Primitive Data Types and Arithmetic Operations CS 0007
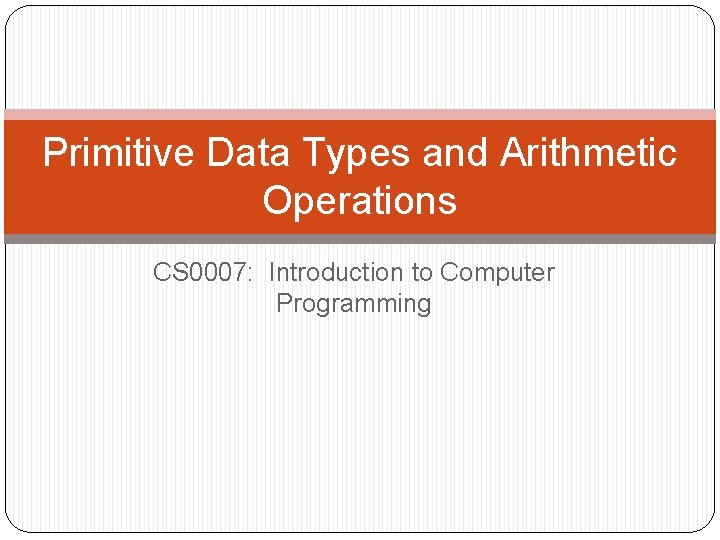
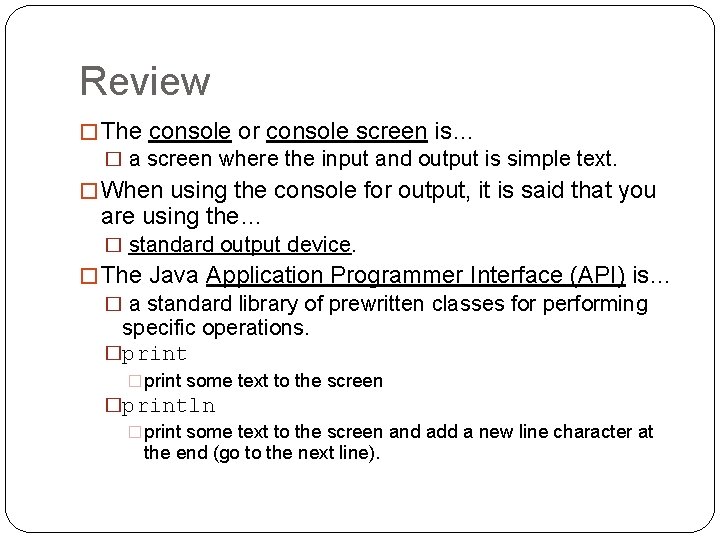
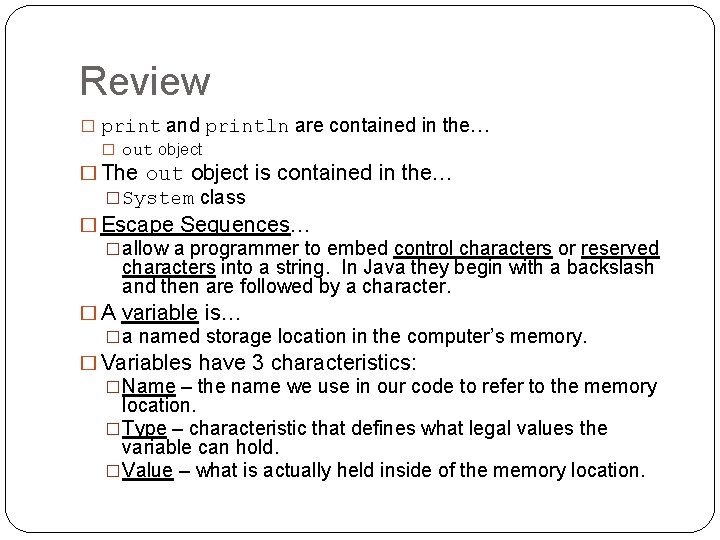
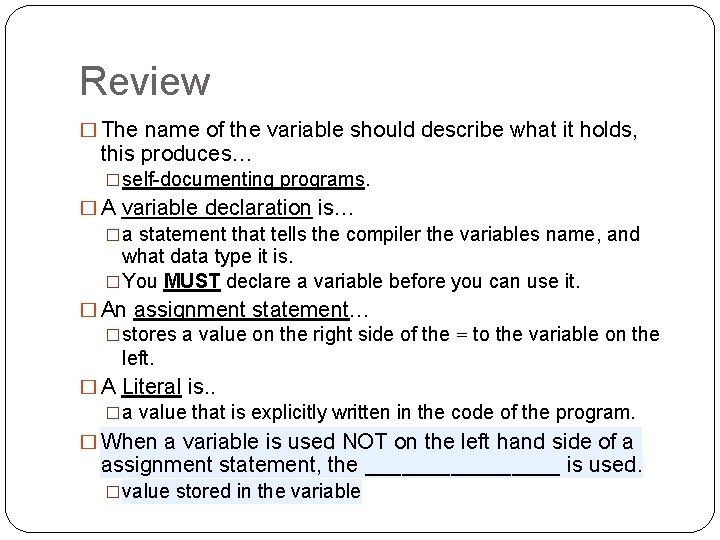
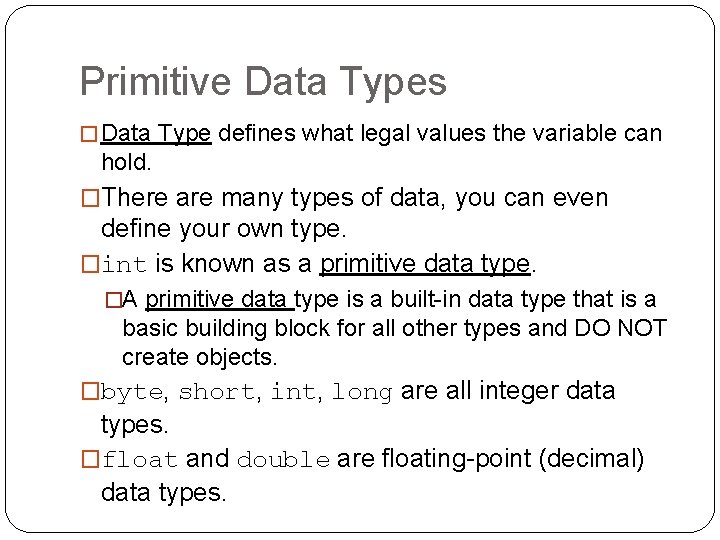
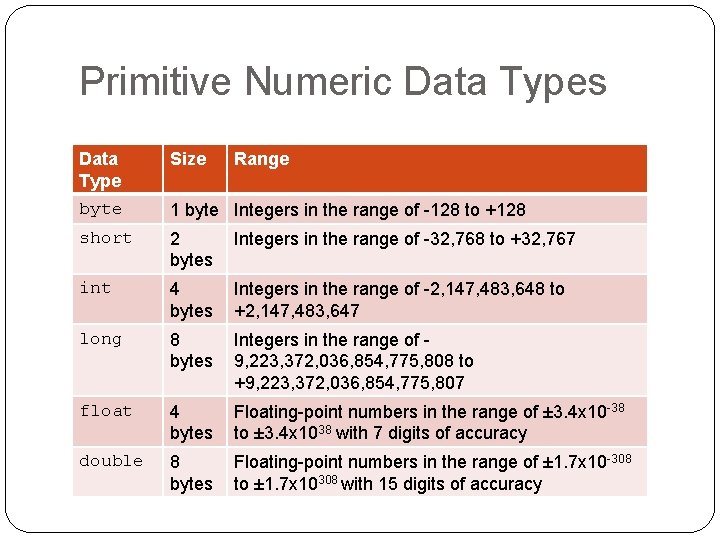
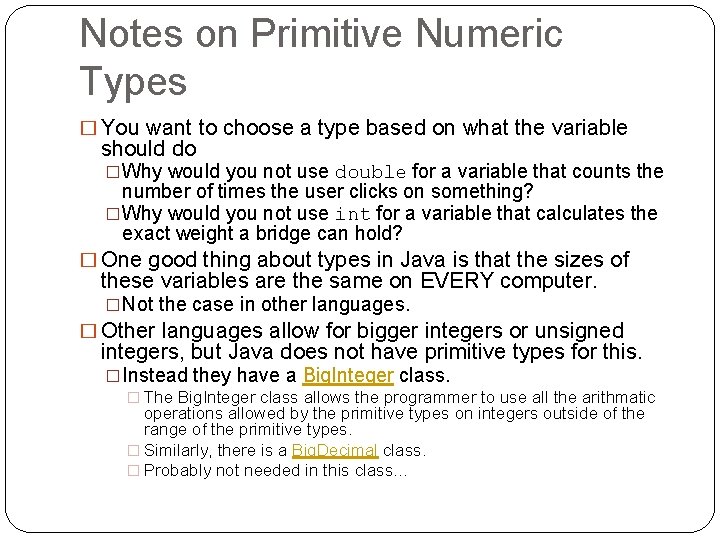
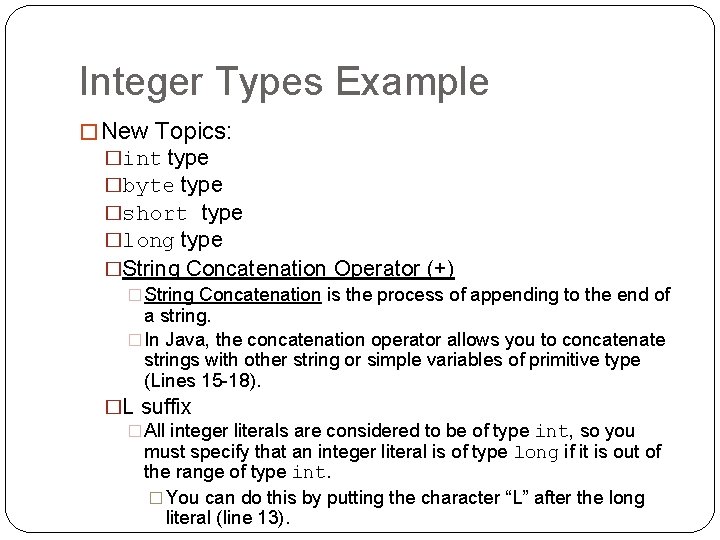
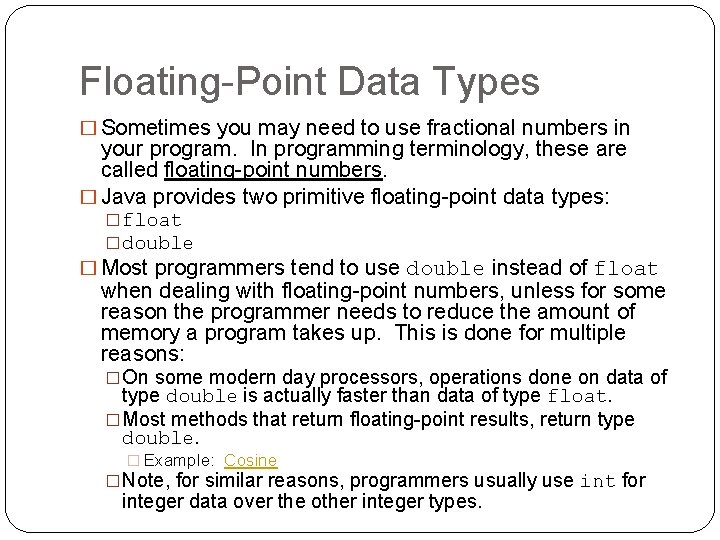
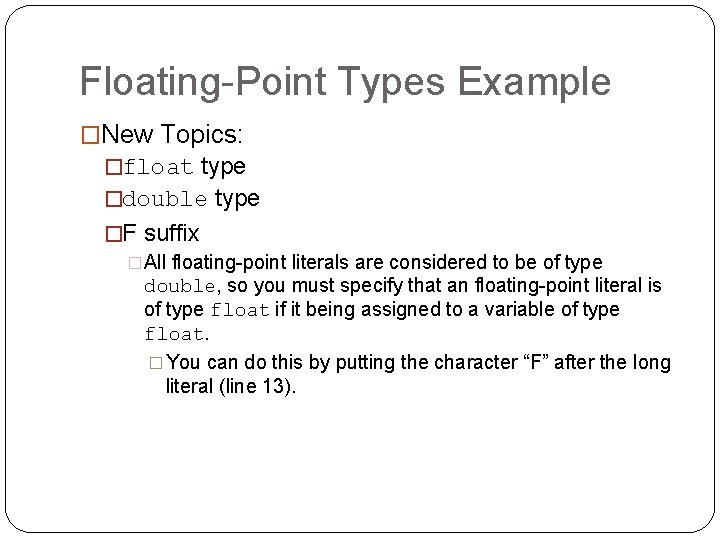
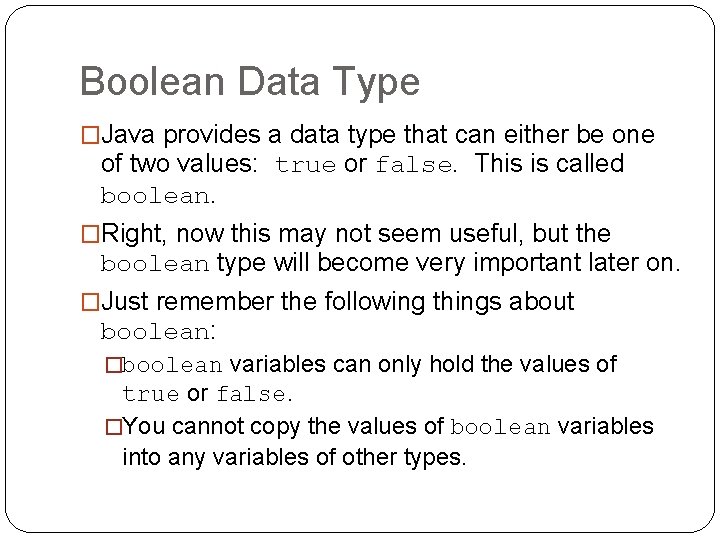
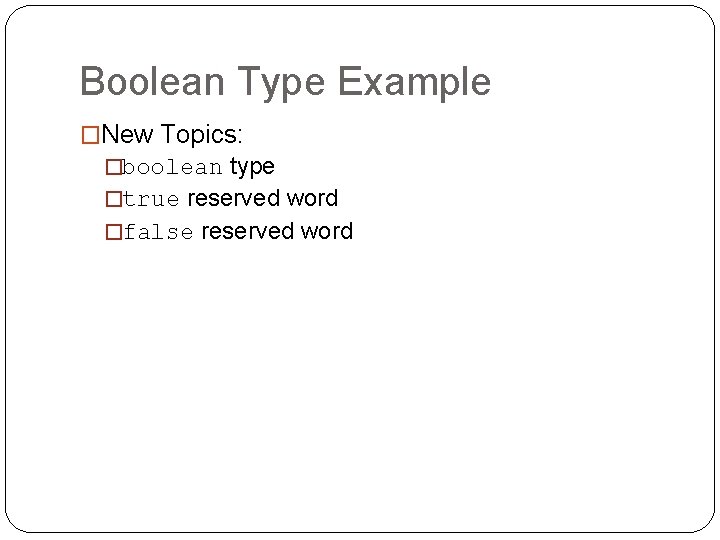
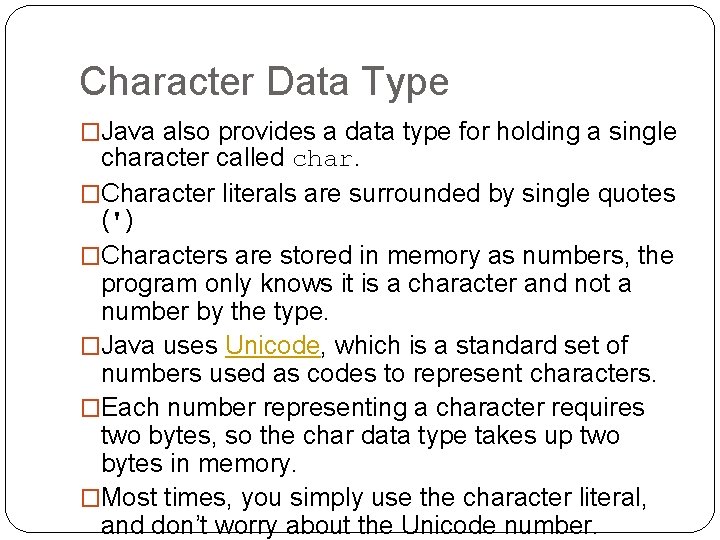
