Casts What are they Why do we need
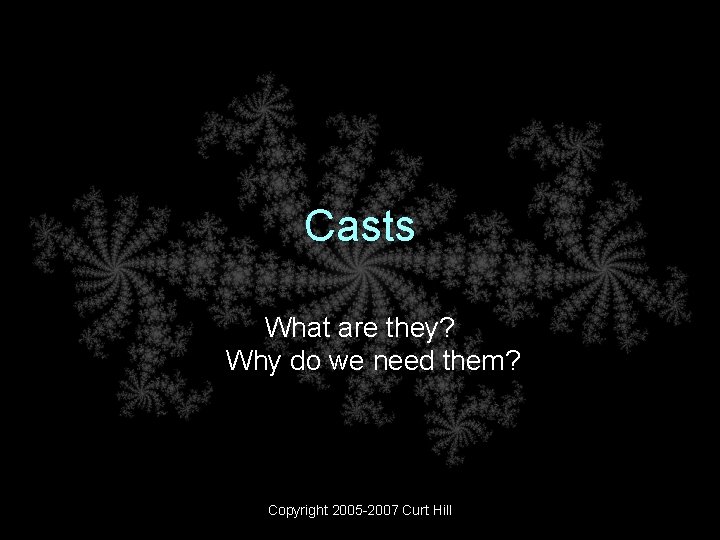
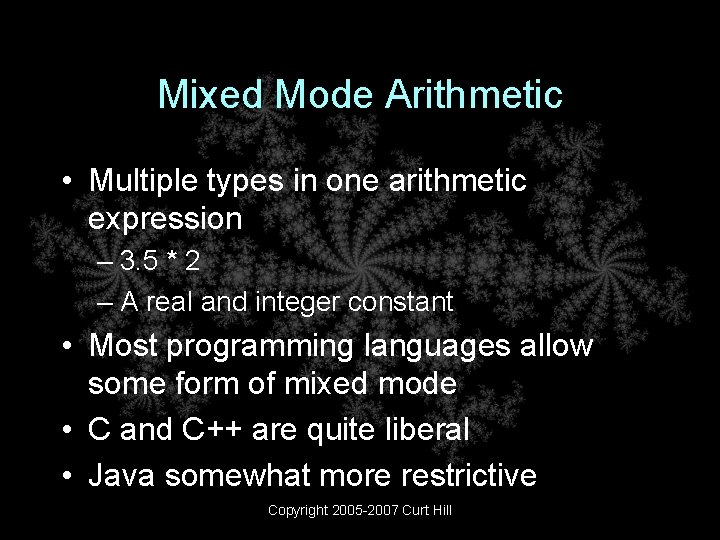
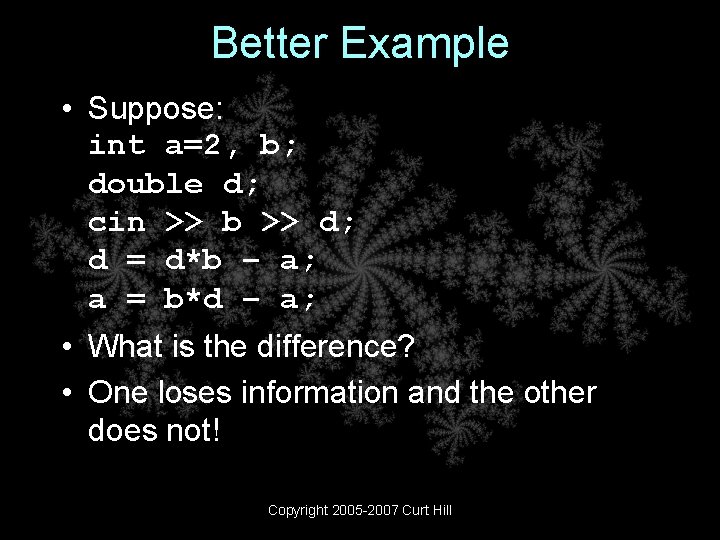
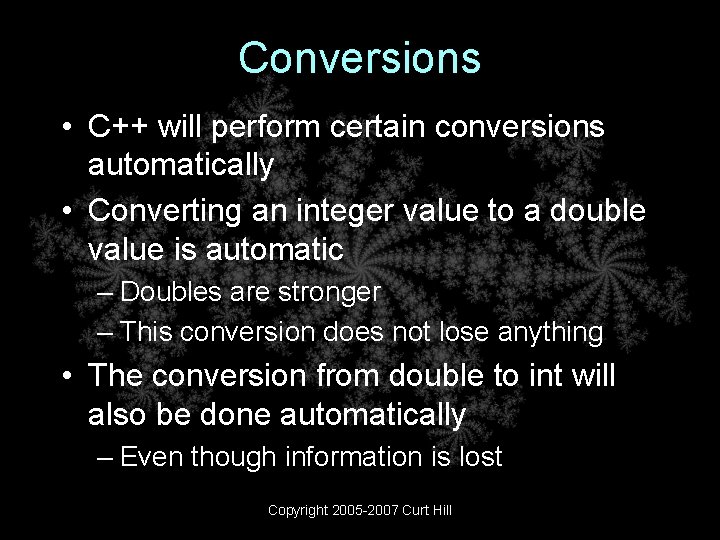
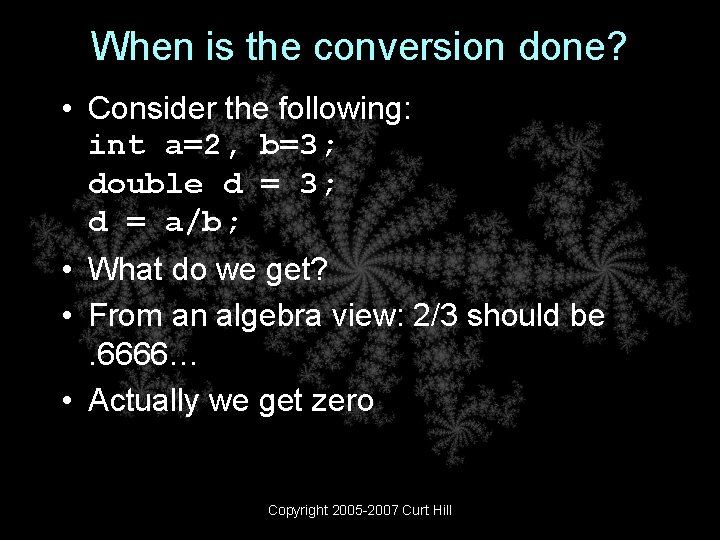
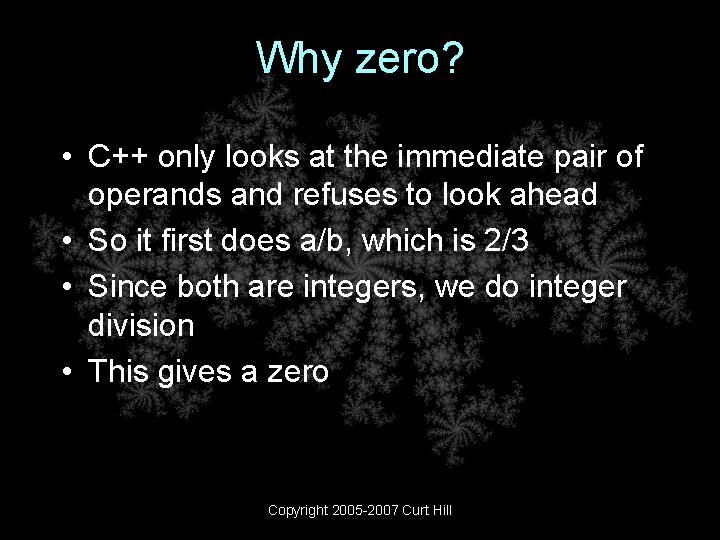
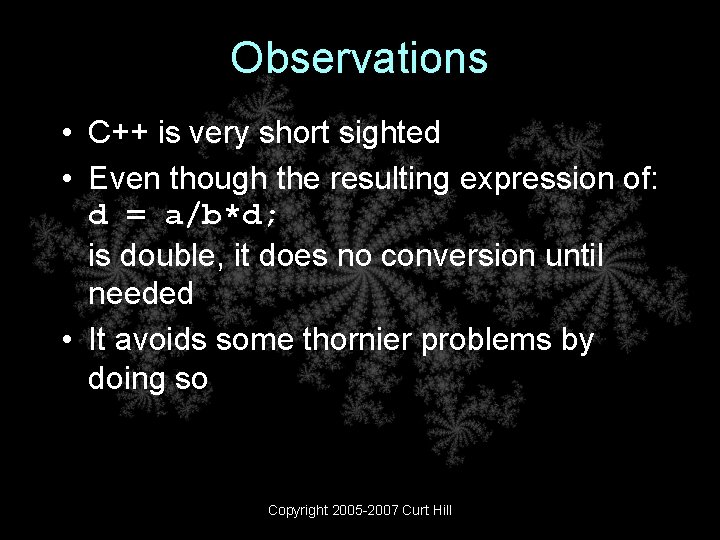
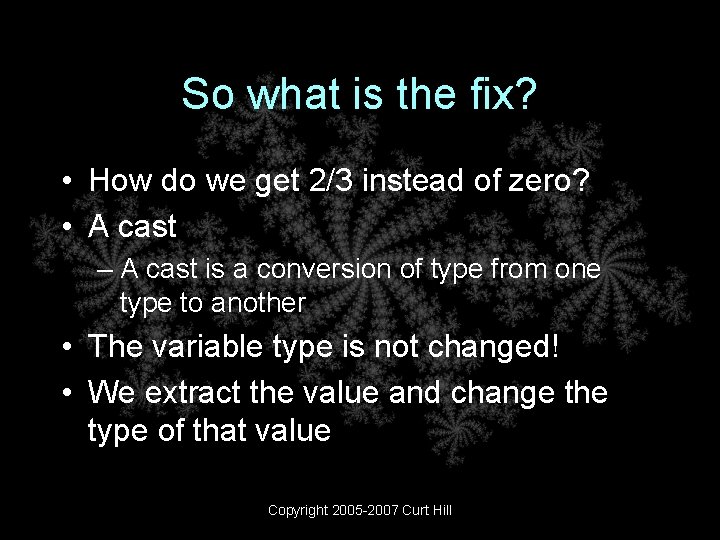
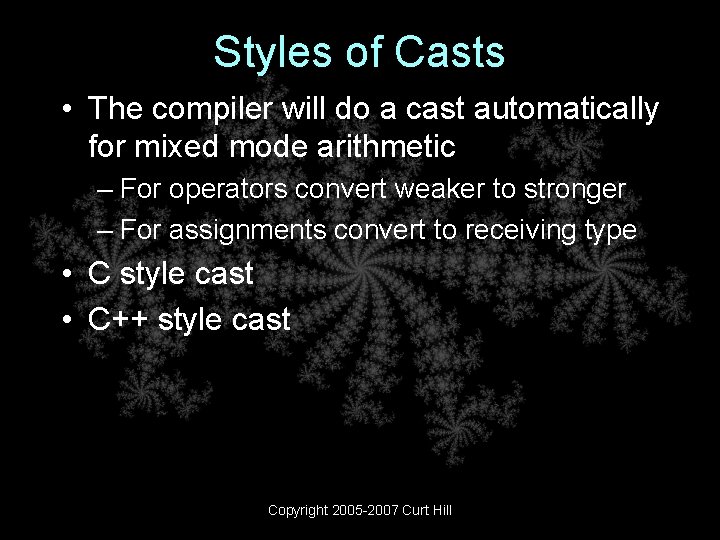
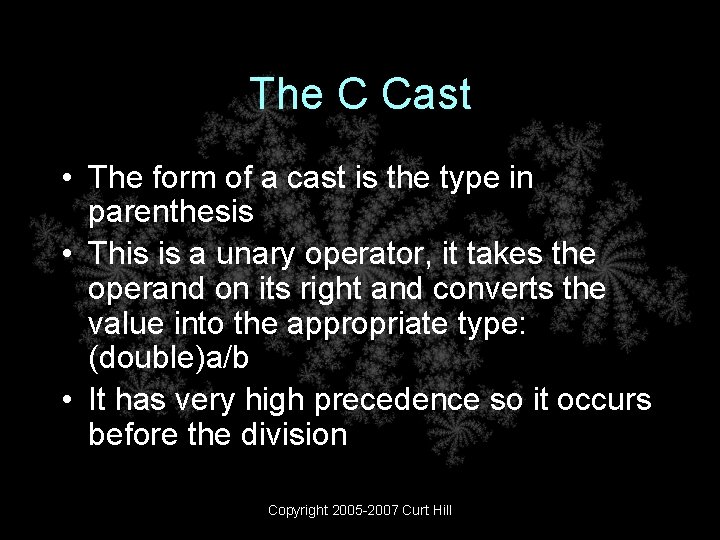
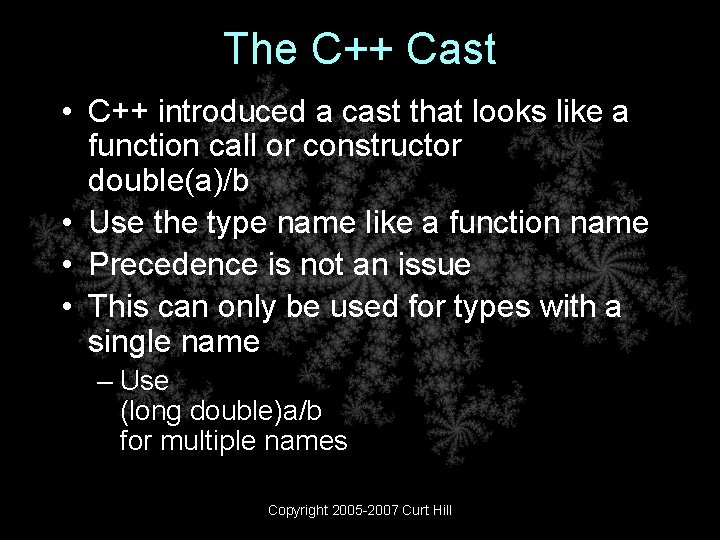
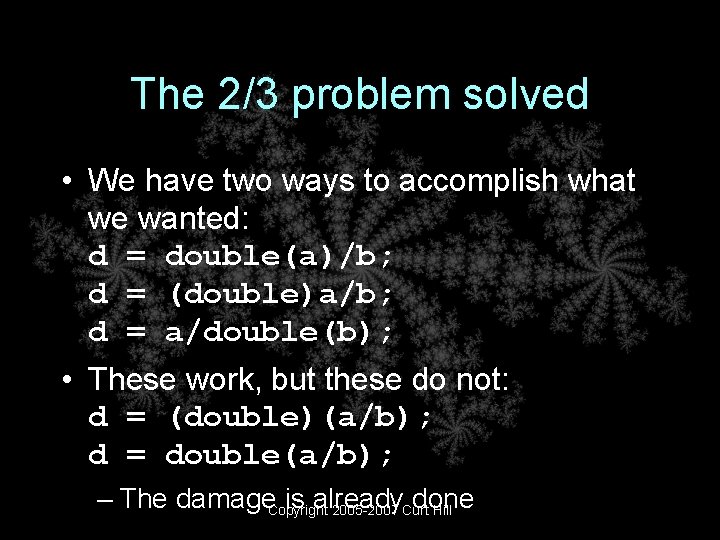
