Advanced Functional Programming Tim Sheard Oregon Graduate Institute
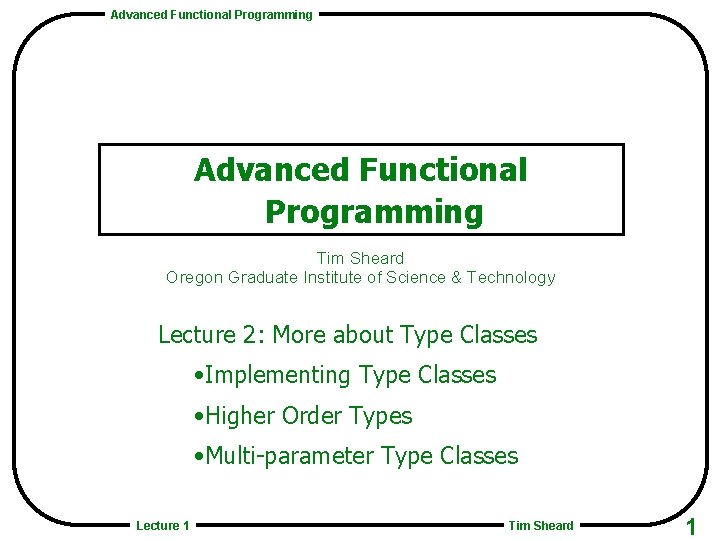
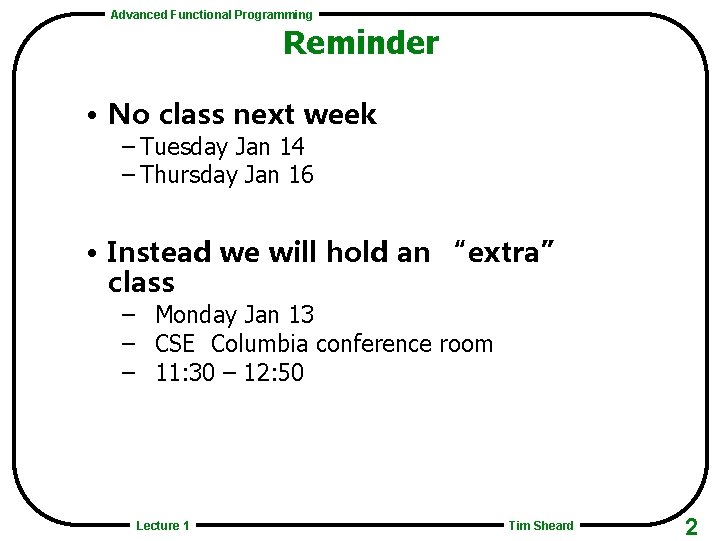
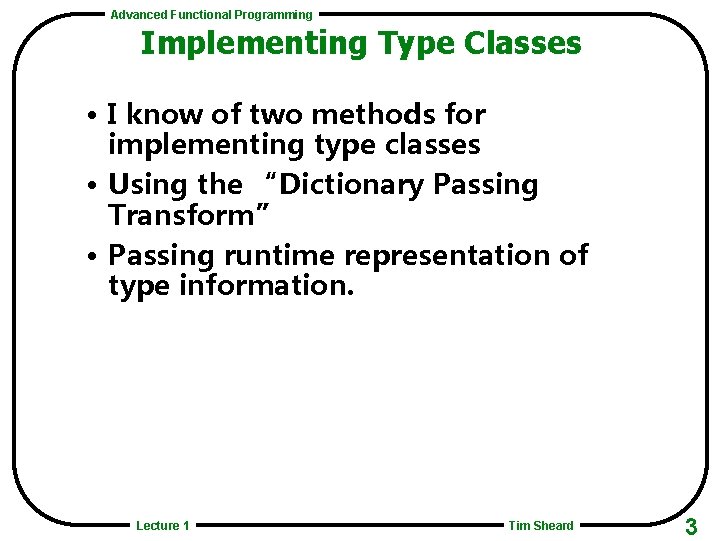
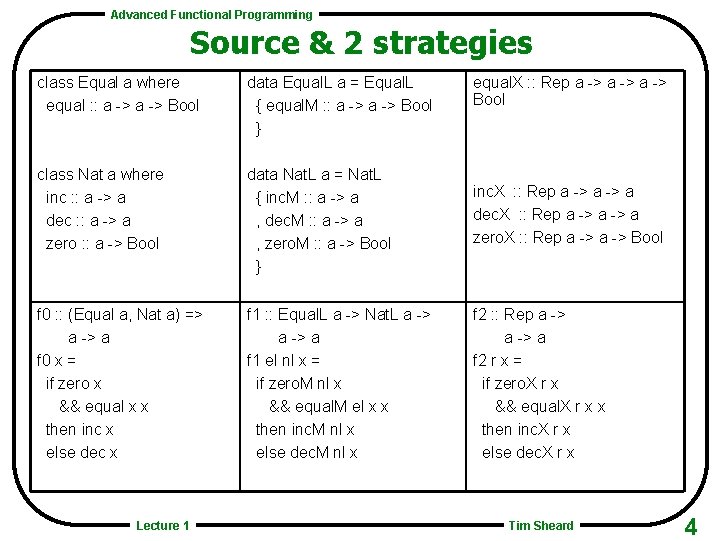
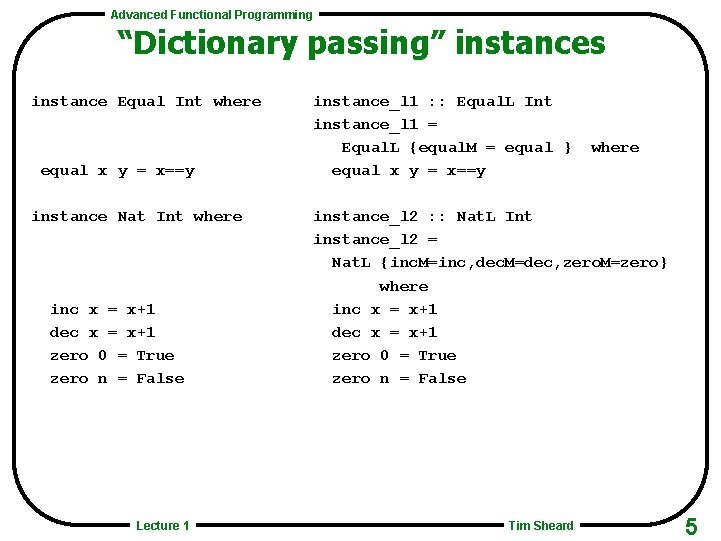
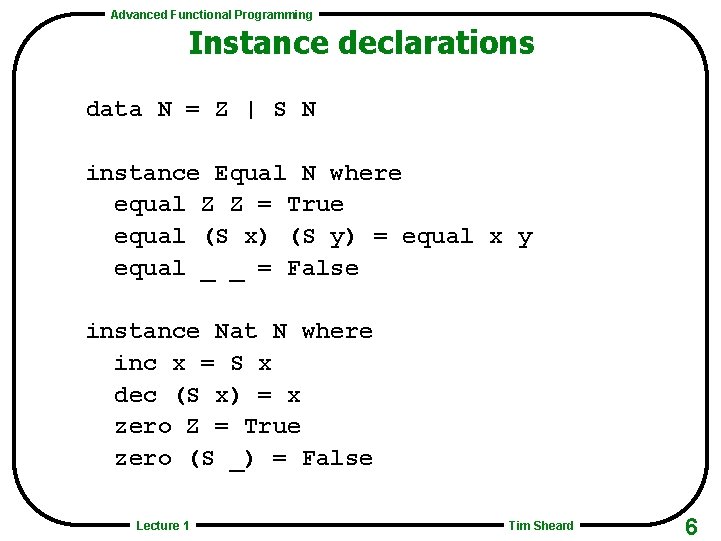
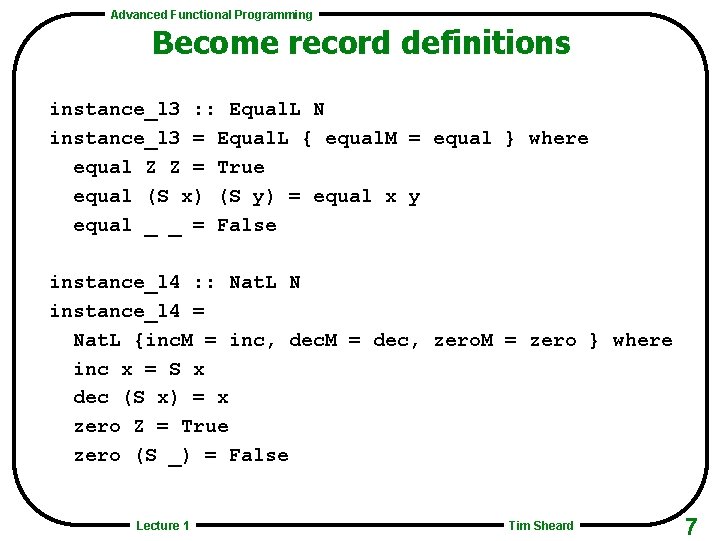
![Advanced Functional Programming Dependent classes instance Equal a => Equal [a] where equal [] Advanced Functional Programming Dependent classes instance Equal a => Equal [a] where equal []](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-8.jpg)
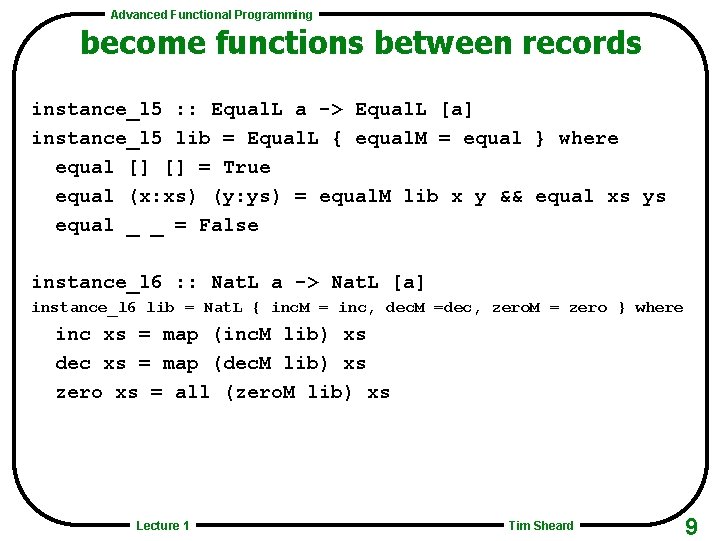
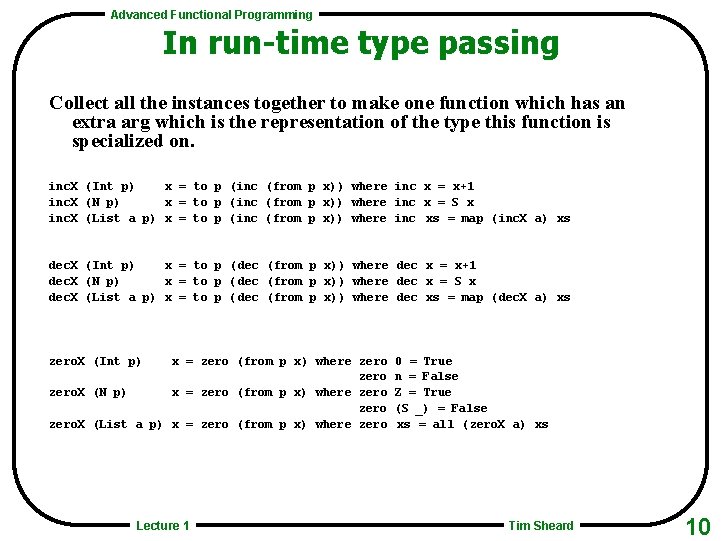
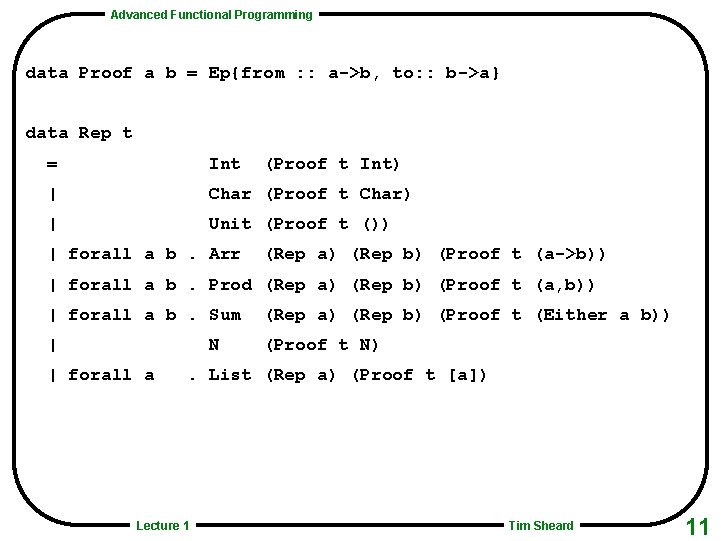
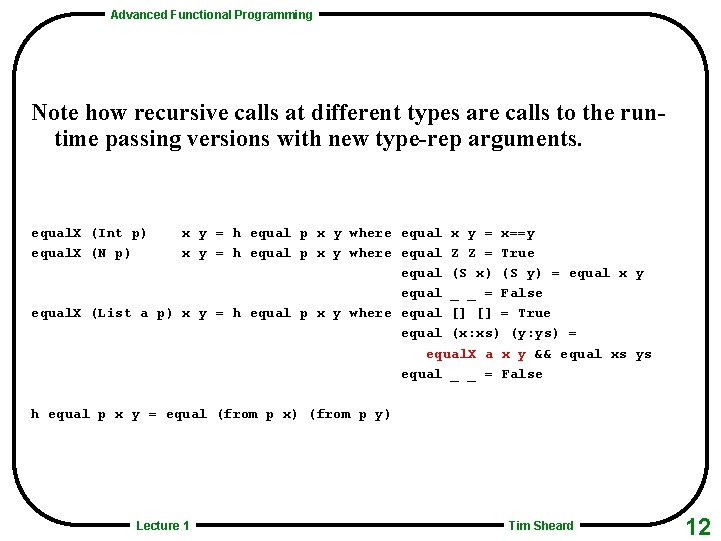
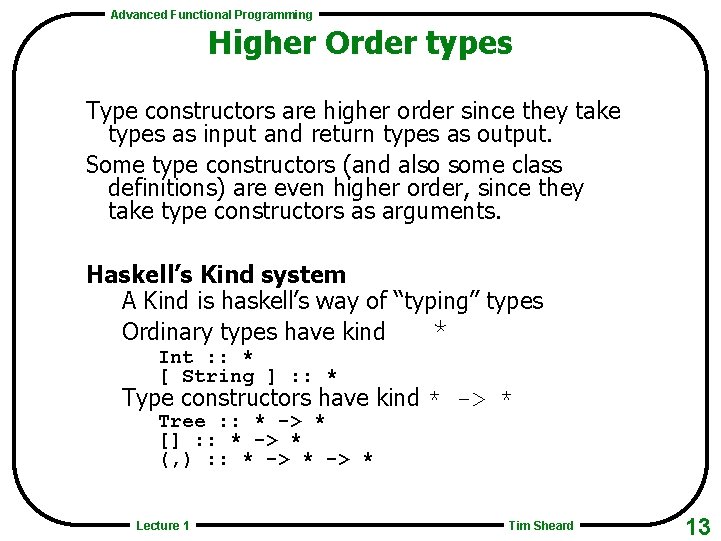
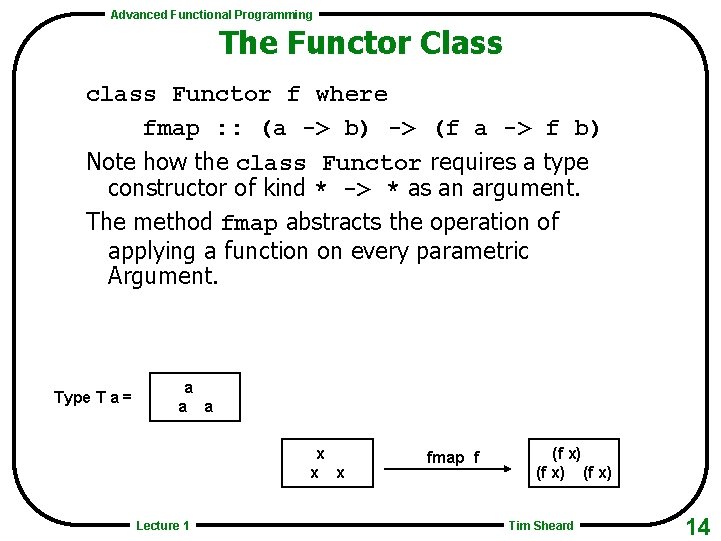
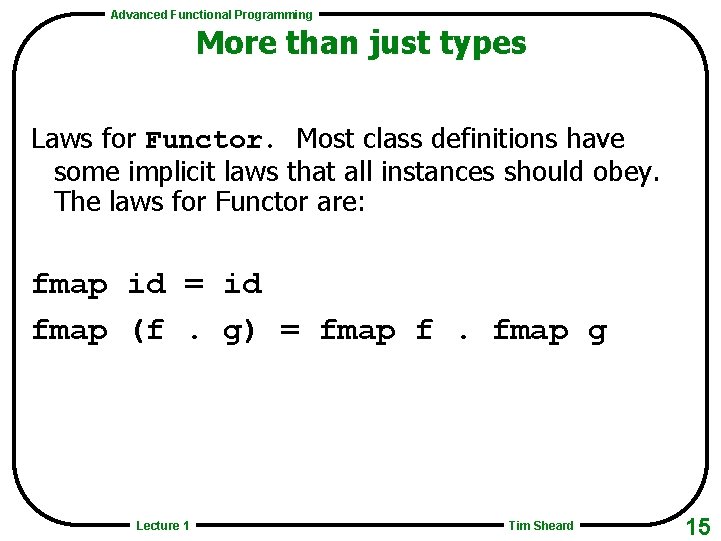
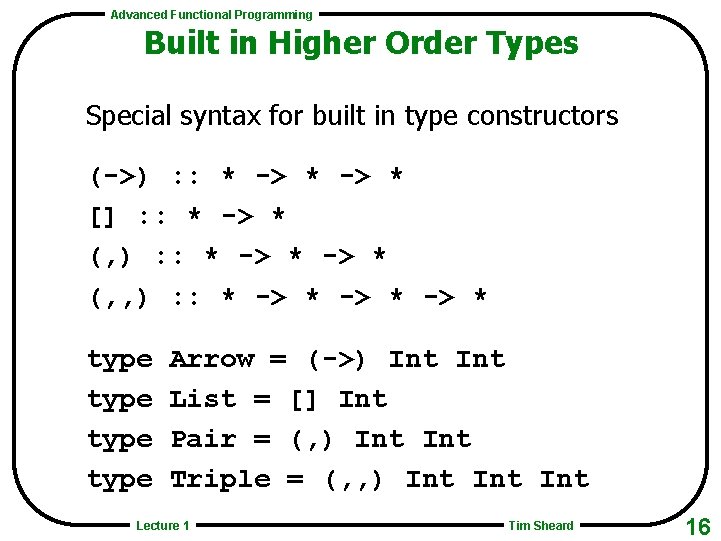
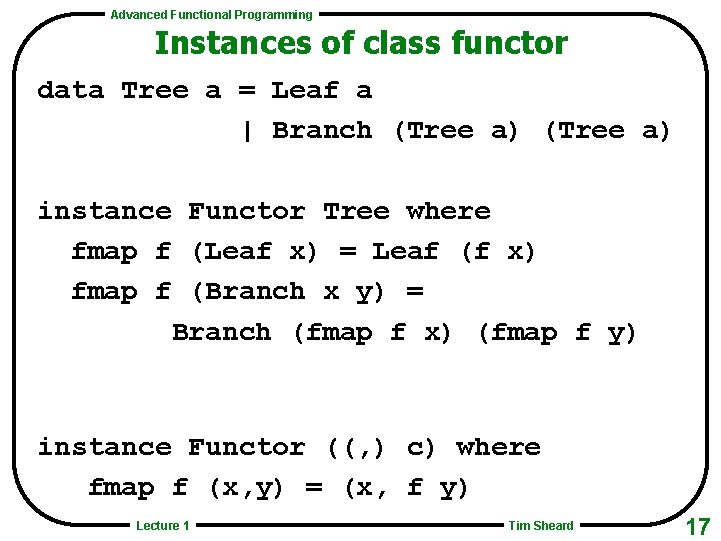
![Advanced Functional Programming More Instances instance Functor [] where fmap f [] = [] Advanced Functional Programming More Instances instance Functor [] where fmap f [] = []](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-18.jpg)
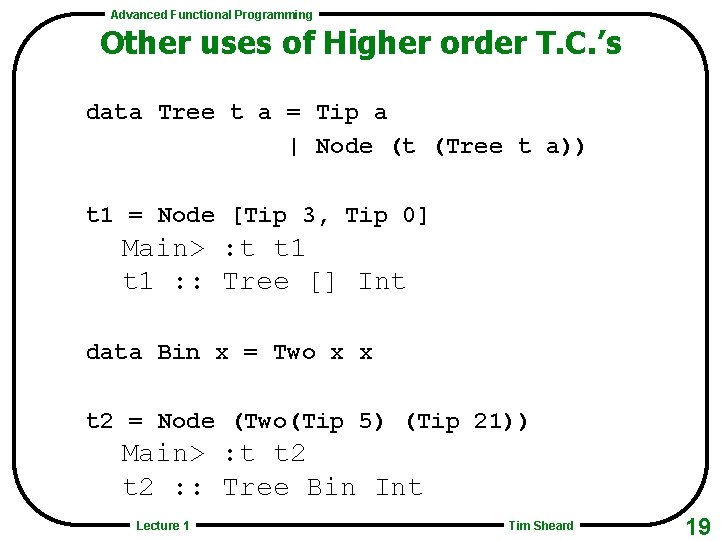
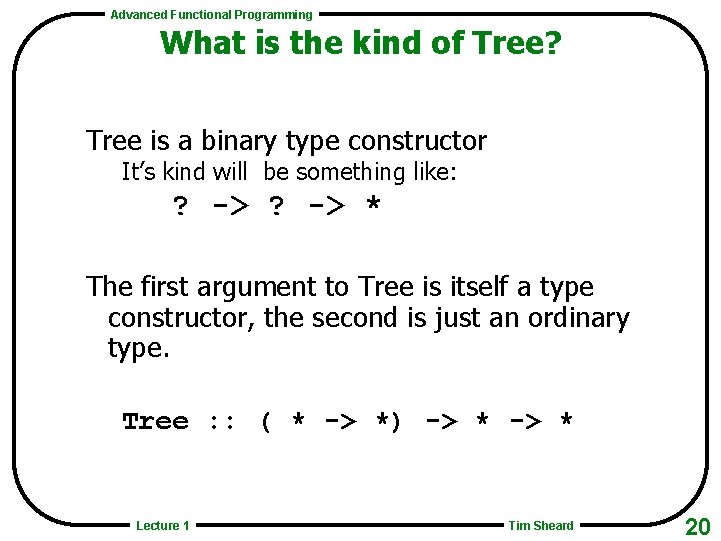
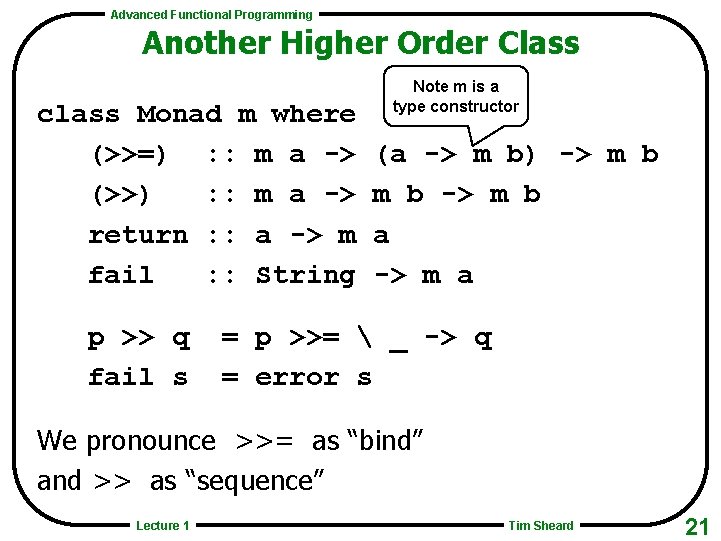
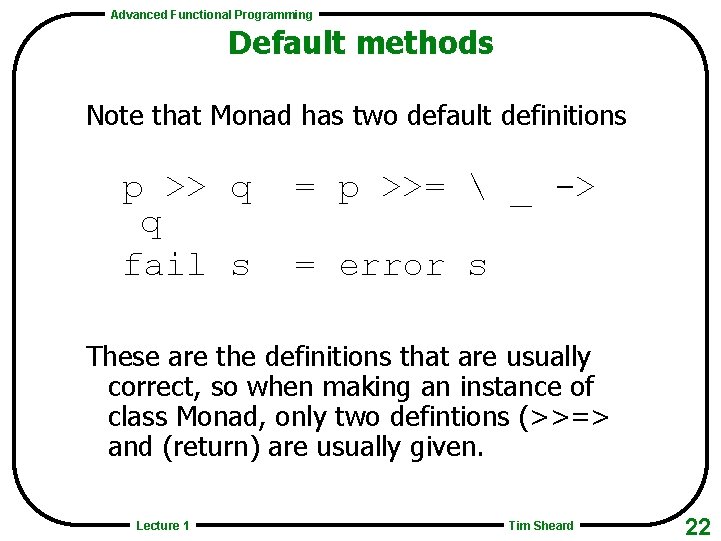
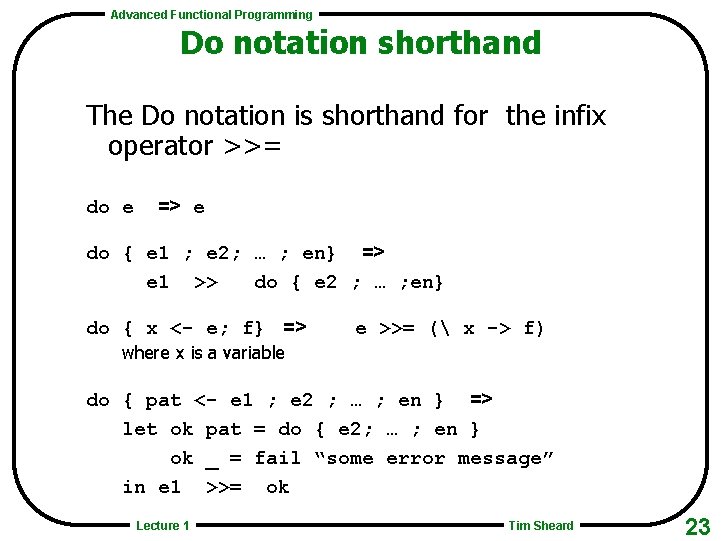
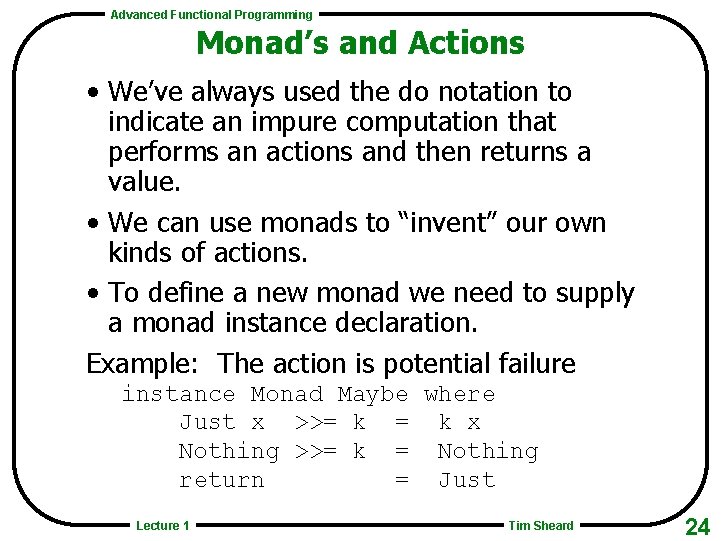
![Advanced Functional Programming Example find : : Eq a => a -> [(a, b)] Advanced Functional Programming Example find : : Eq a => a -> [(a, b)]](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-25.jpg)
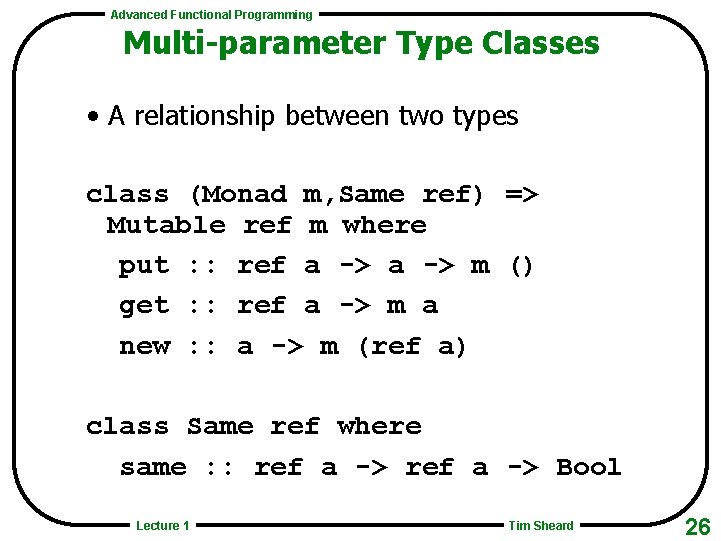
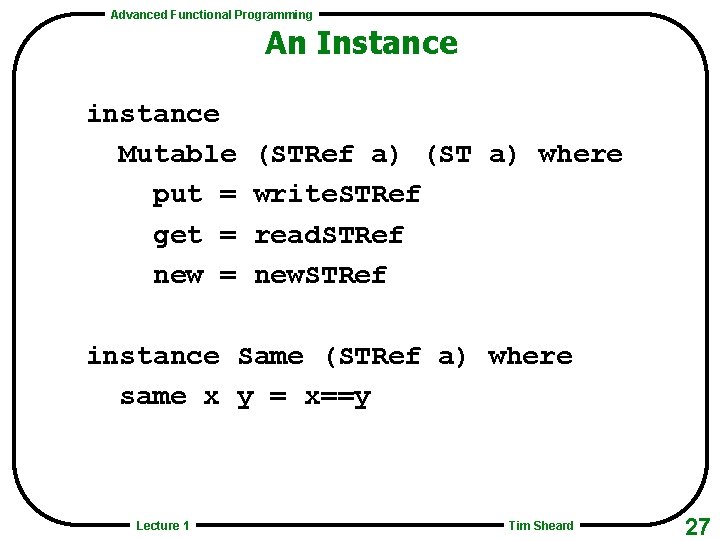
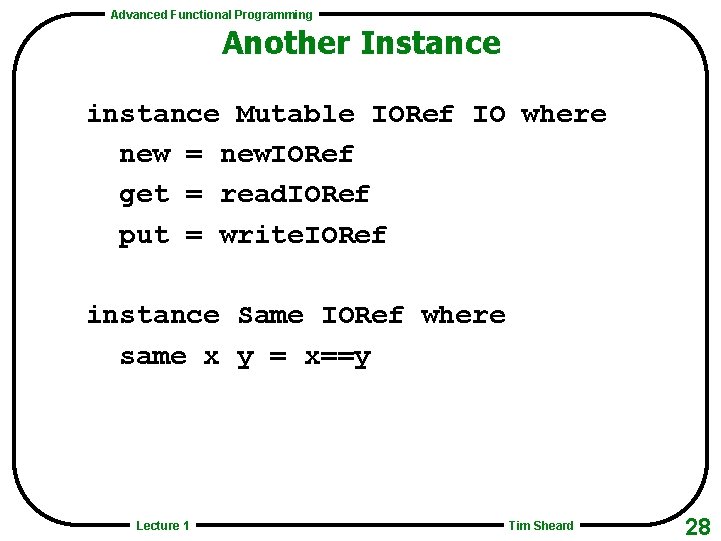
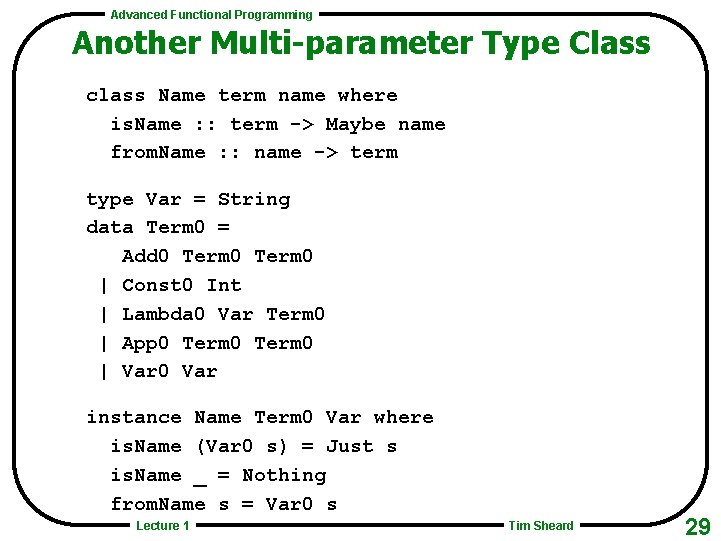
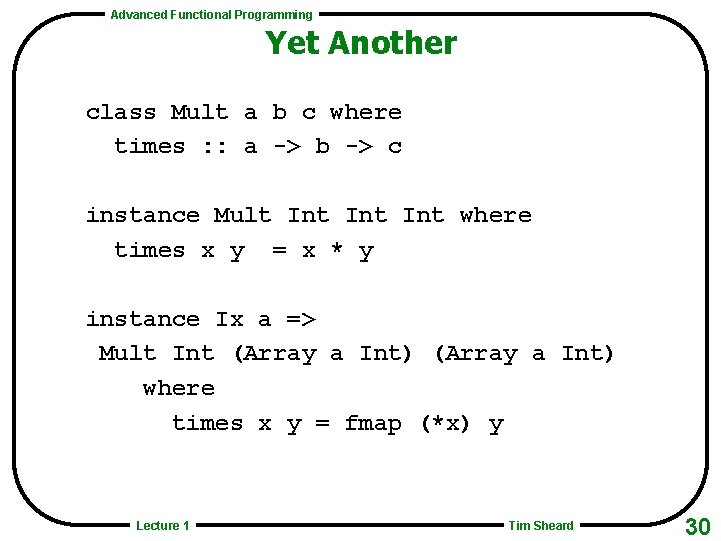
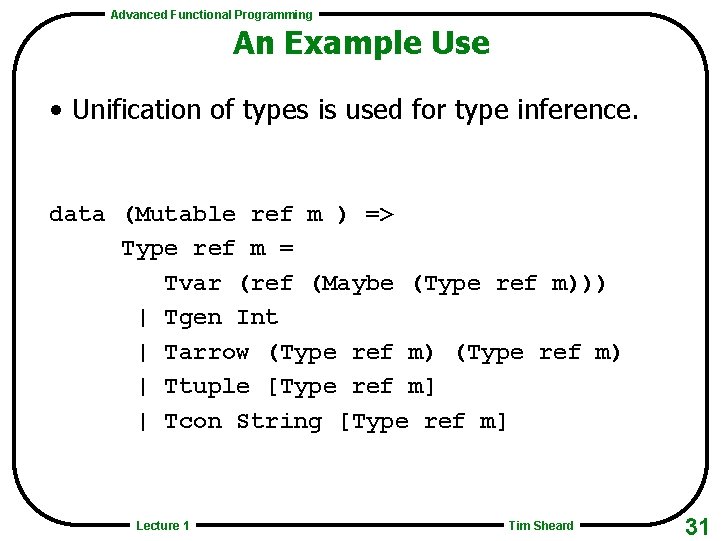
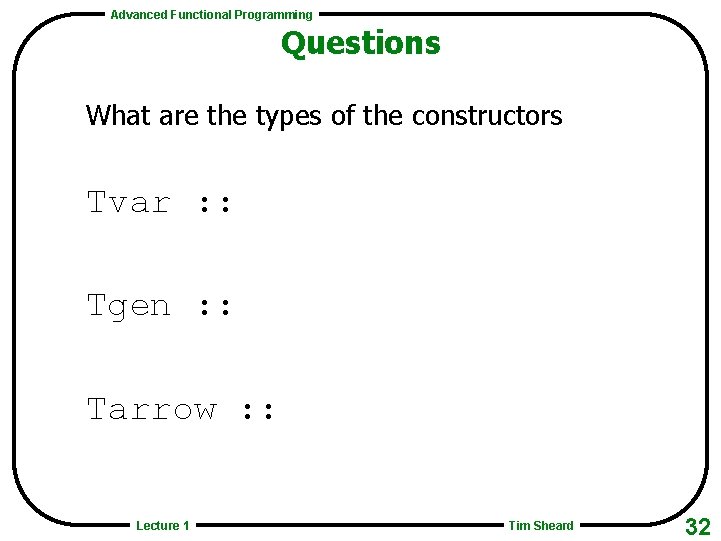
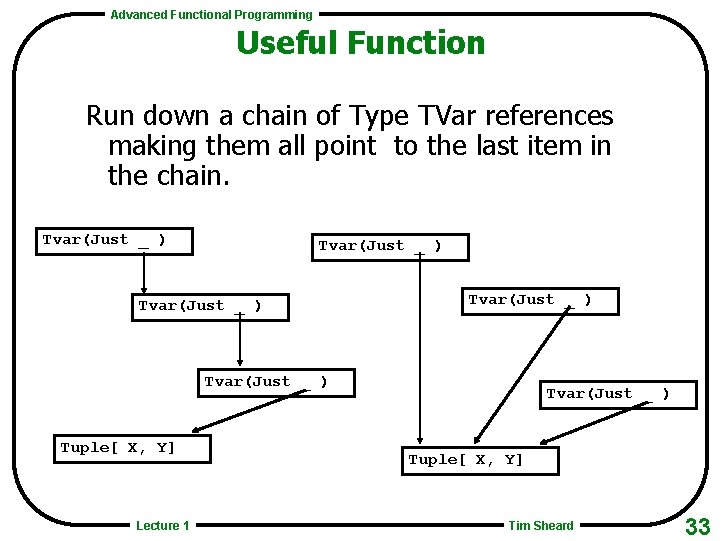
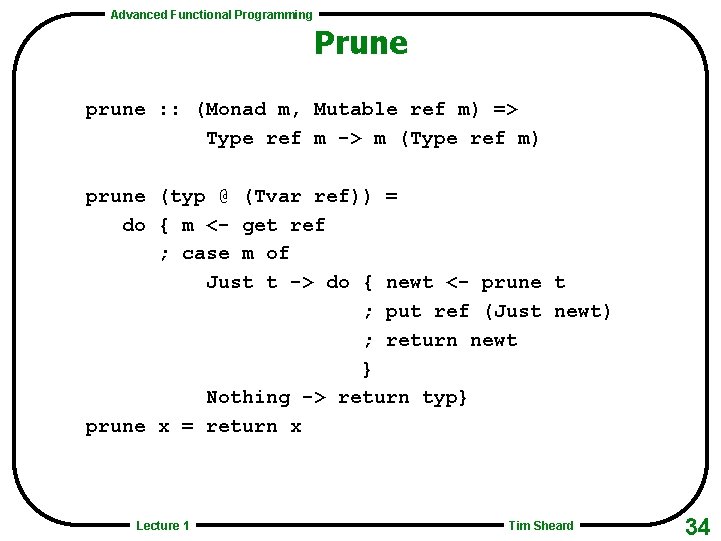
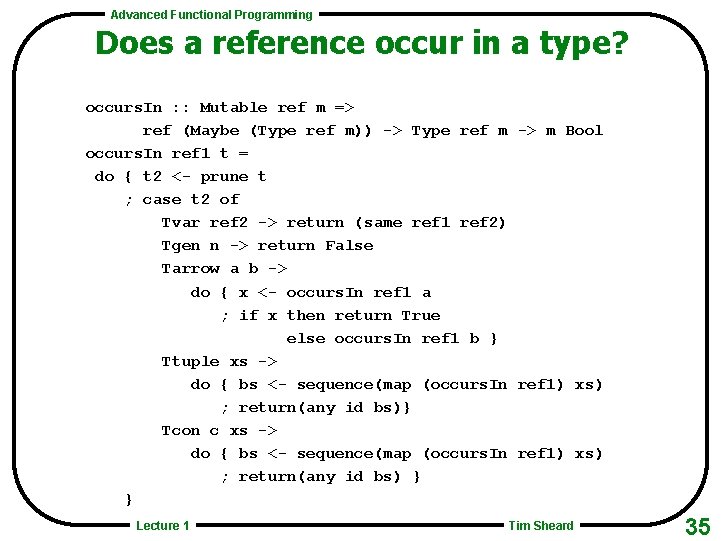
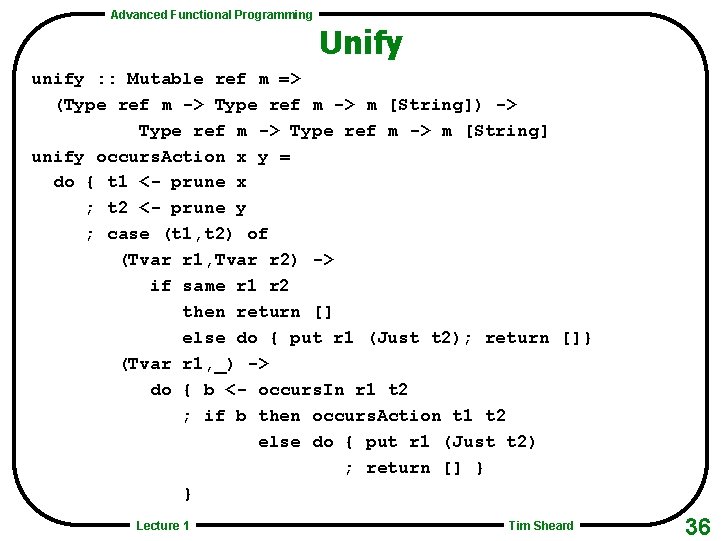
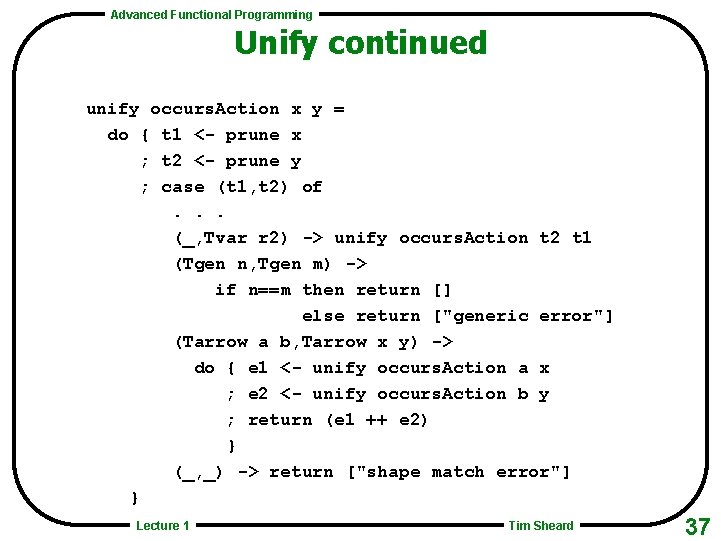
![Advanced Functional Programming Generic Monad Functions sequence : : Monad m => [m a] Advanced Functional Programming Generic Monad Functions sequence : : Monad m => [m a]](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-38.jpg)
