System Calls and IO 1 System Calls versus
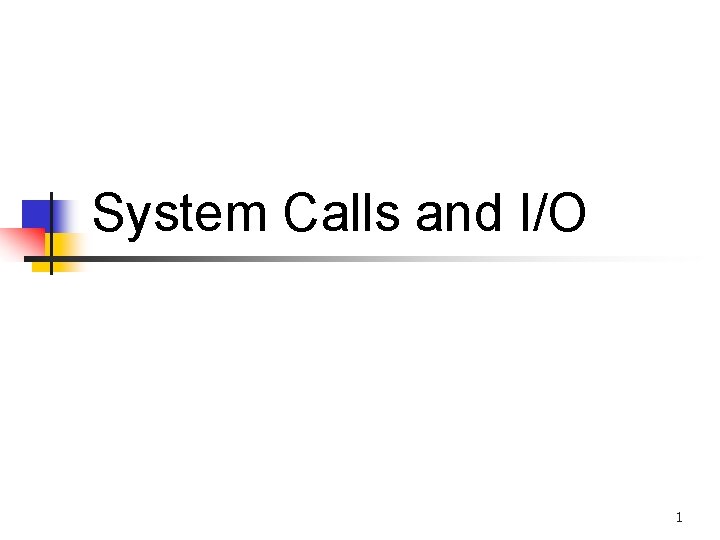
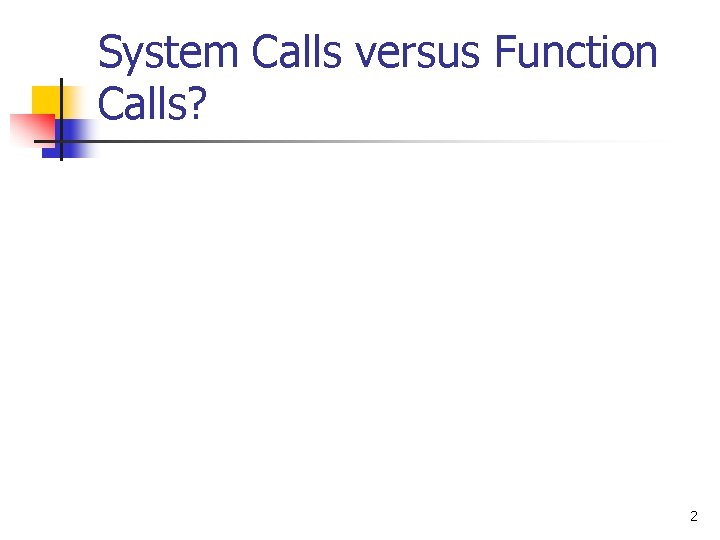
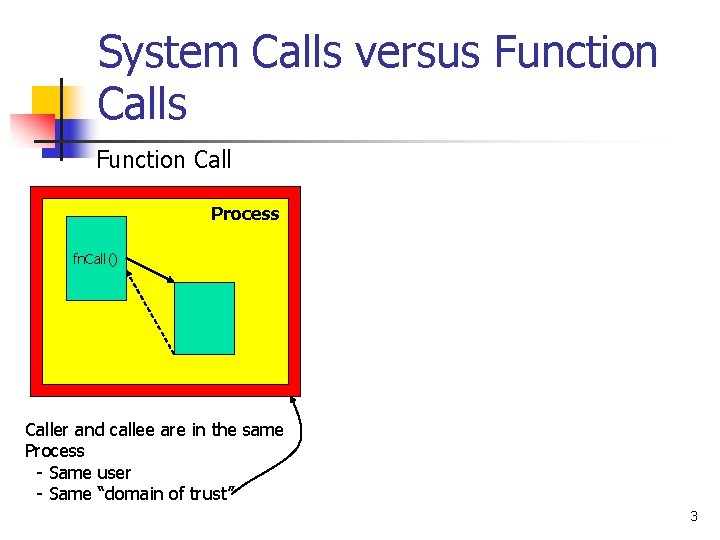
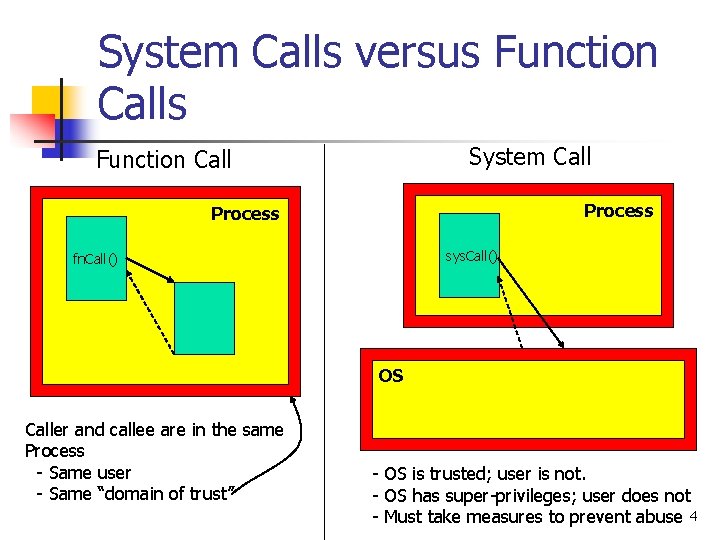
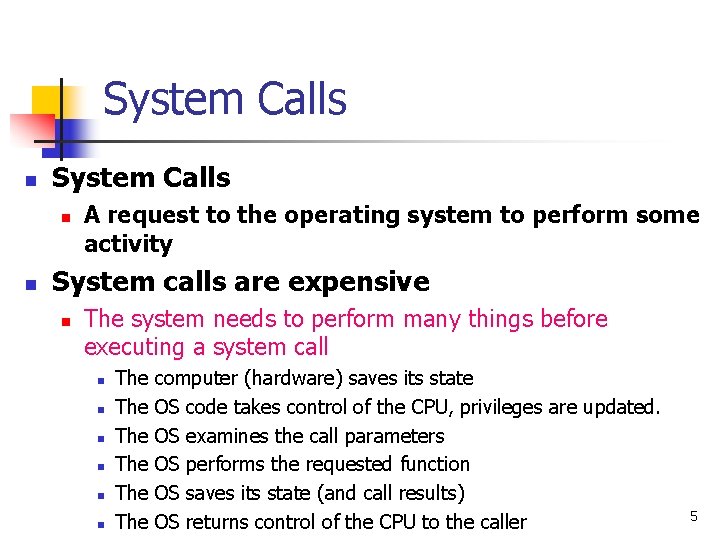
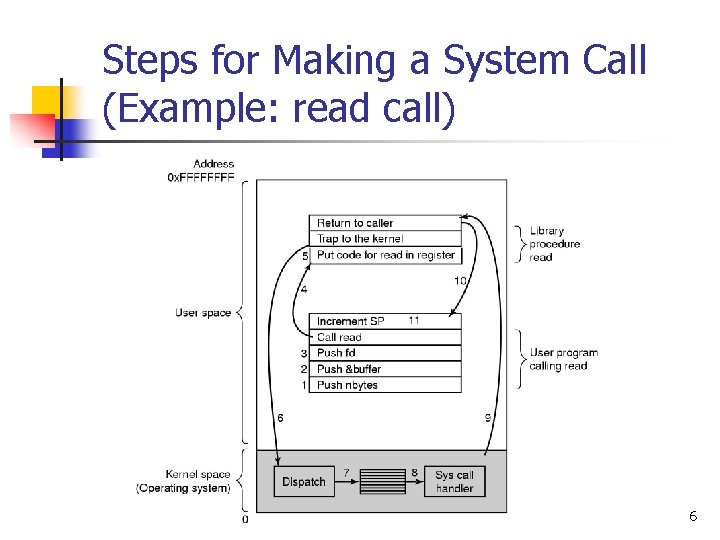
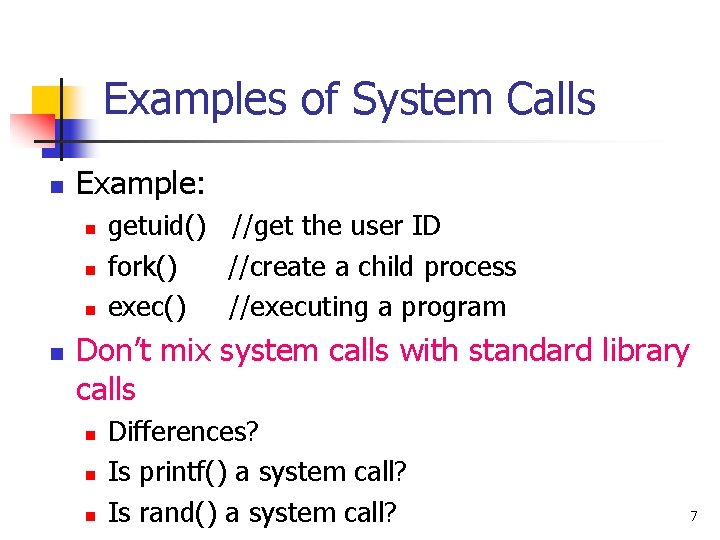
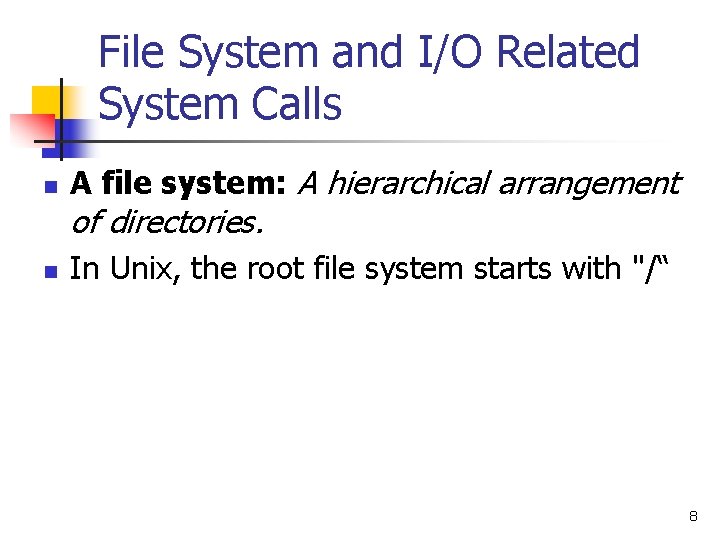
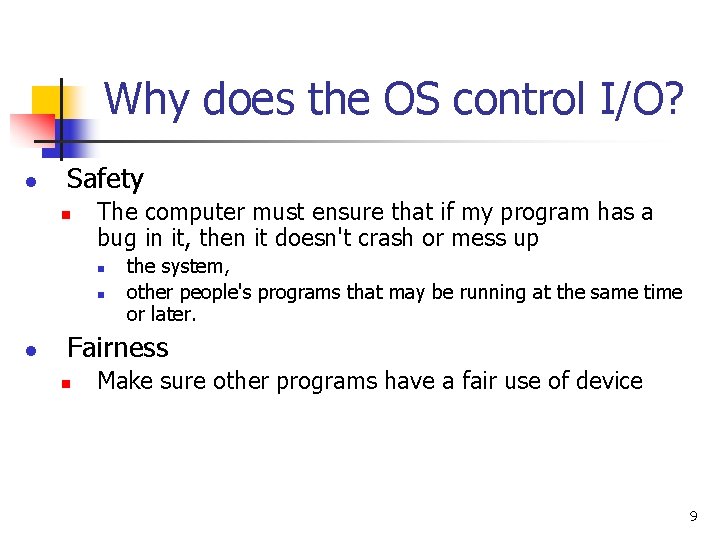
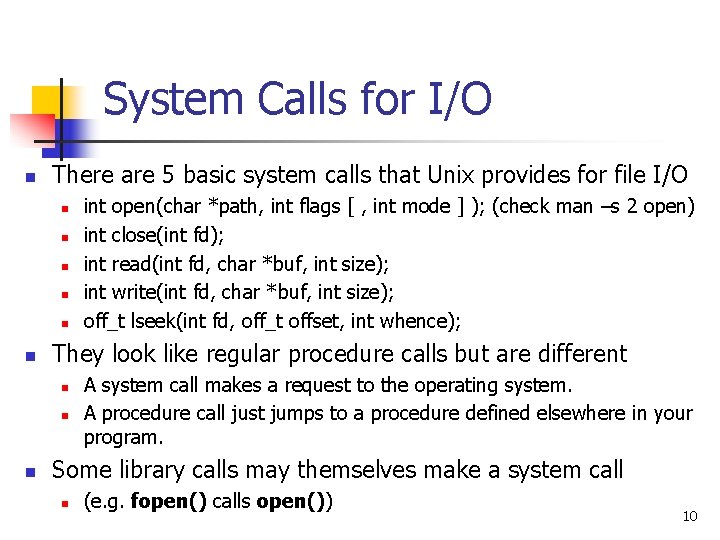
![Open n int open(char *path, int flags [ , int mode ] ) makes Open n int open(char *path, int flags [ , int mode ] ) makes](https://slidetodoc.com/presentation_image/b4f0cd50c521e1975e0b8d1b37f3e525/image-11.jpg)
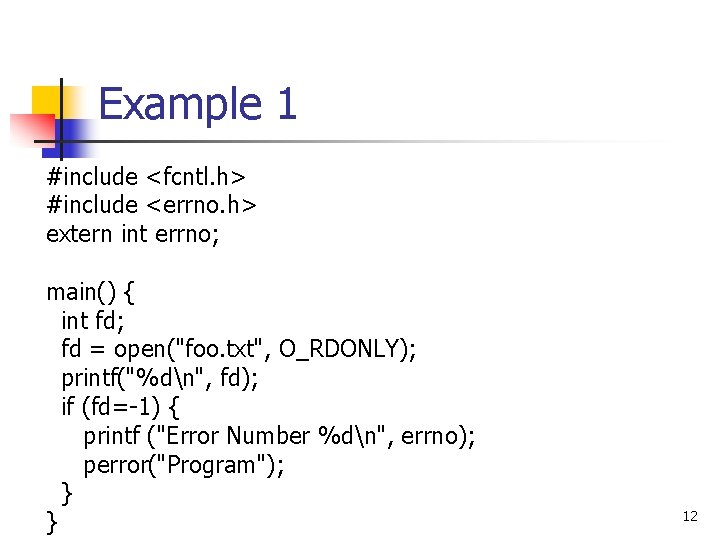
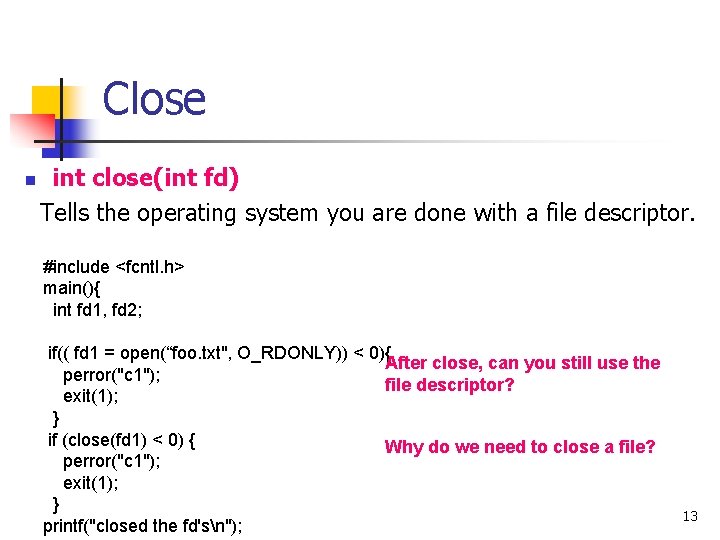
