Python Modules What are Python modules Files with
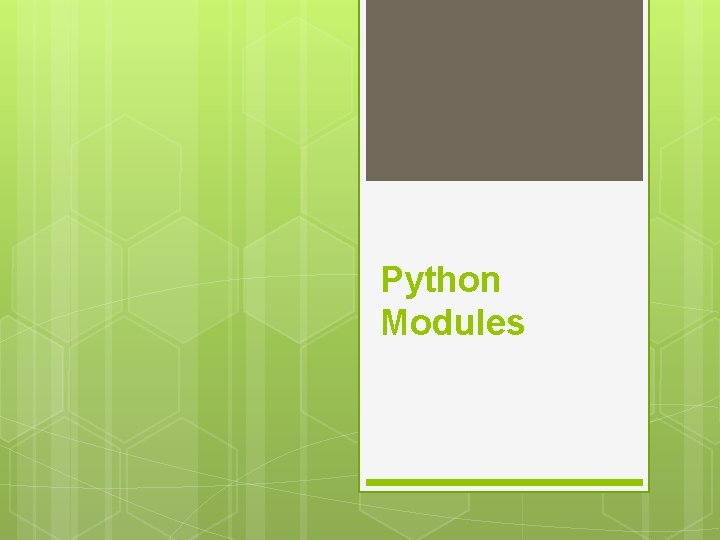
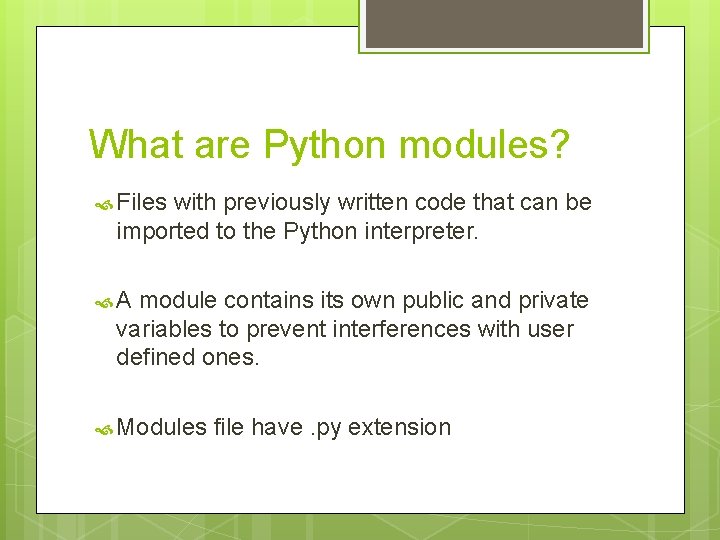
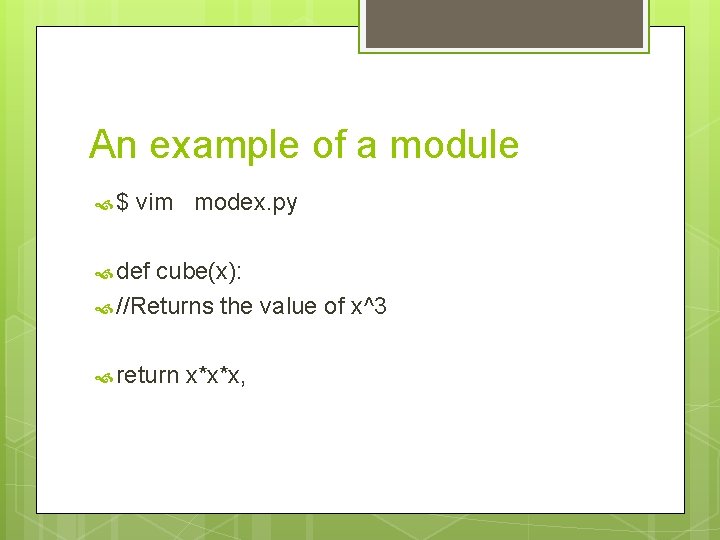
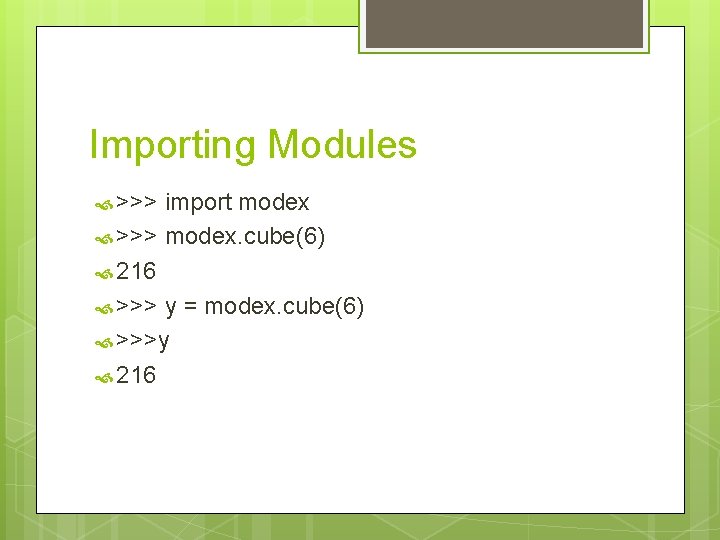
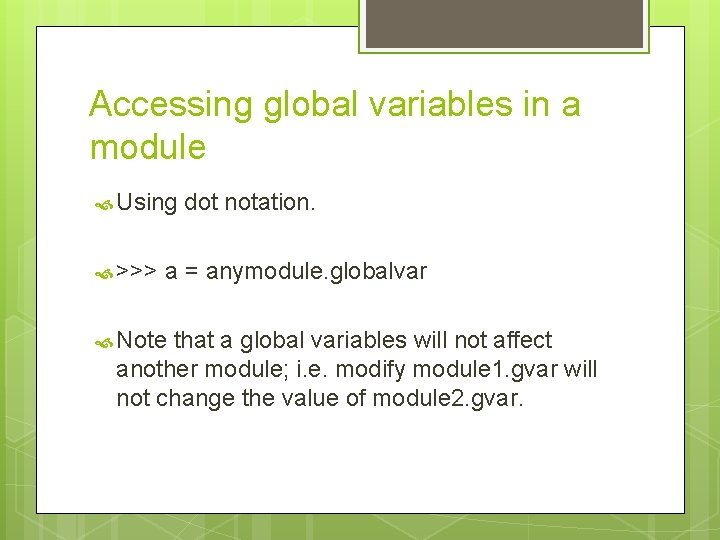
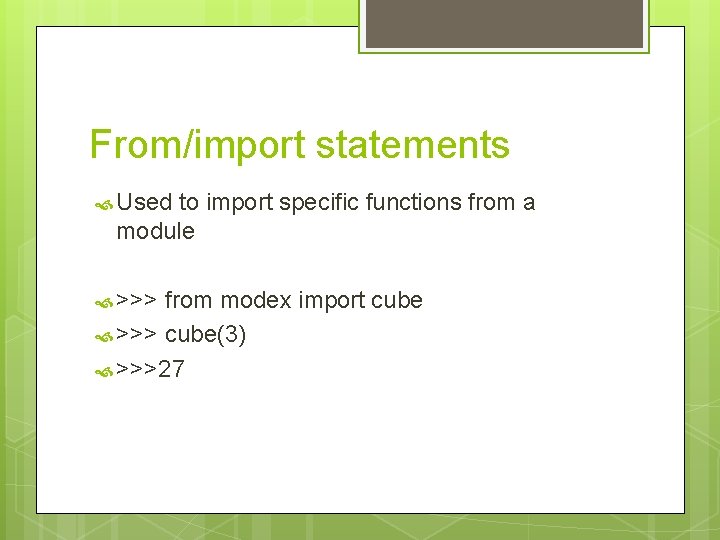
