Lecture 5 Loops 1 Outline n n Introduction
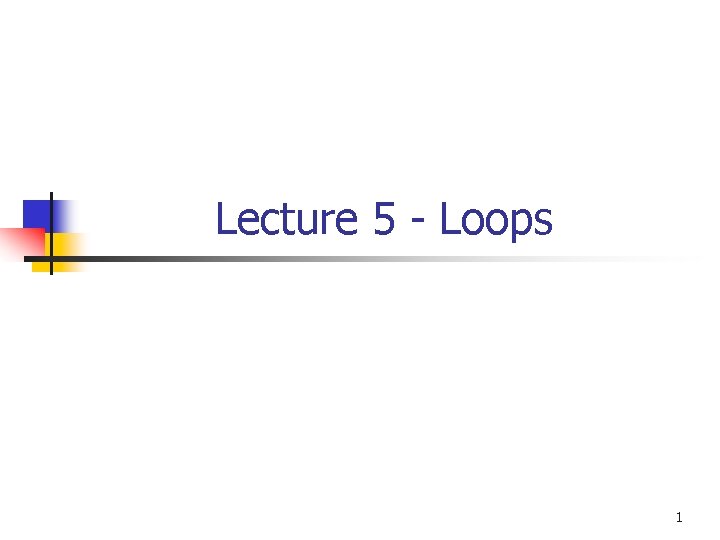
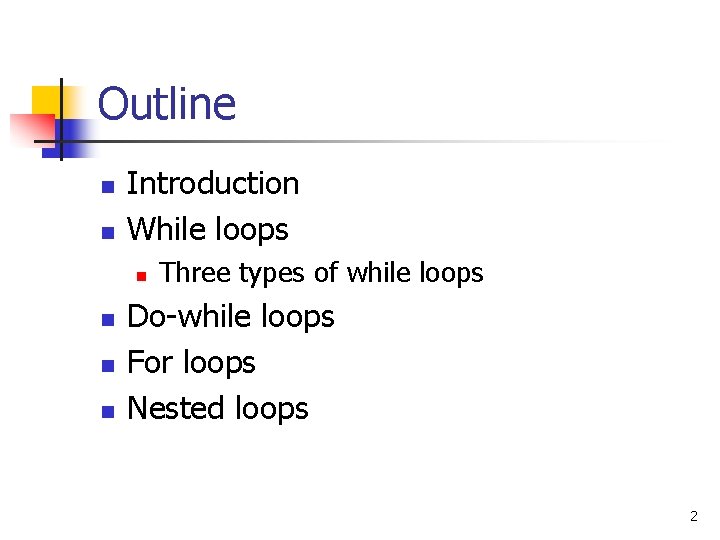
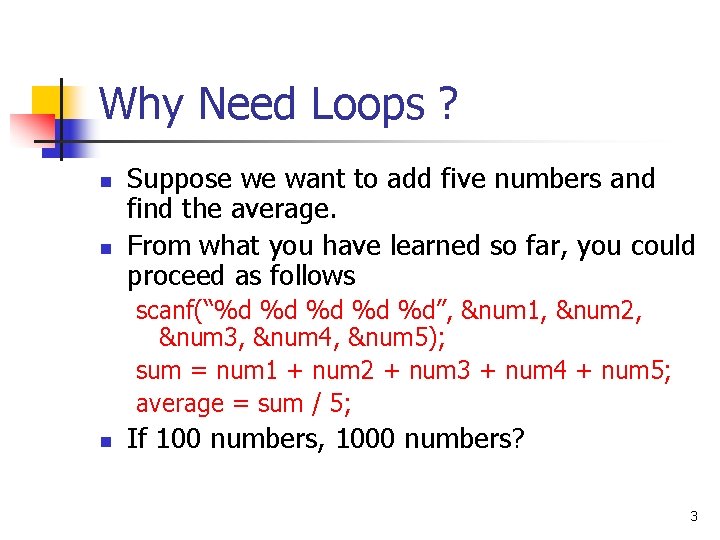
