MachineLevel Programming 2 Control Flow Topics n Condition
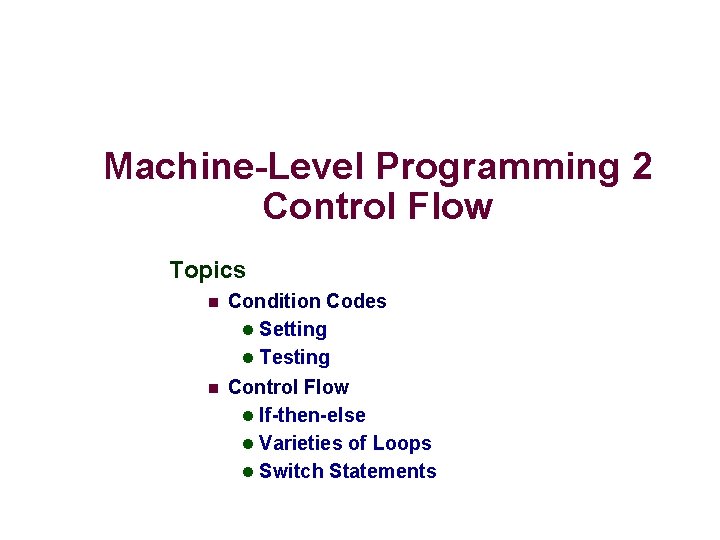
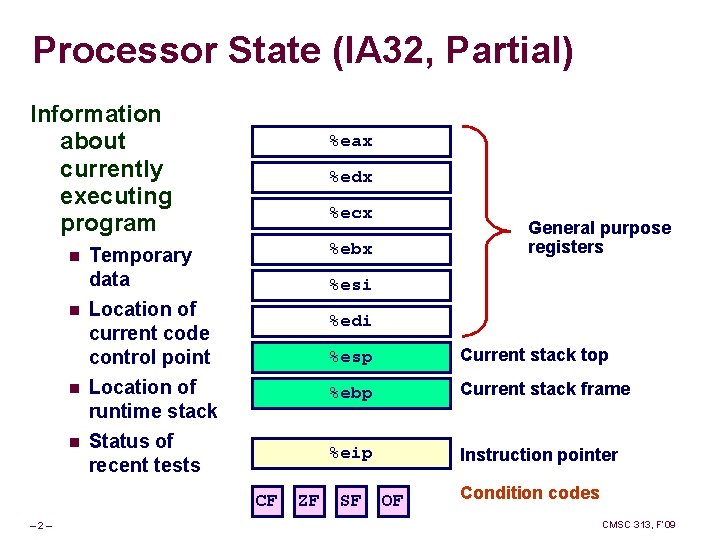
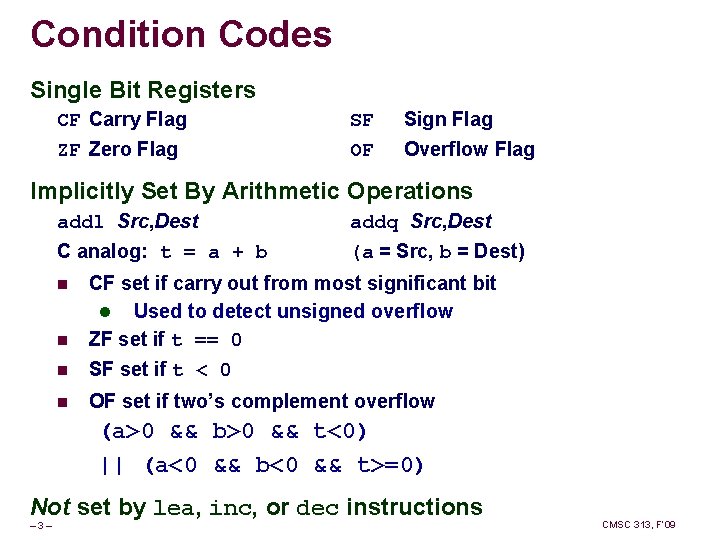
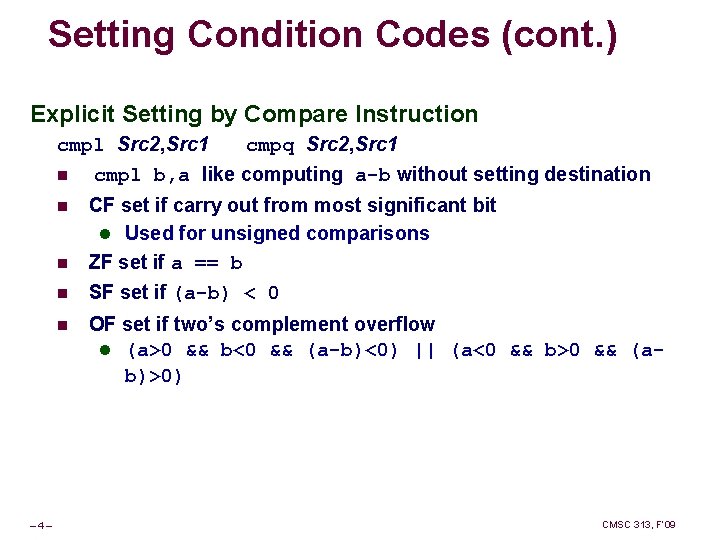
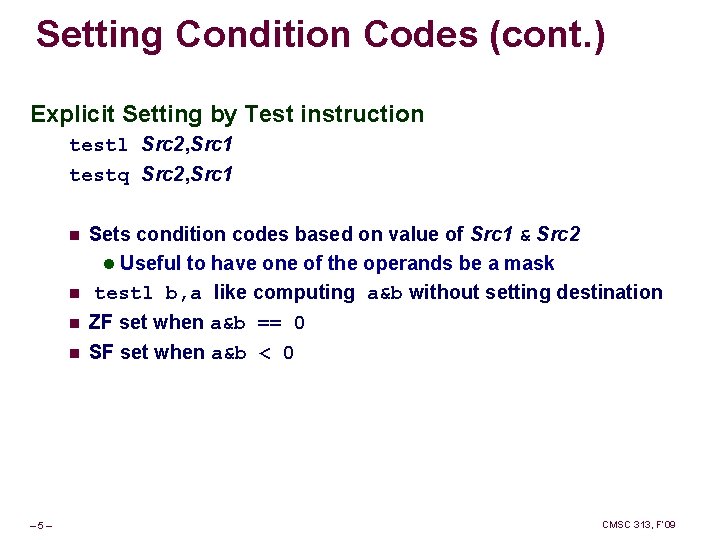
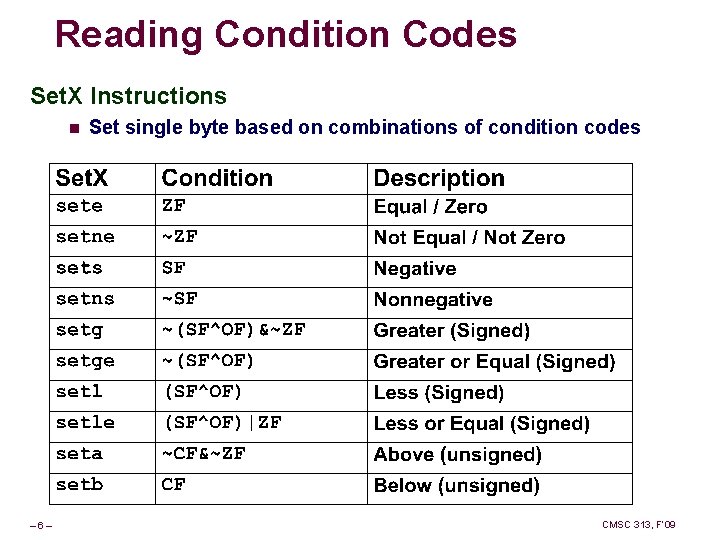
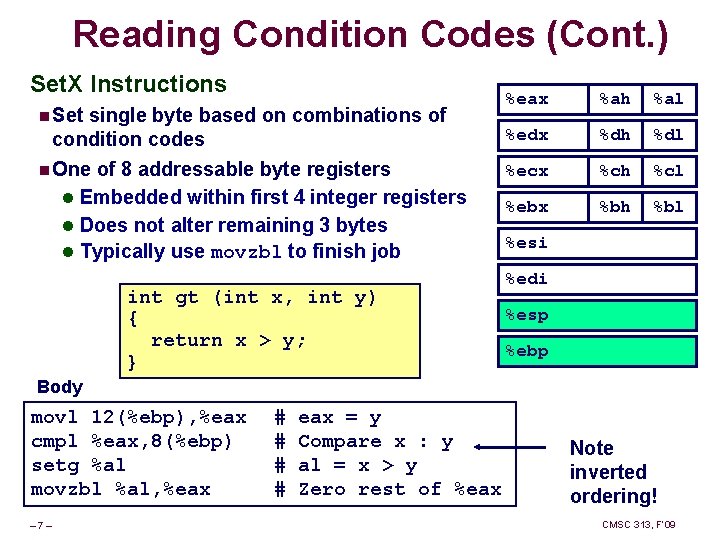
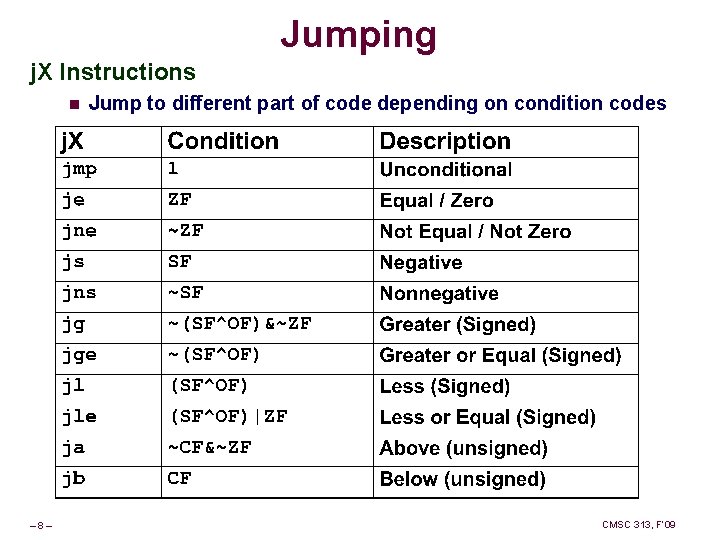
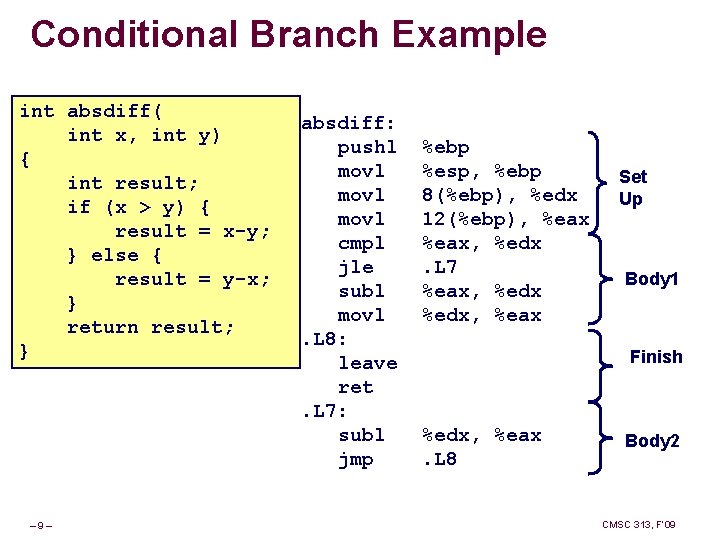
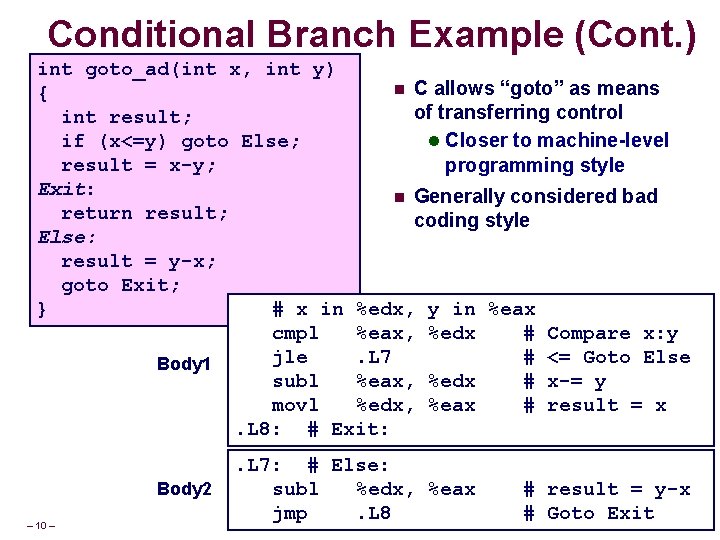
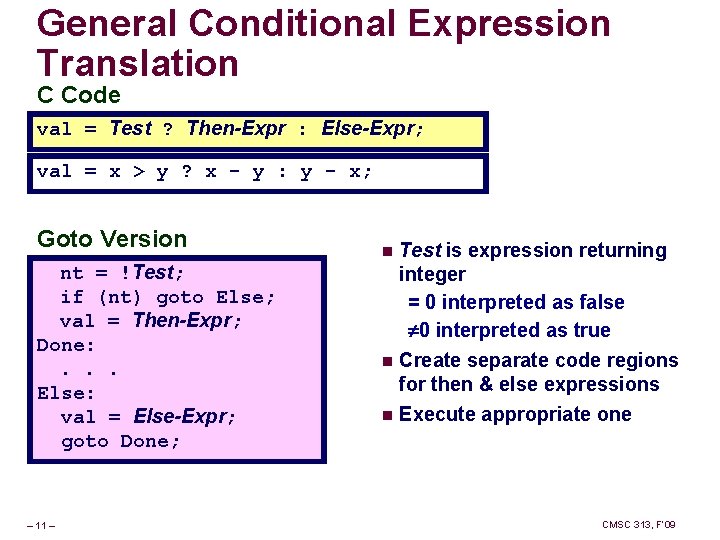
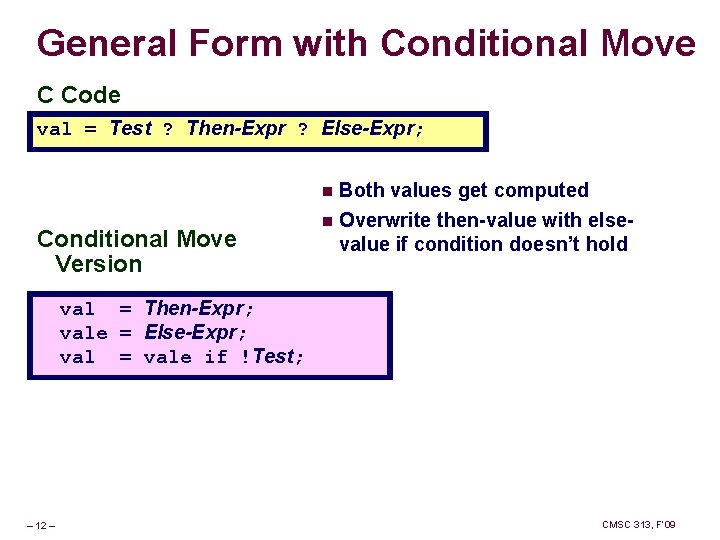
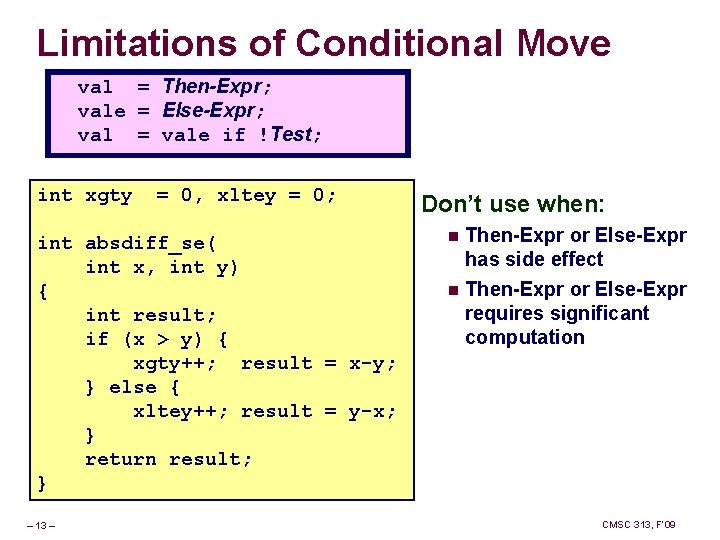
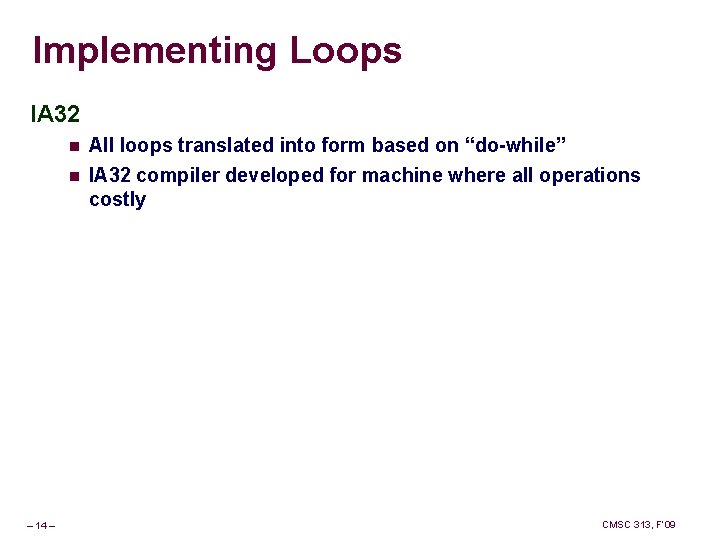
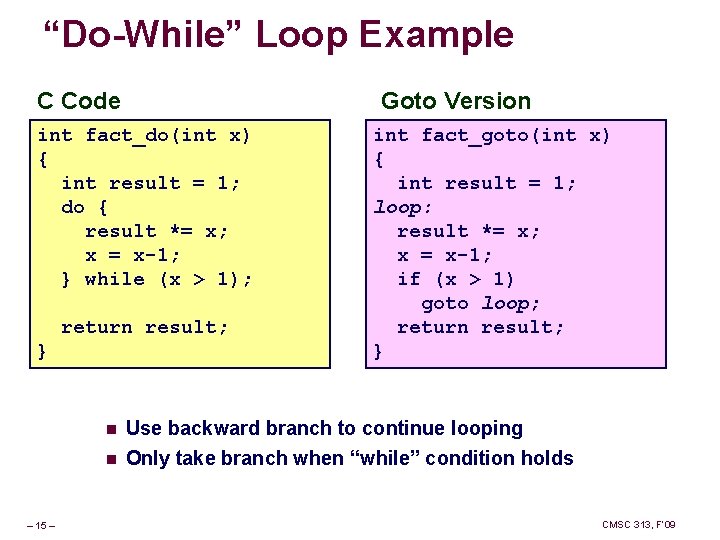
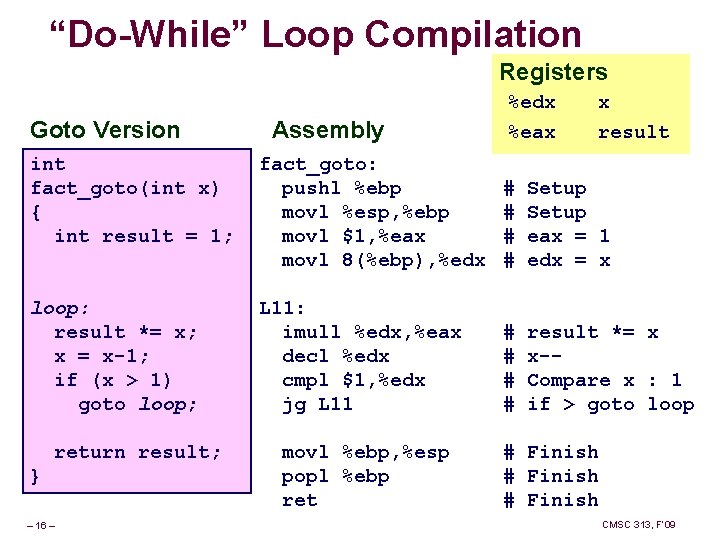
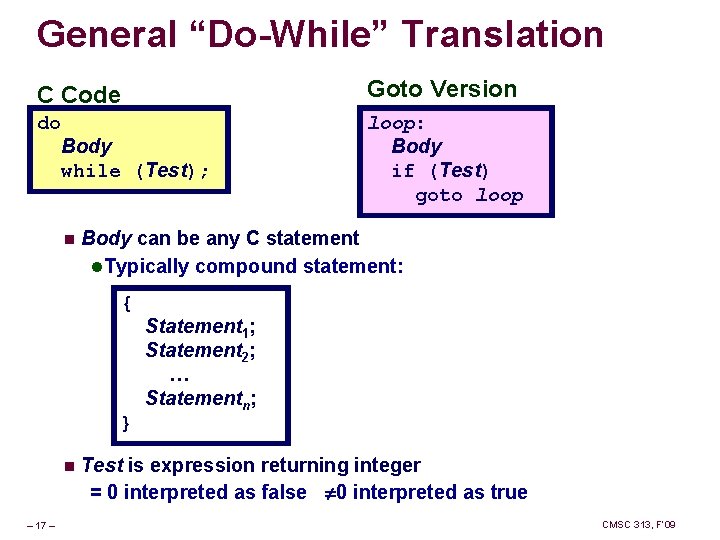
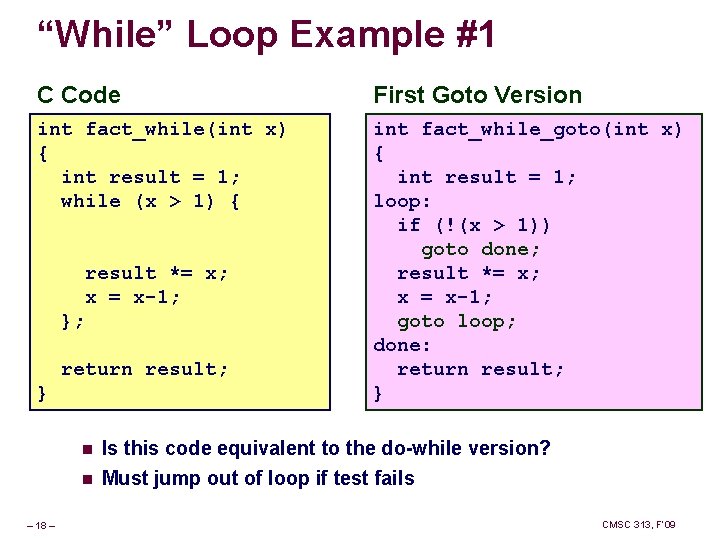
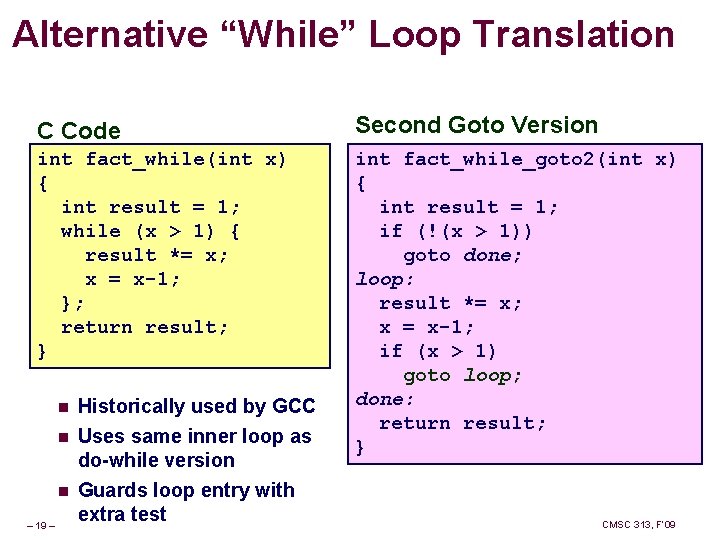
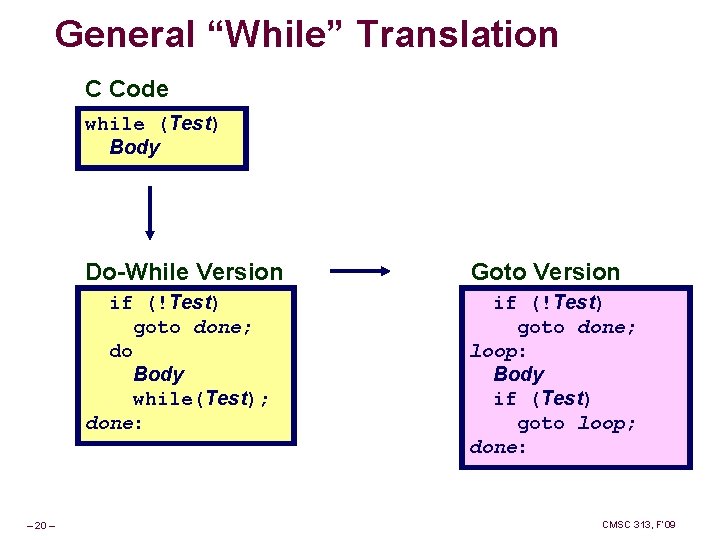
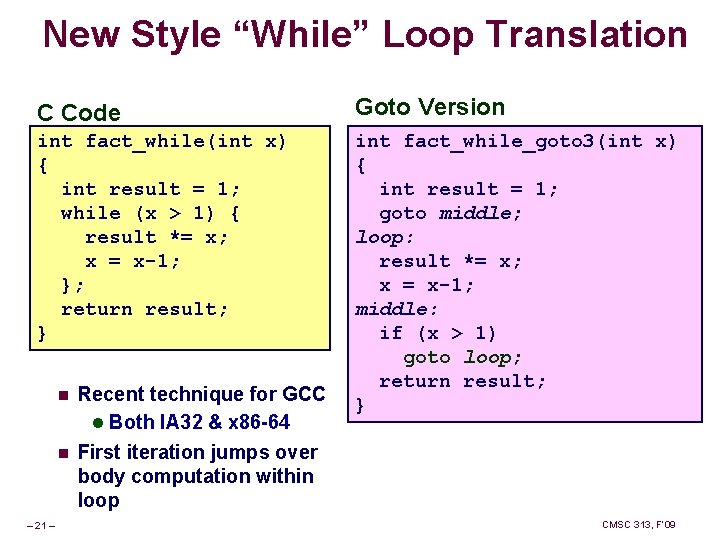
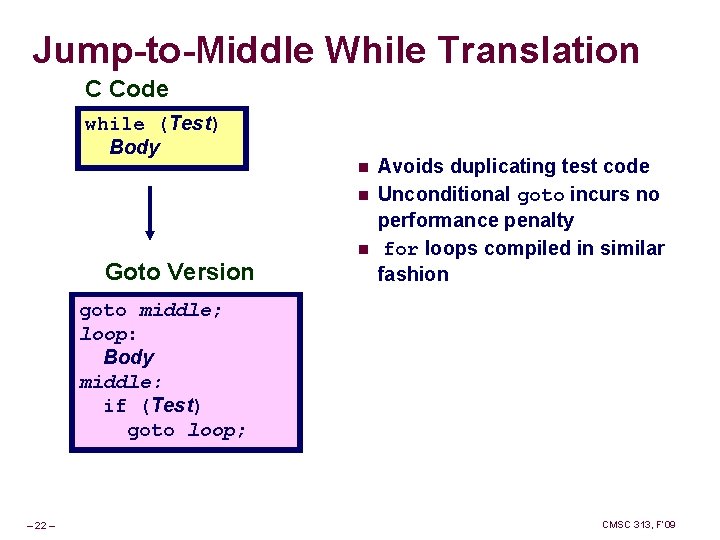
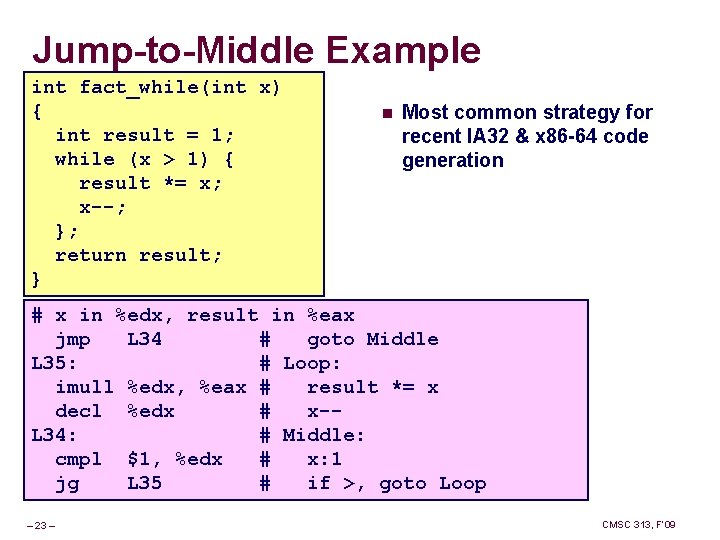
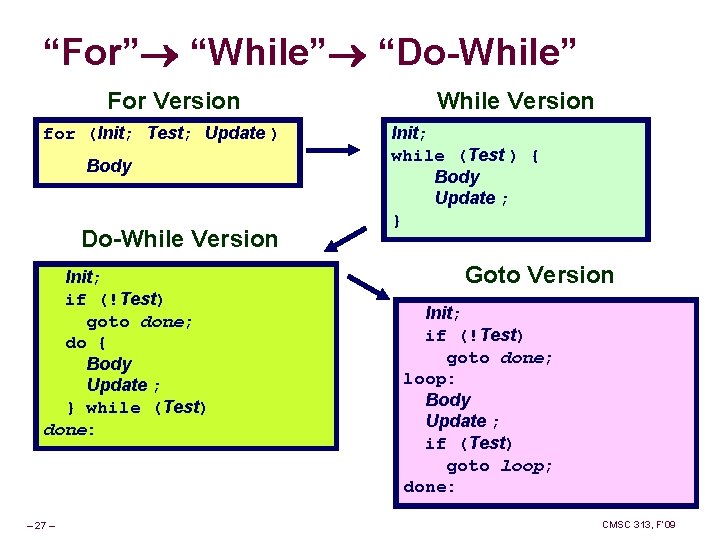
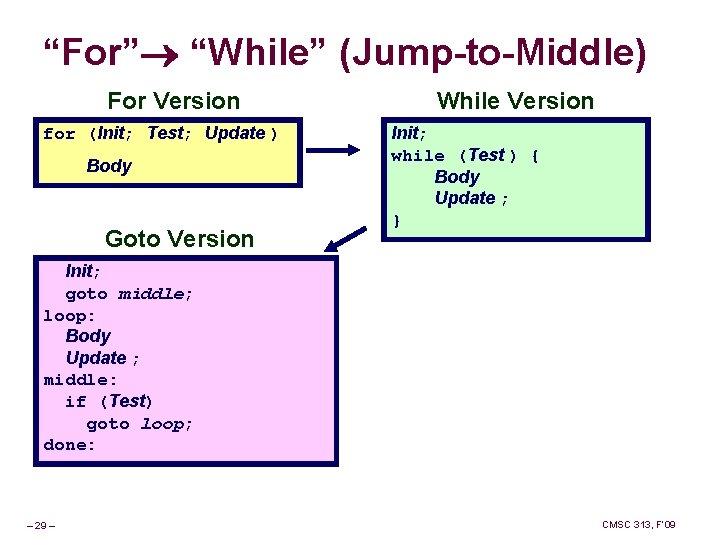
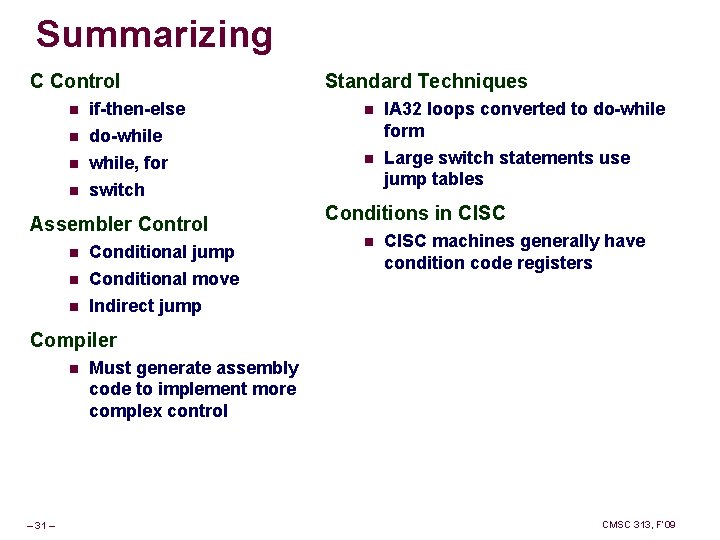
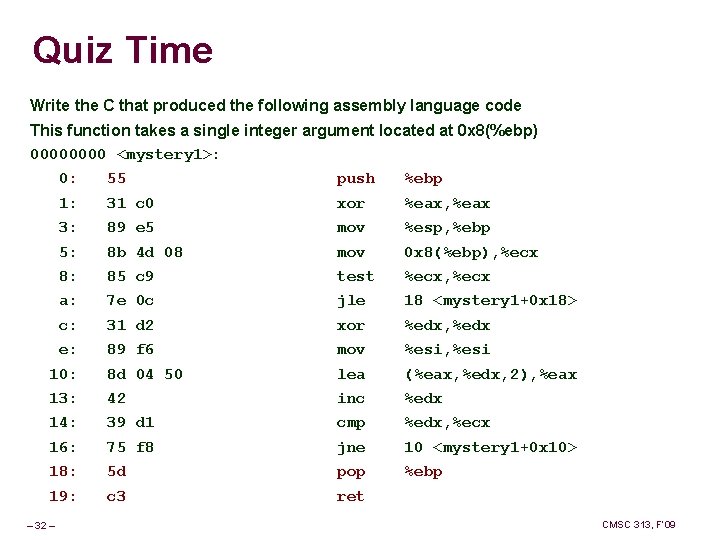
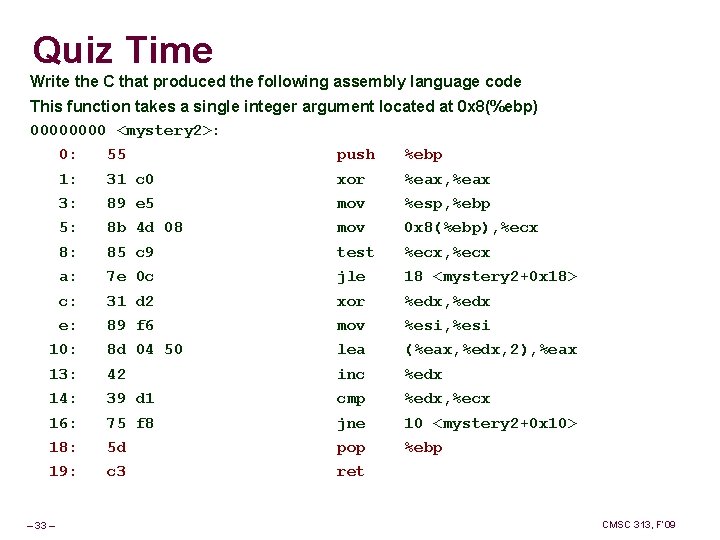
- Slides: 28
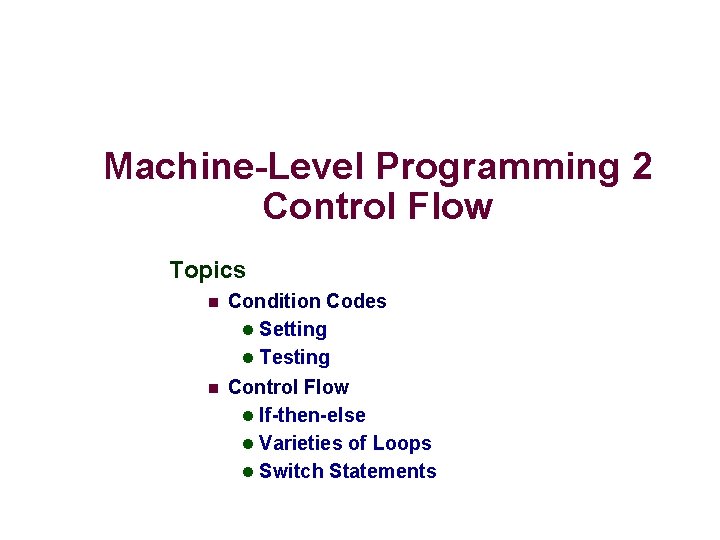
Machine-Level Programming 2 Control Flow Topics n Condition Codes l Setting l Testing n Control Flow l If-then-else l Varieties of Loops l Switch Statements
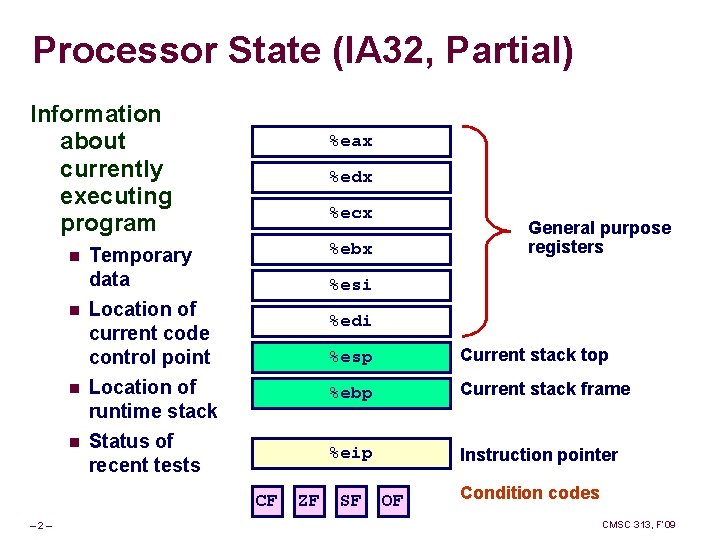
