Recitation File IO File IO File in File
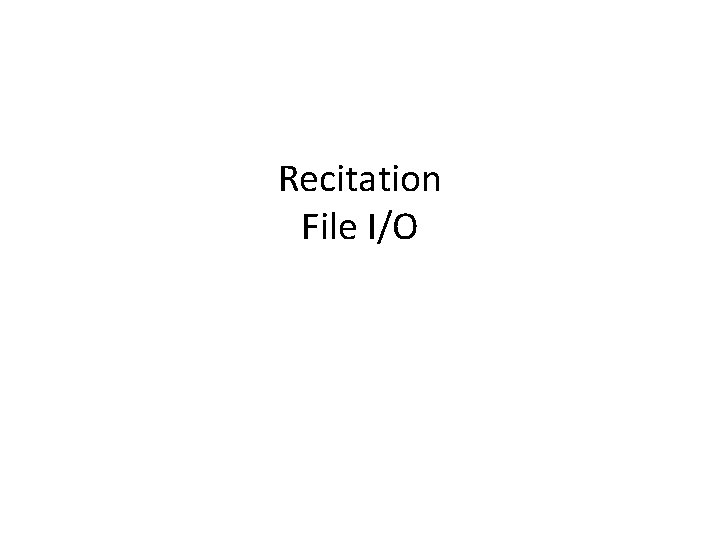
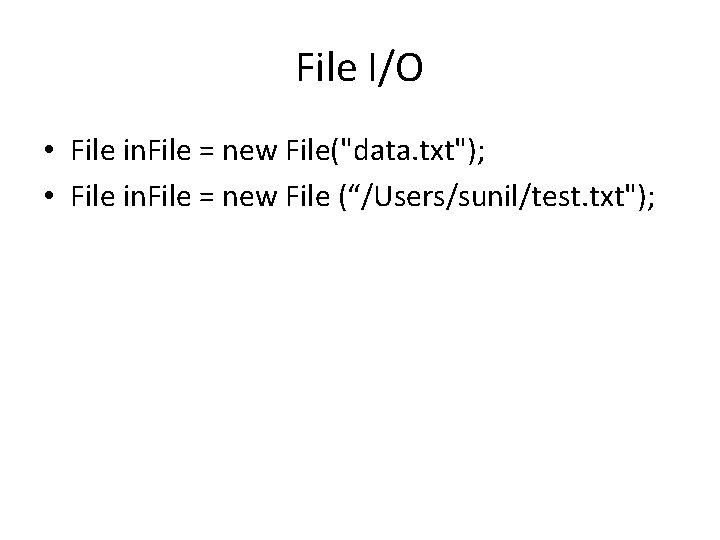
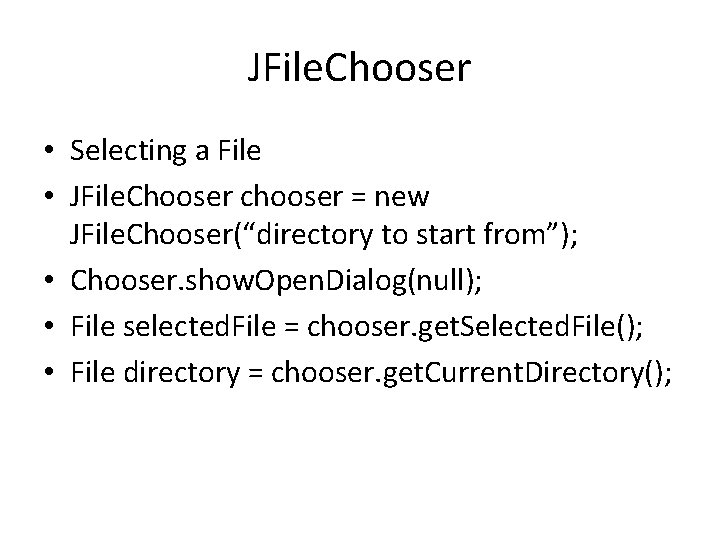
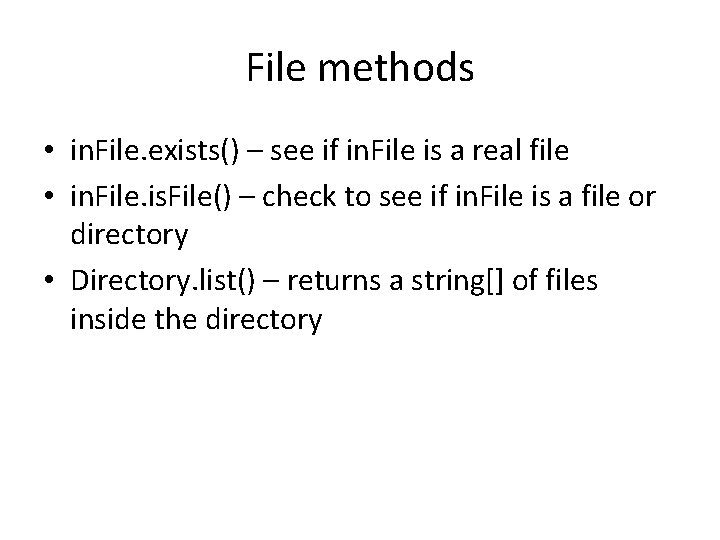
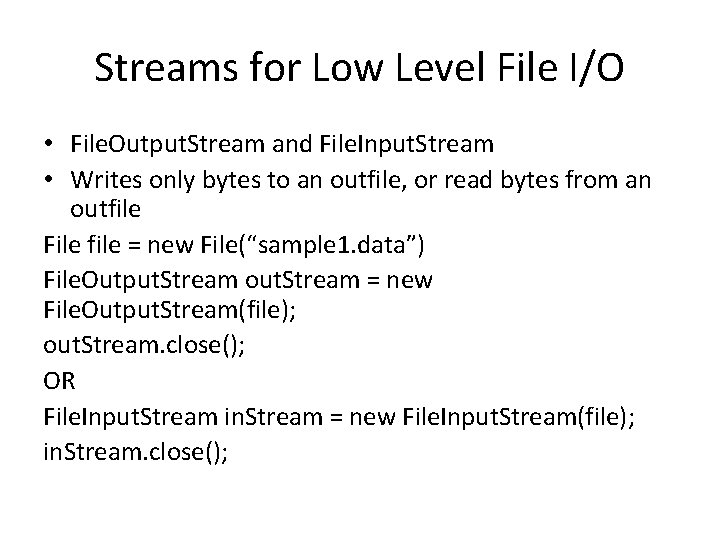
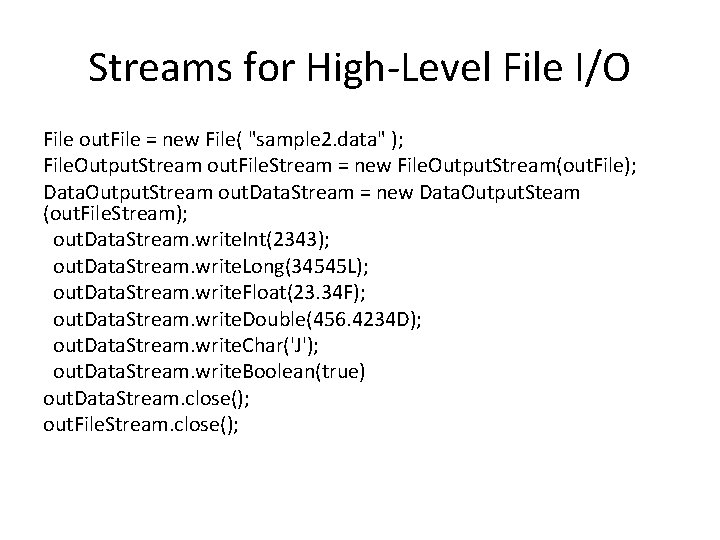
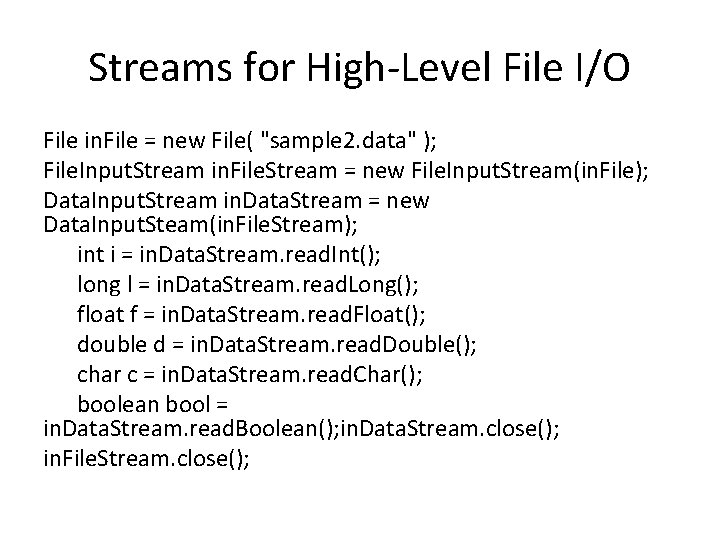
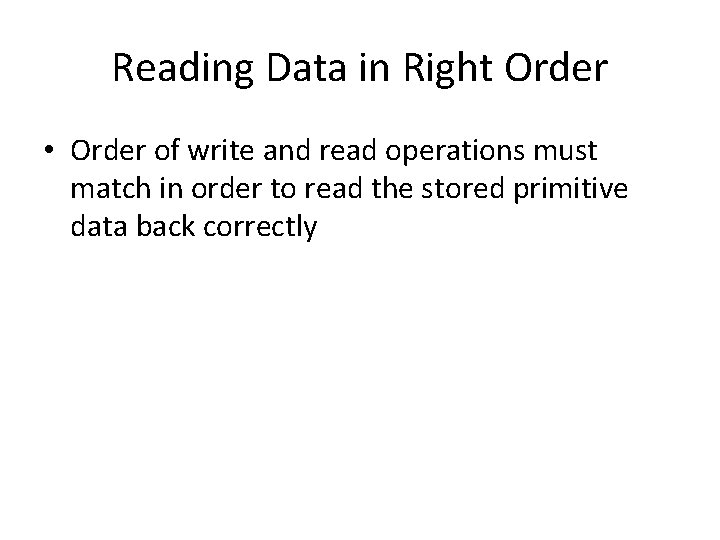
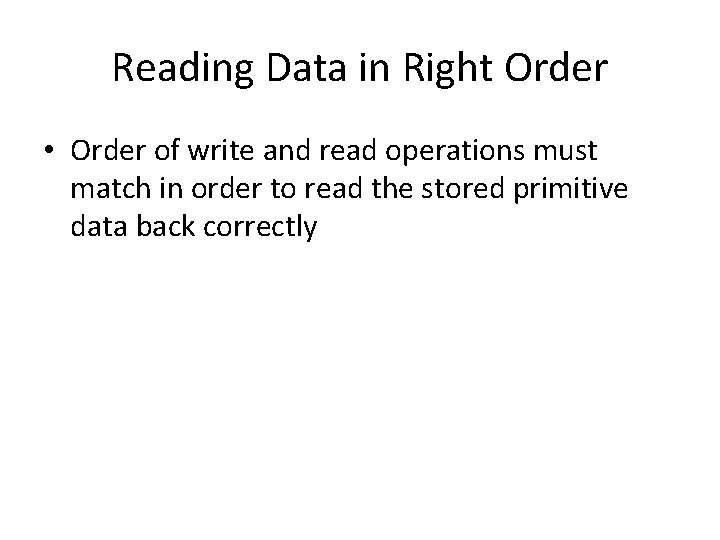
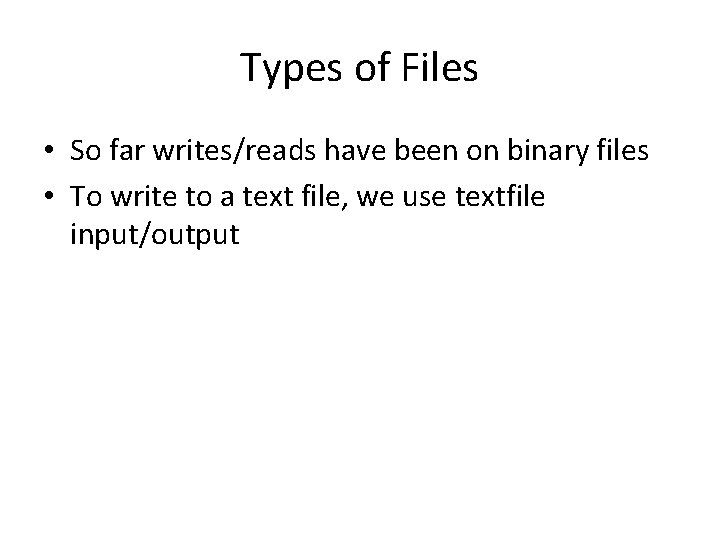
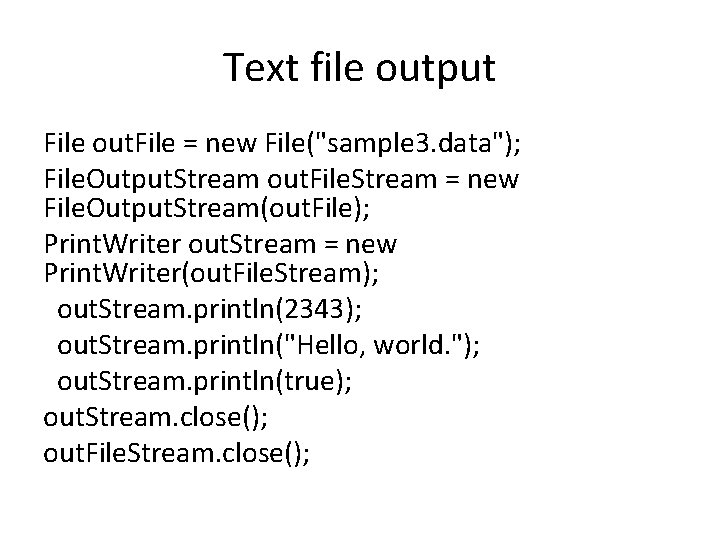
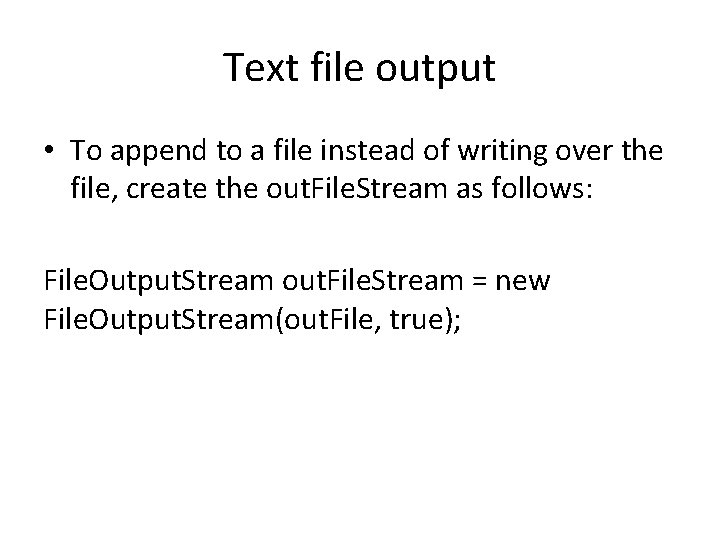
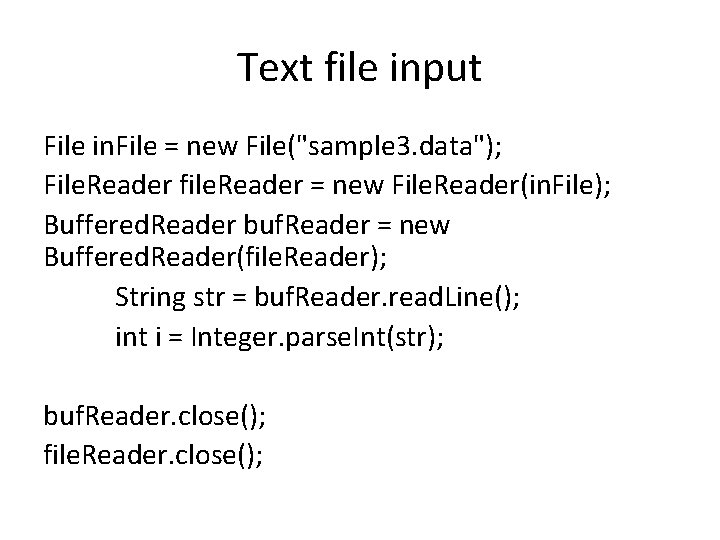
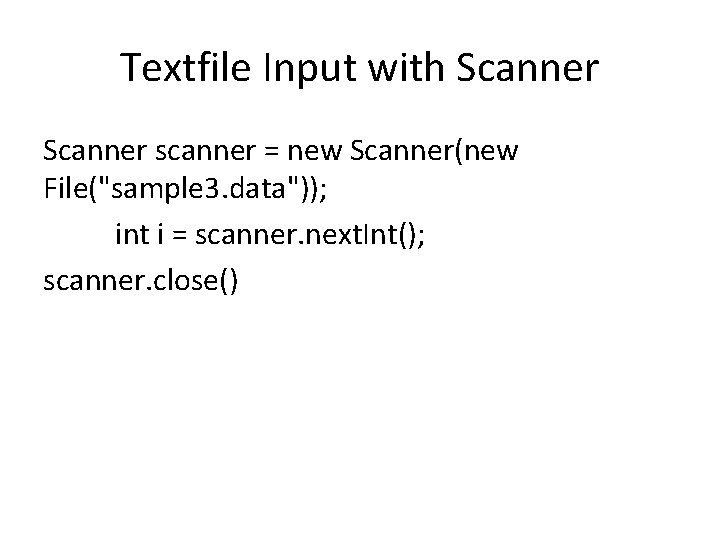
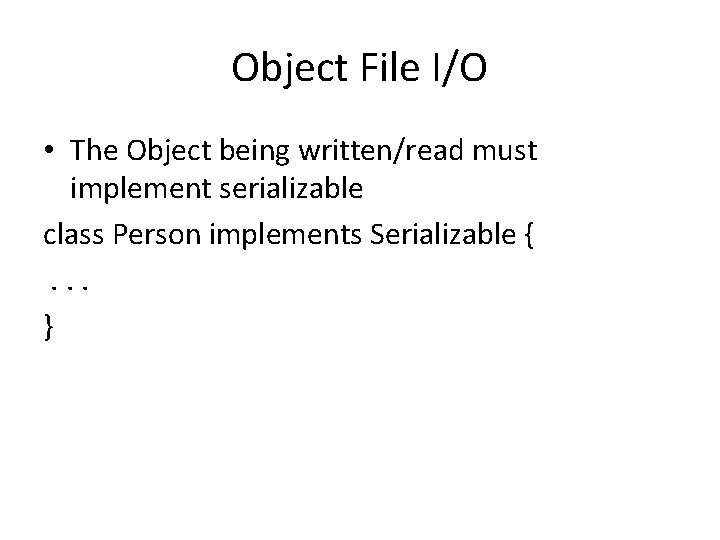
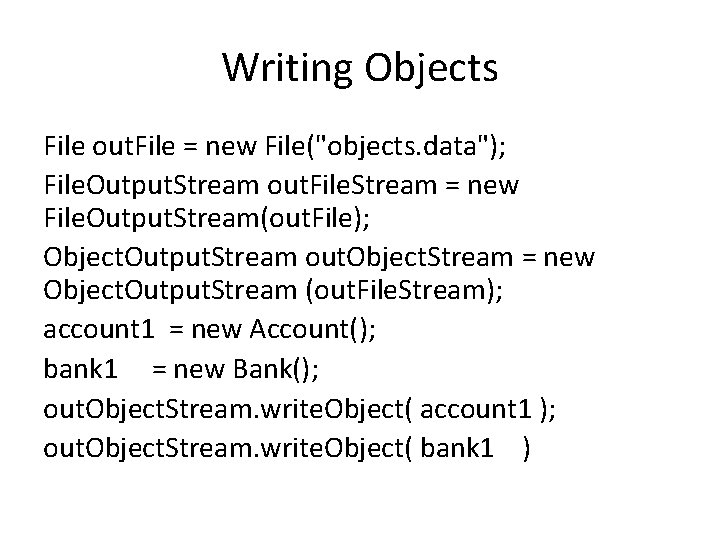
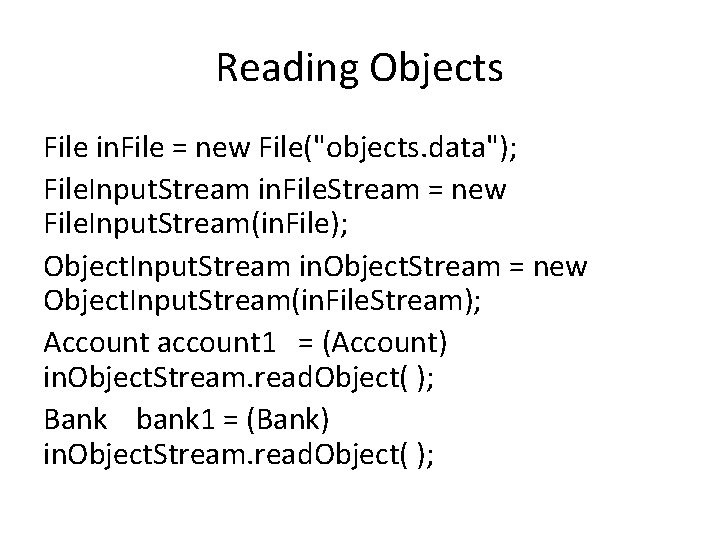
![Writing and Reading Arrays Person[] people = new Person[ N ]; out. Object. Stream. Writing and Reading Arrays Person[] people = new Person[ N ]; out. Object. Stream.](https://slidetodoc.com/presentation_image_h/3121ef977d02dd547b6a280d93960842/image-18.jpg)
