Chapter 2 Primitive Data Types and Operations Liang
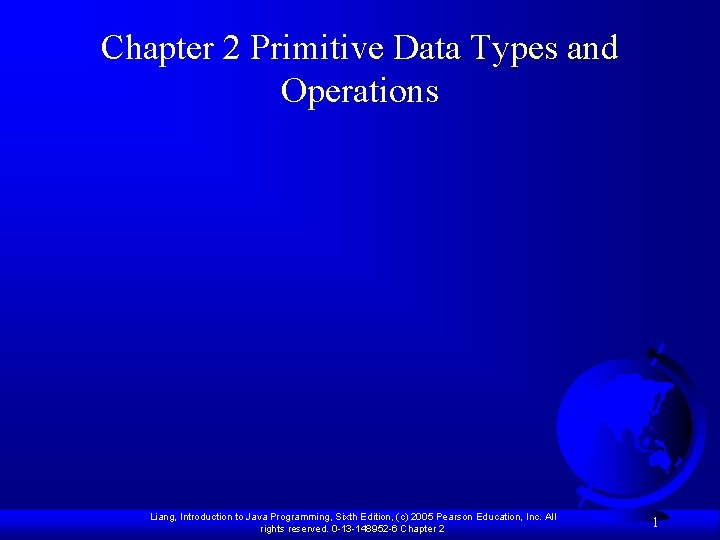
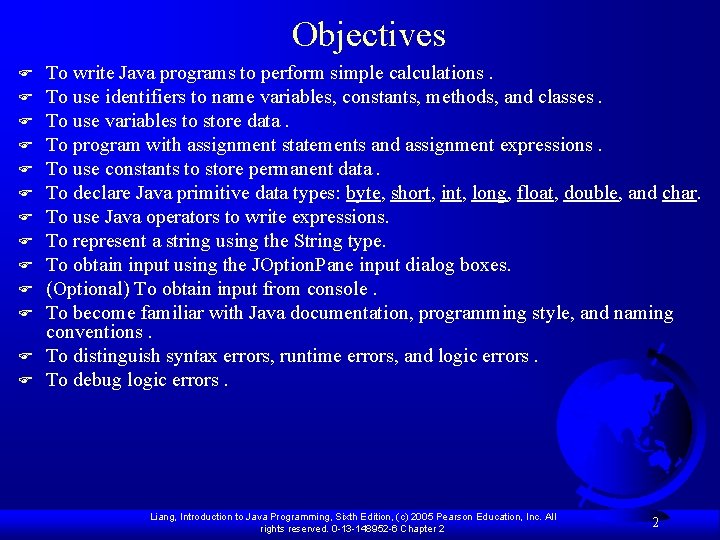
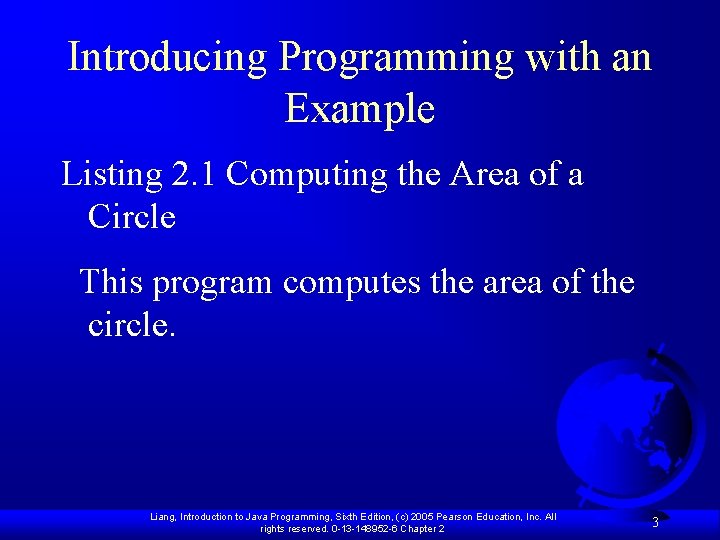
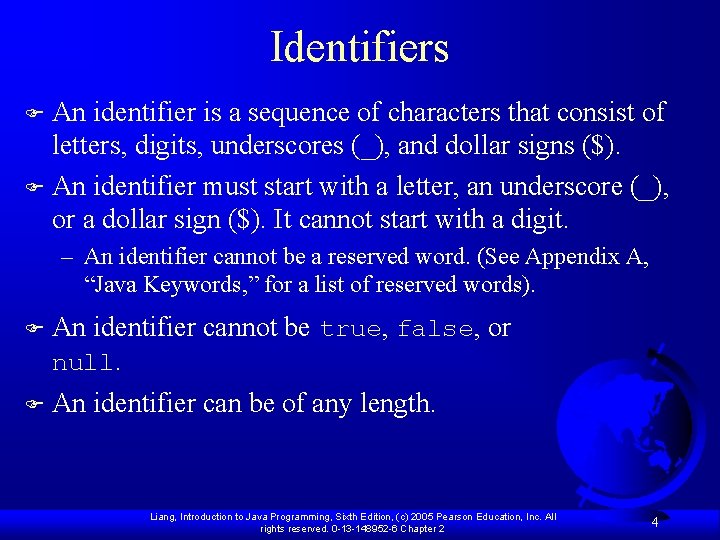
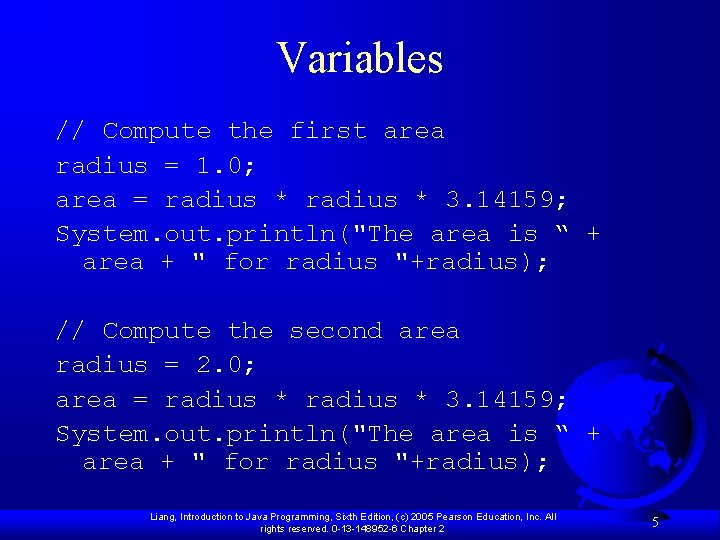
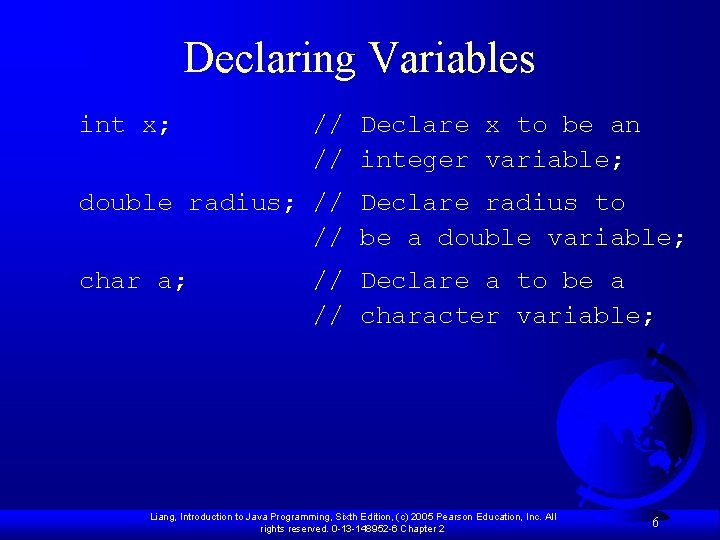
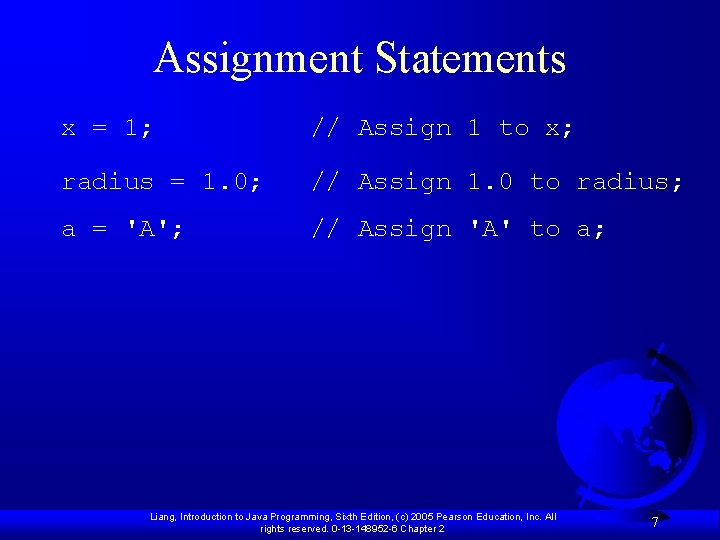
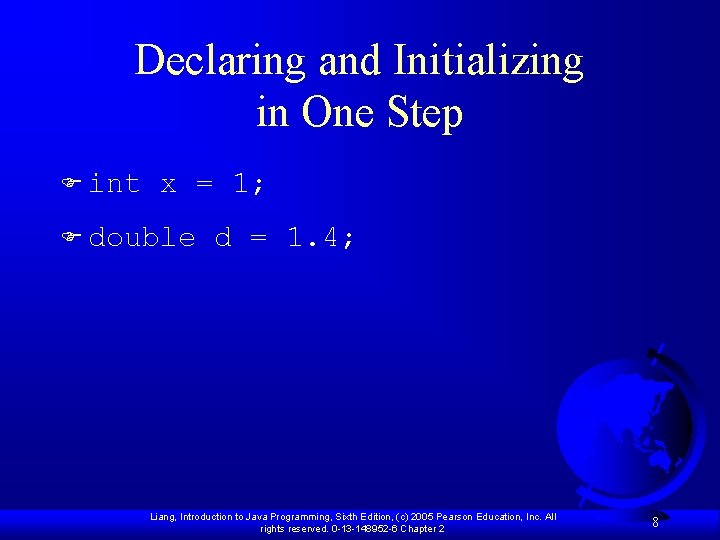
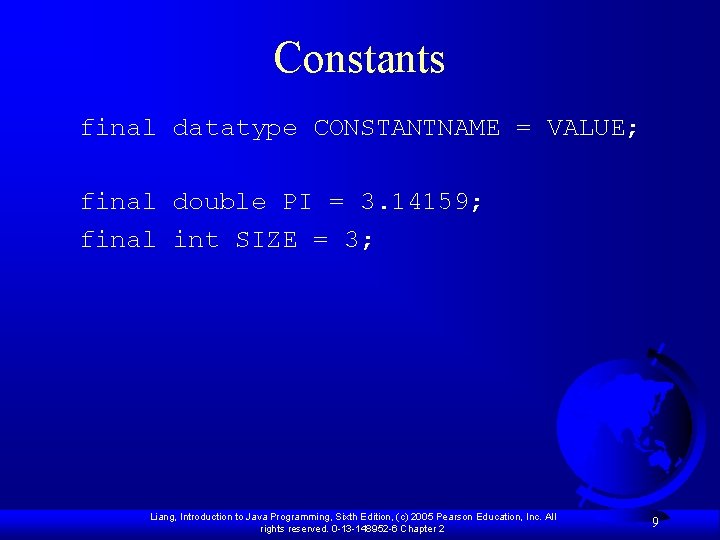
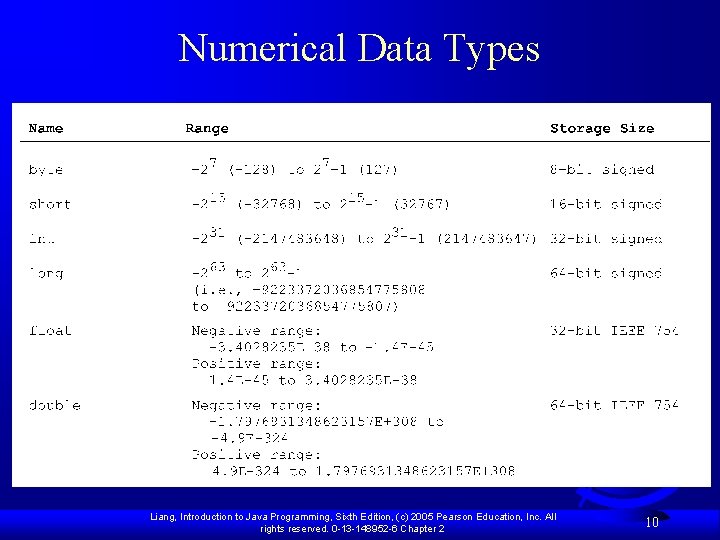
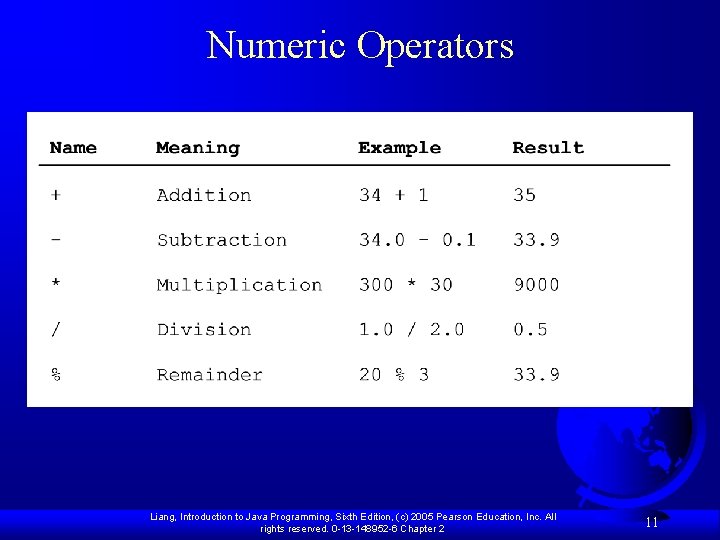
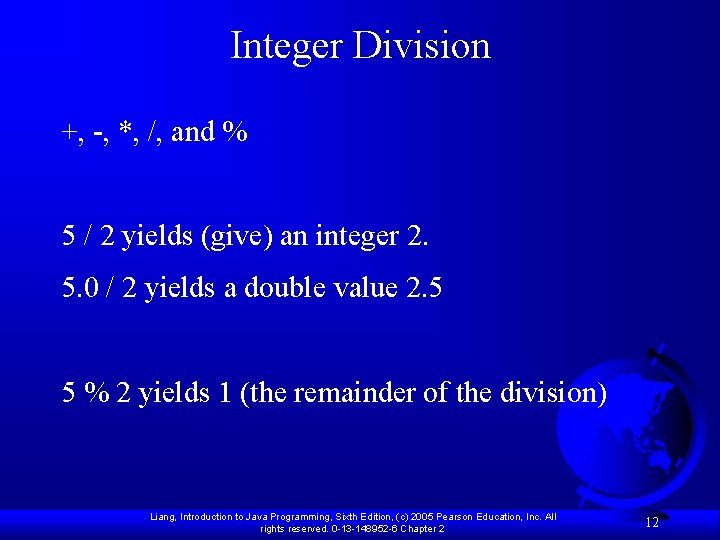
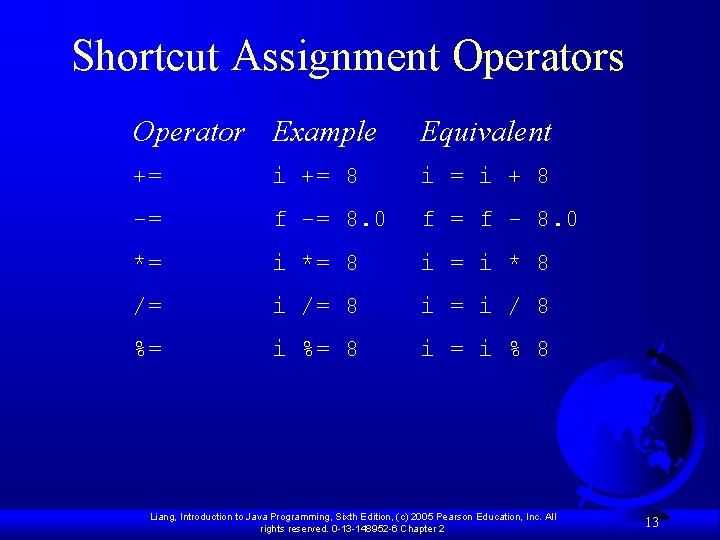
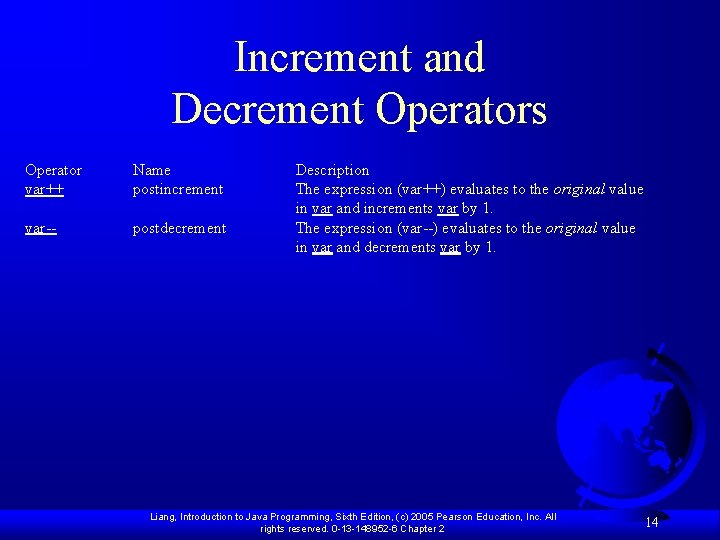
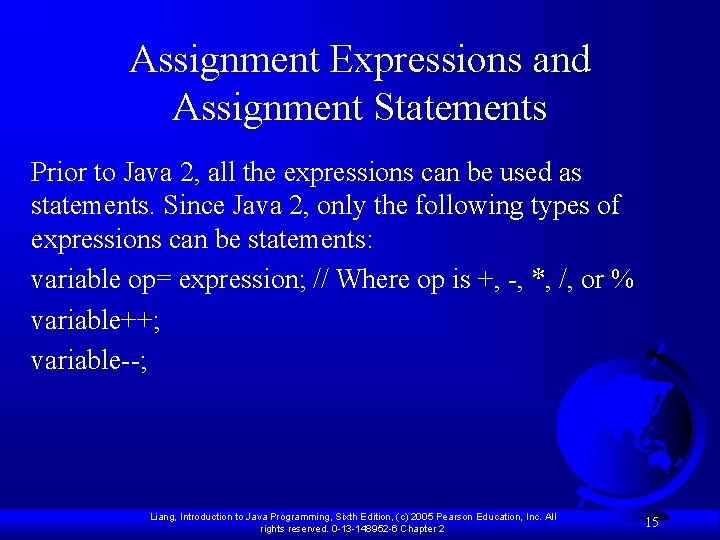
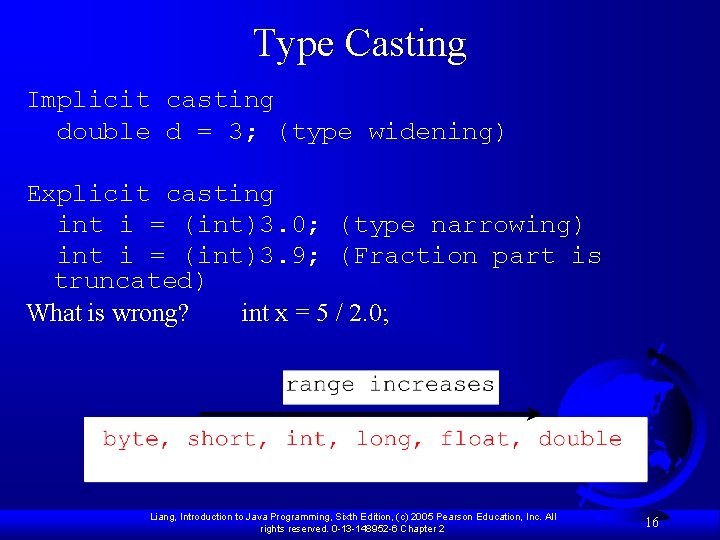
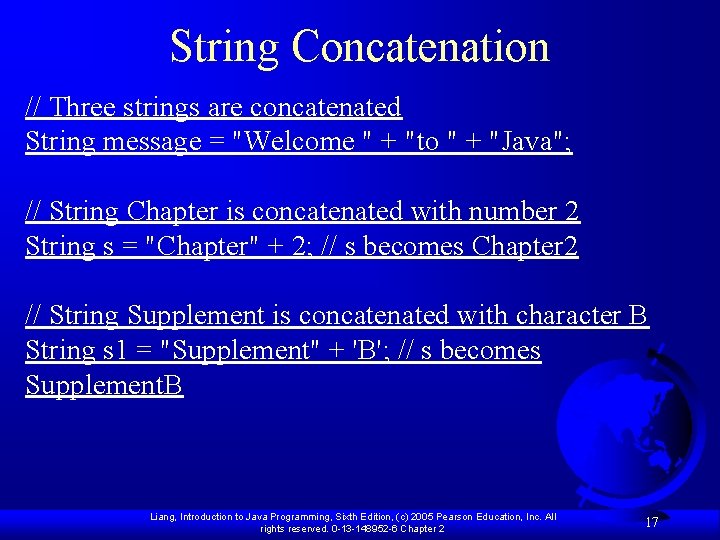
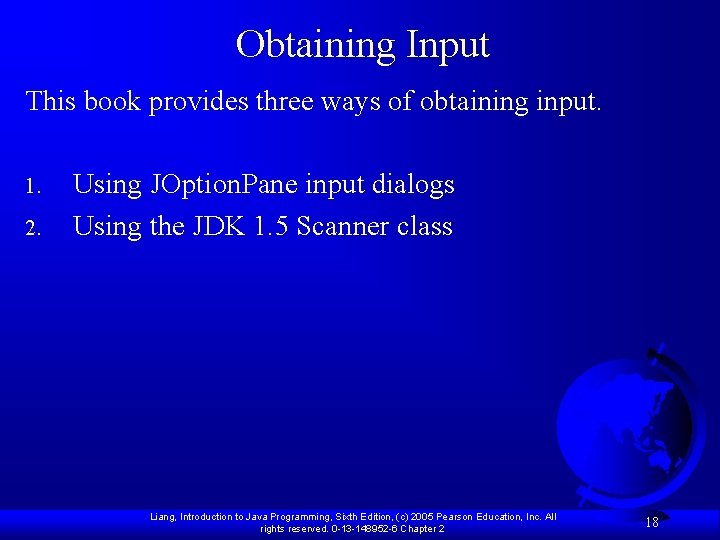
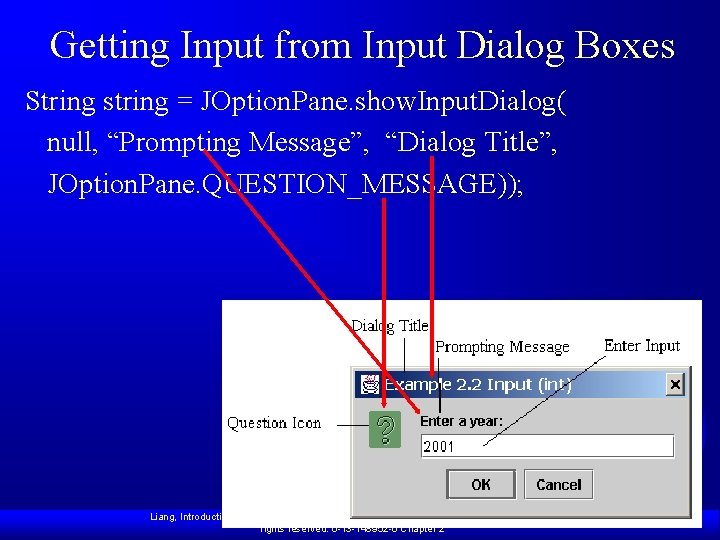
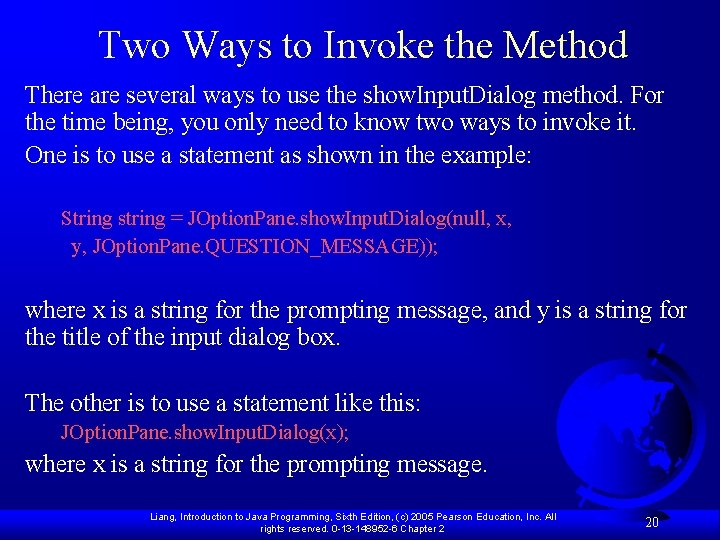
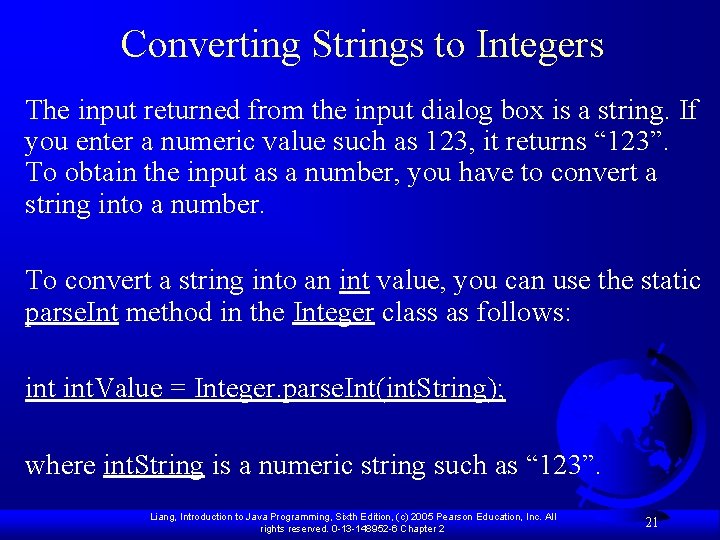
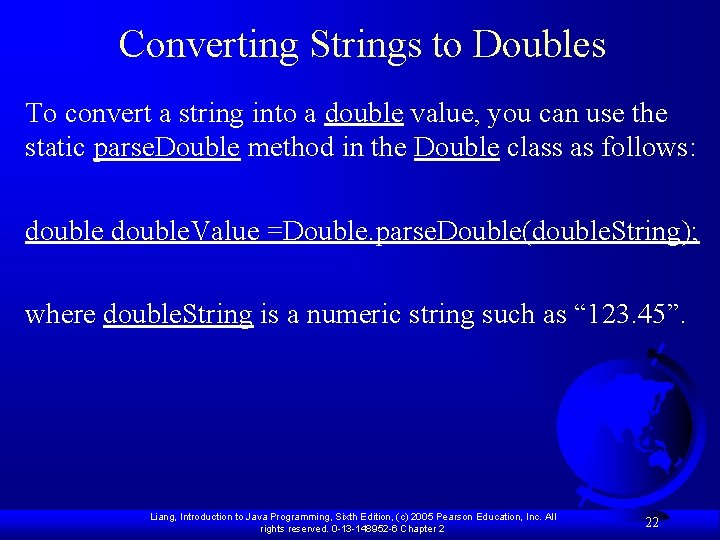
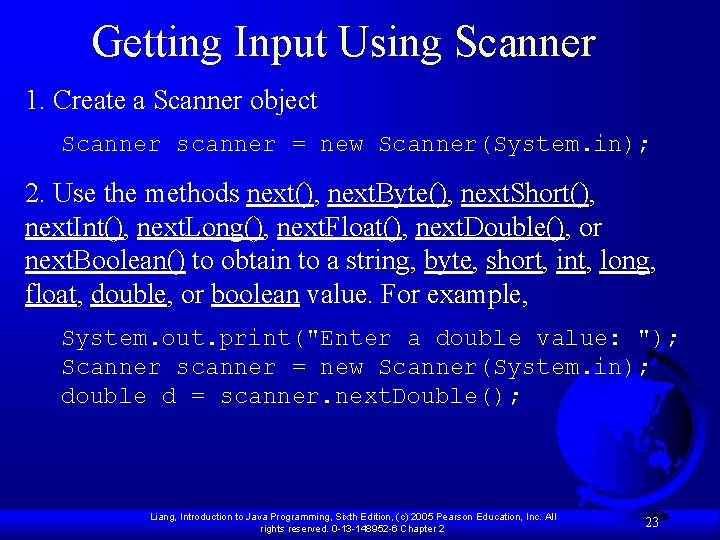
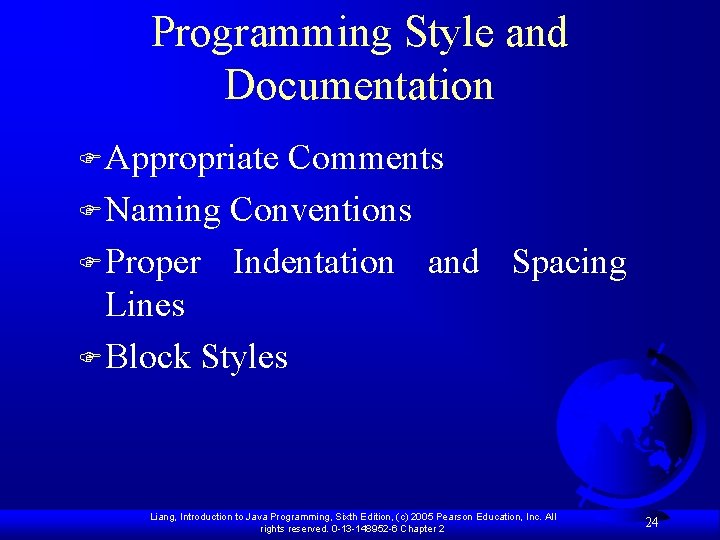
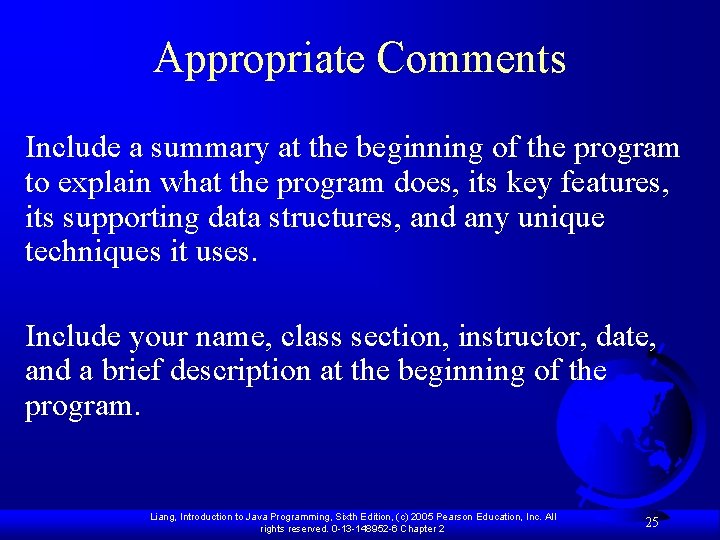
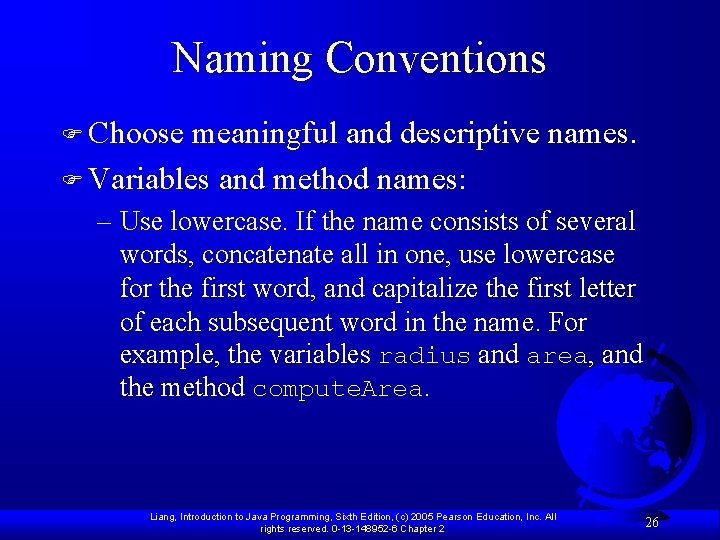
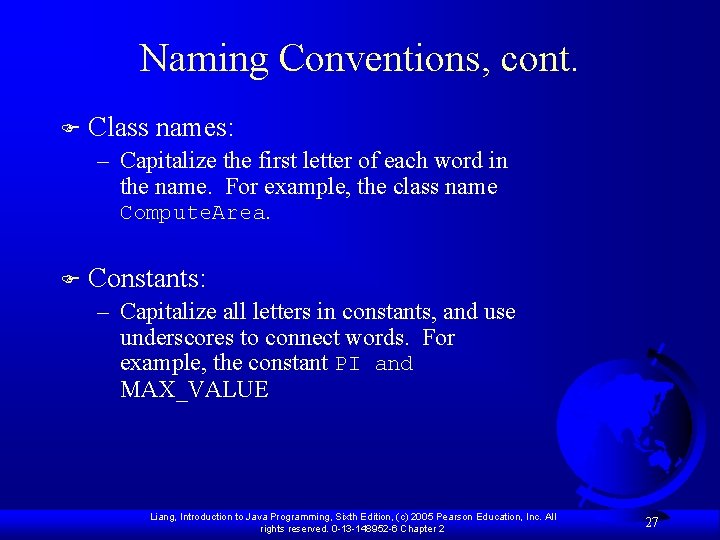
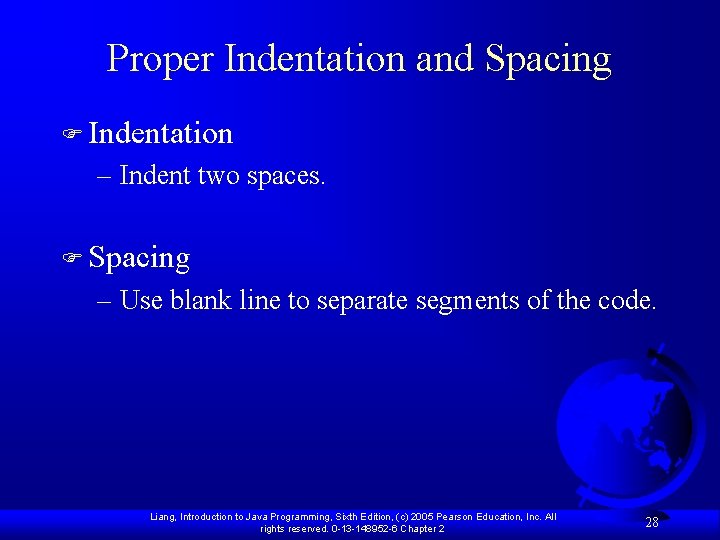
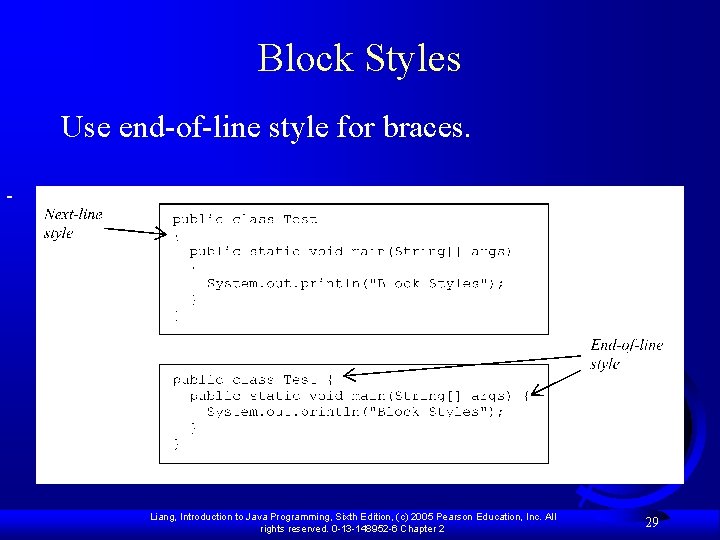
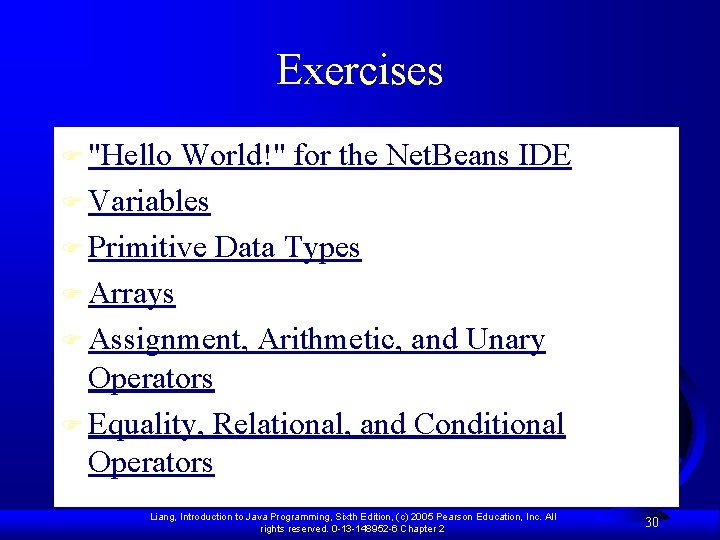
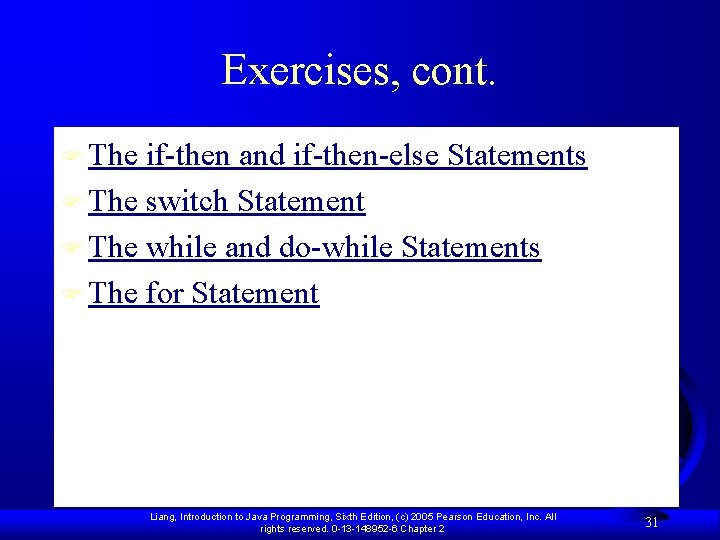
- Slides: 31
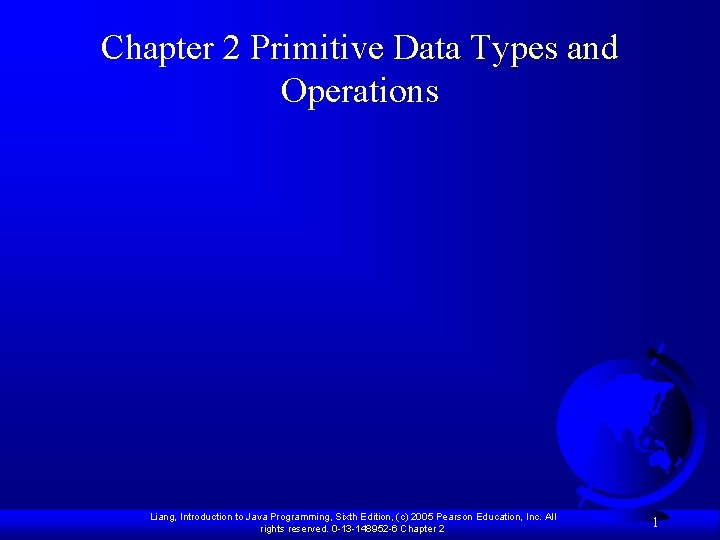
Chapter 2 Primitive Data Types and Operations Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 1
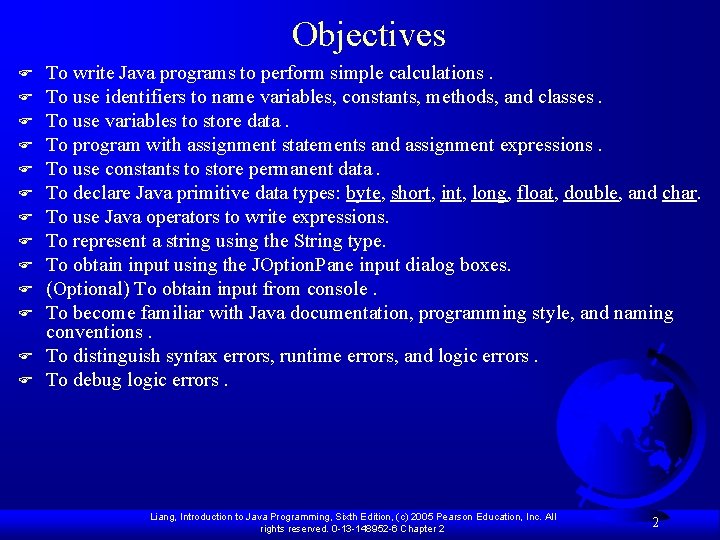