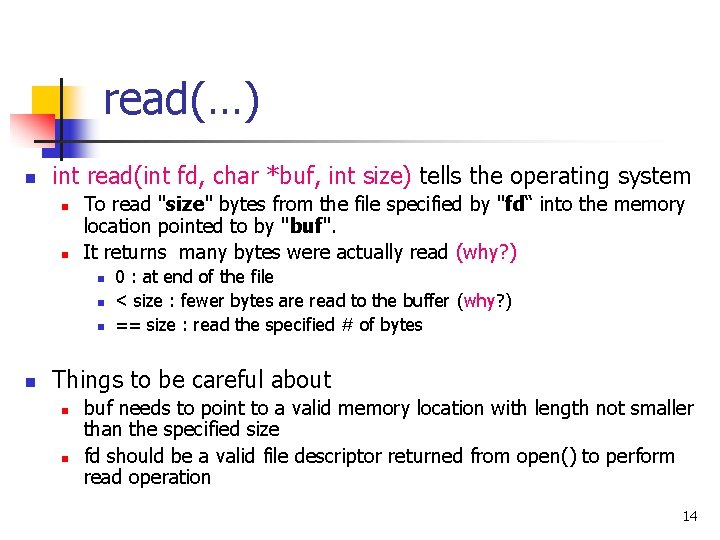
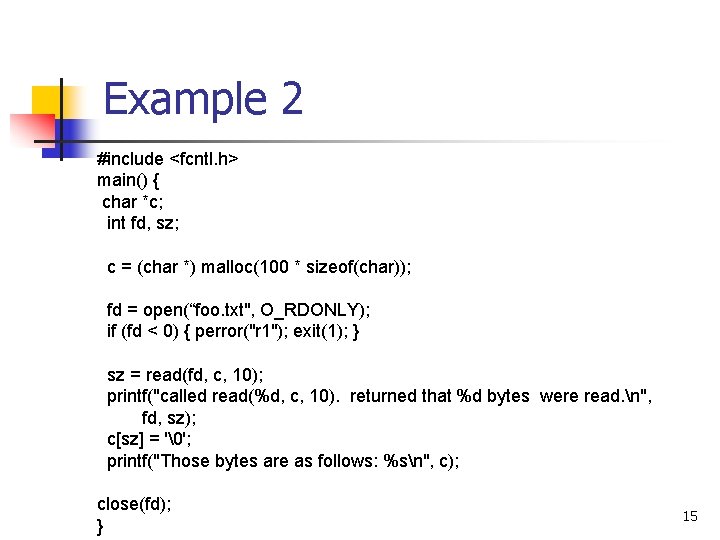
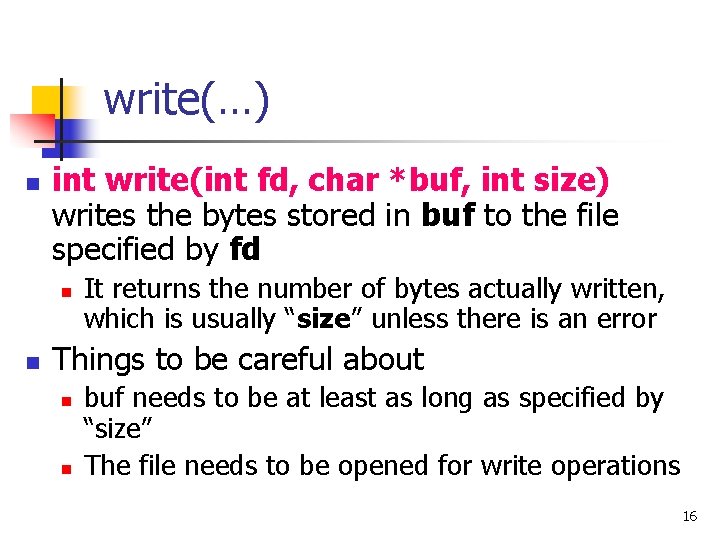
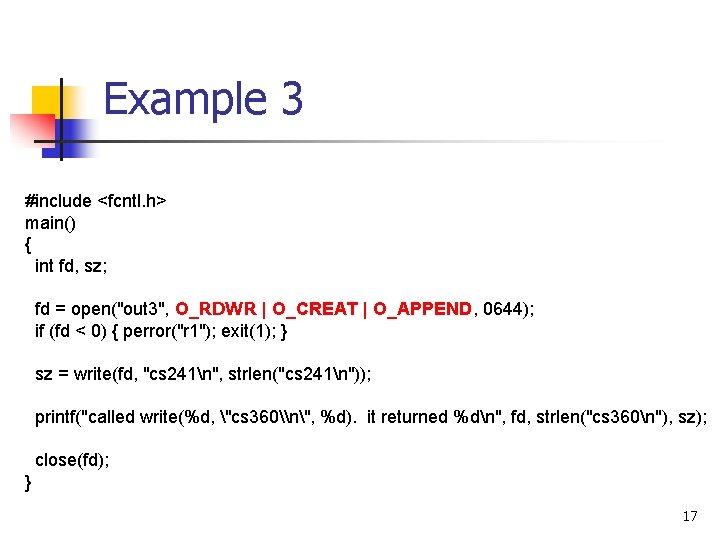
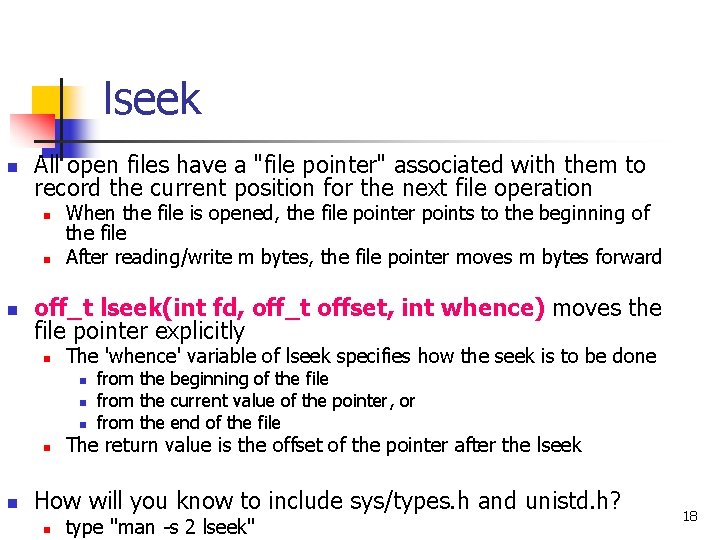
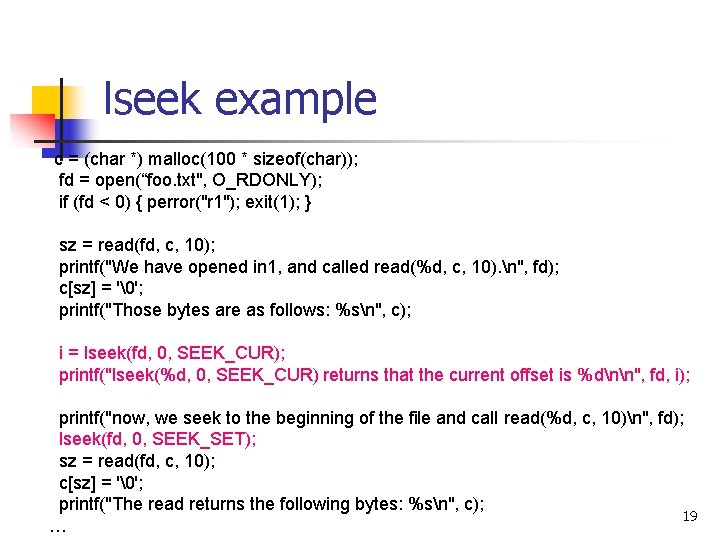
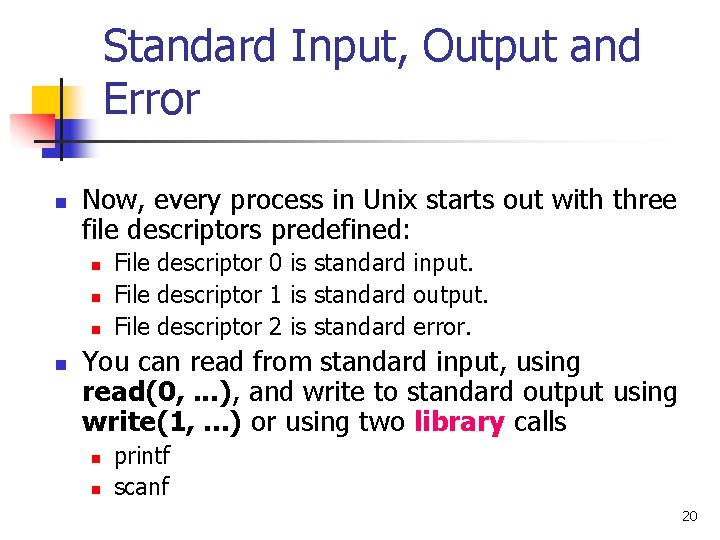
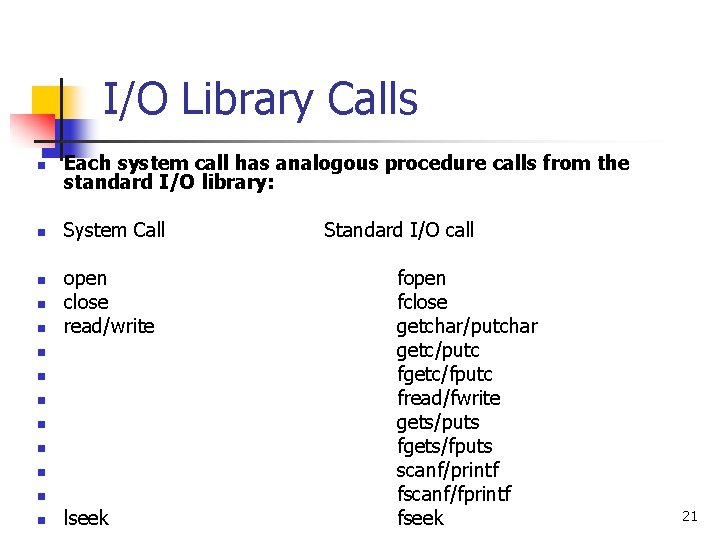
- Slides: 21
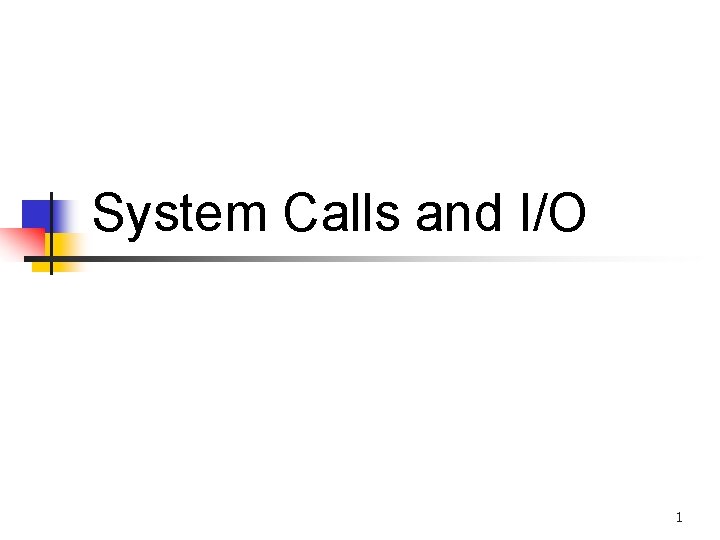
System Calls and I/O 1
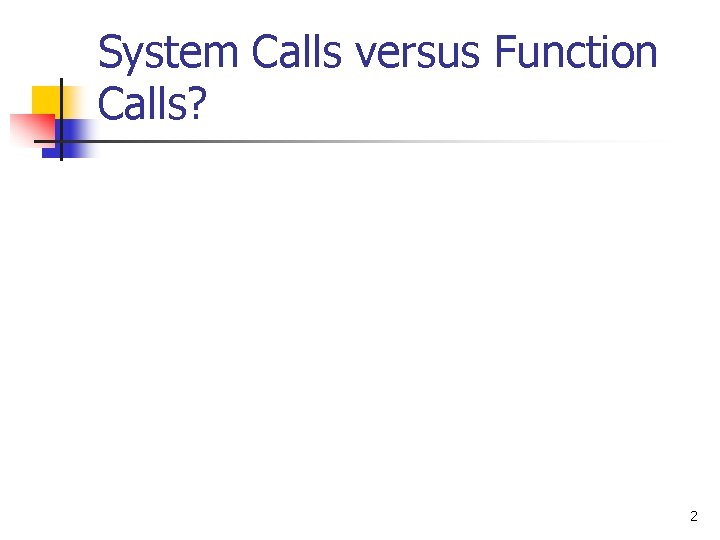
System Calls versus Function Calls? 2
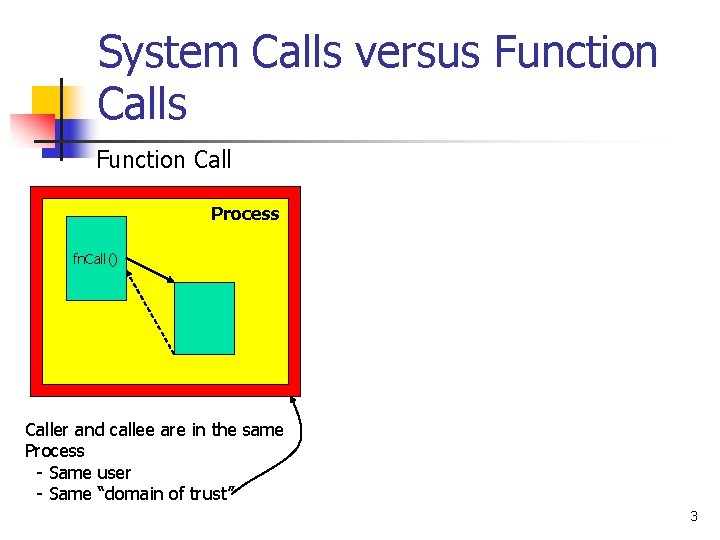
System Calls versus Function Call Process fn. Call() Caller and callee are in the same Process - Same user - Same “domain of trust” 3
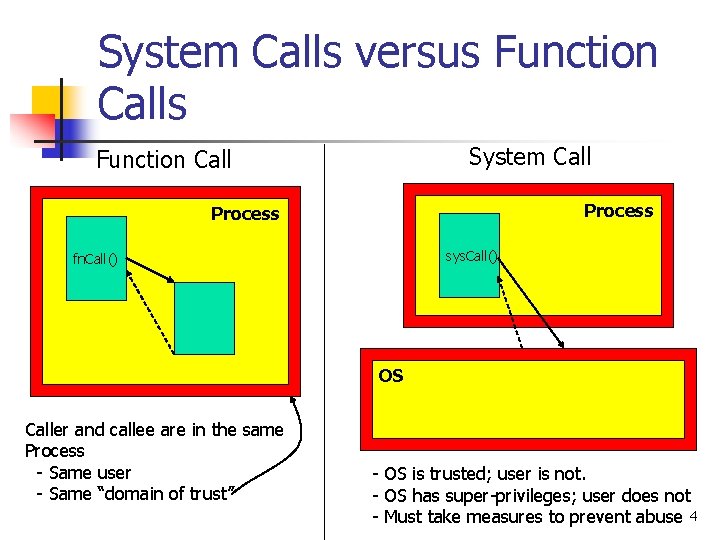
