Memento Design Pattern CECS 277 Fall 2017 Mimi
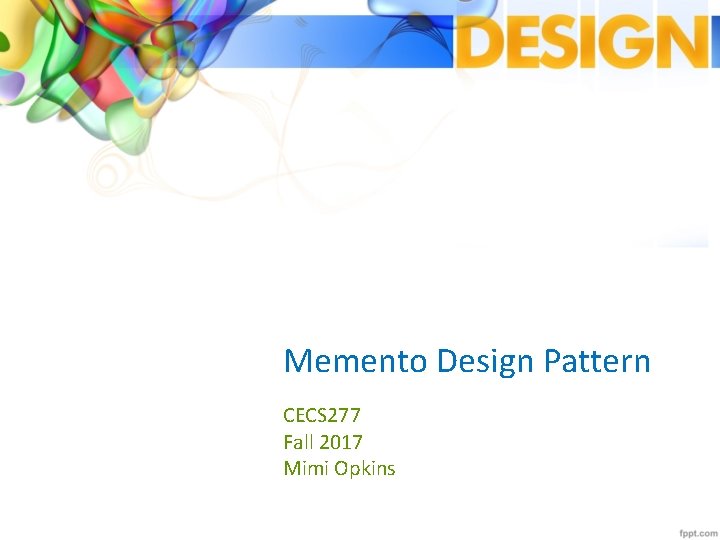
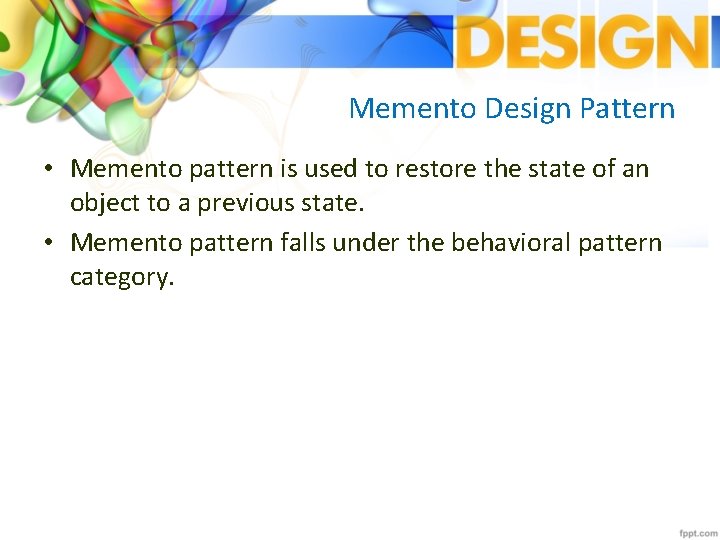
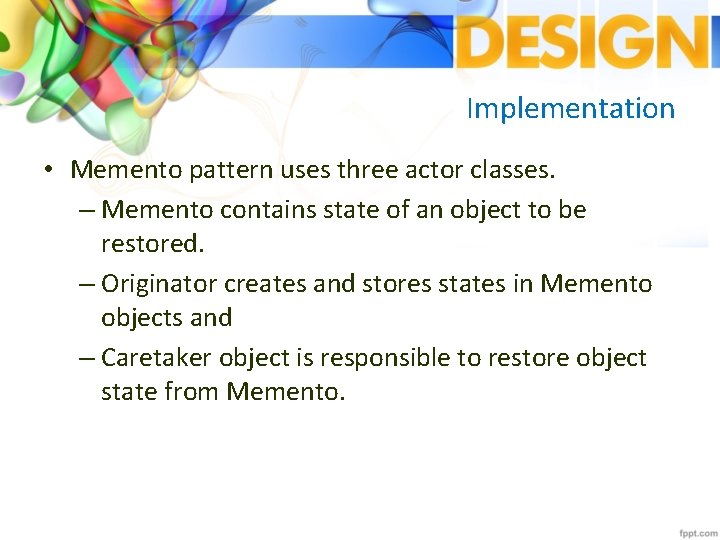
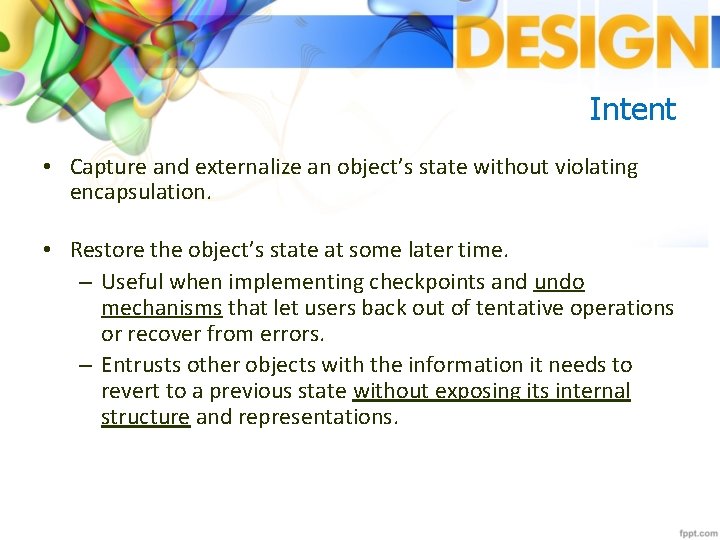
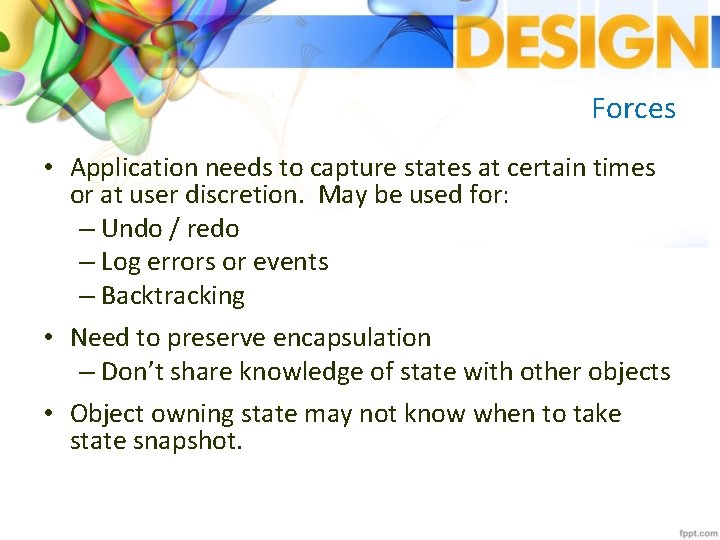
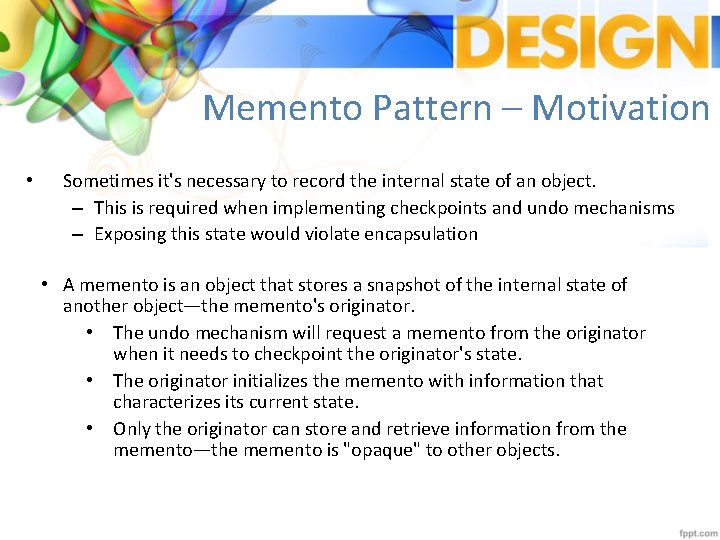
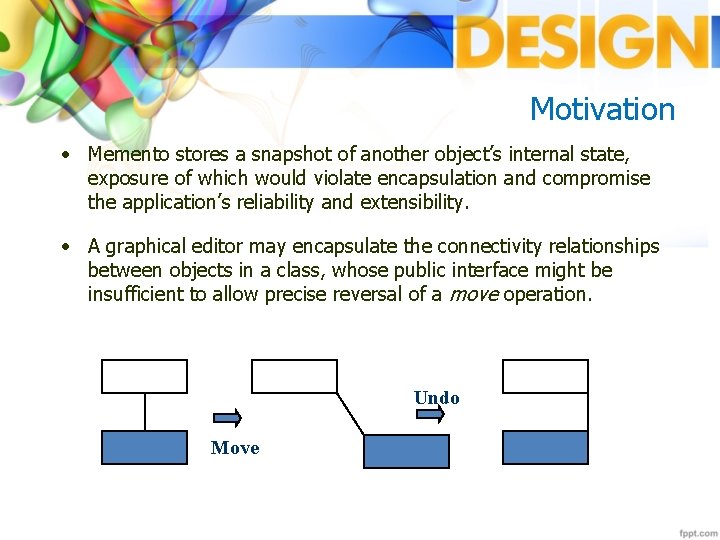
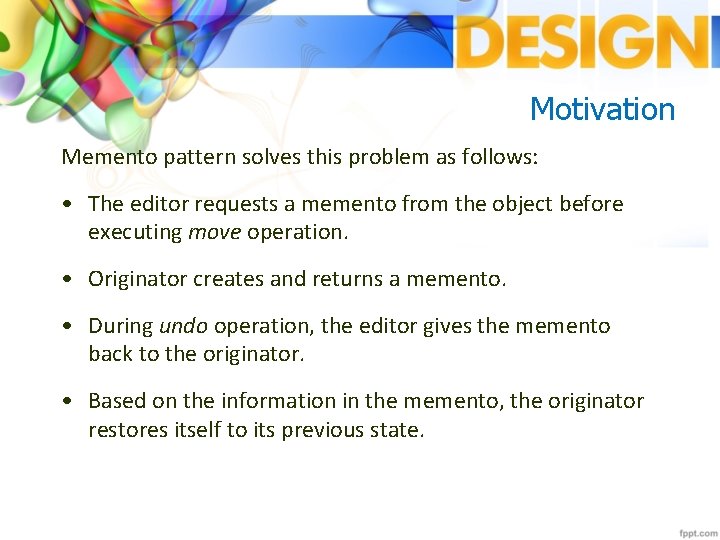
