5 Remote Procedure Call Regular Procedure Calls How
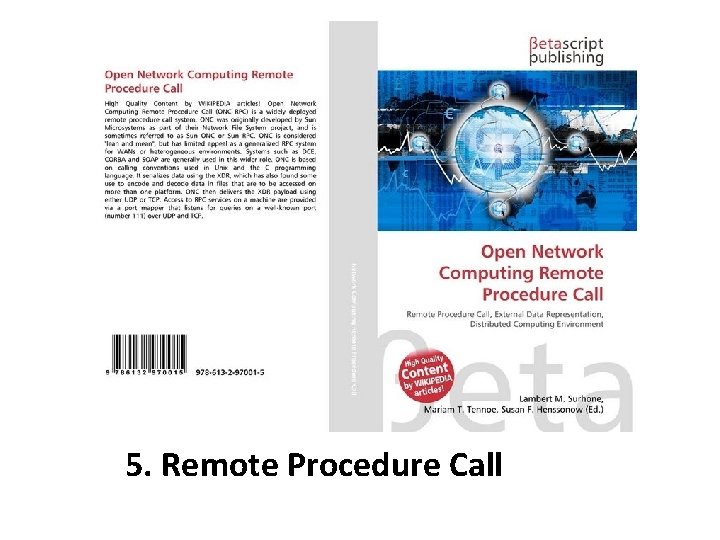
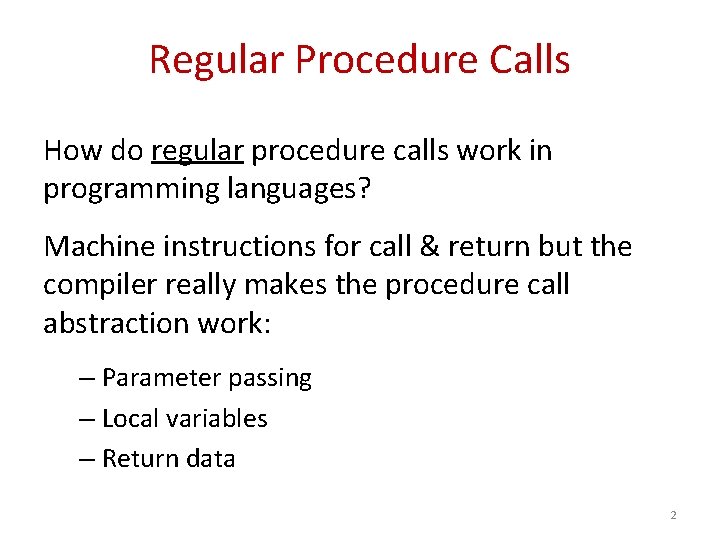
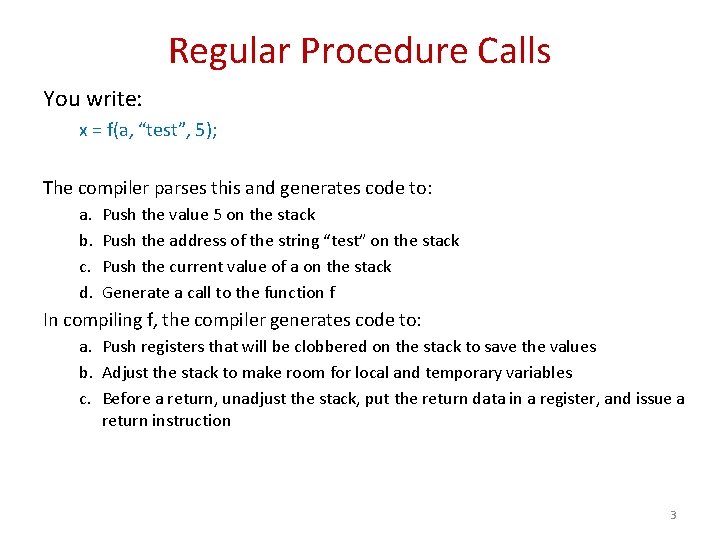
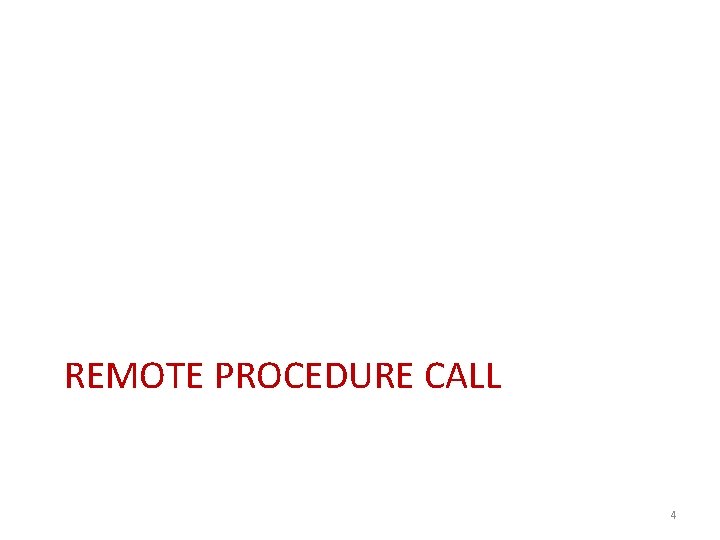
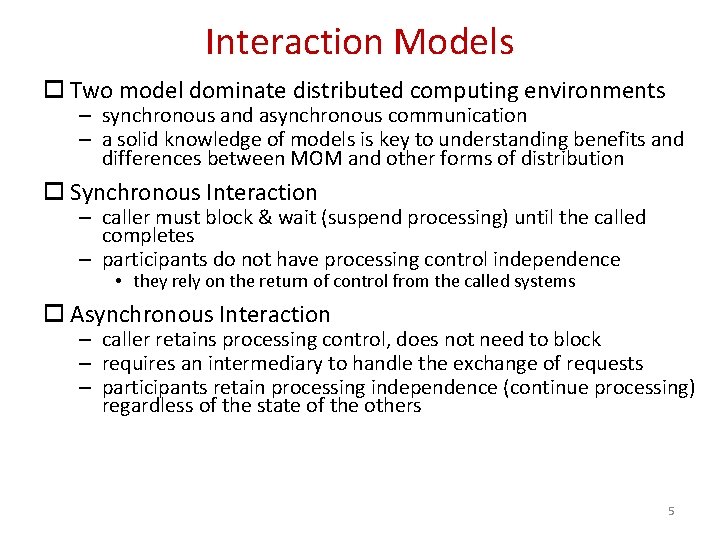
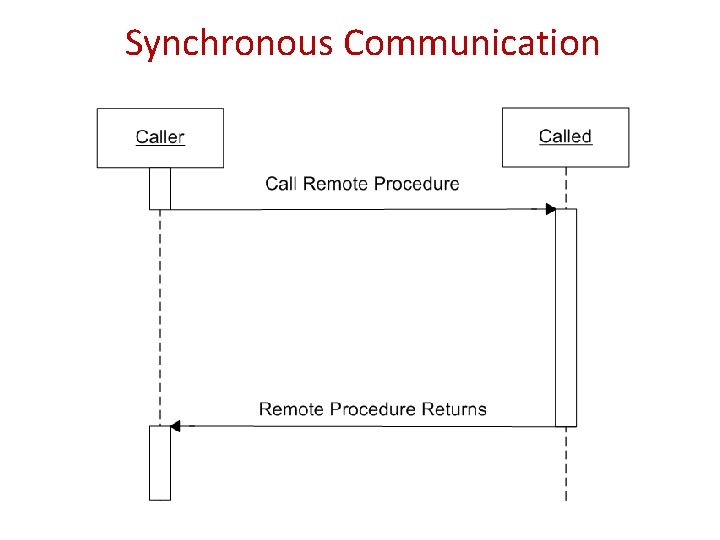
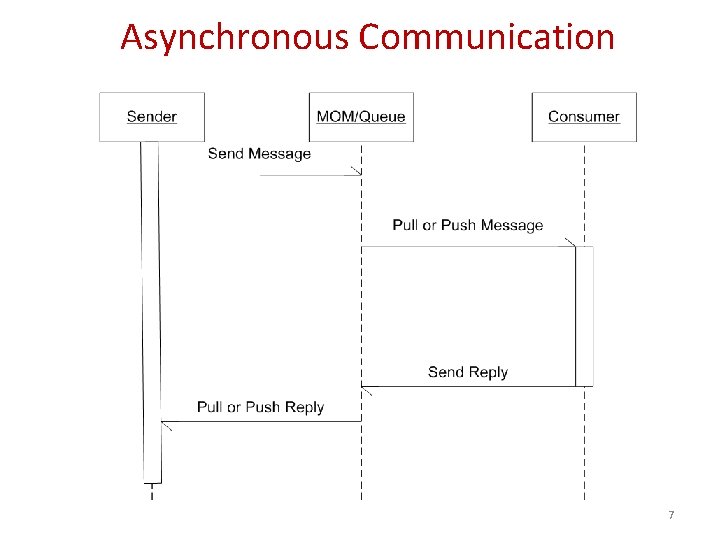
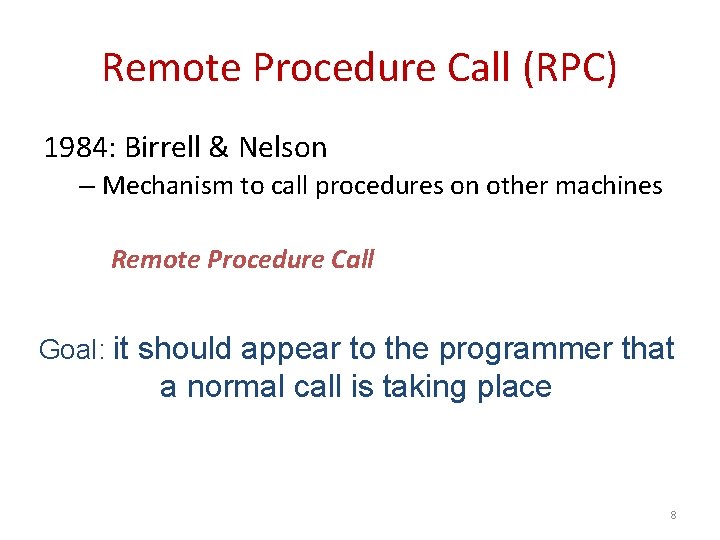
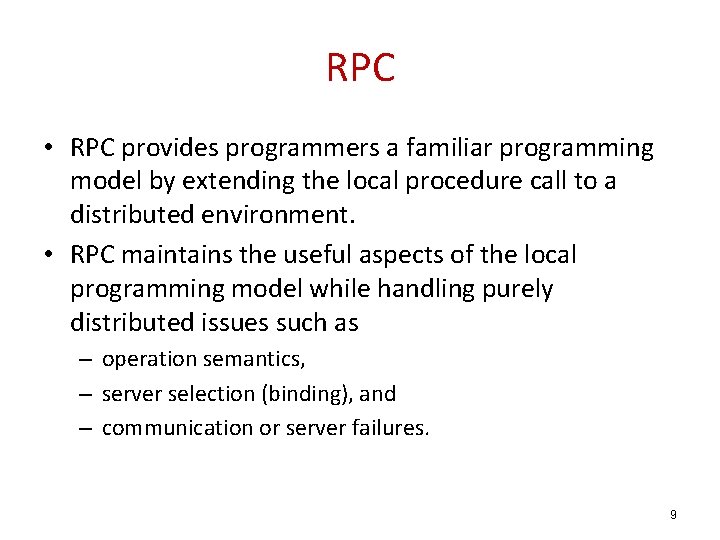
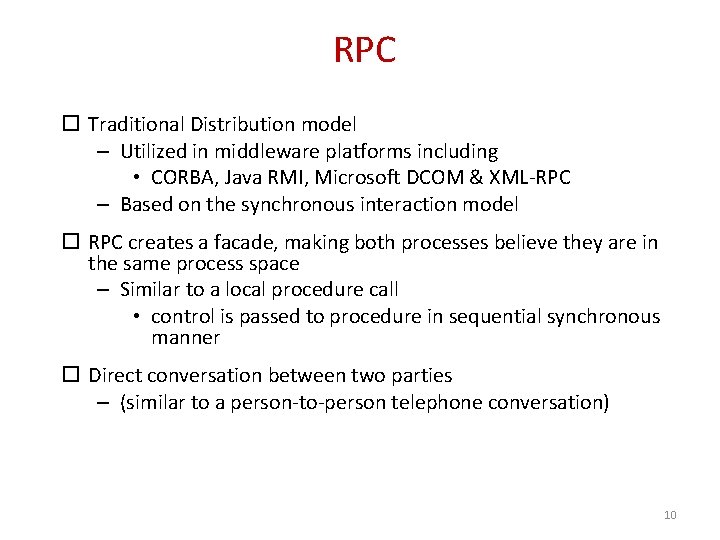
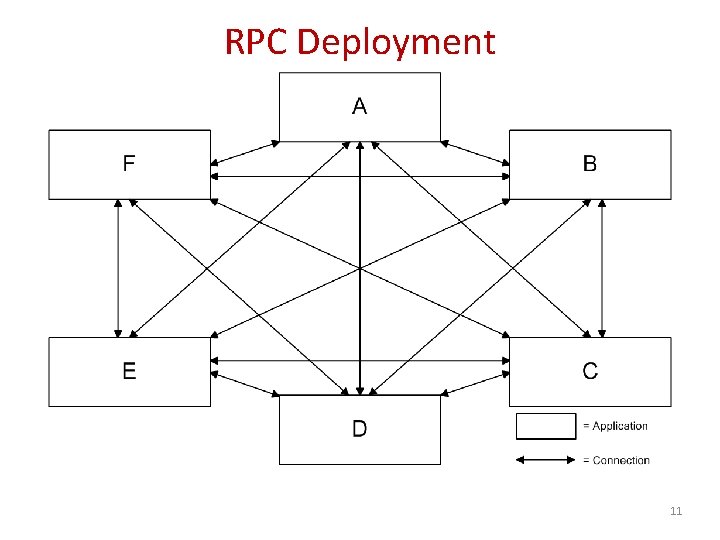
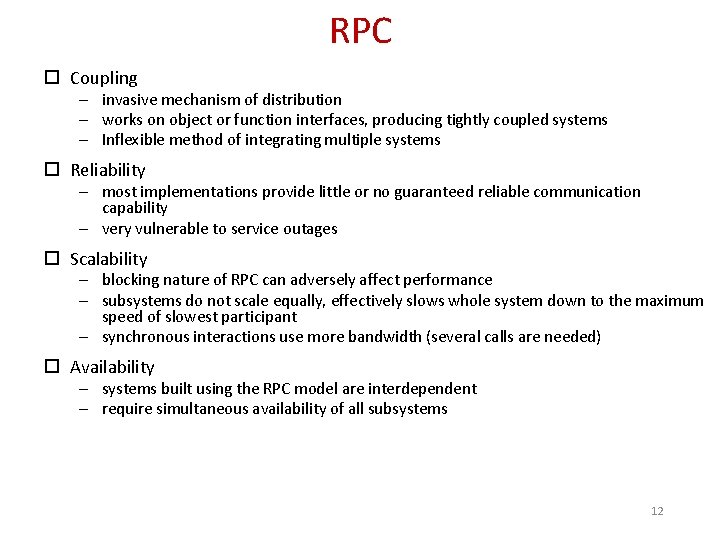
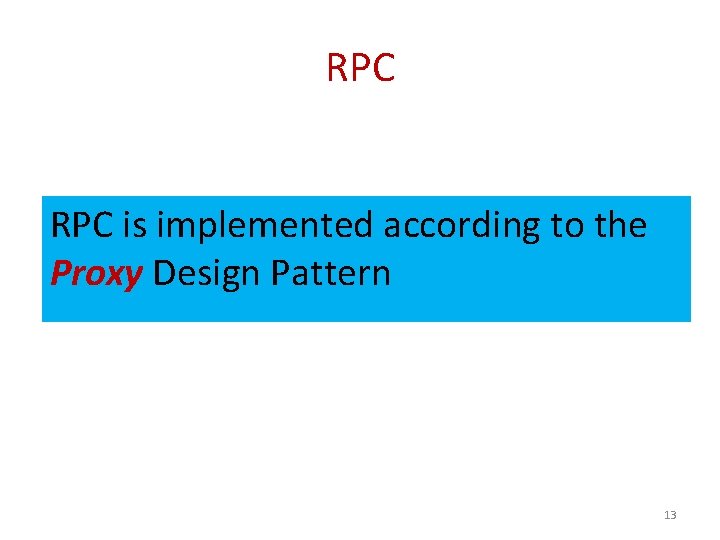
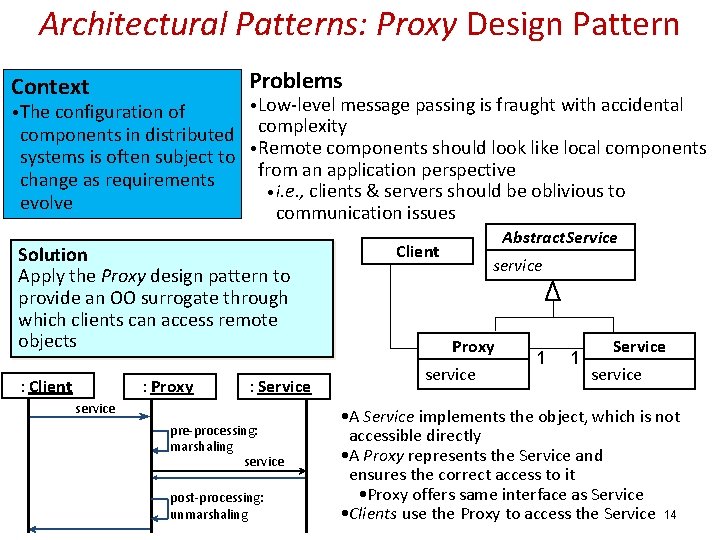
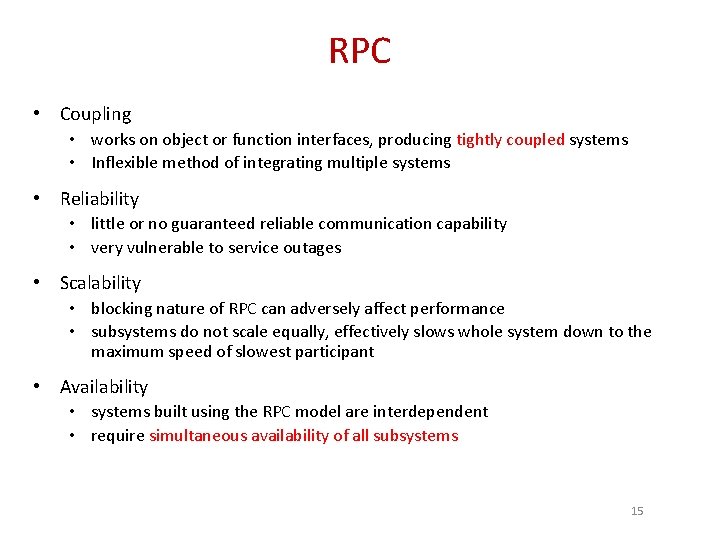
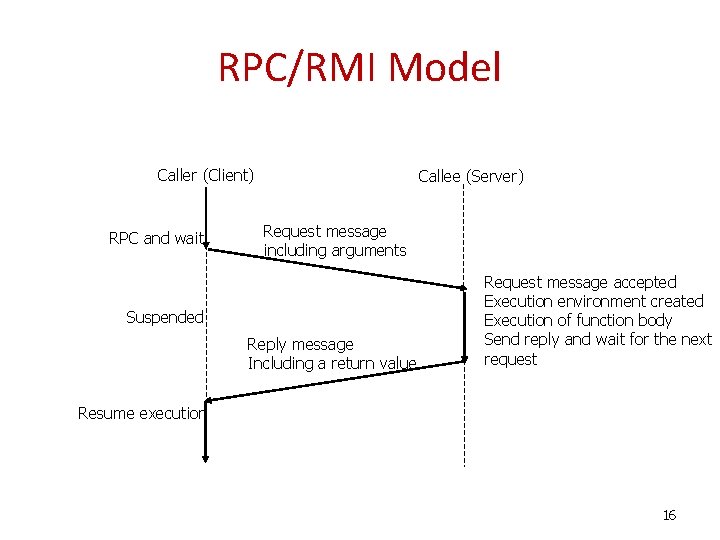
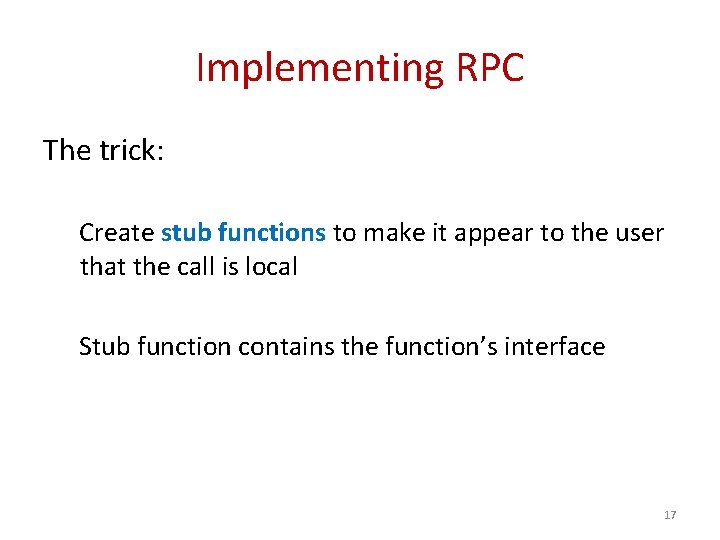
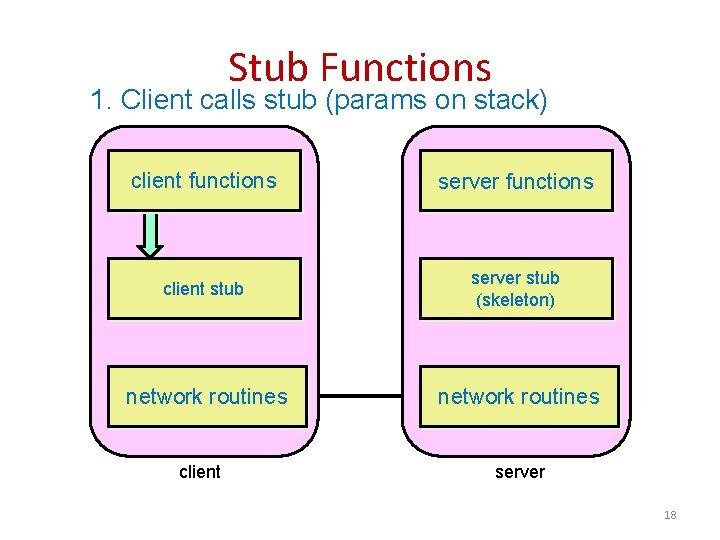
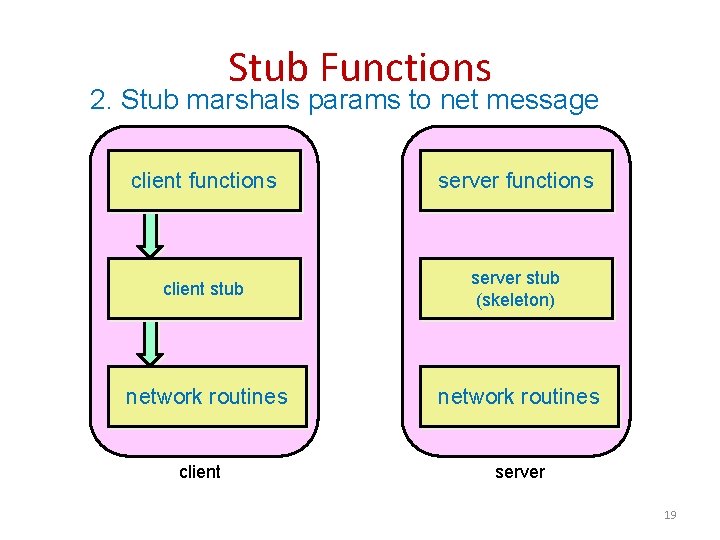
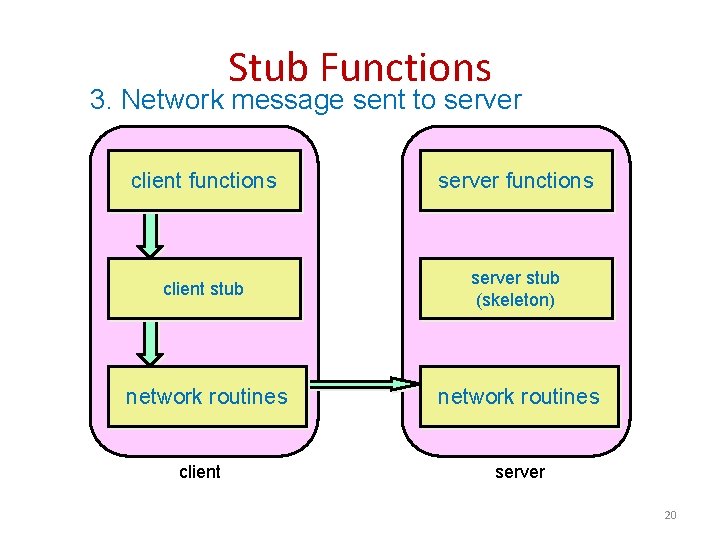
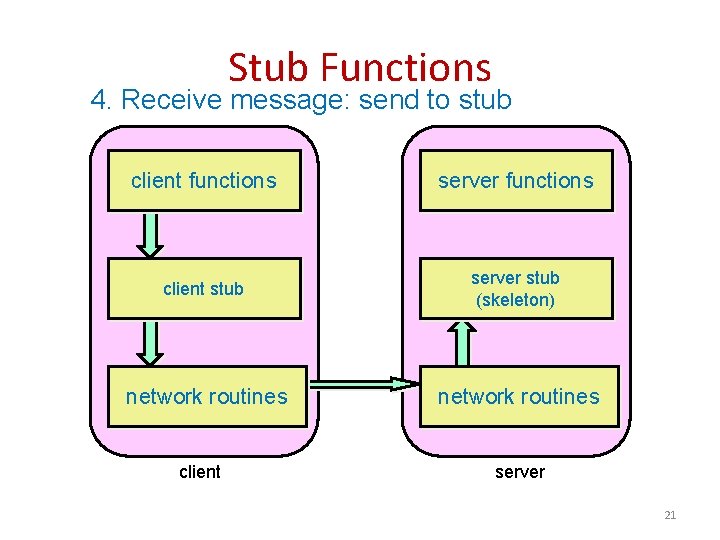
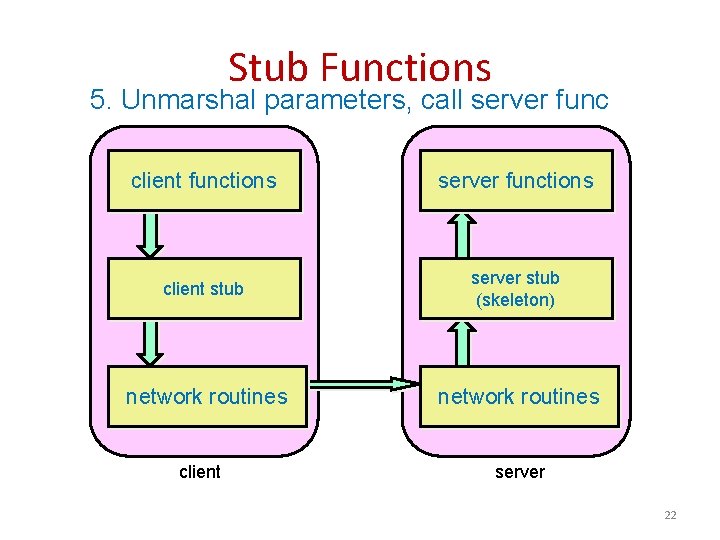
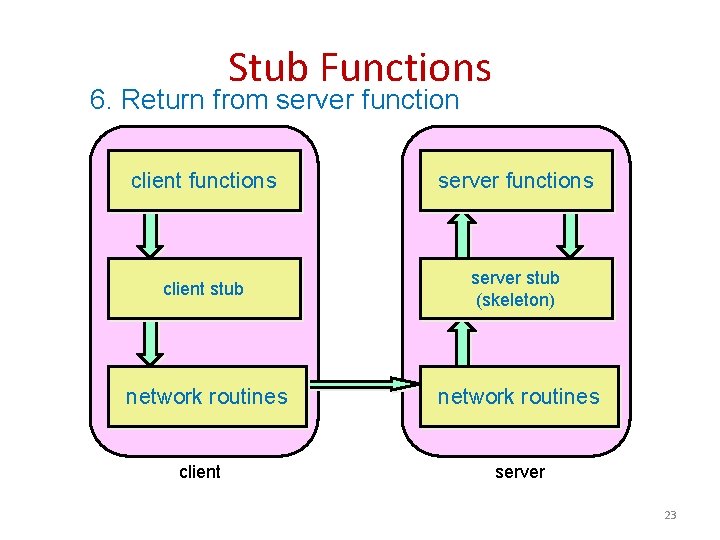
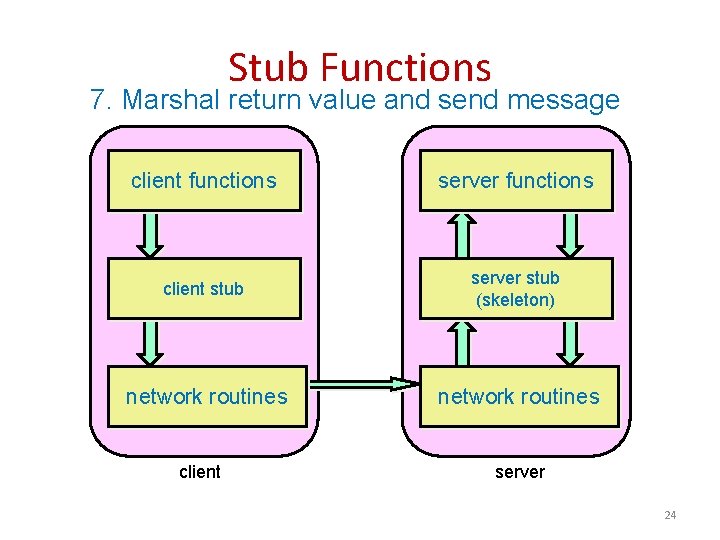
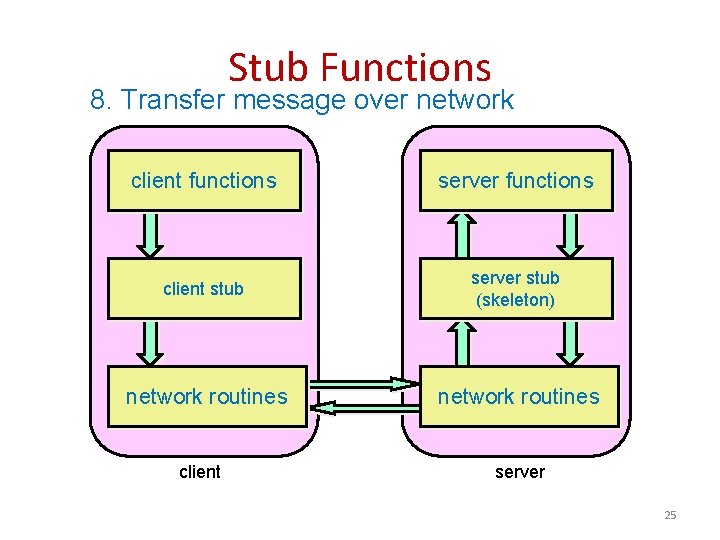
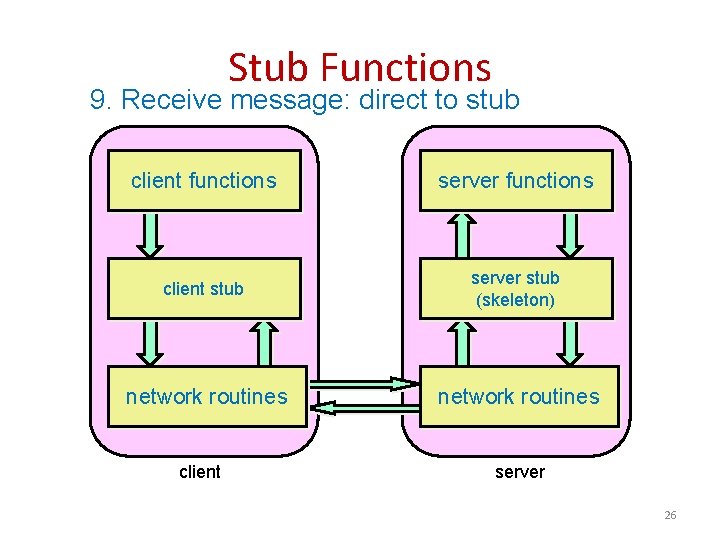
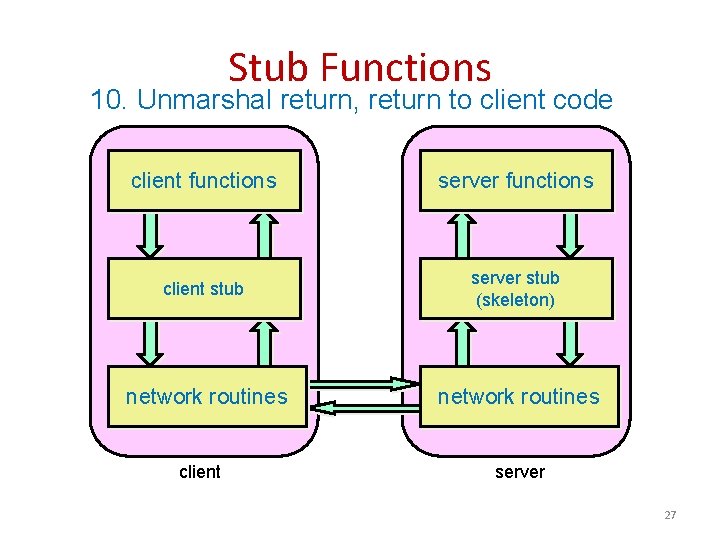
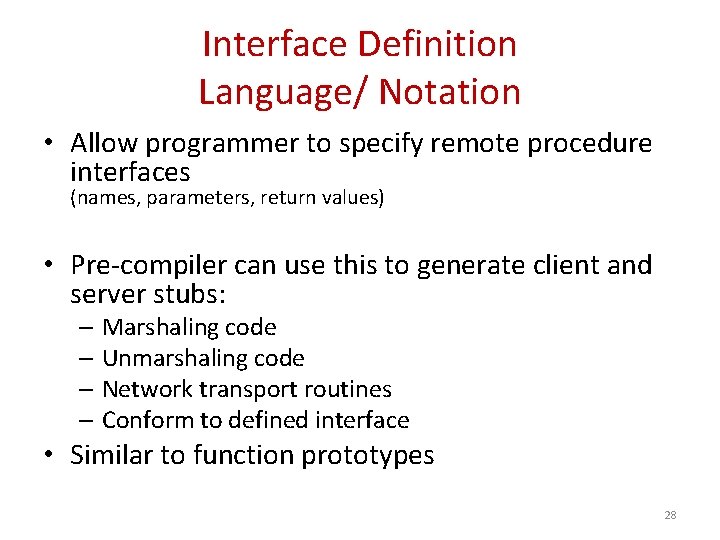
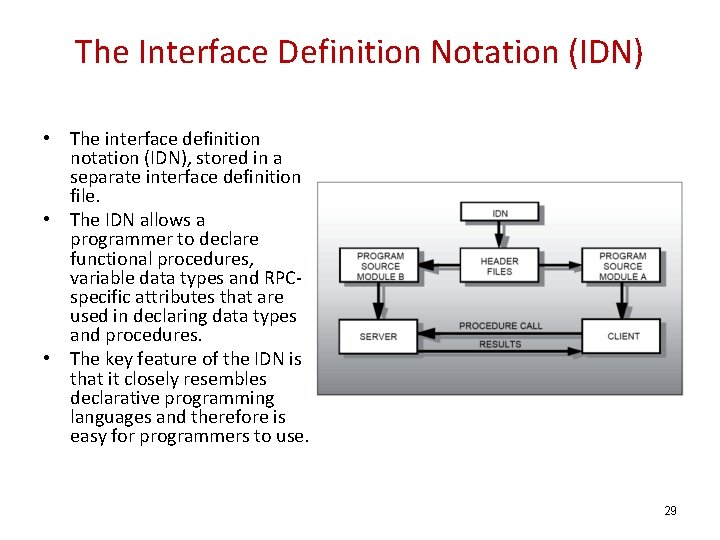
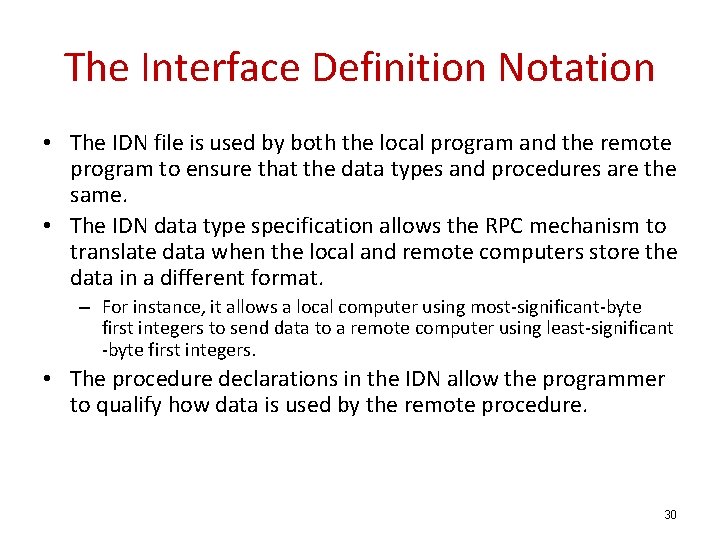
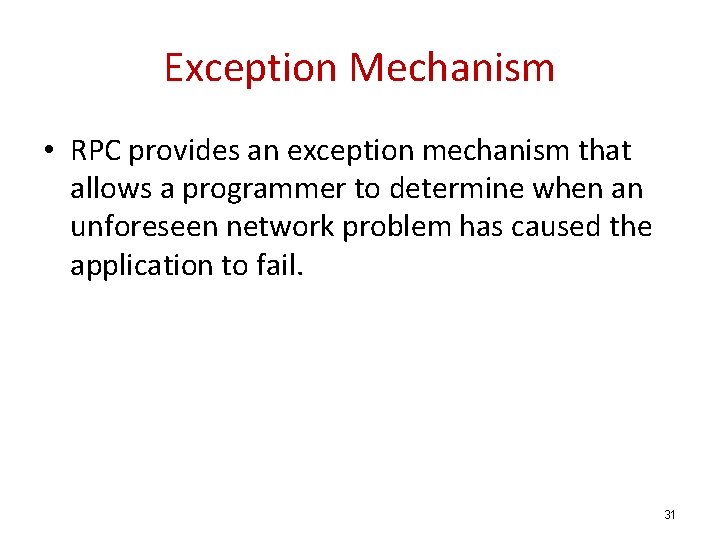
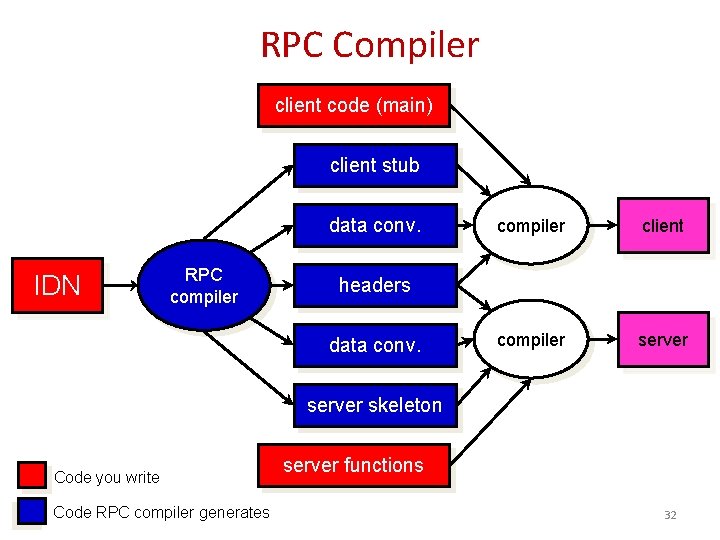
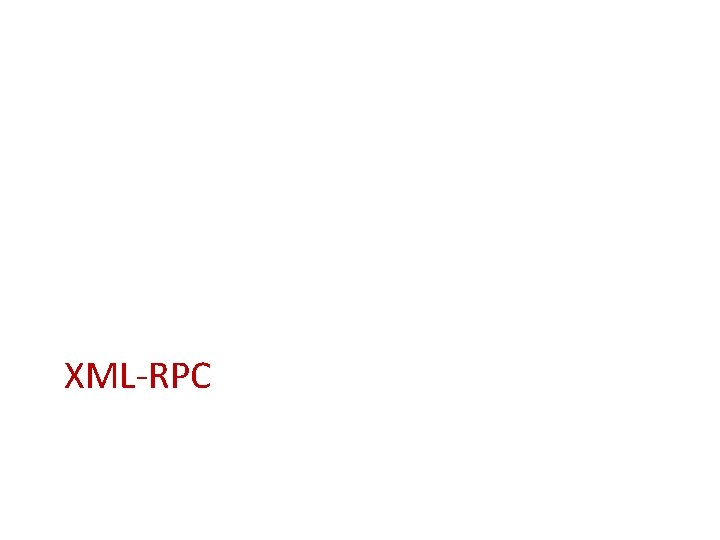
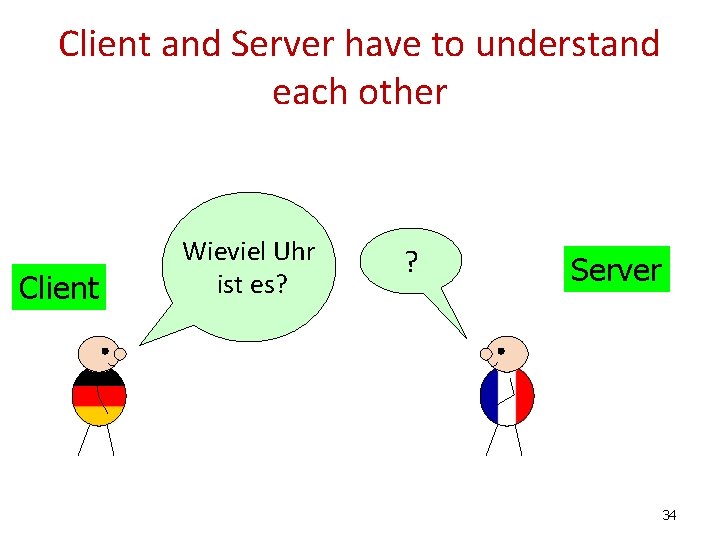
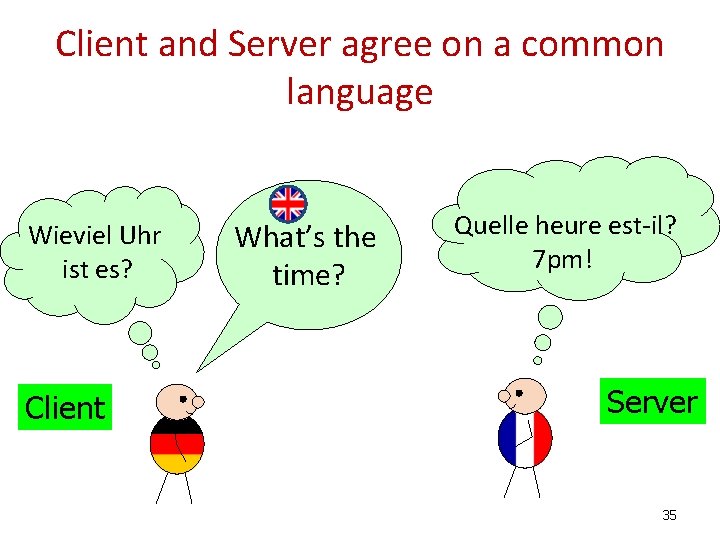
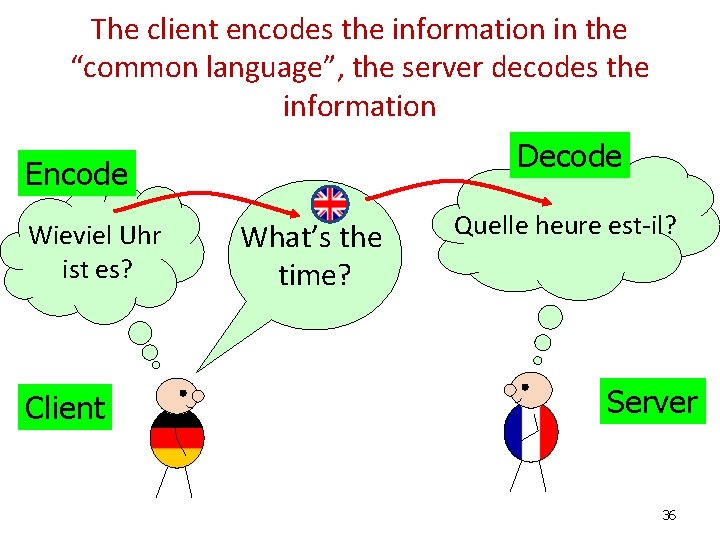
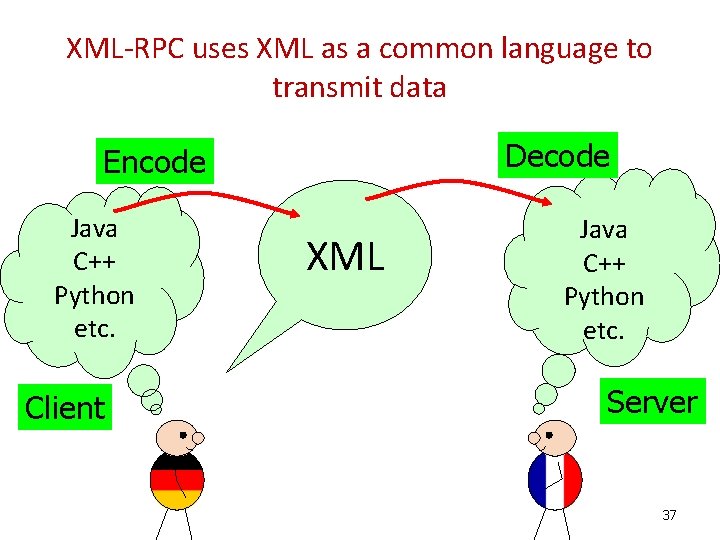
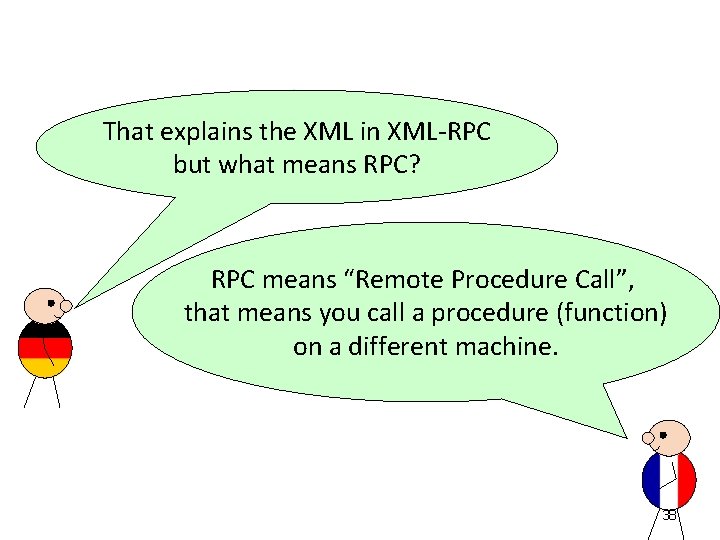
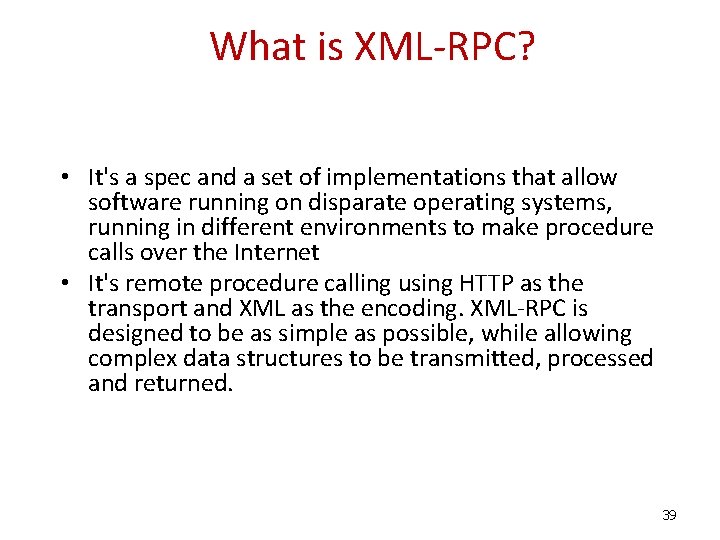
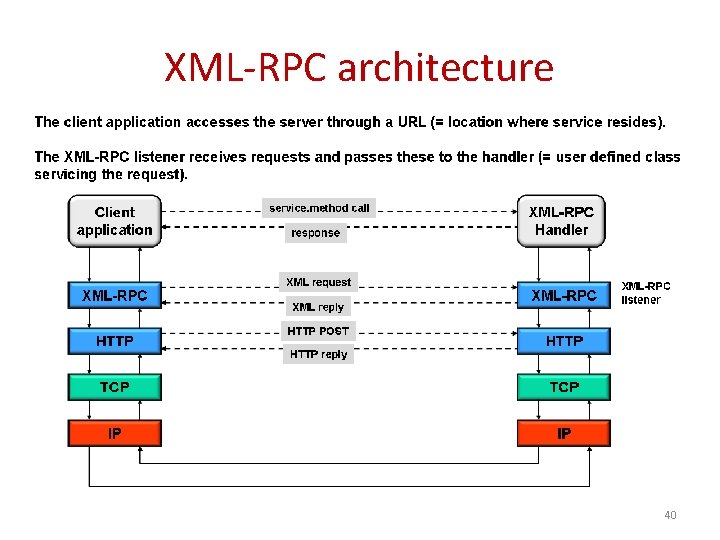
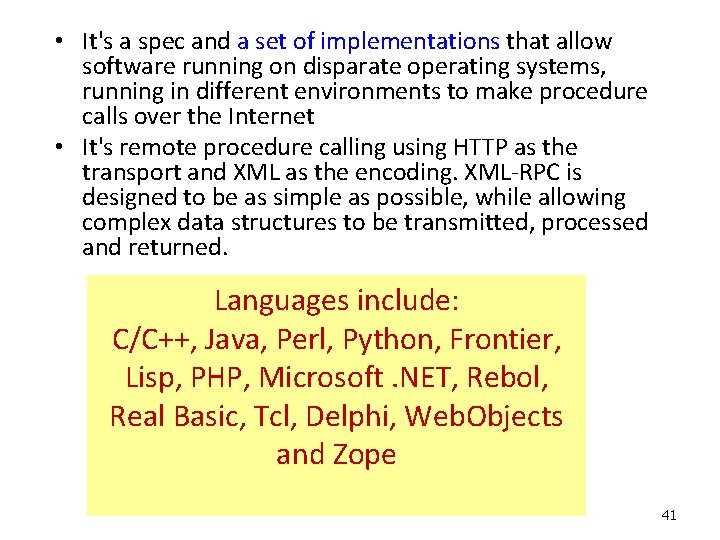