System Calls versus Function Calls System Call Function Call Process sys. Call() fn. Call() OS Caller and callee are in the same Process - Same user - Same “domain of trust” - OS is trusted; user is not. - OS has super-privileges; user does not - Must take measures to prevent abuse 4
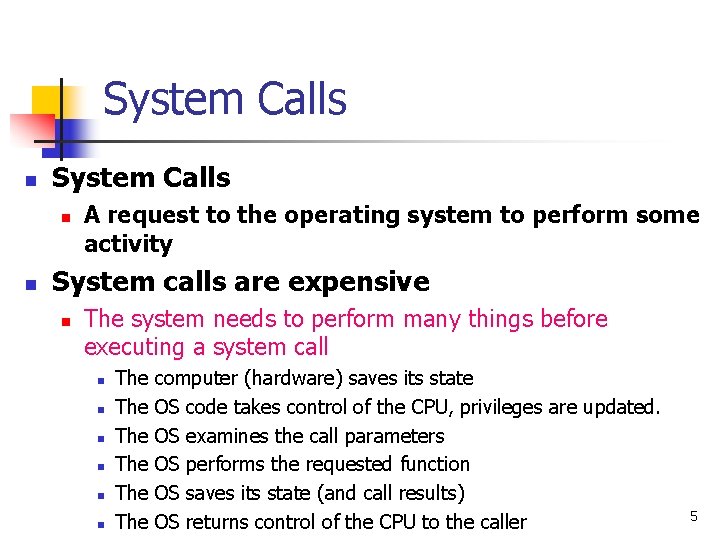
System Calls n n A request to the operating system to perform some activity System calls are expensive n The system needs to perform many things before executing a system call n n n The The The computer (hardware) saves its state OS code takes control of the CPU, privileges are updated. OS examines the call parameters OS performs the requested function OS saves its state (and call results) OS returns control of the CPU to the caller 5
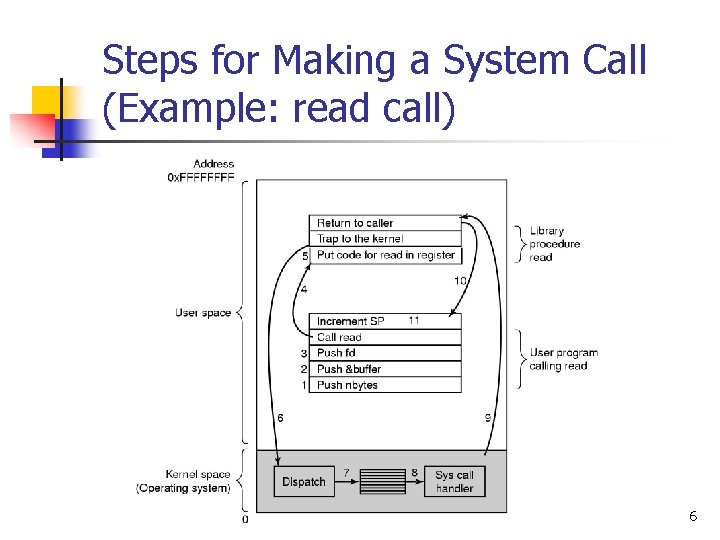
Steps for Making a System Call (Example: read call) 6
