Reference Details copying comparing References You were introduced
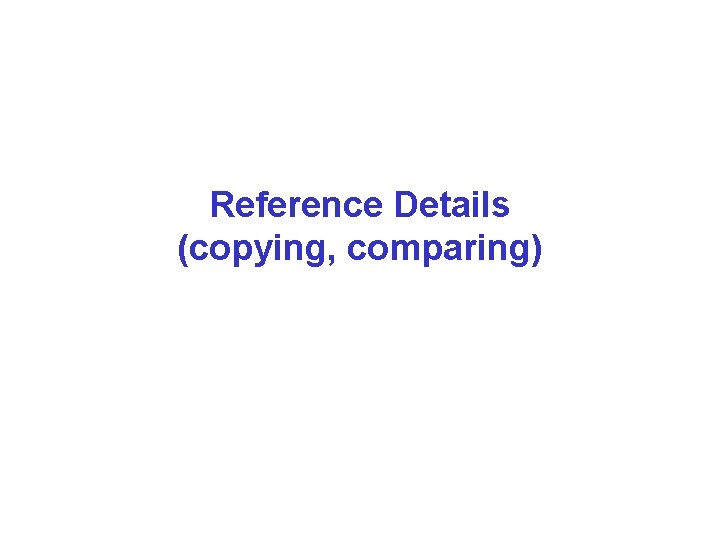
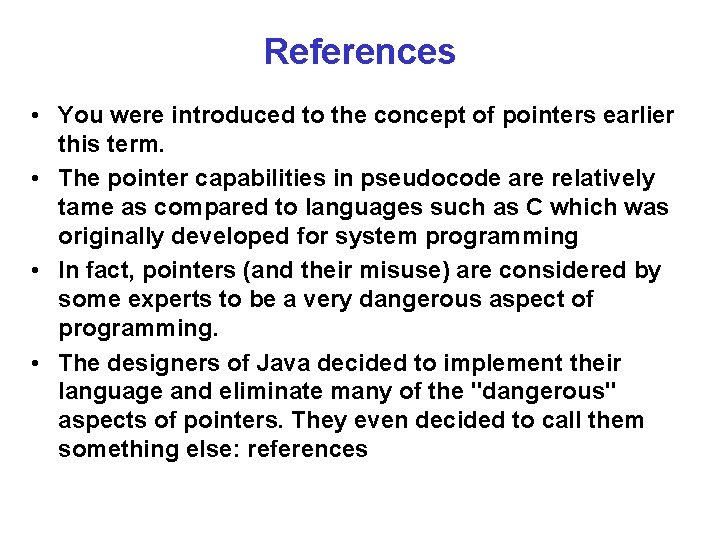
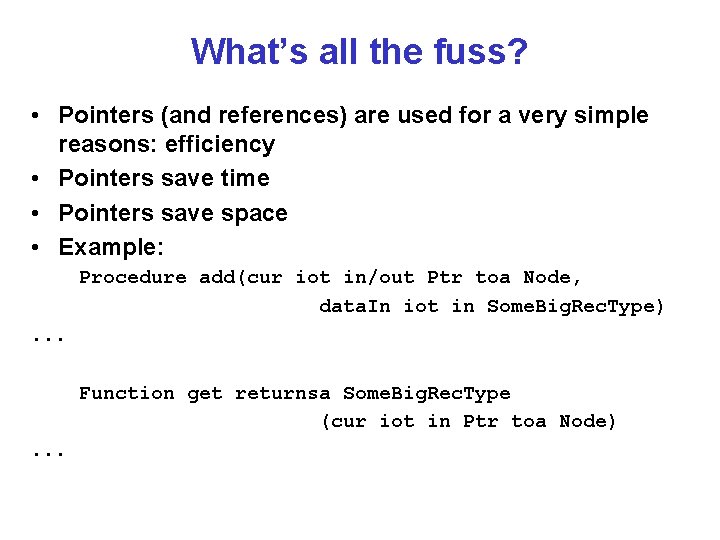
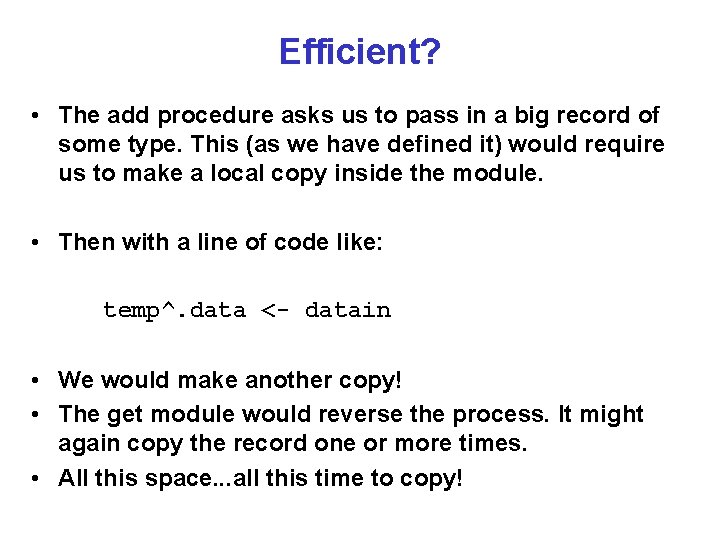
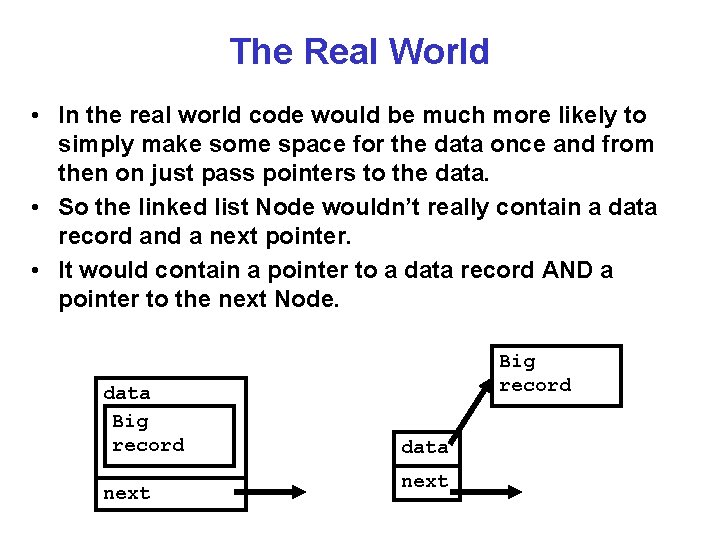
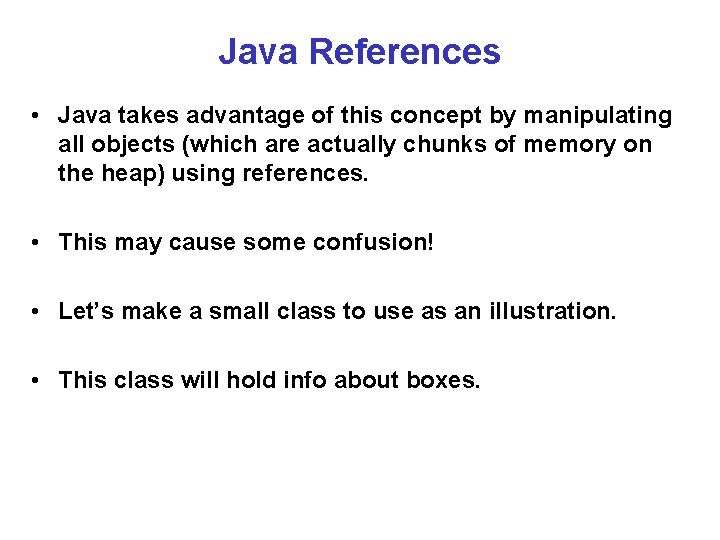
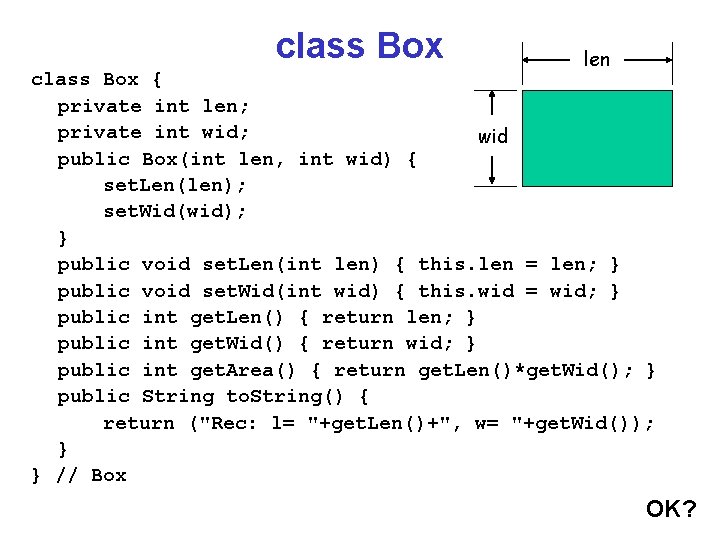
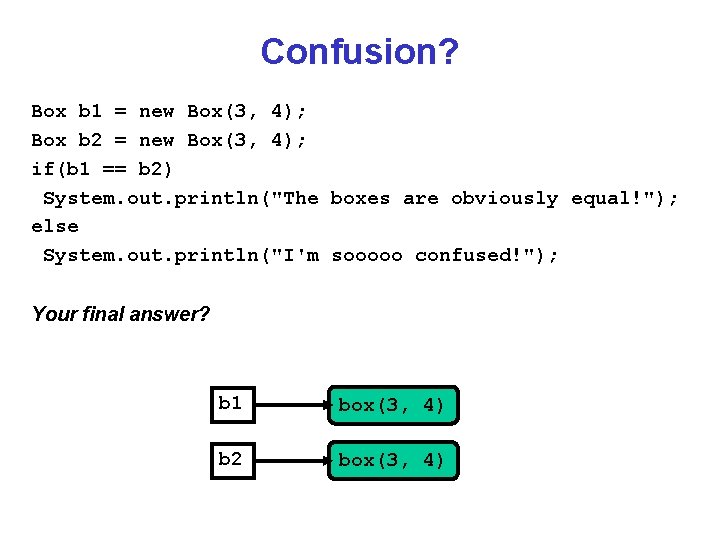
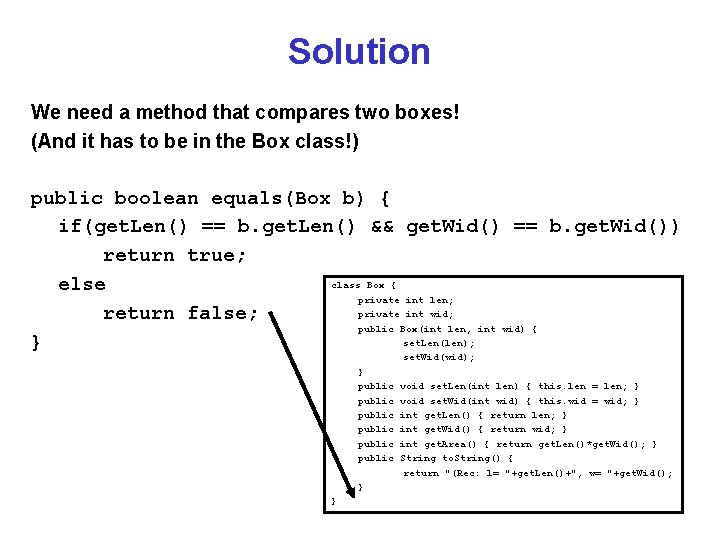
