Programming with Shared Memory Java Threads and Synchronization
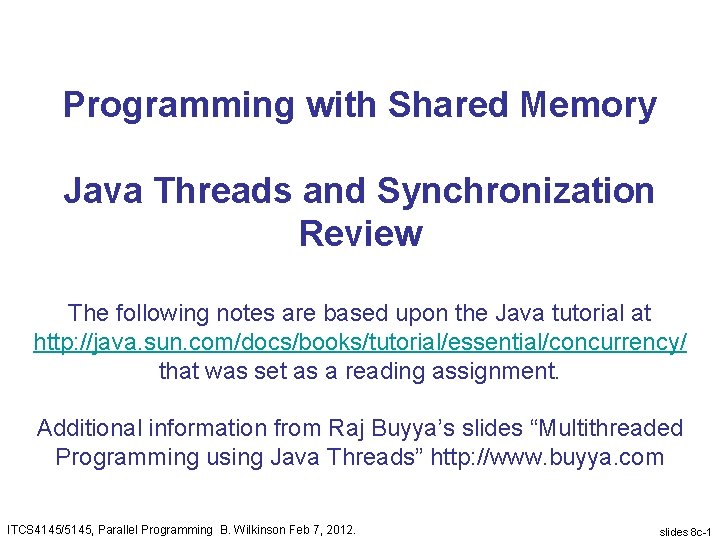
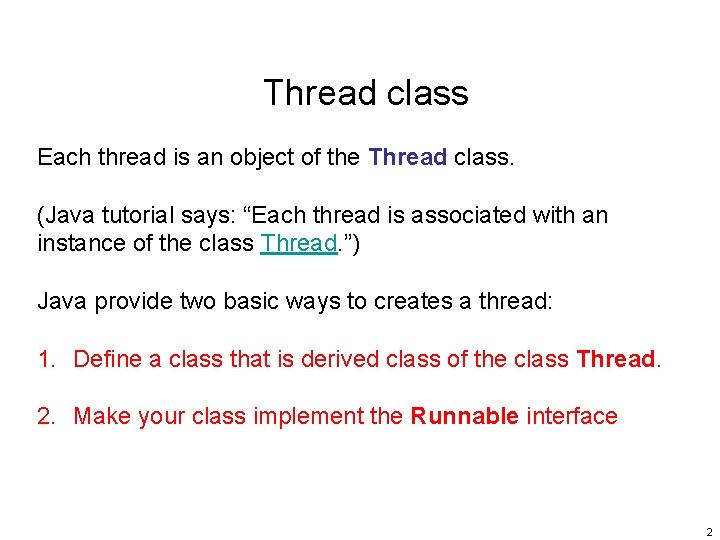
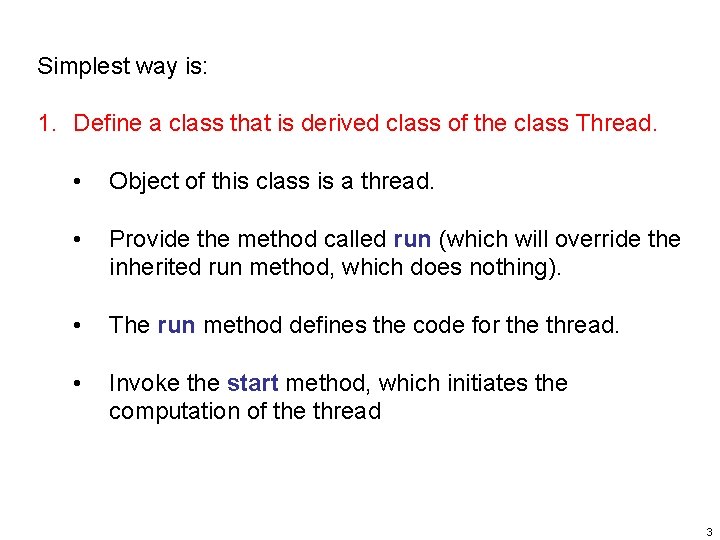
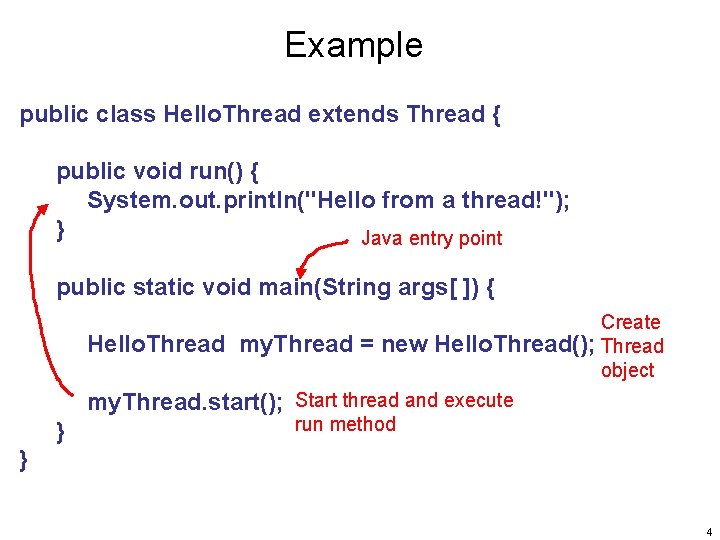
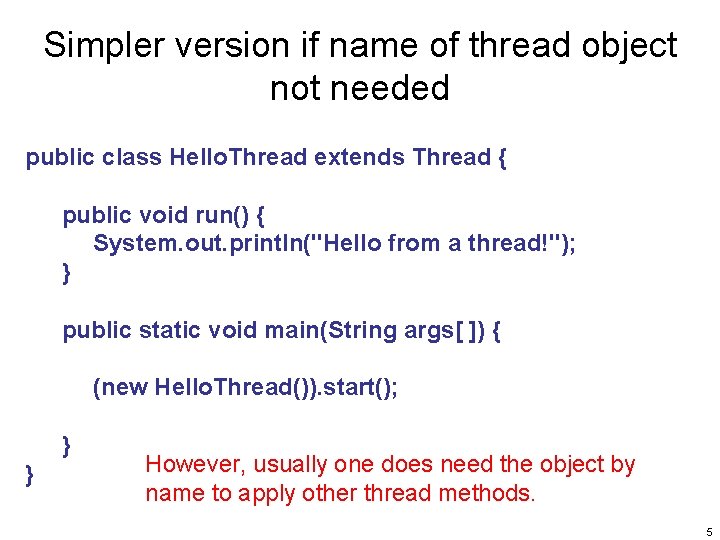
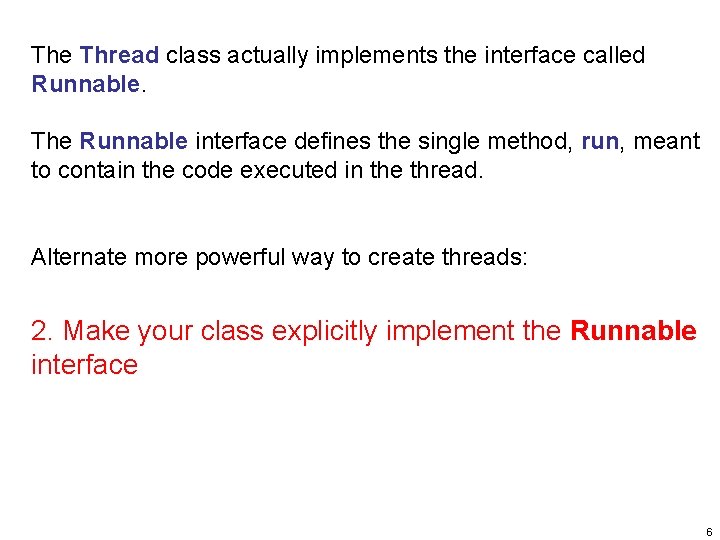
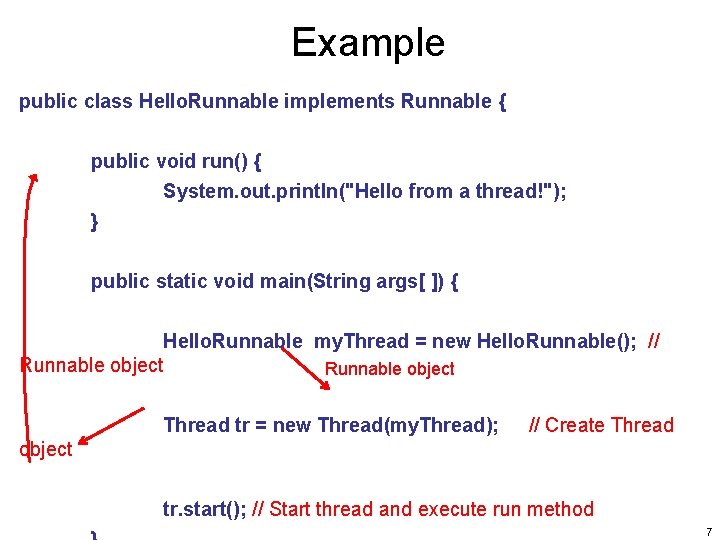
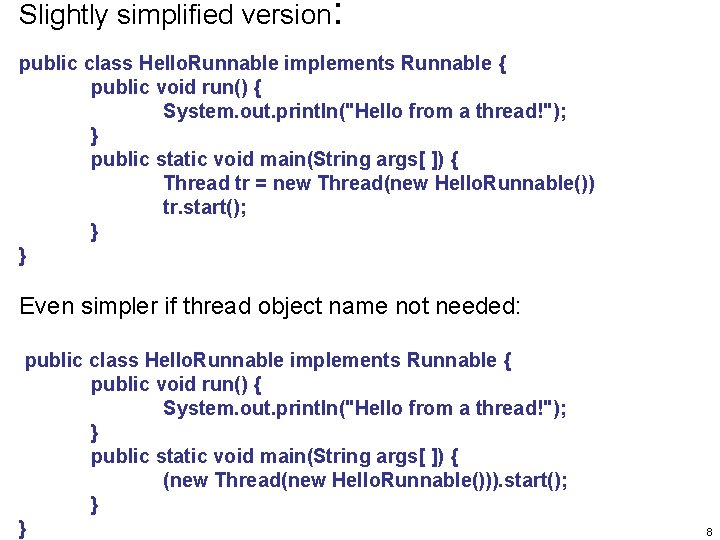
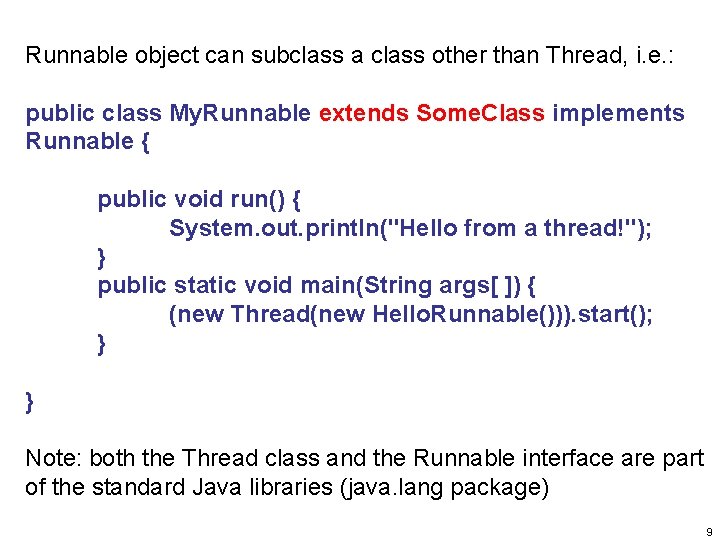
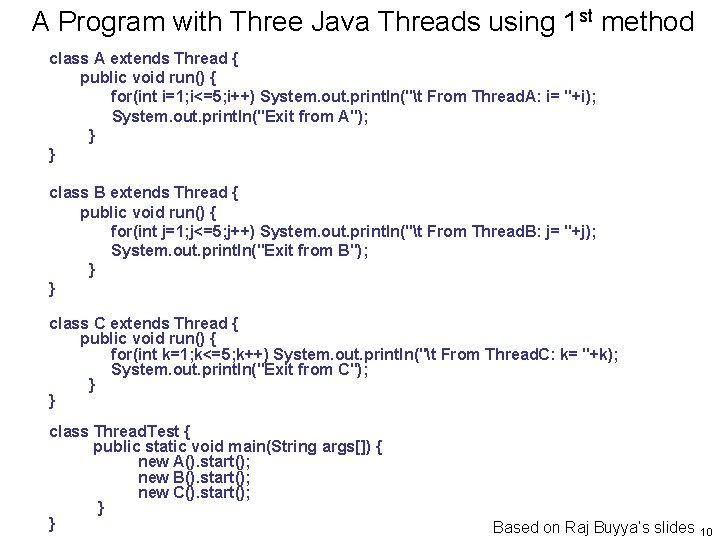
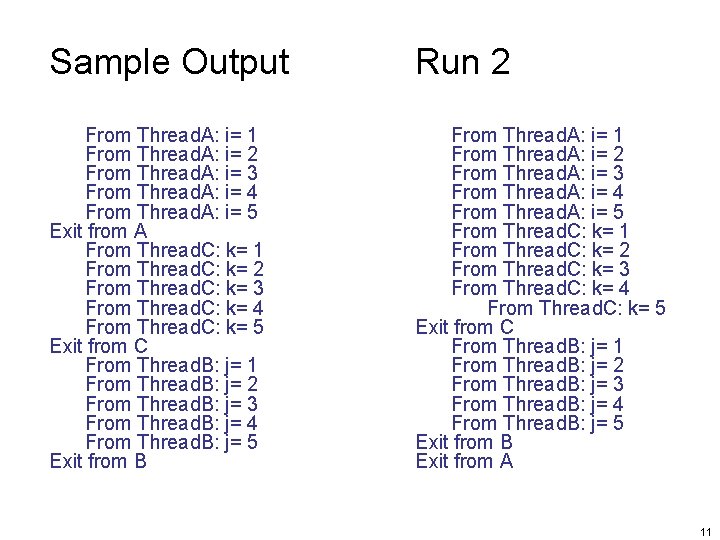
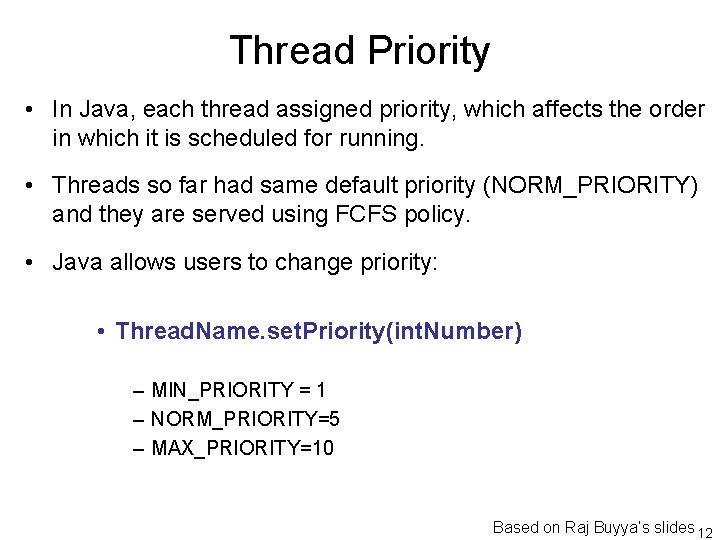
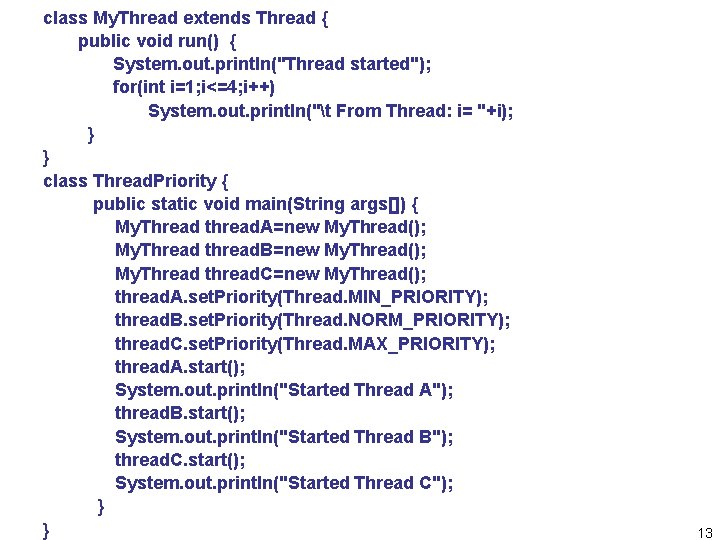
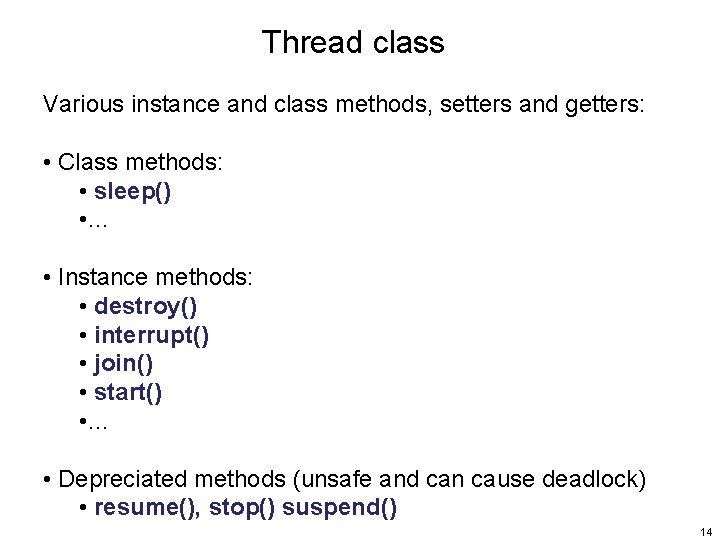
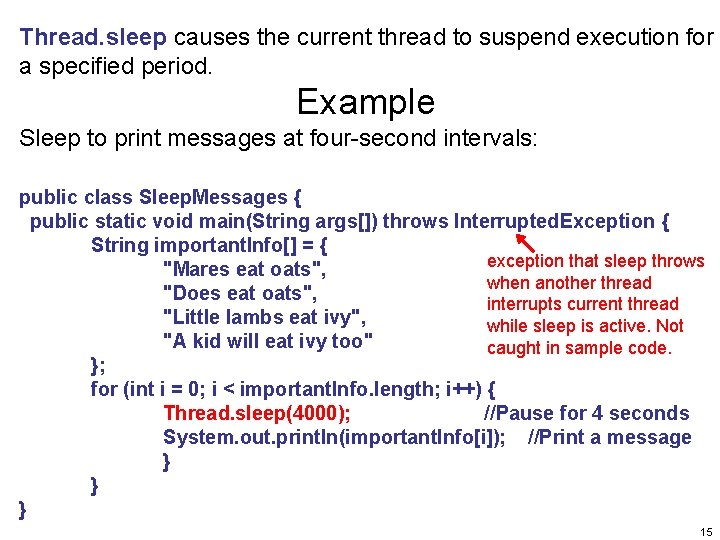
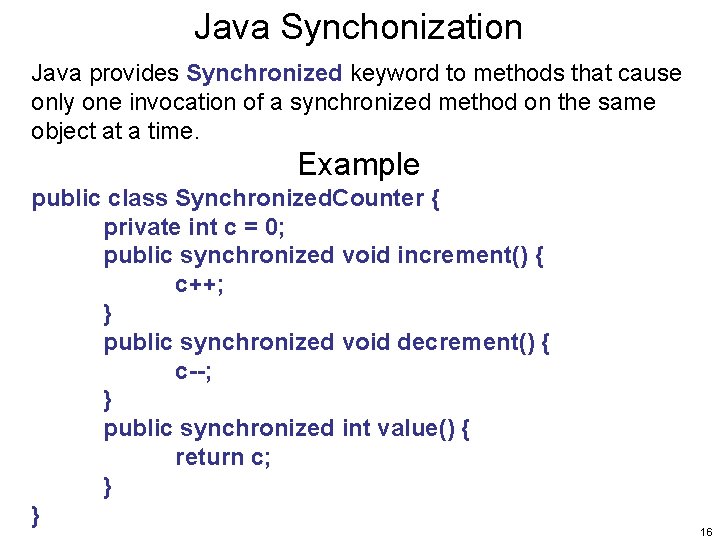
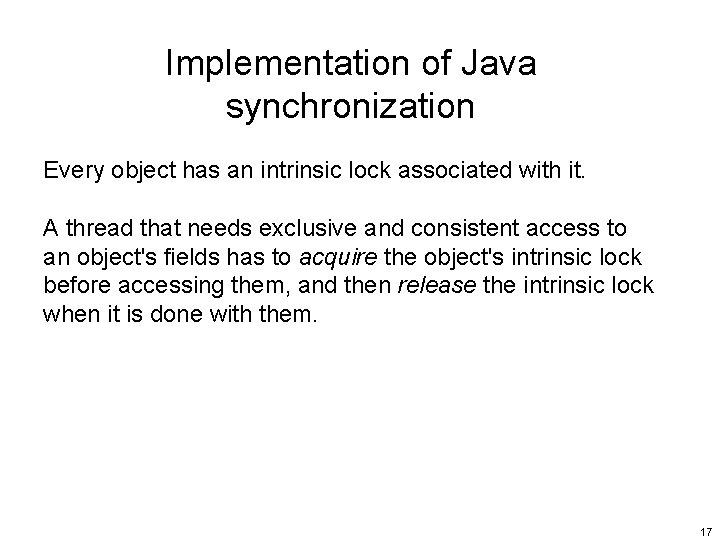
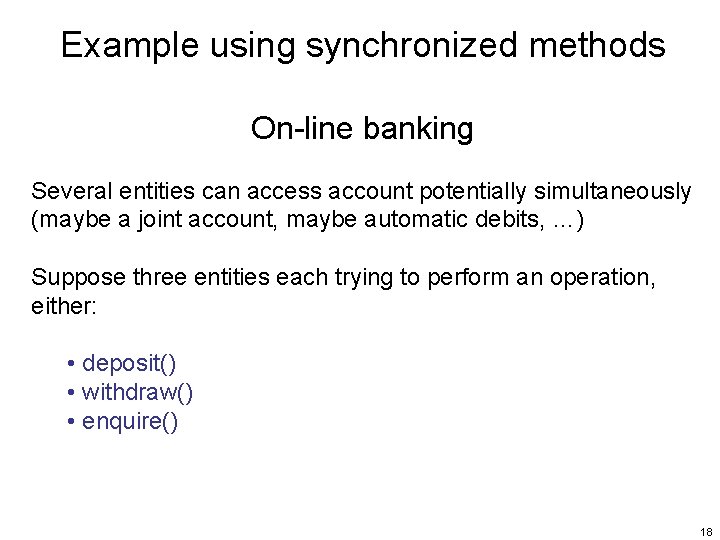
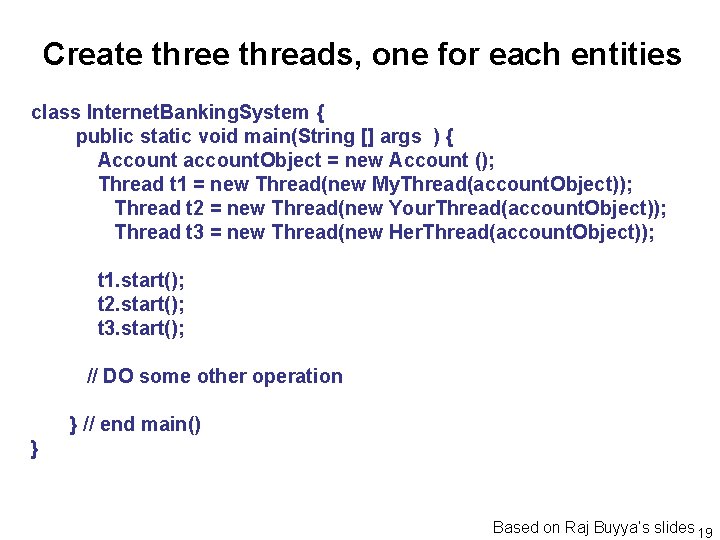
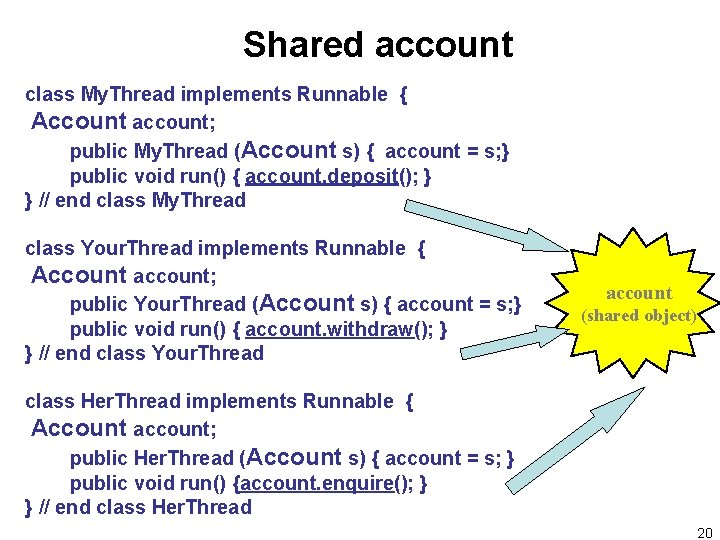
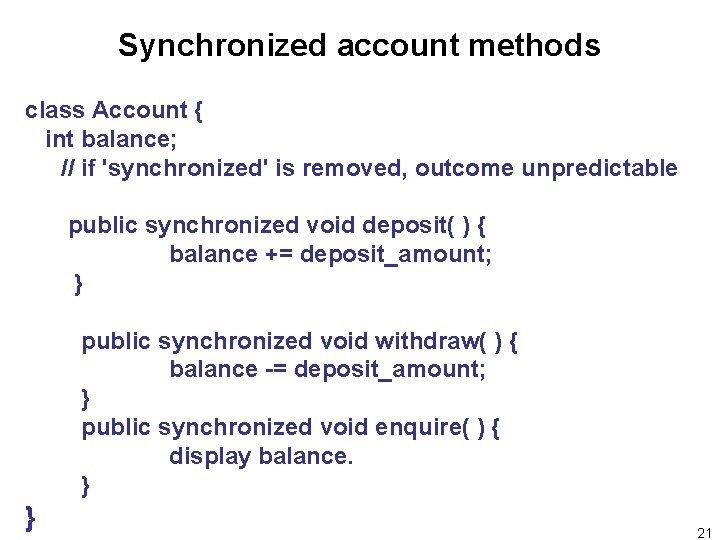
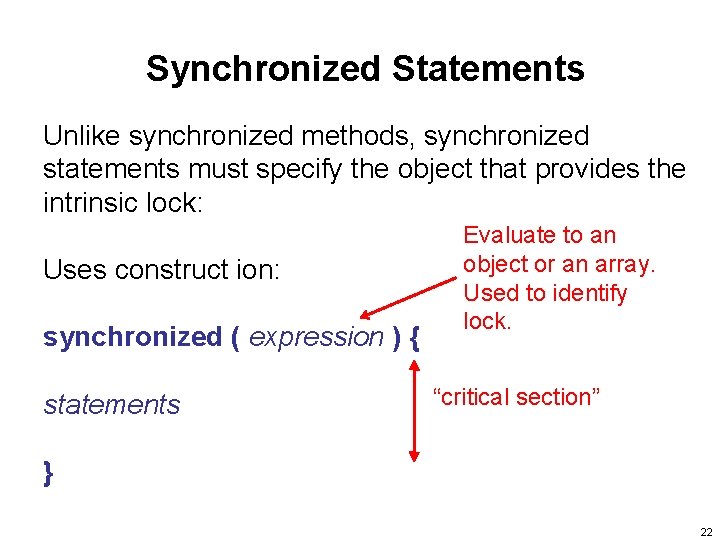
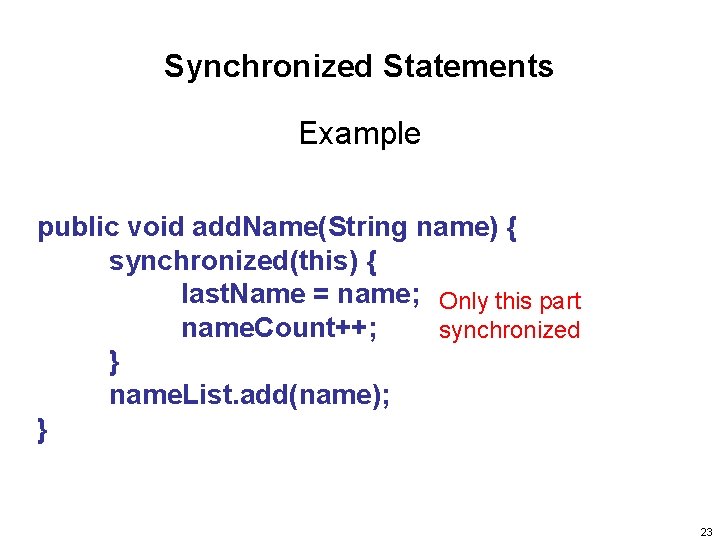
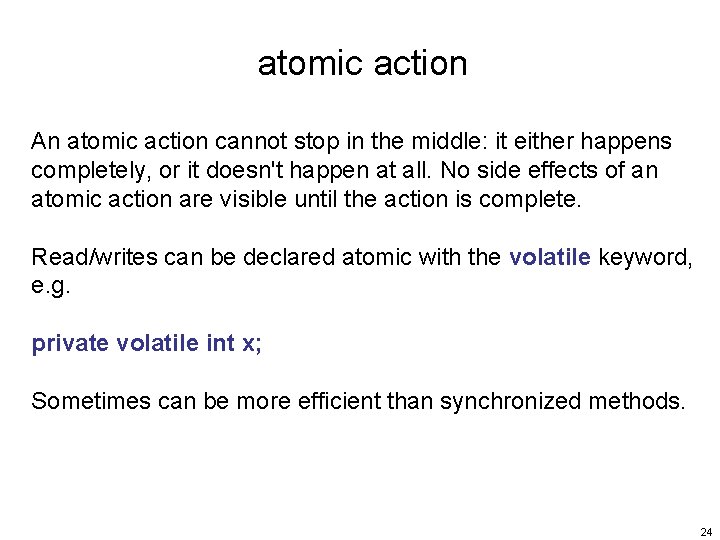
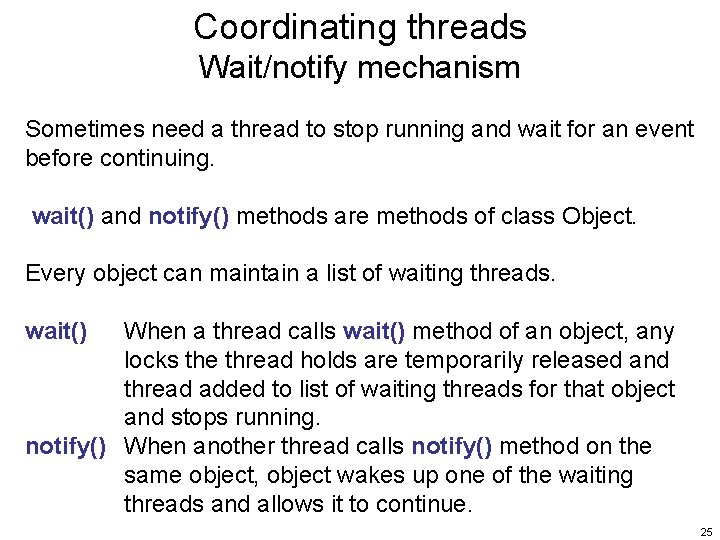
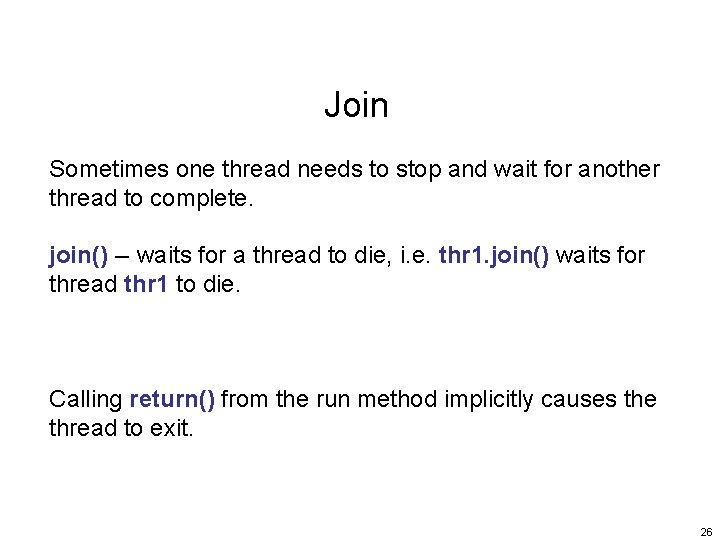
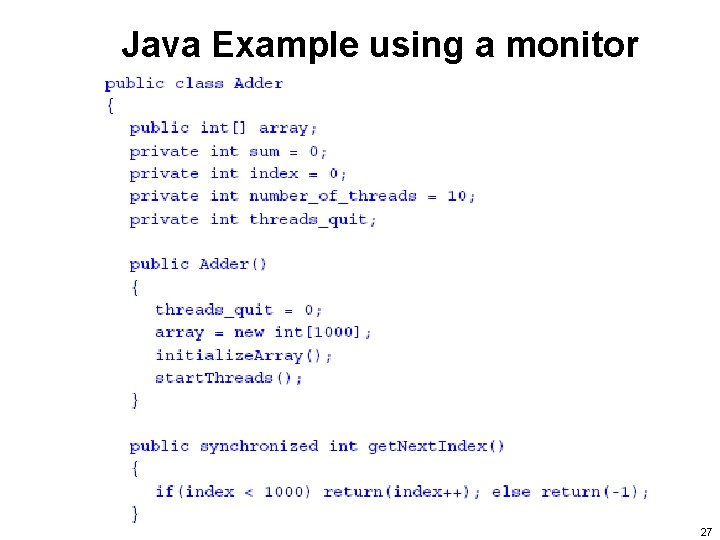
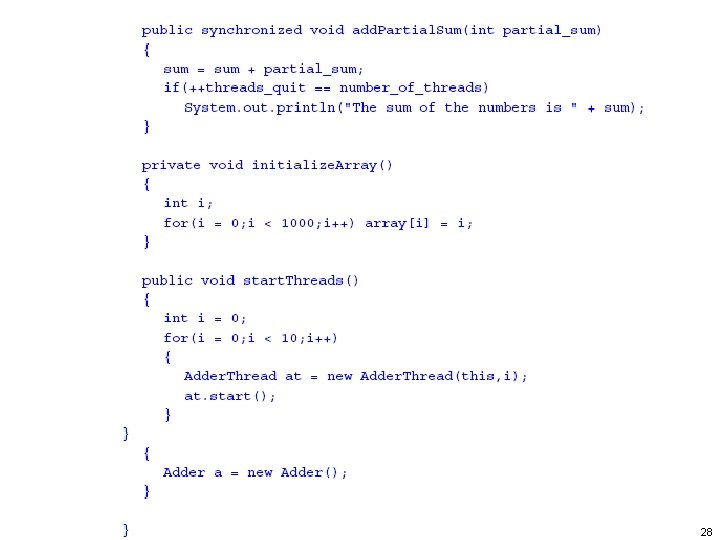
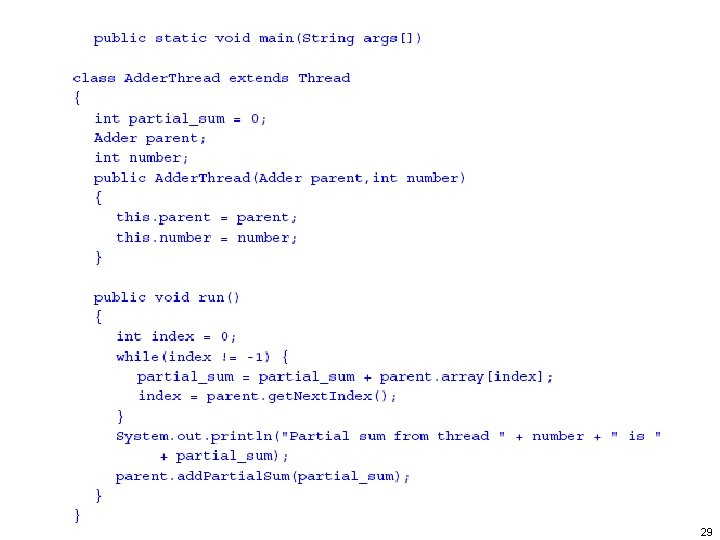
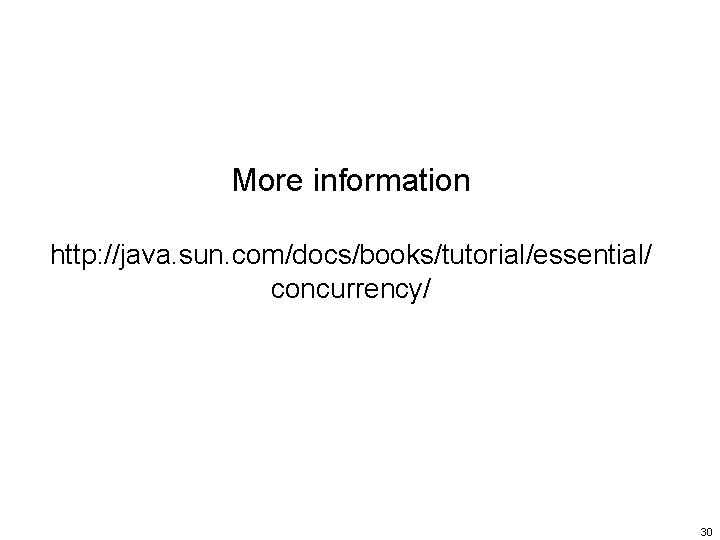
- Slides: 30
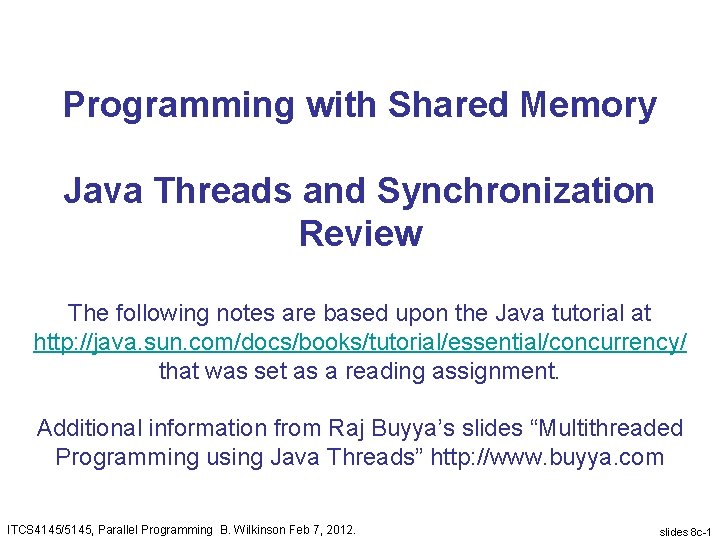
Programming with Shared Memory Java Threads and Synchronization Review The following notes are based upon the Java tutorial at http: //java. sun. com/docs/books/tutorial/essential/concurrency/ that was set as a reading assignment. Additional information from Raj Buyya’s slides “Multithreaded Programming using Java Threads” http: //www. buyya. com ITCS 4145/5145, Parallel Programming B. Wilkinson Feb 7, 2012. slides 8 c-1
