MachineLevel Programming 3 Control Flow Topics n Control
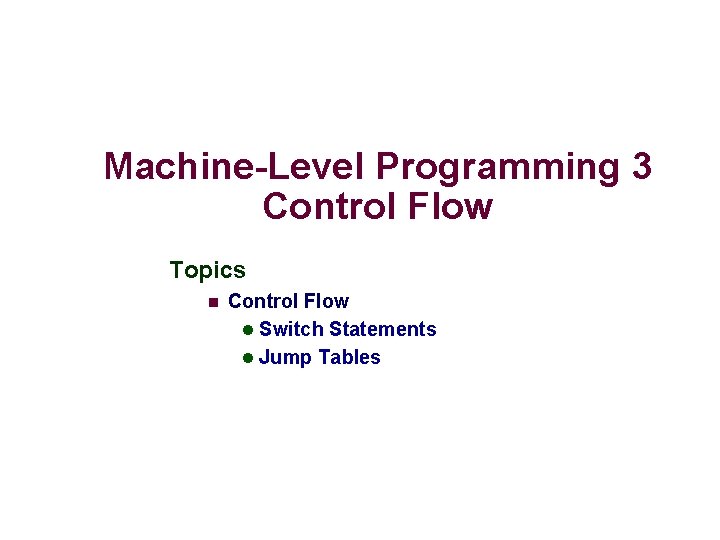
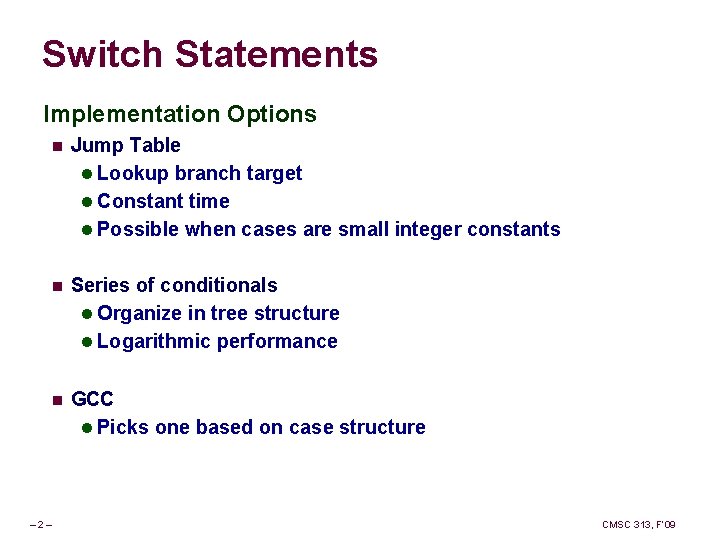
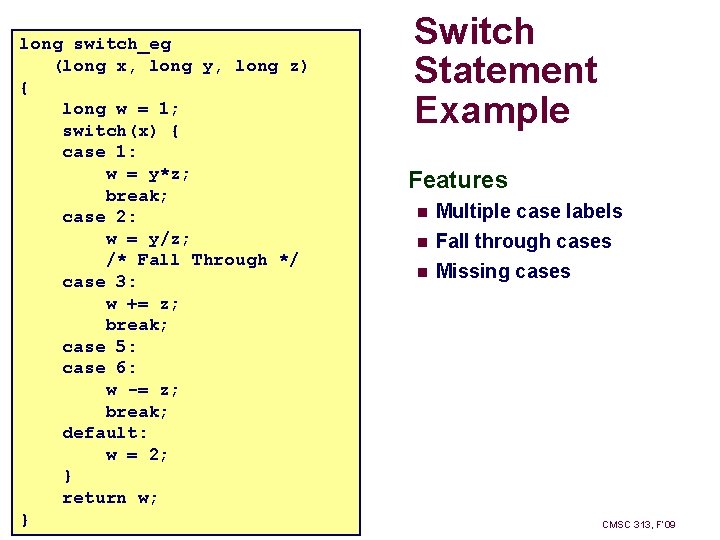
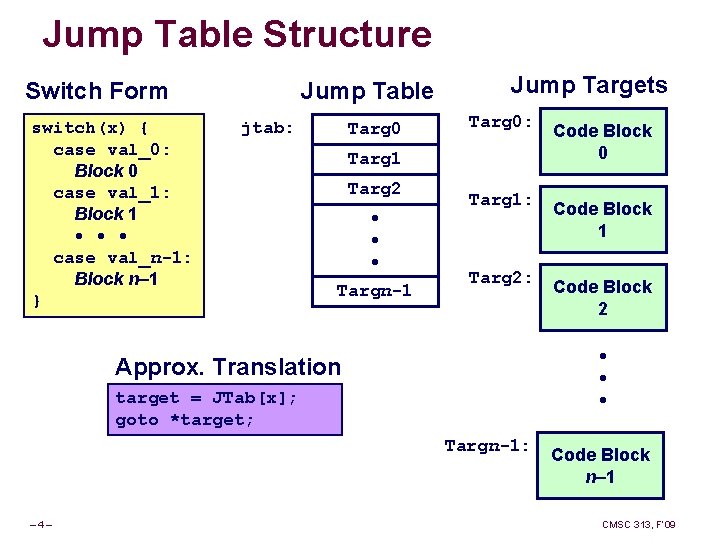
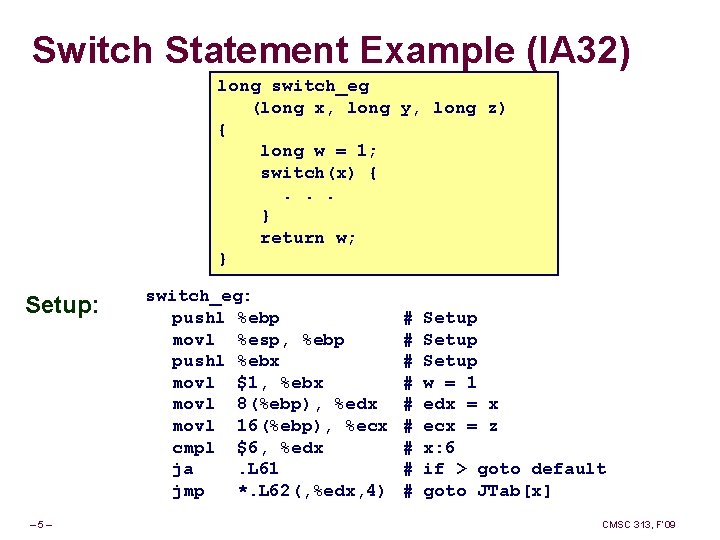
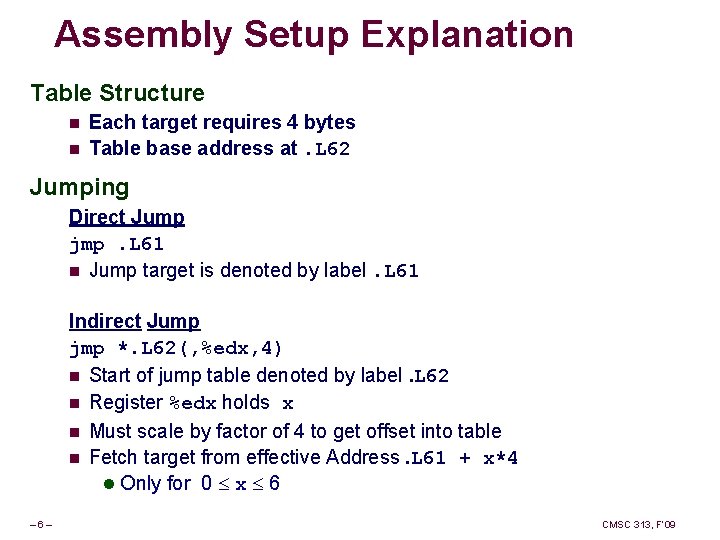
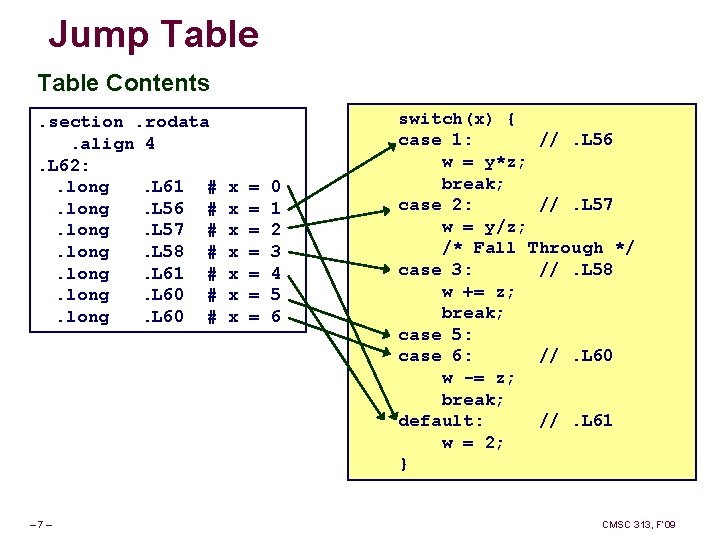
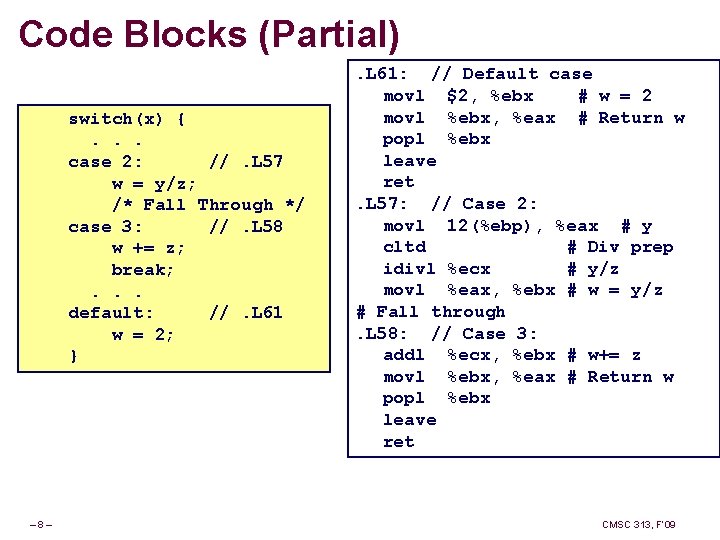
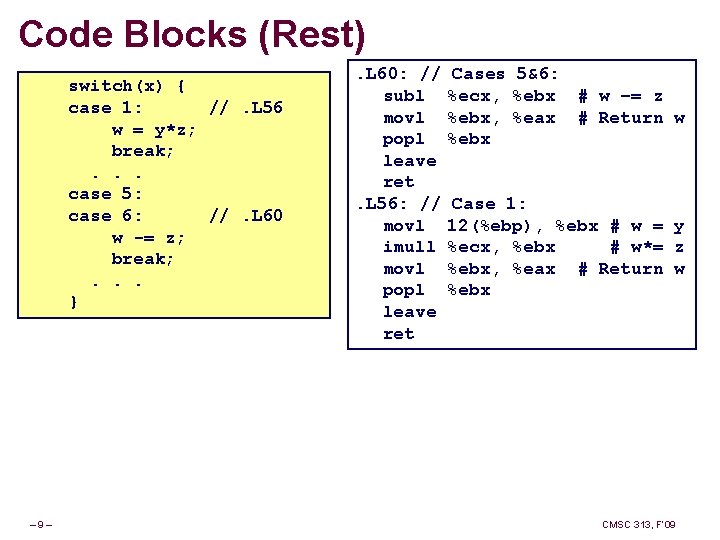
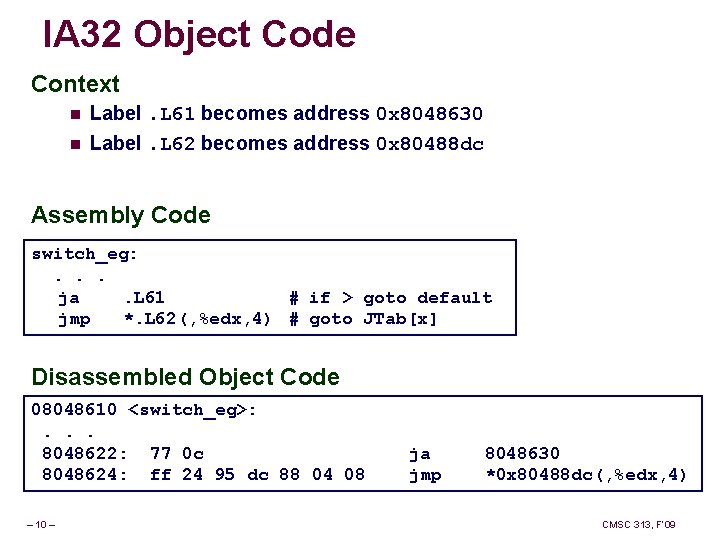
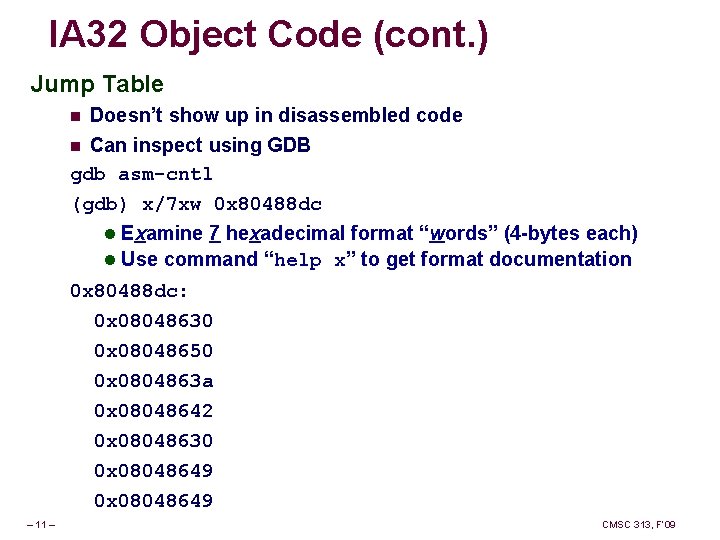
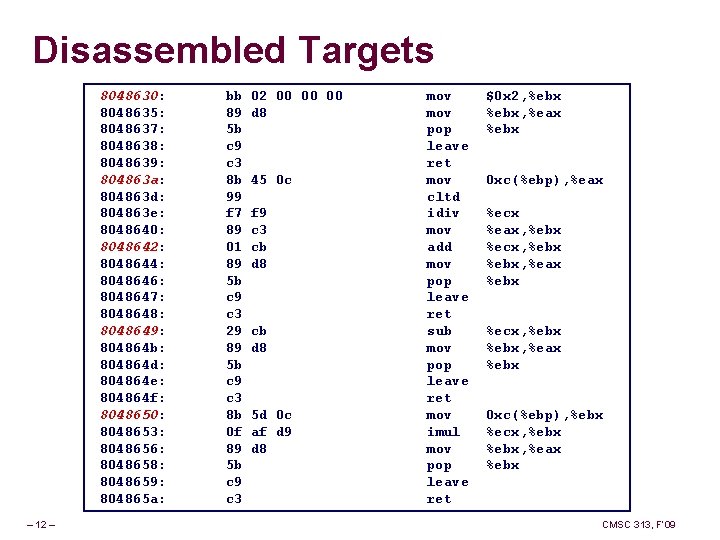
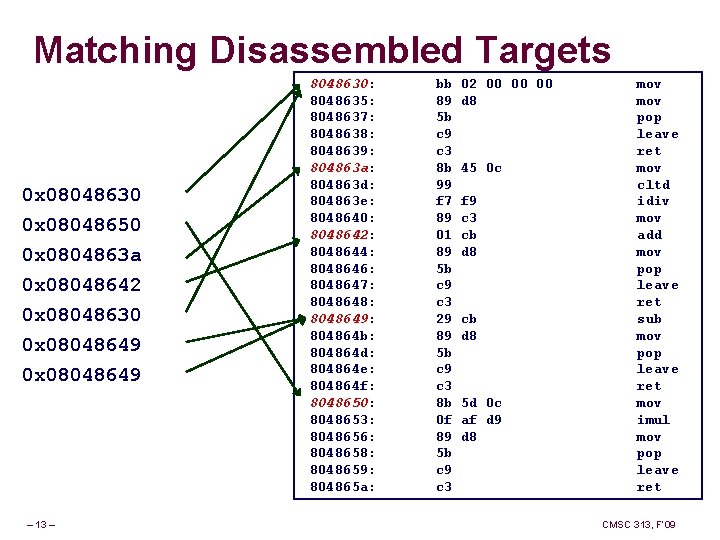
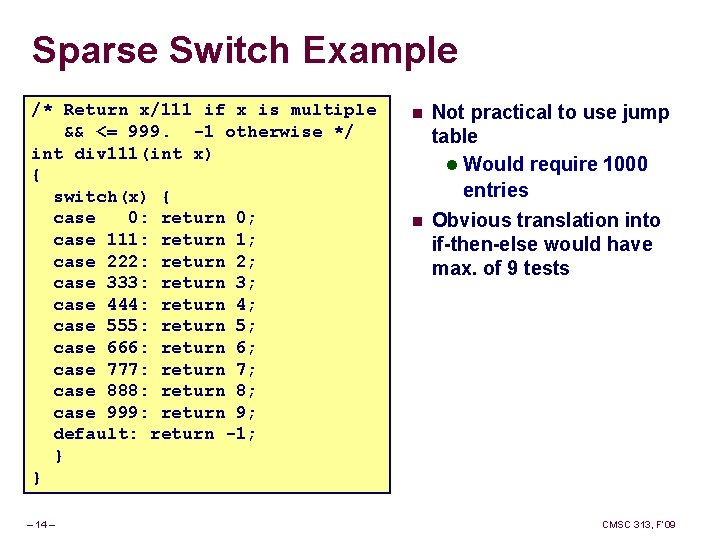
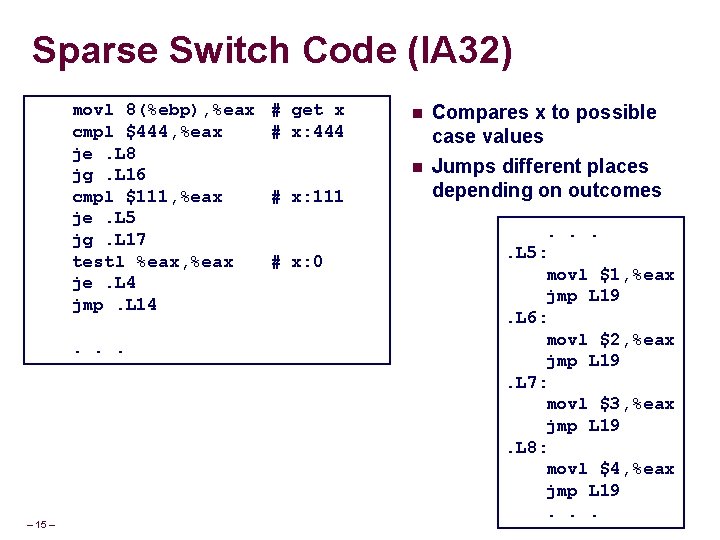
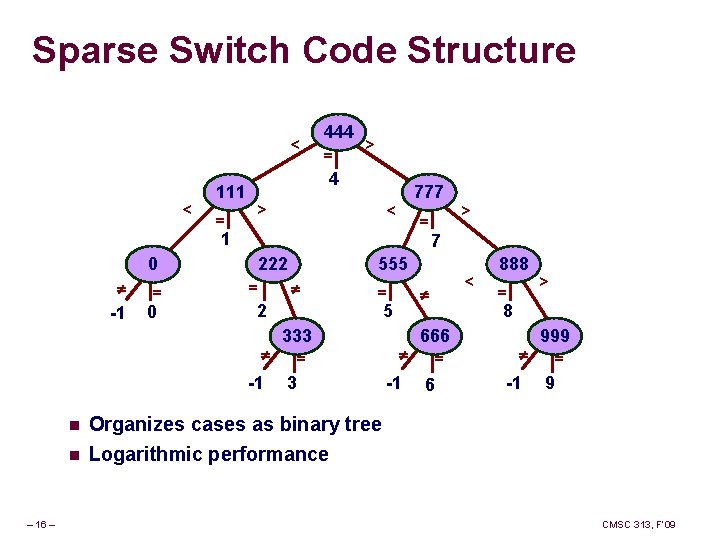
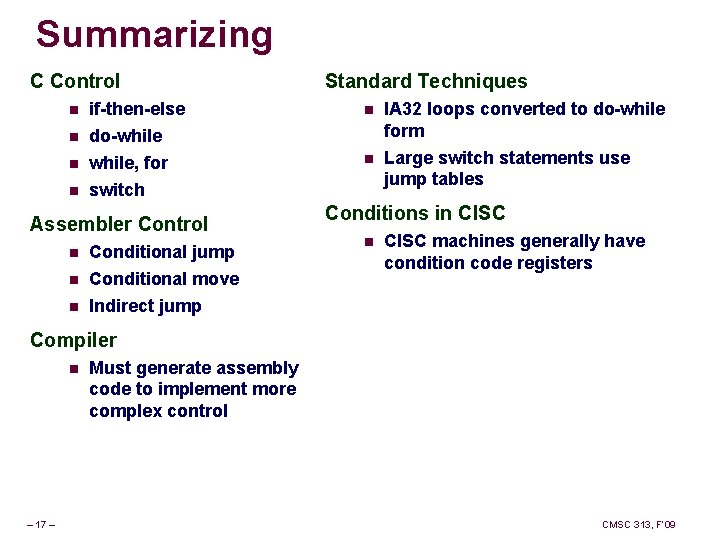
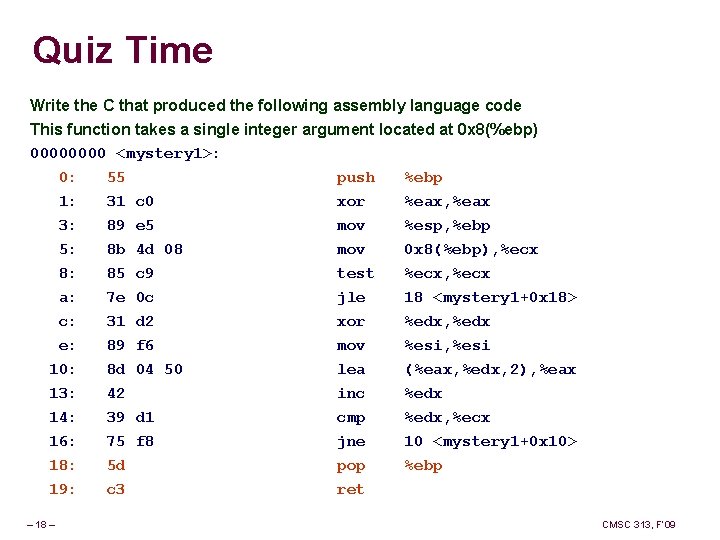
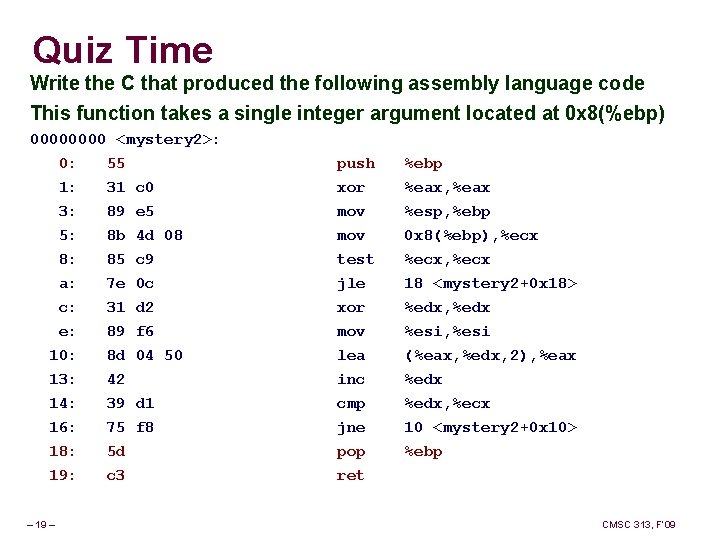
- Slides: 19
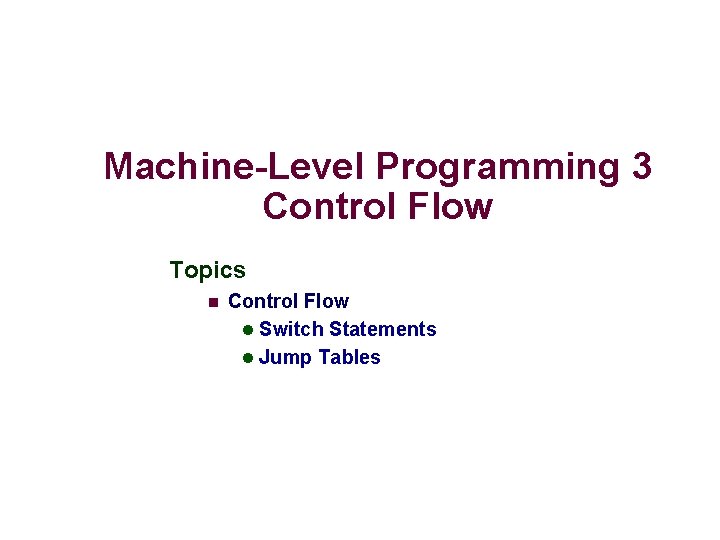
Machine-Level Programming 3 Control Flow Topics n Control Flow l Switch Statements l Jump Tables
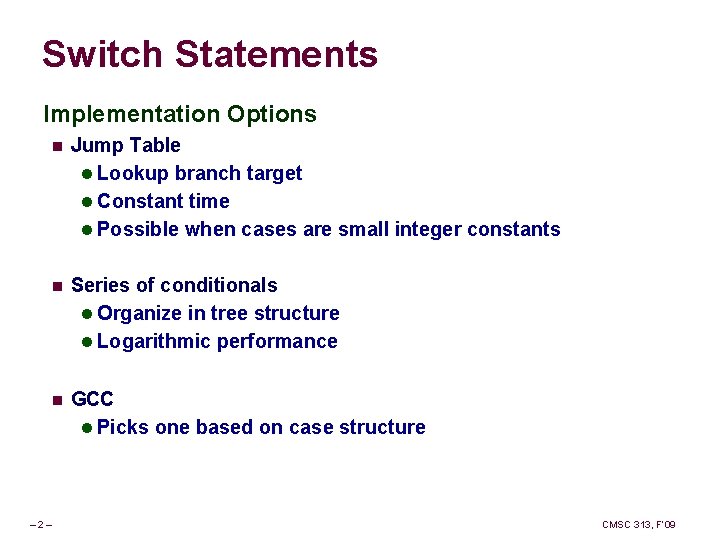
Switch Statements Implementation Options – 2– n Jump Table l Lookup branch target l Constant time l Possible when cases are small integer constants n Series of conditionals l Organize in tree structure l Logarithmic performance n GCC l Picks one based on case structure CMSC 313, F’ 09