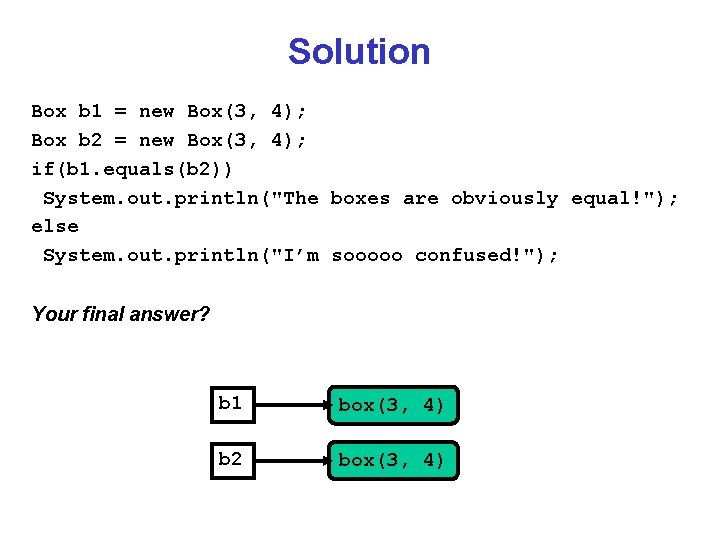
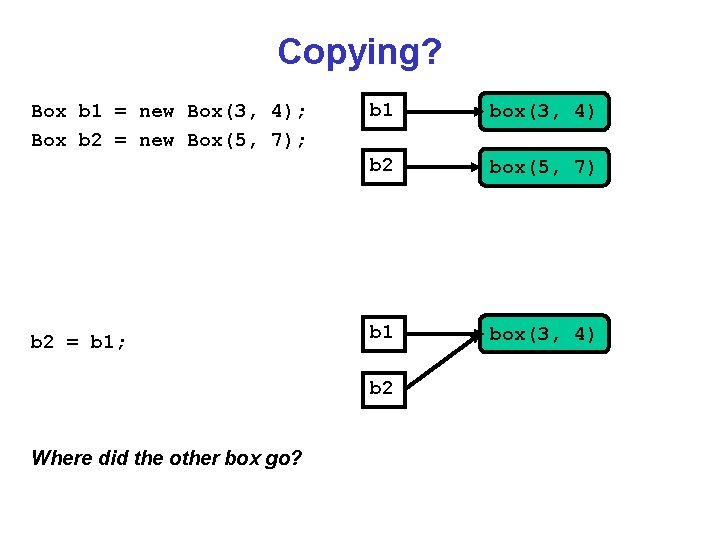
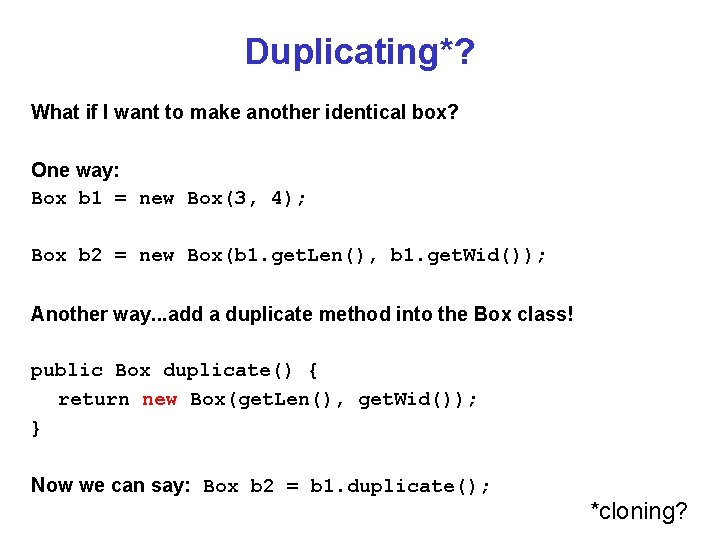
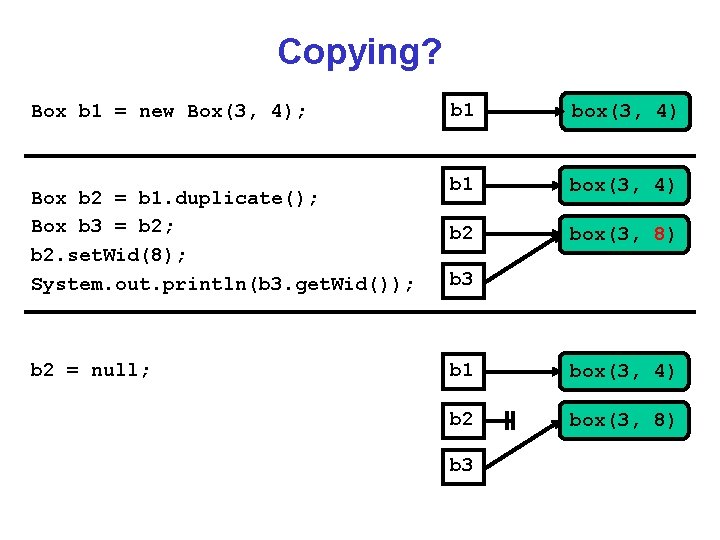
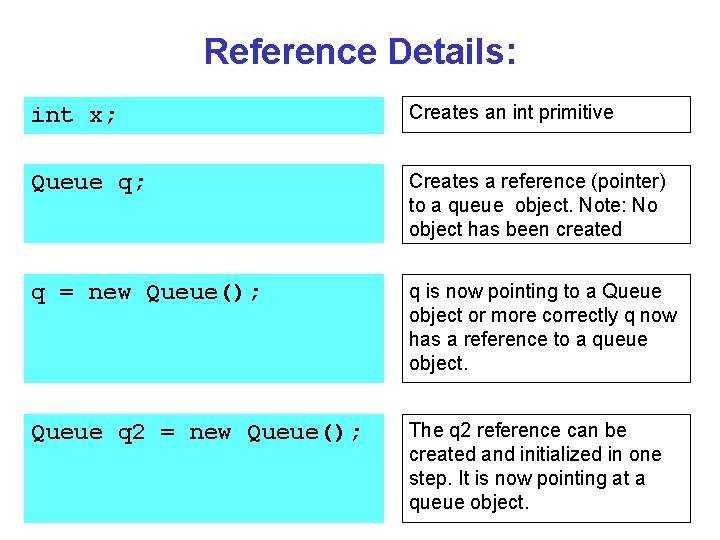
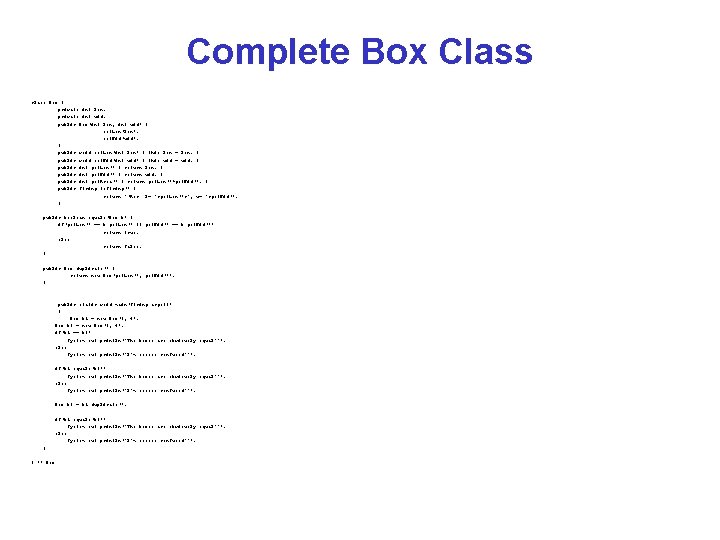
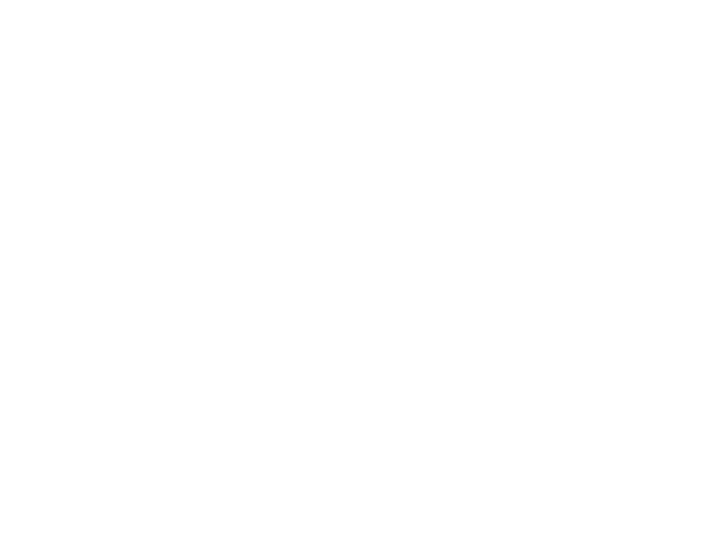
- Slides: 16
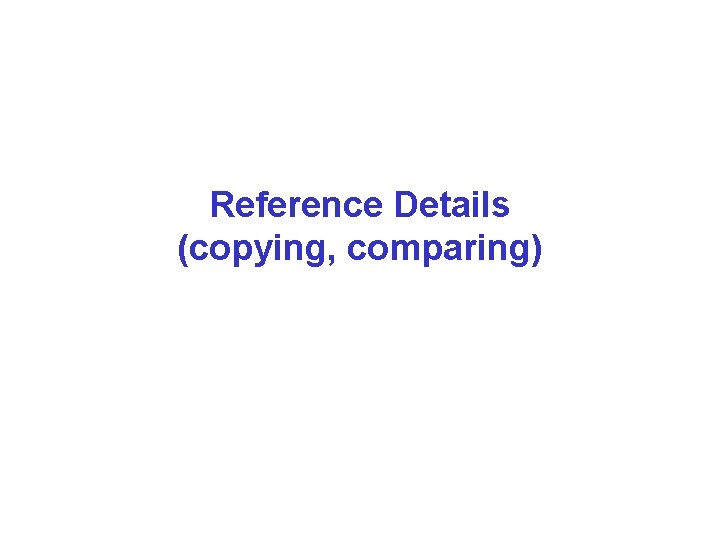
Reference Details (copying, comparing)
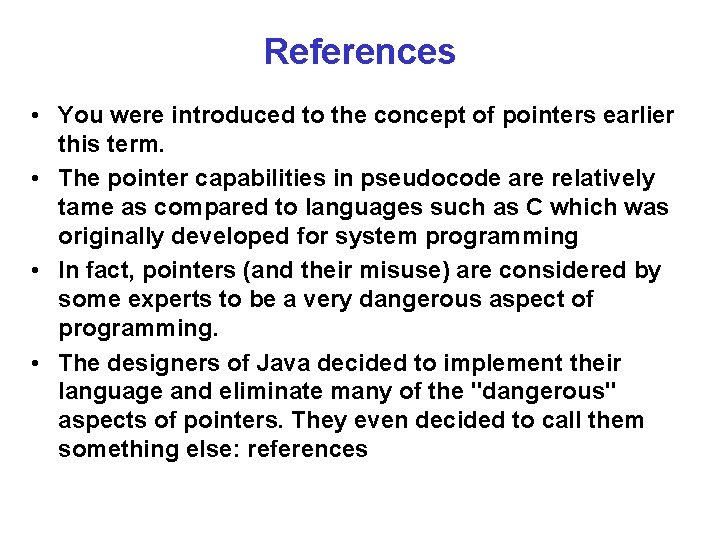
