Procedure Calls Linking Launching Applications Lecture 15 Digital
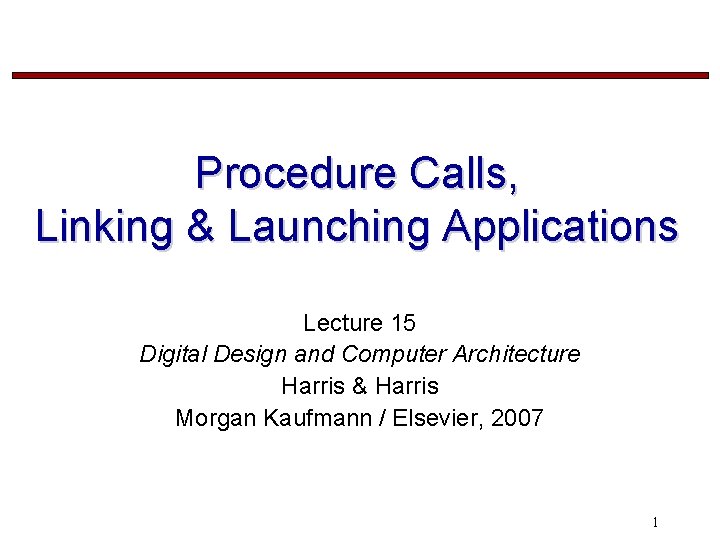
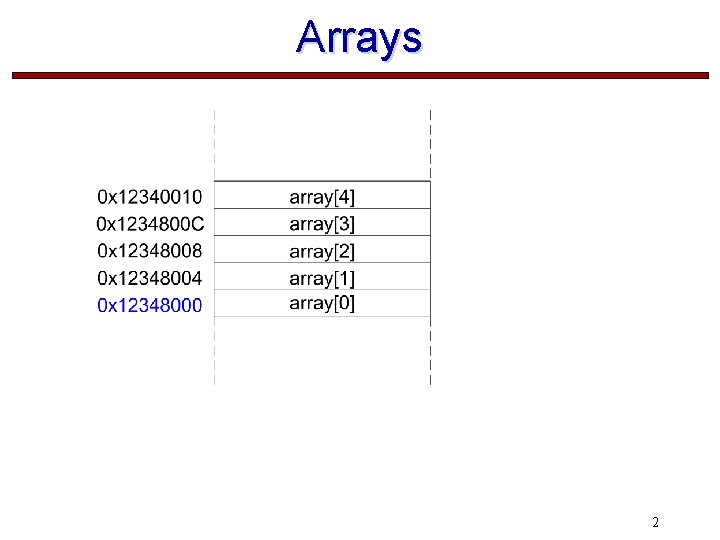
![Arrays // high-level code int array[5]; array[0] = array[0] * 2; array[1] = array[1] Arrays // high-level code int array[5]; array[0] = array[0] * 2; array[1] = array[1]](https://slidetodoc.com/presentation_image_h2/60dd85e81b7d4ee4de0d6af481c4b4c0/image-3.jpg)
![Arrays: Using for Loops // high-level code int array[1000]; int i; for (i=0; i Arrays: Using for Loops // high-level code int array[1000]; int i; for (i=0; i](https://slidetodoc.com/presentation_image_h2/60dd85e81b7d4ee4de0d6af481c4b4c0/image-4.jpg)
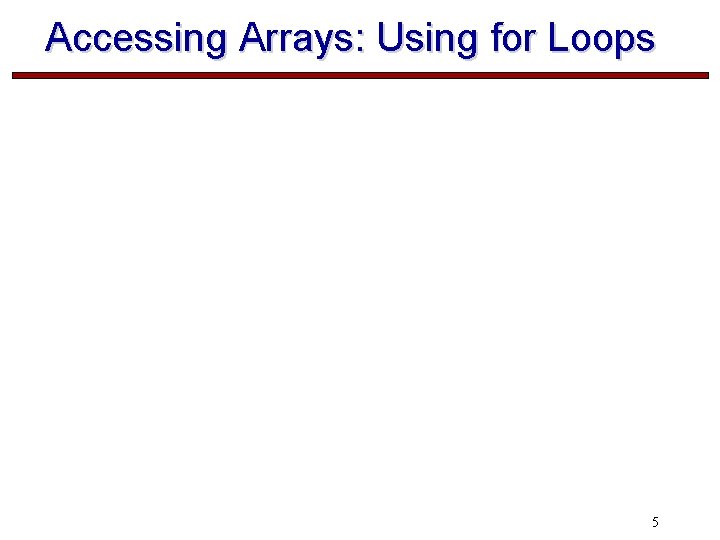
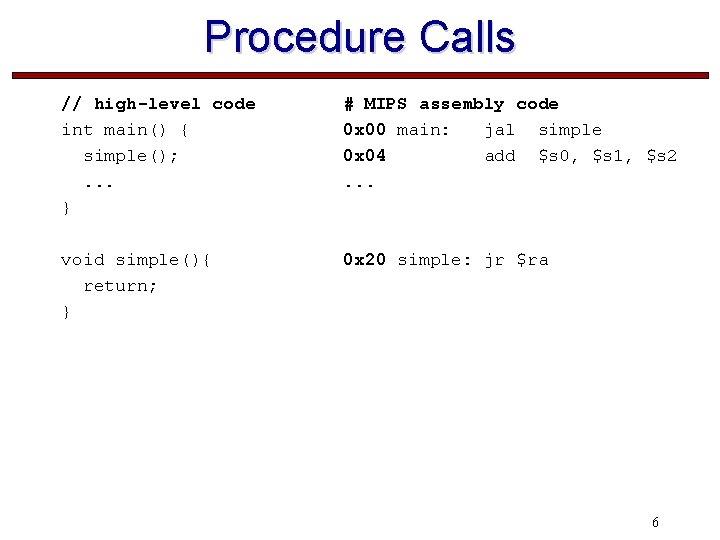
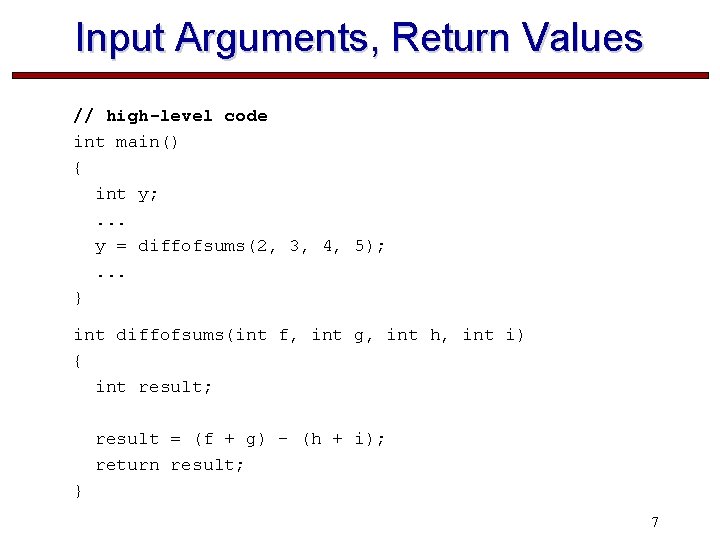
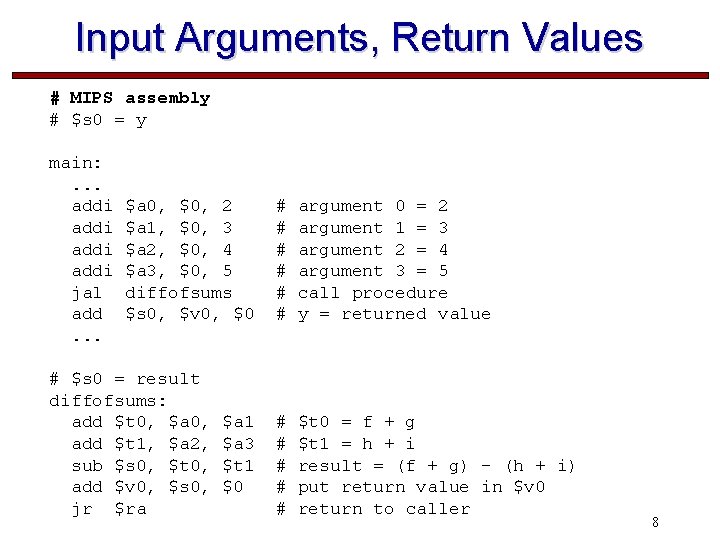
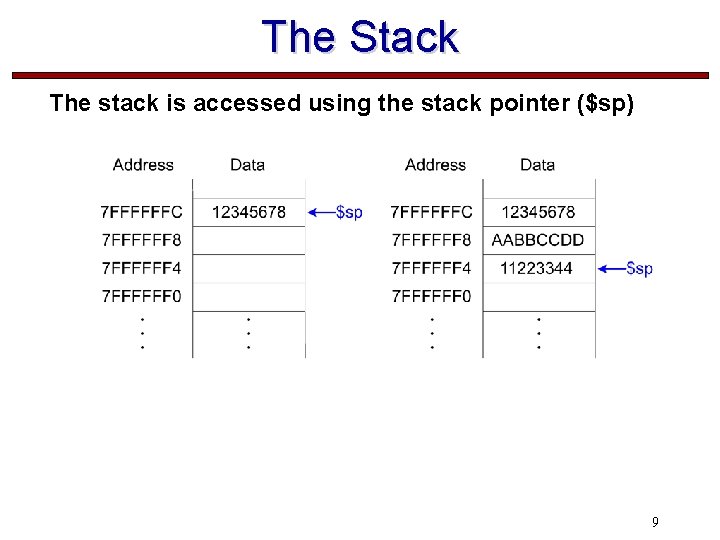
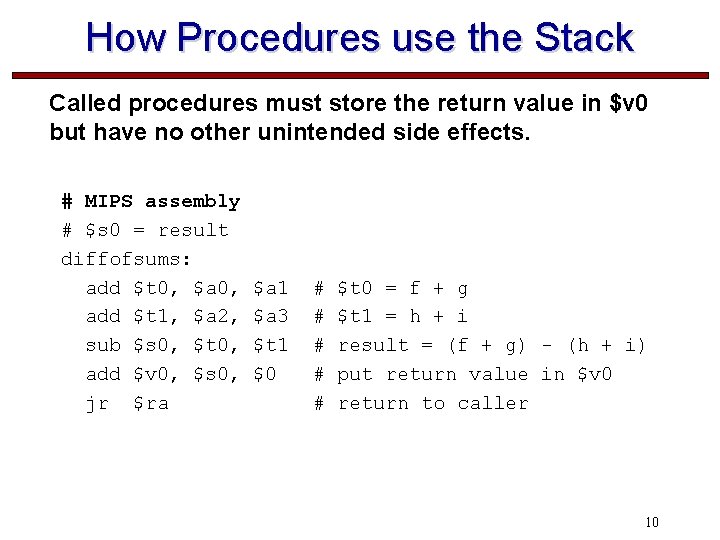
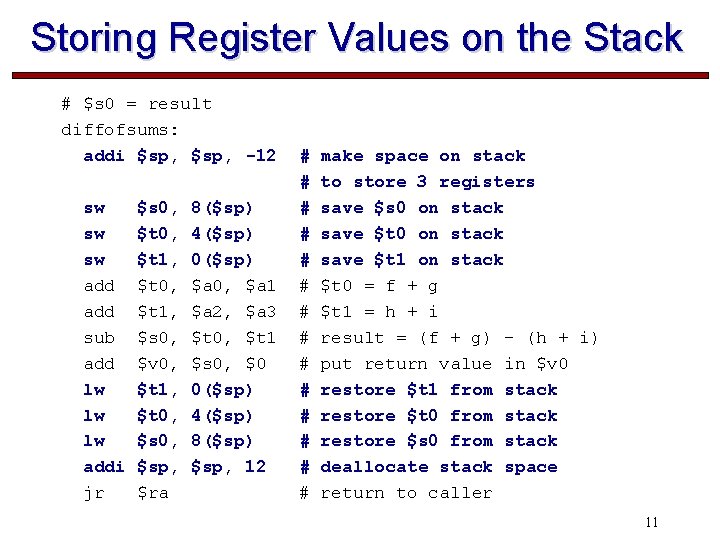
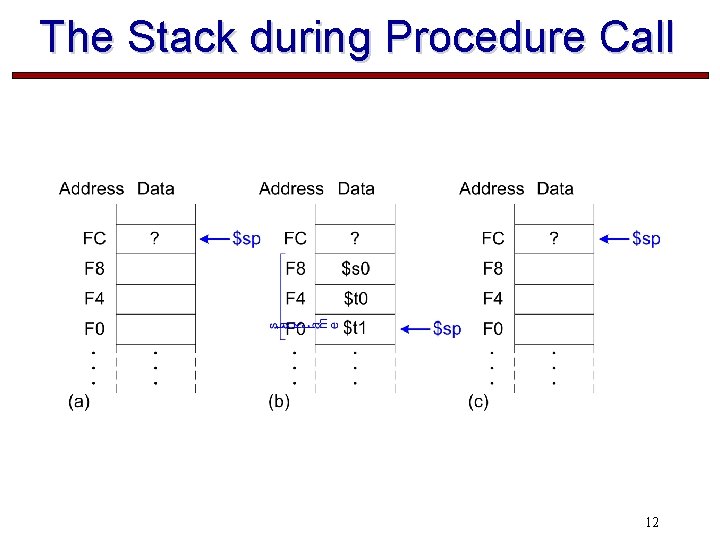
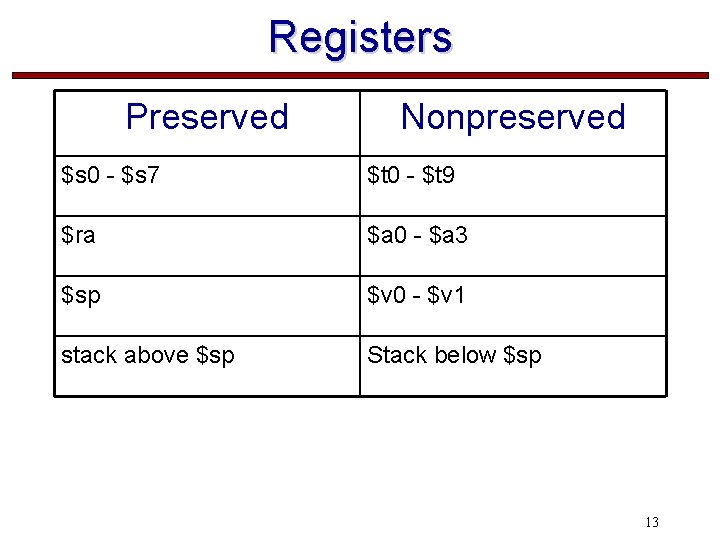
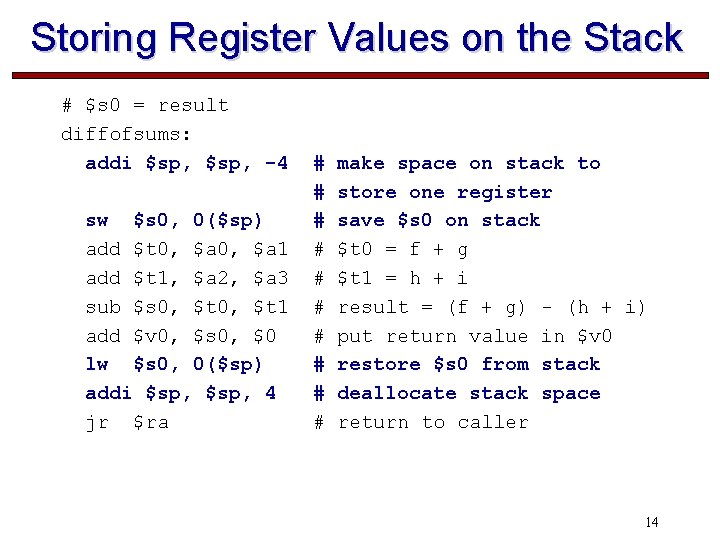
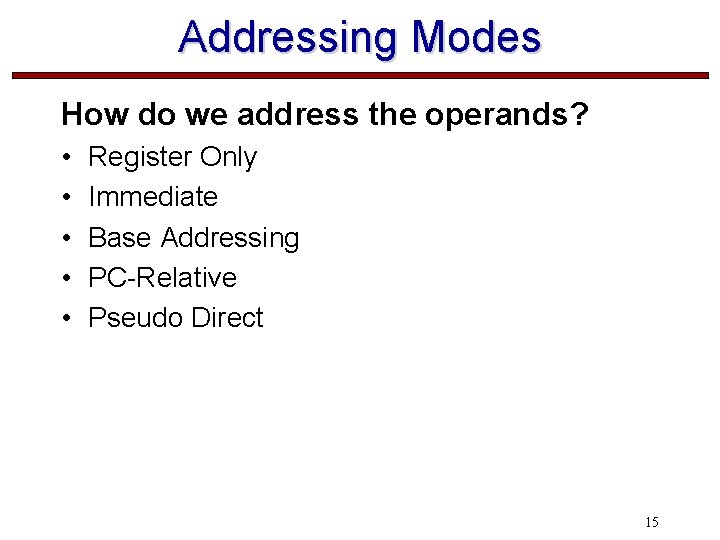
