PHP Using Strings 1 Note There are many
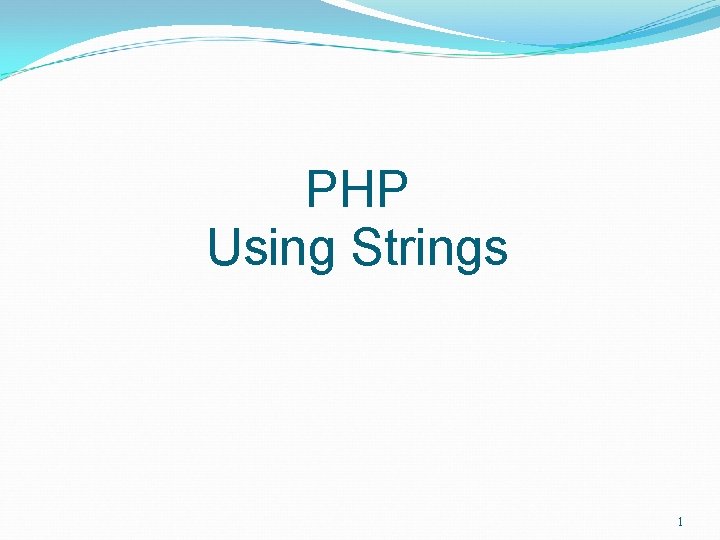
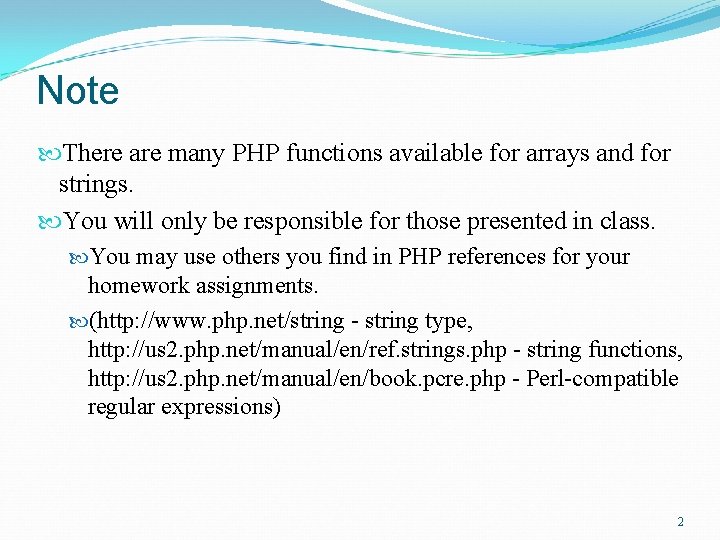
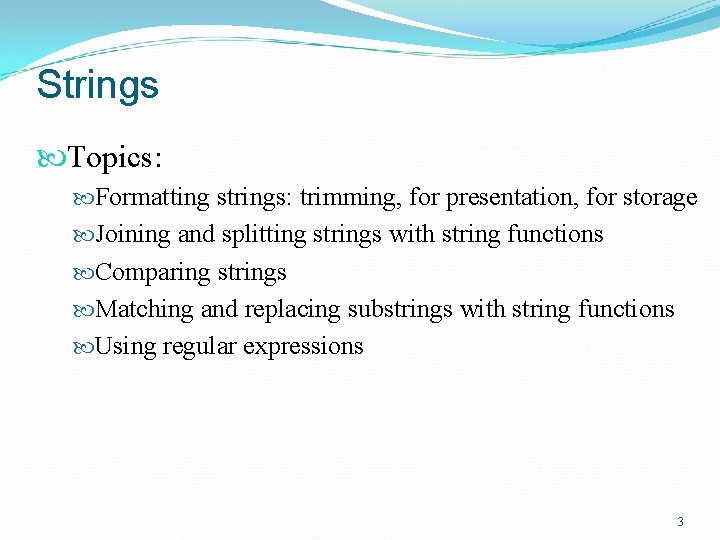
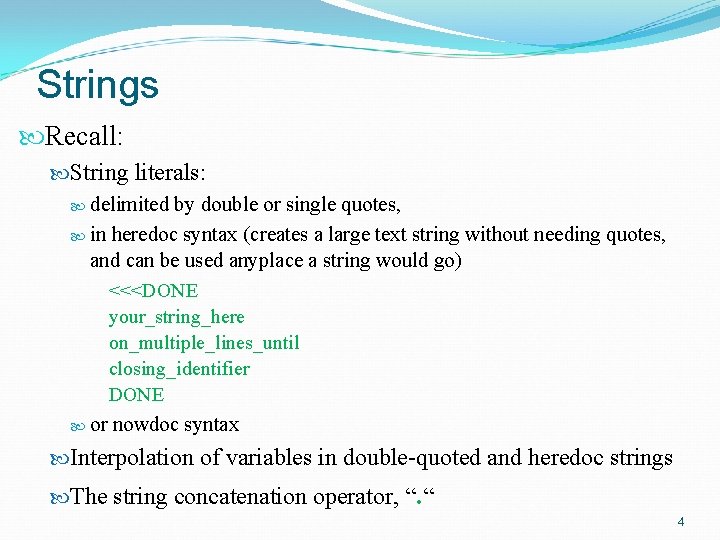
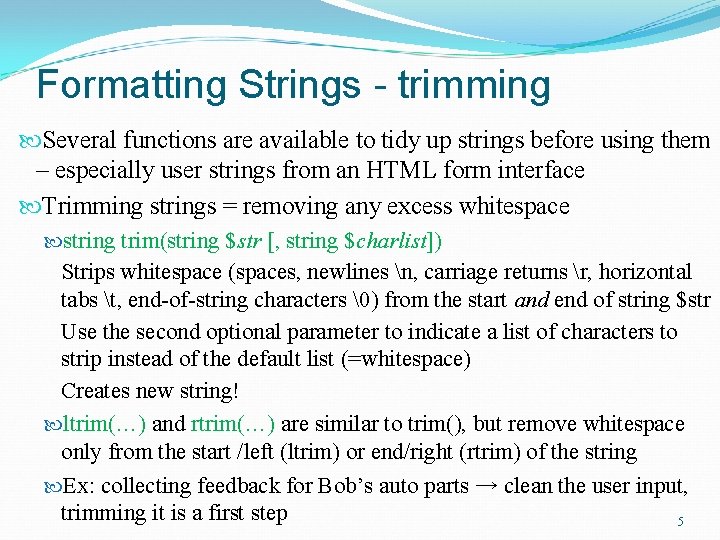
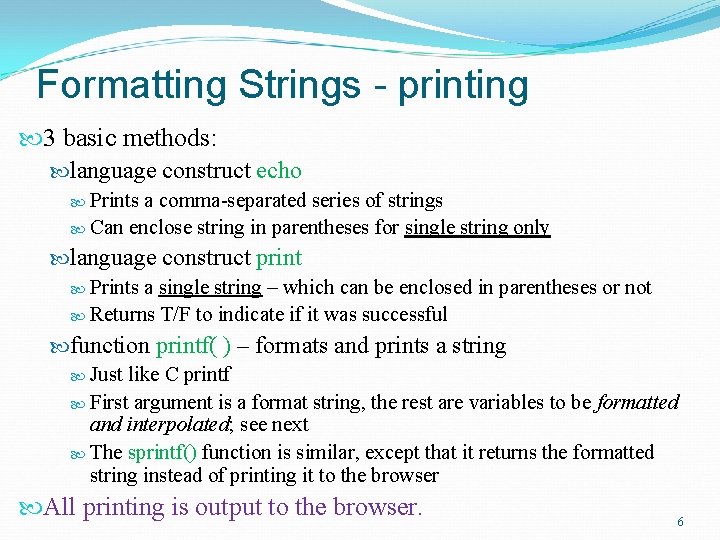
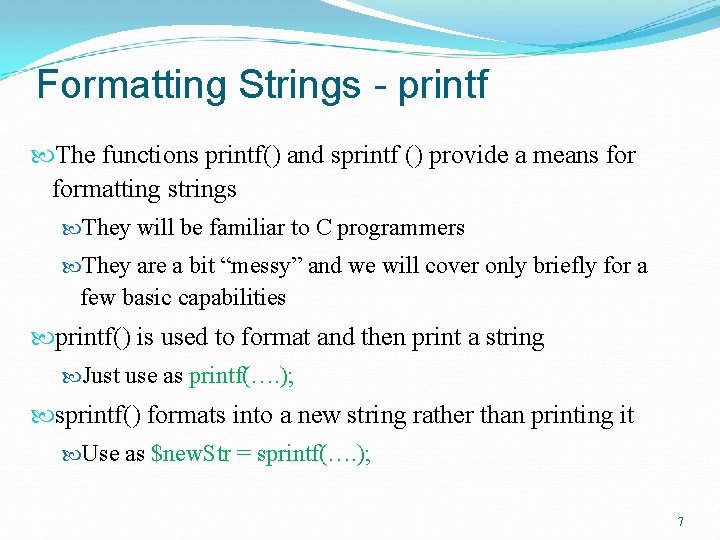
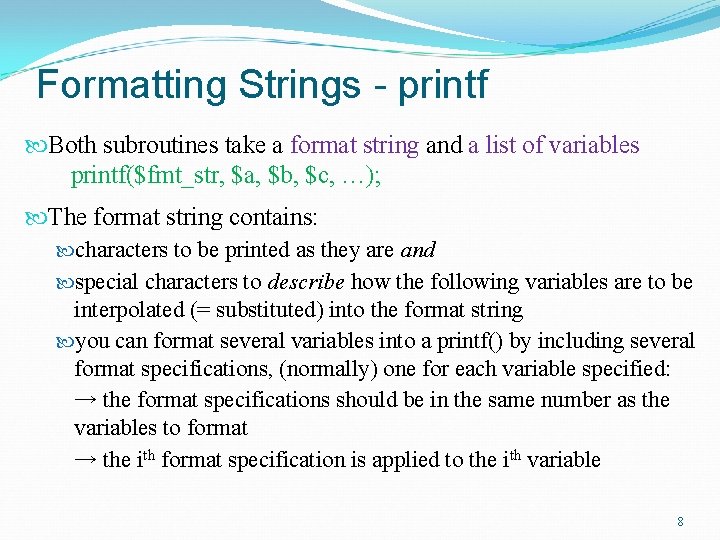
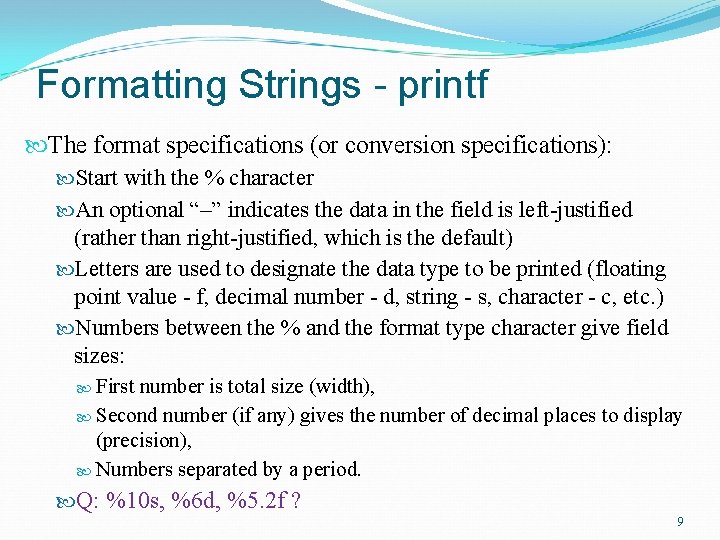
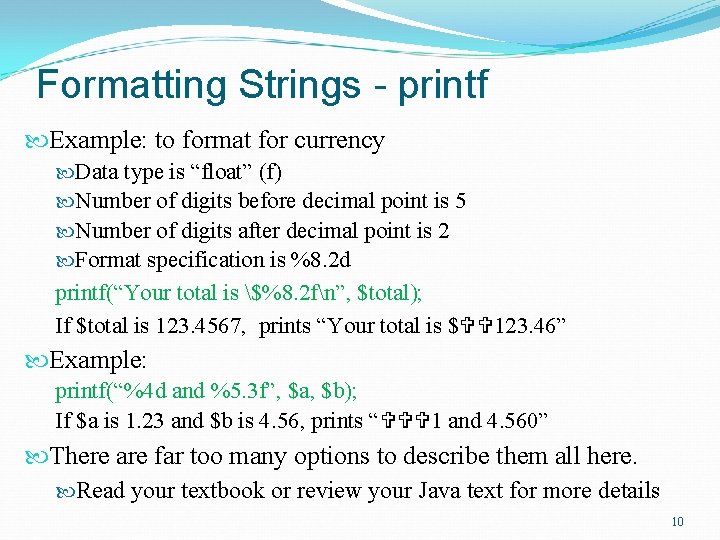
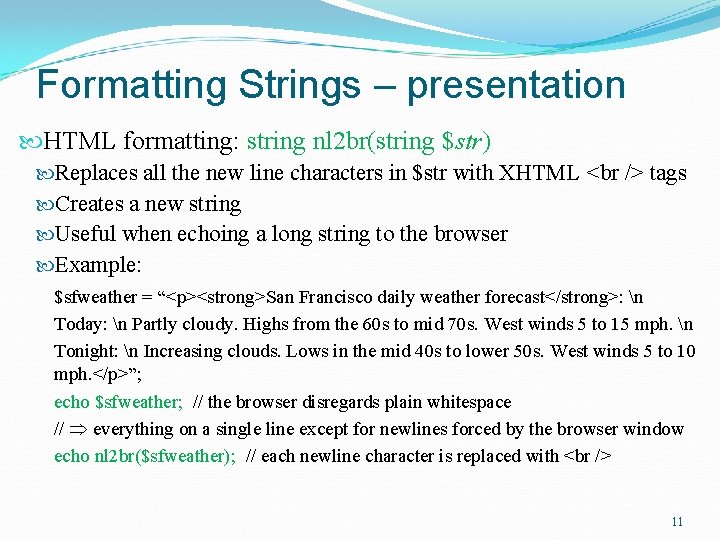
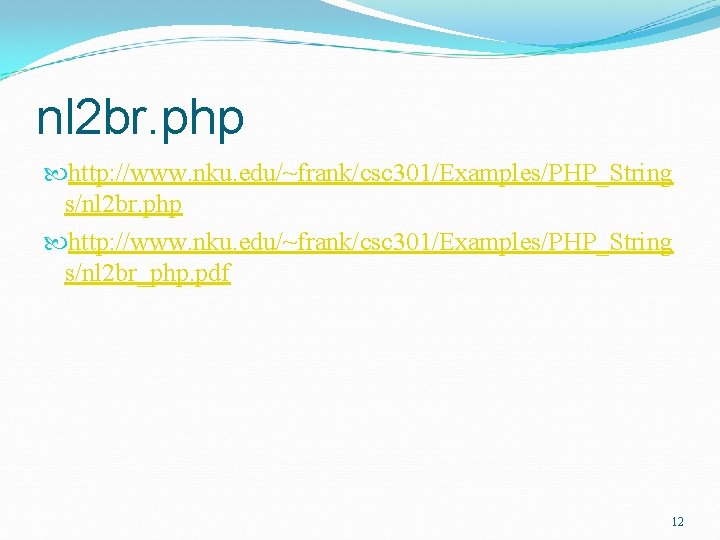
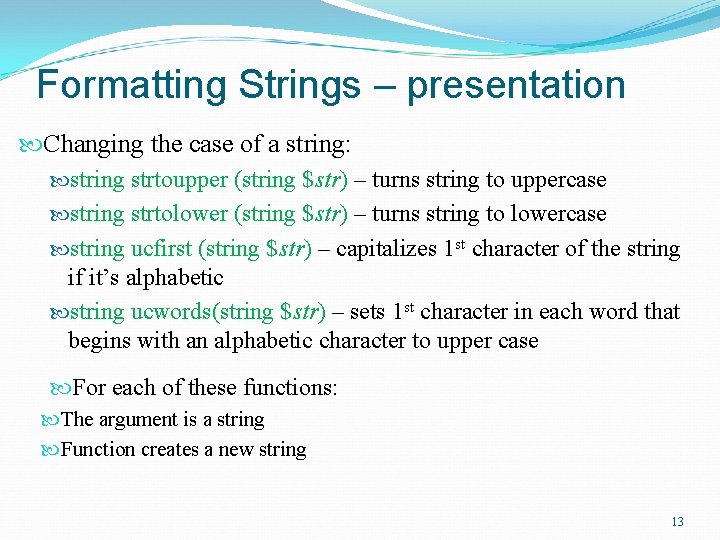
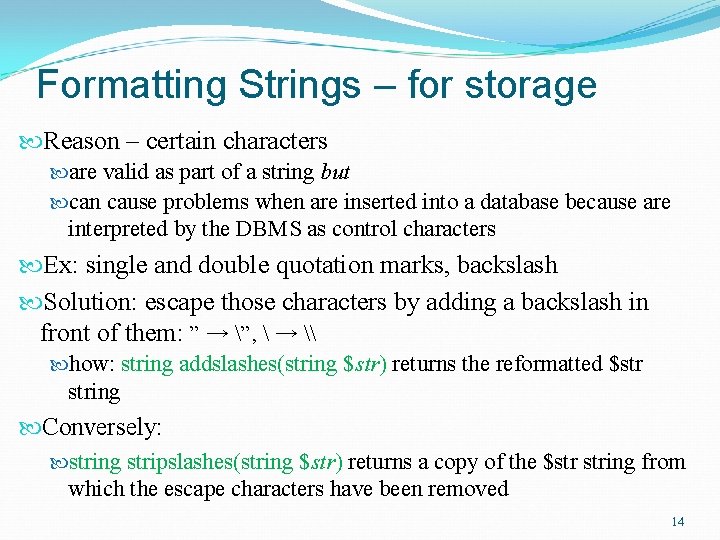
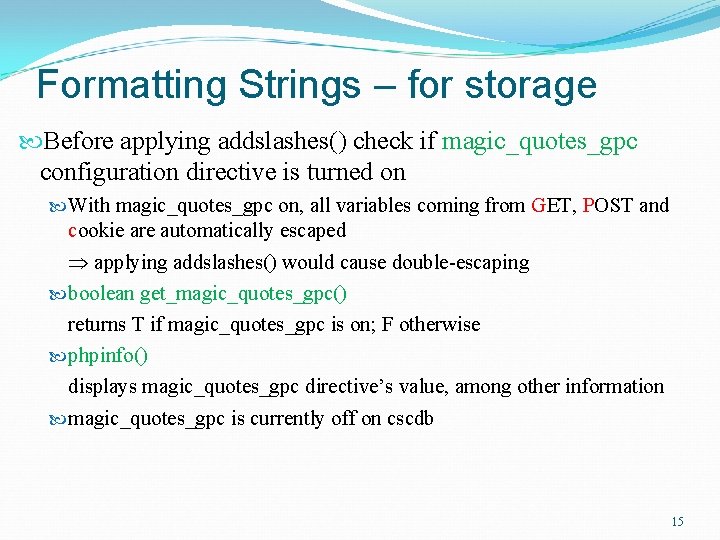
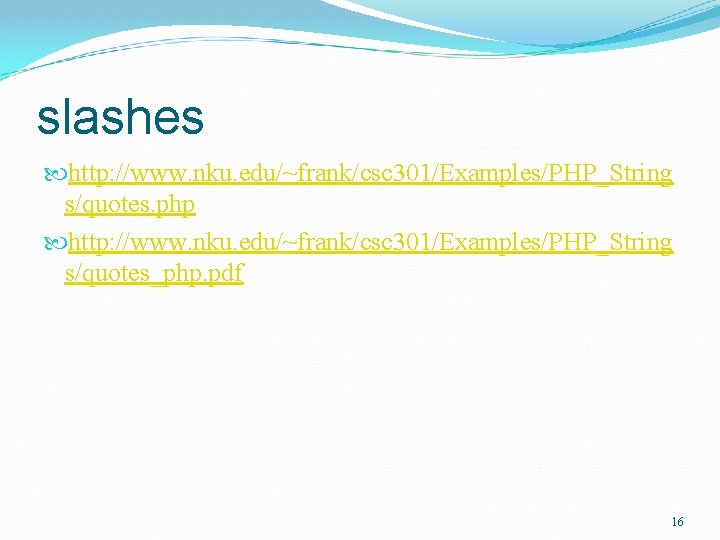
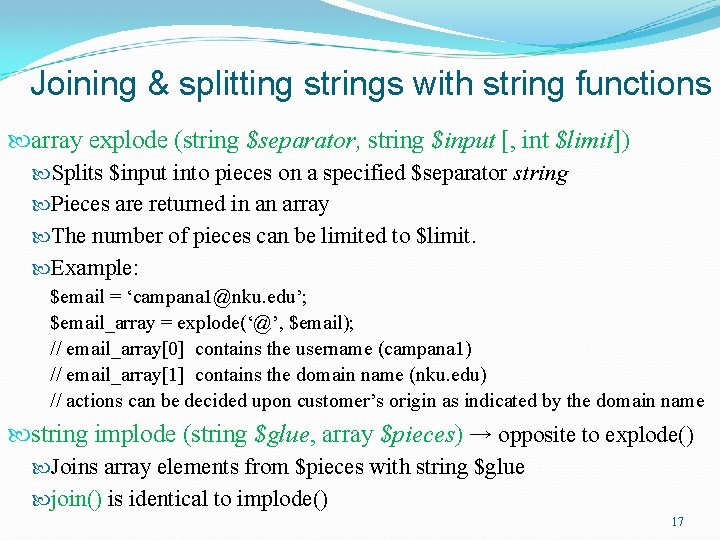
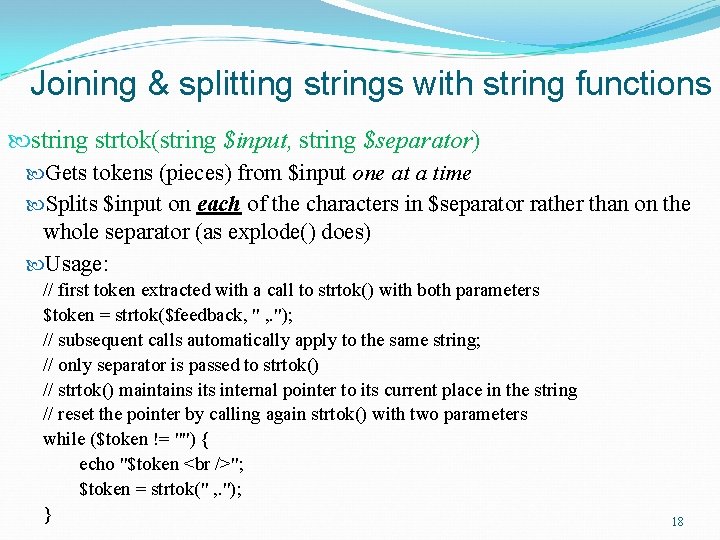
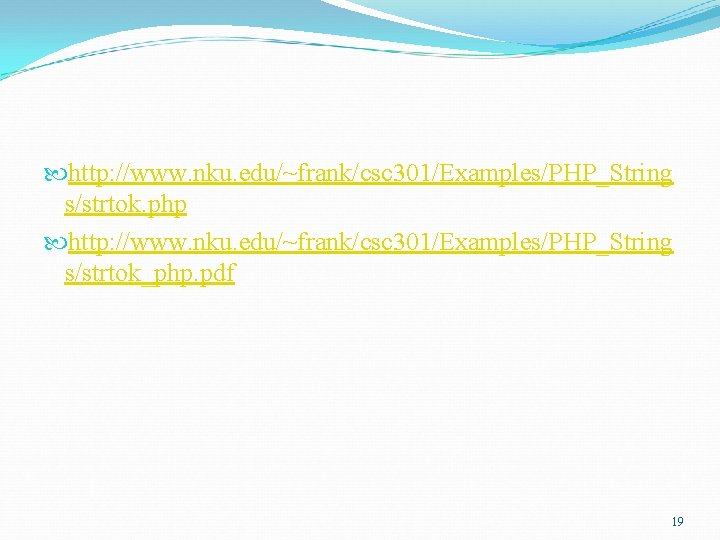
![Joining & splitting strings with string functions string substr(string $str, int $start[, int $length]) Joining & splitting strings with string functions string substr(string $str, int $start[, int $length])](https://slidetodoc.com/presentation_image_h/a17971a3ebc331a2ac56456789a99d6d/image-20.jpg)
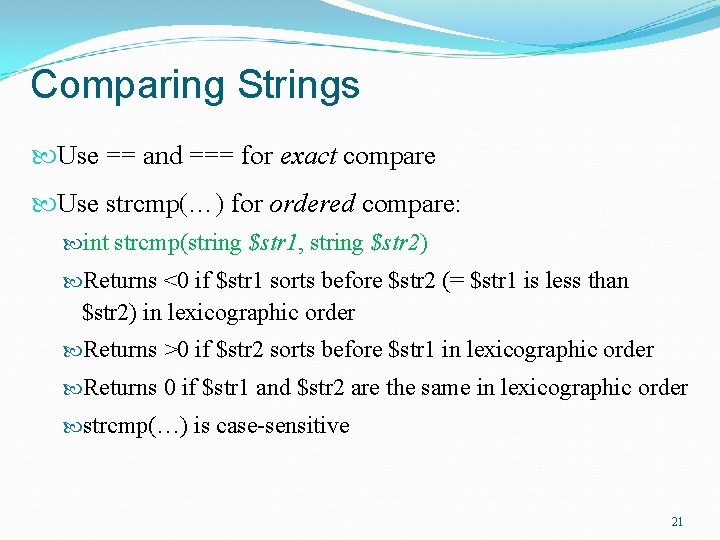
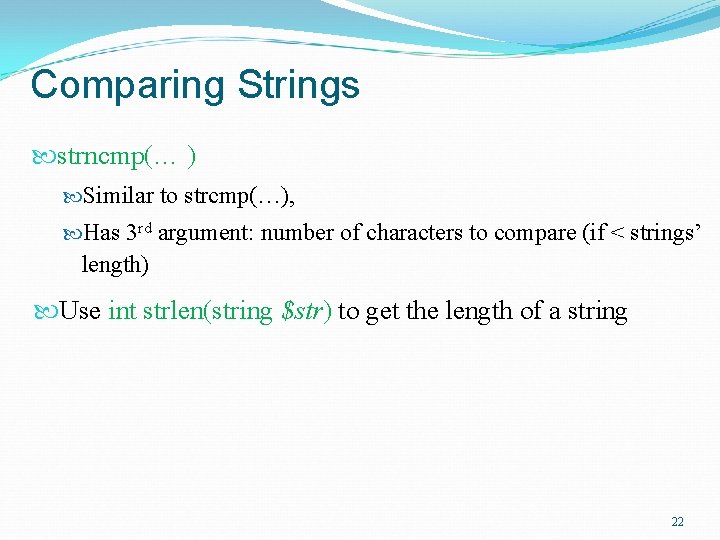
- Slides: 22
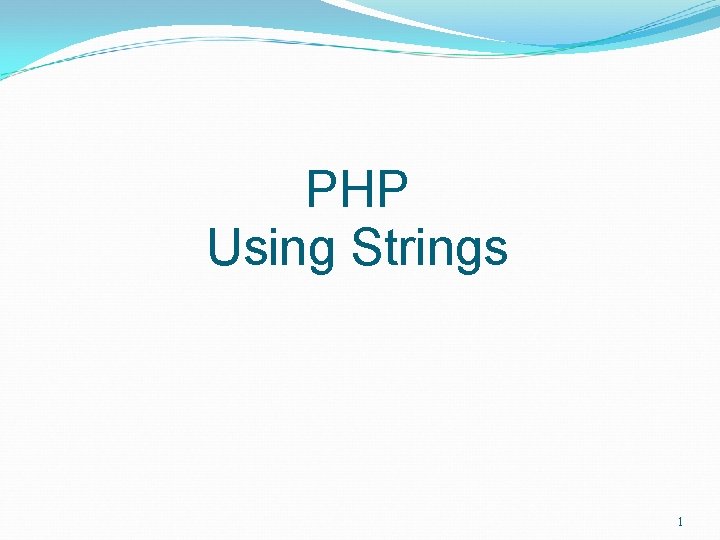
PHP Using Strings 1
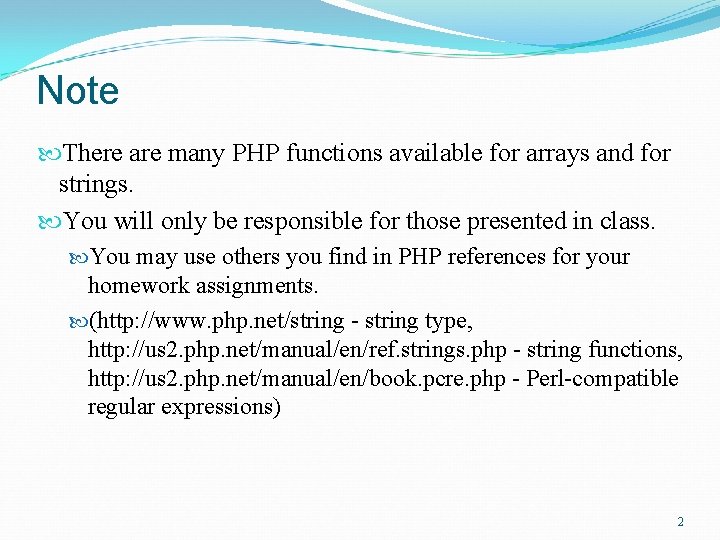
Note There are many PHP functions available for arrays and for strings. You will only be responsible for those presented in class. You may use others you find in PHP references for your homework assignments. (http: //www. php. net/string - string type, http: //us 2. php. net/manual/en/ref. strings. php - string functions, http: //us 2. php. net/manual/en/book. pcre. php - Perl-compatible regular expressions) 2