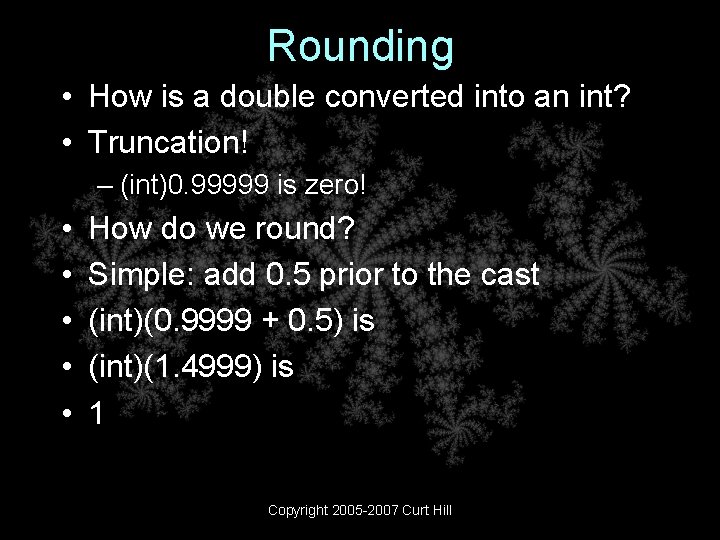
- Slides: 13
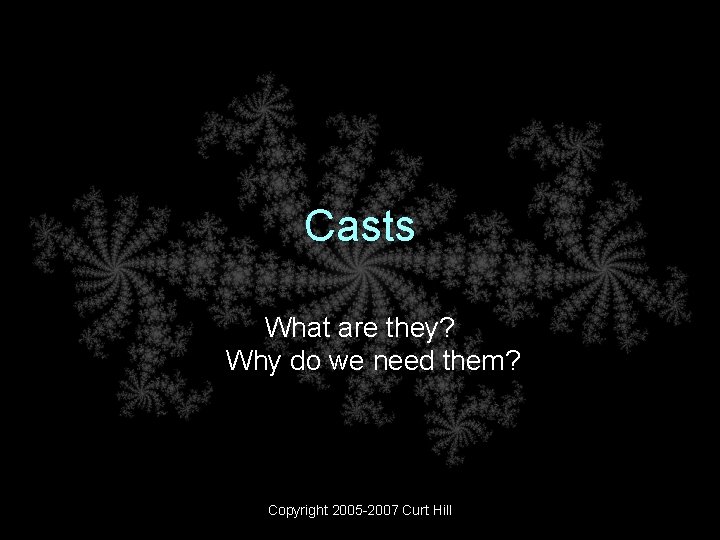
Casts What are they? Why do we need them? Copyright 2005 -2007 Curt Hill
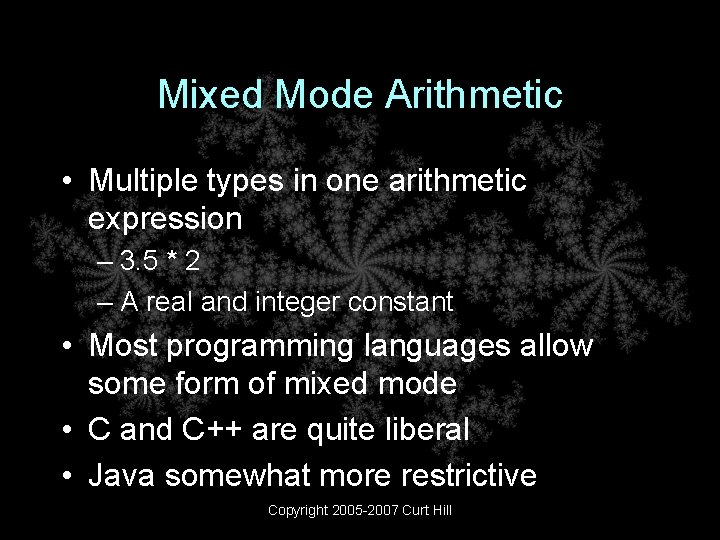
Mixed Mode Arithmetic • Multiple types in one arithmetic expression – 3. 5 * 2 – A real and integer constant • Most programming languages allow some form of mixed mode • C and C++ are quite liberal • Java somewhat more restrictive Copyright 2005 -2007 Curt Hill
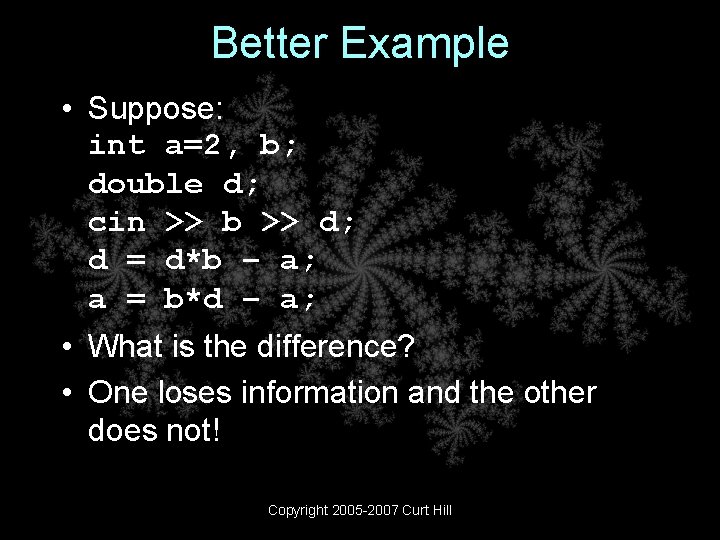
