1 Lecture 5 Pointer Outline Chapter 5 Pointer
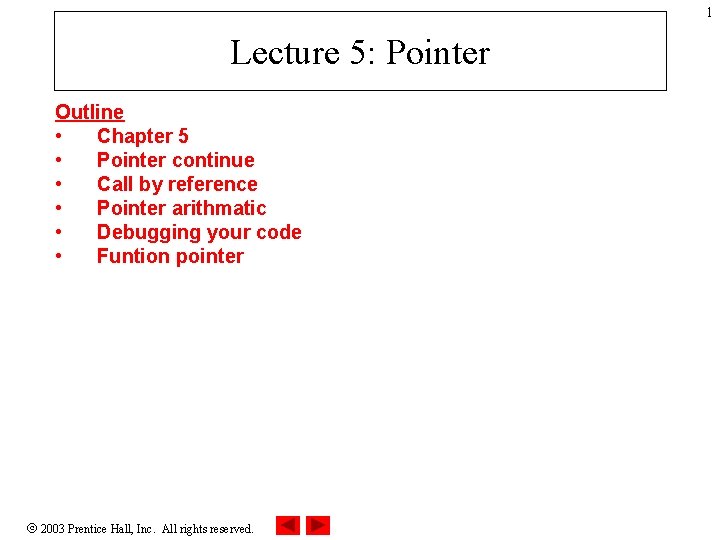
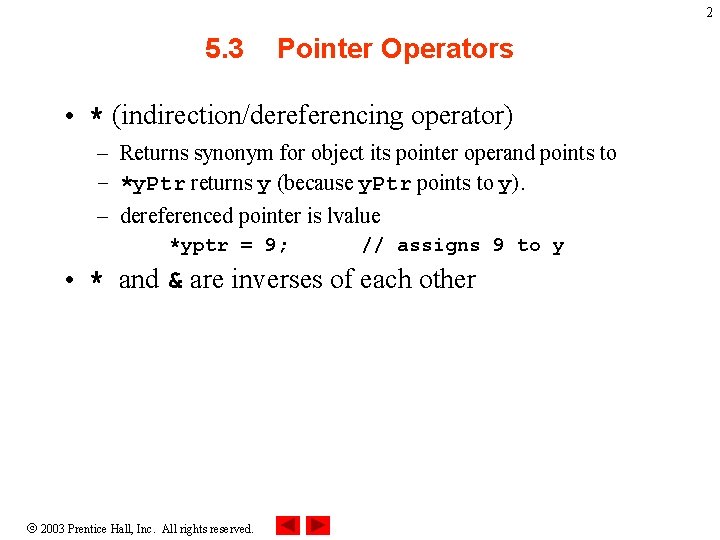
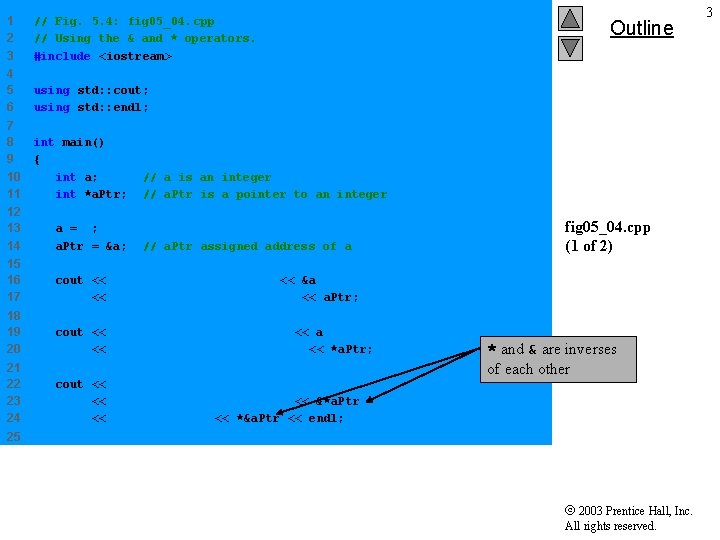
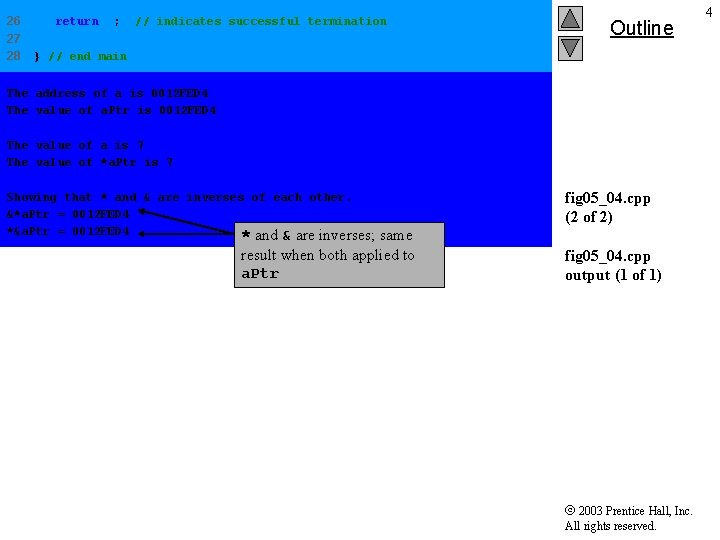
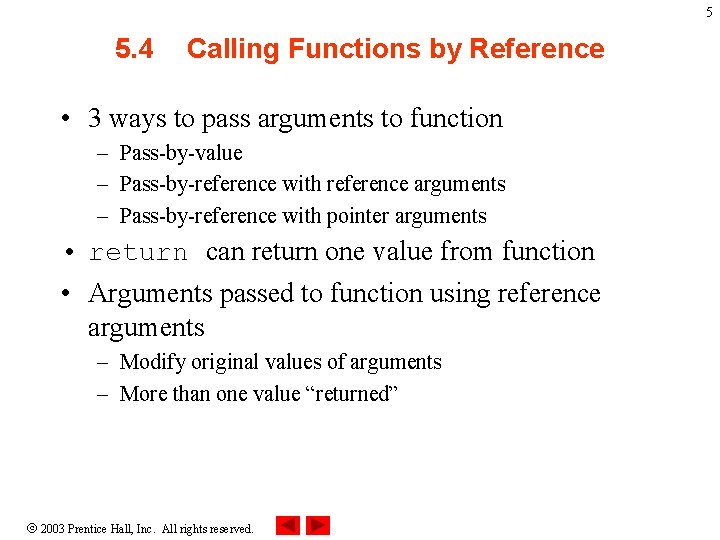
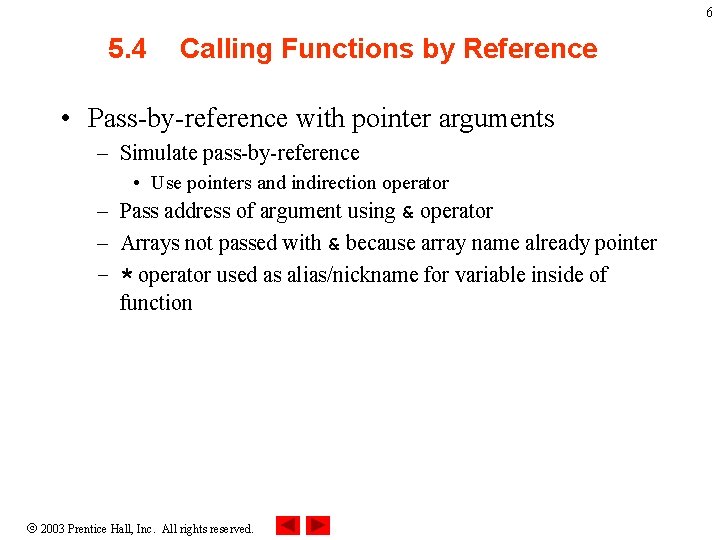
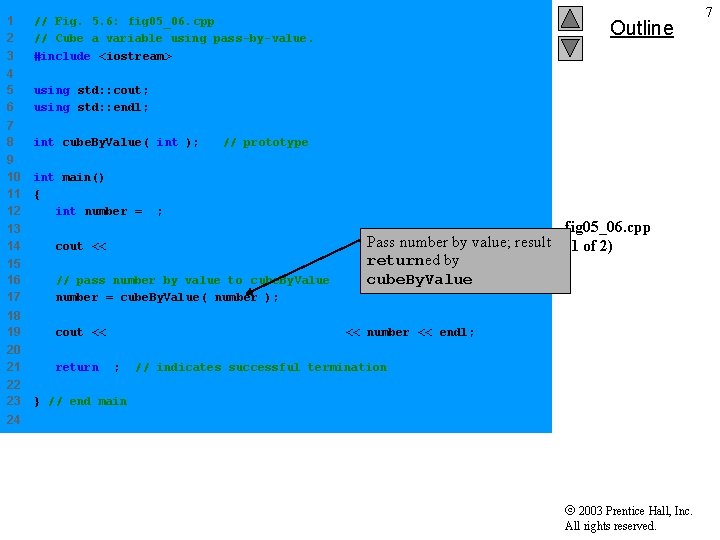
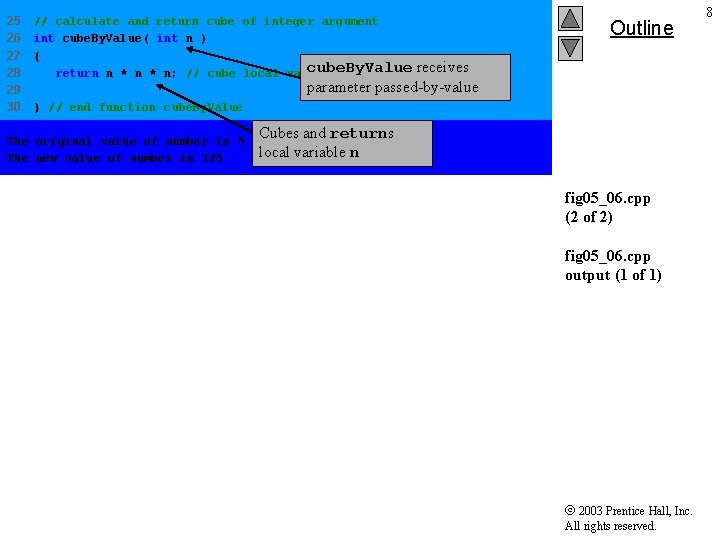
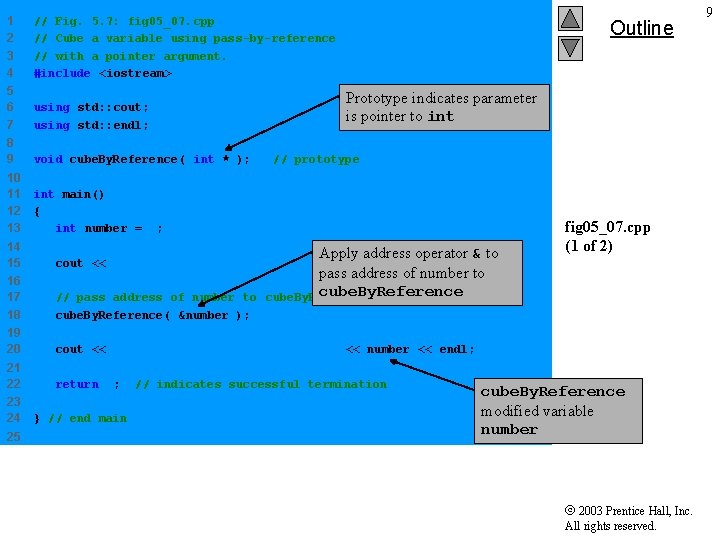
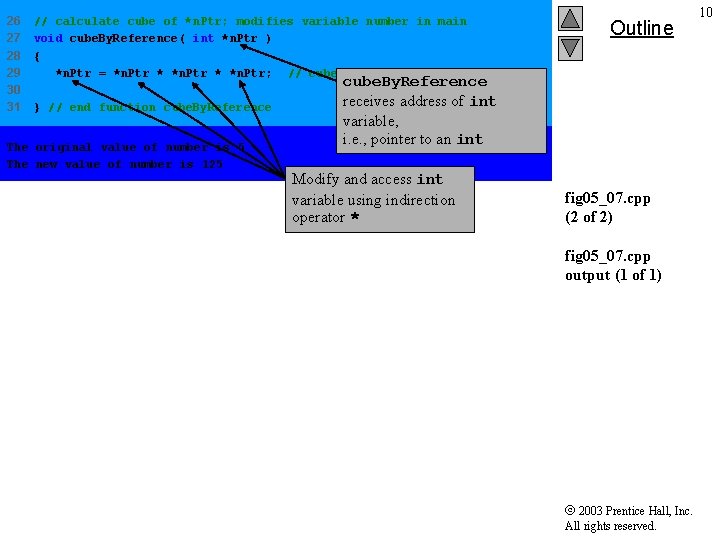
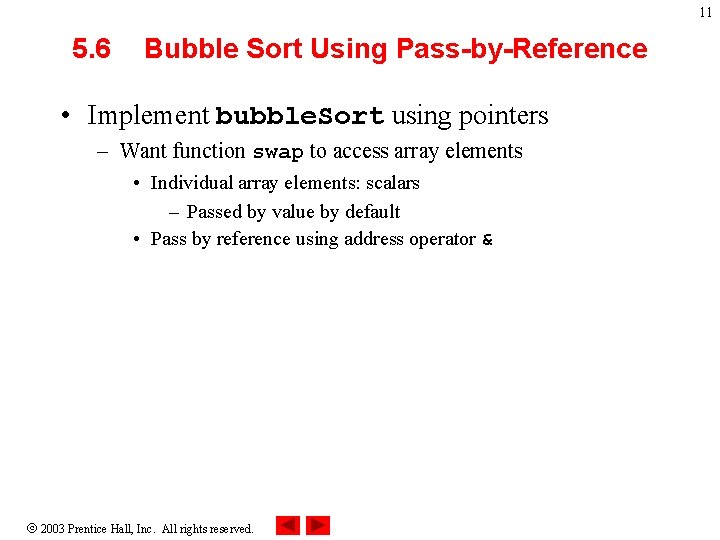
