Pythons math Modules Files Pythons time Classes in
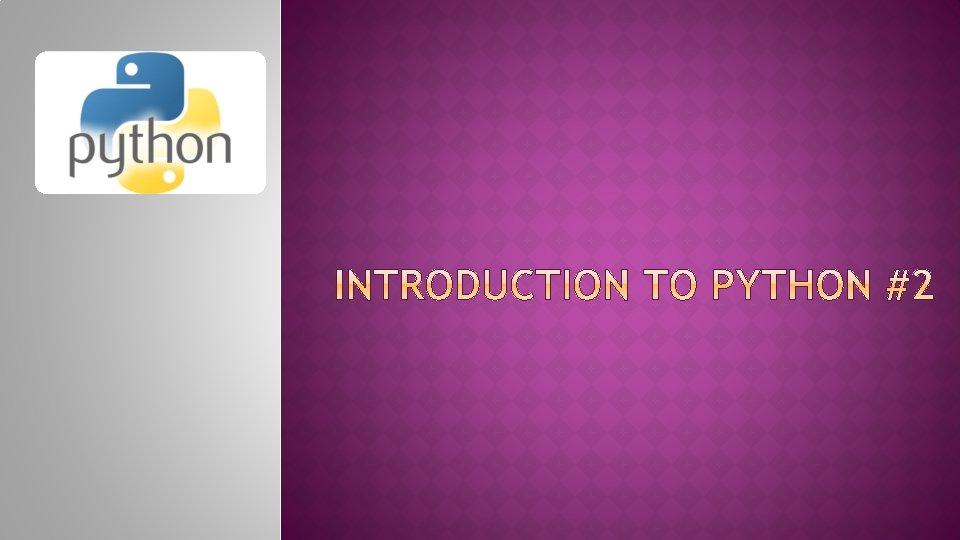
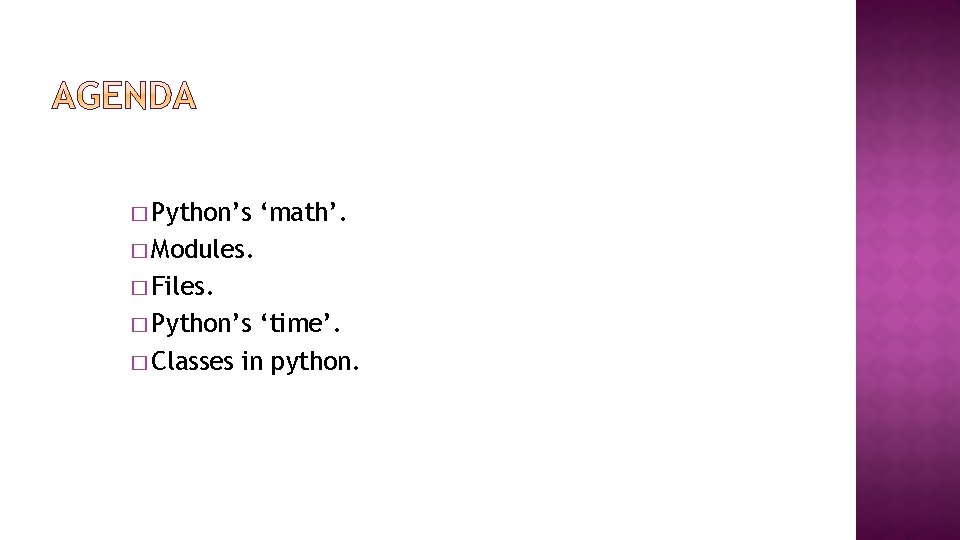
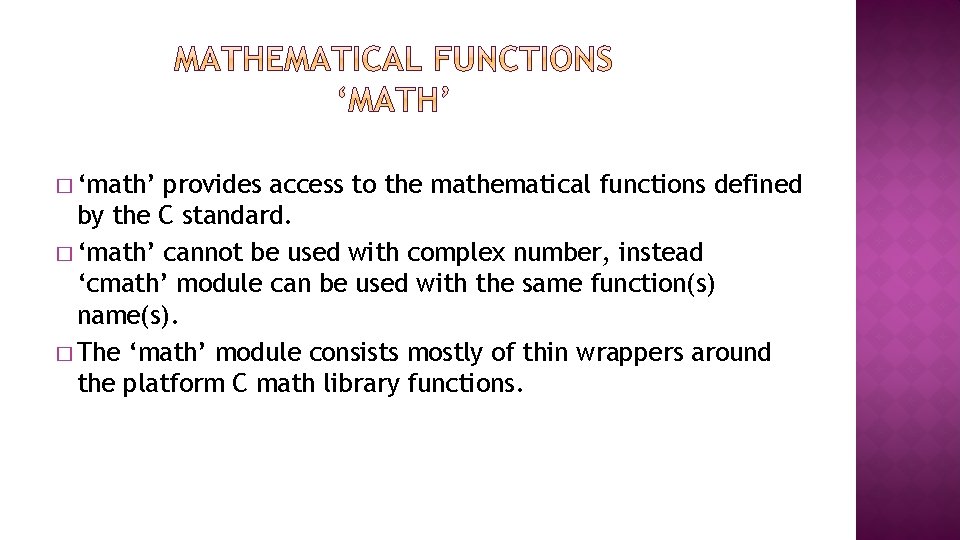
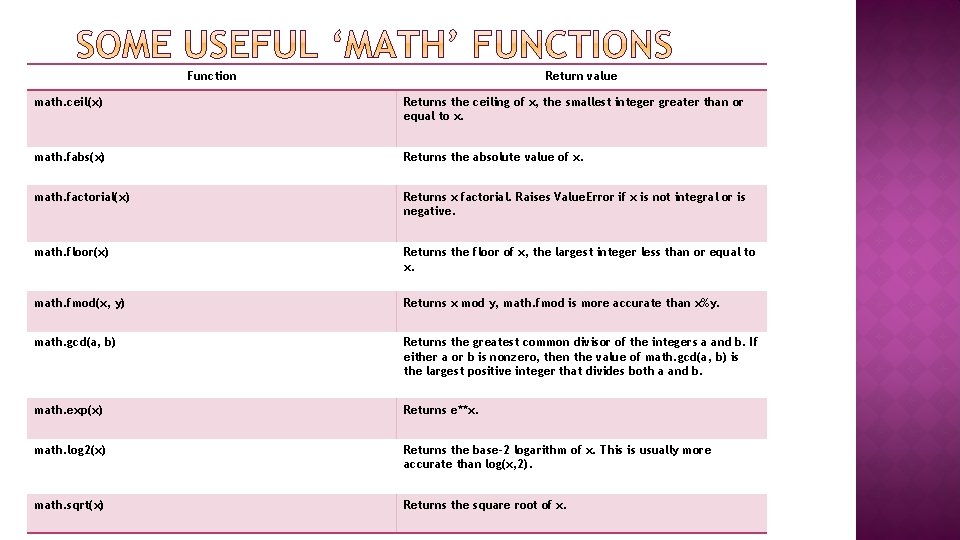