- Slides: 38
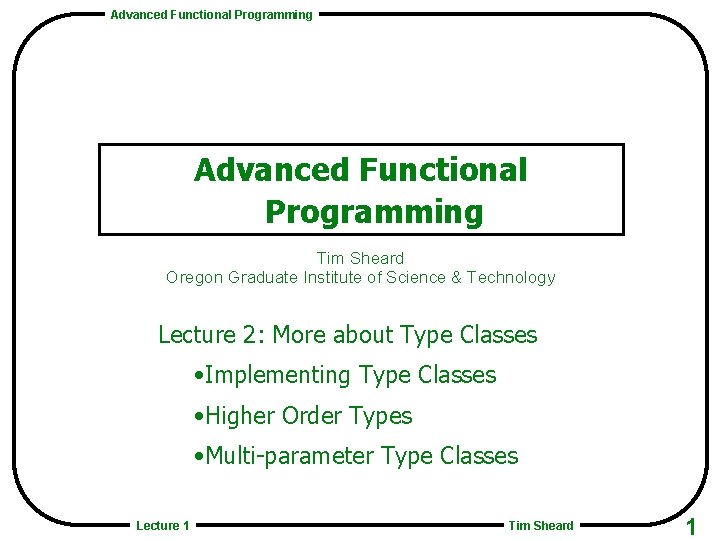
Advanced Functional Programming Tim Sheard Oregon Graduate Institute of Science & Technology Lecture 2: More about Type Classes • Implementing Type Classes • Higher Order Types • Multi-parameter Type Classes Lecture 1 Tim Sheard 1
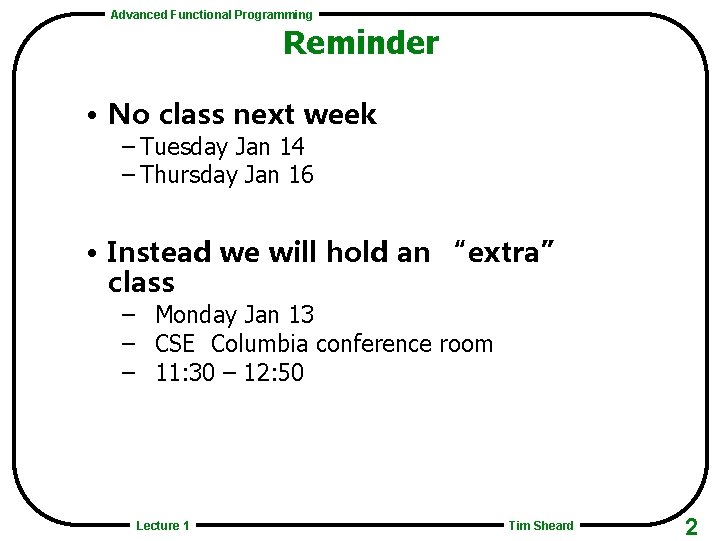
Advanced Functional Programming Reminder • No class next week – Tuesday Jan 14 – Thursday Jan 16 • Instead we will hold an “extra” class – Monday Jan 13 – CSE Columbia conference room – 11: 30 – 12: 50 Lecture 1 Tim Sheard 2
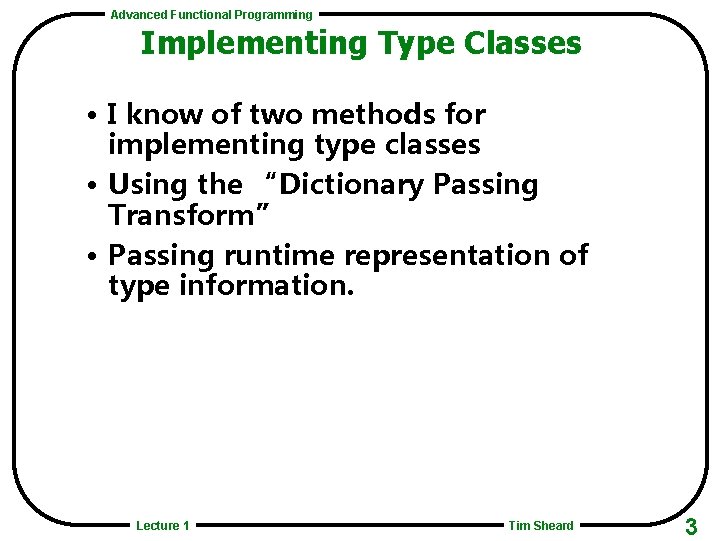
Advanced Functional Programming Implementing Type Classes • I know of two methods for implementing type classes • Using the “Dictionary Passing Transform” • Passing runtime representation of type information. Lecture 1 Tim Sheard 3
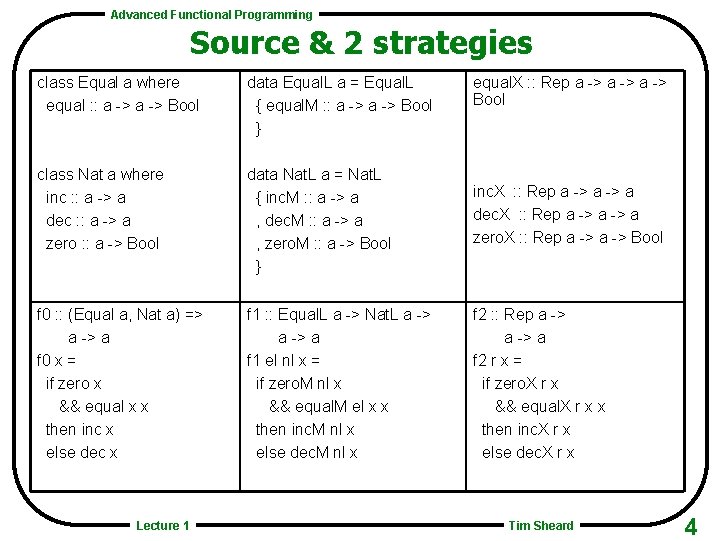
Advanced Functional Programming Source & 2 strategies class Equal a where equal : : a -> Bool data Equal. L a = Equal. L { equal. M : : a -> Bool } class Nat a where inc : : a -> a dec : : a -> a zero : : a -> Bool data Nat. L a = Nat. L { inc. M : : a -> a , dec. M : : a -> a , zero. M : : a -> Bool } inc. X : : Rep a -> a dec. X : : Rep a -> a zero. X : : Rep a -> Bool f 0 : : (Equal a, Nat a) => a -> a f 0 x = if zero x && equal x x then inc x else dec x f 1 : : Equal. L a -> Nat. L a -> a f 1 el nl x = if zero. M nl x && equal. M el x x then inc. M nl x else dec. M nl x f 2 : : Rep a -> a f 2 r x = if zero. X r x && equal. X r x x then inc. X r x else dec. X r x Lecture 1 equal. X : : Rep a -> Bool Tim Sheard 4
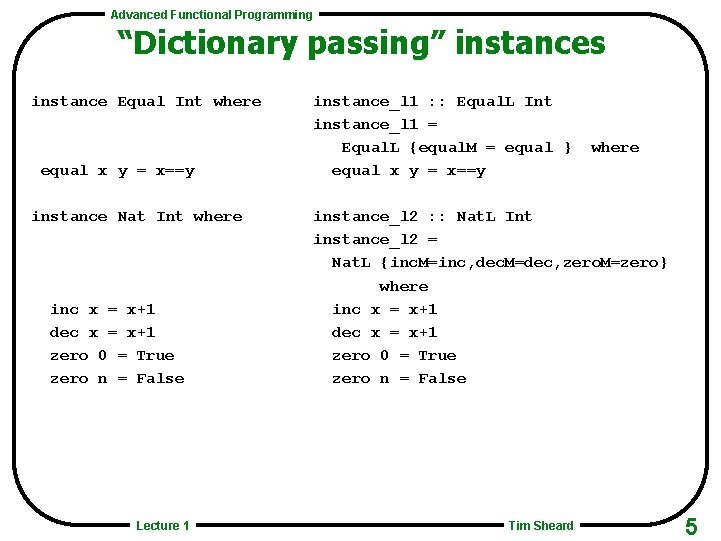
Advanced Functional Programming “Dictionary passing” instances instance Equal Int where equal x y = x==y instance Nat Int where inc x = x+1 dec x = x+1 zero 0 = True zero n = False Lecture 1 instance_l 1 : : Equal. L Int instance_l 1 = Equal. L {equal. M = equal } equal x y = x==y where instance_l 2 : : Nat. L Int instance_l 2 = Nat. L {inc. M=inc, dec. M=dec, zero. M=zero} where inc x = x+1 dec x = x+1 zero 0 = True zero n = False Tim Sheard 5
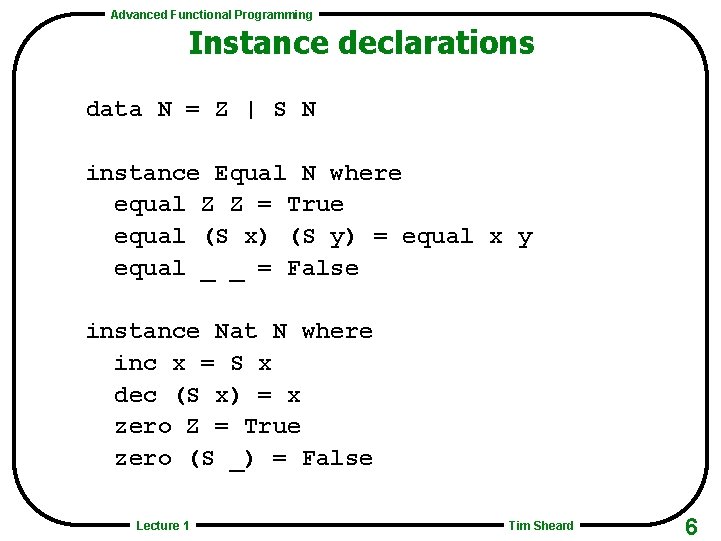
Advanced Functional Programming Instance declarations data N = Z | S N instance Equal N where equal Z Z = True equal (S x) (S y) = equal x y equal _ _ = False instance Nat N where inc x = S x dec (S x) = x zero Z = True zero (S _) = False Lecture 1 Tim Sheard 6