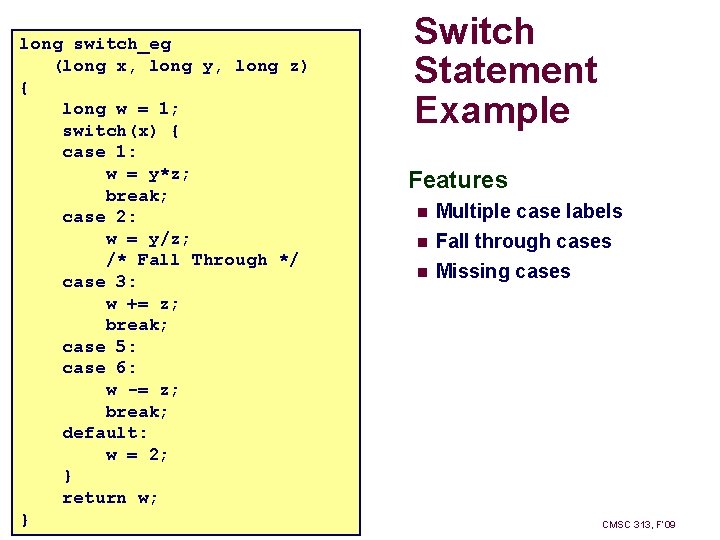
long switch_eg (long x, long y, long z) { long w = 1; switch(x) { case 1: w = y*z; break; case 2: w = y/z; /* Fall Through */ case 3: w += z; break; case 5: case 6: w -= z; break; default: w = 2; } return w; }– 3 – Switch Statement Example Features n Multiple case labels n Fall through cases Missing cases n CMSC 313, F’ 09
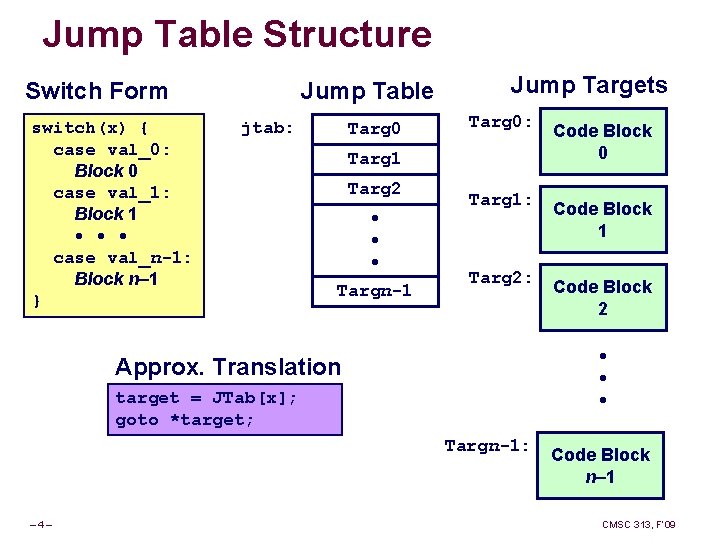
Jump Table Structure Switch Form switch(x) { case val_0: Block 0 case val_1: Block 1 • • • case val_n-1: Block n– 1 } Jump Table jtab: Targ 0 Jump Targets Targ 0: Code Block 0 Targ 1: Code Block 1 Targ 2: Code Block 2 Targ 1 Targ 2 • • • Targn-1 • • • Approx. Translation target = JTab[x]; goto *target; Targn-1: – 4– Code Block n– 1 CMSC 313, F’ 09
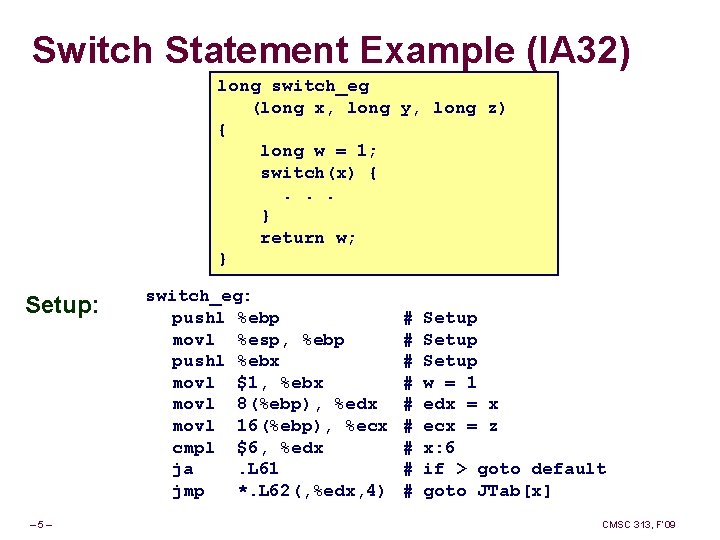
Switch Statement Example (IA 32) long switch_eg (long x, long y, long z) { long w = 1; switch(x) {. . . } return w; } Setup: – 5– switch_eg: pushl %ebp movl %esp, %ebp pushl %ebx movl $1, %ebx movl 8(%ebp), %edx movl 16(%ebp), %ecx cmpl $6, %edx ja. L 61 jmp *. L 62(, %edx, 4) # # # # # Setup w = 1 edx = x ecx = z x: 6 if > goto default goto JTab[x] CMSC 313, F’ 09