Better Example • Suppose: int a=2, b; double d; cin >> b >> d; d = d*b – a; a = b*d – a; • What is the difference? • One loses information and the other does not! Copyright 2005 -2007 Curt Hill
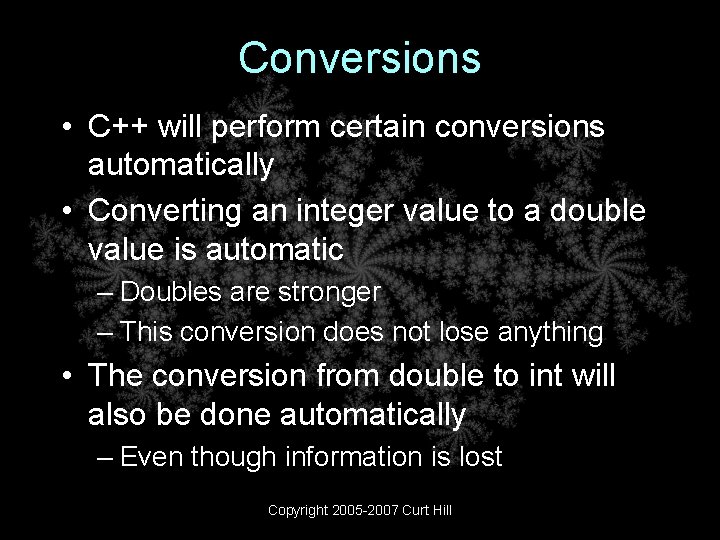
Conversions • C++ will perform certain conversions automatically • Converting an integer value to a double value is automatic – Doubles are stronger – This conversion does not lose anything • The conversion from double to int will also be done automatically – Even though information is lost Copyright 2005 -2007 Curt Hill