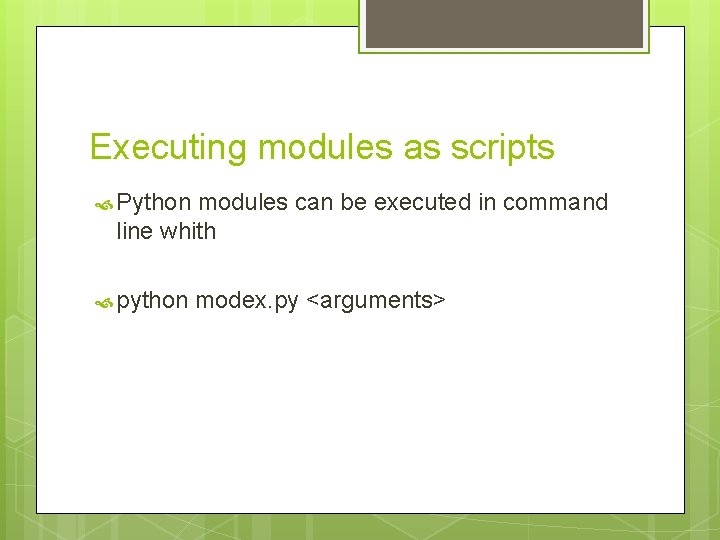
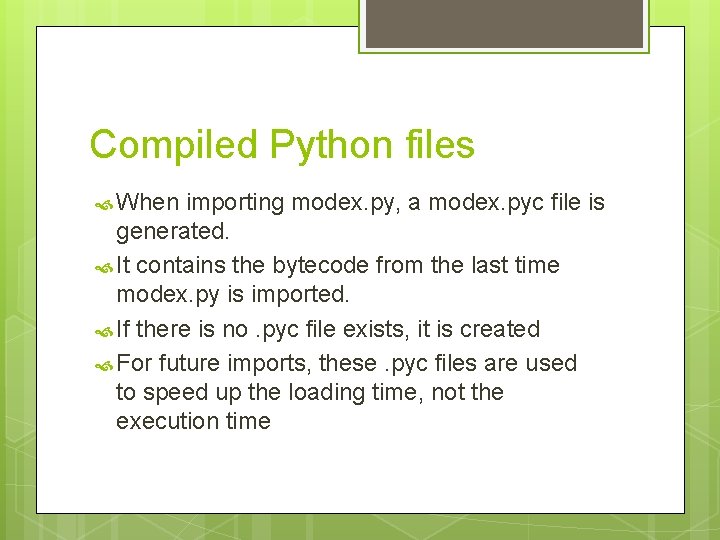
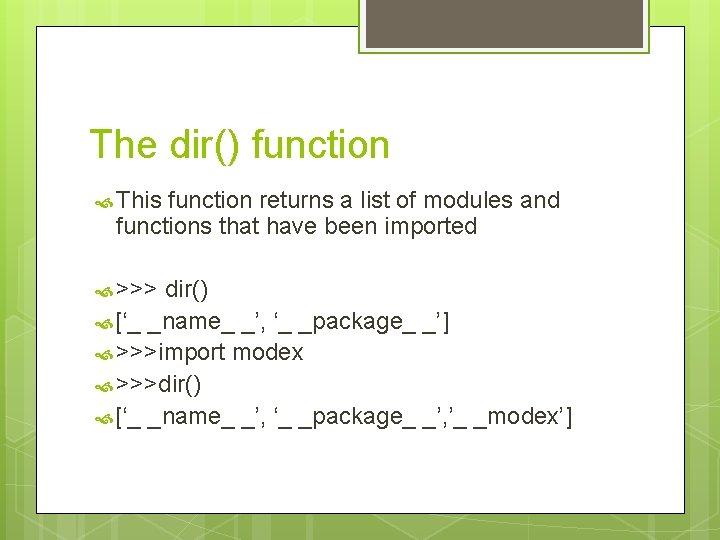
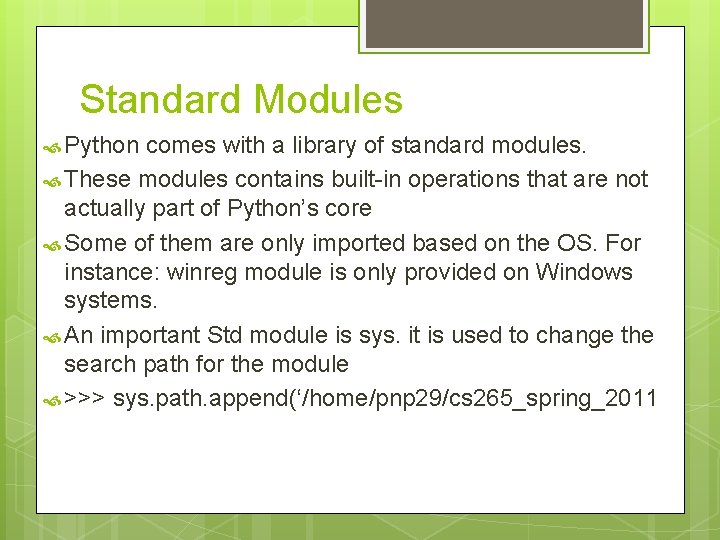
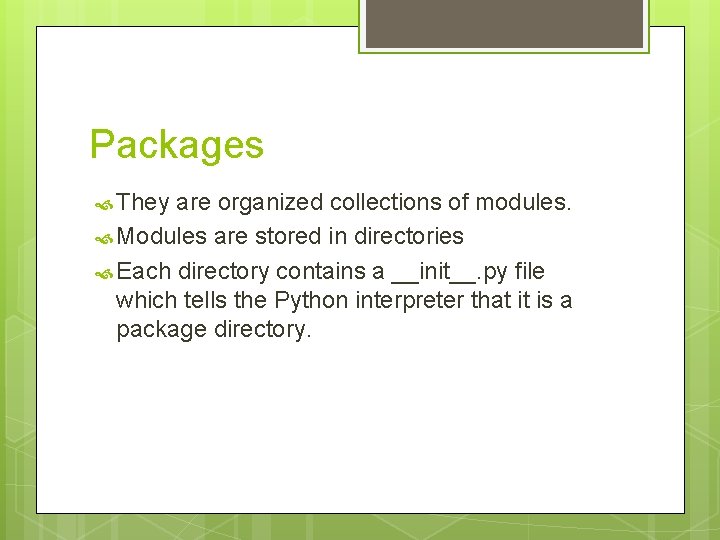
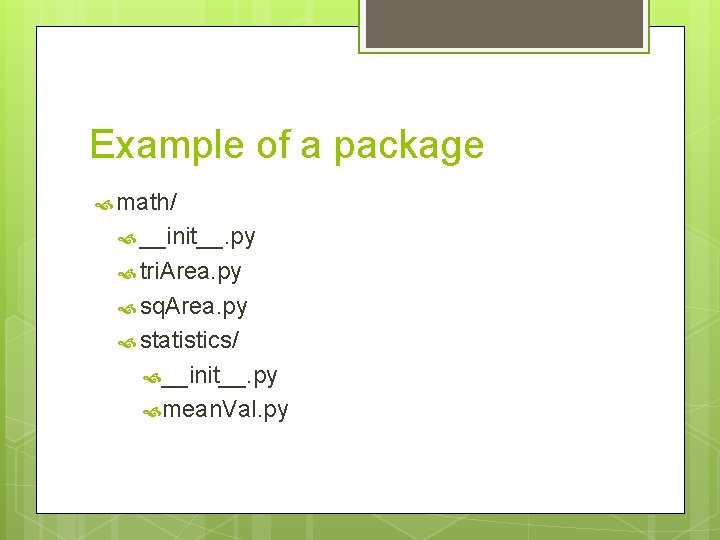
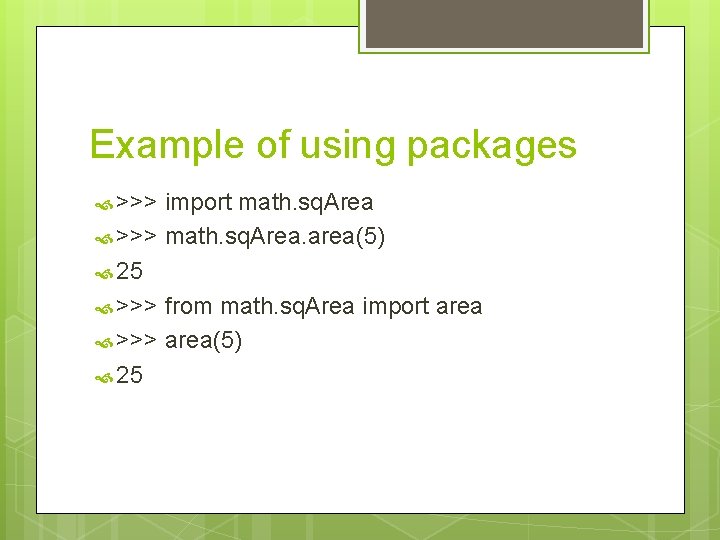
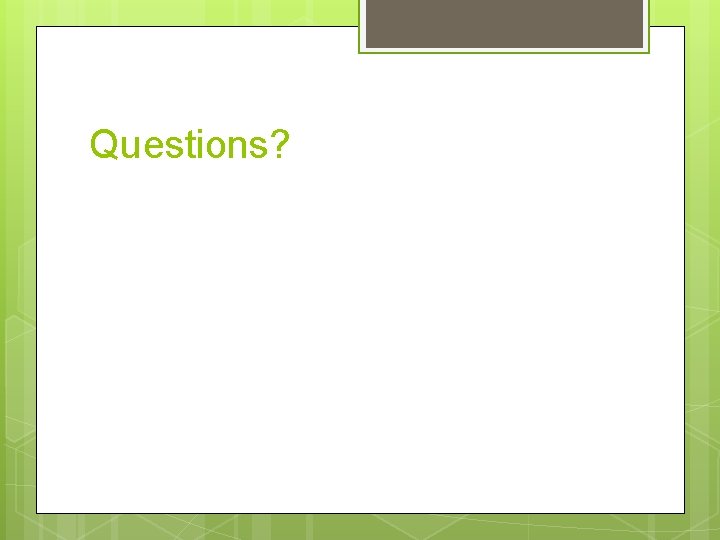