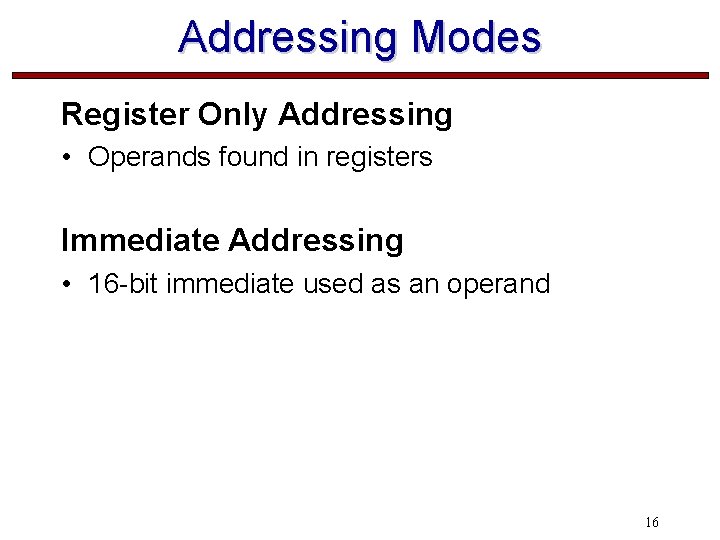
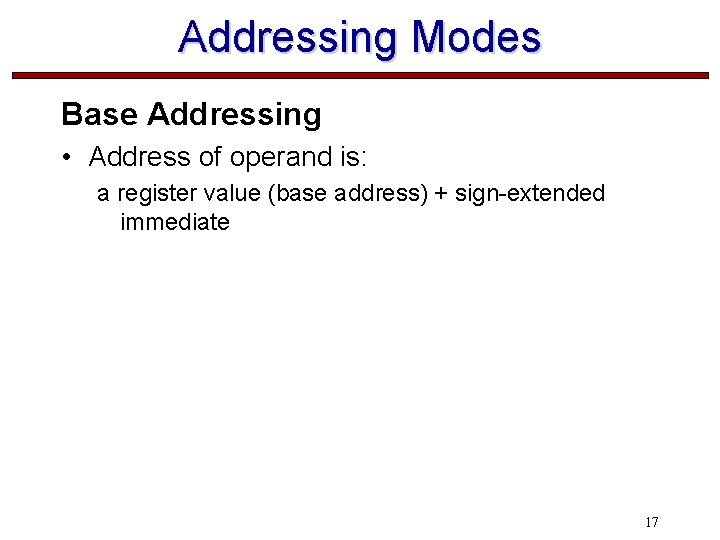
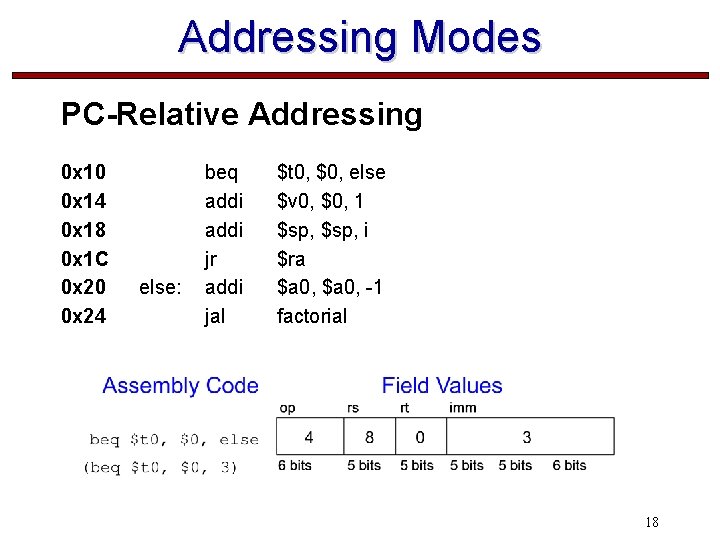
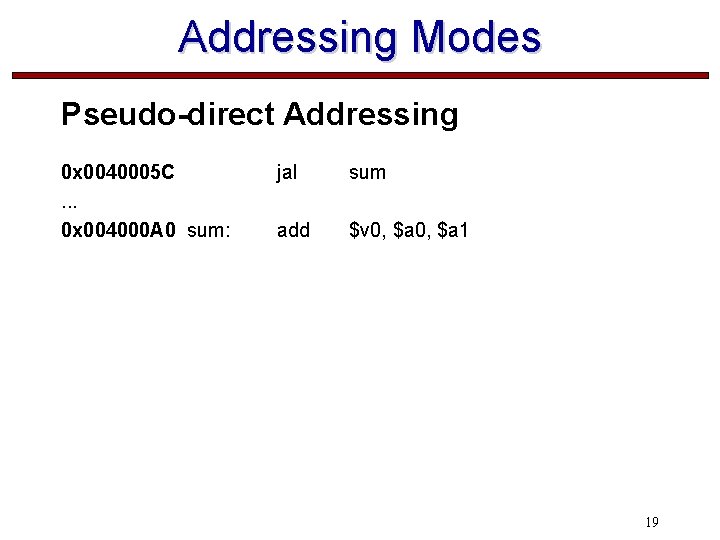
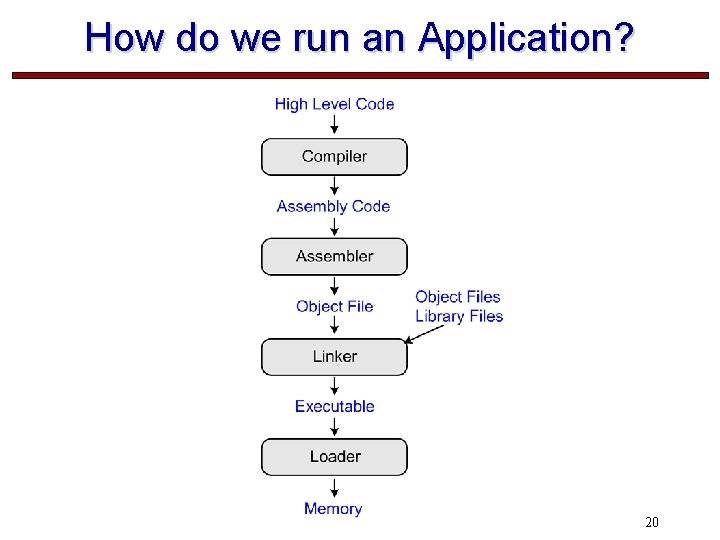
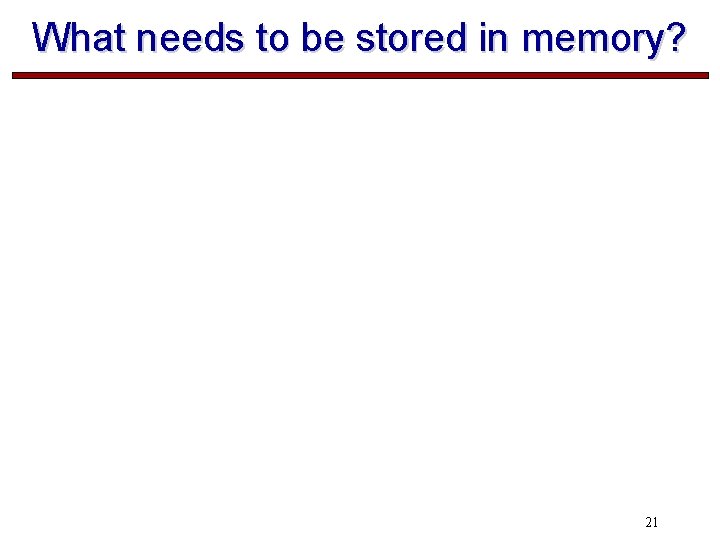
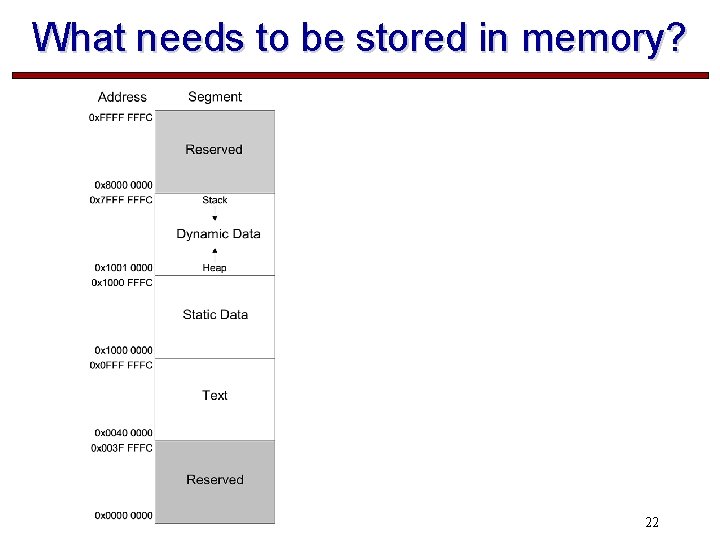
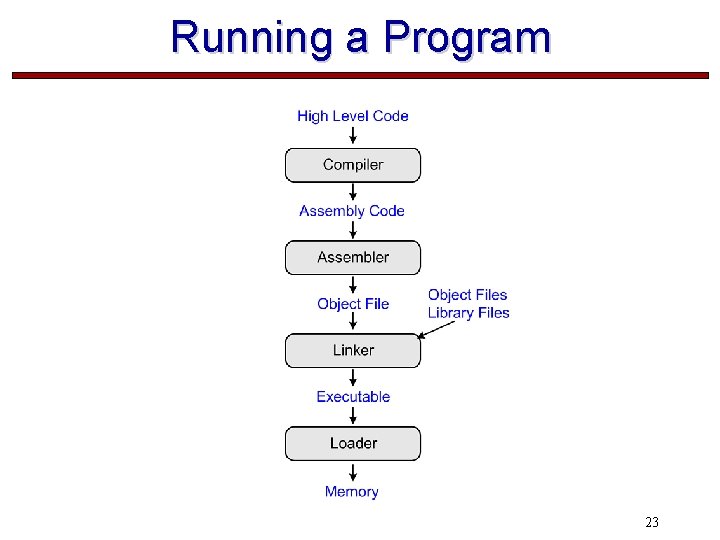
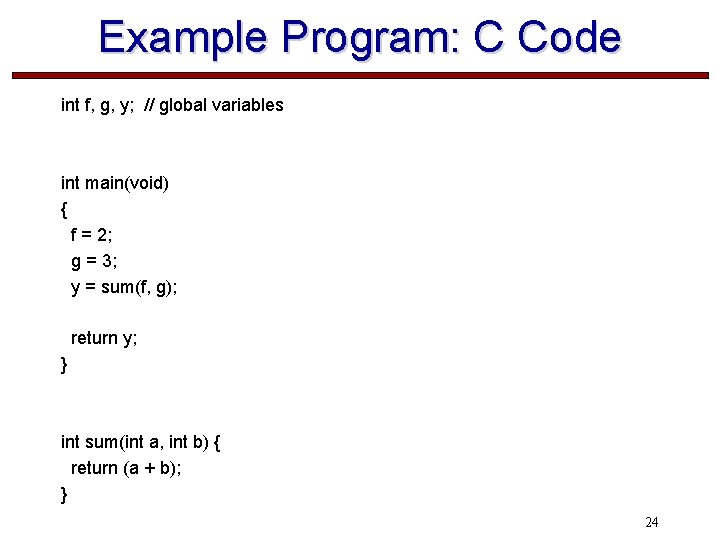
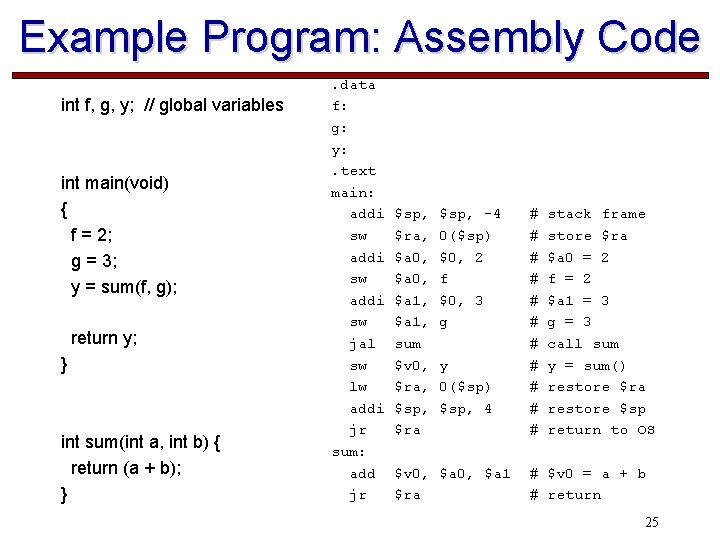
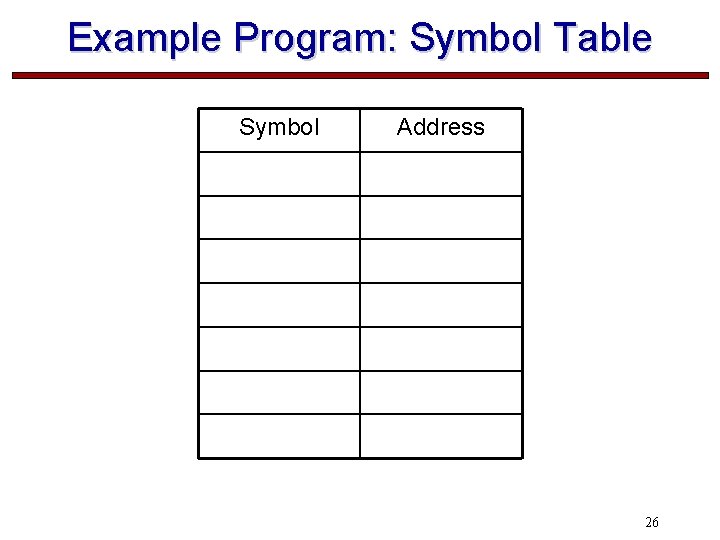
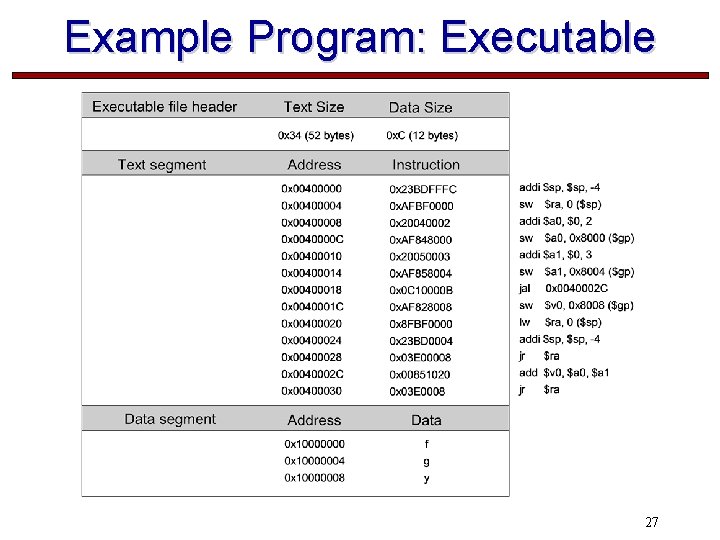
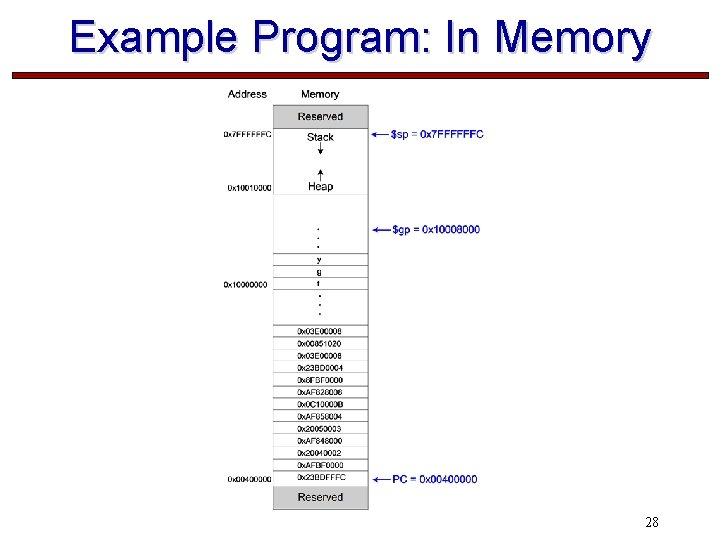
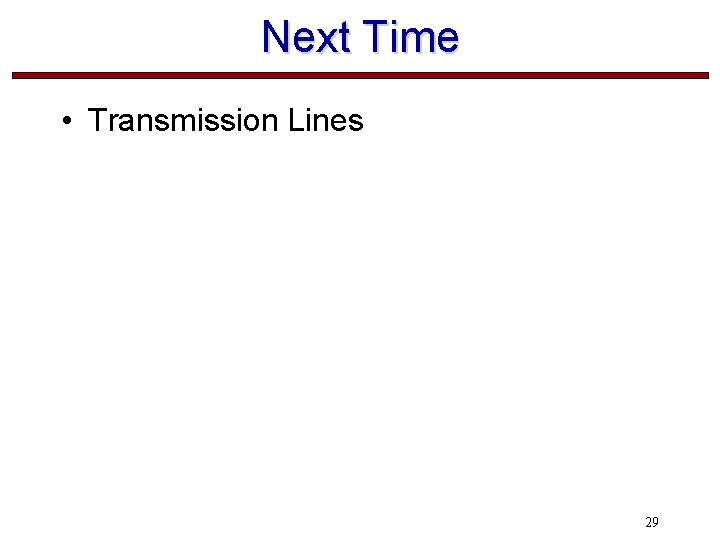
- Slides: 29
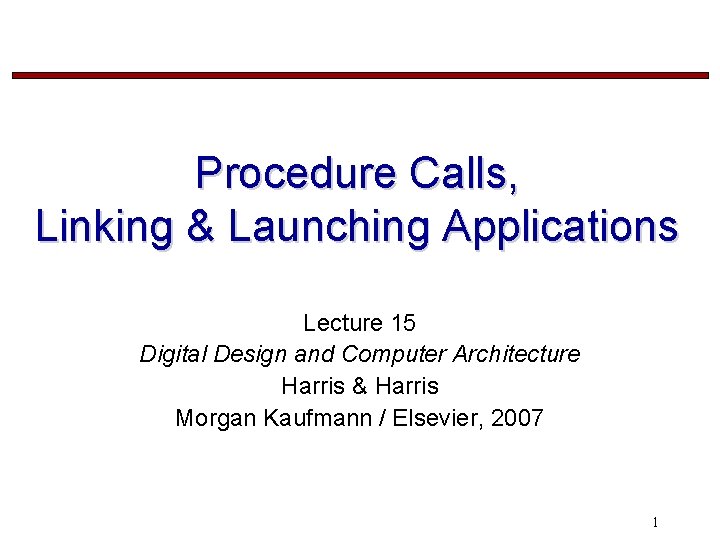
Procedure Calls, Linking & Launching Applications Lecture 15 Digital Design and Computer Architecture Harris & Harris Morgan Kaufmann / Elsevier, 2007 1
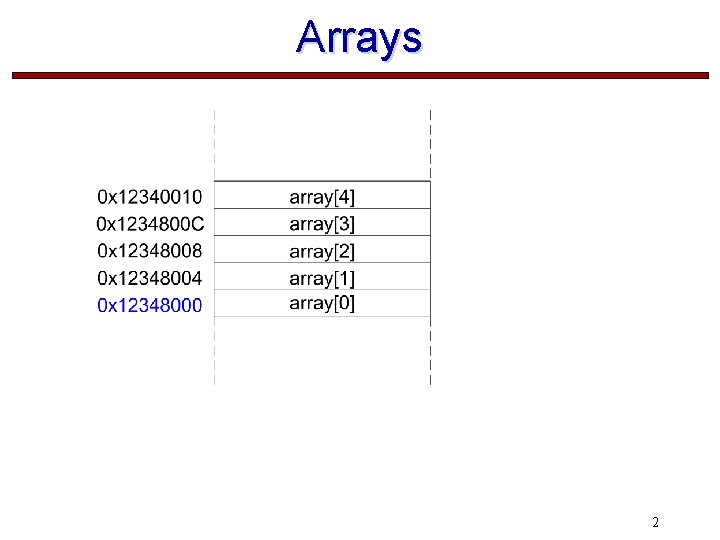
Arrays 2
![Arrays highlevel code int array5 array0 array0 2 array1 array1 Arrays // high-level code int array[5]; array[0] = array[0] * 2; array[1] = array[1]](https://slidetodoc.com/presentation_image_h2/60dd85e81b7d4ee4de0d6af481c4b4c0/image-3.jpg)
