1 Chapter 11 Templates Outline 11 1 11
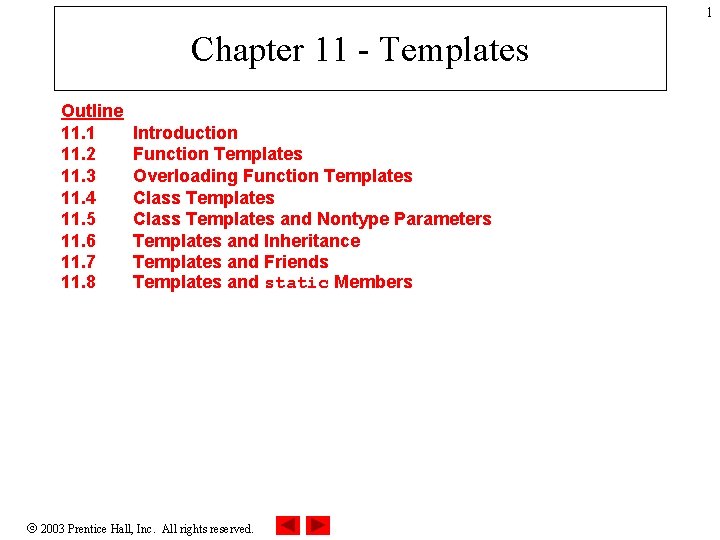
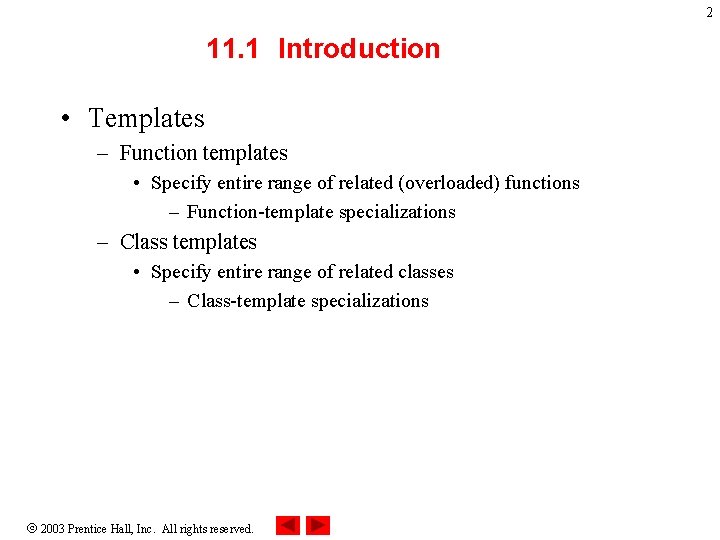
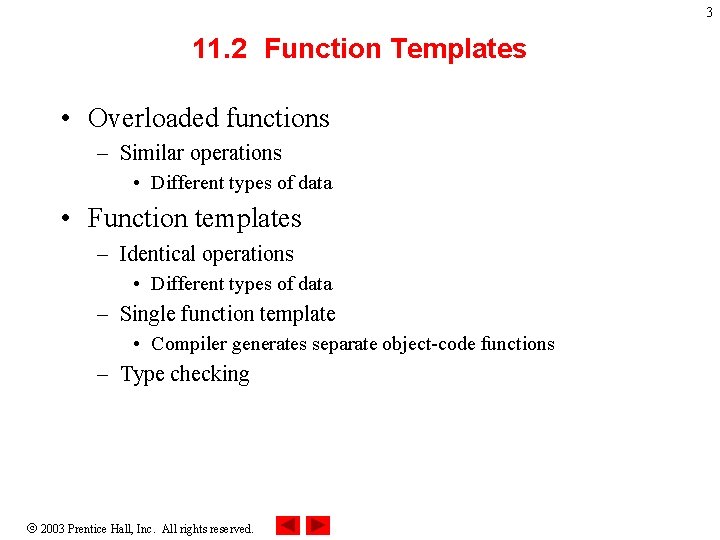
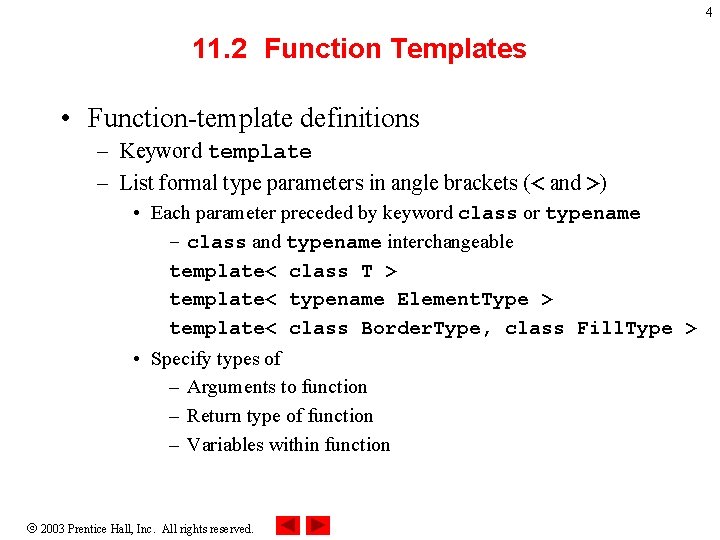
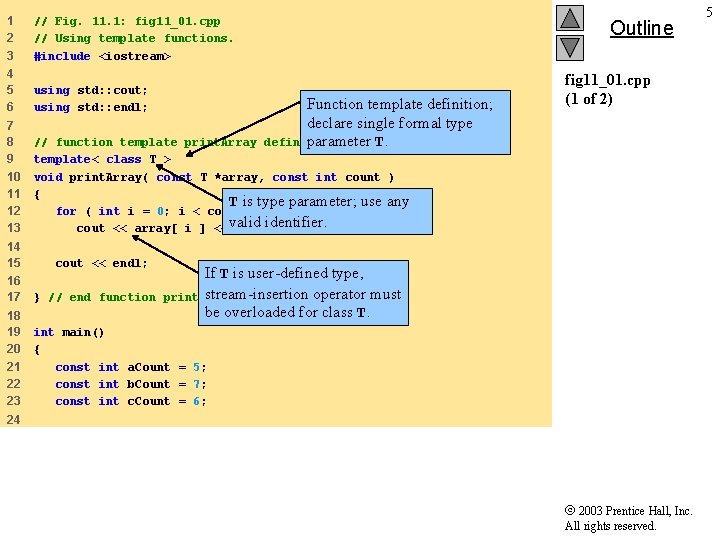
![25 26 27 int a[ a. Count ] = { 1, 2, 3, 4, 25 26 27 int a[ a. Count ] = { 1, 2, 3, 4,](https://slidetodoc.com/presentation_image/6259b293a27b62c923aea46adaab2e3c/image-6.jpg)
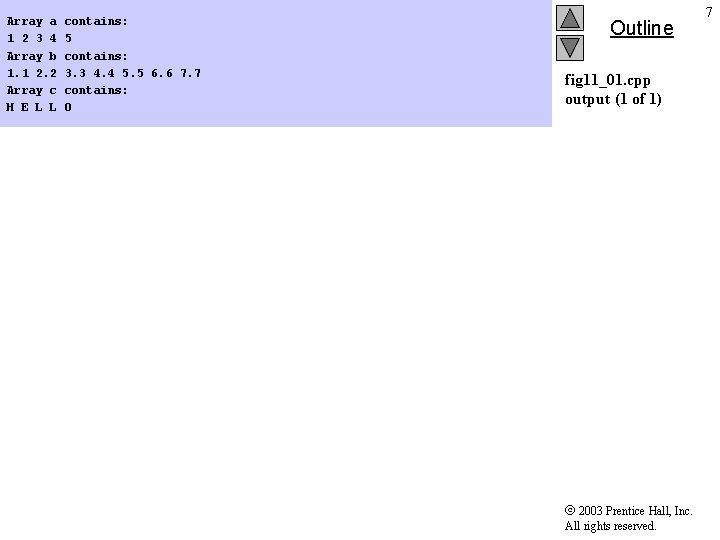
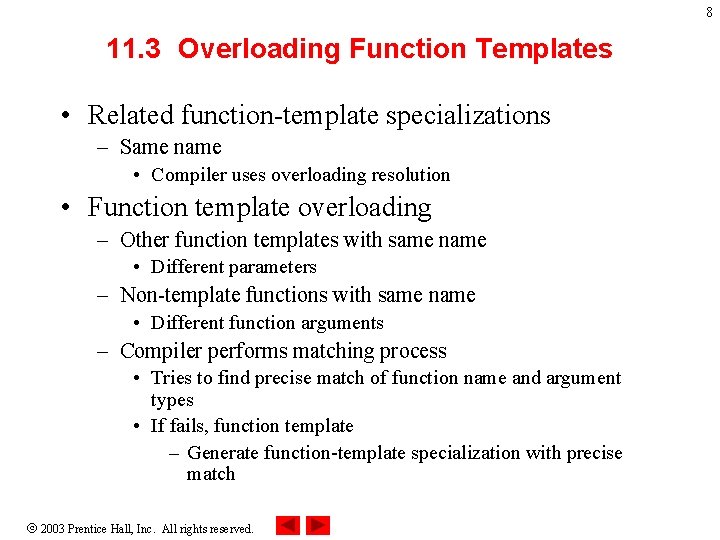
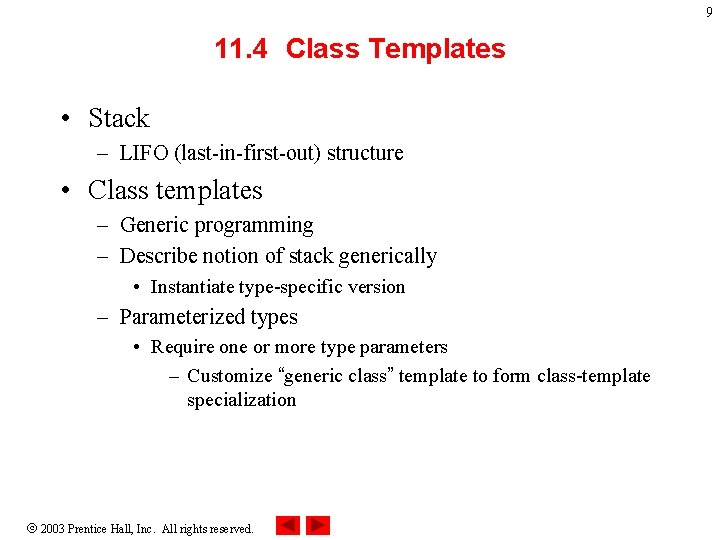
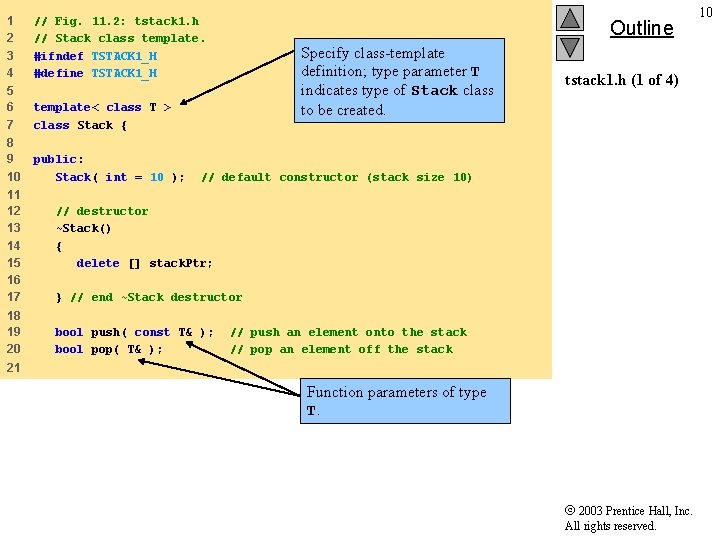
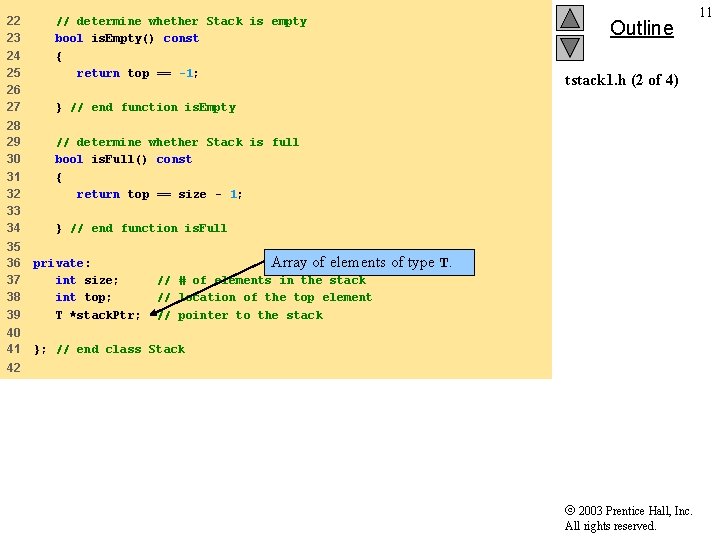
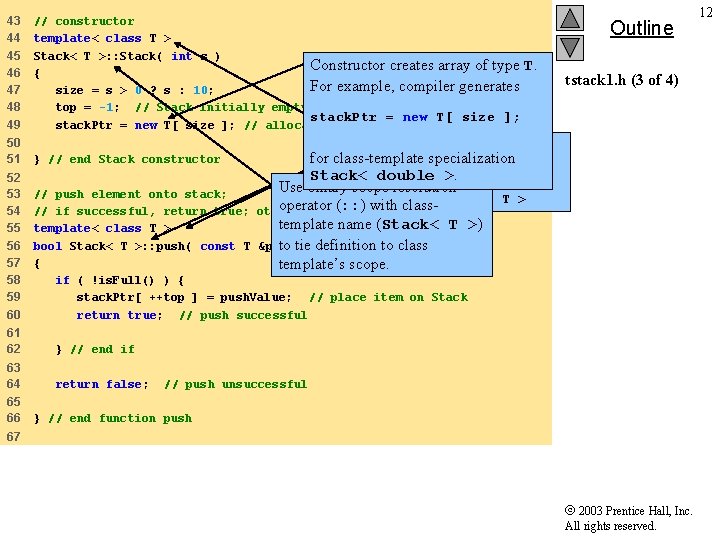
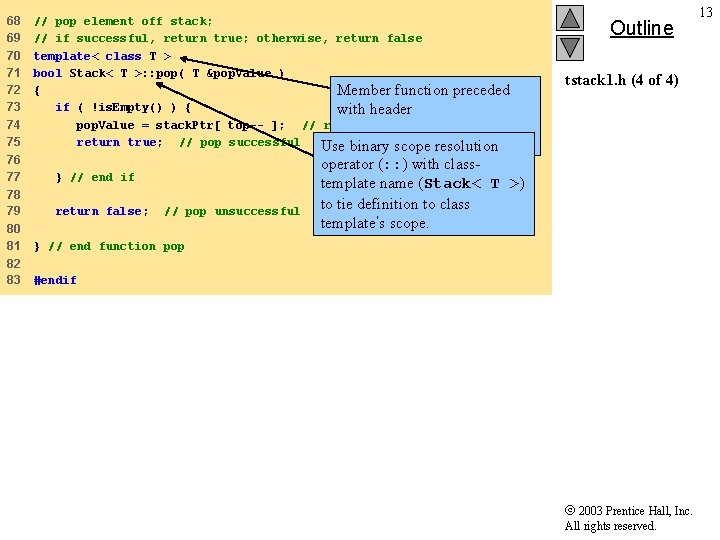
