Sorting and Complexity Sorting is to arrange an
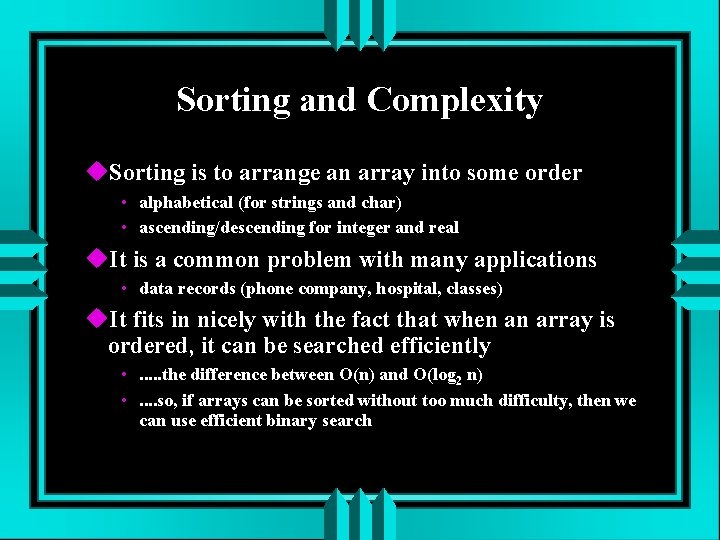
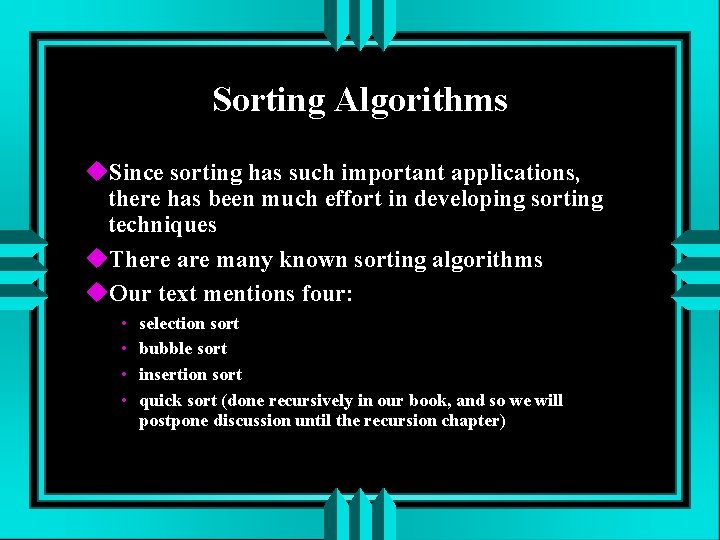
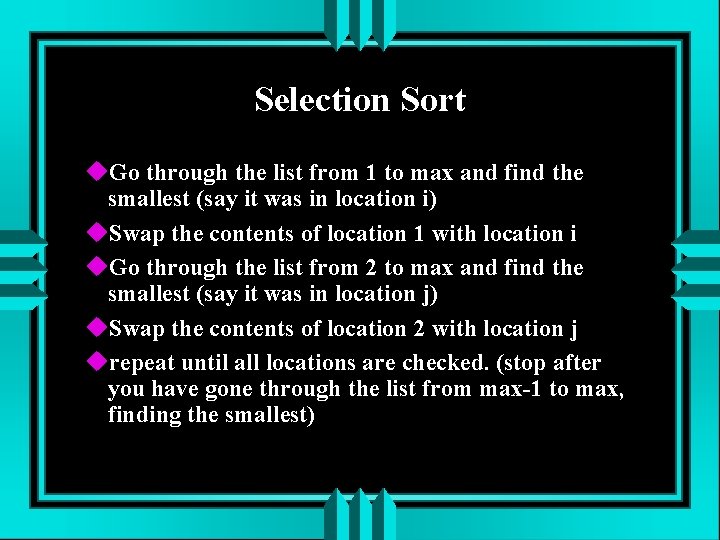
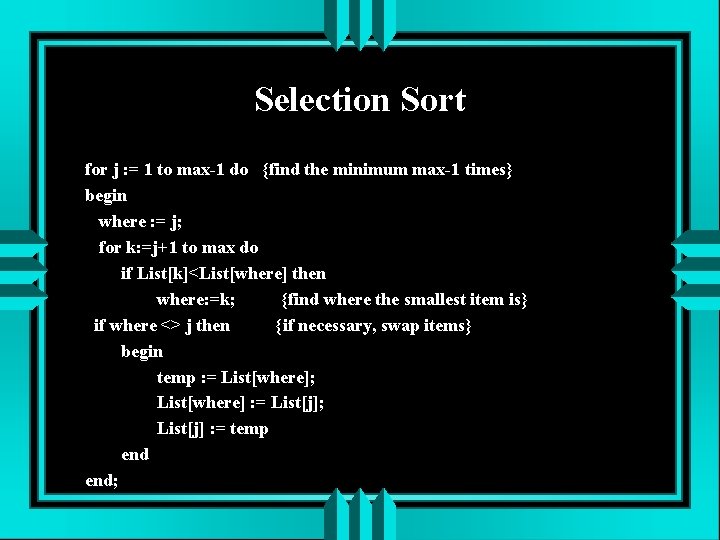
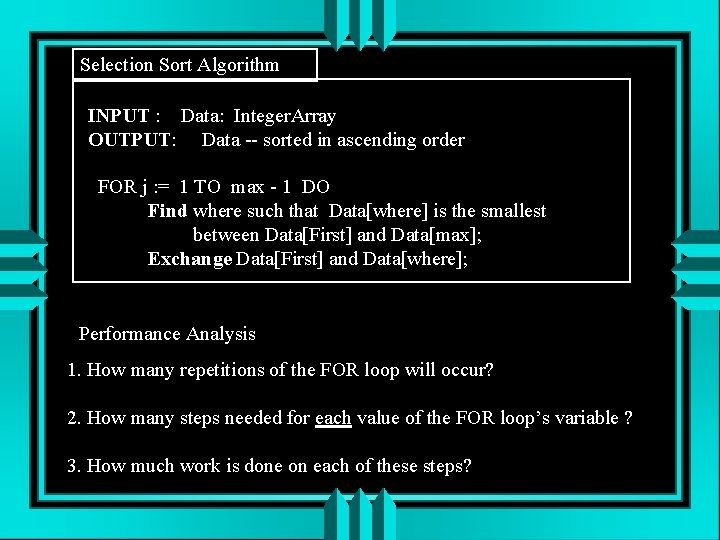
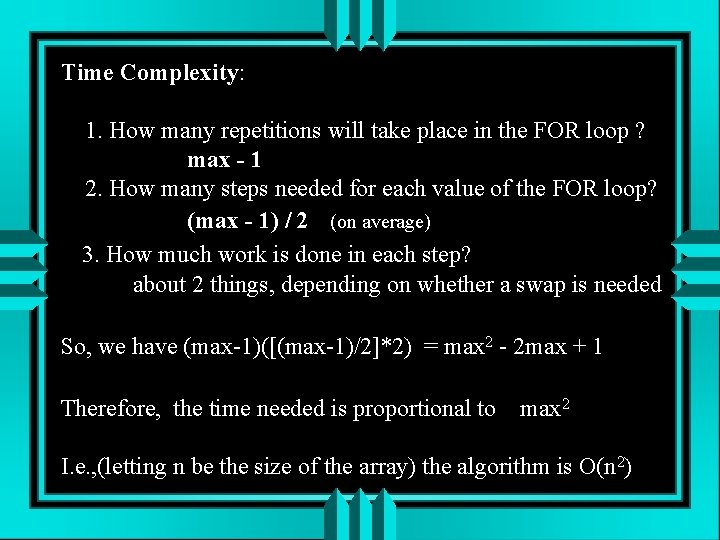
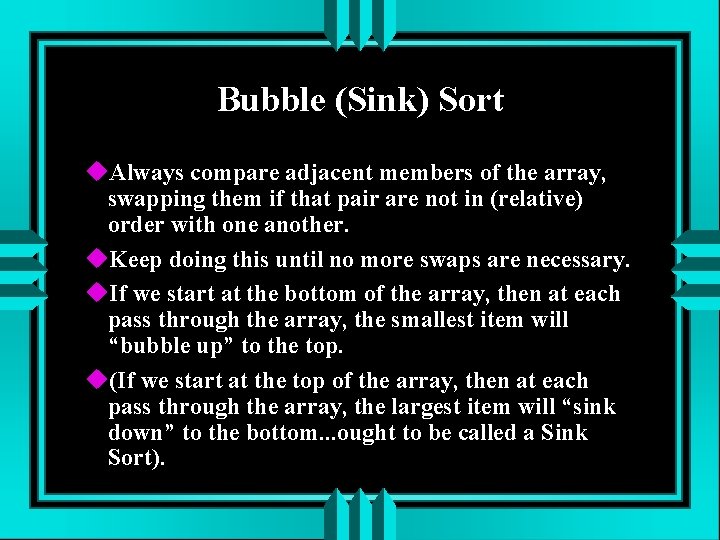
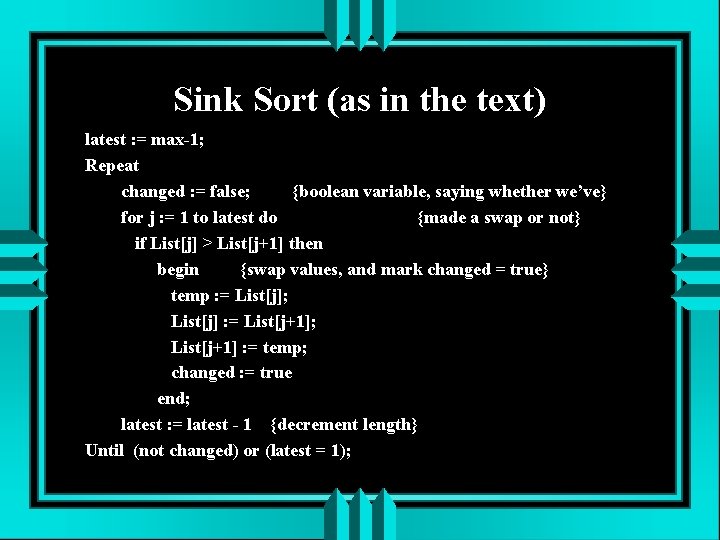
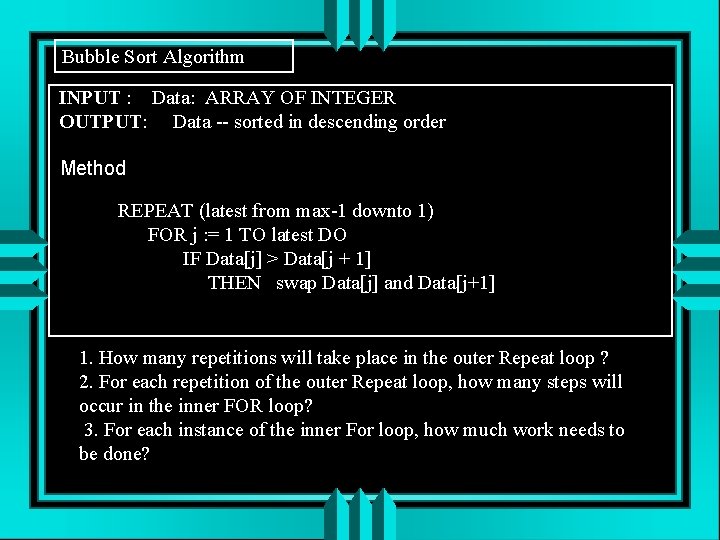
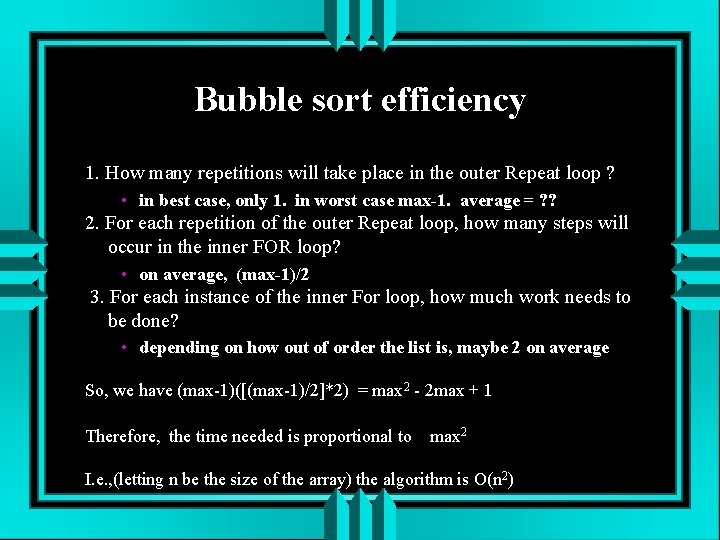
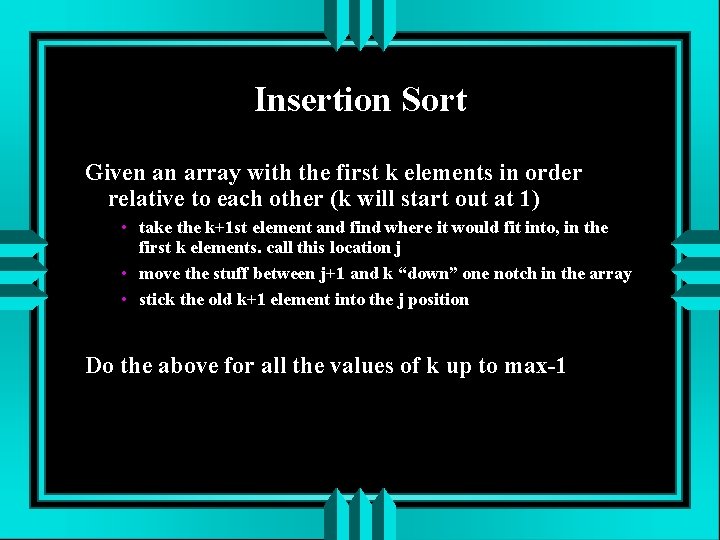
![Insertion Sort For newguy : = 2 to max do begin temp: =List[newguy]; k: Insertion Sort For newguy : = 