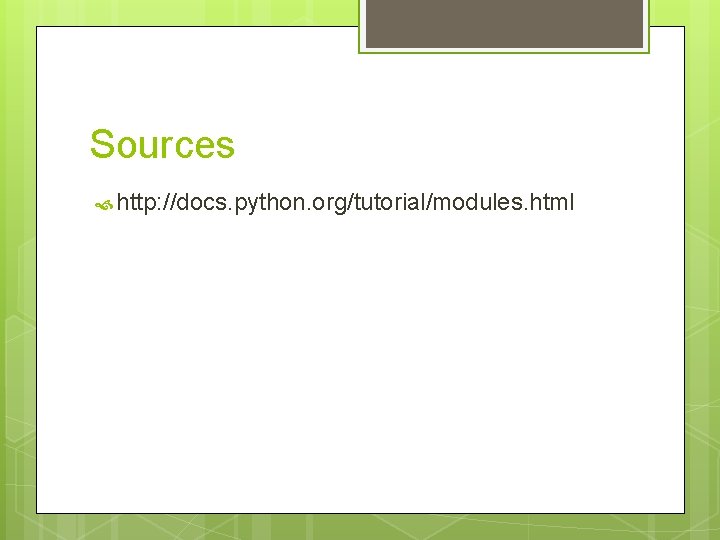
- Slides: 15
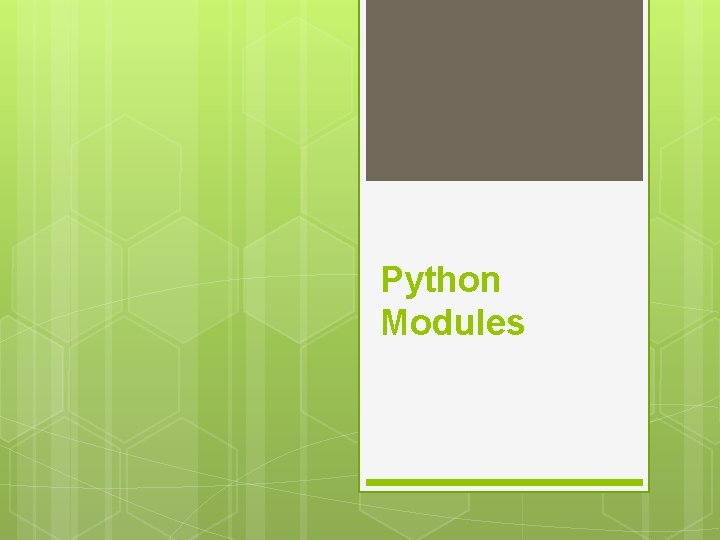
Python Modules
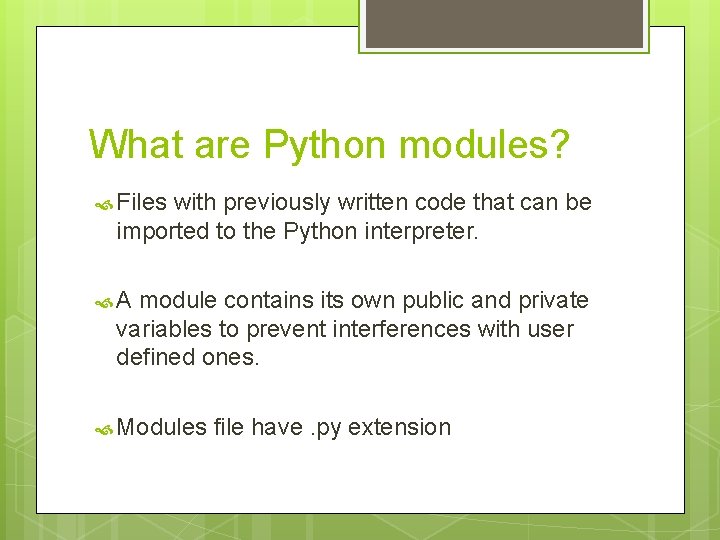
What are Python modules? Files with previously written code that can be imported to the Python interpreter. A module contains its own public and private