References • You were introduced to the concept of pointers earlier this term. • The pointer capabilities in pseudocode are relatively tame as compared to languages such as C which was originally developed for system programming • In fact, pointers (and their misuse) are considered by some experts to be a very dangerous aspect of programming. • The designers of Java decided to implement their language and eliminate many of the "dangerous" aspects of pointers. They even decided to call them something else: references
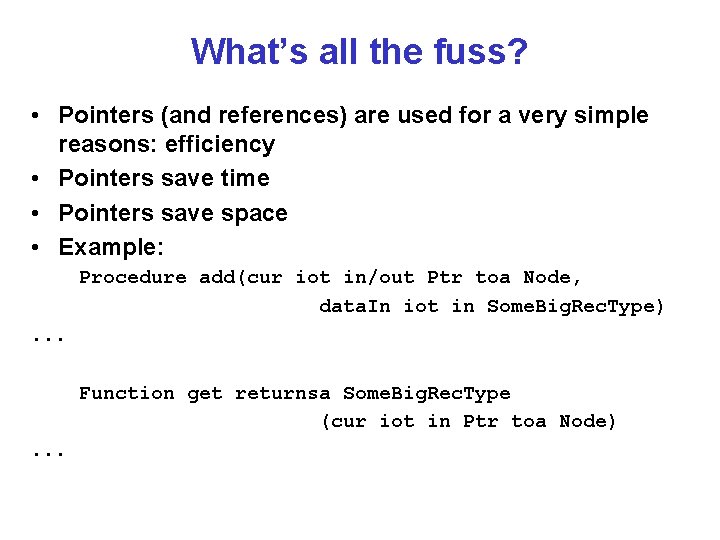
What’s all the fuss? • Pointers (and references) are used for a very simple reasons: efficiency • Pointers save time • Pointers save space • Example: Procedure add(cur iot in/out Ptr toa Node, data. In iot in Some. Big. Rec. Type). . . Function get returnsa Some. Big. Rec. Type (cur iot in Ptr toa Node). . .