Objectives F F F F To write Java programs to perform simple calculations. To use identifiers to name variables, constants, methods, and classes. To use variables to store data. To program with assignment statements and assignment expressions. To use constants to store permanent data. To declare Java primitive data types: byte, short, int, long, float, double, and char. To use Java operators to write expressions. To represent a string using the String type. To obtain input using the JOption. Pane input dialog boxes. (Optional) To obtain input from console. To become familiar with Java documentation, programming style, and naming conventions. To distinguish syntax errors, runtime errors, and logic errors. To debug logic errors. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 2
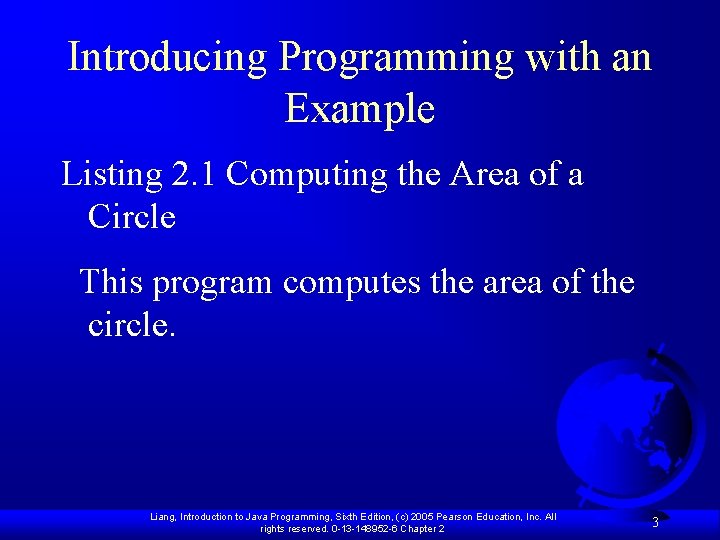
Introducing Programming with an Example Listing 2. 1 Computing the Area of a Circle This program computes the area of the circle. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 3
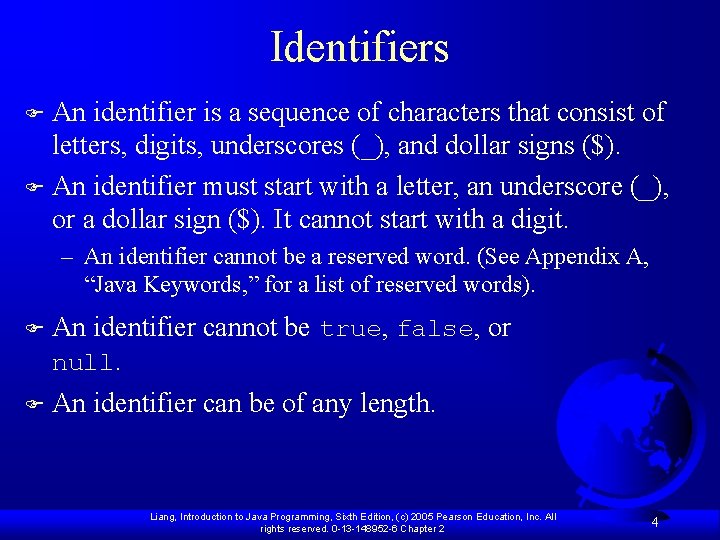
Identifiers An identifier is a sequence of characters that consist of letters, digits, underscores (_), and dollar signs ($). F An identifier must start with a letter, an underscore (_), or a dollar sign ($). It cannot start with a digit. F – An identifier cannot be a reserved word. (See Appendix A, “Java Keywords, ” for a list of reserved words). F An identifier cannot be true, false, or null. F An identifier can be of any length. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 4