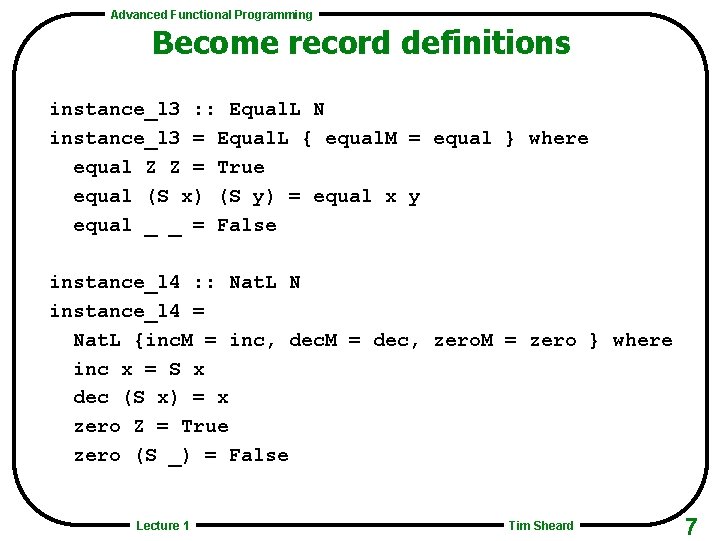
Advanced Functional Programming Become record definitions instance_l 3 : : Equal. L N instance_l 3 = Equal. L { equal. M = equal } where equal Z Z = True equal (S x) (S y) = equal x y equal _ _ = False instance_l 4 : : Nat. L N instance_l 4 = Nat. L {inc. M = inc, dec. M = dec, zero. M = zero } where inc x = S x dec (S x) = x zero Z = True zero (S _) = False Lecture 1 Tim Sheard 7
![Advanced Functional Programming Dependent classes instance Equal a Equal a where equal Advanced Functional Programming Dependent classes instance Equal a => Equal [a] where equal []](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-8.jpg)
Advanced Functional Programming Dependent classes instance Equal a => Equal [a] where equal [] [] = True equal (x: xs) (y: ys) = equal x y && equal xs ys equal _ _ = False instance Nat a => Nat [a] where inc xs = map inc xs dec xs = map dec xs zero xs = all zero xs Lecture 1 Tim Sheard 8
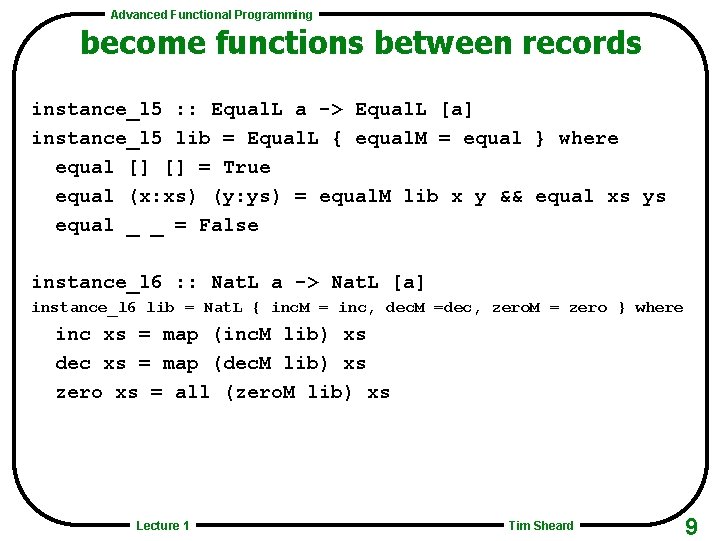
Advanced Functional Programming become functions between records instance_l 5 : : Equal. L a -> Equal. L [a] instance_l 5 lib = Equal. L { equal. M = equal } where equal [] [] = True equal (x: xs) (y: ys) = equal. M lib x y && equal xs ys equal _ _ = False instance_l 6 : : Nat. L a -> Nat. L [a] instance_l 6 lib = Nat. L { inc. M = inc, dec. M =dec, zero. M = zero } where inc xs = map (inc. M lib) xs dec xs = map (dec. M lib) xs zero xs = all (zero. M lib) xs Lecture 1 Tim Sheard 9
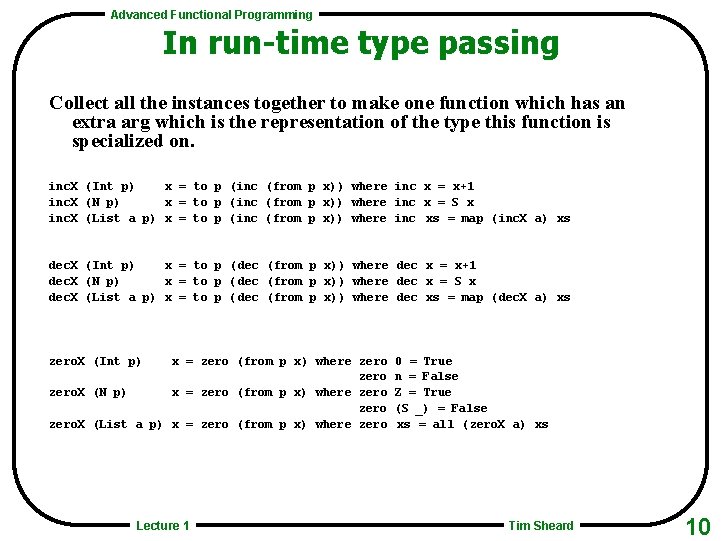
Advanced Functional Programming In run-time type passing Collect all the instances together to make one function which has an extra arg which is the representation of the type this function is specialized on. inc. X (Int p) x = to p (inc (from p x)) where inc x = x+1 inc. X (N p) x = to p (inc (from p x)) where inc x = S x inc. X (List a p) x = to p (inc (from p x)) where inc xs = map (inc. X a) xs dec. X (Int p) x = to p (dec (from p x)) where dec x = x+1 dec. X (N p) x = to p (dec (from p x)) where dec x = S x dec. X (List a p) x = to p (dec (from p x)) where dec xs = map (dec. X a) xs zero. X (Int p) x = zero (from p x) where zero. X (N p) x = zero (from p x) where zero. X (List a p) x = zero (from p x) where zero Lecture 1 0 = True n = False Z = True (S _) = False xs = all (zero. X a) xs Tim Sheard 10
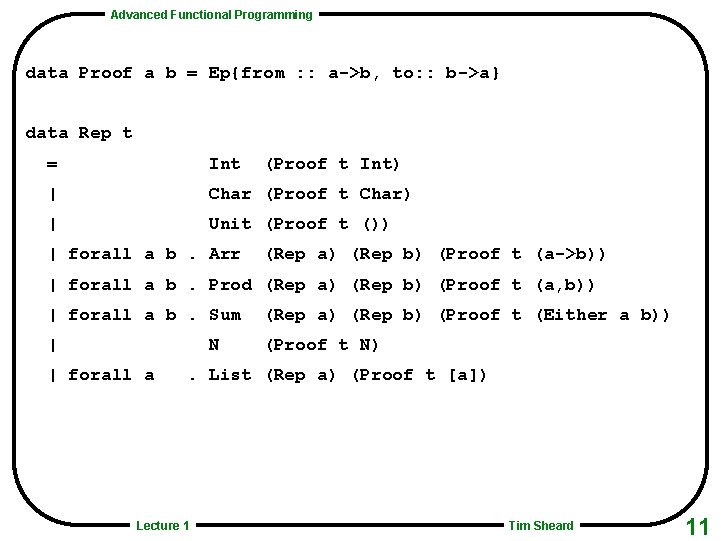
