15 213 Recitation 2 2401 Outline Machine Model
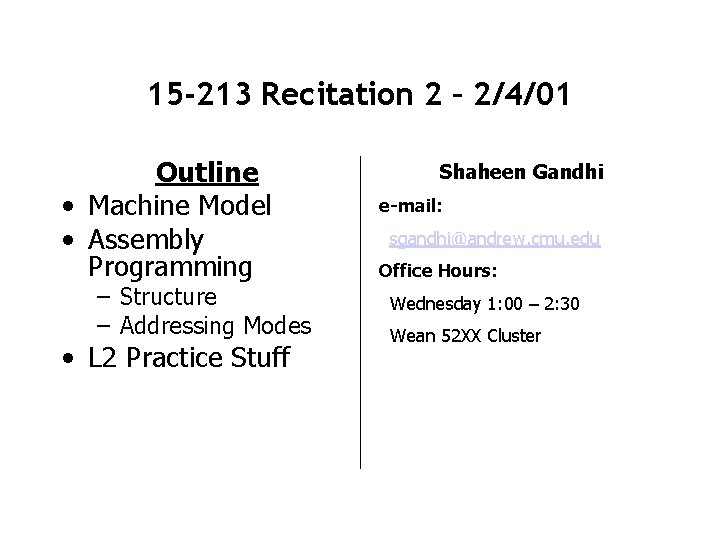
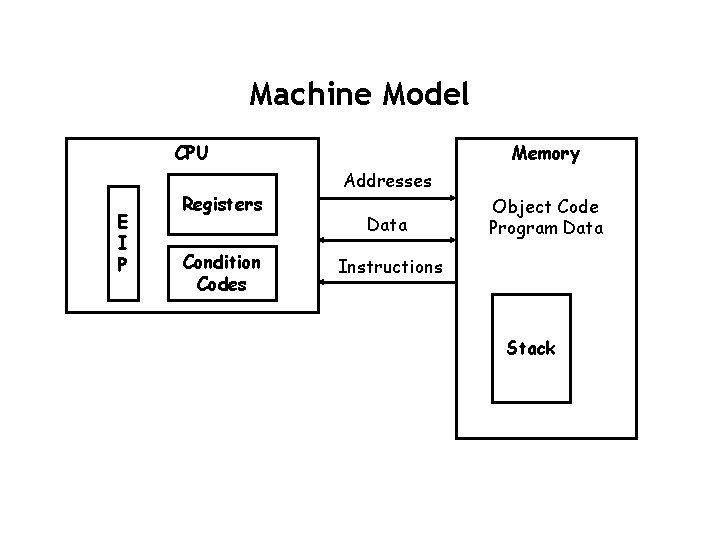
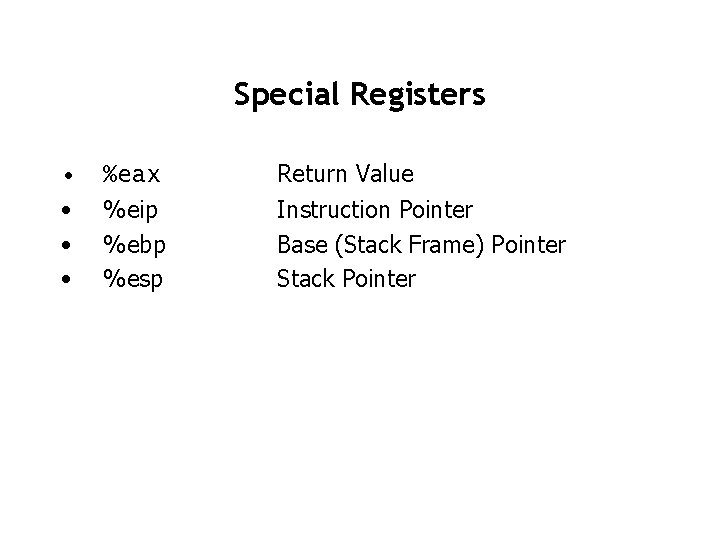
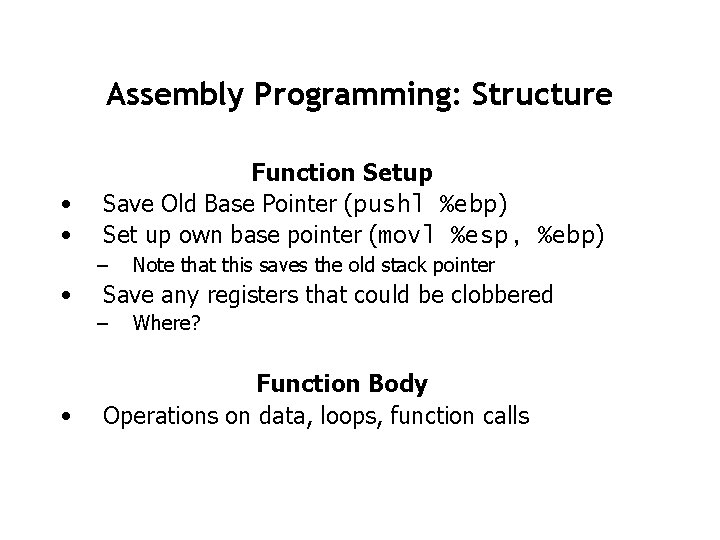
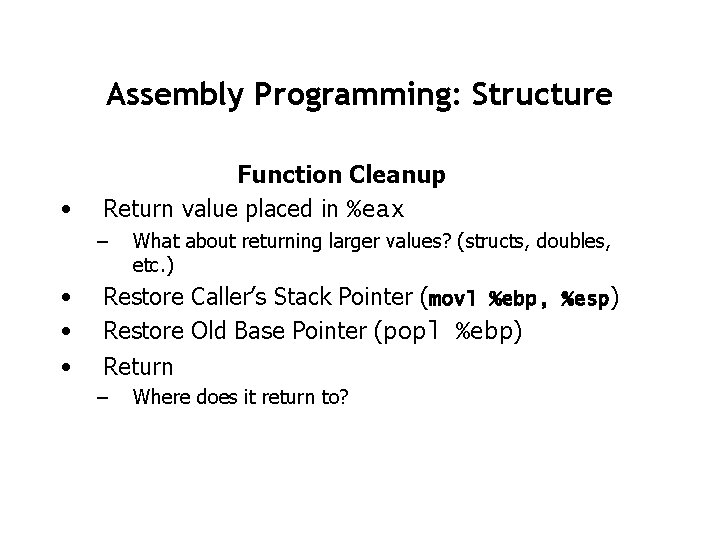
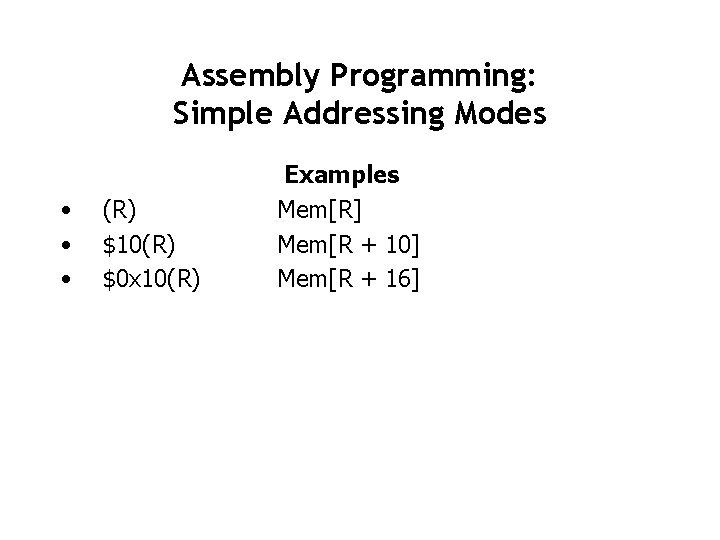
![Assembly Programming: Indexed Addressing Modes Generic Form D(Rb, Ri, S) Mem[Reg[Rb]+S*Reg[Ri]+ D] Examples • Assembly Programming: Indexed Addressing Modes Generic Form D(Rb, Ri, S) Mem[Reg[Rb]+S*Reg[Ri]+ D] Examples •](https://slidetodoc.com/presentation_image_h2/9bfcc4e6fcf3f9549966b46823f0eaba/image-7.jpg)
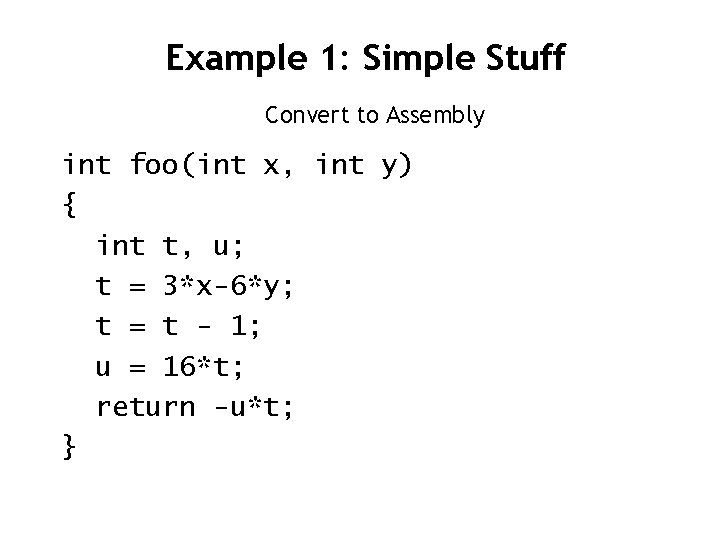