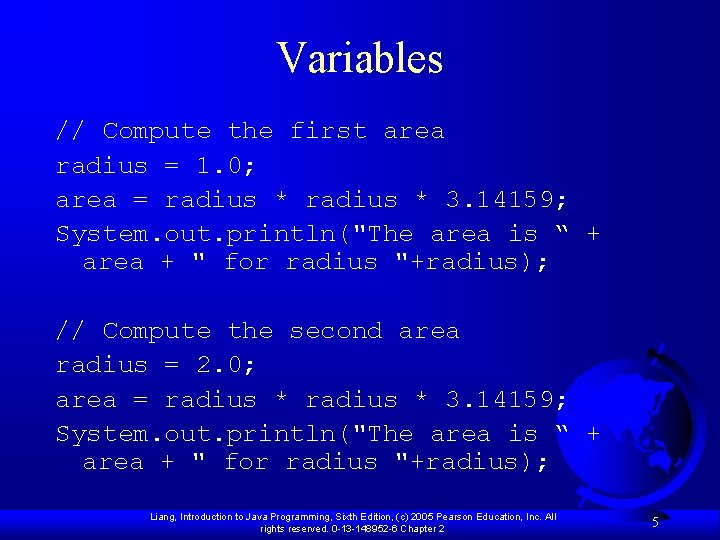
Variables // Compute the first area radius = 1. 0; area = radius * 3. 14159; System. out. println("The area is “ + area + " for radius "+radius); // Compute the second area radius = 2. 0; area = radius * 3. 14159; System. out. println("The area is “ + area + " for radius "+radius); Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 5
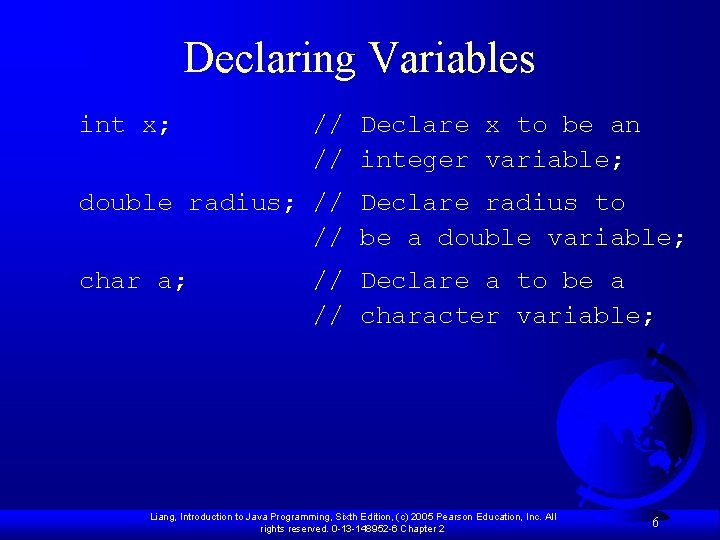
Declaring Variables int x; // Declare x to be an // integer variable; double radius; // Declare radius to // be a double variable; char a; // Declare a to be a // character variable; Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 6
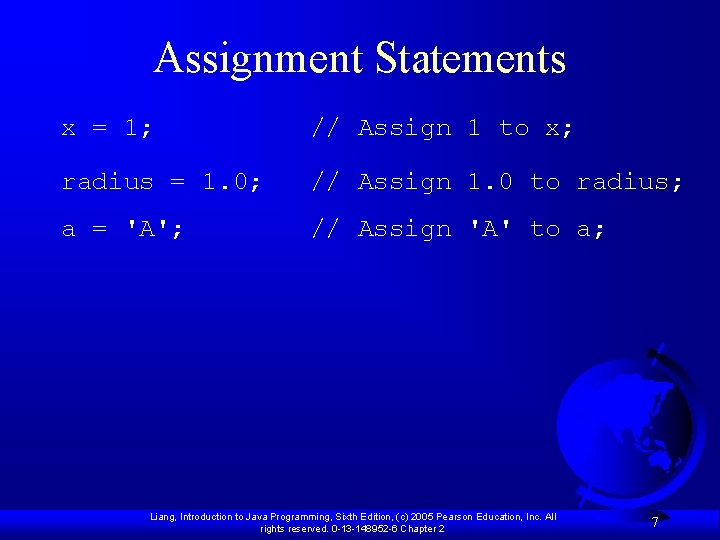
Assignment Statements x = 1; // Assign 1 to x; radius = 1. 0; // Assign 1. 0 to radius; a = 'A'; // Assign 'A' to a; Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 7
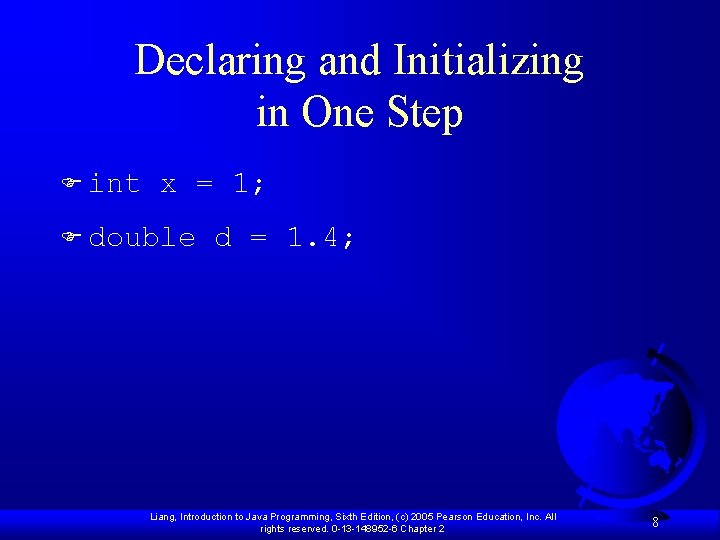
Declaring and Initializing in One Step F int x = 1; F double d = 1. 4; Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 8
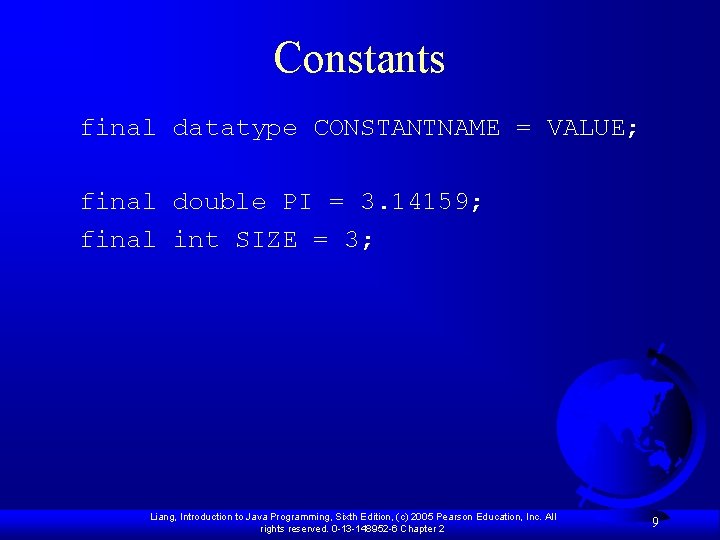
Constants final datatype CONSTANTNAME = VALUE; final double PI = 3. 14159; final int SIZE = 3; Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 9
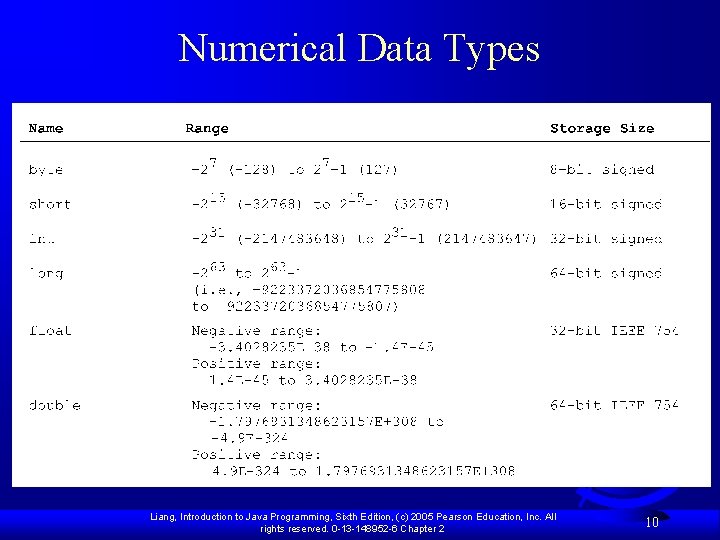
Numerical Data Types Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 10
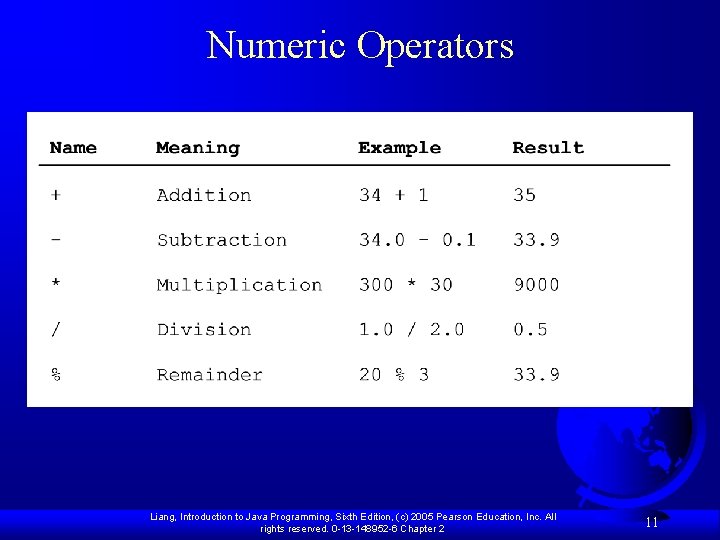
Numeric Operators Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 11
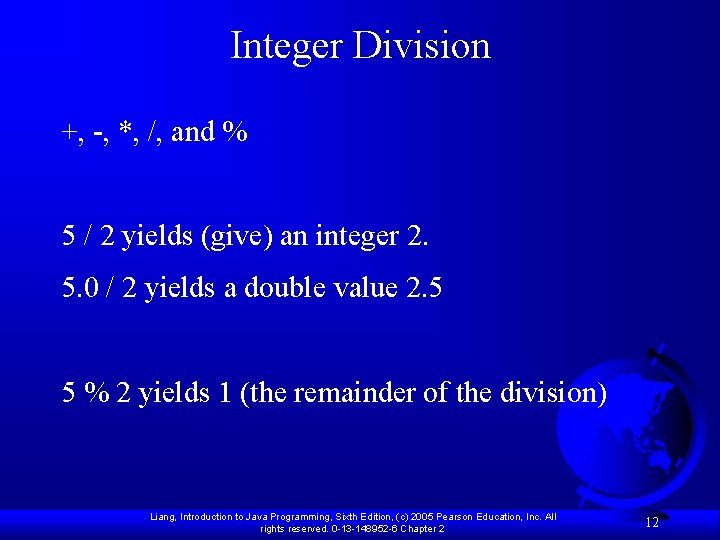
