presents Design Patterns in Qt 4 Alan Ezust
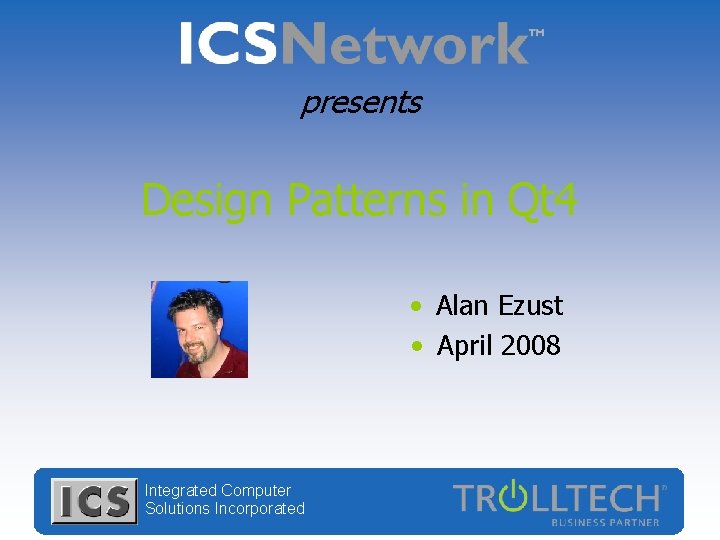
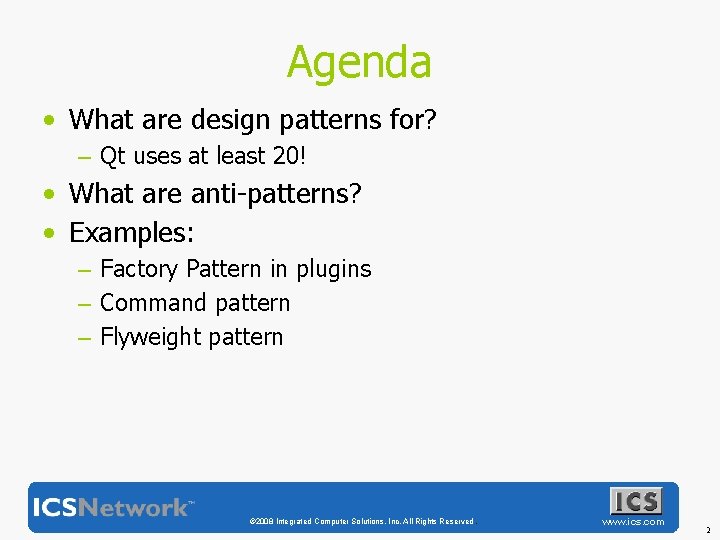
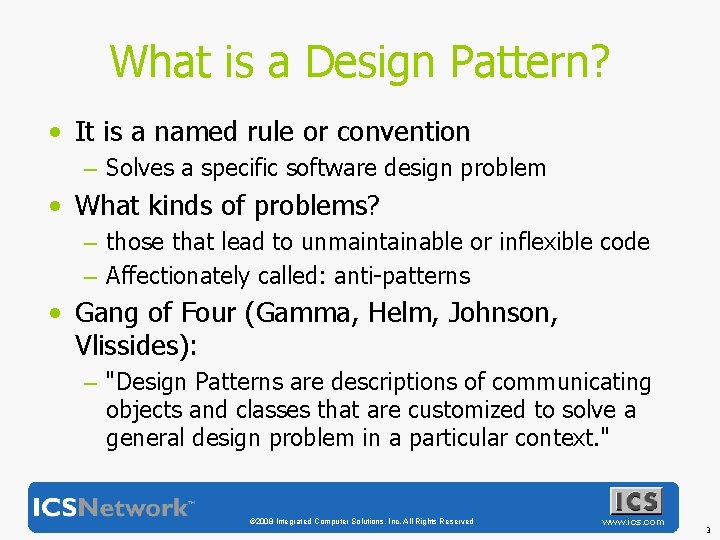
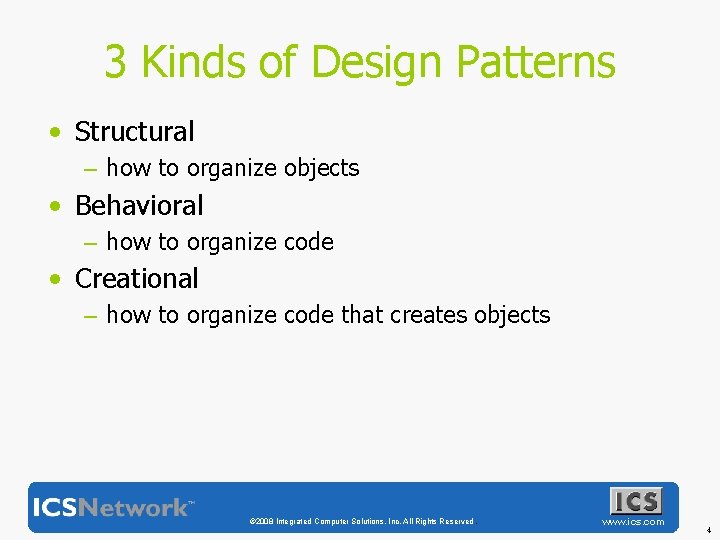
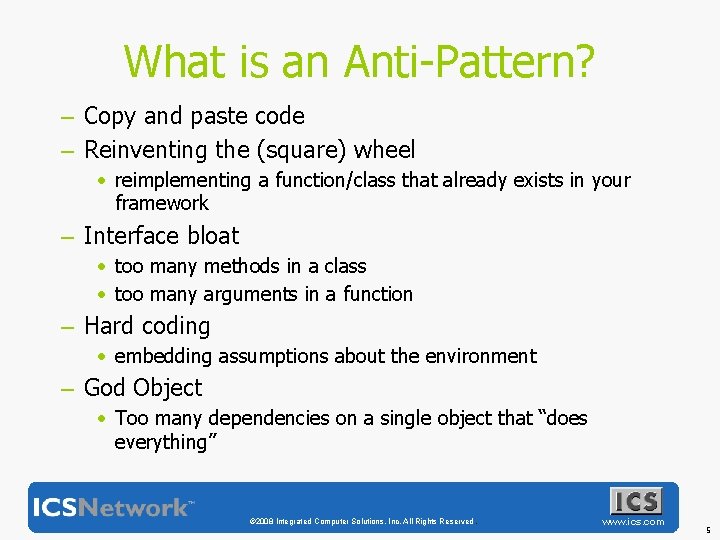
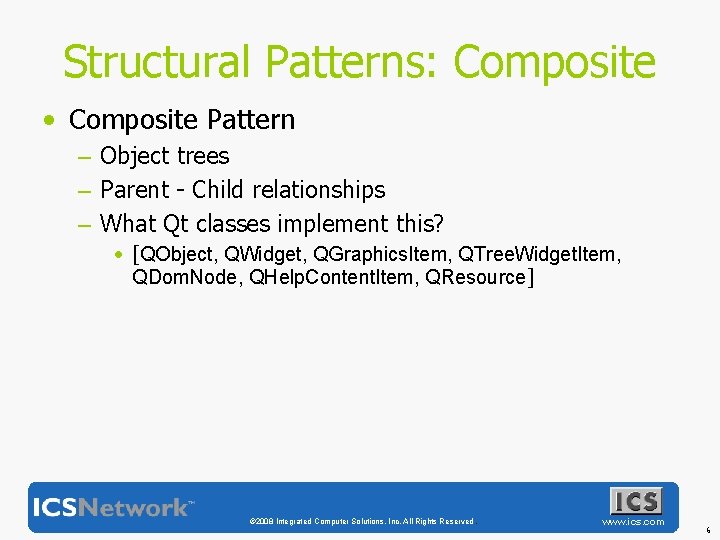
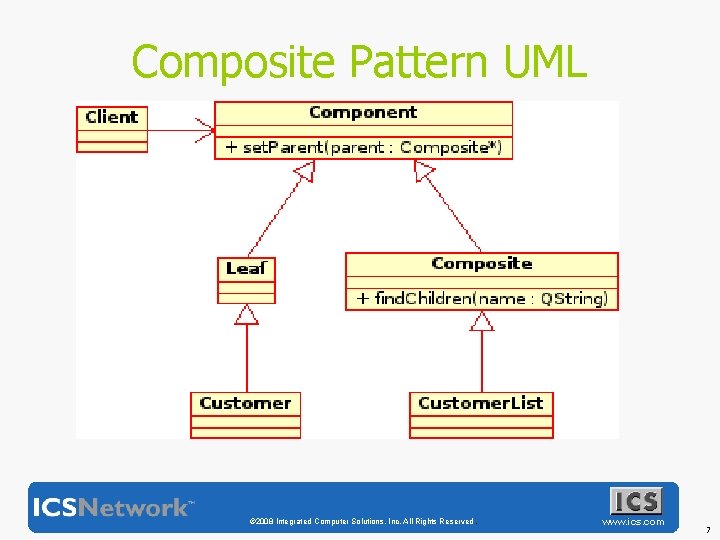
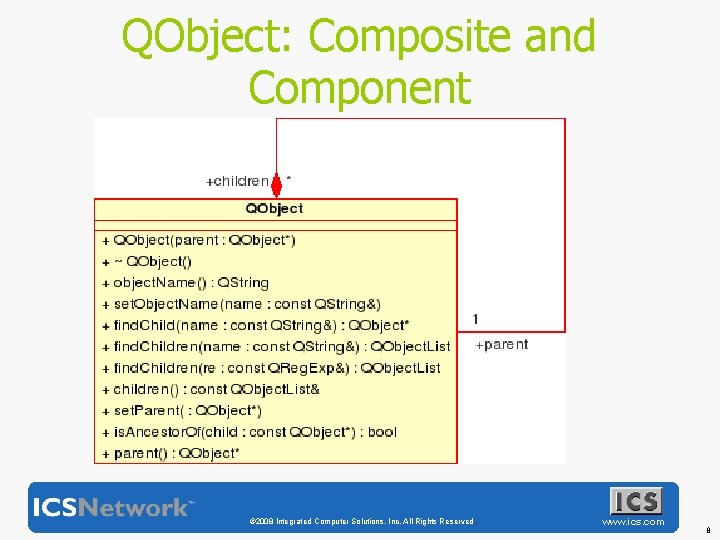
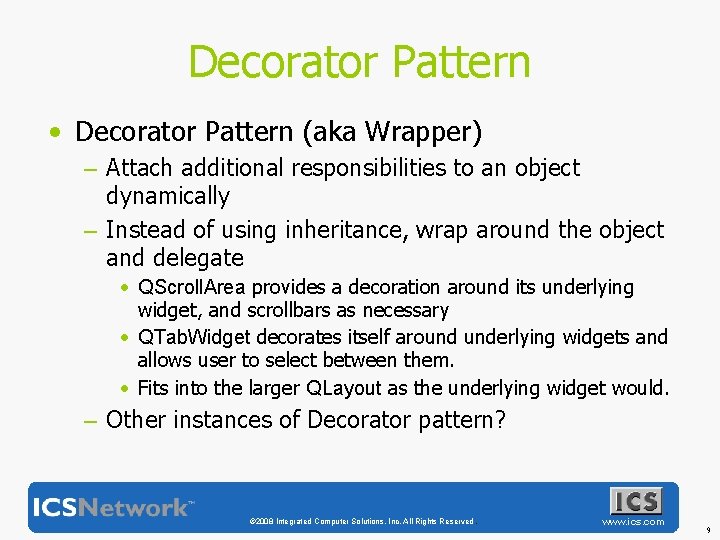
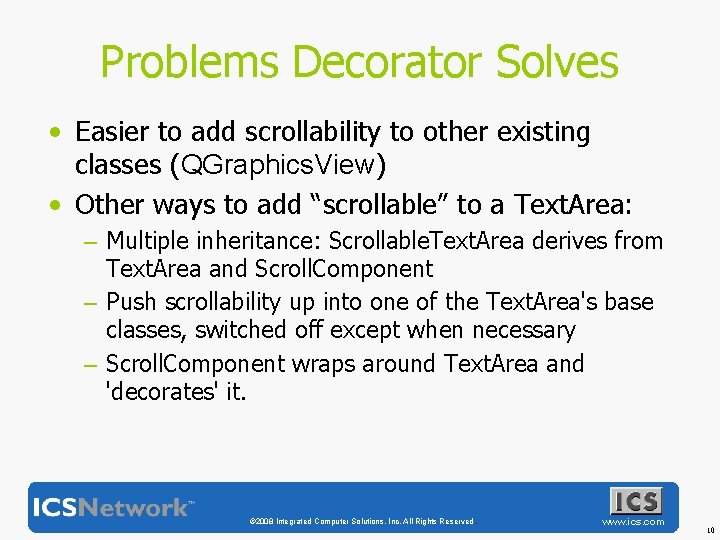
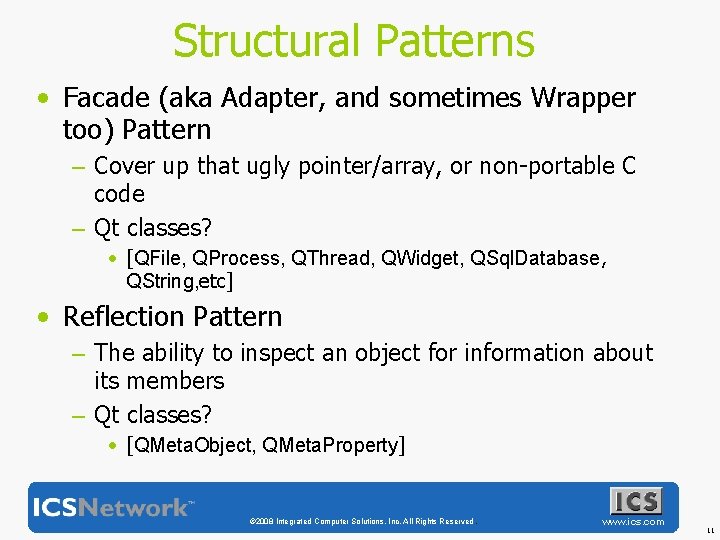
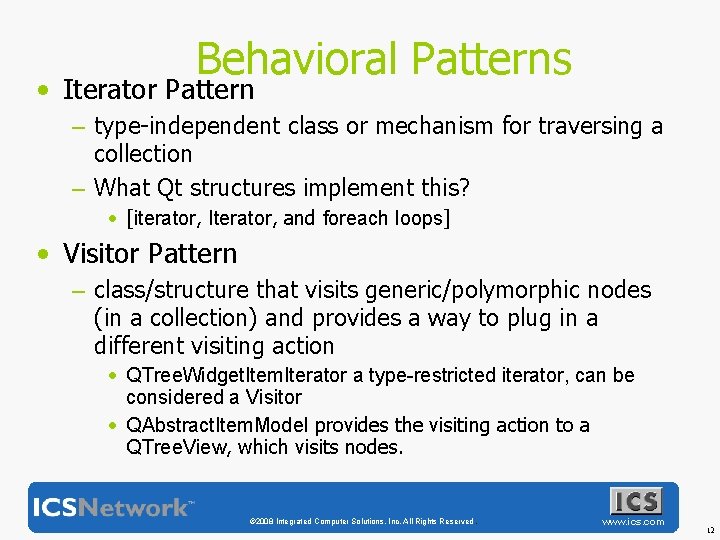
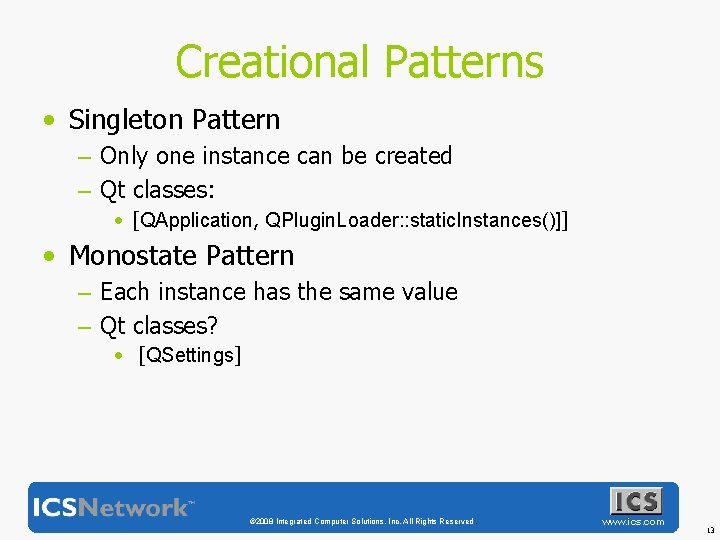
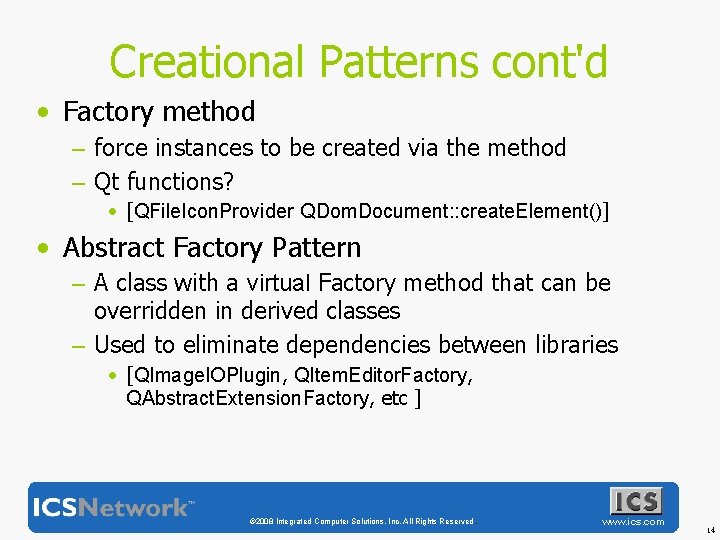
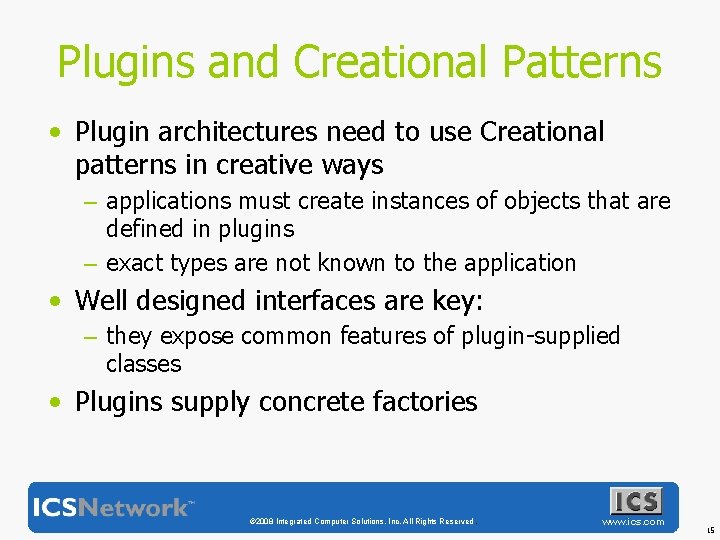
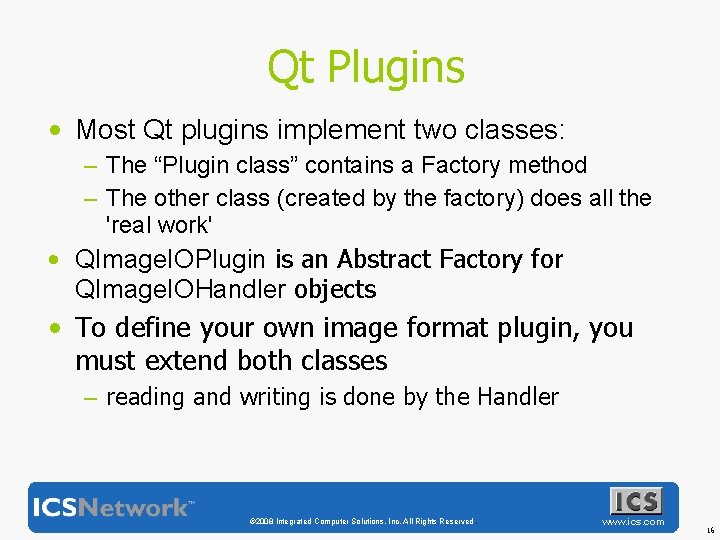
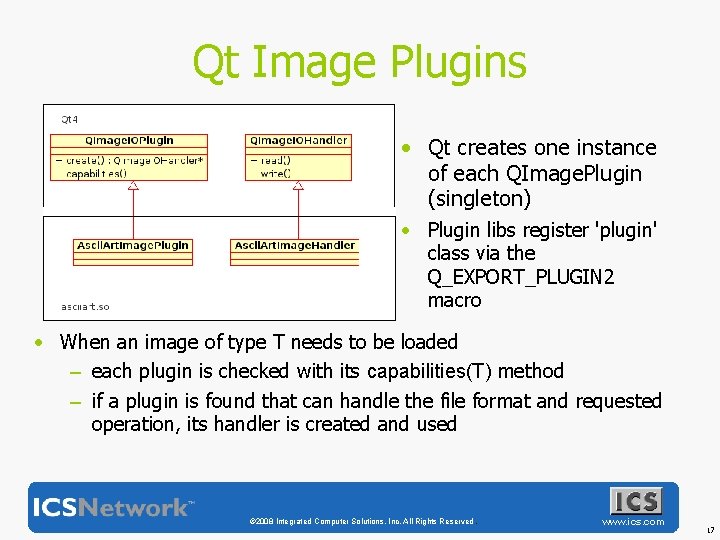
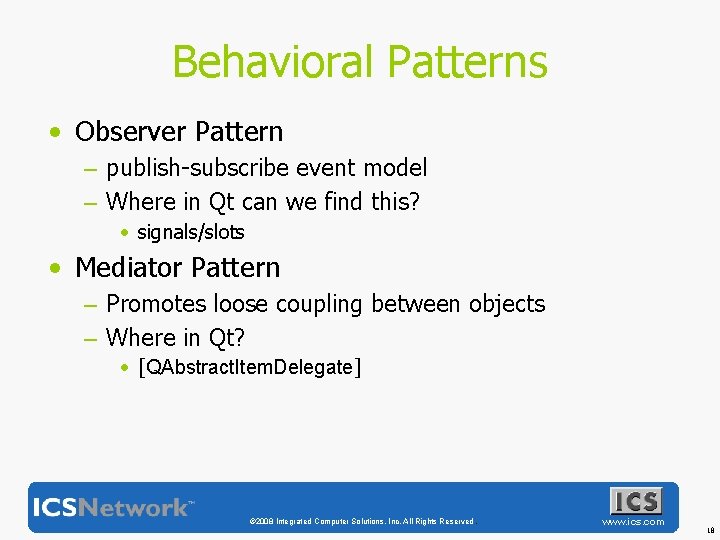
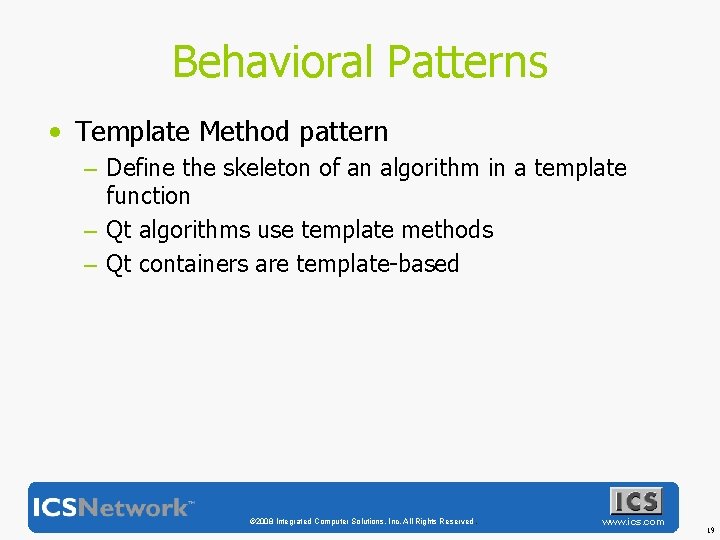
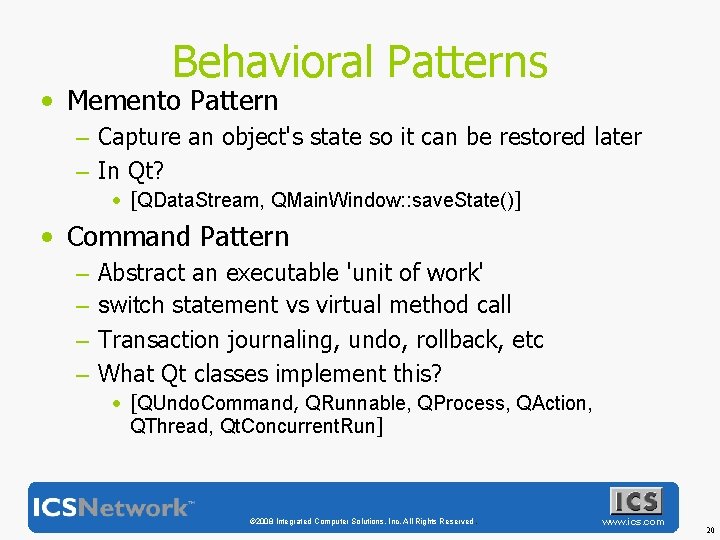
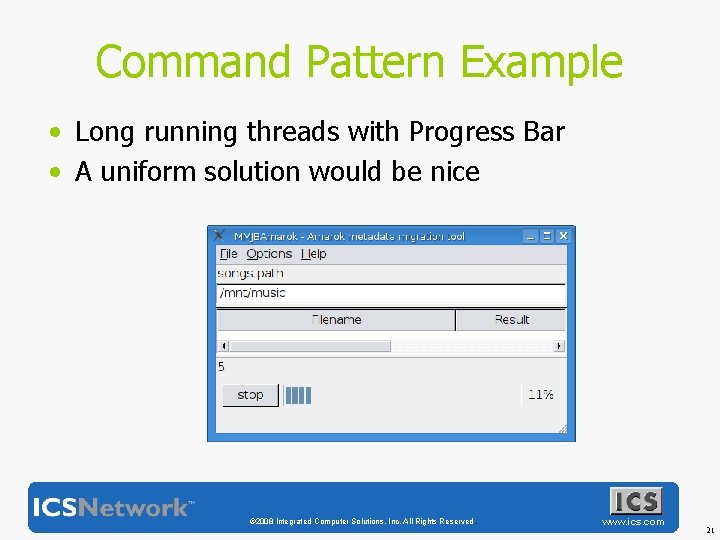
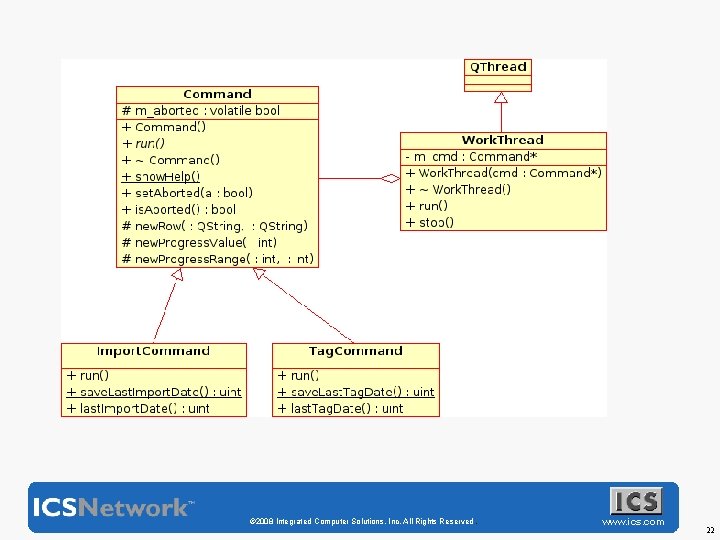
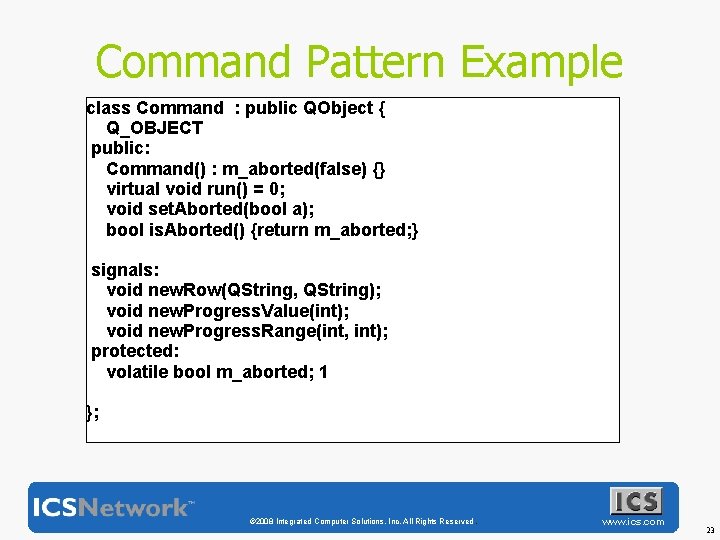
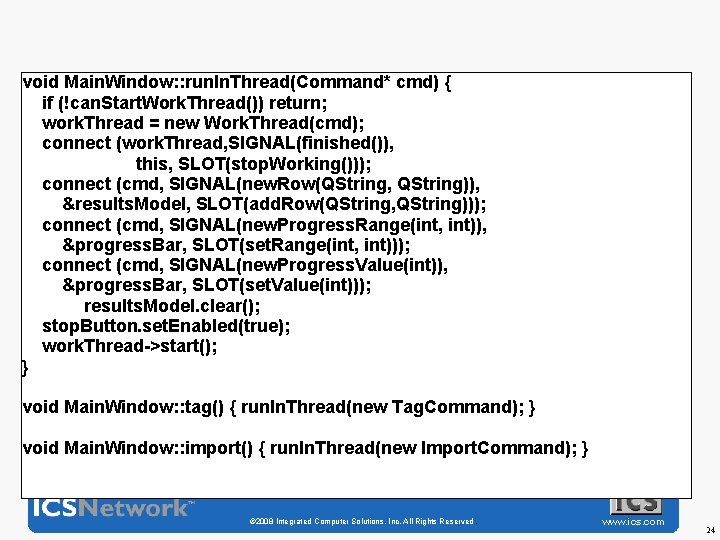
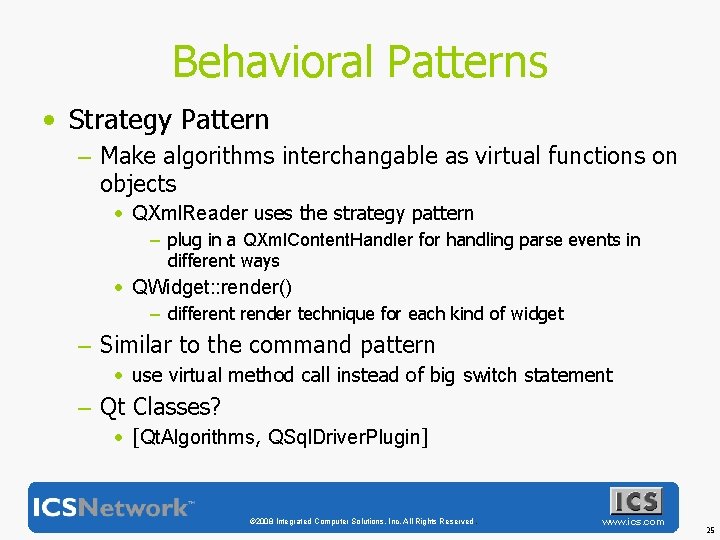
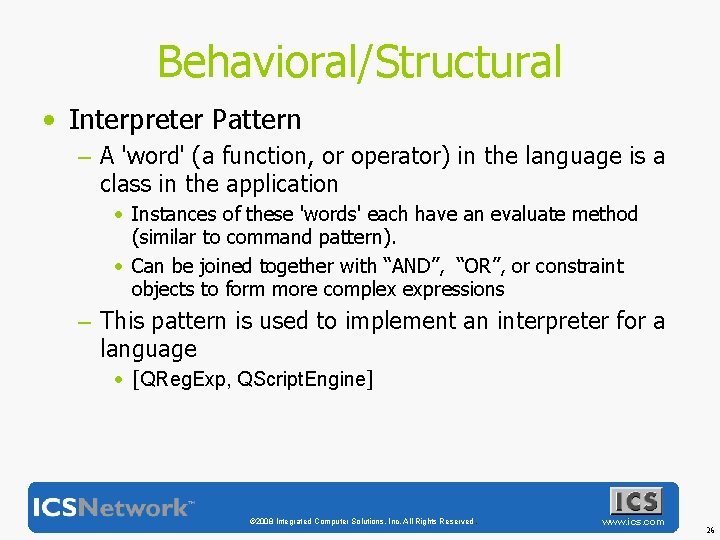
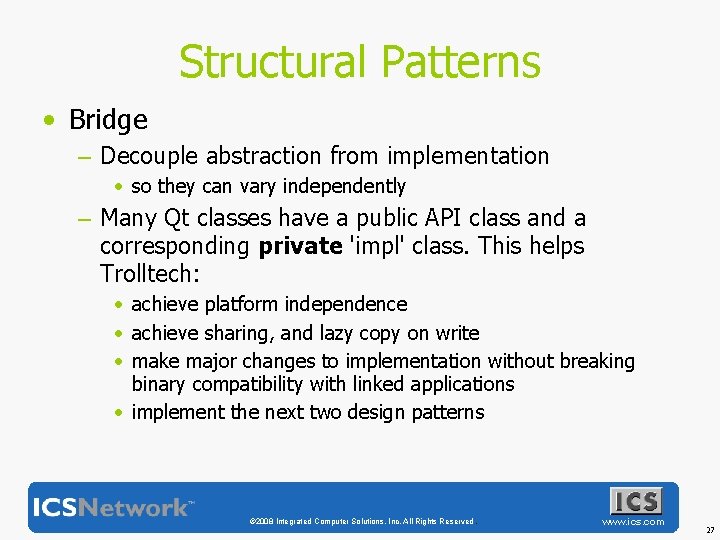
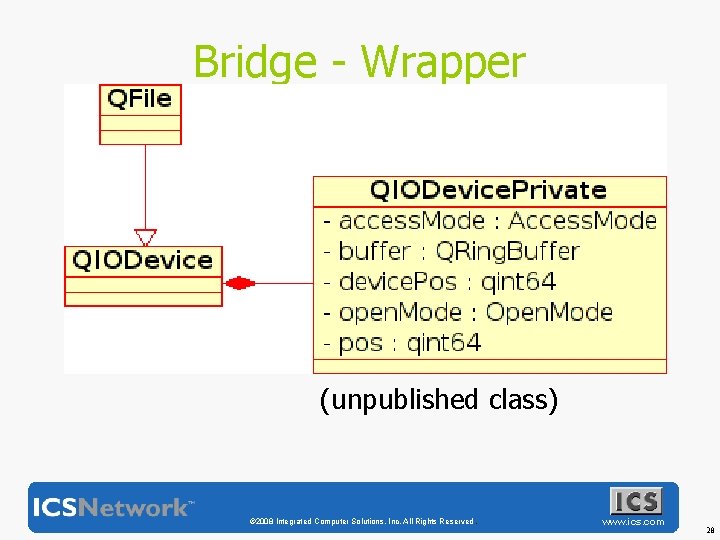
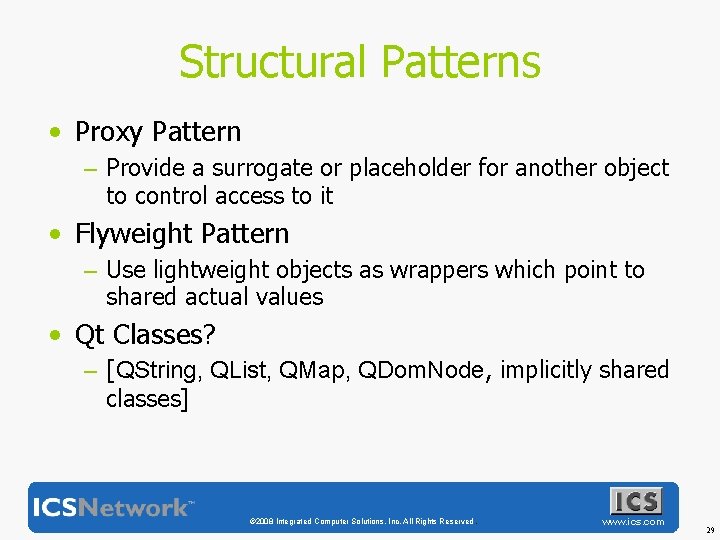
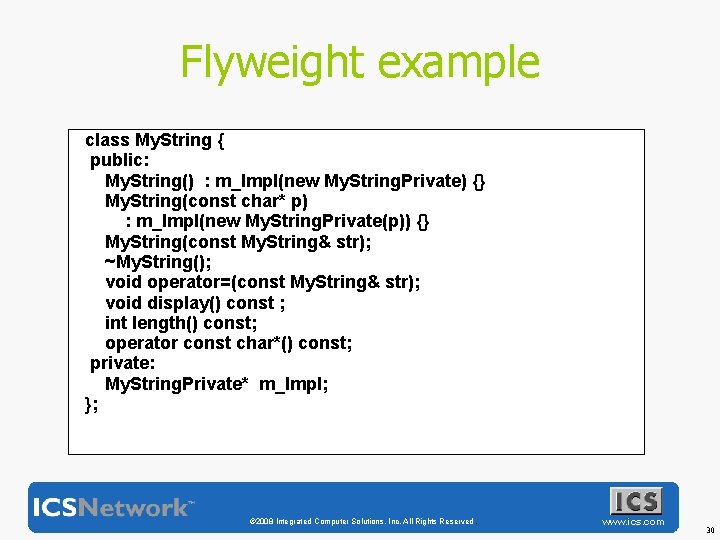
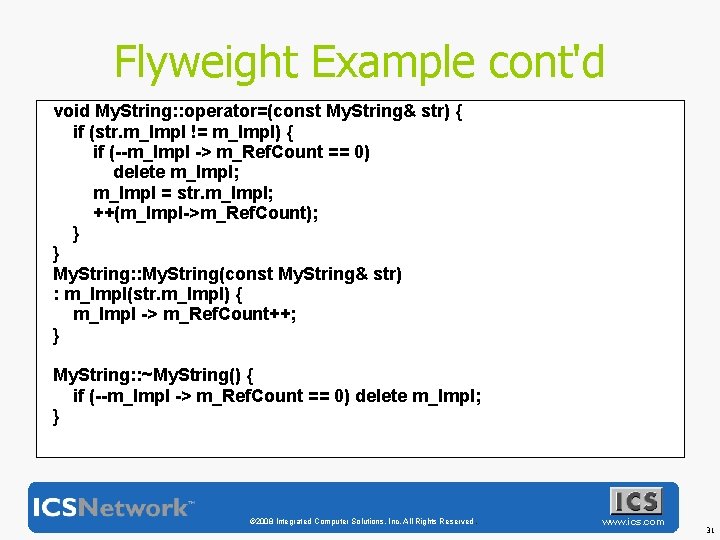
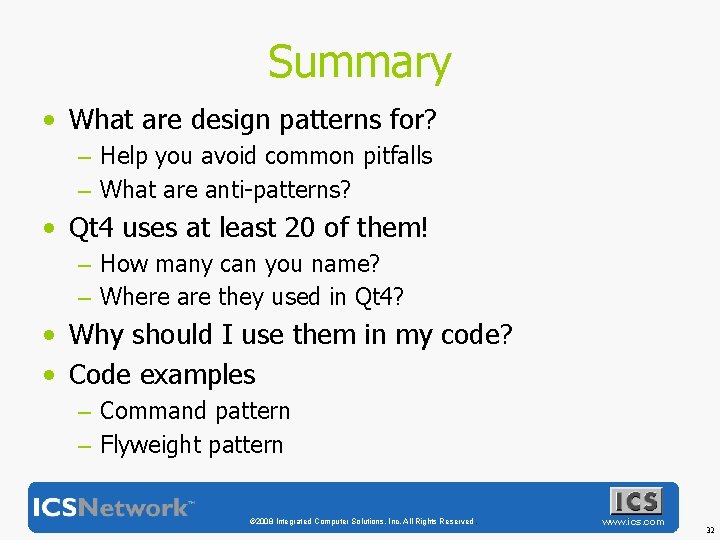
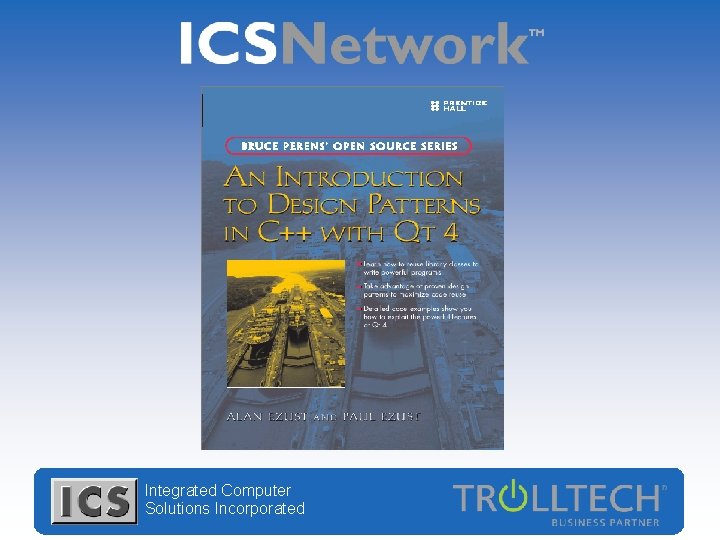
![Bibliography • [Ezust 07] Introduction to Design Patterns in C++ and Qt 4. Alan Bibliography • [Ezust 07] Introduction to Design Patterns in C++ and Qt 4. Alan](https://slidetodoc.com/presentation_image_h/0e3823ec683edc21125f0bc820195e2e/image-34.jpg)
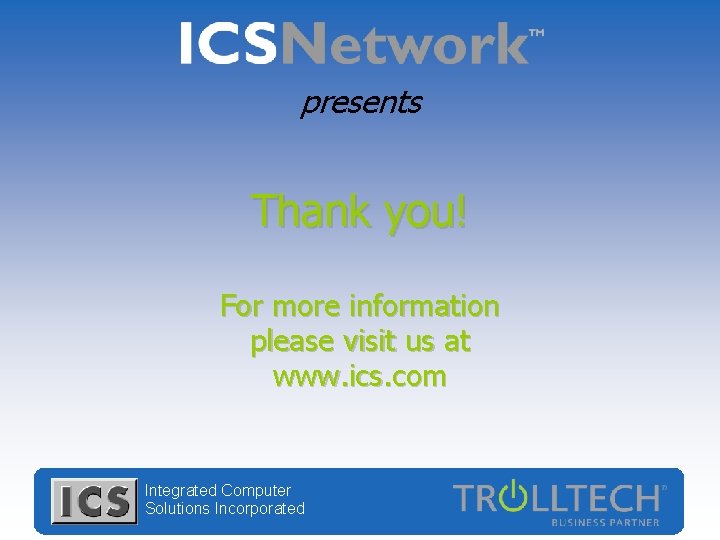
- Slides: 35
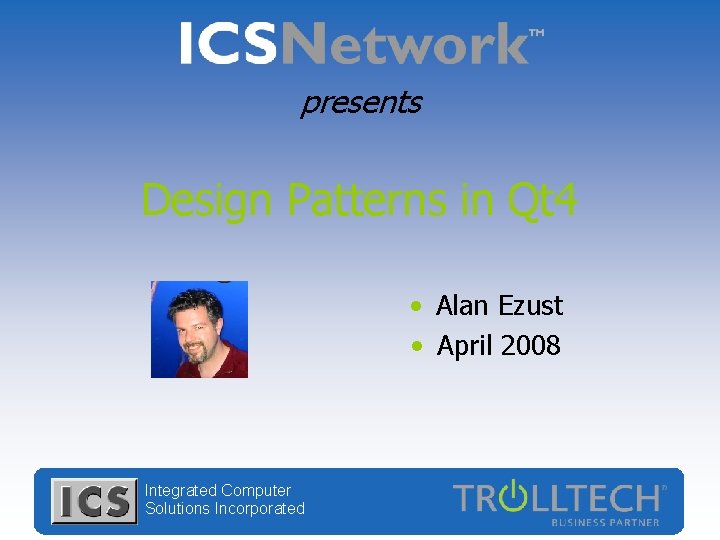
presents Design Patterns in Qt 4 • Alan Ezust • April 2008 Integrated Computer Solutions Incorporated
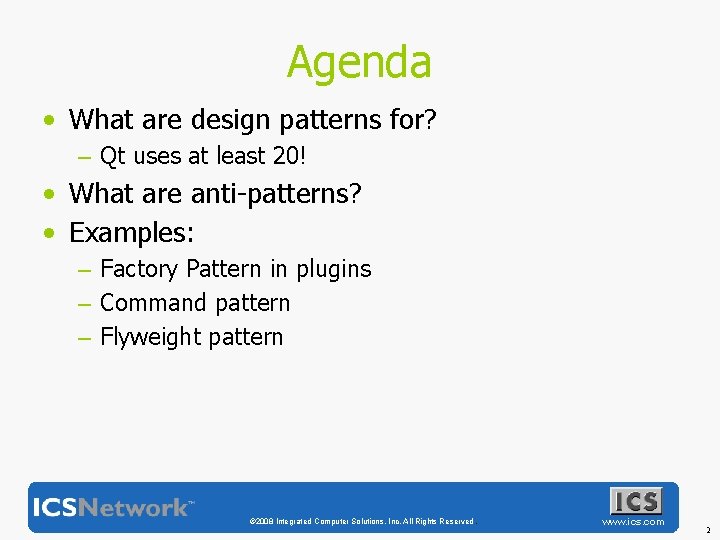
Agenda • What are design patterns for? – Qt uses at least 20! • What are anti-patterns? • Examples: – Factory Pattern in plugins – Command pattern – Flyweight pattern © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 2
