Text File IO Reading Data From and Writing
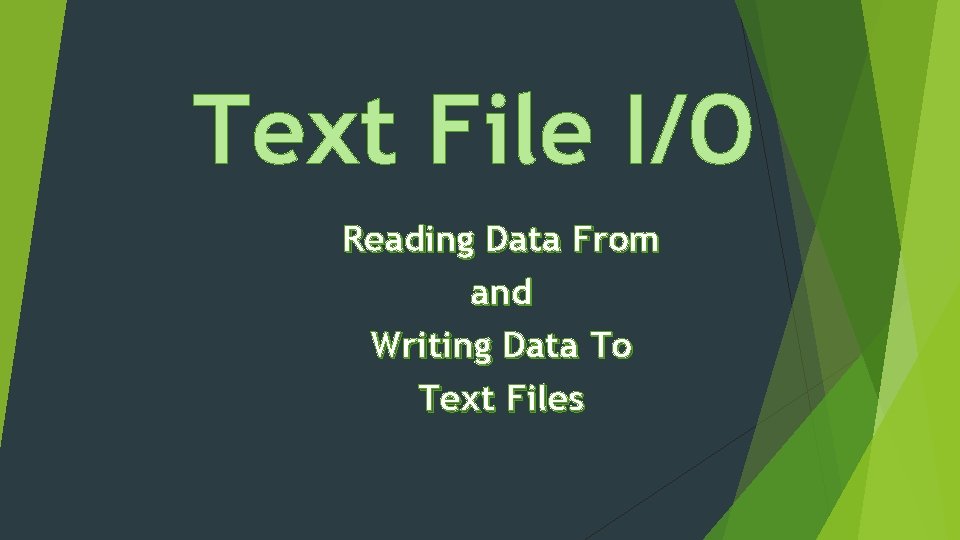
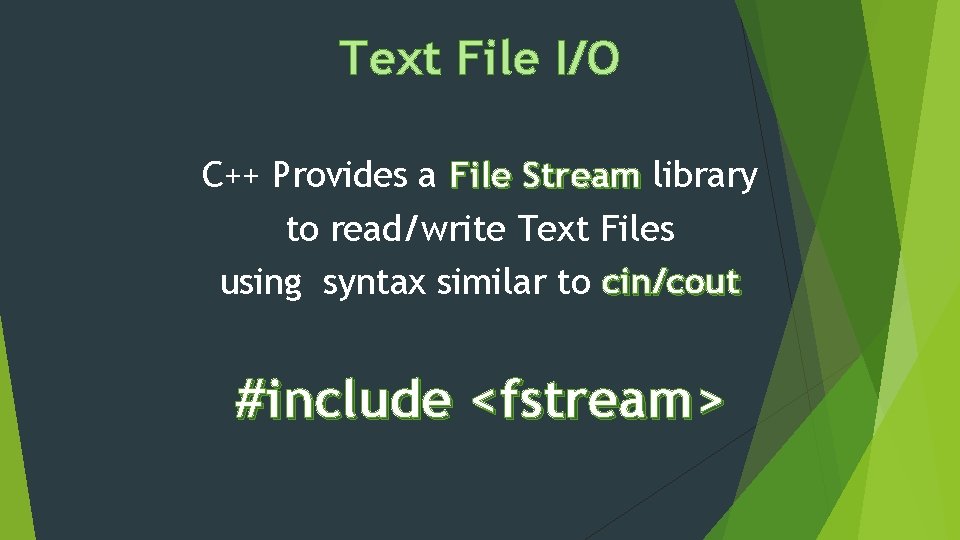
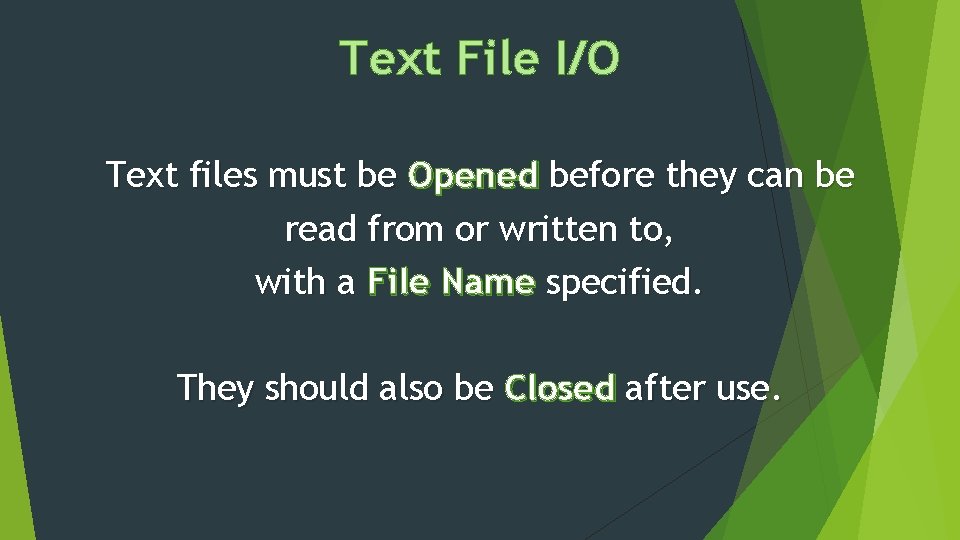
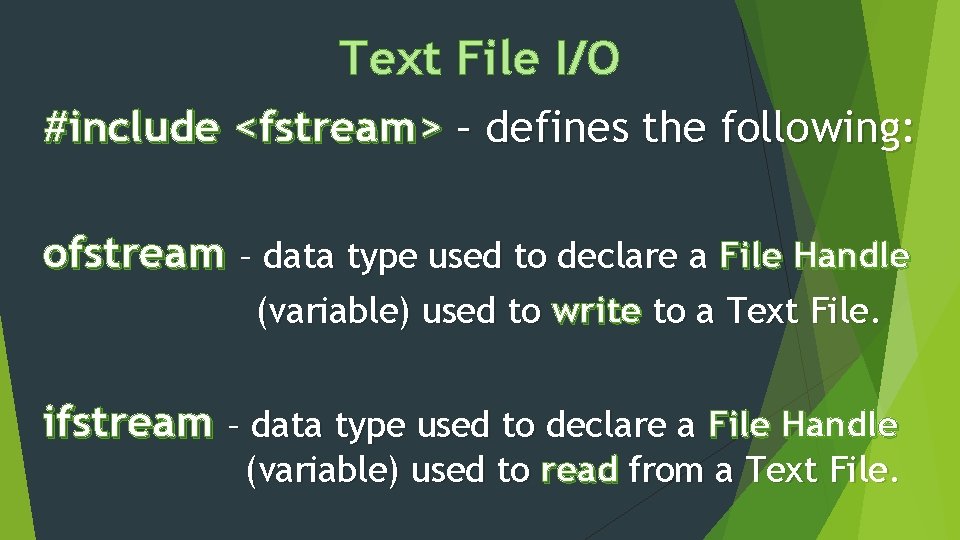
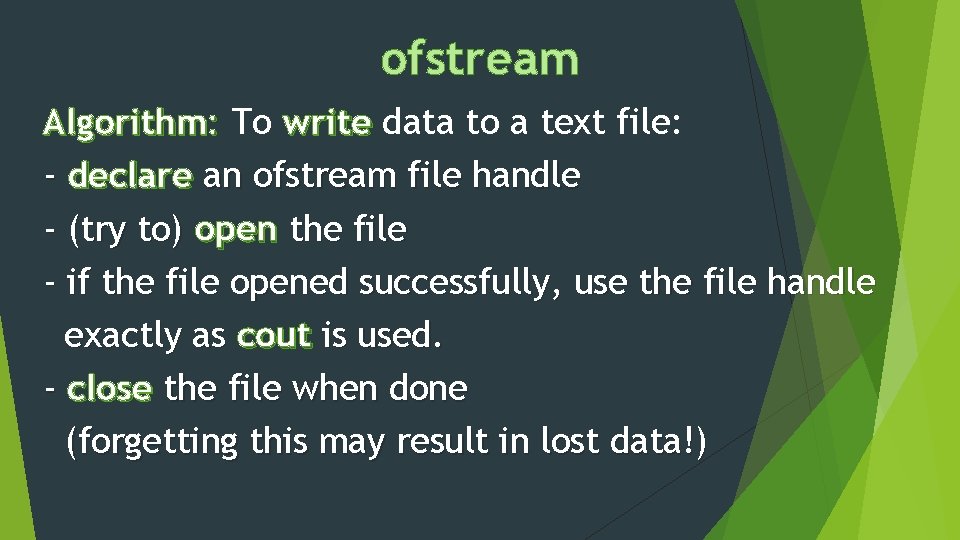
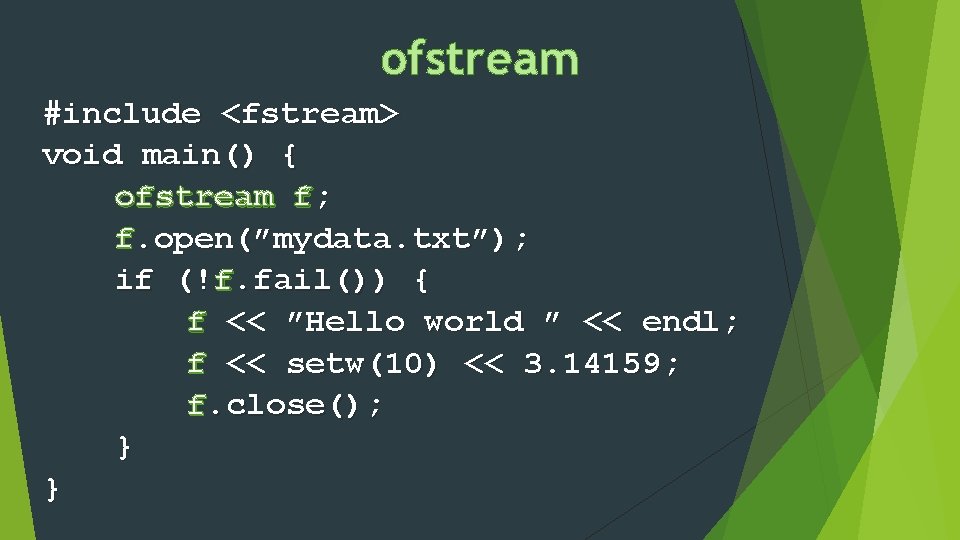
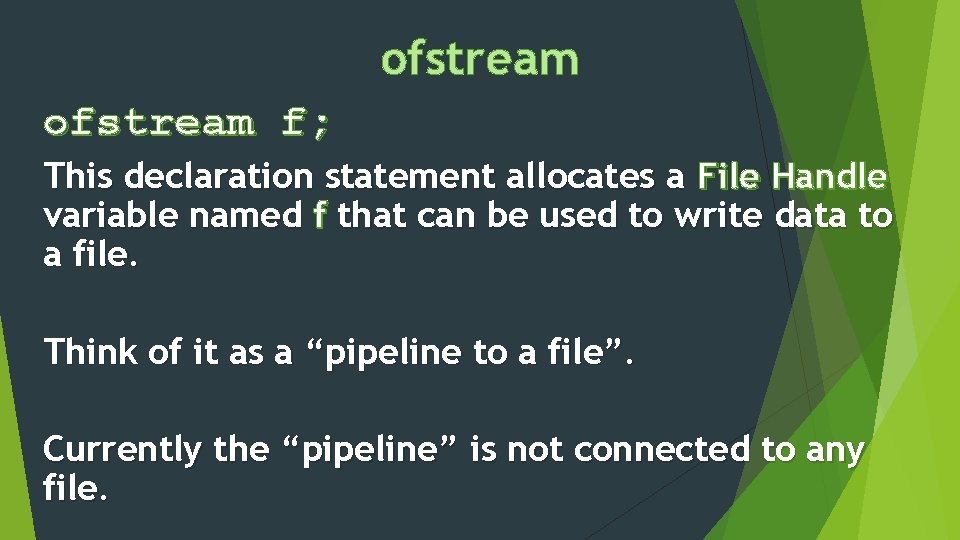
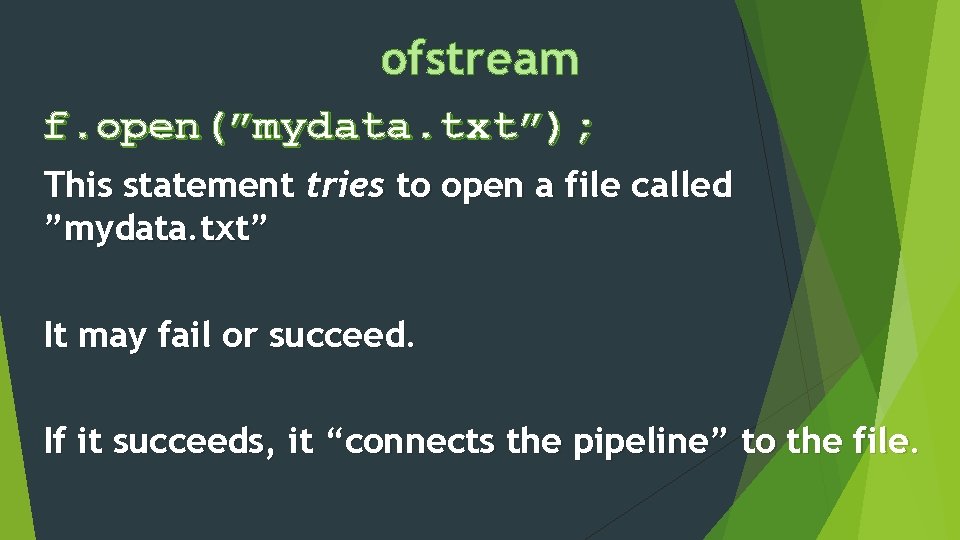
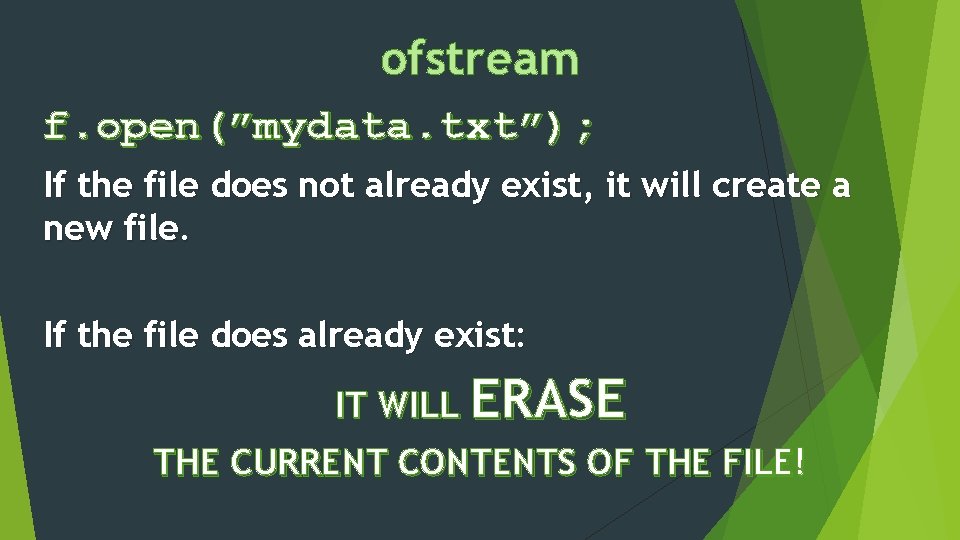