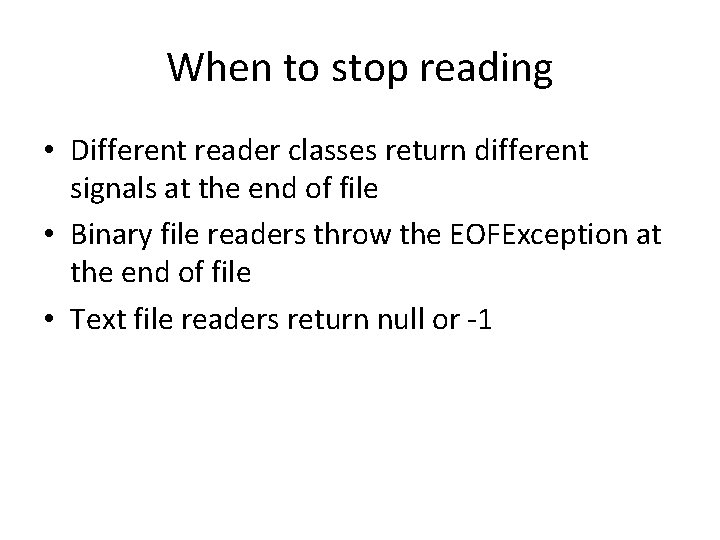
- Slides: 19
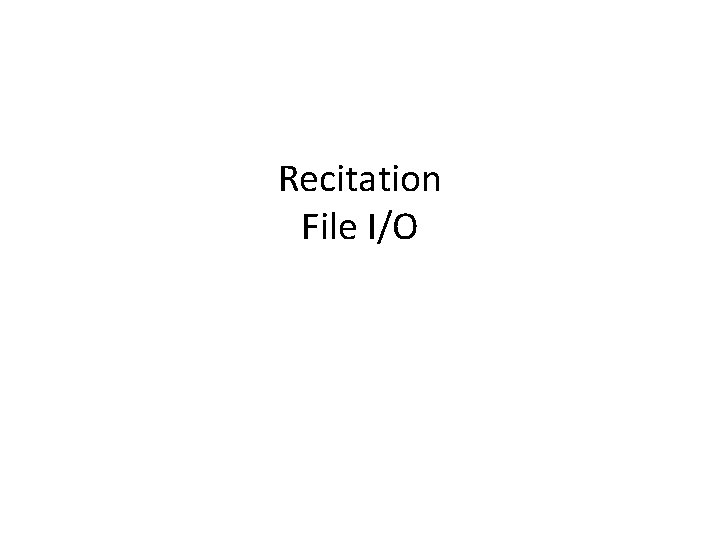
Recitation File I/O
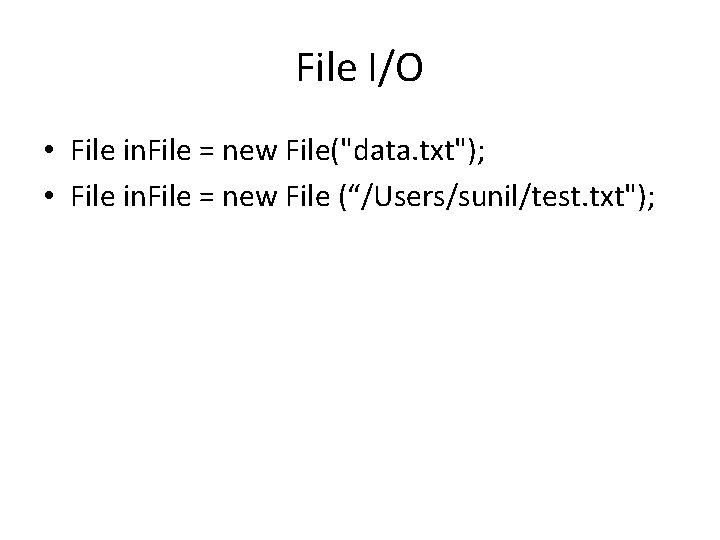
File I/O • File in. File = new File("data. txt"); • File in. File = new File (“/Users/sunil/test. txt");
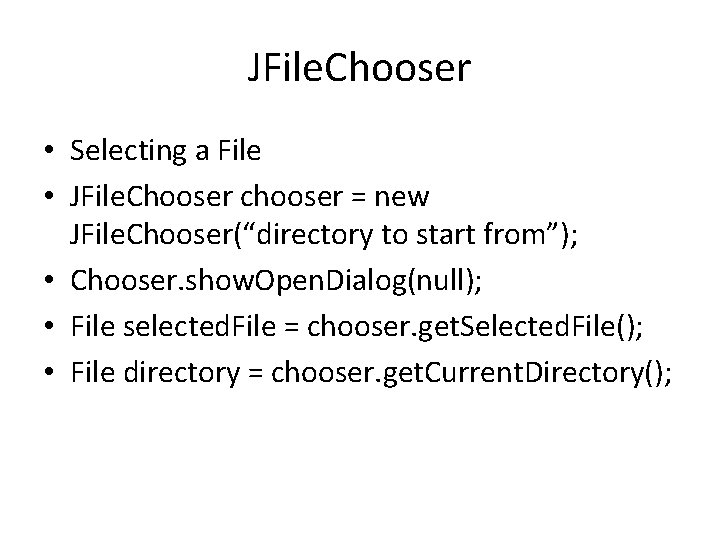
JFile. Chooser • Selecting a File • JFile. Chooser chooser = new JFile. Chooser(“directory to start from”); • Chooser. show. Open. Dialog(null); • File selected. File = chooser. get. Selected. File(); • File directory = chooser. get. Current. Directory();
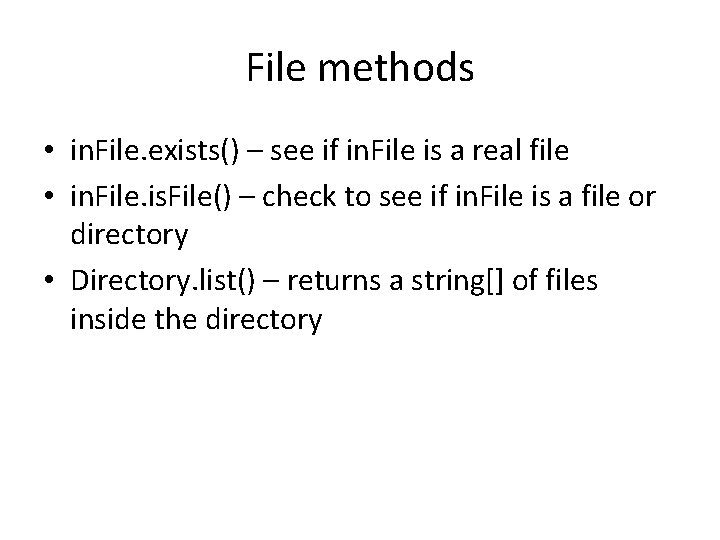
File methods • in. File. exists() – see if in. File is a real file • in. File. is. File() – check to see if in. File is a file or directory • Directory. list() – returns a string[] of files inside the directory
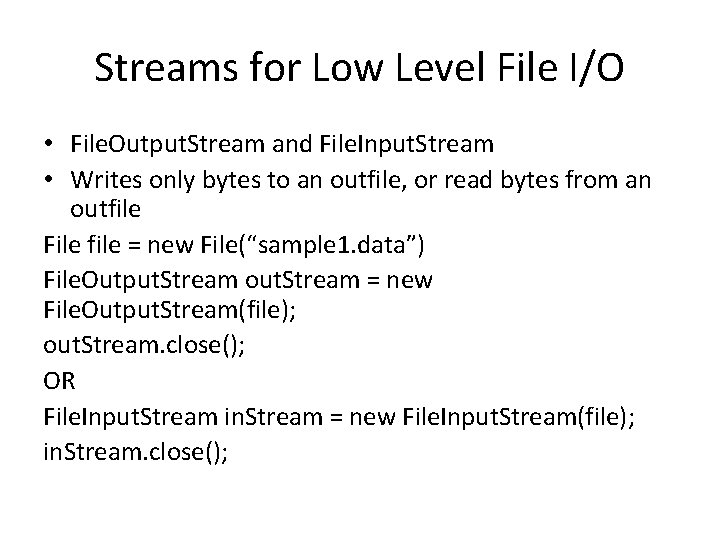