variables to prevent interferences with user defined ones. Modules file have. py extension
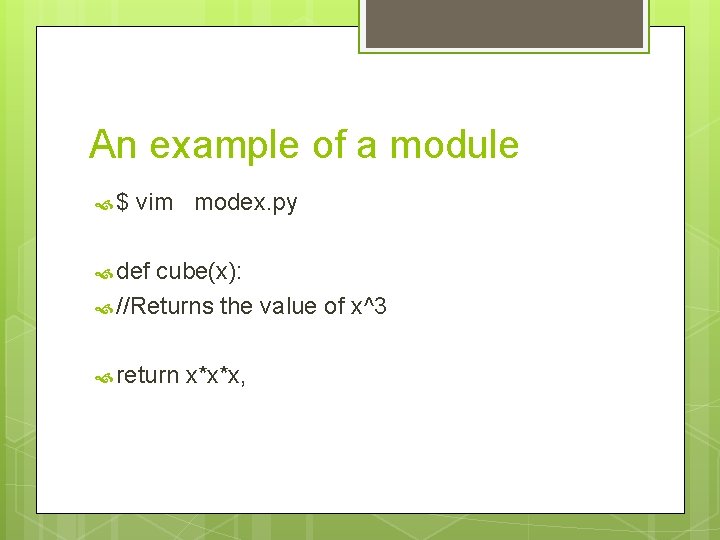
An example of a module $ vim modex. py def cube(x): //Returns the value of x^3 return x*x*x,
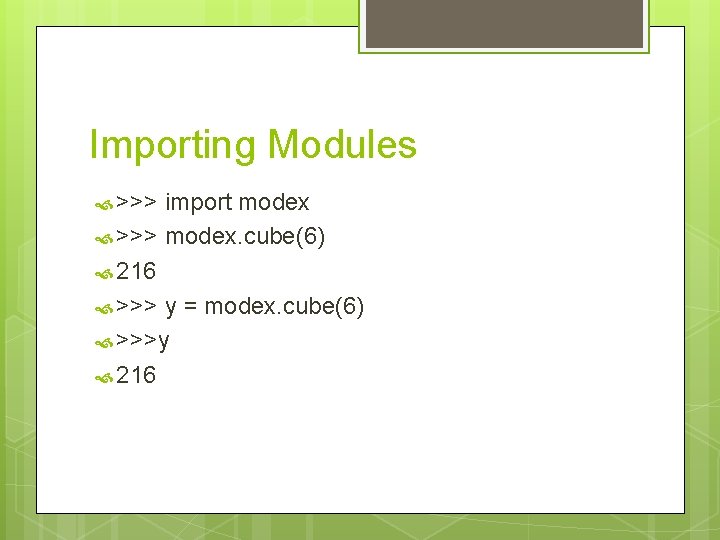