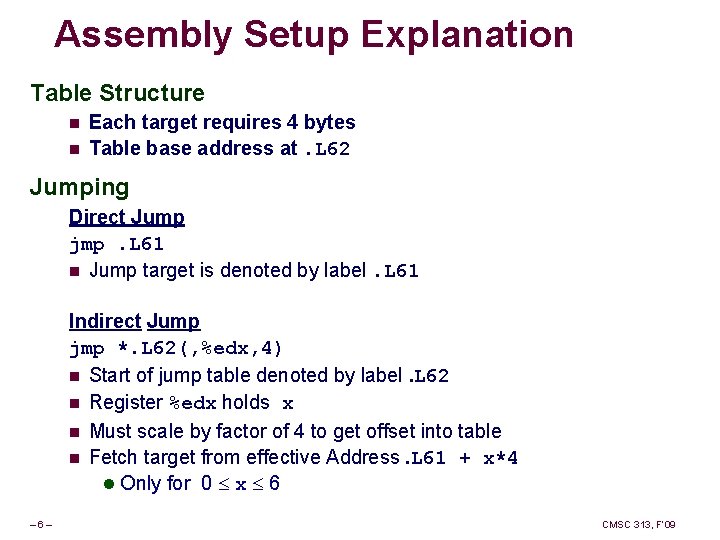
Assembly Setup Explanation Table Structure n n Each target requires 4 bytes Table base address at. L 62 Jumping Direct Jump jmp. L 61 n Jump target is denoted by label. L 61 Indirect Jump jmp *. L 62(, %edx, 4) n Start of jump table denoted by label. L 62 n Register %edx holds x n Must scale by factor of 4 to get offset into table n Fetch target from effective Address. L 61 + x*4 l Only for 0 x 6 – 6– CMSC 313, F’ 09
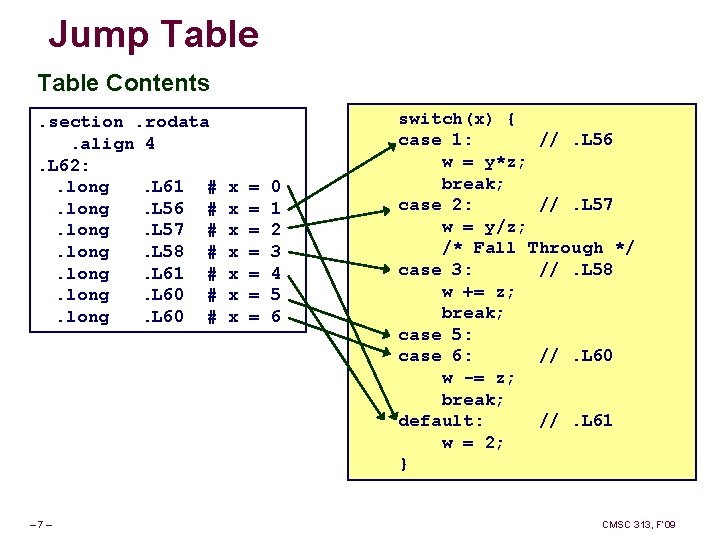
Jump Table Contents. section. rodata. align 4. L 62: . long. L 61 #. long. L 56 #. long. L 57 #. long. L 58 #. long. L 61 #. long. L 60 # – 7– x x x x = = = = 0 1 2 3 4 5 6 switch(x) { case 1: //. L 56 w = y*z; break; case 2: //. L 57 w = y/z; /* Fall Through */ case 3: //. L 58 w += z; break; case 5: case 6: //. L 60 w -= z; break; default: //. L 61 w = 2; } CMSC 313, F’ 09
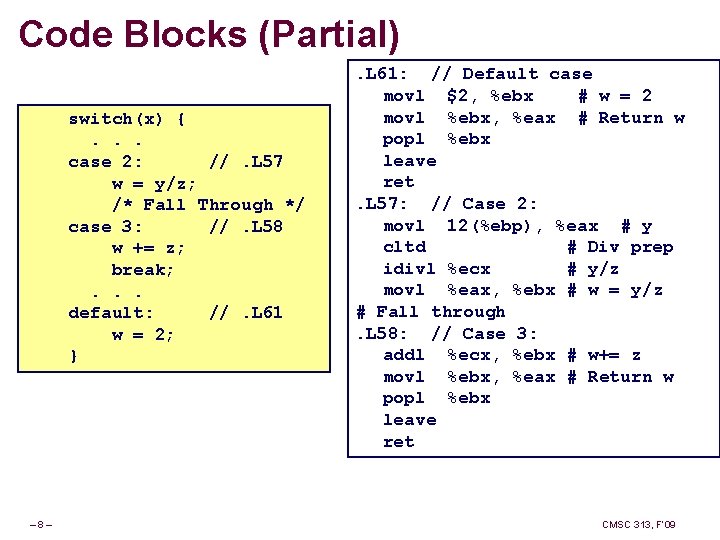
Code Blocks (Partial) switch(x) {. . . case 2: //. L 57 w = y/z; /* Fall Through */ case 3: //. L 58 w += z; break; . . . default: //. L 61 w = 2; } – 8– . L 61: // Default case movl $2, %ebx # w = 2 movl %ebx, %eax # Return w popl %ebx leave ret. L 57: // Case 2: movl 12(%ebp), %eax # y cltd # Div prep idivl %ecx # y/z movl %eax, %ebx # w = y/z # Fall through. L 58: // Case 3: addl %ecx, %ebx # w+= z movl %ebx, %eax # Return w popl %ebx leave ret CMSC 313, F’ 09