Processor State (IA 32, Partial) Information about currently executing program n n %eax %edx %ecx %ebx Temporary data Location of current code control point Location of runtime stack Status of recent tests %esi %edi CF – 2– General purpose registers ZF %esp Current stack top %ebp Current stack frame %eip Instruction pointer SF OF Condition codes CMSC 313, F’ 09
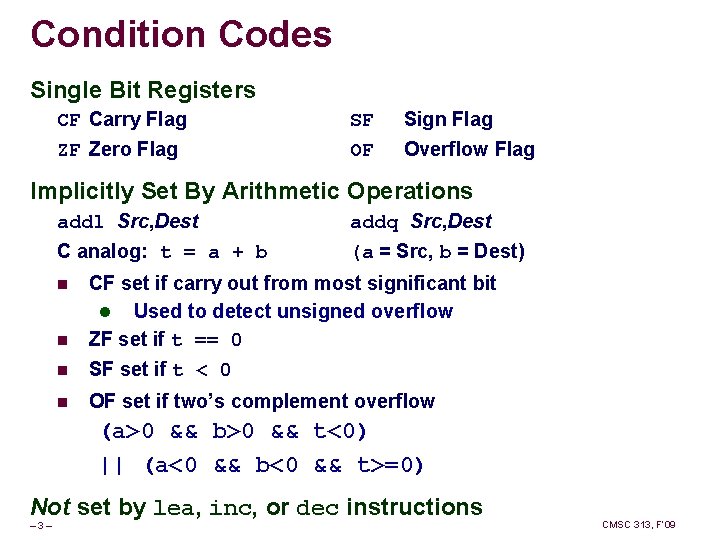
Condition Codes Single Bit Registers CF Carry Flag ZF Zero Flag SF OF Sign Flag Overflow Flag Implicitly Set By Arithmetic Operations addl Src, Dest C analog: t = a + b addq Src, Dest (a = Src, b = Dest) n CF set if carry out from most significant bit l Used to detect unsigned overflow ZF set if t == 0 SF set if t < 0 n OF set if two’s complement overflow n n (a>0 && b>0 && t<0) || (a<0 && b<0 && t>=0) Not set by lea, inc, or dec instructions – 3– CMSC 313, F’ 09
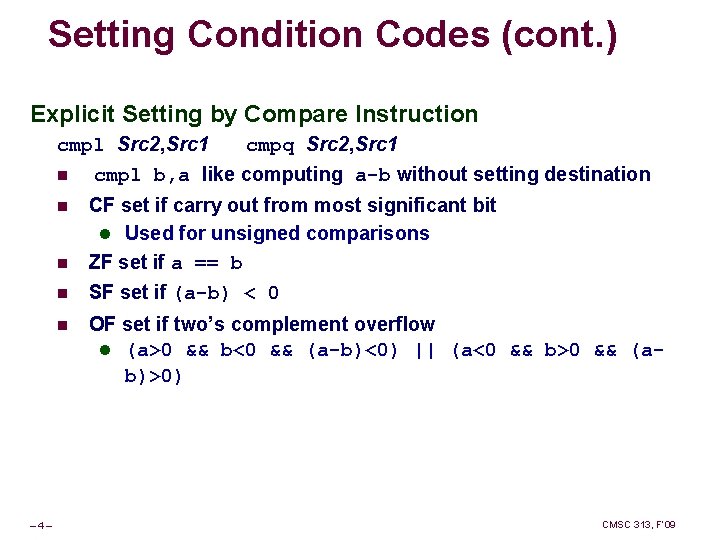
Setting Condition Codes (cont. ) Explicit Setting by Compare Instruction cmpl Src 2, Src 1 cmpq Src 2, Src 1 n cmpl b, a like computing a-b without setting destination n n – 4– CF set if carry out from most significant bit l Used for unsigned comparisons ZF set if a == b SF set if (a-b) < 0 OF set if two’s complement overflow l (a>0 && b<0 && (a-b)<0) || (a<0 && b>0 && (ab)>0) CMSC 313, F’ 09
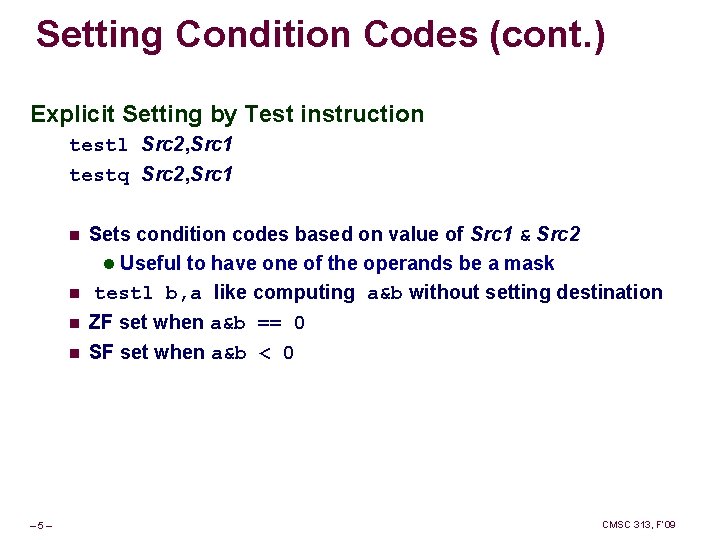
Setting Condition Codes (cont. ) Explicit Setting by Test instruction testl Src 2, Src 1 testq Src 2, Src 1 n Sets condition codes based on value of Src 1 & Src 2 l Useful to have one of the operands be a mask testl b, a like computing a&b without setting destination ZF set when a&b == 0 n SF set when a&b < 0 n n – 5– CMSC 313, F’ 09