Streams for Low Level File I/O • File. Output. Stream and File. Input. Stream • Writes only bytes to an outfile, or read bytes from an outfile File file = new File(“sample 1. data”) File. Output. Stream out. Stream = new File. Output. Stream(file); out. Stream. close(); OR File. Input. Stream in. Stream = new File. Input. Stream(file); in. Stream. close();
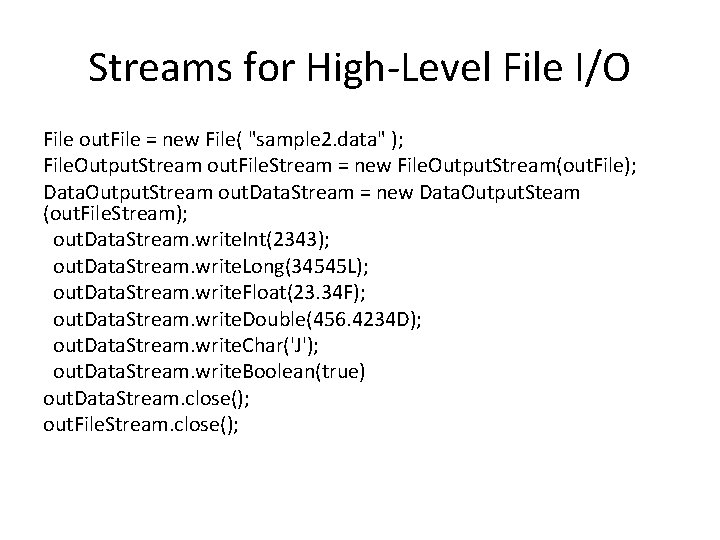
Streams for High-Level File I/O File out. File = new File( "sample 2. data" ); File. Output. Stream out. File. Stream = new File. Output. Stream(out. File); Data. Output. Stream out. Data. Stream = new Data. Output. Steam (out. File. Stream); out. Data. Stream. write. Int(2343); out. Data. Stream. write. Long(34545 L); out. Data. Stream. write. Float(23. 34 F); out. Data. Stream. write. Double(456. 4234 D); out. Data. Stream. write. Char('J'); out. Data. Stream. write. Boolean(true) out. Data. Stream. close(); out. File. Stream. close();