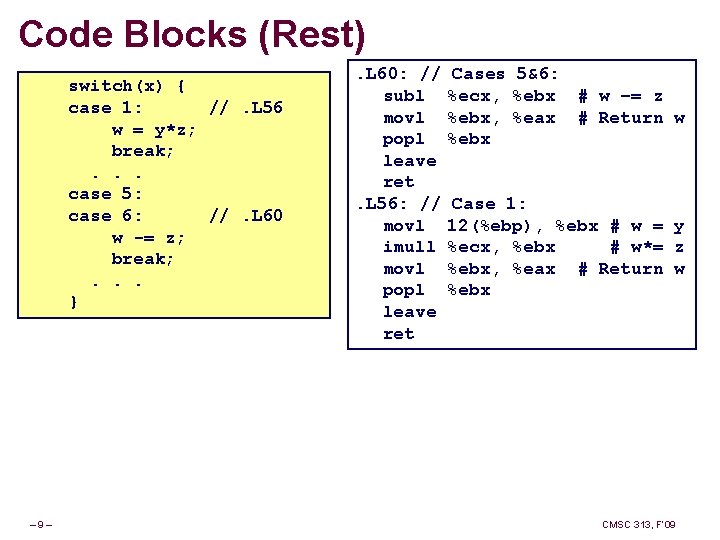
Code Blocks (Rest) switch(x) { case 1: //. L 56 w = y*z; break; . . . case 5: case 6: //. L 60 w -= z; break; . . . } – 9– . L 60: // subl movl popl leave ret. L 56: // movl imull movl popl leave ret Cases 5&6: %ecx, %ebx # w –= z %ebx, %eax # Return w %ebx Case 1: 12(%ebp), %ebx # w = y %ecx, %ebx # w*= z %ebx, %eax # Return w %ebx CMSC 313, F’ 09
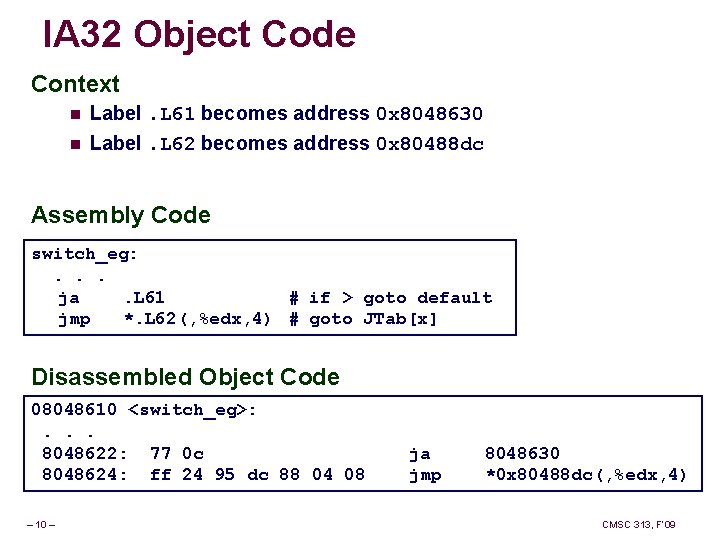
IA 32 Object Code Context n n Label. L 61 becomes address 0 x 8048630 Label. L 62 becomes address 0 x 80488 dc Assembly Code switch_eg: . . . ja. L 61 # if > goto default jmp *. L 62(, %edx, 4) # goto JTab[x] Disassembled Object Code 08048610 <switch_eg>: . . . 8048622: 77 0 c 8048624: ff 24 95 dc 88 04 08 – 10 – ja jmp 8048630 *0 x 80488 dc(, %edx, 4) CMSC 313, F’ 09
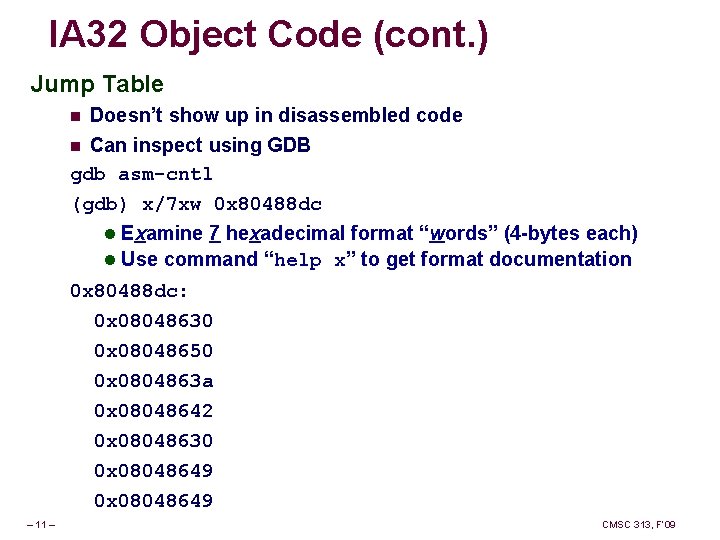
IA 32 Object Code (cont. ) Jump Table n Doesn’t show up in disassembled code Can inspect using GDB gdb asm-cntl (gdb) x/7 xw 0 x 80488 dc l Examine 7 hexadecimal format “words” (4 -bytes each) l Use command “help x” to get format documentation n 0 x 80488 dc: 0 x 08048630 0 x 08048650 0 x 0804863 a 0 x 08048642 0 x 08048630 0 x 08048649 – 11 – CMSC 313, F’ 09