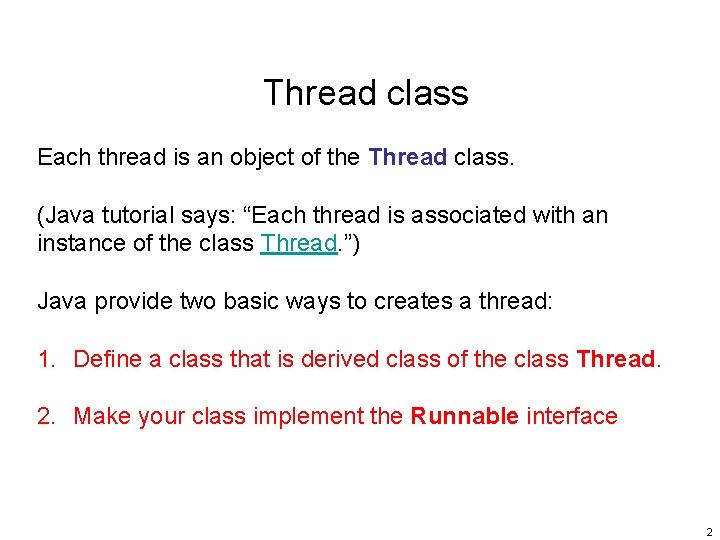
Thread class Each thread is an object of the Thread class. (Java tutorial says: “Each thread is associated with an instance of the class Thread. ”) Java provide two basic ways to creates a thread: 1. Define a class that is derived class of the class Thread. 2. Make your class implement the Runnable interface 2
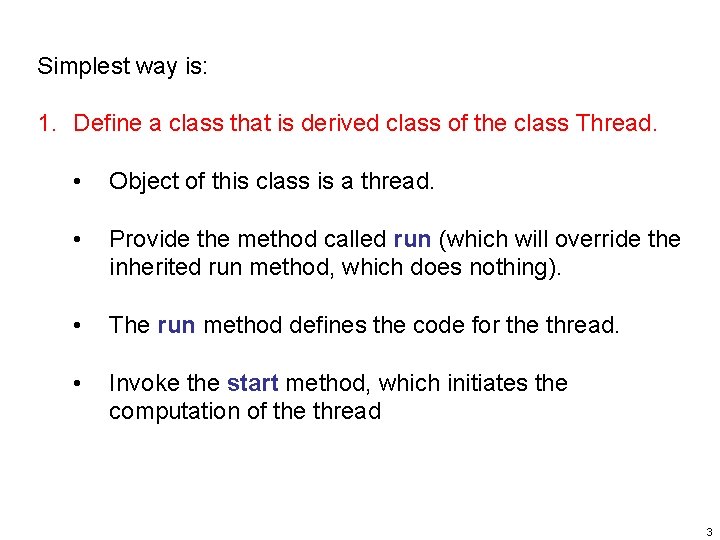
Simplest way is: 1. Define a class that is derived class of the class Thread. • Object of this class is a thread. • Provide the method called run (which will override the inherited run method, which does nothing). • The run method defines the code for the thread. • Invoke the start method, which initiates the computation of the thread 3
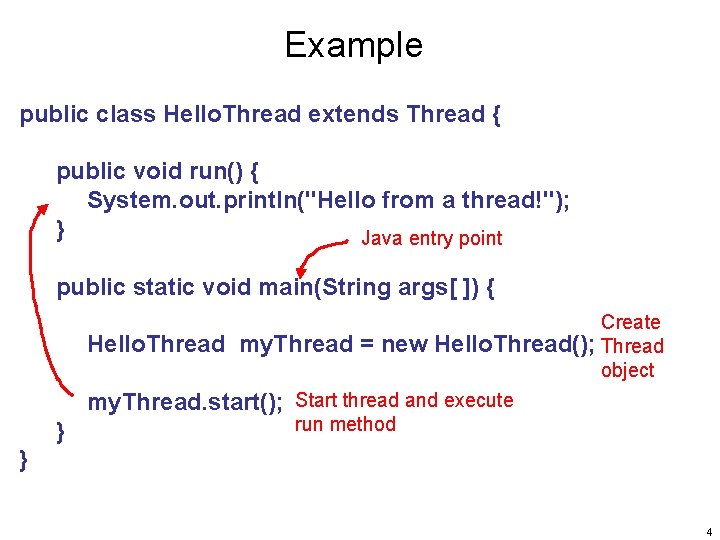
Example public class Hello. Thread extends Thread { public void run() { System. out. println("Hello from a thread!"); } Java entry point public static void main(String args[ ]) { } Create Hello. Thread my. Thread = new Hello. Thread(); Thread object my. Thread. start(); Start thread and execute run method } 4
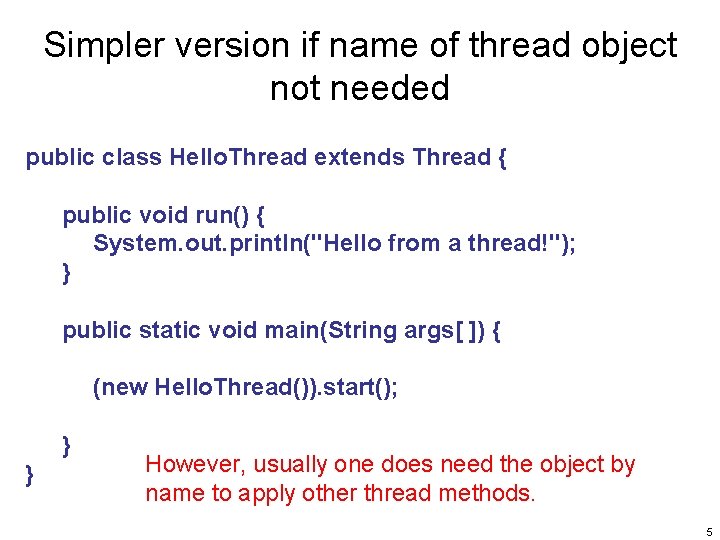
Simpler version if name of thread object not needed public class Hello. Thread extends Thread { public void run() { System. out. println("Hello from a thread!"); } public static void main(String args[ ]) { (new Hello. Thread()). start(); } } However, usually one does need the object by name to apply other thread methods. 5
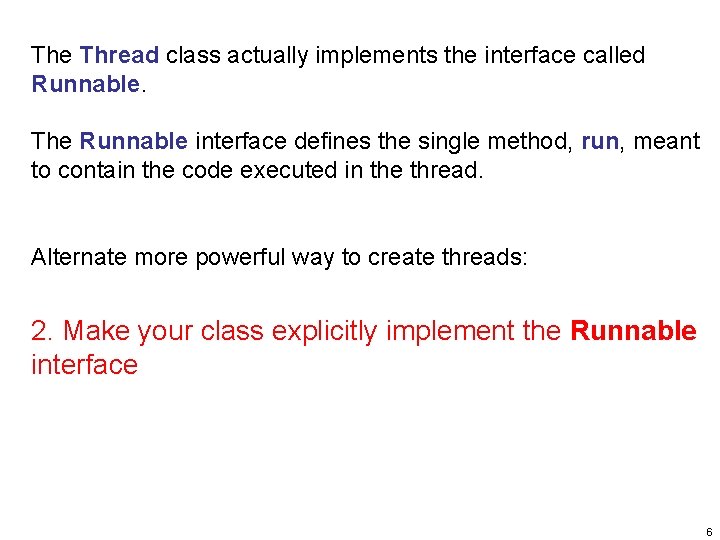
The Thread class actually implements the interface called Runnable. The Runnable interface defines the single method, run, meant to contain the code executed in the thread. Alternate more powerful way to create threads: 2. Make your class explicitly implement the Runnable interface 6