Arrays // high-level code int array[5]; array[0] = array[0] * 2; array[1] = array[1] * 2; # MIPS assembly code # array base address = $s 0 lui $s 0, 0 x 1234 # put 0 x 1234 in upper half of $S 0 ori $s 0, 0 x 8000 # put 0 x 8000 in lower half of $s 0 lw $t 1, 0($s 0) sll $t 1, 1 sw $t 1, 0($s 0) # $t 1 = array[0] # $t 1 = $t 1 * 2 # array[0] = $t 1 lw $t 1, 4($s 0) sll $t 1, 1 sw $t 1, 4($s 0) # $t 1 = array[1] # $t 1 = $t 1 * 2 # array[1] = $t 1 3
![Arrays Using for Loops highlevel code int array1000 int i for i0 i Arrays: Using for Loops // high-level code int array[1000]; int i; for (i=0; i](https://slidetodoc.com/presentation_image_h2/60dd85e81b7d4ee4de0d6af481c4b4c0/image-4.jpg)
Arrays: Using for Loops // high-level code int array[1000]; int i; for (i=0; i < 1000; i = i + 1) array[i] = array[i] * 2; 4
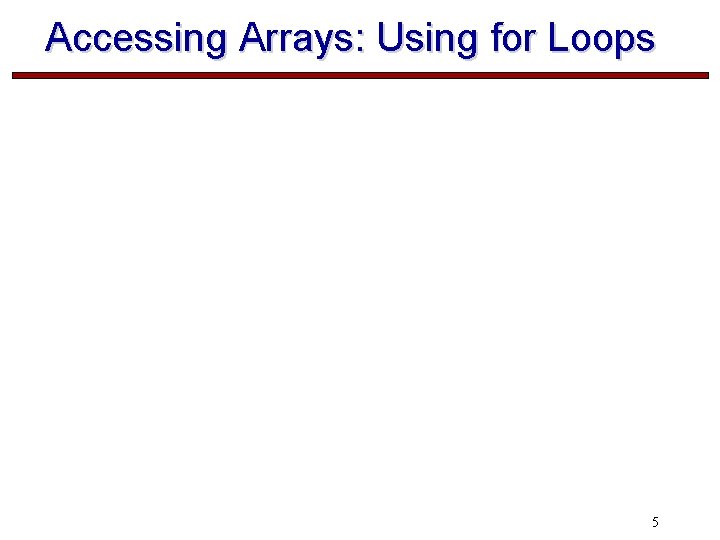
Accessing Arrays: Using for Loops 5
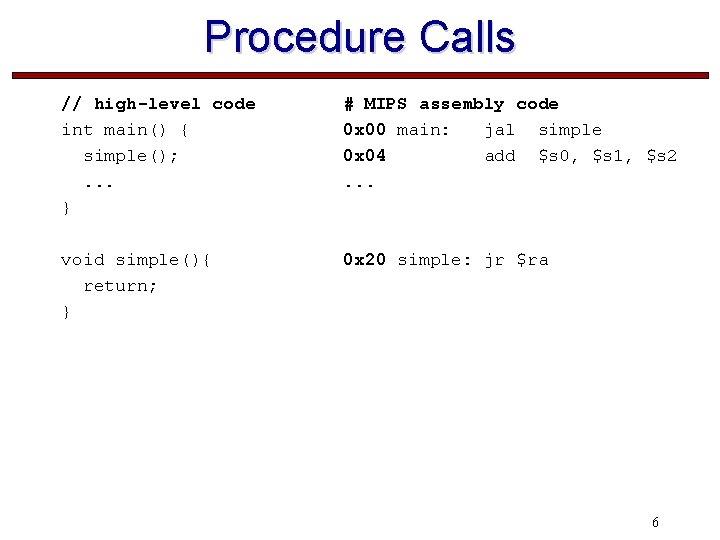
Procedure Calls // high-level code int main() { simple(); . . . } # MIPS assembly code 0 x 00 main: jal simple 0 x 04 add $s 0, $s 1, $s 2. . . void simple(){ return; } 0 x 20 simple: jr $ra 6
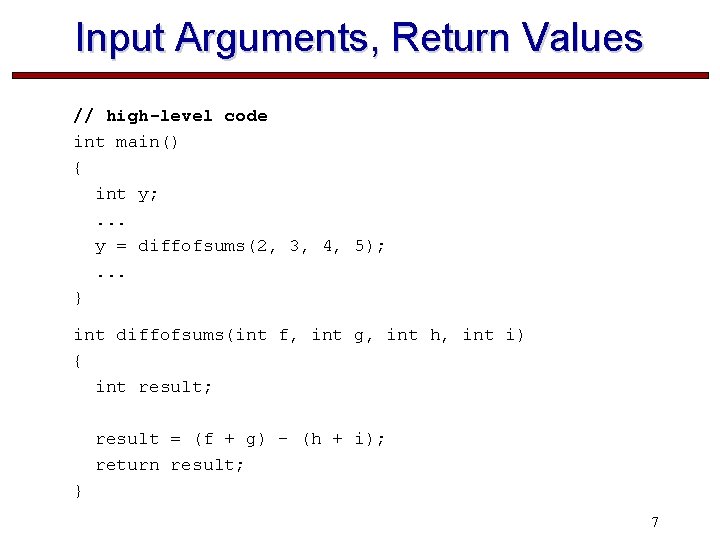