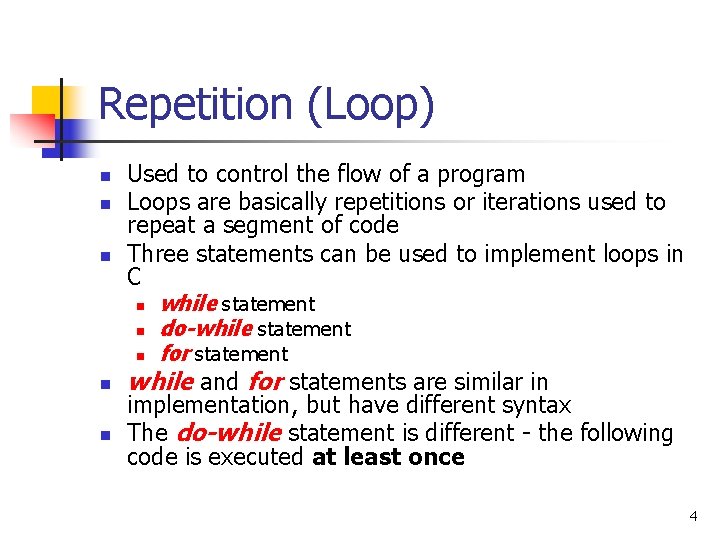
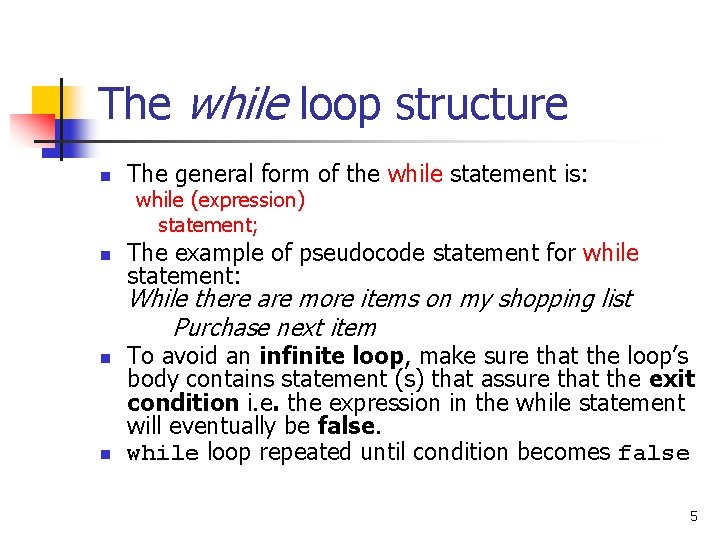
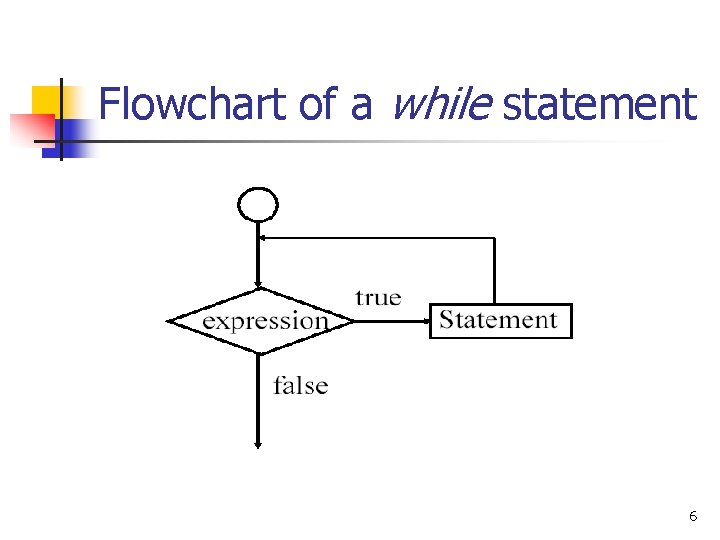