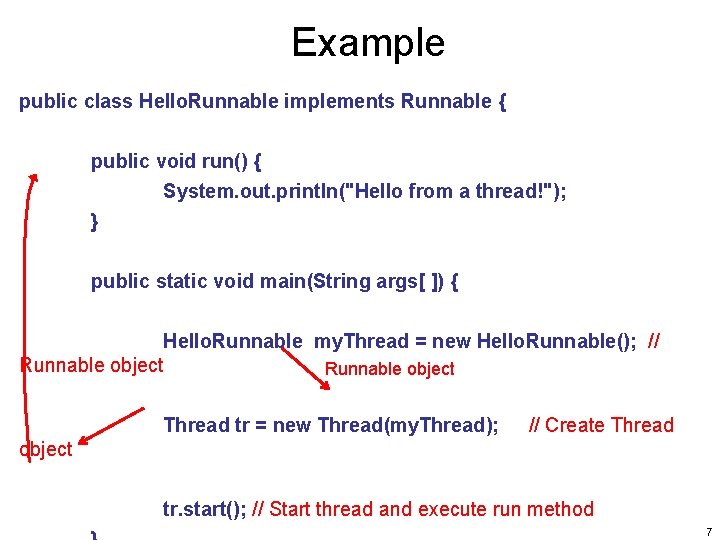
Example public class Hello. Runnable implements Runnable { public void run() { System. out. println("Hello from a thread!"); } public static void main(String args[ ]) { Hello. Runnable my. Thread = new Hello. Runnable(); // Runnable object Thread tr = new Thread(my. Thread); // Create Thread object tr. start(); // Start thread and execute run method 7
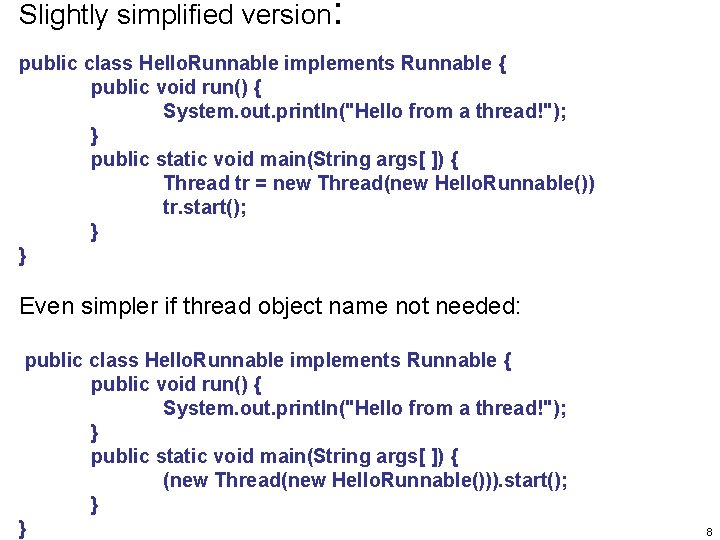
Slightly simplified version: public class Hello. Runnable implements Runnable { public void run() { System. out. println("Hello from a thread!"); } public static void main(String args[ ]) { Thread tr = new Thread(new Hello. Runnable()) tr. start(); } } Even simpler if thread object name not needed: public class Hello. Runnable implements Runnable { public void run() { System. out. println("Hello from a thread!"); } public static void main(String args[ ]) { (new Thread(new Hello. Runnable())). start(); } } 8
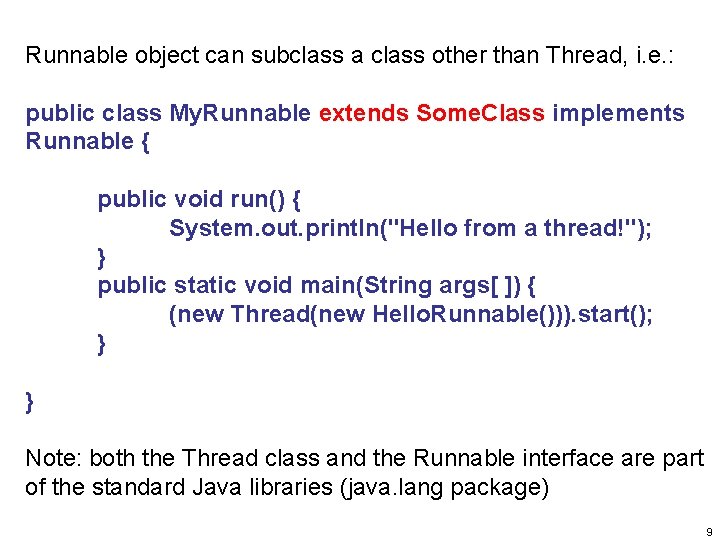
Runnable object can subclass a class other than Thread, i. e. : public class My. Runnable extends Some. Class implements Runnable { public void run() { System. out. println("Hello from a thread!"); } public static void main(String args[ ]) { (new Thread(new Hello. Runnable())). start(); } } Note: both the Thread class and the Runnable interface are part of the standard Java libraries (java. lang package) 9
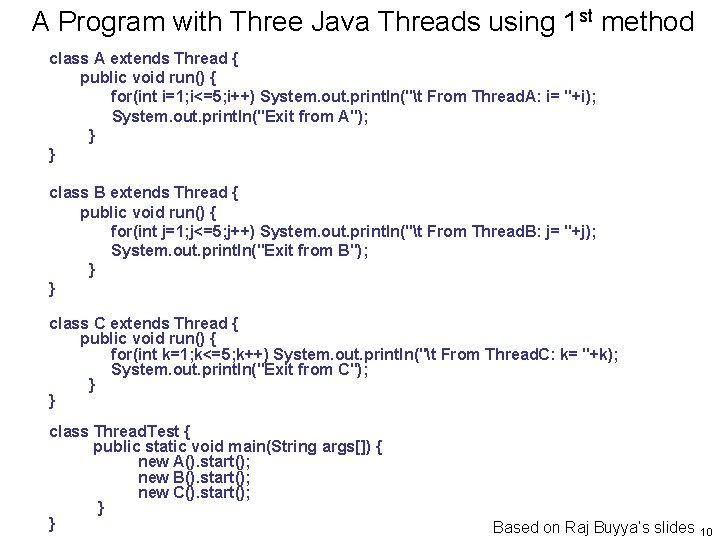
A Program with Three Java Threads using 1 st method class A extends Thread { public void run() { for(int i=1; i<=5; i++) System. out. println("t From Thread. A: i= "+i); System. out. println("Exit from A"); } } class B extends Thread { public void run() { for(int