Advanced Functional Programming data Proof a b = Ep{from : : a->b, to: : b->a} data Rep t = Int | Char (Proof t Char) | Unit (Proof t ()) | forall a b. Arr (Proof t Int) (Rep a) (Rep b) (Proof t (a->b)) | forall a b. Prod (Rep a) (Rep b) (Proof t (a, b)) | forall a b. Sum (Rep a) (Rep b) (Proof t (Either a b)) | (Proof t N) N | forall a . List (Rep a) (Proof t [a]) Lecture 1 Tim Sheard 11
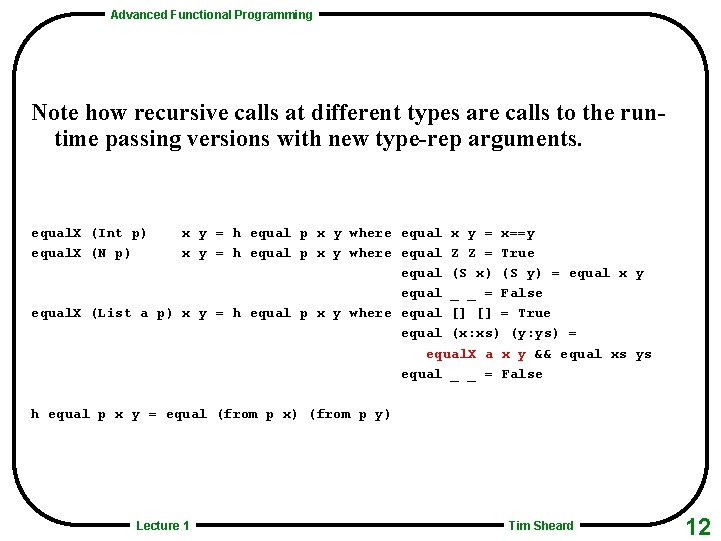
Advanced Functional Programming Note how recursive calls at different types are calls to the runtime passing versions with new type-rep arguments. equal. X (Int p) equal. X (N p) x y = h equal p x y where equal x y = x==y x y = h equal p x y where equal Z Z = True equal (S x) (S y) = equal x y equal _ _ = False equal. X (List a p) x y = h equal p x y where equal [] [] = True equal (x: xs) (y: ys) = equal. X a x y && equal xs ys equal _ _ = False h equal p x y = equal (from p x) (from p y) Lecture 1 Tim Sheard 12
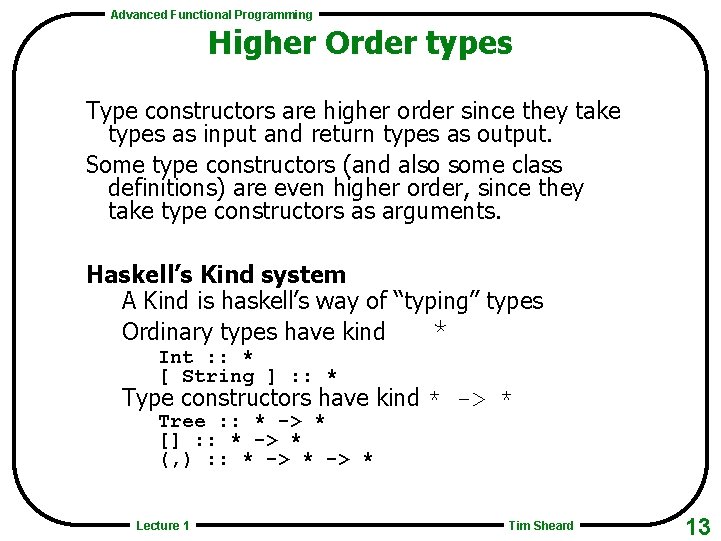
Advanced Functional Programming Higher Order types Type constructors are higher order since they take types as input and return types as output. Some type constructors (and also some class definitions) are even higher order, since they take type constructors as arguments. Haskell’s Kind system A Kind is haskell’s way of “typing” types Ordinary types have kind * Int : : * [ String ] : : * Type constructors have kind * -> * Tree : : * -> * [] : : * -> * (, ) : : * -> * Lecture 1 Tim Sheard 13
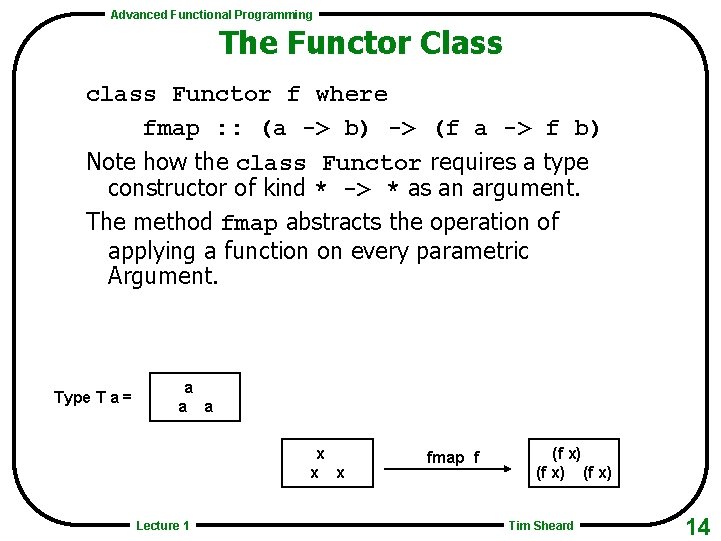
Advanced Functional Programming The Functor Class class Functor f where fmap : : (a -> b) -> (f a -> f b) Note how the class Functor requires a type constructor of kind * -> * as an argument. The method fmap abstracts the operation of applying a function on every parametric Argument. Type T a = a a a x x x Lecture 1 fmap f (f x) Tim Sheard 14
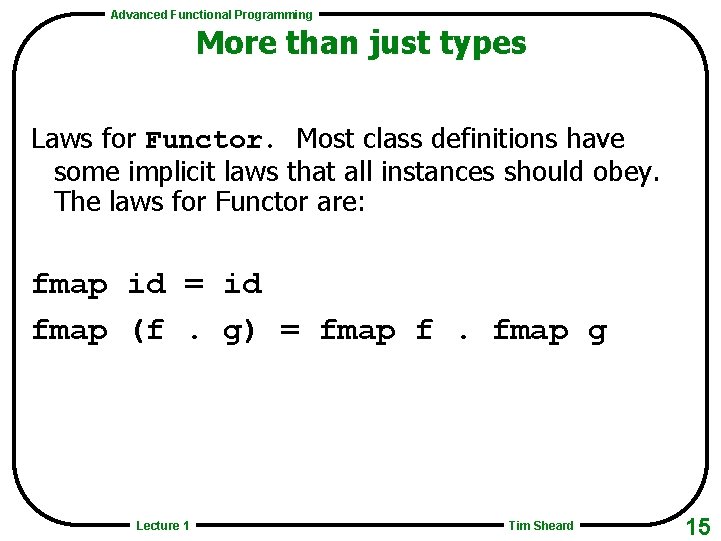
Advanced Functional Programming More than just types Laws for Functor. Most class definitions have some implicit laws that all instances should obey. The laws for Functor are: fmap id = id fmap (f. g) = fmap f. fmap g Lecture 1 Tim Sheard 15
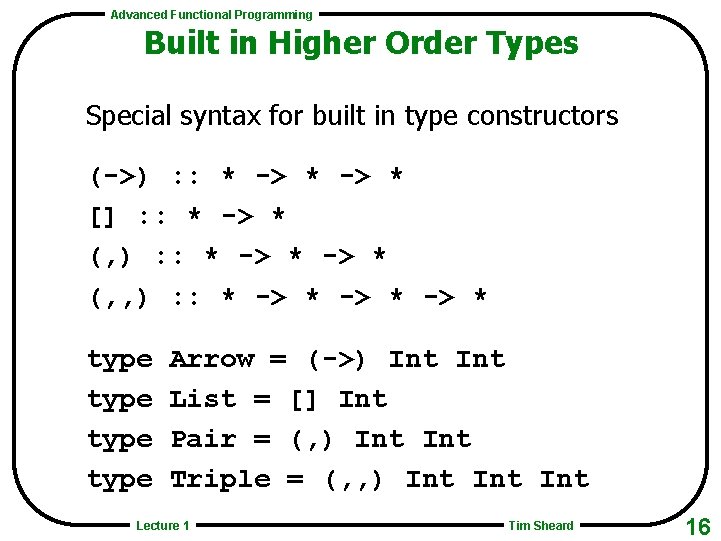
Advanced Functional Programming Built in Higher Order Types Special syntax for built in type constructors (->) : : * -> * [] : : * -> * (, ) : : * -> * (, , ) : : * -> * type Arrow = (->) Int List = [] Int Pair = (, ) Int Triple = (, , ) Int Int Lecture 1 Tim Sheard 16