- Slides: 41
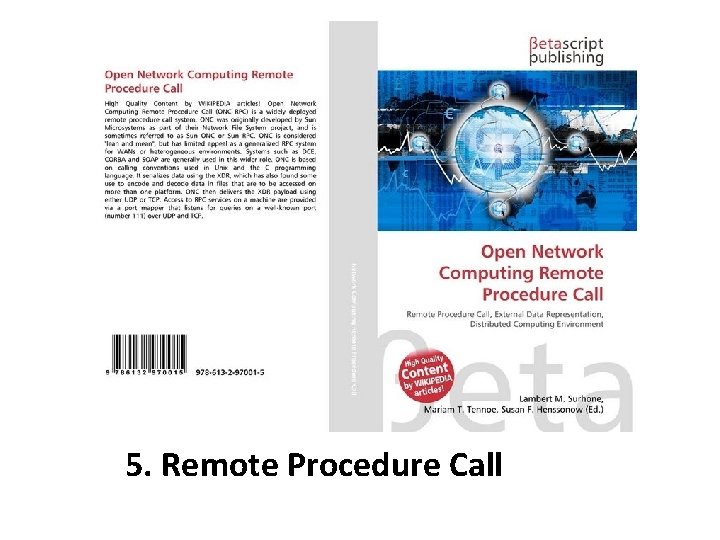
5. Remote Procedure Call
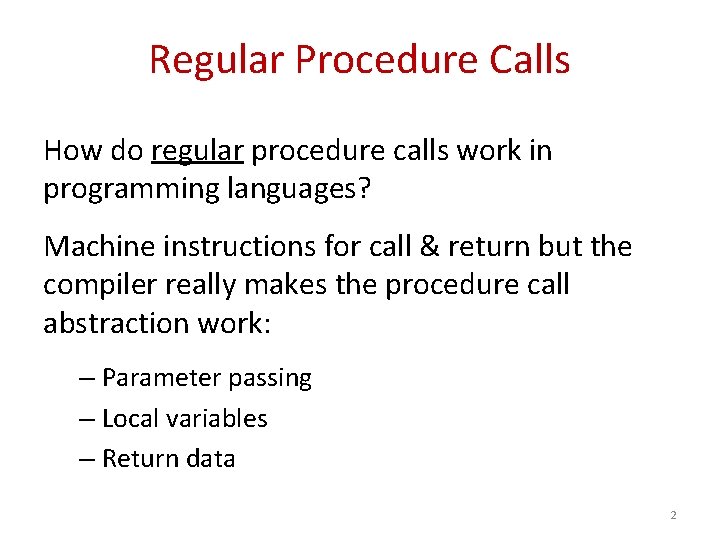
Regular Procedure Calls How do regular procedure calls work in programming languages? Machine instructions for call & return but the compiler really makes the procedure call abstraction work: – Parameter passing – Local variables – Return data 2
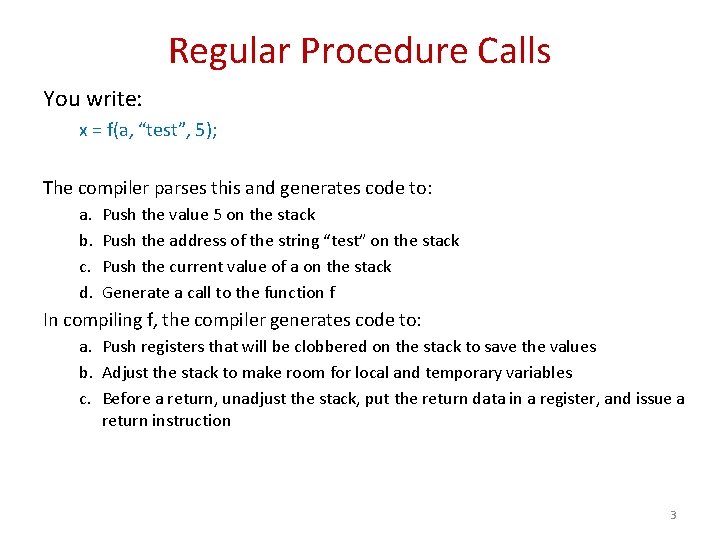
Regular Procedure Calls You write: x = f(a, “test”, 5); The compiler parses this and generates code to: a. b. c. d. Push the value 5 on the stack Push the address of the string “test” on the stack Push the current value of a on the stack Generate a call to the function f In compiling f, the compiler generates code to: a. Push registers that will be clobbered on the stack to save the values b. Adjust the stack to make room for local and temporary variables c. Before a return, unadjust the stack, put the return data in a register, and issue a return instruction 3
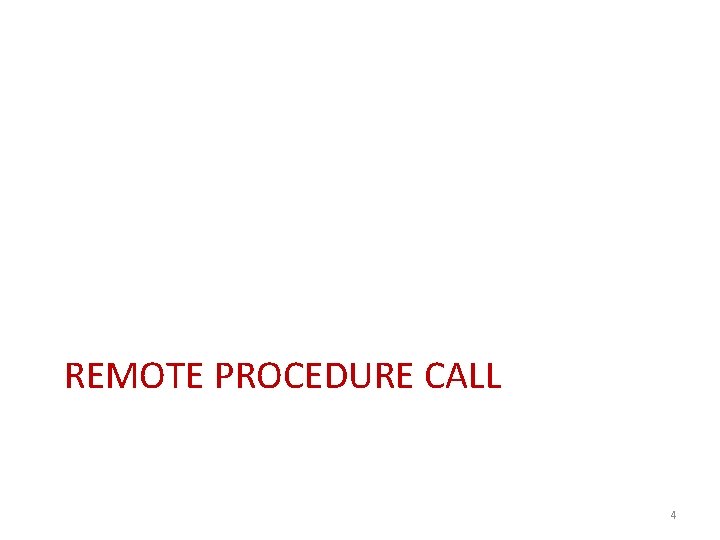
REMOTE PROCEDURE CALL 4
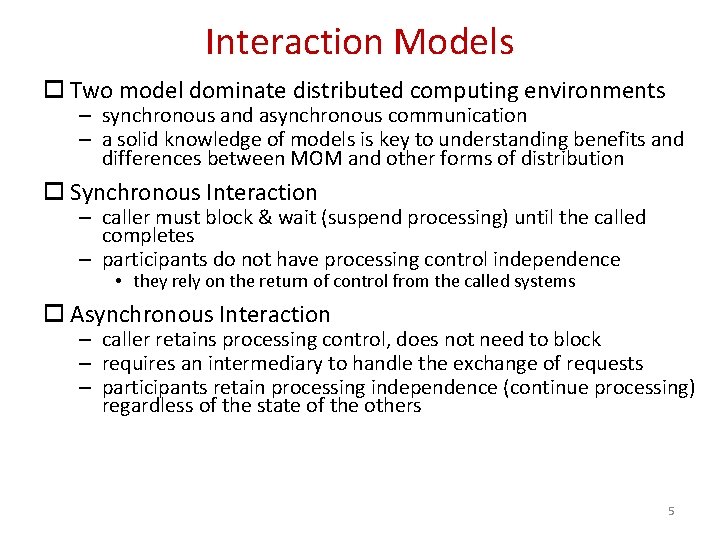
Interaction Models o Two model dominate distributed computing environments – synchronous and asynchronous communication – a solid knowledge of models is key to understanding benefits and differences between MOM and other forms of distribution o Synchronous Interaction – caller must block & wait (suspend processing) until the called completes – participants do not have processing control independence • they rely on the return of control from the called systems o Asynchronous Interaction – caller retains processing control, does not need to block – requires an intermediary to handle the exchange of requests – participants retain processing independence (continue processing) regardless of the state of the others 5