Importing Modules >>> import modex >>> modex. cube(6) 216 >>> y = modex. cube(6) >>>y 216
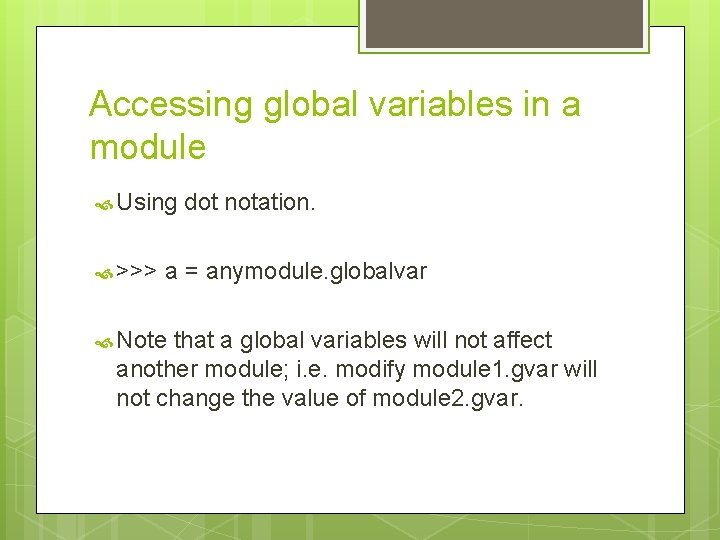
Accessing global variables in a module Using >>> dot notation. a = anymodule. globalvar Note that a global variables will not affect another module; i. e. modify module 1. gvar will not change the value of module 2. gvar.