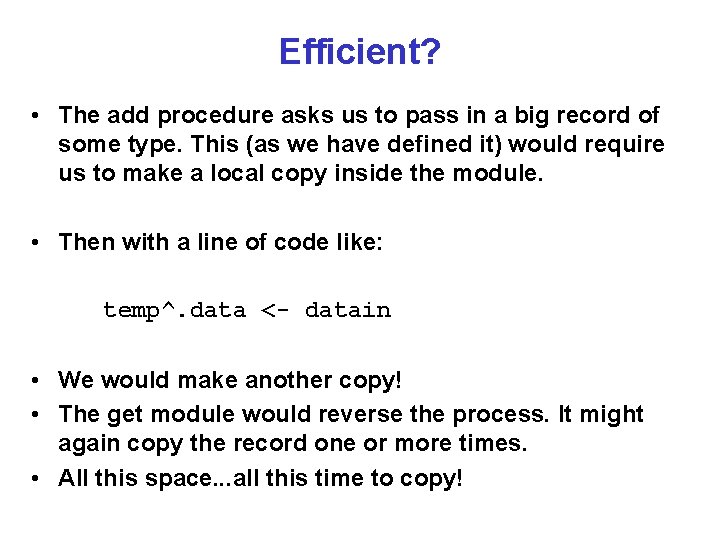
Efficient? • The add procedure asks us to pass in a big record of some type. This (as we have defined it) would require us to make a local copy inside the module. • Then with a line of code like: temp^. data <- datain • We would make another copy! • The get module would reverse the process. It might again copy the record one or more times. • All this space. . . all this time to copy!
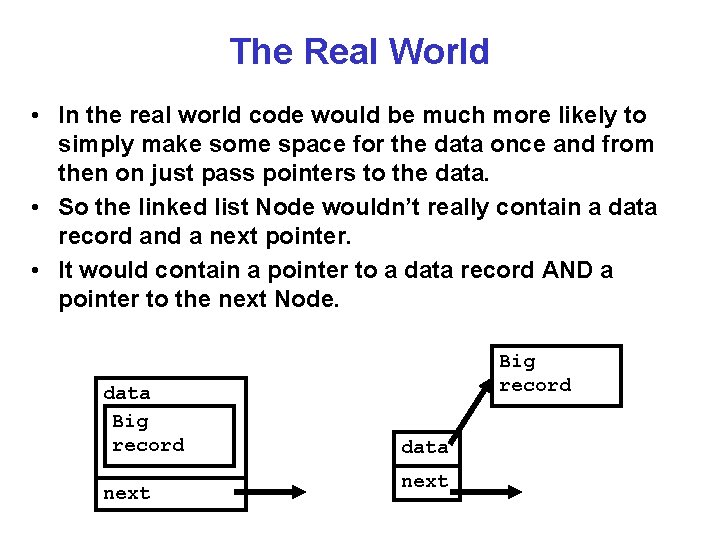
The Real World • In the real world code would be much more likely to simply make some space for the data once and from then on just pass pointers to the data. • So the linked list Node wouldn’t really contain a data record and a next pointer. • It would contain a pointer to a data record AND a pointer to the next Node. data Big record next Big record data next
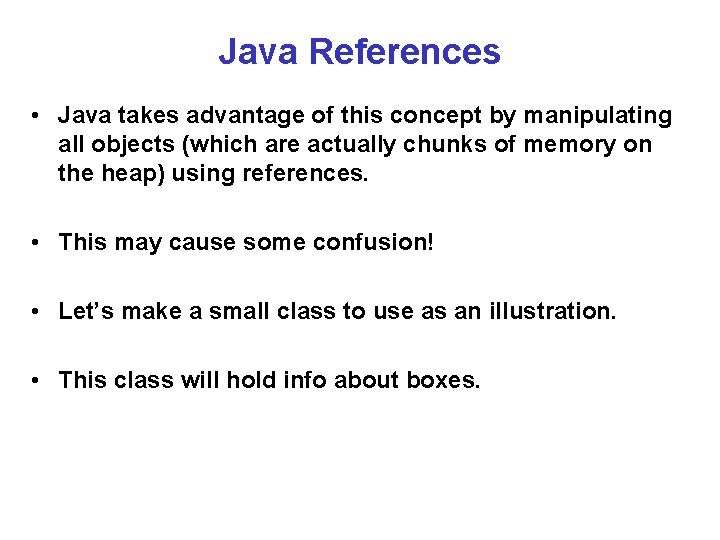
Java References • Java takes advantage of this concept by manipulating all objects (which are actually chunks of memory on the heap) using references. • This may cause some confusion! • Let’s make a small class to use as an illustration. • This class will hold info about boxes.