j=1; j<=5; j++) System. out. println("t From Thread. B: j= "+j); System. out. println("Exit from B"); } } class C extends Thread { public void run() { for(int k=1; k<=5; k++) System. out. println("t From Thread. C: k= "+k); System. out. println("Exit from C"); } } class Thread. Test { public static void main(String args[]) { new A(). start(); new B(). start(); new C(). start(); } } Based on Raj Buyya’s slides 10
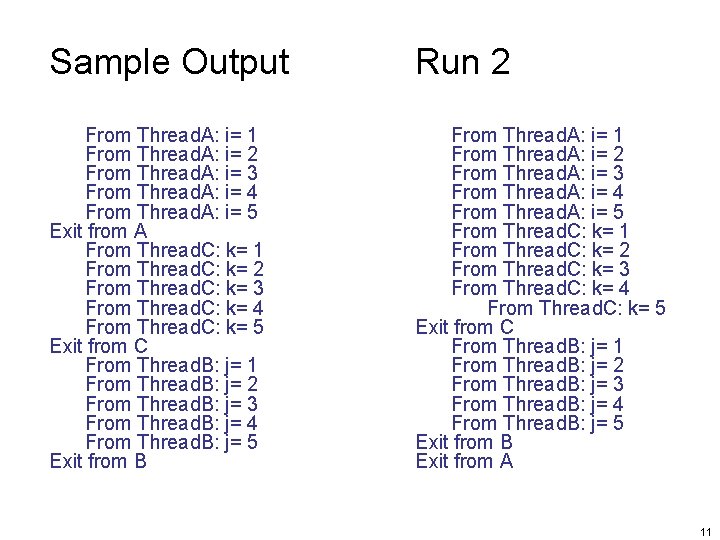
Sample Output Run 2 From Thread. A: i= 1 From Thread. A: i= 2 From Thread. A: i= 3 From Thread. A: i= 4 From Thread. A: i= 5 Exit from A From Thread. C: k= 1 From Thread. C: k= 2 From Thread. C: k= 3 From Thread. C: k= 4 From Thread. C: k= 5 Exit from C From Thread. B: j= 1 From Thread. B: j= 2 From Thread. B: j= 3 From Thread. B: j= 4 From Thread. B: j= 5 Exit from B From Thread. A: i= 1 From Thread. A: i= 2 From Thread. A: i= 3 From Thread. A: i= 4 From Thread. A: i= 5 From Thread. C: k= 1 From Thread. C: k= 2 From Thread. C: k= 3 From Thread. C: k= 4 From Thread. C: k= 5 Exit from C From Thread. B: j= 1 From Thread. B: j= 2 From Thread. B: j= 3 From Thread. B: j= 4 From Thread. B: j= 5 Exit from B Exit from A 11
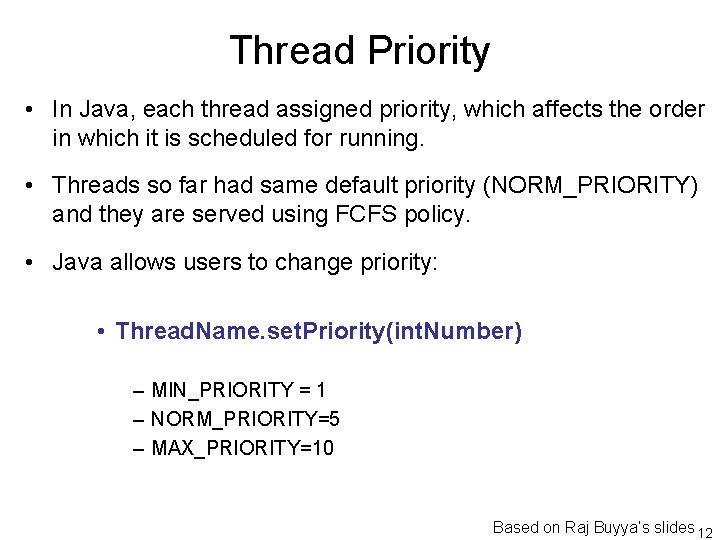
Thread Priority • In Java, each thread assigned priority, which affects the order in which it is scheduled for running. • Threads so far had same default priority (NORM_PRIORITY) and they are served using FCFS policy. • Java allows users to change priority: • Thread. Name. set. Priority(int. Number) – MIN_PRIORITY = 1 – NORM_PRIORITY=5 – MAX_PRIORITY=10 Based on Raj Buyya’s slides 12
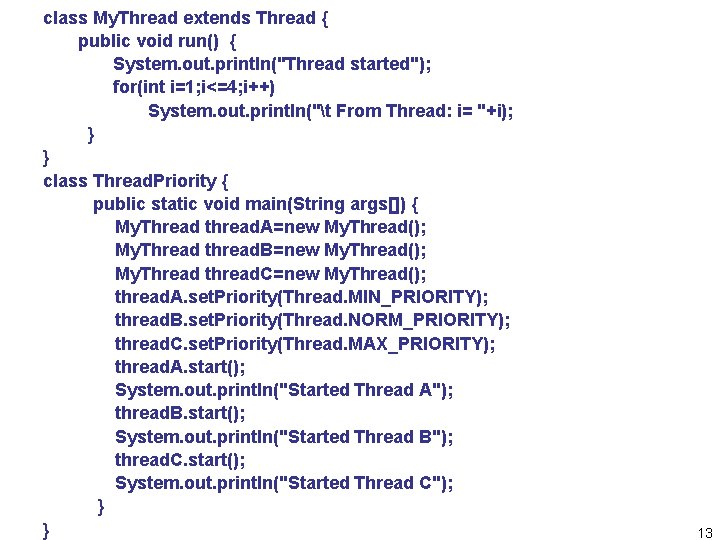