Integer Division +, -, *, /, and % 5 / 2 yields (give) an integer 2. 5. 0 / 2 yields a double value 2. 5 5 % 2 yields 1 (the remainder of the division) Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 12
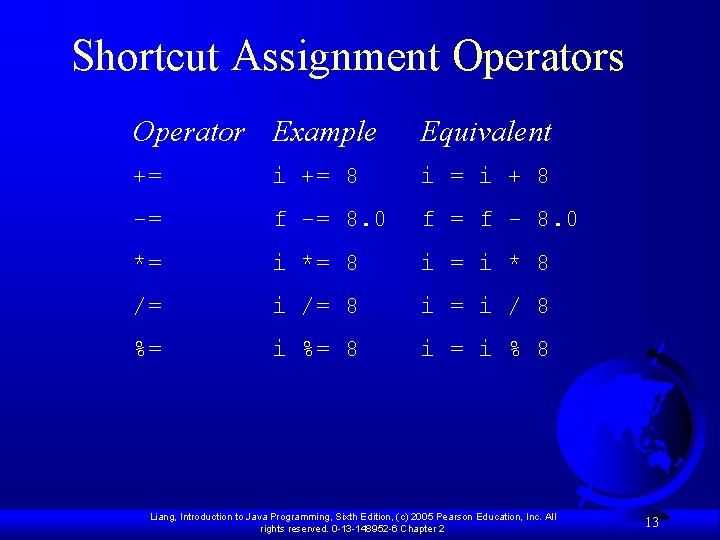
Shortcut Assignment Operators Operator Example Equivalent += i += 8 i = i + 8 -= f -= 8. 0 f = f - 8. 0 *= i *= 8 i = i * 8 /= i /= 8 i = i / 8 %= i %= 8 i = i % 8 Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 13
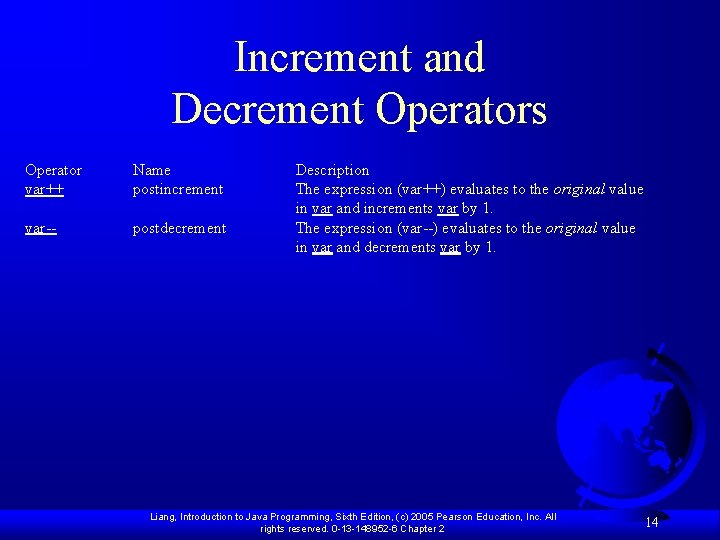
Increment and Decrement Operators Operator var++ Name postincrement var-- postdecrement Description The expression (var++) evaluates to the original value in var and increments var by 1. The expression (var--) evaluates to the original value in var and decrements var by 1. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 14
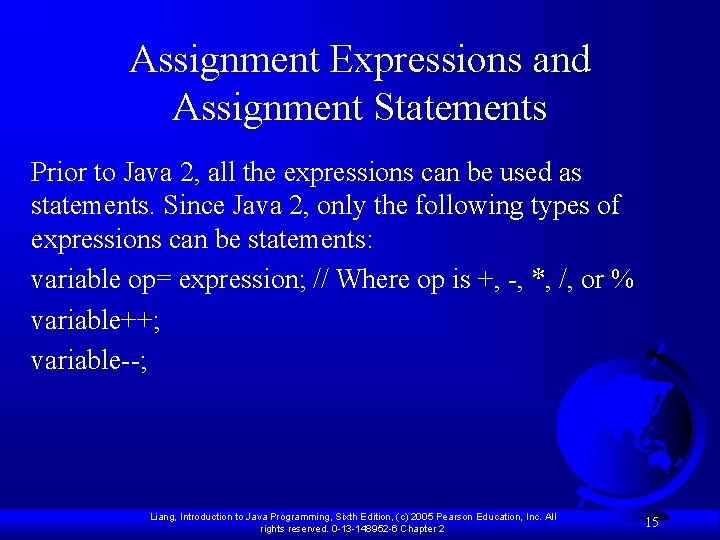
Assignment Expressions and Assignment Statements Prior to Java 2, all the expressions can be used as statements. Since Java 2, only the following types of expressions can be statements: variable op= expression; // Where op is +, -, *, /, or % variable++; variable--; Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 15
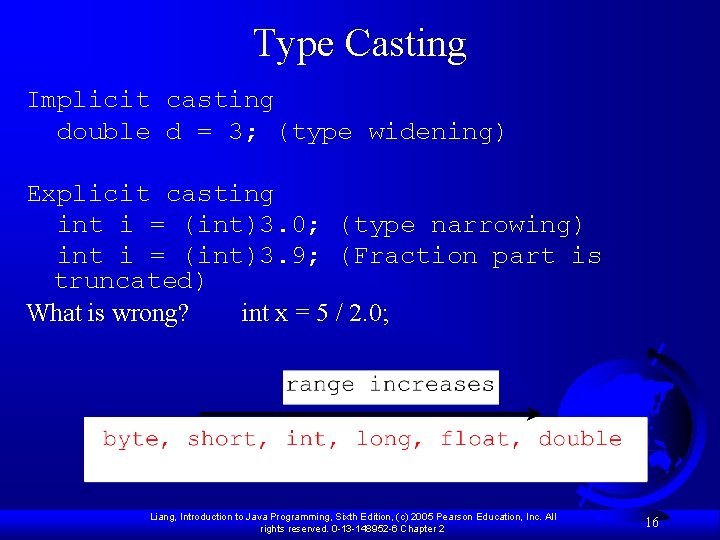
Type Casting Implicit casting double d = 3; (type widening) Explicit casting int i = (int)3. 0; (type narrowing) int i = (int)3. 9; (Fraction part is truncated) What is wrong? int x = 5 / 2. 0; Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 16
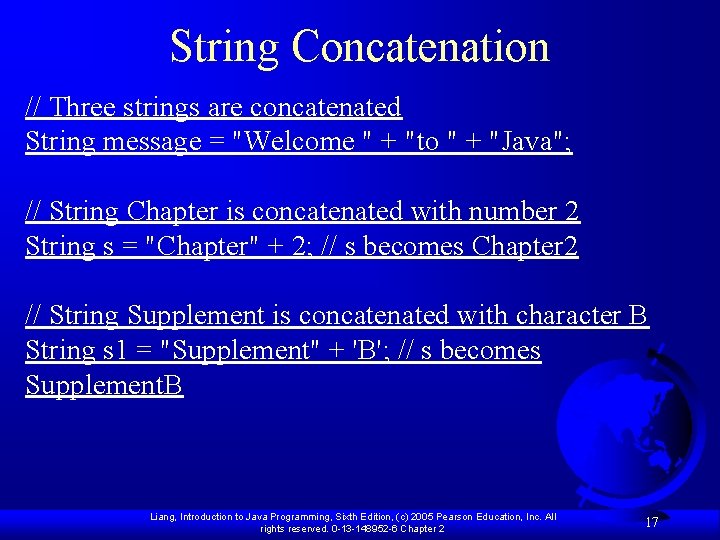