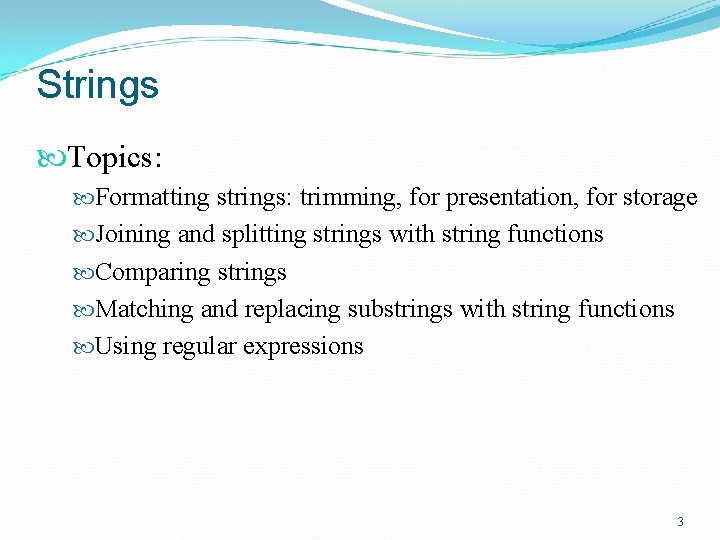
Strings Topics: Formatting strings: trimming, for presentation, for storage Joining and splitting strings with string functions Comparing strings Matching and replacing substrings with string functions Using regular expressions 3
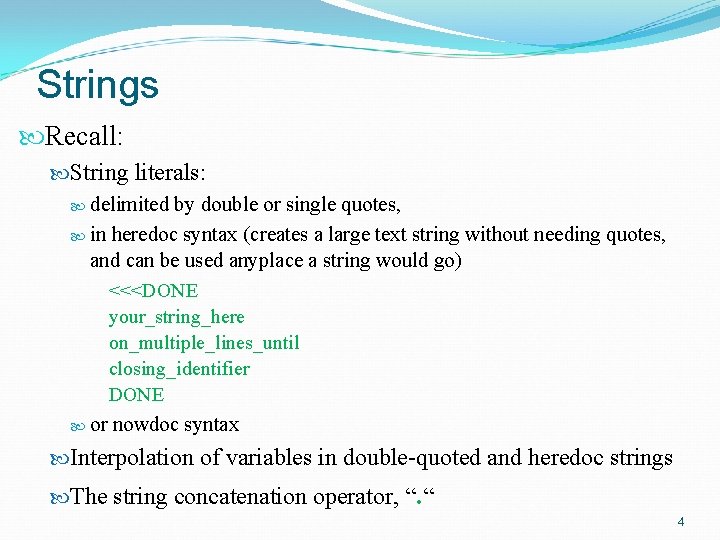
Strings Recall: String literals: delimited by double or single quotes, in heredoc syntax (creates a large text string without needing quotes, and can be used anyplace a string would go) <<<DONE your_string_here on_multiple_lines_until closing_identifier DONE or nowdoc syntax Interpolation of variables in double-quoted and heredoc strings The string concatenation operator, “. “ 4
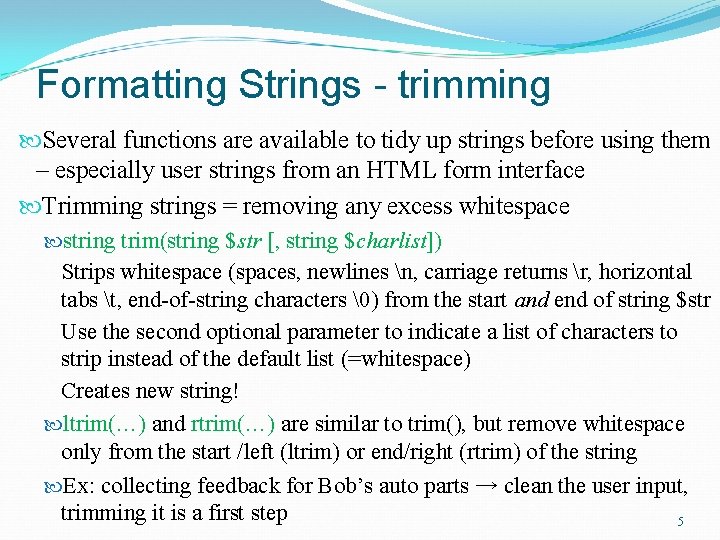
Formatting Strings - trimming Several functions are available to tidy up strings before using them – especially user strings from an HTML form interface Trimming strings = removing any excess whitespace string trim(string $str [, string $charlist]) Strips whitespace (spaces, newlines n, carriage returns r, horizontal tabs t, end-of-string characters ) from the start and end of string $str Use the second optional parameter to indicate a list of characters to strip instead of the default list (=whitespace) Creates new string! ltrim(…) and rtrim(…) are similar to trim(), but remove whitespace only from the start /left (ltrim) or end/right (rtrim) of the string Ex: collecting feedback for Bob’s auto parts → clean the user input, trimming it is a first step 5