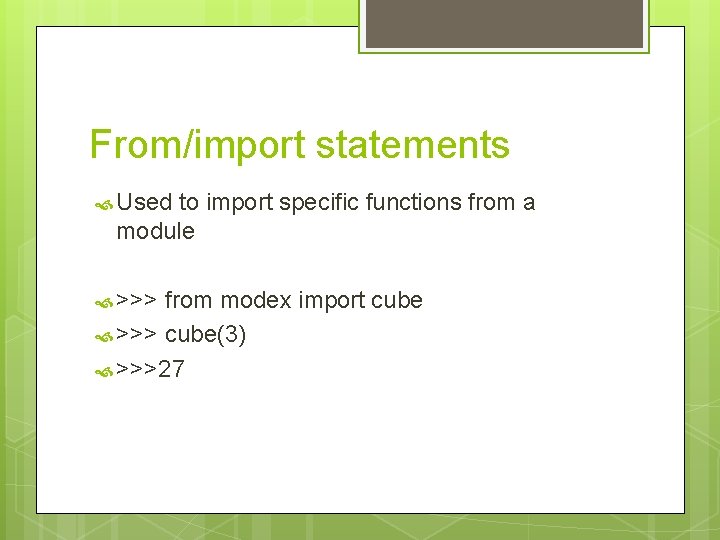
From/import statements Used to import specific functions from a module >>> from modex import cube >>> cube(3) >>>27
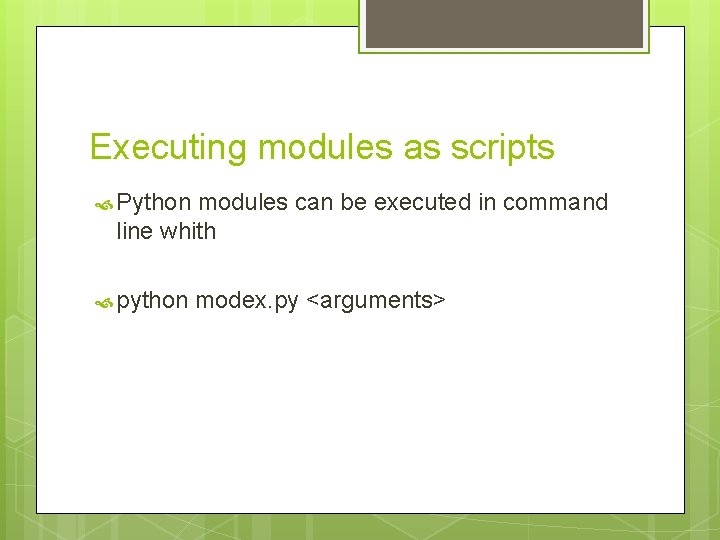
Executing modules as scripts Python modules can be executed in command line whith python modex. py <arguments>
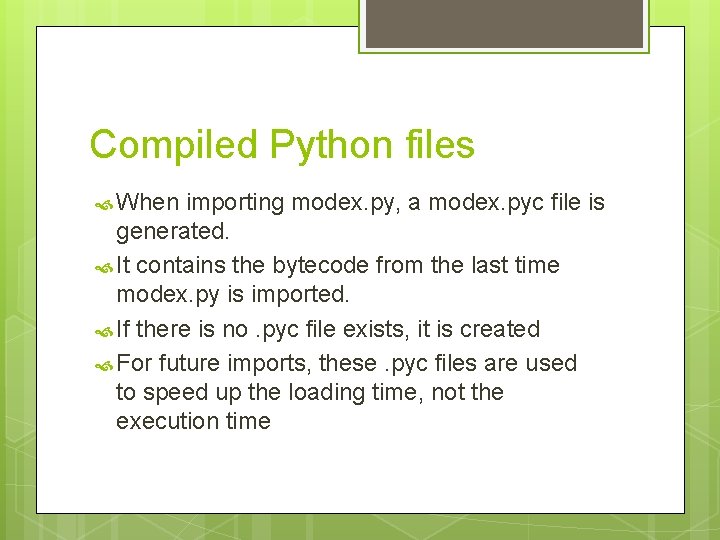
Compiled Python files When importing modex. py, a modex. pyc file is generated. It contains the bytecode from the last time modex. py is imported. If there is no. pyc file exists, it is created For future imports, these. pyc files are used to speed up the loading time, not the execution time
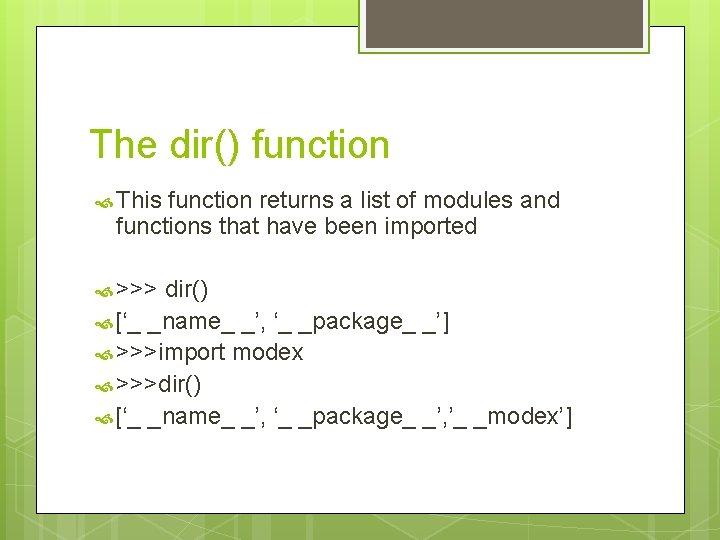