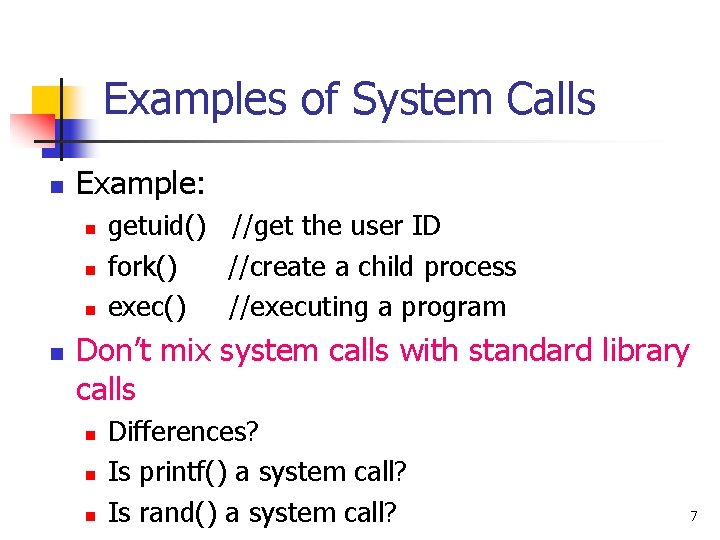
Examples of System Calls n Example: n n getuid() //get the user ID fork() //create a child process exec() //executing a program Don’t mix system calls with standard library calls n n n Differences? Is printf() a system call? Is rand() a system call? 7
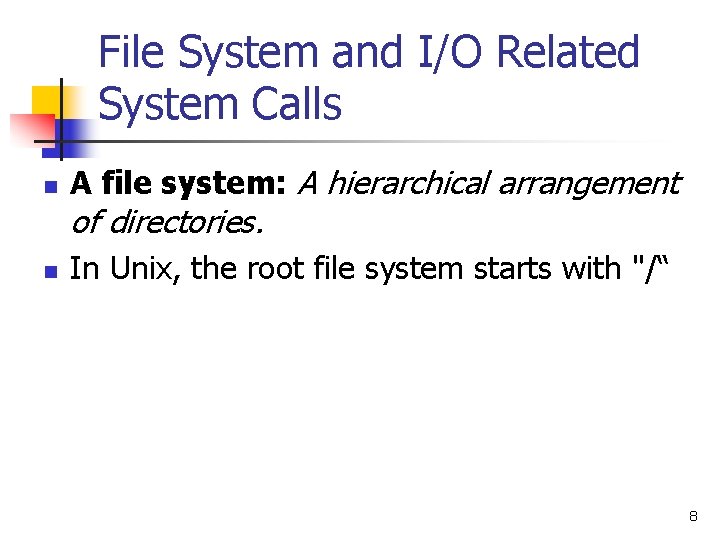
File System and I/O Related System Calls n A file system: A hierarchical arrangement of directories. n In Unix, the root file system starts with "/“ 8
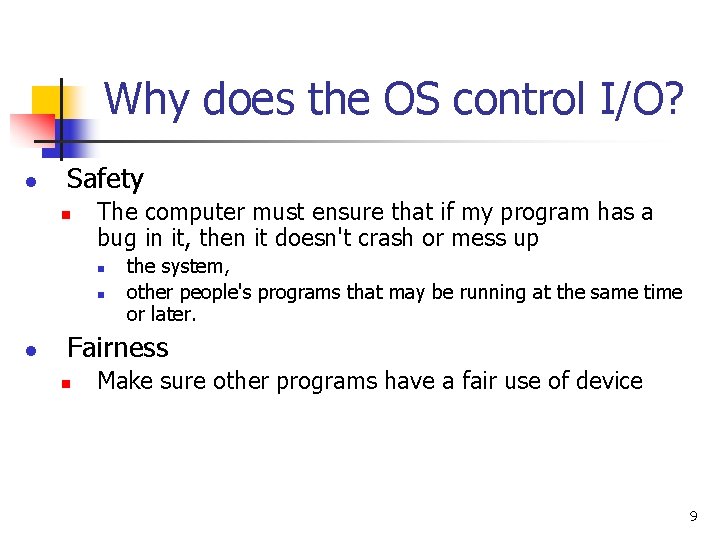
Why does the OS control I/O? l Safety n The computer must ensure that if my program has a bug in it, then it doesn't crash or mess up n n l the system, other people's programs that may be running at the same time or later. Fairness n Make sure other programs have a fair use of device 9