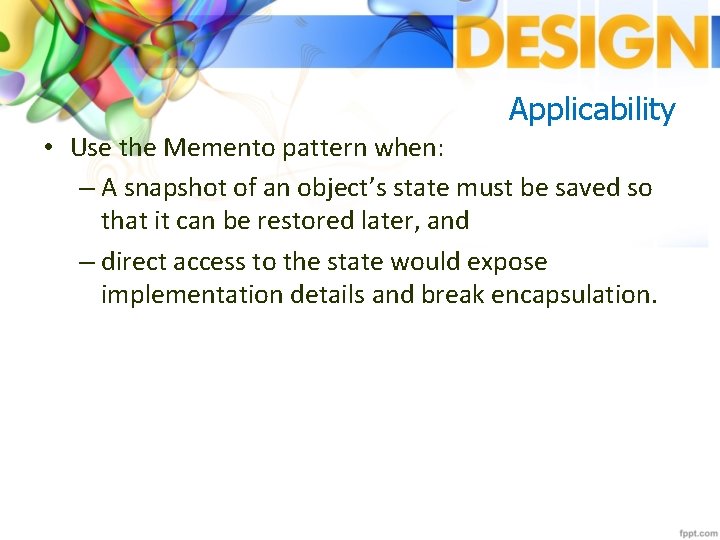
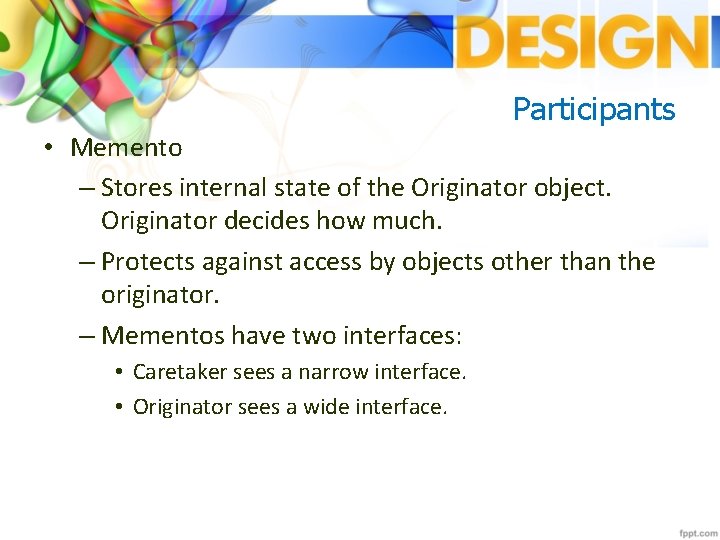
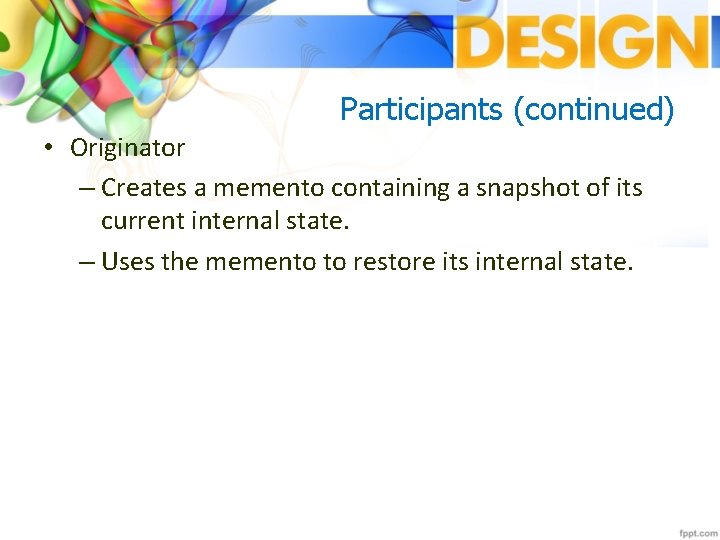
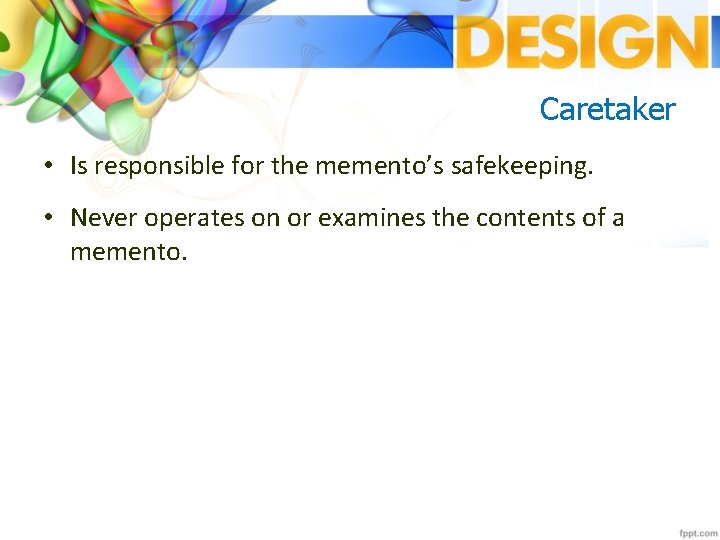
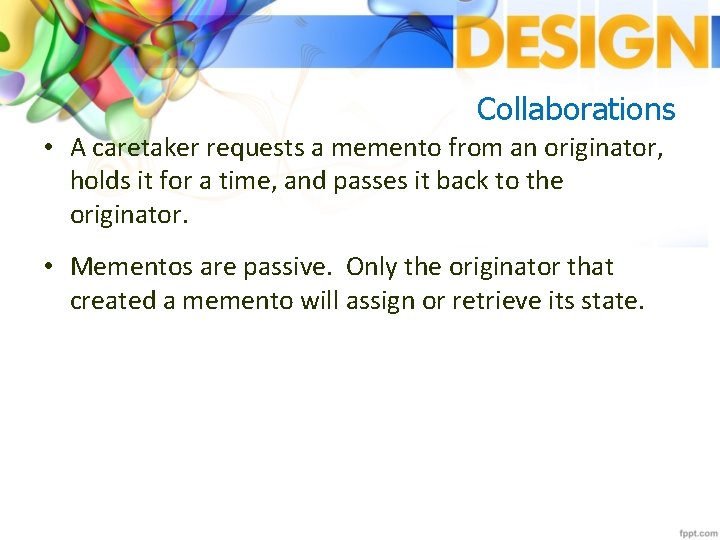
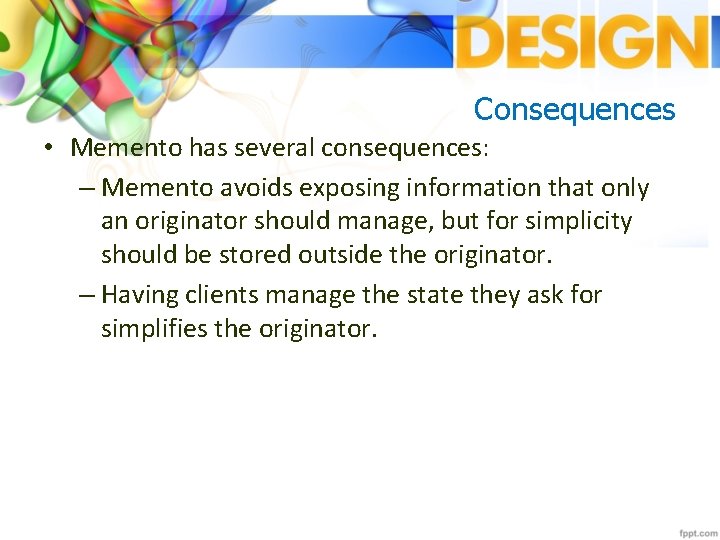
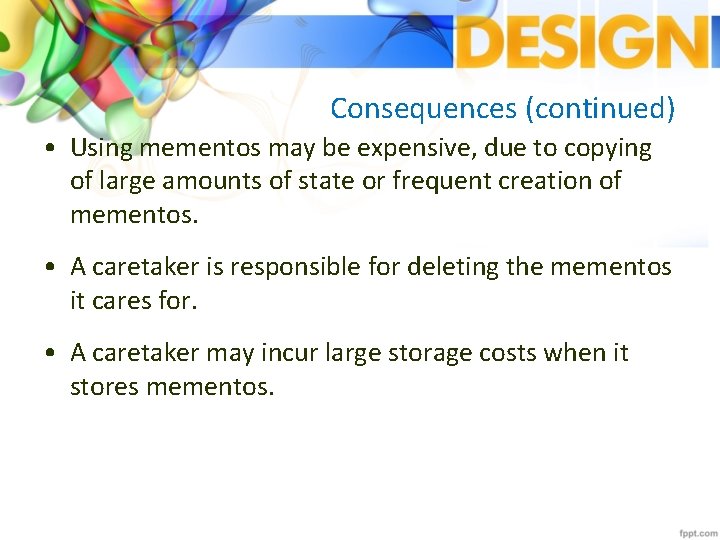
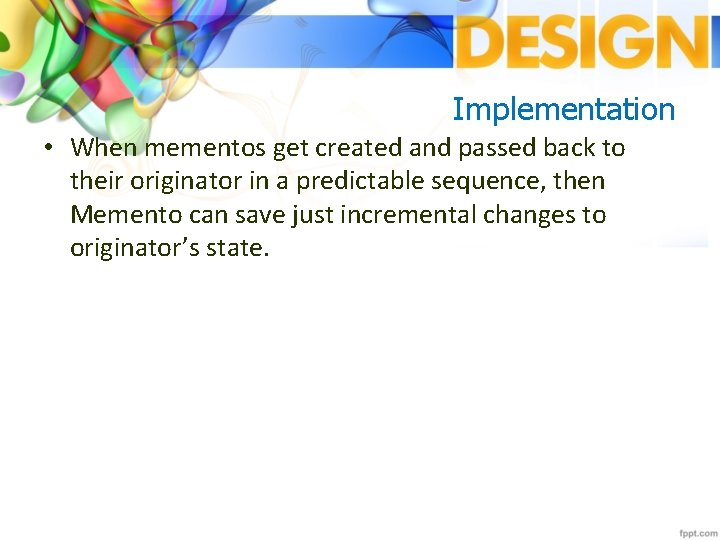