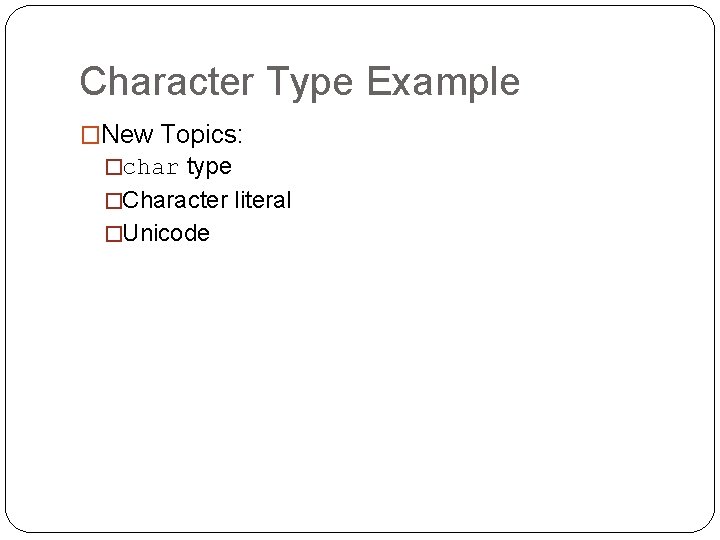
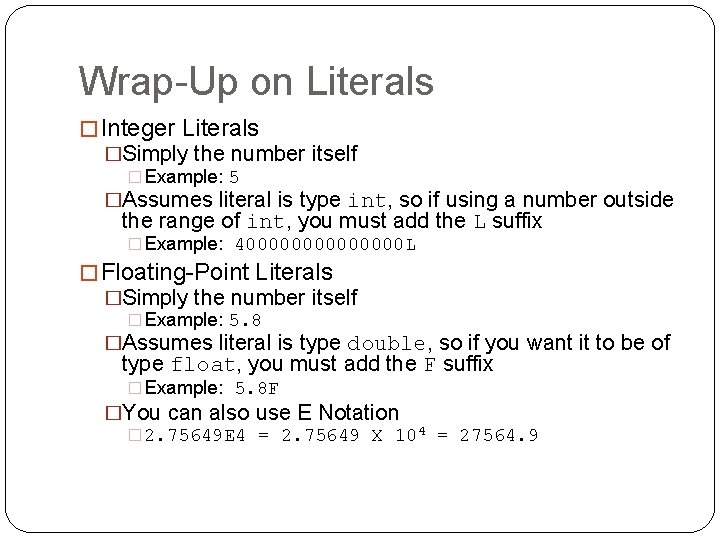
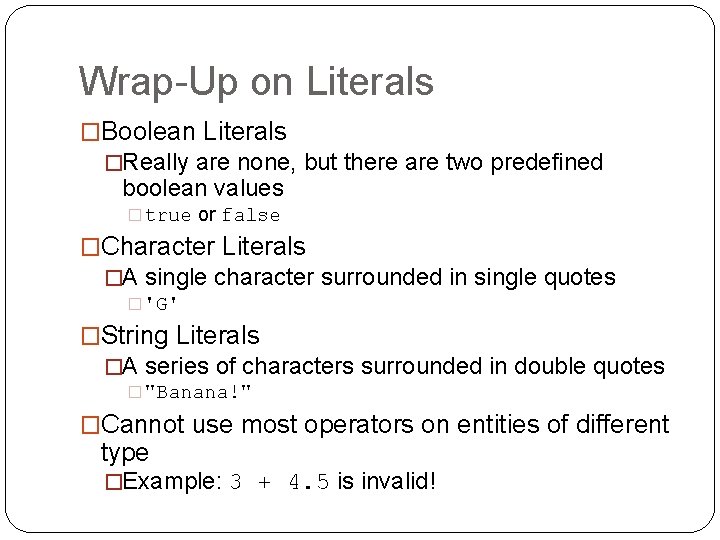
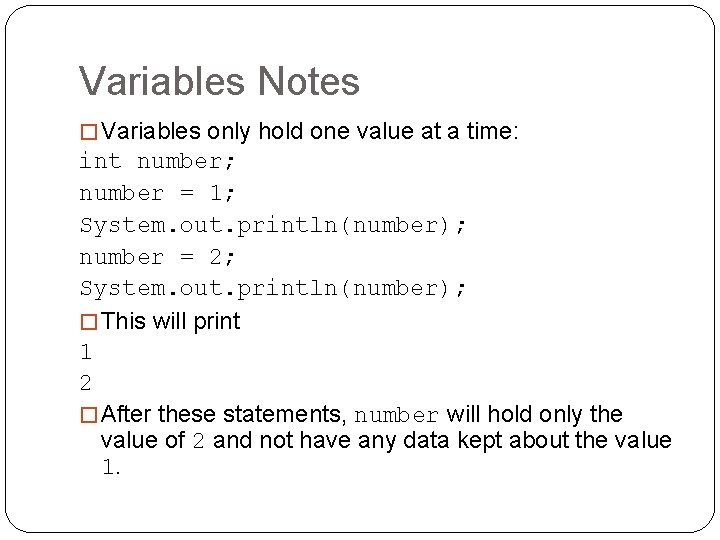
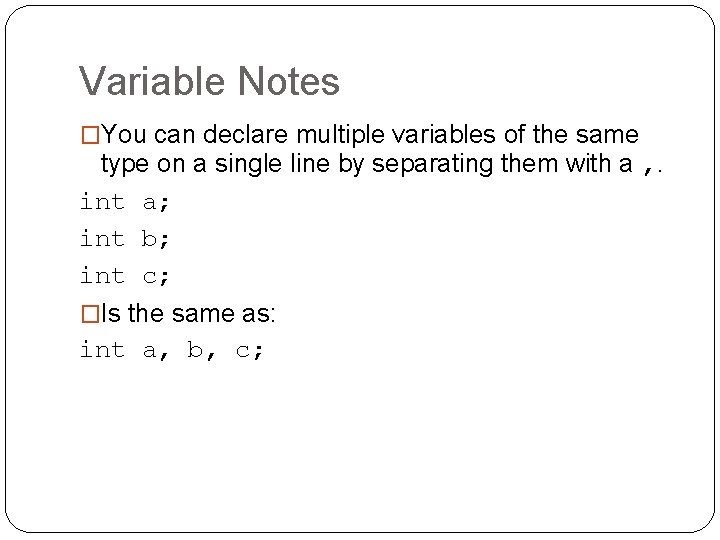
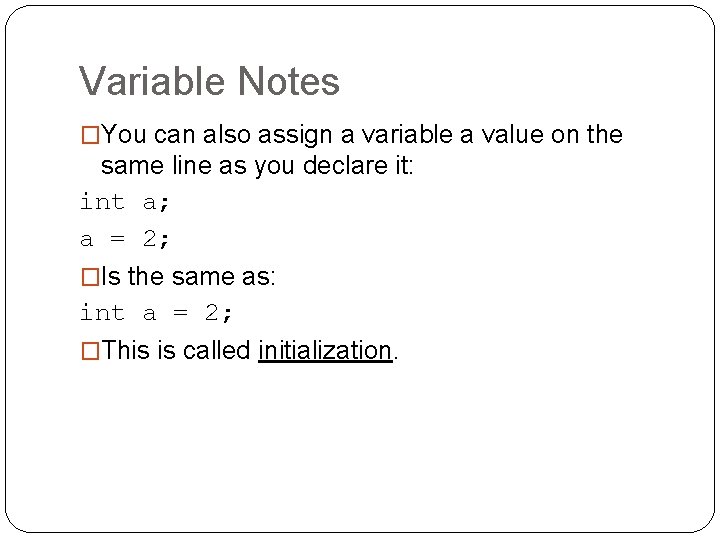
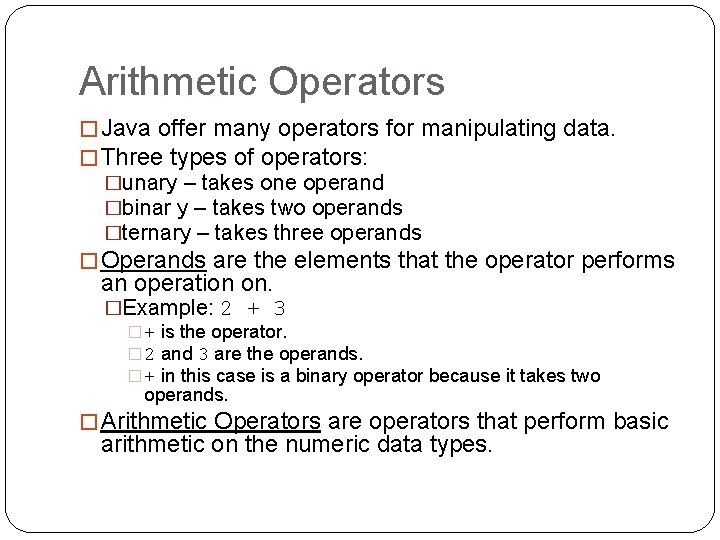
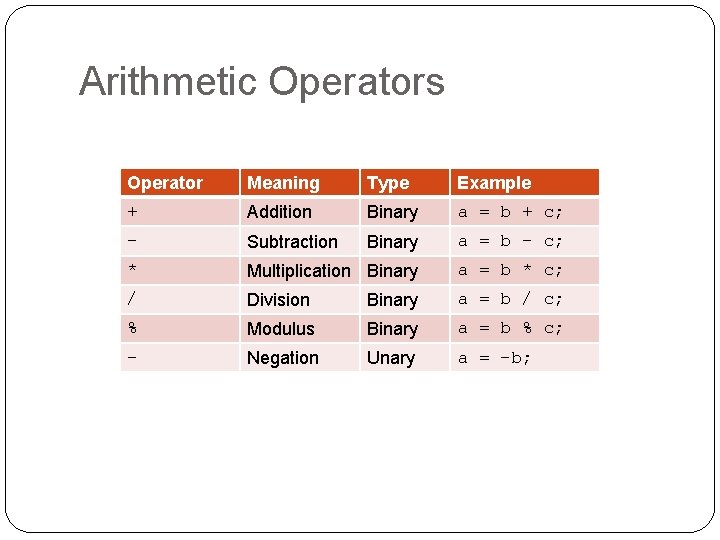
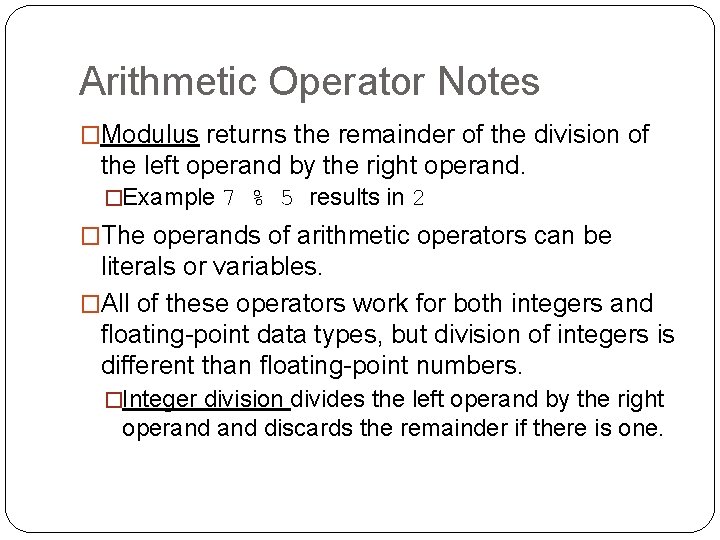
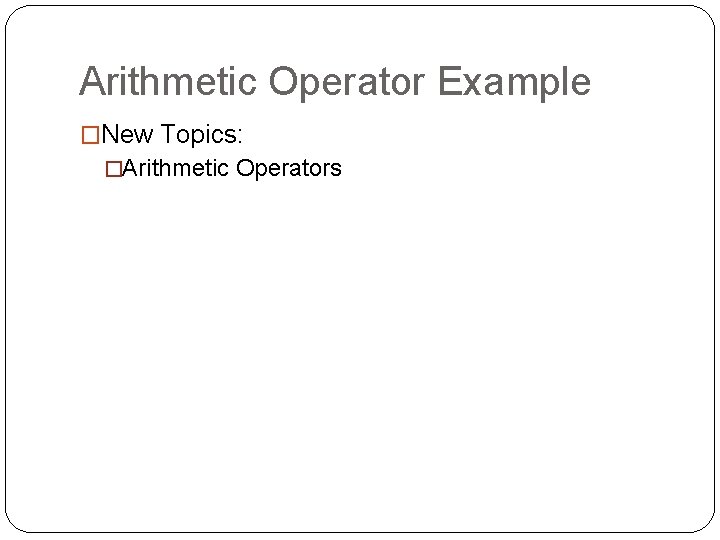
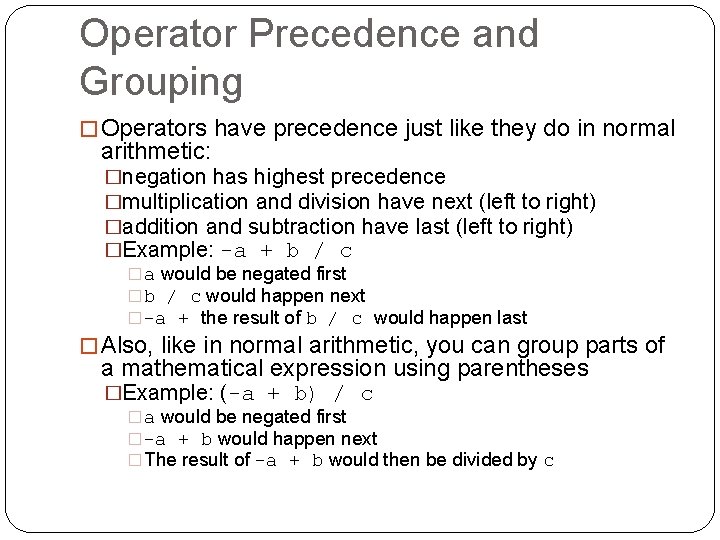
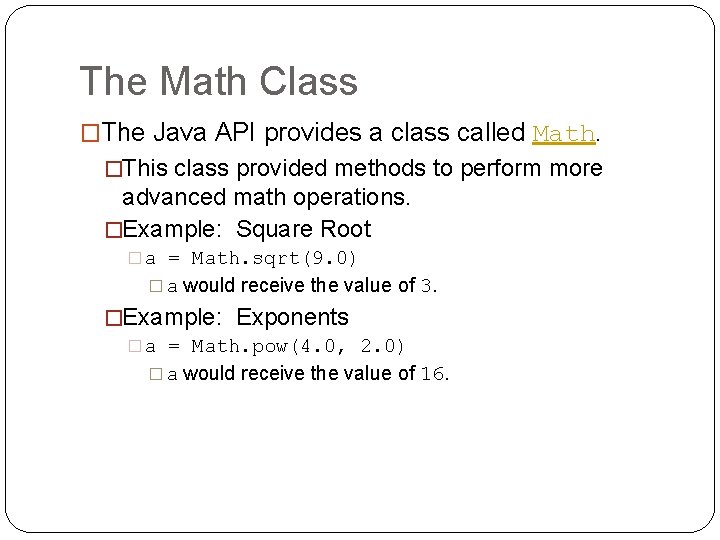
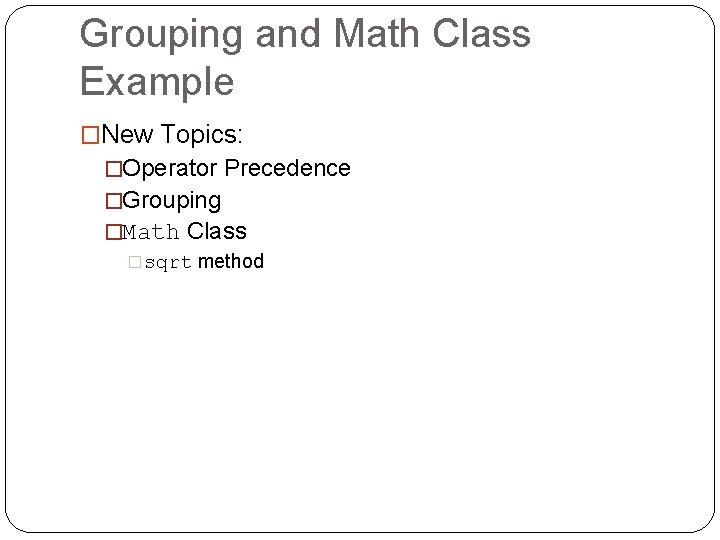
- Slides: 26
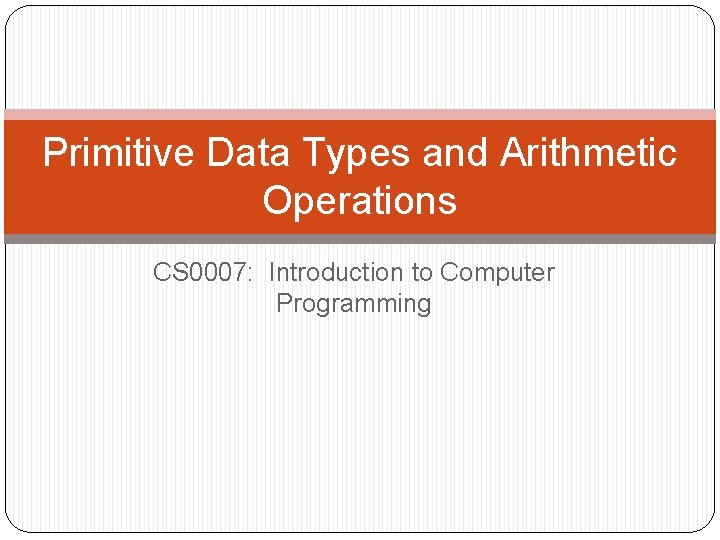
