15 213 Recitation 3 21102 Outline Stacks Procedures
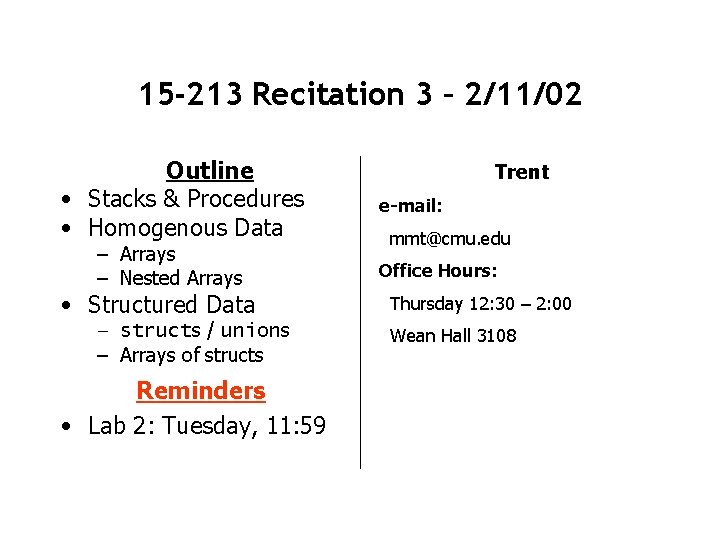
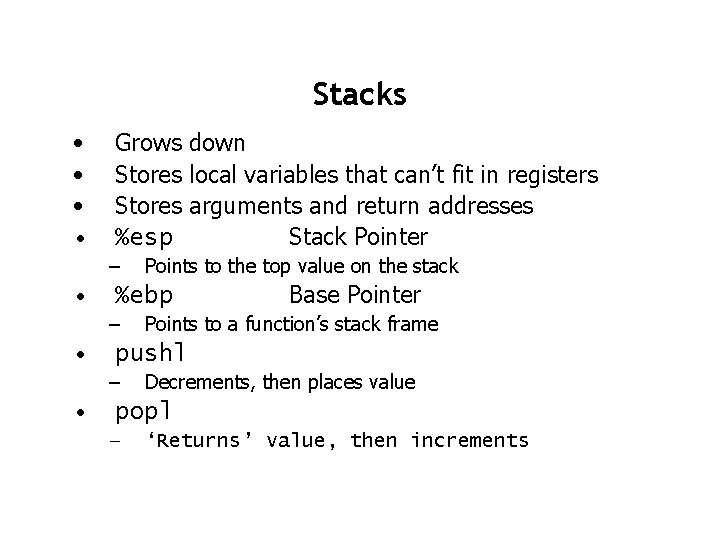
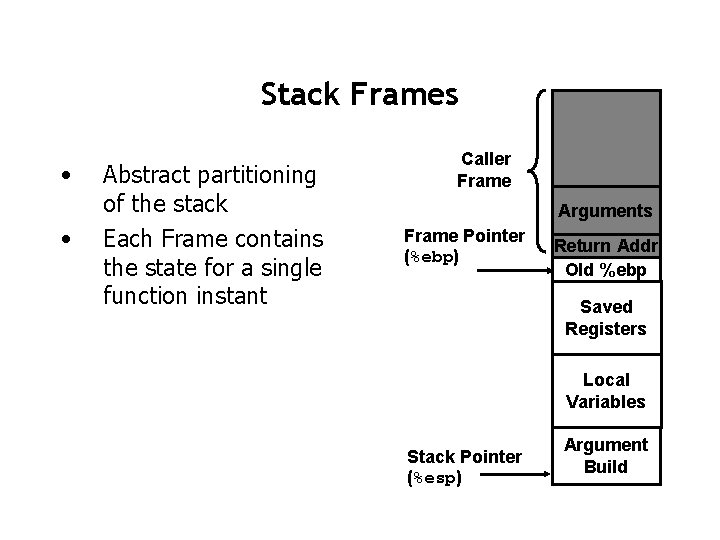
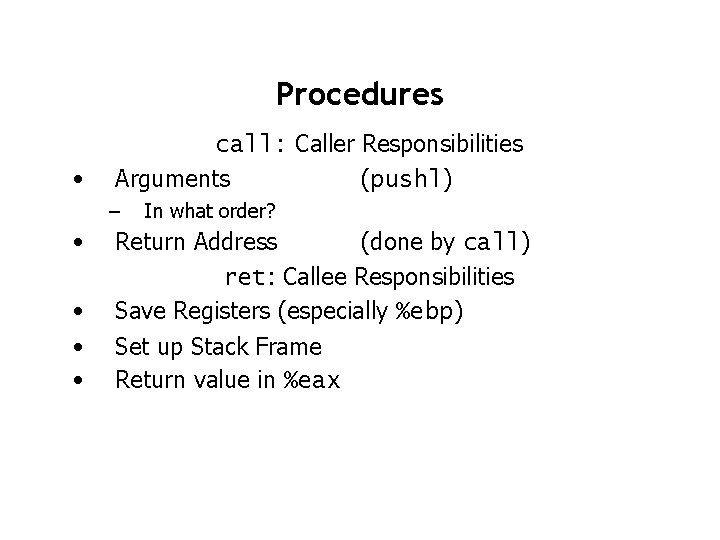
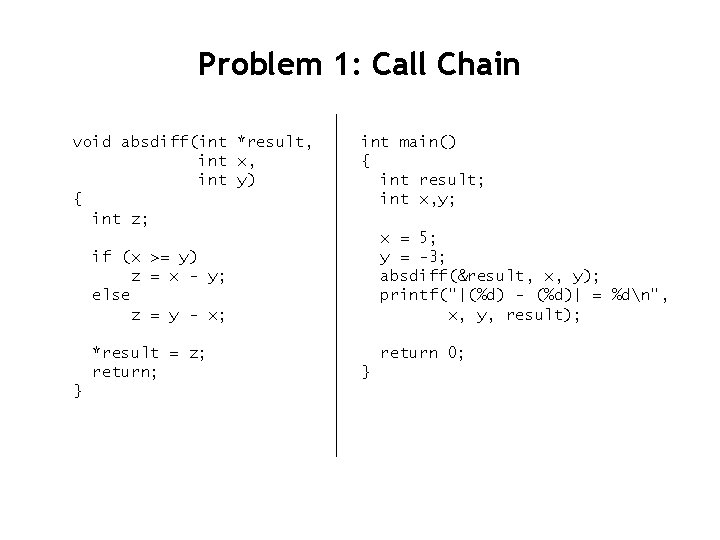
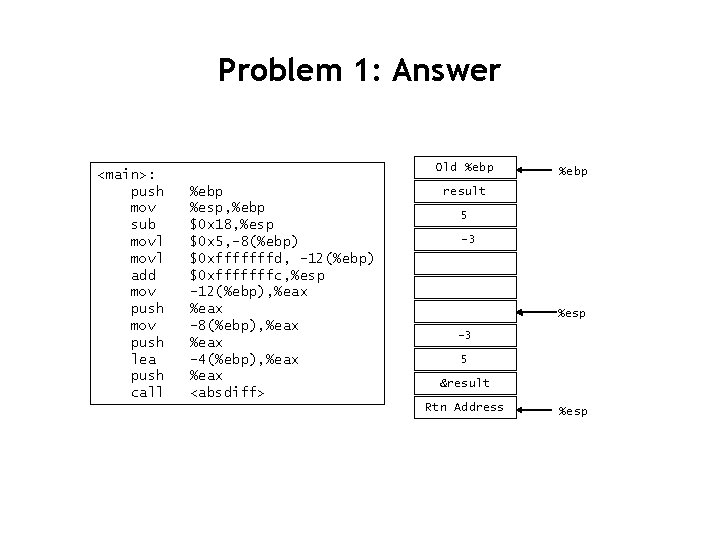
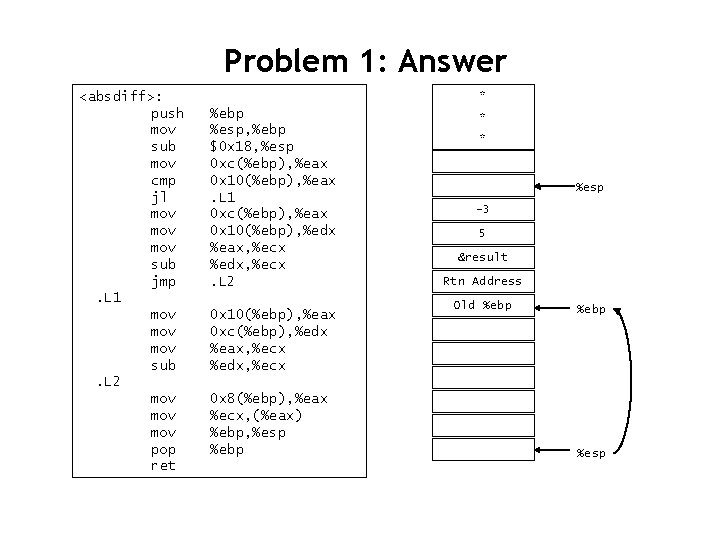
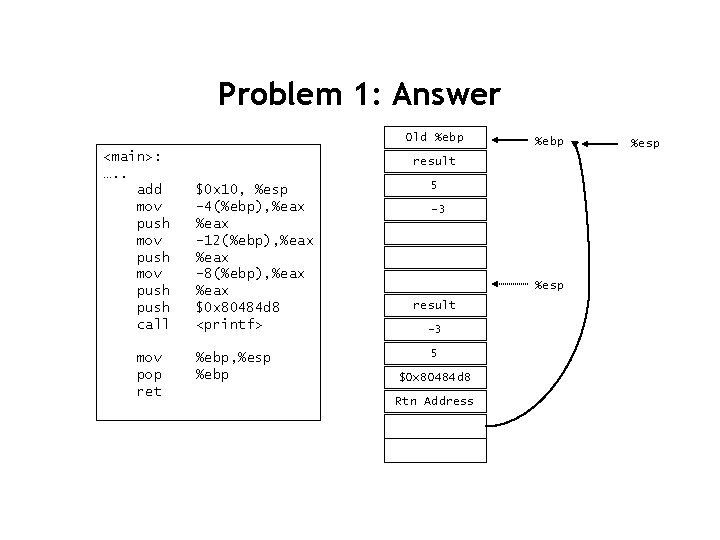
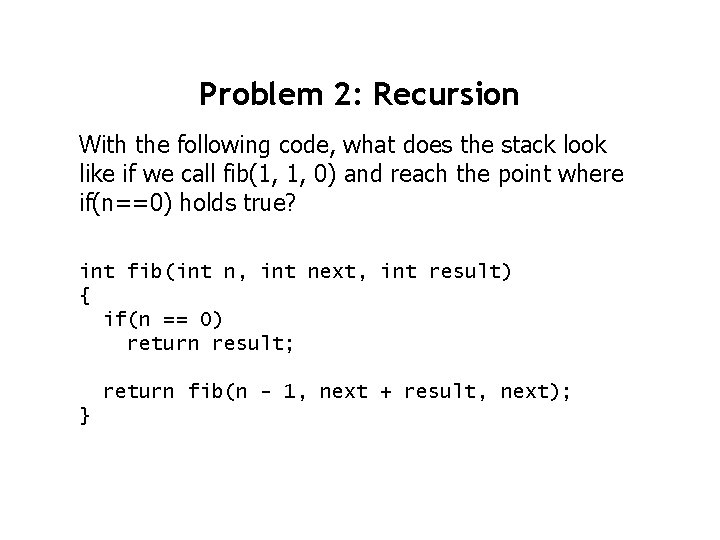
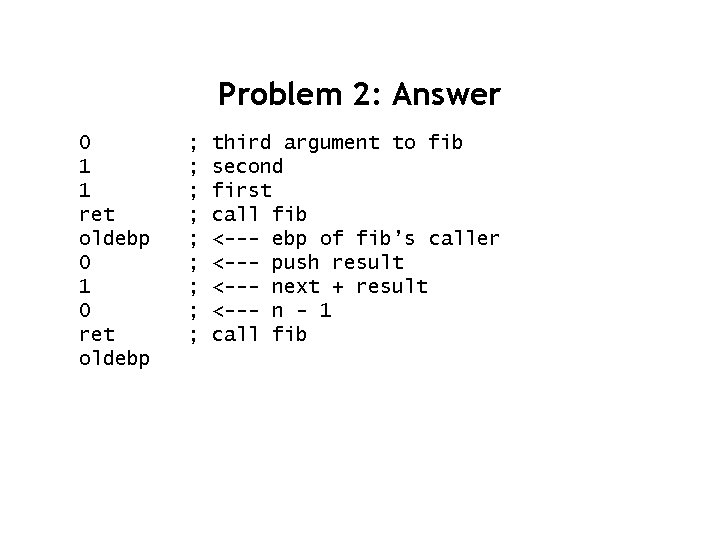
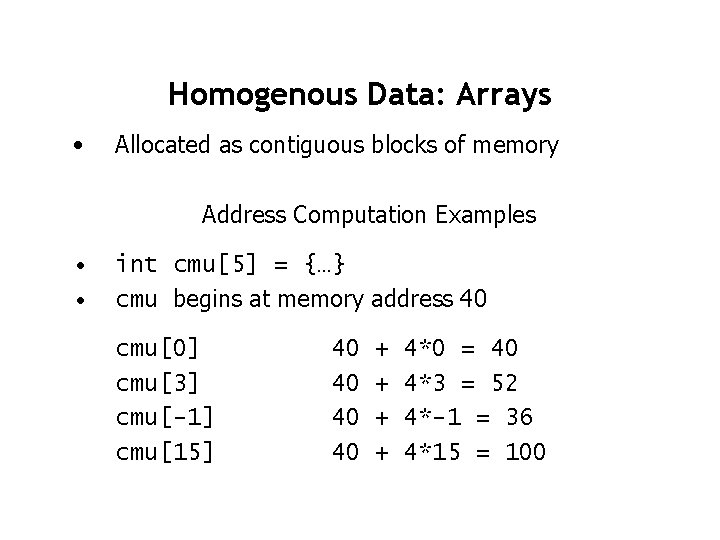
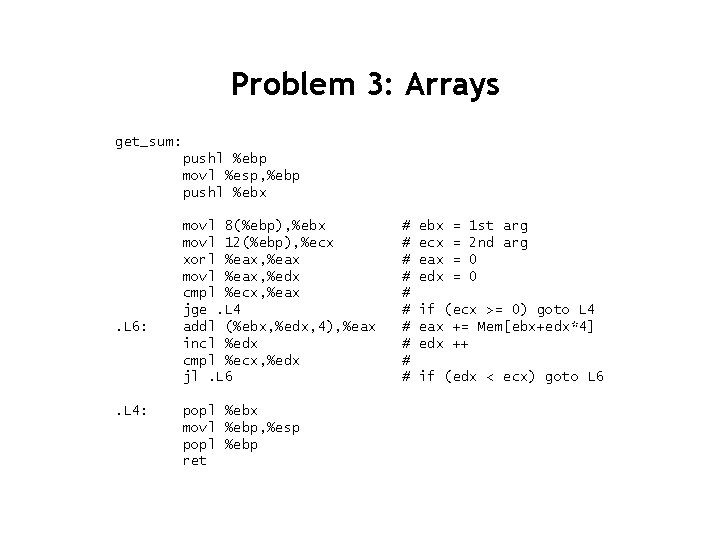
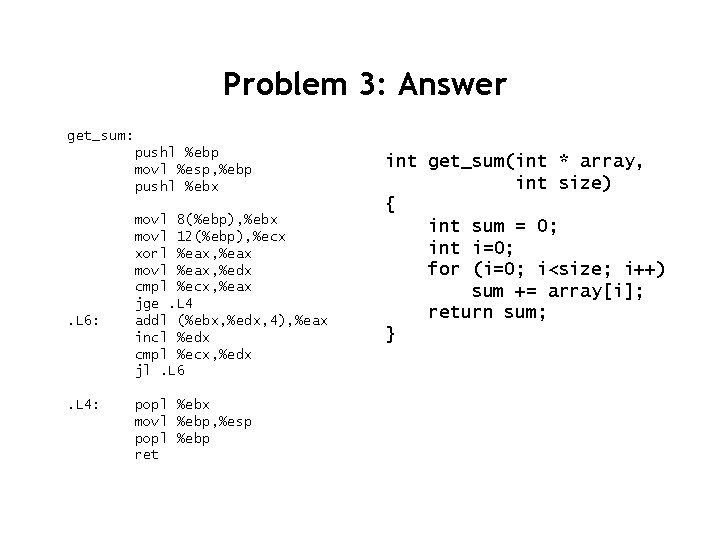
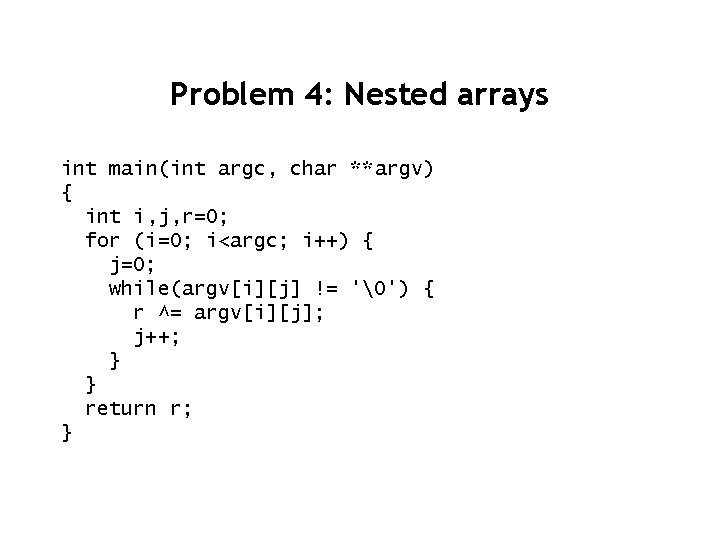
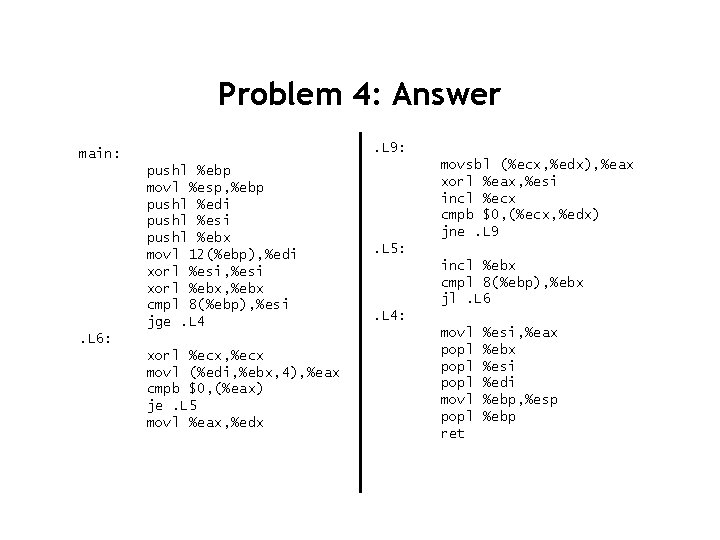
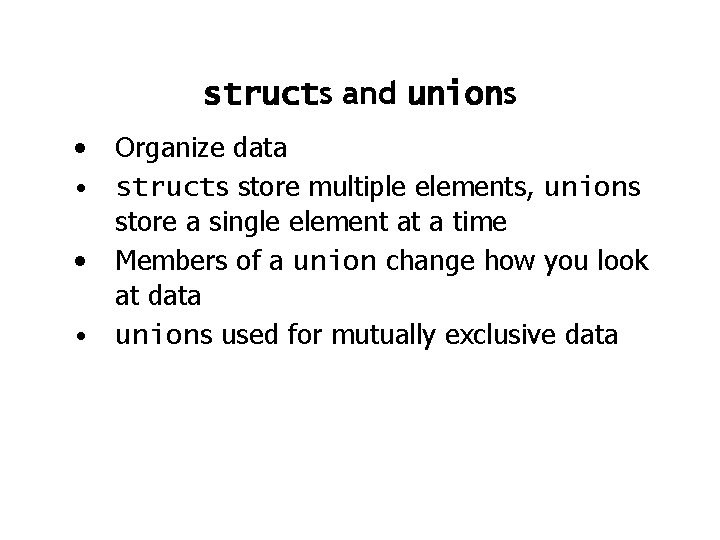