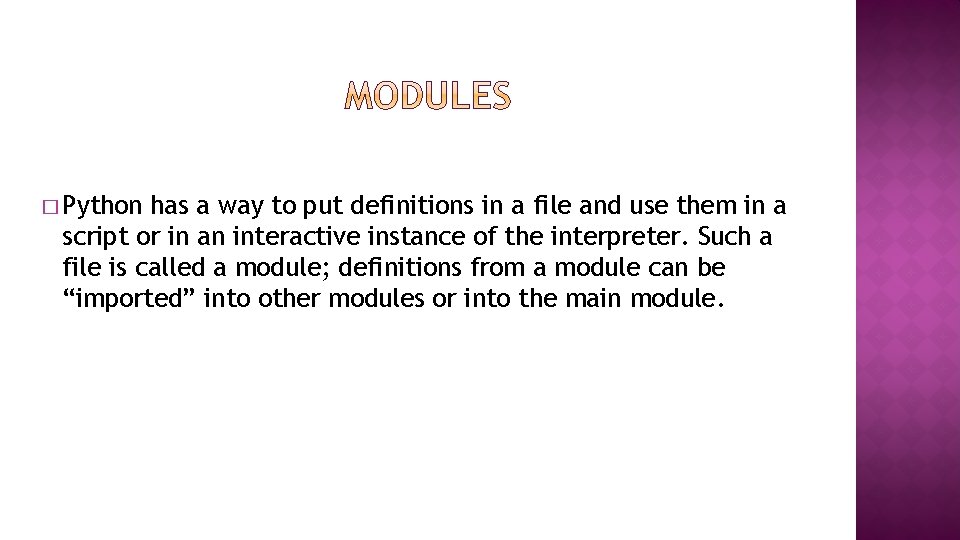
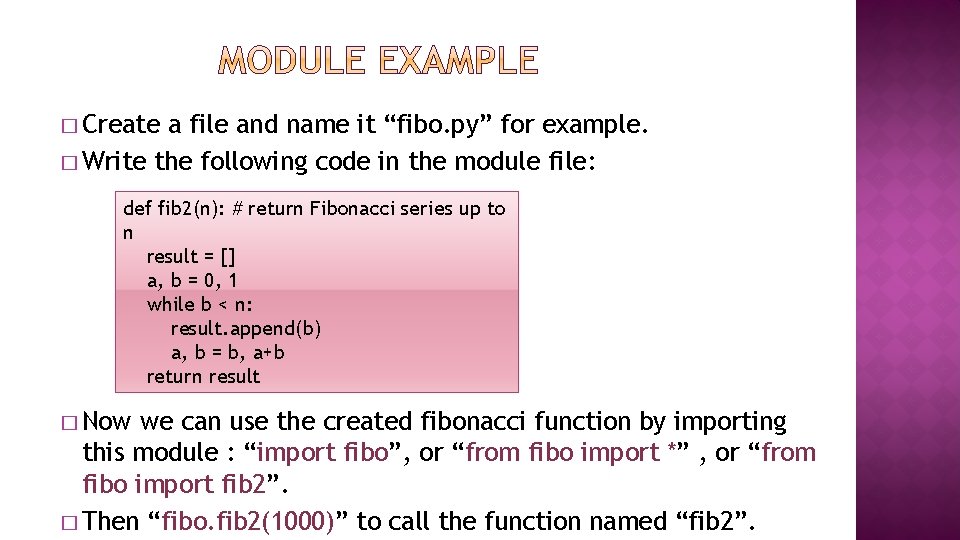
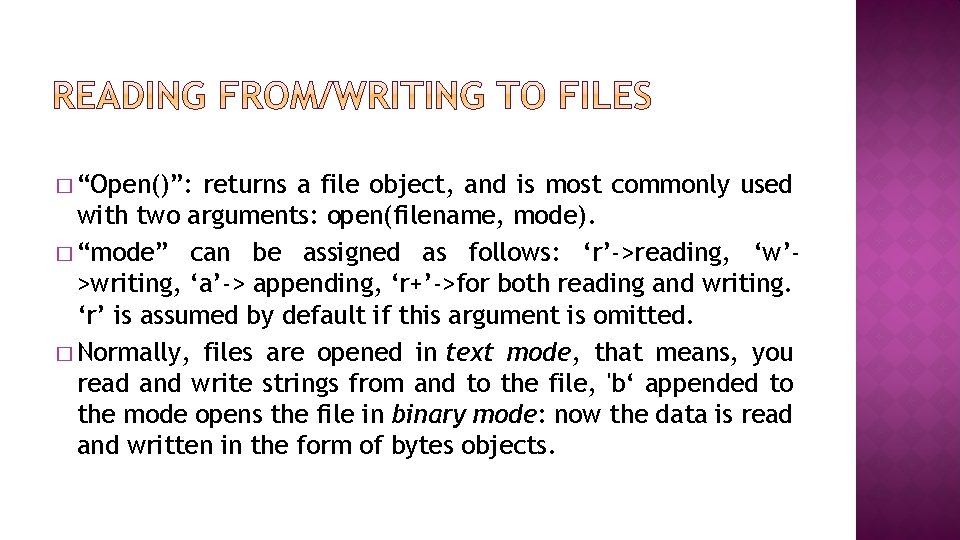
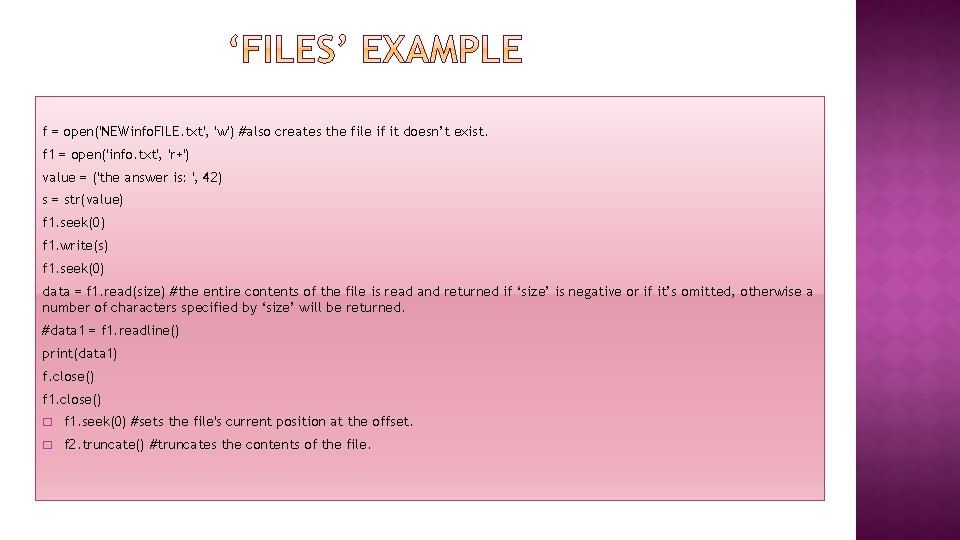
