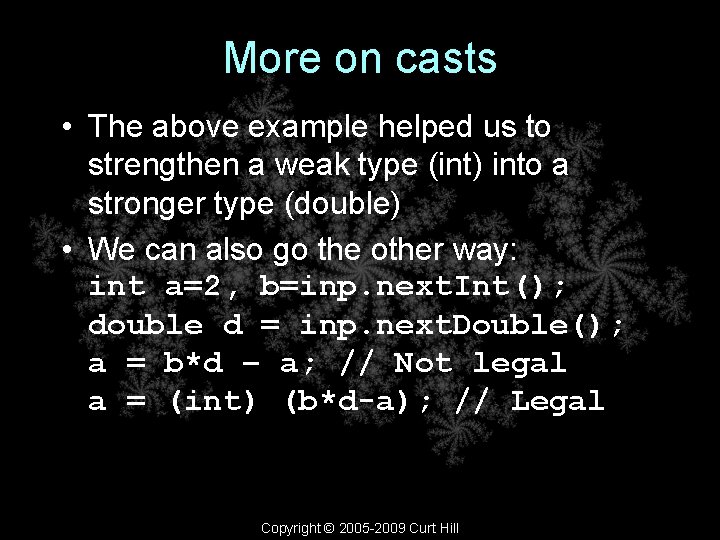
More on casts • The above example helped us to strengthen a weak type (int) into a stronger type (double) • We can also go the other way: int a=2, b=inp. next. Int(); double d = inp. next. Double(); a = b*d – a; // Not legal a = (int) (b*d-a); // Legal Copyright © 2005 -2009 Curt Hill
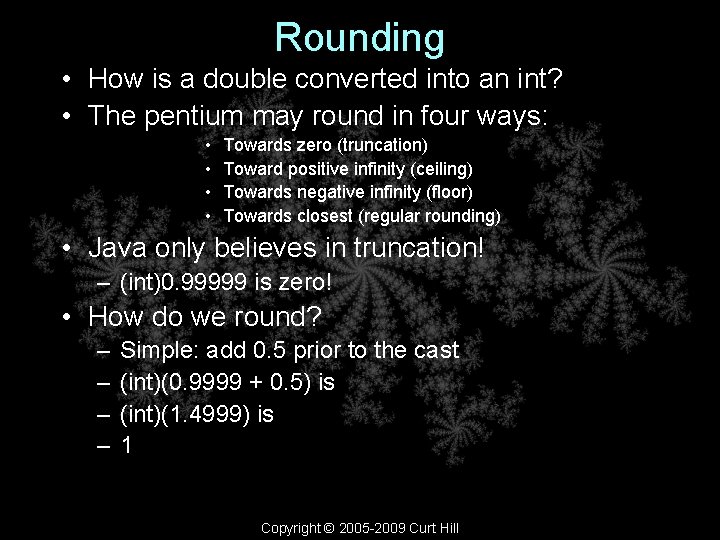
Rounding • How is a double converted into an int? • The pentium may round in four ways: • • Towards zero (truncation) Toward positive infinity (ceiling) Towards negative infinity (floor) Towards closest (regular rounding) • Java only believes in truncation! – (int)0. 99999 is zero! • How do we round? – – Simple: add 0. 5 prior to the cast (int)(0. 9999 + 0. 5) is (int)(1. 4999) is 1 Copyright © 2005 -2009 Curt Hill
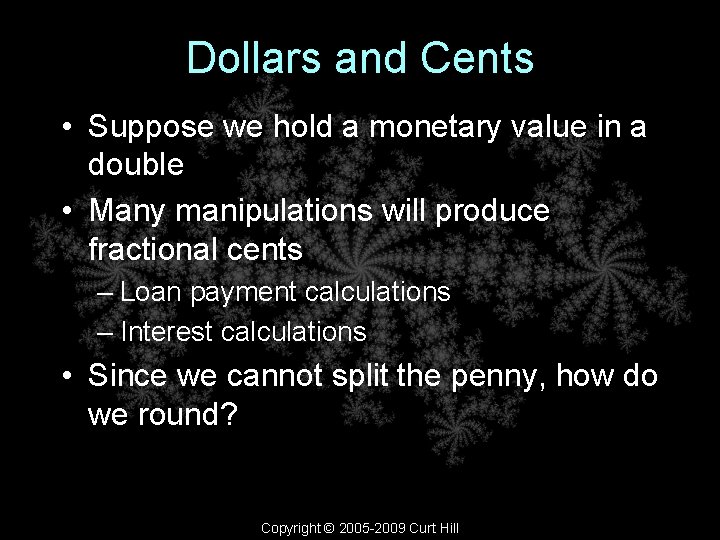
Dollars and Cents • Suppose we hold a monetary value in a double • Many manipulations will produce fractional cents – Loan payment calculations – Interest calculations • Since we cannot split the penny, how do we round? Copyright © 2005 -2009 Curt Hill