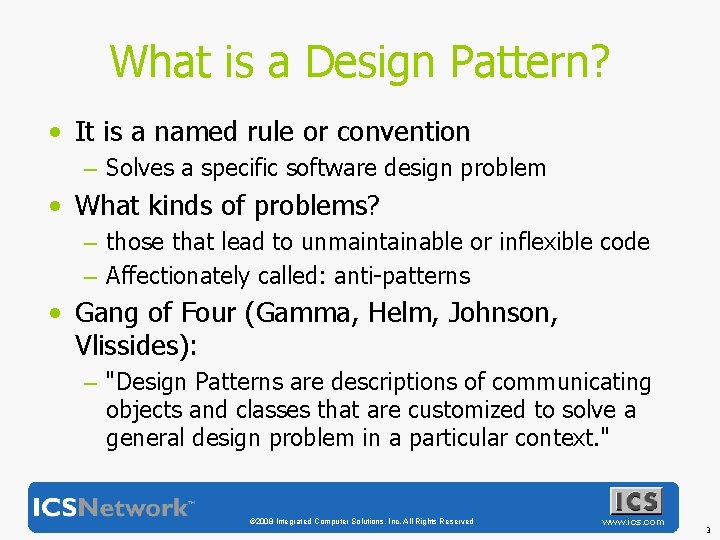
What is a Design Pattern? • It is a named rule or convention – Solves a specific software design problem • What kinds of problems? – those that lead to unmaintainable or inflexible code – Affectionately called: anti-patterns • Gang of Four (Gamma, Helm, Johnson, Vlissides): – "Design Patterns are descriptions of communicating objects and classes that are customized to solve a general design problem in a particular context. " © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 3
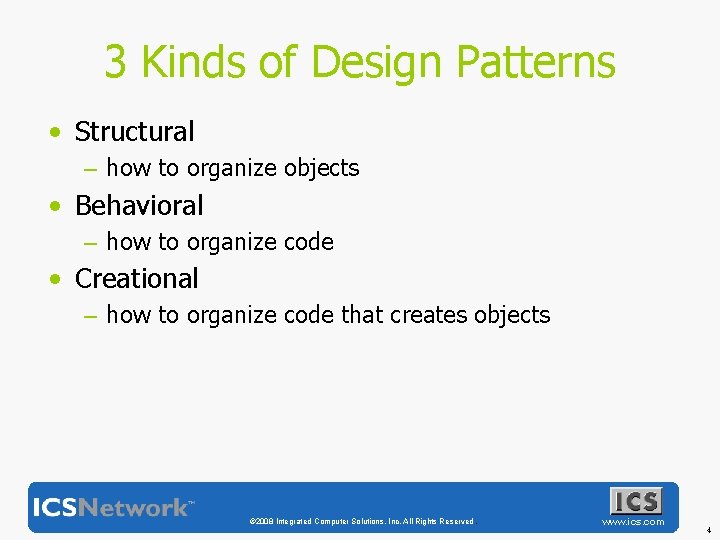
3 Kinds of Design Patterns • Structural – how to organize objects • Behavioral – how to organize code • Creational – how to organize code that creates objects © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 4
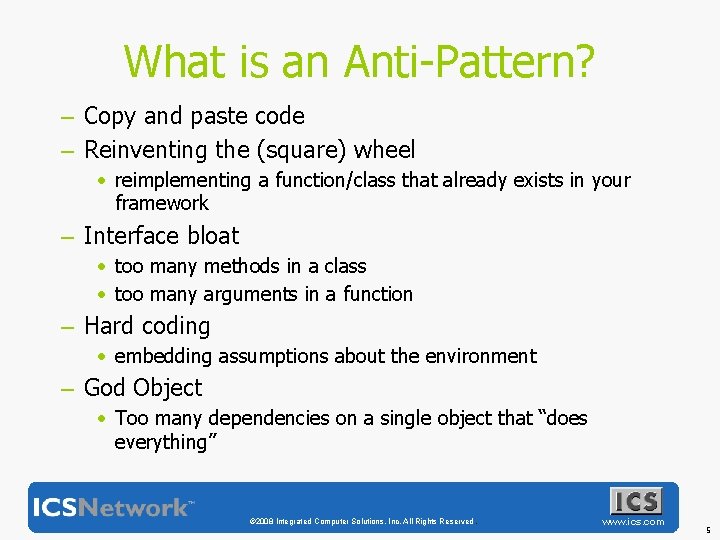
What is an Anti-Pattern? – Copy and paste code – Reinventing the (square) wheel • reimplementing a function/class that already exists in your framework – Interface bloat • too many methods in a class • too many arguments in a function – Hard coding • embedding assumptions about the environment – God Object • Too many dependencies on a single object that “does everything” © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 5
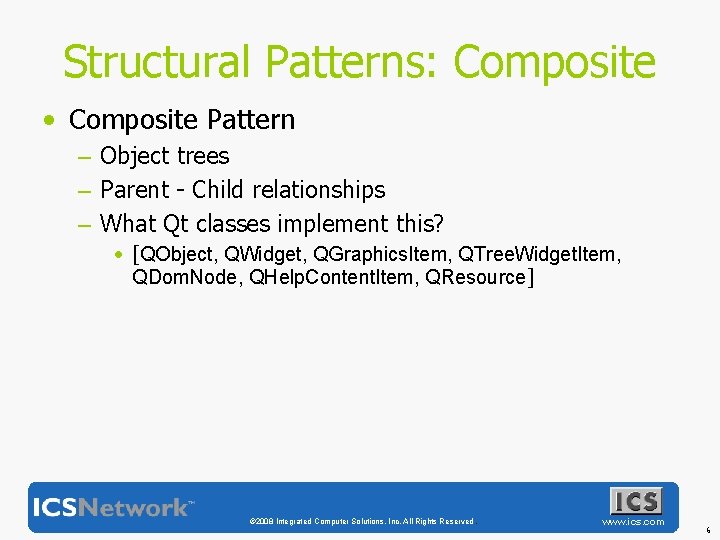
Structural Patterns: Composite • Composite Pattern – Object trees – Parent - Child relationships – What Qt classes implement this? • [QObject, QWidget, QGraphics. Item, QTree. Widget. Item, QDom. Node, QHelp. Content. Item, QResource] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 6
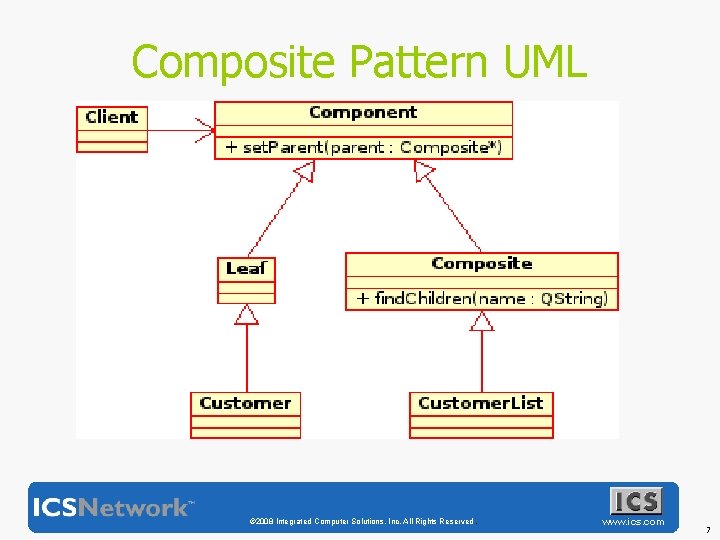
Composite Pattern UML © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 7
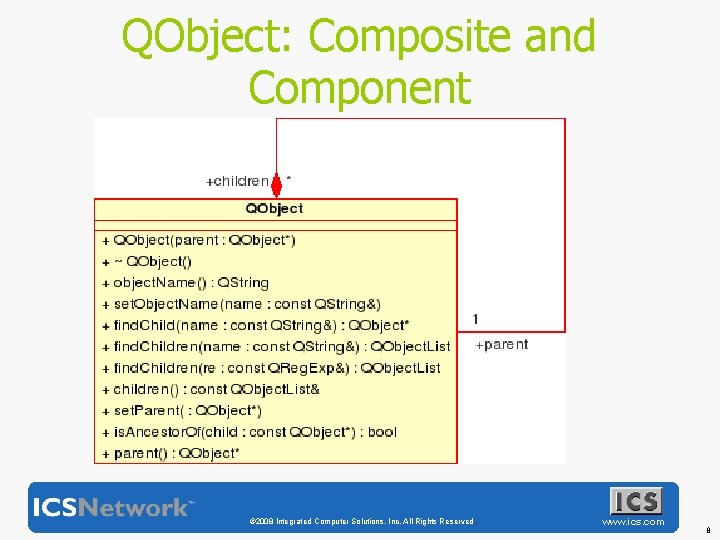
QObject: Composite and Component © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 8
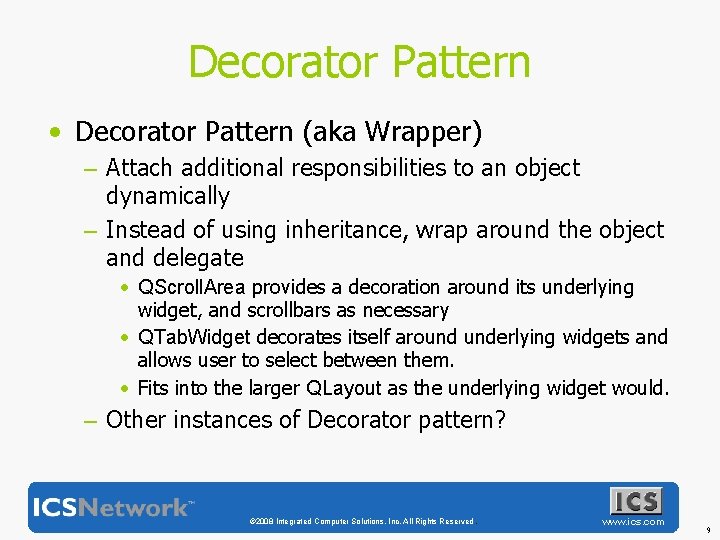
