PROGRAMMING PRE AND POSTCONDITIONS INVARIANTS AND METHOD CONTRACTS
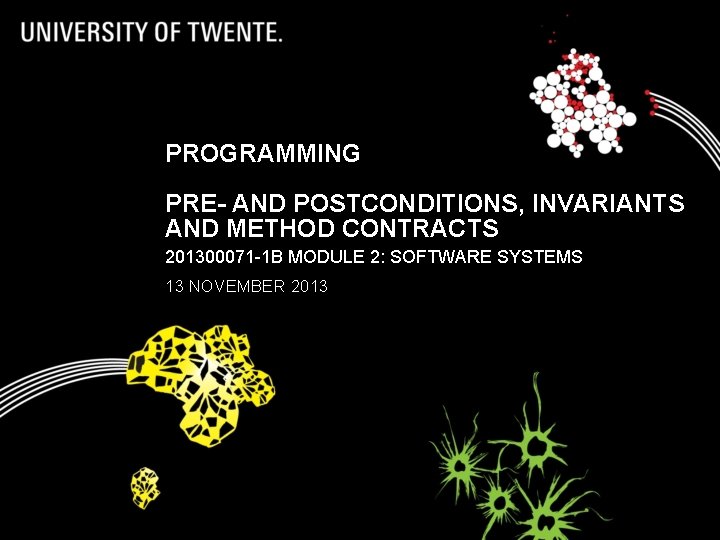
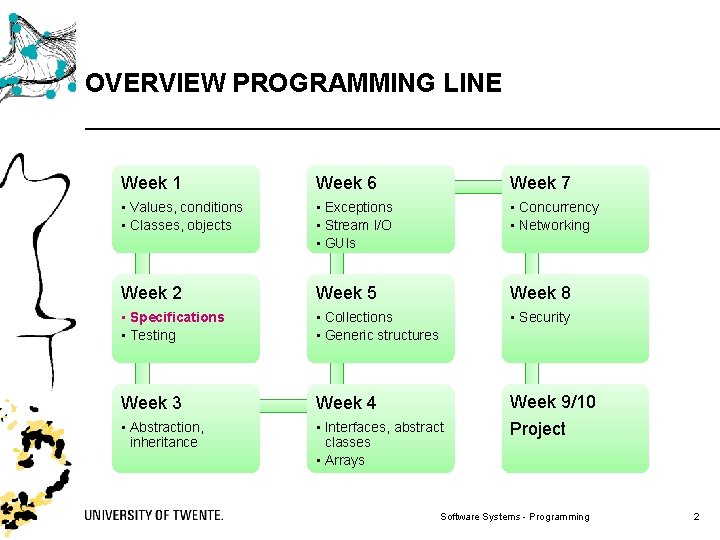
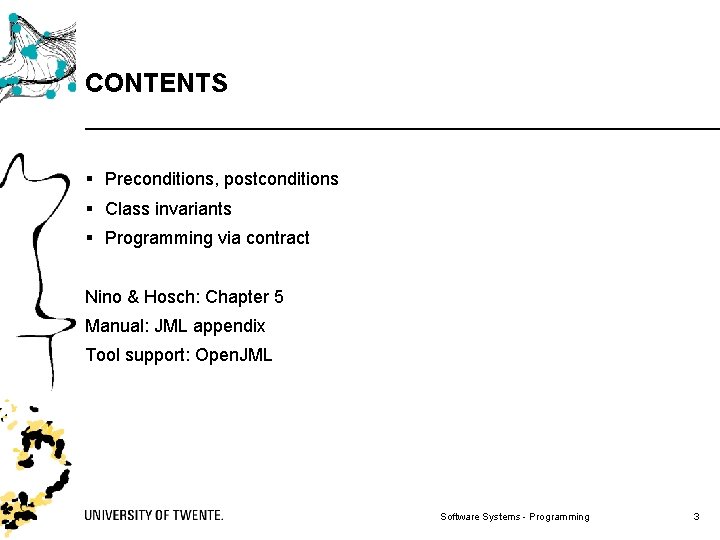
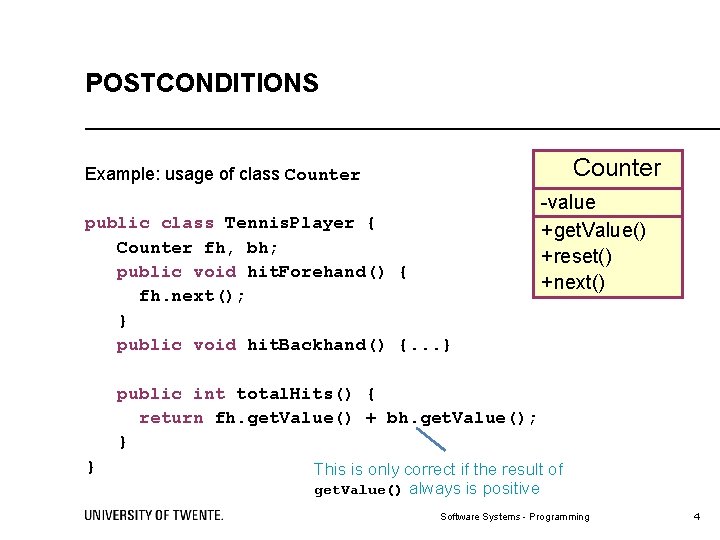
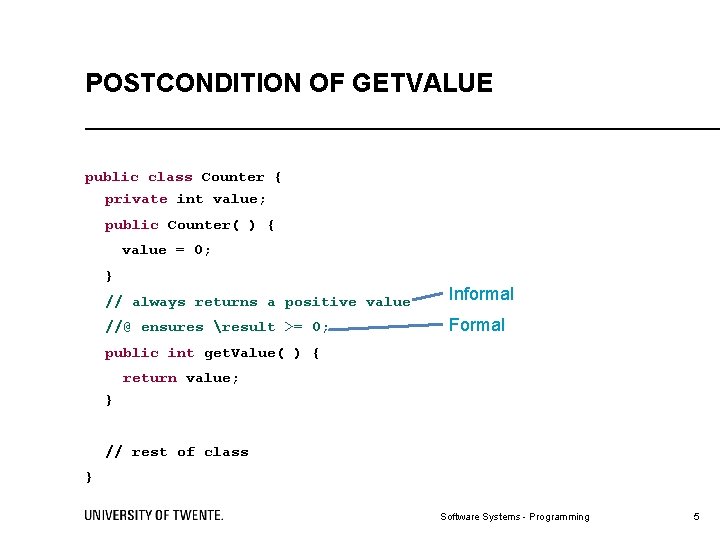
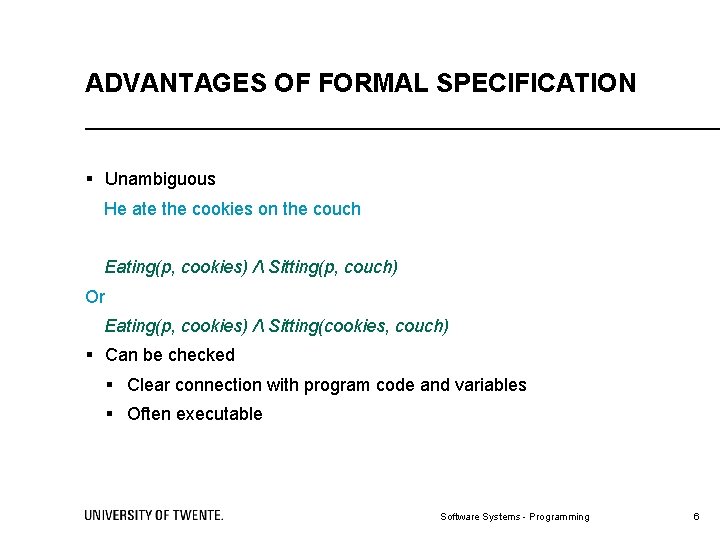
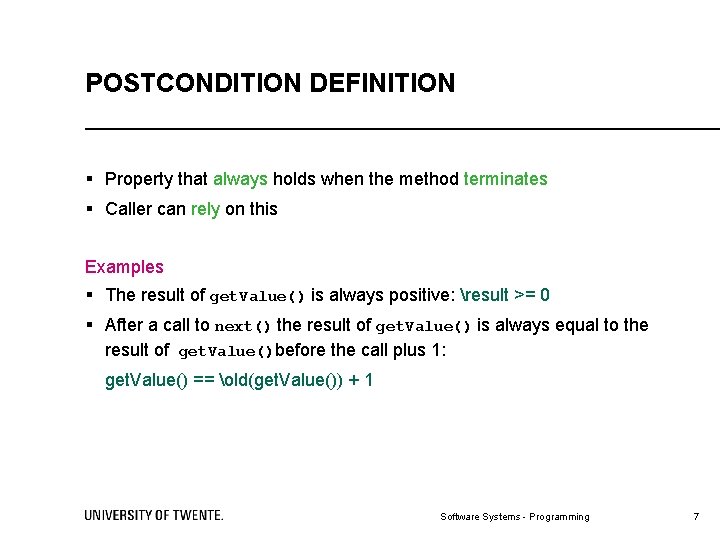
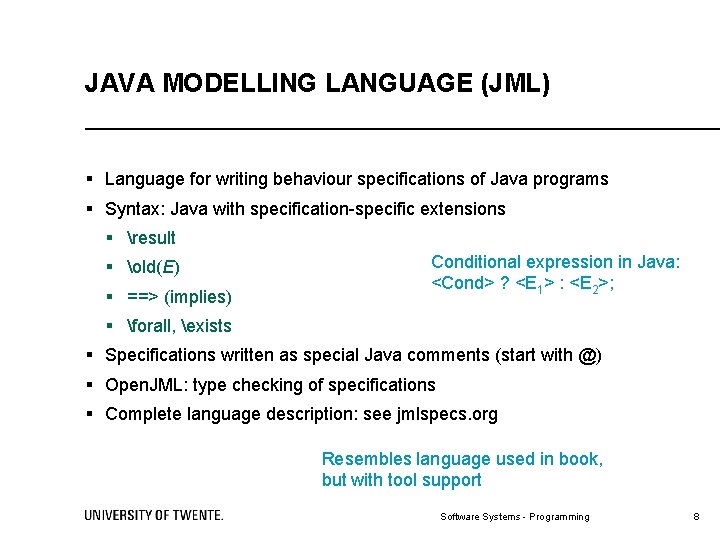
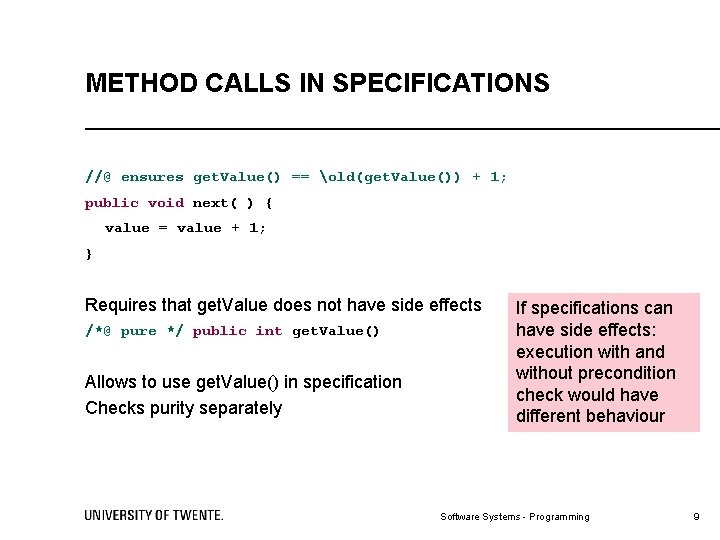
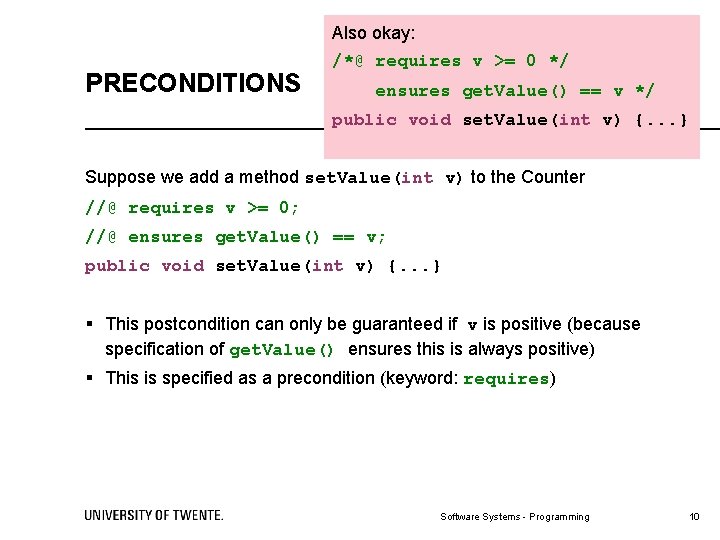
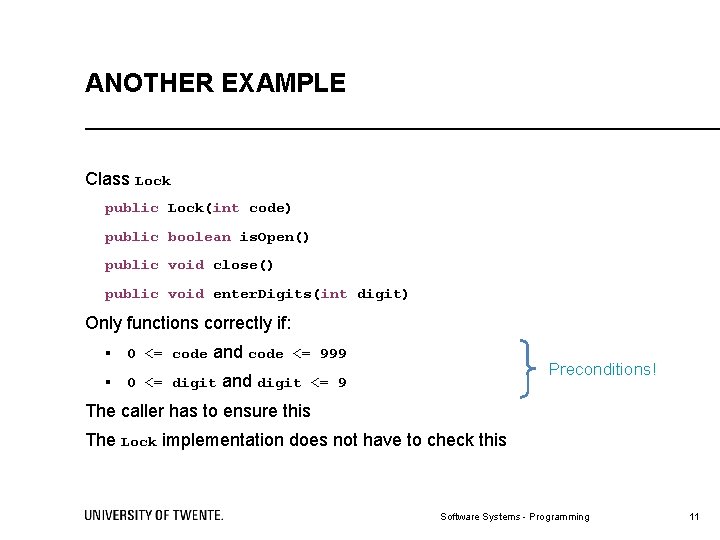
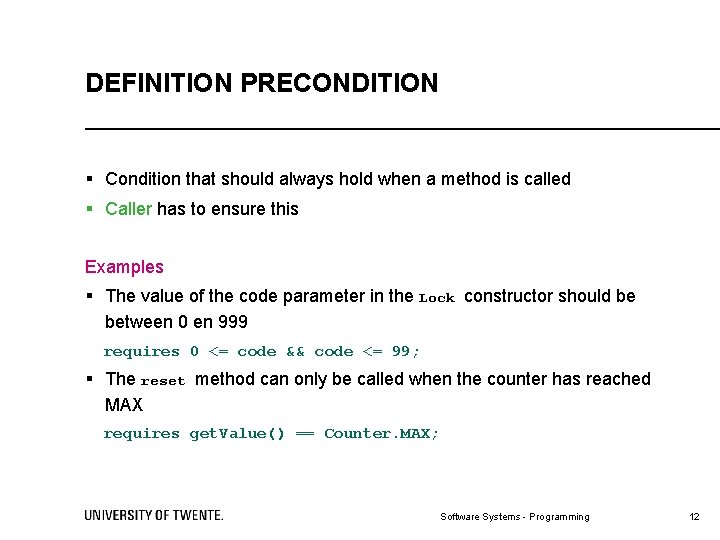
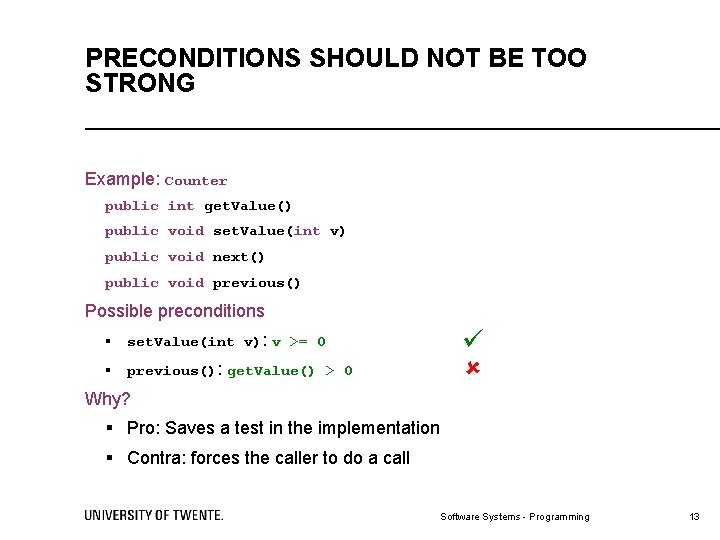
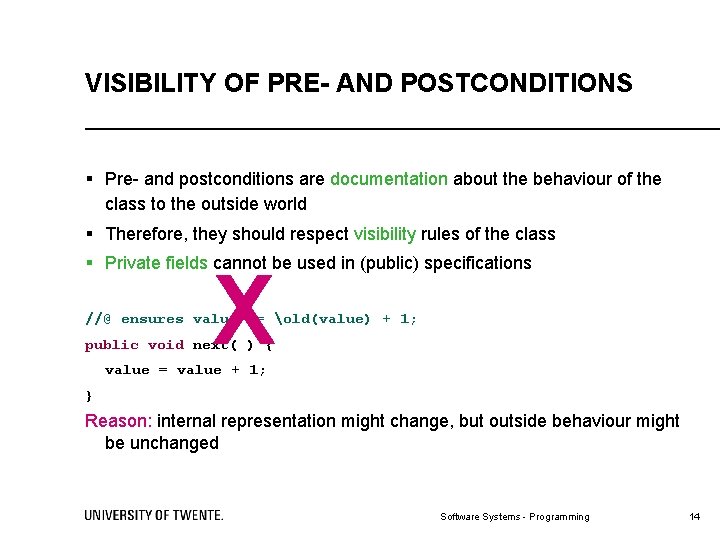
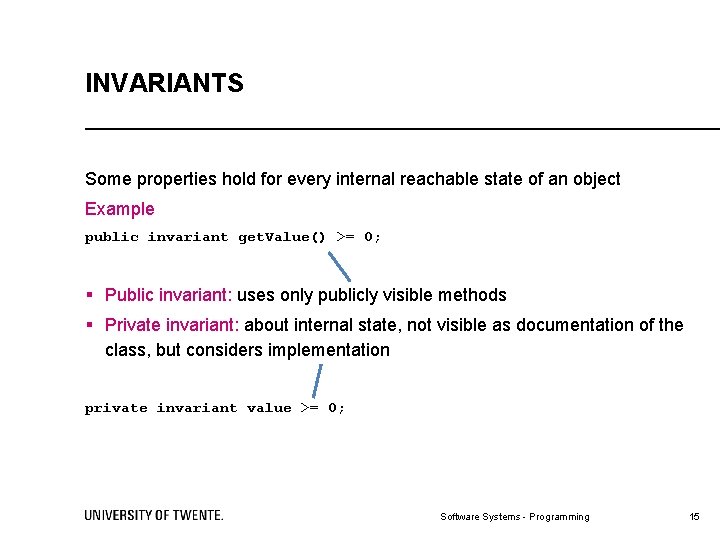
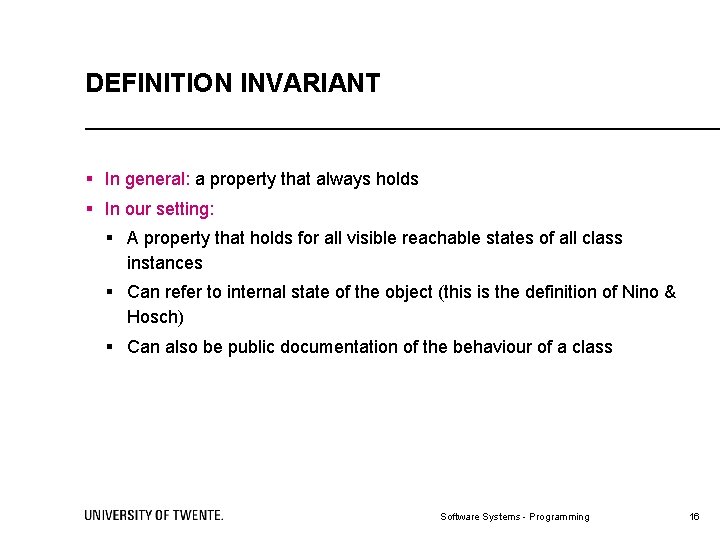
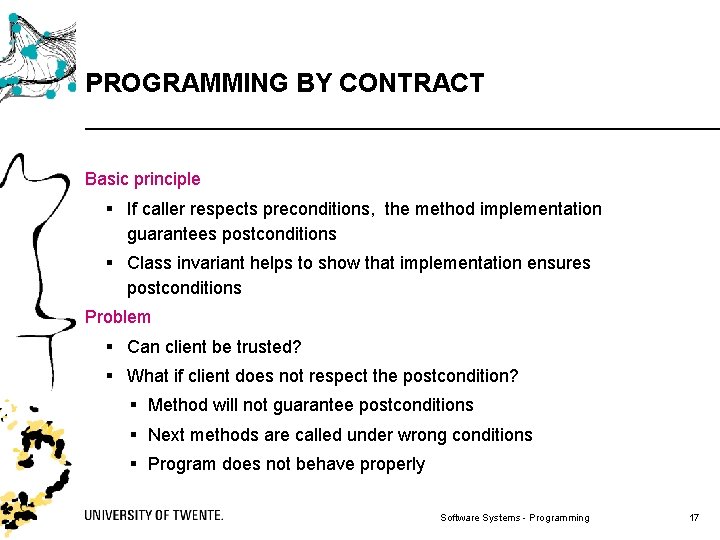
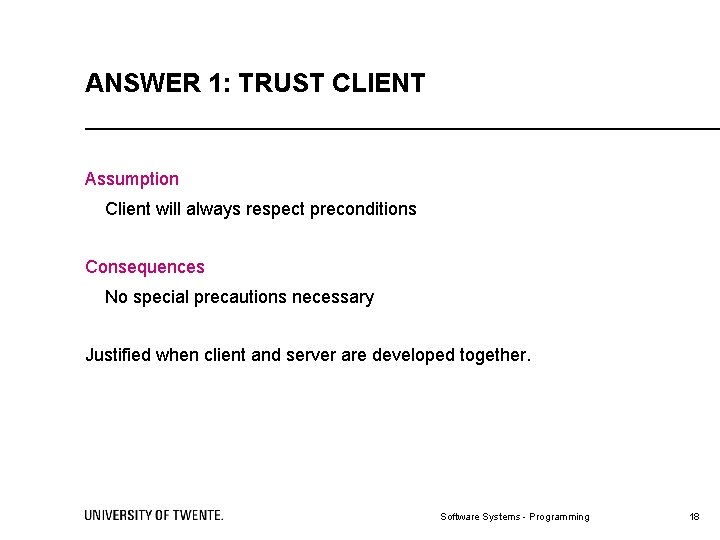
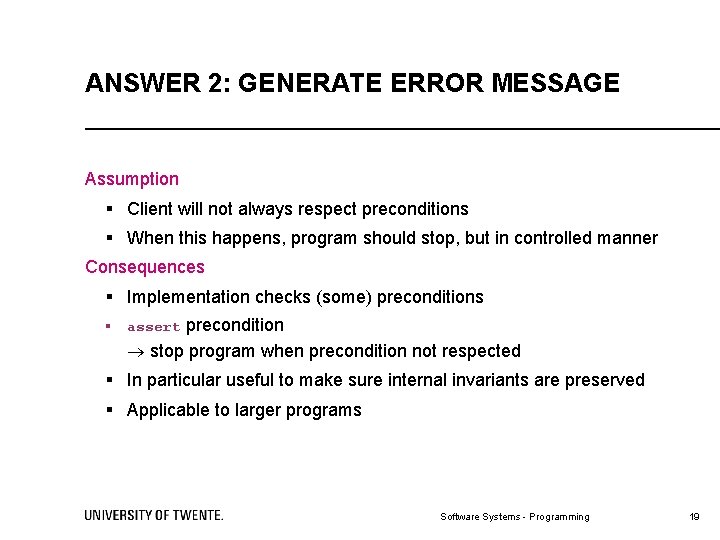
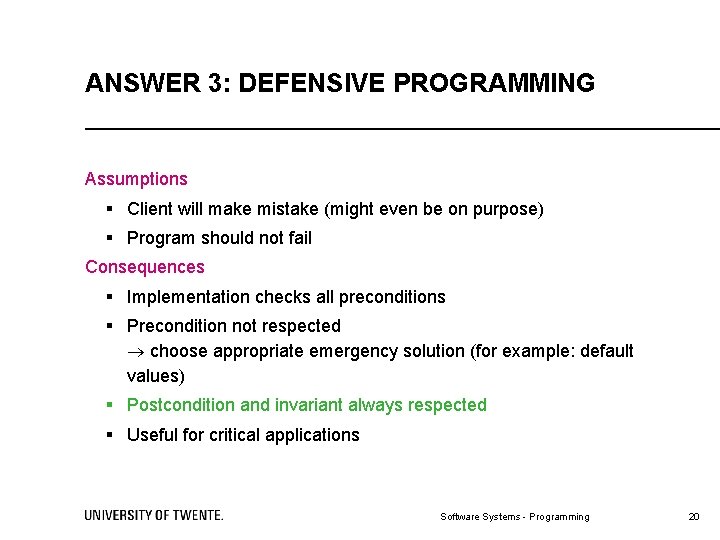
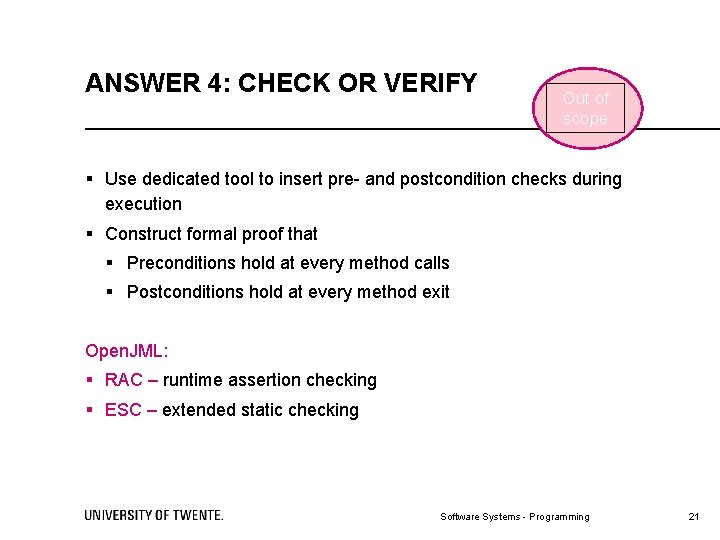
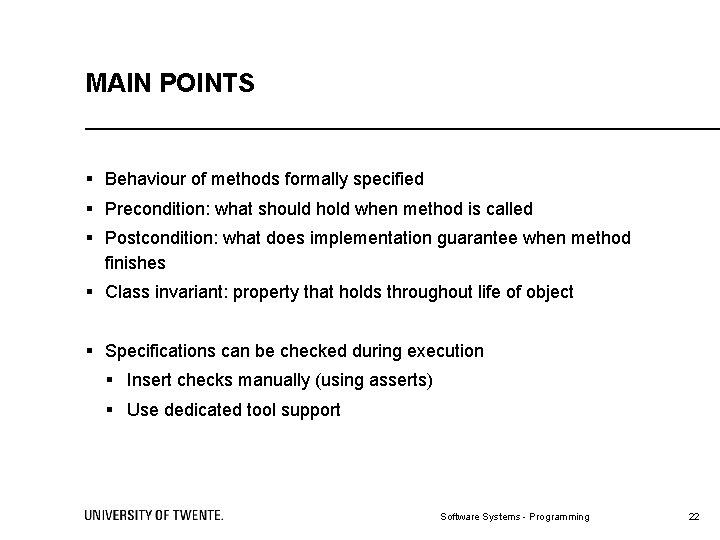
- Slides: 22
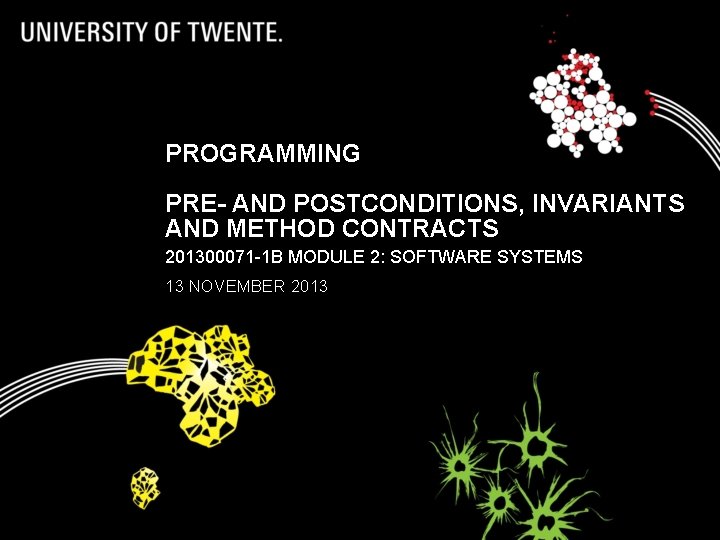
PROGRAMMING PRE- AND POSTCONDITIONS, INVARIANTS AND METHOD CONTRACTS 201300071 -1 B MODULE 2: SOFTWARE SYSTEMS 13 NOVEMBER 2013
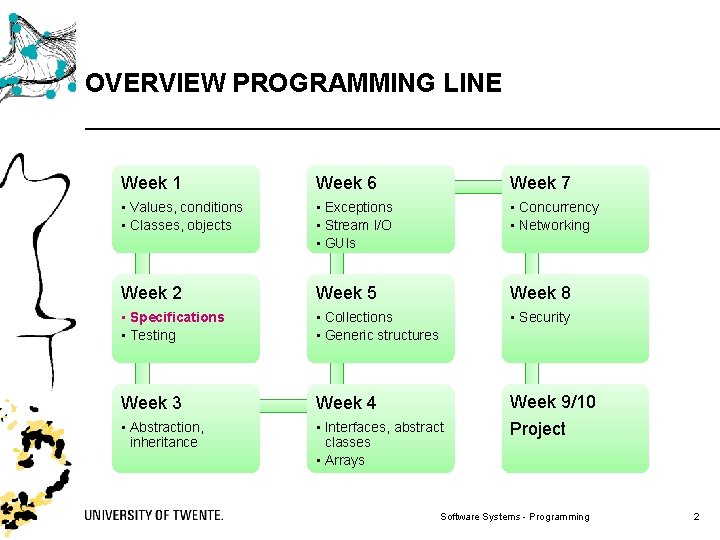