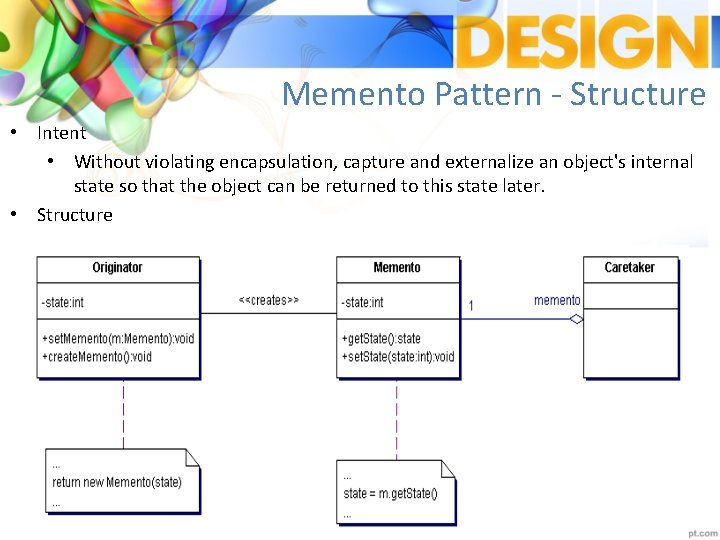
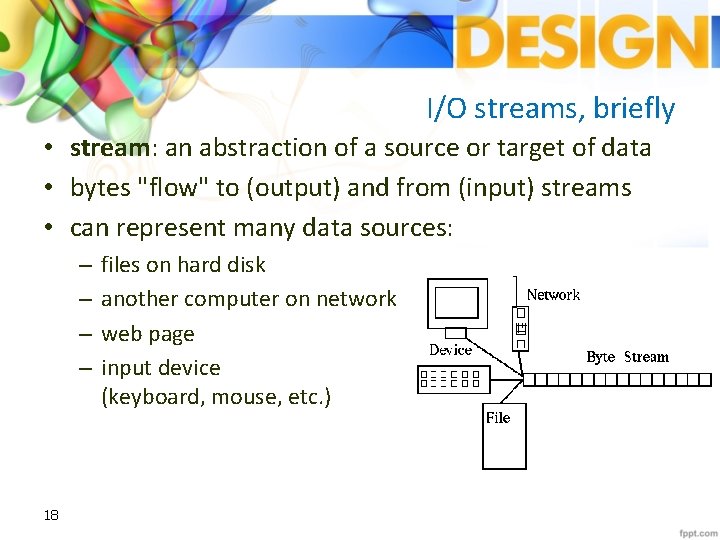
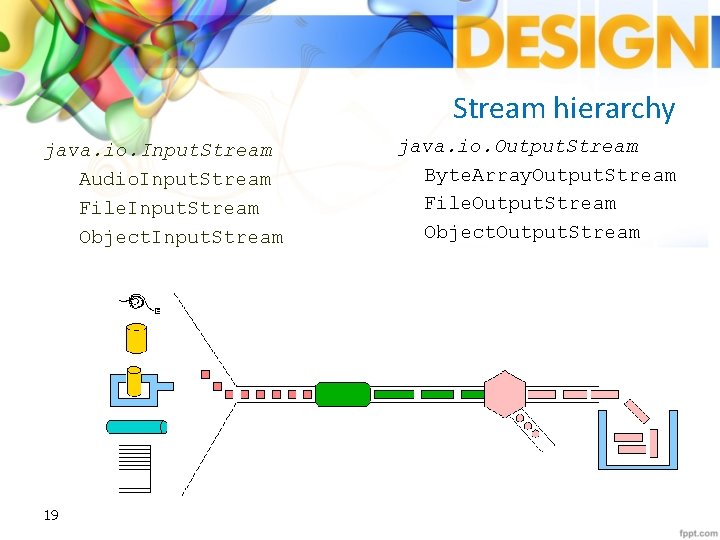
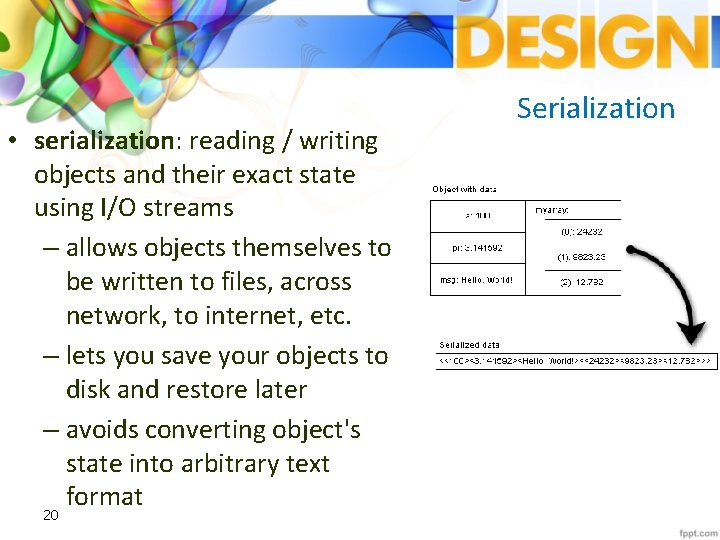
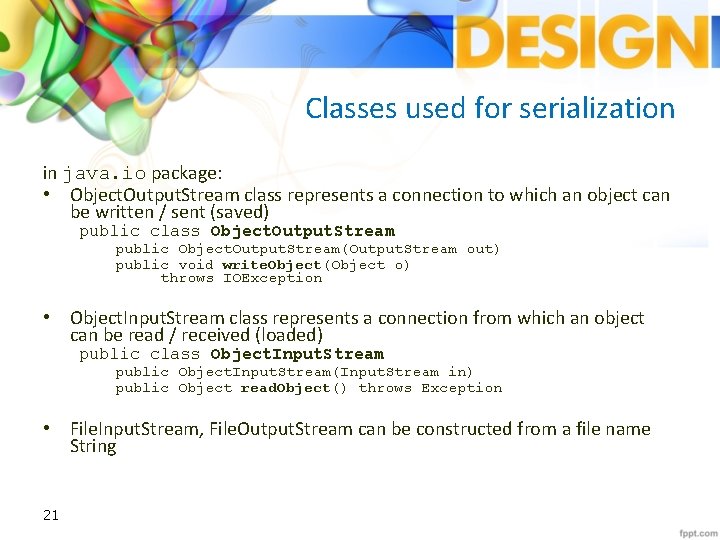
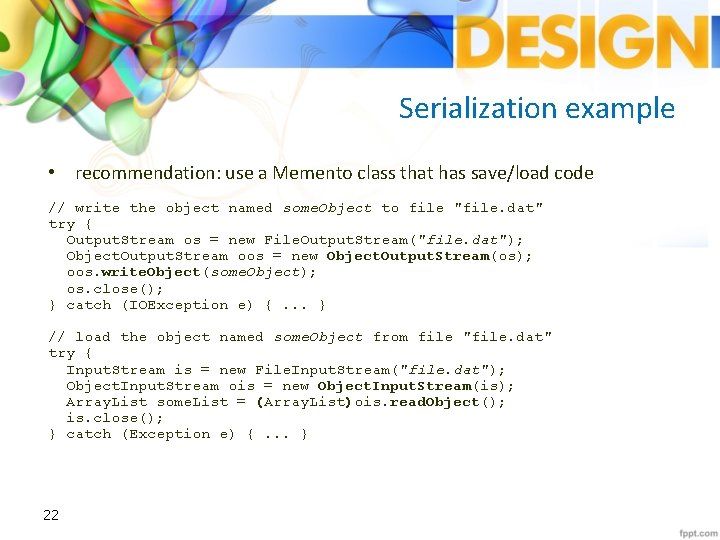
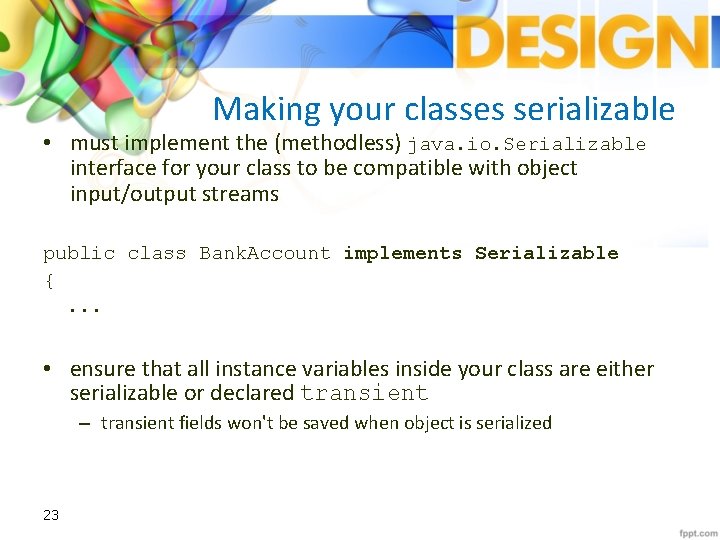
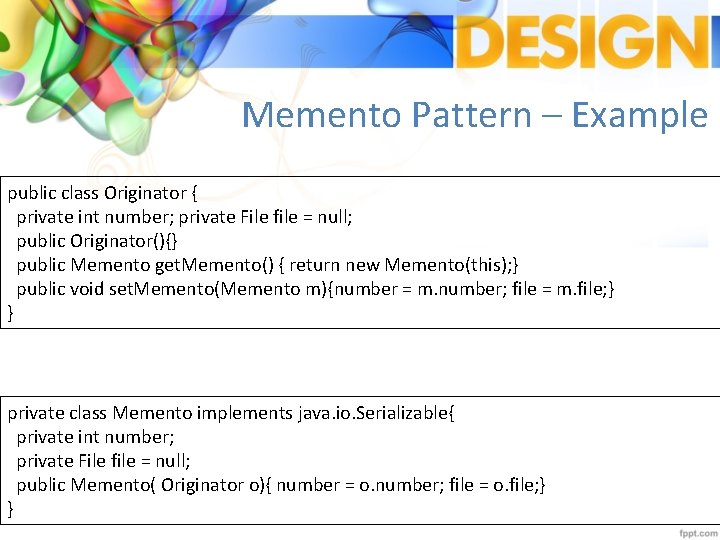
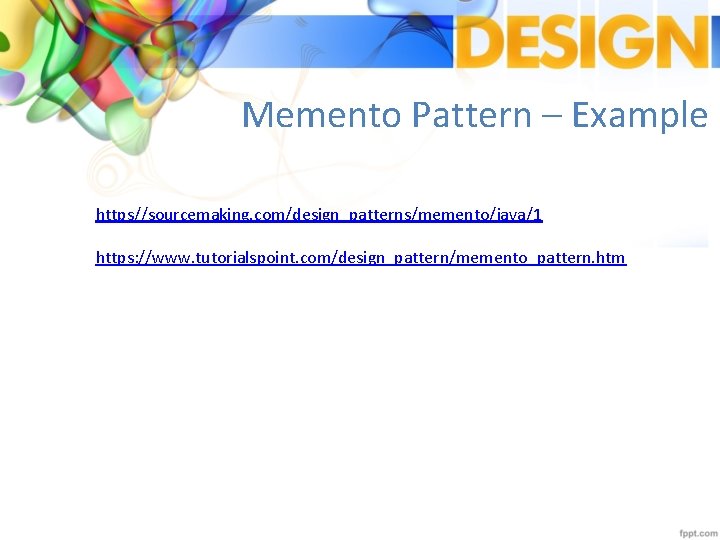