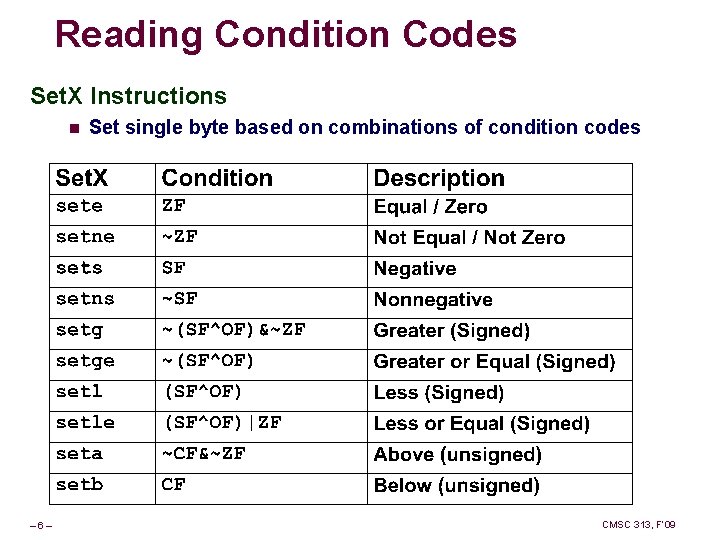
Reading Condition Codes Set. X Instructions n – 6– Set single byte based on combinations of condition codes CMSC 313, F’ 09
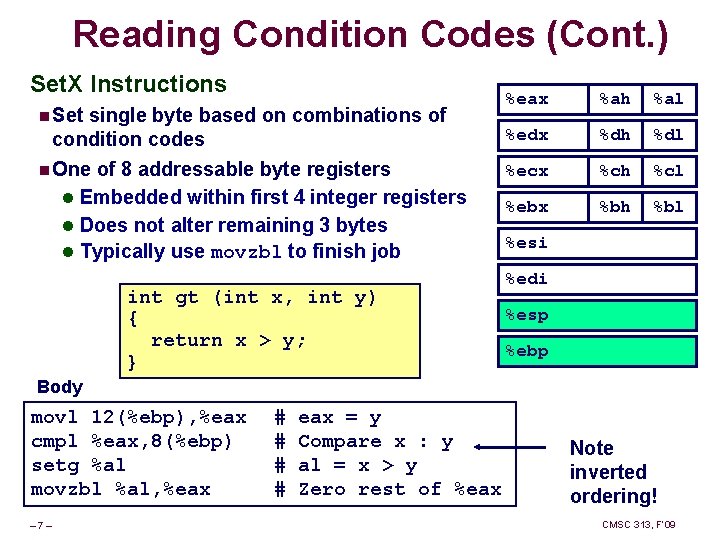
Reading Condition Codes (Cont. ) Set. X Instructions n Set single byte based on combinations of condition codes n One of 8 addressable byte registers l Embedded within first 4 integer registers l Does not alter remaining 3 bytes l Typically use movzbl to finish job int gt (int x, int y) { return x > y; } %eax %ah %al %edx %dh %dl %ecx %ch %cl %ebx %bh %bl %esi %edi %esp %ebp Body movl 12(%ebp), %eax cmpl %eax, 8(%ebp) setg %al movzbl %al, %eax – 7– # # eax = y Compare x : y al = x > y Zero rest of %eax Note inverted ordering! CMSC 313, F’ 09
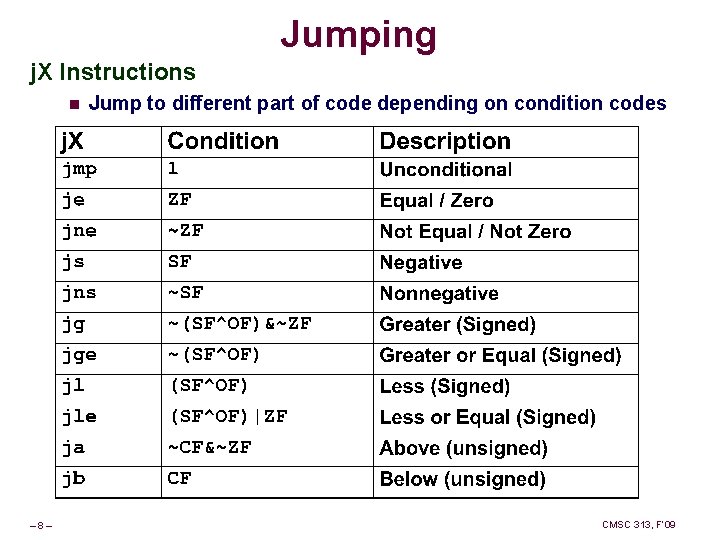
Jumping j. X Instructions n – 8– Jump to different part of code depending on condition codes CMSC 313, F’ 09
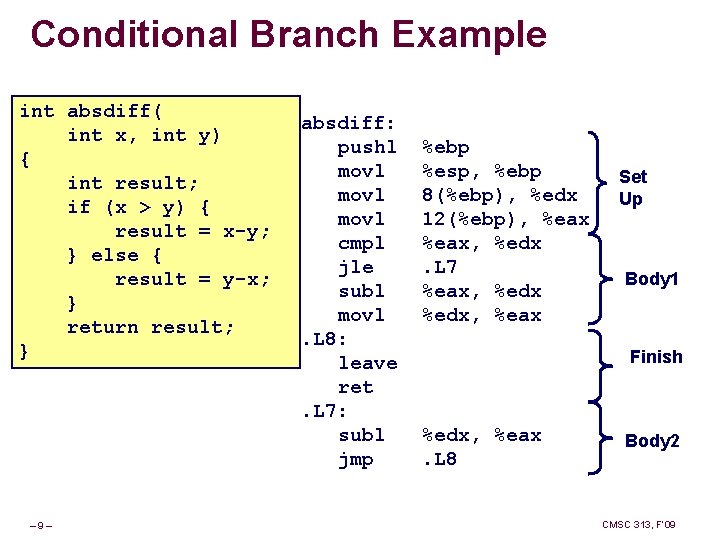
Conditional Branch Example int absdiff( int x, int y) { int result; if (x > y) { result = x-y; } else { result = y-x; } return result; } – 9– absdiff: pushl movl cmpl jle subl movl. L 8: leave ret. L 7: subl jmp %ebp %esp, %ebp 8(%ebp), %edx 12(%ebp), %eax, %edx. L 7 %eax, %edx, %eax Set Up Body 1 Finish %edx, %eax. L 8 Body 2 CMSC 313, F’ 09
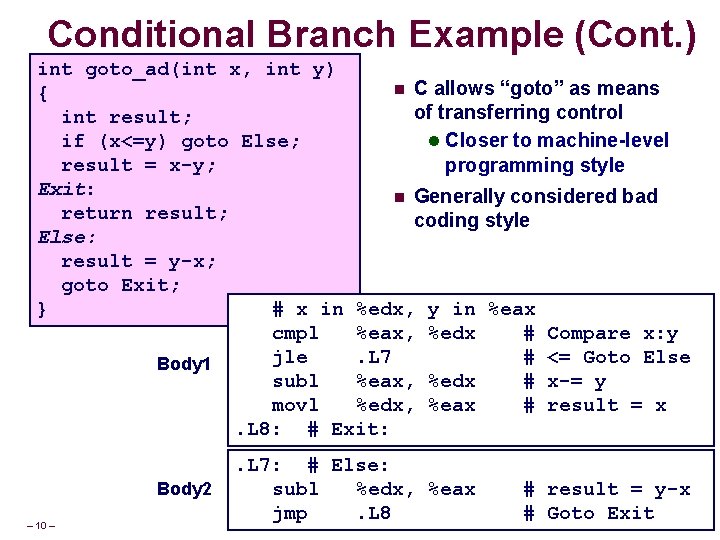