Input Arguments, Return Values // high-level code int main() { int y; . . . y = diffofsums(2, 3, 4, 5); . . . } int diffofsums(int f, int g, int h, int i) { int result; result = (f + g) - (h + i); return result; } 7
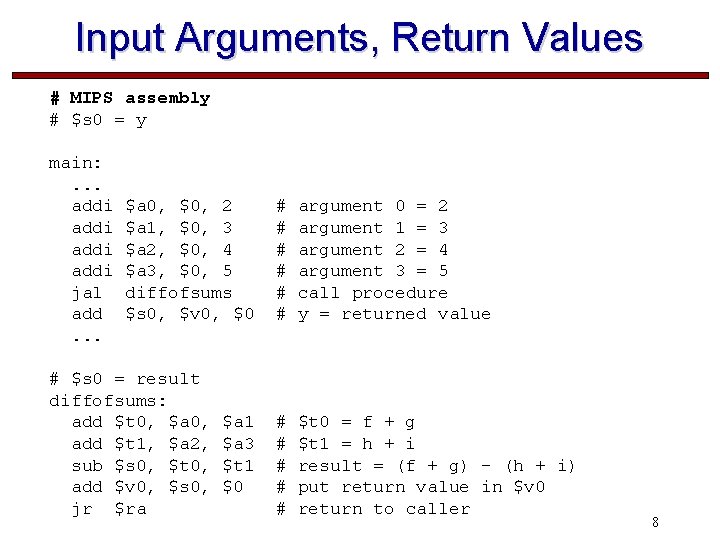
Input Arguments, Return Values # MIPS assembly # $s 0 = y main: . . . addi jal add. . . $a 0, $0, 2 $a 1, $0, 3 $a 2, $0, 4 $a 3, $0, 5 diffofsums $s 0, $v 0, $0 # $s 0 = result diffofsums: add $t 0, $a 0, add $t 1, $a 2, sub $s 0, $t 0, add $v 0, $s 0, jr $ra $a 1 $a 3 $t 1 $0 # # # argument 0 = 2 argument 1 = 3 argument 2 = 4 argument 3 = 5 call procedure y = returned value # # # $t 0 = f + g $t 1 = h + i result = (f + g) - (h + i) put return value in $v 0 return to caller 8
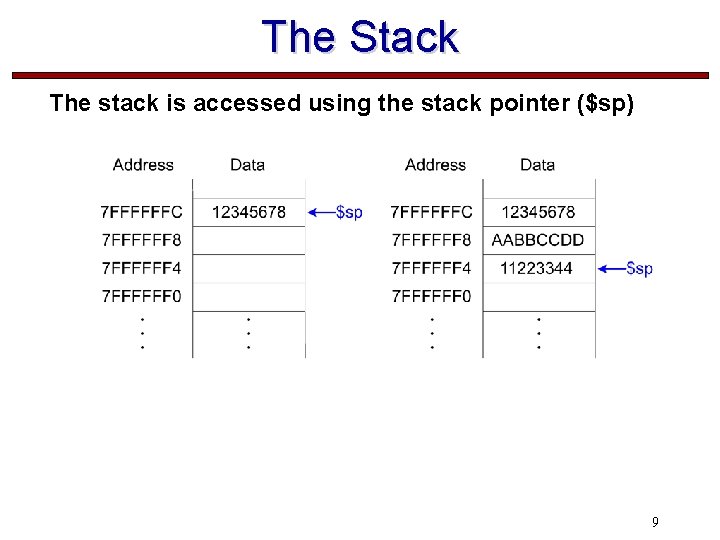
The Stack The stack is accessed using the stack pointer ($sp) 9
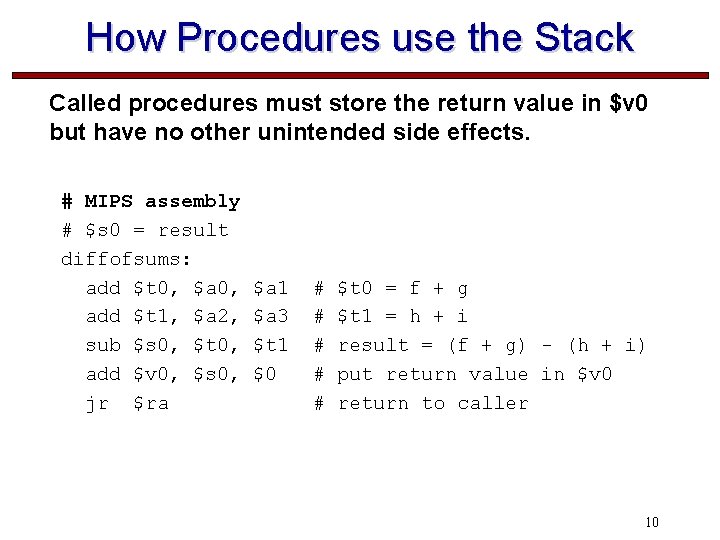
How Procedures use the Stack Called procedures must store the return value in $v 0 but have no other unintended side effects. # MIPS assembly # $s 0 = result diffofsums: add $t 0, $a 0, add $t 1, $a 2, sub $s 0, $t 0, add $v 0, $s 0, jr $ra $a 1 $a 3 $t 1 $0 # # # $t 0 = f + g $t 1 = h + i result = (f + g) - (h + i) put return value in $v 0 return to caller 10