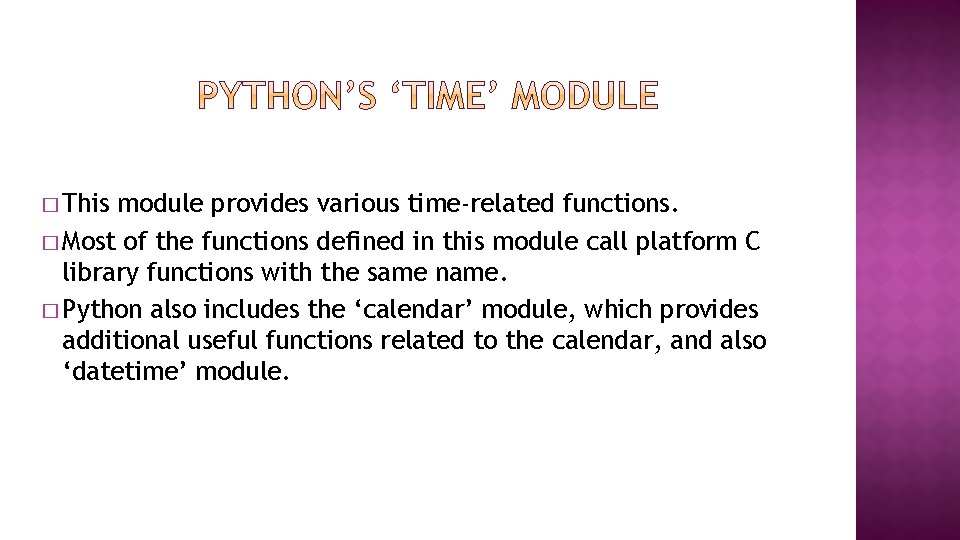
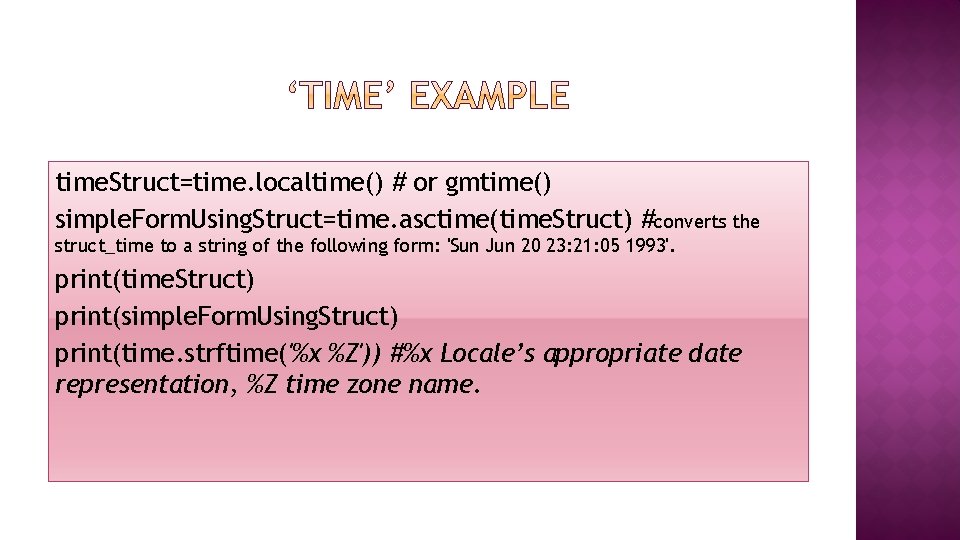
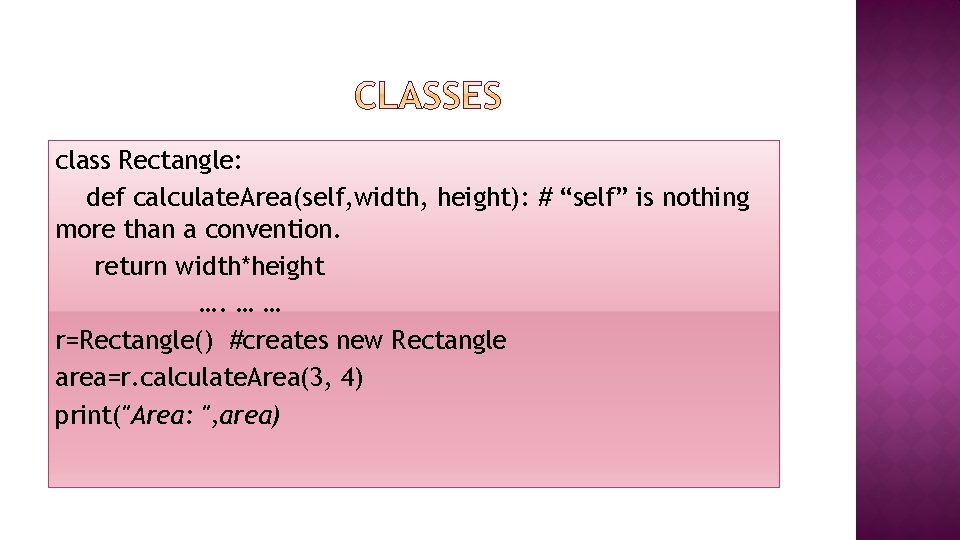
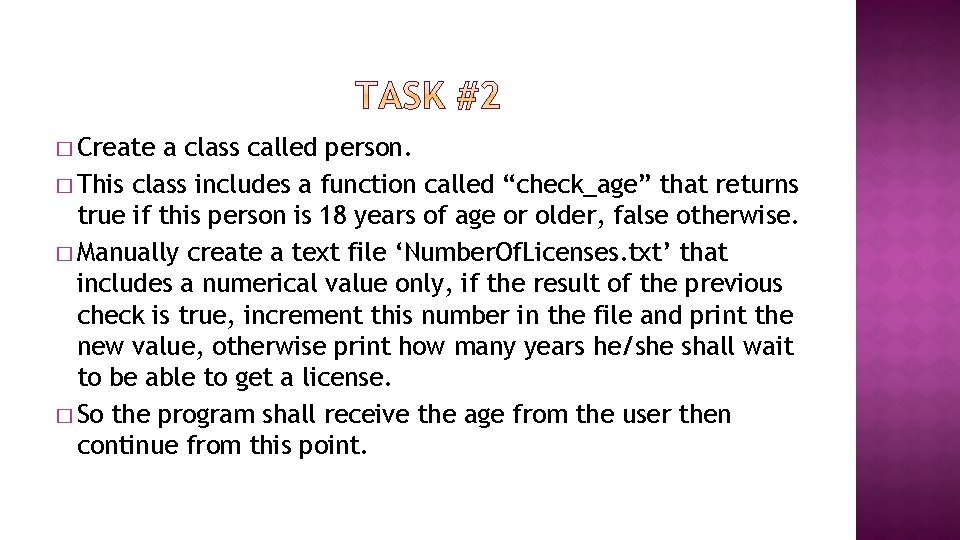
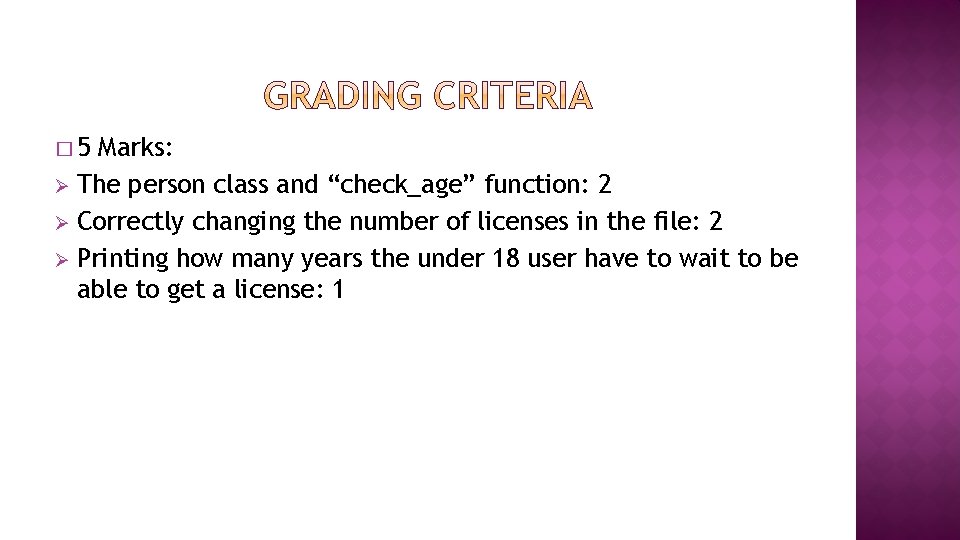