Conditional Branch Example (Cont. ) int goto_ad(int x, int y) n C allows “goto” as means { of transferring control int result; l Closer to machine-level if (x<=y) goto Else; result = x-y; programming style Exit: n Generally considered bad return result; coding style Else: result = y-x; goto Exit; # x in %edx, y in %eax } cmpl %eax, %edx # Compare x: y jle. L 7 # <= Goto Else Body 1 subl %eax, %edx # x-= y movl %edx, %eax # result = x. L 8: # Exit: Body 2 – 10 – . L 7: # Else: subl %edx, %eax jmp. L 8 # result = y-x # Goto CMSC Exit 313, F’ 09
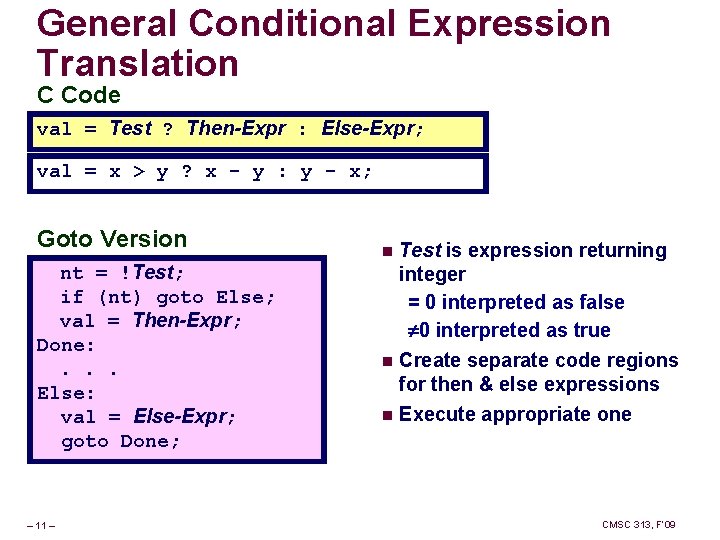
General Conditional Expression Translation C Code val = Test ? Then-Expr : Else-Expr; val = x > y ? x - y : y - x; Goto Version nt = !Test; if (nt) goto Else; val = Then-Expr; Done: . . . Else: val = Else-Expr; goto Done; – 11 – n Test is expression returning integer = 0 interpreted as false 0 interpreted as true Create separate code regions for then & else expressions n Execute appropriate one n CMSC 313, F’ 09
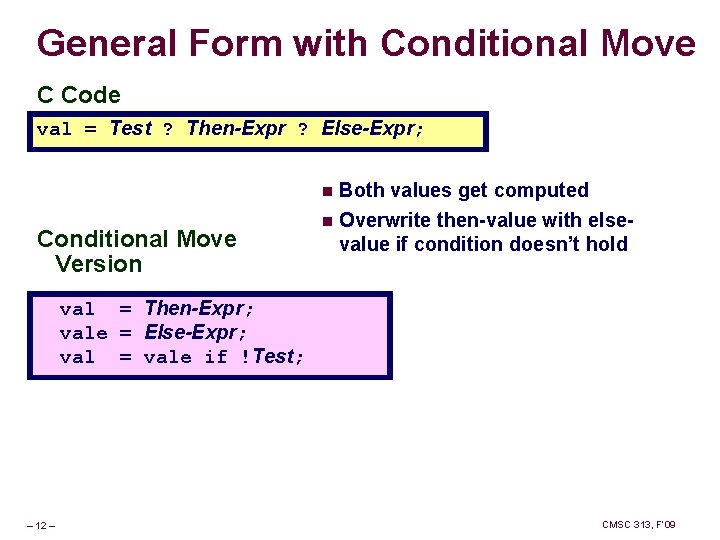
General Form with Conditional Move C Code val = Test ? Then-Expr ? Else-Expr; Both values get computed n Overwrite then-value with elsevalue if condition doesn’t hold n Conditional Move Version val = Then-Expr; vale = Else-Expr; val = vale if !Test; – 12 – CMSC 313, F’ 09
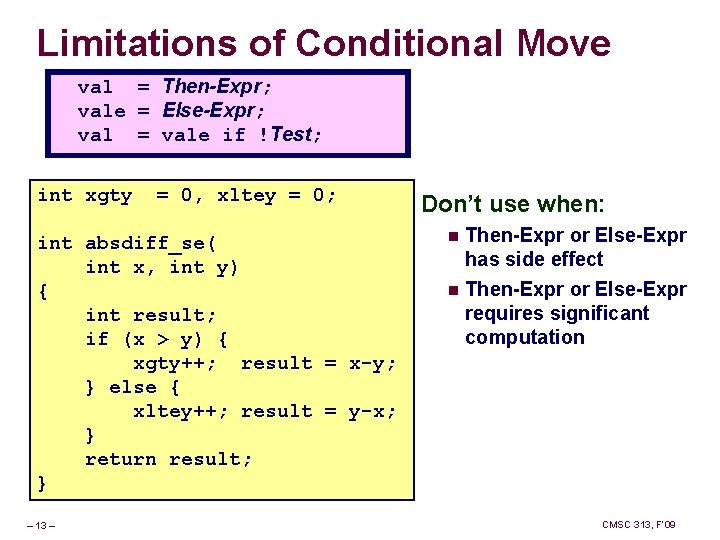
Limitations of Conditional Move val = Then-Expr; vale = Else-Expr; val = vale if !Test; int xgty = 0, xltey = 0; int absdiff_se( int x, int y) { int result; if (x > y) { xgty++; result = x-y; } else { xltey++; result = y-x; } return result; } – 13 – Don’t use when: Then-Expr or Else-Expr has side effect n Then-Expr or Else-Expr requires significant computation n CMSC 313, F’ 09