String Concatenation // Three strings are concatenated String message = "Welcome " + "to " + "Java"; // String Chapter is concatenated with number 2 String s = "Chapter" + 2; // s becomes Chapter 2 // String Supplement is concatenated with character B String s 1 = "Supplement" + 'B'; // s becomes Supplement. B Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 17
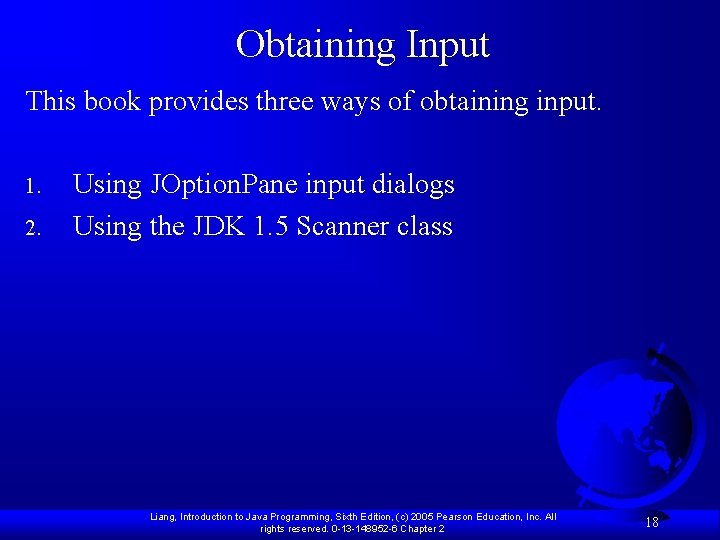
Obtaining Input This book provides three ways of obtaining input. 1. 2. Using JOption. Pane input dialogs Using the JDK 1. 5 Scanner class Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 18
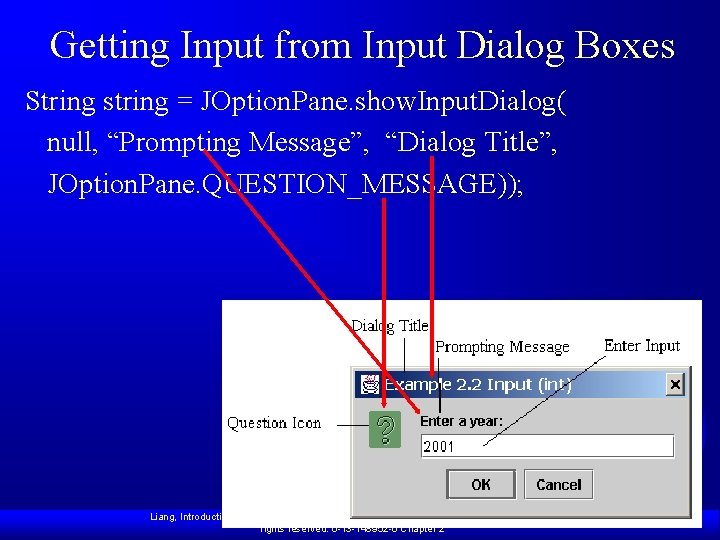
Getting Input from Input Dialog Boxes String string = JOption. Pane. show. Input. Dialog( null, “Prompting Message”, “Dialog Title”, JOption. Pane. QUESTION_MESSAGE)); Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 19
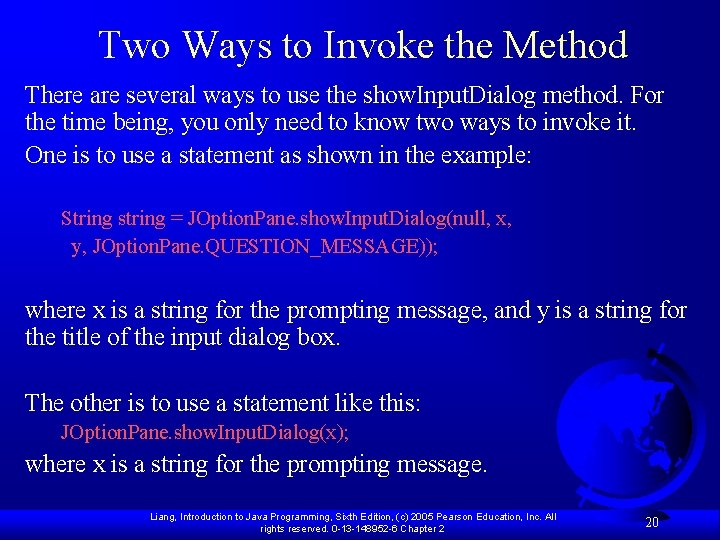
Two Ways to Invoke the Method There are several ways to use the show. Input. Dialog method. For the time being, you only need to know two ways to invoke it. One is to use a statement as shown in the example: String string = JOption. Pane. show. Input. Dialog(null, x, y, JOption. Pane. QUESTION_MESSAGE)); where x is a string for the prompting message, and y is a string for the title of the input dialog box. The other is to use a statement like this: JOption. Pane. show. Input. Dialog(x); where x is a string for the prompting message. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 20
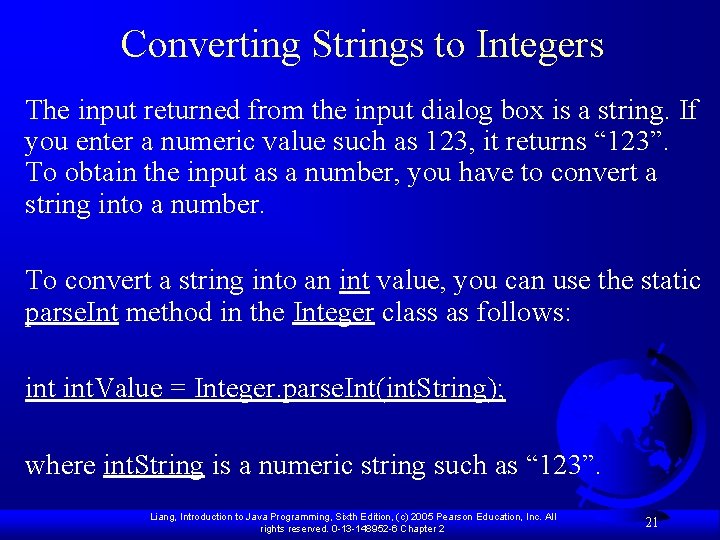