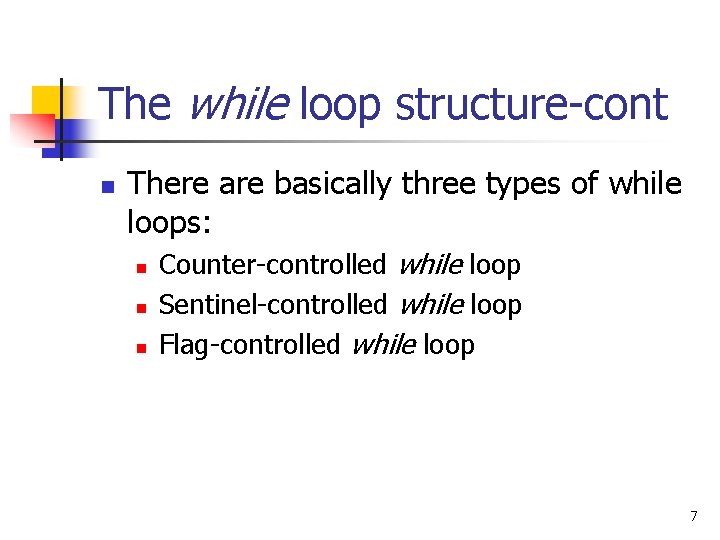
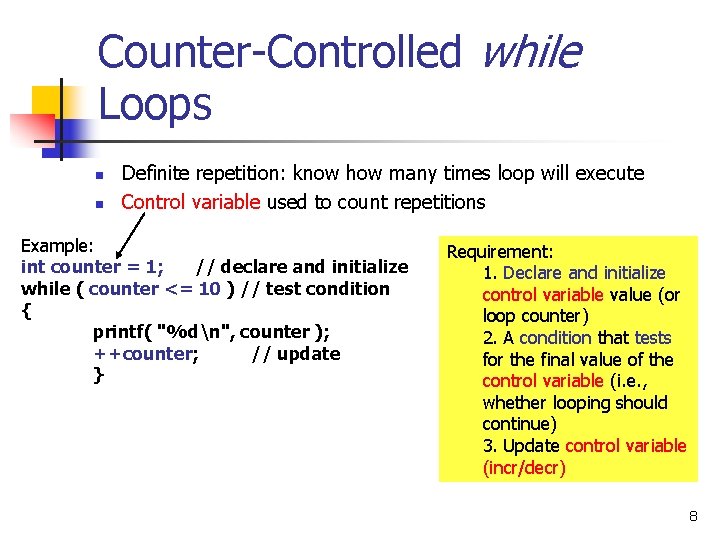
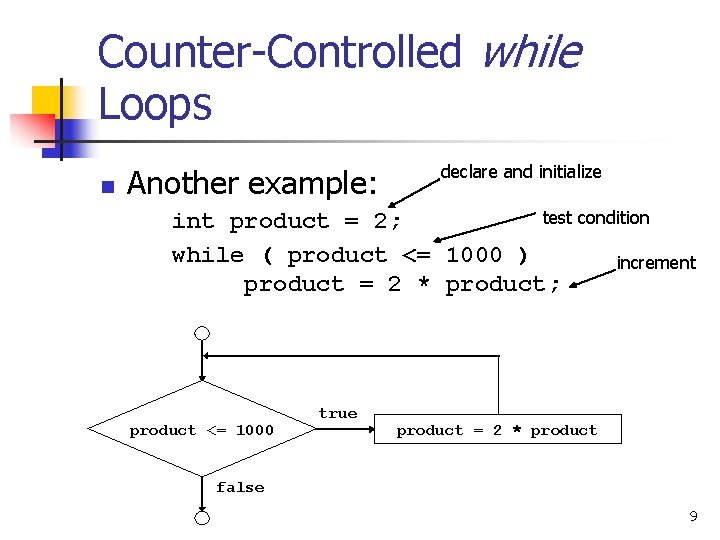
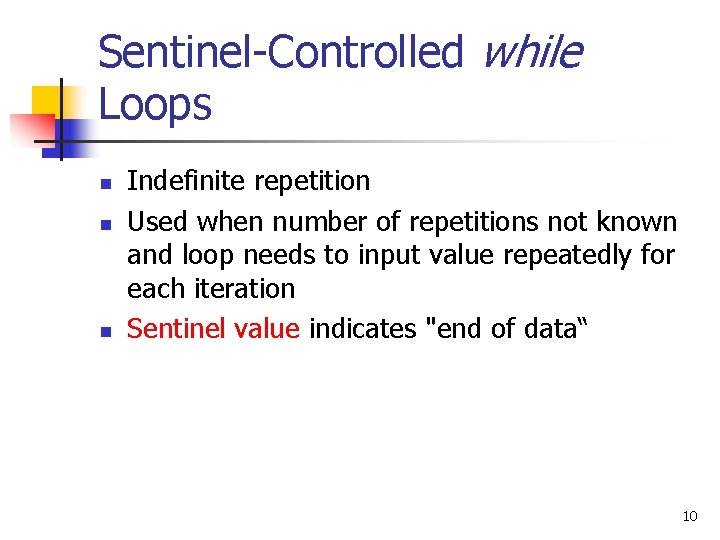