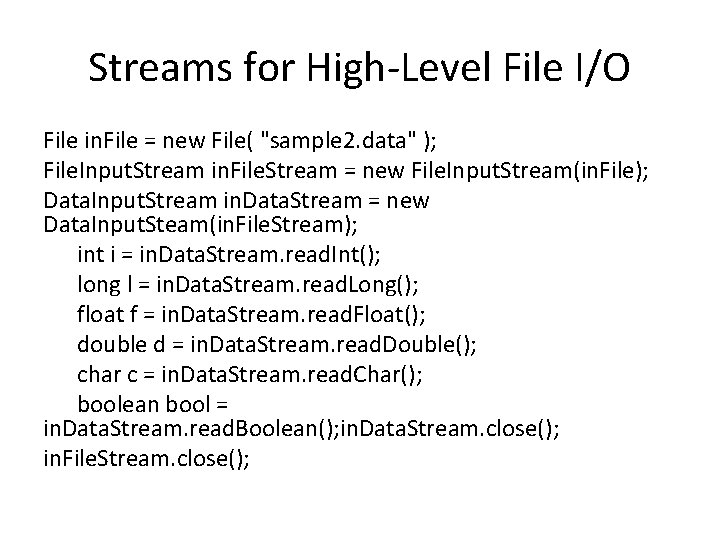
Streams for High-Level File I/O File in. File = new File( "sample 2. data" ); File. Input. Stream in. File. Stream = new File. Input. Stream(in. File); Data. Input. Stream in. Data. Stream = new Data. Input. Steam(in. File. Stream); int i = in. Data. Stream. read. Int(); long l = in. Data. Stream. read. Long(); float f = in. Data. Stream. read. Float(); double d = in. Data. Stream. read. Double(); char c = in. Data. Stream. read. Char(); boolean bool = in. Data. Stream. read. Boolean(); in. Data. Stream. close(); in. File. Stream. close();
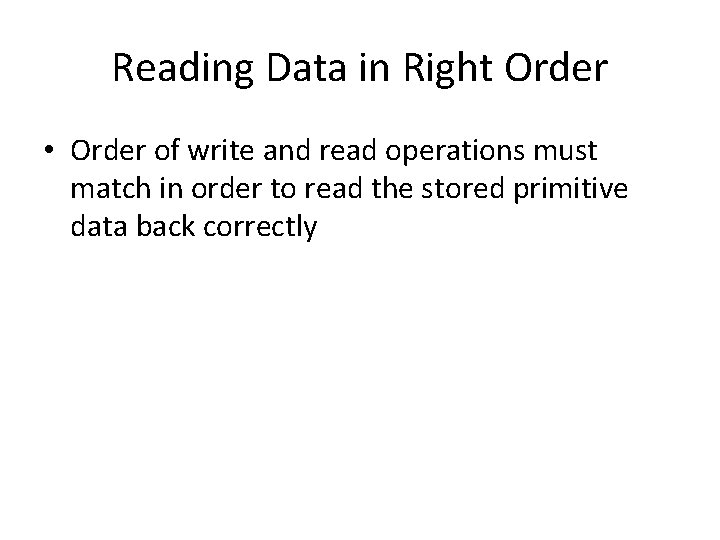
Reading Data in Right Order • Order of write and read operations must match in order to read the stored primitive data back correctly
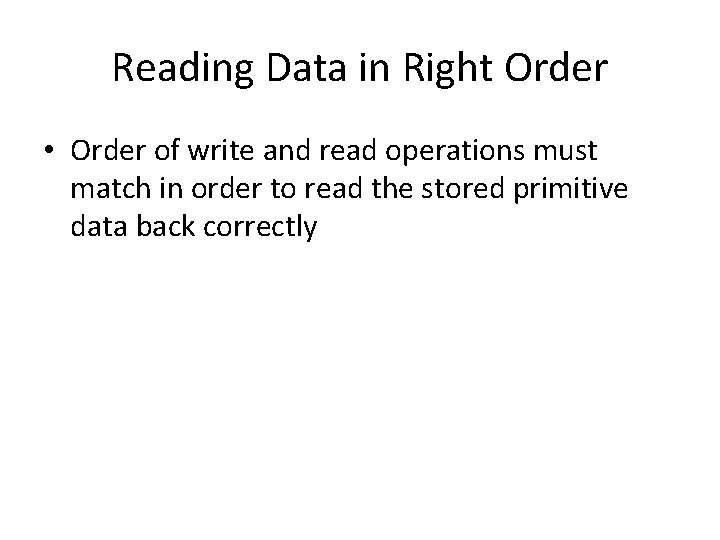
Reading Data in Right Order • Order of write and read operations must match in order to read the stored primitive data back correctly