Primitive Data Types and Arithmetic Operations CS 0007: Introduction to Computer Programming
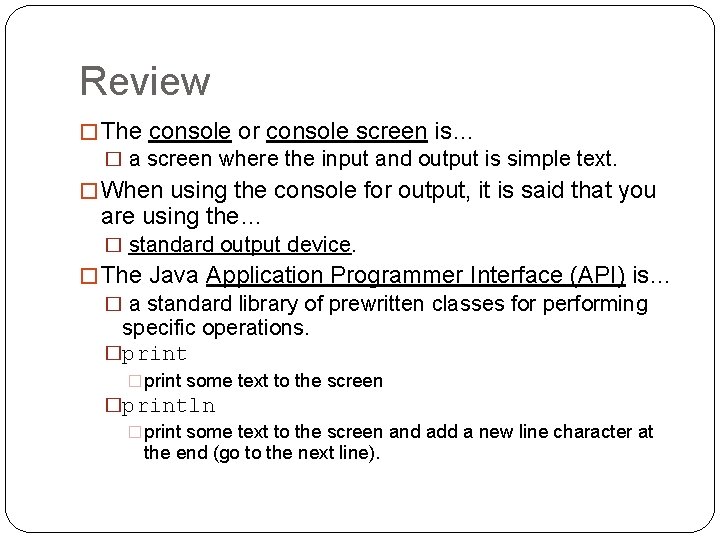
Review � The console or console screen is… � a screen where the input and output is simple text. � When using the console for output, it is said that you are using the… � standard output device. � The Java Application Programmer Interface (API) is… � a standard library of prewritten classes for performing specific operations. �print � print some text to the screen �println � print some text to the screen and add a new line character at the end (go to the next line).
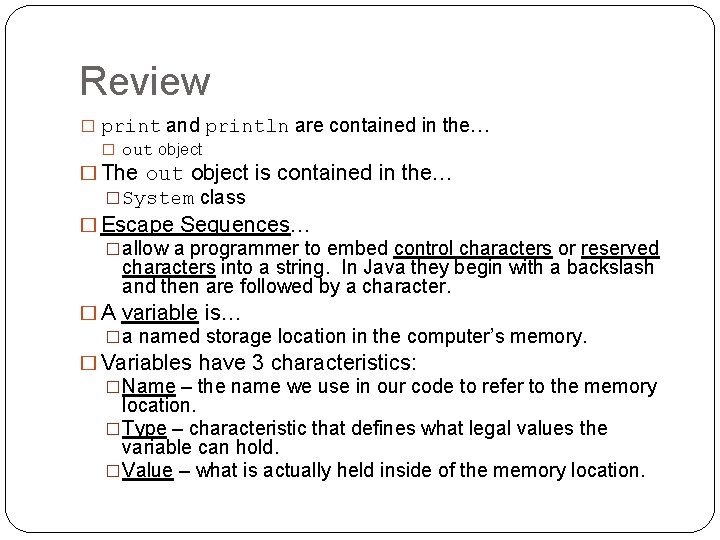
Review � print and println are contained in the… � out object � The out object is contained in the… �System class � Escape Sequences… �allow a programmer to embed control characters or reserved characters into a string. In Java they begin with a backslash and then are followed by a character. � A variable is… �a named storage location in the computer’s memory. � Variables have 3 characteristics: �Name – the name we use in our code to refer to the memory location. �Type – characteristic that defines what legal values the variable can hold. �Value – what is actually held inside of the memory location.