class My. Thread extends Thread { public void run() { System. out. println("Thread started"); for(int i=1; i<=4; i++) System. out. println("t From Thread: i= "+i); } } class Thread. Priority { public static void main(String args[]) { My. Thread thread. A=new My. Thread(); My. Thread thread. B=new My. Thread(); My. Thread thread. C=new My. Thread(); thread. A. set. Priority(Thread. MIN_PRIORITY); thread. B. set. Priority(Thread. NORM_PRIORITY); thread. C. set. Priority(Thread. MAX_PRIORITY); thread. A. start(); System. out. println("Started Thread A"); thread. B. start(); System. out. println("Started Thread B"); thread. C. start(); System. out. println("Started Thread C"); } } 13
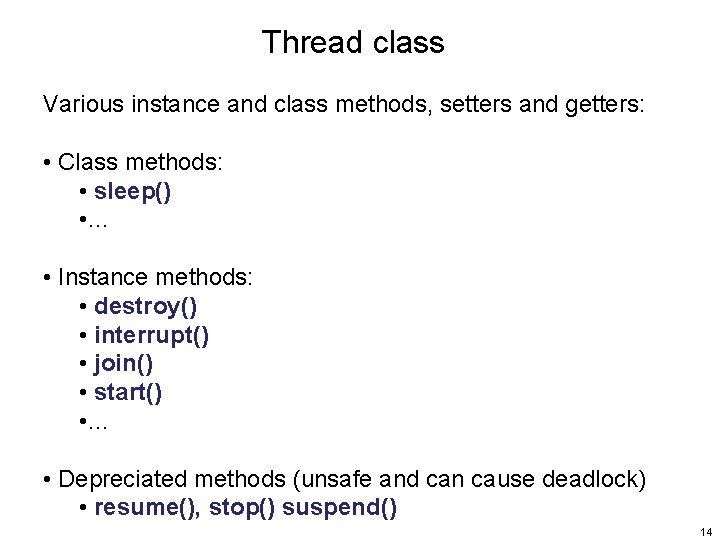
Thread class Various instance and class methods, setters and getters: • Class methods: • sleep() • … • Instance methods: • destroy() • interrupt() • join() • start() • … • Depreciated methods (unsafe and can cause deadlock) • resume(), stop() suspend() 14
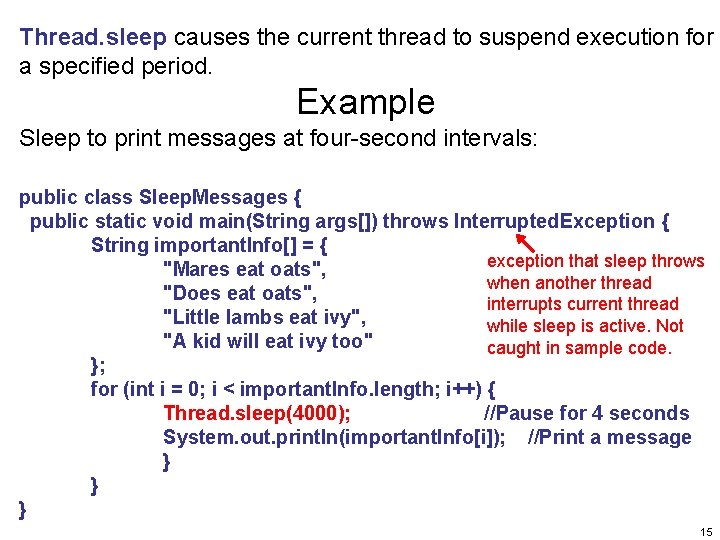
Thread. sleep causes the current thread to suspend execution for a specified period. Example Sleep to print messages at four-second intervals: public class Sleep. Messages { public static void main(String args[]) throws Interrupted. Exception { String important. Info[] = { exception that sleep throws "Mares eat oats", when another thread "Does eat oats", interrupts current thread "Little lambs eat ivy", while sleep is active. Not "A kid will eat ivy too" caught in sample code. }; for (int i = 0; i < important. Info. length; i++) { Thread. sleep(4000); //Pause for 4 seconds System. out. println(important. Info[i]); //Print a message } } } 15
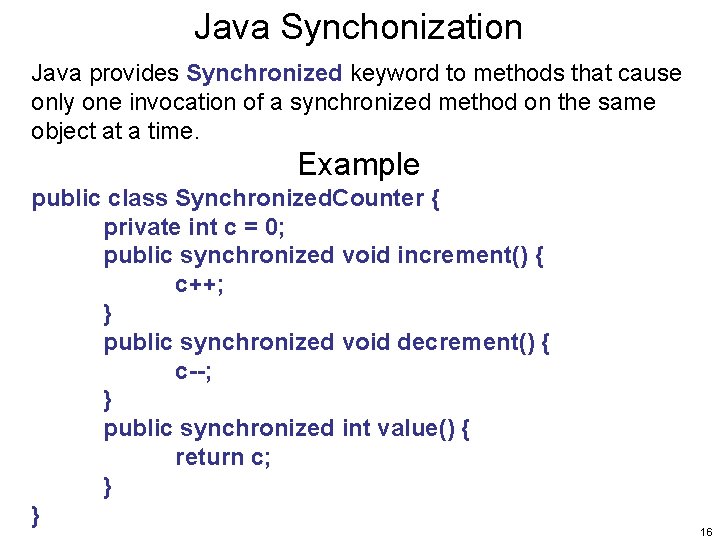
