1 Introducing ASML Classes Structured Values Sets Sequences
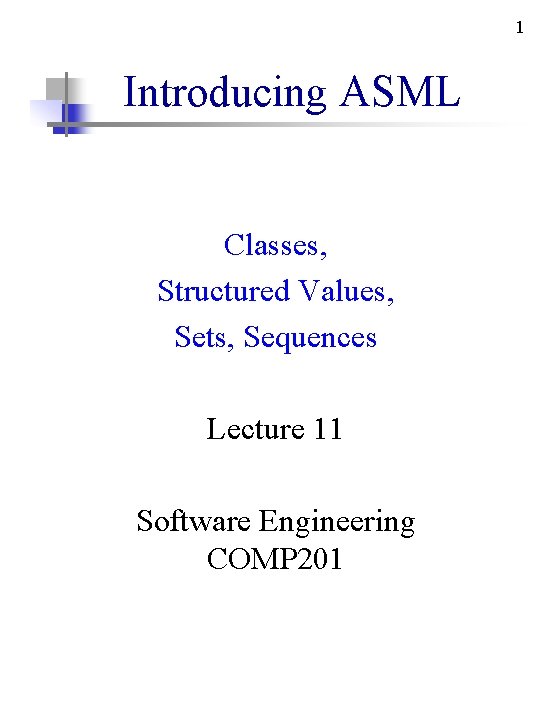
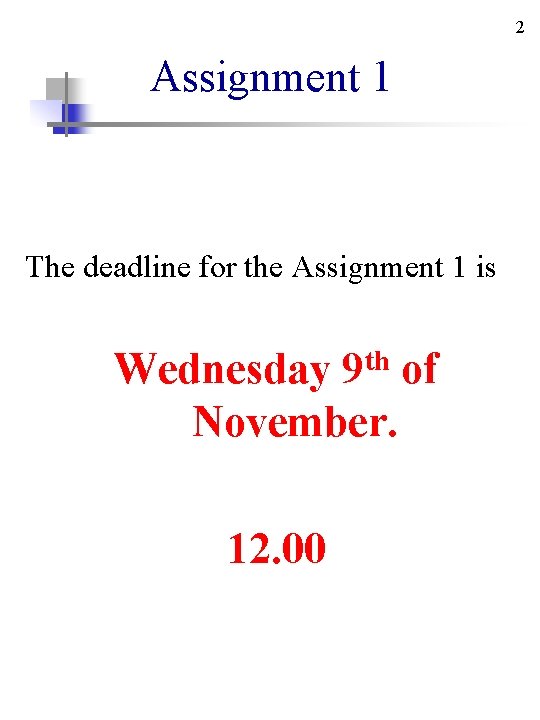
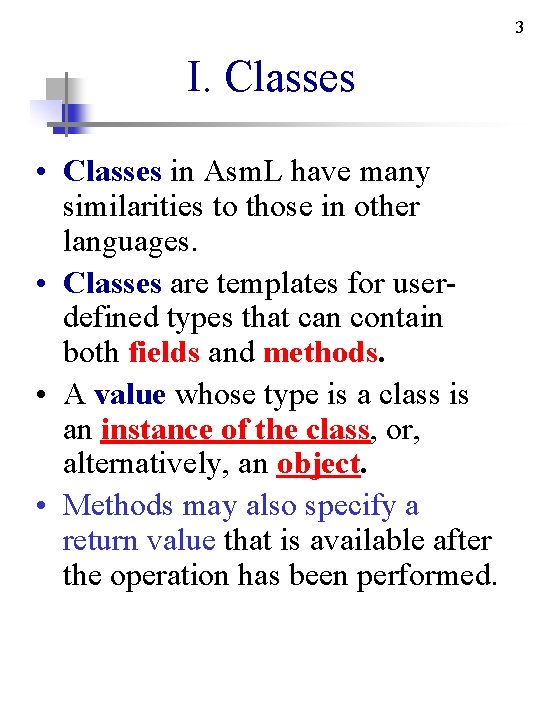
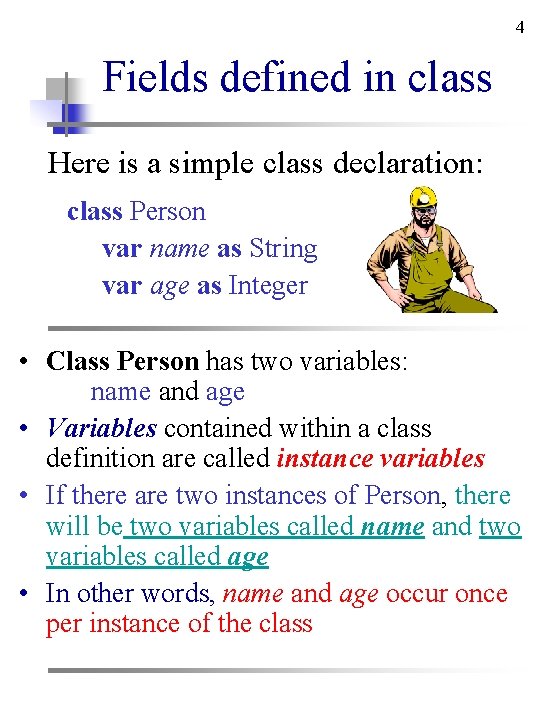
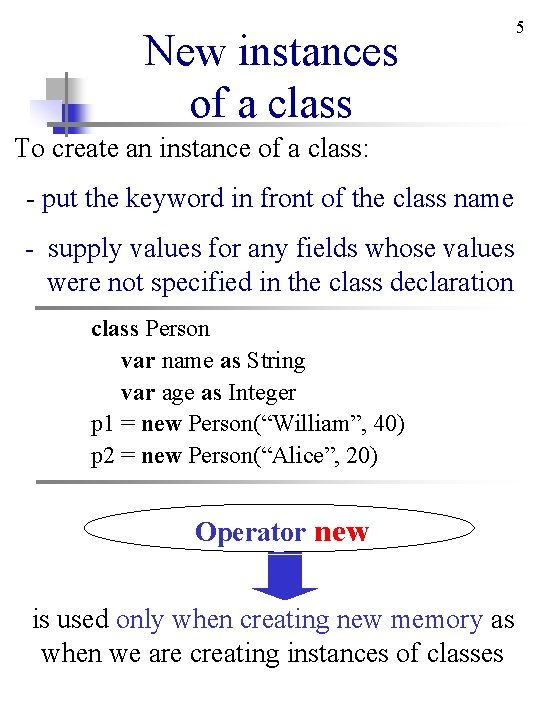
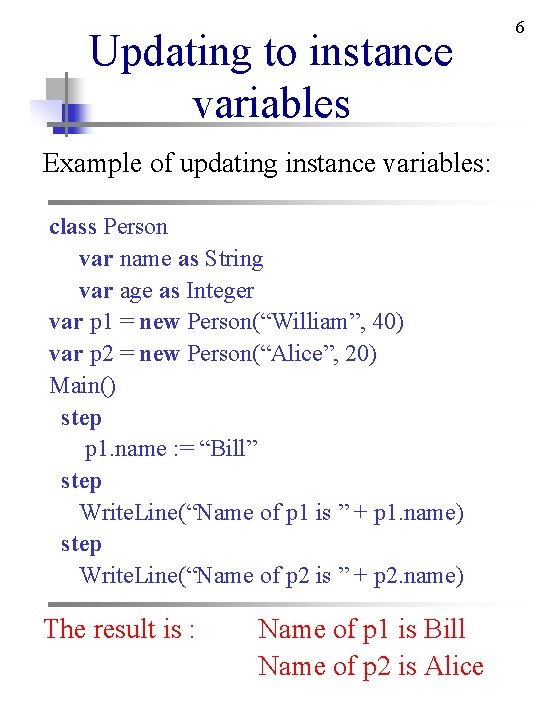
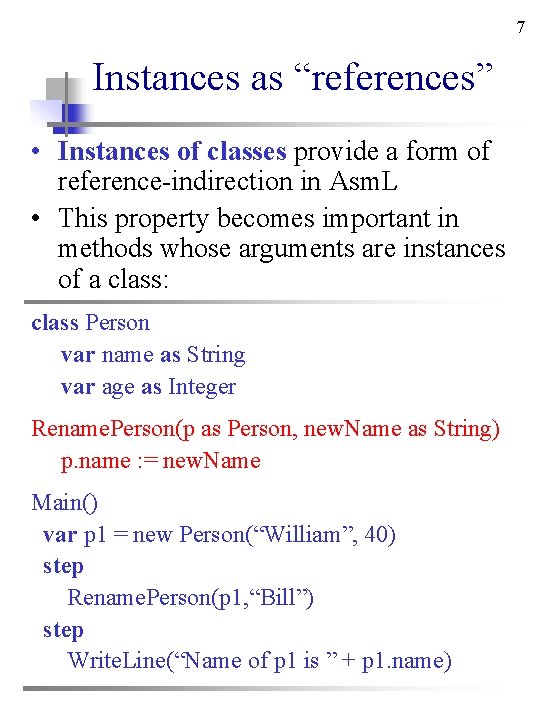
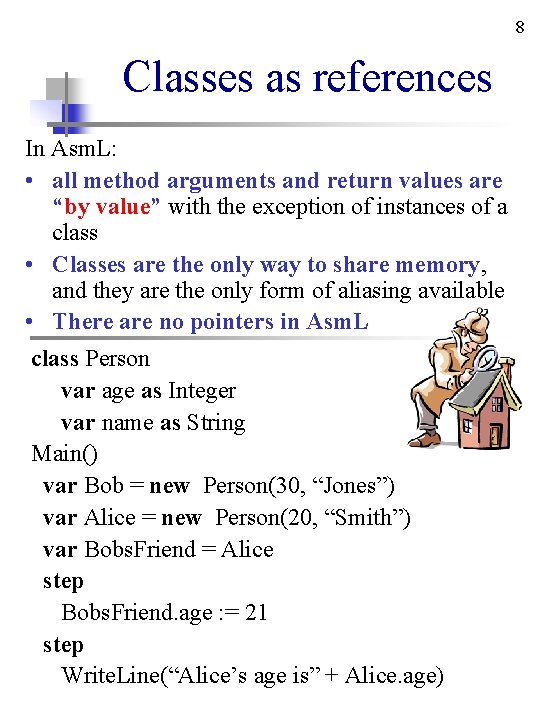