OVERVIEW PROGRAMMING LINE Week 1 Week 6 Week 7 • Values, conditions • Classes, objects • Exceptions • Stream I/O • GUIs • Concurrency • Networking Week 2 Week 5 Week 8 • Specifications • Testing • Collections • Generic structures • Security Week 3 Week 4 Week 9/10 • Abstraction, inheritance • Interfaces, abstract classes • Arrays Project Software Systems - Programming 2
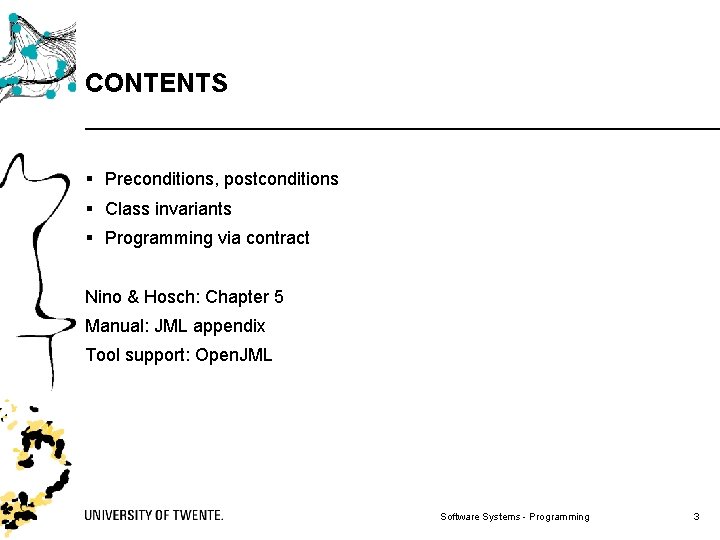
CONTENTS § Preconditions, postconditions § Class invariants § Programming via contract Nino & Hosch: Chapter 5 Manual: JML appendix Tool support: Open. JML Software Systems - Programming 3
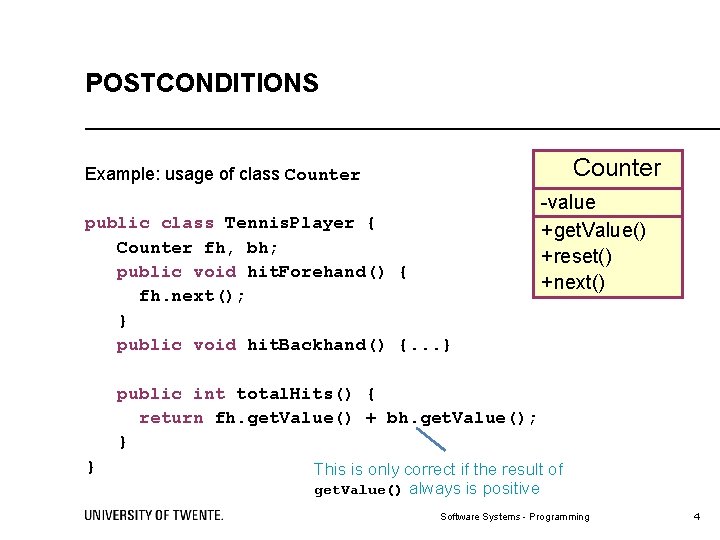
POSTCONDITIONS Counter Example: usage of class Counter public class Tennis. Player { Counter fh, bh; public void hit. Forehand() { fh. next(); } public void hit. Backhand() {. . . } -value +get. Value() +reset() +next() public int total. Hits() { return fh. get. Value() + bh. get. Value(); } } This is only correct if the result of get. Value() always is positive Software Systems - Programming 4
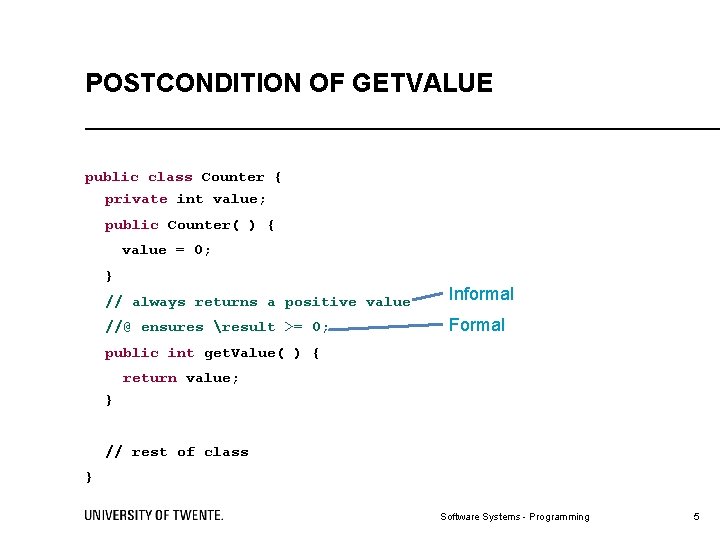
POSTCONDITION OF GETVALUE public class Counter { private int value; public Counter( ) { value = 0; } // always returns a positive value Informal //@ ensures result >= 0; Formal public int get. Value( ) { return value; } // rest of class } Software Systems - Programming 5