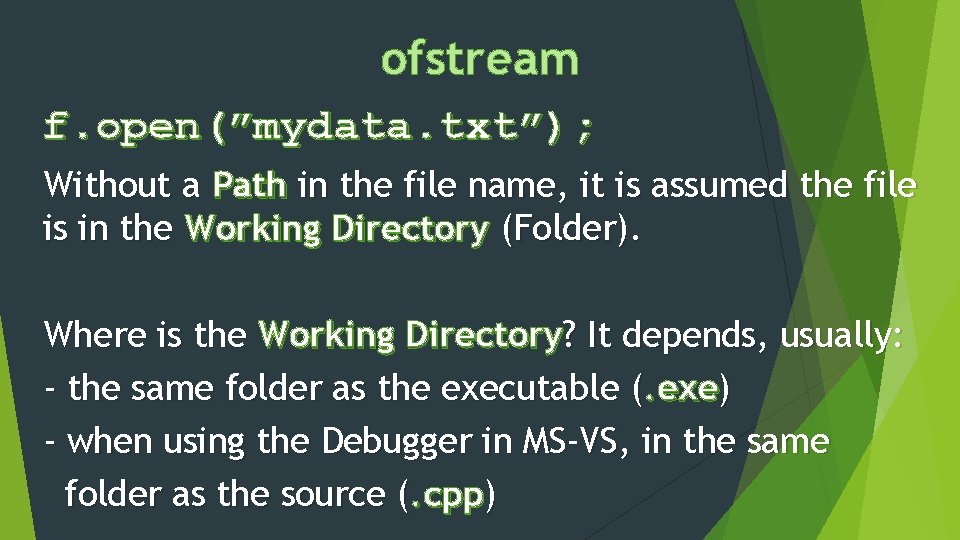
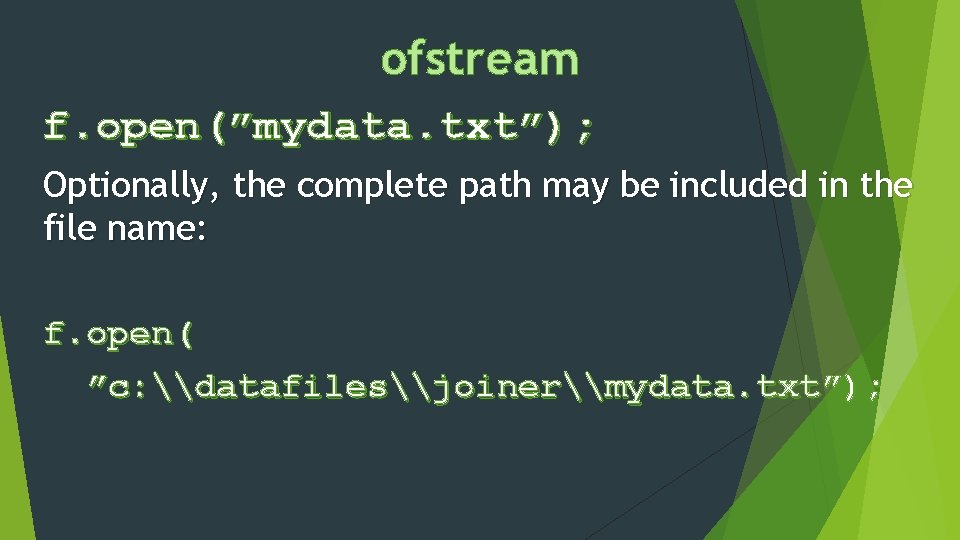
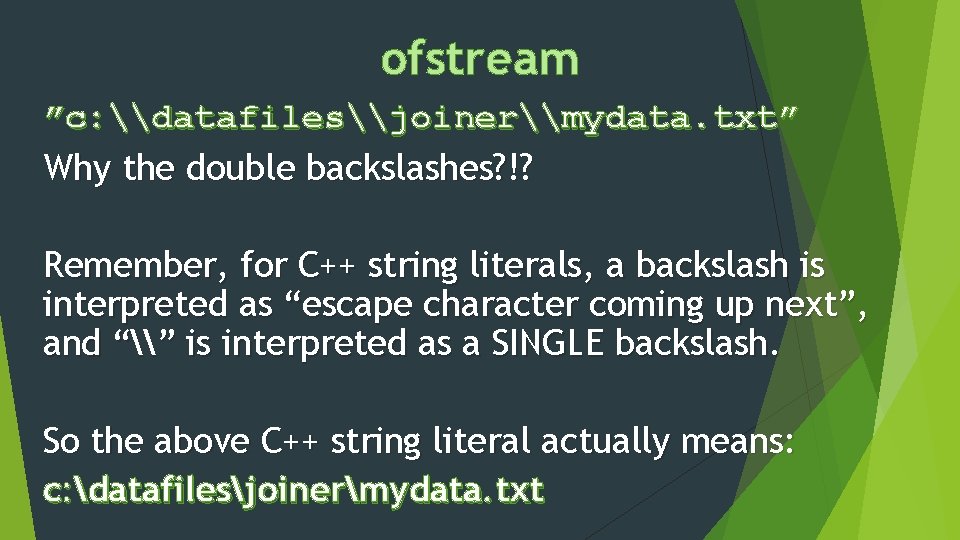
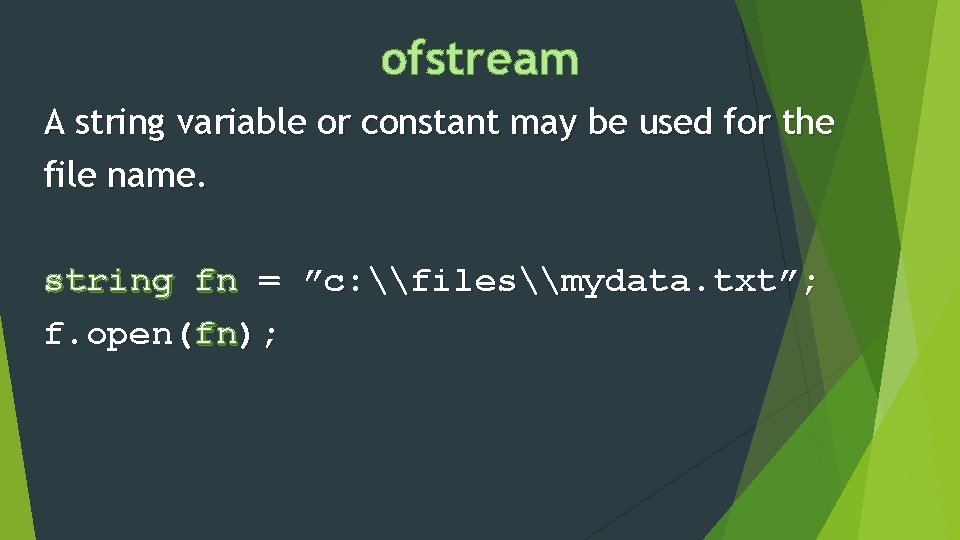
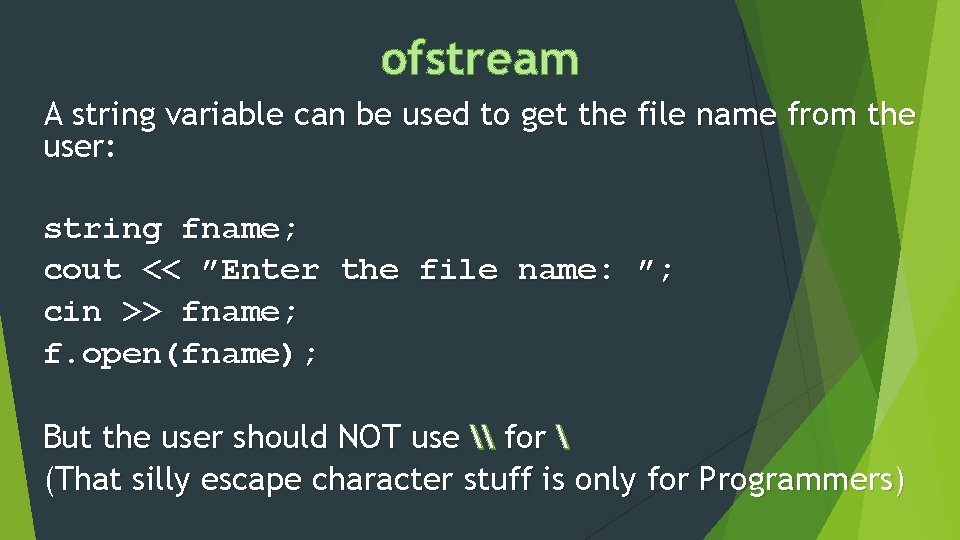
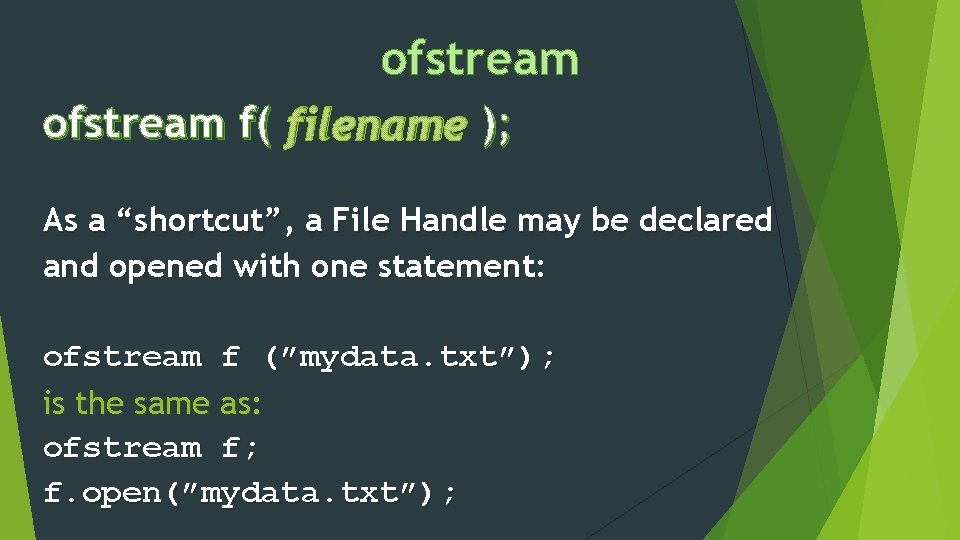
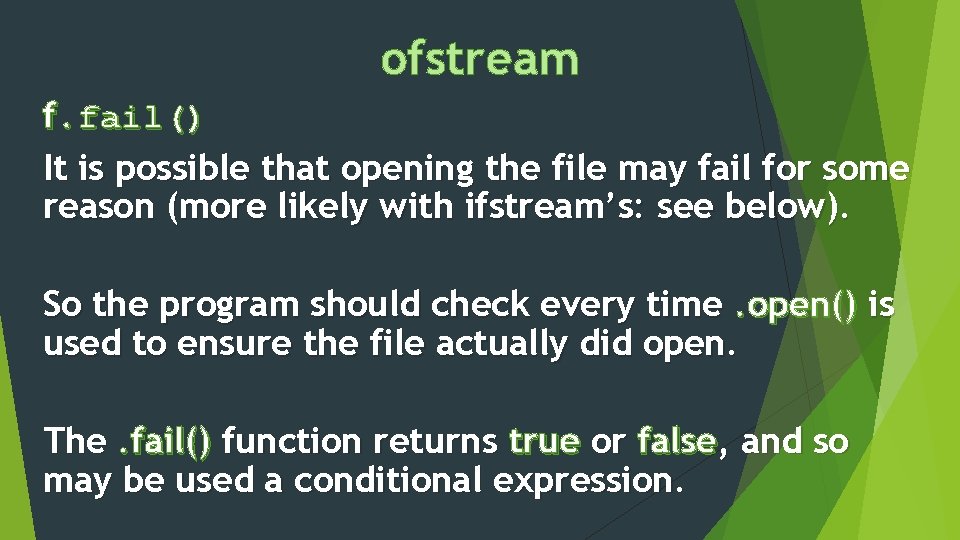
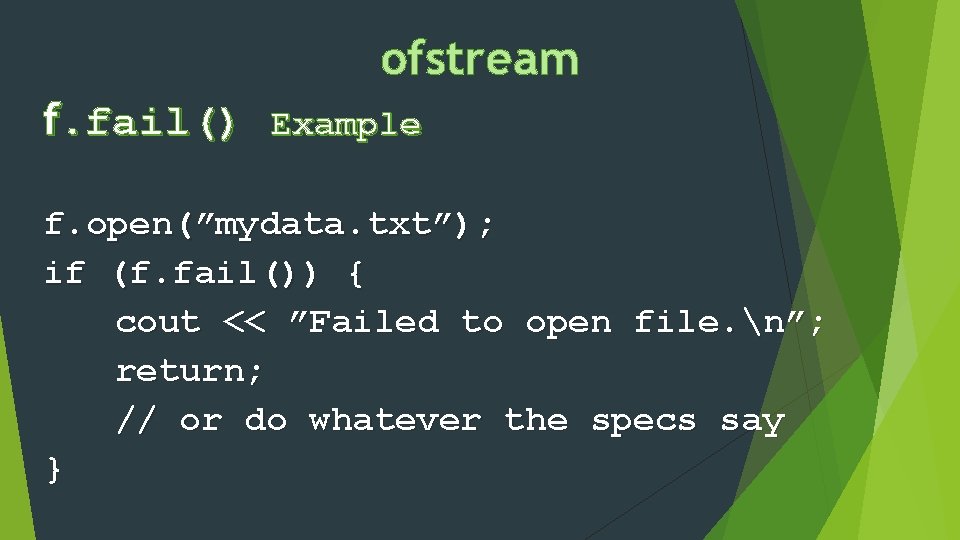
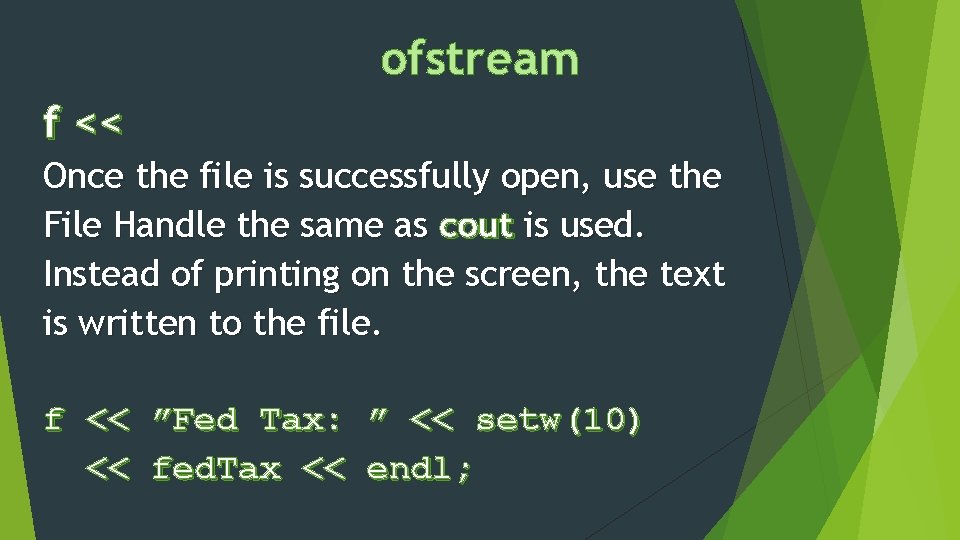