Java Synchonization Java provides Synchronized keyword to methods that cause only one invocation of a synchronized method on the same object at a time. Example public class Synchronized. Counter { private int c = 0; public synchronized void increment() { c++; } public synchronized void decrement() { c--; } public synchronized int value() { return c; } } 16
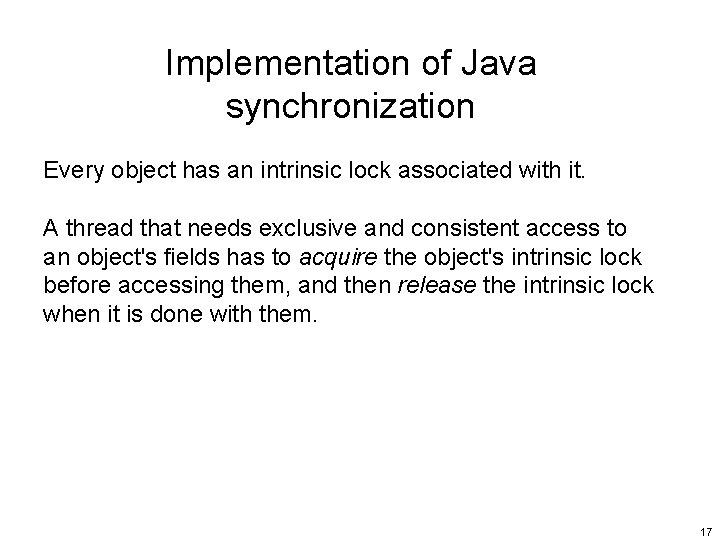
Implementation of Java synchronization Every object has an intrinsic lock associated with it. A thread that needs exclusive and consistent access to an object's fields has to acquire the object's intrinsic lock before accessing them, and then release the intrinsic lock when it is done with them. 17
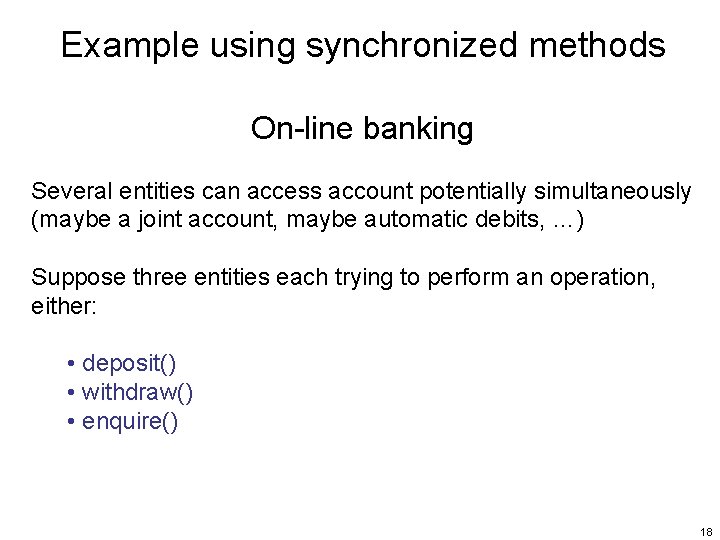
Example using synchronized methods On-line banking Several entities can access account potentially simultaneously (maybe a joint account, maybe automatic debits, …) Suppose three entities each trying to perform an operation, either: • deposit() • withdraw() • enquire() 18
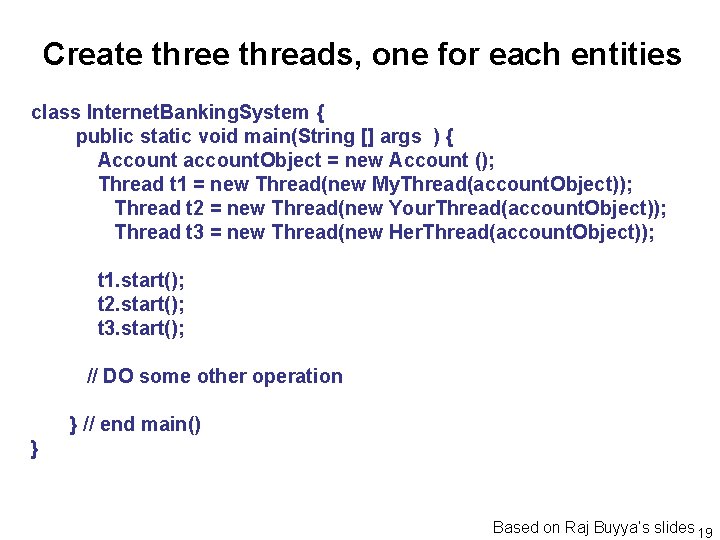
Create threads, one for each entities class Internet. Banking. System { public static void main(String [] args ) { Account account. Object = new Account (); Thread t 1 = new Thread(new My. Thread(account. Object)); Thread t 2 = new Thread(new Your. Thread(account. Object)); Thread t 3 = new Thread(new Her. Thread(account. Object)); t 1. start(); t 2. start(); t 3. start(); // DO some other operation } // end main() } Based on Raj Buyya’s slides 19
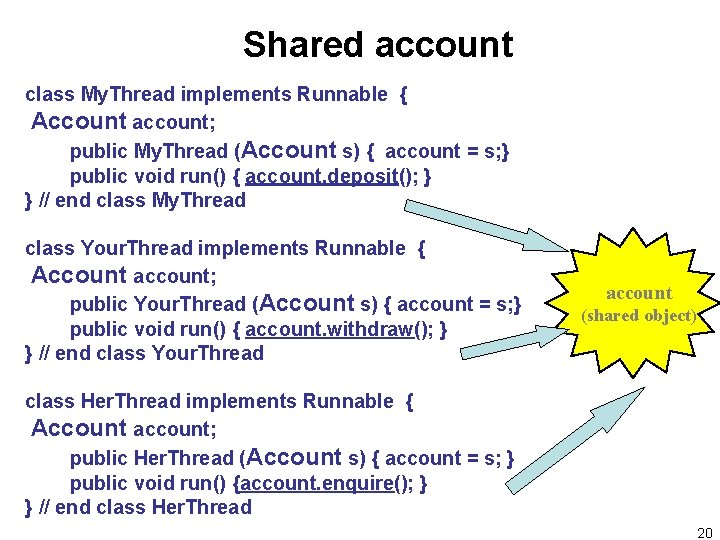
Shared account class My. Thread implements Runnable { Account account; public My. Thread (Account s) { account = s; } public void run() { account. deposit(); } } // end class My. Thread class Your. Thread implements Runnable { Account account; public Your. Thread (Account s) { account = s; } public void run() { account. withdraw(); } } // end class Your. Thread account (shared object) class Her. Thread implements Runnable { Account account; public Her. Thread (Account s) { account = s; } public void run() {account. enquire(); } } // end class Her. Thread 20