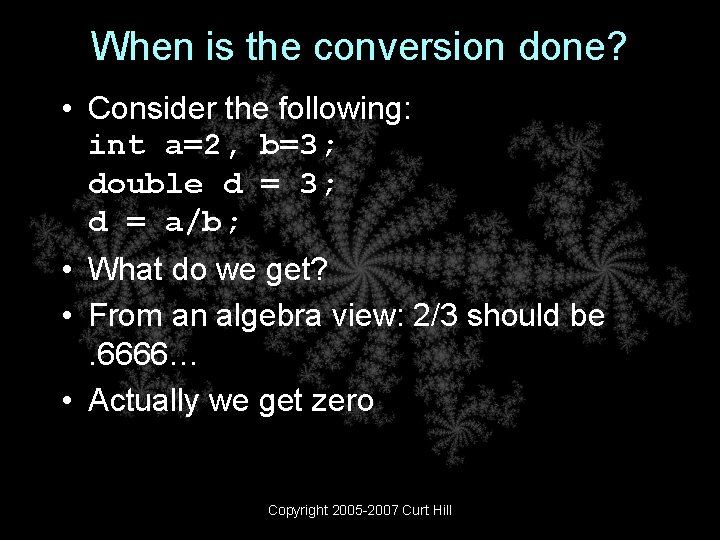
When is the conversion done? • Consider the following: int a=2, b=3; double d = 3; d = a/b; • What do we get? • From an algebra view: 2/3 should be. 6666… • Actually we get zero Copyright 2005 -2007 Curt Hill
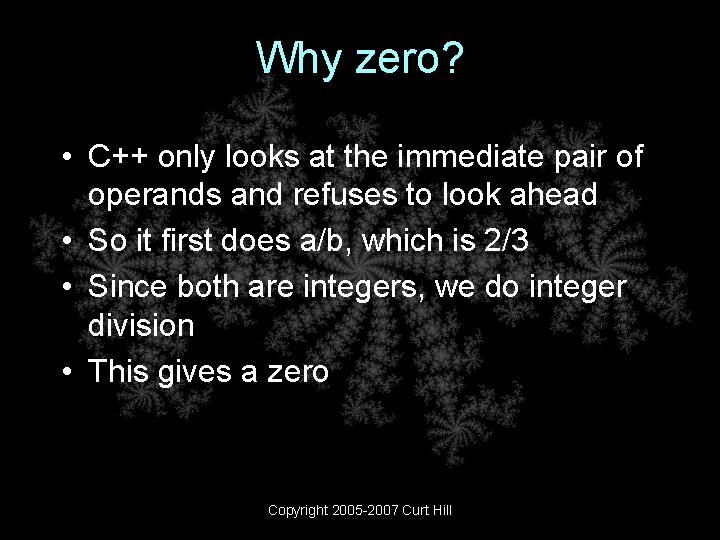
Why zero? • C++ only looks at the immediate pair of operands and refuses to look ahead • So it first does a/b, which is 2/3 • Since both are integers, we do integer division • This gives a zero Copyright 2005 -2007 Curt Hill