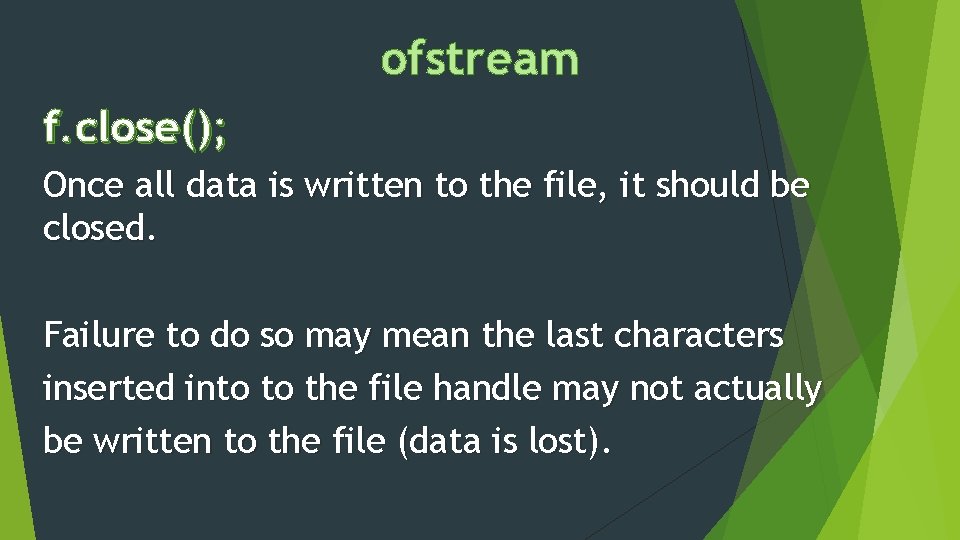
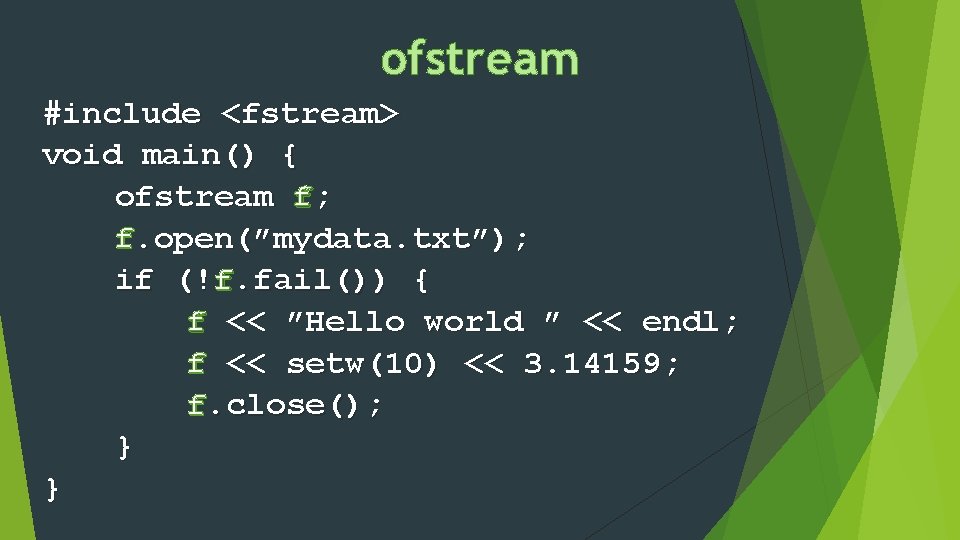
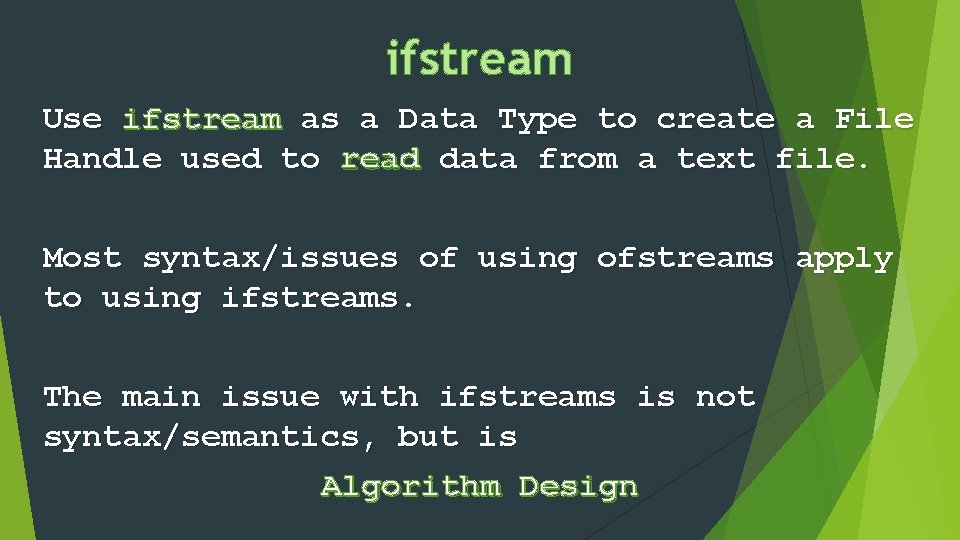
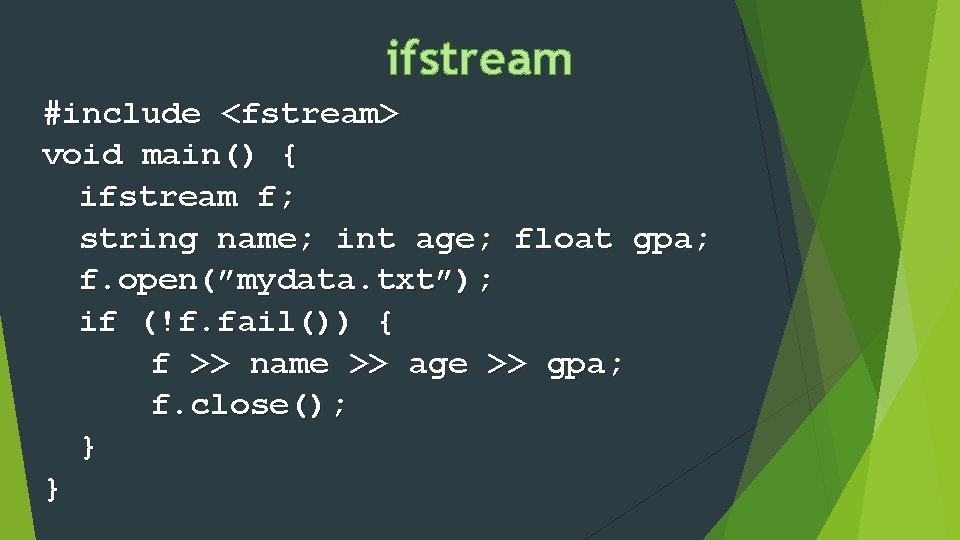
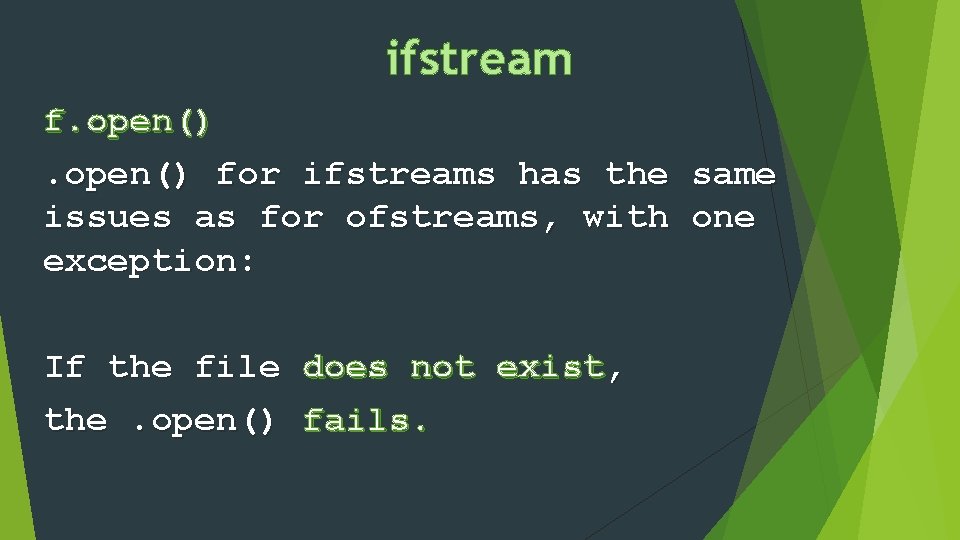
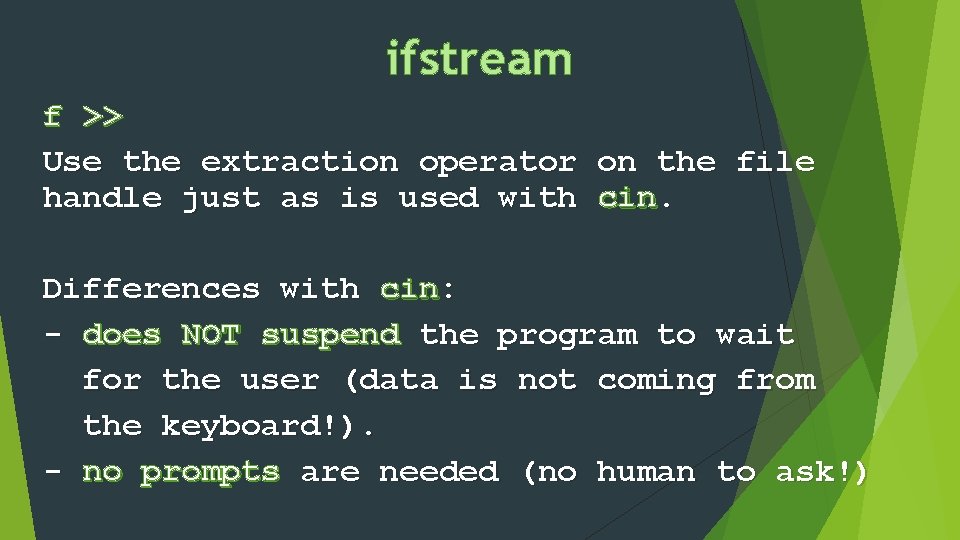
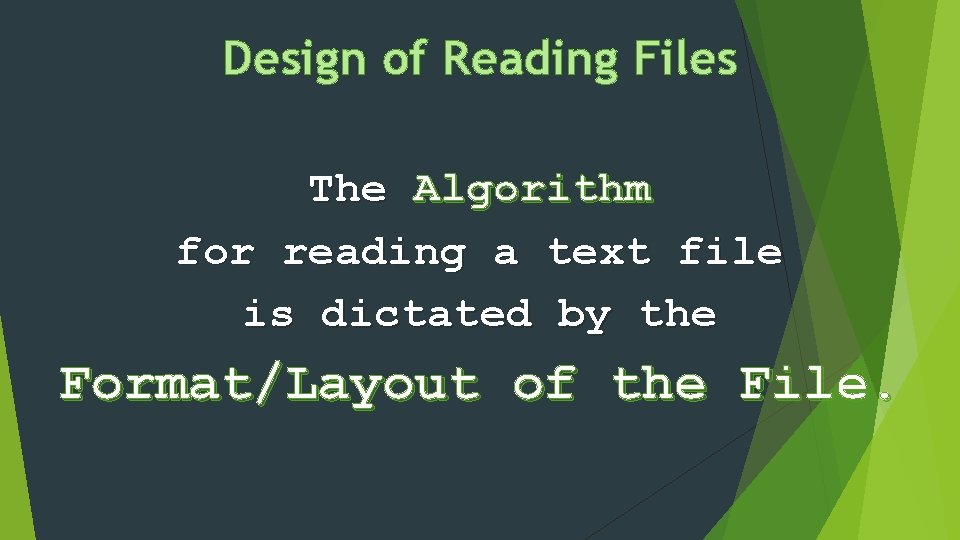
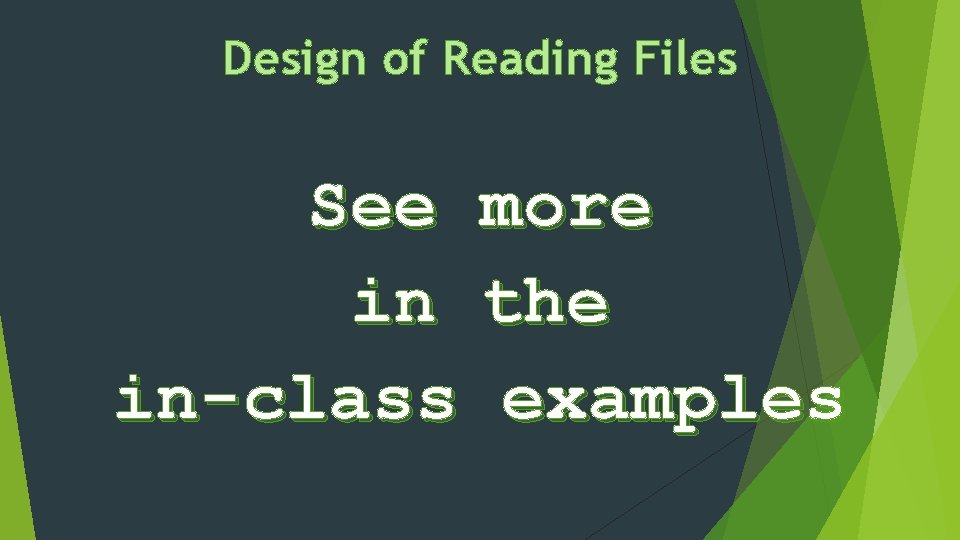
- Slides: 26
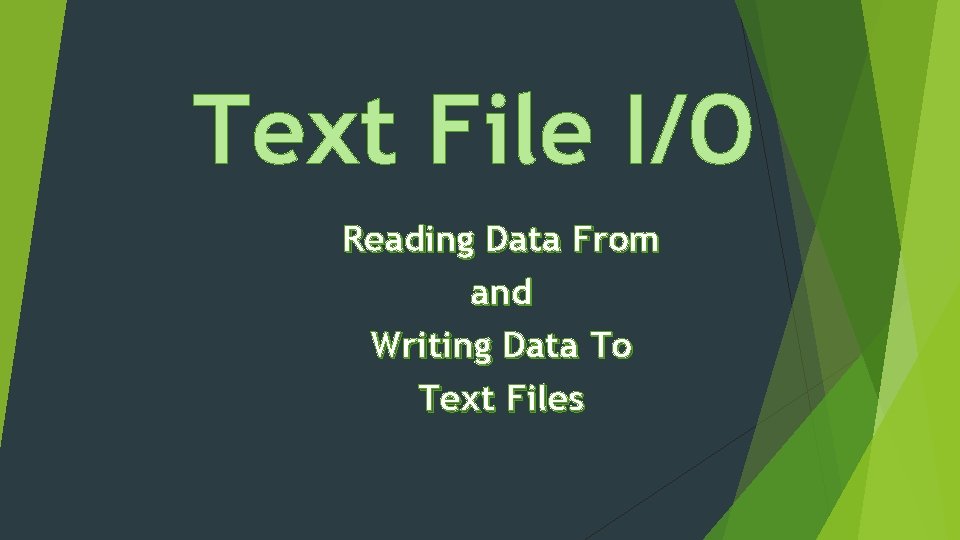