Converting Strings to Integers The input returned from the input dialog box is a string. If you enter a numeric value such as 123, it returns “ 123”. To obtain the input as a number, you have to convert a string into a number. To convert a string into an int value, you can use the static parse. Int method in the Integer class as follows: int. Value = Integer. parse. Int(int. String); where int. String is a numeric string such as “ 123”. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 21
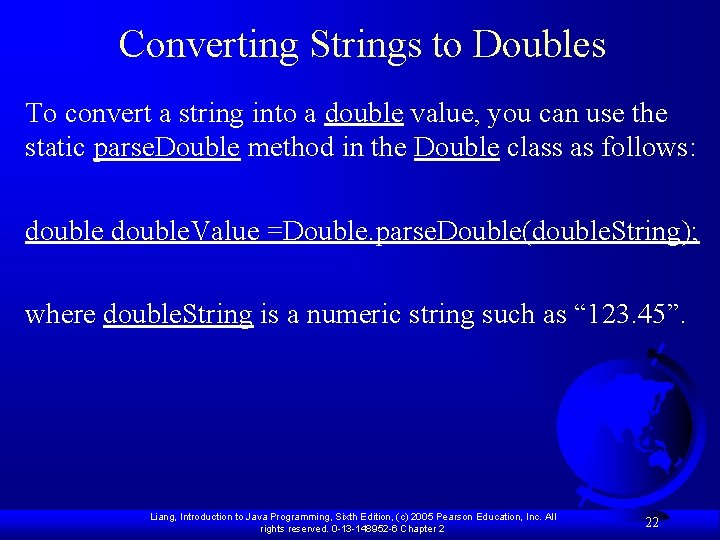
Converting Strings to Doubles To convert a string into a double value, you can use the static parse. Double method in the Double class as follows: double. Value =Double. parse. Double(double. String); where double. String is a numeric string such as “ 123. 45”. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 22
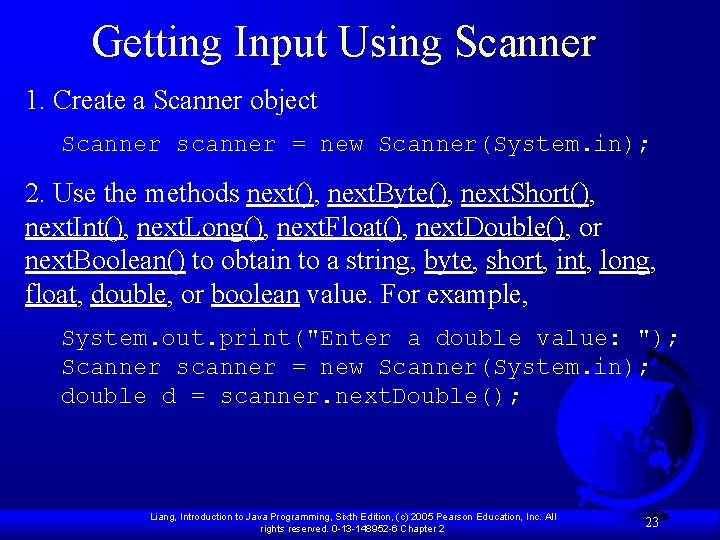
Getting Input Using Scanner 1. Create a Scanner object Scanner scanner = new Scanner(System. in); 2. Use the methods next(), next. Byte(), next. Short(), next. Int(), next. Long(), next. Float(), next. Double(), or next. Boolean() to obtain to a string, byte, short, int, long, float, double, or boolean value. For example, System. out. print("Enter a double value: "); Scanner scanner = new Scanner(System. in); double d = scanner. next. Double(); Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 23
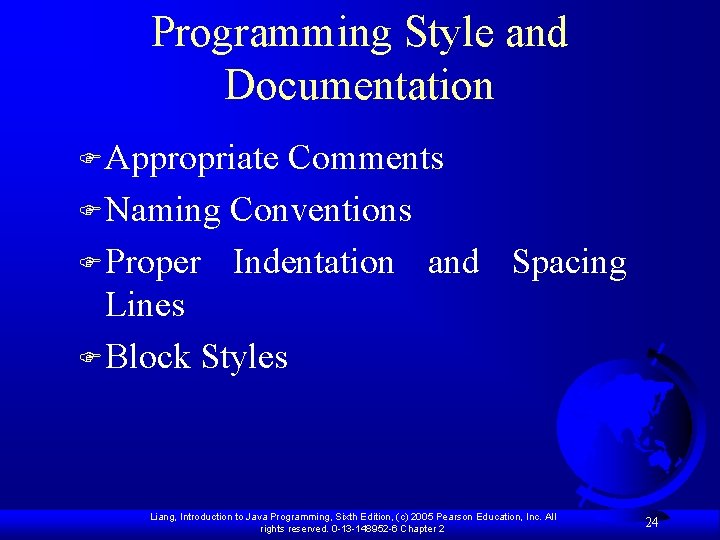