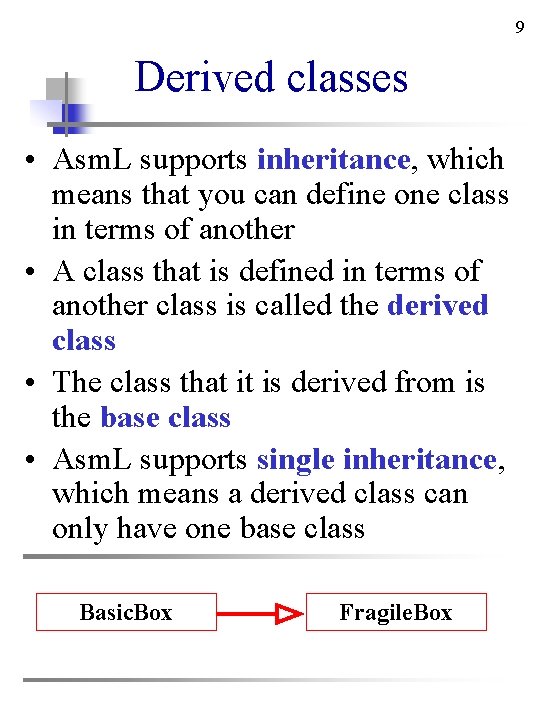
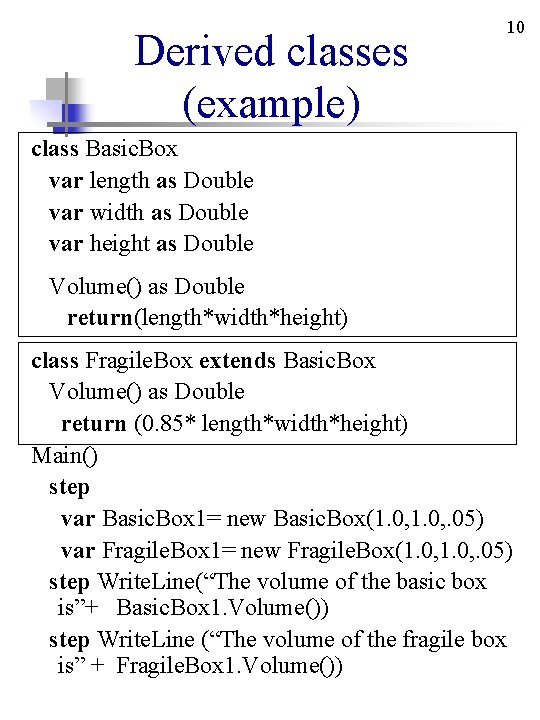
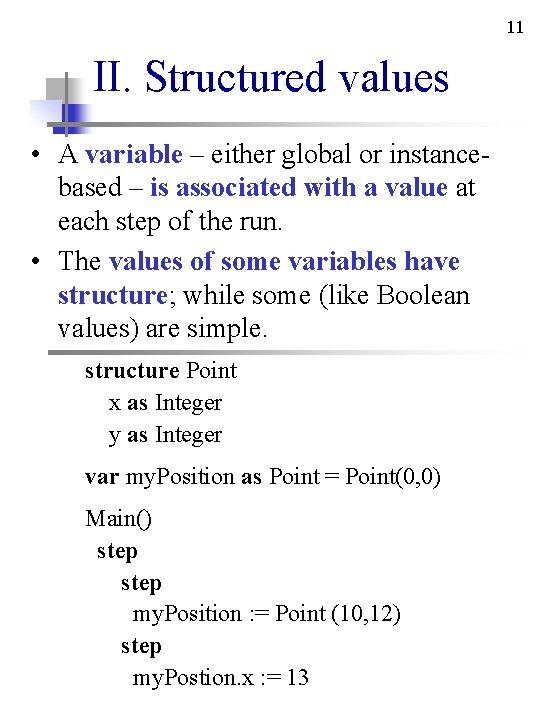
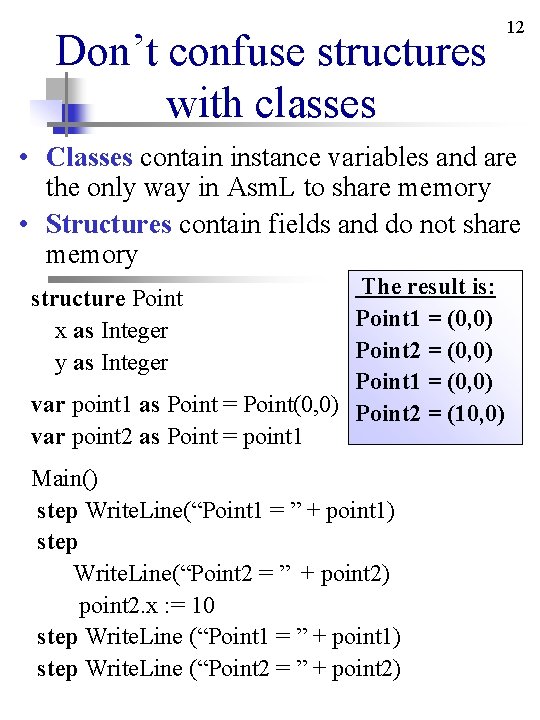
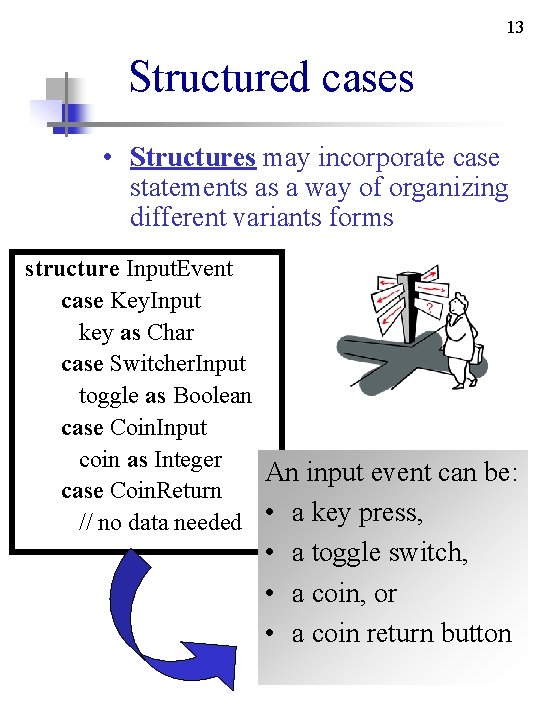
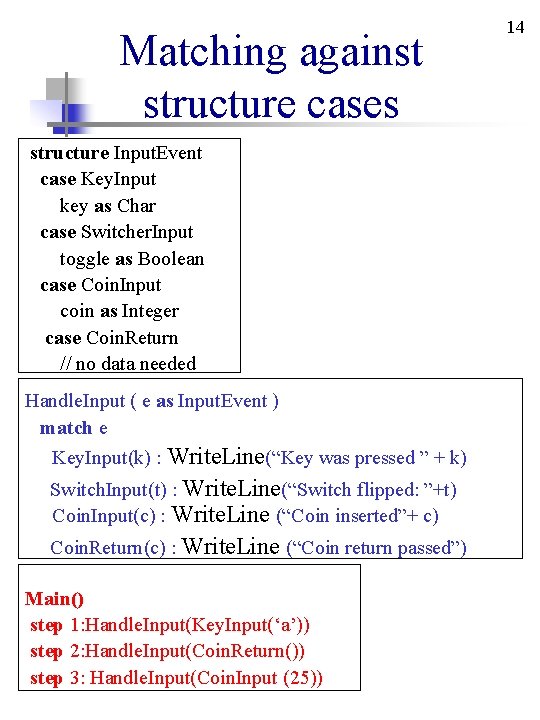
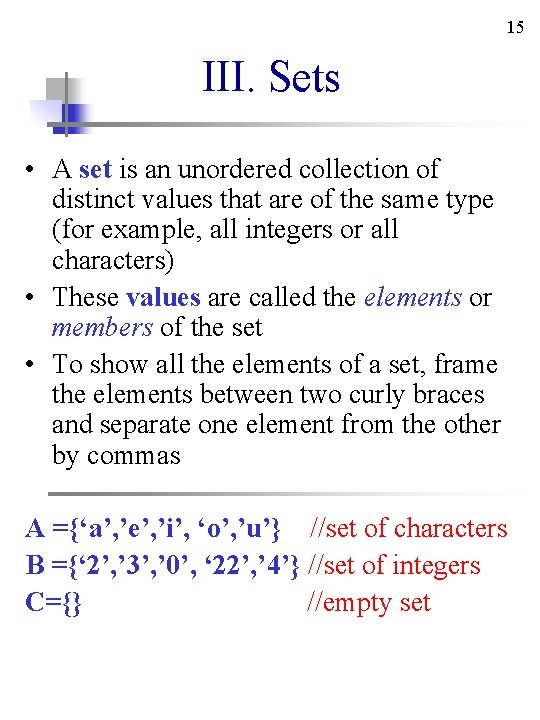
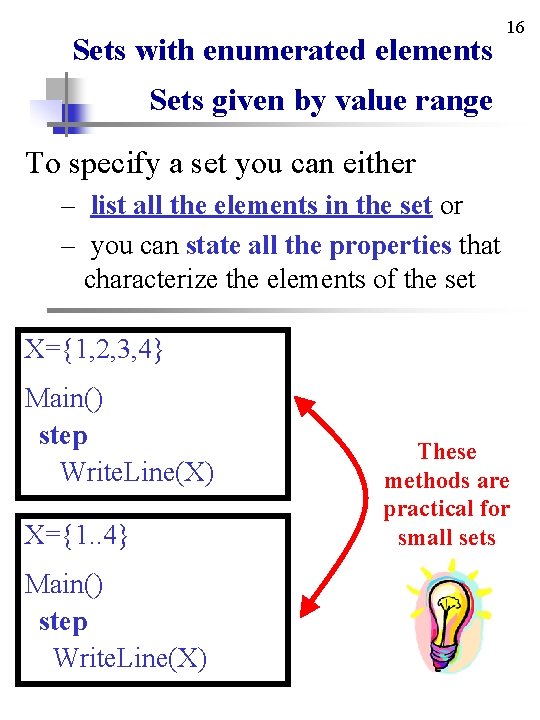
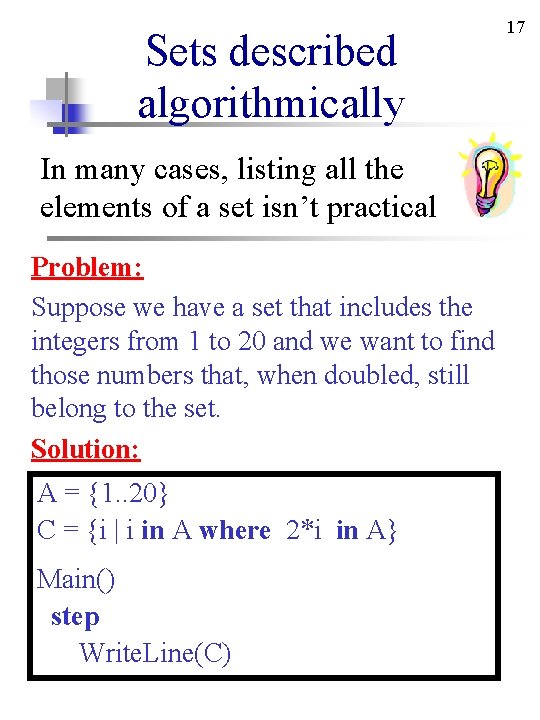