Text File I/O Reading Data From and Writing Data To Text Files
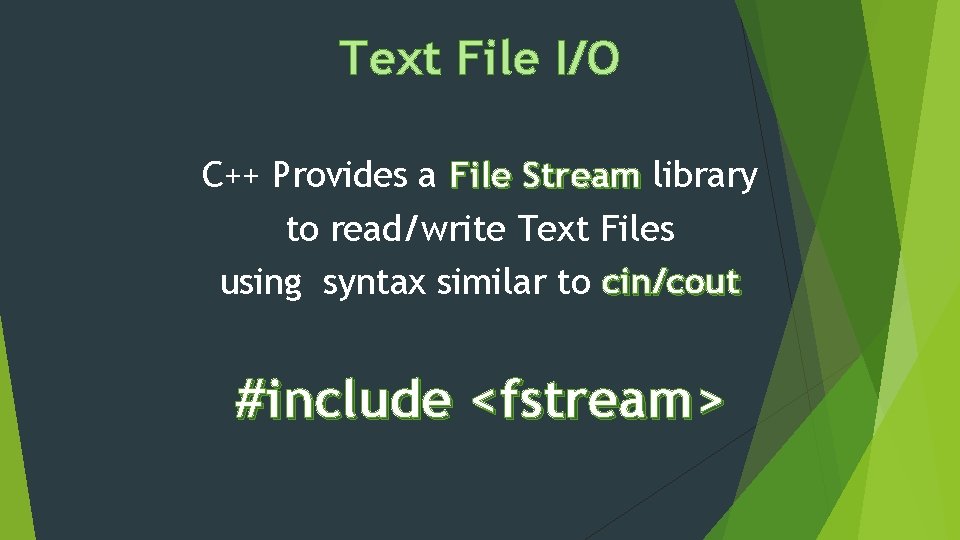
Text File I/O C++ Provides a File Stream library to read/write Text Files using syntax similar to cin/cout #include <fstream>
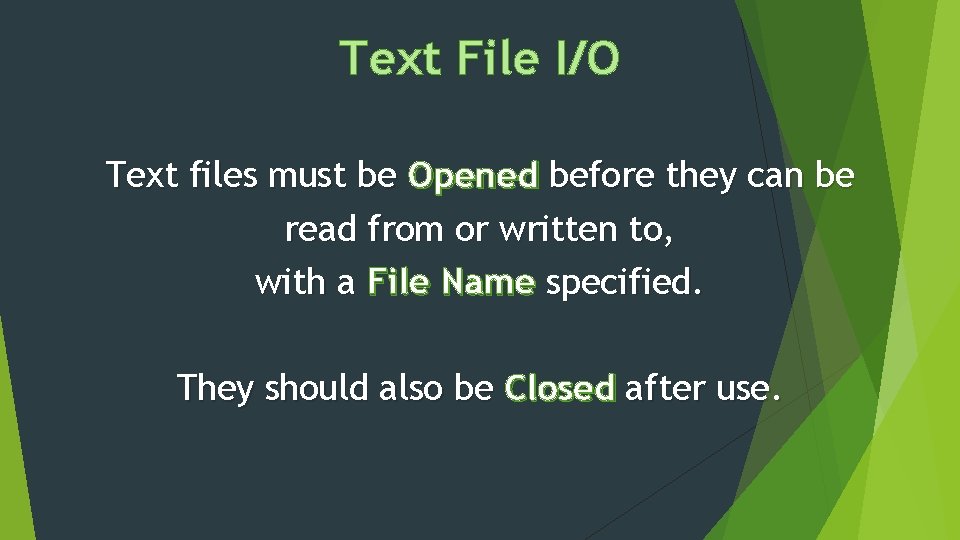
Text File I/O Text files must be Opened before they can be read from or written to, with a File Name specified. They should also be Closed after use.