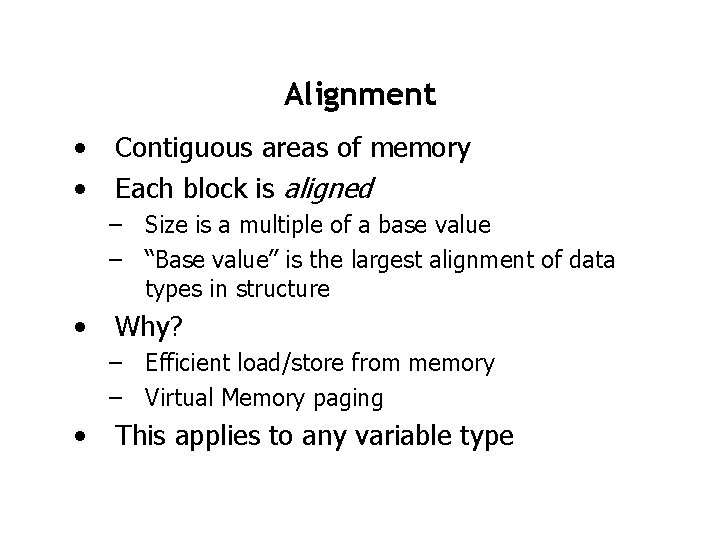
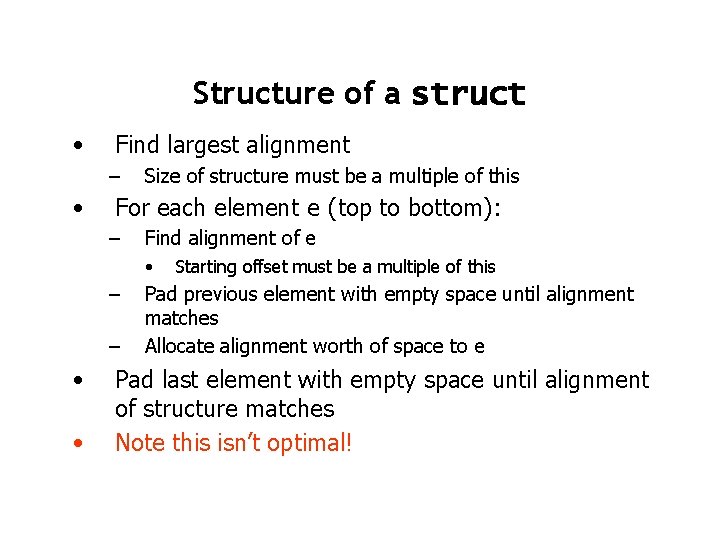
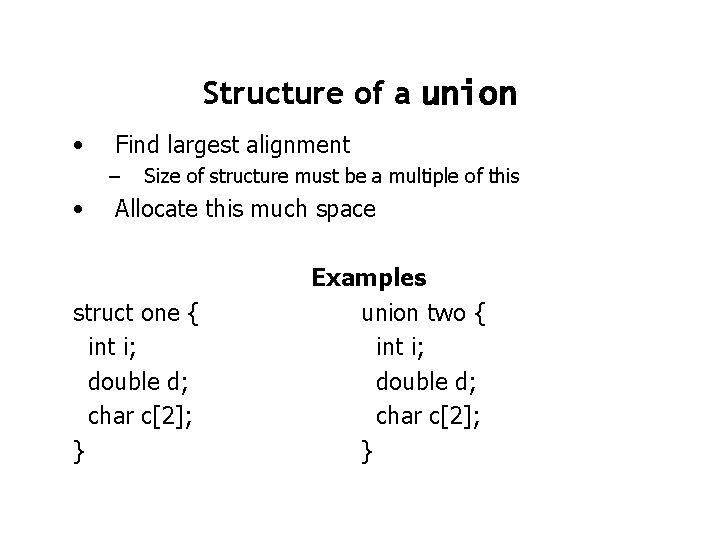
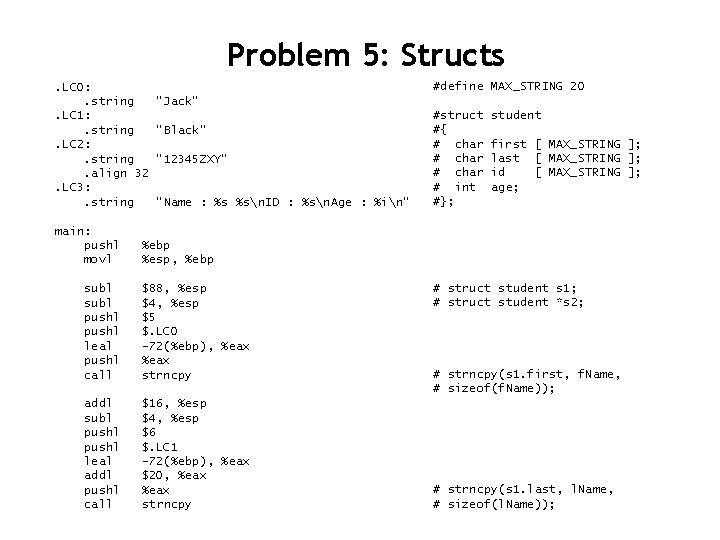
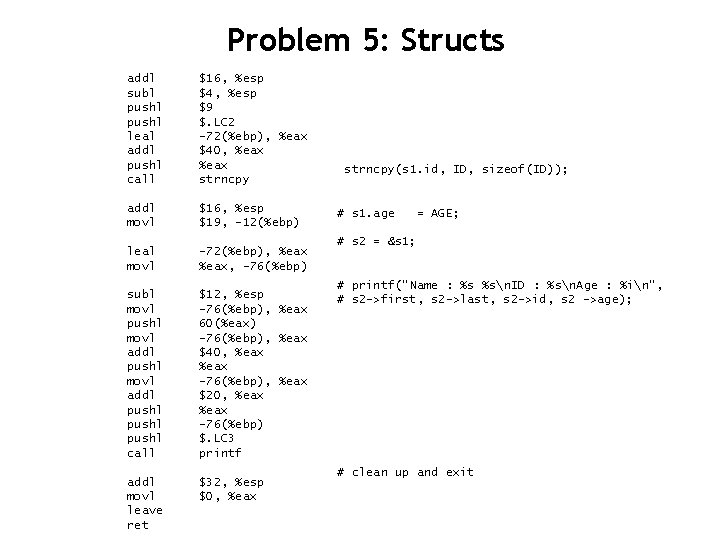
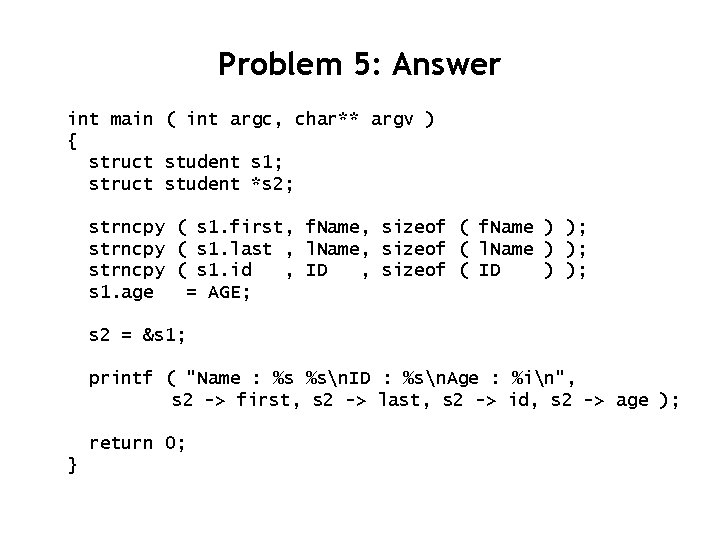
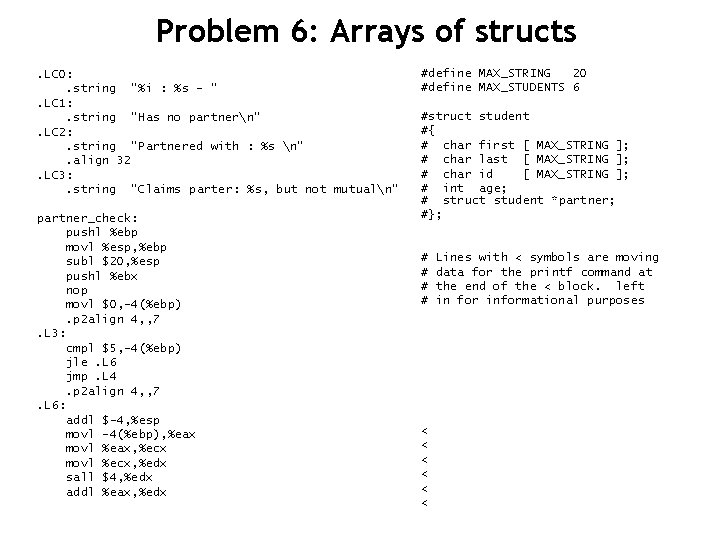
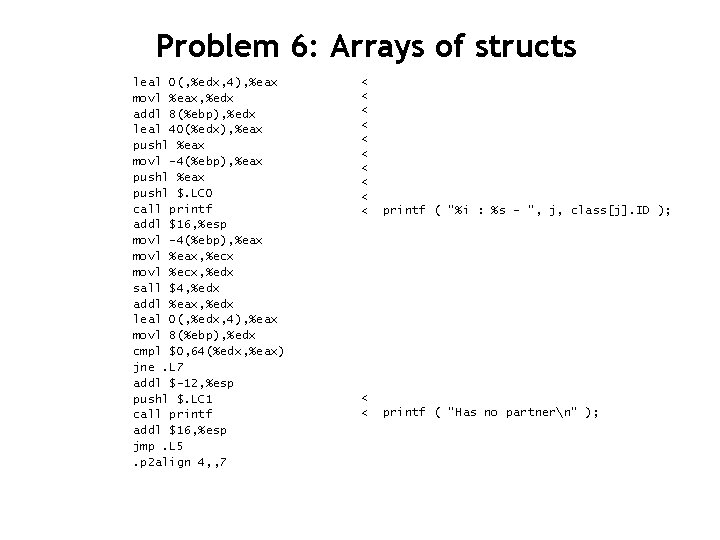
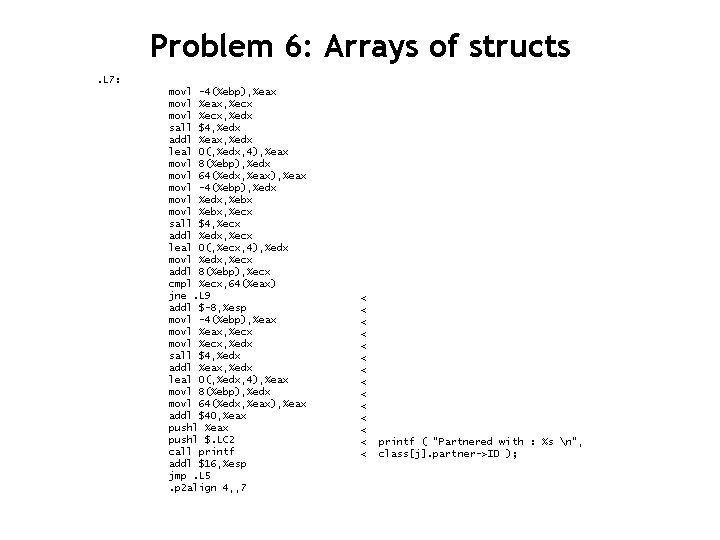
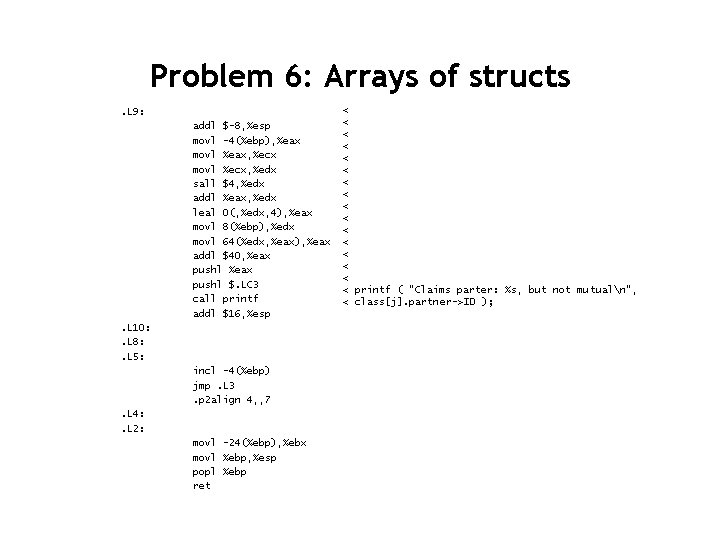
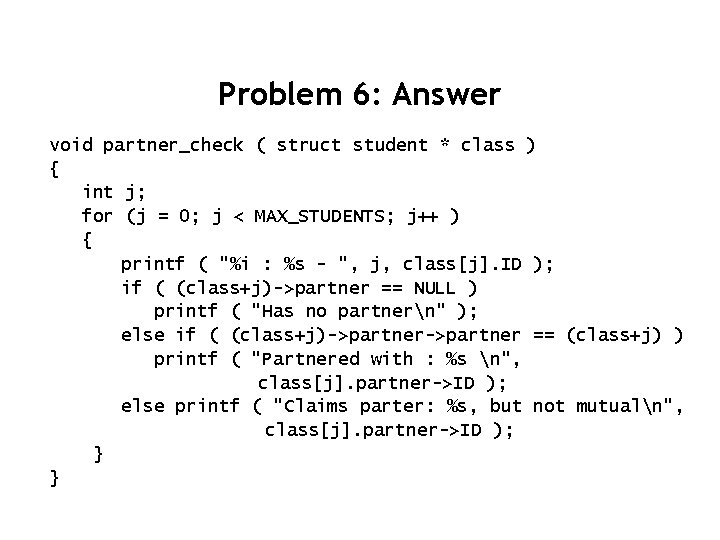
- Slides: 27
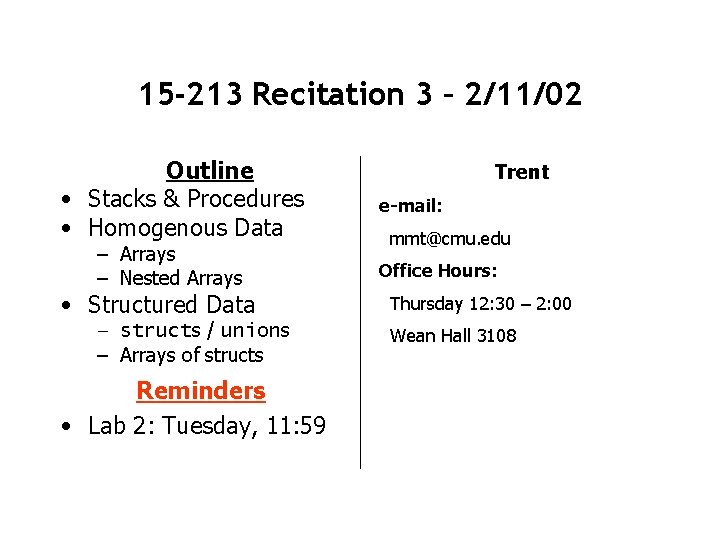
15 -213 Recitation 3 – 2/11/02 Outline • Stacks & Procedures • Homogenous Data – Arrays – Nested Arrays • Structured Data – structs / unions – Arrays of structs Reminders • Lab 2: Tuesday, 11: 59 Trent e-mail: mmt@cmu. edu Office Hours: Thursday 12: 30 – 2: 00 Wean Hall 3108