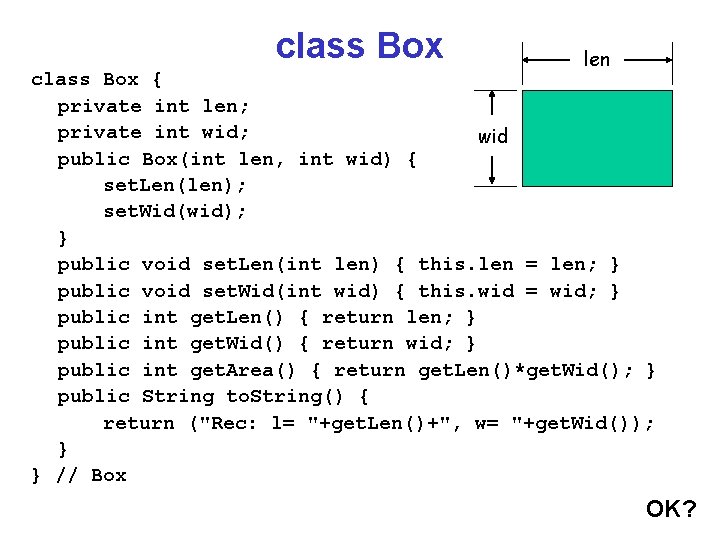
class Box len class Box { private int len; private int wid; wid public Box(int len, int wid) { set. Len(len); set. Wid(wid); } public void set. Len(int len) { this. len = len; } public void set. Wid(int wid) { this. wid = wid; } public int get. Len() { return len; } public int get. Wid() { return wid; } public int get. Area() { return get. Len()*get. Wid(); } public String to. String() { return ("Rec: l= "+get. Len()+", w= "+get. Wid()); } } // Box OK?
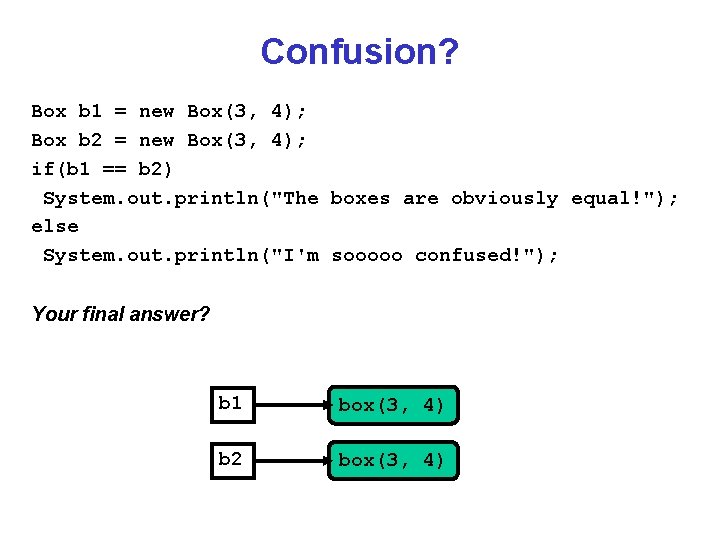
Confusion? Box b 1 = new Box(3, 4); Box b 2 = new Box(3, 4); if(b 1 == b 2) System. out. println("The boxes are obviously equal!"); else System. out. println("I'm sooooo confused!"); Your final answer? b 1 box(3, 4) b 2 box(3, 4)
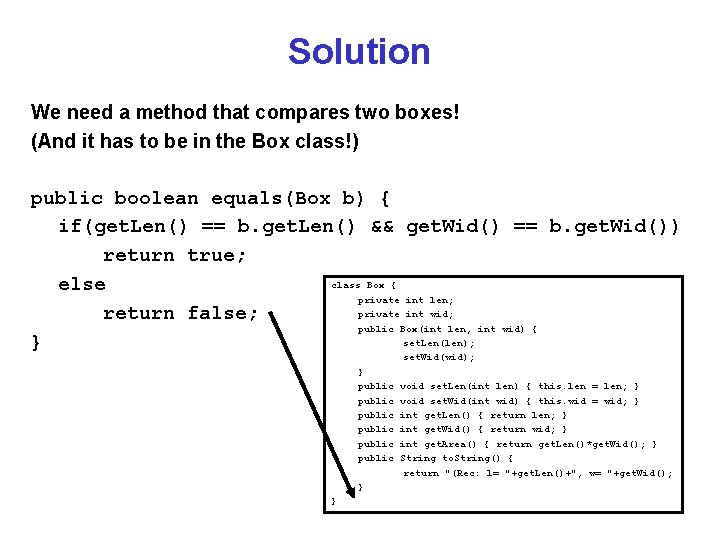
Solution We need a method that compares two boxes! (And it has to be in the Box class!) public boolean equals(Box b) { if(get. Len() == b. get. Len() && get. Wid() == b. get. Wid()) return true; class Box { else private int len; private int wid; return false; public Box(int len, int wid) { set. Len(len); } set. Wid(wid); } public