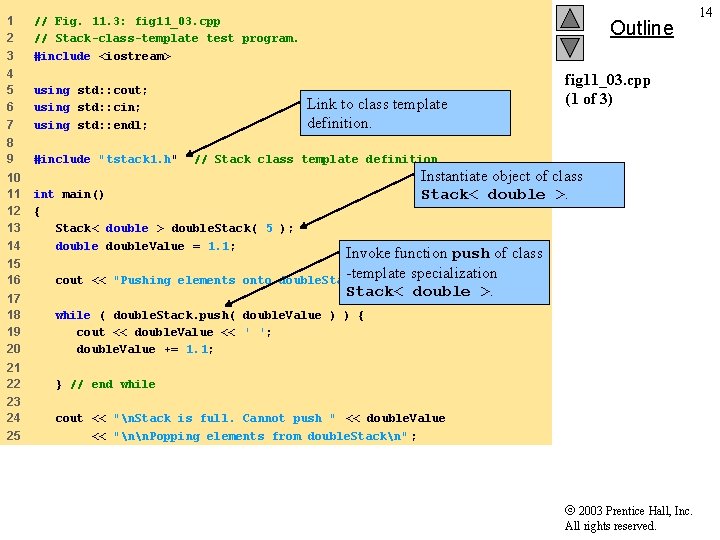
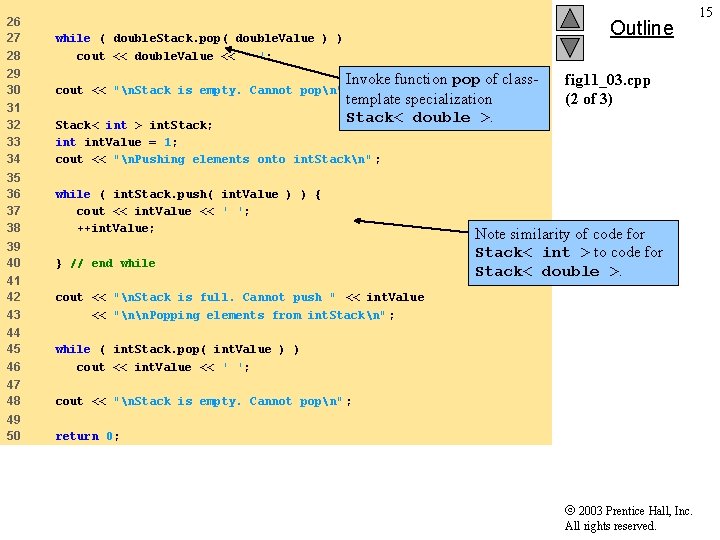
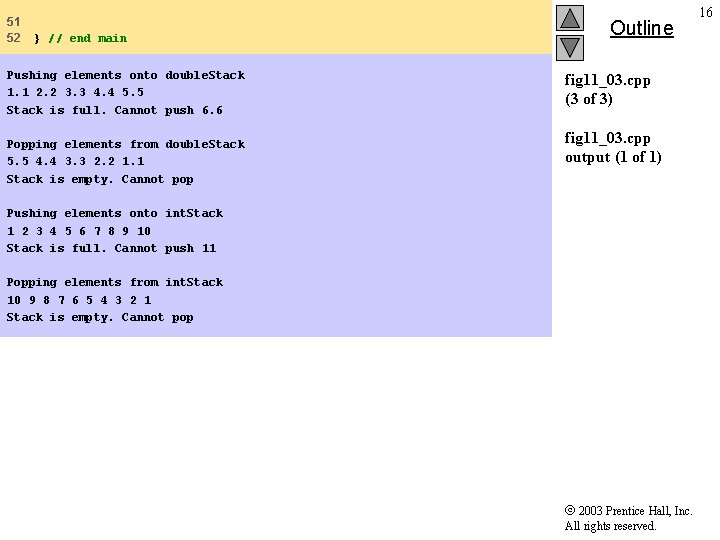
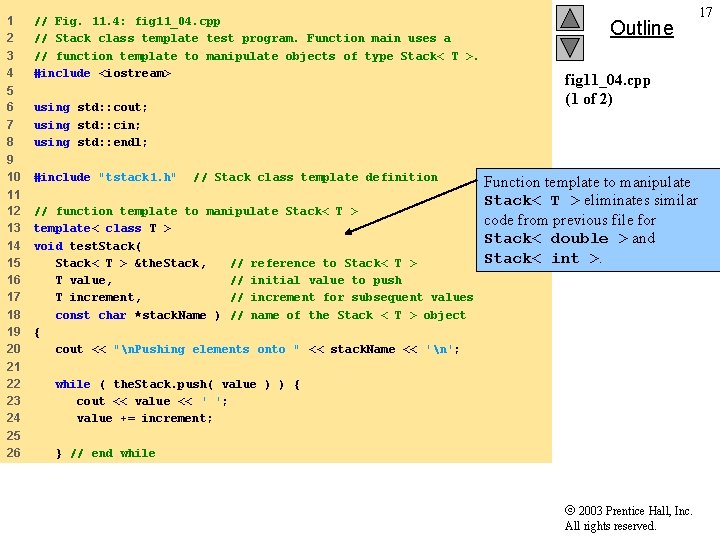
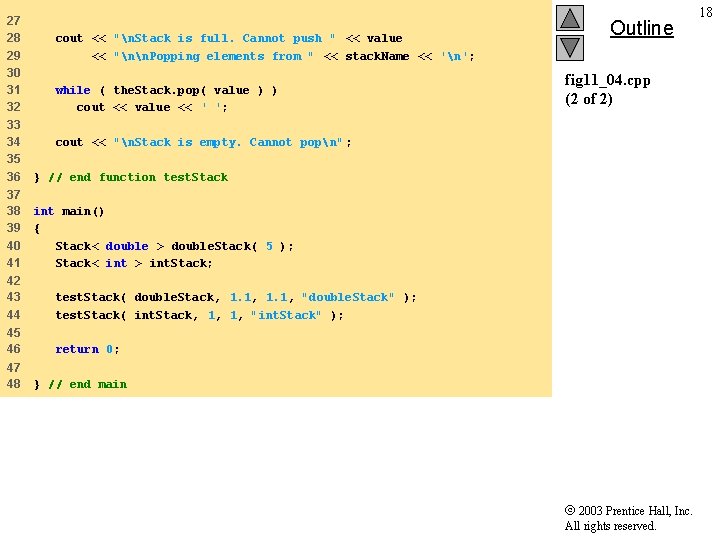
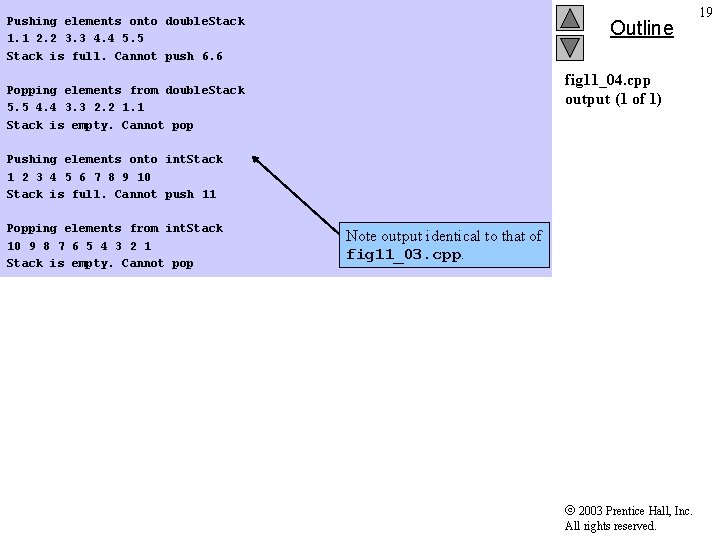
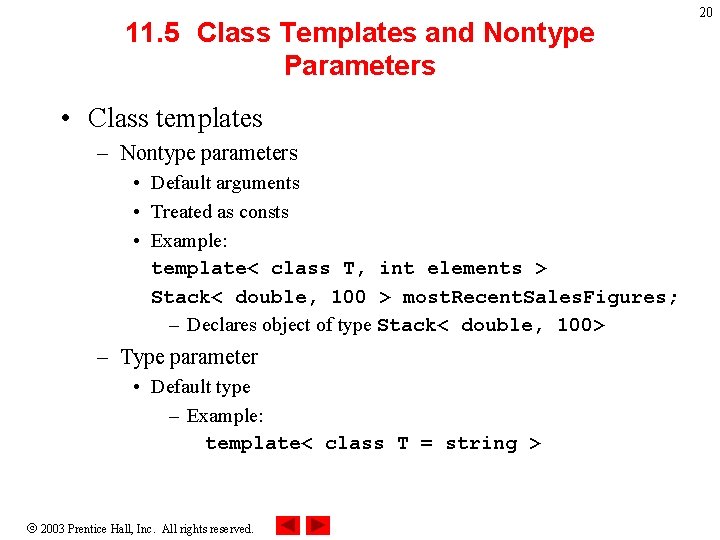
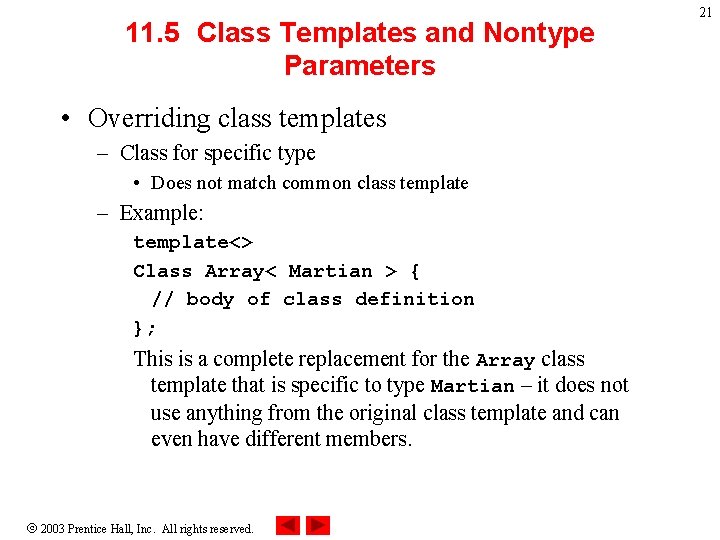
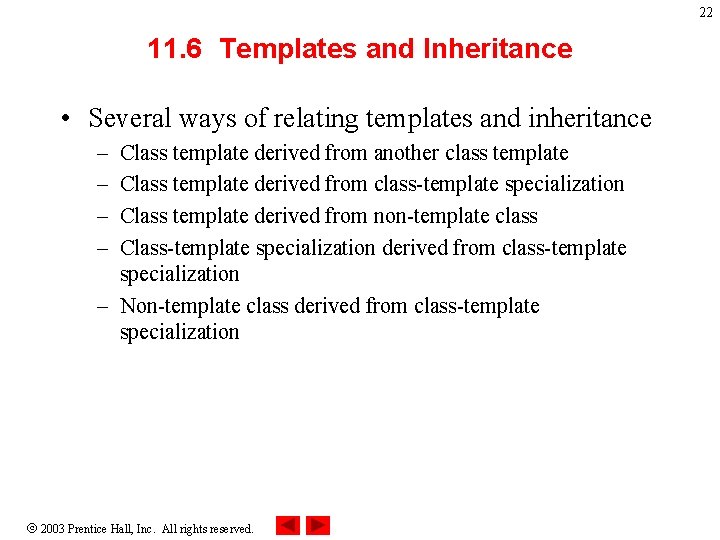
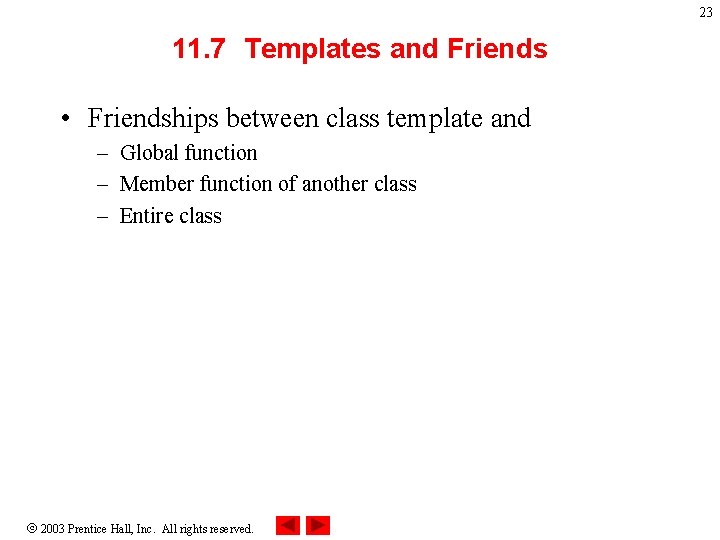
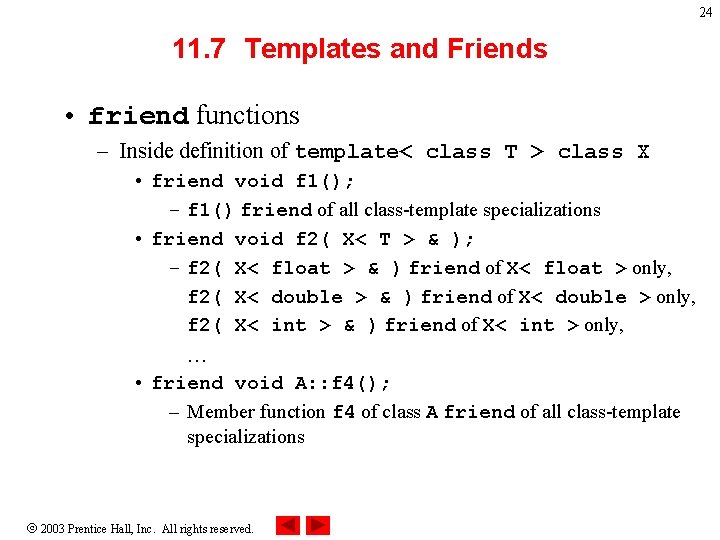
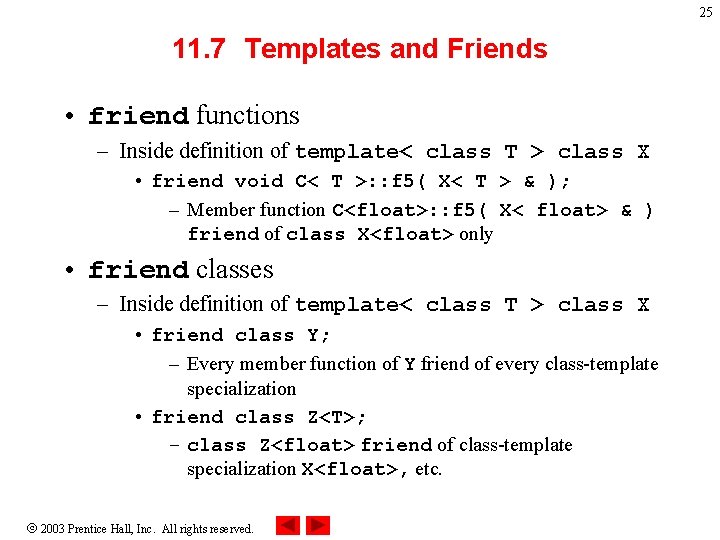
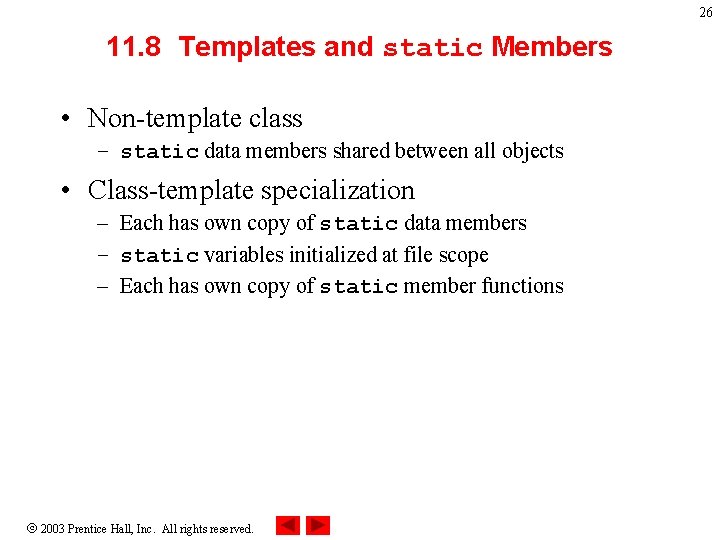
- Slides: 26
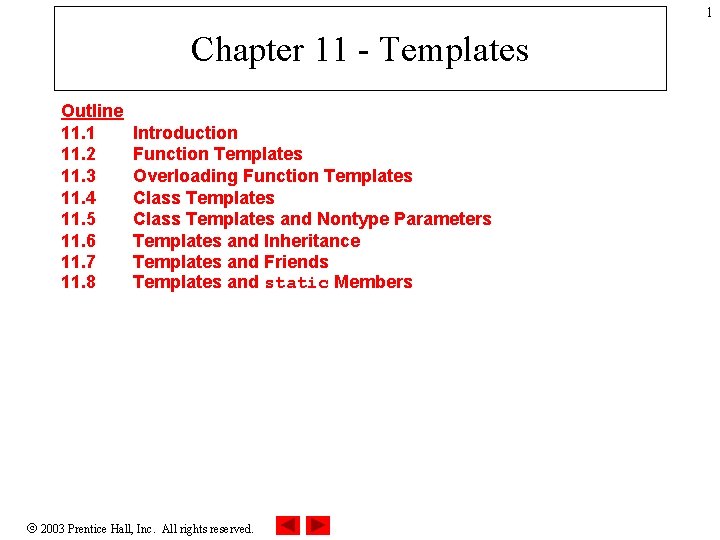