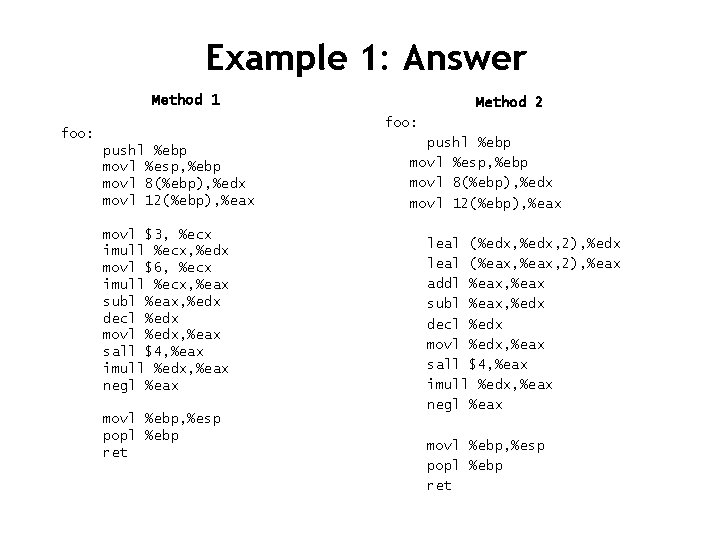
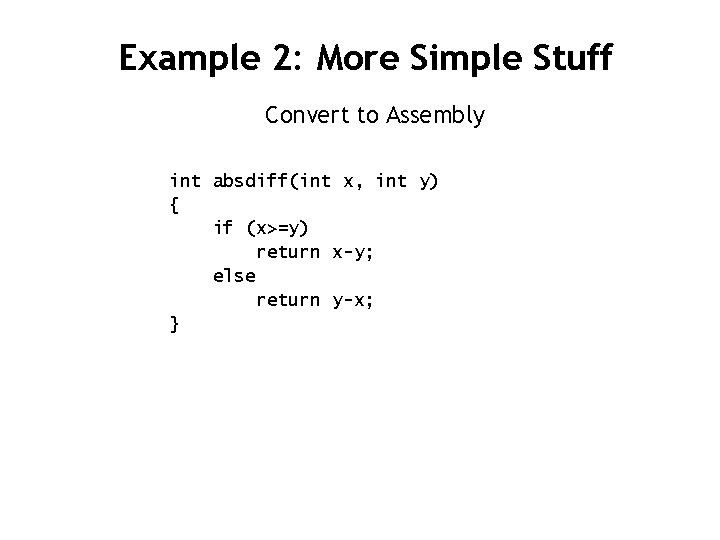
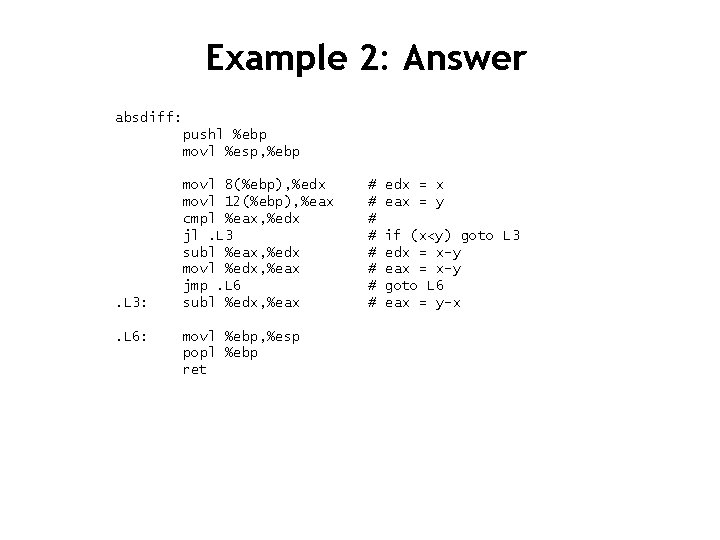
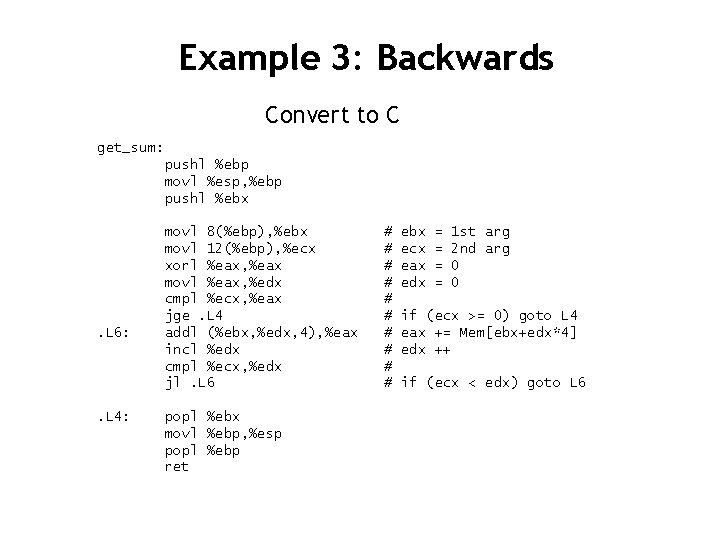
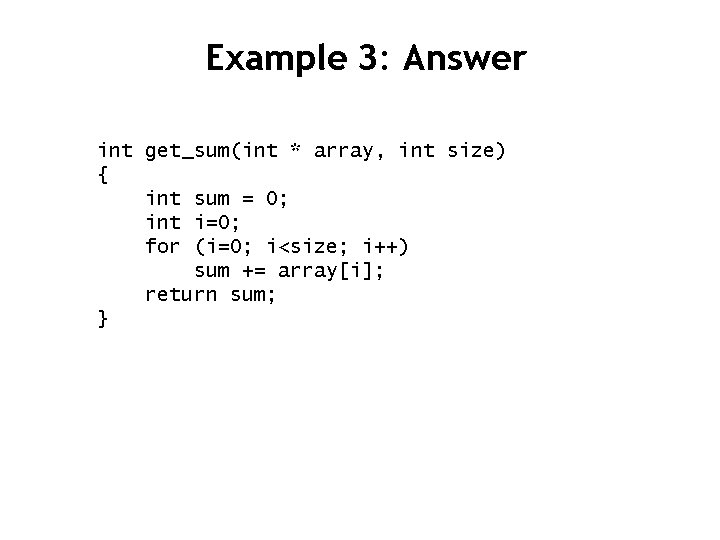
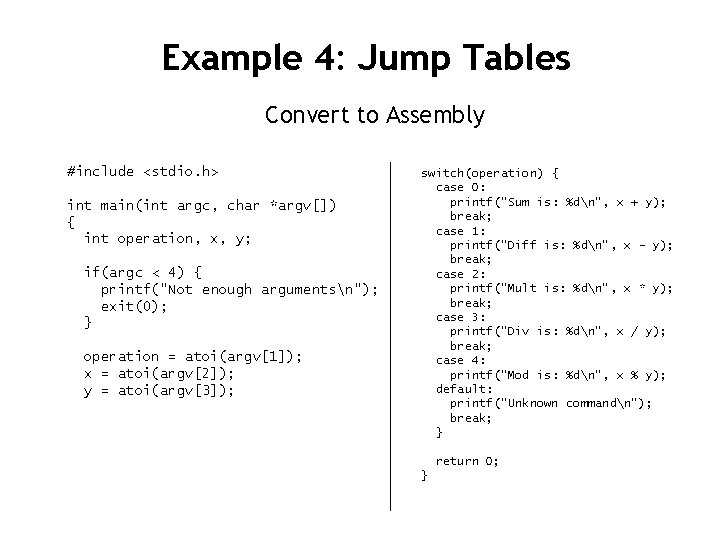
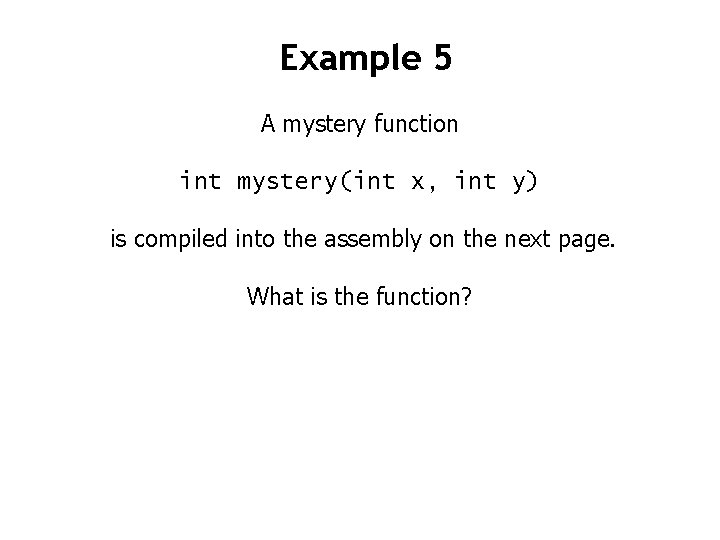
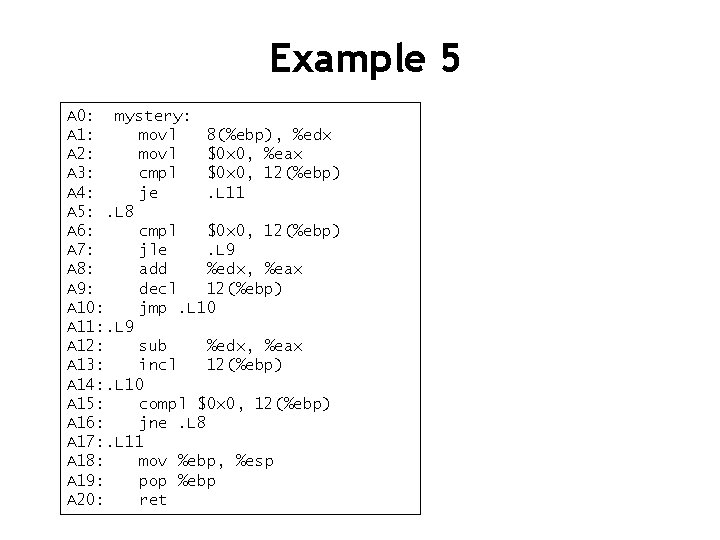
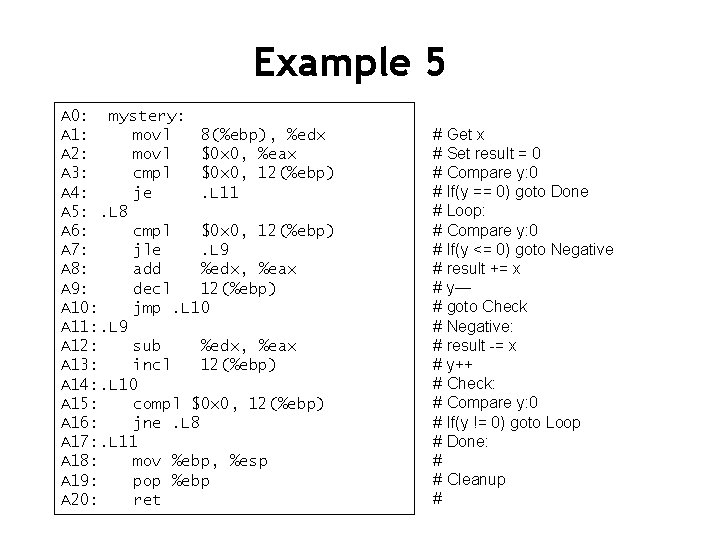
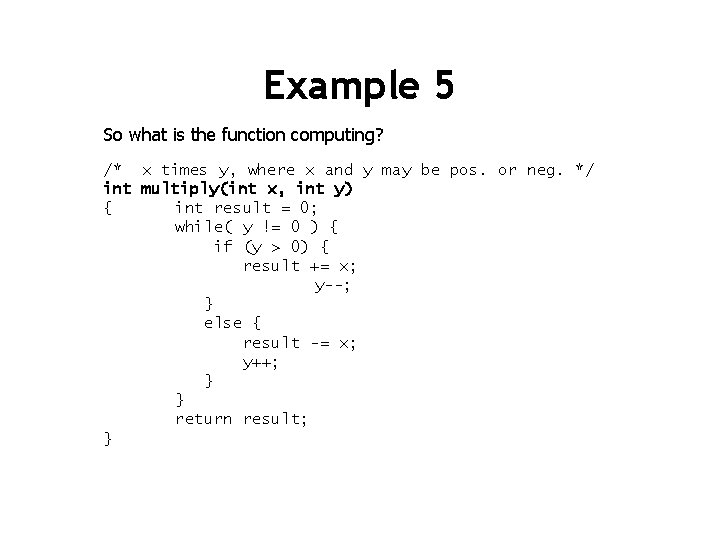
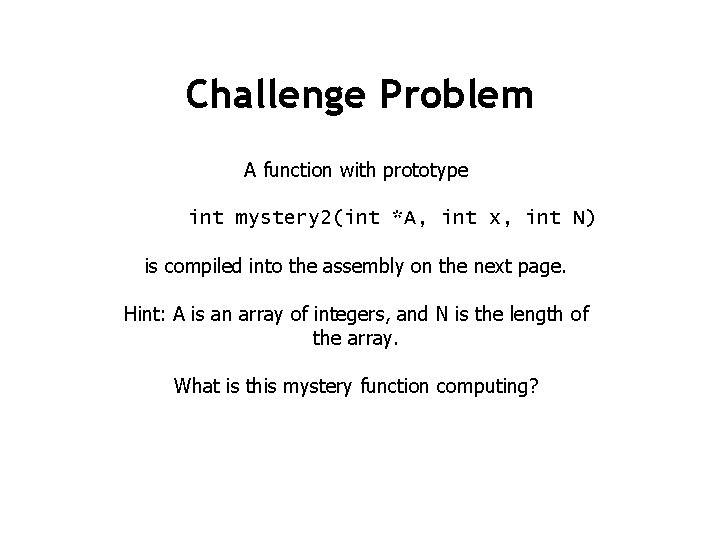
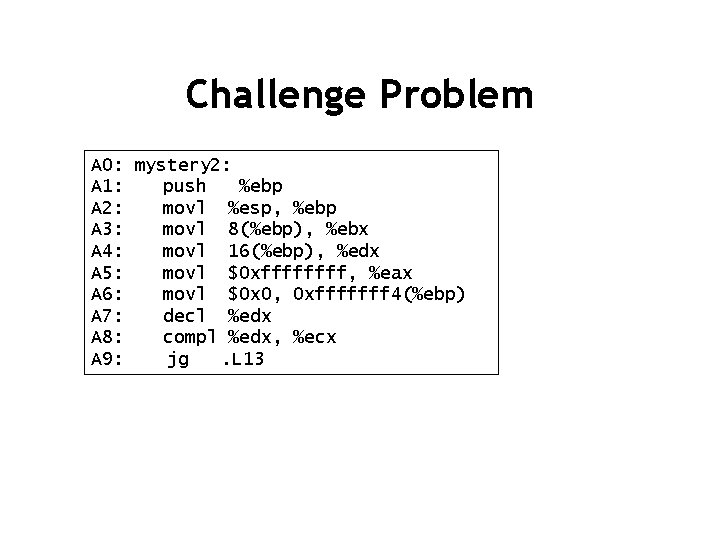
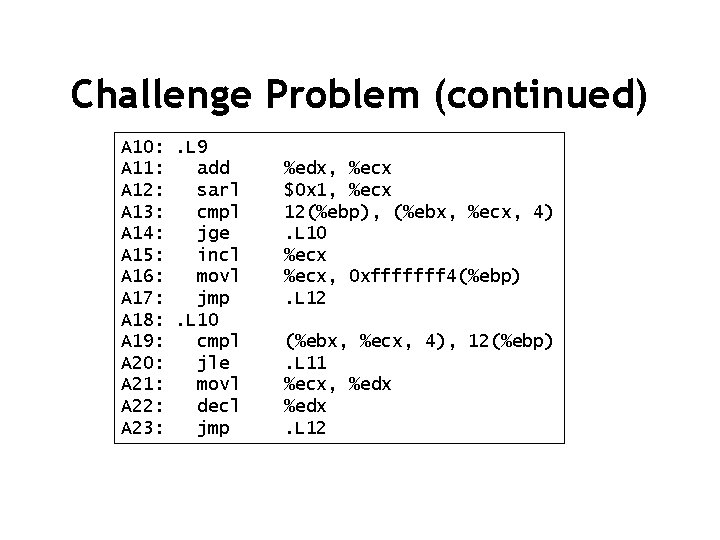
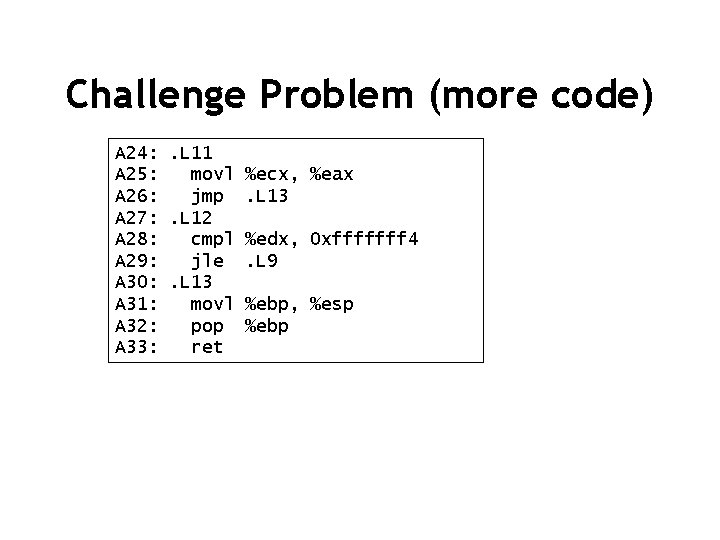
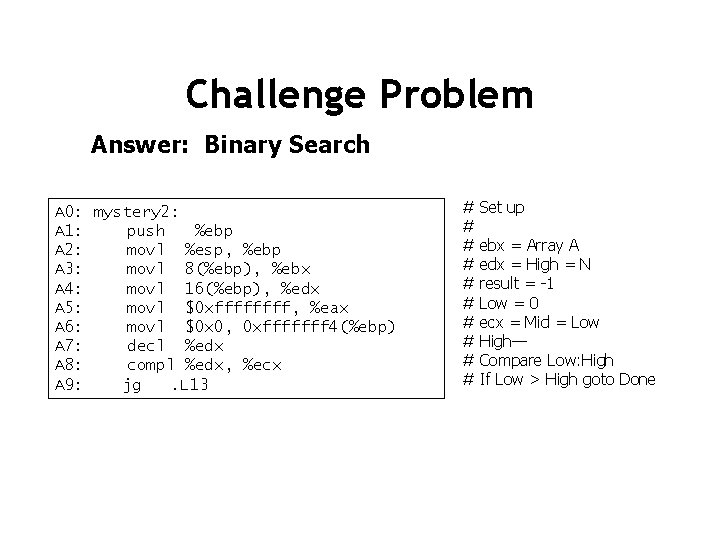
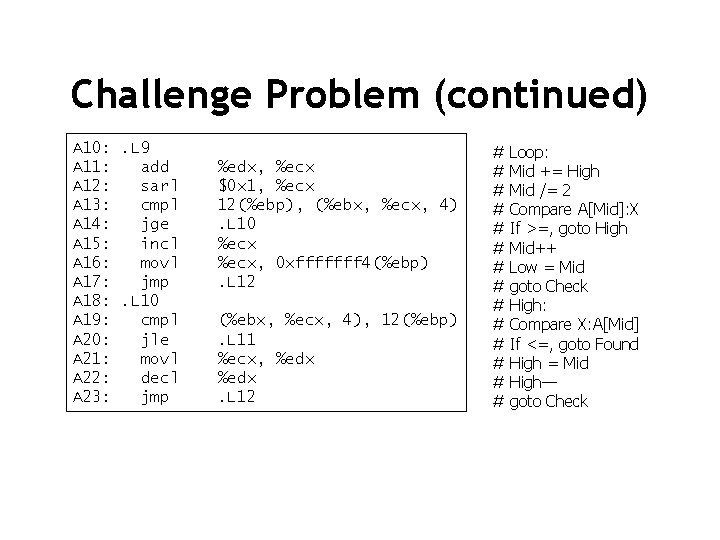