2 to max do begin temp: =List[newguy]; k:](https://slidetodoc.com/presentation_image_h2/6862bb24682445b91e785af4d870b163/image-12.jpg)
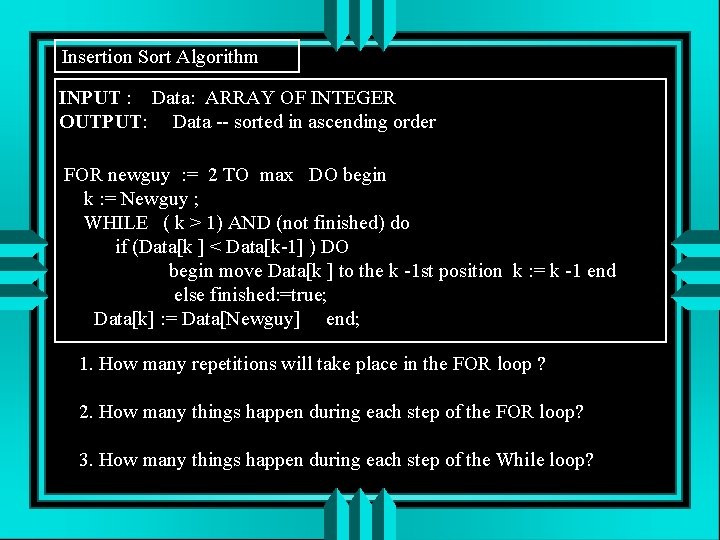
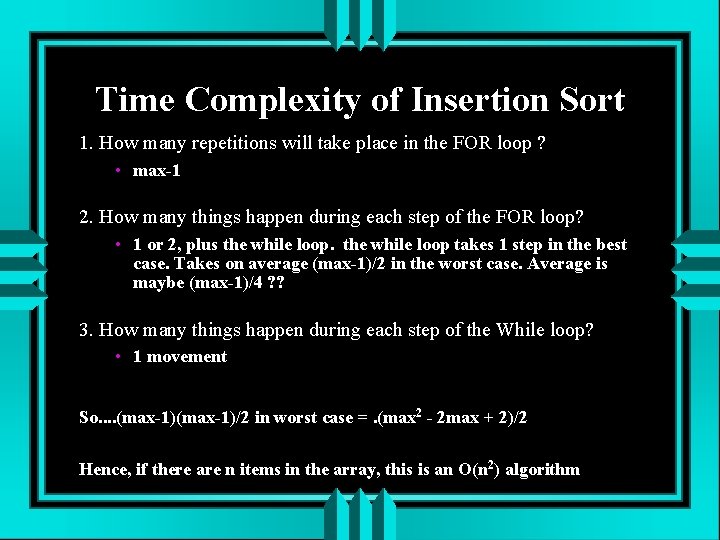
- Slides: 14
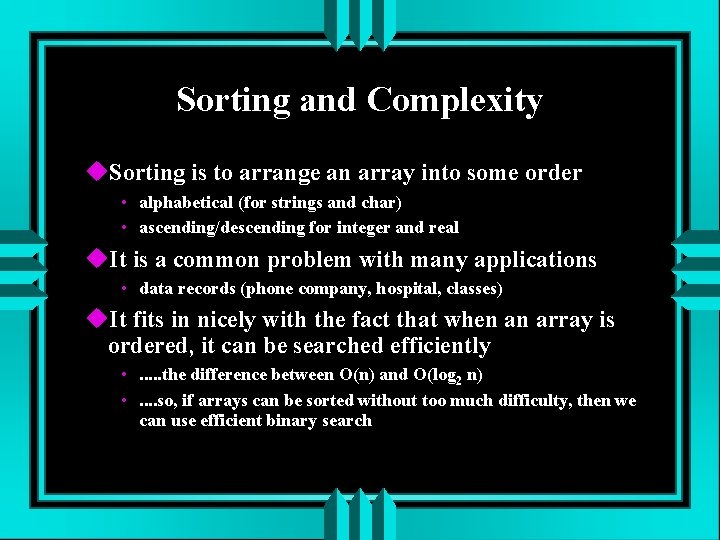
Sorting and Complexity Sorting is to arrange an array into some order • alphabetical (for strings and char) • ascending/descending for integer and real It is a common problem with many applications • data records (phone company, hospital, classes) It fits in nicely with the fact that when an array is ordered, it can be searched