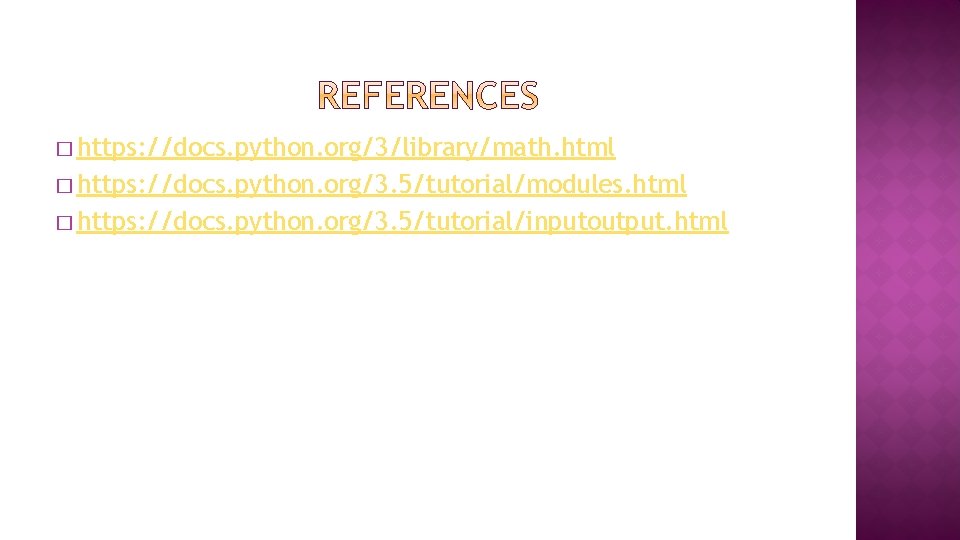
- Slides: 14
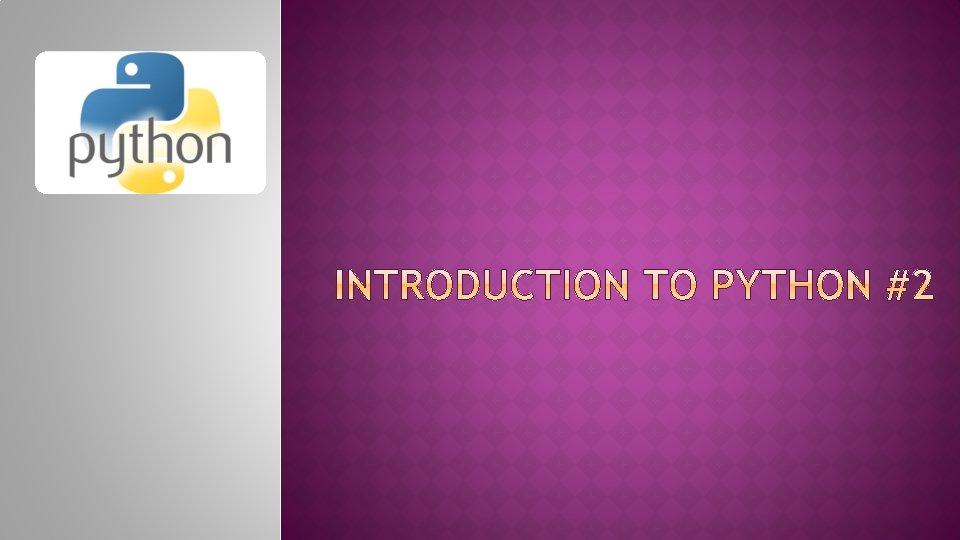
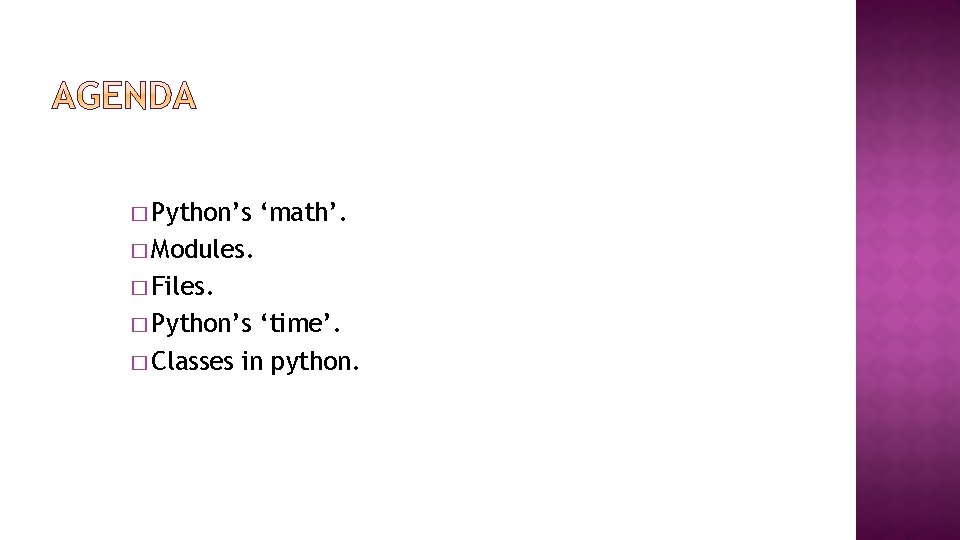
� Python’s ‘math’. � Modules. � Files. � Python’s ‘time’. � Classes in python.