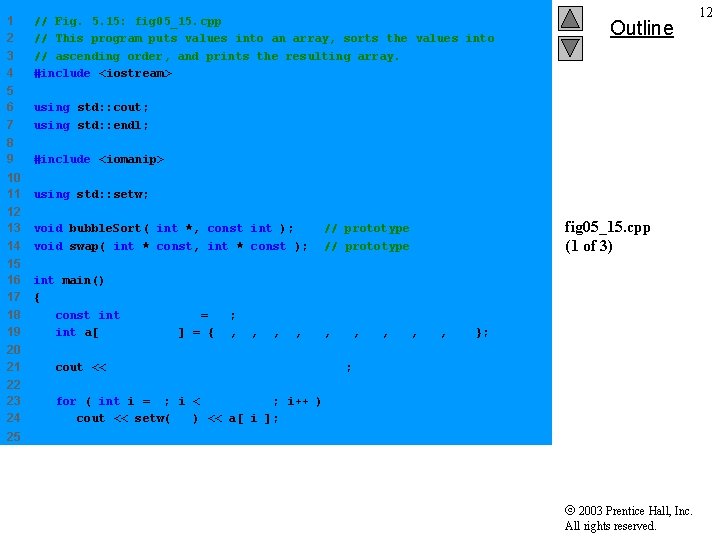
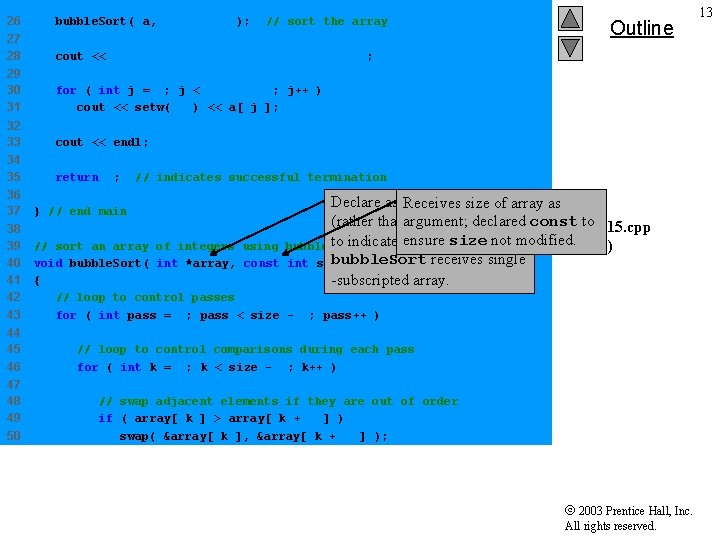
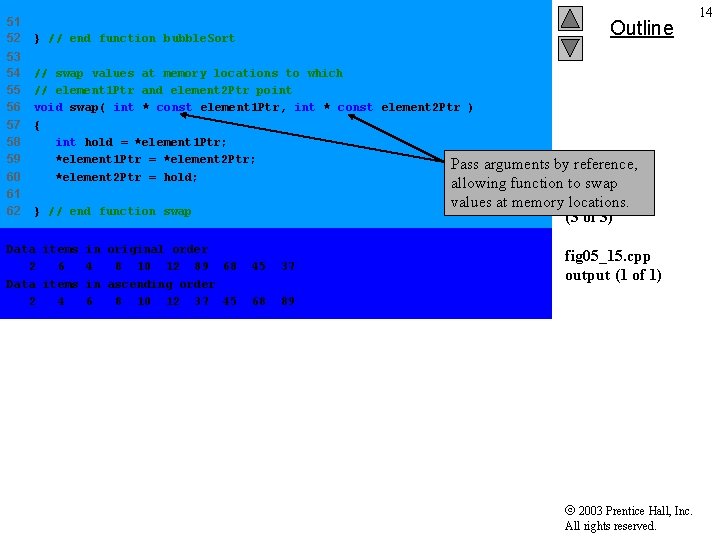
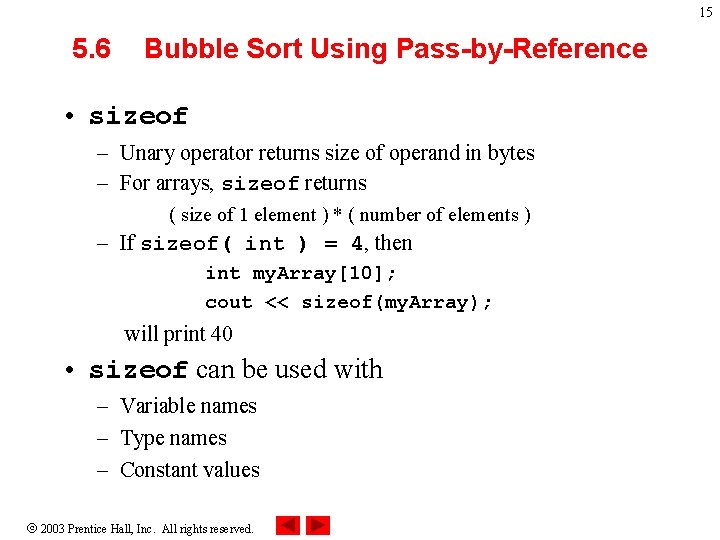
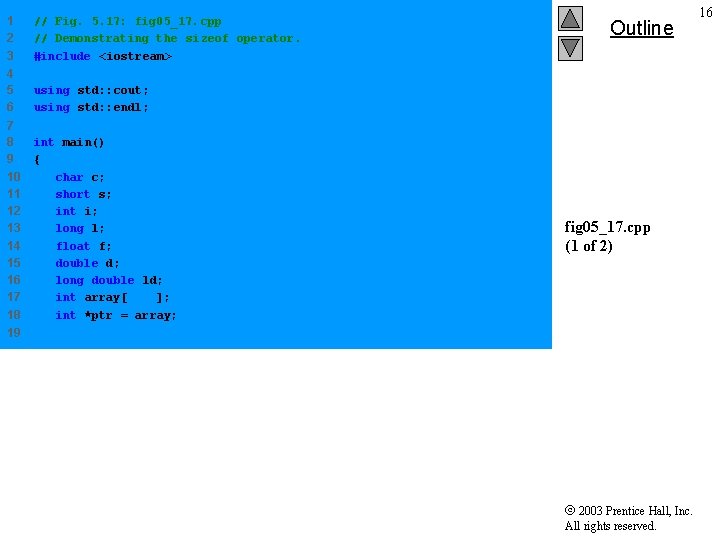
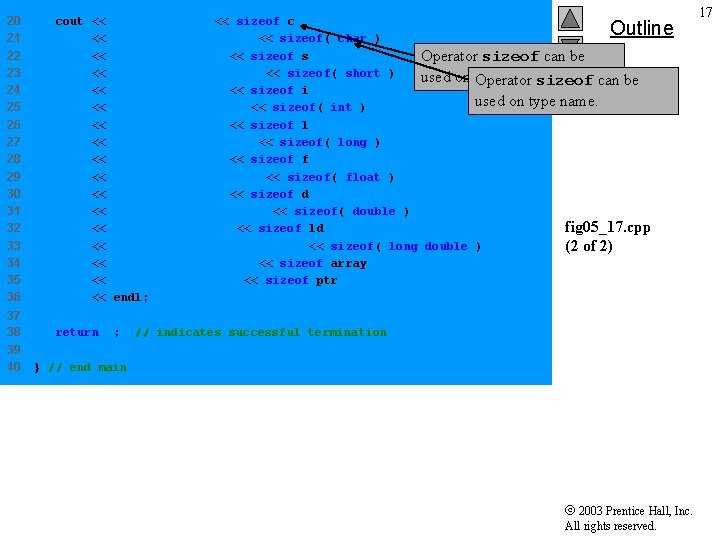
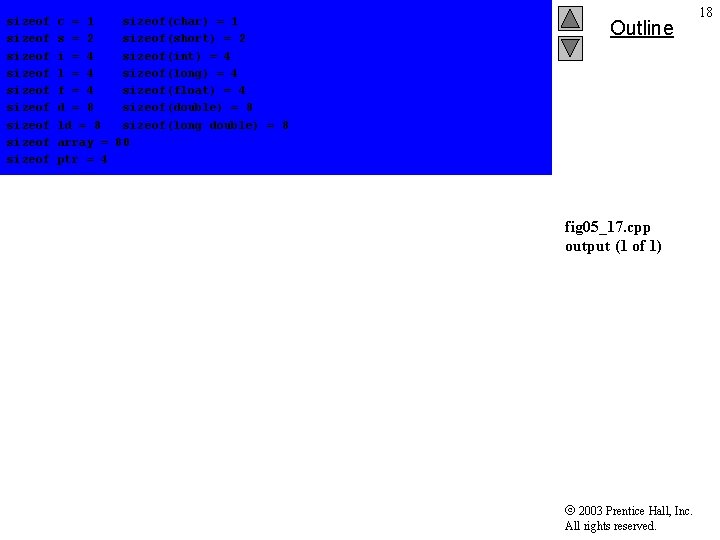
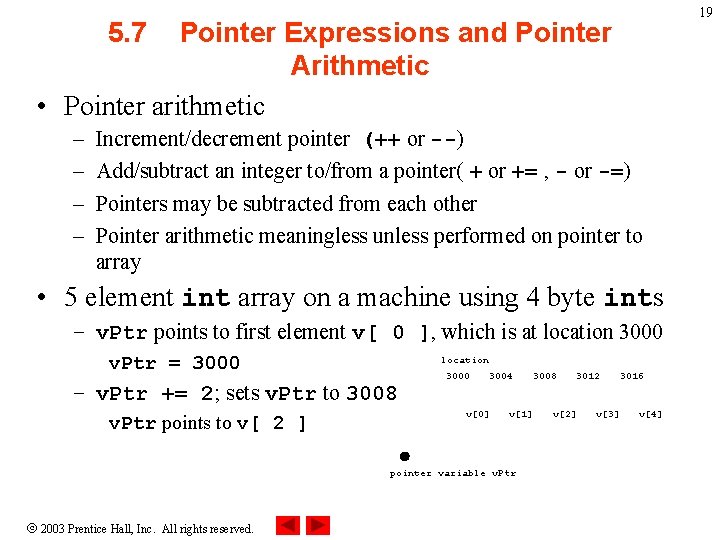
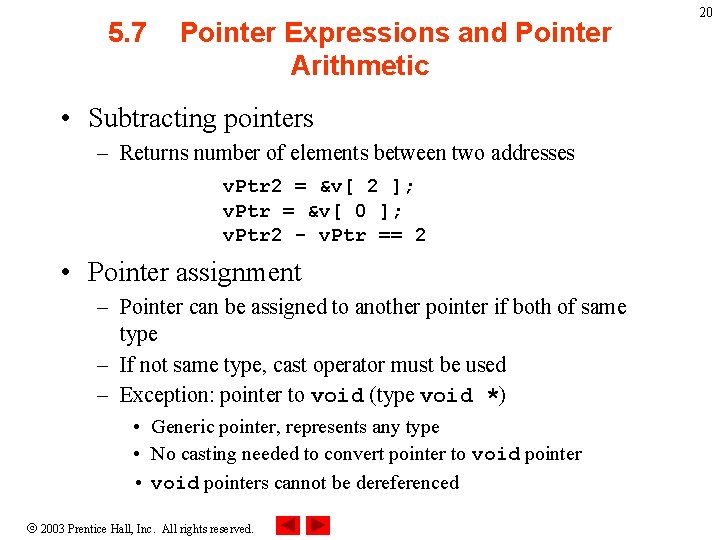
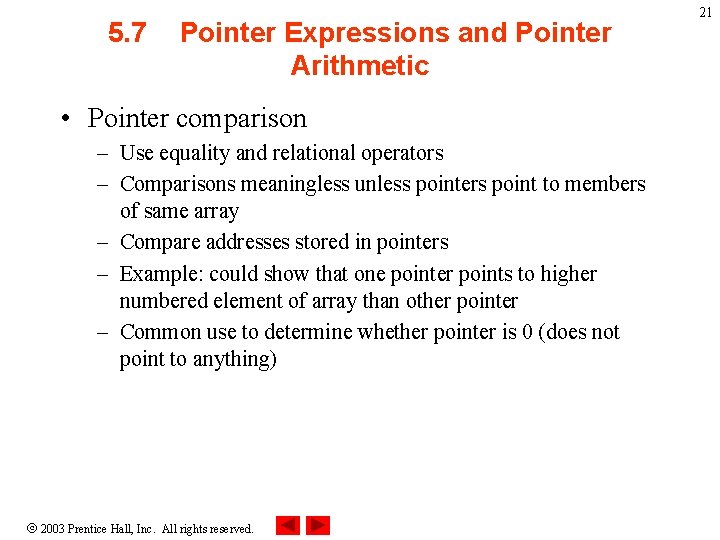
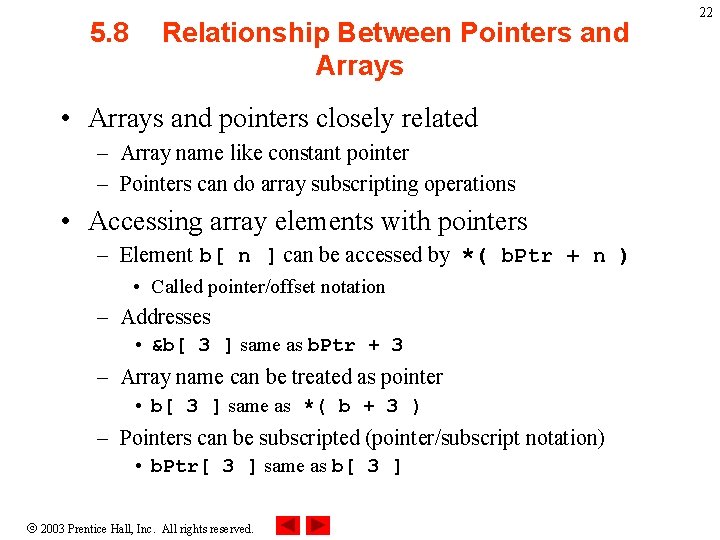
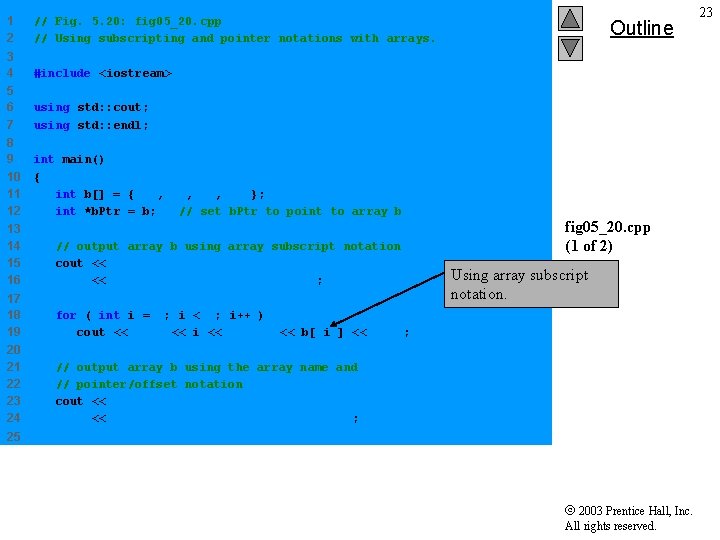
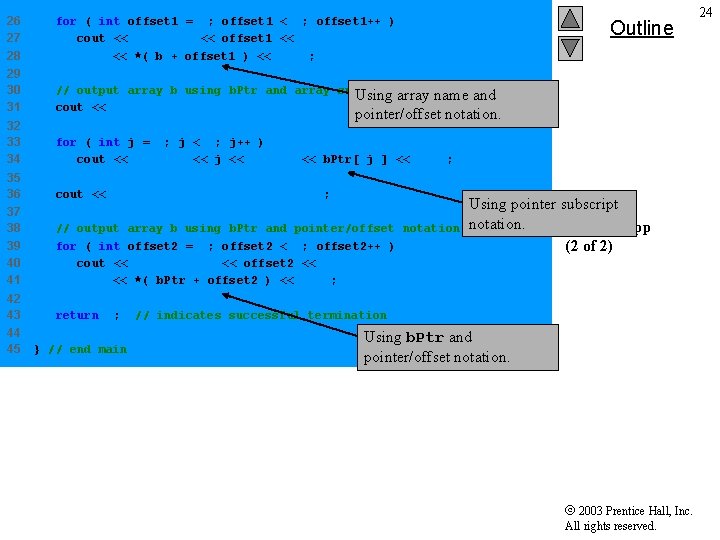