efficiently • . . . the difference between O(n) and O(log 2 n) • . . so, if arrays can be sorted without too much difficulty, then we can use efficient binary search
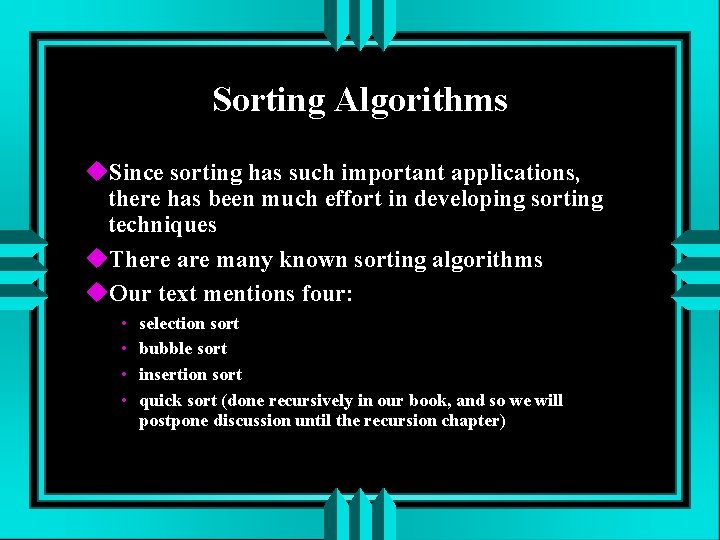
Sorting Algorithms Since sorting has such important applications, there has been much effort in developing sorting techniques There are many known sorting algorithms Our text mentions four: • • selection sort bubble sort insertion sort quick sort (done recursively in our book, and so we will postpone discussion until the recursion chapter)
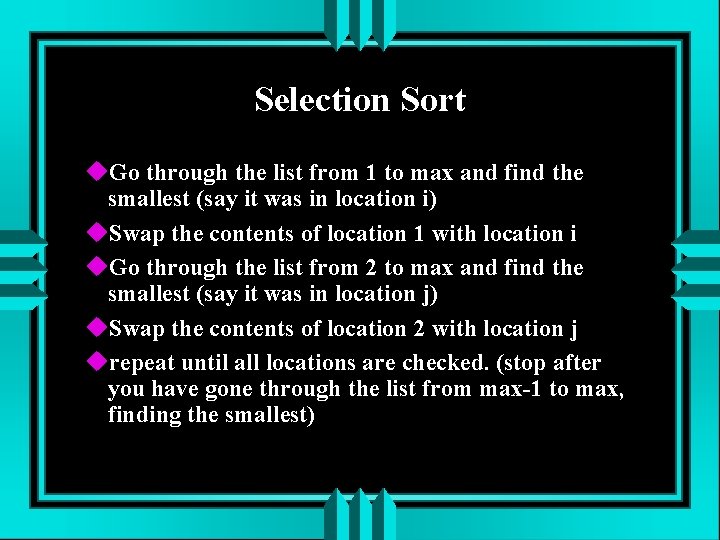