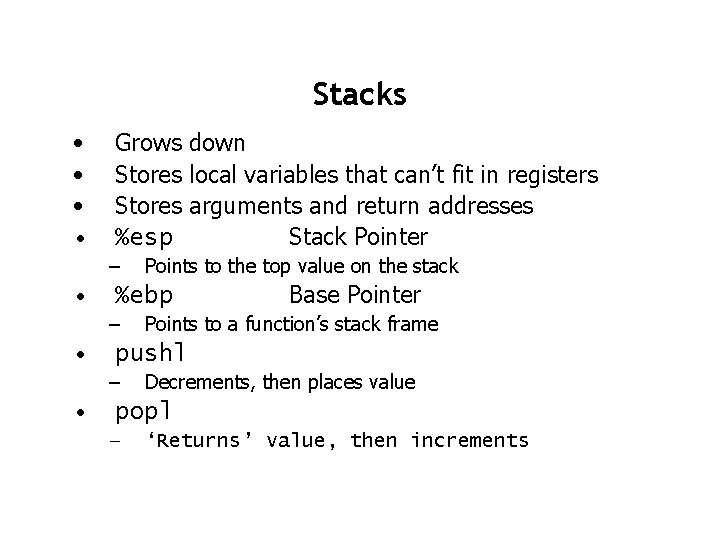
Stacks • • Grows down Stores local variables that can’t fit in registers Stores arguments and return addresses %esp Stack Pointer – • %ebp – • Base Pointer Points to a function’s stack frame pushl – • Points to the top value on the stack Decrements, then places value popl – ‘Returns’ value, then increments
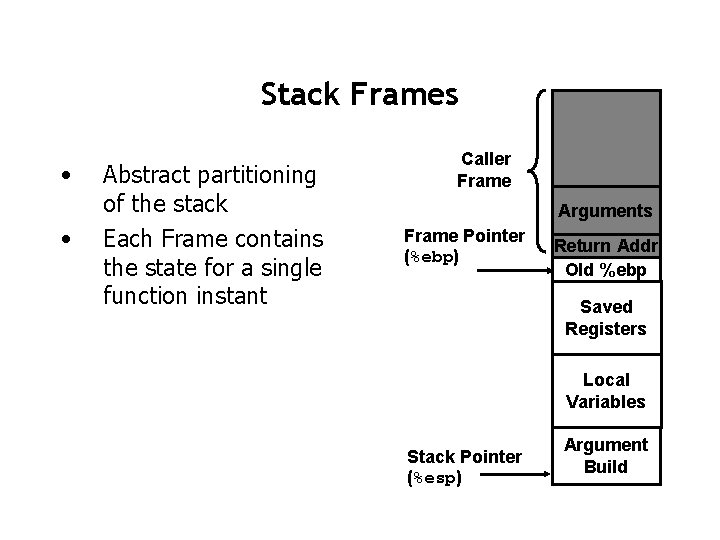
Stack Frames • • Abstract partitioning of the stack Each Frame contains the state for a single function instant Caller Frame Arguments Frame Pointer (%ebp) Return Addr Old %ebp Saved Registers Local Variables Stack Pointer (%esp) Argument Build