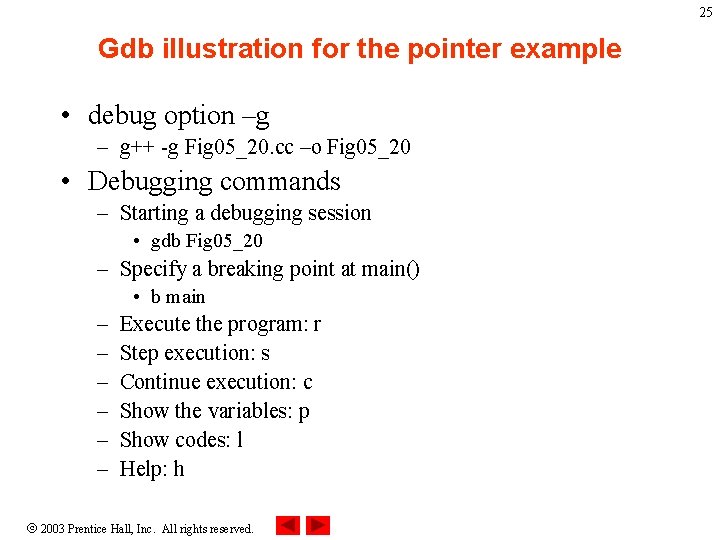
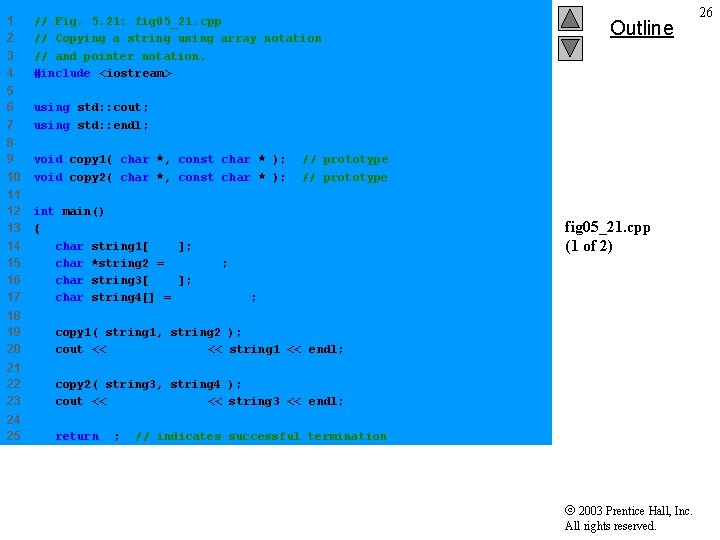
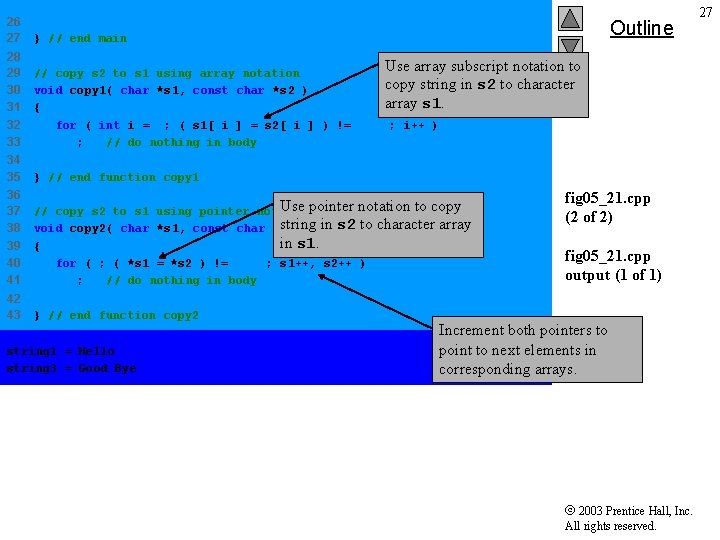
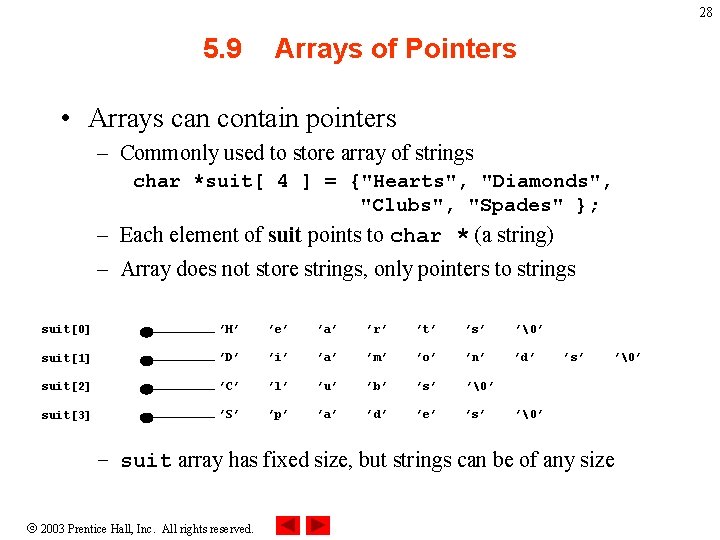
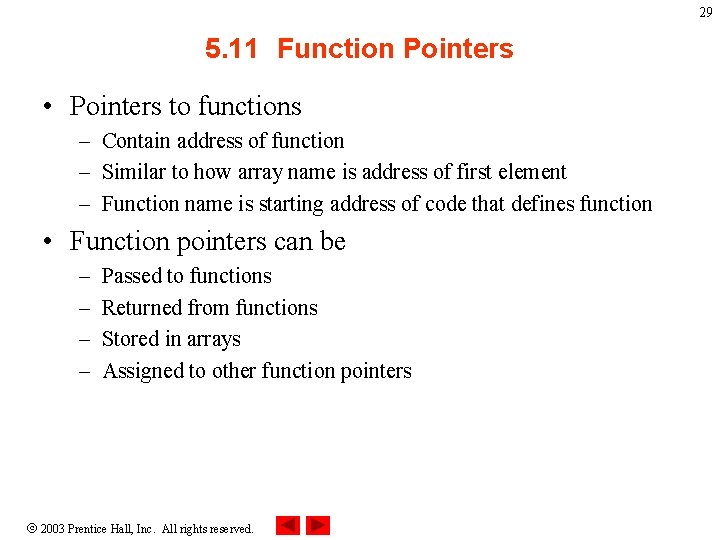
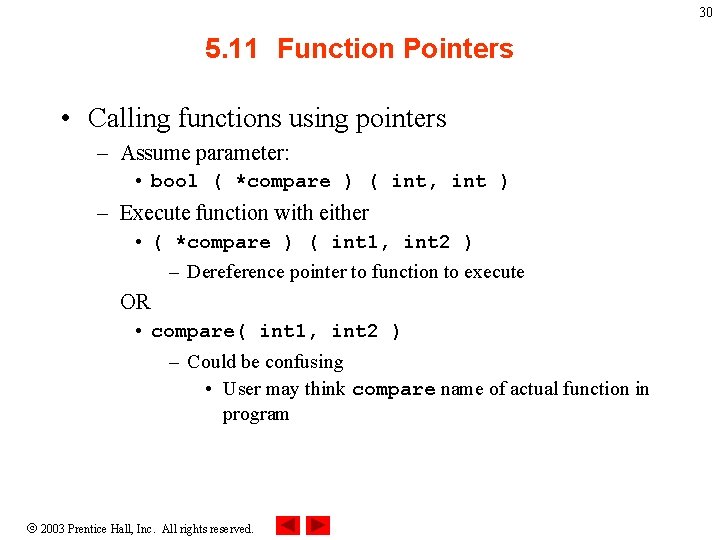
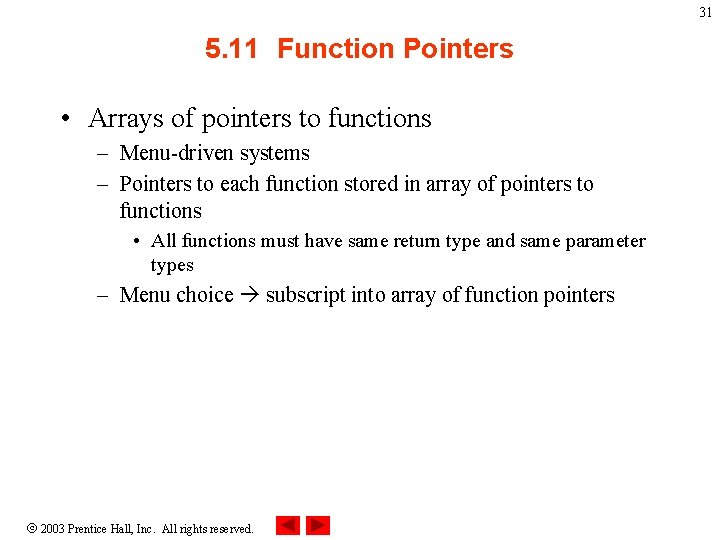
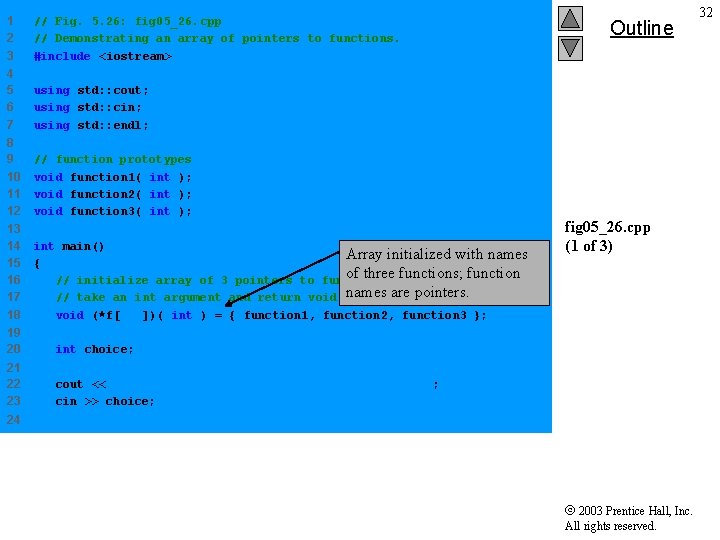
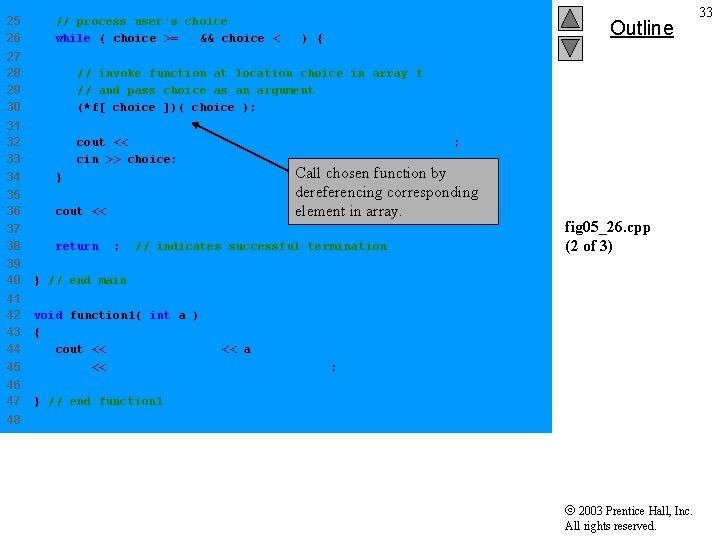
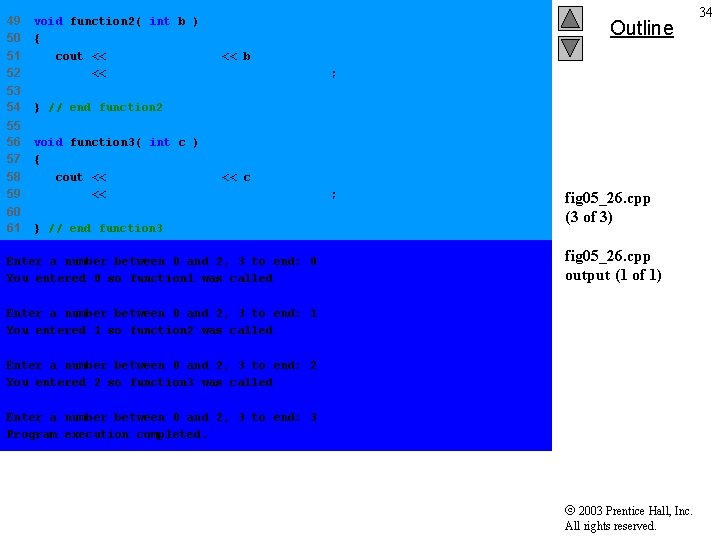
- Slides: 34
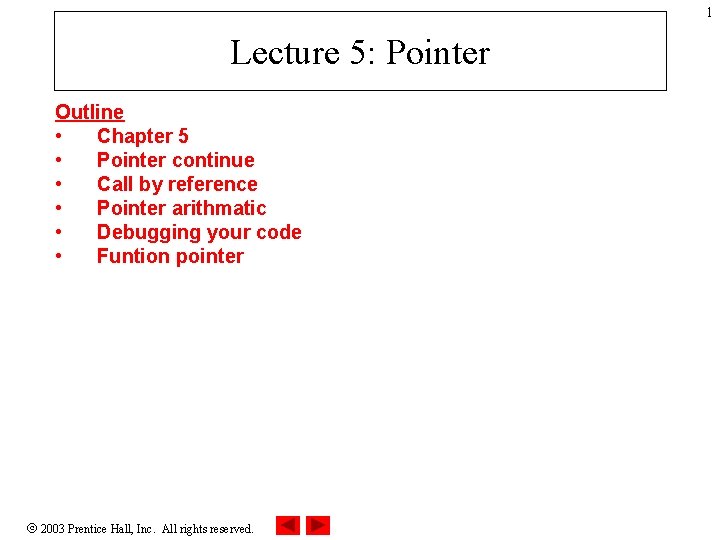