Selection Sort Go through the list from 1 to max and find the smallest (say it was in location i) Swap the contents of location 1 with location i Go through the list from 2 to max and find the smallest (say it was in location j) Swap the contents of location 2 with location j repeat until all locations are checked. (stop after you have gone through the list from max-1 to max, finding the smallest)
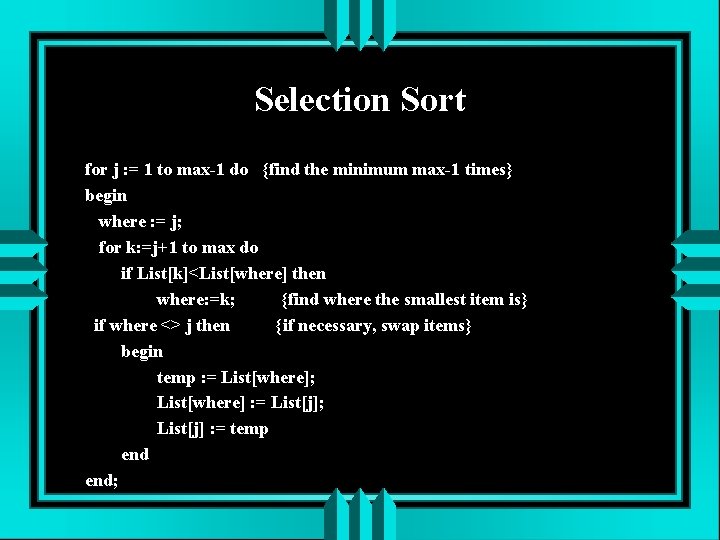
Selection Sort for j : = 1 to max-1 do {find the minimum max-1 times} begin where : = j; for k: =j+1 to max do if List[k]<List[where] then where: =k; {find where the smallest item