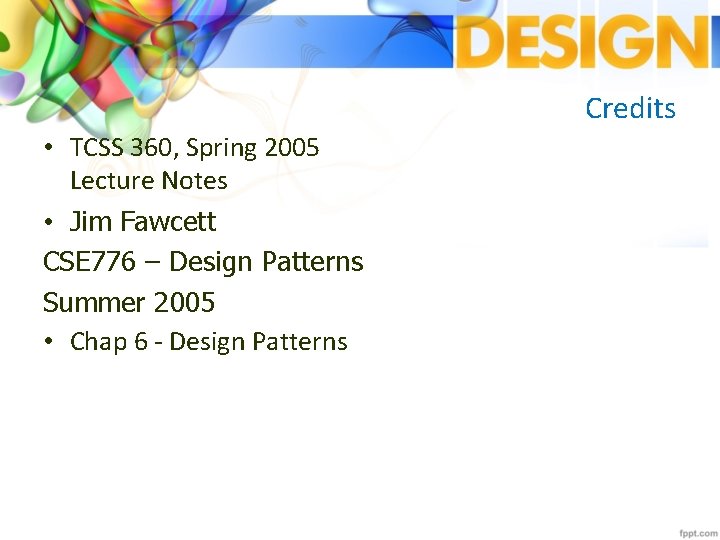
- Slides: 26
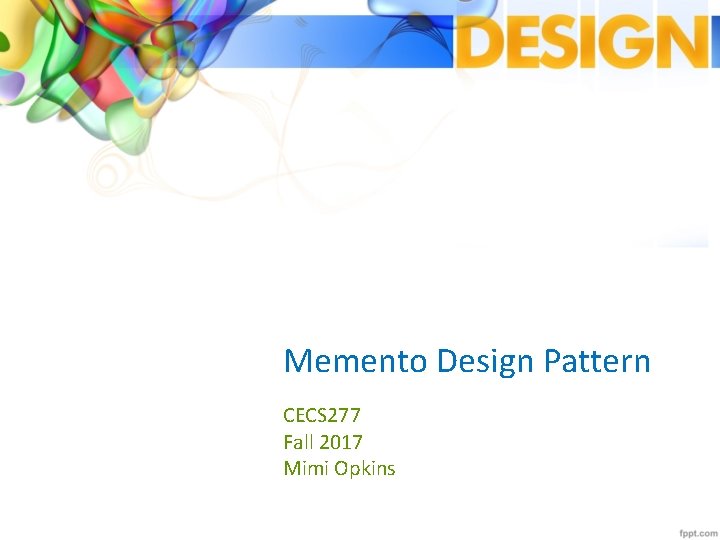
Memento Design Pattern CECS 277 Fall 2017 Mimi Opkins
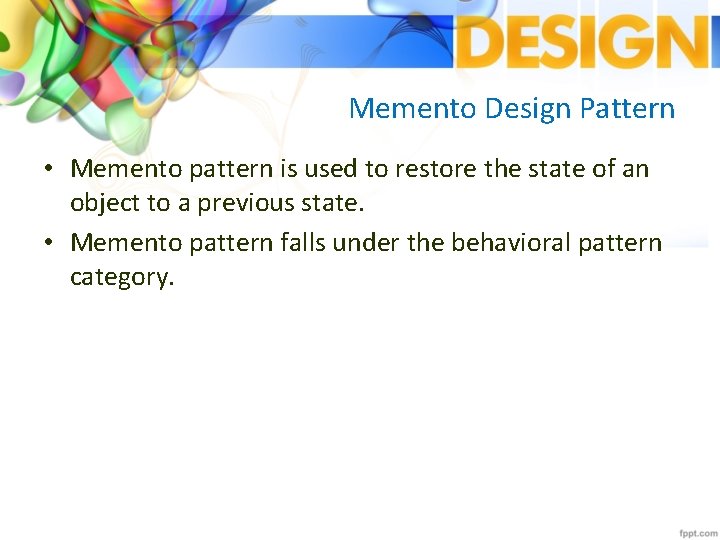
Memento Design Pattern • Memento pattern is used to restore the state of an object to a previous state. • Memento pattern falls under the behavioral pattern category.