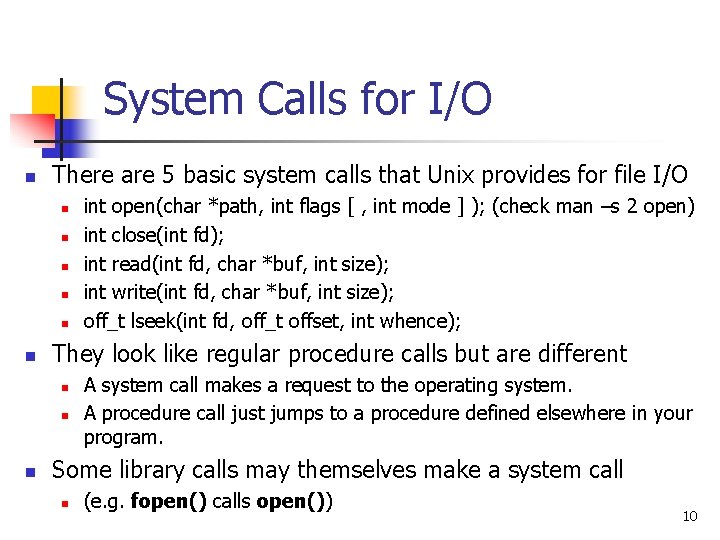
System Calls for I/O n There are 5 basic system calls that Unix provides for file I/O n n n They look like regular procedure calls but are different n n n int open(char *path, int flags [ , int mode ] ); (check man –s 2 open) int close(int fd); int read(int fd, char *buf, int size); int write(int fd, char *buf, int size); off_t lseek(int fd, off_t offset, int whence); A system call makes a request to the operating system. A procedure call just jumps to a procedure defined elsewhere in your program. Some library calls may themselves make a system call n (e. g. fopen() calls open()) 10