is} if where <> j then {if necessary, swap items} begin temp : = List[where]; List[where] : = List[j]; List[j] : = temp end;
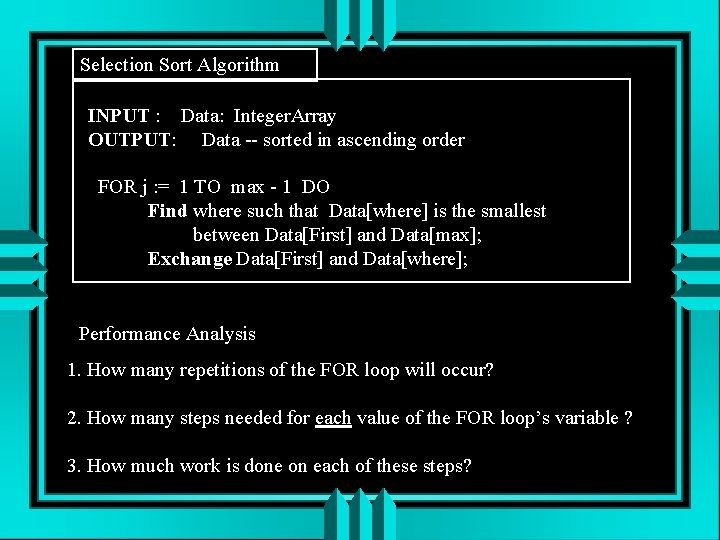
Selection Sort Algorithm INPUT : Data: Integer. Array OUTPUT: Data -- sorted in ascending order FOR j : = 1 TO max - 1 DO Find where such that Data[where] is the smallest between Data[First] and Data[max]; Exchange Data[First] and Data[where]; Performance Analysis 1. How many repetitions of the FOR loop will occur? 2. How many steps needed for each value of the FOR loop’s variable ? 3. How much work is done on each of these steps?