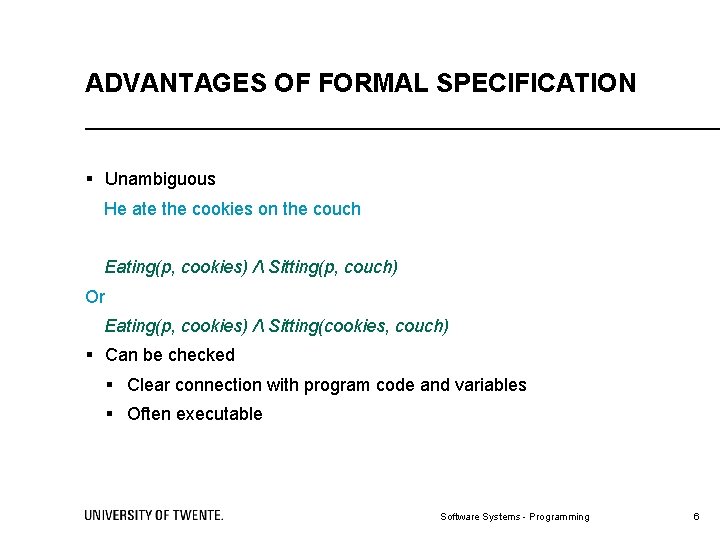
ADVANTAGES OF FORMAL SPECIFICATION § Unambiguous He ate the cookies on the couch Eating(p, cookies) / Sitting(p, couch) Or Eating(p, cookies) / Sitting(cookies, couch) § Can be checked § Clear connection with program code and variables § Often executable Software Systems - Programming 6
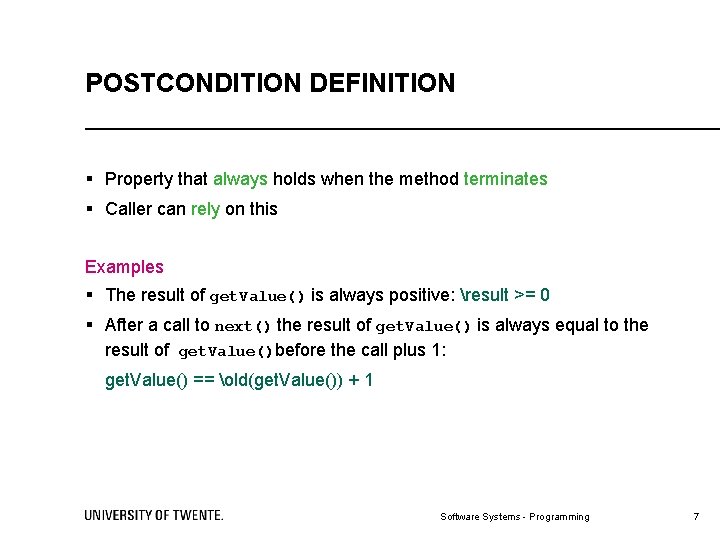
POSTCONDITION DEFINITION § Property that always holds when the method terminates § Caller can rely on this Examples § The result of get. Value() is always positive: result >= 0 § After a call to next() the result of get. Value() is always equal to the result of get. Value()before the call plus 1: get. Value() == old(get. Value()) + 1 Software Systems - Programming 7
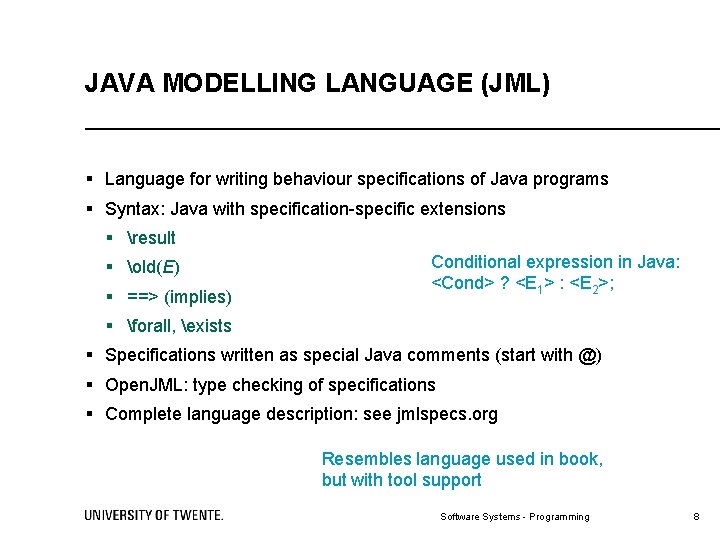
JAVA MODELLING LANGUAGE (JML) § Language for writing behaviour specifications of Java programs § Syntax: Java with specification-specific extensions § result § old(E) § ==> (implies) Conditional expression in Java: <Cond> ? <E 1> : <E 2>; § forall, exists § Specifications written as special Java comments (start with @) § Open. JML: type checking of specifications § Complete language description: see jmlspecs. org Resembles language used in book, but with tool support Software Systems - Programming 8