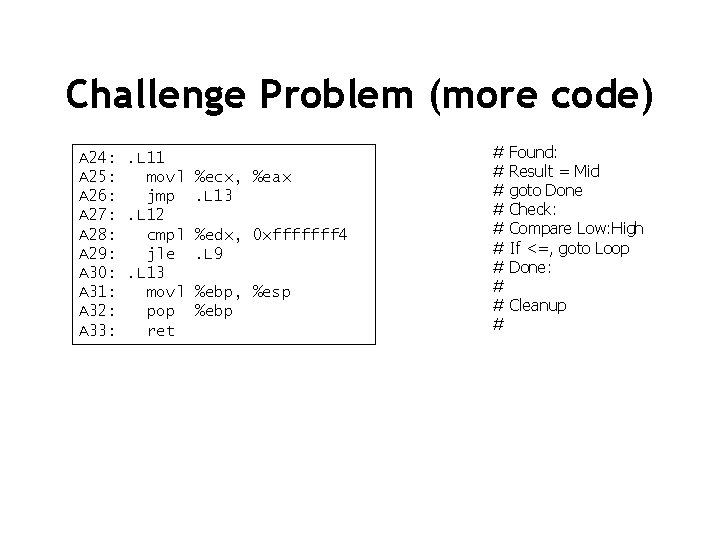
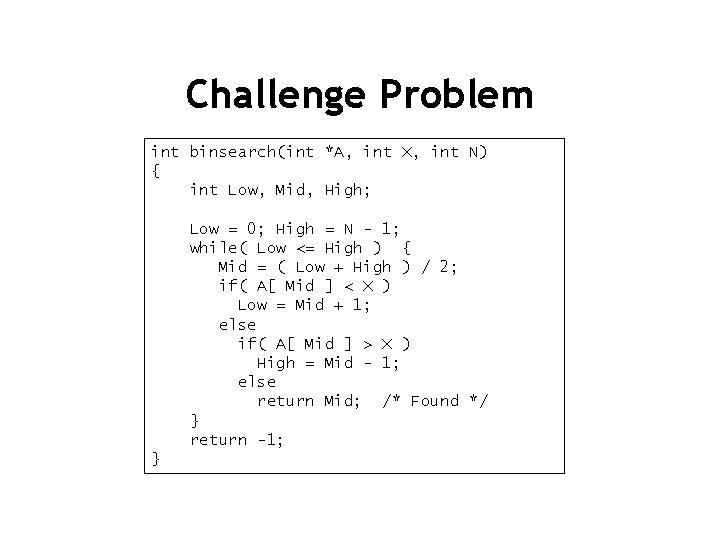
- Slides: 26
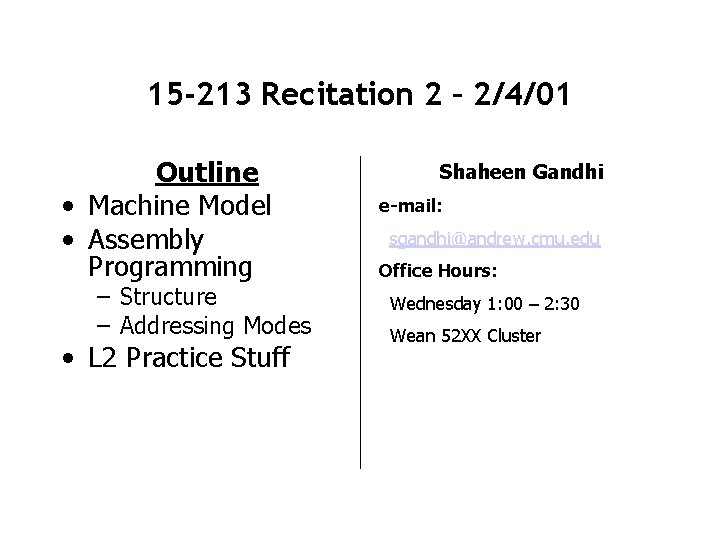
15 -213 Recitation 2 – 2/4/01 Outline • Machine Model • Assembly Programming – Structure – Addressing Modes • L 2 Practice Stuff Shaheen Gandhi e-mail: sgandhi@andrew. cmu. edu Office Hours: Wednesday 1: 00 – 2: 30 Wean 52 XX Cluster
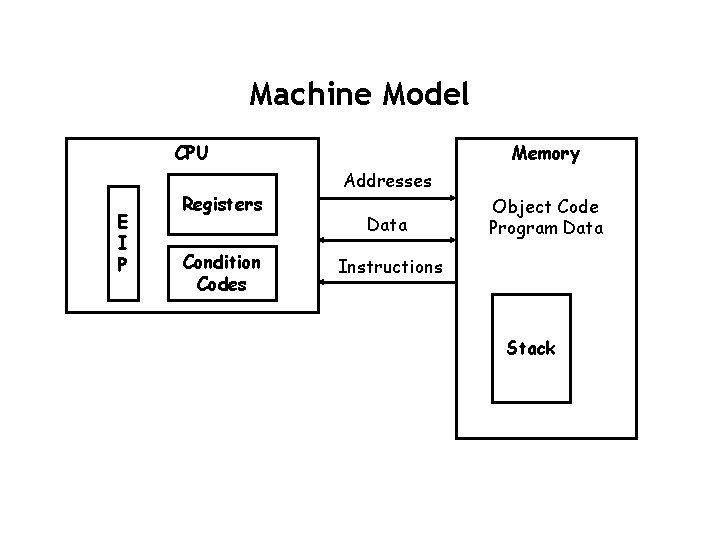
Machine Model CPU E I P Registers Condition Codes Memory Addresses Data Object Code Program Data Instructions Stack
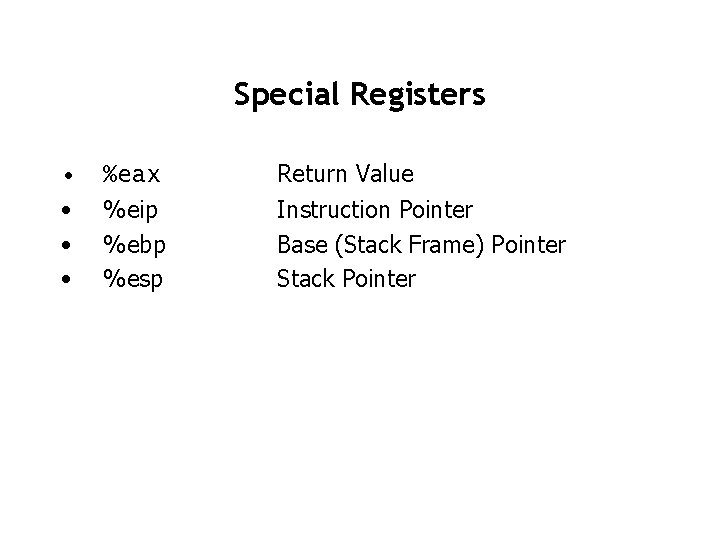
Special Registers • • %eax %eip %ebp %esp Return Value Instruction Pointer Base (Stack Frame) Pointer Stack Pointer