1 Chapter 11 - Templates Outline 11. 1 11. 2 11. 3 11. 4 11. 5 11. 6 11. 7 11. 8 Introduction Function Templates Overloading Function Templates Class Templates and Nontype Parameters Templates and Inheritance Templates and Friends Templates and static Members 2003 Prentice Hall, Inc. All rights reserved.
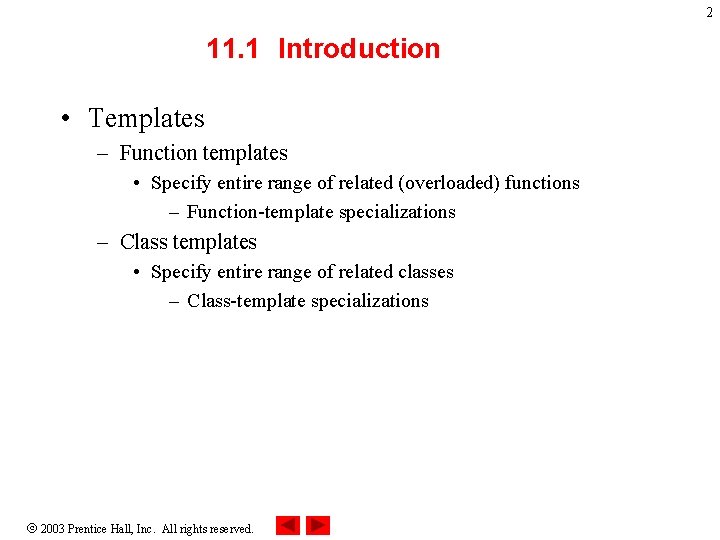
2 11. 1 Introduction • Templates – Function templates • Specify entire range of related (overloaded) functions – Function-template specializations – Class templates • Specify entire range of related classes – Class-template specializations 2003 Prentice Hall, Inc. All rights reserved.
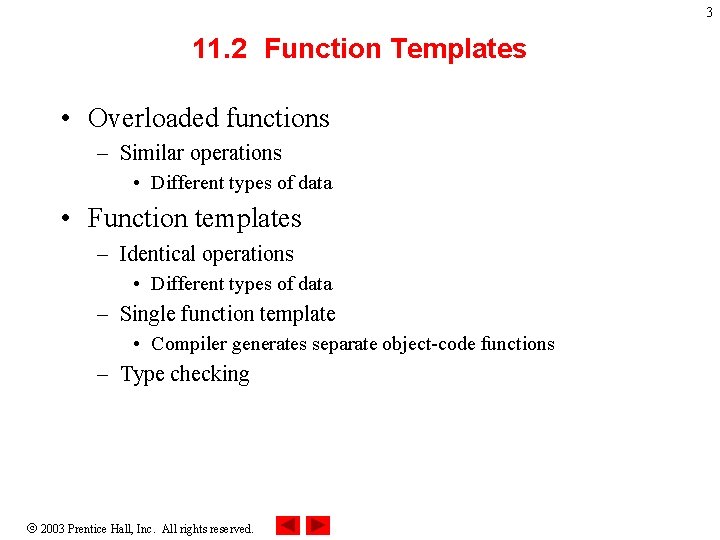
3 11. 2 Function Templates • Overloaded functions – Similar operations • Different types of data • Function templates – Identical