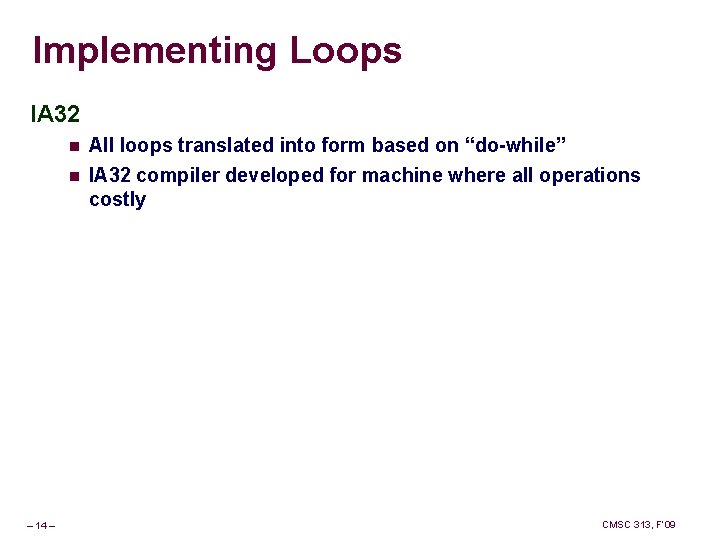
Implementing Loops IA 32 – 14 – n All loops translated into form based on “do-while” n IA 32 compiler developed for machine where all operations costly CMSC 313, F’ 09
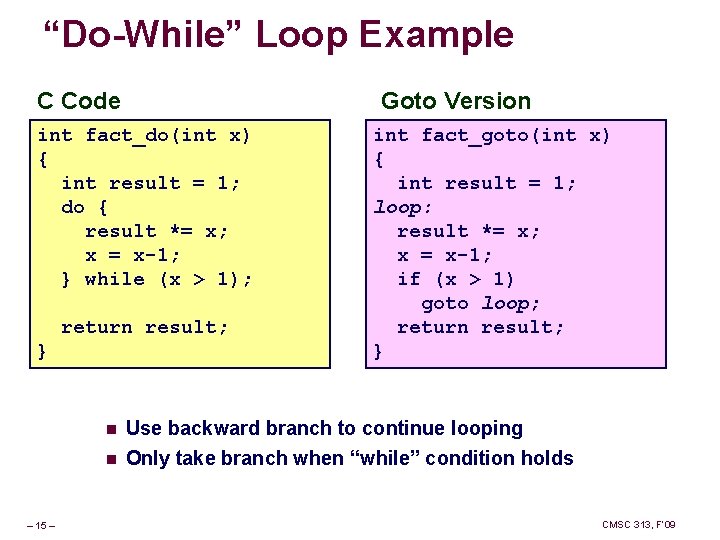
“Do-While” Loop Example C Code Goto Version int fact_do(int x) { int result = 1; do { result *= x; x = x-1; } while (x > 1); return result; } n n – 15 – int fact_goto(int x) { int result = 1; loop: result *= x; x = x-1; if (x > 1) goto loop; return result; } Use backward branch to continue looping Only take branch when “while” condition holds CMSC 313, F’ 09
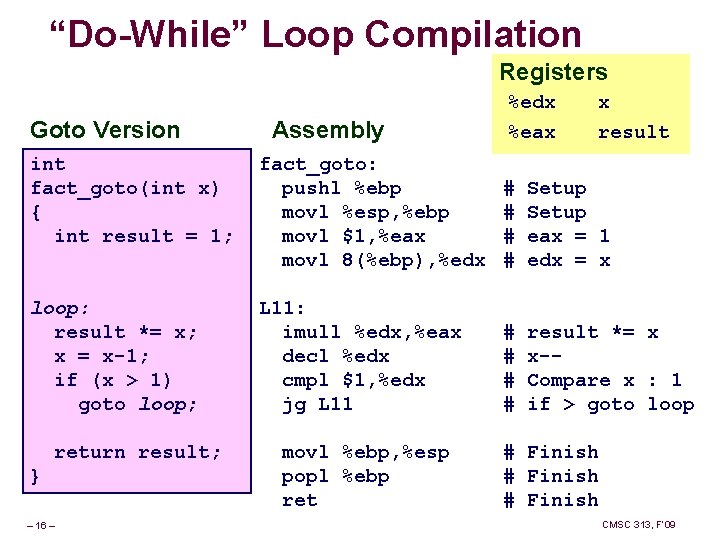
“Do-While” Loop Compilation Registers Goto Version Assembly %edx %eax x result int fact_goto(int x) { int result = 1; fact_goto: pushl %ebp movl %esp, %ebp movl $1, %eax movl 8(%ebp), %edx # # Setup eax = 1 edx = x loop: result *= x; x = x-1; if (x > 1) goto loop; L 11: imull %edx, %eax decl %edx cmpl $1, %edx jg L 11 # # result *= x x-Compare x : 1 if > goto loop return result; } – 16 – movl %ebp, %esp popl %ebp ret # Finish CMSC 313, F’ 09
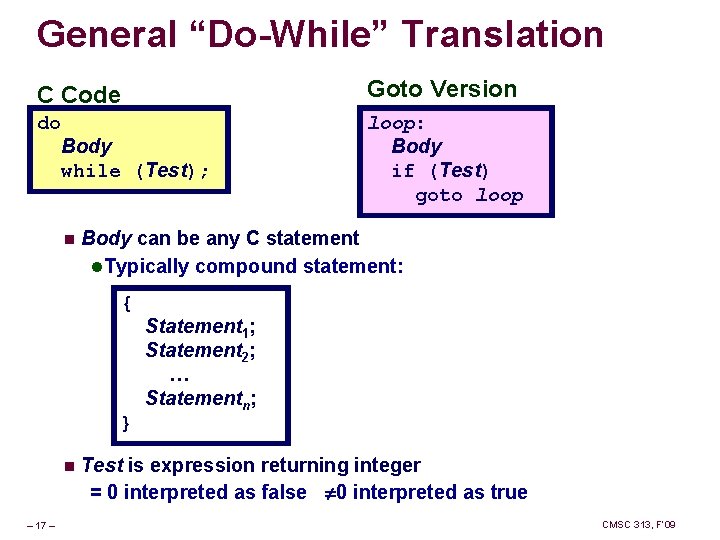
General “Do-While” Translation C Code Goto Version do loop: Body if (Test) goto loop Body while (Test); n Body can be any C statement l. Typically compound statement: { } n – 17 – Statement 1; Statement 2; … Statementn; Test is expression returning integer = 0 interpreted as false 0 interpreted as true CMSC 313, F’ 09