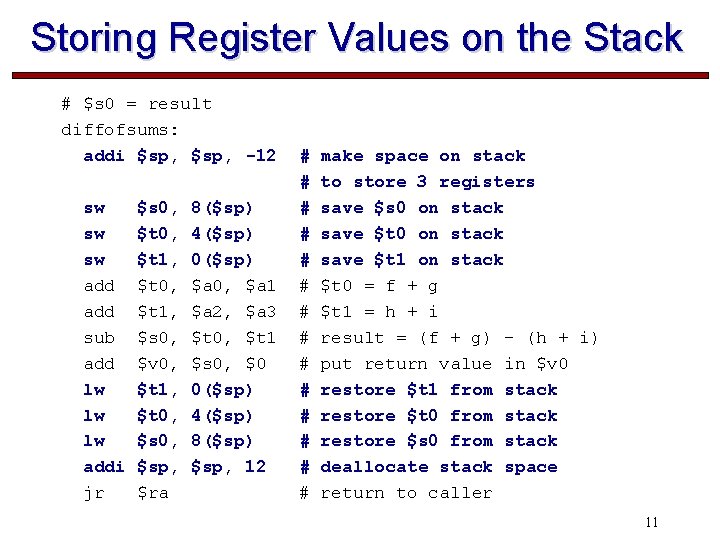
Storing Register Values on the Stack # $s 0 = result diffofsums: addi $sp, -12 sw sw sw add sub add lw lw lw addi jr $s 0, $t 1, $t 0, $t 1, $s 0, $v 0, $t 1, $t 0, $sp, $ra 8($sp) 4($sp) 0($sp) $a 0, $a 1 $a 2, $a 3 $t 0, $t 1 $s 0, $0 0($sp) 4($sp) 8($sp) $sp, 12 # # # # make space on stack to store 3 registers save $s 0 on stack save $t 1 on stack $t 0 = f + g $t 1 = h + i result = (f + g) - (h + i) put return value in $v 0 restore $t 1 from stack restore $t 0 from stack restore $s 0 from stack deallocate stack space return to caller 11
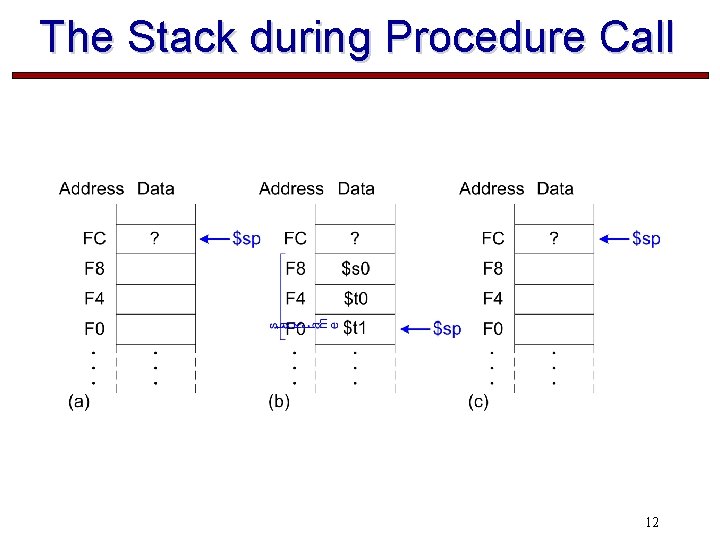
The Stack during Procedure Call 12
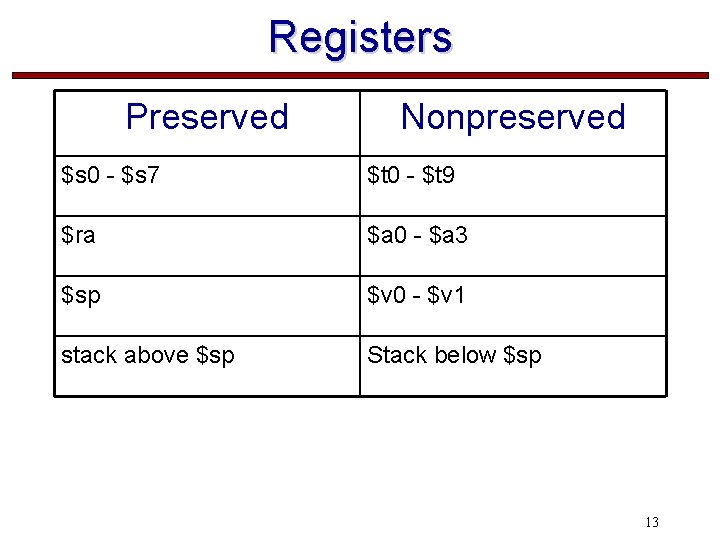
Registers Preserved Nonpreserved $s 0 - $s 7 $t 0 - $t 9 $ra $a 0 - $a 3 $sp $v 0 - $v 1 stack above $sp Stack below $sp 13
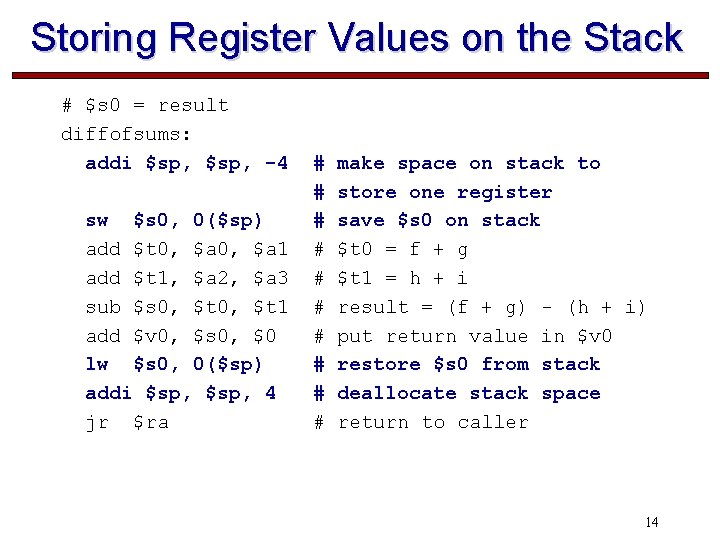
Storing Register Values on the Stack # $s 0 = result diffofsums: addi $sp, -4 sw $s 0, 0($sp) add $t 0, $a 1 add $t 1, $a 2, $a 3 sub $s 0, $t 1 add $v 0, $s 0, $0 lw $s 0, 0($sp) addi $sp, 4 jr $ra # # # # # make space on stack to store one register save $s 0 on stack $t 0 = f + g $t 1 = h + i result = (f + g) - (h + i) put return value in $v 0 restore $s 0 from stack deallocate stack space return to caller 14
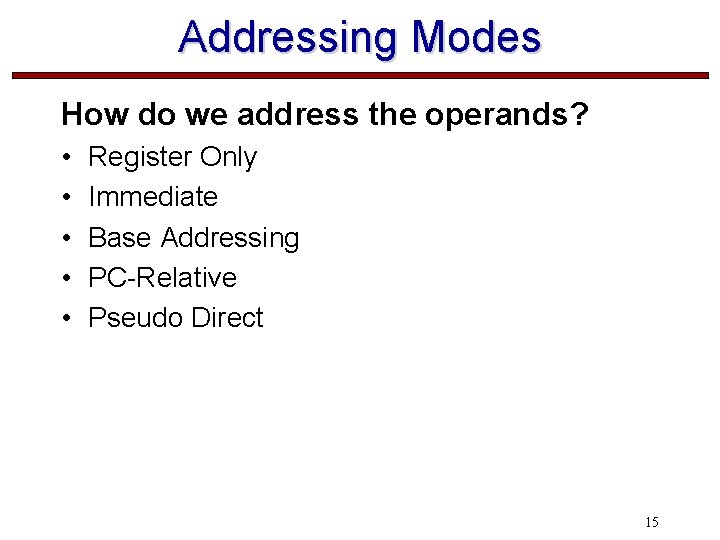
Addressing Modes How do we address the operands? • • • Register Only Immediate Base Addressing PC-Relative Pseudo Direct 15
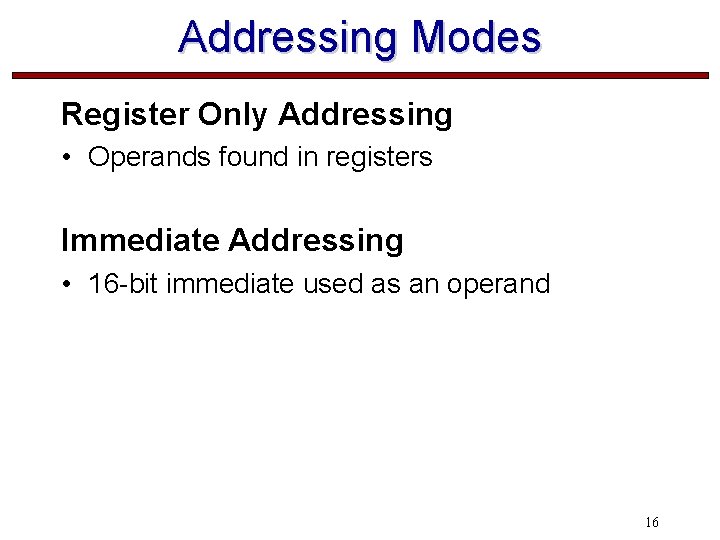
Addressing Modes Register Only Addressing • Operands found in registers Immediate Addressing • 16 -bit immediate used as an operand 16
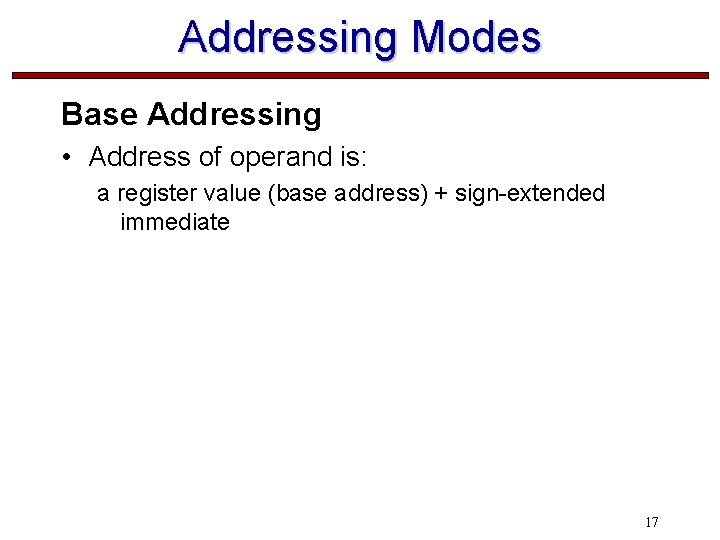
Addressing Modes Base Addressing • Address of operand is: a register value (base address) + sign-extended immediate 17
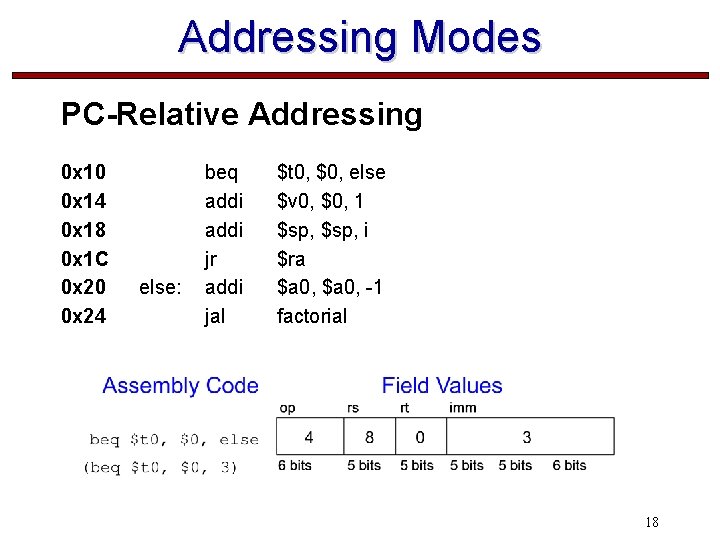
Addressing Modes PC-Relative Addressing 0 x 10 0 x 14 0 x 18 0 x 1 C 0 x 20 0 x 24 else: beq addi jr addi jal $t 0, $0, else $v 0, $0, 1 $sp, i $ra $a 0, -1 factorial 18
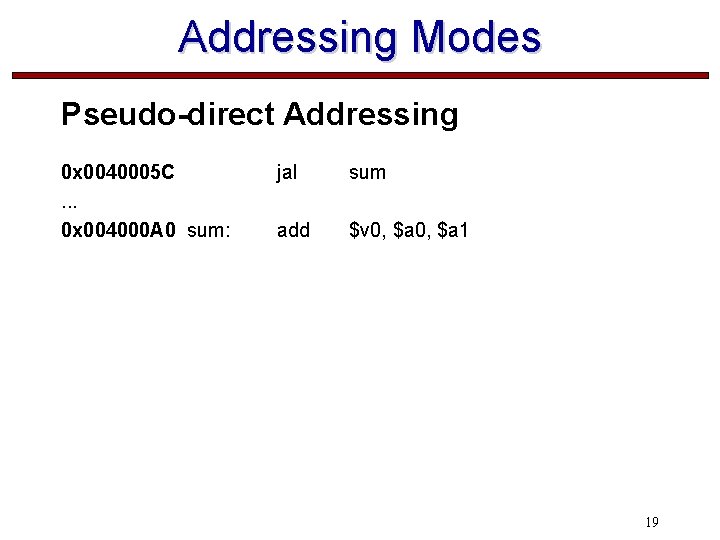
Addressing Modes Pseudo-direct Addressing 0 x 0040005 C. . . 0 x 004000 A 0 sum: jal sum add $v 0, $a 1 19
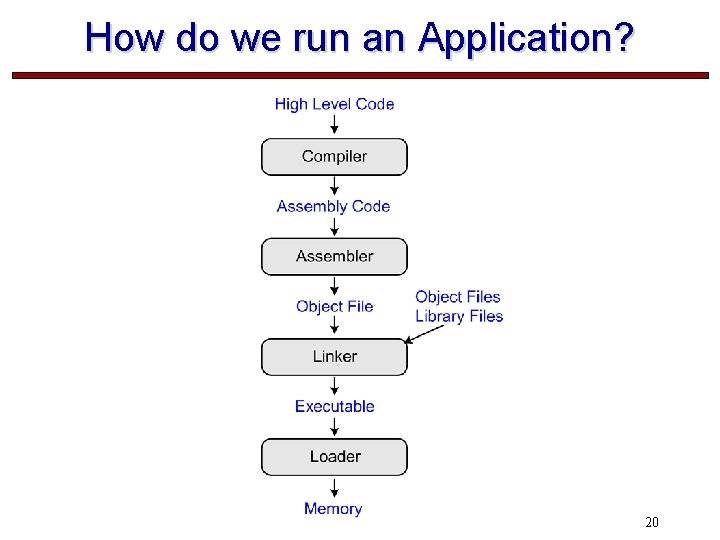
How do we run an Application? 20