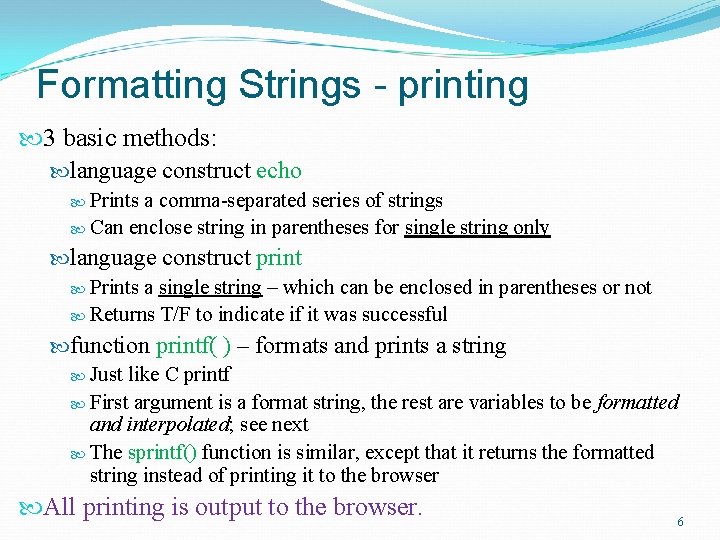
Formatting Strings - printing 3 basic methods: language construct echo Prints a comma-separated series of strings Can enclose string in parentheses for single string only language construct print Prints a single string – which can be enclosed in parentheses or not Returns T/F to indicate if it was successful function printf( ) – formats and prints a string Just like C printf First argument is a format string, the rest are variables to be formatted and interpolated; see next The sprintf() function is similar, except that it returns the formatted string instead of printing it to the browser All printing is output to the browser. 6
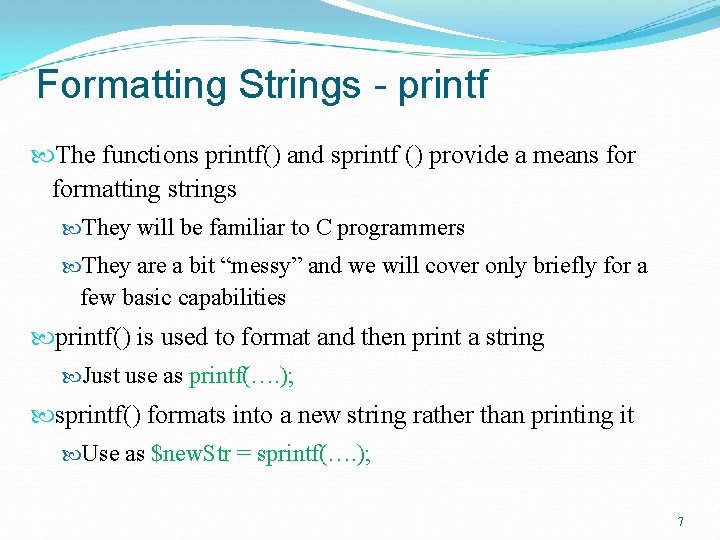
Formatting Strings - printf The functions printf() and sprintf () provide a means formatting strings They will be familiar to C programmers They are a bit “messy” and we will cover only briefly for a few basic capabilities printf() is used to format and then print a string Just use as printf(…. ); sprintf() formats into a new string rather than printing it Use as $new. Str = sprintf(…. ); 7
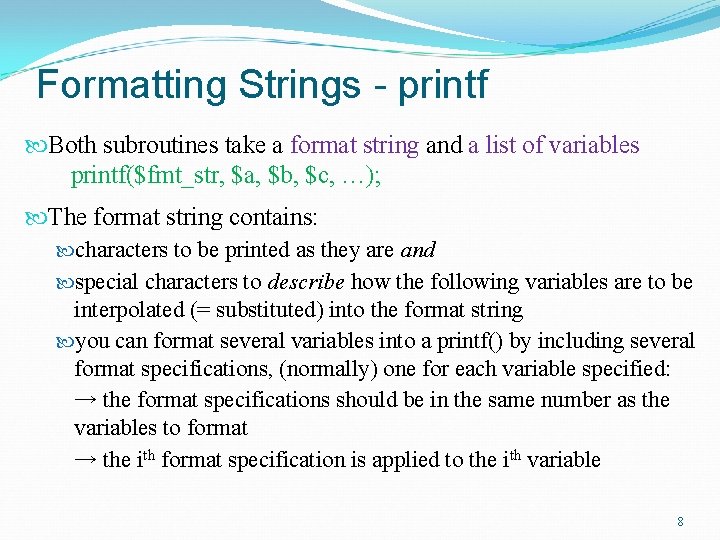
Formatting Strings - printf Both subroutines take a format string and a list of variables printf($fmt_str, $a, $b, $c, …); The format string contains: characters to be printed as they are and special characters to describe how the following variables are to be interpolated (= substituted) into the format string you can format several variables into a printf() by including several format specifications, (normally) one for each variable specified: → the format specifications should be in the same number as the variables to format → the ith format specification is applied to the ith variable 8