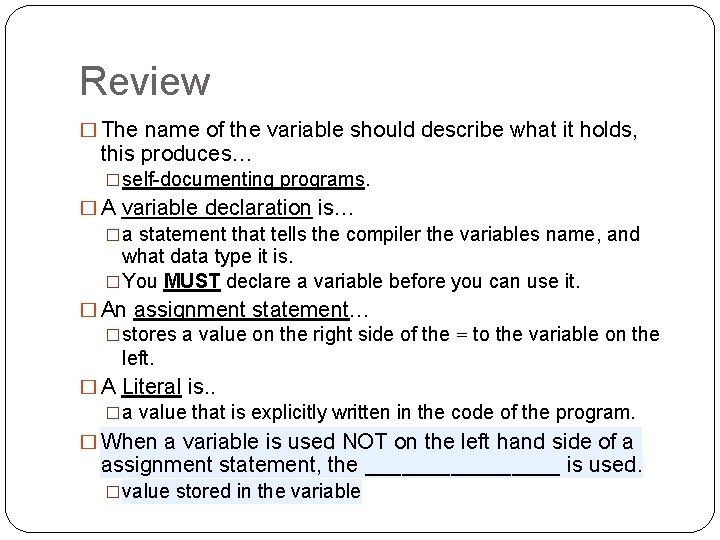
Review � The name of the variable should describe what it holds, this produces… �self-documenting programs. � A variable declaration is… �a statement that tells the compiler the variables name, and what data type it is. �You MUST declare a variable before you can use it. � An assignment statement… �stores a value on the right side of the = to the variable on the left. � A Literal is. . �a value that is explicitly written in the code of the program. � When a variable is used NOT on the left hand side of a assignment statement, the ________ is used. �value stored in the variable
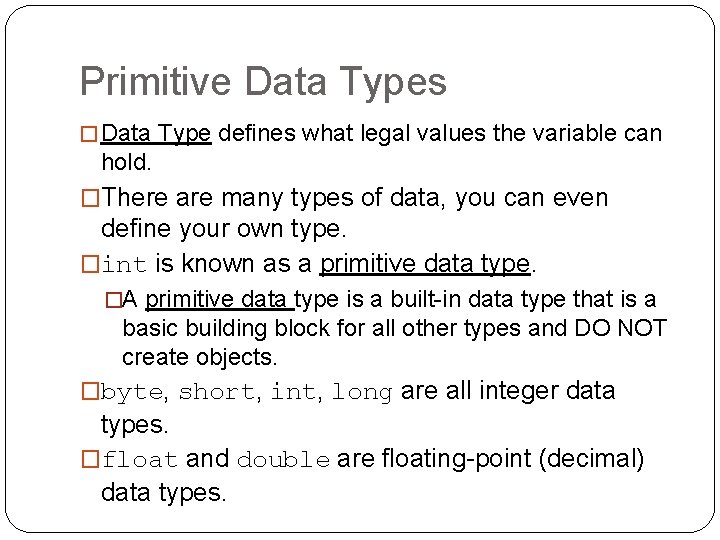
Primitive Data Types � Data Type defines what legal values the variable can hold. �There are many types of data, you can even define your own type. �int is known as a primitive data type. �A primitive data type is a built-in data type that is a basic building block for all other types and DO NOT create objects. �byte, short, int, long are all integer data types. �float and double are floating-point (decimal) data types.
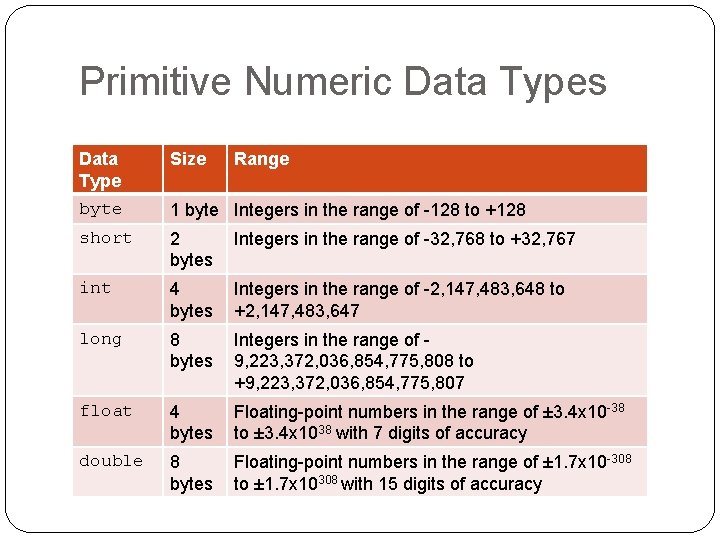
Primitive Numeric Data Types Data Type Size Range byte 1 byte Integers in the range of -128 to +128 short 2 bytes Integers in the range of -32, 768 to +32, 767 int 4 bytes Integers in the range of -2, 147, 483, 648 to +2, 147, 483, 647 long 8 bytes Integers in the range of 9, 223, 372, 036, 854, 775, 808 to +9, 223, 372, 036, 854, 775, 807 float 4 bytes Floating-point numbers in the range of ± 3. 4 x 10 -38 to ± 3. 4 x 1038 with 7 digits of accuracy double 8 bytes Floating-point numbers in the range of ± 1. 7 x 10 -308 to ± 1. 7 x 10308 with 15 digits of accuracy
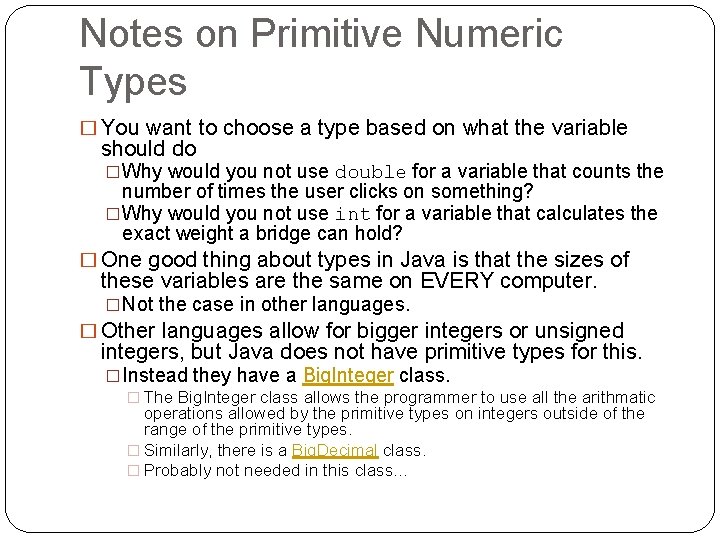
Notes on Primitive Numeric Types � You want to choose a type based on what the variable should do �Why would you not use double for a variable that counts the number of times the user clicks on something? �Why would you not use int for a variable that calculates the exact weight a bridge can hold? � One good thing about types in Java is that the sizes of these variables are the same on EVERY computer. �Not the case in other languages. � Other languages allow for bigger integers or unsigned integers, but Java does not have primitive types for this. �Instead they have a Big. Integer class. � The Big. Integer class allows the programmer to use all the arithmatic operations allowed by the primitive types on integers outside of the range of the primitive types. � Similarly, there is a Big. Decimal class. � Probably not needed in this class…
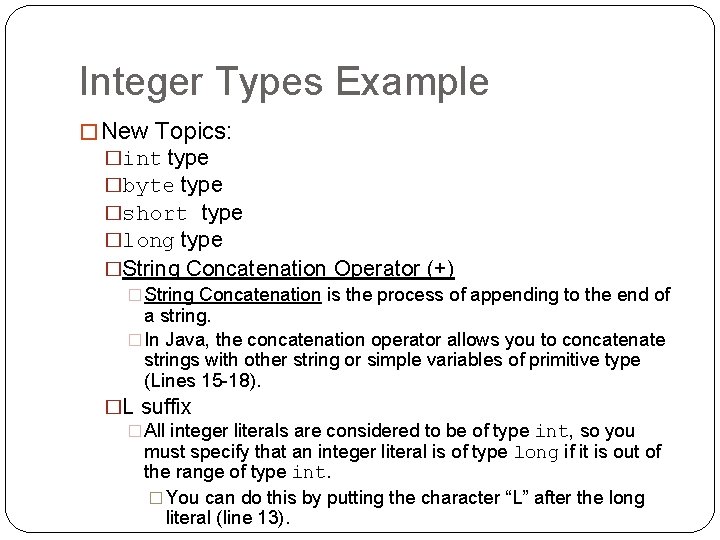
Integer Types Example � New Topics: �int type �byte type �short type �long type �String Concatenation Operator (+) � String Concatenation is the process of appending to the end of a string. � In Java, the concatenation operator allows you to concatenate strings with other string or simple variables of primitive type (Lines 15 -18). �L suffix � All integer literals are considered to be of type int, so you must specify that an integer literal is of type long if it is out of the range of type int. � You can do this by putting the character “L” after the long literal (line 13).