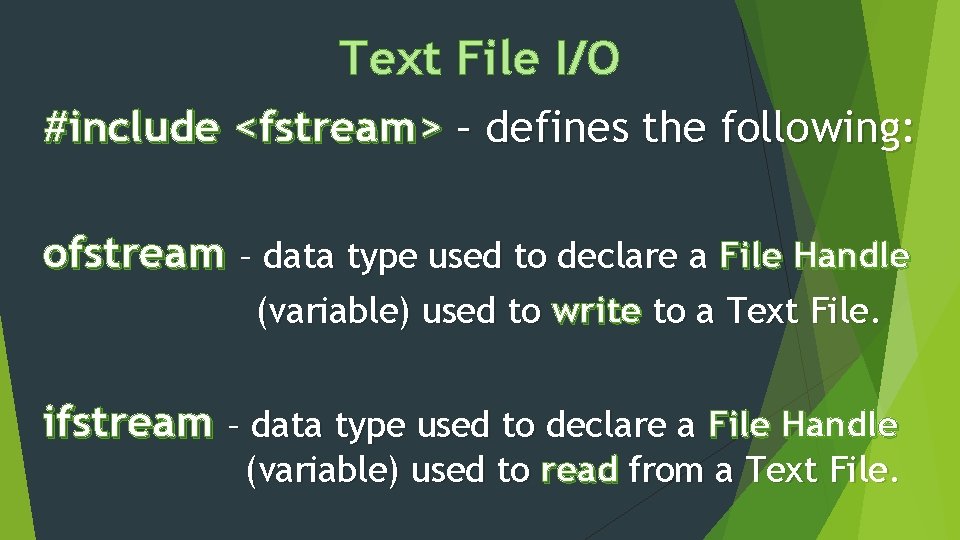
Text File I/O #include <fstream> – defines the following: ofstream – data type used to declare a File Handle (variable) used to write to a Text File. ifstream – data type used to declare a File Handle (variable) used to read from a Text File.
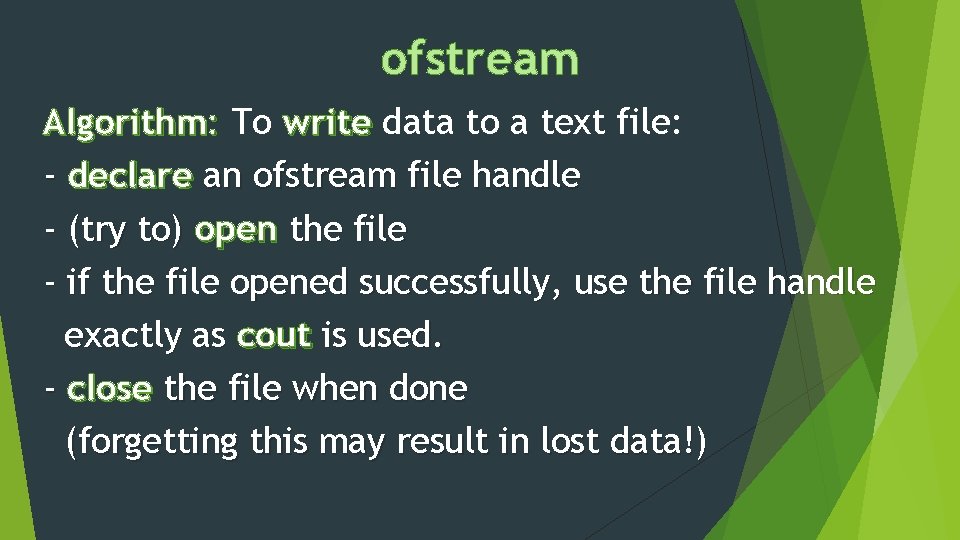
ofstream Algorithm: To write data to a text file: - declare an ofstream file handle - (try to) open the file - if the file opened successfully, use the file handle exactly as cout is used. - close the file when done (forgetting this may result in lost data!)
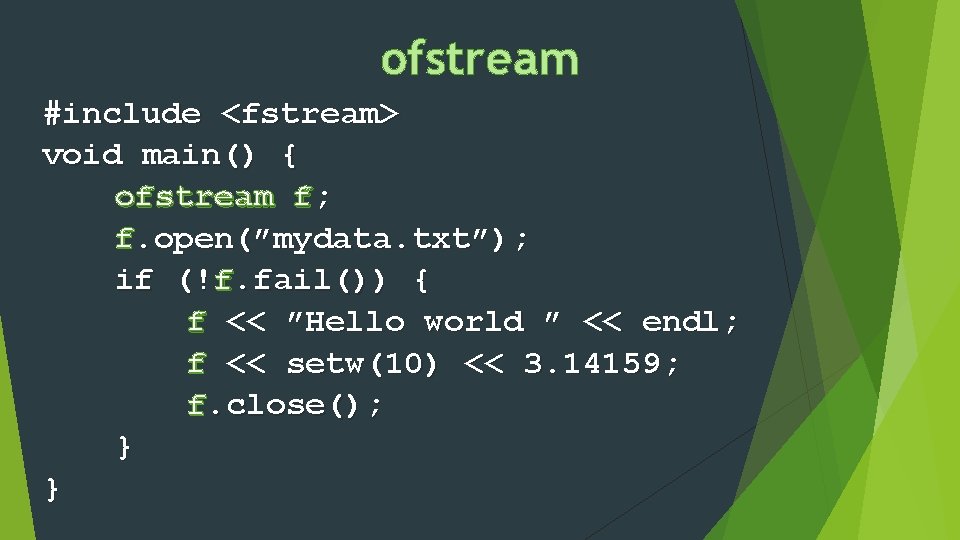
ofstream #include <fstream> void main() { ofstream f; f. open(”mydata. txt”); if (!f. fail()) { f << ”Hello world ” << endl; f << setw(10) << 3. 14159; f. close(); } }
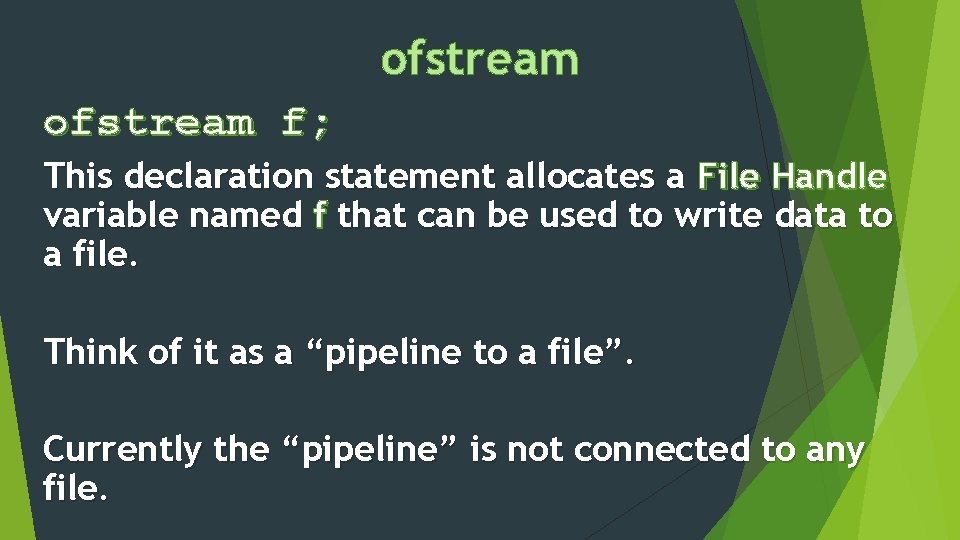
ofstream f; This declaration statement allocates a File Handle variable named f that can be used to write data to a file. Think of it as a “pipeline to a file”. Currently the “pipeline” is not connected to any file.
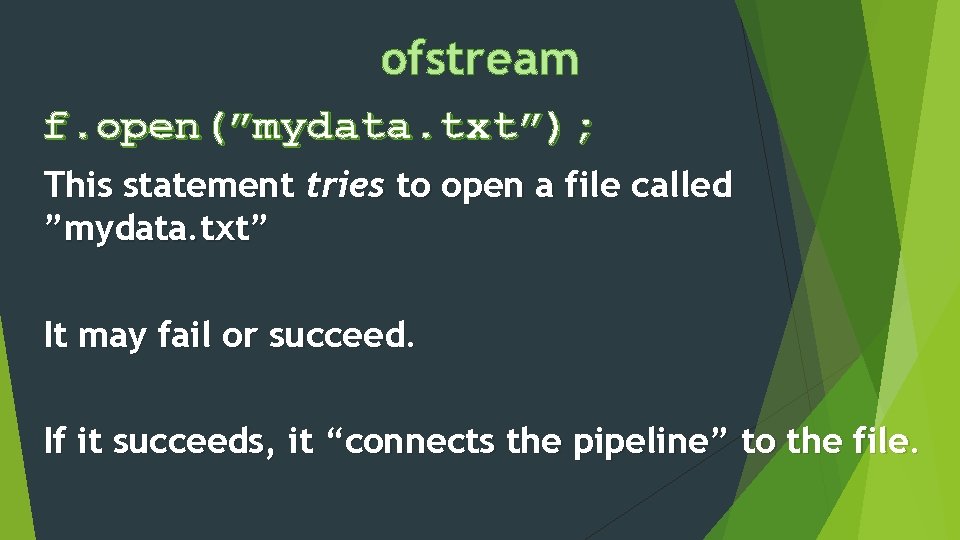
ofstream f. open(”mydata. txt”); This statement tries to open a file called ”mydata. txt” It may fail or succeed. If it succeeds, it “connects the pipeline” to the file.