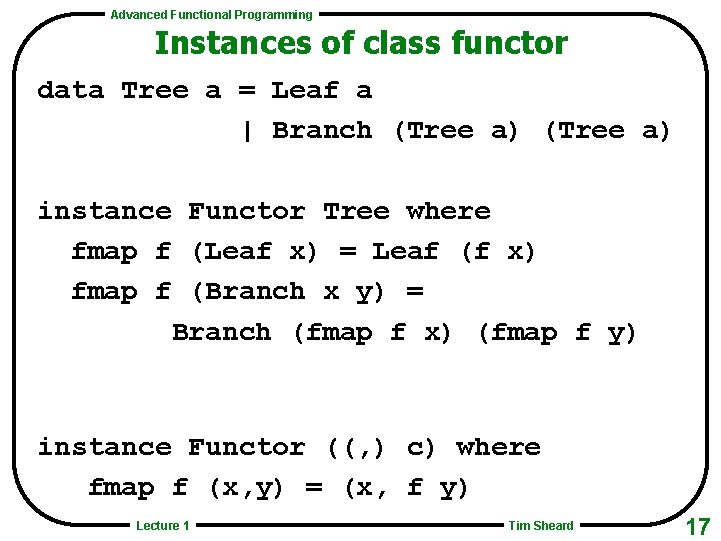
Advanced Functional Programming Instances of class functor data Tree a = Leaf a | Branch (Tree a) instance Functor Tree where fmap f (Leaf x) = Leaf (f x) fmap f (Branch x y) = Branch (fmap f x) (fmap f y) instance Functor ((, ) c) where fmap f (x, y) = (x, f y) Lecture 1 Tim Sheard 17
![Advanced Functional Programming More Instances instance Functor where fmap f Advanced Functional Programming More Instances instance Functor [] where fmap f [] = []](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-18.jpg)
Advanced Functional Programming More Instances instance Functor [] where fmap f [] = [] fmap f (x: xs) = f x : fmap f xs instance Functor Maybe where fmap f Nothing = Nothing fmap f (Just x) = Just (f x) Lecture 1 Tim Sheard 18
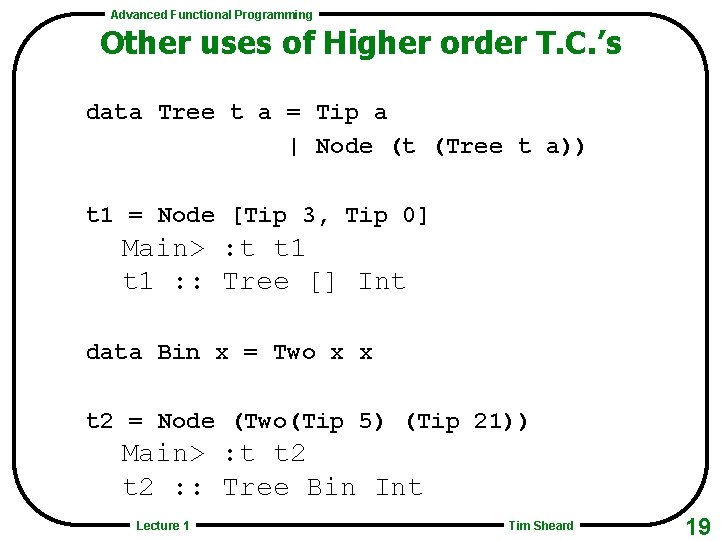
Advanced Functional Programming Other uses of Higher order T. C. ’s data Tree t a = Tip a | Node (t (Tree t a)) t 1 = Node [Tip 3, Tip 0] Main> : t t 1 : : Tree [] Int data Bin x = Two x x t 2 = Node (Two(Tip 5) (Tip 21)) Main> : t t 2 : : Tree Bin Int Lecture 1 Tim Sheard 19
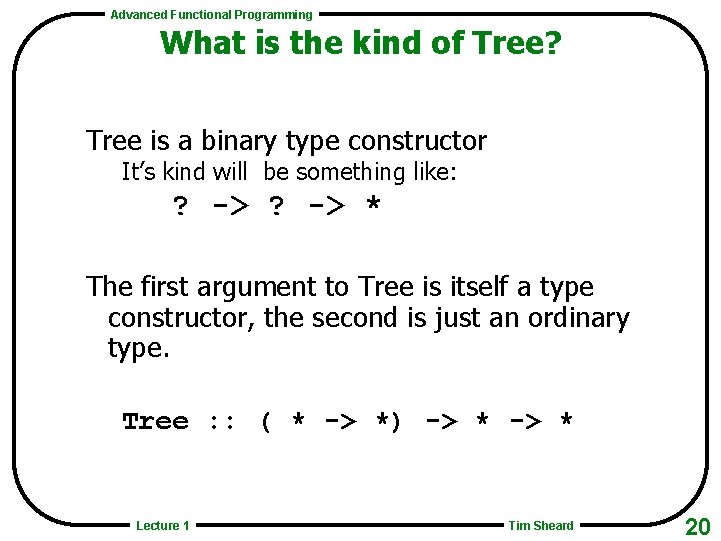
Advanced Functional Programming What is the kind of Tree? Tree is a binary type constructor It’s kind will be something like: ? -> * The first argument to Tree is itself a type constructor, the second is just an ordinary type. Tree : : ( * -> *) -> * Lecture 1 Tim Sheard 20
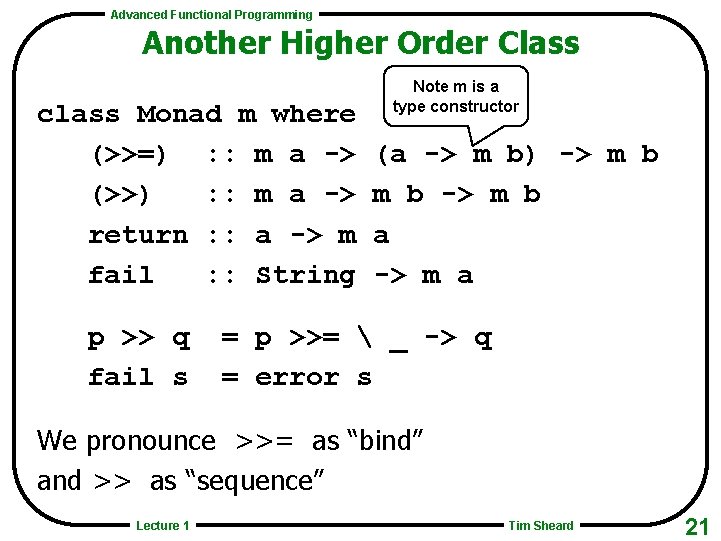
Advanced Functional Programming Another Higher Order Class class Monad m where (>>=) : : m a -> (>>) : : m a -> return : : a -> m fail : : String p >> q fail s Note m is a type constructor (a -> m b) -> m b a -> m a = p >>= _ -> q = error s We pronounce >>= as “bind” and >> as “sequence” Lecture 1 Tim Sheard 21
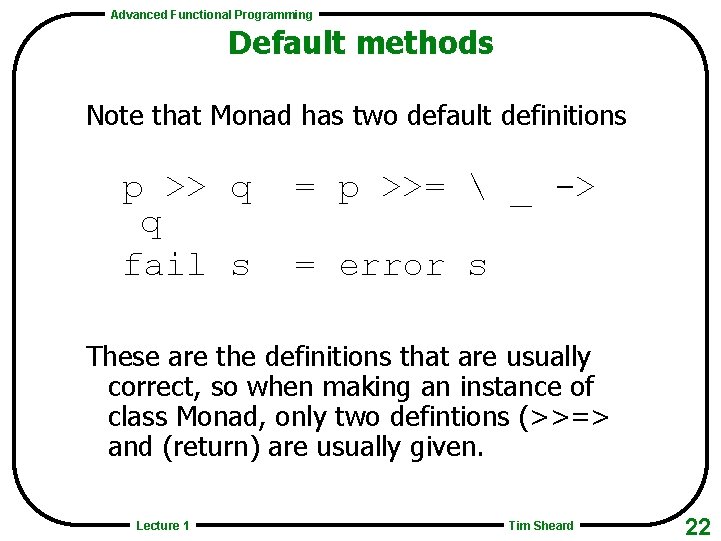
Advanced Functional Programming Default methods Note that Monad has two default definitions p >> q q fail s = p >>= _ -> = error s These are the definitions that are usually correct, so when making an instance of class Monad, only two defintions (>>=> and (return) are usually given. Lecture 1 Tim Sheard 22
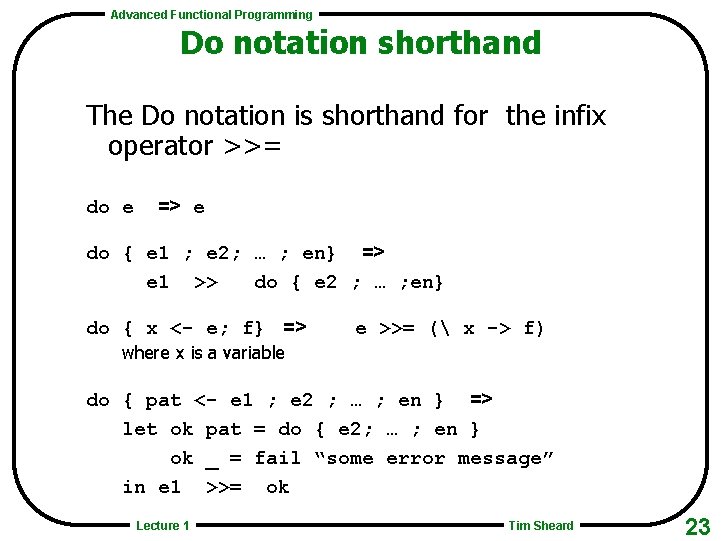