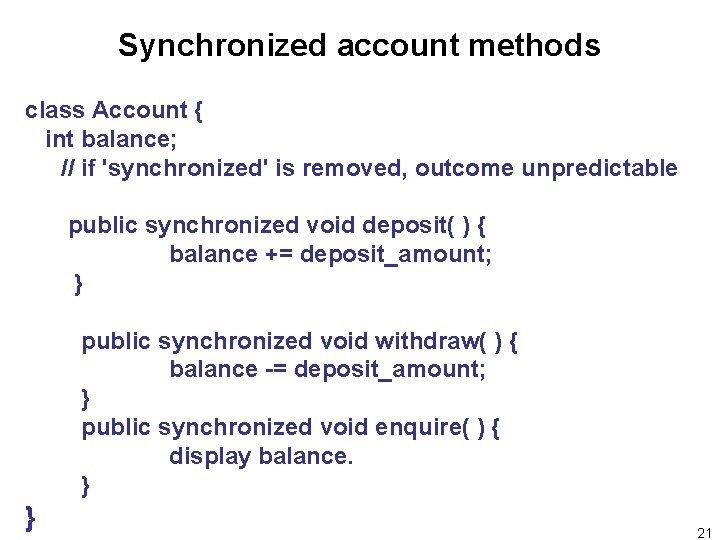
Synchronized account methods class Account { int balance; // if 'synchronized' is removed, outcome unpredictable public synchronized void deposit( ) { balance += deposit_amount; } public synchronized void withdraw( ) { balance -= deposit_amount; } public synchronized void enquire( ) { display balance. } } 21
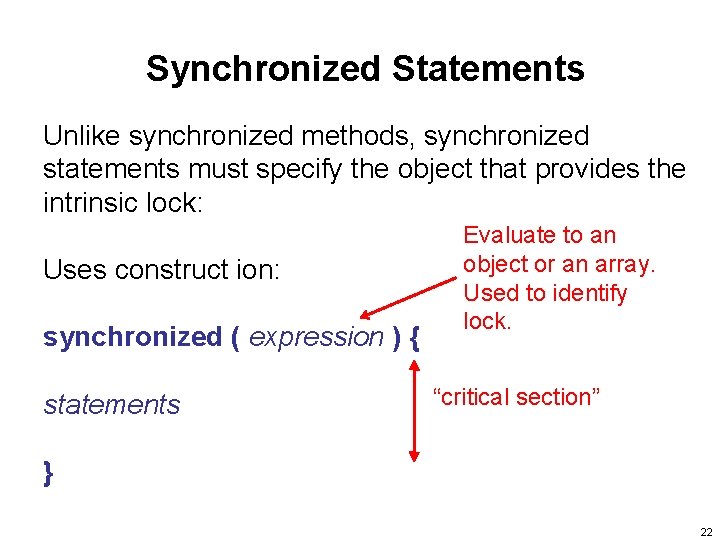
Synchronized Statements Unlike synchronized methods, synchronized statements must specify the object that provides the intrinsic lock: Uses construct ion: synchronized ( expression ) { statements Evaluate to an object or an array. Used to identify lock. “critical section” } 22
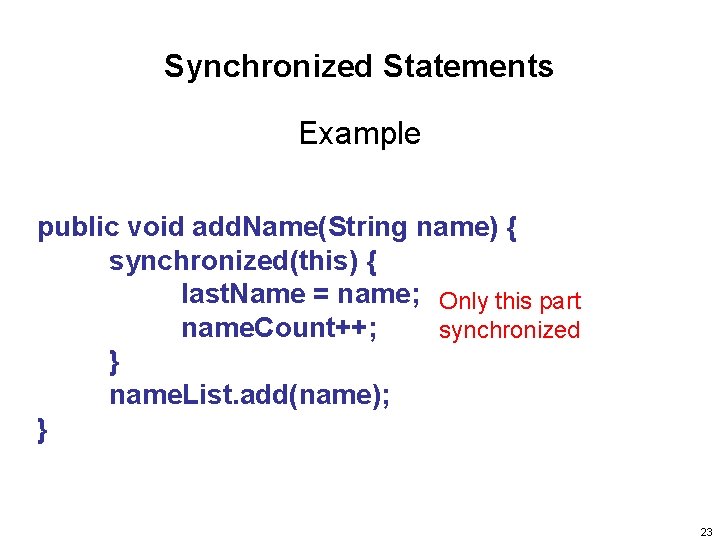
Synchronized Statements Example public void add. Name(String name) { synchronized(this) { last. Name = name; Only this part name. Count++; synchronized } name. List. add(name); } 23
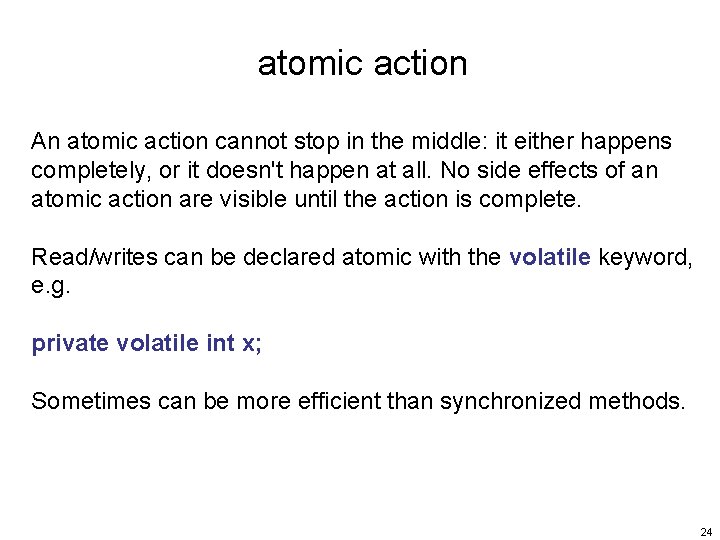
atomic action An atomic action cannot stop in the middle: it either happens completely, or it doesn't happen at all. No side effects of an atomic action are visible until the action is complete. Read/writes can be declared atomic with the volatile keyword, e. g. private volatile int x; Sometimes can be more efficient than synchronized methods. 24
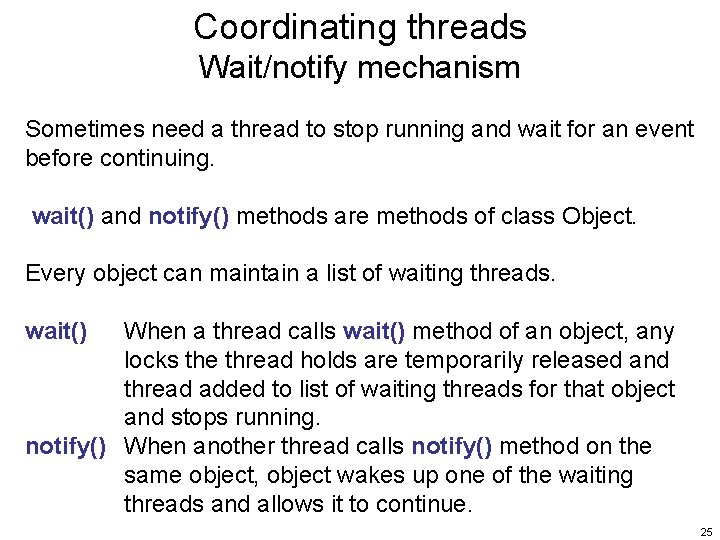