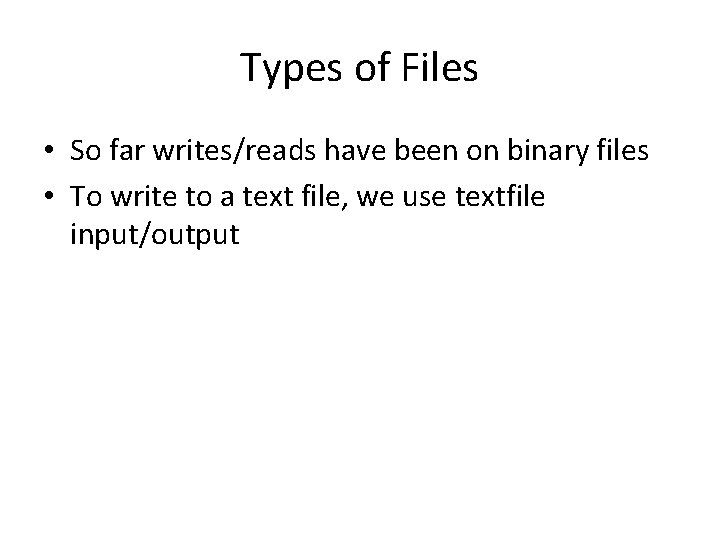
Types of Files • So far writes/reads have been on binary files • To write to a text file, we use textfile input/output
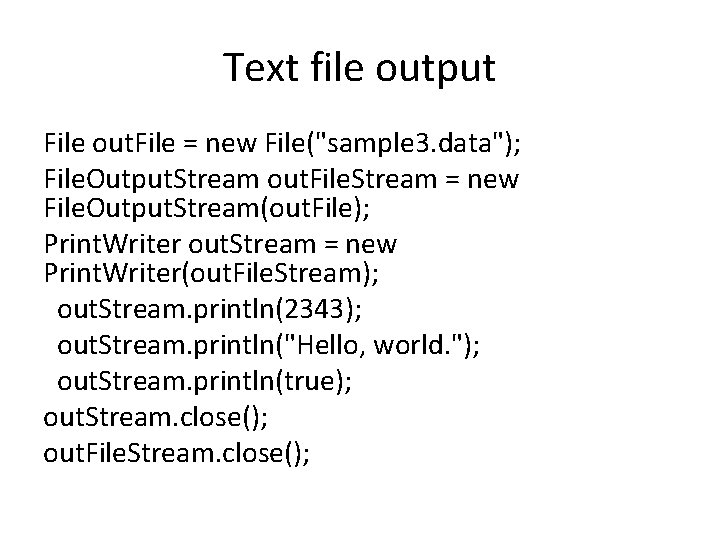
Text file output File out. File = new File("sample 3. data"); File. Output. Stream out. File. Stream = new File. Output. Stream(out. File); Print. Writer out. Stream = new Print. Writer(out. File. Stream); out. Stream. println(2343); out. Stream. println("Hello, world. "); out. Stream. println(true); out. Stream. close(); out. File. Stream. close();
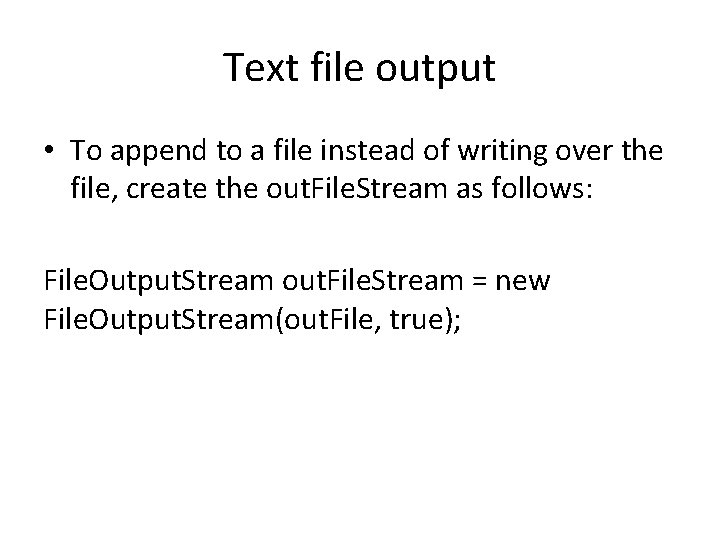
Text file output • To append to a file instead of writing over the file, create the out. File. Stream as follows: File. Output. Stream out. File. Stream = new File. Output. Stream(out. File, true);
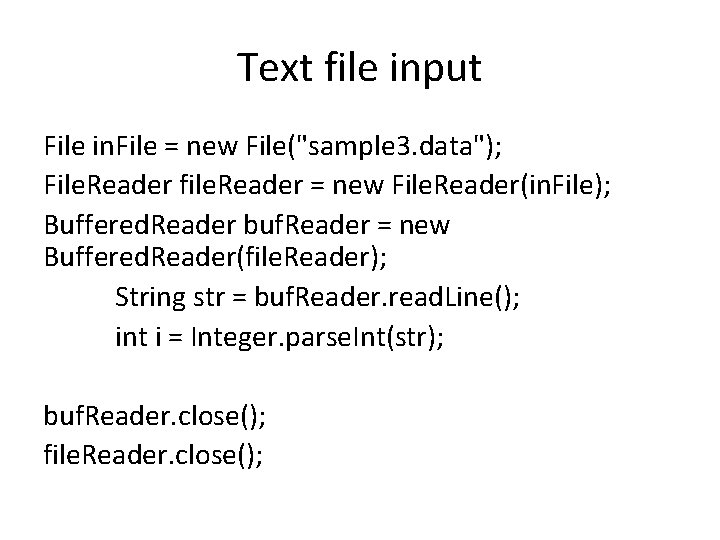