Programming Style and Documentation F Appropriate Comments F Naming Conventions F Proper Indentation and Spacing Lines F Block Styles Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 24
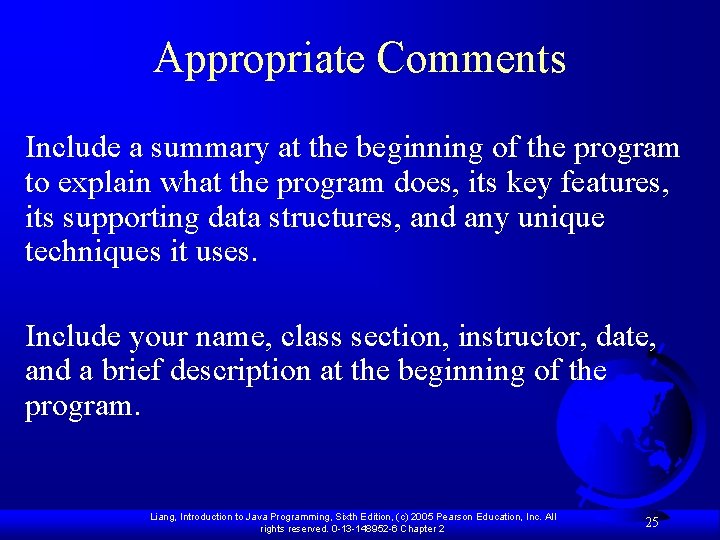
Appropriate Comments Include a summary at the beginning of the program to explain what the program does, its key features, its supporting data structures, and any unique techniques it uses. Include your name, class section, instructor, date, and a brief description at the beginning of the program. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 25
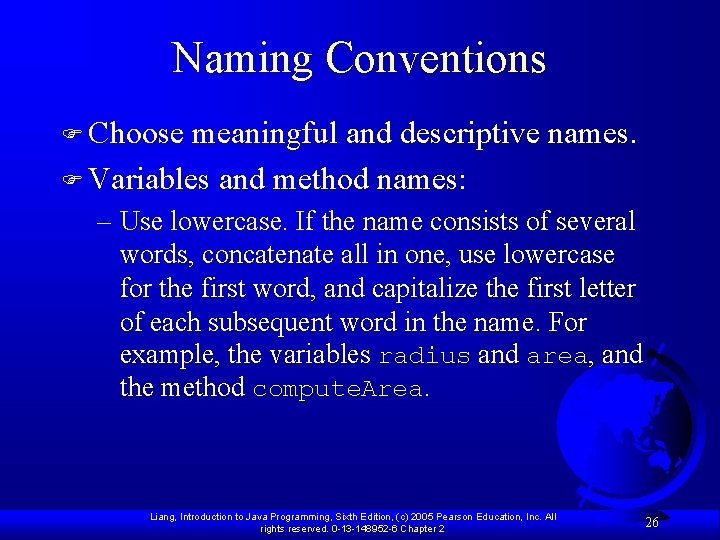
Naming Conventions F Choose meaningful and descriptive names. F Variables and method names: – Use lowercase. If the name consists of several words, concatenate all in one, use lowercase for the first word, and capitalize the first letter of each subsequent word in the name. For example, the variables radius and area, and the method compute. Area. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 26
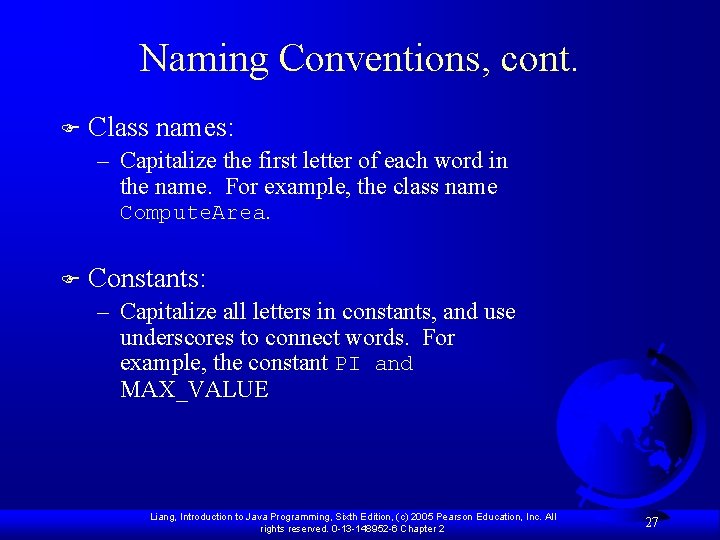
Naming Conventions, cont. F Class names: – Capitalize the first letter of each word in the name. For example, the class name Compute. Area. F Constants: – Capitalize all letters in constants, and use underscores to connect words. For example, the constant PI and MAX_VALUE Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 27
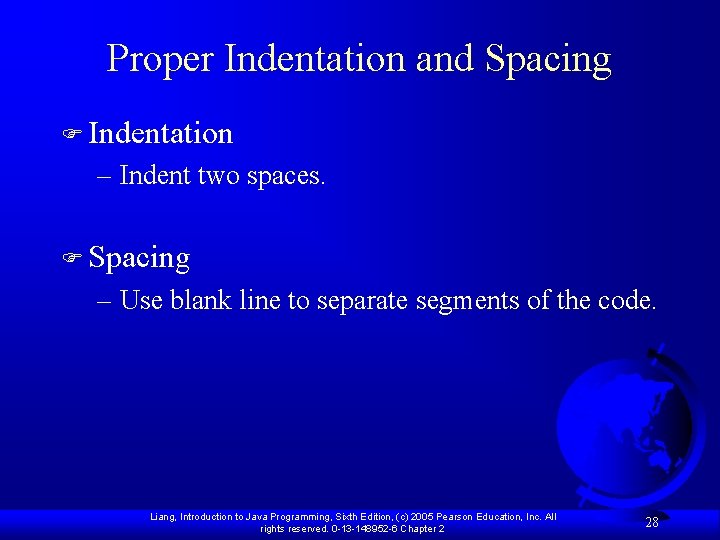
Proper Indentation and Spacing F Indentation – Indent two spaces. F Spacing – Use blank line to separate segments of the code. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 28