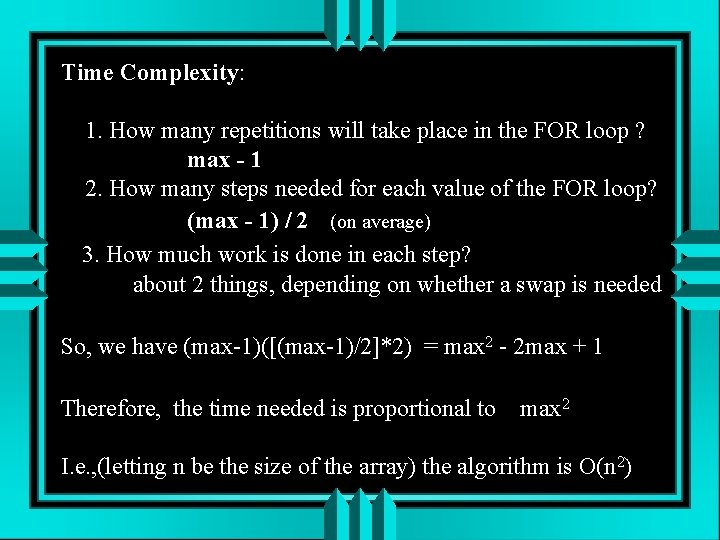
Time Complexity: 1. How many repetitions will take place in the FOR loop ? max - 1 2. How many steps needed for each value of the FOR loop? (max - 1) / 2 (on average) 3. How much work is done in each step? about 2 things, depending on whether a swap is needed So, we have (max-1)([(max-1)/2]*2) = max 2 - 2 max + 1 Therefore, the time needed is proportional to max 2 I. e. , (letting n be the size of the array) the algorithm is O(n 2)
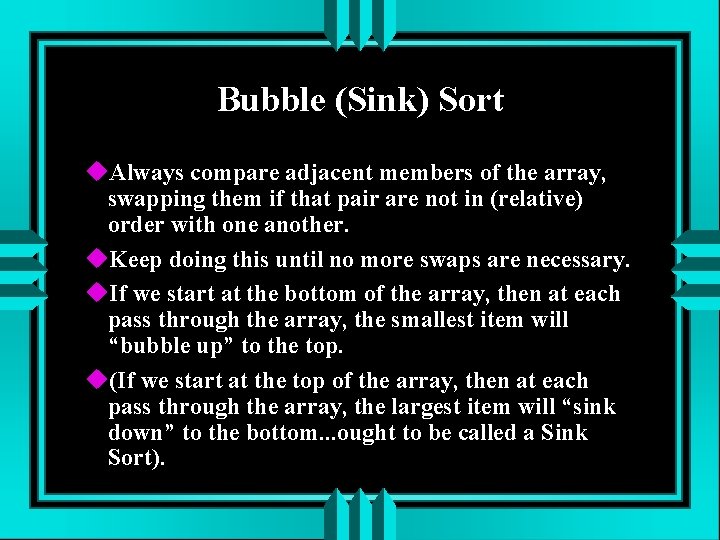
Bubble (Sink) Sort Always compare adjacent members of the array, swapping them if that pair are not in (relative) order with one another. Keep doing this until no more swaps are necessary. If we start at the bottom of the array, then at each pass through the array, the smallest item will “bubble up” to the top. (If we start at the top of the array, then at each pass through the array, the largest item will “sink down” to the bottom. . . ought to be called a Sink Sort).
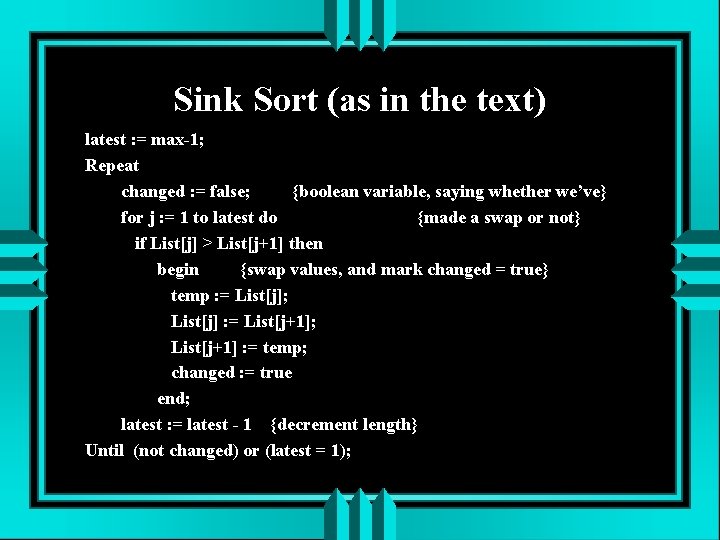
Sink Sort (as in the text) latest : = max-1; Repeat changed : = false; {boolean variable, saying whether we’ve} for j : = 1 to latest do {made a swap or not} if List[j] > List[j+1] then begin {swap values, and mark changed = true} temp : = List[j]; List[j] : = List[j+1]; List[j+1] : = temp; changed : = true end; latest : = latest - 1 {decrement length} Until (not changed) or (latest = 1);
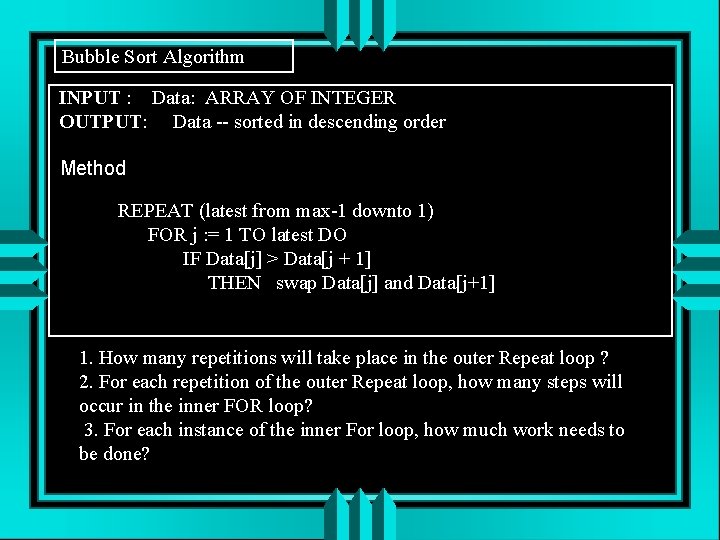