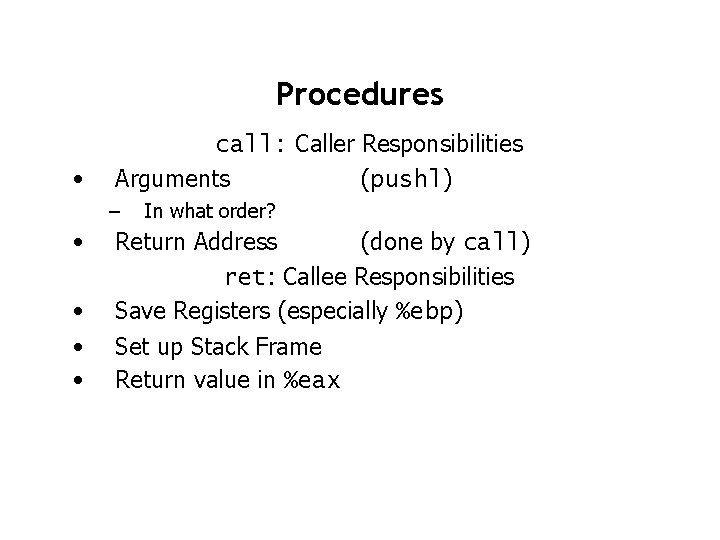
Procedures • call: Caller Responsibilities Arguments (pushl) – • • In what order? Return Address (done by call) ret: Callee Responsibilities Save Registers (especially %ebp) Set up Stack Frame Return value in %eax
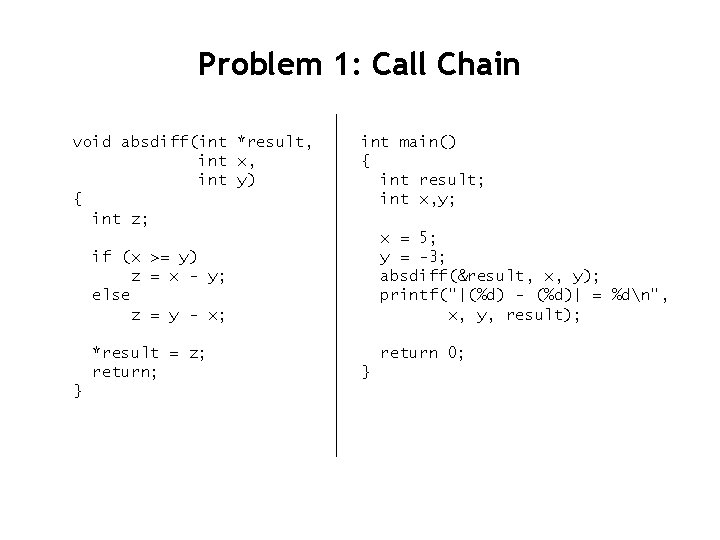
Problem 1: Call Chain void absdiff(int *result, int x, int y) { int z; int main() { int result; int x, y; x = 5; y = -3; absdiff(&result, x, y); printf("|(%d) - (%d)| = %dn", x, y, result); if (x >= y) z = x - y; else z = y - x; *result = z; return; } return 0; }
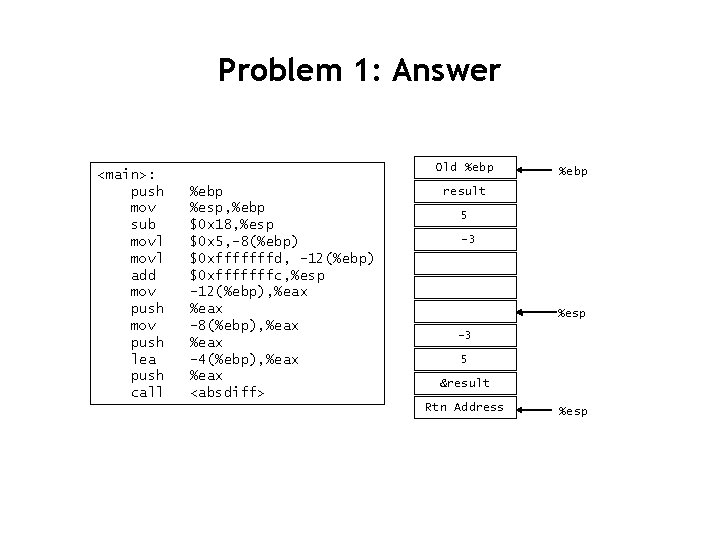