1 Lecture 5: Pointer Outline • Chapter 5 • Pointer continue • Call by reference • Pointer arithmatic • Debugging your code • Funtion pointer 2003 Prentice Hall, Inc. All rights reserved.
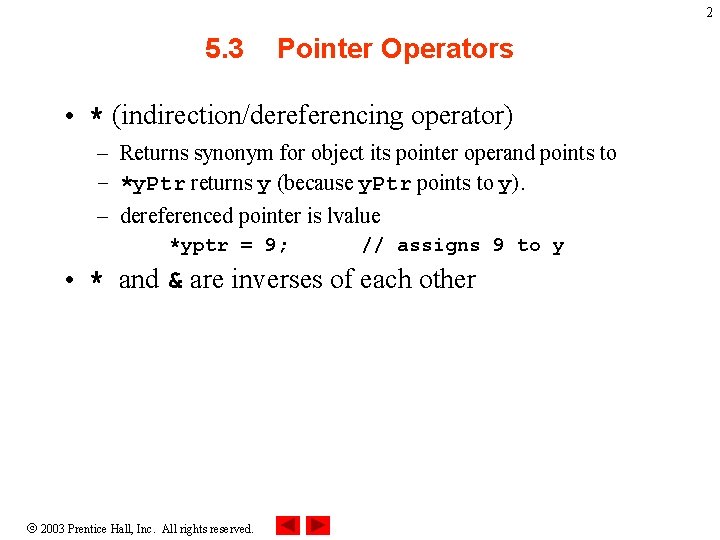
2 5. 3 Pointer Operators • * (indirection/dereferencing operator) – Returns synonym for object its pointer operand points to – *y. Ptr returns y (because y. Ptr points to y). – dereferenced pointer is lvalue *yptr = 9; // assigns 9 to y • * and & are inverses of each other 2003 Prentice Hall, Inc. All rights reserved.