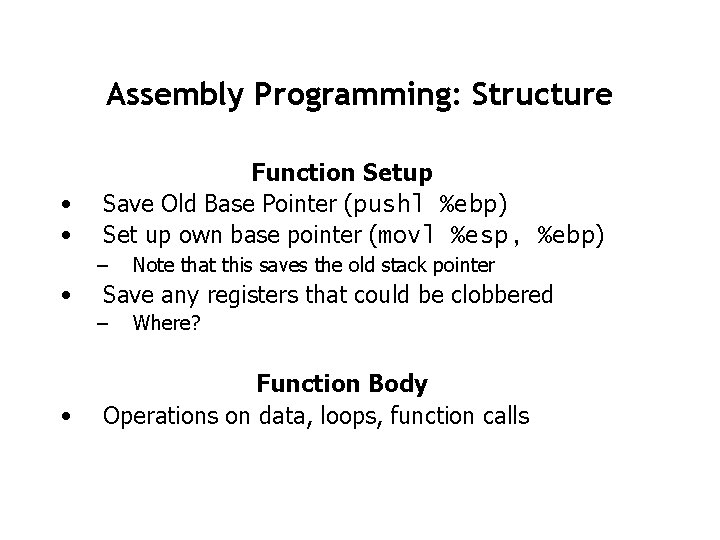
Assembly Programming: Structure • • Function Setup Save Old Base Pointer (pushl %ebp) Set up own base pointer (movl %esp, %ebp) – • Save any registers that could be clobbered – • Note that this saves the old stack pointer Where? Function Body Operations on data, loops, function calls
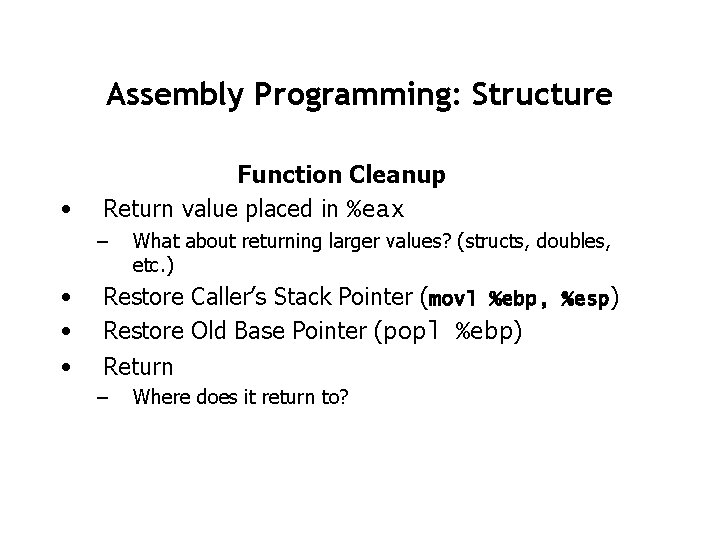
Assembly Programming: Structure • Function Cleanup Return value placed in %eax – • • • What about returning larger values? (structs, doubles, etc. ) Restore Caller’s Stack Pointer (movl %ebp, %esp) Restore Old Base Pointer (popl %ebp) Return – Where does it return to?