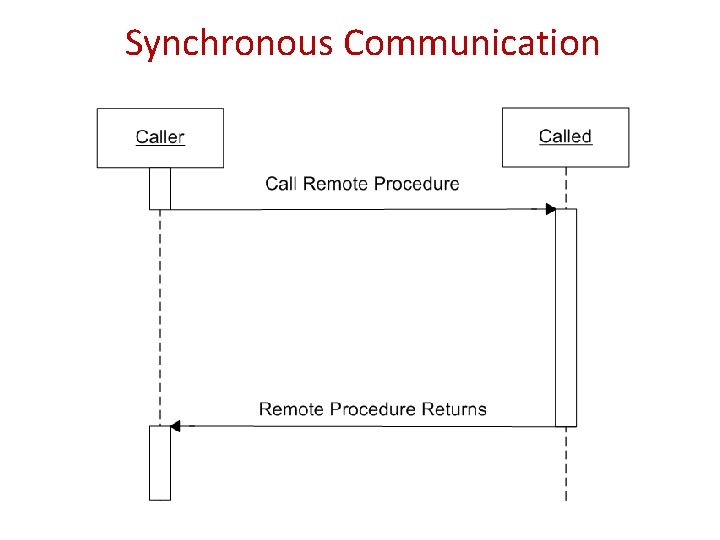
Synchronous Communication
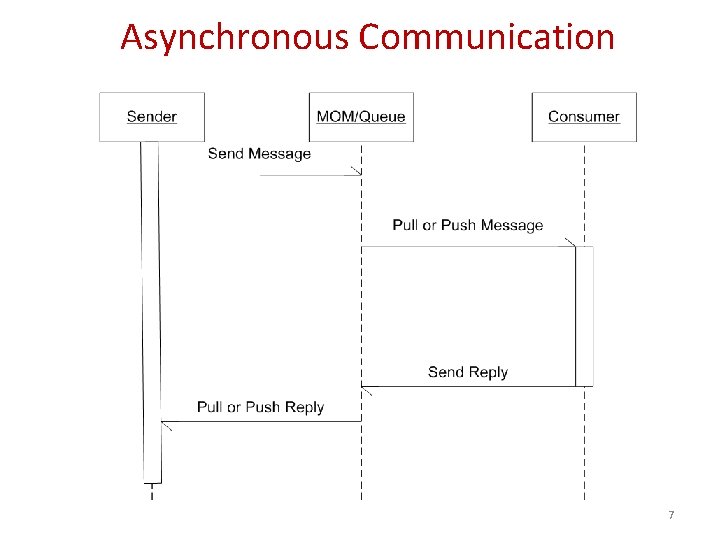
Asynchronous Communication 7
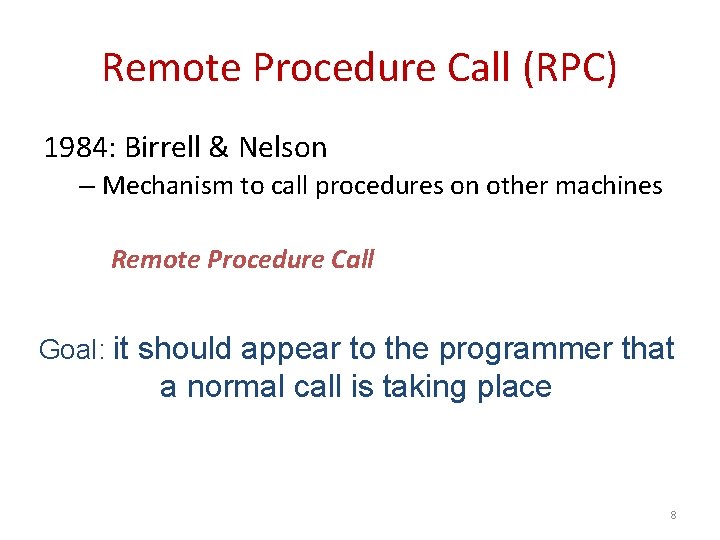
Remote Procedure Call (RPC) 1984: Birrell & Nelson – Mechanism to call procedures on other machines Remote Procedure Call Goal: it should appear to the programmer that a normal call is taking place 8
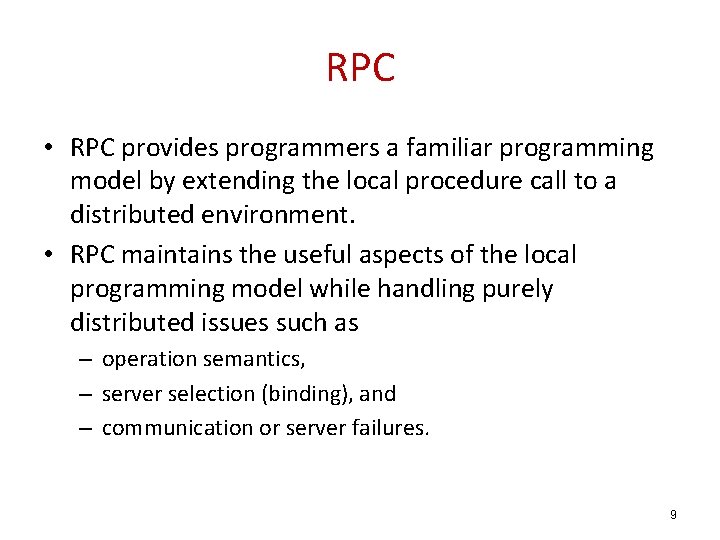
RPC • RPC provides programmers a familiar programming model by extending the local procedure call to a distributed environment. • RPC maintains the useful aspects of the local programming model while handling purely distributed issues such as – operation semantics, – server selection (binding), and – communication or server failures. 9
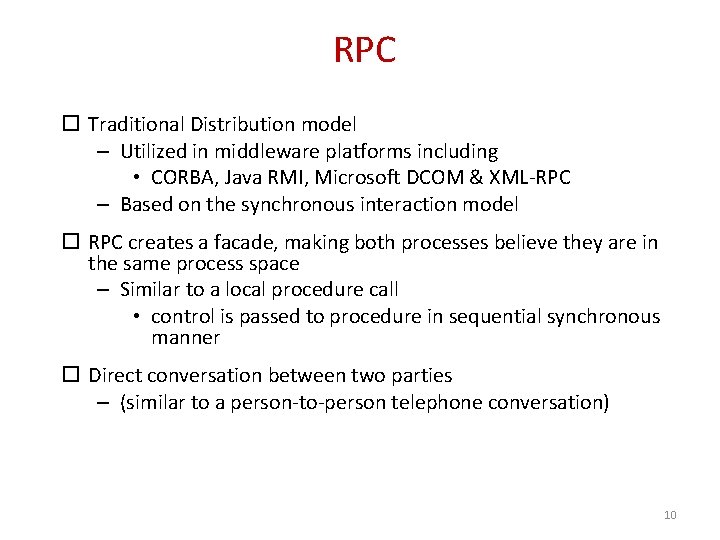
RPC o Traditional Distribution model – Utilized in middleware platforms including • CORBA, Java RMI, Microsoft DCOM & XML-RPC – Based on the synchronous interaction model o RPC creates a facade, making both processes believe they are in the same process space – Similar to a local procedure call • control is passed to procedure in sequential synchronous manner o Direct conversation between two parties – (similar to a person-to-person telephone conversation) 10
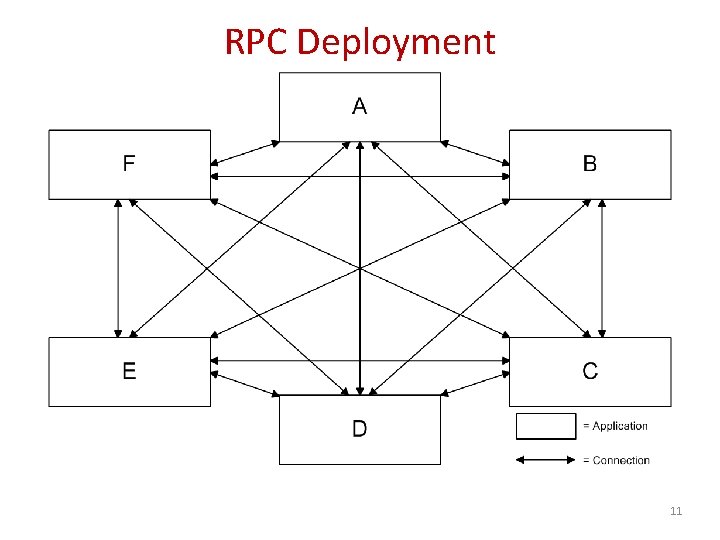
RPC Deployment 11
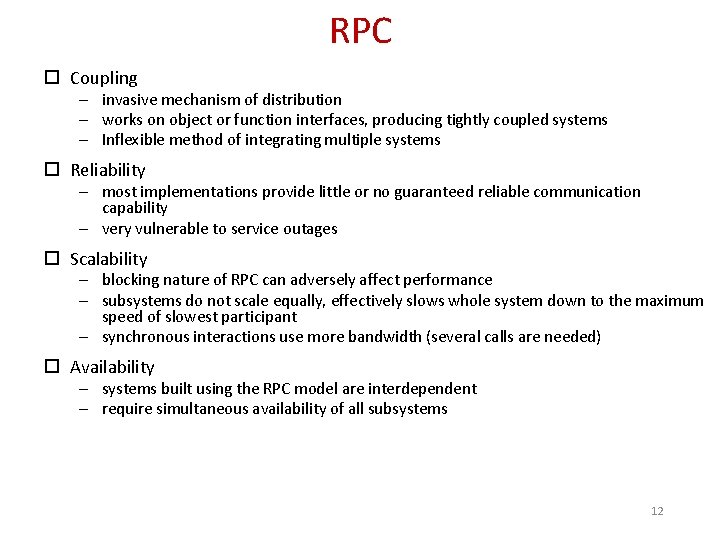