operations • Different types of data – Single function template • Compiler generates separate object-code functions – Type checking 2003 Prentice Hall, Inc. All rights reserved.
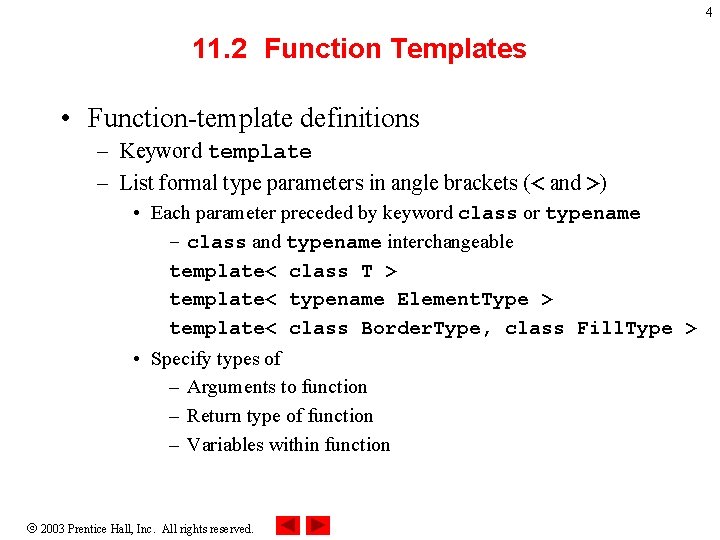
4 11. 2 Function Templates • Function-template definitions – Keyword template – List formal type parameters in angle brackets (< and >) • Each parameter preceded by keyword class or typename – class and typename interchangeable template< class T > template< typename Element. Type > template< class Border. Type, class Fill. Type > • Specify types of – Arguments to function – Return type of function – Variables within function 2003 Prentice Hall, Inc. All rights reserved.