public } } void set. Len(int len) { this. len = len; } void set. Wid(int wid) { this. wid = wid; } int get. Len() { return len; } int get. Wid() { return wid; } int get. Area() { return get. Len()*get. Wid(); } String to. String() { return "(Rec: l= "+get. Len()+", w= "+get. Wid();
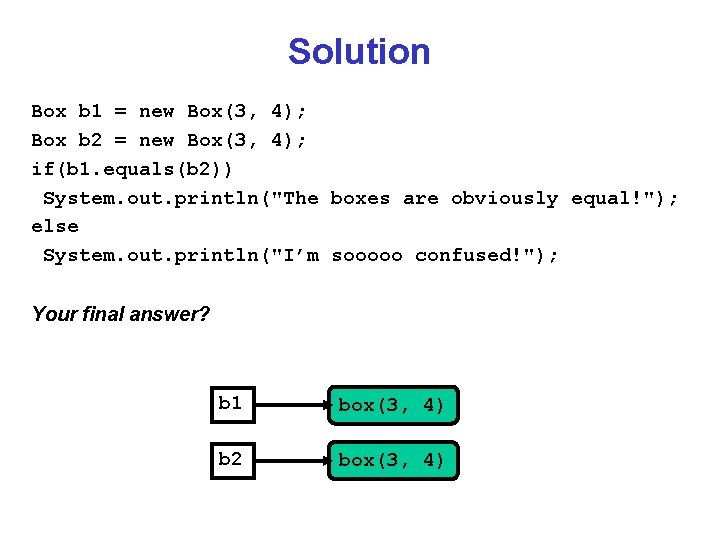
Solution Box b 1 = new Box(3, 4); Box b 2 = new Box(3, 4); if(b 1. equals(b 2)) System. out. println("The boxes are obviously equal!"); else System. out. println("I’m sooooo confused!"); Your final answer? b 1 box(3, 4) b 2 box(3, 4)
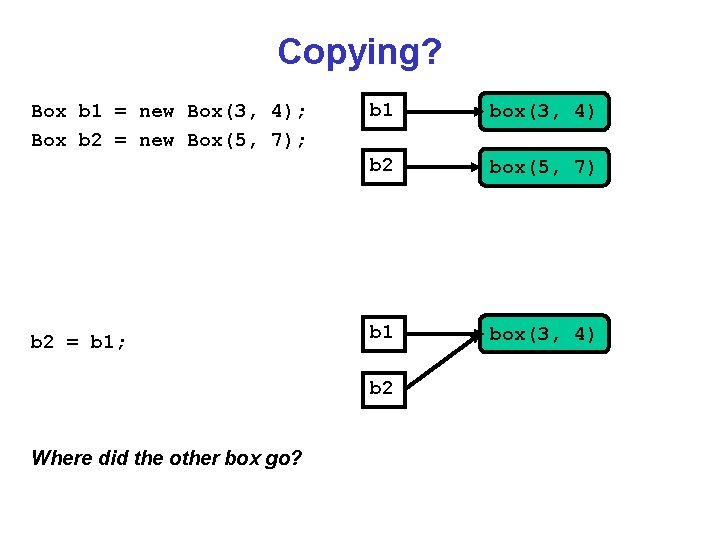
Copying? Box b 1 = new Box(3, 4); Box b 2 = new Box(5, 7); b 2 = b 1; b 1 box(3, 4) b 2 box(5, 7) b 1 box(3, 4) b 2 Where did the other box go?
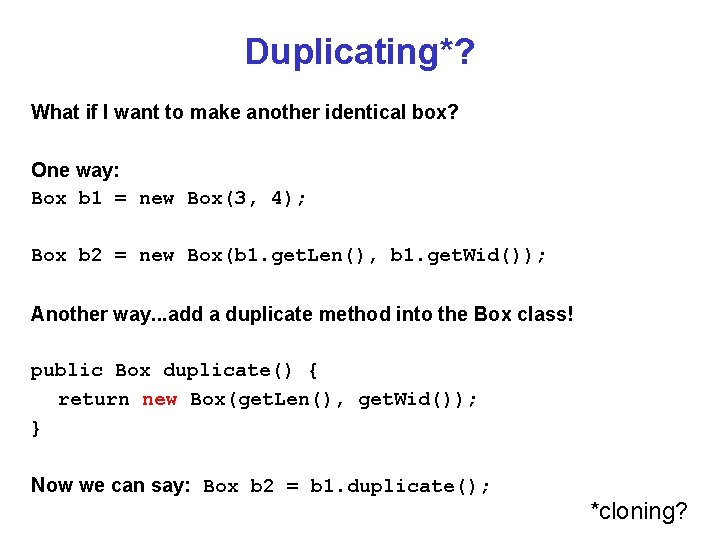
Duplicating*? What if I want to make another identical box? One way: Box b 1 = new Box(3, 4); Box b 2 = new Box(b 1. get. Len(), b 1. get. Wid()); Another way. . . add a duplicate method into the Box class! public Box duplicate() { return new Box(get. Len(), get. Wid()); } Now we can say: Box b 2 = b 1. duplicate(); *cloning?