Decorator Pattern • Decorator Pattern (aka Wrapper) – Attach additional responsibilities to an object dynamically – Instead of using inheritance, wrap around the object and delegate • QScroll. Area provides a decoration around its underlying widget, and scrollbars as necessary • QTab. Widget decorates itself around underlying widgets and allows user to select between them. • Fits into the larger QLayout as the underlying widget would. – Other instances of Decorator pattern? © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 9
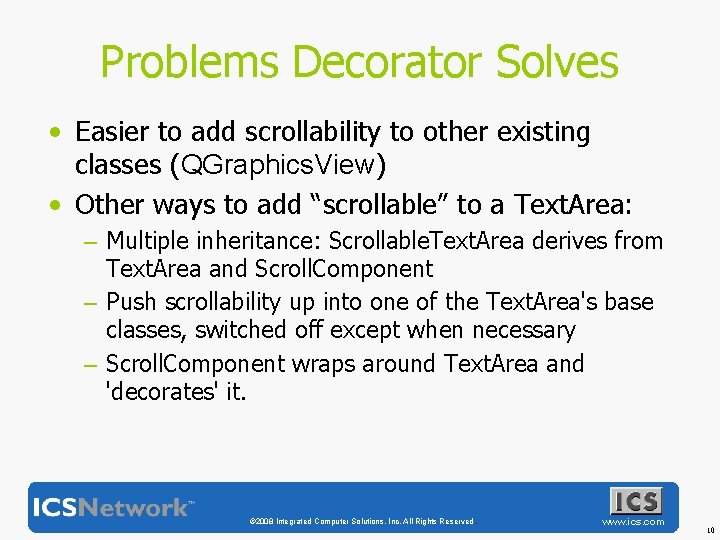
Problems Decorator Solves • Easier to add scrollability to other existing classes (QGraphics. View) • Other ways to add “scrollable” to a Text. Area: – Multiple inheritance: Scrollable. Text. Area derives from Text. Area and Scroll. Component – Push scrollability up into one of the Text. Area's base classes, switched off except when necessary – Scroll. Component wraps around Text. Area and 'decorates' it. © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 10
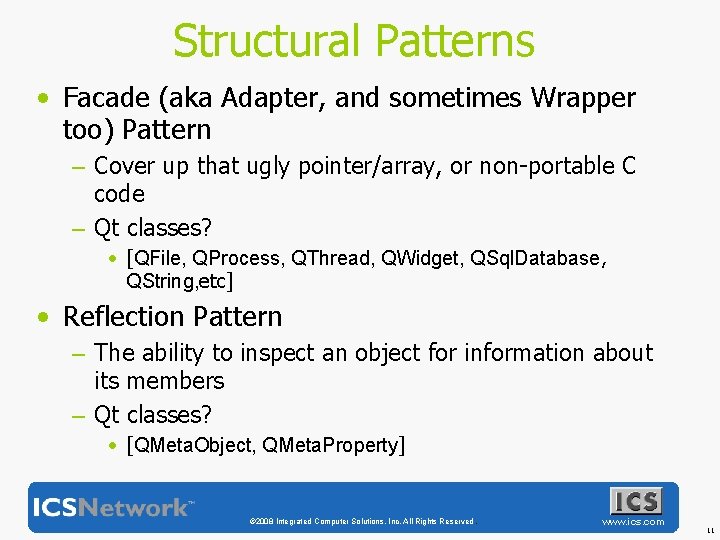
Structural Patterns • Facade (aka Adapter, and sometimes Wrapper too) Pattern – Cover up that ugly pointer/array, or non-portable C code – Qt classes? • [QFile, QProcess, QThread, QWidget, QSql. Database, QString, etc] • Reflection Pattern – The ability to inspect an object for information about its members – Qt classes? • [QMeta. Object, QMeta. Property] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 11
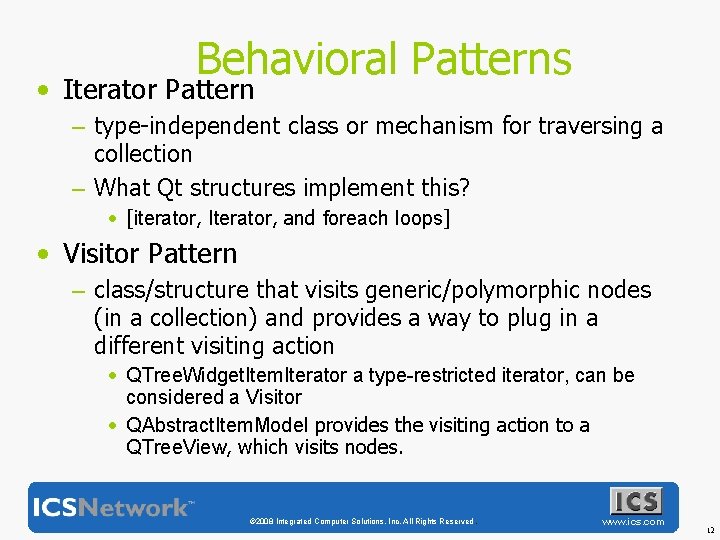
Behavioral Patterns • Iterator Pattern – type-independent class or mechanism for traversing a collection – What Qt structures implement this? • [iterator, Iterator, and foreach loops] • Visitor Pattern – class/structure that visits generic/polymorphic nodes (in a collection) and provides a way to plug in a different visiting action • QTree. Widget. Item. Iterator a type-restricted iterator, can be considered a Visitor • QAbstract. Item. Model provides the visiting action to a QTree. View, which visits nodes. © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 12
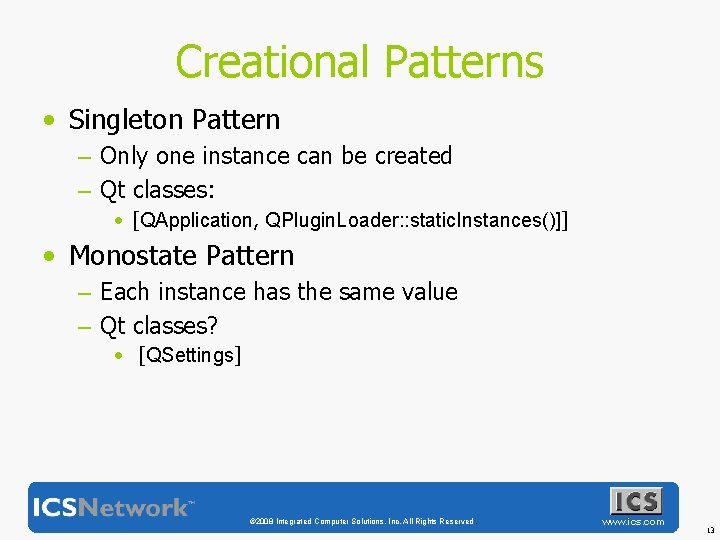