RPC o Coupling – invasive mechanism of distribution – works on object or function interfaces, producing tightly coupled systems – Inflexible method of integrating multiple systems o Reliability – most implementations provide little or no guaranteed reliable communication capability – very vulnerable to service outages o Scalability – blocking nature of RPC can adversely affect performance – subsystems do not scale equally, effectively slows whole system down to the maximum speed of slowest participant – synchronous interactions use more bandwidth (several calls are needed) o Availability – systems built using the RPC model are interdependent – require simultaneous availability of all subsystems 12
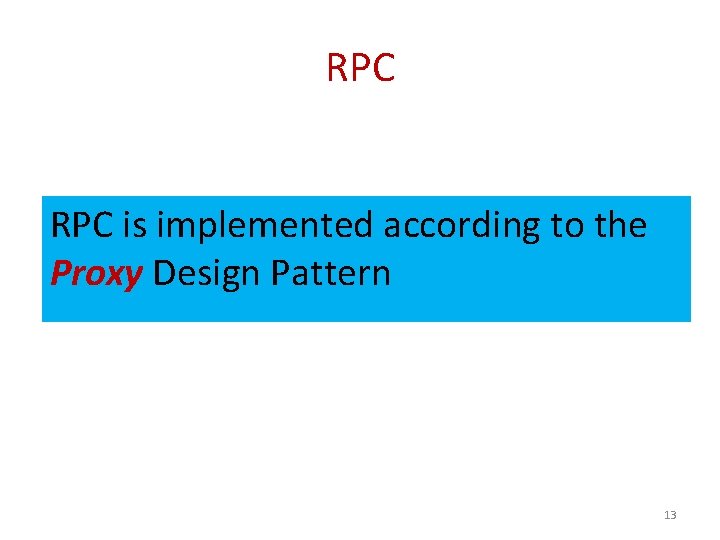
RPC is implemented according to the Proxy Design Pattern 13
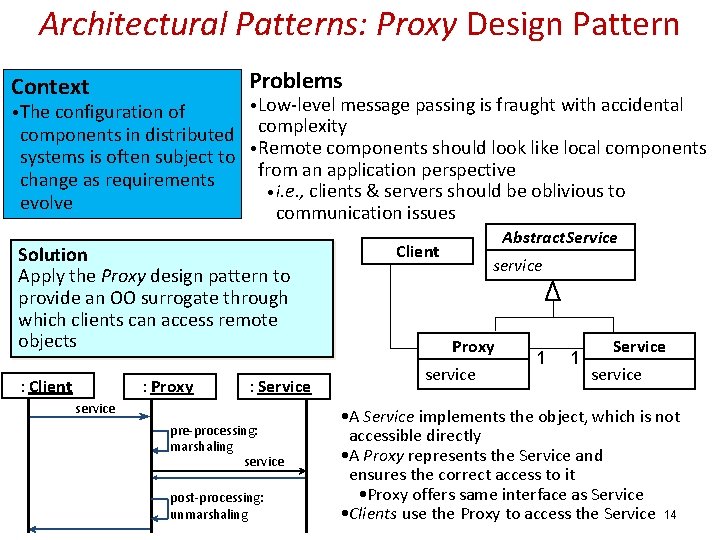
Architectural Patterns: Proxy Design Pattern Problems Context • The configuration of • Low-level message passing is fraught with accidental components in distributed complexity systems is often subject to • Remote components should look like local components from an application perspective change as requirements • i. e. , clients & servers should be oblivious to evolve communication issues Solution Apply the Proxy design pattern to provide an OO surrogate through which clients can access remote objects : Client : Proxy : Service service pre-processing: marshaling service post-processing: unmarshaling Abstract. Service Client service Proxy service 1 1 Service service • A Service implements the object, which is not accessible directly • A Proxy represents the Service and ensures the correct access to it • Proxy offers same interface as Service • Clients use the Proxy to access the Service 14
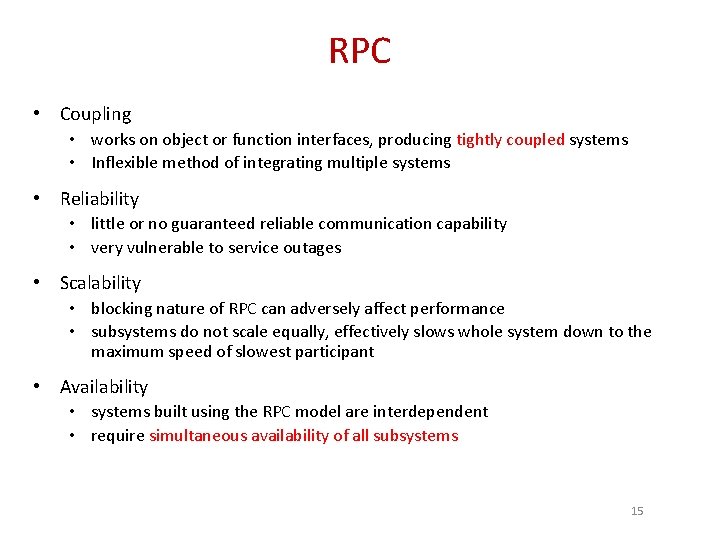