Coordinating threads Wait/notify mechanism Sometimes need a thread to stop running and wait for an event before continuing. wait() and notify() methods are methods of class Object. Every object can maintain a list of waiting threads. wait() When a thread calls wait() method of an object, any locks the thread holds are temporarily released and thread added to list of waiting threads for that object and stops running. notify() When another thread calls notify() method on the same object, object wakes up one of the waiting threads and allows it to continue. 25
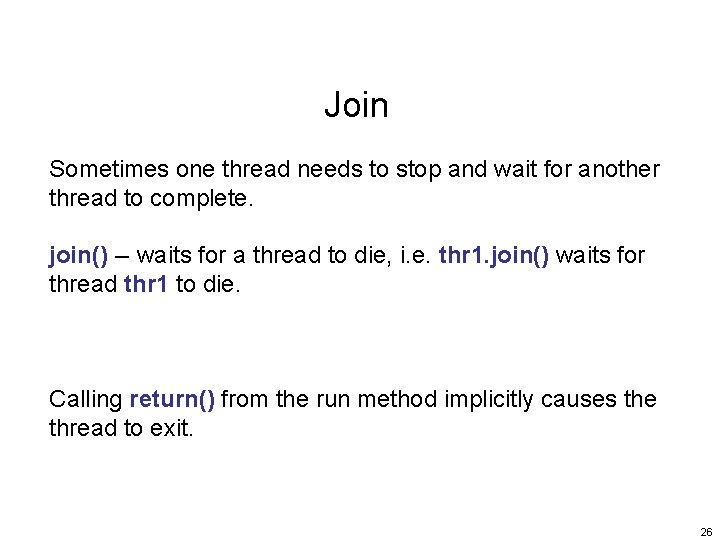
Join Sometimes one thread needs to stop and wait for another thread to complete. join() -- waits for a thread to die, i. e. thr 1. join() waits for thread thr 1 to die. Calling return() from the run method implicitly causes the thread to exit. 26
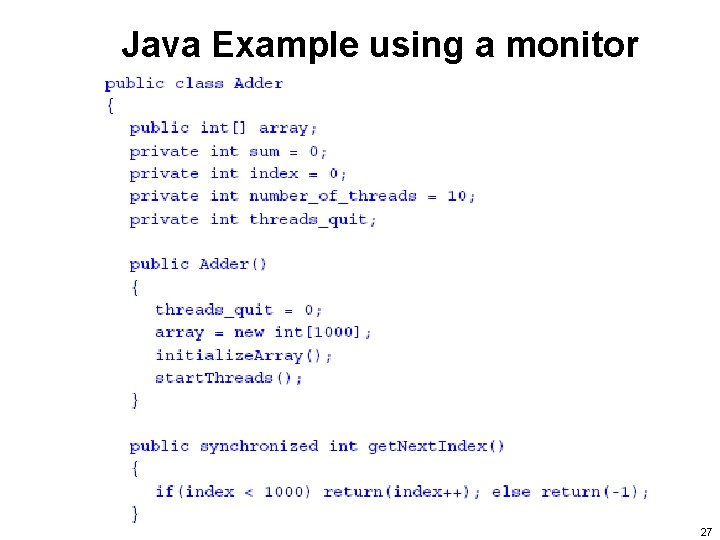
Java Example using a monitor 27
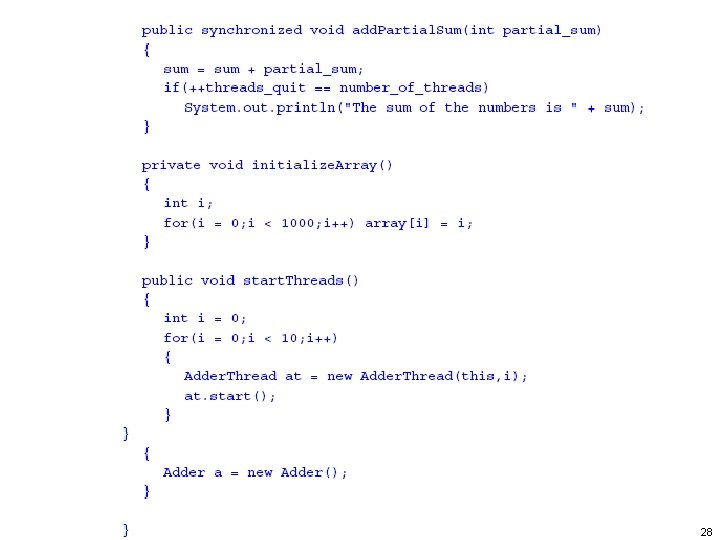
28
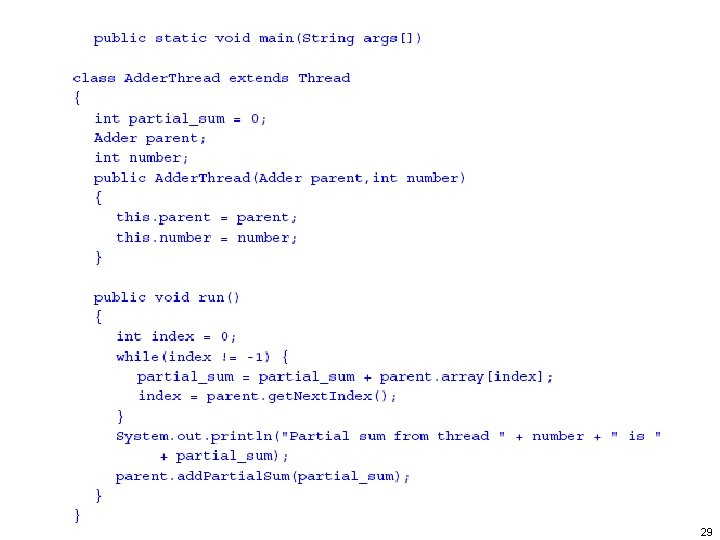
29
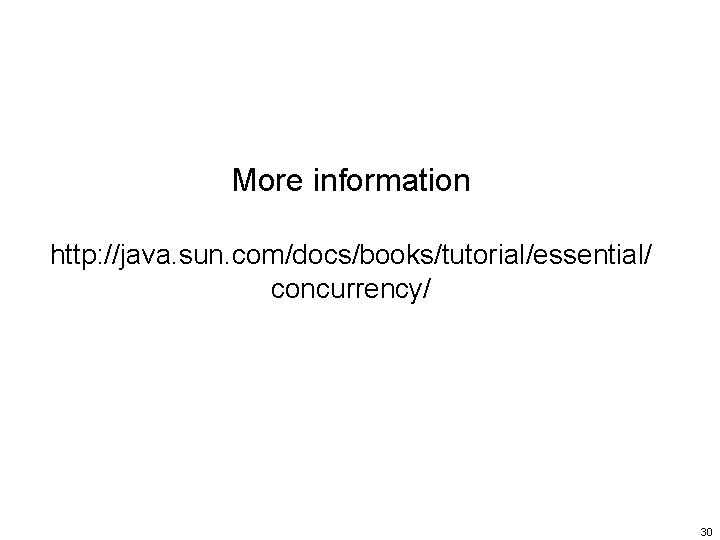
More information http: //java. sun. com/docs/books/tutorial/essential/ concurrency/ 30
Shared memory in java
Shared memory in unix
Shared vs distributed memory
Pthreads tutorial
Synchronization algorithms and concurrent programming
Threads java
Threads em java
Distributed shared memory architecture tutorialspoint
Share a single centralized memory
Shared memory linux
Symmetric shared memory architecture
Cuda bank conflict
Symmetric shared memory architecture
Distributed shared memory
Distributed shared memory
Shared memory nedir
Design issues of distributed shared memory
Acc shared memory
Shared virtual memory
Advantages and disadvantages of mimd
Show the detailed abstract view of dsm
What is shared memory
Memory mapped file
Internal memory and external memory
Primary memory and secondary memory
Page fault
Process and threads
Process and threads
Sockets and threads
Process and threads in operating system
Problem solving