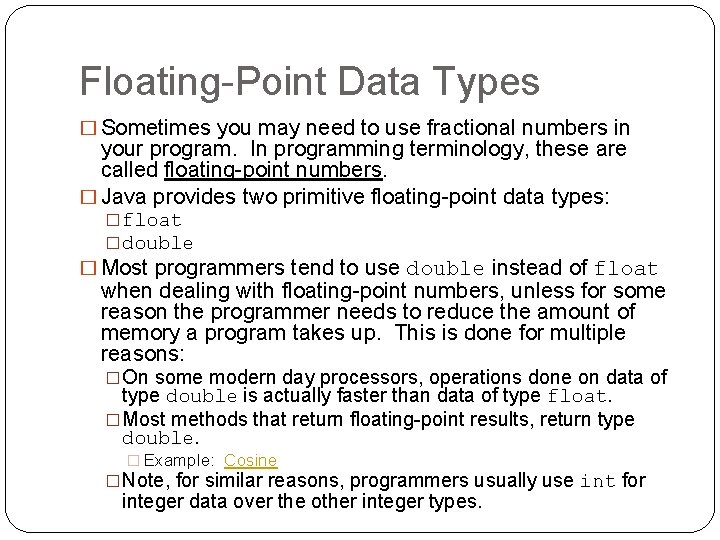
Floating-Point Data Types � Sometimes you may need to use fractional numbers in your program. In programming terminology, these are called floating-point numbers. � Java provides two primitive floating-point data types: �float �double � Most programmers tend to use double instead of float when dealing with floating-point numbers, unless for some reason the programmer needs to reduce the amount of memory a program takes up. This is done for multiple reasons: �On some modern day processors, operations done on data of type double is actually faster than data of type float. �Most methods that return floating-point results, return type double. � Example: Cosine �Note, for similar reasons, programmers usually use int for integer data over the other integer types.
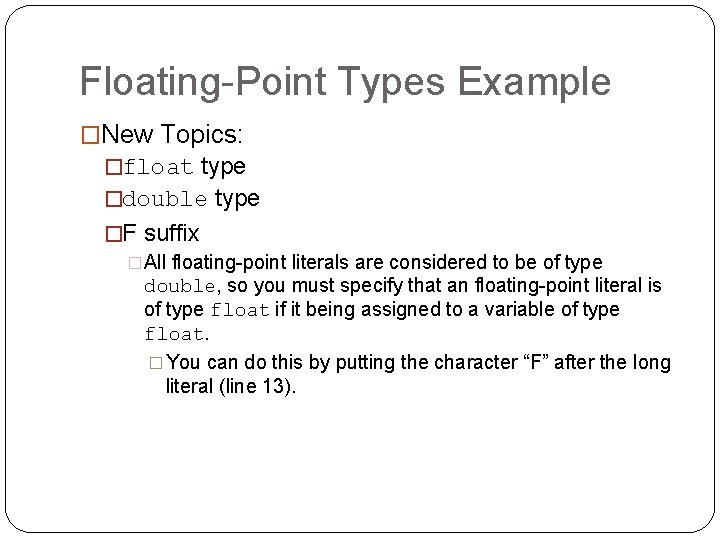
Floating-Point Types Example �New Topics: �float type �double type �F suffix �All floating-point literals are considered to be of type double, so you must specify that an floating-point literal is of type float if it being assigned to a variable of type float. � You can do this by putting the character “F” after the long literal (line 13).
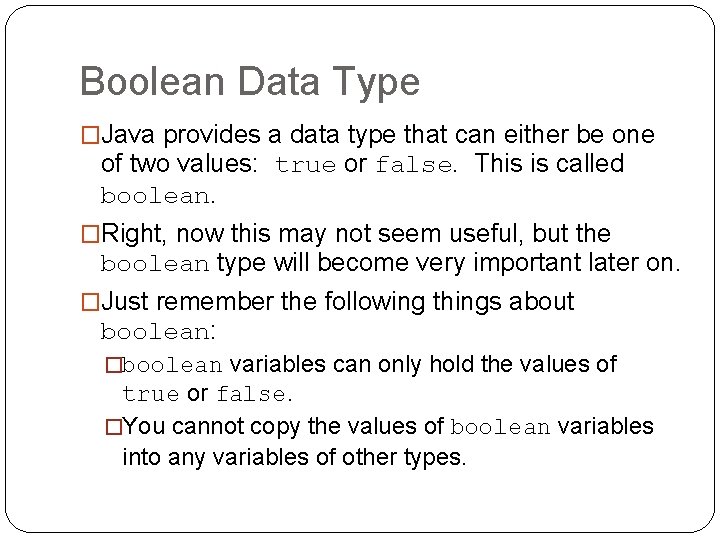
Boolean Data Type �Java provides a data type that can either be one of two values: true or false. This is called boolean. �Right, now this may not seem useful, but the boolean type will become very important later on. �Just remember the following things about boolean: �boolean variables can only hold the values of true or false. �You cannot copy the values of boolean variables into any variables of other types.
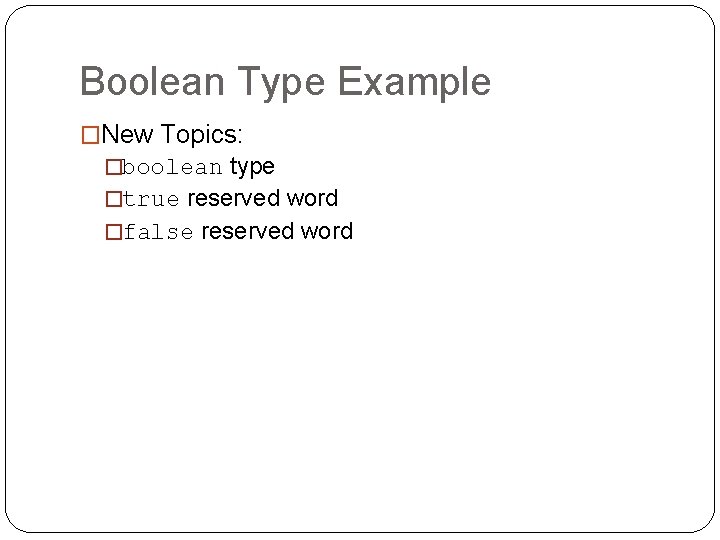
Boolean Type Example �New Topics: �boolean type �true reserved word �false reserved word
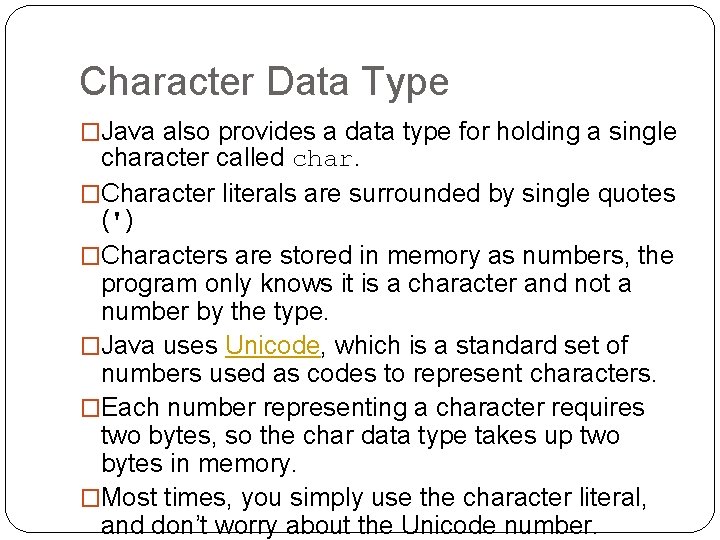
Character Data Type �Java also provides a data type for holding a single character called char. �Character literals are surrounded by single quotes (') �Characters are stored in memory as numbers, the program only knows it is a character and not a number by the type. �Java uses Unicode, which is a standard set of numbers used as codes to represent characters. �Each number representing a character requires two bytes, so the char data type takes up two bytes in memory. �Most times, you simply use the character literal, and don’t worry about the Unicode number.