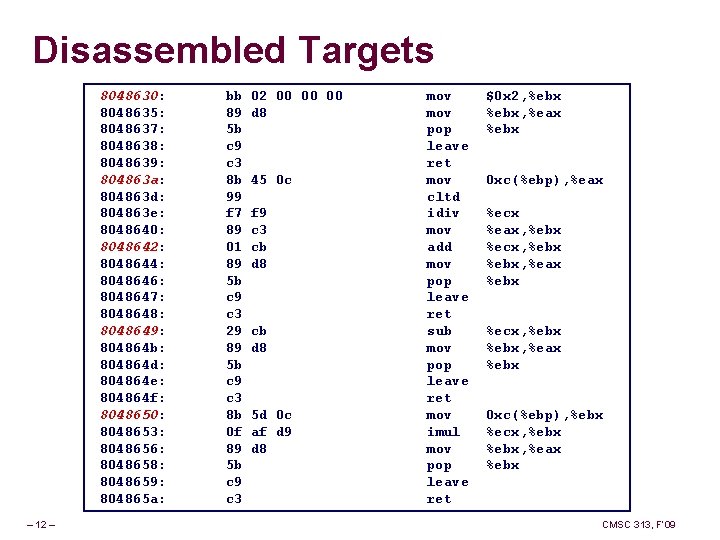
Disassembled Targets 8048630: 8048635: 8048637: 8048638: 8048639: 804863 a: 804863 d: 804863 e: 8048640: 8048642: 8048644: 8048646: 8048647: 8048648: 8048649: 804864 b: 804864 d: 804864 e: 804864 f: 8048650: 8048653: 8048656: 8048658: 8048659: 804865 a: – 12 – bb 89 5 b c 9 c 3 8 b 99 f 7 89 01 89 5 b c 9 c 3 29 89 5 b c 9 c 3 8 b 0 f 89 5 b c 9 c 3 02 00 00 00 d 8 45 0 c f 9 c 3 cb d 8 5 d 0 c af d 9 d 8 mov pop leave ret mov cltd idiv mov add mov pop leave ret sub mov pop leave ret mov imul mov pop leave ret $0 x 2, %ebx, %eax %ebx 0 xc(%ebp), %eax %ecx %eax, %ebx %ecx, %ebx, %eax %ebx 0 xc(%ebp), %ebx %ecx, %ebx, %eax %ebx CMSC 313, F’ 09
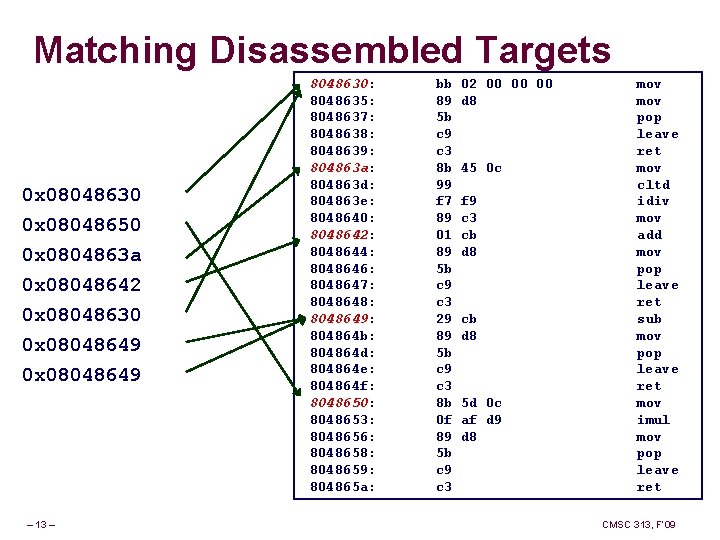
Matching Disassembled Targets 0 x 08048630 0 x 08048650 0 x 0804863 a 0 x 08048642 0 x 08048630 0 x 08048649 – 13 – 8048630: 8048635: 8048637: 8048638: 8048639: 804863 a: 804863 d: 804863 e: 8048640: 8048642: 8048644: 8048646: 8048647: 8048648: 8048649: 804864 b: 804864 d: 804864 e: 804864 f: 8048650: 8048653: 8048656: 8048658: 8048659: 