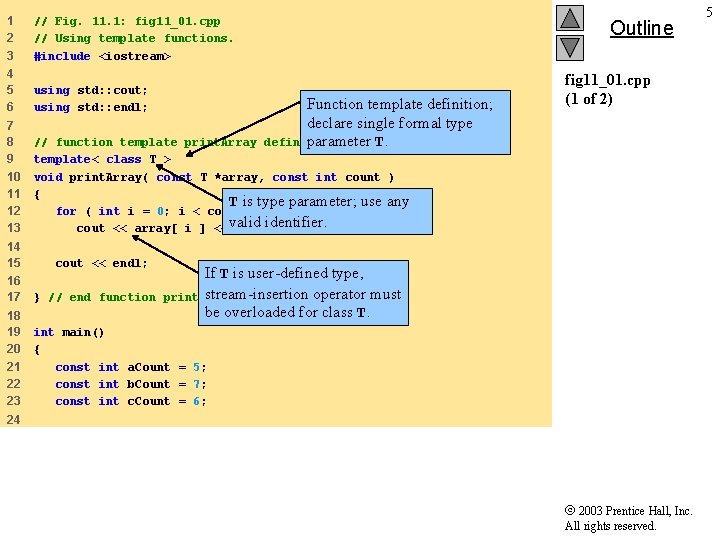
1 2 3 // Fig. 11. 1: fig 11_01. cpp // Using template functions. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 12 13 template< class T > void print. Array( const T *array, const int count ) { T is type parameter; use any for ( int i = 0; i < count; i++ ) valid cout << array[ i ] << " "; identifier. 14 15 cout << endl; 16 17 18 19 20 21 22 23 Function template definition; declare single formal type // function template print. Array definition parameter T. Outline fig 11_01. cpp (1 of 2) If T is user-defined type, stream-insertion operator must } // end function print. Array be overloaded for class T. int main() { const int a. Count = 5; const int b. Count = 7; const int c. Count = 6; 24 2003 Prentice Hall, Inc. All rights reserved. 5
![25 26 27 int a a Count 1 2 3 4 25 26 27 int a[ a. Count ] = { 1, 2, 3, 4,](https://slidetodoc.com/presentation_image/6259b293a27b62c923aea46adaab2e3c/image-6.jpg)
25 26 27 int a[ a. Count ] = { 1, 2, 3, 4, 5 }; double b[ b. Count ] = { 1. 1, 2. 2, 3. 3, 4. 4, 5. 5, 6. 6, 7. 7 }; char c[ c. Count ] = "HELLO"; // 6 th position for null 28 29 cout << "Array a contains: " << endl; 30 31 32 // call integer function-template specialization print. Array( a, a. Count ); 33 34 cout << "Array b contains: " << endl; 35 36 37 38 39 Outline fig 11_01. cpp (2 of 2) Compiler infers T is double; instantiates function-template specialization where T is Creates complete function-template specialization for printing // call double function-template specialization double. array of ints: print. Array( b, b. Count ); 43 44 Compiler infers T is char; void print. Array( const int *array, const int count ) cout << "Array c contains: " << endl; { instantiates function-template for ( int i = 0; i < count; i++ ) specialization where T is // call character function-template specialization cout << array[ i ] << " " print. Array( c, c. Count ); char. cout << endl; } // end function print. Array return 0; 45 46 } // end main 40 41 42 2003 Prentice Hall, Inc. All rights reserved. 6
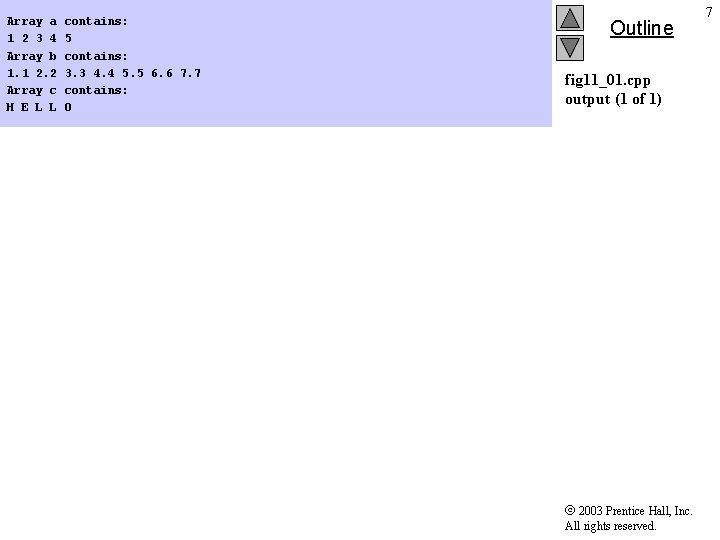
Array a contains: 1 2 3 4 5 Array b contains: 1. 1 2. 2 3. 3 4. 4 5. 5 6. 6 7. 7 Array c contains: H E L L O Outline fig 11_01. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 7
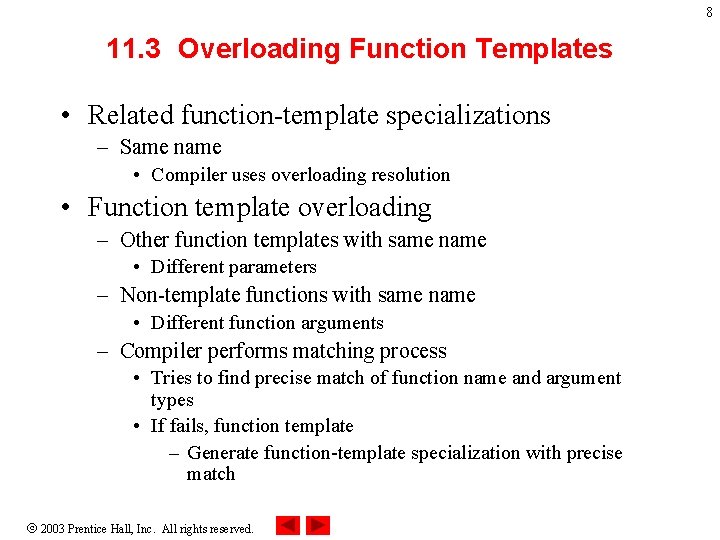
8 11. 3 Overloading Function Templates • Related function-template specializations – Same name • Compiler uses overloading resolution • Function template overloading – Other function templates with same name • Different parameters – Non-template functions with same name • Different function arguments – Compiler performs matching process • Tries to find precise match of function name and argument types • If fails, function template – Generate function-template specialization with precise match 2003 Prentice Hall, Inc. All rights reserved.
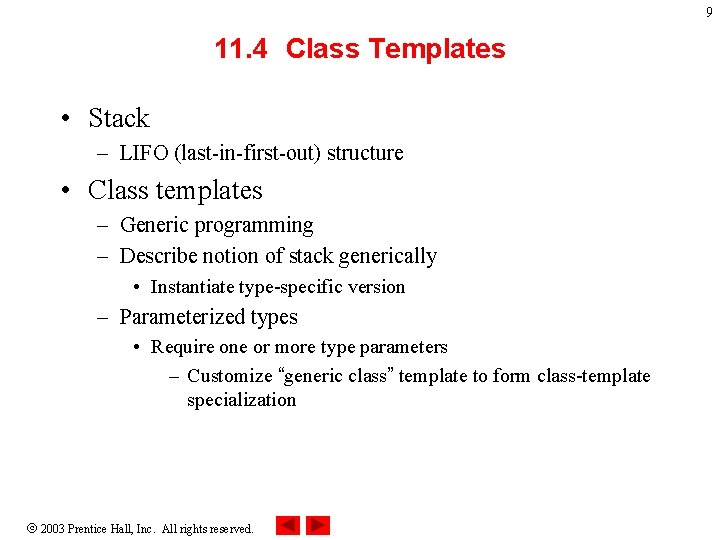
9 11. 4 Class Templates • Stack – LIFO (last-in-first-out) structure • Class templates – Generic programming – Describe notion of stack generically • Instantiate type-specific version – Parameterized types • Require one or more type parameters – Customize “generic class” template to form class-template specialization 2003 Prentice Hall, Inc. All rights reserved.