Advanced Functional Programming Do notation shorthand The Do notation is shorthand for the infix operator >>= do e => e do { e 1 ; e 2; … ; en} => e 1 >> do { e 2 ; … ; en} do { x <- e; f} => e >>= ( x -> f) where x is a variable do { pat <- e 1 ; e 2 ; … ; en } => let ok pat = do { e 2; … ; en } ok _ = fail “some error message” in e 1 >>= ok Lecture 1 Tim Sheard 23
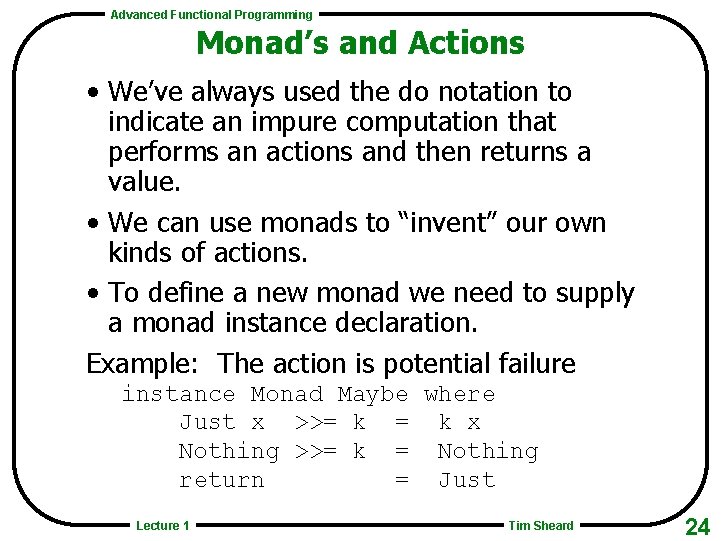
Advanced Functional Programming Monad’s and Actions • We’ve always used the do notation to indicate an impure computation that performs an actions and then returns a value. • We can use monads to “invent” our own kinds of actions. • To define a new monad we need to supply a monad instance declaration. Example: The action is potential failure instance Monad Maybe where Just x >>= k x Nothing >>= k = Nothing return = Just Lecture 1 Tim Sheard 24
![Advanced Functional Programming Example find Eq a a a b Advanced Functional Programming Example find : : Eq a => a -> [(a, b)]](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-25.jpg)
Advanced Functional Programming Example find : : Eq a => a -> [(a, b)] -> Maybe b find x [] = Nothing find x ((y, a): ys) = if x == y then Just a else find x ys test a c x = do { b <- find a x; return (c+b) } What is the type of test? What does it return if the find fails? Lecture 1 Tim Sheard 25
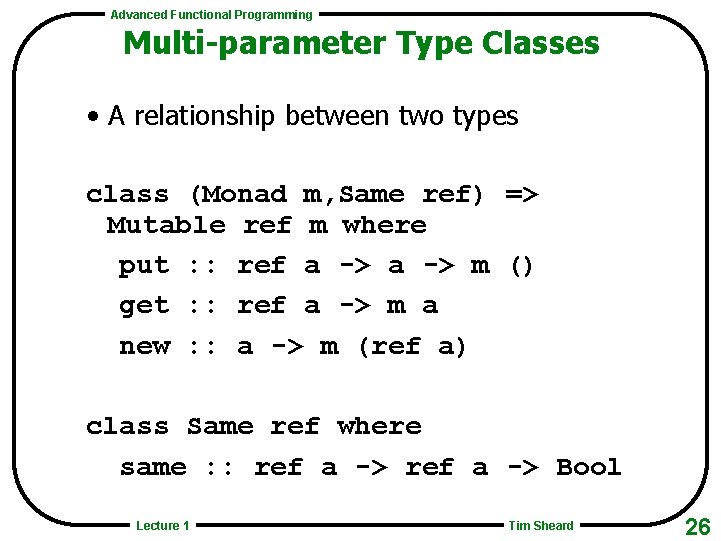
Advanced Functional Programming Multi-parameter Type Classes • A relationship between two types class (Monad m, Same ref) => Mutable ref m where put : : ref a -> m () get : : ref a -> m a new : : a -> m (ref a) class Same ref where same : : ref a -> Bool Lecture 1 Tim Sheard 26
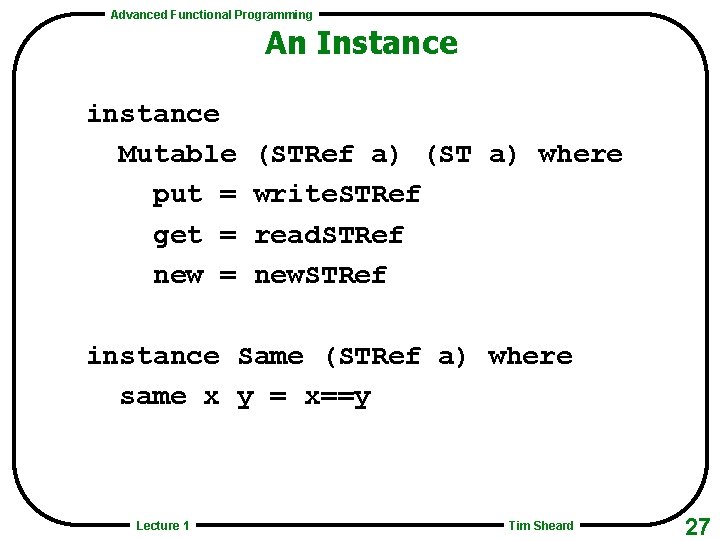
Advanced Functional Programming An Instance instance Mutable put = get = new = (STRef a) (ST a) where write. STRef read. STRef new. STRef instance Same (STRef a) where same x y = x==y Lecture 1 Tim Sheard 27
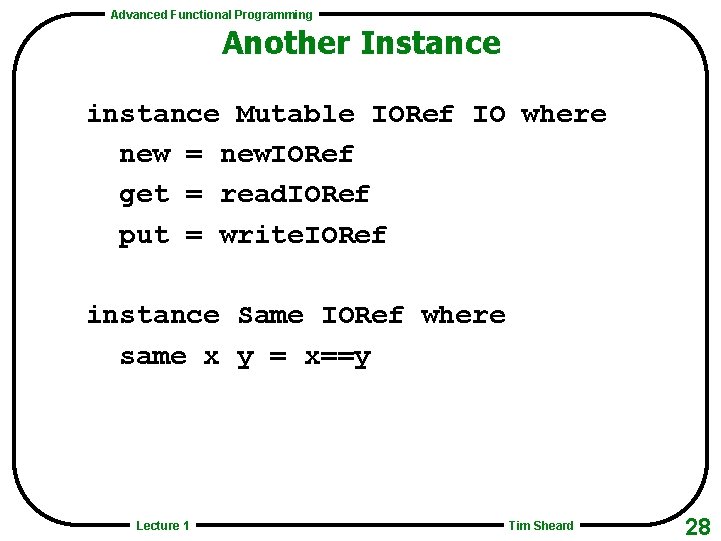
Advanced Functional Programming Another Instance instance Mutable IORef IO where new = new. IORef get = read. IORef put = write. IORef instance Same IORef where same x y = x==y Lecture 1 Tim Sheard 28
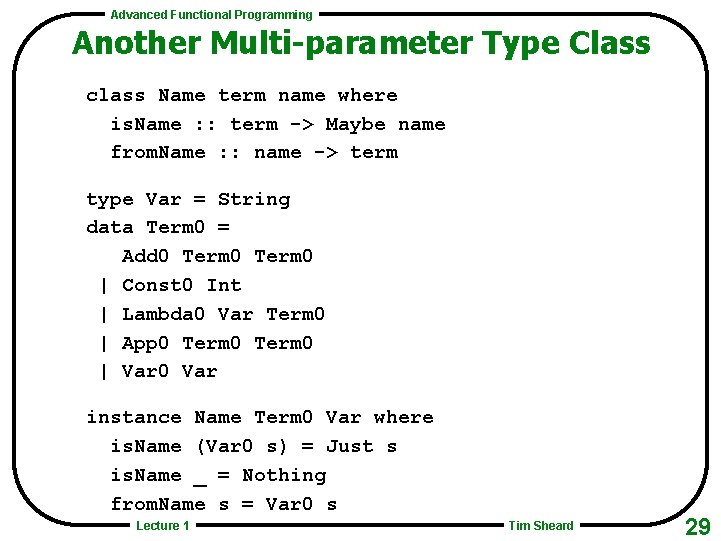
Advanced Functional Programming Another Multi-parameter Type Class class Name term name where is. Name : : term -> Maybe name from. Name : : name -> term type Var = String data Term 0 = Add 0 Term 0 | Const 0 Int | Lambda 0 Var Term 0 | App 0 Term 0 | Var 0 Var instance Name Term 0 Var where is. Name (Var 0 s) = Just s is. Name _ = Nothing from. Name s = Var 0 s Lecture 1 Tim Sheard 29