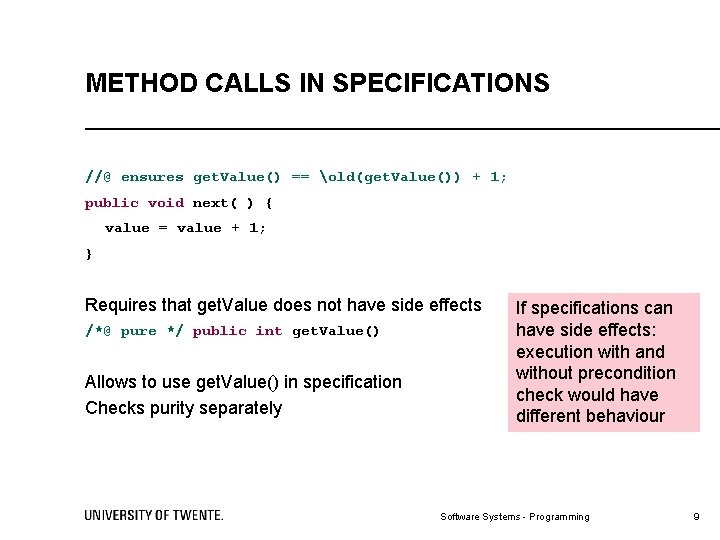
METHOD CALLS IN SPECIFICATIONS //@ ensures get. Value() == old(get. Value()) + 1; public void next( ) { value = value + 1; } Requires that get. Value does not have side effects /*@ pure */ public int get. Value() Allows to use get. Value() in specification Checks purity separately If specifications can have side effects: execution with and without precondition check would have different behaviour Software Systems - Programming 9
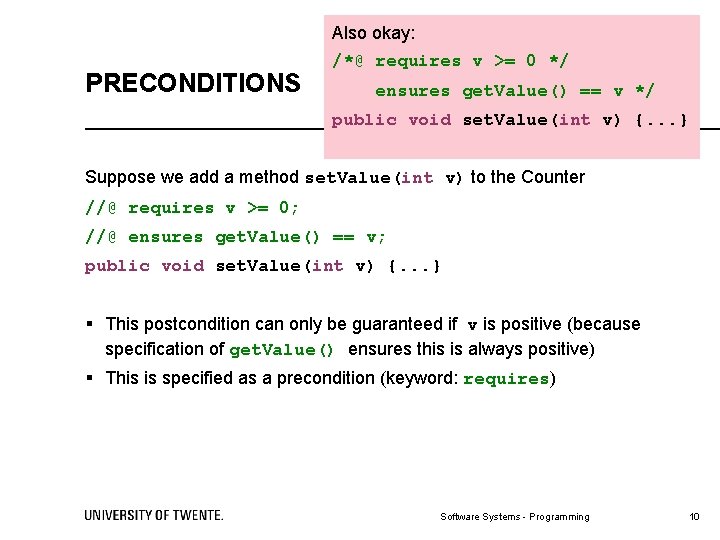
Also okay: PRECONDITIONS /*@ requires v >= 0 */ ensures get. Value() == v */ public void set. Value(int v) {. . . } Suppose we add a method set. Value(int v) to the Counter //@ requires v >= 0; //@ ensures get. Value() == v; public void set. Value(int v) {. . . } § This postcondition can only be guaranteed if v is positive (because specification of get. Value() ensures this is always positive) § This is specified as a precondition (keyword: requires) Software Systems - Programming 10
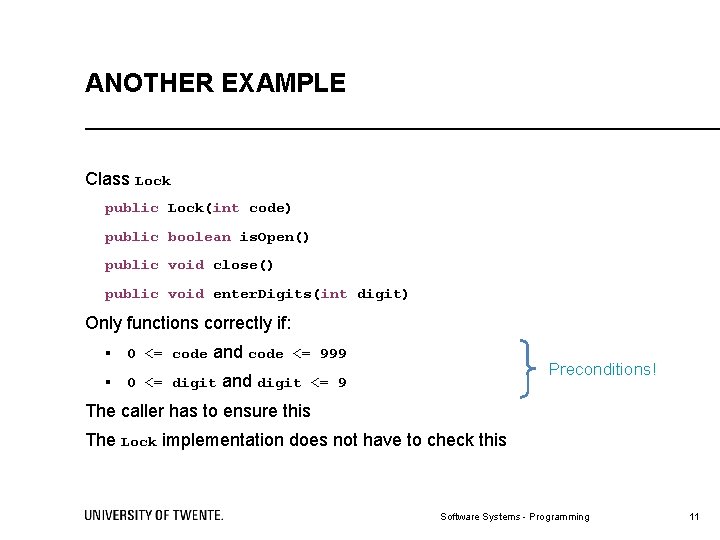