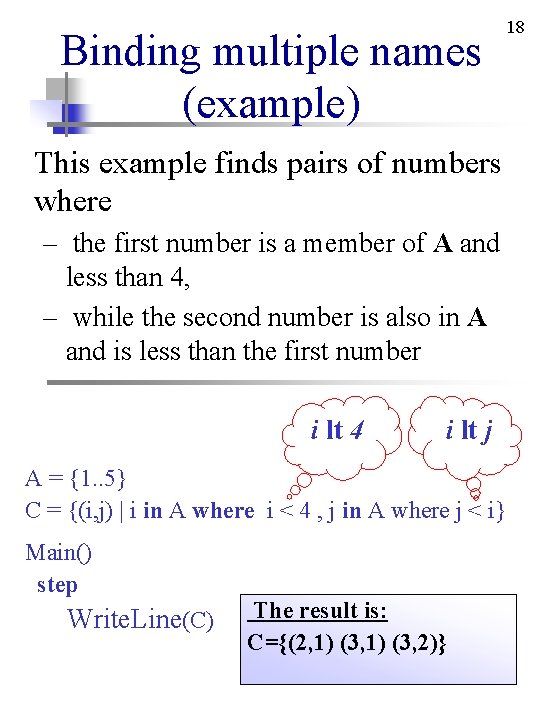
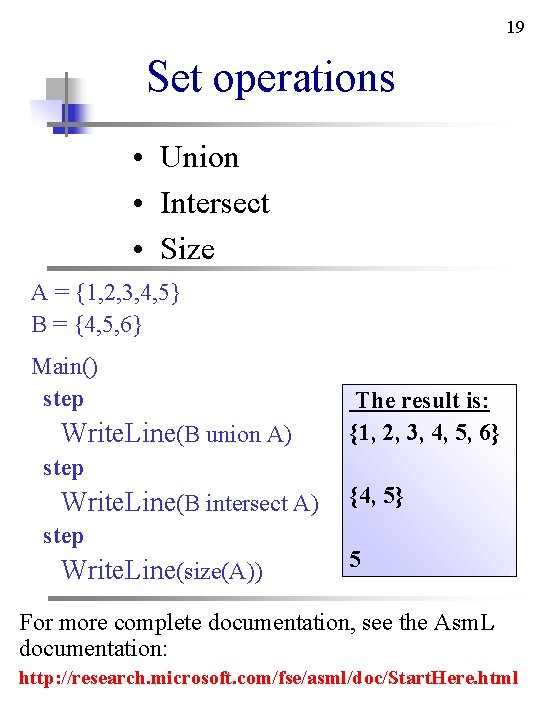
- Slides: 19
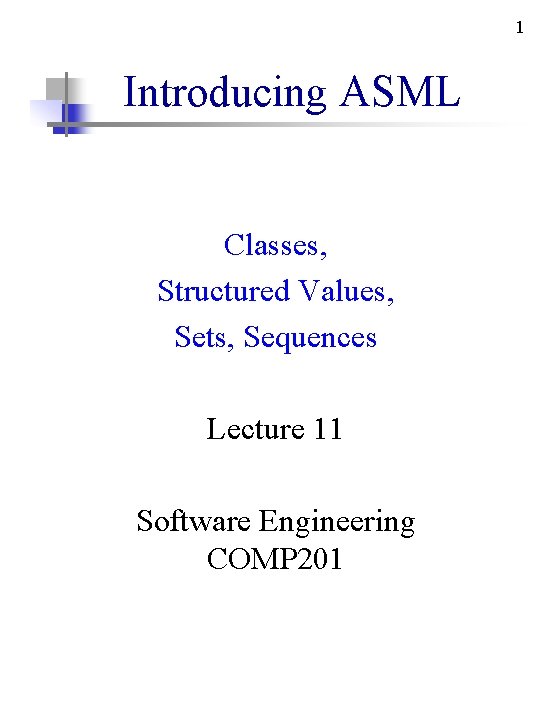
1 Introducing ASML Classes, Structured Values, Sets, Sequences Lecture 11 Software Engineering COMP 201
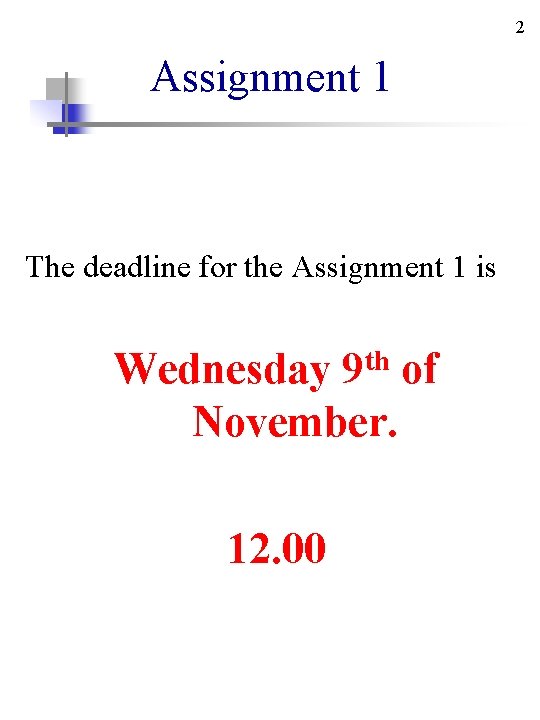
2 Assignment 1 The deadline for the Assignment 1 is Wednesday 9 th of November. 12. 00
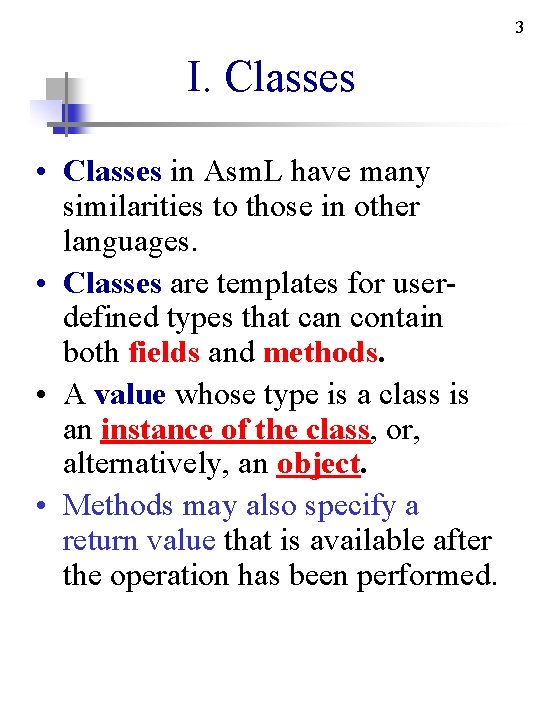
3 I. Classes • Classes in Asm. L have many similarities to those in other languages. • Classes are templates for userdefined types that can contain both fields and methods. • A value whose type is a class is an instance of the class, or, alternatively, an object. • Methods may also specify a return value that is available after the operation has been performed.