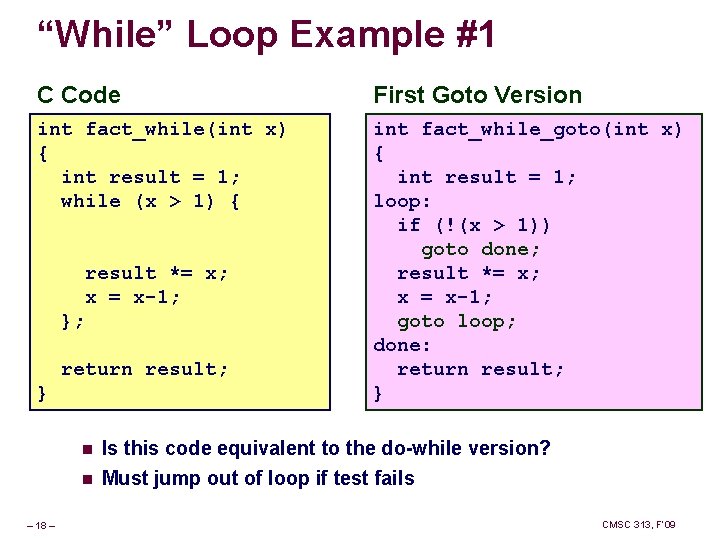
“While” Loop Example #1 C Code First Goto Version int fact_while(int x) { int result = 1; while (x > 1) { int fact_while_goto(int x) { int result = 1; loop: if (!(x > 1)) goto done; result *= x; x = x-1; goto loop; done: return result; } result *= x; x = x-1; }; return result; } n n – 18 – Is this code equivalent to the do-while version? Must jump out of loop if test fails CMSC 313, F’ 09
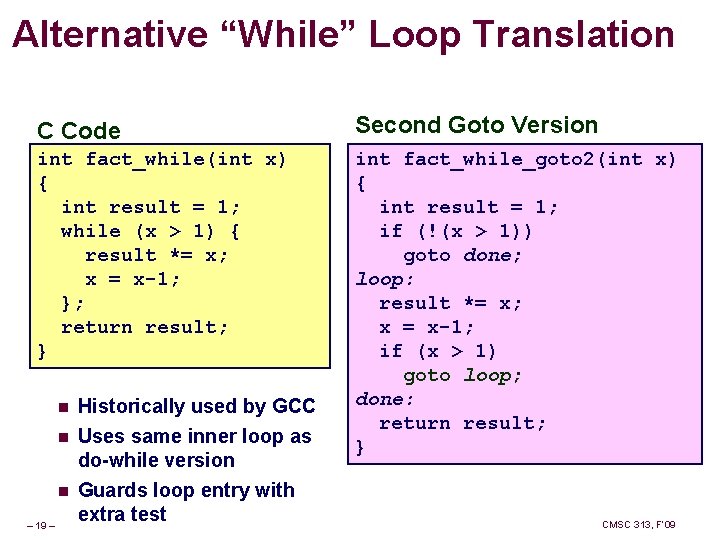
Alternative “While” Loop Translation C Code Second Goto Version int fact_while(int x) { int result = 1; while (x > 1) { result *= x; x = x-1; }; return result; } int fact_while_goto 2(int x) { int result = 1; if (!(x > 1)) goto done; loop: result *= x; x = x-1; if (x > 1) goto loop; done: return result; } n n n – 19 – Historically used by GCC Uses same inner loop as do-while version Guards loop entry with extra test CMSC 313, F’ 09
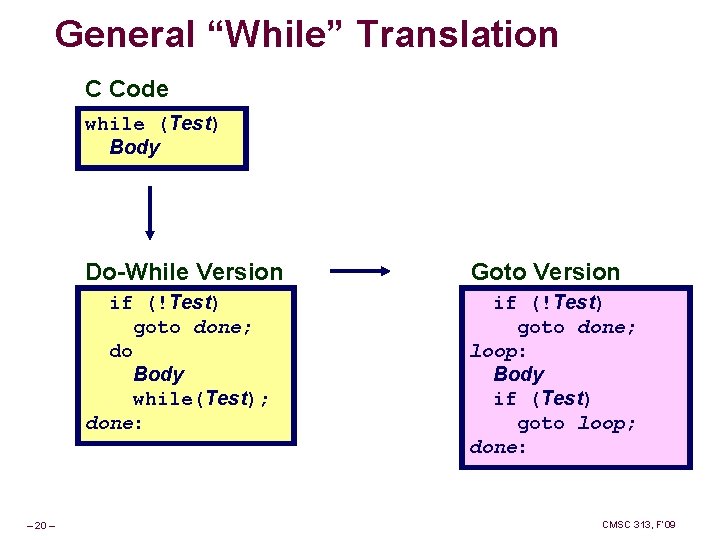
General “While” Translation C Code while (Test) Body – 20 – Do-While Version Goto Version if (!Test) goto done; do Body while(Test); done: if (!Test) goto done; loop: Body if (Test) goto loop; done: CMSC 313, F’ 09
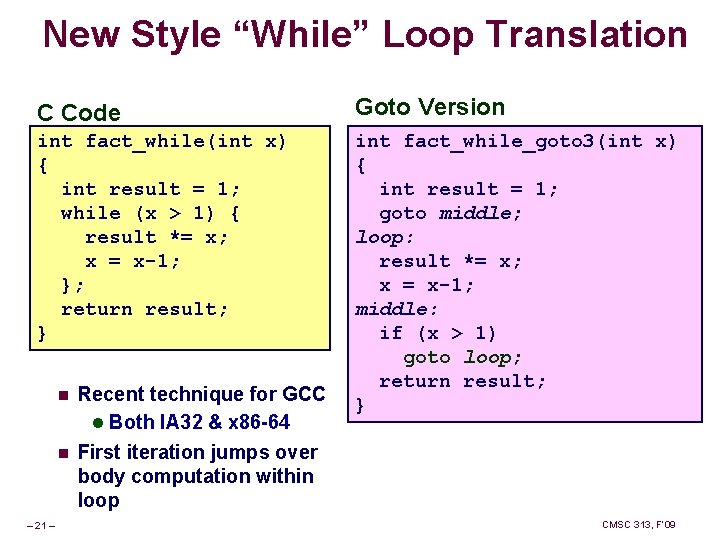
New Style “While” Loop Translation C Code Goto Version int fact_while(int x) { int result = 1; while (x > 1) { result *= x; x = x-1; }; return result; } int fact_while_goto 3(int x) { int result = 1; goto middle; loop: result *= x; x = x-1; middle: if (x > 1) goto loop; return result; } – 21 – n Recent technique for GCC l Both IA 32 & x 86 -64 n First iteration jumps over body computation within loop CMSC 313, F’ 09