Creational Patterns • Singleton Pattern – Only one instance can be created – Qt classes: • [QApplication, QPlugin. Loader: : static. Instances()]] • Monostate Pattern – Each instance has the same value – Qt classes? • [QSettings] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 13
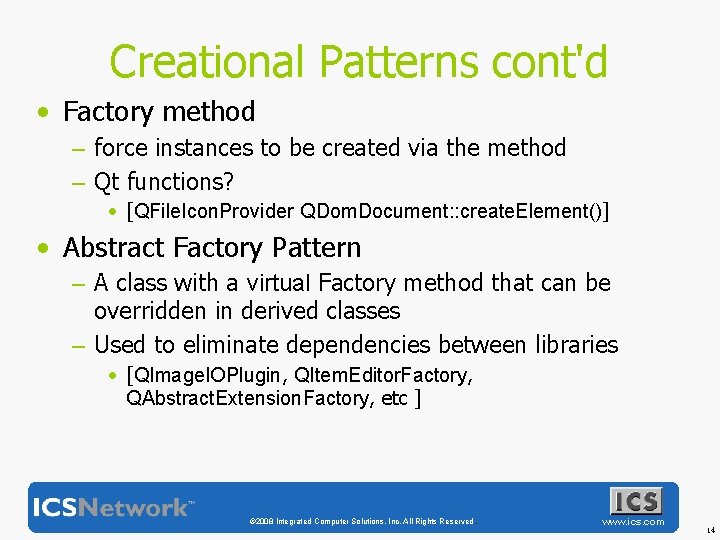
Creational Patterns cont'd • Factory method – force instances to be created via the method – Qt functions? • [QFile. Icon. Provider QDom. Document: : create. Element()] • Abstract Factory Pattern – A class with a virtual Factory method that can be overridden in derived classes – Used to eliminate dependencies between libraries • [QImage. IOPlugin, QItem. Editor. Factory, QAbstract. Extension. Factory, etc ] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 14
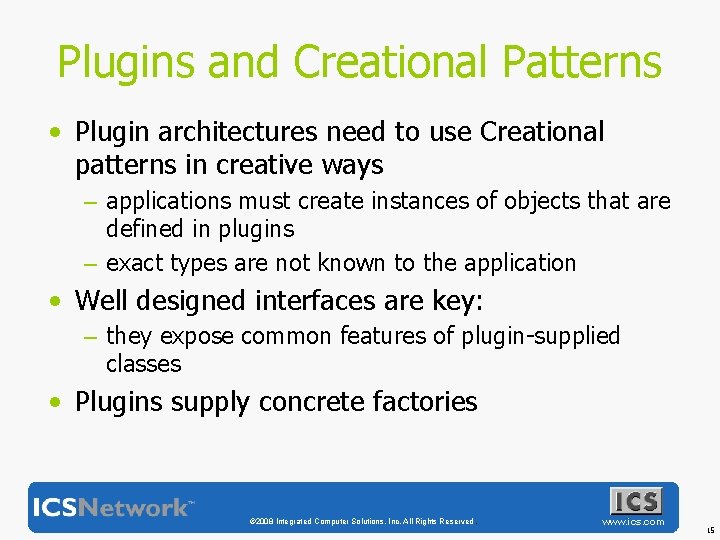
Plugins and Creational Patterns • Plugin architectures need to use Creational patterns in creative ways – applications must create instances of objects that are defined in plugins – exact types are not known to the application • Well designed interfaces are key: – they expose common features of plugin-supplied classes • Plugins supply concrete factories © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 15
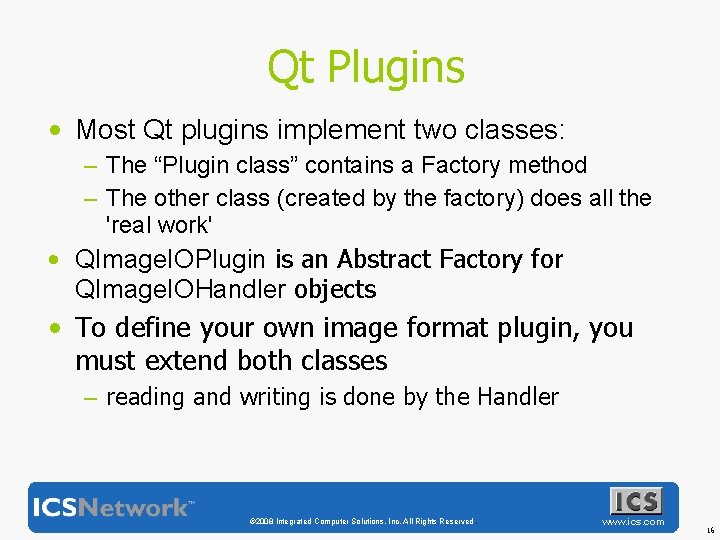
Qt Plugins • Most Qt plugins implement two classes: – The “Plugin class” contains a Factory method – The other class (created by the factory) does all the 'real work' • QImage. IOPlugin is an Abstract Factory for QImage. IOHandler objects • To define your own image format plugin, you must extend both classes – reading and writing is done by the Handler © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 16
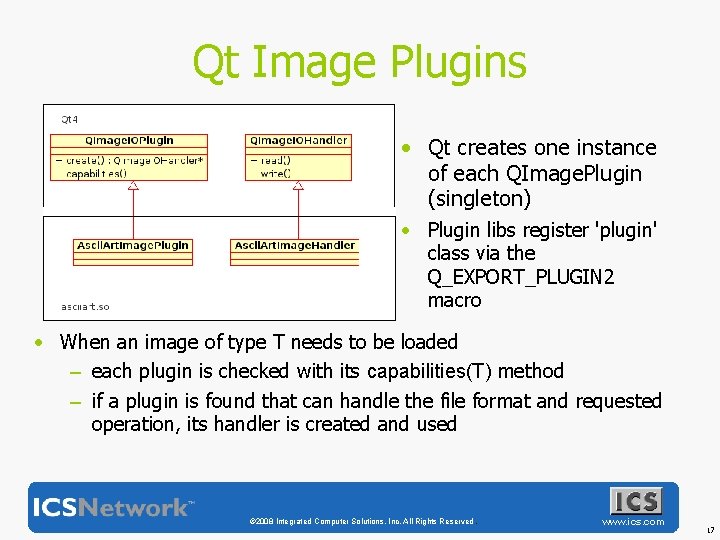
Qt Image Plugins • Qt creates one instance of each QImage. Plugin (singleton) • Plugin libs register 'plugin' class via the Q_EXPORT_PLUGIN 2 macro • When an image of type T needs to be loaded – each plugin is checked with its capabilities(T) method – if a plugin is found that can handle the file format and requested operation, its handler is created and used © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 17