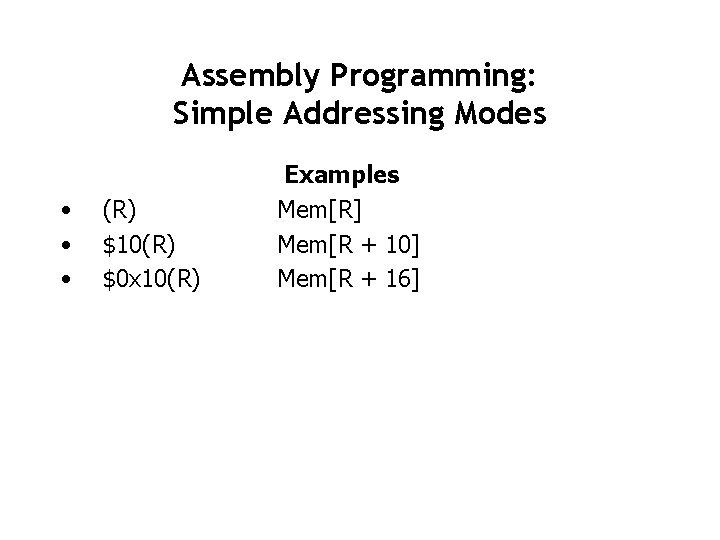
Assembly Programming: Simple Addressing Modes • • • (R) $10(R) $0 x 10(R) Examples Mem[R] Mem[R + 10] Mem[R + 16]
![Assembly Programming Indexed Addressing Modes Generic Form DRb Ri S MemRegRbSRegRi D Examples Assembly Programming: Indexed Addressing Modes Generic Form D(Rb, Ri, S) Mem[Reg[Rb]+S*Reg[Ri]+ D] Examples •](https://slidetodoc.com/presentation_image_h2/9bfcc4e6fcf3f9549966b46823f0eaba/image-7.jpg)
Assembly Programming: Indexed Addressing Modes Generic Form D(Rb, Ri, S) Mem[Reg[Rb]+S*Reg[Ri]+ D] Examples • • • (Rb, Ri) D(Rb, Ri) (Rb, Ri, S) Mem[Reg[Rb]+Reg[Ri]] Mem[Reg[Rb]+Reg[Ri]+D] Mem[Reg[Rb]+S*Reg[Ri]]
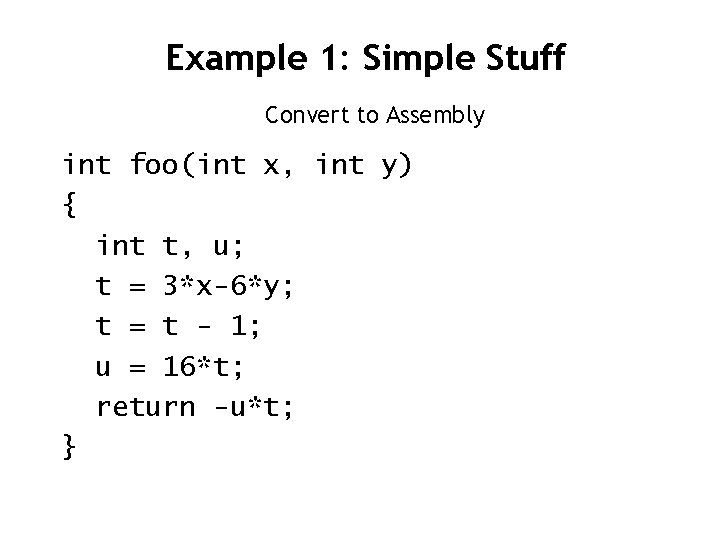
Example 1: Simple Stuff Convert to Assembly int foo(int x, int y) { int t, u; t = 3*x-6*y; t = t - 1; u = 16*t; return -u*t; }
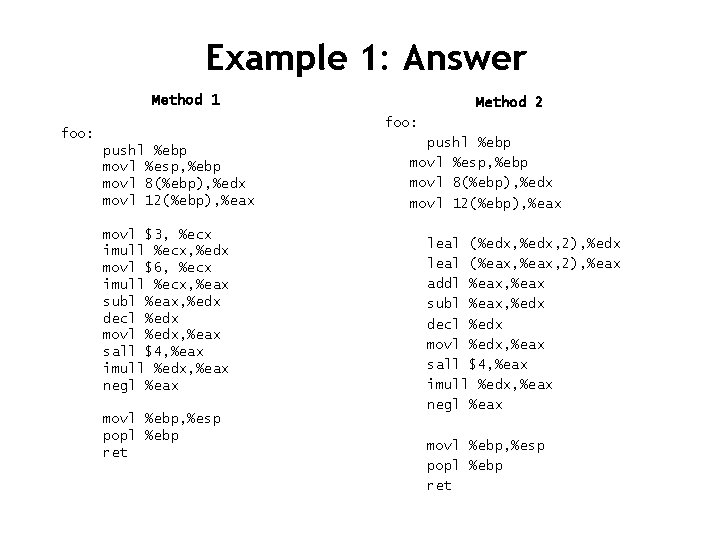
Example 1: Answer Method 1 Method 2 foo: pushl %ebp movl %esp, %ebp movl 8(%ebp), %edx movl 12(%ebp), %eax movl $3, %ecx imull %ecx, %edx movl $6, %ecx imull %ecx, %eax subl %eax, %edx decl %edx movl %edx, %eax sall $4, %eax imull %edx, %eax negl %eax movl %ebp, %esp popl %ebp ret pushl %ebp movl %esp, %ebp movl 8(%ebp), %edx movl 12(%ebp), %eax leal (%edx, 2), %edx leal (%eax, 2), %eax addl %eax, %eax subl %eax, %edx decl %edx movl %edx, %eax sall $4, %eax imull %edx, %eax negl %eax movl %ebp, %esp popl %ebp ret
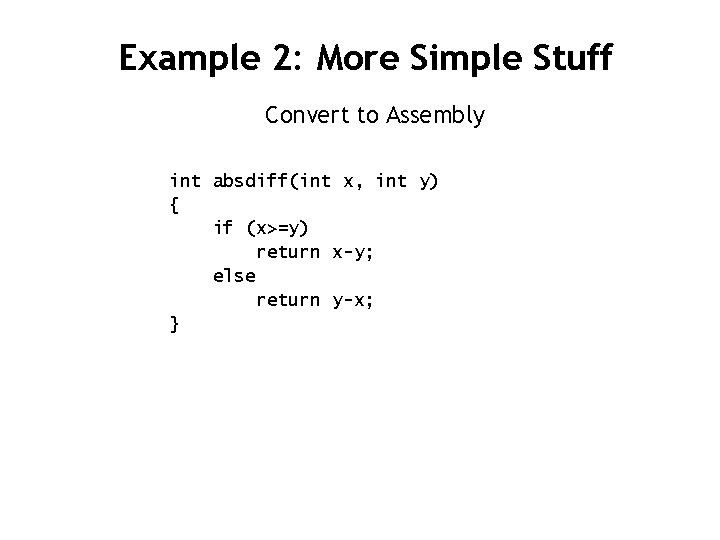
Example 2: More Simple Stuff Convert to Assembly int absdiff(int x, int y) { if (x>=y) return x-y; else return y-x; }
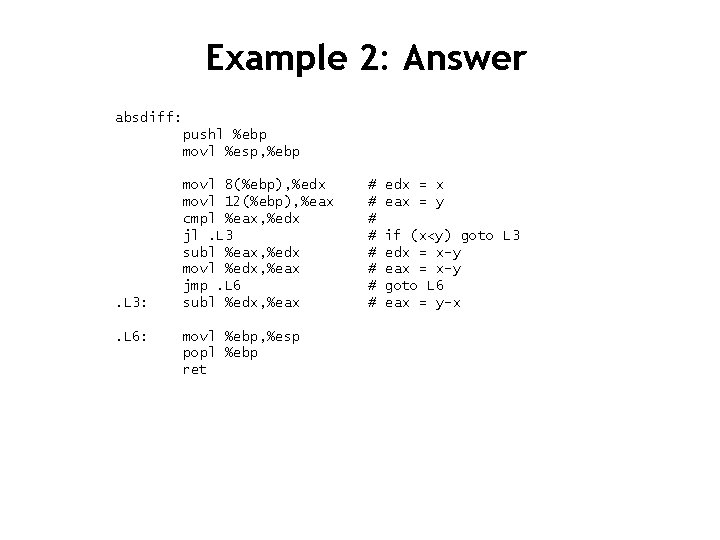