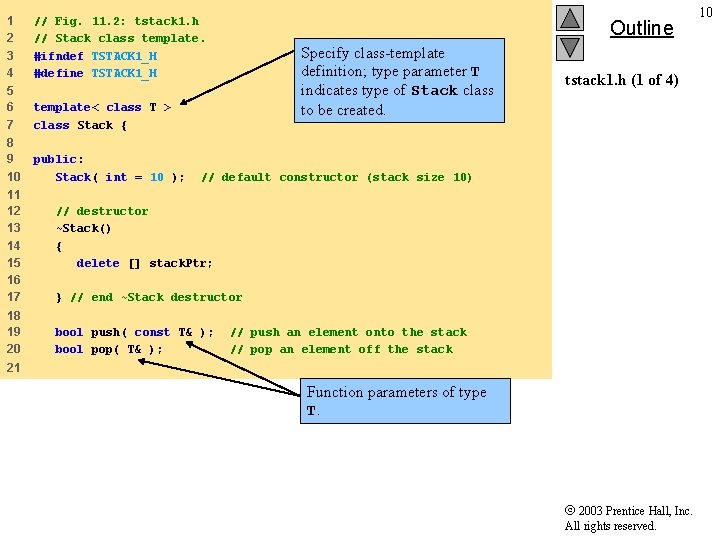
1 2 3 4 // Fig. 11. 2: tstack 1. h // Stack class template. #ifndef TSTACK 1_H #define TSTACK 1_H 5 6 7 template< class T > class Stack { 8 9 10 public: Stack( int = 10 ); // default constructor (stack size 10) 11 12 13 14 15 16 17 // destructor ~Stack() { delete [] stack. Ptr; } // end ~Stack destructor 18 19 20 bool push( const T& ); // push an element onto the stack bool pop( T& ); // pop an element off the stack Outline Specify class-template definition; type parameter T indicates type of Stack class to be created. tstack 1. h (1 of 4) 21 Function parameters of type T. 2003 Prentice Hall, Inc. All rights reserved. 10
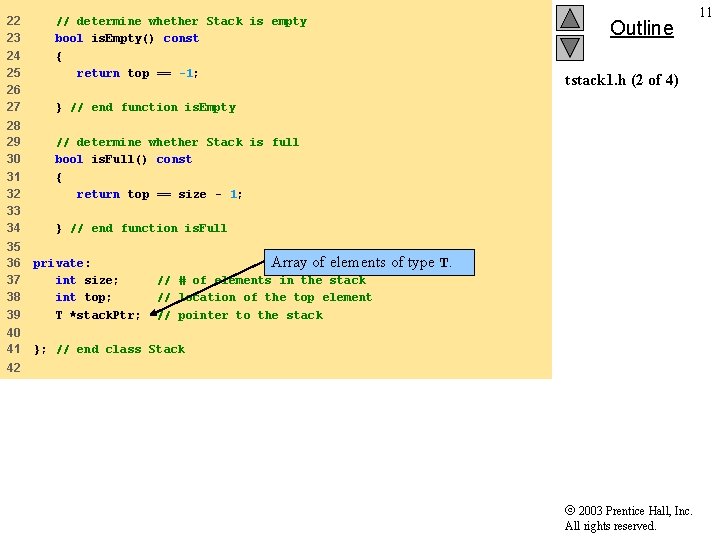
22 23 24 25 26 27 // determine whether Stack is empty bool is. Empty() const { return top == -1; } // end function is. Empty 28 29 30 31 32 33 34 // determine whether Stack is full bool is. Full() const { return top == size - 1; } // end function is. Full 35 36 37 38 39 private: Array of elements int size; // # of elements in the stack int top; // location of the top element T *stack. Ptr; // pointer to the stack 40 41 }; // end class Stack Outline tstack 1. h (2 of 4) of type T. 42 2003 Prentice Hall, Inc. All rights reserved. 11
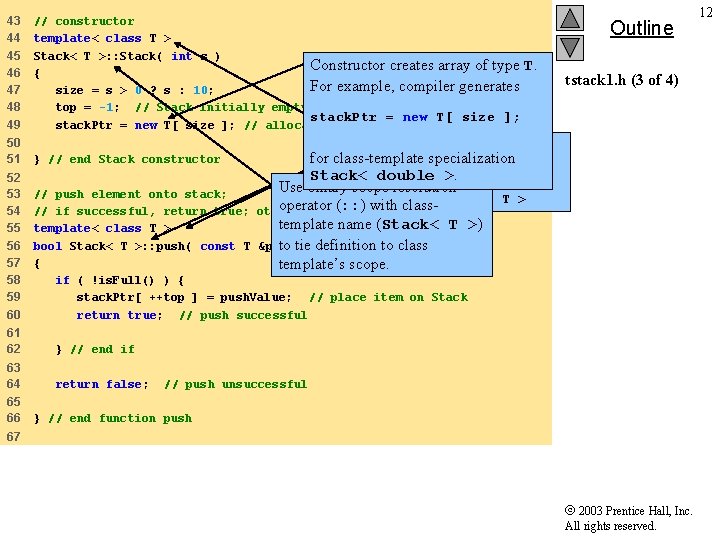
43 44 45 46 47 48 49 // constructor template< class T > Stack< T >: : Stack( int s ) Constructor creates array of type { For example, compiler generates size = s > 0 ? s : 10; top = -1; // Stack initially empty stack. Ptr = new T[ size ]; // allocate memory for elements 50 51 } // end Stack constructor 52 53 54 55 56 57 58 59 60 if ( !is. Full() ) { stack. Ptr[ ++top ] = push. Value; // place item on Stack return true; // push successful Outline T. tstack 1. h (3 of 4) Member functions preceded for class-template specialization with header Stack< double >. Use binary scope resolution // push element onto stack; operator (: : ) template< class T > with class// if successful, return true; otherwise, return false template name (Stack< T >) template< class T > bool Stack< T >: : push( const T &push. Value ) to tie definition to class { template’s scope. 61 62 } // end if 63 64 return false; // push unsuccessful 65 66 } // end function push 67 2003 Prentice Hall, Inc. All rights reserved. 12
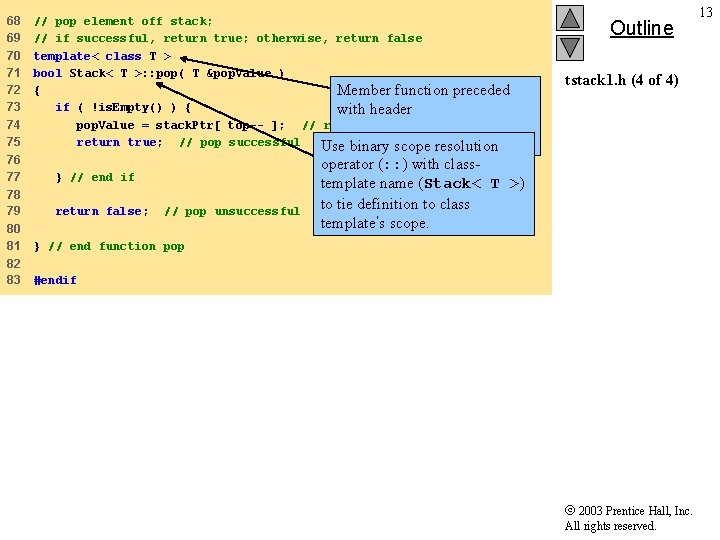
68 69 70 71 72 73 74 75 76 77 // pop element off stack; // if successful, return true; otherwise, return false template< class T > bool Stack< T >: : pop( T &pop. Value ) { Member function preceded if ( !is. Empty() ) { with header pop. Value = stack. Ptr[ top-- ]; // remove item from Stack return true; // pop successful template< class T > Use binary scope resolution } // end if 78 79 return false; // pop unsuccessful 80 81 } // end function pop 82 83 #endif Outline tstack 1. h (4 of 4) operator (: : ) with classtemplate name (Stack< T >) to tie definition to class template’s scope. 2003 Prentice Hall, Inc. All rights reserved. 13
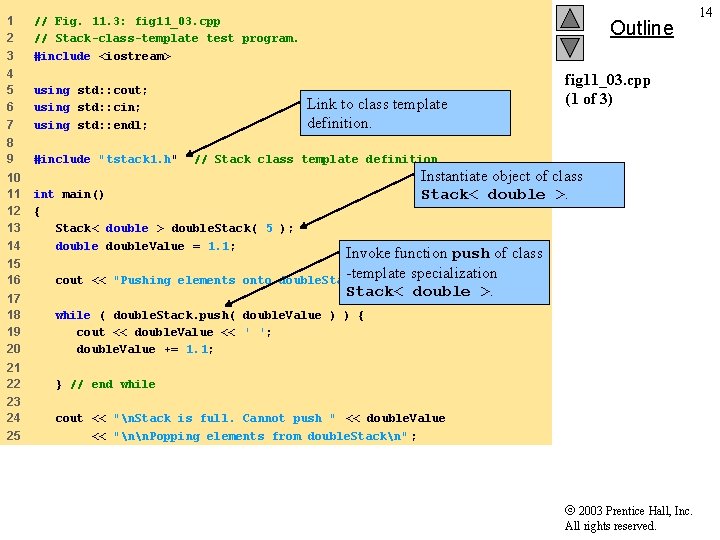
1 2 3 // Fig. 11. 3: fig 11_03. cpp // Stack-class-template test program. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 #include "tstack 1. h" // Stack class template definition 10 11 12 13 14 15 16 17 18 19 20 Outline Link to class template definition. int main() { Stack< double > double. Stack( 5 ); double. Value = 1. 1; fig 11_03. cpp (1 of 3) Instantiate object of class Stack< double >. Invoke function push of class -template specialization cout << "Pushing elements onto double. Stackn" ; Stack< double >. while ( double. Stack. push( double. Value ) ) { cout << double. Value << ' '; double. Value += 1. 1; 21 22 } // end while 23 24 25 cout << "n. Stack is full. Cannot push " << double. Value << "nn. Popping elements from double. Stackn" ; 2003 Prentice Hall, Inc. All rights reserved. 14
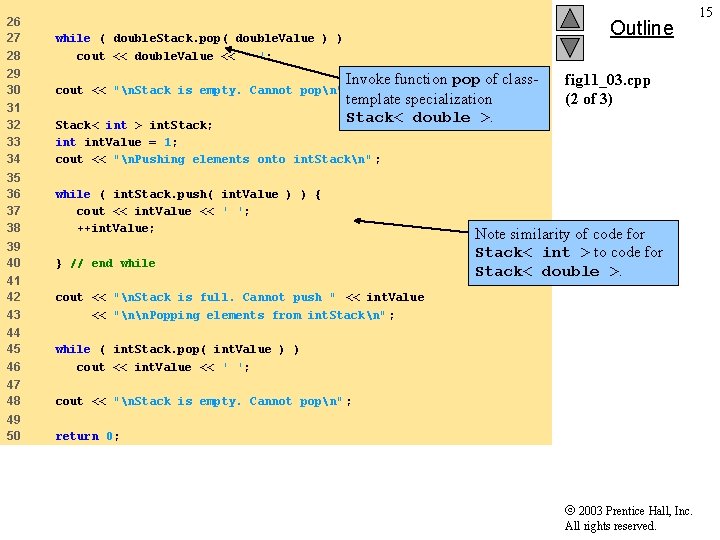
26 27 28 while ( double. Stack. pop( double. Value ) ) cout << double. Value << ' '; 29 30 cout << "n. Stack is empty. Cannot popn" ; 31 32 33 34 Stack< int > int. Stack; int. Value = 1; cout << "n. Pushing elements onto int. Stackn" ; 35 36 37 38 while ( int. Stack. push( int. Value ) ) { cout << int. Value << ' '; ++int. Value; 39 40 } // end while 41 42 43 cout << "n. Stack is full. Cannot push " << int. Value << "nn. Popping elements from int. Stackn" ; 44 45 46 while ( int. Stack. pop( int. Value ) ) cout << int. Value << ' '; 47 48 cout << "n. Stack is empty. Cannot popn" ; 49 50 return 0; Outline Invoke function pop of classtemplate specialization Stack< double >. fig 11_03. cpp (2 of 3) Note similarity of code for Stack< int > to code for Stack< double >. 2003 Prentice Hall, Inc. All rights reserved. 15