ANOTHER EXAMPLE Class Lock public Lock(int code) public boolean is. Open() public void close() public void enter. Digits(int digit) Only functions correctly if: § 0 <= code and code <= 999 Preconditions! § 0 <= digit and digit <= 9 The caller has to ensure this The Lock implementation does not have to check this Software Systems - Programming 11
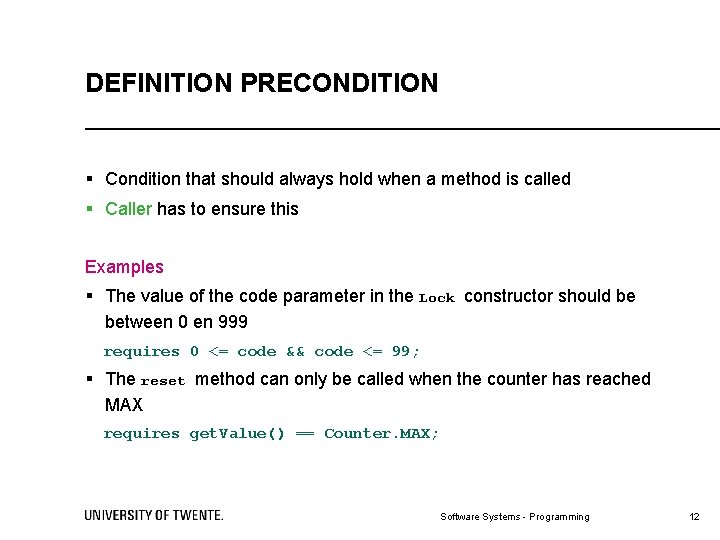
DEFINITION PRECONDITION § Condition that should always hold when a method is called § Caller has to ensure this Examples § The value of the code parameter in the Lock constructor should be between 0 en 999 requires 0 <= code && code <= 99; § The reset method can only be called when the counter has reached MAX requires get. Value() == Counter. MAX; Software Systems - Programming 12
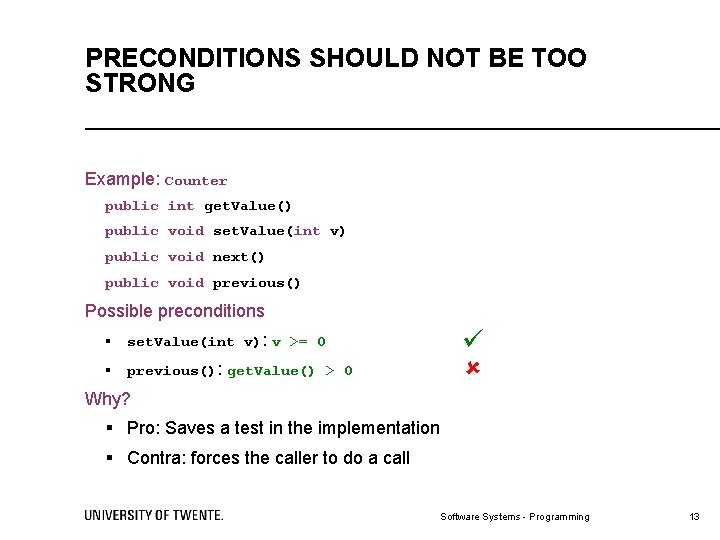
PRECONDITIONS SHOULD NOT BE TOO STRONG Example: Counter public int get. Value() public void set. Value(int v) public void next() public void previous() Possible preconditions § set. Value(int v): v >= 0 § previous(): get. Value() > 0 Why? § Pro: Saves a test in the implementation § Contra: forces the caller to do a call Software Systems - Programming 13
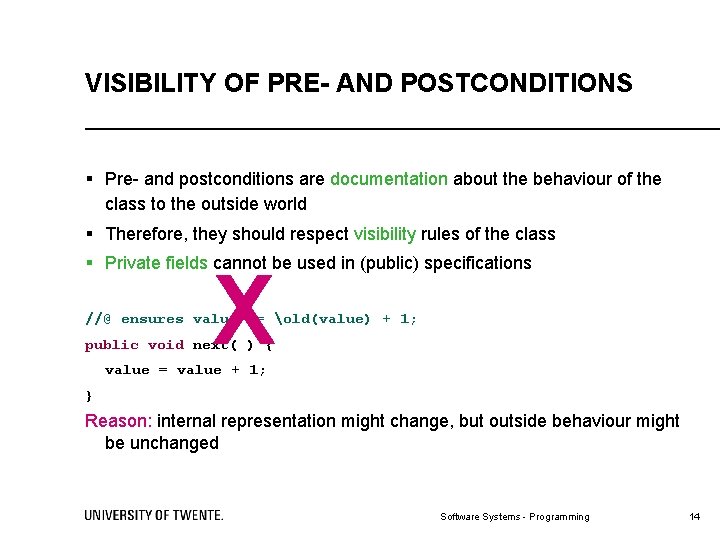