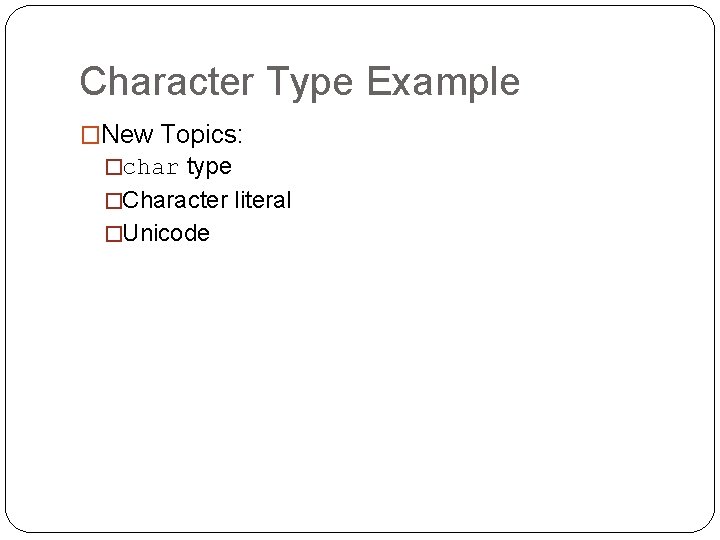
Character Type Example �New Topics: �char type �Character literal �Unicode
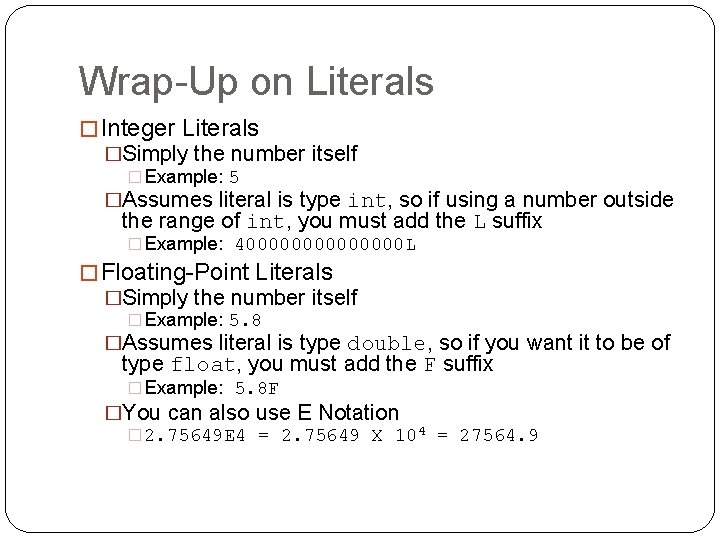
Wrap-Up on Literals � Integer Literals �Simply the number itself � Example: 5 �Assumes literal is type int, so if using a number outside the range of int, you must add the L suffix � Example: 40000000 L � Floating-Point Literals �Simply the number itself � Example: 5. 8 �Assumes literal is type double, so if you want it to be of type float, you must add the F suffix � Example: 5. 8 F �You can also use E Notation � 2. 75649 E 4 = 2. 75649 X 104 = 27564. 9
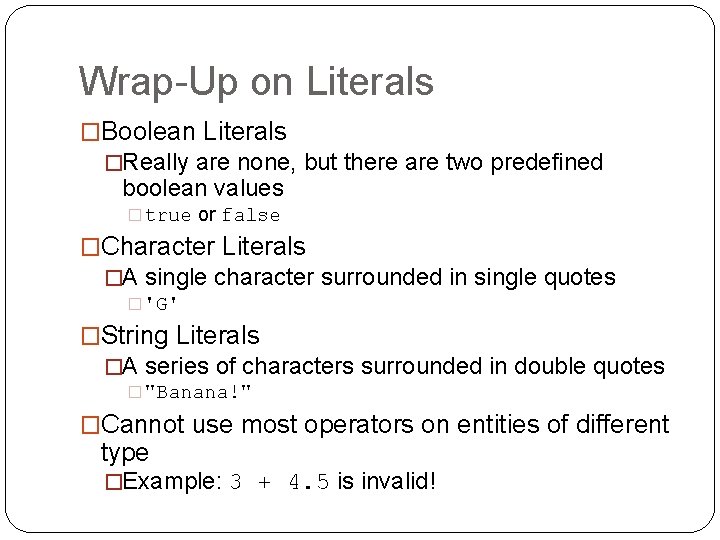
Wrap-Up on Literals �Boolean Literals �Really are none, but there are two predefined boolean values �true or false �Character Literals �A single character surrounded in single quotes �'G' �String Literals �A series of characters surrounded in double quotes �"Banana!" �Cannot use most operators on entities of different type �Example: 3 + 4. 5 is invalid!
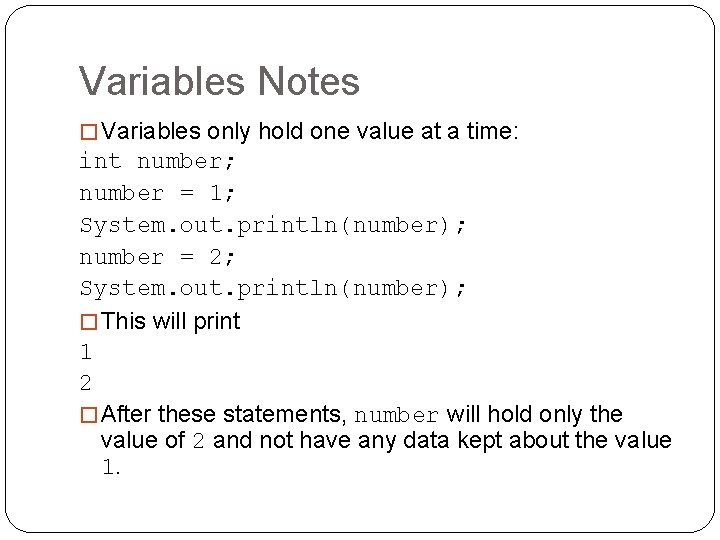
Variables Notes � Variables only hold one value at a time: int number; number = 1; System. out. println(number); number = 2; System. out. println(number); � This will print 1 2 � After these statements, number will hold only the value of 2 and not have any data kept about the value 1.
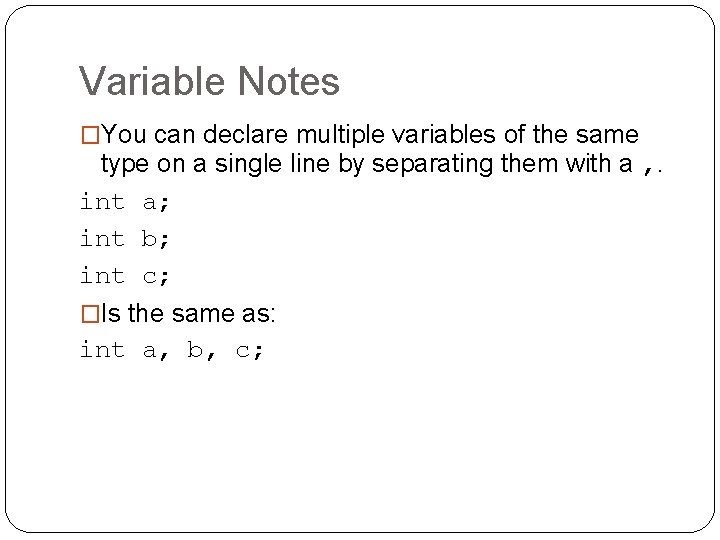
Variable Notes �You can declare multiple variables of the same type on a single line by separating them with a , . int a; int b; int c; �Is the same as: int a, b, c;
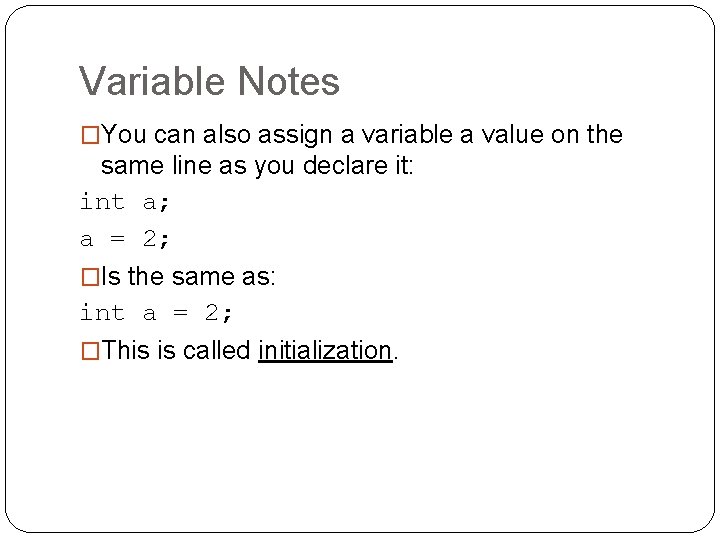
Variable Notes �You can also assign a variable a value on the same line as you declare it: int a; a = 2; �Is the same as: int a = 2; �This is called initialization.
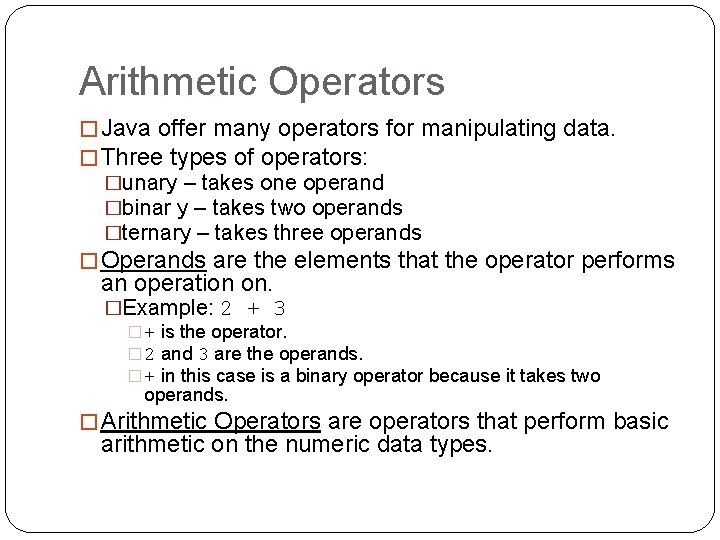
Arithmetic Operators � Java offer many operators for manipulating data. � Three types of operators: �unary – takes one operand �binar y – takes two operands �ternary – takes three operands � Operands are the elements that the operator performs an operation on. �Example: 2 + 3 � + is the operator. � 2 and 3 are the operands. � + in this case is a binary operator because it takes two operands. � Arithmetic Operators are operators that perform basic arithmetic on the numeric data types.