Problem 1: Answer <main>: push mov sub movl add mov push lea push call Old %ebp %esp, %ebp $0 x 18, %esp $0 x 5, -8(%ebp) $0 xfffffffd, -12(%ebp) $0 xfffffffc, %esp -12(%ebp), %eax -8(%ebp), %eax -4(%ebp), %eax <absdiff> %ebp result 5 -3 %esp -3 5 &result Rtn Address %esp
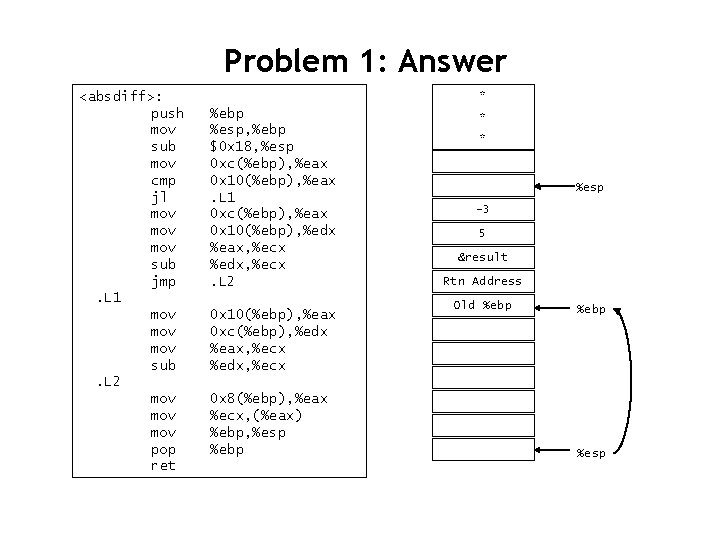
Problem 1: Answer <absdiff>: push mov sub mov cmp jl mov mov sub jmp. L 1 mov mov sub. L 2 mov mov pop ret * %ebp %esp, %ebp $0 x 18, %esp 0 xc(%ebp), %eax 0 x 10(%ebp), %eax. L 1 0 xc(%ebp), %eax 0 x 10(%ebp), %edx %eax, %ecx %edx, %ecx. L 2 0 x 10(%ebp), %eax 0 xc(%ebp), %edx %eax, %ecx %edx, %ecx 0 x 8(%ebp), %eax %ecx, (%eax) %ebp, %esp %ebp * * %esp -3 5 &result Rtn Address Old %ebp %esp
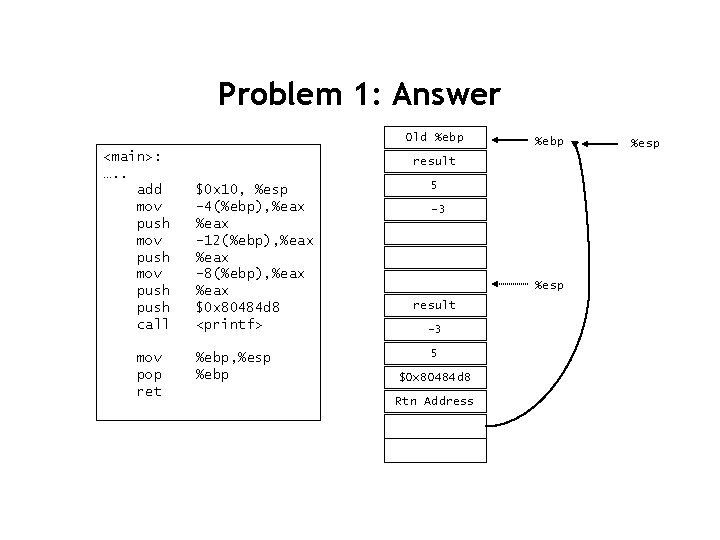