The dir() function This function returns a list of modules and functions that have been imported >>> dir() [‘_ _name_ _’, ‘_ _package_ _’] >>>import modex >>>dir() [‘_ _name_ _’, ‘_ _package_ _’, ’_ _modex’]
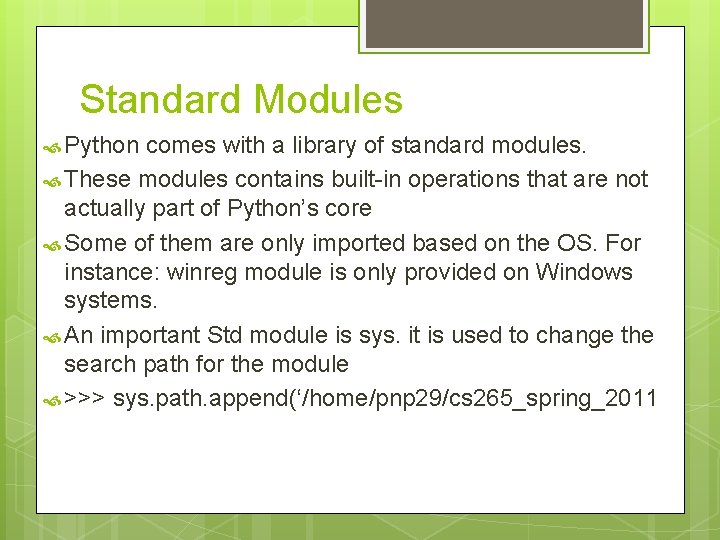
Standard Modules Python comes with a library of standard modules. These modules contains built-in operations that are not actually part of Python’s core Some of them are only imported based on the OS. For instance: winreg module is only provided on Windows systems. An important Std module is sys. it is used to change the search path for the module >>> sys. path. append(‘/home/pnp 29/cs 265_spring_2011
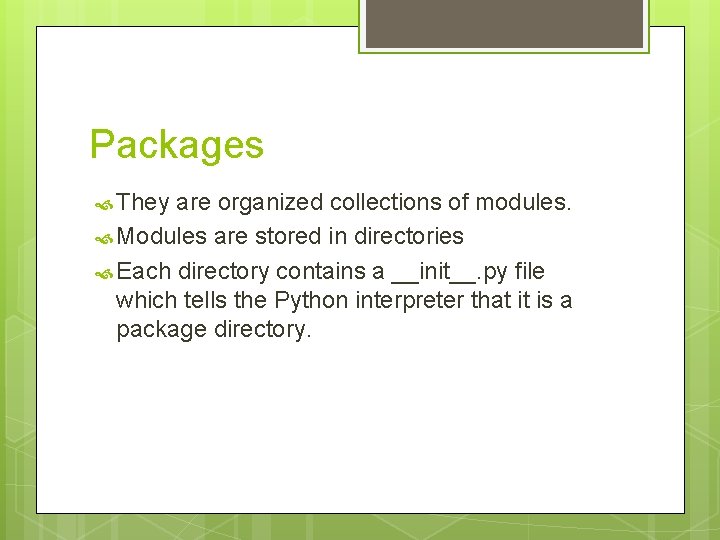
Packages They are organized collections of modules. Modules are stored in directories Each directory contains a __init__. py file which tells the Python interpreter that it is a package directory.