VISIBILITY OF PRE- AND POSTCONDITIONS § Pre- and postconditions are documentation about the behaviour of the class to the outside world § Therefore, they should respect visibility rules of the class § Private fields cannot be used in (public) specifications X //@ ensures value == old(value) + 1; public void next( ) { value = value + 1; } Reason: internal representation might change, but outside behaviour might be unchanged Software Systems - Programming 14
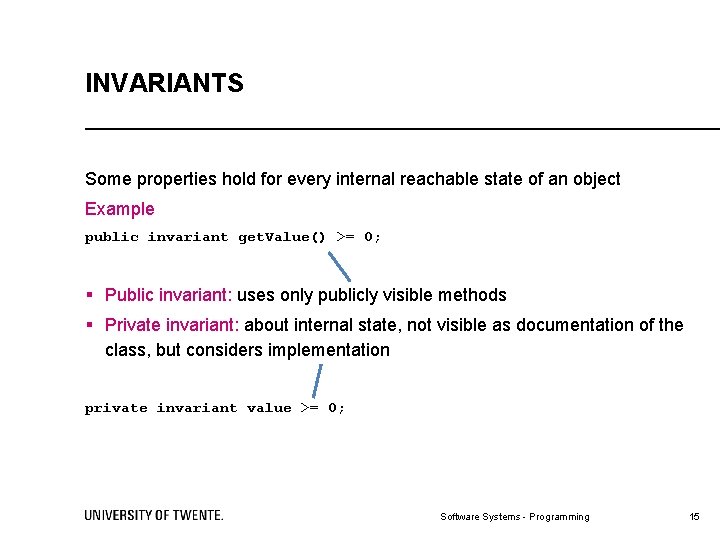
INVARIANTS Some properties hold for every internal reachable state of an object Example public invariant get. Value() >= 0; § Public invariant: uses only publicly visible methods § Private invariant: about internal state, not visible as documentation of the class, but considers implementation private invariant value >= 0; Software Systems - Programming 15
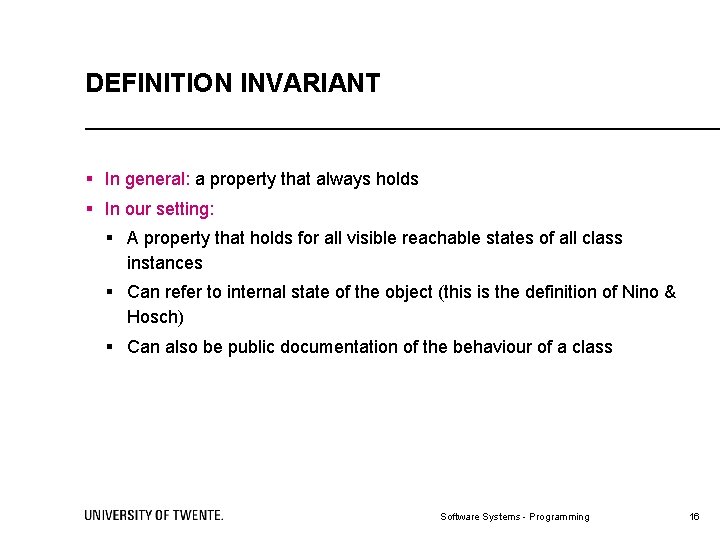
DEFINITION INVARIANT § In general: a property that always holds § In our setting: § A property that holds for all visible reachable states of all class instances § Can refer to internal state of the object (this is the definition of Nino & Hosch) § Can also be public documentation of the behaviour of a class Software Systems - Programming 16