RPC • Coupling • works on object or function interfaces, producing tightly coupled systems • Inflexible method of integrating multiple systems • Reliability • little or no guaranteed reliable communication capability • very vulnerable to service outages • Scalability • blocking nature of RPC can adversely affect performance • subsystems do not scale equally, effectively slows whole system down to the maximum speed of slowest participant • Availability • systems built using the RPC model are interdependent • require simultaneous availability of all subsystems 15
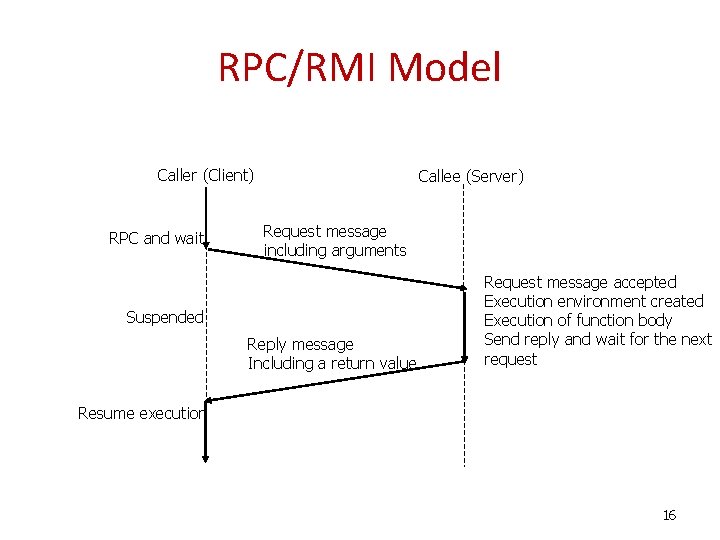
RPC/RMI Model Caller (Client) RPC and wait Callee (Server) Request message including arguments Suspended Reply message Including a return value Request message accepted Execution environment created Execution of function body Send reply and wait for the next request Resume execution 16
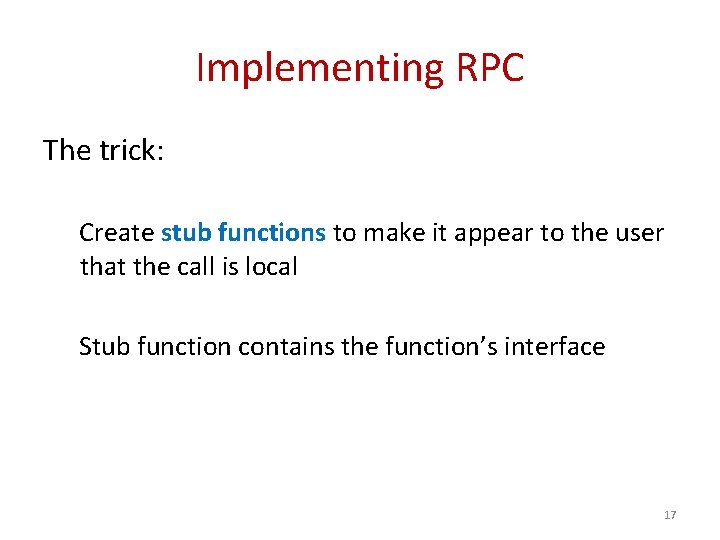
Implementing RPC The trick: Create stub functions to make it appear to the user that the call is local Stub function contains the function’s interface 17
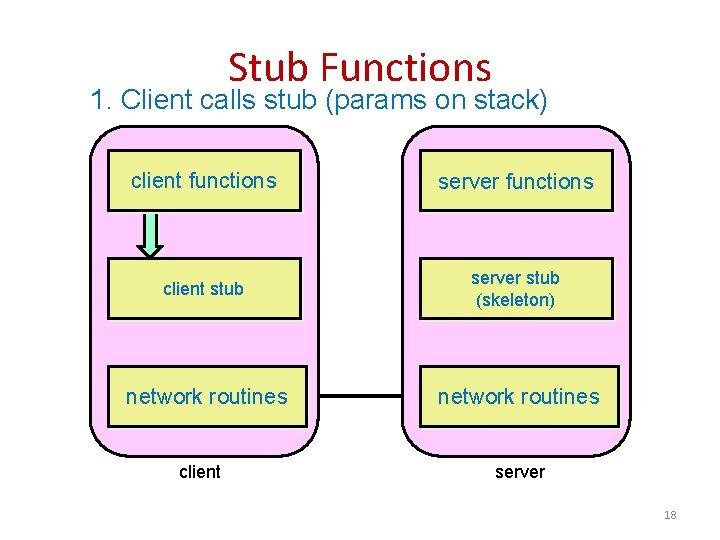
Stub Functions 1. Client calls stub (params on stack) client functions server functions client stub server stub (skeleton) network routines client server 18
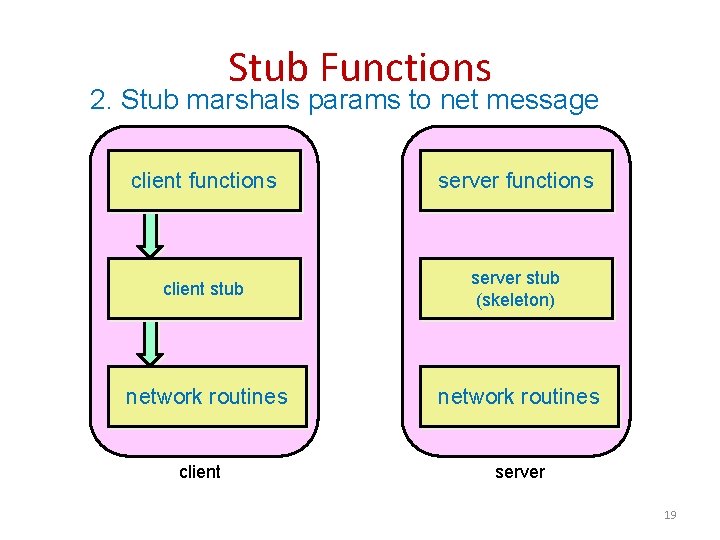
Stub Functions 2. Stub marshals params to net message client functions server functions client stub server stub (skeleton) network routines client server 19
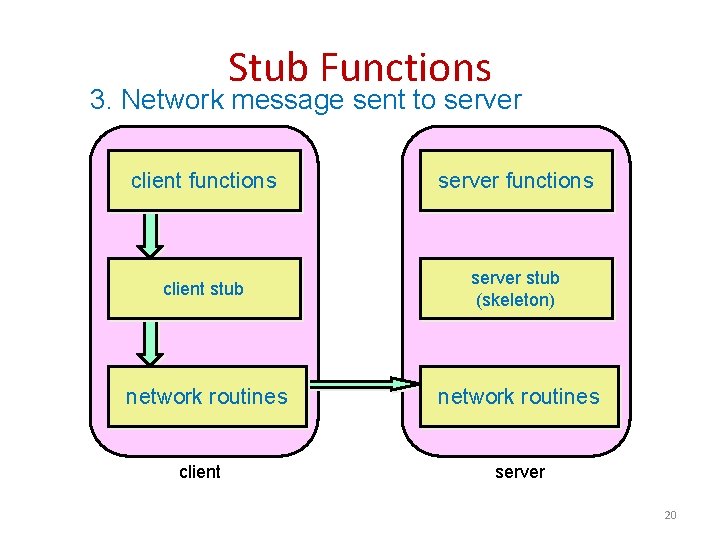
Stub Functions 3. Network message sent to server client functions server functions client stub server stub (skeleton) network routines client server 20
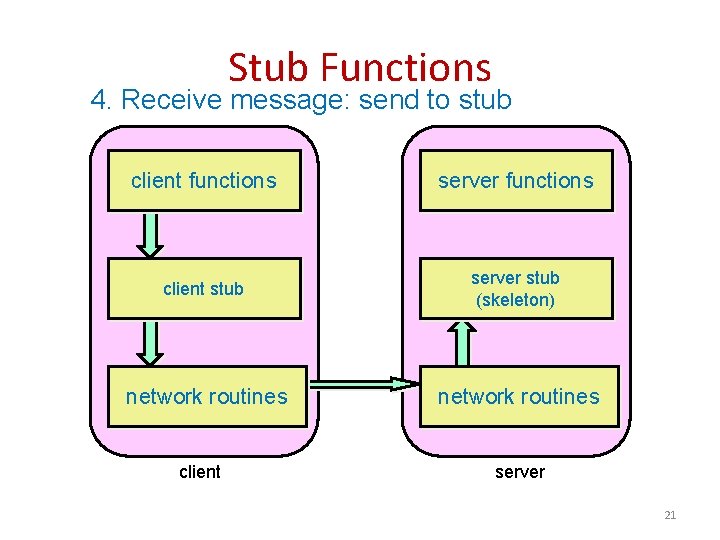
Stub Functions 4. Receive message: send to stub client functions server functions client stub server stub (skeleton) network routines client server 21