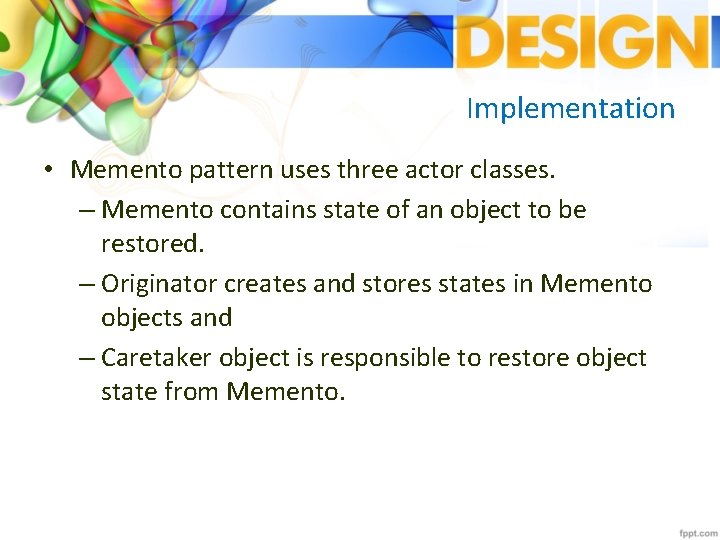
Implementation • Memento pattern uses three actor classes. – Memento contains state of an object to be restored. – Originator creates and stores states in Memento objects and – Caretaker object is responsible to restore object state from Memento.
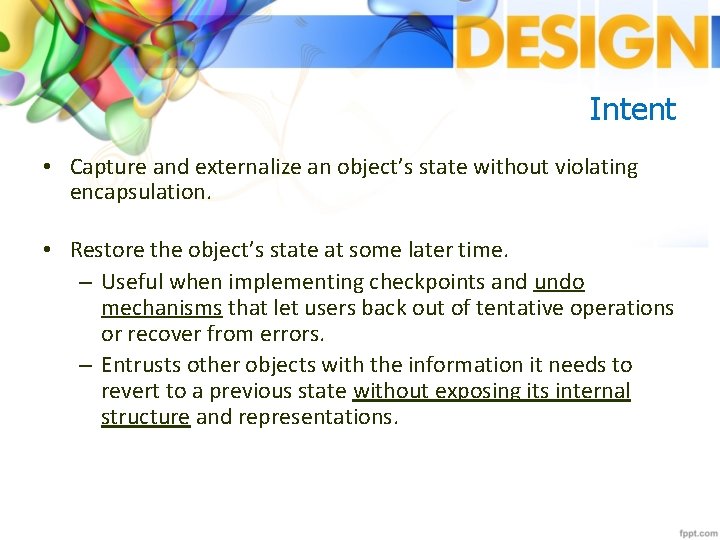
Intent • Capture and externalize an object’s state without violating encapsulation. • Restore the object’s state at some later time. – Useful when implementing checkpoints and undo mechanisms that let users back out of tentative operations or recover from errors. – Entrusts other objects with the information it needs to revert to a previous state without exposing its internal structure and representations.
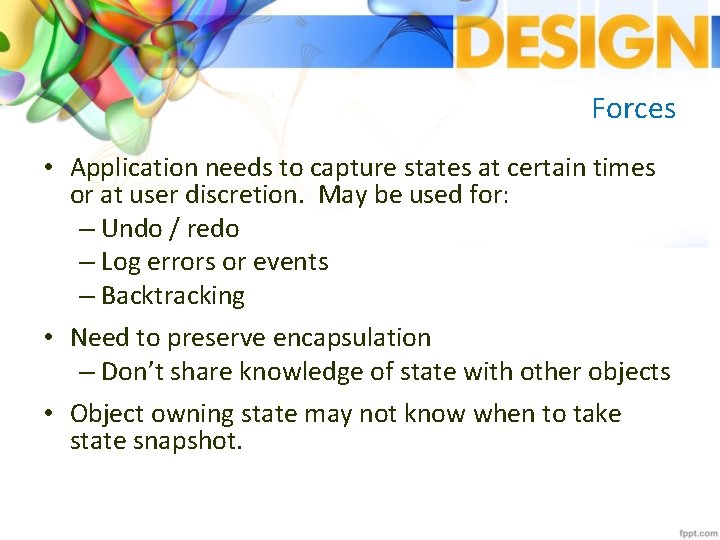
Forces • Application needs to capture states at certain times or at user discretion. May be used for: – Undo / redo – Log errors or events – Backtracking • Need to preserve encapsulation – Don’t share knowledge of state with other objects • Object owning state may not know when to take state snapshot.
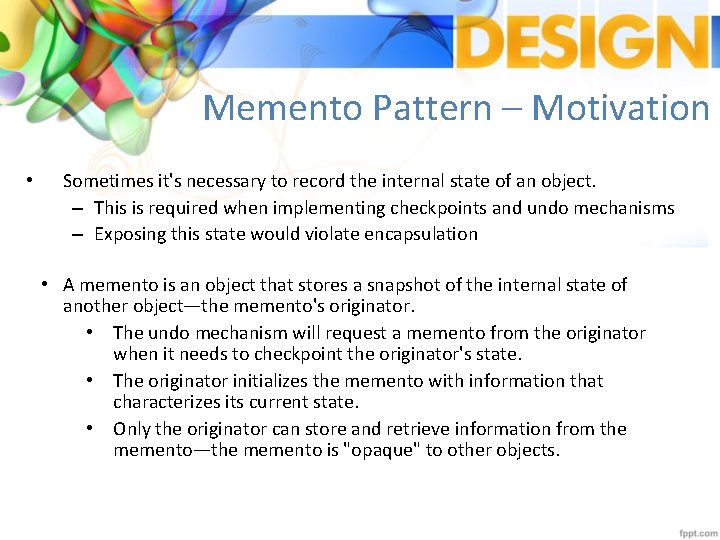
Memento Pattern – Motivation • Sometimes it's necessary to record the internal state of an object. – This is required when implementing checkpoints and undo mechanisms – Exposing this state would violate encapsulation • A memento is an object that stores a snapshot of the internal state of another object—the memento's originator. • The undo mechanism will request a memento from the originator when it needs to checkpoint the originator's state. • The originator initializes the memento with information that characterizes its current state. • Only the originator can store and retrieve information from the memento—the memento is "opaque" to other objects.
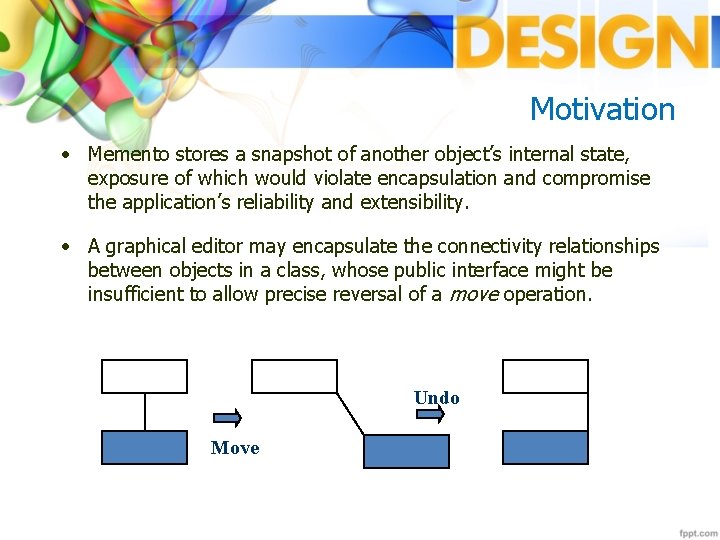
Motivation • Memento stores a snapshot of another object’s internal state, exposure of which would violate encapsulation and compromise the application’s reliability and extensibility. • A graphical editor may encapsulate the connectivity relationships between objects in a class, whose public interface might be insufficient to allow precise reversal of a move operation. Undo Move
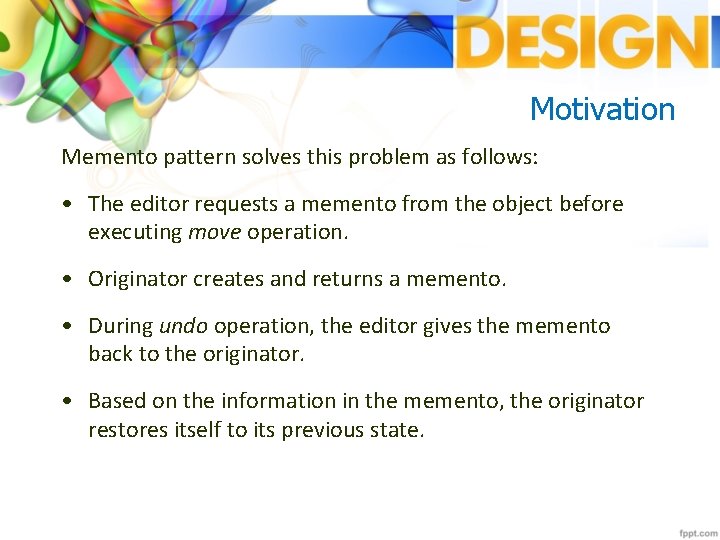
Motivation Memento pattern solves this problem as follows: • The editor requests a memento from the object before executing move operation. • Originator creates and returns a memento. • During undo operation, the editor gives the memento back to the originator. • Based on the information in the memento, the originator restores itself to its previous state.