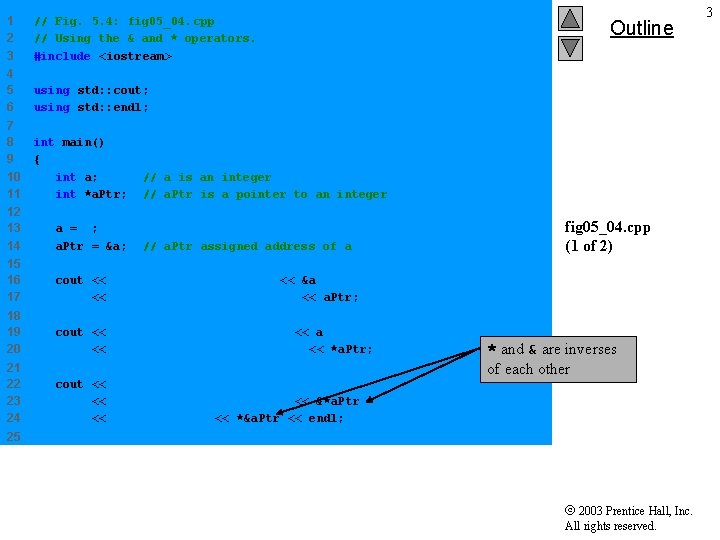
1 2 3 // Fig. 5. 4: fig 05_04. cpp // Using the & and * operators. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 int main() { int a; int *a. Ptr; 12 13 14 a = 7; a. Ptr = &a; 15 16 17 cout << "The address of a is " << &a << "n. The value of a. Ptr is " << a. Ptr; 18 19 20 cout << "nn. The value of a is " << a << "n. The value of *a. Ptr is " << *a. Ptr; 21 22 23 24 cout << "nn. Showing that * and & are inverses of " << "each other. n&*a. Ptr = " << &*a. Ptr << "n*&a. Ptr = " << *&a. Ptr << endl; Outline // a is an integer // a. Ptr is a pointer to an integer // a. Ptr assigned address of a fig 05_04. cpp (1 of 2) * and & are inverses of each other 25 2003 Prentice Hall, Inc. All rights reserved. 3
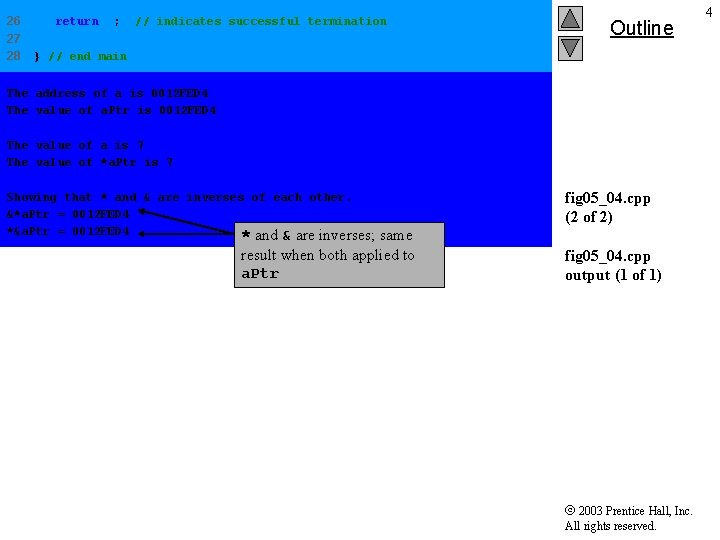
26 27 28 return 0; // indicates successful termination Outline } // end main The address of a is 0012 FED 4 The value of a. Ptr is 0012 FED 4 The value of a is 7 The value of *a. Ptr is 7 Showing that * and & are inverses of each other. &*a. Ptr = 0012 FED 4 *&a. Ptr = 0012 FED 4 * and & are inverses; same result when both applied to a. Ptr fig 05_04. cpp (2 of 2) fig 05_04. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 4
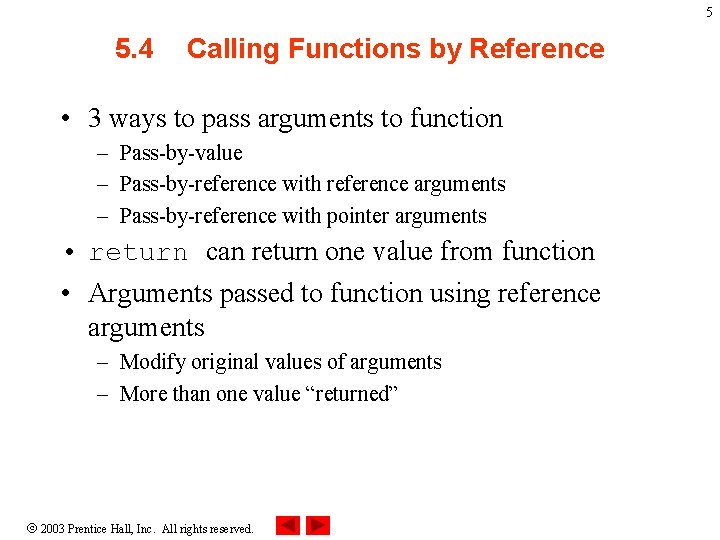
5 5. 4 Calling Functions by Reference • 3 ways to pass arguments to function – Pass-by-value – Pass-by-reference with reference arguments – Pass-by-reference with pointer arguments • return can return one value from function • Arguments passed to function using reference arguments – Modify original values of arguments – More than one value “returned” 2003 Prentice Hall, Inc. All rights reserved.
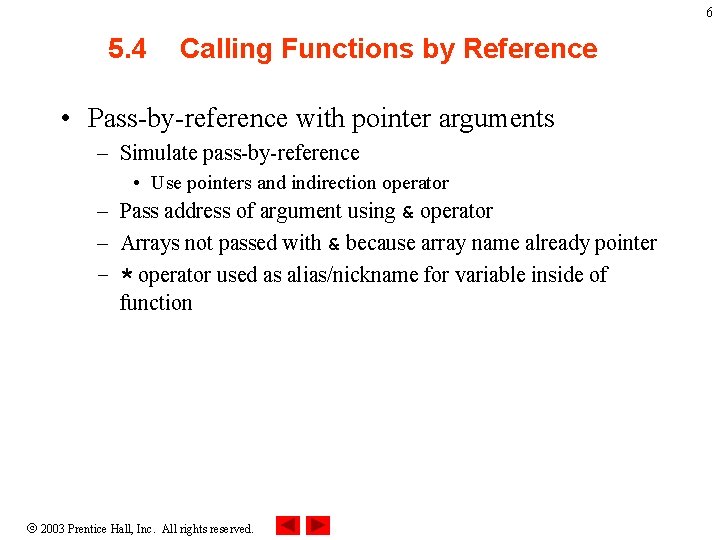
6 5. 4 Calling Functions by Reference • Pass-by-reference with pointer arguments – Simulate pass-by-reference • Use pointers and indirection operator – Pass address of argument using & operator – Arrays not passed with & because array name already pointer – * operator used as alias/nickname for variable inside of function 2003 Prentice Hall, Inc. All rights reserved.
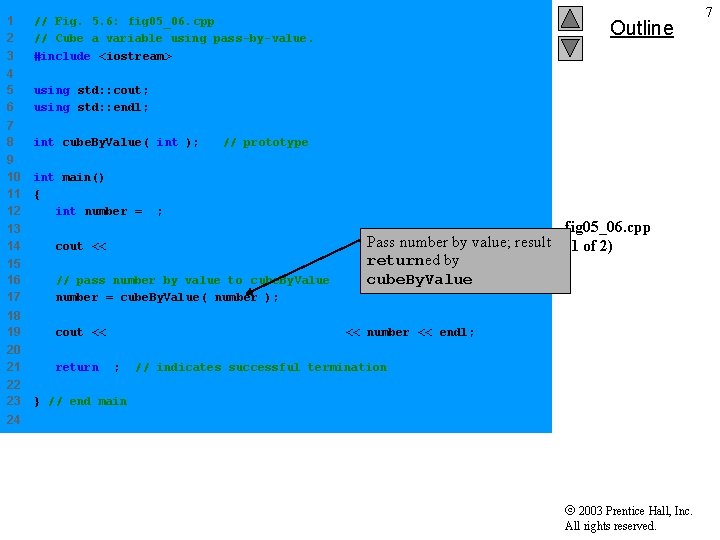
1 2 3 // Fig. 5. 6: fig 05_06. cpp // Cube a variable using pass-by-value. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 int cube. By. Value( int ); 9 10 11 12 int main() { int number = 5; Outline // prototype fig 05_06. cpp Pass number by value; result << number; (1 of 2) returned by cube. By. Value 13 14 cout << "The original value of number is " 15 16 17 // pass number by value to cube. By. Value number = cube. By. Value( number ); 18 19 cout << "n. The new value of number is " << number << endl; 20 21 return 0; 22 23 // indicates successful termination } // end main 24 2003 Prentice Hall, Inc. All rights reserved. 7
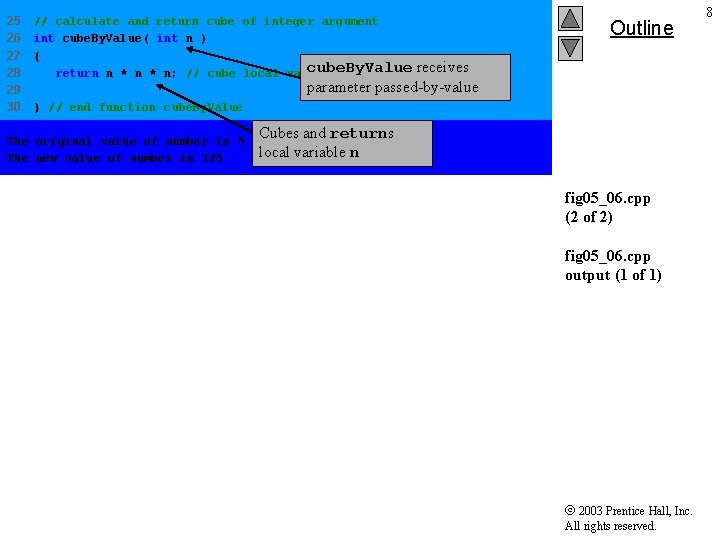
25 26 27 28 29 30 // calculate and return cube of integer argument int cube. By. Value( int n ) { cube. By. Value receives return n * n; // cube local variable n and return result Outline parameter passed-by-value } // end function cube. By. Value The original value of number is 5 The new value of number is 125 Cubes and returns local variable n fig 05_06. cpp (2 of 2) fig 05_06. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 8
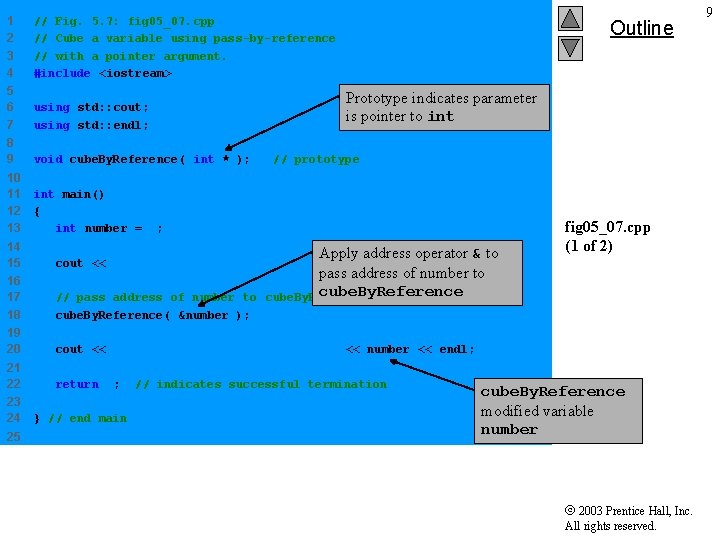
1 2 3 4 // Fig. 5. 7: fig 05_07. cpp // Cube a variable using pass-by-reference // with a pointer argument. #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 void cube. By. Reference( int * ); 10 11 12 13 int main() { int number = 5; Outline Prototype indicates parameter is pointer to int // prototype 14 15 cout << "The original value of number is " << number; 16 17 18 // pass address of number to cube. By. Reference( &number ); 19 20 cout << "n. The new value of number is " << number << endl; 21 22 return 0; 23 24 25 } // end main Apply address operator & to pass address of number to cube. By. Reference // indicates successful termination fig 05_07. cpp (1 of 2) cube. By. Reference modified variable number 2003 Prentice Hall, Inc. All rights reserved. 9