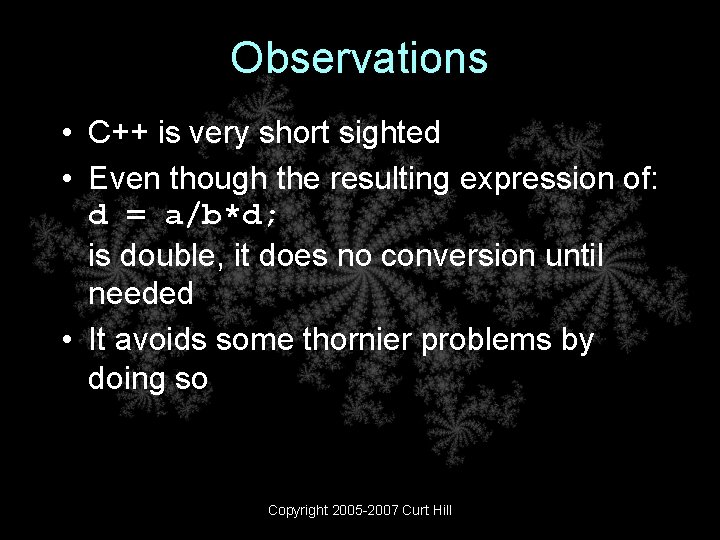
Observations • C++ is very short sighted • Even though the resulting expression of: d = a/b*d; is double, it does no conversion until needed • It avoids some thornier problems by doing so Copyright 2005 -2007 Curt Hill
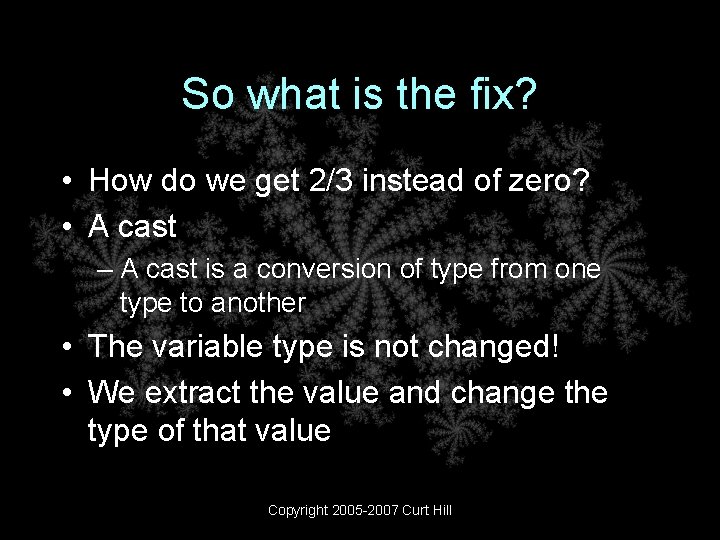
So what is the fix? • How do we get 2/3 instead of zero? • A cast – A cast is a conversion of type from one type to another • The variable type is not changed! • We extract the value and change the type of that value Copyright 2005 -2007 Curt Hill