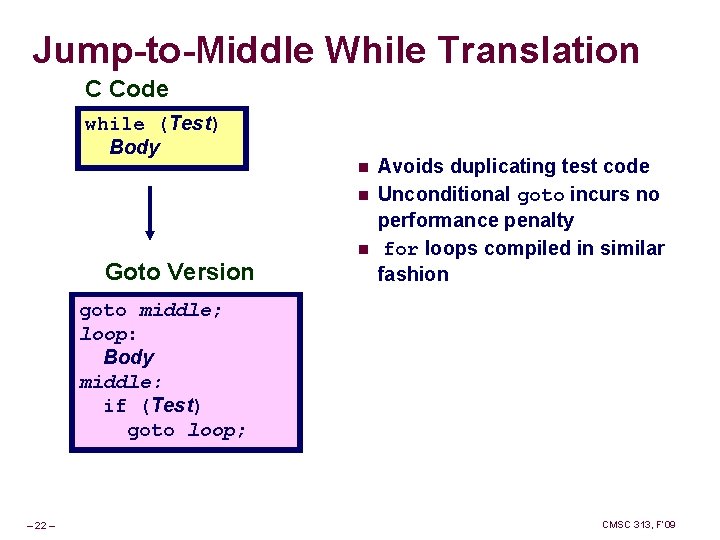
Jump-to-Middle While Translation C Code while (Test) Body n n Goto Version n Avoids duplicating test code Unconditional goto incurs no performance penalty for loops compiled in similar fashion goto middle; loop: Body middle: if (Test) goto loop; – 22 – CMSC 313, F’ 09
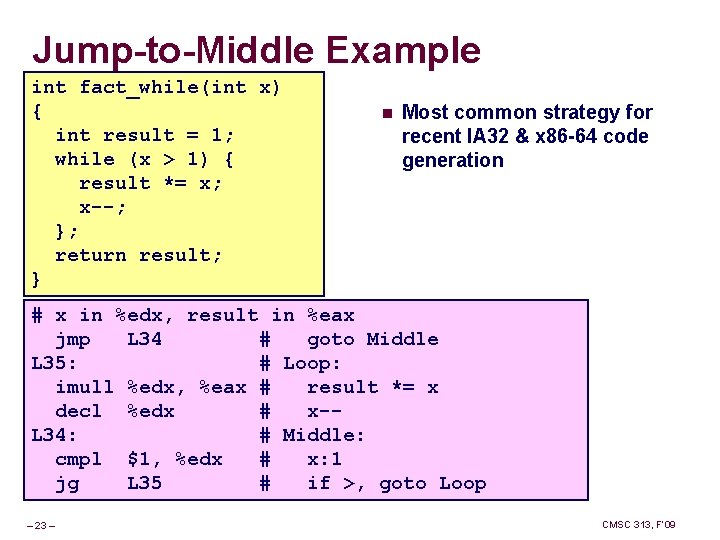
Jump-to-Middle Example int fact_while(int x) { int result = 1; while (x > 1) { result *= x; x--; }; return result; } n Most common strategy for recent IA 32 & x 86 -64 code generation # x in %edx, result in %eax jmp L 34 # goto Middle L 35: # Loop: imull %edx, %eax # result *= x decl %edx # x-L 34: # Middle: cmpl $1, %edx # x: 1 jg L 35 # if >, goto Loop – 23 – CMSC 313, F’ 09
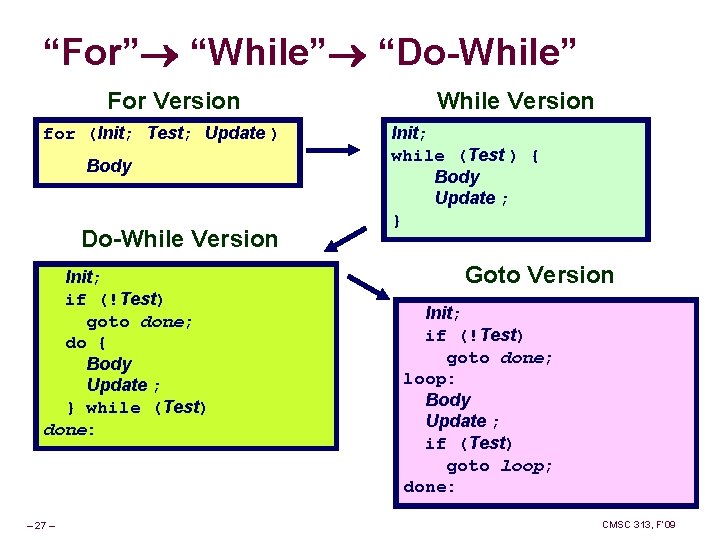
“For” “While” “Do-While” For Version for (Init; Test; Update ) Body Do-While Version Init; if (!Test) goto done; do { Body Update ; } while (Test) done: – 27 – While Version Init; while (Test ) { Body Update ; } Goto Version Init; if (!Test) goto done; loop: Body Update ; if (Test) goto loop; done: CMSC 313, F’ 09
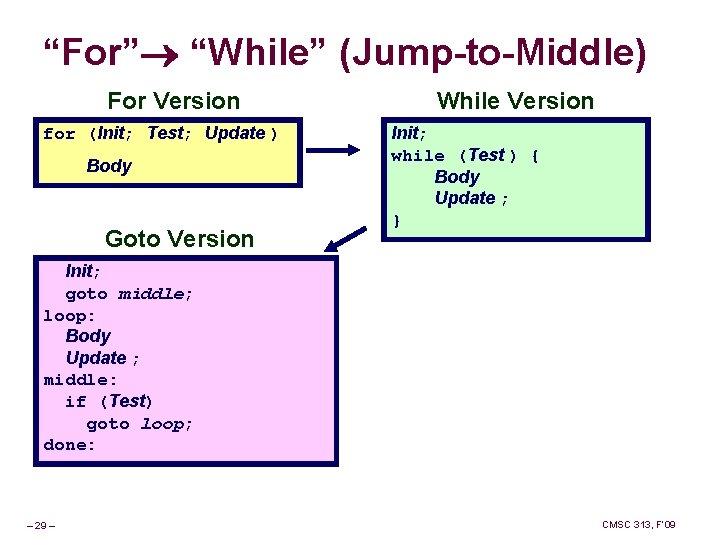
“For” “While” (Jump-to-Middle) For Version for (Init; Test; Update ) Body Goto Version While Version Init; while (Test ) { Body Update ; } Init; goto middle; loop: Body Update ; middle: if (Test) goto loop; done: – 29 – CMSC 313, F’ 09
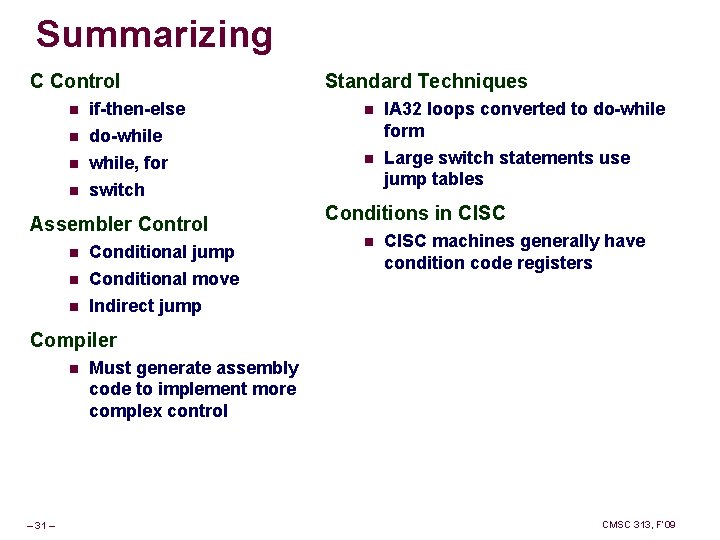