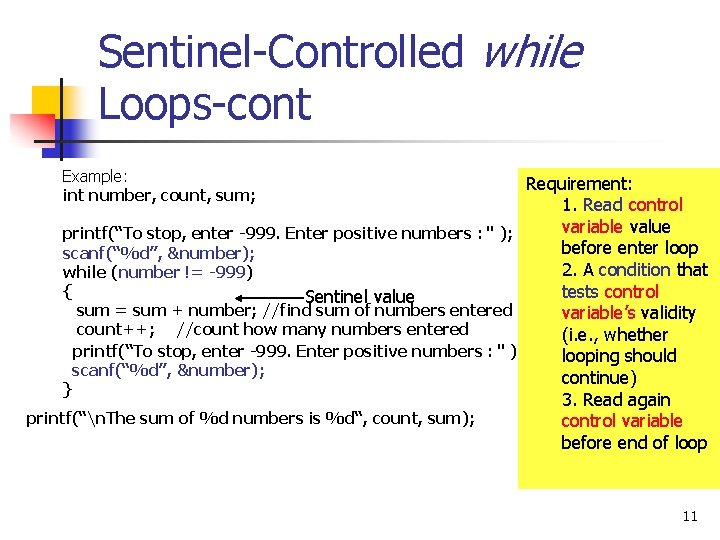
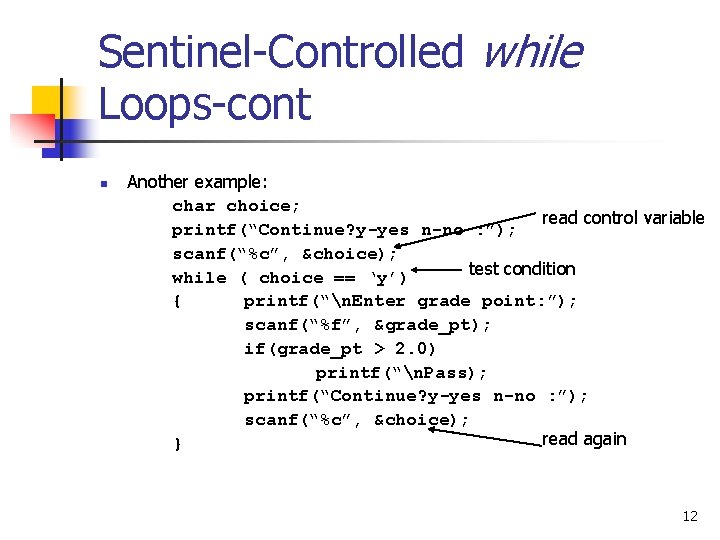
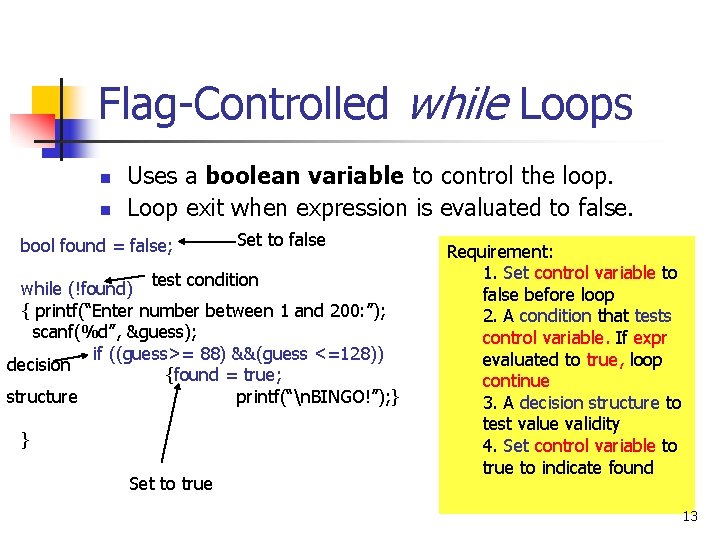