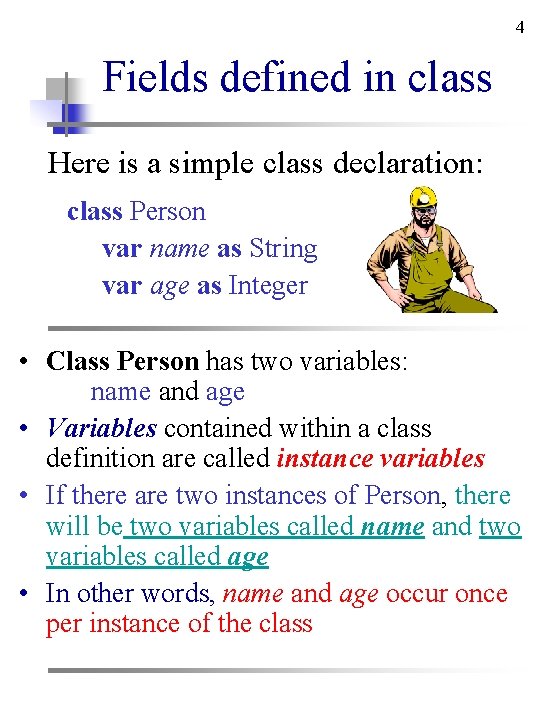
4 Fields defined in class Here is a simple class declaration: class Person var name as String var age as Integer • Class Person has two variables: name and age • Variables contained within a class definition are called instance variables • If there are two instances of Person, there will be two variables called name and two variables called age • In other words, name and age occur once per instance of the class
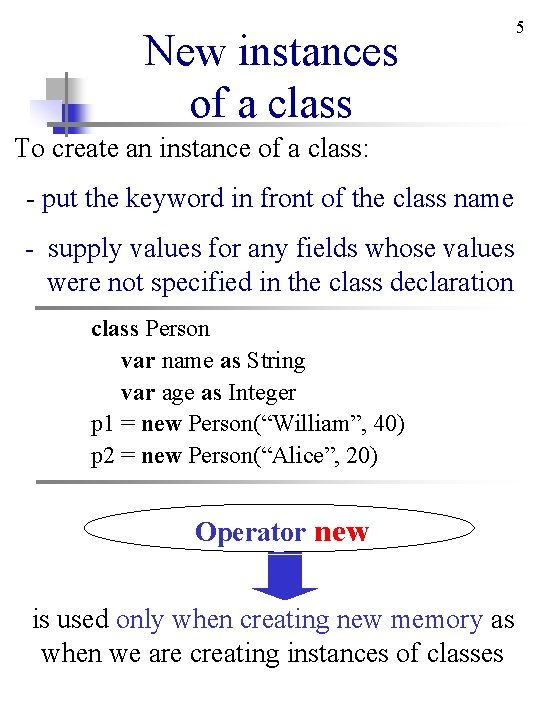
New instances of a class To create an instance of a class: - put the keyword in front of the class name - supply values for any fields whose values were not specified in the class declaration class Person var name as String var age as Integer p 1 = new Person(“William”, 40) p 2 = new Person(“Alice”, 20) Operator new is used only when creating new memory as when we are creating instances of classes 5
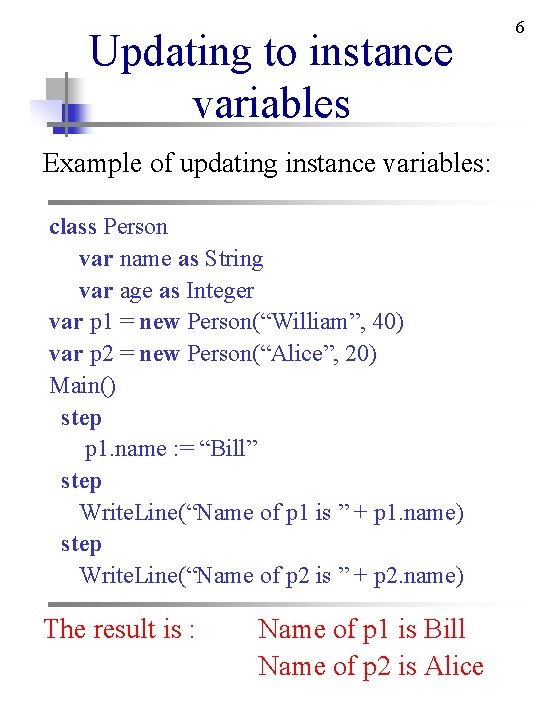
Updating to instance variables Example of updating instance variables: class Person var name as String var age as Integer var p 1 = new Person(“William”, 40) var p 2 = new Person(“Alice”, 20) Main() step p 1. name : = “Bill” step Write. Line(“Name of p 1 is ” + p 1. name) step Write. Line(“Name of p 2 is ” + p 2. name) The result is : Name of p 1 is Bill Name of p 2 is Alice 6
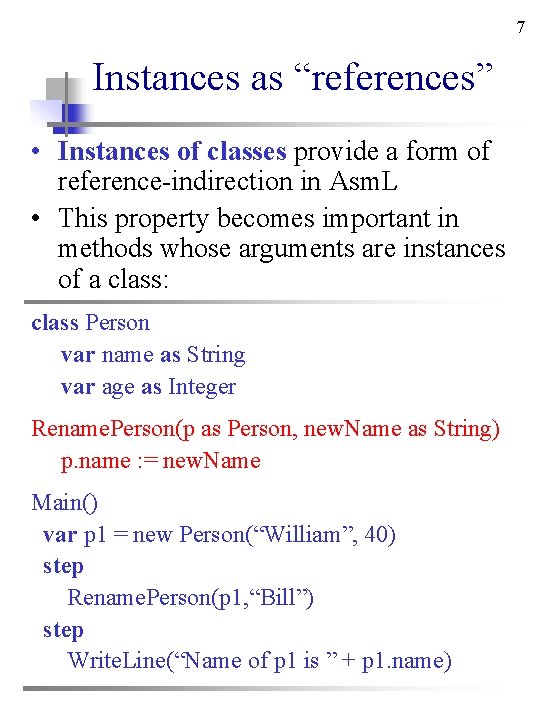
7 Instances as “references” • Instances of classes provide a form of reference-indirection in Asm. L • This property becomes important in methods whose arguments are instances of a class: class Person var name as String var age as Integer Rename. Person(p as Person, new. Name as String) p. name : = new. Name Main() var p 1 = new Person(“William”, 40) step Rename. Person(p 1, “Bill”) step Write. Line(“Name of p 1 is ” + p 1. name)
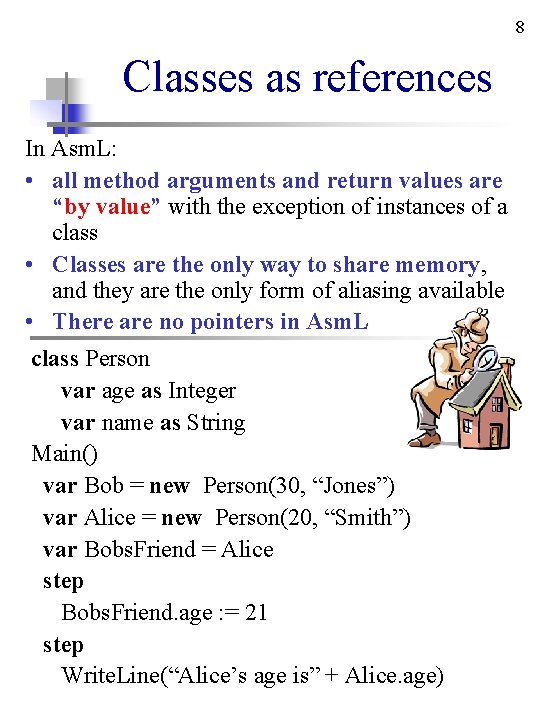