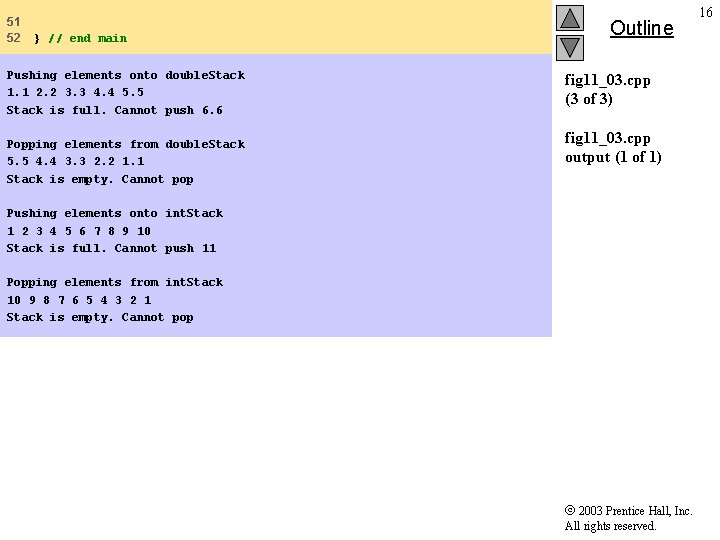
51 52 } // end main Pushing elements onto double. Stack 1. 1 2. 2 3. 3 4. 4 5. 5 Stack is full. Cannot push 6. 6 Popping elements from double. Stack 5. 5 4. 4 3. 3 2. 2 1. 1 Stack is empty. Cannot pop Outline fig 11_03. cpp (3 of 3) fig 11_03. cpp output (1 of 1) Pushing elements onto int. Stack 1 2 3 4 5 6 7 8 9 10 Stack is full. Cannot push 11 Popping elements from int. Stack 10 9 8 7 6 5 4 3 2 1 Stack is empty. Cannot pop 2003 Prentice Hall, Inc. All rights reserved. 16
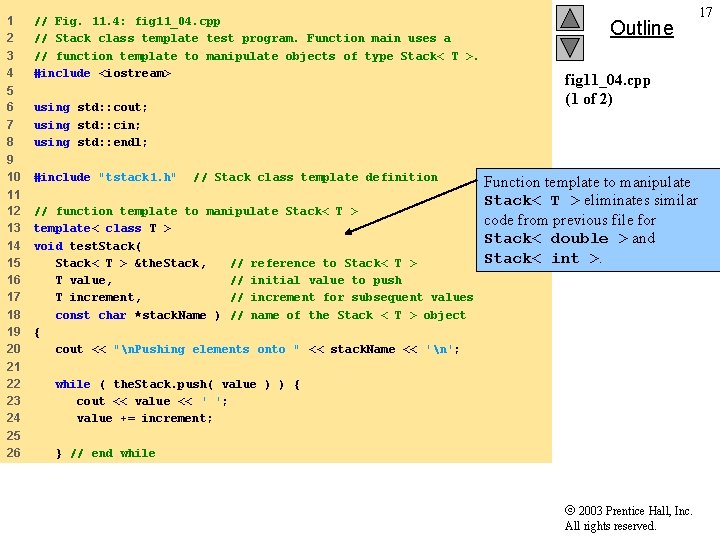
1 2 3 4 // Fig. 11. 4: fig 11_04. cpp // Stack class template test program. Function main uses a // function template to manipulate objects of type Stack< T >. #include <iostream> 5 6 7 8 using std: : cout; using std: : cin; using std: : endl; 9 10 #include "tstack 1. h" // Stack class template definition 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 // function template to manipulate Stack< T > template< class T > void test. Stack( Stack< T > &the. Stack, // reference to Stack< T > T value, // initial value to push T increment, // increment for subsequent values const char *stack. Name ) // name of the Stack < T > object { cout << "n. Pushing elements onto " << stack. Name << 'n'; while ( the. Stack. push( value ) ) { cout << value << ' '; value += increment; } // end while Outline fig 11_04. cpp (1 of 2) Function template to manipulate Stack< T > eliminates similar code from previous file for Stack< double > and Stack< int >. 2003 Prentice Hall, Inc. All rights reserved. 17
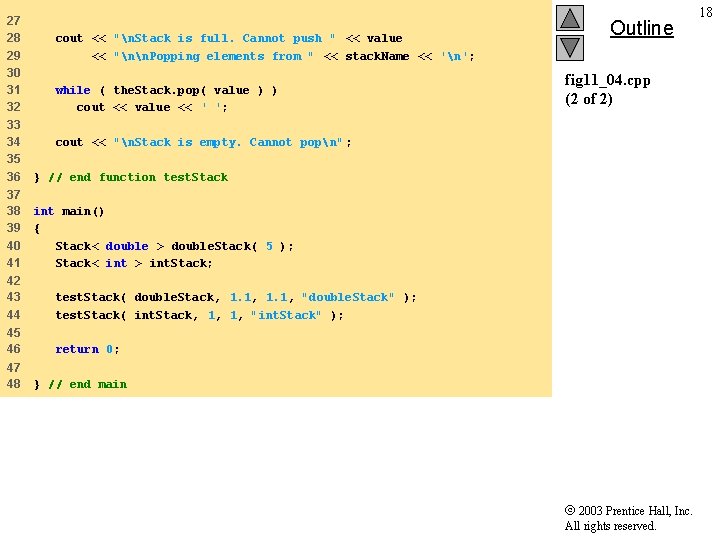
27 28 29 30 31 32 33 34 35 36 cout << "n. Stack is full. Cannot push " << value << "nn. Popping elements from " << stack. Name << 'n'; while ( the. Stack. pop( value ) ) cout << value << ' '; cout << "n. Stack is empty. Cannot popn" ; } // end function test. Stack 37 38 39 40 41 int main() { Stack< double > double. Stack( 5 ); Stack< int > int. Stack; 42 43 44 test. Stack( double. Stack, 1. 1, "double. Stack" ); test. Stack( int. Stack, 1, 1, "int. Stack" ); 45 46 return 0; 47 48 } // end main Outline fig 11_04. cpp (2 of 2) 2003 Prentice Hall, Inc. All rights reserved. 18
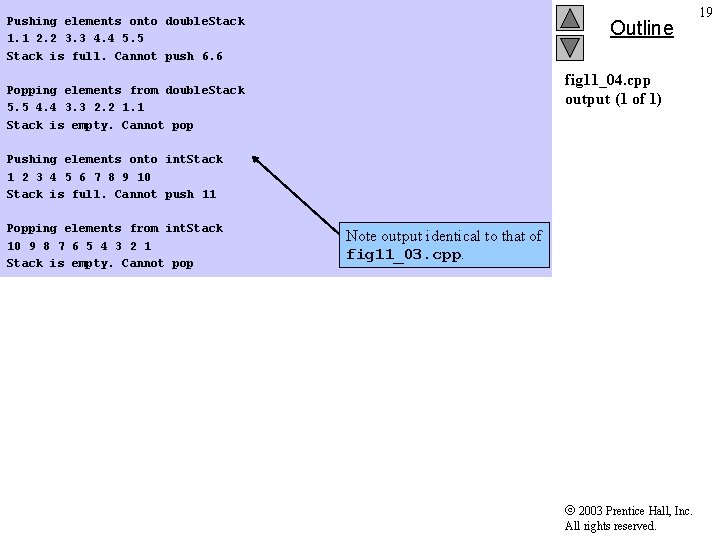
Pushing elements onto double. Stack 1. 1 2. 2 3. 3 4. 4 5. 5 Stack is full. Cannot push 6. 6 Outline Popping elements from double. Stack 5. 5 4. 4 3. 3 2. 2 1. 1 Stack is empty. Cannot pop fig 11_04. cpp output (1 of 1) Pushing elements onto int. Stack 1 2 3 4 5 6 7 8 9 10 Stack is full. Cannot push 11 Popping elements from int. Stack 10 9 8 7 6 5 4 3 2 1 Stack is empty. Cannot pop Note output identical to that of fig 11_03. cpp. 2003 Prentice Hall, Inc. All rights reserved. 19
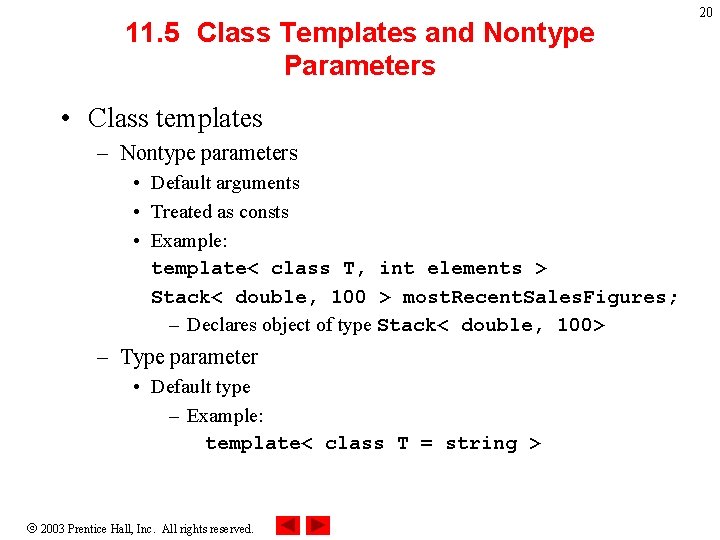
11. 5 Class Templates and Nontype Parameters • Class templates – Nontype parameters • Default arguments • Treated as consts • Example: template< class T, int elements > Stack< double, 100 > most. Recent. Sales. Figures; – Declares object of type Stack< double, 100> – Type parameter • Default type – Example: template< class T = string > 2003 Prentice Hall, Inc. All rights reserved. 20
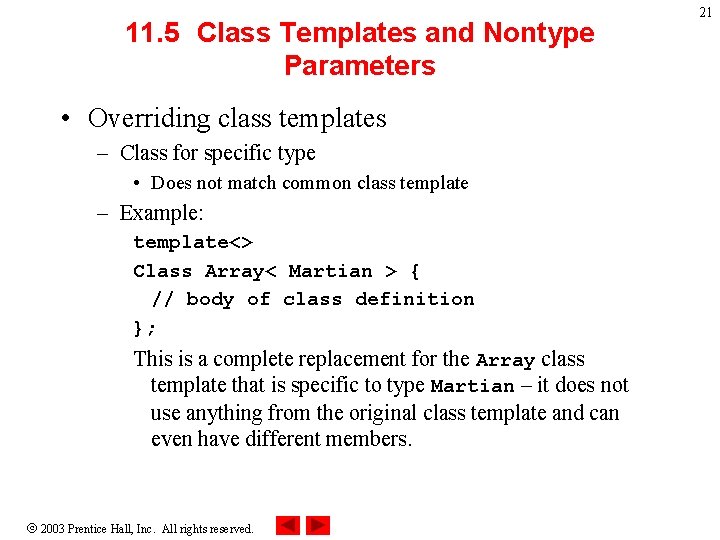
11. 5 Class Templates and Nontype Parameters • Overriding class templates – Class for specific type • Does not match common class template – Example: template<> Class Array< Martian > { // body of class definition }; This is a complete replacement for the Array class template that is specific to type Martian – it does not use anything from the original class template and can even have different members. 2003 Prentice Hall, Inc. All rights reserved. 21
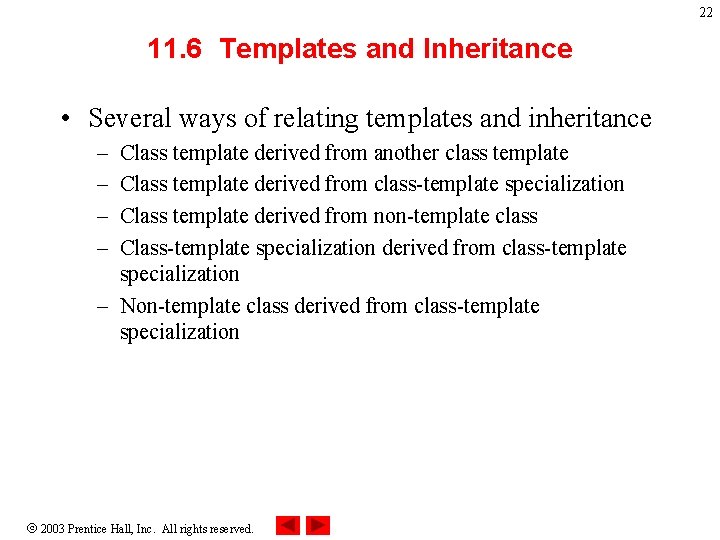
22 11. 6 Templates and Inheritance • Several ways of relating templates and inheritance – – Class template derived from another class template Class template derived from class-template specialization Class template derived from non-template class Class-template specialization derived from class-template specialization – Non-template class derived from class-template specialization 2003 Prentice Hall, Inc. All rights reserved.