804865 a: bb 89 5 b c 9 c 3 8 b 99 f 7 89 01 89 5 b c 9 c 3 29 89 5 b c 9 c 3 8 b 0 f 89 5 b c 9 c 3 02 00 00 00 d 8 45 0 c f 9 c 3 cb d 8 5 d 0 c af d 9 d 8 mov pop leave ret mov cltd idiv mov add mov pop leave ret sub mov pop leave ret mov imul mov pop leave ret CMSC 313, F’ 09
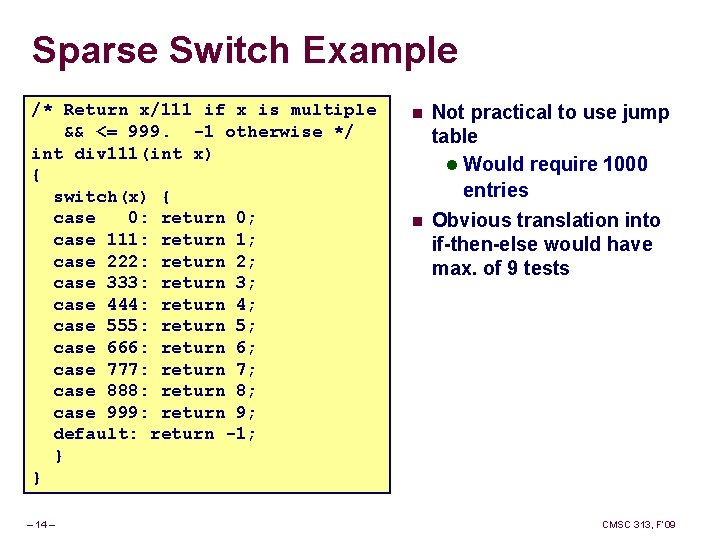
Sparse Switch Example /* Return x/111 if x is multiple && <= 999. -1 otherwise */ int div 111(int x) { switch(x) { case 0: return 0; case 111: return 1; case 222: return 2; case 333: return 3; case 444: return 4; case 555: return 5; case 666: return 6; case 777: return 7; case 888: return 8; case 999: return 9; default: return -1; } } – 14 – n Not practical to use jump table l Would require 1000 entries n Obvious translation into if-then-else would have max. of 9 tests CMSC 313, F’ 09
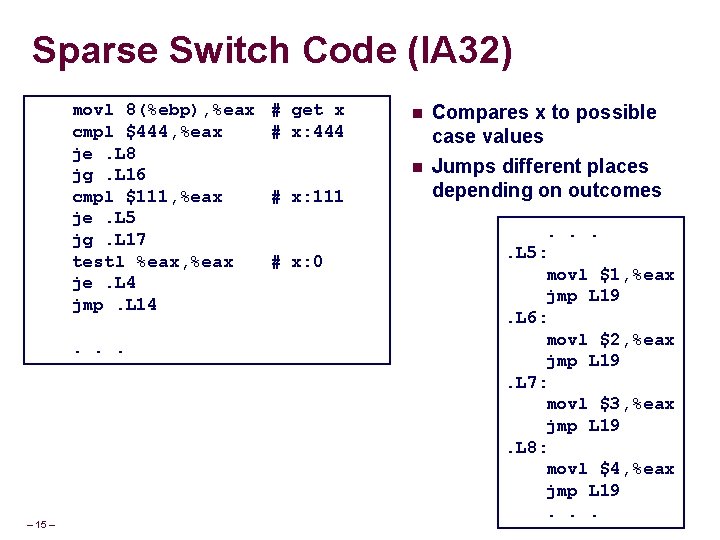
Sparse Switch Code (IA 32) movl 8(%ebp), %eax cmpl $444, %eax je. L 8 jg. L 16 cmpl $111, %eax je. L 5 jg. L 17 testl %eax, %eax je. L 4 jmp. L 14. . . – 15 – # get x # x: 444 # x: 111 # x: 0 n Compares x to possible case values n Jumps different places depending on outcomes. . L 5: movl $1, %eax jmp L 19. L 6: movl $2, %eax jmp L 19. L 7: movl $3, %eax jmp L 19. L 8: movl $4, %eax jmp L 19. . . CMSC 313, F’ 09