Summarizing C Control Standard Techniques n n if-then-else do-while IA 32 loops converted to do-while form n while, for n n switch Large switch statements use jump tables n Assembler Control n n n Conditional jump Conditional move Indirect jump Conditions in CISC machines generally have condition code registers Compiler n – 31 – Must generate assembly code to implement more complex control CMSC 313, F’ 09
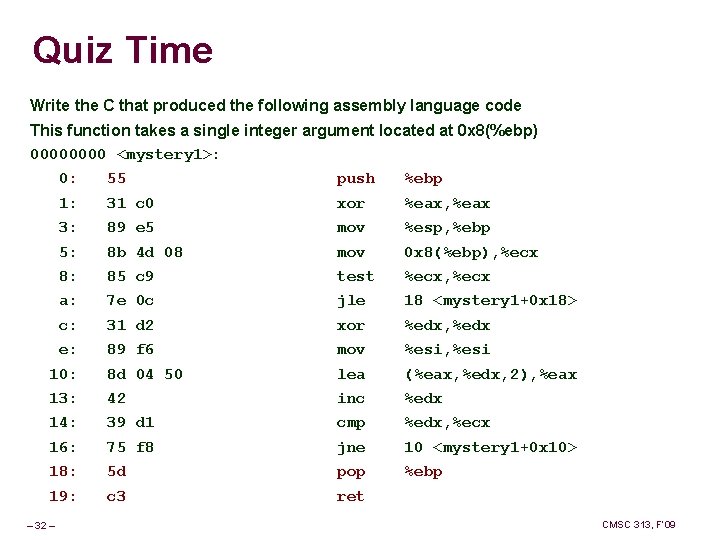
Quiz Time Write the C that produced the following assembly language code This function takes a single integer argument located at 0 x 8(%ebp) 0000 <mystery 1>: 0: 55 push %ebp 1: 31 c 0 xor %eax, %eax 3: 89 e 5 mov %esp, %ebp 5: 8 b 4 d 08 mov 0 x 8(%ebp), %ecx 8: 85 c 9 test %ecx, %ecx a: 7 e 0 c jle 18 <mystery 1+0 x 18> c: 31 d 2 xor %edx, %edx e: 89 f 6 mov %esi, %esi 10: 8 d 04 50 lea (%eax, %edx, 2), %eax 13: 42 inc %edx 14: 39 d 1 cmp %edx, %ecx 16: 75 f 8 jne 10 <mystery 1+0 x 10> 18: 5 d pop %ebp 19: c 3 ret – 32 – CMSC 313, F’ 09
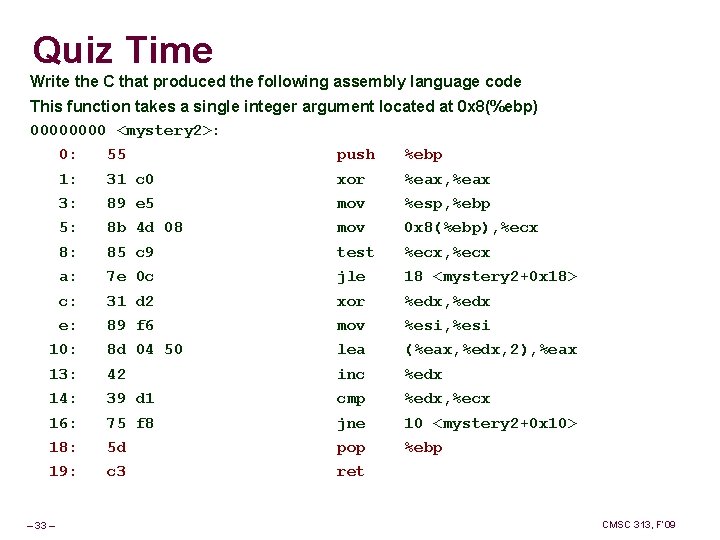
Quiz Time Write the C that produced the following assembly language code This function takes a single integer argument located at 0 x 8(%ebp) 0000 <mystery 2>: 0: 55 push %ebp 1: 31 c 0 xor %eax, %eax 3: 89 e 5 mov %esp, %ebp 5: 8 b 4 d 08 mov 0 x 8(%ebp), %ecx 8: 85 c 9 test %ecx, %ecx a: 7 e 0 c jle 18 <mystery 2+0 x 18> c: 31 d 2 xor %edx, %edx e: 89 f 6 mov %esi, %esi 10: 8 d 04 50 lea (%eax, %edx, 2), %eax 13: 42 inc %edx 14: 39 d 1 cmp %edx, %ecx 16: 75 f 8 jne 10 <mystery 2+0 x 10> 18: 5 d pop %ebp 19: c 3 ret – 33 – CMSC 313, F’ 09