![Open n int openchar path int flags int mode makes Open n int open(char *path, int flags [ , int mode ] ) makes](https://slidetodoc.com/presentation_image/b4f0cd50c521e1975e0b8d1b37f3e525/image-11.jpg)
Open n int open(char *path, int flags [ , int mode ] ) makes a request to the operating system to use a file. n n The 'path' argument specifies the file you would like to use The 'flags' and 'mode' arguments specify how you would like to use it. If the operating system approves your request, it will return a file descriptor to you. This is a non-negative integer. Any future accesses to this file needs to provide this file descriptor If it returns -1, then you have been denied access, and check the value of the variable "errno" to determine why (use perror()). 11
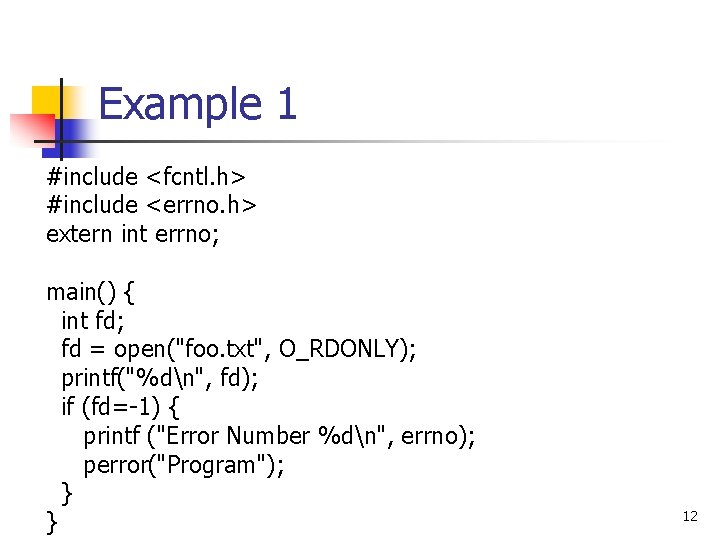