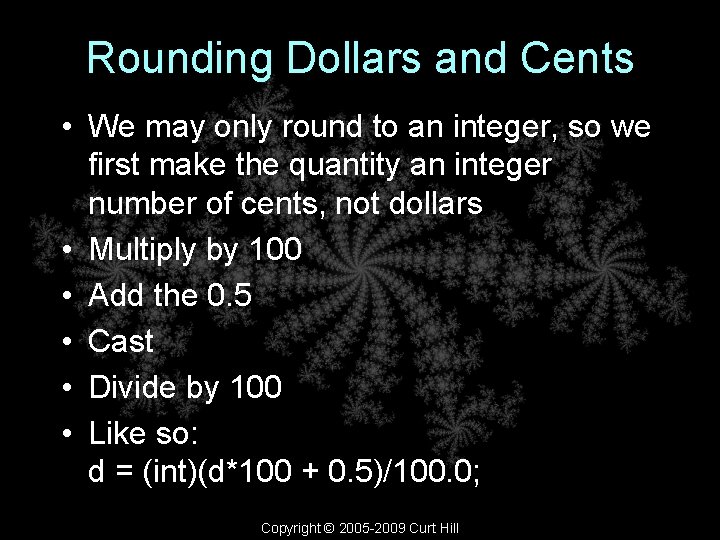
Rounding Dollars and Cents • We may only round to an integer, so we first make the quantity an integer number of cents, not dollars • Multiply by 100 • Add the 0. 5 • Cast • Divide by 100 • Like so: d = (int)(d*100 + 0. 5)/100. 0; Copyright © 2005 -2009 Curt Hill
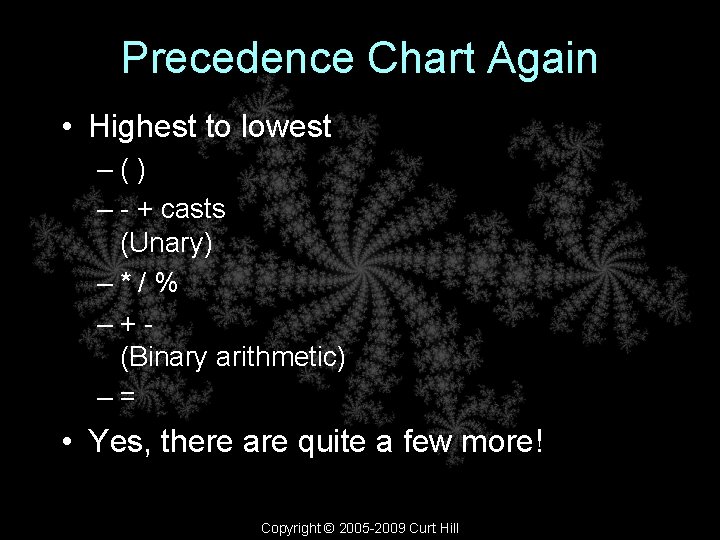
Precedence Chart Again • Highest to lowest –() – - + casts (Unary) –*/% –+(Binary arithmetic) –= • Yes, there are quite a few more! Copyright © 2005 -2009 Curt Hill
Mikael ferm
Pictures
Hyaline casts in urine
Centrifuged
Flute casts
The chestnut casts his flambeaux
Minerva cast ortho
Values of trigonometric ratios
Urinary
Ua hyaline casts/lpf qn
Urine cast
Theres no fear in love
Horizontal
Right triangle trigonometry solving word problems
Don't ask why why why
How do plants get nitrogen