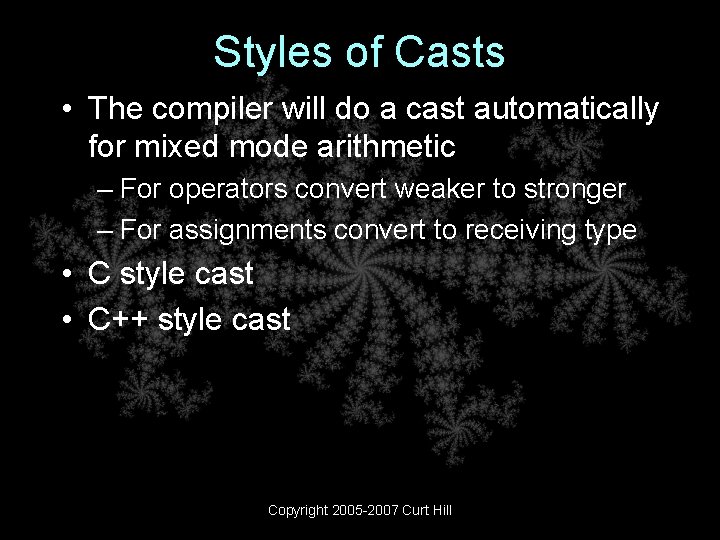
Styles of Casts • The compiler will do a cast automatically for mixed mode arithmetic – For operators convert weaker to stronger – For assignments convert to receiving type • C style cast • C++ style cast Copyright 2005 -2007 Curt Hill
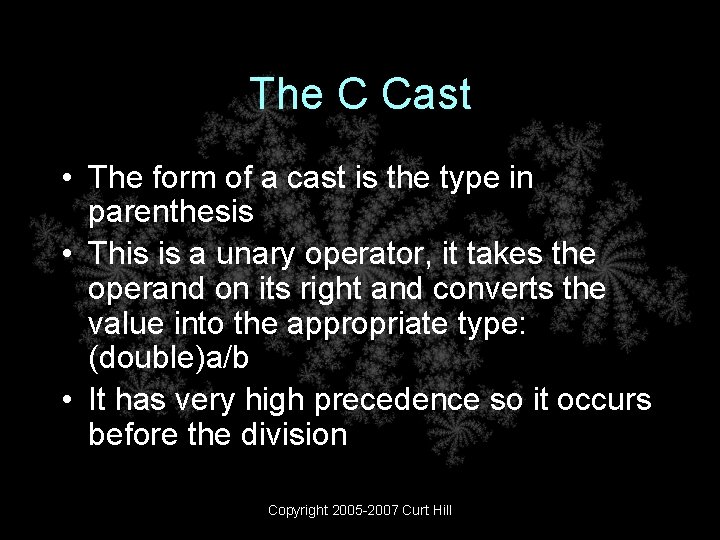
The C Cast • The form of a cast is the type in parenthesis • This is a unary operator, it takes the operand on its right and