8 Classes as references In Asm. L: • all method arguments and return values are “by value” with the exception of instances of a class • Classes are the only way to share memory, and they are the only form of aliasing available • There are no pointers in Asm. L class Person var age as Integer var name as String Main() var Bob = new Person(30, “Jones”) var Alice = new Person(20, “Smith”) var Bobs. Friend = Alice step Bobs. Friend. age : = 21 step Write. Line(“Alice’s age is” + Alice. age)
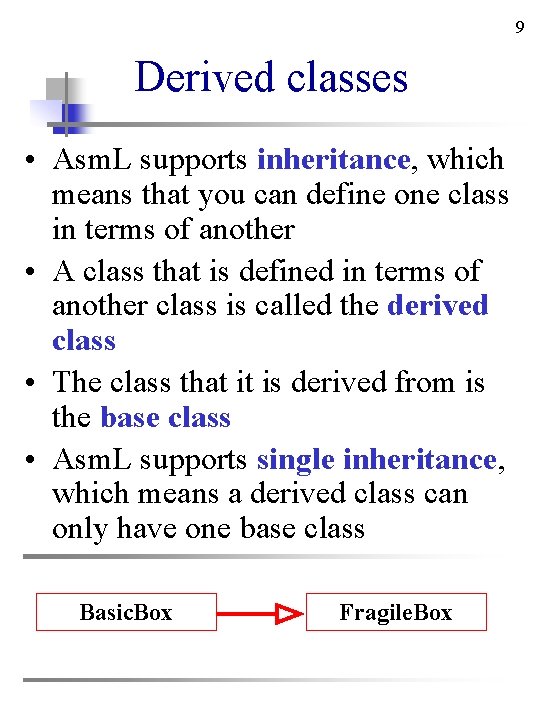
9 Derived classes • Asm. L supports inheritance, which means that you can define one class in terms of another • A class that is defined in terms of another class is called the derived class • The class that it is derived from is the base class • Asm. L supports single inheritance, which means a derived class can only have one base class Basic. Box Fragile. Box
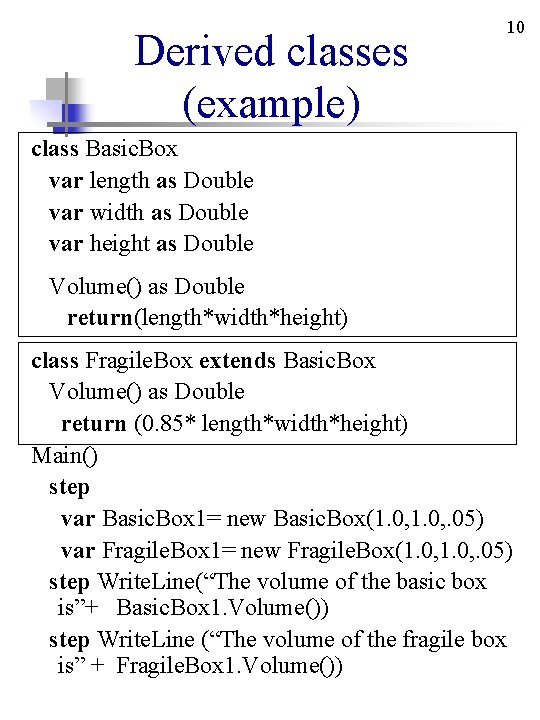
Derived classes (example) 10 class Basic. Box var length as Double var width as Double var height as Double Volume() as Double return(length*width*height) class Fragile. Box extends Basic. Box Volume() as Double return (0. 85* length*width*height) Main() step var Basic. Box 1= new Basic. Box(1. 0, . 05) var Fragile. Box 1= new Fragile. Box(1. 0, . 05) step Write. Line(“The volume of the basic box is”+ Basic. Box 1. Volume()) step Write. Line (“The volume of the fragile box is” + Fragile. Box 1. Volume())
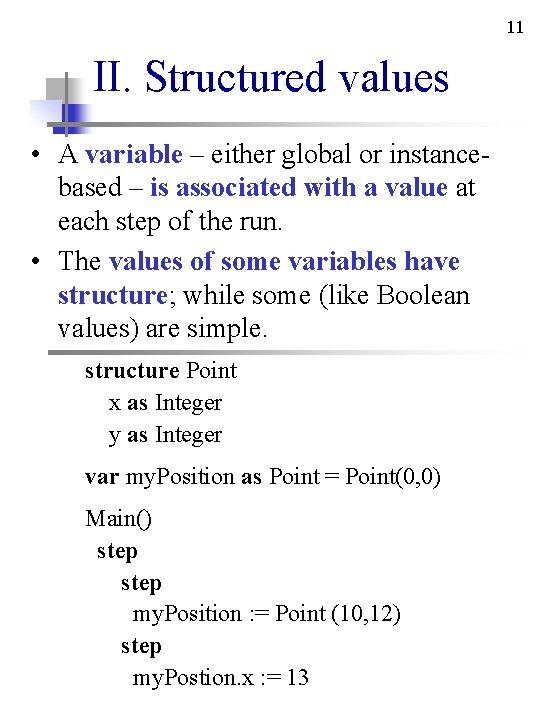
11 II. Structured values • A variable – either global or instancebased – is associated with a value at each step of the run. • The values of some variables have structure; while some (like Boolean values) are simple. structure Point x as Integer y as Integer var my. Position as Point = Point(0, 0) Main() step my. Position : = Point (10, 12) step my. Postion. x : = 13
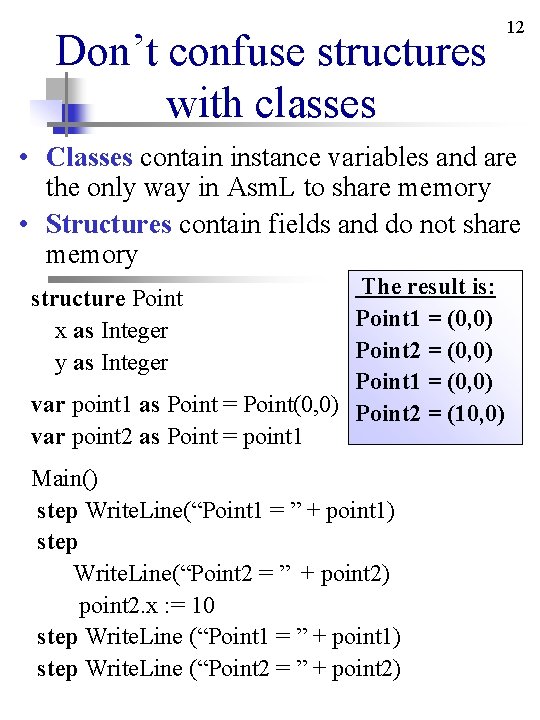