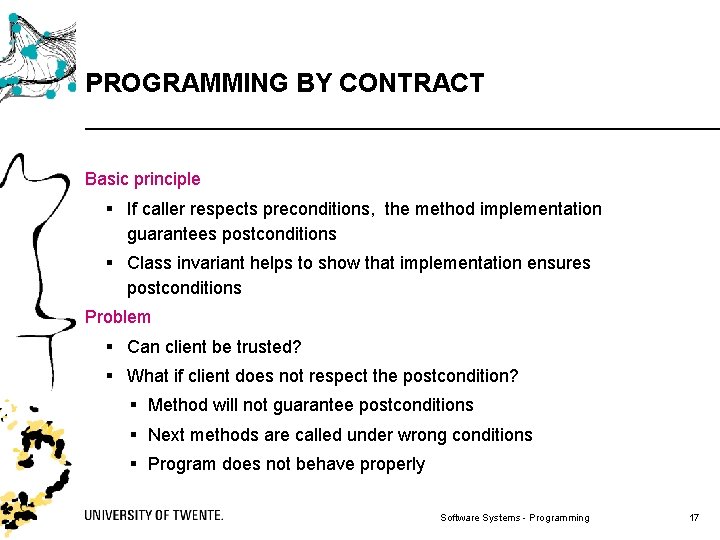
PROGRAMMING BY CONTRACT Basic principle § If caller respects preconditions, the method implementation guarantees postconditions § Class invariant helps to show that implementation ensures postconditions Problem § Can client be trusted? § What if client does not respect the postcondition? § Method will not guarantee postconditions § Next methods are called under wrong conditions § Program does not behave properly Software Systems - Programming 17
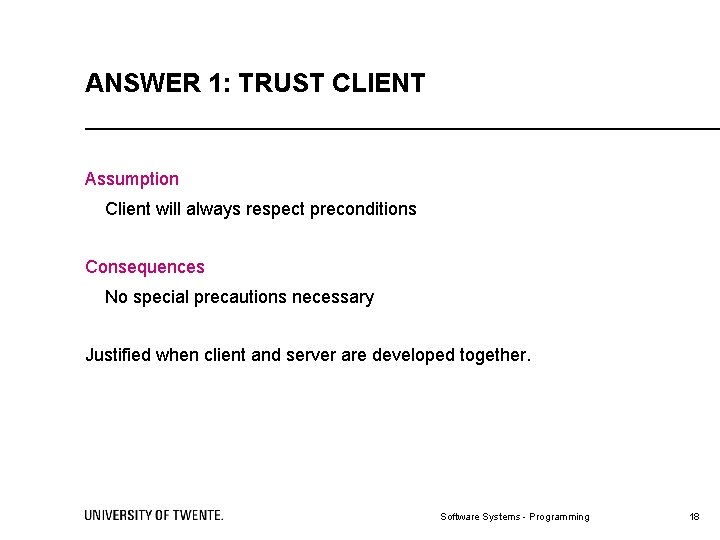
ANSWER 1: TRUST CLIENT Assumption Client will always respect preconditions Consequences No special precautions necessary Justified when client and server are developed together. Software Systems - Programming 18
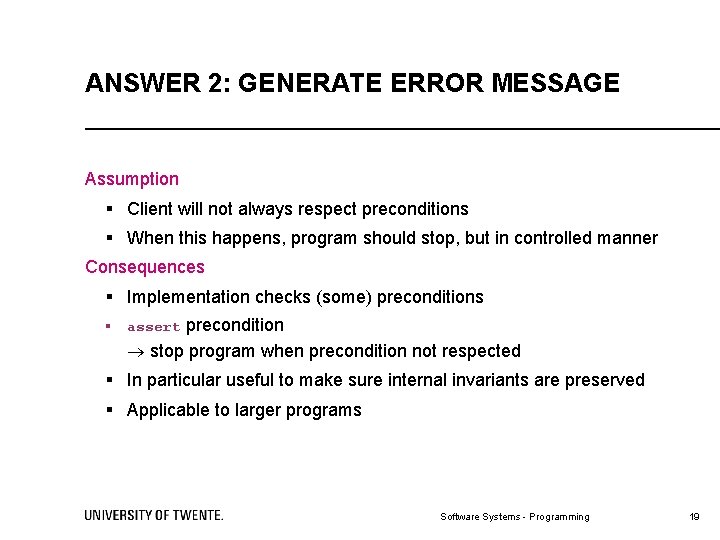
ANSWER 2: GENERATE ERROR MESSAGE Assumption § Client will not always respect preconditions § When this happens, program should stop, but in controlled manner Consequences § Implementation checks (some) preconditions § assert precondition stop program when precondition not respected § In particular useful to make sure internal invariants are preserved § Applicable to larger programs Software Systems - Programming 19
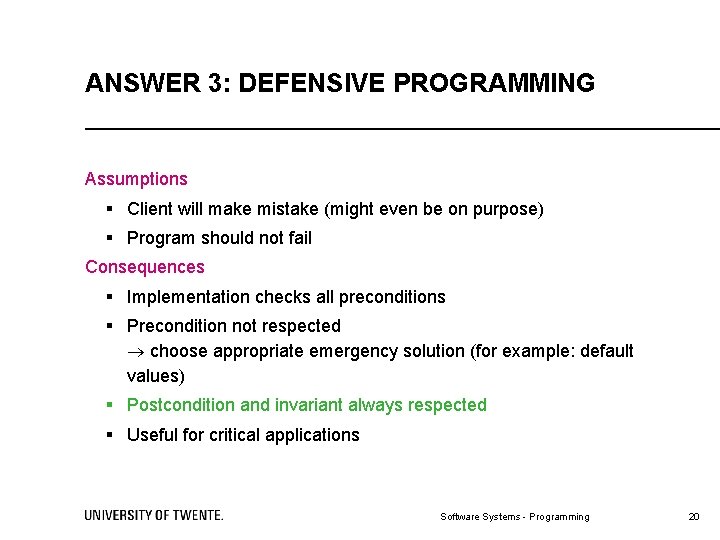