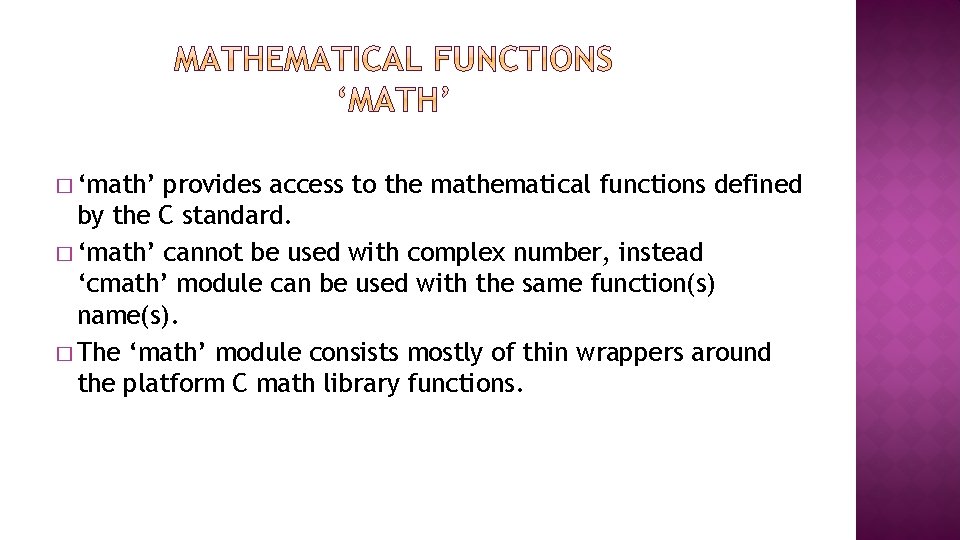
� ‘math’ provides access to the mathematical functions defined by the C standard. � ‘math’ cannot be used with complex number, instead ‘cmath’ module can be used with the same function(s) name(s). � The ‘math’ module consists mostly of thin wrappers around the platform C math library functions.
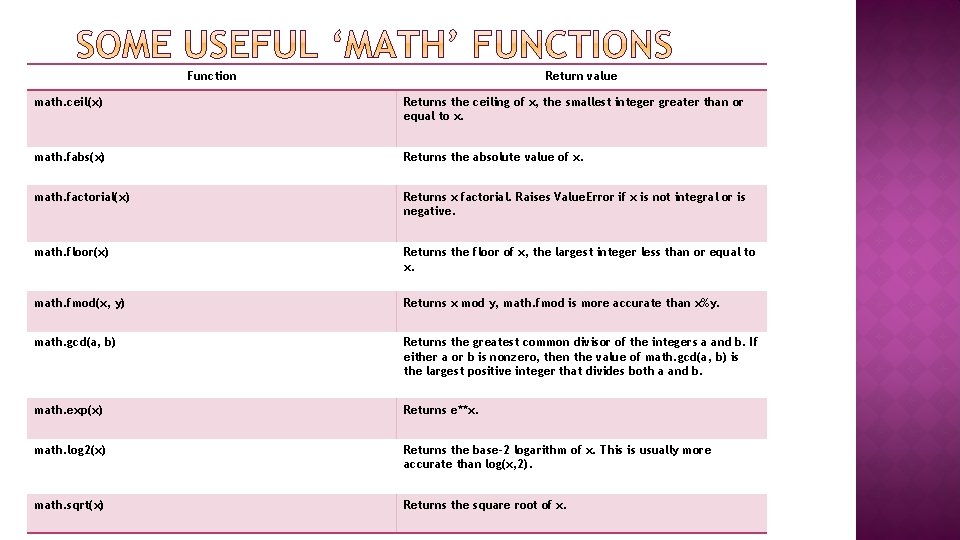
Function Return value math. ceil(x) Returns the ceiling of x, the smallest integer greater than or equal to x. math. fabs(x) Returns the absolute value of x. math. factorial(x) Returns x factorial. Raises Value. Error if x is not integral or is negative. math. floor(x) Returns the floor of x, the largest integer less than or equal to x. math. fmod(x, y) Returns x mod y, math. fmod is more accurate than x%y. math. gcd(a, b) Returns the greatest common divisor of the integers a and b. If either a or b is nonzero, then the value of math. gcd(a, b) is the largest positive integer that divides both a and b. math. exp(x) Returns e**x. math. log 2(x) Returns the base-2 logarithm of x. This is usually more accurate than log(x, 2). math. sqrt(x) Returns the square root of x.