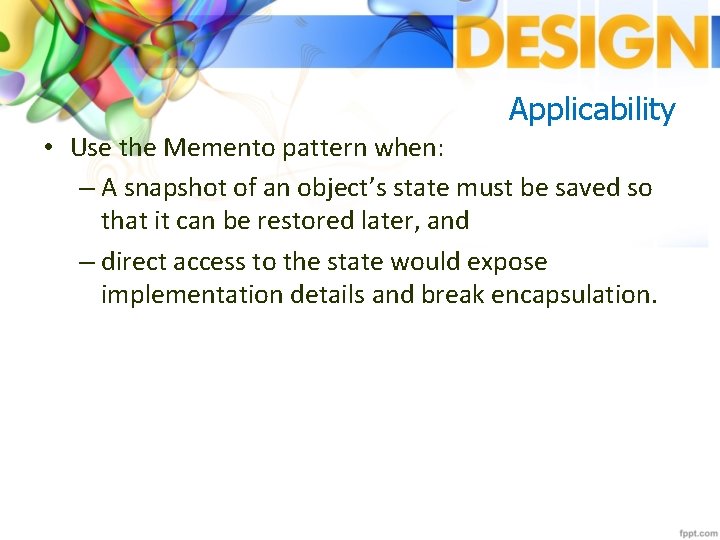
Applicability • Use the Memento pattern when: – A snapshot of an object’s state must be saved so that it can be restored later, and – direct access to the state would expose implementation details and break encapsulation.
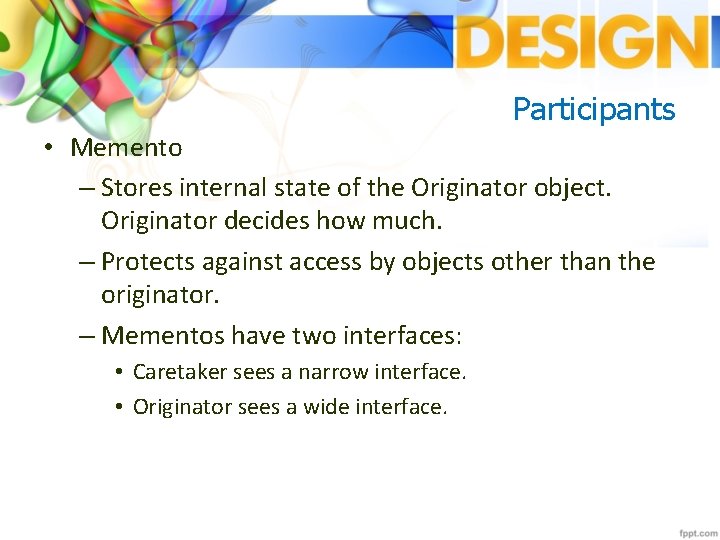
Participants • Memento – Stores internal state of the Originator object. Originator decides how much. – Protects against access by objects other than the originator. – Mementos have two interfaces: • Caretaker sees a narrow interface. • Originator sees a wide interface.
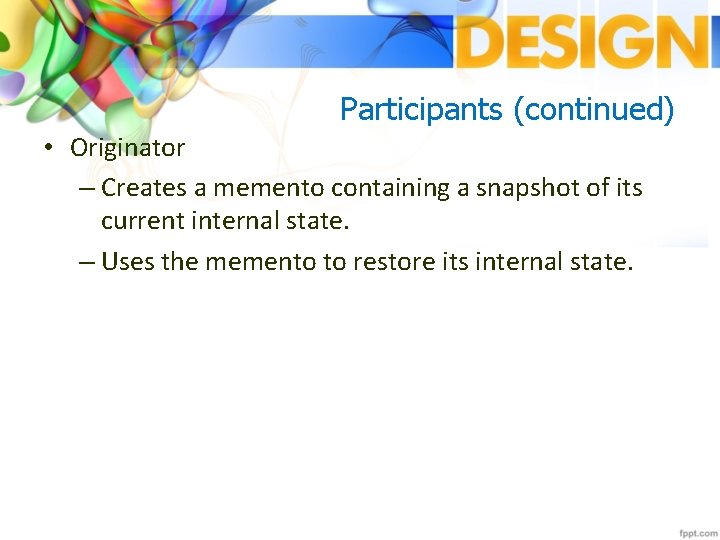
Participants (continued) • Originator – Creates a memento containing a snapshot of its current internal state. – Uses the memento to restore its internal state.
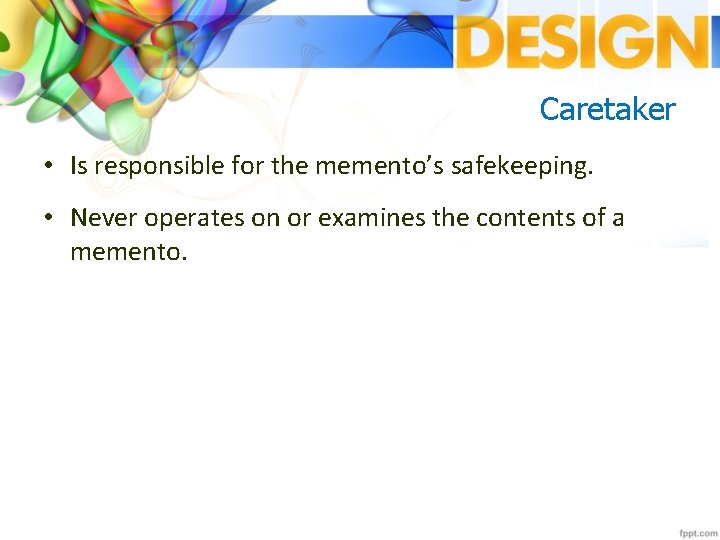
Caretaker • Is responsible for the memento’s safekeeping. • Never operates on or examines the contents of a memento.
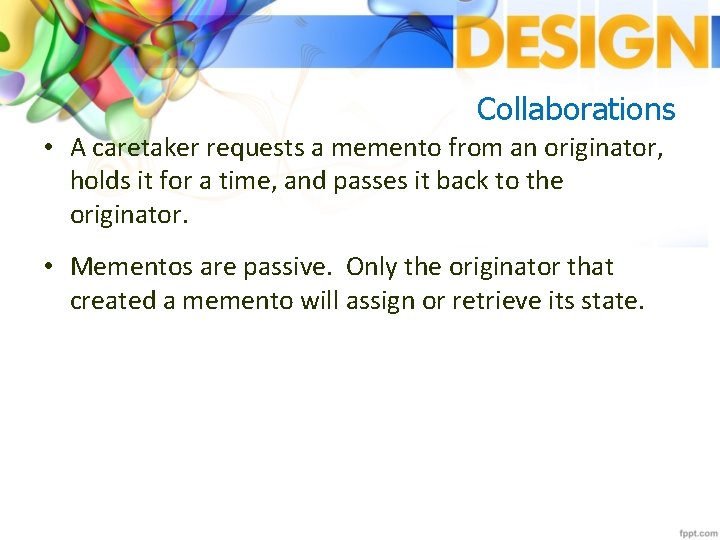
Collaborations • A caretaker requests a memento from an originator, holds it for a time, and passes it back to the originator. • Mementos are passive. Only the originator that created a memento will assign or retrieve its state.
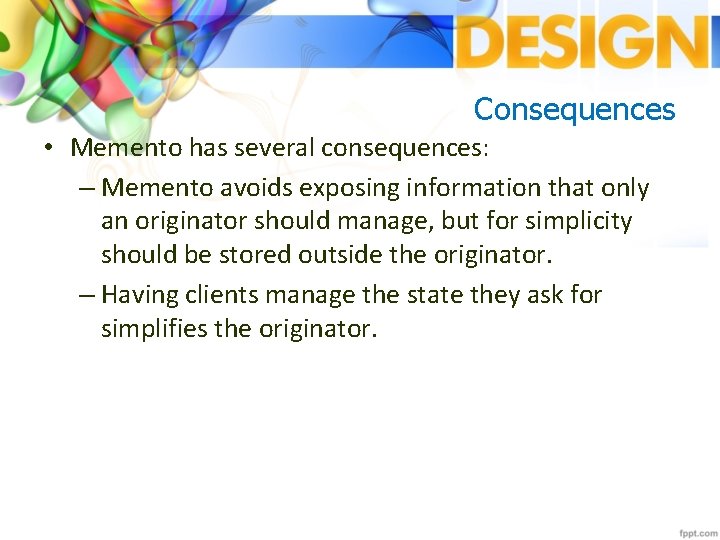
Consequences • Memento has several consequences: – Memento avoids exposing information that only an originator should manage, but for simplicity should be stored outside the originator. – Having clients manage the state they ask for simplifies the originator.