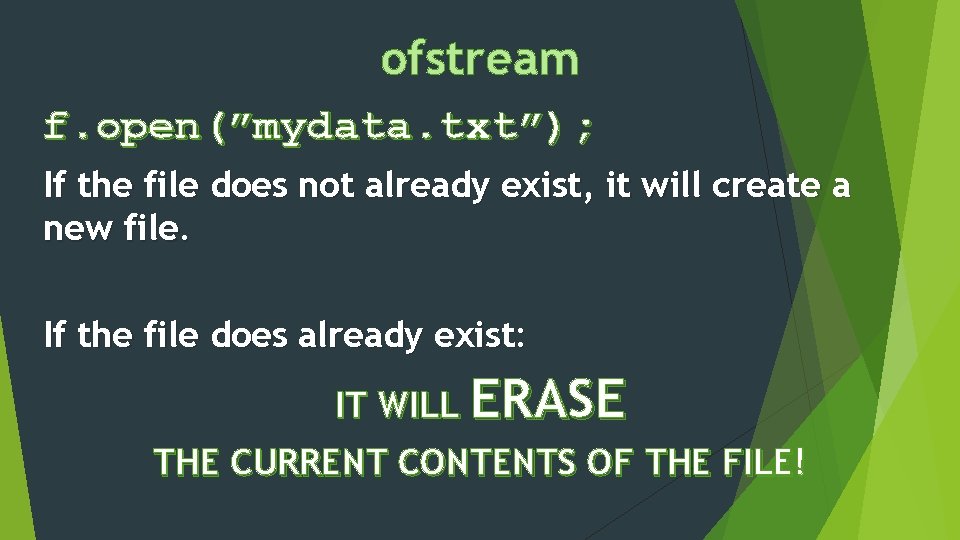
ofstream f. open(”mydata. txt”); If the file does not already exist, it will create a new file. If the file does already exist: IT WILL ERASE THE CURRENT CONTENTS OF THE FILE!
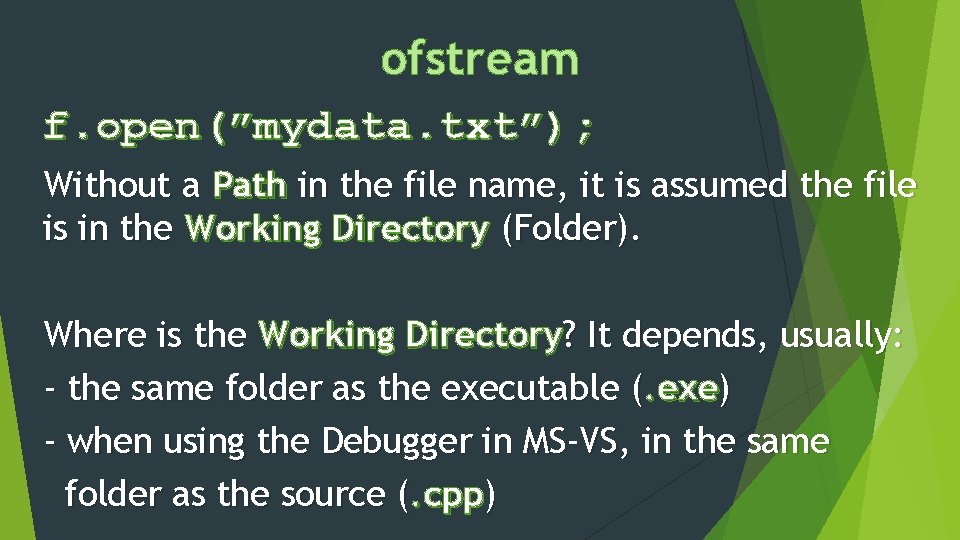
ofstream f. open(”mydata. txt”); Without a Path in the file name, it is assumed the file is in the Working Directory (Folder). Where is the Working Directory? It depends, usually: - the same folder as the executable (. exe) - when using the Debugger in MS-VS, in the same folder as the source (. cpp)
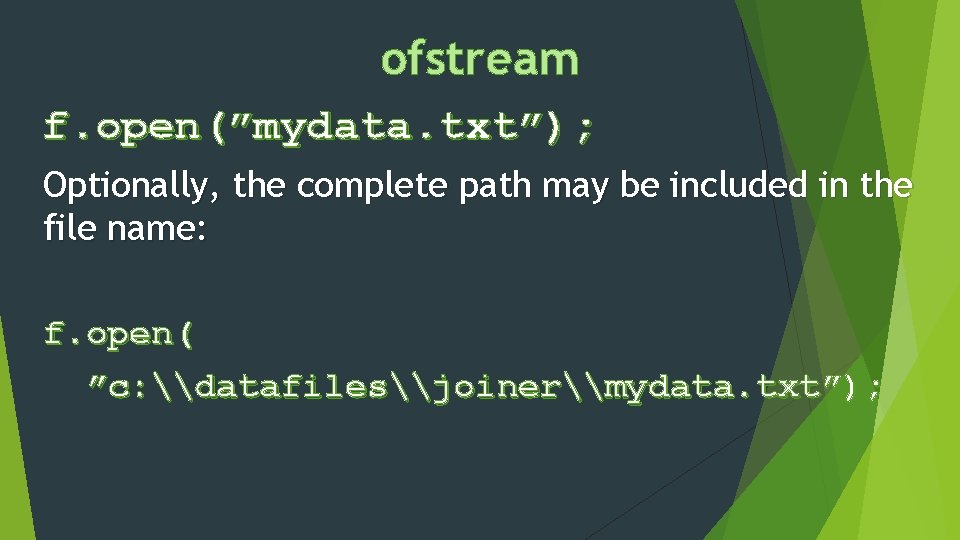
ofstream f. open(”mydata. txt”); Optionally, the complete path may be included in the file name: f. open( ”c: \datafiles\joiner\mydata. txt”);
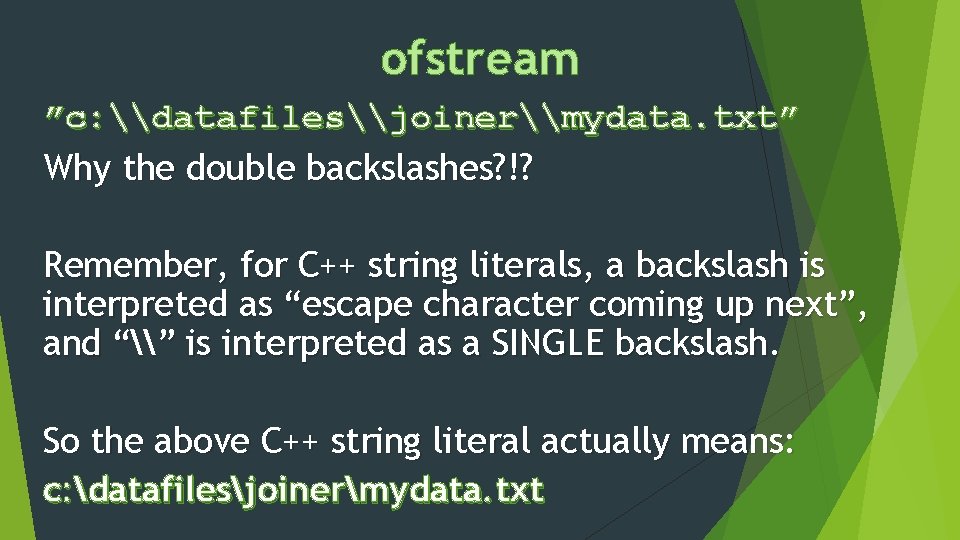
ofstream ”c: \datafiles\joiner\mydata. txt” Why the double backslashes? !? Remember, for C++ string literals, a backslash is interpreted as “escape character coming up next”, and “\” is interpreted as a SINGLE backslash. So the above C++ string literal actually means: c: datafilesjoinermydata. txt
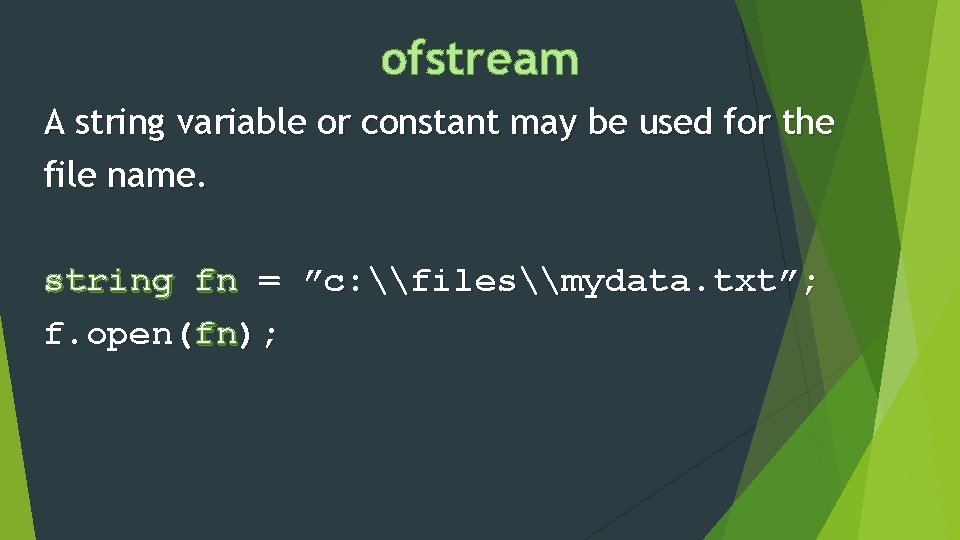
ofstream A string variable or constant may be used for the file name. string fn = ”c: \files\mydata. txt”; f. open(fn);
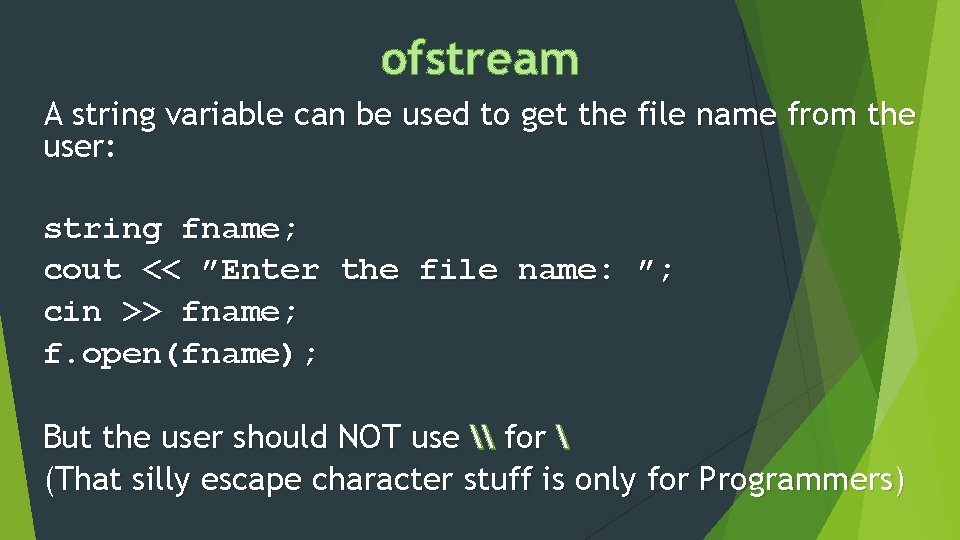