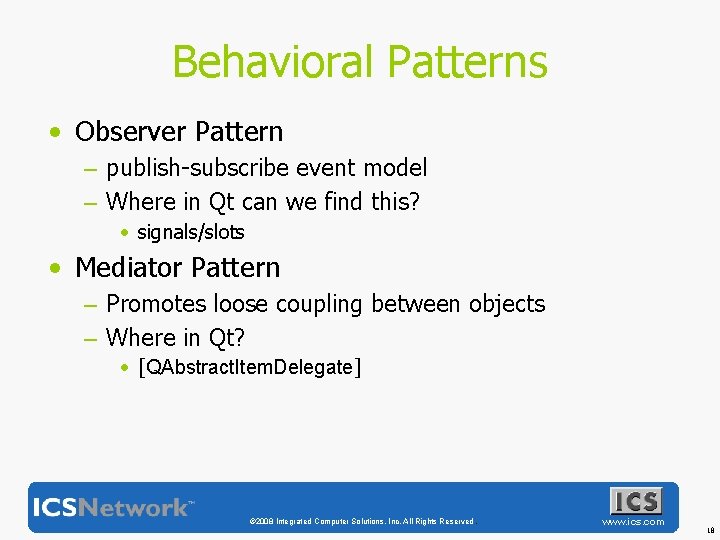
Behavioral Patterns • Observer Pattern – publish-subscribe event model – Where in Qt can we find this? • signals/slots • Mediator Pattern – Promotes loose coupling between objects – Where in Qt? • [QAbstract. Item. Delegate] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 18
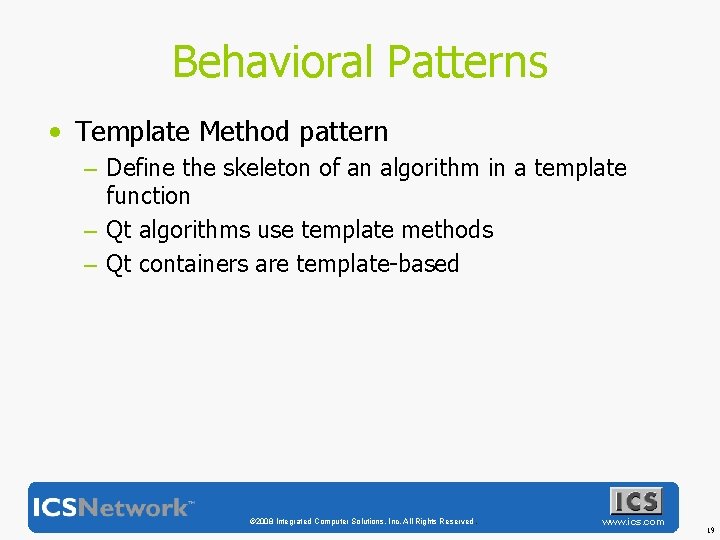
Behavioral Patterns • Template Method pattern – Define the skeleton of an algorithm in a template function – Qt algorithms use template methods – Qt containers are template-based © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 19
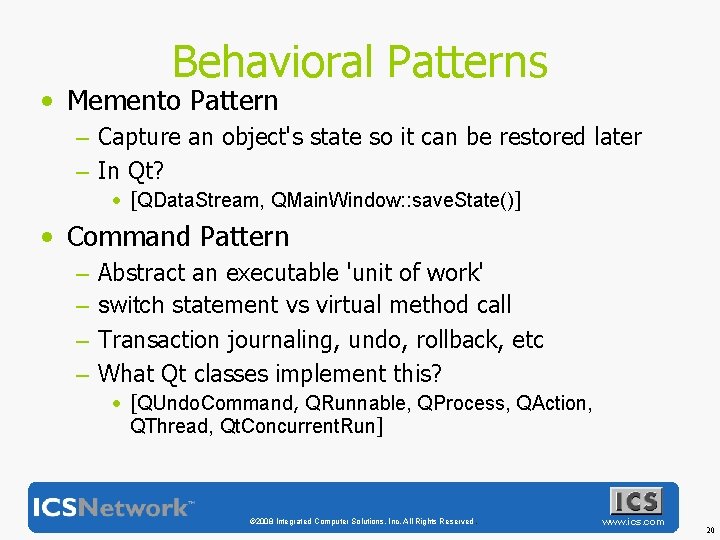
Behavioral Patterns • Memento Pattern – Capture an object's state so it can be restored later – In Qt? • [QData. Stream, QMain. Window: : save. State()] • Command Pattern – – Abstract an executable 'unit of work' switch statement vs virtual method call Transaction journaling, undo, rollback, etc What Qt classes implement this? • [QUndo. Command, QRunnable, QProcess, QAction, QThread, Qt. Concurrent. Run] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 20
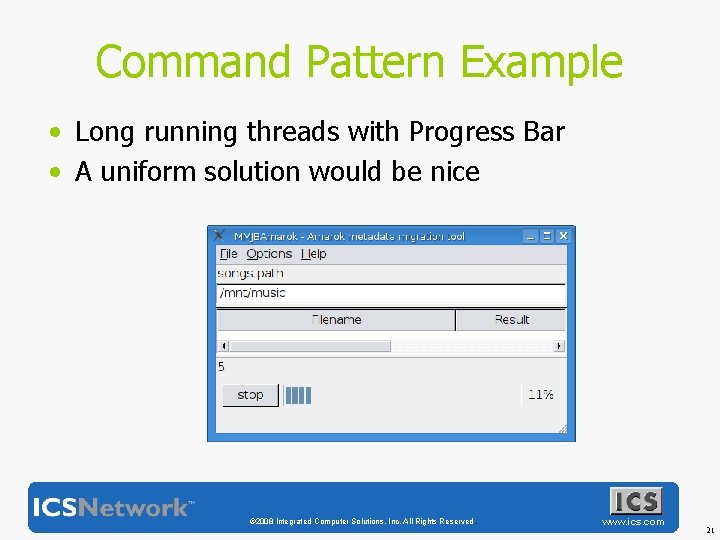
Command Pattern Example • Long running threads with Progress Bar • A uniform solution would be nice © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 21
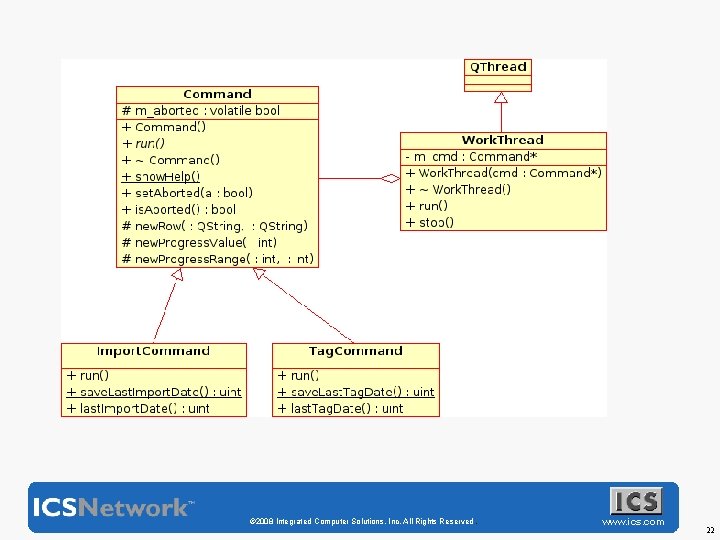
© 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 22
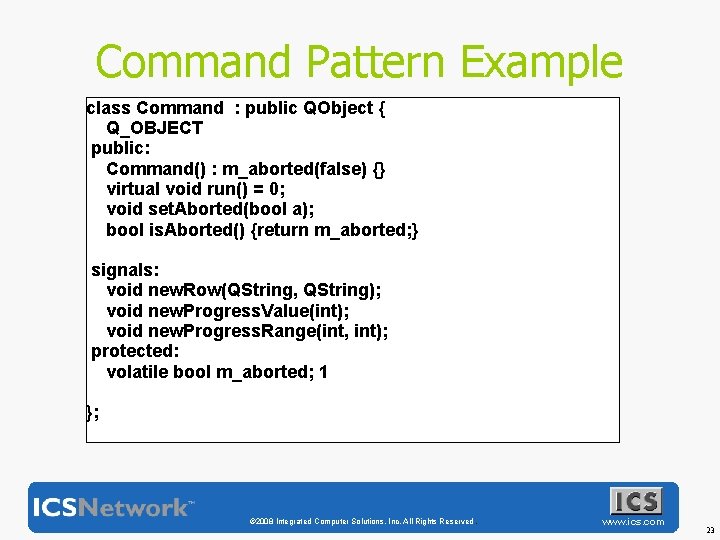
Command Pattern Example class Command : public QObject { Q_OBJECT public: Command() : m_aborted(false) {} virtual void run() = 0; void set. Aborted(bool a); bool is. Aborted() {return m_aborted; } signals: void new. Row(QString, QString); void new. Progress. Value(int); void new. Progress. Range(int, int); protected: volatile bool m_aborted; 1 }; © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 23
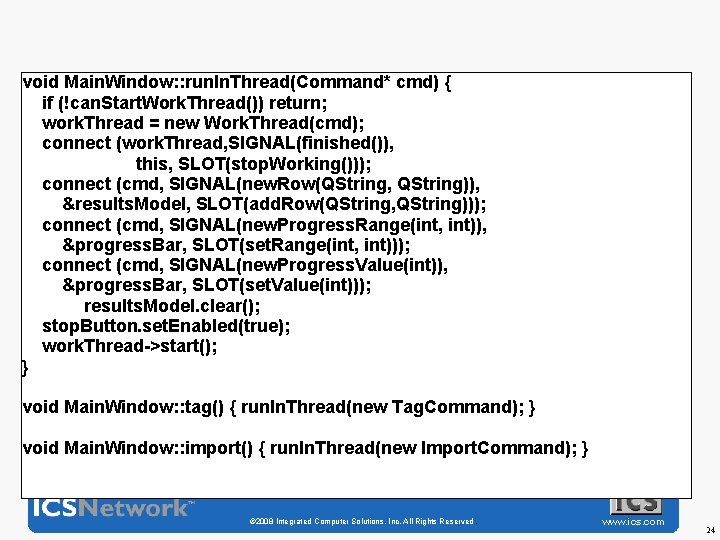