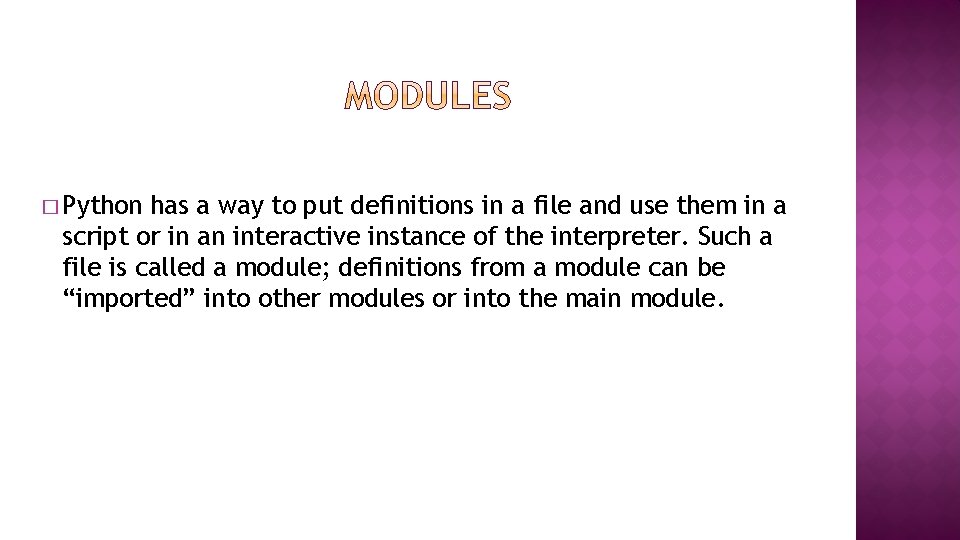
� Python has a way to put definitions in a file and use them in a script or in an interactive instance of the interpreter. Such a file is called a module; definitions from a module can be “imported” into other modules or into the main module.
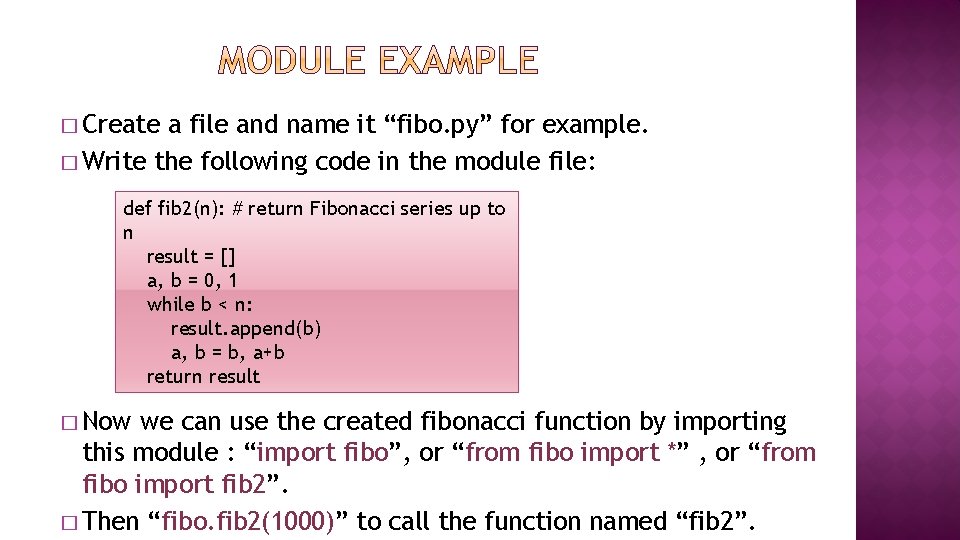
� Create a file and name it “fibo. py” for example. � Write the following code in the module file: def fib 2(n): # return Fibonacci series up to n result = [] a, b = 0, 1 while b < n: result. append(b) a, b = b, a+b return result � Now we can use the created fibonacci function by importing this module : “import fibo”, or “from fibo import *” , or “from fibo import fib 2”. � Then “fibo. fib 2(1000)” to call the function named “fib 2”.
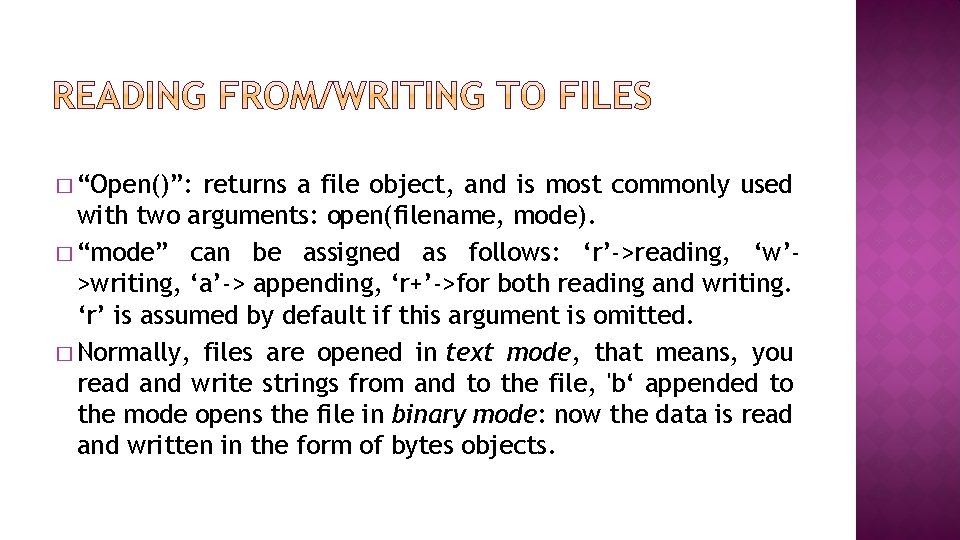
� “Open()”: returns a file object, and is most commonly used with two arguments: open(filename, mode). � “mode” can be assigned as follows: ‘r’->reading, ‘w’>writing, ‘a’-> appending, ‘r+’->for both reading and writing. ‘r’ is assumed by default if this argument is omitted. � Normally, files are opened in text mode, that means, you read and write strings from and to the file, 'b‘ appended to the mode opens the file in binary mode: now the data is read and written in the form of bytes objects.
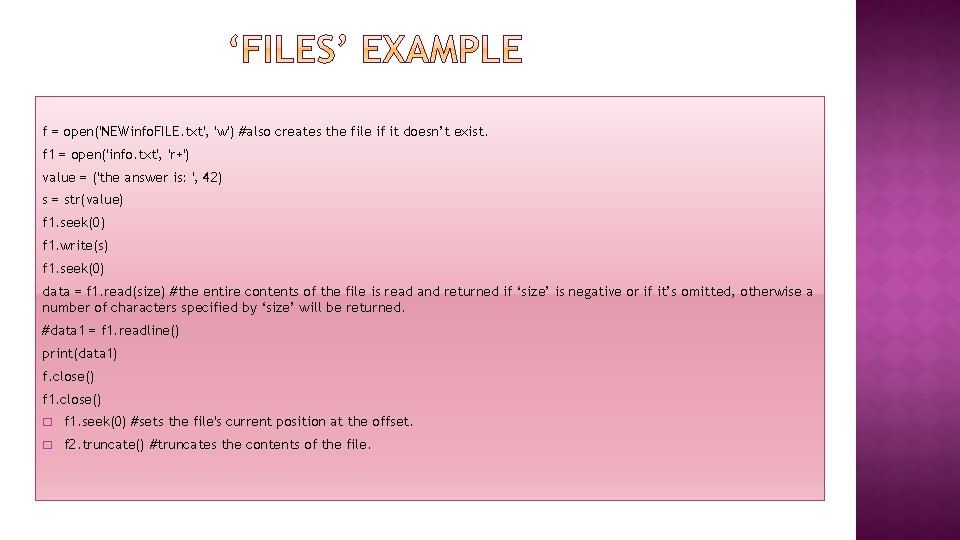
f = open('NEWinfo. FILE. txt', 'w') #also creates the file if it doesn’t exist. f 1 = open('info. txt', 'r+') value = ('the answer is: ', 42) s = str(value) f 1. seek(0) f 1. write(s) f 1. seek(0) data = f 1. read(size) #the entire contents of the file is read and returned if ‘size’ is negative or if it’s omitted, otherwise a number of characters specified by ‘size’ will be returned. #data 1 = f 1. readline() print(data 1) f. close() f 1. close() � f 1. seek(0) #sets the file's current position at the offset. � f 2. truncate() #truncates the contents of the file.