Example 2: Answer absdiff: pushl %ebp movl %esp, %ebp . L 3: . L 6: movl 8(%ebp), %edx movl 12(%ebp), %eax cmpl %eax, %edx jl. L 3 subl %eax, %edx movl %edx, %eax jmp. L 6 subl %edx, %eax movl %ebp, %esp popl %ebp ret # # # # edx = x eax = y if (x<y) goto L 3 edx = x-y eax = x-y goto L 6 eax = y-x
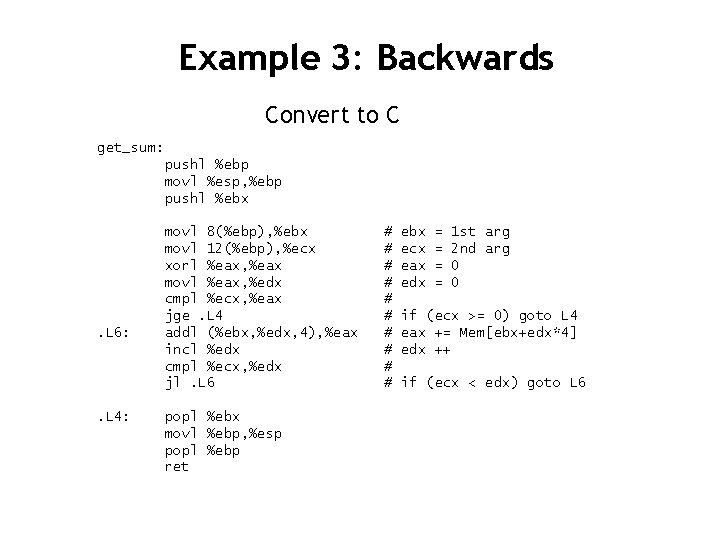
Example 3: Backwards Convert to C get_sum: pushl %ebp movl %esp, %ebp pushl %ebx . L 6: . L 4: movl 8(%ebp), %ebx movl 12(%ebp), %ecx xorl %eax, %eax movl %eax, %edx cmpl %ecx, %eax jge. L 4 addl (%ebx, %edx, 4), %eax incl %edx cmpl %ecx, %edx jl. L 6 popl %ebx movl %ebp, %esp popl %ebp ret # # # # # ebx ecx eax edx = = 1 st arg 2 nd arg 0 0 if (ecx >= 0) goto L 4 eax += Mem[ebx+edx*4] edx ++ if (ecx < edx) goto L 6
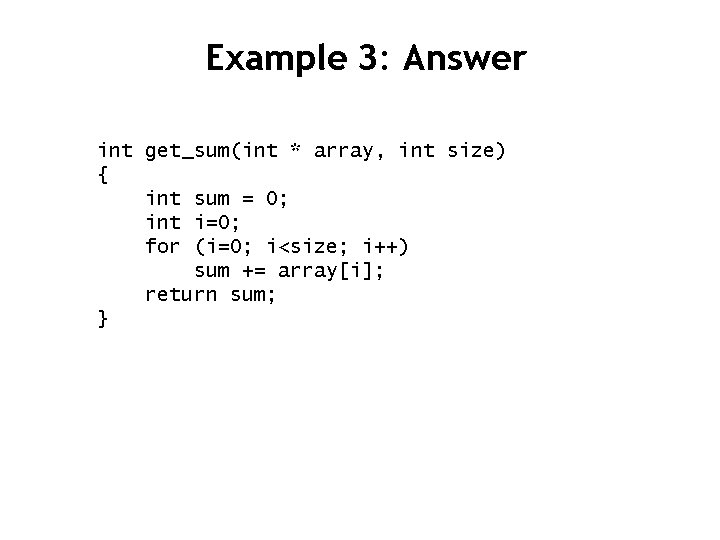
Example 3: Answer int get_sum(int * array, int size) { int sum = 0; int i=0; for (i=0; i<size; i++) sum += array[i]; return sum; }
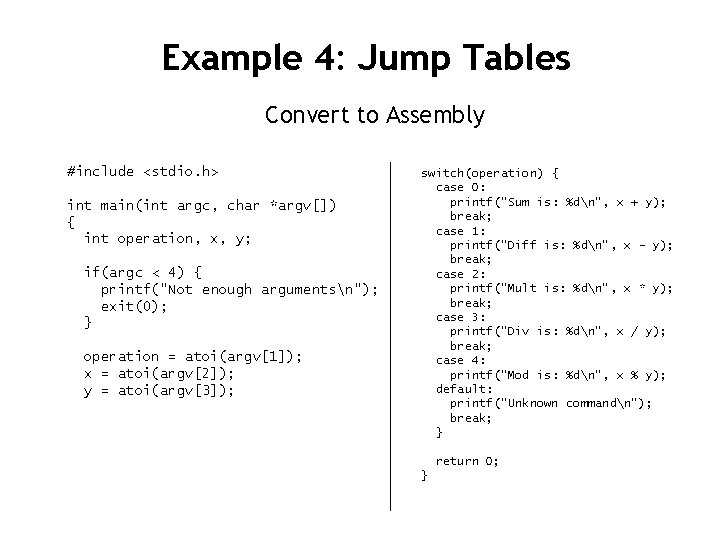
Example 4: Jump Tables Convert to Assembly #include <stdio. h> int main(int argc, char *argv[]) { int operation, x, y; if(argc < 4) { printf("Not enough argumentsn"); exit(0); } operation = atoi(argv[1]); x = atoi(argv[2]); y = atoi(argv[3]); switch(operation) { case 0: printf("Sum is: %dn", x + y); break; case 1: printf("Diff is: %dn", x - y); break; case 2: printf("Mult is: %dn", x * y); break; case 3: printf("Div is: %dn", x / y); break; case 4: printf("Mod is: %dn", x % y); default: printf("Unknown commandn"); break; } return 0; }
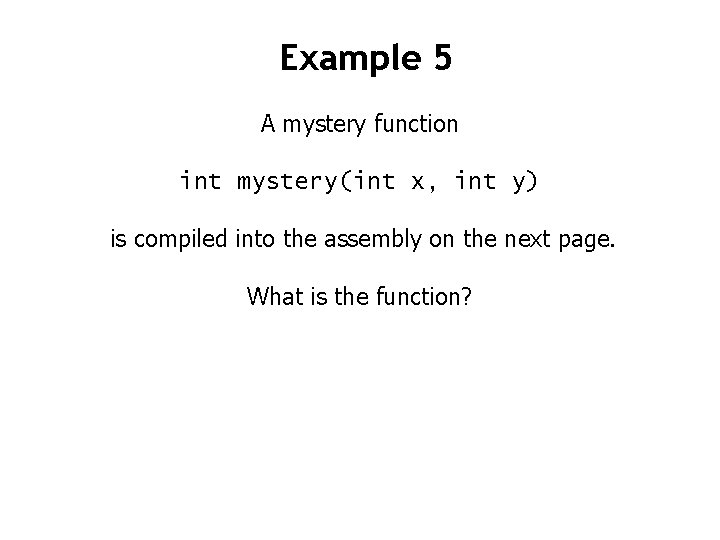
Example 5 A mystery function int mystery(int x, int y) is compiled into the assembly on the next page. What is the function?