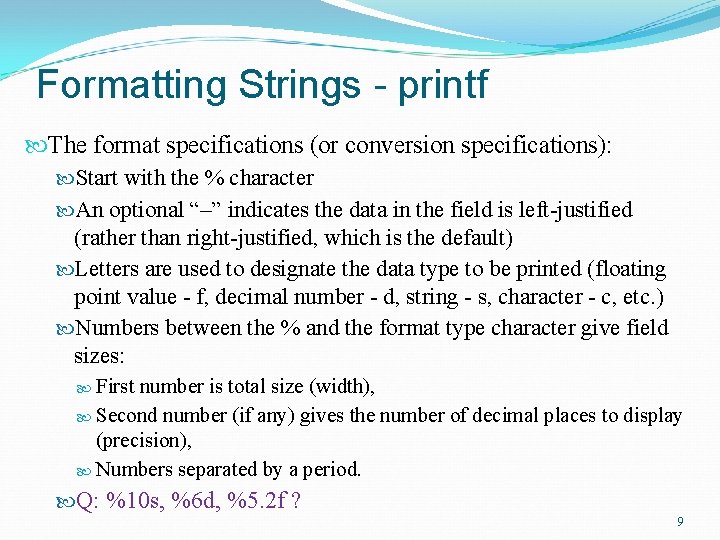
Formatting Strings - printf The format specifications (or conversion specifications): Start with the % character An optional “–” indicates the data in the field is left-justified (rather than right-justified, which is the default) Letters are used to designate the data type to be printed (floating point value - f, decimal number - d, string - s, character - c, etc. ) Numbers between the % and the format type character give field sizes: First number is total size (width), Second number (if any) gives the number of decimal places to display (precision), Numbers separated by a period. Q: %10 s, %6 d, %5. 2 f ? 9
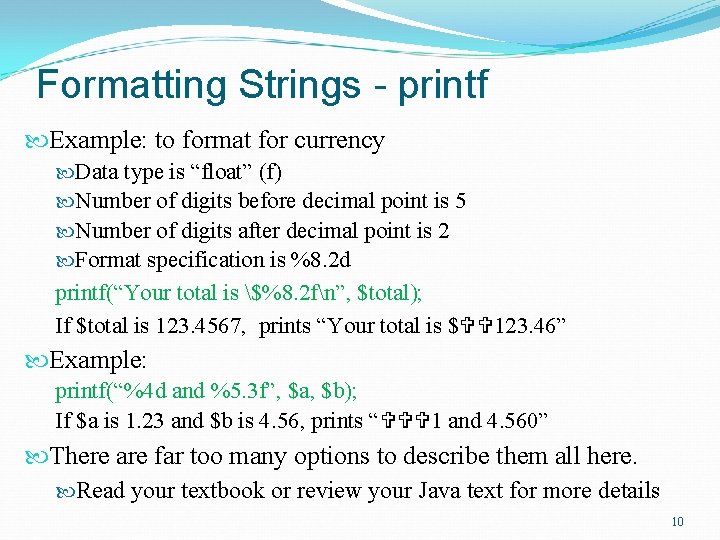
Formatting Strings - printf Example: to format for currency Data type is “float” (f) Number of digits before decimal point is 5 Number of digits after decimal point is 2 Format specification is %8. 2 d printf(“Your total is $%8. 2 fn”, $total); If $total is 123. 4567, prints “Your total is $ 123. 46” Example: printf(“%4 d and %5. 3 f”, $a, $b); If $a is 1. 23 and $b is 4. 56, prints “ 1 and 4. 560” There are far too many options to describe them all here. Read your textbook or review your Java text for more details 10
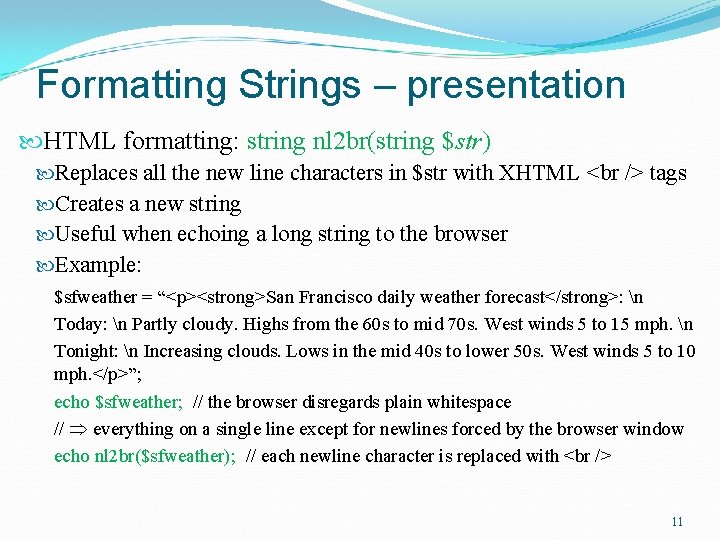