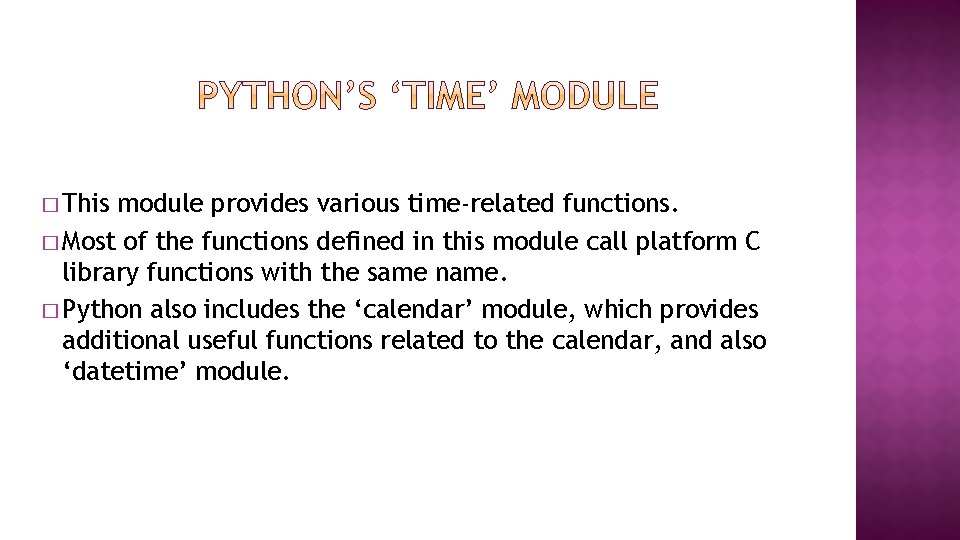
� This module provides various time-related functions. � Most of the functions defined in this module call platform C library functions with the same name. � Python also includes the ‘calendar’ module, which provides additional useful functions related to the calendar, and also ‘datetime’ module.
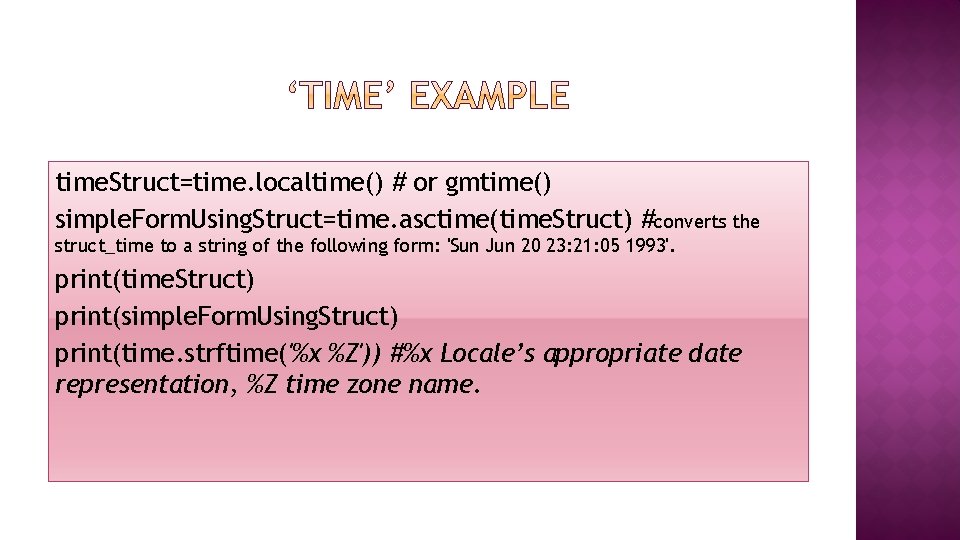
time. Struct=time. localtime() # or gmtime() simple. Form. Using. Struct=time. asctime(time. Struct) #converts the struct_time to a string of the following form: 'Sun Jun 20 23: 21: 05 1993'. print(time. Struct) print(simple. Form. Using. Struct) print(time. strftime('%x %Z')) #%x Locale’s appropriate date representation, %Z time zone name.