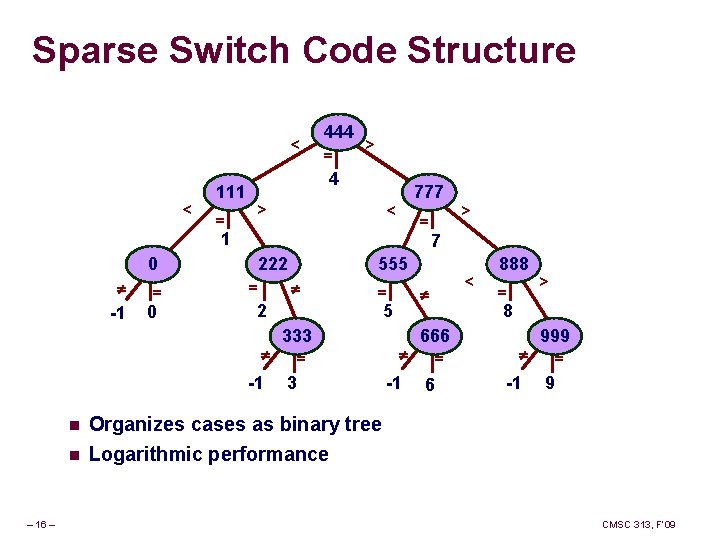
Sparse Switch Code Structure < < 111 = 444 = > 4 > 777 < = 1 7 0 -1 = 0 222 2 -1 n – 16 – 555 = n > 5 333 = 3 < = -1 888 = > 8 666 = 6 -1 999 = 9 Organizes cases as binary tree Logarithmic performance CMSC 313, F’ 09
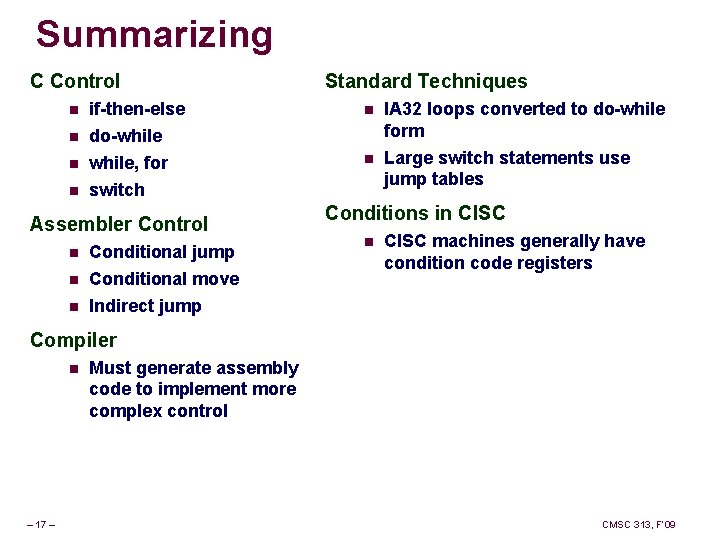
Summarizing C Control Standard Techniques n n if-then-else do-while IA 32 loops converted to do-while form n while, for n n switch Large switch statements use jump tables n Assembler Control n n n Conditional jump Conditional move Indirect jump Conditions in CISC machines generally have condition code registers Compiler n – 17 – Must generate assembly code to implement more complex control CMSC 313, F’ 09
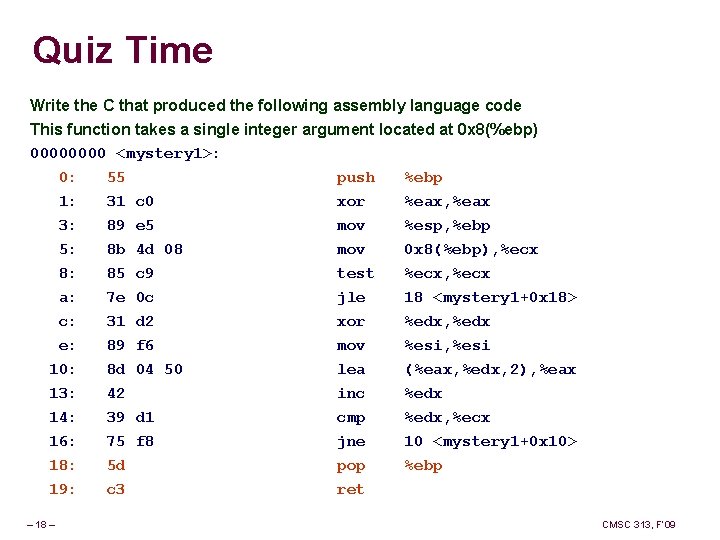
Quiz Time Write the C that produced the following assembly language code This function takes a single integer argument located at 0 x 8(%ebp) 0000 <mystery 1>: 0: 55 push %ebp 1: 31 c 0 xor %eax, %eax 3: 89 e 5 mov %esp, %ebp 5: 8 b 4 d 08 mov 0 x 8(%ebp), %ecx 8: 85 c 9 test %ecx, %ecx a: 7 e 0 c jle 18 <mystery 1+0 x 18> c: 31 d 2 xor %edx, %edx e: 89 f 6 mov %esi, %esi 8 d 04 50 42 39 d 1 75 f 8 5 d c 3 lea inc cmp jne pop ret (%eax, %edx, 2), %eax %edx, %ecx 10 <mystery 1+0 x 10> %ebp 10: 13: 14: 16: 18: 19: – 18 – CMSC 313, F’ 09