Formatting Strings – presentation HTML formatting: string nl 2 br(string $str) Replaces all the new line characters in $str with XHTML tags Creates a new string Useful when echoing a long string to the browser Example: $sfweather = “<p><strong>San Francisco daily weather forecast</strong>: n Today: n Partly cloudy. Highs from the 60 s to mid 70 s. West winds 5 to 15 mph. n Tonight: n Increasing clouds. Lows in the mid 40 s to lower 50 s. West winds 5 to 10 mph. </p>”; echo $sfweather; // the browser disregards plain whitespace // everything on a single line except for newlines forced by the browser window echo nl 2 br($sfweather); // each newline character is replaced with 11
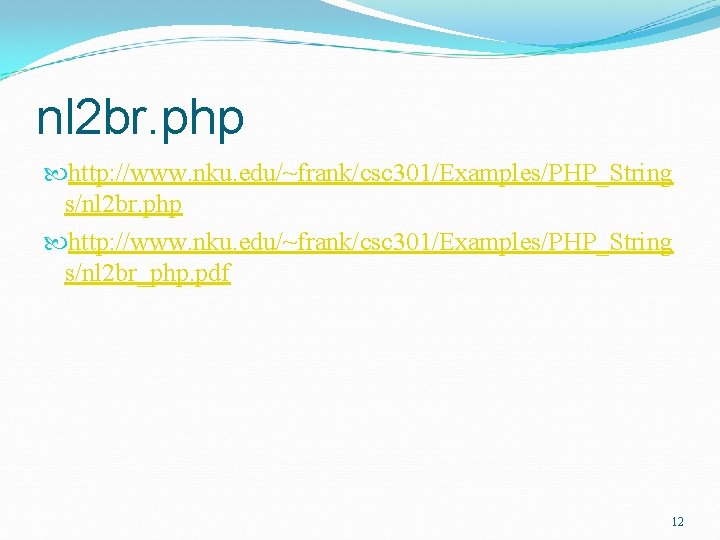
nl 2 br. php http: //www. nku. edu/~frank/csc 301/Examples/PHP_String s/nl 2 br_php. pdf 12
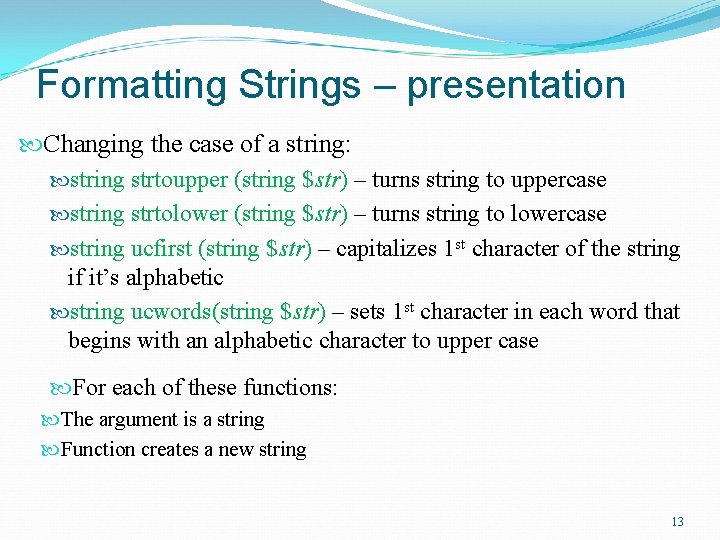
Formatting Strings – presentation Changing the case of a string: string strtoupper (string $str) – turns string to uppercase string strtolower (string $str) – turns string to lowercase string ucfirst (string $str) – capitalizes 1 st character of the string if it’s alphabetic string ucwords(string $str) – sets 1 st character in each word that begins with an alphabetic character to upper case For each of these functions: The argument is a string Function creates a new string 13
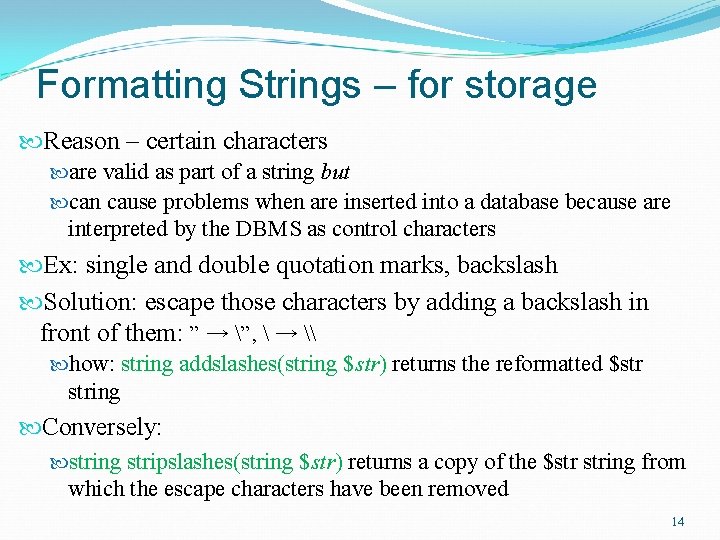
Formatting Strings – for storage Reason – certain characters are valid as part of a string but can cause problems when are inserted into a database because are interpreted by the DBMS as control characters Ex: single and double quotation marks, backslash Solution: escape those characters by adding a backslash in front of them: ” → ”, → \ how: string addslashes(string $str) returns the reformatted $str string Conversely: string stripslashes(string $str) returns a copy of the $str string from which the escape characters have been removed 14