converts the value into the appropriate type: (double)a/b • It has very high precedence so it occurs before the division Copyright 2005 -2007 Curt Hill
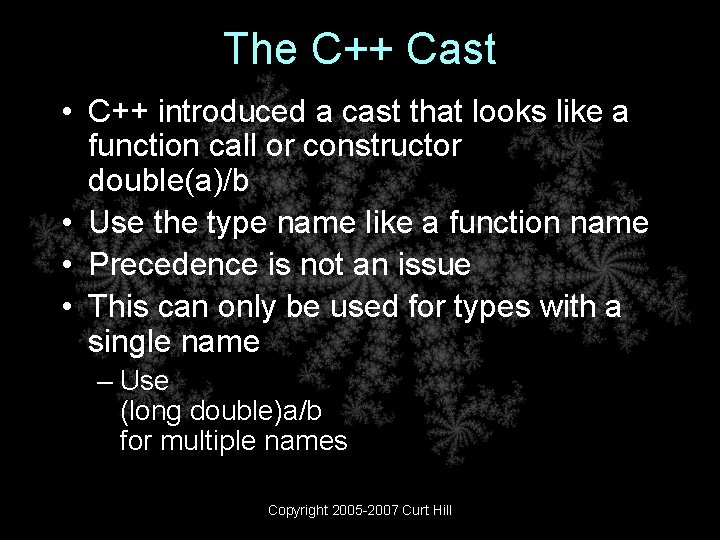
The C++ Cast • C++ introduced a cast that looks like a function call or constructor double(a)/b • Use the type name like a function name • Precedence is not an issue • This can only be used for types with a single name – Use (long double)a/b for multiple names Copyright 2005 -2007 Curt Hill
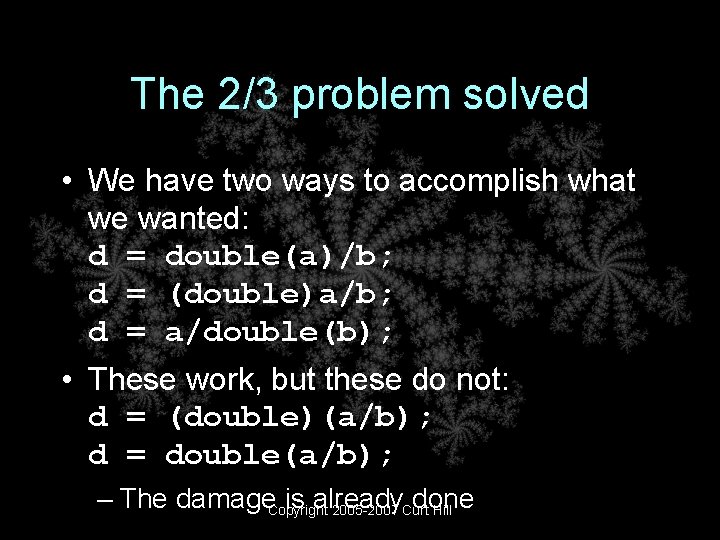