void Main. Window: : run. In. Thread(Command* cmd) { if (!can. Start. Work. Thread()) return; work. Thread = new Work. Thread(cmd); connect (work. Thread, SIGNAL(finished()), this, SLOT(stop. Working())); connect (cmd, SIGNAL(new. Row(QString, QString)), &results. Model, SLOT(add. Row(QString, QString))); connect (cmd, SIGNAL(new. Progress. Range(int, int)), &progress. Bar, SLOT(set. Range(int, int))); connect (cmd, SIGNAL(new. Progress. Value(int)), &progress. Bar, SLOT(set. Value(int))); results. Model. clear(); stop. Button. set. Enabled(true); work. Thread->start(); } void Main. Window: : tag() { run. In. Thread(new Tag. Command); } void Main. Window: : import() { run. In. Thread(new Import. Command); } © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 24
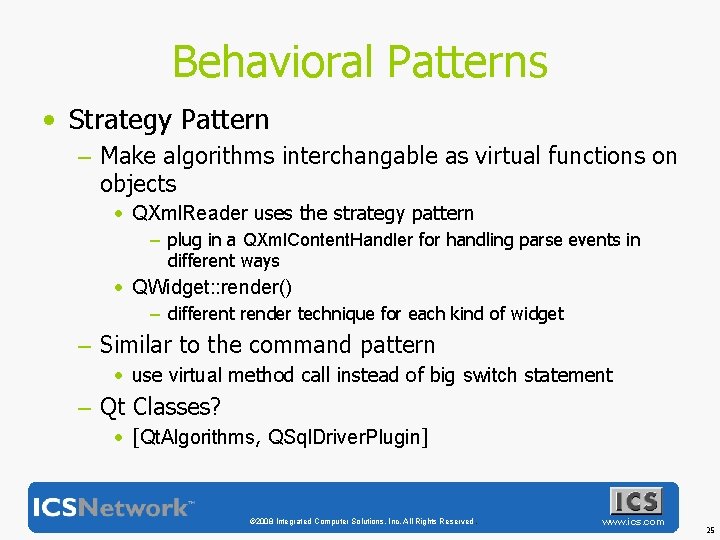
Behavioral Patterns • Strategy Pattern – Make algorithms interchangable as virtual functions on objects • QXml. Reader uses the strategy pattern – plug in a QXml. Content. Handler for handling parse events in different ways • QWidget: : render() – different render technique for each kind of widget – Similar to the command pattern • use virtual method call instead of big switch statement – Qt Classes? • [Qt. Algorithms, QSql. Driver. Plugin] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 25
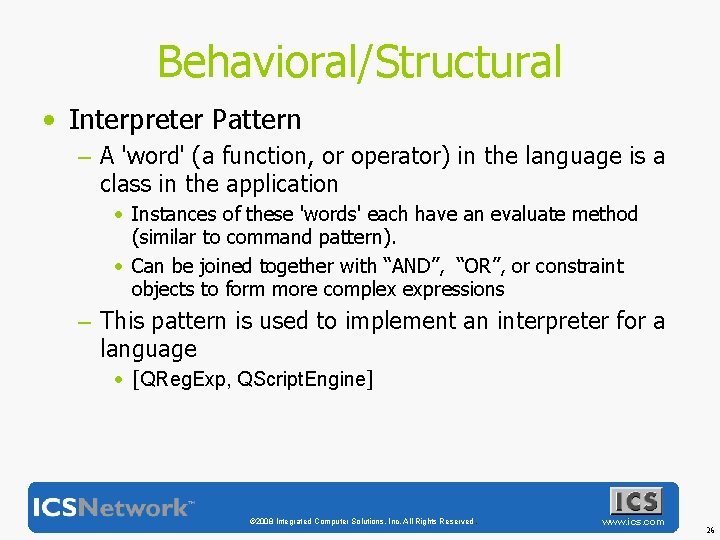
Behavioral/Structural • Interpreter Pattern – A 'word' (a function, or operator) in the language is a class in the application • Instances of these 'words' each have an evaluate method (similar to command pattern). • Can be joined together with “AND”, “OR”, or constraint objects to form more complex expressions – This pattern is used to implement an interpreter for a language • [QReg. Exp, QScript. Engine] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 26
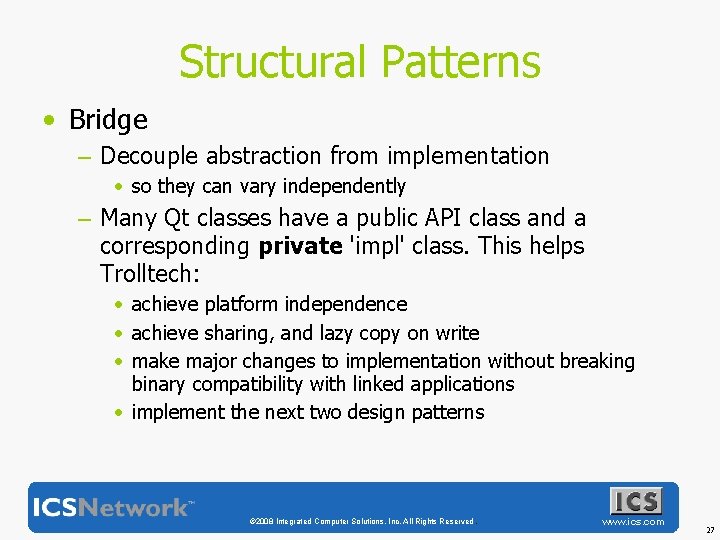
Structural Patterns • Bridge – Decouple abstraction from implementation • so they can vary independently – Many Qt classes have a public API class and a corresponding private 'impl' class. This helps Trolltech: • achieve platform independence • achieve sharing, and lazy copy on write • make major changes to implementation without breaking binary compatibility with linked applications • implement the next two design patterns © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 27