Text file input File in. File = new File("sample 3. data"); File. Reader file. Reader = new File. Reader(in. File); Buffered. Reader buf. Reader = new Buffered. Reader(file. Reader); String str = buf. Reader. read. Line(); int i = Integer. parse. Int(str); buf. Reader. close(); file. Reader. close();
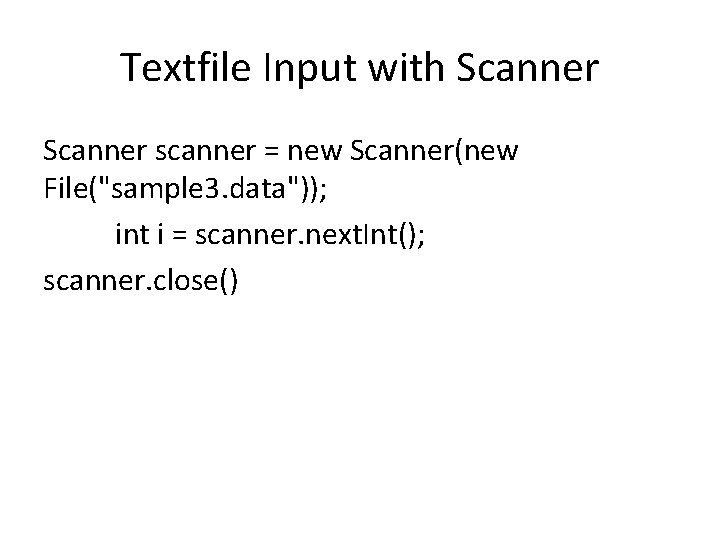
Textfile Input with Scanner scanner = new Scanner(new File("sample 3. data")); int i = scanner. next. Int(); scanner. close()
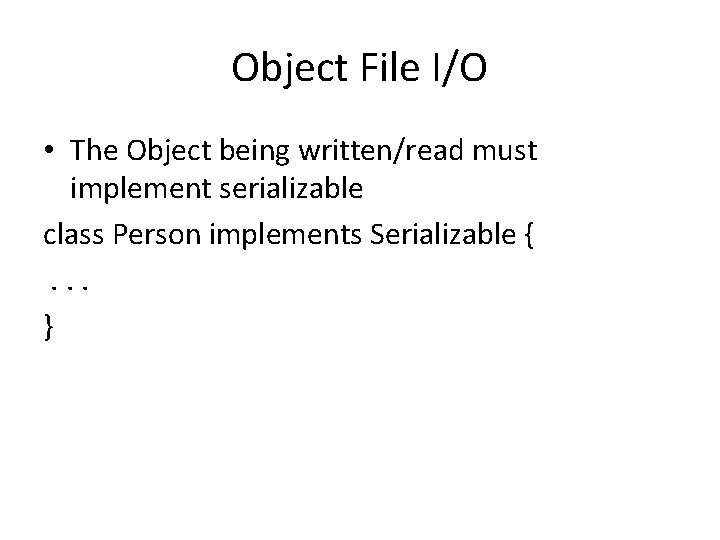
Object File I/O • The Object being written/read must implement serializable class Person implements Serializable {. . . }
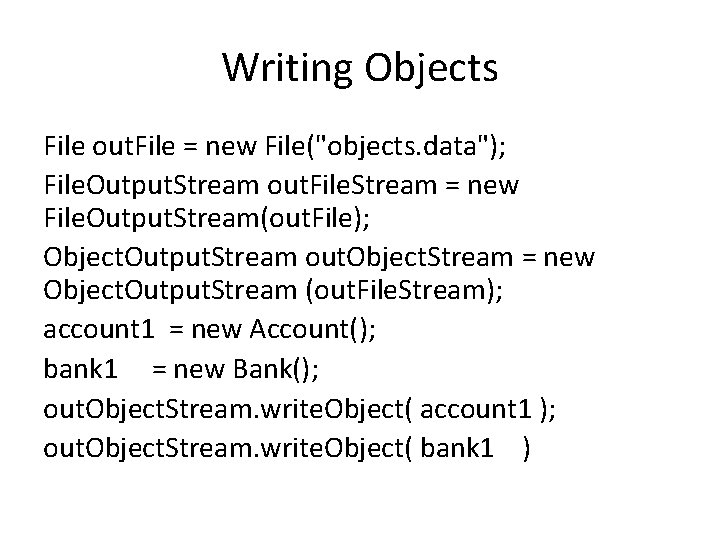
Writing Objects File out. File = new File("objects. data"); File. Output. Stream out. File. Stream = new File. Output. Stream(out. File); Object. Output. Stream out. Object. Stream = new Object. Output. Stream (out. File. Stream); account 1 = new Account(); bank 1 = new Bank(); out. Object. Stream. write. Object( account 1 ); out. Object. Stream. write. Object( bank 1 )