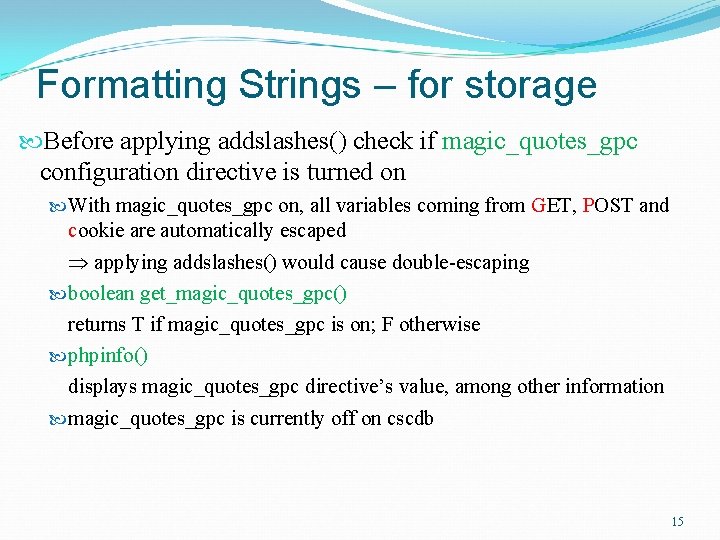
Formatting Strings – for storage Before applying addslashes() check if magic_quotes_gpc configuration directive is turned on With magic_quotes_gpc on, all variables coming from GET, POST and cookie are automatically escaped applying addslashes() would cause double-escaping boolean get_magic_quotes_gpc() returns T if magic_quotes_gpc is on; F otherwise phpinfo() displays magic_quotes_gpc directive’s value, among other information magic_quotes_gpc is currently off on cscdb 15
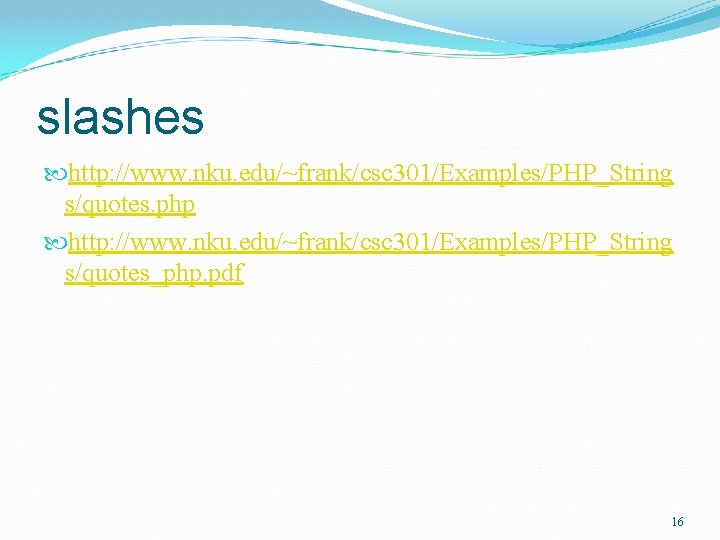
slashes http: //www. nku. edu/~frank/csc 301/Examples/PHP_String s/quotes. php http: //www. nku. edu/~frank/csc 301/Examples/PHP_String s/quotes_php. pdf 16
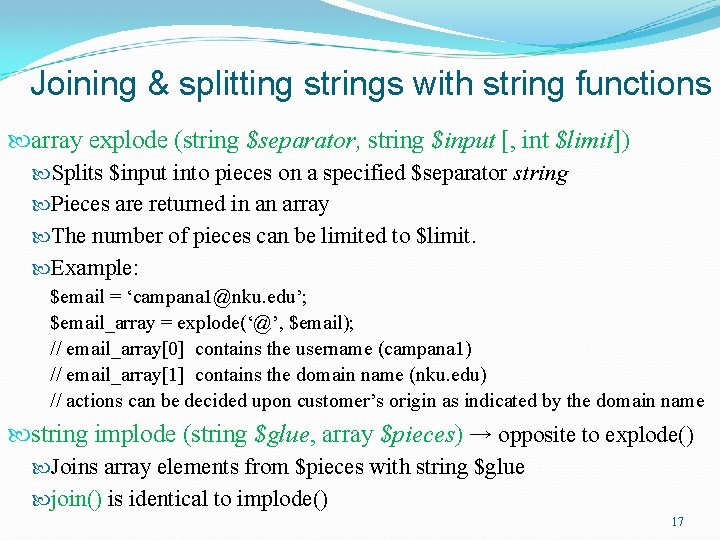
Joining & splitting strings with string functions array explode (string $separator, string $input [, int $limit]) Splits $input into pieces on a specified $separator string Pieces are returned in an array The number of pieces can be limited to $limit. Example: $email = ‘campana 1@nku. edu’; $email_array = explode(‘@’, $email); // email_array[0] contains the username (campana 1) // email_array[1] contains the domain name (nku. edu) // actions can be decided upon customer’s origin as indicated by the domain name string implode (string $glue, array $pieces) → opposite to explode() Joins array elements from $pieces with string $glue join() is identical to implode() 17
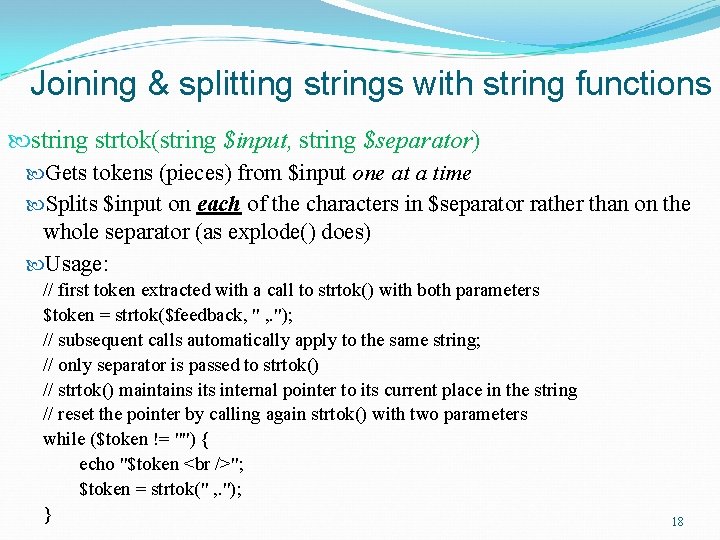