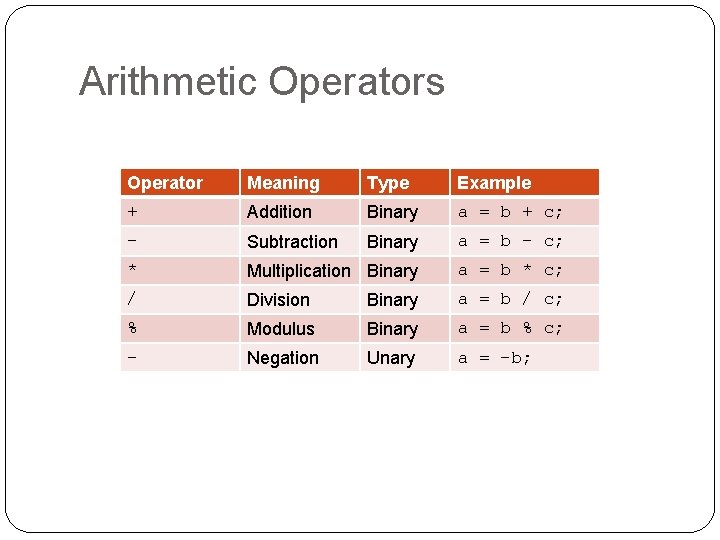
Arithmetic Operators Operator Meaning Type Example + Addition Binary a = b + c; - Subtraction Binary a = b - c; * Multiplication Binary a = b * c; / Division Binary a = b / c; % Modulus Binary a = b % c; - Negation Unary a = -b;
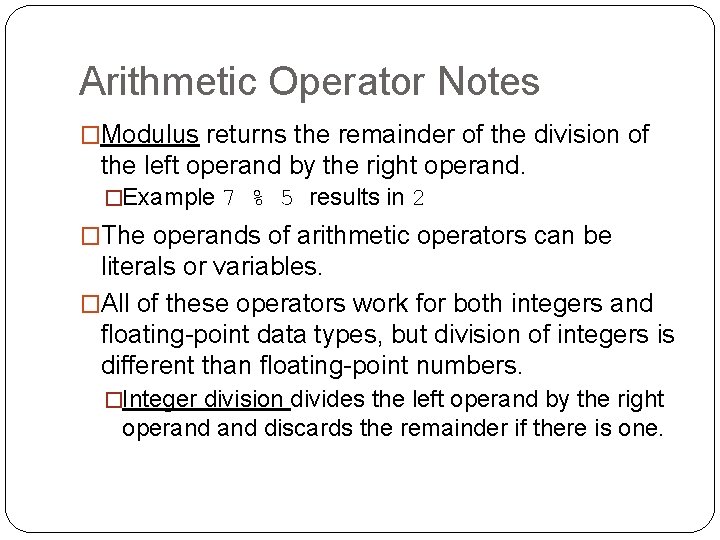
Arithmetic Operator Notes �Modulus returns the remainder of the division of the left operand by the right operand. �Example 7 % 5 results in 2 �The operands of arithmetic operators can be literals or variables. �All of these operators work for both integers and floating-point data types, but division of integers is different than floating-point numbers. �Integer division divides the left operand by the right operand discards the remainder if there is one.
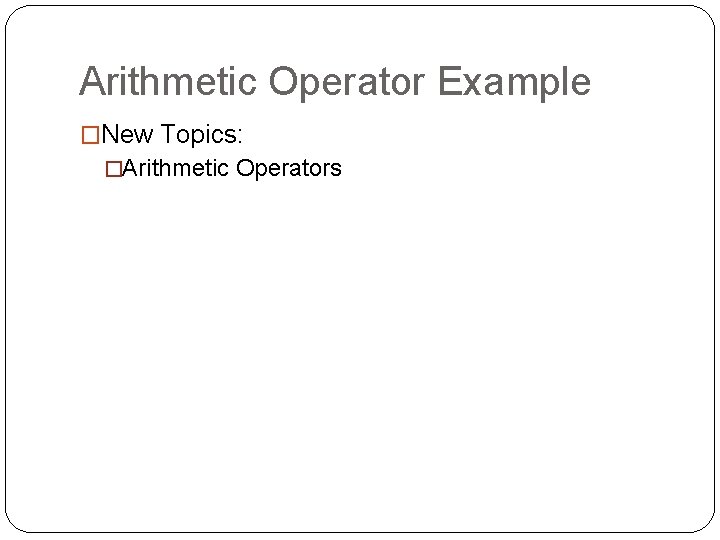
Arithmetic Operator Example �New Topics: �Arithmetic Operators
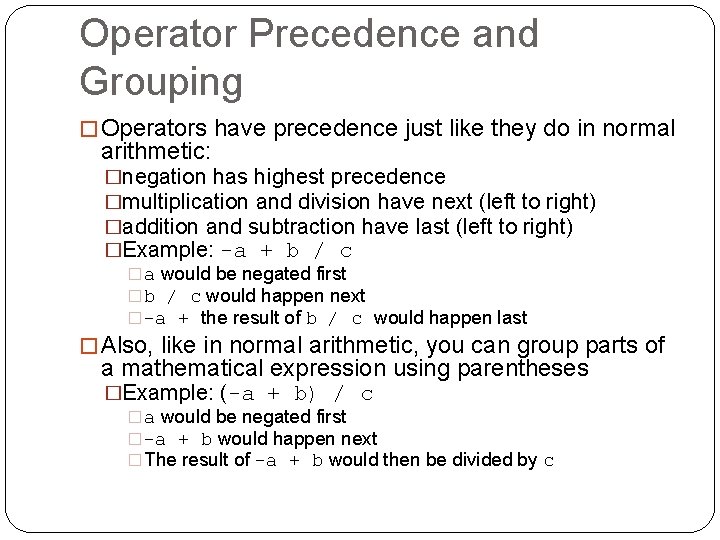
Operator Precedence and Grouping � Operators have precedence just like they do in normal arithmetic: �negation has highest precedence �multiplication and division have next (left to right) �addition and subtraction have last (left to right) �Example: -a + b / c � a would be negated first � b / c would happen next � -a + the result of b / c would happen last � Also, like in normal arithmetic, you can group parts of a mathematical expression using parentheses �Example: (-a + b) / c � a would be negated first � -a + b would happen next � The result of -a + b would then be divided by c
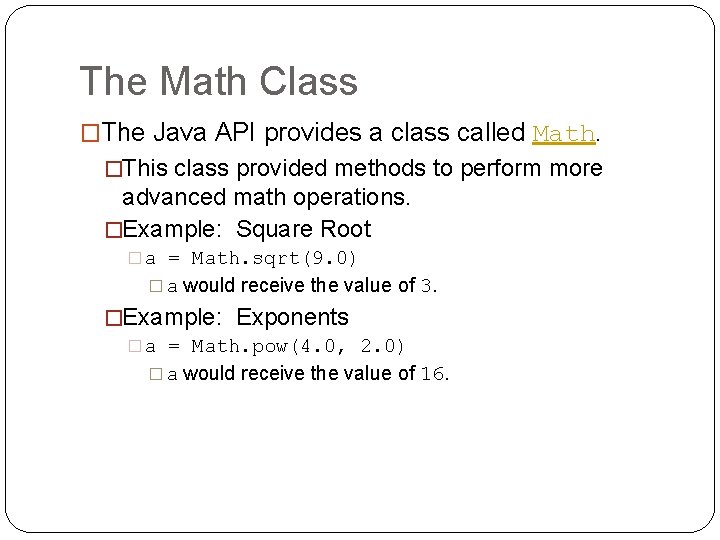
The Math Class �The Java API provides a class called Math. �This class provided methods to perform more advanced math operations. �Example: Square Root �a = Math. sqrt(9. 0) �a would receive the value of 3. �Example: Exponents �a = Math. pow(4. 0, 2. 0) � a would receive the value of 16.
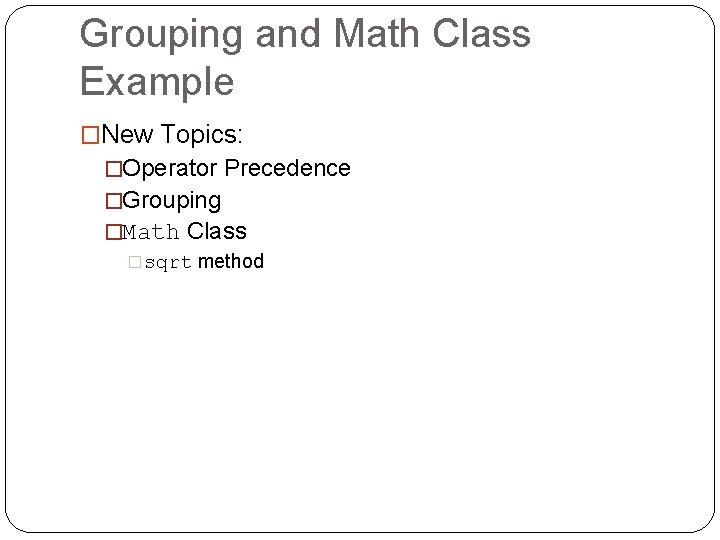
Grouping and Math Class Example �New Topics: �Operator Precedence �Grouping �Math Class �sqrt method
Data structures unit 1
Classification of data structure
Geia-hb-0007
Agent 0007
Counting primitive operations examples
Counting primitive operations
Counting primitive operations
Rtl register transfer language
Arithmetic operationsarithmetic operations
The objective of sharpening spatial filter is to
Modular arithmetic
Explain floating point arithmetic operations with example
Why array index starts with 0
Unit 1 primitive types
Non primitive data structure
Data representation and computer arithmetic
Arithmetic verbs in cobol
Standard deviation grouped data calculator
Mean for ungrouped data
Arithmetic mean for ungrouped data
Weighted mean
Arithmetic mean formula for grouped data
What is mean
Primitive root of a number in cryptography
Semaphores provide a primitive yet powerful and flexible
Greek mythology study guide
Arithmetic and geometric sequences and series