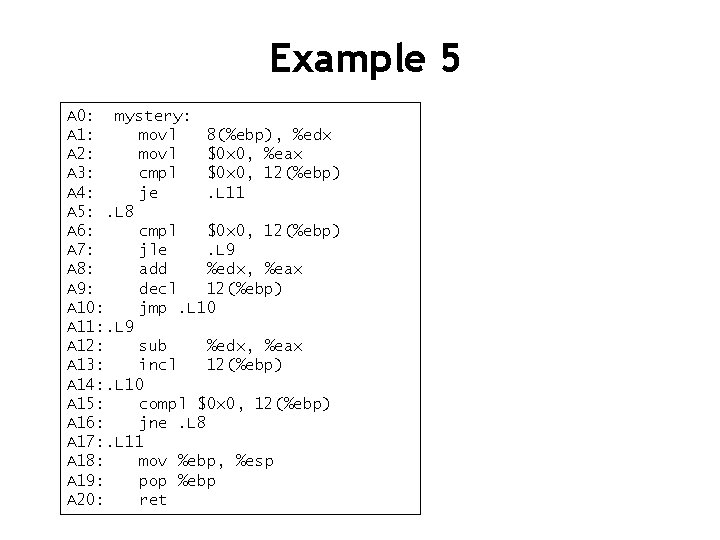
Example 5 A 0: mystery: A 1: movl 8(%ebp), %edx A 2: movl $0 x 0, %eax A 3: cmpl $0 x 0, 12(%ebp) A 4: je. L 11 A 5: . L 8 A 6: cmpl $0 x 0, 12(%ebp) A 7: jle. L 9 A 8: add %edx, %eax A 9: decl 12(%ebp) A 10: jmp. L 10 A 11: . L 9 A 12: sub %edx, %eax A 13: incl 12(%ebp) A 14: . L 10 A 15: compl $0 x 0, 12(%ebp) A 16: jne. L 8 A 17: . L 11 A 18: mov %ebp, %esp A 19: pop %ebp A 20: ret
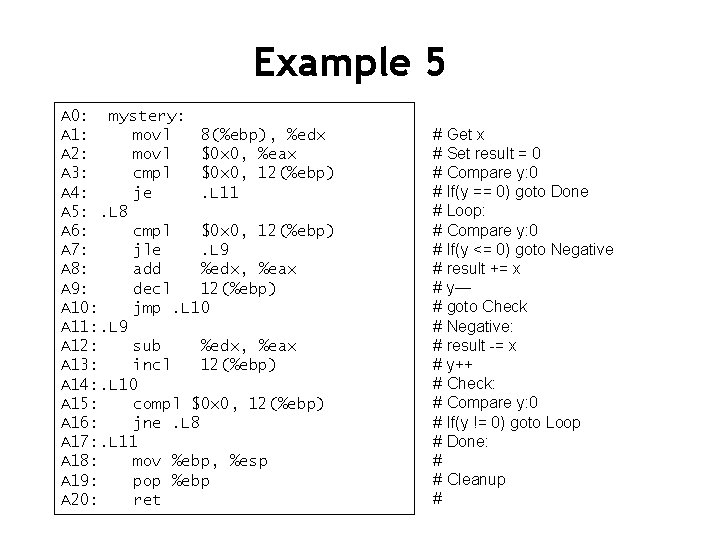
Example 5 A 0: mystery: A 1: movl 8(%ebp), %edx A 2: movl $0 x 0, %eax A 3: cmpl $0 x 0, 12(%ebp) A 4: je. L 11 A 5: . L 8 A 6: cmpl $0 x 0, 12(%ebp) A 7: jle. L 9 A 8: add %edx, %eax A 9: decl 12(%ebp) A 10: jmp. L 10 A 11: . L 9 A 12: sub %edx, %eax A 13: incl 12(%ebp) A 14: . L 10 A 15: compl $0 x 0, 12(%ebp) A 16: jne. L 8 A 17: . L 11 A 18: mov %ebp, %esp A 19: pop %ebp A 20: ret # Get x # Set result = 0 # Compare y: 0 # If(y == 0) goto Done # Loop: # Compare y: 0 # If(y <= 0) goto Negative # result += x # y— # goto Check # Negative: # result -= x # y++ # Check: # Compare y: 0 # If(y != 0) goto Loop # Done: # # Cleanup #
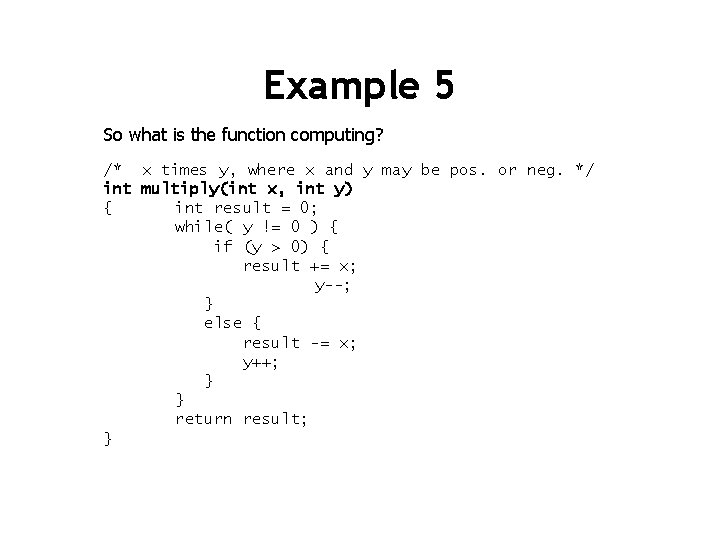
Example 5 So what is the function computing? /* x times y, where x and y may be pos. or neg. */ int multiply(int x, int y) { int result = 0; while( y != 0 ) { if (y > 0) { result += x; y--; } else { result -= x; y++; } } return result; }
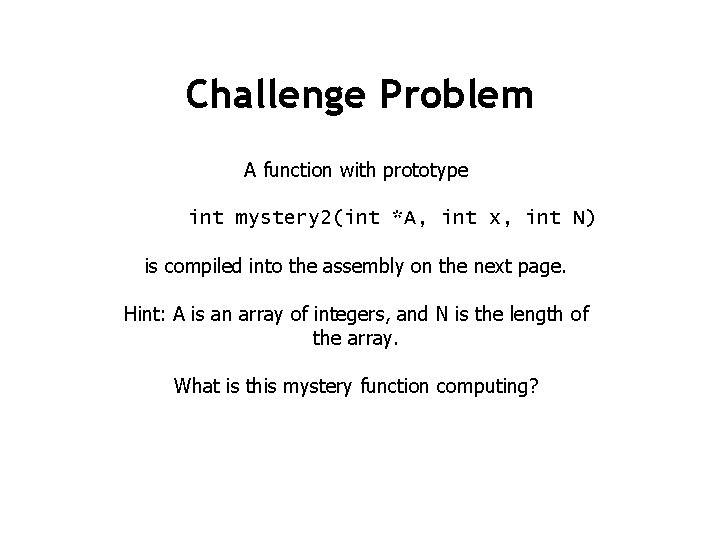
Challenge Problem A function with prototype int mystery 2(int *A, int x, int N) is compiled into the assembly on the next page. Hint: A is an array of integers, and N is the length of the array. What is this mystery function computing?
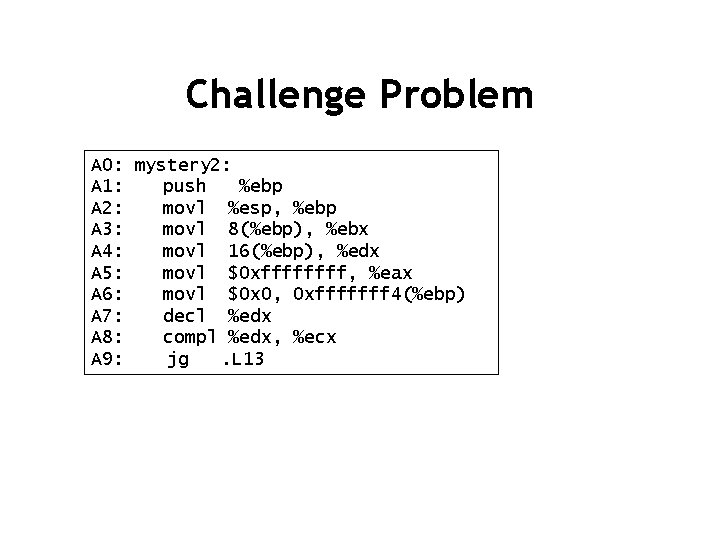
Challenge Problem A 0: mystery 2: A 1: push %ebp A 2: movl %esp, %ebp A 3: movl 8(%ebp), %ebx A 4: movl 16(%ebp), %edx A 5: movl $0 xffff, %eax A 6: movl $0 x 0, 0 xfffffff 4(%ebp) A 7: decl %edx A 8: compl %edx, %ecx A 9: jg. L 13
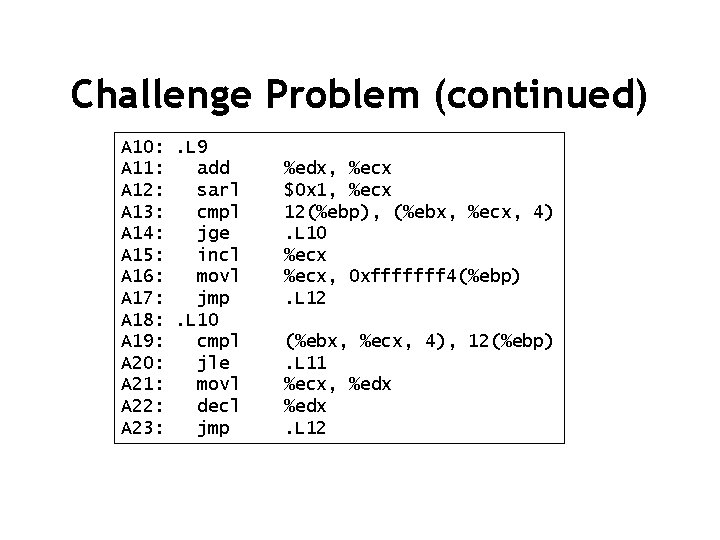
Challenge Problem (continued) A 10: . L 9 A 11: add A 12: sarl A 13: cmpl A 14: jge A 15: incl A 16: movl A 17: jmp A 18: . L 10 A 19: cmpl A 20: jle A 21: movl A 22: decl A 23: jmp %edx, %ecx $0 x 1, %ecx 12(%ebp), (%ebx, %ecx, 4). L 10 %ecx, 0 xfffffff 4(%ebp). L 12 (%ebx, %ecx, 4), 12(%ebp). L 11 %ecx, %edx. L 12