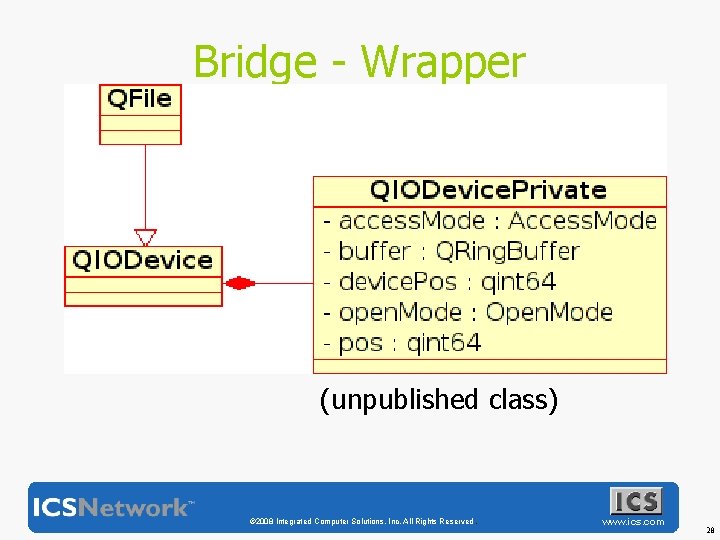
Bridge - Wrapper (unpublished class) © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 28
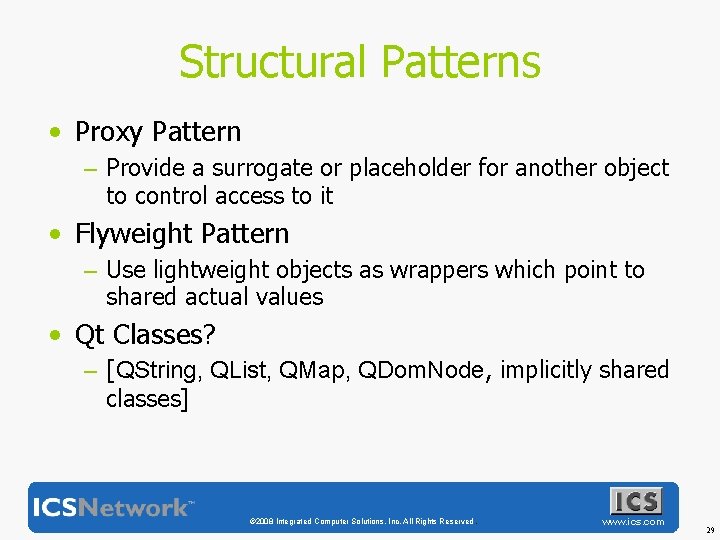
Structural Patterns • Proxy Pattern – Provide a surrogate or placeholder for another object to control access to it • Flyweight Pattern – Use lightweight objects as wrappers which point to shared actual values • Qt Classes? – [QString, QList, QMap, QDom. Node, implicitly shared classes] © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 29
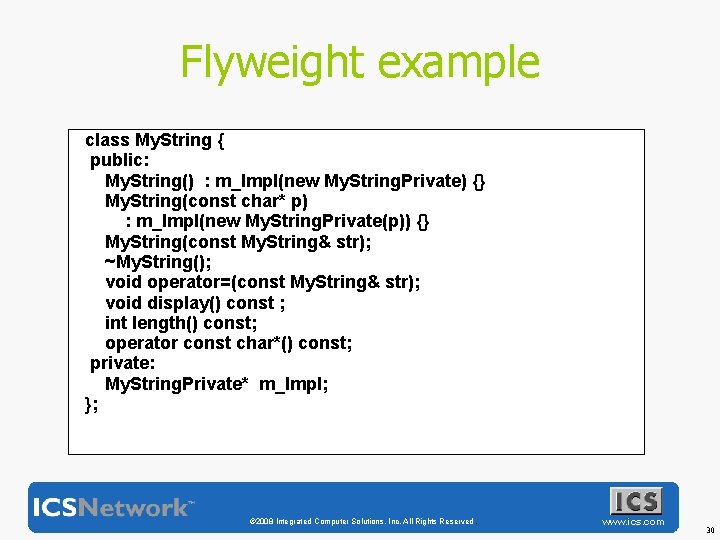
Flyweight example class My. String { public: My. String() : m_Impl(new My. String. Private) {} My. String(const char* p) : m_Impl(new My. String. Private(p)) {} My. String(const My. String& str); ~My. String(); void operator=(const My. String& str); void display() const ; int length() const; operator const char*() const; private: My. String. Private* m_Impl; }; © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 30
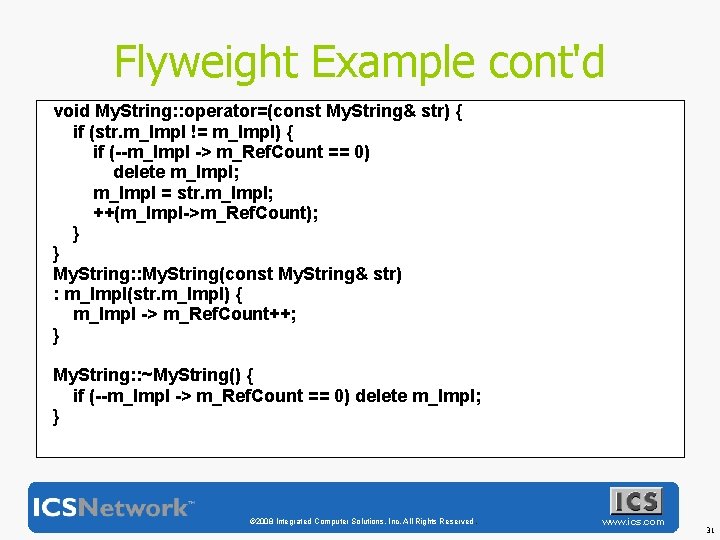
Flyweight Example cont'd void My. String: : operator=(const My. String& str) { if (str. m_Impl != m_Impl) { if (--m_Impl -> m_Ref. Count == 0) delete m_Impl; m_Impl = str. m_Impl; ++(m_Impl->m_Ref. Count); } } My. String: : My. String(const My. String& str) : m_Impl(str. m_Impl) { m_Impl -> m_Ref. Count++; } My. String: : ~My. String() { if (--m_Impl -> m_Ref. Count == 0) delete m_Impl; } © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 31
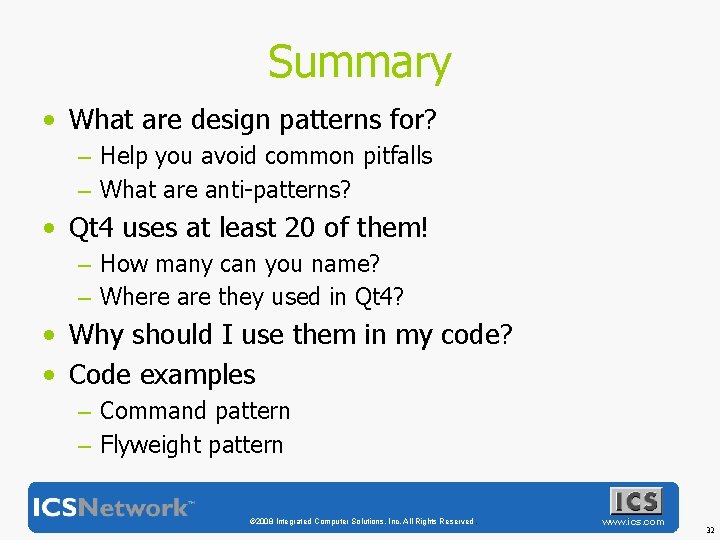
Summary • What are design patterns for? – Help you avoid common pitfalls – What are anti-patterns? • Qt 4 uses at least 20 of them! – How many can you name? – Where are they used in Qt 4? • Why should I use them in my code? • Code examples – Command pattern – Flyweight pattern © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 32
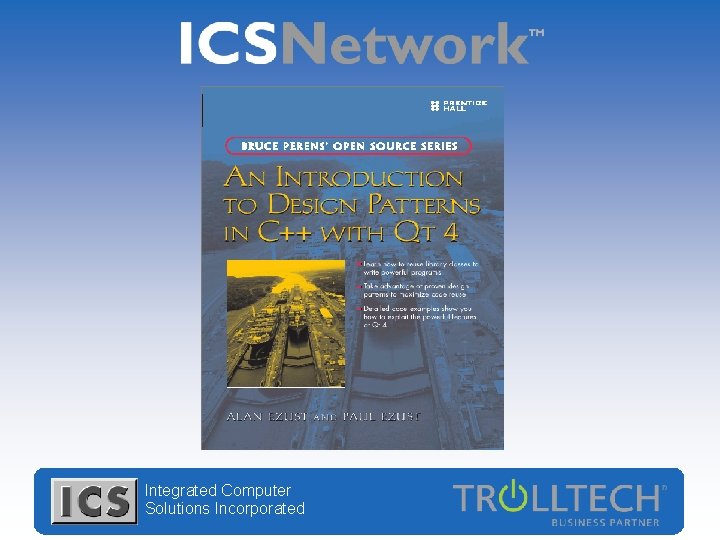
presents Integrated Computer Solutions Incorporated