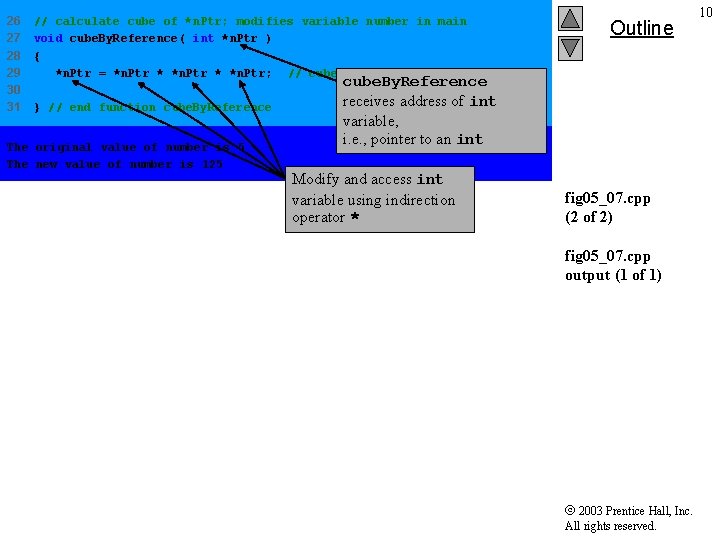
26 27 28 29 30 31 // calculate cube of *n. Ptr; modifies variable number in main void cube. By. Reference( int *n. Ptr ) { *n. Ptr = *n. Ptr * *n. Ptr; // cube *n. Ptr } // end function cube. By. Reference The original value of number is 5 The new value of number is 125 Outline cube. By. Reference receives address of int variable, i. e. , pointer to an int Modify and access int variable using indirection operator * fig 05_07. cpp (2 of 2) fig 05_07. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 10
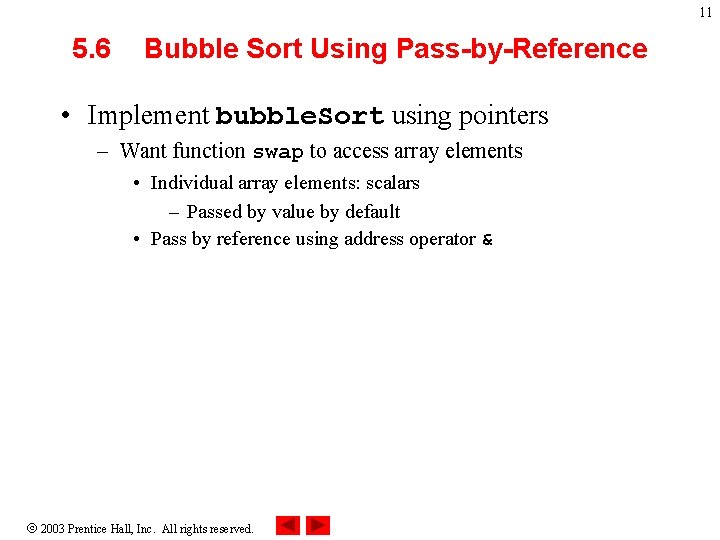
11 5. 6 Bubble Sort Using Pass-by-Reference • Implement bubble. Sort using pointers – Want function swap to access array elements • Individual array elements: scalars – Passed by value by default • Pass by reference using address operator & 2003 Prentice Hall, Inc. All rights reserved.
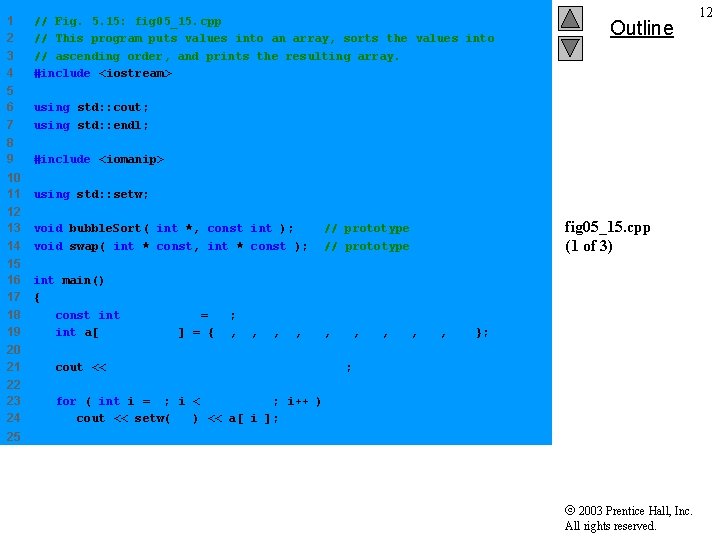
1 2 3 4 // Fig. 5. 15: fig 05_15. cpp // This program puts values into an array, sorts the values into // ascending order, and prints the resulting array. #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 #include <iomanip> 10 11 using std: : setw; 12 13 14 void bubble. Sort( int *, const int ); void swap( int * const, int * const ); 15 16 17 18 19 int main() { const int array. Size = 10; int a[ array. Size ] = { 2, 6, 4, 8, 10, 12, 89, 68, 45, 37 }; // prototype 20 21 cout << "Data items in original ordern" ; 22 23 24 for ( int i = 0; i < array. Size; i++ ) cout << setw( 4 ) << a[ i ]; Outline fig 05_15. cpp (1 of 3) 25 2003 Prentice Hall, Inc. All rights reserved. 12
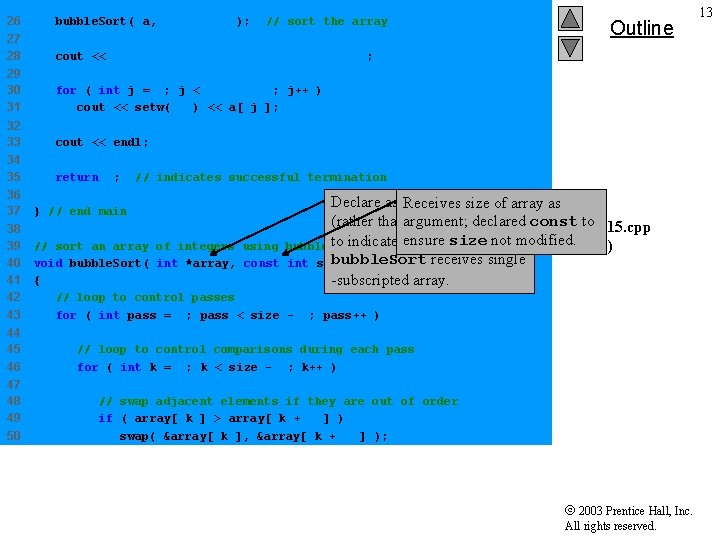
26 bubble. Sort( a, array. Size ); 27 28 cout << "n. Data items in ascending ordern" ; 29 30 31 for ( int j = 0; j < array. Size; j++ ) cout << setw( 4 ) << a[ j ]; 32 33 cout << endl; 34 35 return 0; // sort the array // indicates successful termination 36 37 } // end main 38 39 40 41 42 43 // sort an array of integers using void bubble. Sort( int *array, const { // loop to control passes for ( int pass = 0; pass < size - 1; pass++ ) 44 45 46 47 48 49 50 Outline Declare as int *array Receives size of array as declared const to (rather thanargument; int array[]) fig 05_15. cpp ensure size not modified. indicate function bubble to sort algorithm (2 of 3) bubble. Sort receives single int size ) -subscripted array. // loop to control comparisons during each pass for ( int k = 0; k < size - 1; k++ ) // swap adjacent elements if they are out of order if ( array[ k ] > array[ k + 1 ] ) swap( &array[ k ], &array[ k + 1 ] ); 2003 Prentice Hall, Inc. All rights reserved. 13
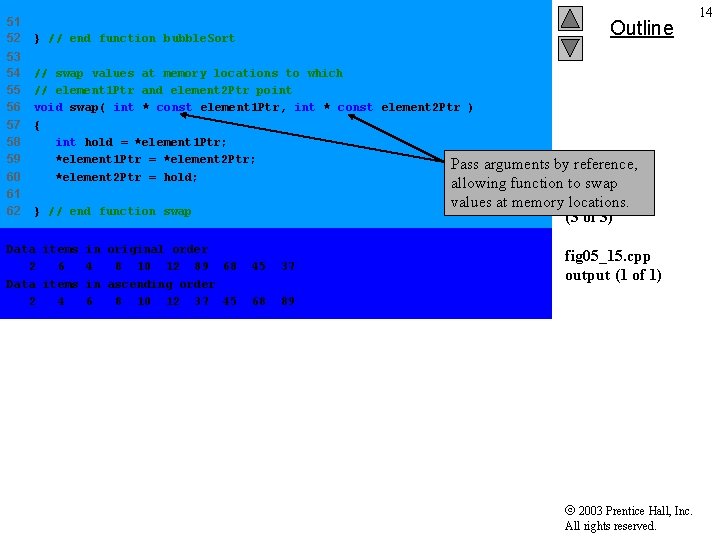
51 52 53 54 55 56 57 58 59 60 61 62 Outline } // end function bubble. Sort // swap values at memory locations to which // element 1 Ptr and element 2 Ptr point void swap( int * const element 1 Ptr, int * const element 2 Ptr ) { int hold = *element 1 Ptr; *element 1 Ptr = *element 2 Ptr; Pass *element 2 Ptr = hold; arguments by reference, allowing function to swap values at memoryfig 05_15. cpp locations. (3 of 3) } // end function swap Data items in original order 2 6 4 8 10 12 89 68 Data items in ascending order 2 4 6 8 10 12 37 45 45 37 68 89 fig 05_15. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 14
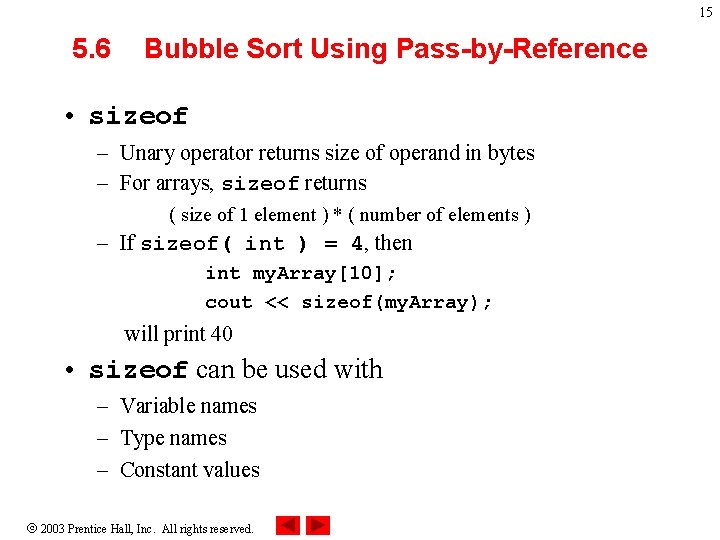
15 5. 6 Bubble Sort Using Pass-by-Reference • sizeof – Unary operator returns size of operand in bytes – For arrays, sizeof returns ( size of 1 element ) * ( number of elements ) – If sizeof( int ) = 4, then int my. Array[10]; cout << sizeof(my. Array); will print 40 • sizeof can be used with – Variable names – Type names – Constant values 2003 Prentice Hall, Inc. All rights reserved.