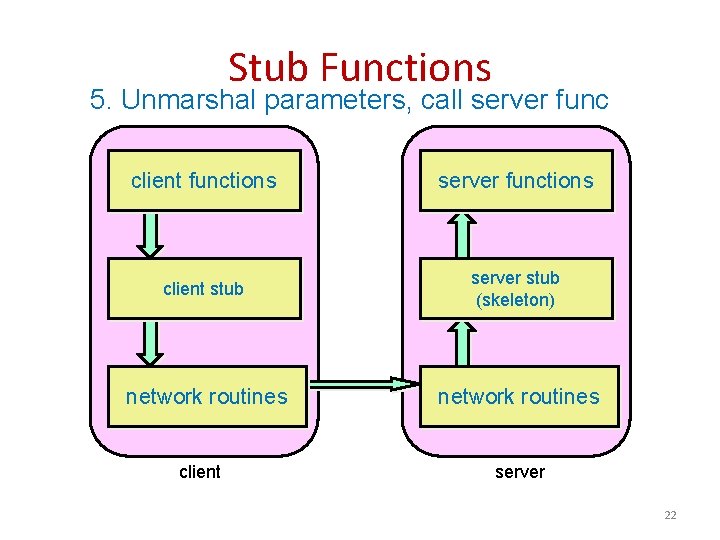
Stub Functions 5. Unmarshal parameters, call server func client functions server functions client stub server stub (skeleton) network routines client server 22
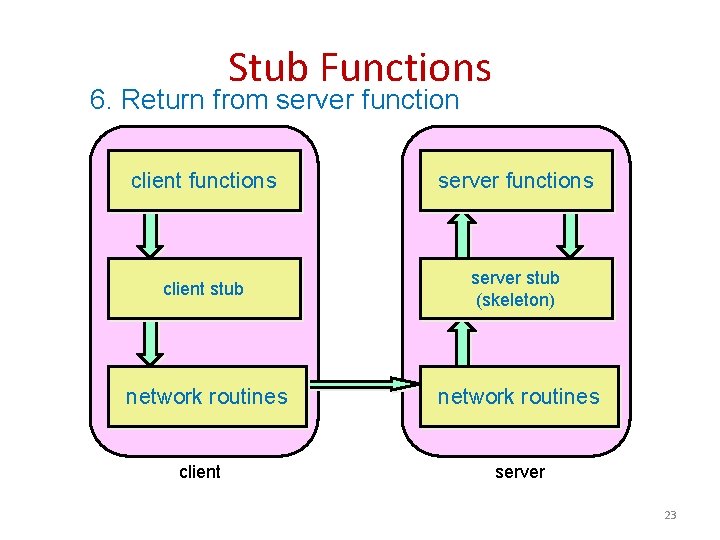
Stub Functions 6. Return from server function client functions server functions client stub server stub (skeleton) network routines client server 23
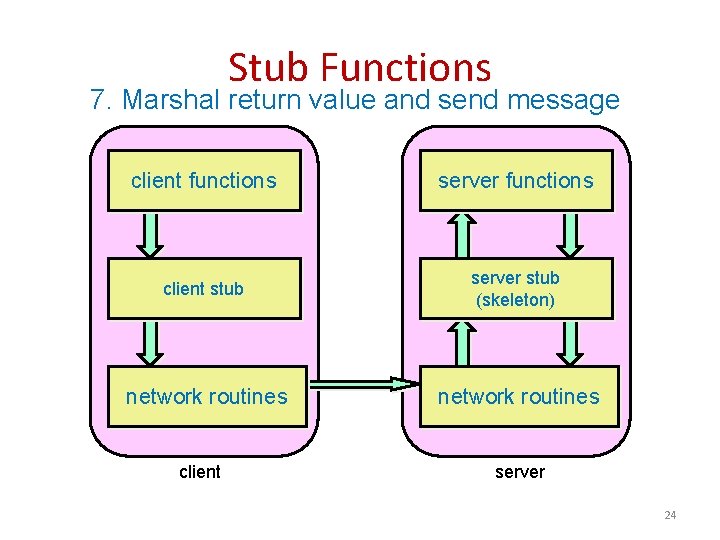
Stub Functions 7. Marshal return value and send message client functions server functions client stub server stub (skeleton) network routines client server 24
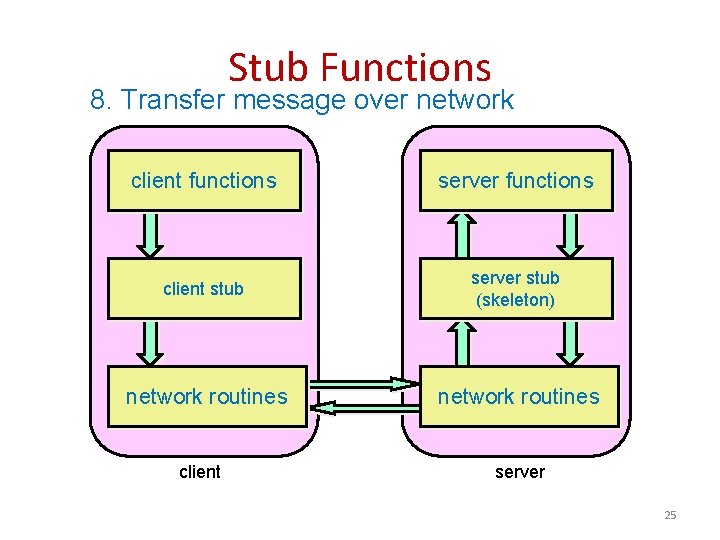
Stub Functions 8. Transfer message over network client functions server functions client stub server stub (skeleton) network routines client server 25
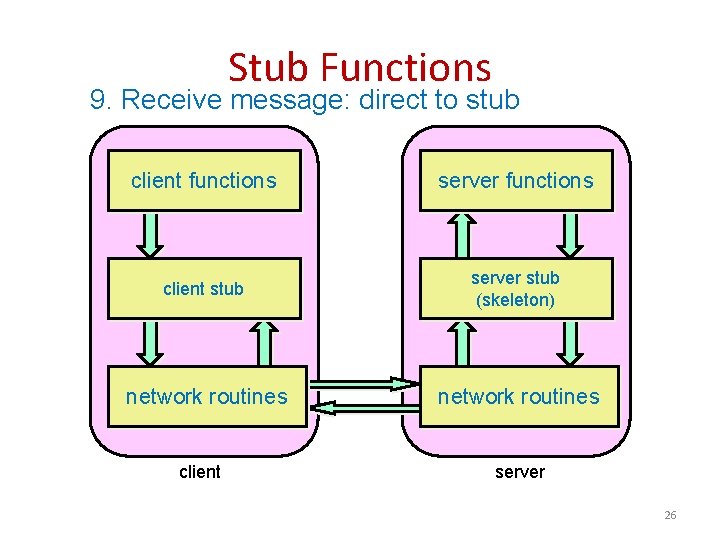
Stub Functions 9. Receive message: direct to stub client functions server functions client stub server stub (skeleton) network routines client server 26
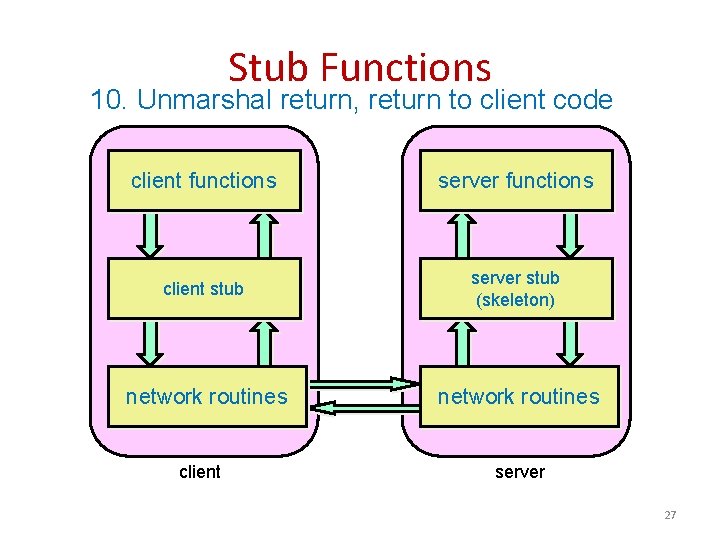
Stub Functions 10. Unmarshal return, return to client code client functions server functions client stub server stub (skeleton) network routines client server 27
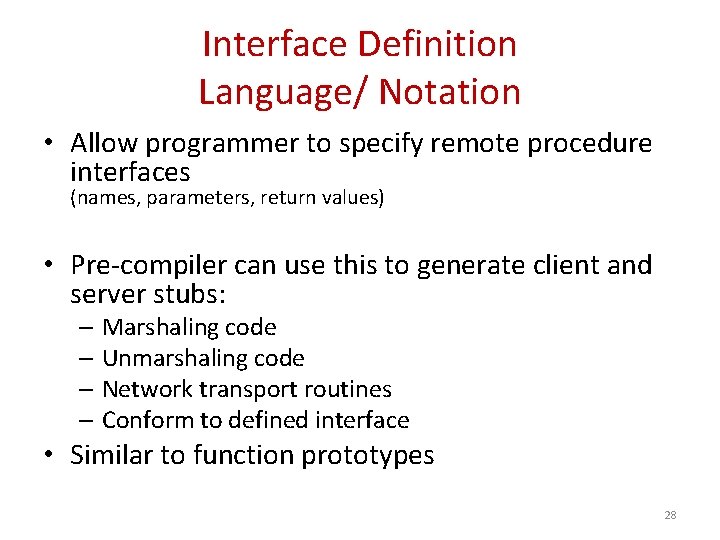
Interface Definition Language/ Notation • Allow programmer to specify remote procedure interfaces (names, parameters, return values) • Pre-compiler can use this to generate client and server stubs: – Marshaling code – Unmarshaling code – Network transport routines – Conform to defined interface • Similar to function prototypes 28
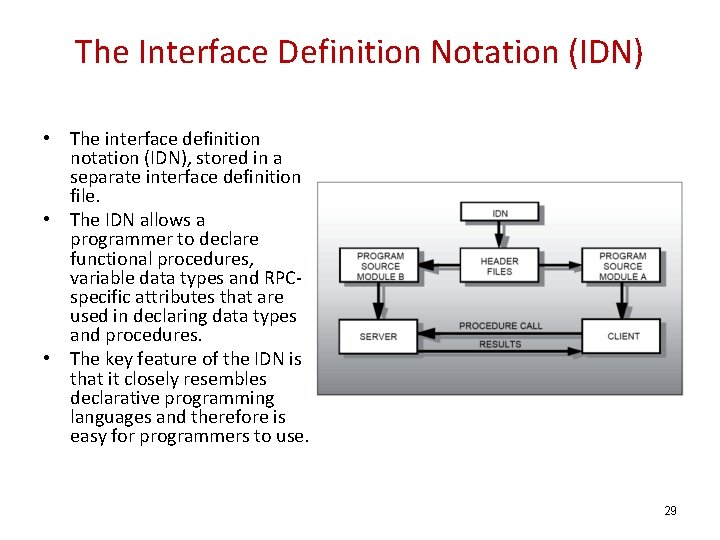