Problem 1: Answer Old %ebp <main>: …. . add mov push call mov pop ret %ebp result $0 x 10, %esp -4(%ebp), %eax -12(%ebp), %eax -8(%ebp), %eax $0 x 80484 d 8 <printf> %ebp, %esp %ebp 5 -3 %esp result -3 5 $0 x 80484 d 8 Rtn Address %esp
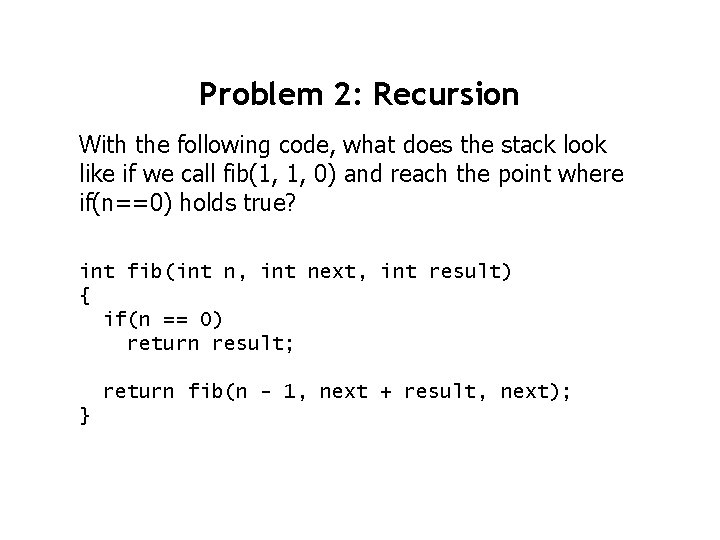
Problem 2: Recursion With the following code, what does the stack look like if we call fib(1, 1, 0) and reach the point where if(n==0) holds true? int fib(int n, int next, int result) { if(n == 0) return result; return fib(n - 1, next + result, next); }
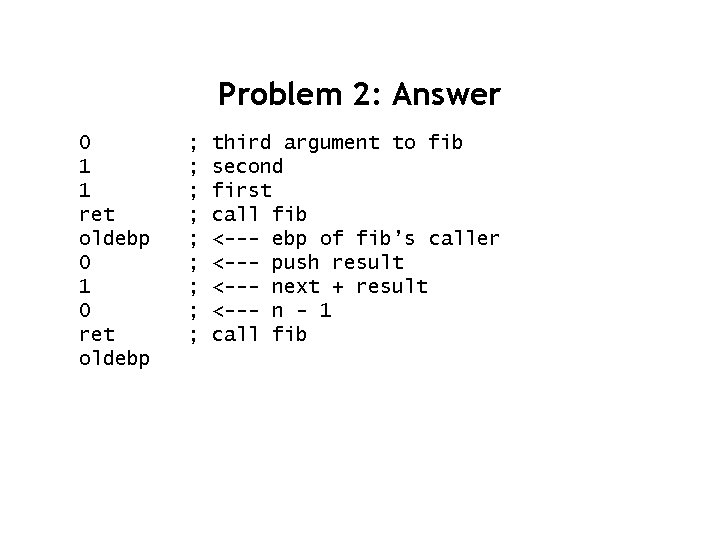
Problem 2: Answer 0 1 1 ret oldebp 0 1 0 ret oldebp ; ; ; ; ; third argument to fib second first call fib <--- ebp of fib’s caller <--- push result <--- next + result <--- n - 1 call fib