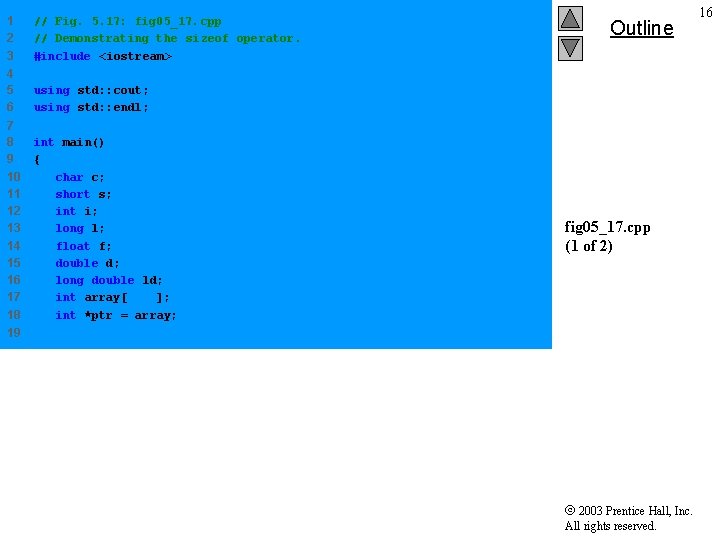
1 2 3 // Fig. 5. 17: fig 05_17. cpp // Demonstrating the sizeof operator. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 12 13 14 15 16 17 18 int main() { char c; short s; int i; long l; float f; double d; long double ld; int array[ 20 ]; int *ptr = array; Outline fig 05_17. cpp (1 of 2) 19 2003 Prentice Hall, Inc. All rights reserved. 16
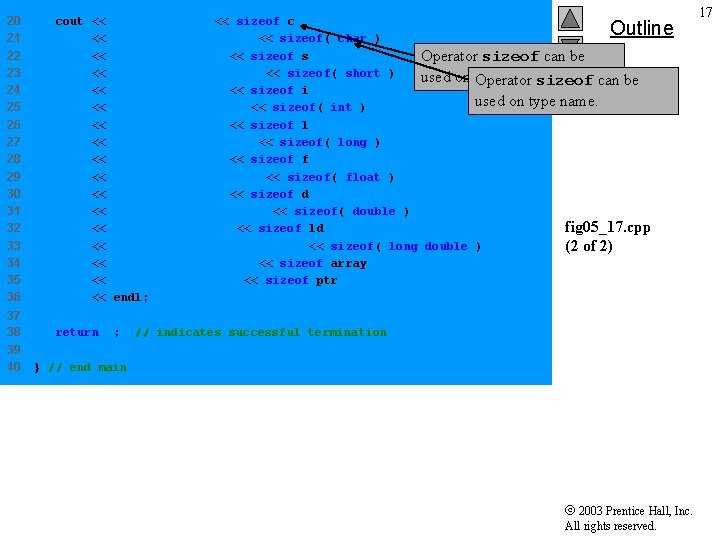
20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 cout << << << << << 37 38 return 0; 39 40 "sizeof c = " << sizeof c "tsizeof(char) = " << sizeof( char ) "nsizeof s = " << sizeof s Operator sizeof can be "tsizeof(short) = " << sizeof( short ) used on variable Operatorname. sizeof can be "nsizeof i = " << sizeof i used on type name. "tsizeof(int) = " << sizeof( int ) "nsizeof l = " << sizeof l "tsizeof(long) = " << sizeof( long ) "nsizeof f = " << sizeof f "tsizeof(float) = " << sizeof( float ) "nsizeof d = " << sizeof d "tsizeof(double) = " << sizeof( double ) fig 05_17. cpp "nsizeof ld = " << sizeof ld "tsizeof(long double) = " << sizeof( long double ) (2 of 2) "nsizeof array = " << sizeof array "nsizeof ptr = " << sizeof ptr endl; Outline // indicates successful termination } // end main 2003 Prentice Hall, Inc. All rights reserved. 17
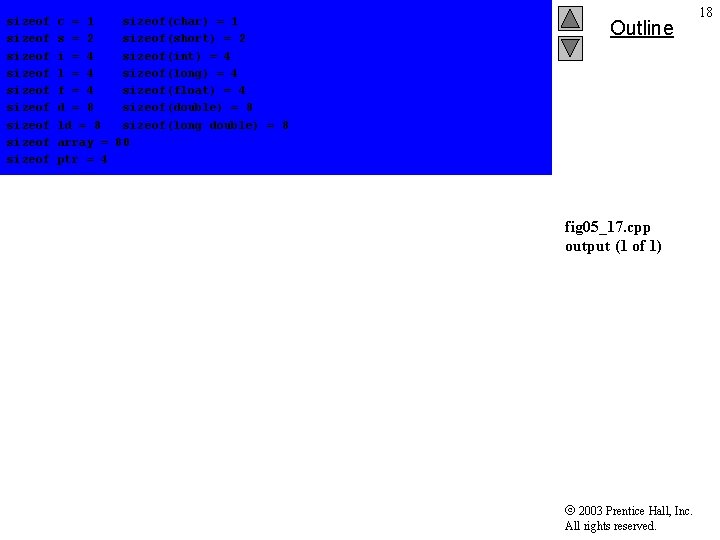
sizeof sizeof sizeof c = 1 sizeof(char) = 1 s = 2 sizeof(short) = 2 i = 4 sizeof(int) = 4 l = 4 sizeof(long) = 4 f = 4 sizeof(float) = 4 d = 8 sizeof(double) = 8 ld = 8 sizeof(long double) = 8 array = 80 ptr = 4 Outline fig 05_17. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 18
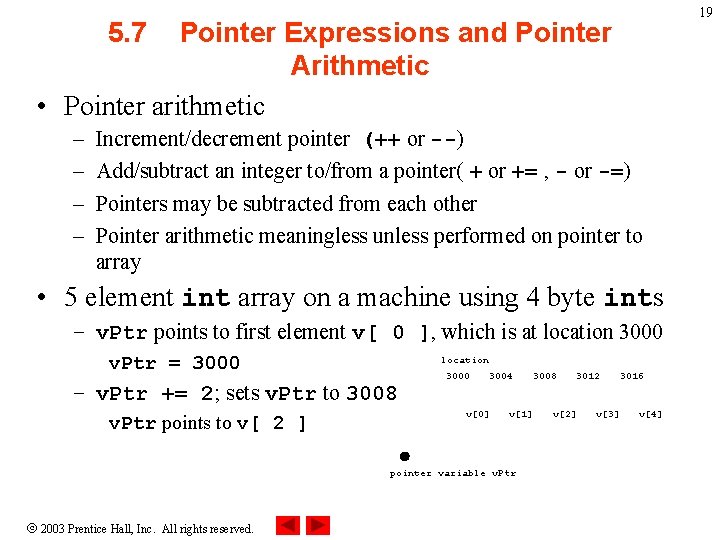
19 5. 7 Pointer Expressions and Pointer Arithmetic • Pointer arithmetic – – Increment/decrement pointer (++ or --) Add/subtract an integer to/from a pointer( + or += , - or -=) Pointers may be subtracted from each other Pointer arithmetic meaningless unless performed on pointer to array • 5 element int array on a machine using 4 byte ints – v. Ptr points to first element v[ 0 ], which is at location 3000 v. Ptr = 3000 location – v. Ptr += 2; sets v. Ptr to 3008 v. Ptr points to v[ 2 ] 3000 3004 v[0] v[1] pointer variable v. Ptr 2003 Prentice Hall, Inc. All rights reserved. 3008 3012 v[2] 3016 v[3] v[4]
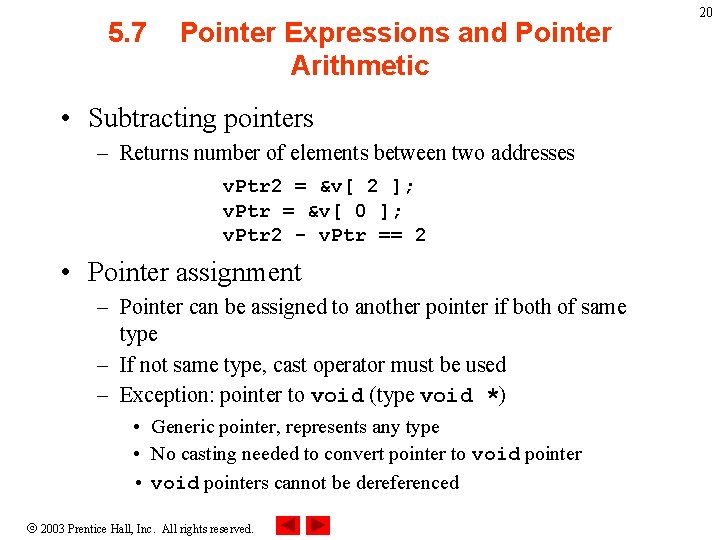
5. 7 Pointer Expressions and Pointer Arithmetic • Subtracting pointers – Returns number of elements between two addresses v. Ptr 2 = &v[ 2 ]; v. Ptr = &v[ 0 ]; v. Ptr 2 - v. Ptr == 2 • Pointer assignment – Pointer can be assigned to another pointer if both of same type – If not same type, cast operator must be used – Exception: pointer to void (type void *) • Generic pointer, represents any type • No casting needed to convert pointer to void pointer • void pointers cannot be dereferenced 2003 Prentice Hall, Inc. All rights reserved. 20
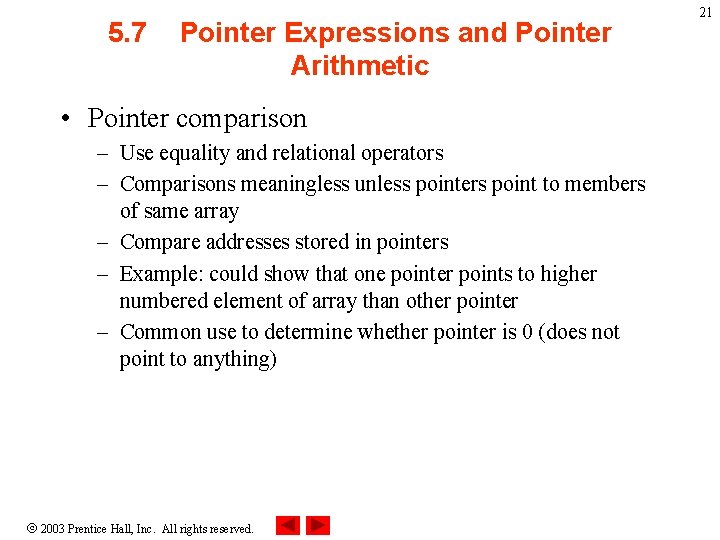
5. 7 Pointer Expressions and Pointer Arithmetic • Pointer comparison – Use equality and relational operators – Comparisons meaningless unless pointers point to members of same array – Compare addresses stored in pointers – Example: could show that one pointer points to higher numbered element of array than other pointer – Common use to determine whether pointer is 0 (does not point to anything) 2003 Prentice Hall, Inc. All rights reserved. 21
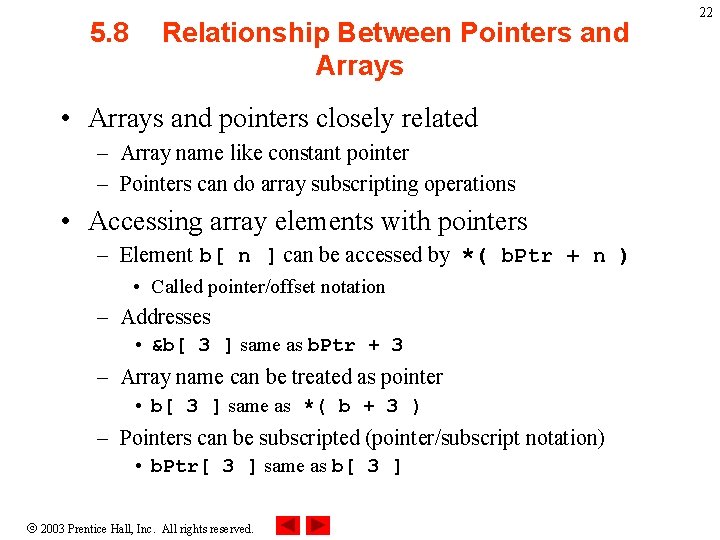