Don’t confuse structures with classes 12 • Classes contain instance variables and are the only way in Asm. L to share memory • Structures contain fields and do not share memory The result is: Point 1 = (0, 0) Point 2 = (0, 0) Point 1 = (0, 0) var point 1 as Point = Point(0, 0) Point 2 = (10, 0) var point 2 as Point = point 1 structure Point x as Integer y as Integer Main() step Write. Line(“Point 1 = ” + point 1) step Write. Line(“Point 2 = ” + point 2) point 2. x : = 10 step Write. Line (“Point 1 = ” + point 1) step Write. Line (“Point 2 = ” + point 2)
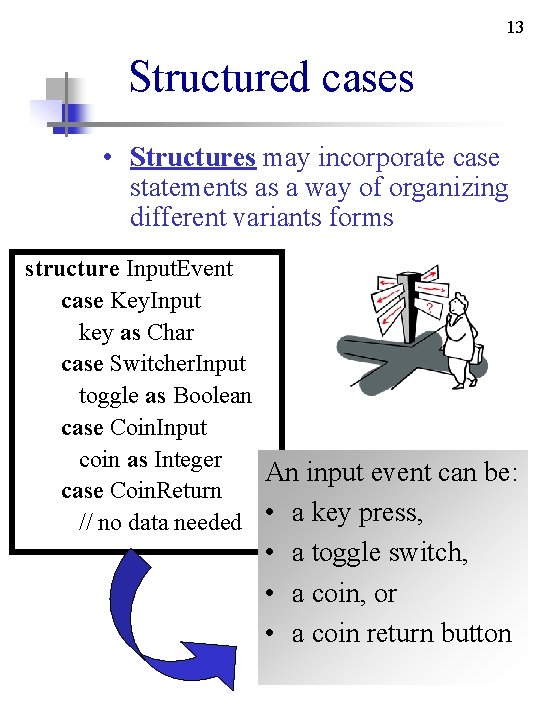
13 Structured cases • Structures may incorporate case statements as a way of organizing different variants forms structure Input. Event case Key. Input key as Char case Switcher. Input toggle as Boolean case Coin. Input coin as Integer An input event can be: case Coin. Return // no data needed • a key press, • a toggle switch, • a coin, or • a coin return button
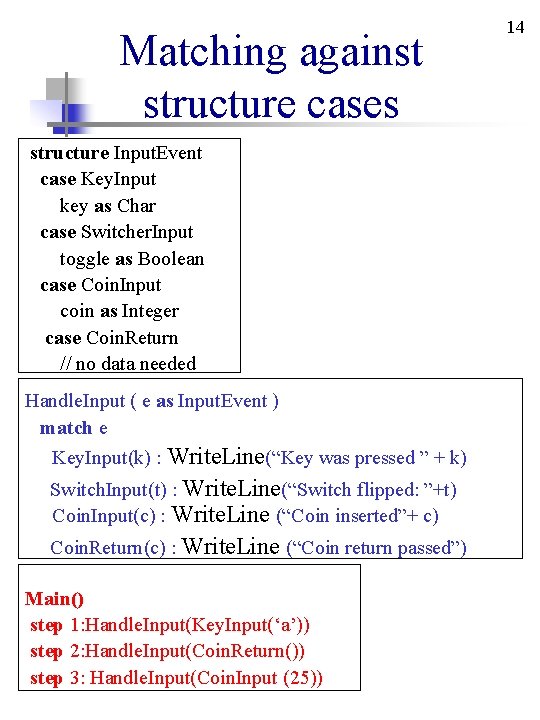
Matching against structure cases structure Input. Event case Key. Input key as Char case Switcher. Input toggle as Boolean case Coin. Input coin as Integer case Coin. Return // no data needed Handle. Input ( e as Input. Event ) match e Key. Input(k) : Write. Line(“Key was pressed ” + k) Switch. Input(t) : Write. Line(“Switch flipped: ”+t) Coin. Input(c) : Write. Line (“Coin inserted”+ c) Coin. Return(c) : Write. Line (“Coin return passed”) Main() step 1: Handle. Input(Key. Input(‘a’)) step 2: Handle. Input(Coin. Return()) step 3: Handle. Input(Coin. Input (25)) 14
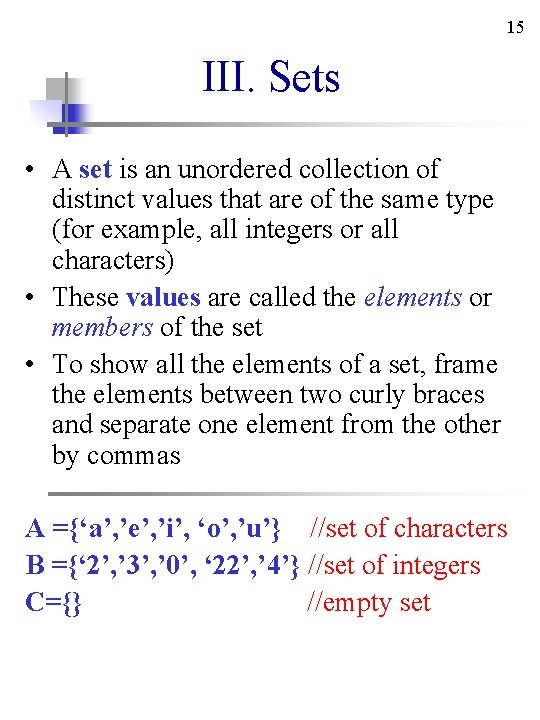
15 III. Sets • A set is an unordered collection of distinct values that are of the same type (for example, all integers or all characters) • These values are called the elements or members of the set • To show all the elements of a set, frame the elements between two curly braces and separate one element from the other by commas A ={‘a’, ’e’, ’i’, ‘o’, ’u’} //set of characters B ={‘ 2’, ’ 3’, ’ 0’, ‘ 22’, ’ 4’} //set of integers C={} //empty set
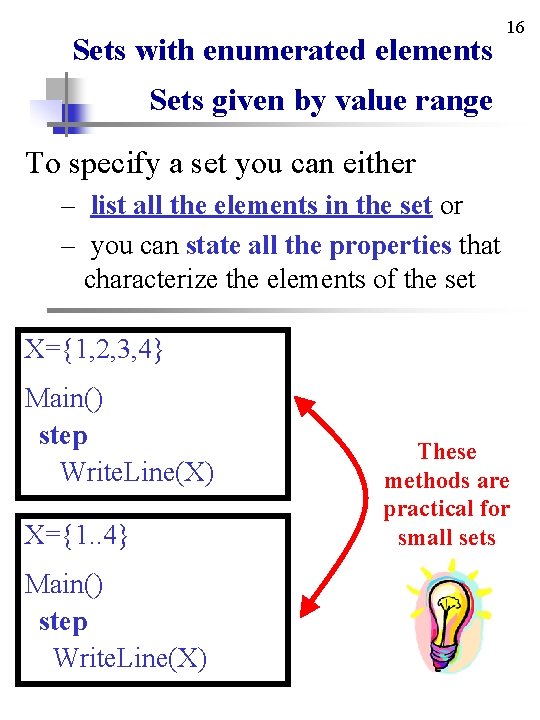