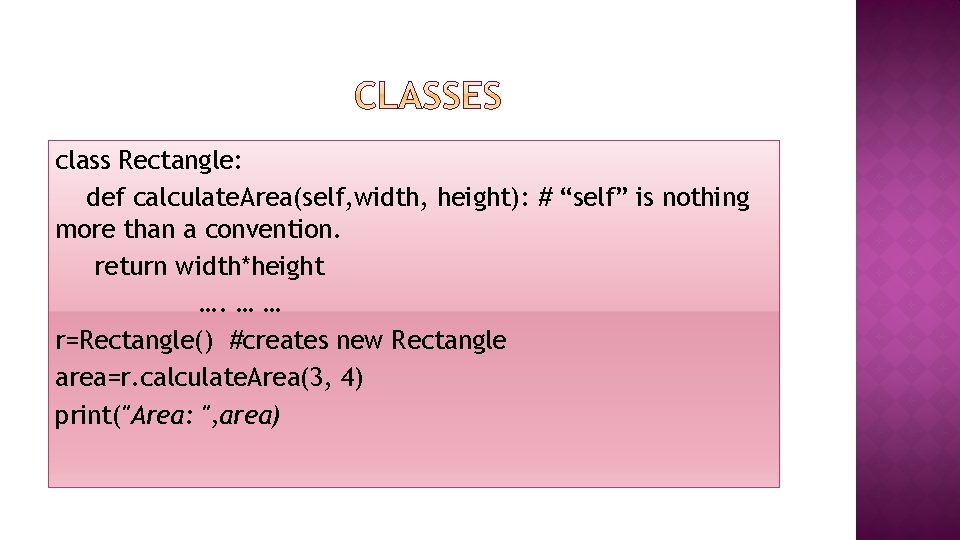
class Rectangle: def calculate. Area(self, width, height): # “self” is nothing more than a convention. return width*height …. … … r=Rectangle() #creates new Rectangle area=r. calculate. Area(3, 4) print("Area: ", area)
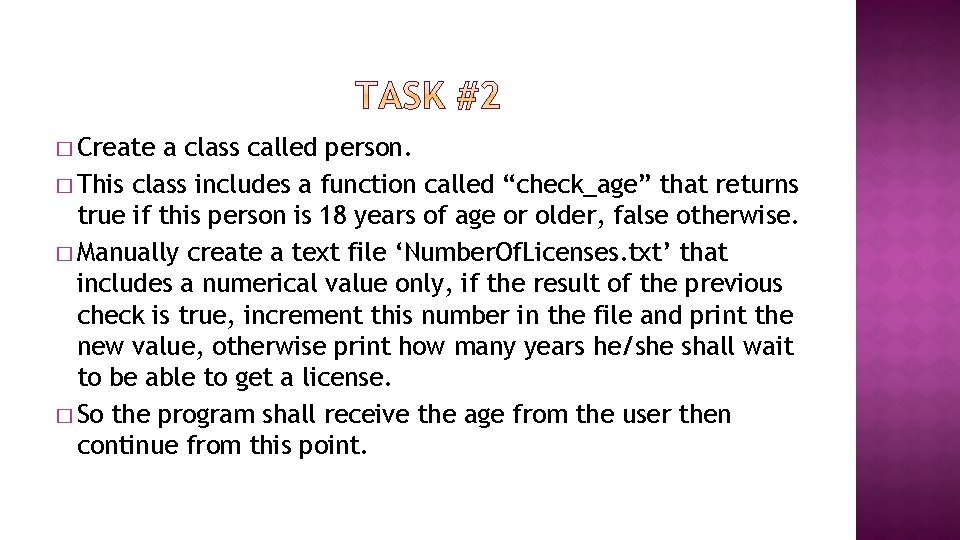
� Create a class called person. � This class includes a function called “check_age” that returns true if this person is 18 years of age or older, false otherwise. � Manually create a text file ‘Number. Of. Licenses. txt’ that includes a numerical value only, if the result of the previous check is true, increment this number in the file and print the new value, otherwise print how many years he/she shall wait to be able to get a license. � So the program shall receive the age from the user then continue from this point.
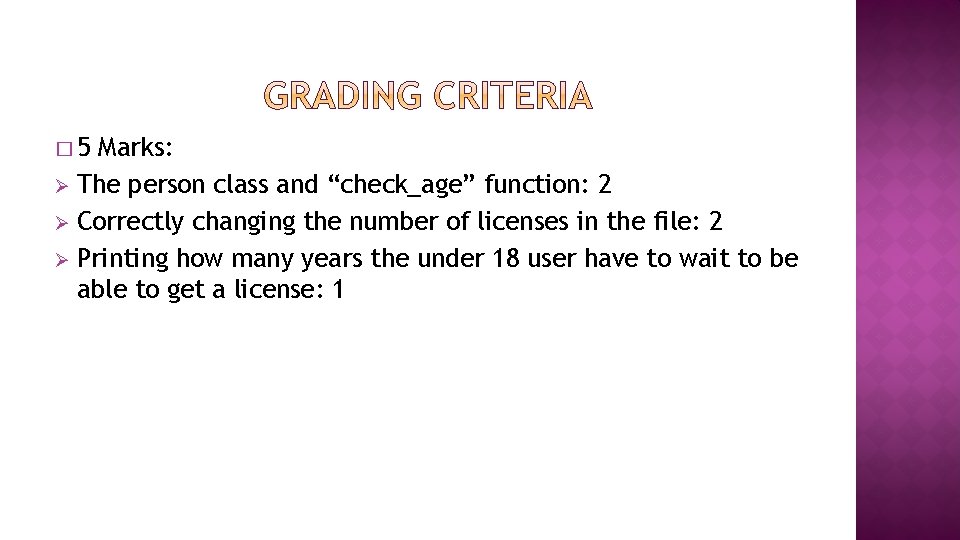
� 5 Marks: Ø The person class and “check_age” function: 2 Ø Correctly changing the number of licenses in the file: 2 Ø Printing how many years the under 18 user have to wait to be able to get a license: 1
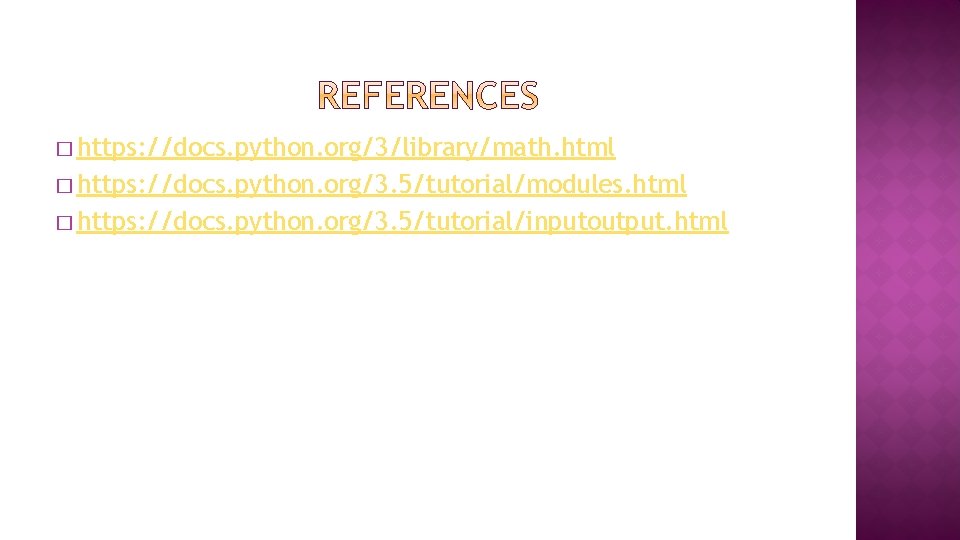
� https: //docs. python. org/3/library/math. html � https: //docs. python. org/3. 5/tutorial/modules. html � https: //docs. python. org/3. 5/tutorial/inputoutput. html
Dot powai files are binary files
Cjis level 4 certification
Ncic hosts restricted files and non-restricted files
Nys math modules
Classe e subclasse de palavras 7. ano
Pre ap classes vs regular classes
Elapsed time
Math game math hit the button
A collection of web page
Dedup chunk store
Tsd export files
Rftp
Qrp files
Emc unity simulator
Files2u