The 2/3 problem solved • We have two ways to accomplish what we wanted: d = double(a)/b; d = (double)a/b; d = a/double(b); • These work, but these do not: d = (double)(a/b); d = double(a/b); – The damage. Copyright is already done 2005 -2007 Curt Hill
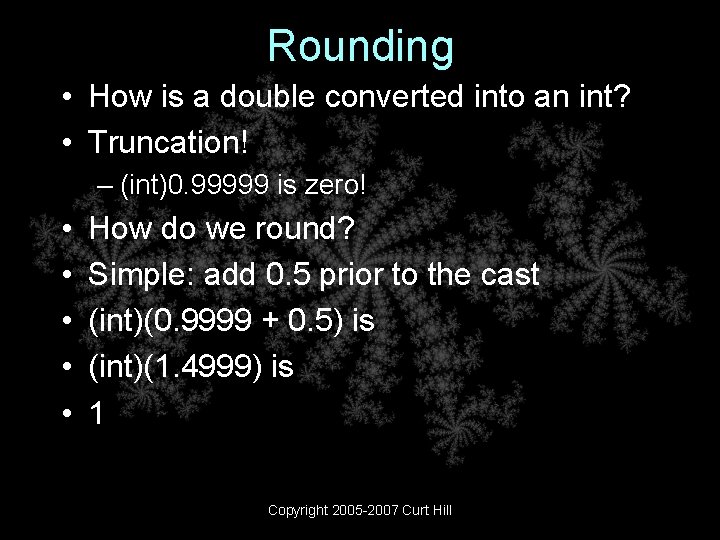
Rounding • How is a double converted into an int? • Truncation! – (int)0. 99999 is zero! • • • How do we round? Simple: add 0. 5 prior to the cast (int)(0. 9999 + 0. 5) is (int)(1. 4999) is 1 Copyright 2005 -2007 Curt Hill