5. 8 Relationship Between Pointers and Arrays • Arrays and pointers closely related – Array name like constant pointer – Pointers can do array subscripting operations • Accessing array elements with pointers – Element b[ n ] can be accessed by *( b. Ptr + n ) • Called pointer/offset notation – Addresses • &b[ 3 ] same as b. Ptr + 3 – Array name can be treated as pointer • b[ 3 ] same as *( b + 3 ) – Pointers can be subscripted (pointer/subscript notation) • b. Ptr[ 3 ] same as b[ 3 ] 2003 Prentice Hall, Inc. All rights reserved. 22
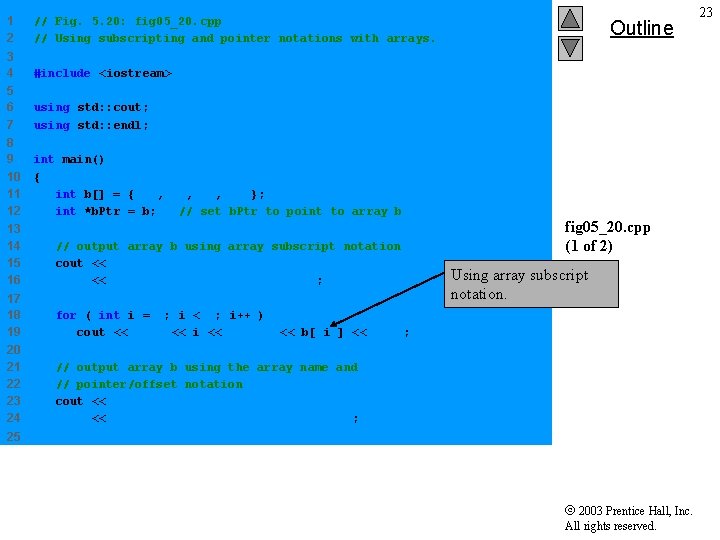
1 2 // Fig. 5. 20: fig 05_20. cpp // Using subscripting and pointer notations with arrays. 3 4 #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 10 11 12 int main() { int b[] = { 10, 20, 30, 40 }; int *b. Ptr = b; // set b. Ptr to point to array b 13 14 15 16 // output array b using array subscript notation cout << "Array b printed with: n" << "Array subscript notationn" ; 17 18 19 for ( int i = 0; i < 4; i++ ) cout << "b[" << i << "] = " << b[ i ] << 'n'; 20 21 22 23 24 // output array b using the array name and // pointer/offset notation cout << "n. Pointer/offset notation where " << "the pointer is the array namen" ; Outline fig 05_20. cpp (1 of 2) Using array subscript notation. 25 2003 Prentice Hall, Inc. All rights reserved. 23
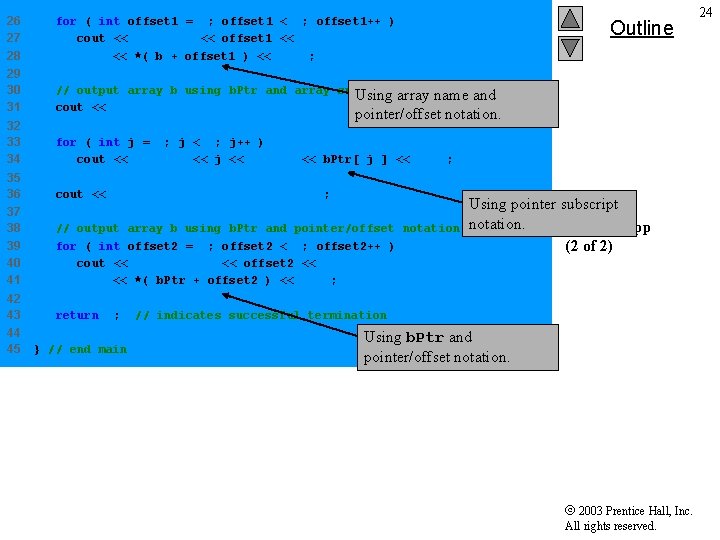
26 27 28 for ( int offset 1 = 0; offset 1 < 4; offset 1++ ) cout << "*(b + " << offset 1 << ") = " << *( b + offset 1 ) << 'n'; 29 30 31 // output array b using b. Ptr and array subscript notation Using array name cout << "n. Pointer subscript notationn"; 32 33 34 for ( int j = 0; j < 4; j++ ) cout << "b. Ptr[" << j << "] = " << b. Ptr[ j ] << 'n'; 35 36 cout << "n. Pointer/offset notationn"; 37 38 39 40 41 // output array b using b. Ptr and pointer/offset notation for ( int offset 2 = 0; offset 2 < 4; offset 2++ ) cout << "*(b. Ptr + " << offset 2 << ") = " << *( b. Ptr + offset 2 ) << 'n'; 42 43 return 0; 44 45 Outline and pointer/offset notation. } // end main Using pointer subscript notation. fig 05_20. cpp (2 of 2) // indicates successful termination Using b. Ptr and pointer/offset notation. 2003 Prentice Hall, Inc. All rights reserved. 24
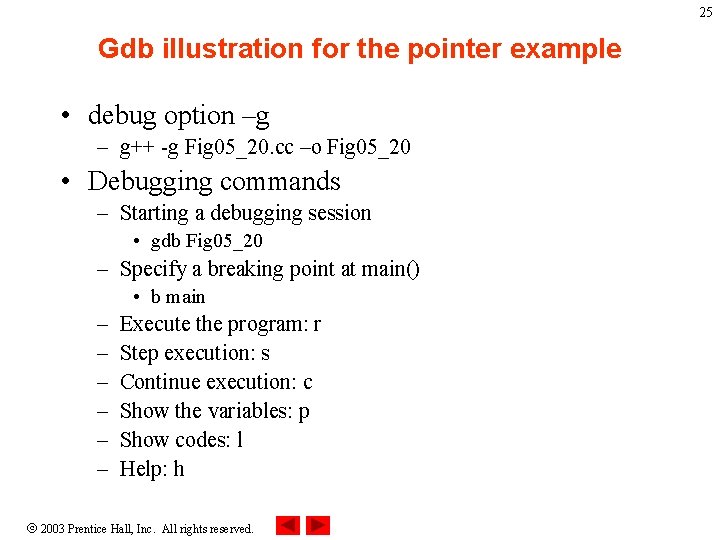
25 Gdb illustration for the pointer example • debug option –g – g++ -g Fig 05_20. cc –o Fig 05_20 • Debugging commands – Starting a debugging session • gdb Fig 05_20 – Specify a breaking point at main() • b main – – – Execute the program: r Step execution: s Continue execution: c Show the variables: p Show codes: l Help: h 2003 Prentice Hall, Inc. All rights reserved.
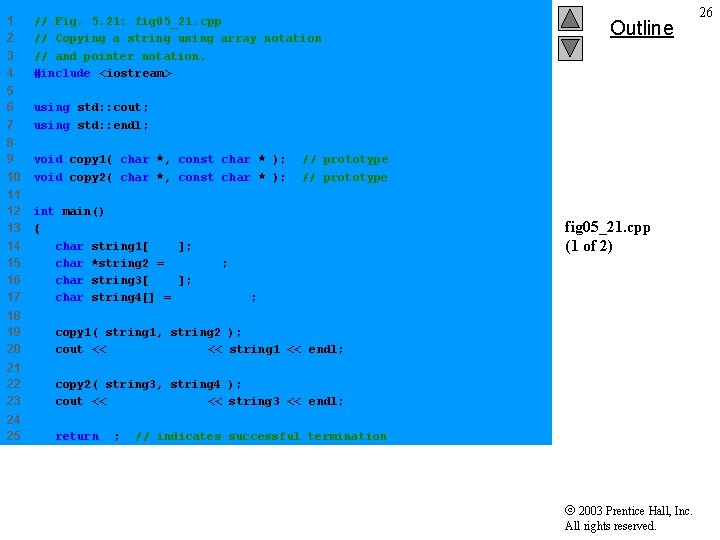
1 2 3 4 // Fig. 5. 21: fig 05_21. cpp // Copying a string using array notation // and pointer notation. #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 10 void copy 1( char *, const char * ); void copy 2( char *, const char * ); 11 12 13 14 15 16 17 int main() { char string 1[ 10 ]; char *string 2 = "Hello"; char string 3[ 10 ]; char string 4[] = "Good Bye"; Outline // prototype 18 19 20 copy 1( string 1, string 2 ); cout << "string 1 = " << string 1 << endl; 21 22 23 copy 2( string 3, string 4 ); cout << "string 3 = " << string 3 << endl; 24 25 return 0; fig 05_21. cpp (1 of 2) // indicates successful termination 2003 Prentice Hall, Inc. All rights reserved. 26
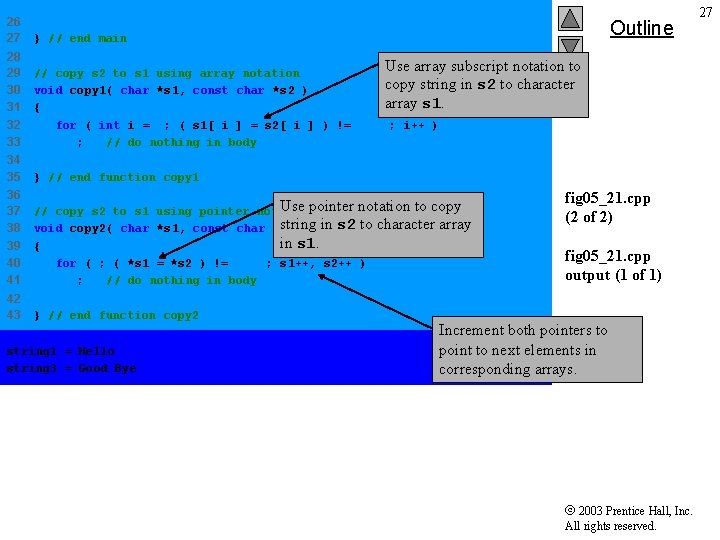
26 27 } // end main 28 29 30 31 32 33 Use array subscript notation to // copy s 2 to s 1 using array notation copy string in s 2 to character void copy 1( char *s 1, const char *s 2 ) array s 1. { for ( int i = 0; ( s 1[ i ] = s 2[ i ] ) != '