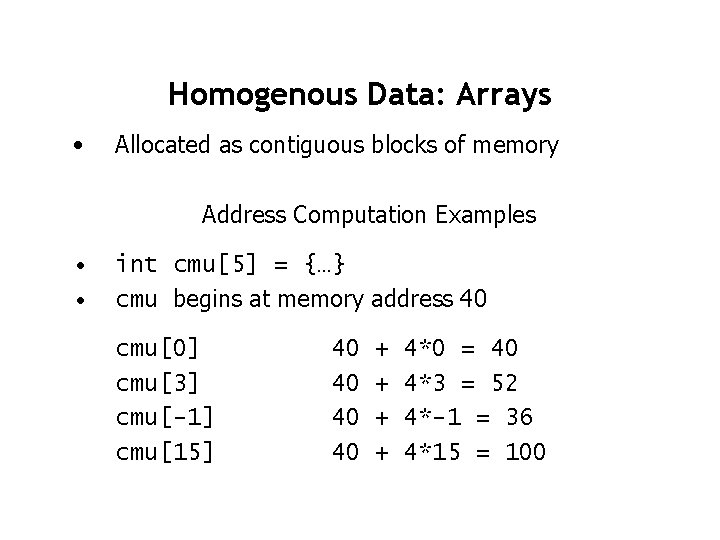
Homogenous Data: Arrays • Allocated as contiguous blocks of memory Address Computation Examples • • int cmu[5] = {…} cmu begins at memory address 40 cmu[0] cmu[3] cmu[-1] cmu[15] 40 40 + + 4*0 = 40 4*3 = 52 4*-1 = 36 4*15 = 100
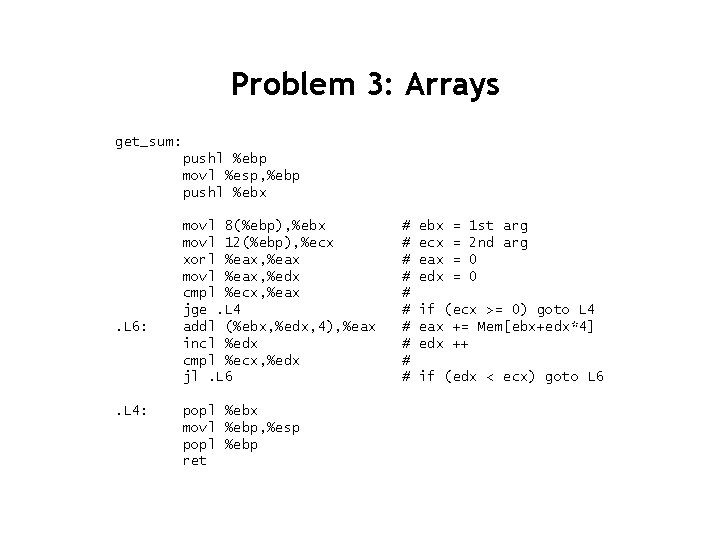
Problem 3: Arrays get_sum: pushl %ebp movl %esp, %ebp pushl %ebx . L 6: . L 4: movl 8(%ebp), %ebx movl 12(%ebp), %ecx xorl %eax, %eax movl %eax, %edx cmpl %ecx, %eax jge. L 4 addl (%ebx, %edx, 4), %eax incl %edx cmpl %ecx, %edx jl. L 6 popl %ebx movl %ebp, %esp popl %ebp ret # # # # # ebx ecx eax edx = = 1 st arg 2 nd arg 0 0 if (ecx >= 0) goto L 4 eax += Mem[ebx+edx*4] edx ++ if (edx < ecx) goto L 6