ANSWER 3: DEFENSIVE PROGRAMMING Assumptions § Client will make mistake (might even be on purpose) § Program should not fail Consequences § Implementation checks all preconditions § Precondition not respected choose appropriate emergency solution (for example: default values) § Postcondition and invariant always respected § Useful for critical applications Software Systems - Programming 20
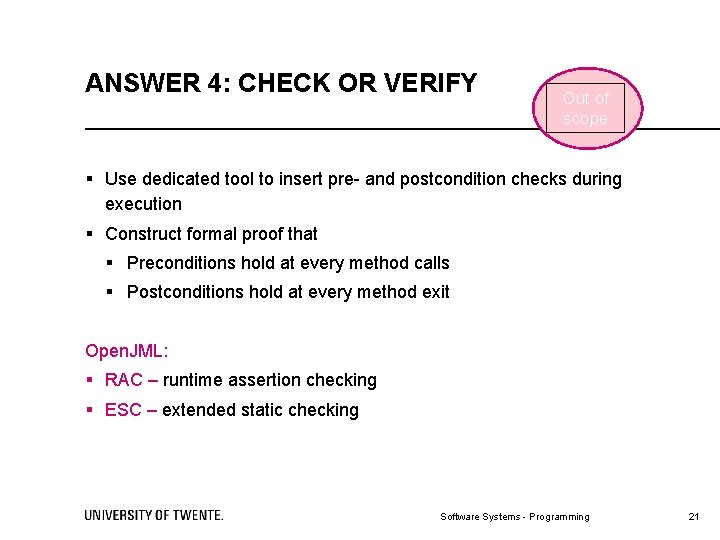
ANSWER 4: CHECK OR VERIFY Out of scope § Use dedicated tool to insert pre- and postcondition checks during execution § Construct formal proof that § Preconditions hold at every method calls § Postconditions hold at every method exit Open. JML: § RAC – runtime assertion checking § ESC – extended static checking Software Systems - Programming 21
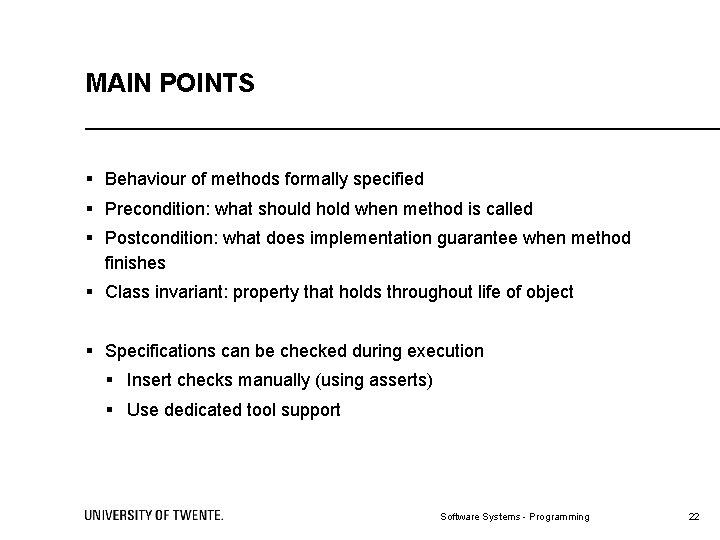
MAIN POINTS § Behaviour of methods formally specified § Precondition: what should hold when method is called § Postcondition: what does implementation guarantee when method finishes § Class invariant: property that holds throughout life of object § Specifications can be checked during execution § Insert checks manually (using asserts) § Use dedicated tool support Software Systems - Programming 22