ofstream A string variable can be used to get the file name from the user: string fname; cout << ”Enter the file name: ”; cin >> fname; f. open(fname); But the user should NOT use \ for (That silly escape character stuff is only for Programmers)
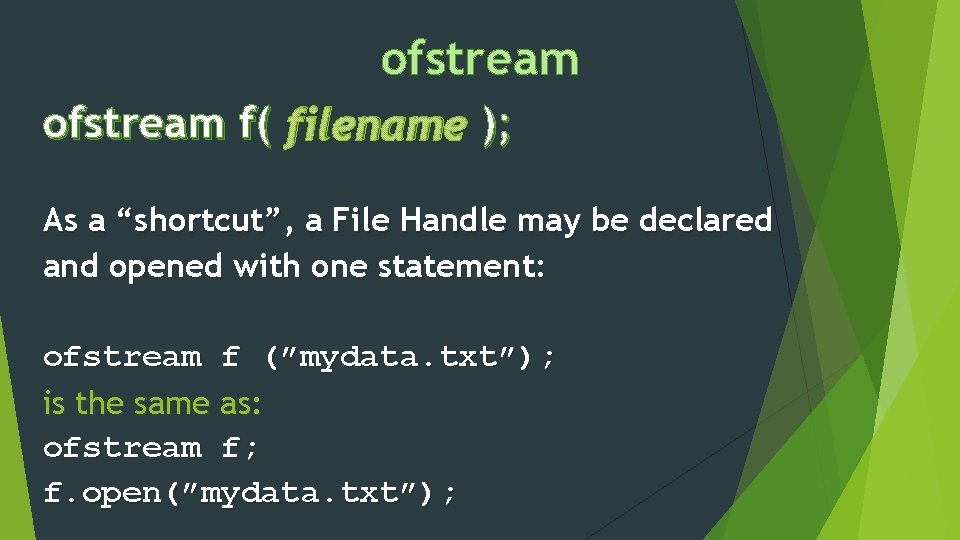
ofstream f( filename ); As a “shortcut”, a File Handle may be declared and opened with one statement: ofstream f (”mydata. txt”); is the same as: ofstream f; f. open(”mydata. txt”);
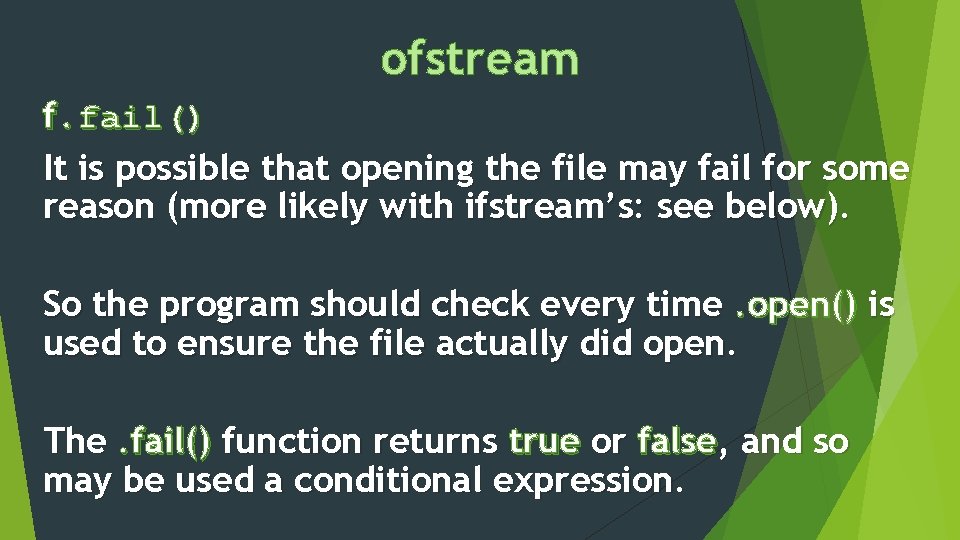
ofstream f. fail() It is possible that opening the file may fail for some reason (more likely with ifstream’s: see below). So the program should check every time. open() is used to ensure the file actually did open. The. fail() function returns true or false, and so may be used a conditional expression.
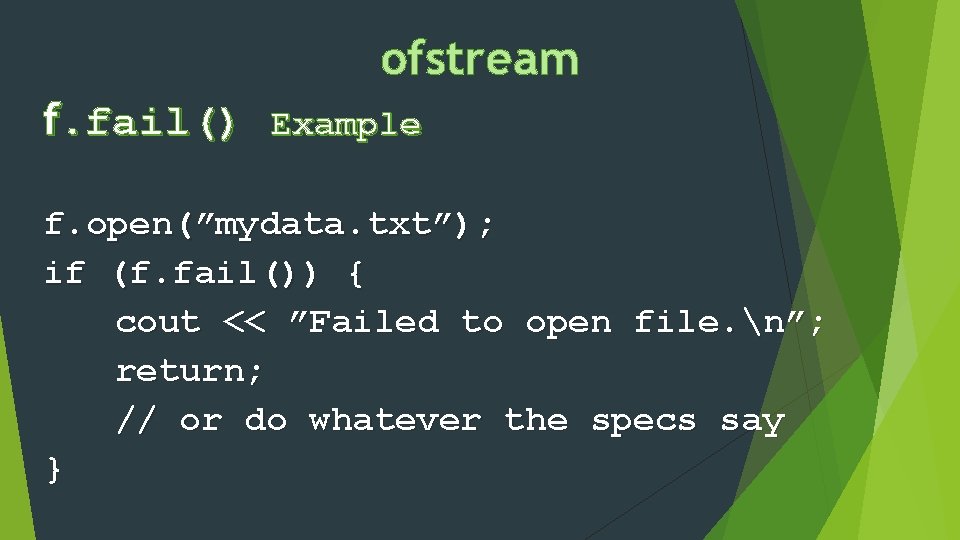
ofstream f. fail() Example f. open(”mydata. txt”); if (f. fail()) { cout << ”Failed to open file. n”; return; // or do whatever the specs say }
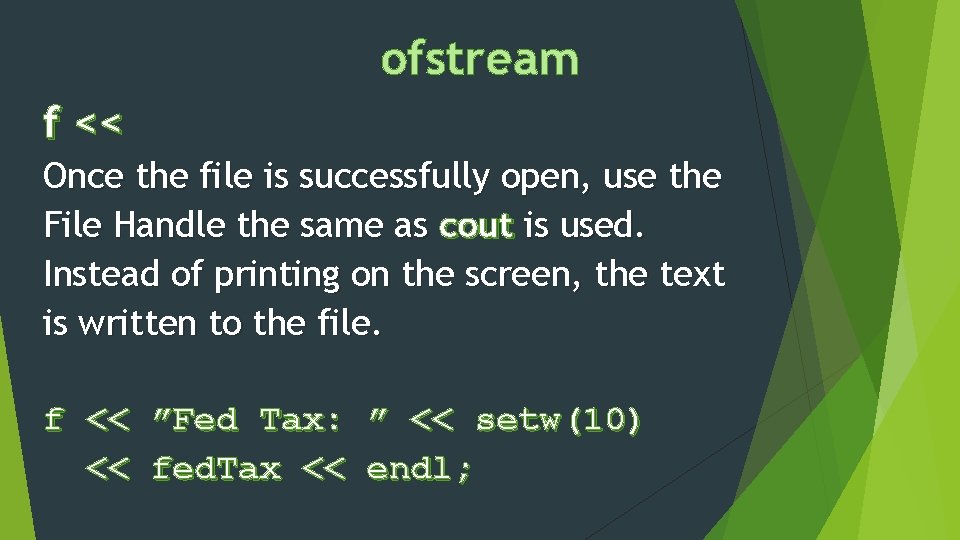
ofstream f << Once the file is successfully open, use the File Handle the same as cout is used. Instead of printing on the screen, the text is written to the file. f << ”Fed Tax: ” << setw(10) << fed. Tax << endl;
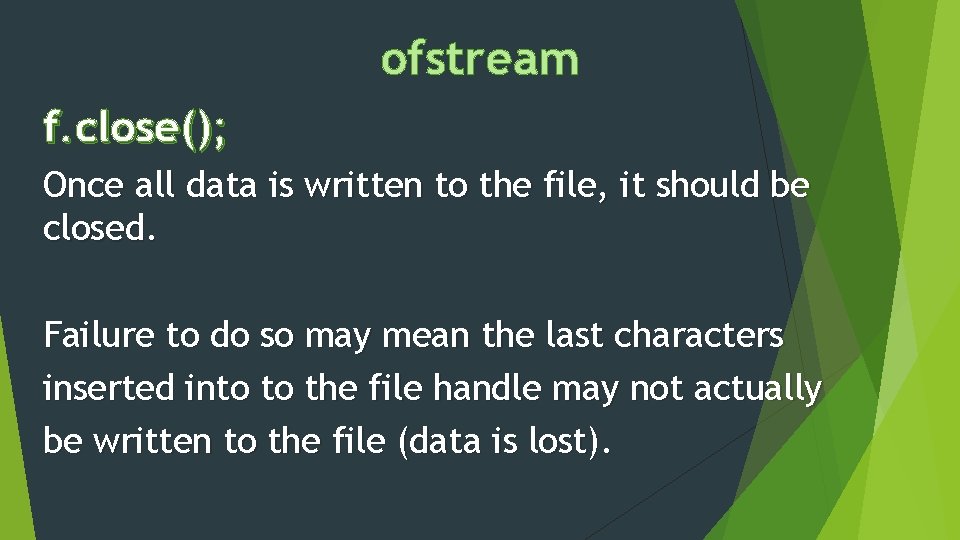
ofstream f. close(); Once all data is written to the file, it should be closed. Failure to do so may mean the last characters inserted into to the file handle may not actually be written to the file (data is lost).