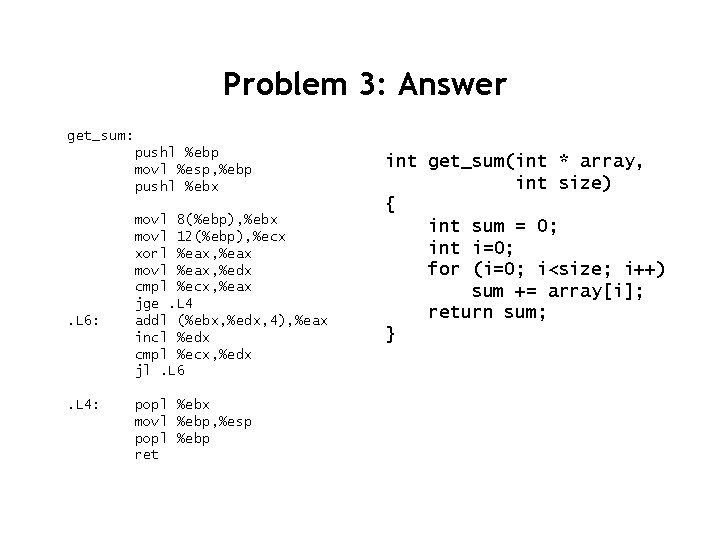
Problem 3: Answer get_sum: pushl %ebp movl %esp, %ebp pushl %ebx . L 6: . L 4: movl 8(%ebp), %ebx movl 12(%ebp), %ecx xorl %eax, %eax movl %eax, %edx cmpl %ecx, %eax jge. L 4 addl (%ebx, %edx, 4), %eax incl %edx cmpl %ecx, %edx jl. L 6 popl %ebx movl %ebp, %esp popl %ebp ret int get_sum(int * array, int size) { int sum = 0; int i=0; for (i=0; i<size; i++) sum += array[i]; return sum; }
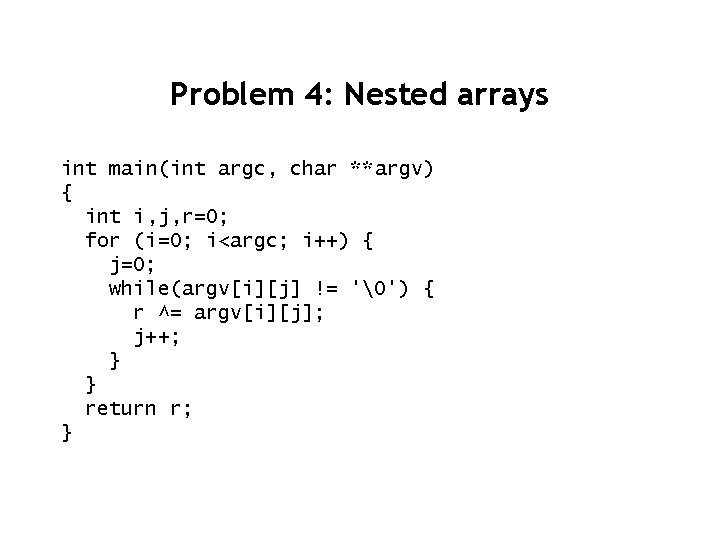
Problem 4: Nested arrays int main(int argc, char **argv) { int i, j, r=0; for (i=0; i<argc; i++) { j=0; while(argv[i][j] != '