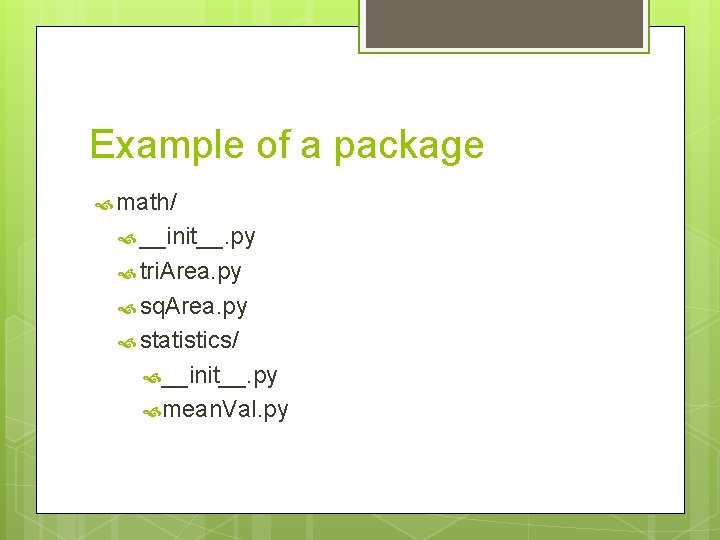
Example of a package math/ __init__. py tri. Area. py sq. Area. py statistics/ __init__. py mean. Val. py
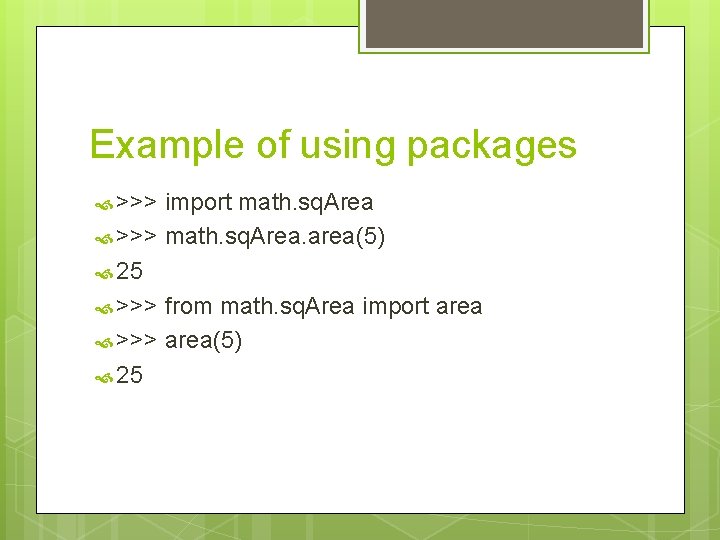
Example of using packages >>> import math. sq. Area >>> math. sq. Area. area(5) 25 >>> from math. sq. Area import area >>> area(5) 25
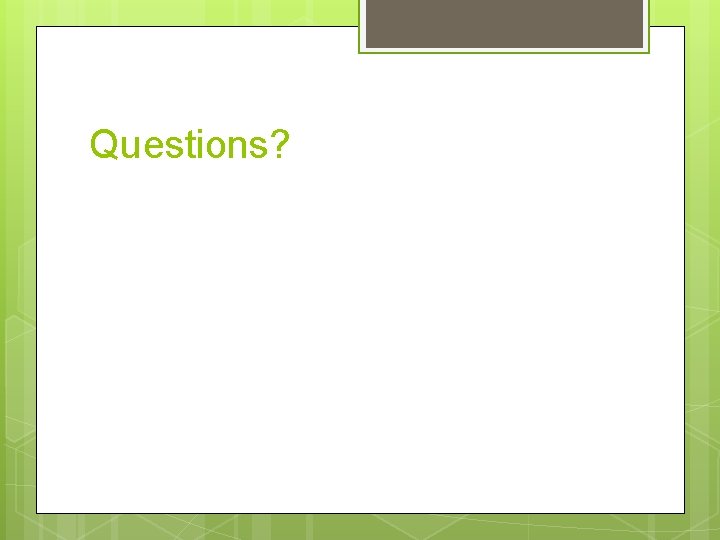
Questions?
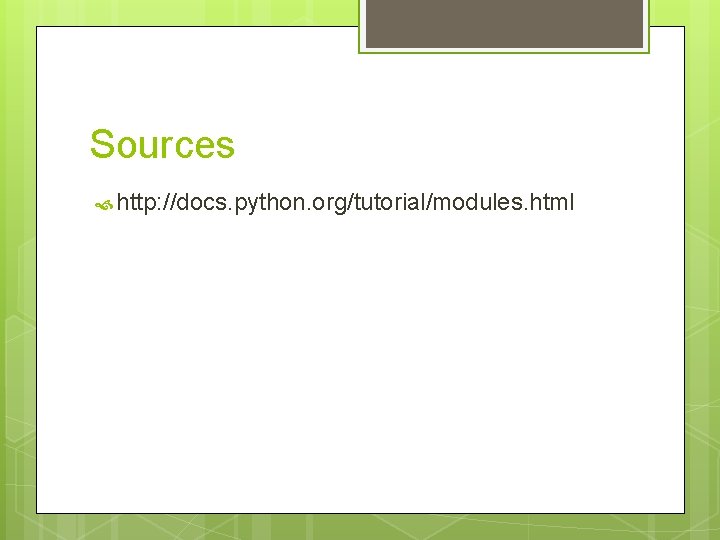
Sources http: //docs. python. org/tutorial/modules. html
Insidan region jh
Ncic hosts restricted files and non-restricted files
File mode python
Cjis level 4 certification
Open python files
Finished files are the result
Edl files
Ibverbs header files not found
File handling in c language
Eznec antenna
Azure files deduplication
Attach2dynamics
Bash startup files
Lrk v4 trojans
Steve reich midi files
Flash to unity converter