Joining & splitting strings with string functions string strtok(string $input, string $separator) Gets tokens (pieces) from $input one at a time Splits $input on each of the characters in $separator rather than on the whole separator (as explode() does) Usage: // first token extracted with a call to strtok() with both parameters $token = strtok($feedback, " , . "); // subsequent calls automatically apply to the same string; // only separator is passed to strtok() // strtok() maintains its internal pointer to its current place in the string // reset the pointer by calling again strtok() with two parameters while ($token != "") { echo "$token "; $token = strtok(" , . "); } 18
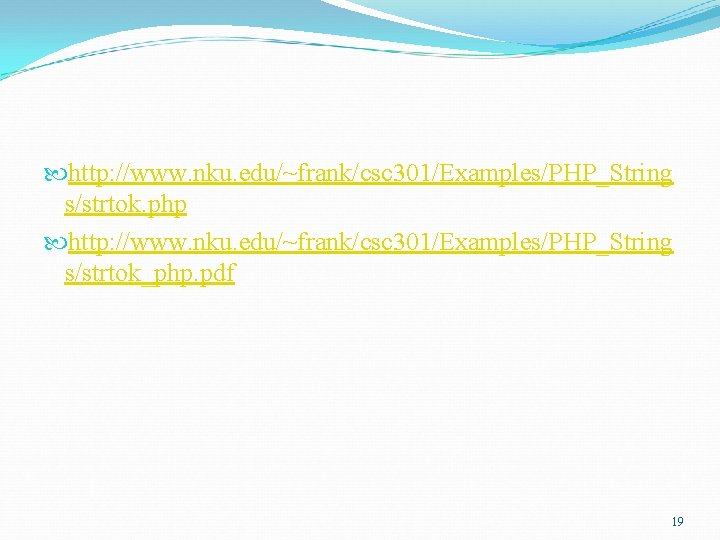
http: //www. nku. edu/~frank/csc 301/Examples/PHP_String s/strtok. php http: //www. nku. edu/~frank/csc 301/Examples/PHP_String s/strtok_php. pdf 19
![Joining splitting strings with string functions string substrstring str int start int length Joining & splitting strings with string functions string substr(string $str, int $start[, int $length])](https://slidetodoc.com/presentation_image_h/a17971a3ebc331a2ac56456789a99d6d/image-20.jpg)
Joining & splitting strings with string functions string substr(string $str, int $start[, int $length]) Called with 1 positive argument (start): returns the substring of $str from the $start position to the end of the string Called with 1 negative argument (start): returns the substring of $str from the end of the string - |$start| characters to the end of the string Called with 2 arguments (start and length): If $length is positive: specifies the number of characters to return starting