Example 1 #include <fcntl. h> #include <errno. h> extern int errno; main() { int fd; fd = open("foo. txt", O_RDONLY); printf("%dn", fd); if (fd=-1) { printf ("Error Number %dn", errno); perror("Program"); } } 12
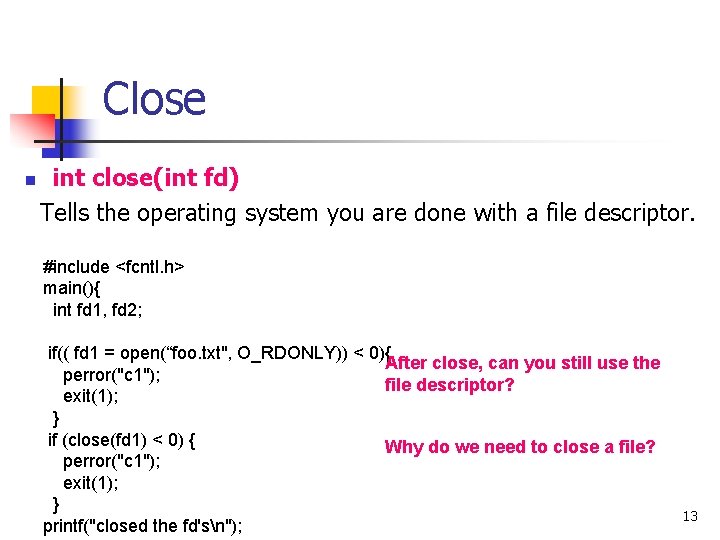
Close n int close(int fd) Tells the operating system you are done with a file descriptor. #include <fcntl. h> main(){ int fd 1, fd 2; if(( fd 1 = open(“foo. txt", O_RDONLY)) < 0){ After close, can you still use the perror("c 1"); file descriptor? exit(1); } if (close(fd 1) < 0) { Why do we need to close a file? perror("c 1"); exit(1); } printf("closed the fd'sn"); 13
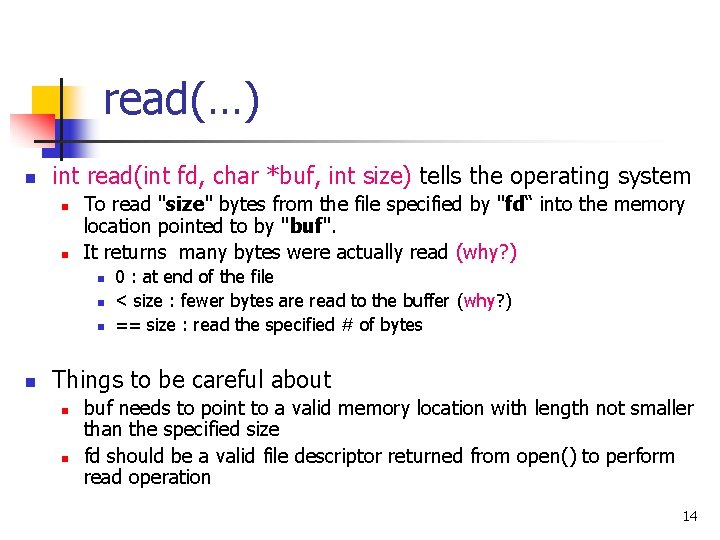
read(…) n int read(int fd, char *buf, int size) tells the operating system n n To read "size" bytes from the file