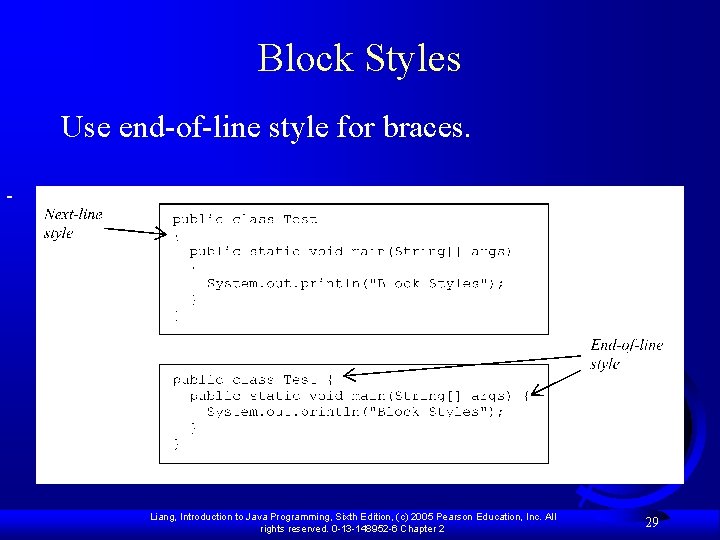
Block Styles Use end-of-line style for braces. Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 29
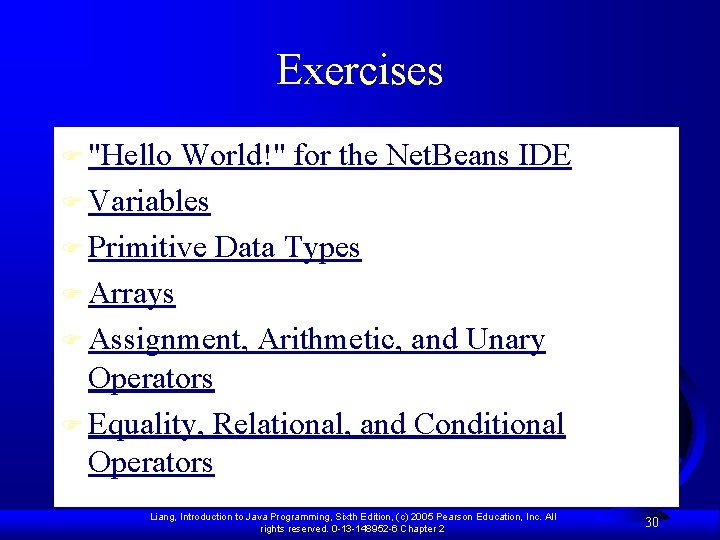
Exercises F "Hello World!" for the Net. Beans IDE F Variables F Primitive Data Types F Arrays F Assignment, Arithmetic, and Unary Operators F Equality, Relational, and Conditional Operators Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 30
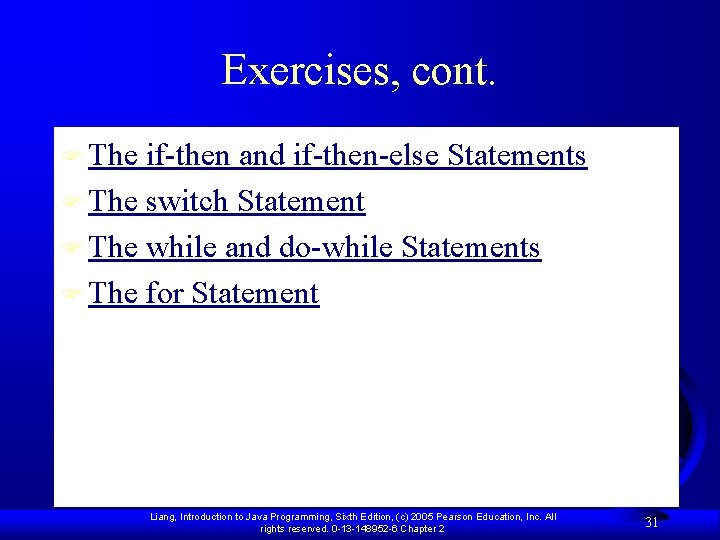
Exercises, cont. F The if-then and if-then-else Statements F The switch Statement F The while and do-while Statements F The for Statement Liang, Introduction to Java Programming, Sixth Edition, (c) 2005 Pearson Education, Inc. All rights reserved. 0 -13 -148952 -6 Chapter 2 31
Data structures unit 1
Data structure primitive and non primitive
Counting primitive operations examples
Counting primitive operations
Counting primitive operations
Richard seow yung liang
Daniel liang introduction to java programming
Zhuo shi wo li liang
Zhong hanliang
Yuanxing liang
Amber liang
Liang fontdemo.java
Liang fontdemo.java
Nama perusahaan abjad
Randy liang
David teo choon liang
How was ma liang like?
Zong-liang yang
Liang jianhui
Google zhang liang games
Chia liang cheng
Otk keuangan kelas 11
Mechanical engineering umbc
Zong-liang yang
Siemens sans bold
Dr liang hao nan
Pengertian arsip dan kearsipan
Anticorps anti-nucléaire positif moucheté
Kathleen liang
Edmund liang
Peranan sistem peredaran darah dalam pengangkutan bahan
Bob liang