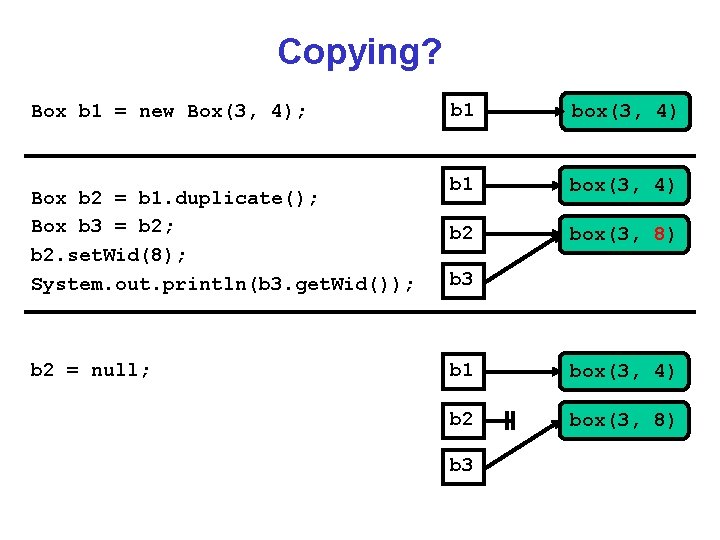
Copying? Box b 1 = new Box(3, 4); Box b 2 = b 1. duplicate(); Box b 3 = b 2; b 2. set. Wid(8); System. out. println(b 3. get. Wid()); b 2 = null; b 1 box(3, 4) b 2 box(3, 8) b 3
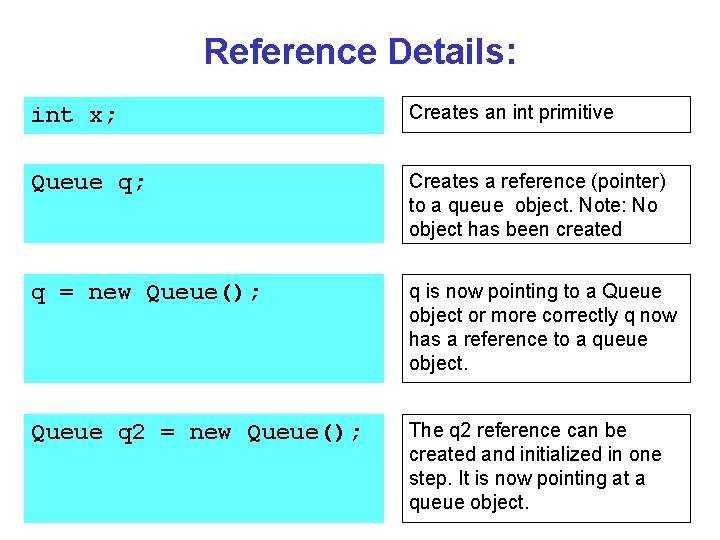
Reference Details: int x; Creates an int primitive Queue q; Creates a reference (pointer) to a queue object. Note: No object has been created q = new Queue(); q is now pointing to a Queue object or more correctly q now has a reference to a queue object. Queue q 2 = new Queue(); The q 2 reference can be created and initialized in one step. It is now pointing at a queue object.
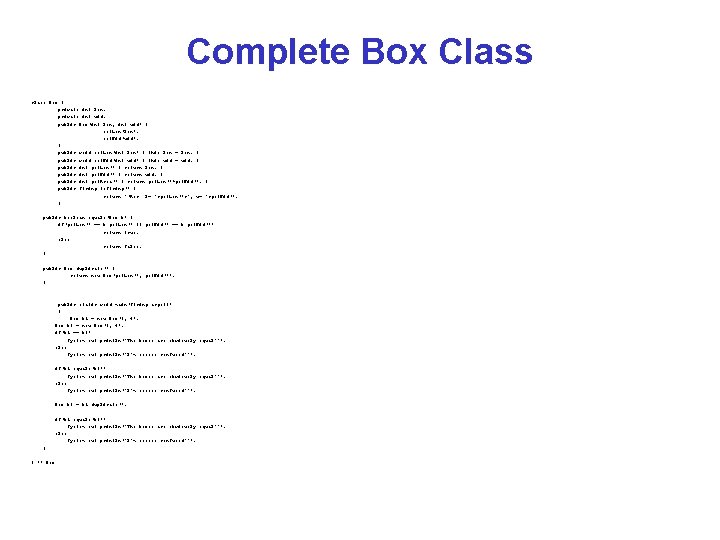
Complete Box Class class Box { private int len; private int wid; public Box(int len, int wid) { set. Len(len); set. Wid(wid); } public void set. Len(int len) { this. len = len; } public void set. Wid(int wid) { this. wid = wid; } public int get. Len() { return len; } public int get. Wid() { return wid; } public int get. Area() { return get. Len()*get. Wid(); } public String to. String() { return "(Rec: l= "+get. Len()+", w= "+get. Wid(); } public boolean equals(Box b) { if(get. Len() == b. get. Len() && get. Wid() == b. get. Wid()) return true; else return false; } public Box duplicate() { return new Box(get. Len(), get. Wid()); } public static void main(String args[]) { Box b 1 = new Box(3, 4); Box b 2 = new Box(3, 4); if(b 1 == b 2) System. out. println("The boxes are obviously equal!"); else System. out. println("I'm sooooo confused!"); if(b 1. equals(b 2)) System. out. println("The boxes are obviously equal!"); else System. out. println("I'm sooooo confused!"); Box b 3 = b 1. duplicate(); if(b 1. equals(b 3)) System. out. println("The boxes are obviously equal!"); else System. out. println("I'm sooooo confused!"); } } // Box
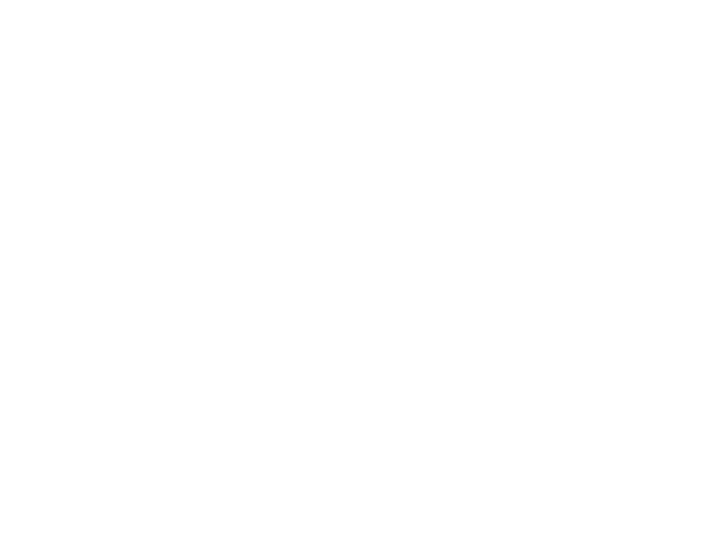
Evil of copying
Copy the given table in your notebook put a check
Copying a segment
C copy struct
[email protected]
The principal enzyme involved in dna replication is
Copying gc
Major vs minor details
Minor and major supporting details
4 figure and 6 figure grid references
Reference node and non reference node
Reference node and non reference node
You remember what you were doing at this time yesterday
What were you doing at this time yesterday
God loved you before you were born
Singular and plural he she it
You become what you always were a very big fish