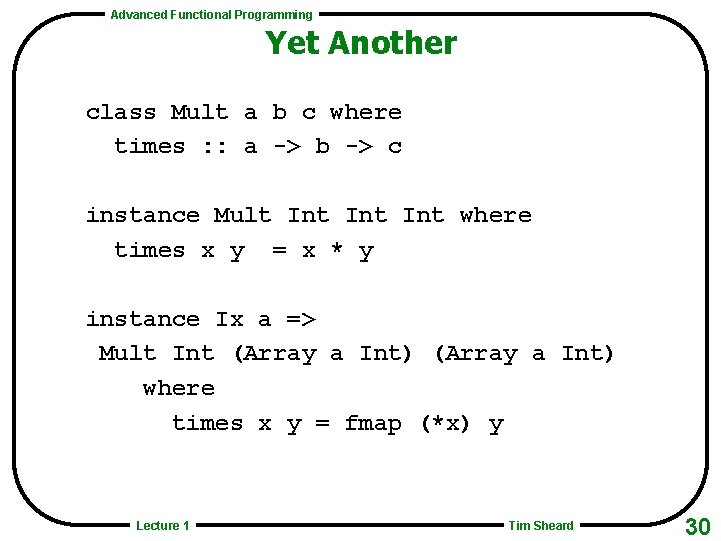
Advanced Functional Programming Yet Another class Mult a b c where times : : a -> b -> c instance Mult Int Int where times x y = x * y instance Ix a => Mult Int (Array a Int) where times x y = fmap (*x) y Lecture 1 Tim Sheard 30
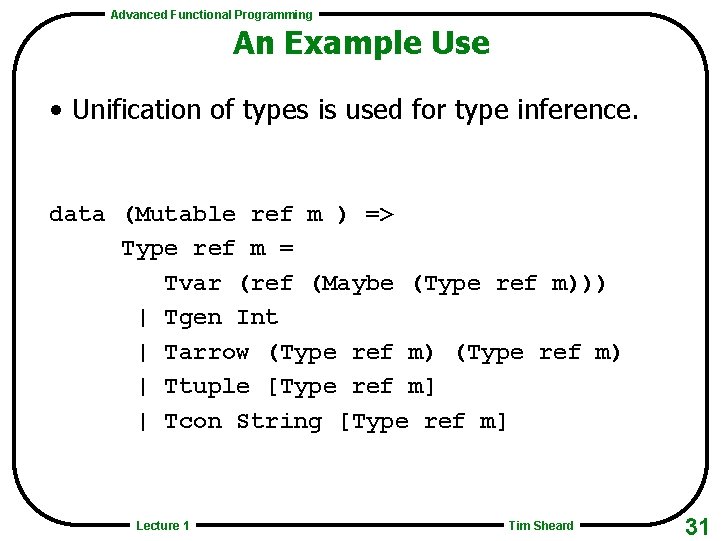
Advanced Functional Programming An Example Use • Unification of types is used for type inference. data (Mutable ref m ) => Type ref m = Tvar (ref (Maybe (Type ref m))) | Tgen Int | Tarrow (Type ref m) | Ttuple [Type ref m] | Tcon String [Type ref m] Lecture 1 Tim Sheard 31
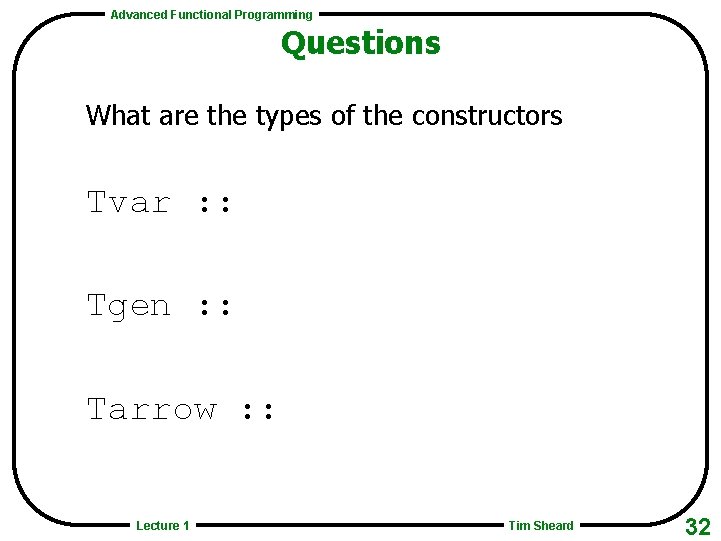
Advanced Functional Programming Questions What are the types of the constructors Tvar : : Tgen : : Tarrow : : Lecture 1 Tim Sheard 32
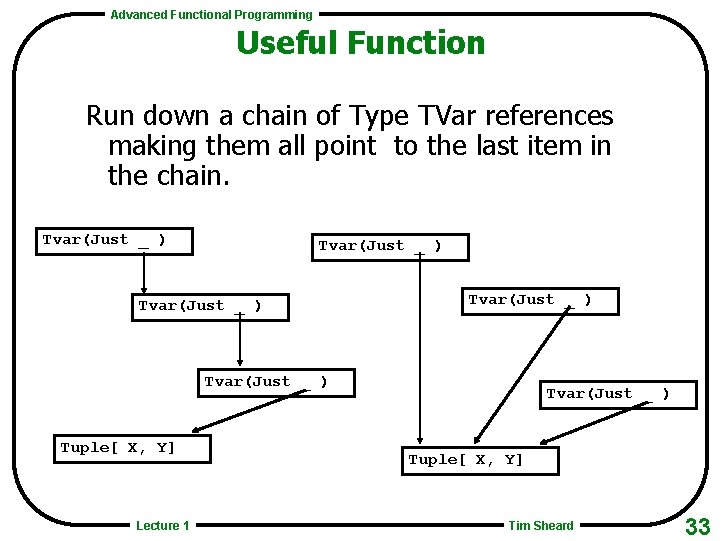
Advanced Functional Programming Useful Function Run down a chain of Type TVar references making them all point to the last item in the chain. Tvar(Just _ ) Tvar(Just _ ) Tuple[ X, Y] Lecture 1 Tvar(Just _ ) Tuple[ X, Y] Tim Sheard 33
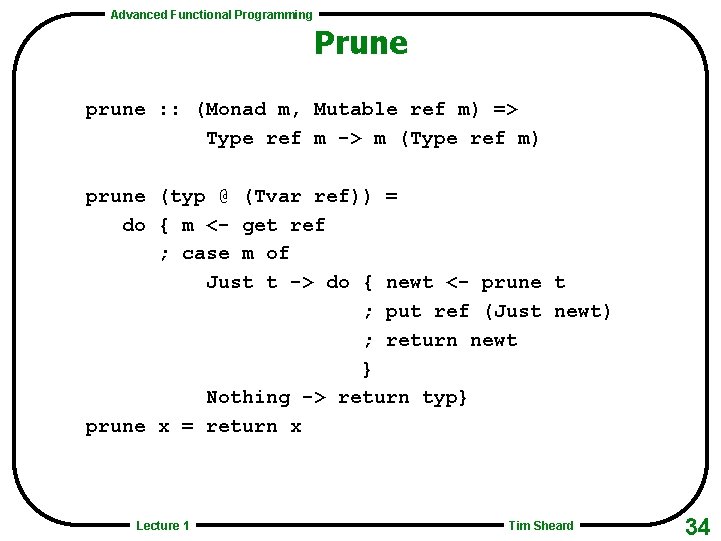
Advanced Functional Programming Prune prune : : (Monad m, Mutable ref m) => Type ref m -> m (Type ref m) prune (typ @ (Tvar ref)) = do { m <- get ref ; case m of Just t -> do { newt <- prune t ; put ref (Just newt) ; return newt } Nothing -> return typ} prune x = return x Lecture 1 Tim Sheard 34
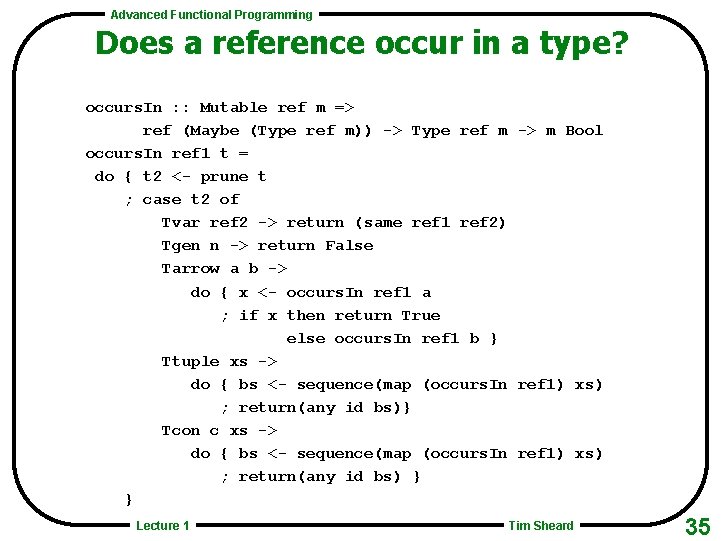
Advanced Functional Programming Does a reference occur in a type? occurs. In : : Mutable ref m => ref (Maybe (Type ref m)) -> Type ref m -> m Bool occurs. In ref 1 t = do { t 2 <- prune t ; case t 2 of Tvar ref 2 -> return (same ref 1 ref 2) Tgen n -> return False Tarrow a b -> do { x <- occurs. In ref 1 a ; if x then return True else occurs. In ref 1 b } Ttuple xs -> do { bs <- sequence(map (occurs. In ref 1) xs) ; return(any id bs)} Tcon c xs -> do { bs <- sequence(map (occurs. In ref 1) xs) ; return(any id bs) } } Lecture 1 Tim Sheard 35
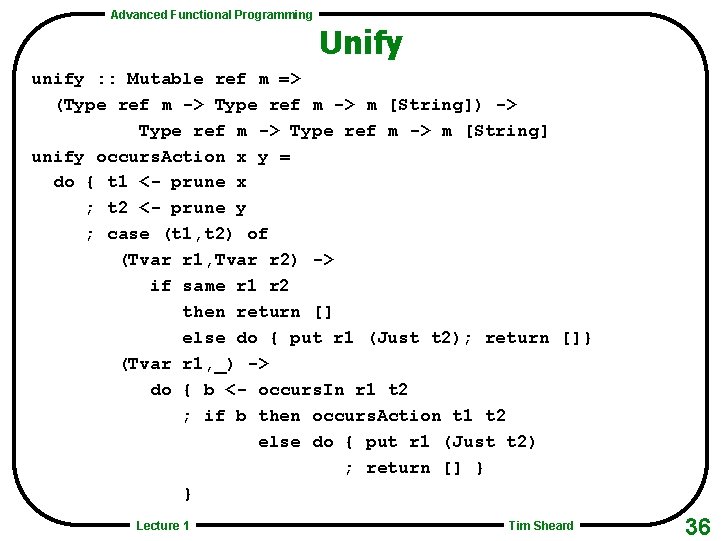
Advanced Functional Programming Unify unify : : Mutable ref m => (Type ref m -> m [String]) -> Type ref m -> m [String] unify occurs. Action x y = do { t 1 <- prune x ; t 2 <- prune y ; case (t 1, t 2) of (Tvar r 1, Tvar r 2) -> if same r 1 r 2 then return [] else do { put r 1 (Just t 2); return []} (Tvar r 1, _) -> do { b <- occurs. In r 1 t 2 ; if b then occurs. Action t 1 t 2 else do { put r 1 (Just t 2) ; return [] } } Lecture 1 Tim Sheard 36