Sets with enumerated elements 16 Sets given by value range To specify a set you can either – list all the elements in the set or – you can state all the properties that characterize the elements of the set X={1, 2, 3, 4} Main() step Write. Line(X) X={1. . 4} Main() step Write. Line(X) These methods are practical for small sets
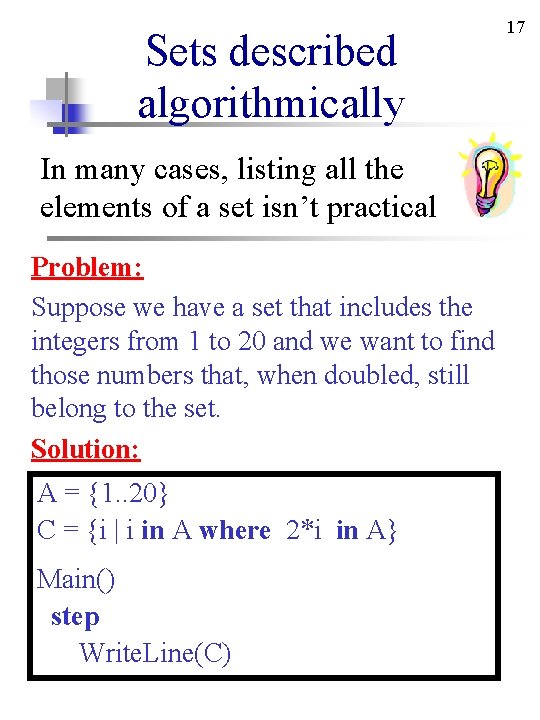
Sets described algorithmically In many cases, listing all the elements of a set isn’t practical Problem: Suppose we have a set that includes the integers from 1 to 20 and we want to find those numbers that, when doubled, still belong to the set. Solution: A = {1. . 20} C = {i | i in A where 2*i in A} Main() step Write. Line(C) 17
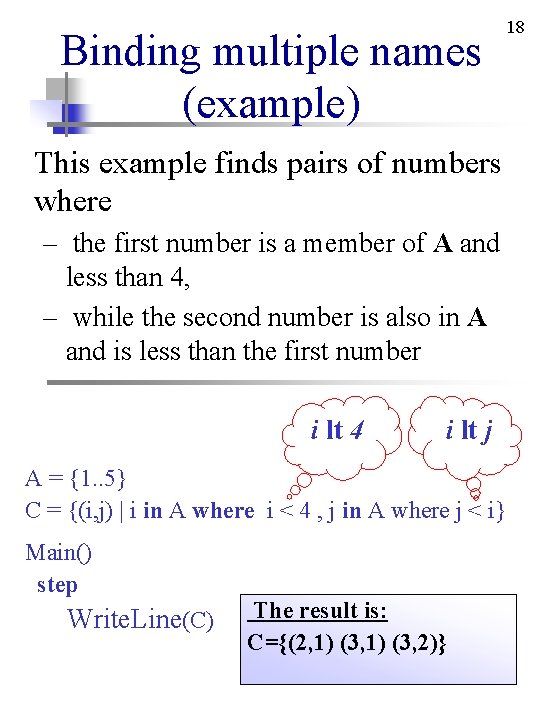
Binding multiple names (example) This example finds pairs of numbers where – the first number is a member of A and less than 4, – while the second number is also in A and is less than the first number i lt 4 i lt j A = {1. . 5} C = {(i, j) | i in A where i < 4 , j in A where j < i} Main() step Write. Line(C) The result is: C={(2, 1) (3, 2)} 18
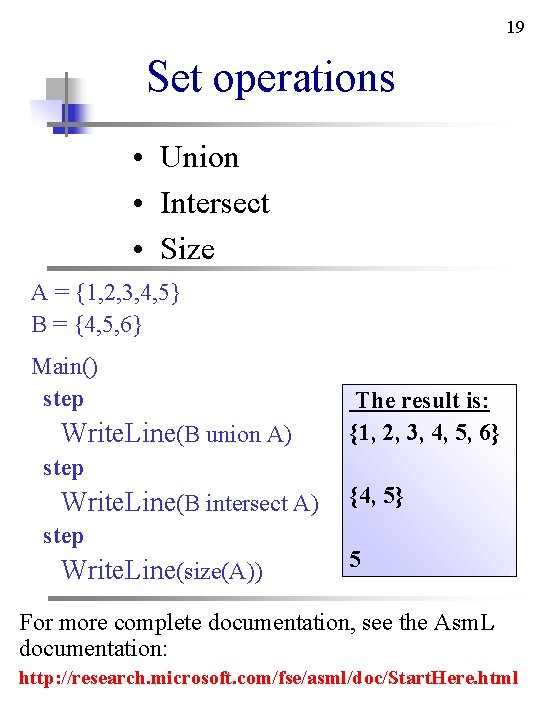
19 Set operations • Union • Intersect • Size A = {1, 2, 3, 4, 5} B = {4, 5, 6} Main() step Write. Line(B union A) The result is: {1, 2, 3, 4, 5, 6} step Write. Line(B intersect A) step Write. Line(size(A)) {4, 5} 5 For more complete documentation, see the Asm. L documentation: http: //research. microsoft. com/fse/asml/doc/Start. Here. html
Asml values
Arithmetic sequence sigma notation
Akü asml
Asml pas 5500 / 100d
Euv system install engineer
Rga meting
Structured analysis and structured design (sa/sd)
Types of interviews structured semi structured unstructured
Determinantes demonstrativos
Pre ap classes vs regular classes
Western values vs eastern values
Types of values in human values
Machiavellian personality
An individual's enduring tendency to feel
______ have only two possible values 0 and 1. *
Introduce yourself
Introducing family members in french
Introducing new market offerings ppt
Khdmdcm
Ma