The Interface Definition Notation (IDN) • The interface definition notation (IDN), stored in a separate interface definition file. • The IDN allows a programmer to declare functional procedures, variable data types and RPCspecific attributes that are used in declaring data types and procedures. • The key feature of the IDN is that it closely resembles declarative programming languages and therefore is easy for programmers to use. 29
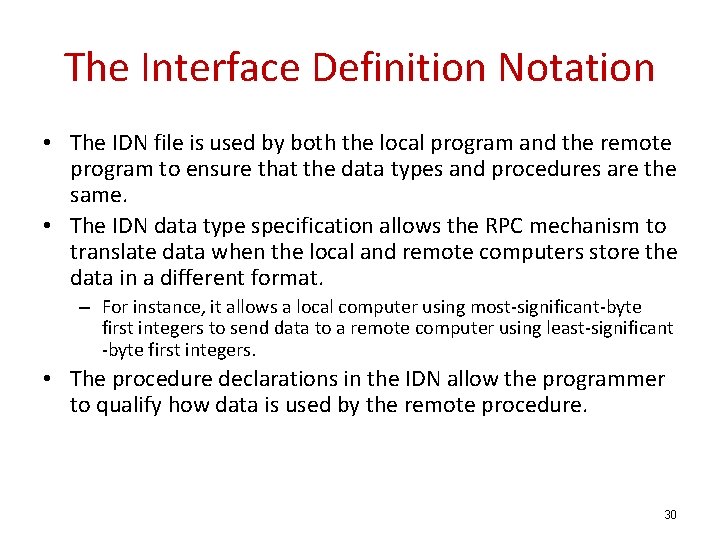
The Interface Definition Notation • The IDN file is used by both the local program and the remote program to ensure that the data types and procedures are the same. • The IDN data type specification allows the RPC mechanism to translate data when the local and remote computers store the data in a different format. – For instance, it allows a local computer using most-significant-byte first integers to send data to a remote computer using least-significant -byte first integers. • The procedure declarations in the IDN allow the programmer to qualify how data is used by the remote procedure. 30
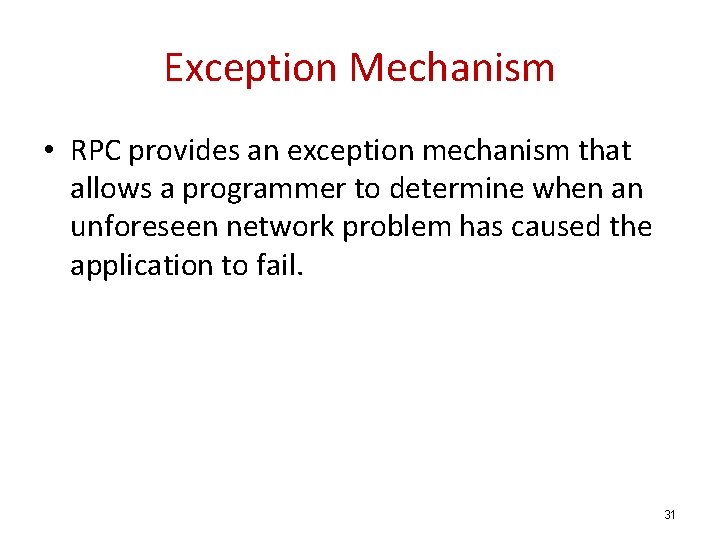
Exception Mechanism • RPC provides an exception mechanism that allows a programmer to determine when an unforeseen network problem has caused the application to fail. 31
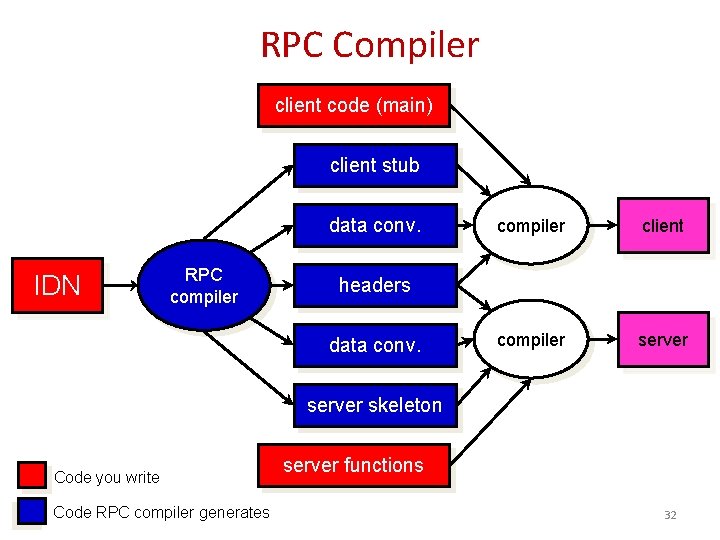
RPC Compiler client code (main) client stub data conv. IDN RPC compiler client compiler server headers data conv. server skeleton Code you write Code RPC compiler generates server functions 32
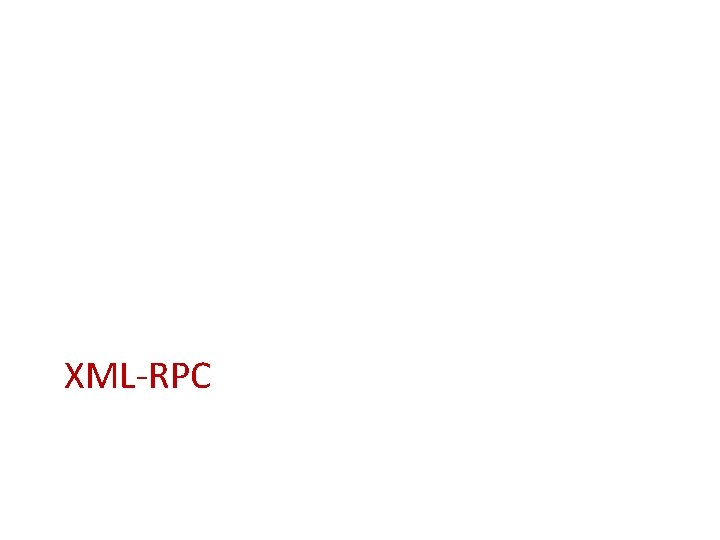
XML-RPC
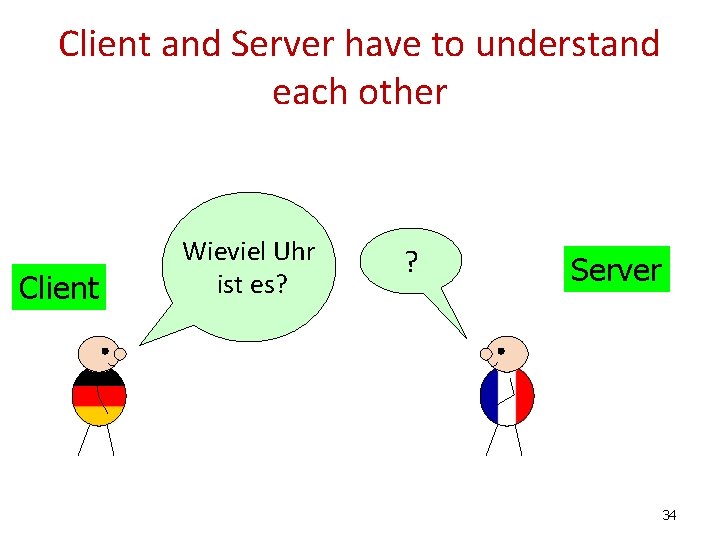
Client and Server have to understand each other Client Wieviel Uhr ist es? ? Server 34
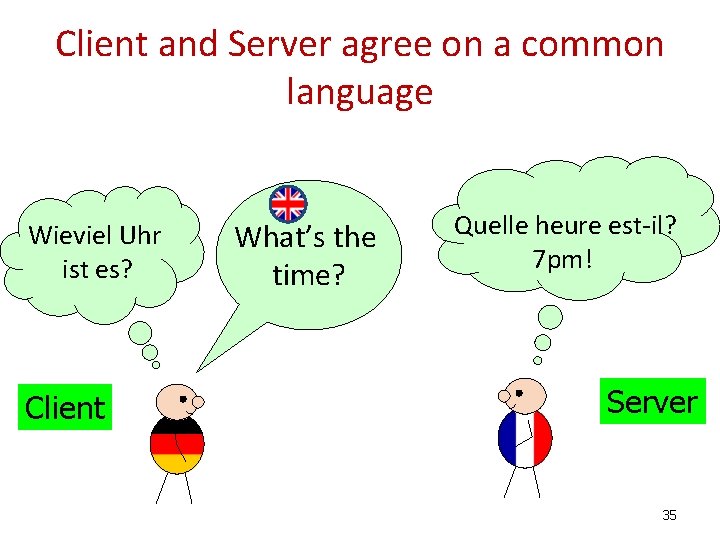
Client and Server agree on a common language Wieviel Uhr ist es? Client What’s the time? Quelle heure est-il? 7 pm! Server 35