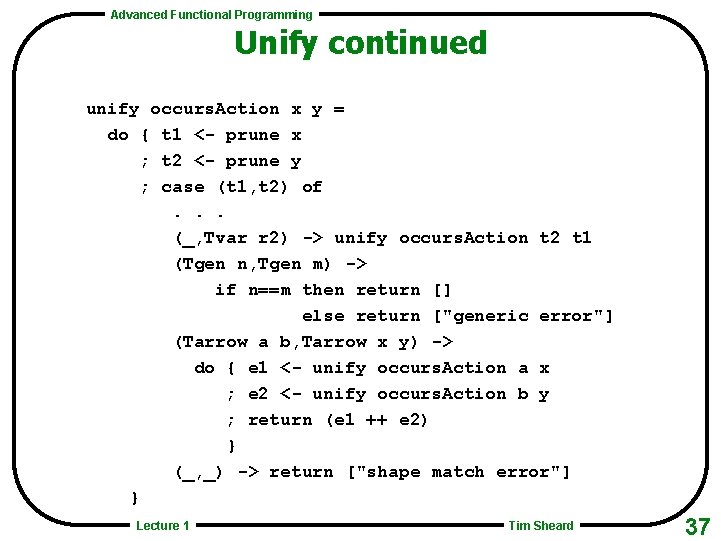
Advanced Functional Programming Unify continued unify occurs. Action x y = do { t 1 <- prune x ; t 2 <- prune y ; case (t 1, t 2) of. . . (_, Tvar r 2) -> unify occurs. Action t 2 t 1 (Tgen n, Tgen m) -> if n==m then return [] else return ["generic error"] (Tarrow a b, Tarrow x y) -> do { e 1 <- unify occurs. Action a x ; e 2 <- unify occurs. Action b y ; return (e 1 ++ e 2) } (_, _) -> return ["shape match error"] } Lecture 1 Tim Sheard 37
![Advanced Functional Programming Generic Monad Functions sequence Monad m m a Advanced Functional Programming Generic Monad Functions sequence : : Monad m => [m a]](https://slidetodoc.com/presentation_image_h/8c956412791b51552f3a52f60cbf47f3/image-38.jpg)
Advanced Functional Programming Generic Monad Functions sequence : : Monad m => [m a] -> m [a] sequence = foldr mcons (return []) where mcons p q = do { x <- p ; xs <- q ; return (x: xs) } map. M : : Monad m => (a -> m b) -> [a] -> m [b] map. M f as = sequence (map f as) Lecture 1 Tim Sheard 38
Tim sheard
Graduate institute of electronics engineering
Jill bamburg
Ntu
Eca graduate institute
Graduate institute of electronics engineering
Graduate institute of electronics engineering
Graduate institute of electronics engineering
Oregon forest resources institute
Passive space maintainer
Functional and non functional plasma enzymes
Enzymes of blood plasma
Functional and non functional
Advanced dynamic programming
Advanced internet programming
Design of assembler in system programming
Assembly language programming
Advanced programming in java
Procedural vs functional
Fundamentals of functional programming language
Functional programming scheme
Elm programming language
Functional programming roadmap
Lisp functional programming
Typed functional programming
Fundamentals of functional programming language
Xkcd programming languages
Cosc 4p41
Is lisp a functional programming language
Functional decomposition programming
Lisp functional programming
Functional programming
Sas institute v world programming
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Components of system programming
Linear vs integer programming
Programing adalah
Zeta phi beta membership intake process manual