Bubble Sort Algorithm INPUT : Data: ARRAY OF INTEGER OUTPUT: Data -- sorted in descending order Method REPEAT (latest from max-1 downto 1) FOR j : = 1 TO latest DO IF Data[j] > Data[j + 1] THEN swap Data[j] and Data[j+1] 1. How many repetitions will take place in the outer Repeat loop ? 2. For each repetition of the outer Repeat loop, how many steps will occur in the inner FOR loop? 3. For each instance of the inner For loop, how much work needs to be done?
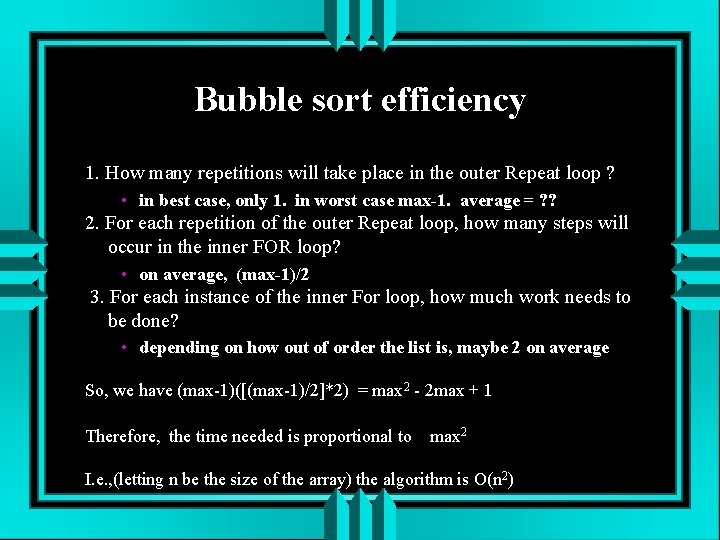
Bubble sort efficiency 1. How many repetitions will take place in the outer Repeat loop ? • in best case, only 1. in worst case max-1. average = ? ? 2. For each repetition of the outer Repeat loop, how many steps will occur in the inner FOR loop? • on average, (max-1)/2 3. For each instance of the inner For loop, how much work needs to be done? • depending on how out of order the list is, maybe 2 on average So, we have (max-1)([(max-1)/2]*2) = max 2 - 2 max + 1 Therefore, the time needed is proportional to max 2 I. e. , (letting n be the size of the array) the algorithm is O(n 2)
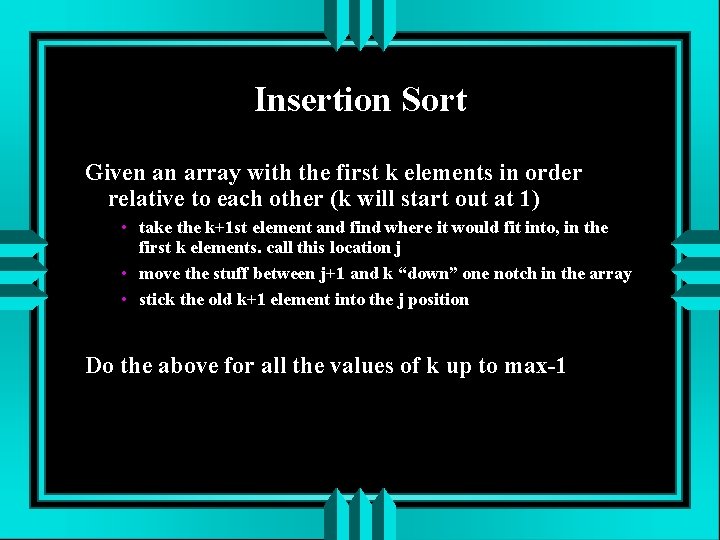
Insertion Sort Given an array with the first k elements in order relative to each other (k will start out at 1) • take the k+1 st element and find where it would fit into, in the first k elements. call this location j • move the stuff between j+1 and k “down” one notch in the array • stick the old k+1 element into the j position Do the above for all the values of k up to max-1
![Insertion Sort For newguy 2 to max do begin temp Listnewguy k Insertion Sort For newguy : = 2 to max do begin temp: =List[newguy]; k:](https://slidetodoc.com/presentation_image_h2/6862bb24682445b91e785af4d870b163/image-12.jpg)
Insertion Sort For newguy : = 2 to max do begin temp: =List[newguy]; k: =newguy; finished: =false; {a boolean to say whether we’re finished} While (k>1) and (not finished) do { looking where to put} if temp < List[k-1] then {the newguy} begin List[k]: =List[k-1]; k : = k-1 end else finished: = true; {we foundwhere to put the newguy} List[k] : = temp end;