![Bibliography Ezust 07 Introduction to Design Patterns in C and Qt 4 Alan Bibliography • [Ezust 07] Introduction to Design Patterns in C++ and Qt 4. Alan](https://slidetodoc.com/presentation_image_h/0e3823ec683edc21125f0bc820195e2e/image-34.jpg)
Bibliography • [Ezust 07] Introduction to Design Patterns in C++ and Qt 4. Alan Ezust and Paul Ezust. 2007, Prentice Hall. 0 -13 -187905 -7. • [Fowler 04] UML Distilled. Third Edition. Martin Fowler. 2004. Addison Wesley. 0 -321 -19368 -7. • [Gamma 95] Design Patterns. Elements of Reusable Object-Oriented Software. Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides. 1995. Addison-Wesley. 0 -201 -63361 -2. • [Thelin 07] Foundations of Qt Development. Johan Thelin. 2007. Apress. 978 -1 -59059 -831 -3 • [Blanchette 08] C++ GUI Programming with Qt 4, 2/E. Jasmin Blanchette and Mark Summerfield. 2008, Prentice Hall. 0 -13 -235416 © 2008 Integrated Computer Solutions, Inc. All Rights Reserved. www. ics. com 34
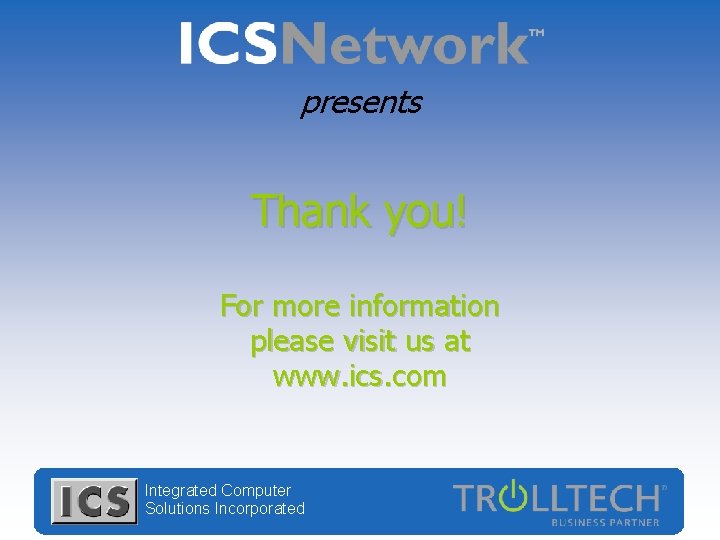
presents Thank you! For more information please visit us at www. ics. com Integrated Computer Solutions Incorporated
Az ezüst vegyjele
Térfogat jele
Ezüst kolloid dm
Dating serves several important functions that include:
Eclat algorithm
Systems analysis and design alan dennis
Alan dennis system analysis design
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
System analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Systems analysis and design alan dennis
Identify the text structure of the following passage
Presents from my aunts in pakistan
Presents from my aunts in pakistan poem analysis
Business agility metrics
16 3 darwin presents his case answer key
Section 15-3 darwin presents his case
Gurmit paid £21 for five presents
Sue palmer skeletons
Zids and zods answers
Prose writing that presents and explains ideas
What is nonfiction prose
Zids and zods
Where does “snowflake girl” help to deliver presents
Bienvenue au culte d'adoration
Is thesis statement and main idea the same
A 26 year old female presents
This presents
A dramatic work that presents the downfall of a character