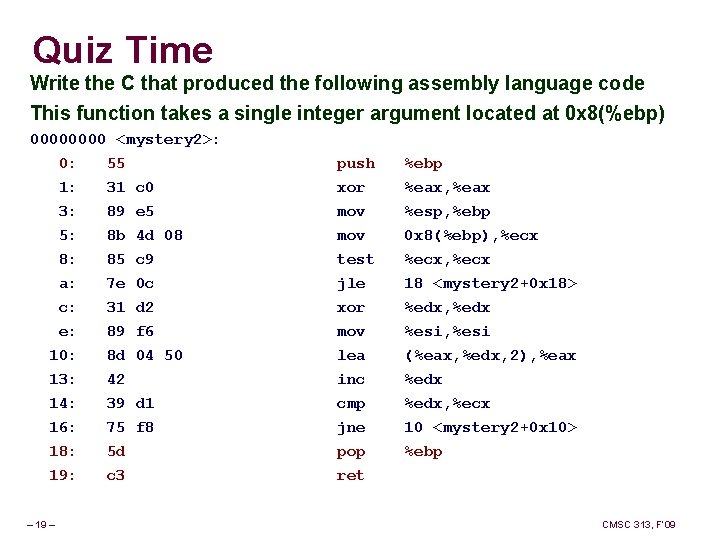
Quiz Time Write the C that produced the following assembly language code This function takes a single integer argument located at 0 x 8(%ebp) 0000 <mystery 2>: 0: 55 1: 31 c 0 %ebp %eax, %eax 3: 89 e 5 mov %esp, %ebp 5: 8 b 4 d 08 mov 0 x 8(%ebp), %ecx 8: 85 c 9 test %ecx, %ecx a: 7 e 0 c jle 18 <mystery 2+0 x 18> 31 89 8 d 42 39 75 5 d c 3 xor mov lea inc cmp jne pop ret %edx, %edx %esi, %esi (%eax, %edx, 2), %eax %edx, %ecx 10 <mystery 2+0 x 10> %ebp c: e: 10: 13: 14: 16: 18: 19: – 19 – push xor d 2 f 6 04 50 d 1 f 8 CMSC 313, F’ 09
Data flow vs control flow
Control flow and data flow computers
Control flow vs transaction flow
Stock control e flow control
Flow and error control
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Runtime programming
Linear vs integer programming
Definisi integer
Flow programming
Flow programming language
Air entrainment nebulizer
Simple mask flow rate
T piece oxygen delivery
Define streamline and turbulent flow
Internal flow vs external flow
Ecological succession
Oikos meaning
Transform flow and transaction flow in software engineering