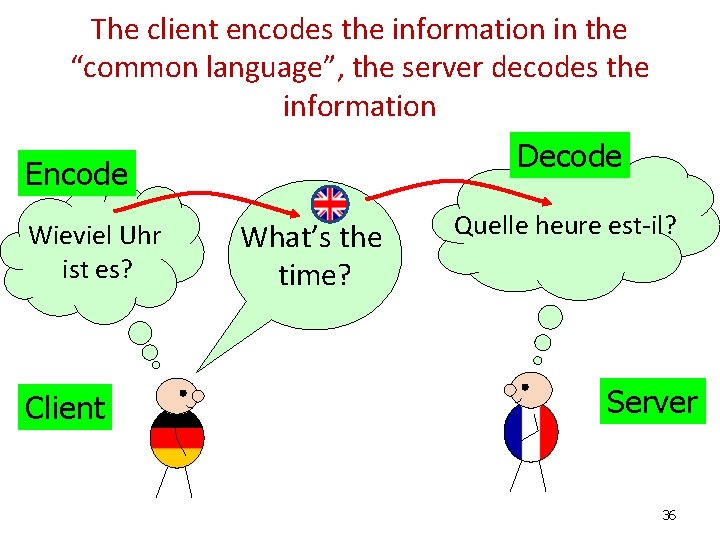
The client encodes the information in the “common language”, the server decodes the information Decode Encode Wieviel Uhr ist es? Client What’s the time? Quelle heure est-il? Server 36
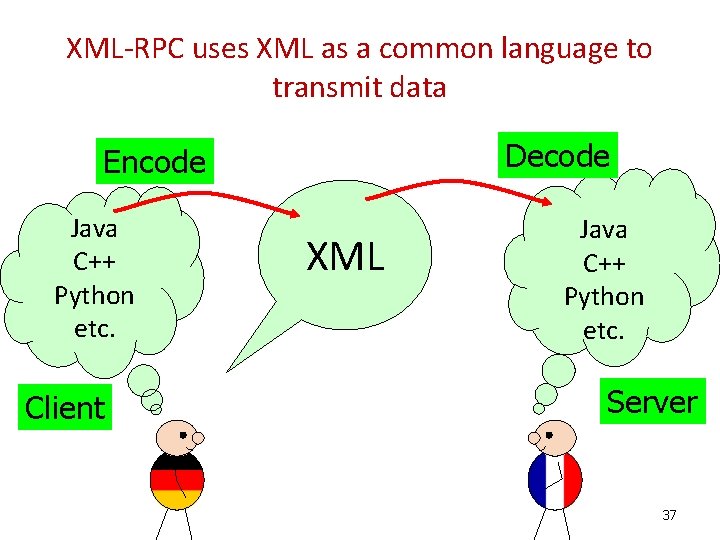
XML-RPC uses XML as a common language to transmit data Decode Encode Java C++ Python etc. Client XML Java C++ Python etc. Server 37
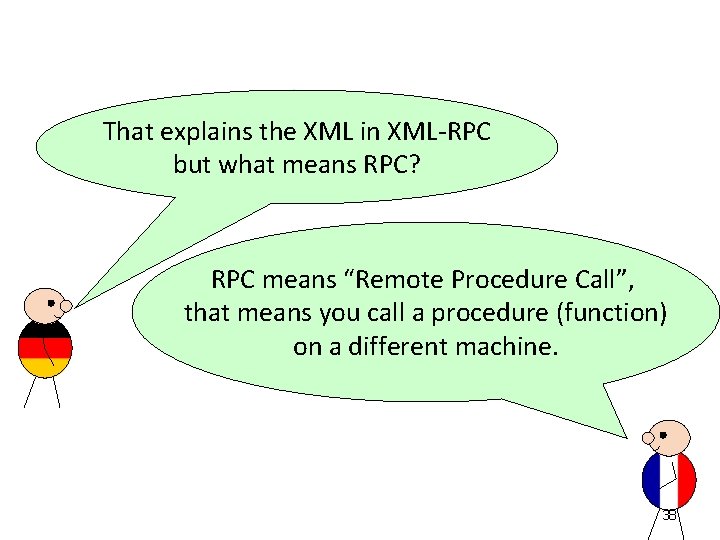
That explains the XML in XML-RPC but what means RPC? RPC means “Remote Procedure Call”, that means you call a procedure (function) on a different machine. 38
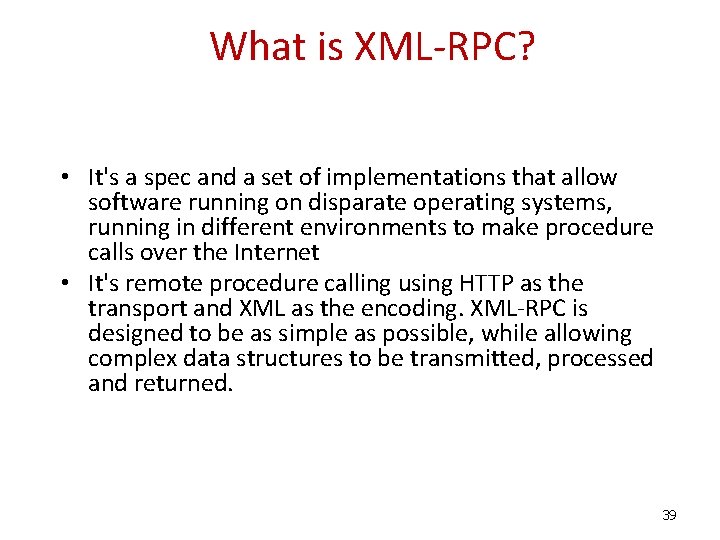
What is XML-RPC? • It's a spec and a set of implementations that allow software running on disparate operating systems, running in different environments to make procedure calls over the Internet • It's remote procedure calling using HTTP as the transport and XML as the encoding. XML-RPC is designed to be as simple as possible, while allowing complex data structures to be transmitted, processed and returned. 39
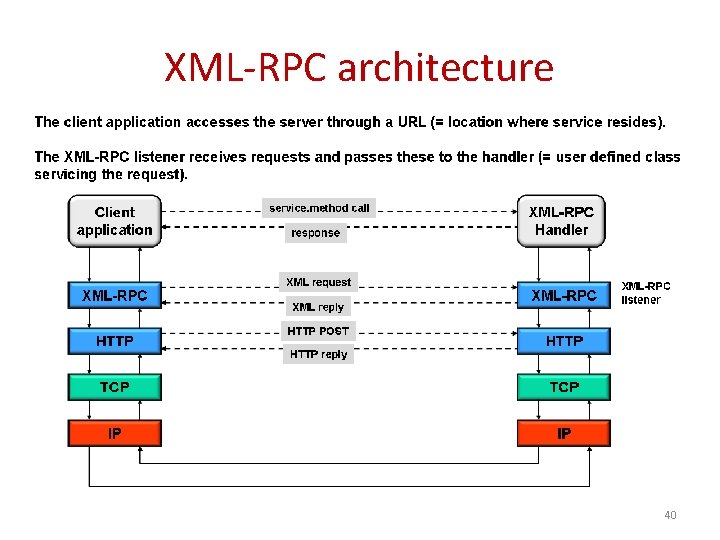
XML-RPC architecture 40
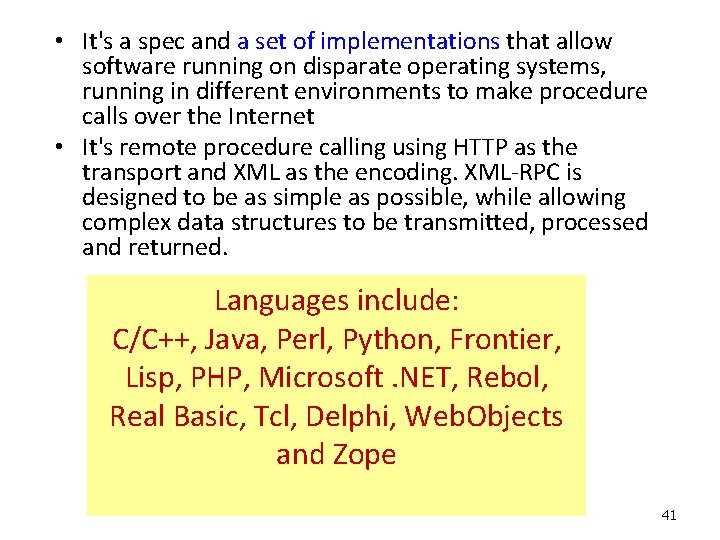
• It's a spec and a set of implementations that allow software running on disparate operating systems, running in different environments to make procedure calls over the Internet • It's remote procedure calling using HTTP as the transport and XML as the encoding. XML-RPC is designed to be as simple as possible, while allowing complex data structures to be transmitted, processed and returned. Languages include: C/C++, Java, Perl, Python, Frontier, Lisp, PHP, Microsoft. NET, Rebol, Real Basic, Tcl, Delphi, Web. Objects and Zope 41