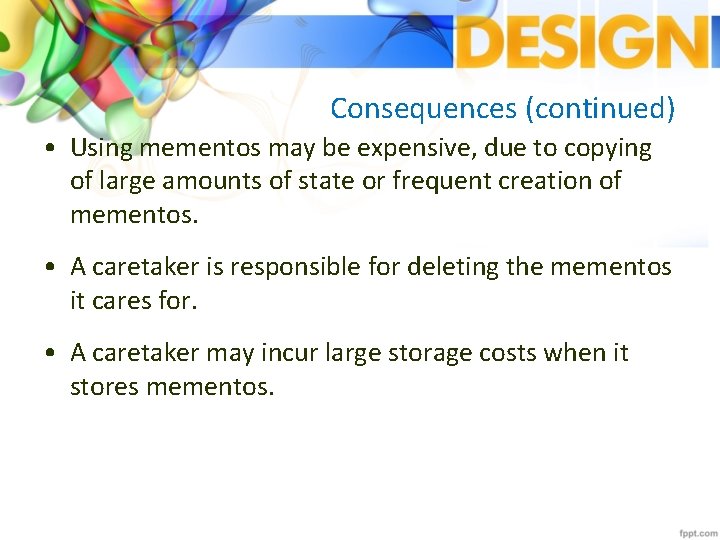
Consequences (continued) • Using mementos may be expensive, due to copying of large amounts of state or frequent creation of mementos. • A caretaker is responsible for deleting the mementos it cares for. • A caretaker may incur large storage costs when it stores mementos.
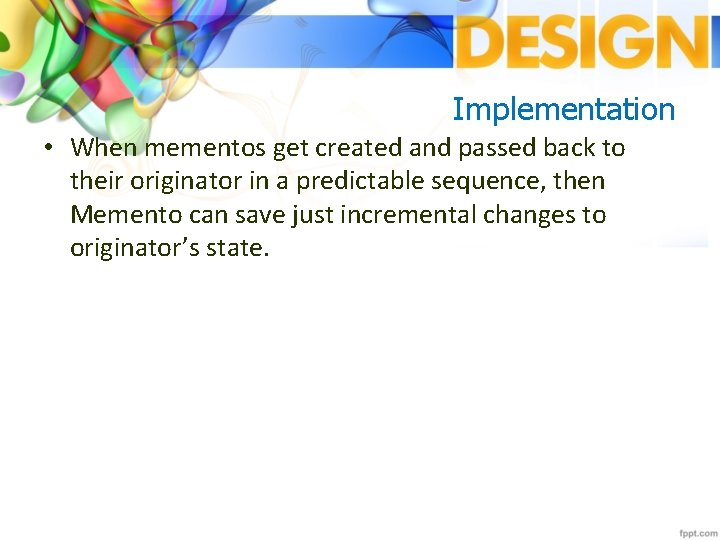
Implementation • When mementos get created and passed back to their originator in a predictable sequence, then Memento can save just incremental changes to originator’s state.
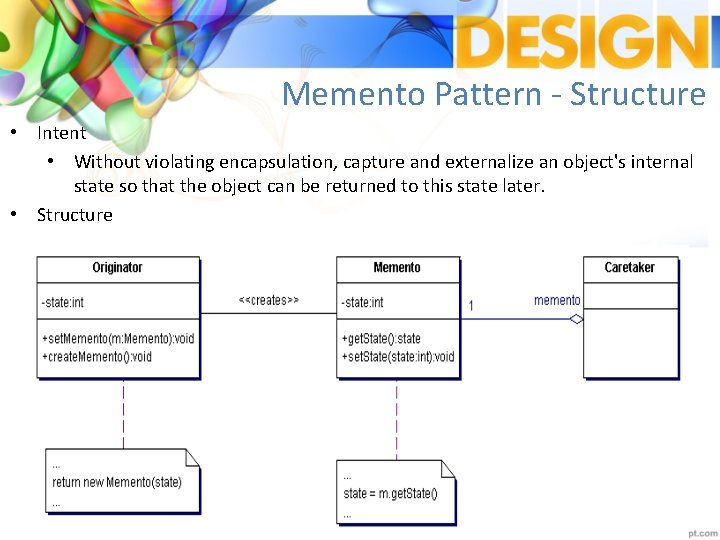
Memento Pattern - Structure • Intent • Without violating encapsulation, capture and externalize an object's internal state so that the object can be returned to this state later. • Structure
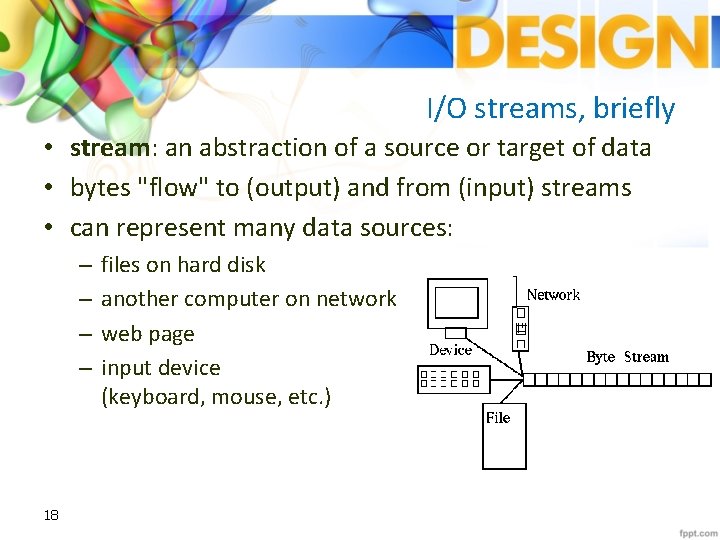
I/O streams, briefly • stream: an abstraction of a source or target of data • bytes "flow" to (output) and from (input) streams • can represent many data sources: – – 18 files on hard disk another computer on network web page input device (keyboard, mouse, etc. )
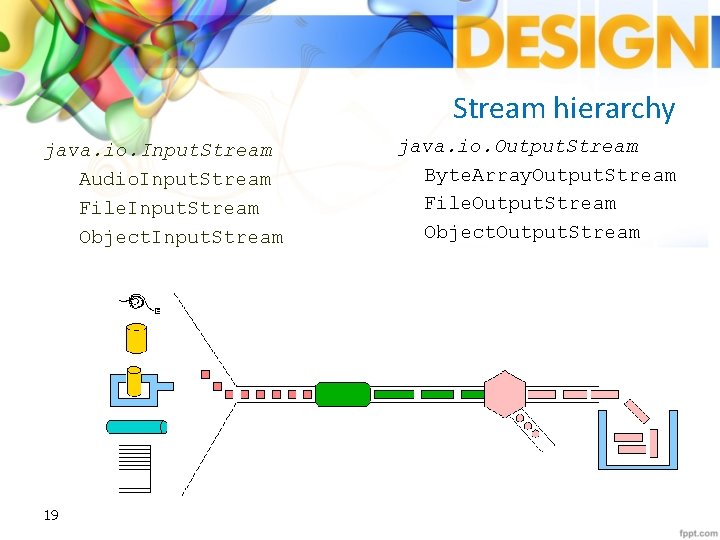
Stream hierarchy java. io. Input. Stream Audio. Input. Stream File. Input. Stream Object. Input. Stream 19 java. io. Output. Stream Byte. Array. Output. Stream File. Output. Stream Object. Output. Stream
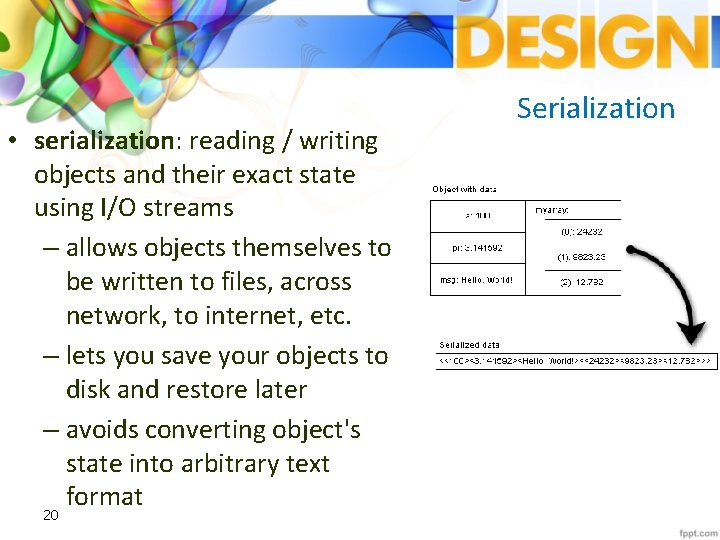
• serialization: reading / writing objects and their exact state using I/O streams – allows objects themselves to be written to files, across network, to internet, etc. – lets you save your objects to disk and restore later – avoids converting object's state into arbitrary text format 20 Serialization
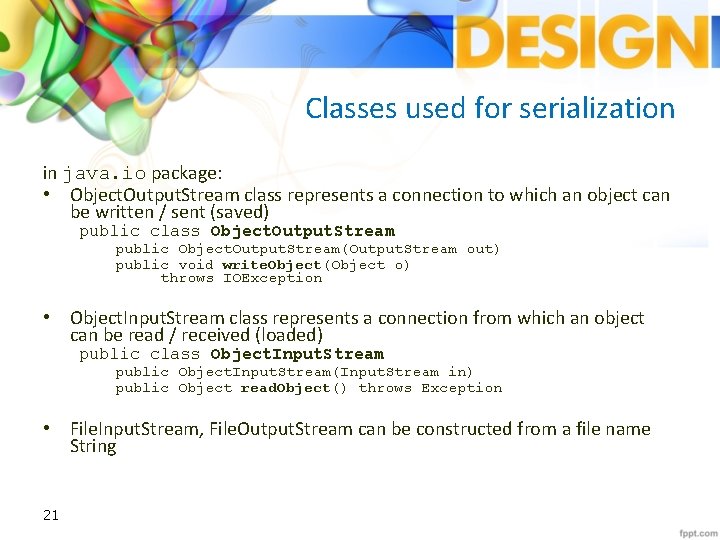
Classes used for serialization in java. io package: • Object. Output. Stream class represents a connection to which an object can be written / sent (saved) public class Object. Output. Stream public Object. Output. Stream(Output. Stream out) public void write. Object(Object o) throws IOException • Object. Input. Stream class represents a connection from which an object can be read / received (loaded) public class Object. Input. Stream public Object. Input. Stream(Input. Stream in) public Object read. Object() throws Exception • File. Input. Stream, File. Output. Stream can be constructed from a file name String 21
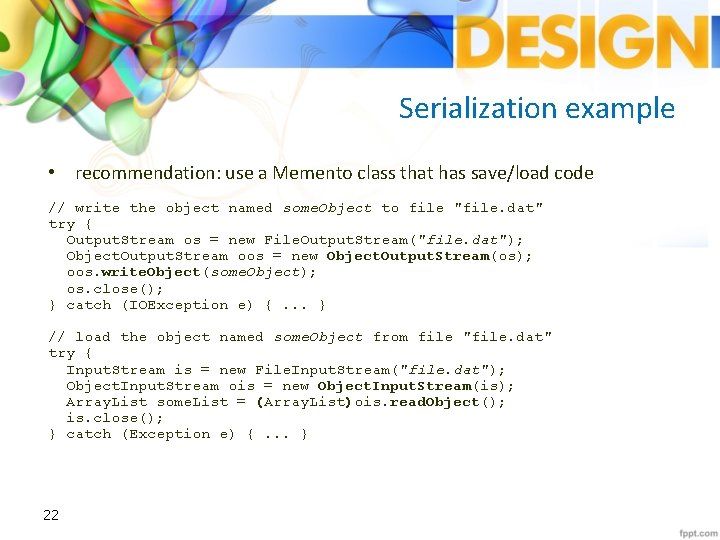