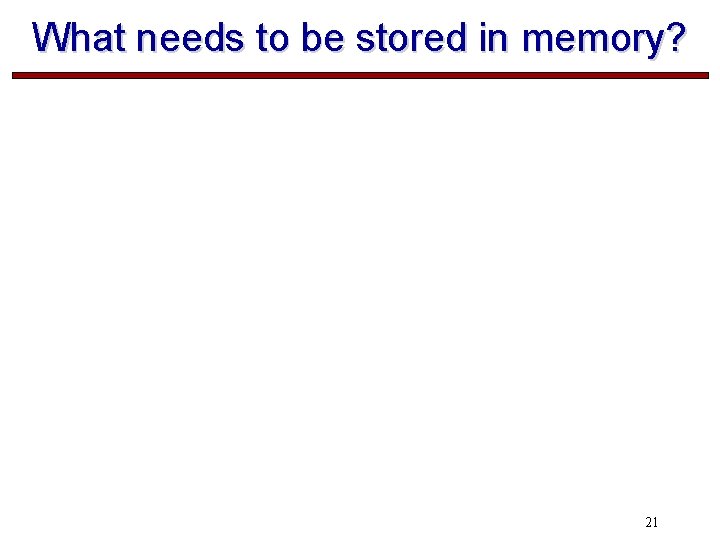
What needs to be stored in memory? 21
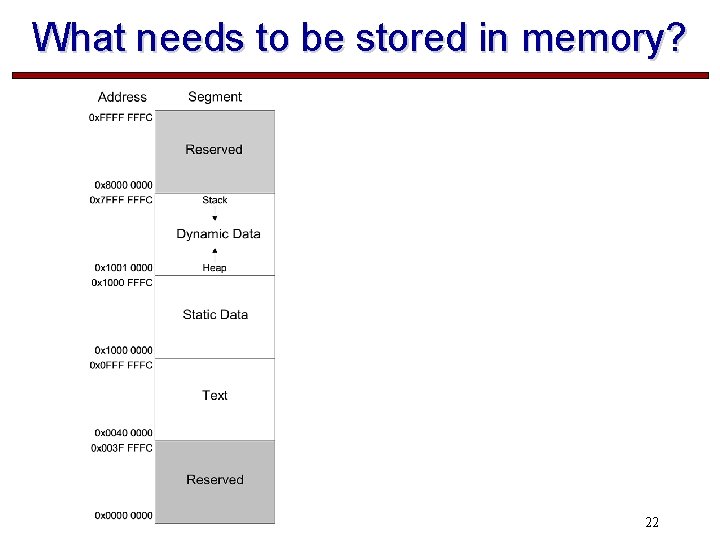
What needs to be stored in memory? 22
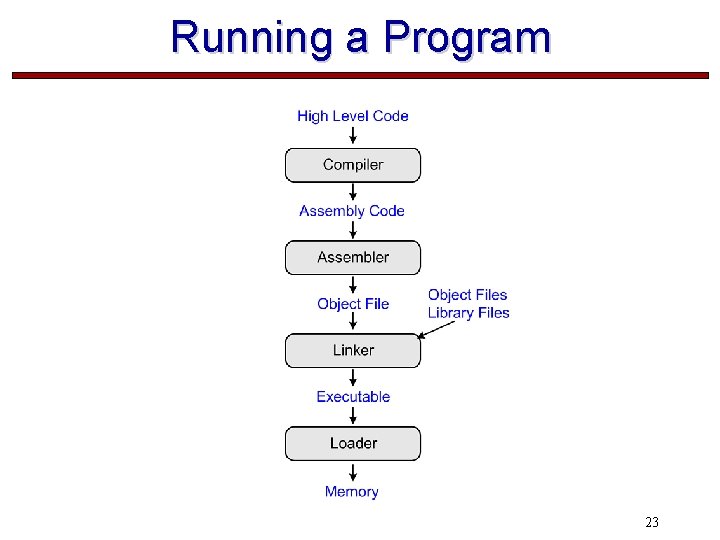
Running a Program 23
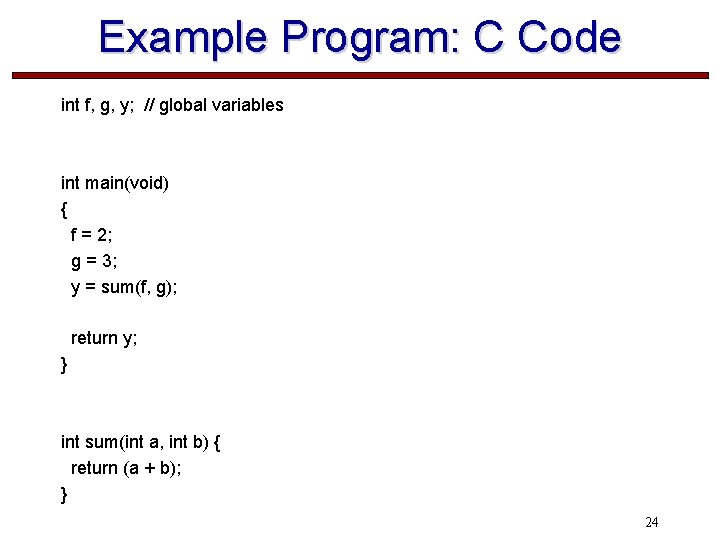
Example Program: C Code int f, g, y; // global variables int main(void) { f = 2; g = 3; y = sum(f, g); return y; } int sum(int a, int b) { return (a + b); } 24
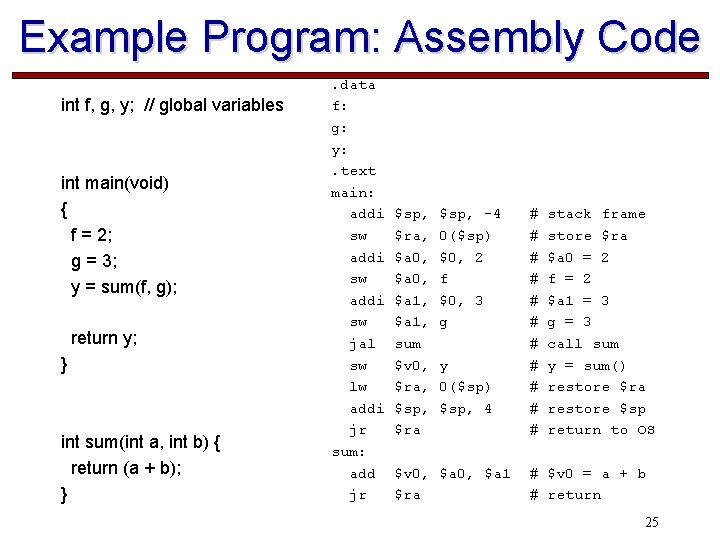
Example Program: Assembly Code int f, g, y; // global variables int main(void) { f = 2; g = 3; y = sum(f, g); return y; } int sum(int a, int b) { return (a + b); } . data f: g: y: . text main: addi sw jal sw lw addi jr sum: add jr $sp, $ra, $a 0, $a 1, sum $v 0, $ra, $sp, $ra $sp, -4 0($sp) $0, 2 f $0, 3 g y 0($sp) $sp, 4 $v 0, $a 1 $ra # # # stack frame store $ra $a 0 = 2 f = 2 $a 1 = 3 g = 3 call sum y = sum() restore $ra restore $sp return to OS # $v 0 = a + b # return 25
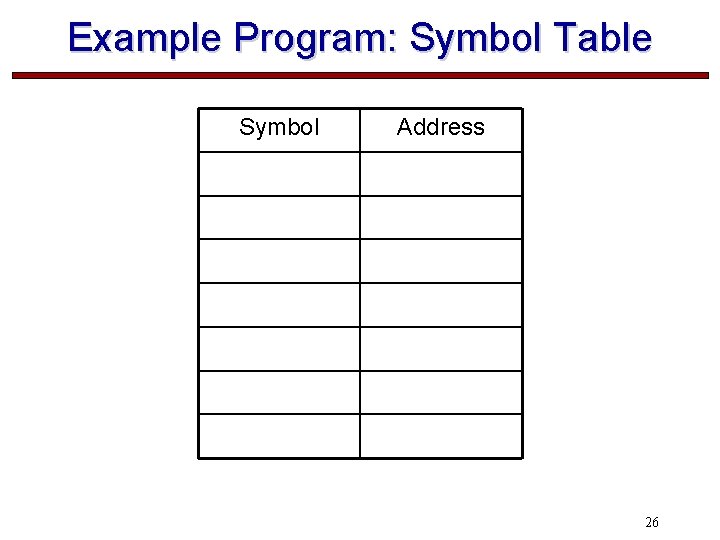
Example Program: Symbol Table Symbol Address 26
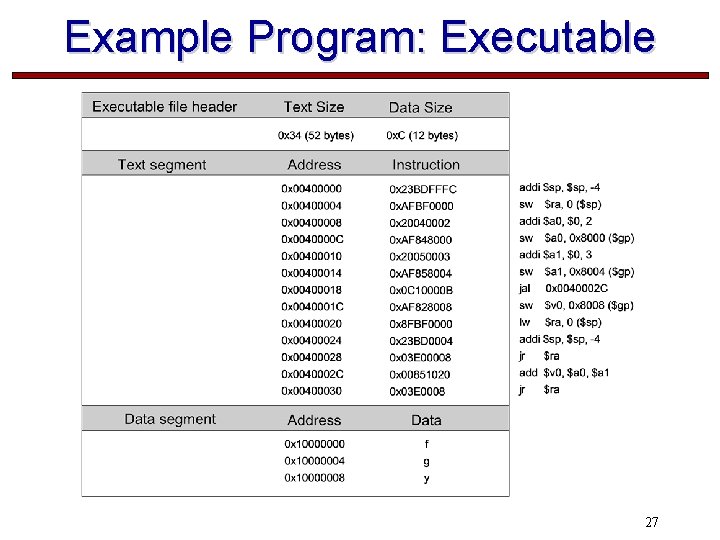
Example Program: Executable 27