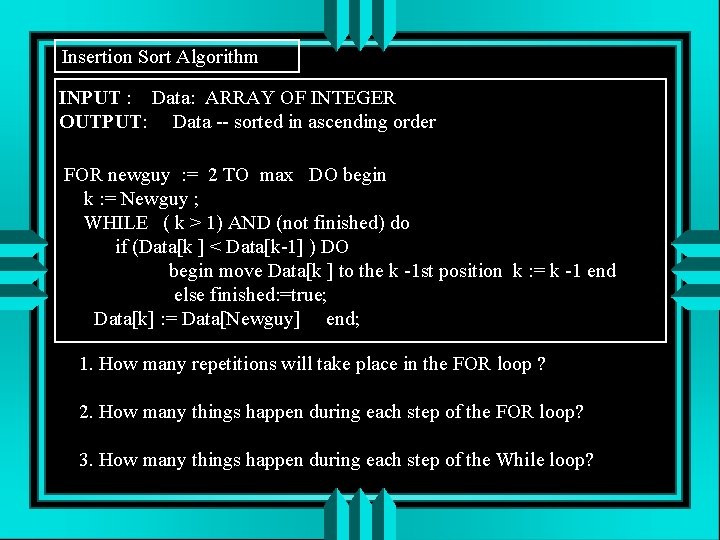
Insertion Sort Algorithm INPUT : Data: ARRAY OF INTEGER OUTPUT: Data -- sorted in ascending order FOR newguy : = 2 TO max DO begin k : = Newguy ; WHILE ( k > 1) AND (not finished) do if (Data[k ] < Data[k-1] ) DO begin move Data[k ] to the k -1 st position k : = k -1 end else finished: =true; Data[k] : = Data[Newguy] end; 1. How many repetitions will take place in the FOR loop ? 2. How many things happen during each step of the FOR loop? 3. How many things happen during each step of the While loop?
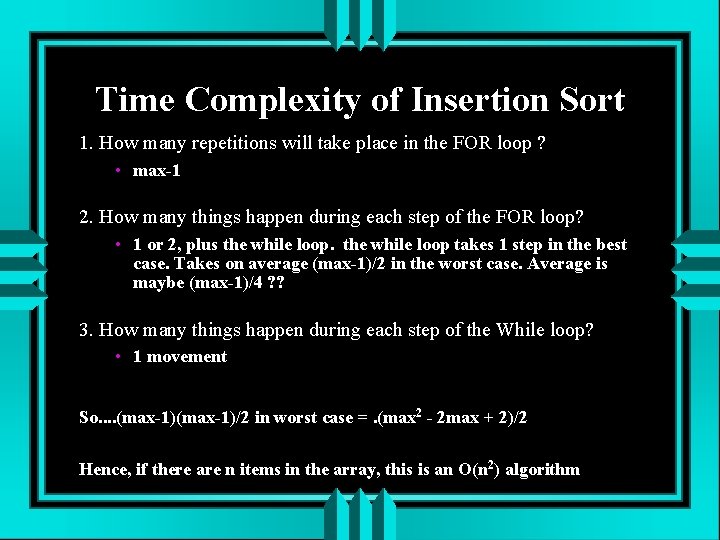
Time Complexity of Insertion Sort 1. How many repetitions will take place in the FOR loop ? • max-1 2. How many things happen during each step of the FOR loop? • 1 or 2, plus the while loop takes 1 step in the best case. Takes on average (max-1)/2 in the worst case. Average is maybe (max-1)/4 ? ? 3. How many things happen during each step of the While loop? • 1 movement So. . (max-1)/2 in worst case =. (max 2 - 2 max + 2)/2 Hence, if there are n items in the array, this is an O(n 2) algorithm
Internal vs external sorting
For loop space complexity
Simplify4−2m2+4m2+1−3m+3−m
Arranged marriage disadvantage
Space complexity bfs
The kaplan model
Language ne demek
Spin glasses and complexity
Depth and complexity icons patterns
Depth and complexity prompts
Linear search average case
Depending on the incident size and complexity
Robert venturi complexity and contradiction in architecture
Kaplan's icons
Divide and conquer complexity