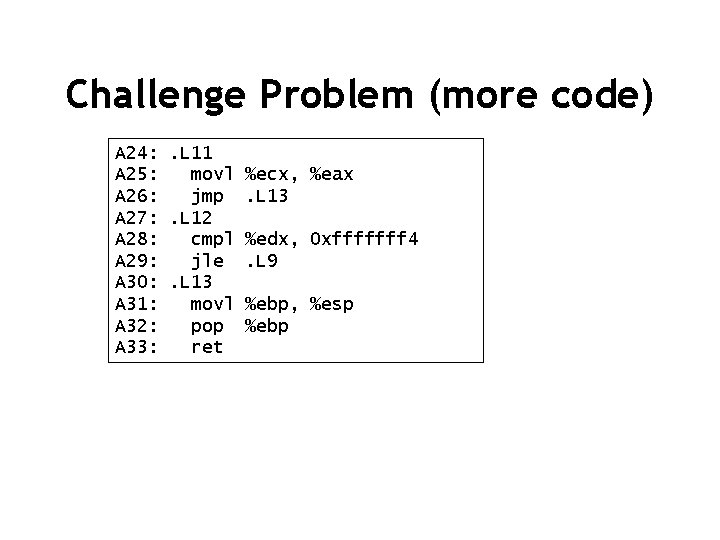
Challenge Problem (more code) A 24: . L 11 A 25: movl A 26: jmp A 27: . L 12 A 28: cmpl A 29: jle A 30: . L 13 A 31: movl A 32: pop A 33: ret %ecx, %eax. L 13 %edx, 0 xfffffff 4. L 9 %ebp, %esp %ebp
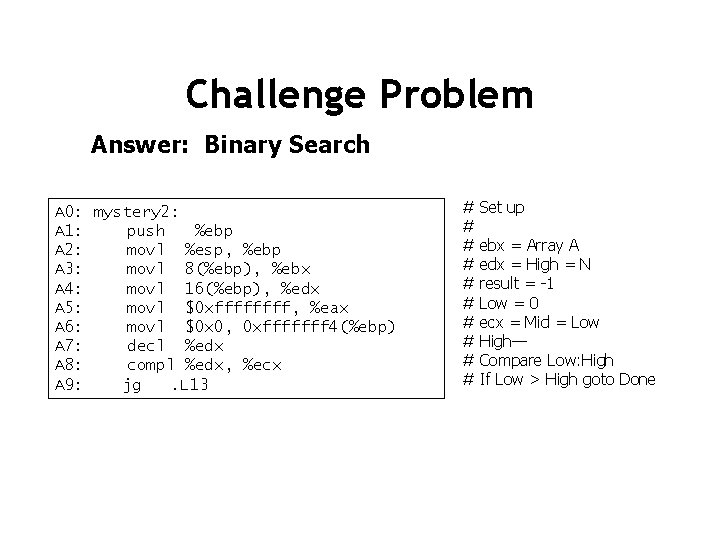
Challenge Problem Answer: Binary Search A 0: mystery 2: A 1: push %ebp A 2: movl %esp, %ebp A 3: movl 8(%ebp), %ebx A 4: movl 16(%ebp), %edx A 5: movl $0 xffff, %eax A 6: movl $0 x 0, 0 xfffffff 4(%ebp) A 7: decl %edx A 8: compl %edx, %ecx A 9: jg. L 13 # # # # # Set up ebx = Array A edx = High = N result = -1 Low = 0 ecx = Mid = Low High— Compare Low: High If Low > High goto Done
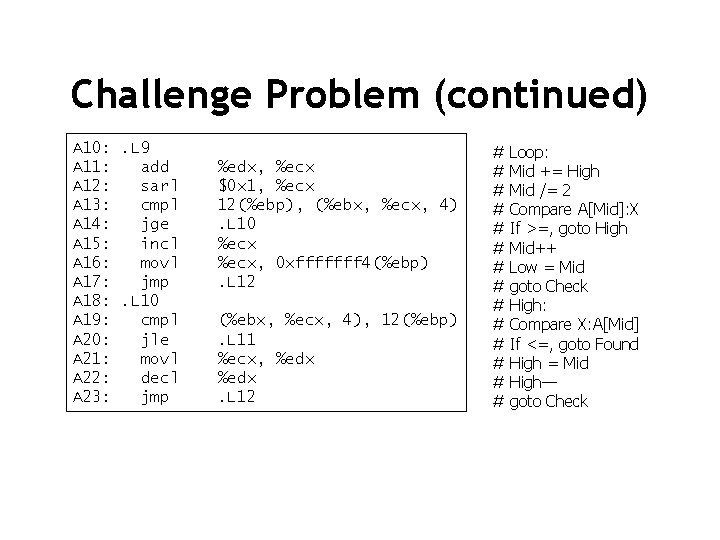
Challenge Problem (continued) A 10: . L 9 A 11: add A 12: sarl A 13: cmpl A 14: jge A 15: incl A 16: movl A 17: jmp A 18: . L 10 A 19: cmpl A 20: jle A 21: movl A 22: decl A 23: jmp %edx, %ecx $0 x 1, %ecx 12(%ebp), (%ebx, %ecx, 4). L 10 %ecx, 0 xfffffff 4(%ebp). L 12 (%ebx, %ecx, 4), 12(%ebp). L 11 %ecx, %edx. L 12 # # # # Loop: Mid += High Mid /= 2 Compare A[Mid]: X If >=, goto High Mid++ Low = Mid goto Check High: Compare X: A[Mid] If <=, goto Found High = Mid High— goto Check
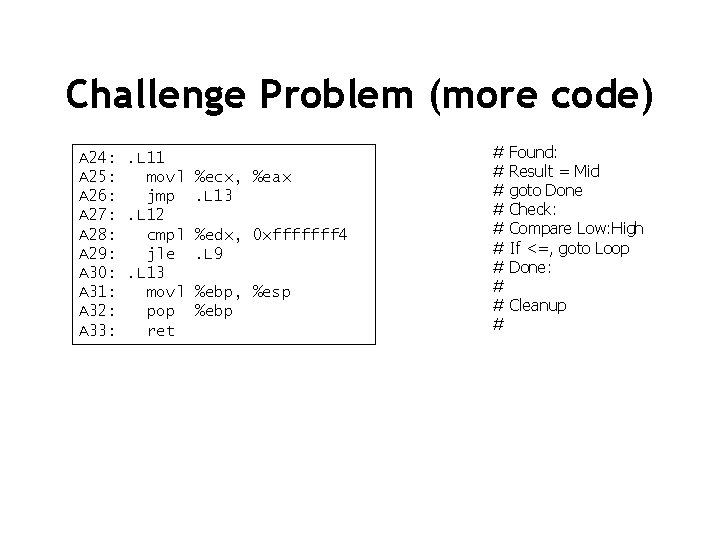
Challenge Problem (more code) A 24: . L 11 A 25: movl A 26: jmp A 27: . L 12 A 28: cmpl A 29: jle A 30: . L 13 A 31: movl A 32: pop A 33: ret %ecx, %eax. L 13 %edx, 0 xfffffff 4. L 9 %ebp, %esp %ebp # # # # # Found: Result = Mid goto Done Check: Compare Low: High If <=, goto Loop Done: Cleanup
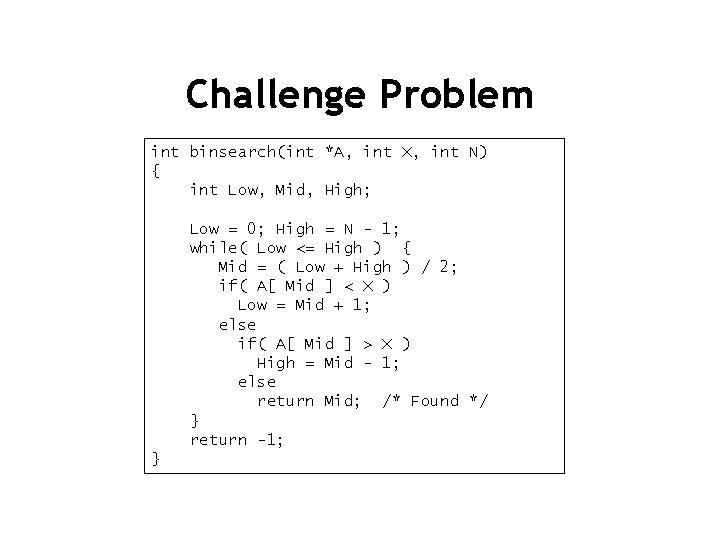
Challenge Problem int binsearch(int *A, int X, int N) { int Low, Mid, High; Low = 0; High = N - 1; while( Low <= High ) { Mid = ( Low + High ) / 2; if( A[ Mid ] < X ) Low = Mid + 1; else if( A[ Mid ] > X ) High = Mid - 1; else return Mid; /* Found */ } return -1; }
Outline 213
Outline 213
15 213
Rio_readlineb
Hft 2401 exam 1
Cits2401
Dd form 2406
Biol 2401
Cs 2401
Math 2401
Math 2401
Cs 2401
Passive recitation
Namaz posture 1
Is the rote recitation of a memorized written message
Poem recitation objectives
Tips for reciting poetry
What is meant by etiquette of recitation of the holy quran
Recitation les machines
Criteria for judging performance
Récitation les hiboux
What is sentence outline
123+132+321+312
Sbi 213
Northwestern cs 213
Cow ceng
Cmu 213