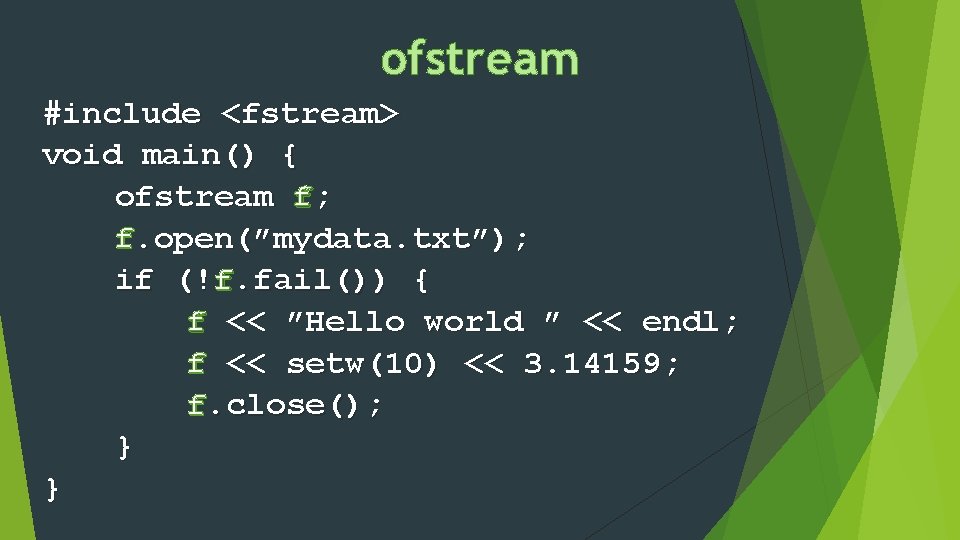
ofstream #include <fstream> void main() { ofstream f; f. open(”mydata. txt”); if (!f. fail()) { f << ”Hello world ” << endl; f << setw(10) << 3. 14159; f. close(); } }
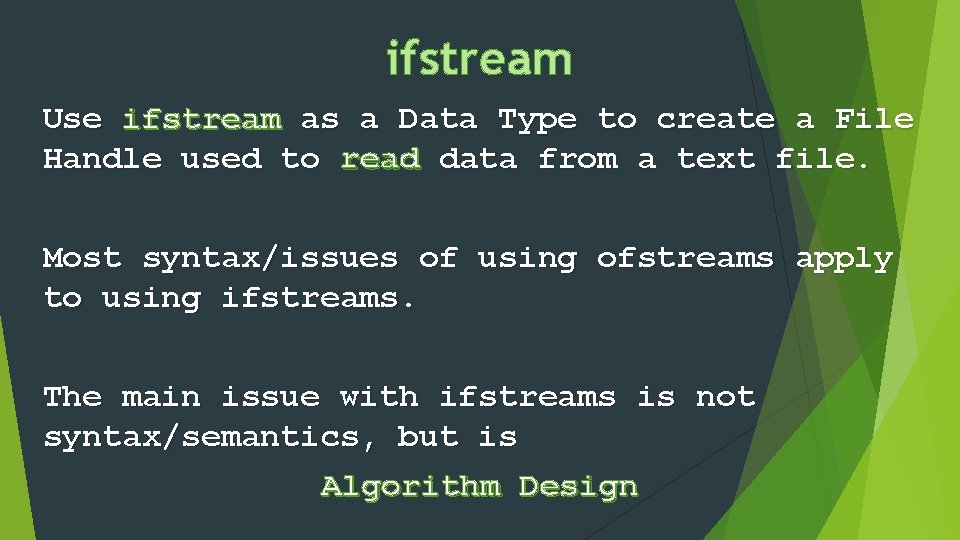
ifstream Use ifstream as a Data Type to create a File Handle used to read data from a text file. Most syntax/issues of using ofstreams apply to using ifstreams. The main issue with ifstreams is not syntax/semantics, but is Algorithm Design
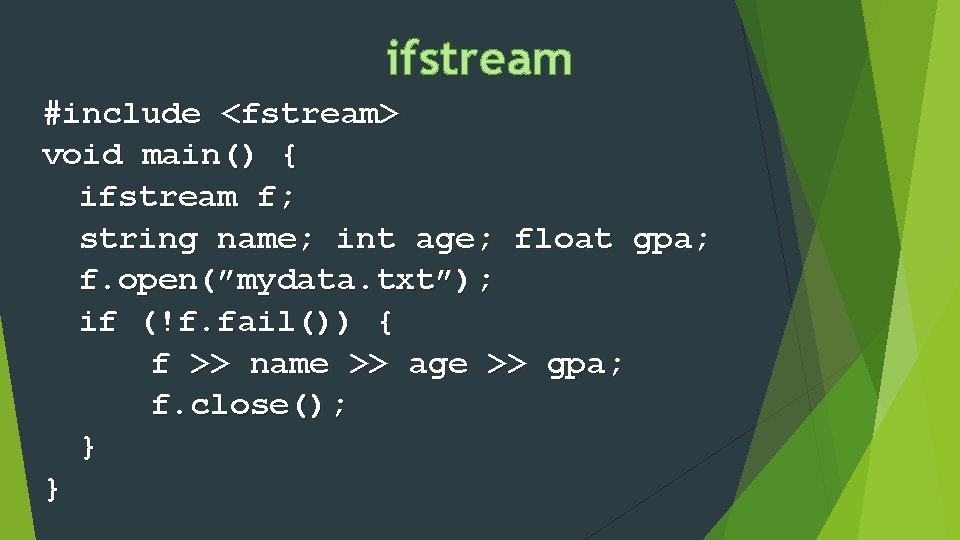
ifstream #include <fstream> void main() { ifstream f; string name; int age; float gpa; f. open(”mydata. txt”); if (!f. fail()) { f >> name >> age >> gpa; f. close(); } }
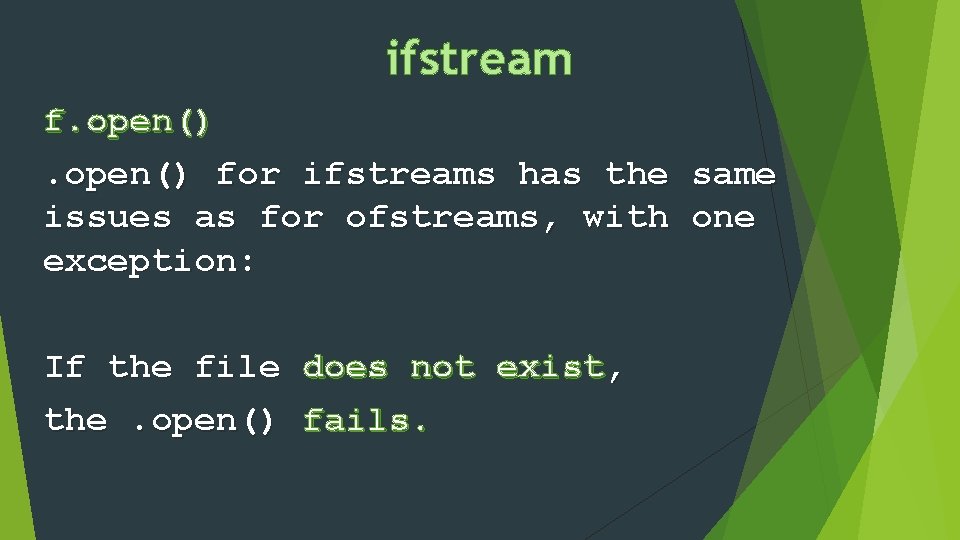
ifstream f. open() for ifstreams has the same issues as for ofstreams, with one exception: If the file does not exist, the. open() fails.
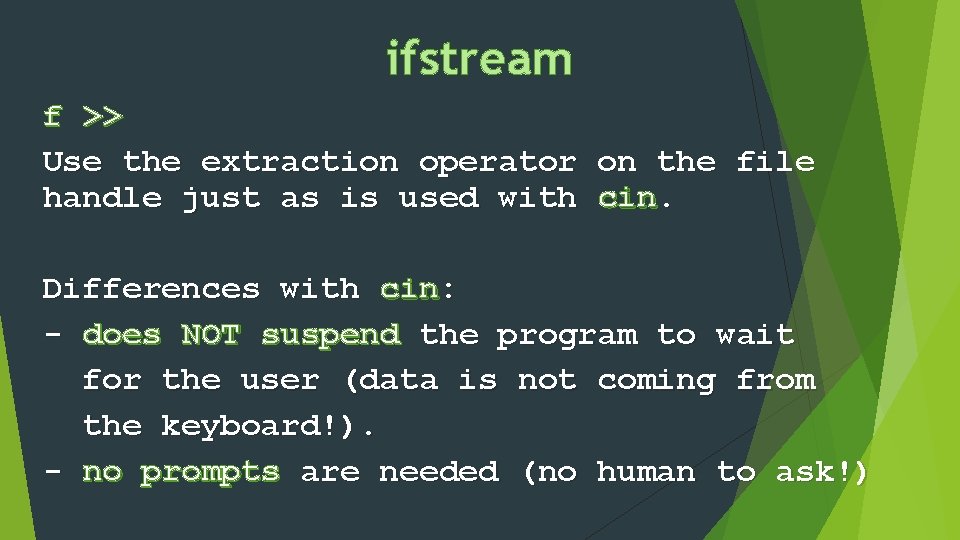
ifstream f >> Use the extraction operator handle just as is used with on the file cin. Differences with cin: - does NOT suspend the program to wait for the user (data is not coming from the keyboard!). - no prompts are needed (no human to ask!)
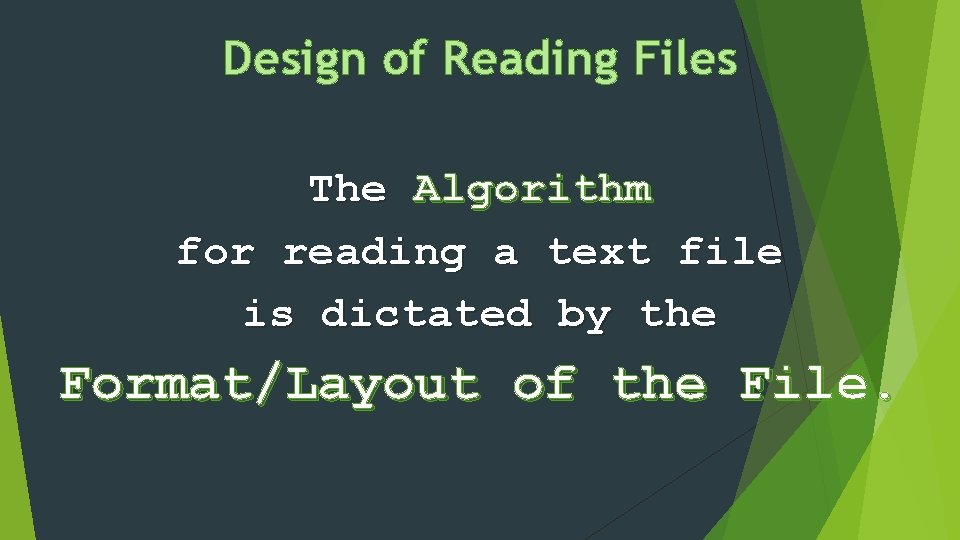
Design of Reading Files The Algorithm for reading a text file is dictated by the Format/Layout of the File.
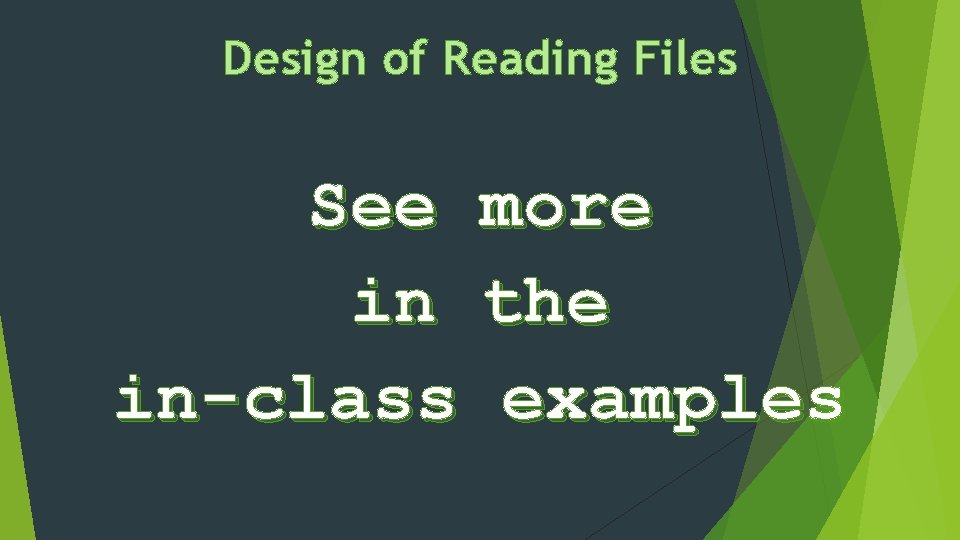
Design of Reading Files See more in the in-class examples
File-file yang dibuat oleh user pada jenis file di linux
Making connections images
Markup tag tells the web browser
Pre reading while reading and post reading activities
Physical image vs logical image
Fungsi dari create file pada operasi-operasi file (cont.)
Remote file access in distributed file system
In a file-oriented information system, a transaction file
Value of money in symbol
Aims of teaching reading
Types of reading skills
Real definition of extensive reading
Extensive reading
Intensive reading definition
Bmi html
Text file
Fhand python
Read the text and answer questions
Summer's reading hots
Factfile
Summarizing is a powerful
Reading and writing numbers 3/4
Exploration in creative reading and writing
Reading writing speaking and listening
Academic english reading and writing across the disciplines
Reading and writing quarter 3
Paper 1 explorations in creative reading and writing