from position $start If $length is negative: function returns the substring from $start position to the end of the string - |$length | position Note: string position starts at 0 Extracting a single character: $string{$index} $index is 0 -based character count from start of string 20
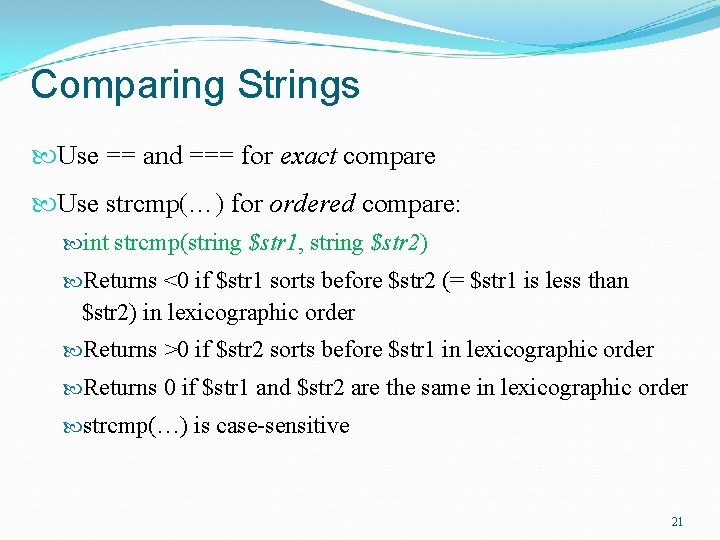
Comparing Strings Use == and === for exact compare Use strcmp(…) for ordered compare: int strcmp(string $str 1, string $str 2) Returns <0 if $str 1 sorts before $str 2 (= $str 1 is less than $str 2) in lexicographic order Returns >0 if $str 2 sorts before $str 1 in lexicographic order Returns 0 if $str 1 and $str 2 are the same in lexicographic order strcmp(…) is case-sensitive 21
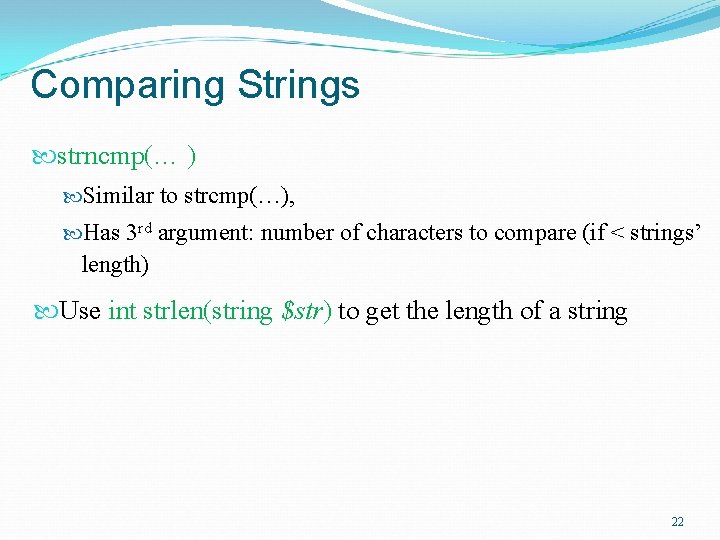
Comparing Strings strncmp(… ) Similar to strcmp(…), Has 3 rd argument: number of characters to compare (if < strings’ length) Use int strlen(string $str) to get the length of a string 22
Insidan region jh
What are permutations and combinations
Web.facebook.com.php
Php php://input
Difference between note making and note taking
Signal words example
Difference between note making and note taking
Financial documents in order
What is debit note
Nota debit adalah
Note taking and note making
Simple discount example problems with solutions
Assembly array of strings
Strings in java
Spring problems mechanics
Flexible pattern matching in strings
Rate of energy transfer by sinusoidal waves on strings
Comparing strings in python
Pointers and strings
Sludoy bamboo zither drawing
Micheal league
Rhyming strings
A type of cipher that uses multiple alphabetic strings.