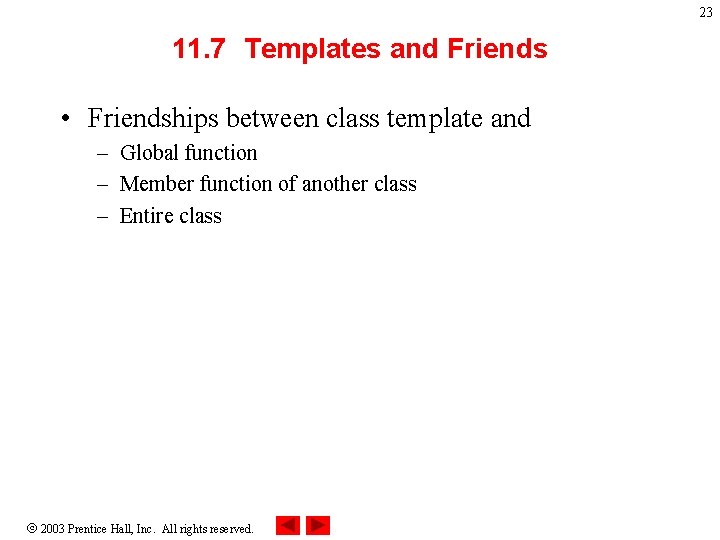
23 11. 7 Templates and Friends • Friendships between class template and – Global function – Member function of another class – Entire class 2003 Prentice Hall, Inc. All rights reserved.
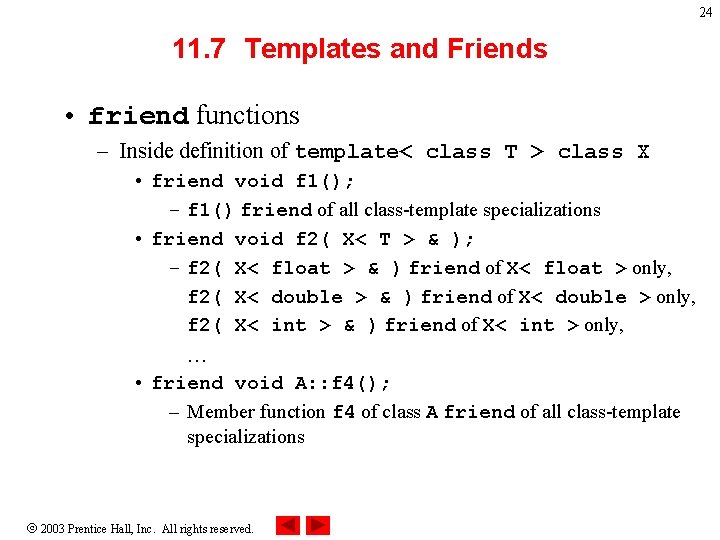
24 11. 7 Templates and Friends • friend functions – Inside definition of template< class T > class X • friend void f 1(); – f 1() friend of all class-template specializations • friend void f 2( X< T > & ); – f 2( X< float > & ) friend of X< float > only, f 2( X< double > & ) friend of X< double > only, f 2( X< int > & ) friend of X< int > only, … • friend void A: : f 4(); – Member function f 4 of class A friend of all class-template specializations 2003 Prentice Hall, Inc. All rights reserved.
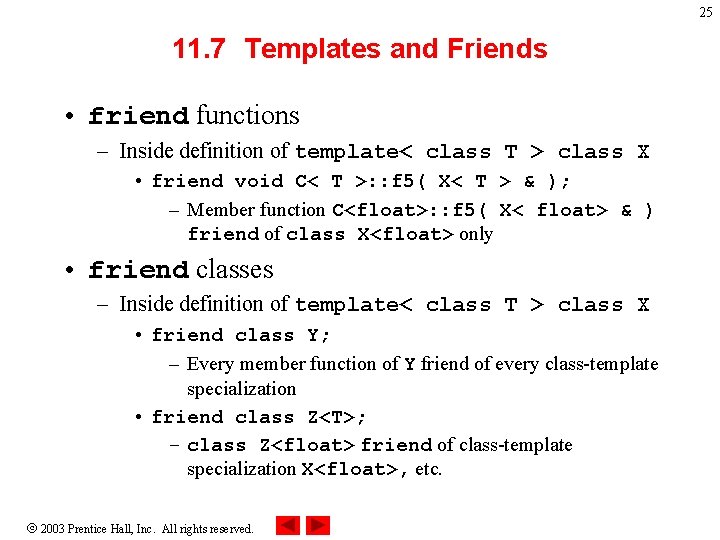
25 11. 7 Templates and Friends • friend functions – Inside definition of template< class T > class X • friend void C< T >: : f 5( X< T > & ); – Member function C<float>: : f 5( X< float> & ) friend of class X<float> only • friend classes – Inside definition of template< class T > class X • friend class Y; – Every member function of Y friend of every class-template specialization • friend class Z<T>; – class Z<float> friend of class-template specialization X<float>, etc. 2003 Prentice Hall, Inc. All rights reserved.
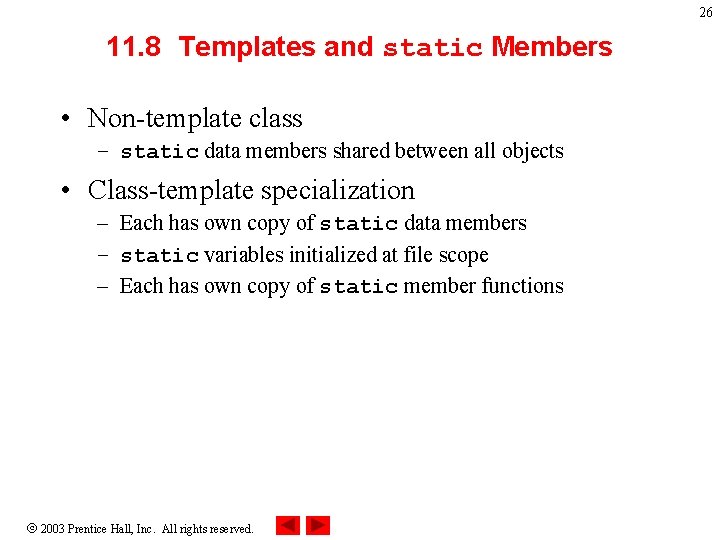
26 11. 8 Templates and static Members • Non-template class – static data members shared between all objects • Class-template specialization – Each has own copy of static data members – static variables initialized at file scope – Each has own copy of static member functions 2003 Prentice Hall, Inc. All rights reserved.
Quotation sandwiches
Chapter 8 templates
Rotary powerpoint template
What is meta commentary
Examples of metacommentary
Metacommentary definition
Templates in c++
Scentsy flyer templates
Dental scheduling template
Qbr presentation template
Ipsc stage design templates
Marketo landing page templates
Project kpi template
Templates in c++
Sejarah pathfinder ppt
Dreamweaver templates tutorials
Powerpoim
All ppt.com
Allppt.com _ free powerpoint templates, diagrams and charts
Allppt.cpm
Ppt template free islamic
Qapi meeting minutes template
Charticulator templates
These are mental templates by which we organize our worlds.
Change management heat map template
Naysayer template
Cms xlc templates