Serialization example • recommendation: use a Memento class that has save/load code // write the object named some. Object to file "file. dat" try { Output. Stream os = new File. Output. Stream("file. dat"); Object. Output. Stream oos = new Object. Output. Stream(os); oos. write. Object(some. Object); os. close(); } catch (IOException e) {. . . } // load the object named some. Object from file "file. dat" try { Input. Stream is = new File. Input. Stream("file. dat"); Object. Input. Stream ois = new Object. Input. Stream(is); Array. List some. List = (Array. List)ois. read. Object(); is. close(); } catch (Exception e) {. . . } 22
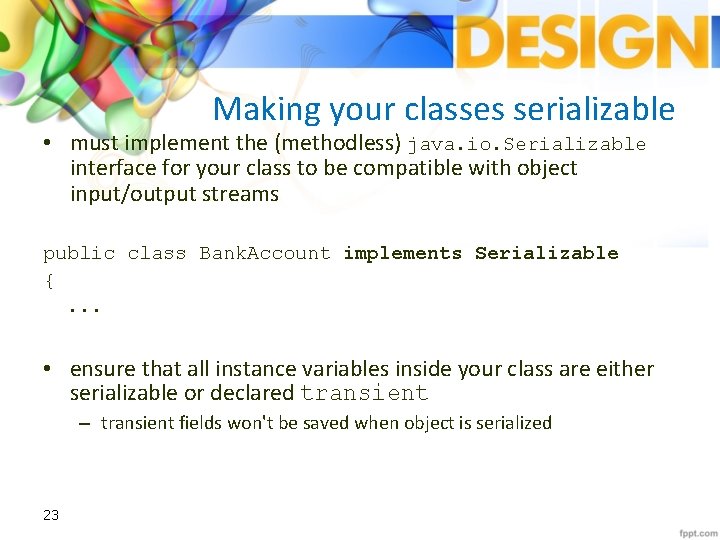
Making your classes serializable • must implement the (methodless) java. io. Serializable interface for your class to be compatible with object input/output streams public class Bank. Account implements Serializable {. . . • ensure that all instance variables inside your class are either serializable or declared transient – transient fields won't be saved when object is serialized 23
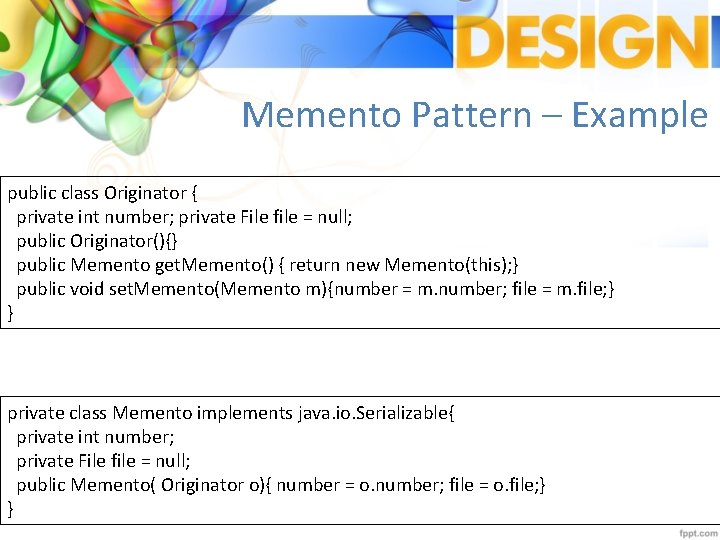
Memento Pattern – Example public class Originator { private int number; private File file = null; public Originator(){} public Memento get. Memento() { return new Memento(this); } public void set. Memento(Memento m){number = m. number; file = m. file; } } private class Memento implements java. io. Serializable{ private int number; private File file = null; public Memento( Originator o){ number = o. number; file = o. file; } }
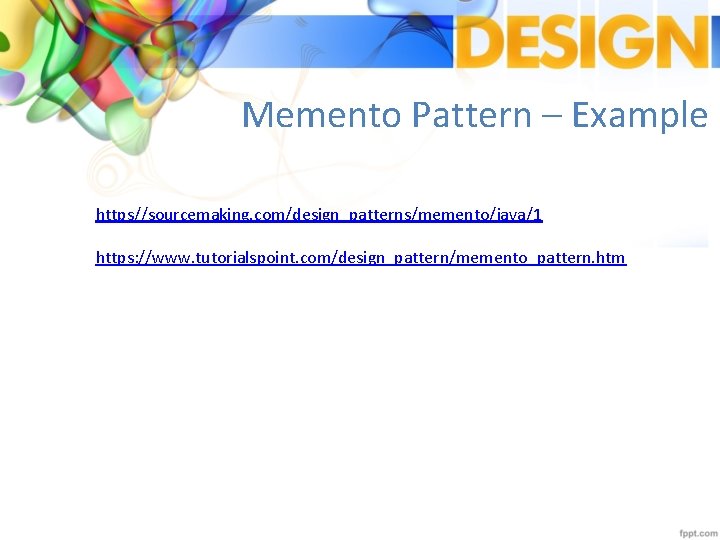
Memento Pattern – Example https//sourcemaking. com/design_patterns/memento/java/1 https: //www. tutorialspoint. com/design_pattern/memento_pattern. htm
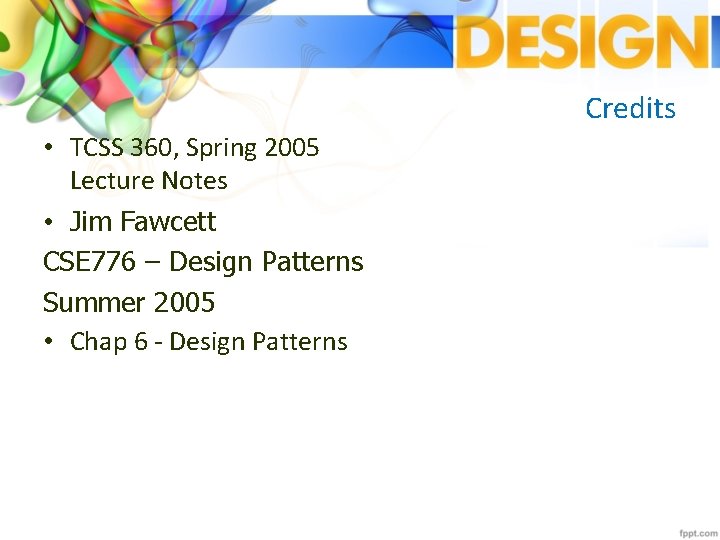
Credits • TCSS 360, Spring 2005 Lecture Notes • Jim Fawcett CSE 776 – Design Patterns Summer 2005 • Chap 6 - Design Patterns
Cecs 277
Opkins
The fire dragon species implements the reptile interface
Mimi opkins
Cecs 277
Cecs 277
De patron
Memento design pattern java
Memento pattern java
Cecs 323
Cecs 474
Cecs 474
Cecs 343
Cecs 474
Opkins
277 480 transformer bank
277/480 transformer bank
+277 isd code
277 / 60
Opa 277
2017 dvhimss annual fall conference
Barock geschichte hintergrund
Barok
Memento mori hkbp
Patron de diseño facade
Jason penny
Memento de l'élingueur