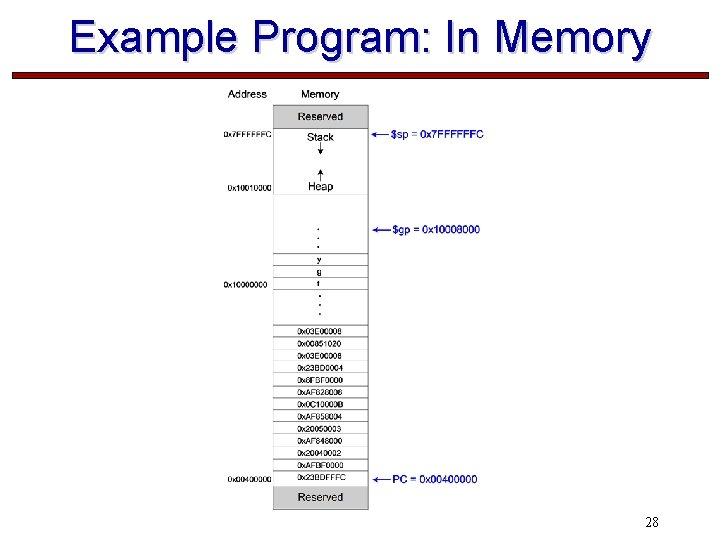
Example Program: In Memory 28
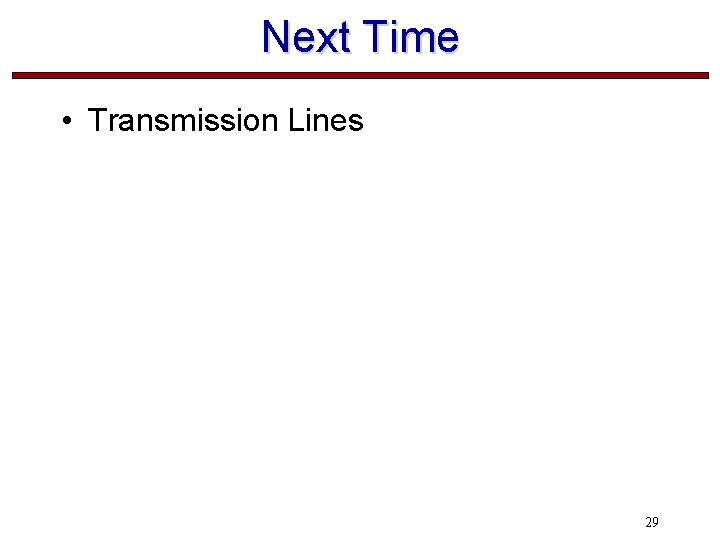
Next Time • Transmission Lines 29
Dynamic picture
01:640:244 lecture notes - lecture 15: plat, idah, farad
Tahap keluarga
Tony and sue are launching yard darts
Contoh backgrounder press release
Mst physics 1135
Launching the nation section 1 answers
Entrepreneurship successfully launching new ventures
Personal entrepreneurial competencies meaning
Chapter 9 launching a new republic
Tost winch
Parenting/expanding stage
Chapter 10 launching the new ship of state
Launching the nation section 1 answers
Chapter 10 launching the new ship of state
Chapter 2 section 4 launching the new nation
When at rest on the launching pad the force
Launching customer
Chapter 9 launching a new republic
H.i.n.k.merupakan akronim dari :
Digital goods ecommerce
Digital data digital signals
Digital data digital signals
E-commerce: digital markets, digital goods
Data encoding techniques
"key international"
E-commerce digital markets digital goods
Double meaning story
Themes in an inspector calls
It is a repetitive process in which algorithm calls itself.