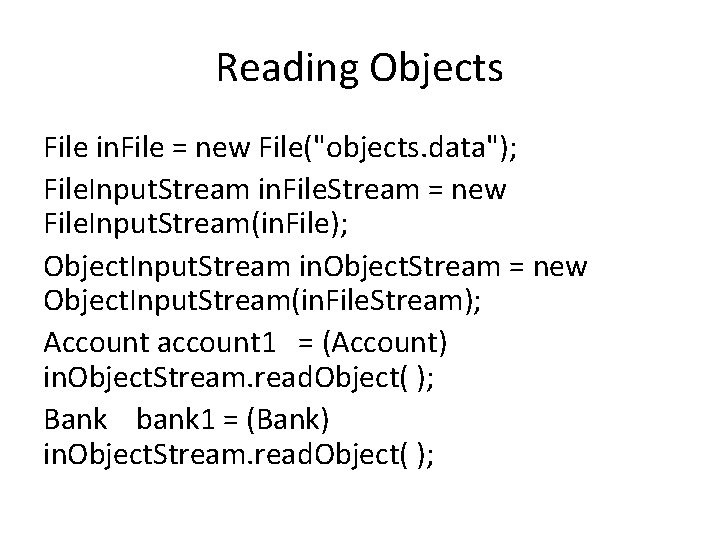
Reading Objects File in. File = new File("objects. data"); File. Input. Stream in. File. Stream = new File. Input. Stream(in. File); Object. Input. Stream in. Object. Stream = new Object. Input. Stream(in. File. Stream); Account account 1 = (Account) in. Object. Stream. read. Object( ); Bank bank 1 = (Bank) in. Object. Stream. read. Object( );
![Writing and Reading Arrays Person people new Person N out Object Stream Writing and Reading Arrays Person[] people = new Person[ N ]; out. Object. Stream.](https://slidetodoc.com/presentation_image_h/3121ef977d02dd547b6a280d93960842/image-18.jpg)
Writing and Reading Arrays Person[] people = new Person[ N ]; out. Object. Stream. write. Object ( people ); Person[ ] people = (Person[]) in. Object. Stream. read. Object ( )
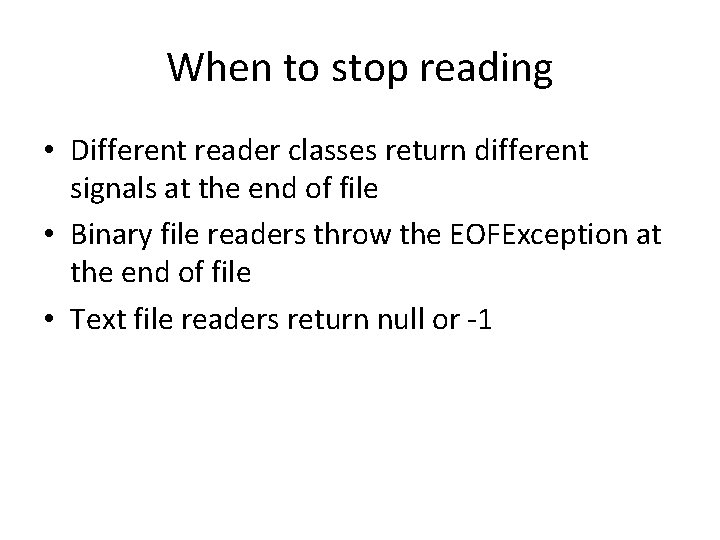
When to stop reading • Different reader classes return different signals at the end of file • Binary file readers throw the EOFException at the end of file • Text file readers return null or -1
Récitation les hiboux
Quood posture 8 in english
What is meant by etiquette of recitation of the holy quran
Impromptu speaking business
Recitation les machines
Objectives of poem recitation
Performance rubrics criteria
Active recitation
Expressive poems for recitation
File-file yang dibuat oleh user pada jenis file di linux
Distributed file system
An html file is a text file containing small markup tags
Physical image vs logical image
In a file-oriented information system, a transaction file
Fungsi dari create file pada operasi-operasi file (cont.)
Avi file format advantages and disadvantages
Ps0310 file not found
An advantage of a batch general ledger system (gls) is that
Outline steps to be taken when creating a sequential file
Physical register file