specified by "fd“ into the memory location pointed to by "buf". It returns many bytes were actually read (why? ) n n 0 : at end of the file < size : fewer bytes are read to the buffer (why? ) == size : read the specified # of bytes Things to be careful about n n buf needs to point to a valid memory location with length not smaller than the specified size fd should be a valid file descriptor returned from open() to perform read operation 14
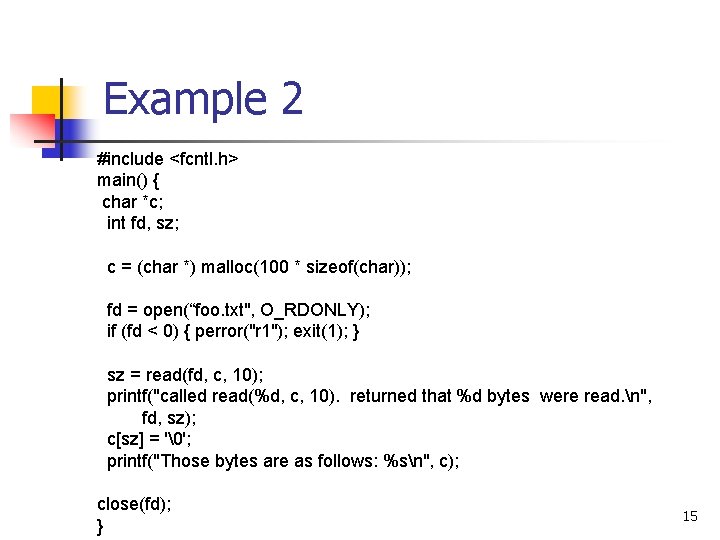
Example 2 #include <fcntl. h> main() { char *c; int fd, sz; c = (char *) malloc(100 * sizeof(char)); fd = open(“foo. txt", O_RDONLY); if (fd < 0) { perror("r 1"); exit(1); } sz = read(fd, c, 10); printf("called read(%d, c, 10). returned that %d bytes were read. n", fd, sz); c[sz] = '