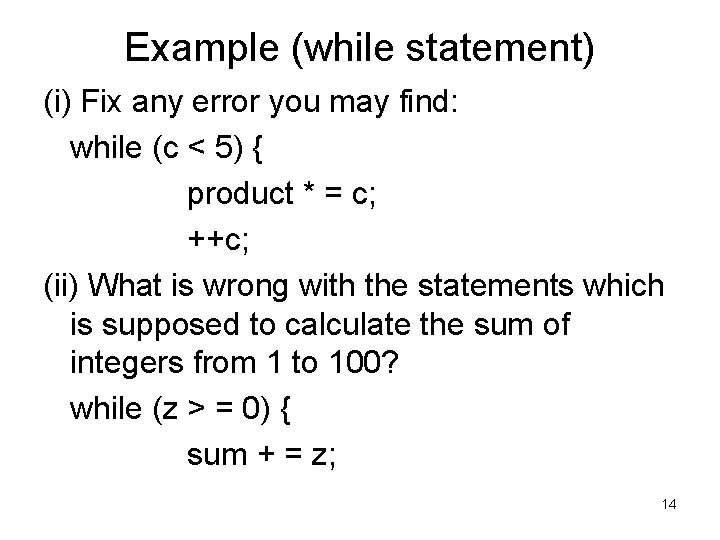
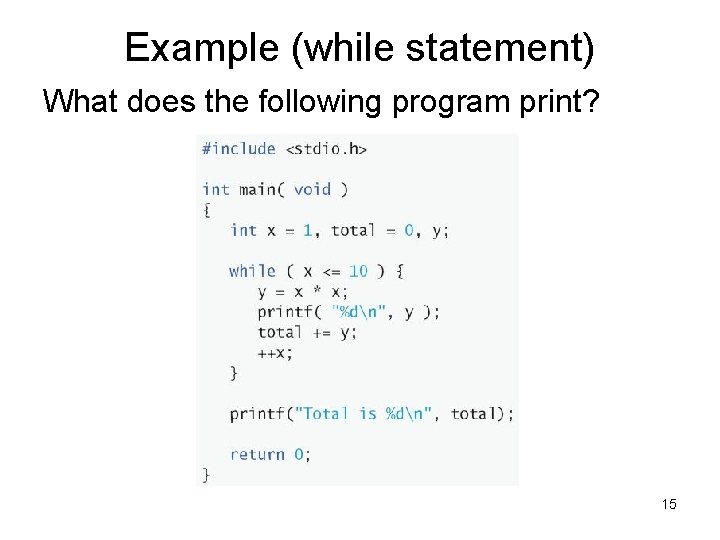
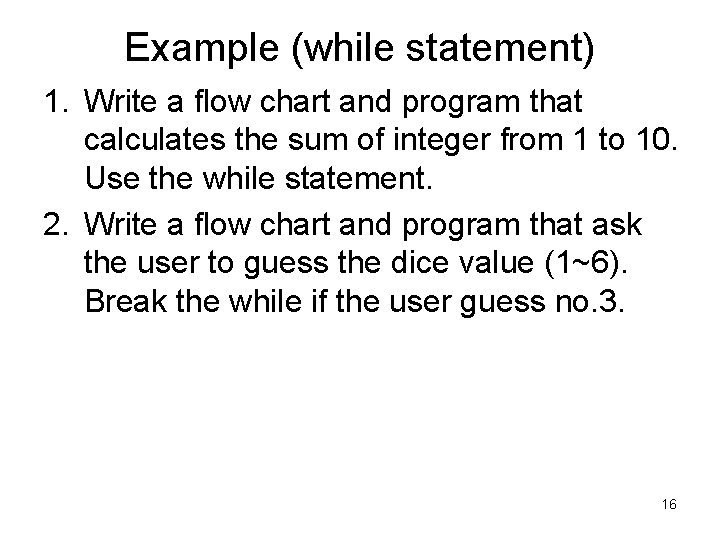
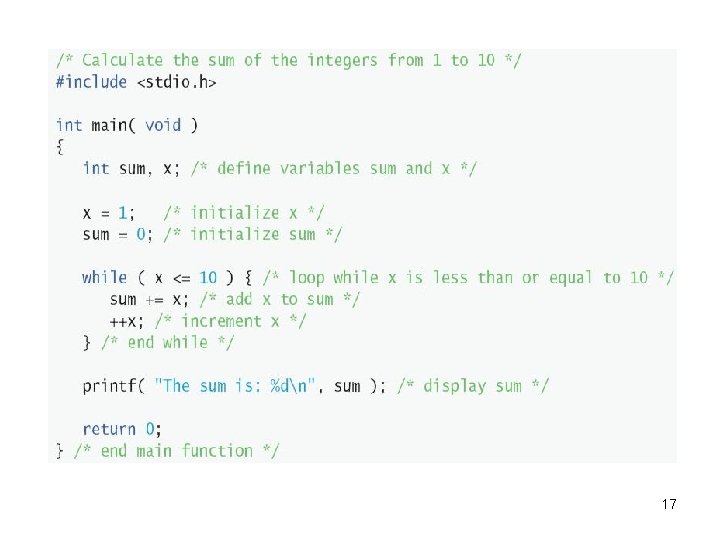
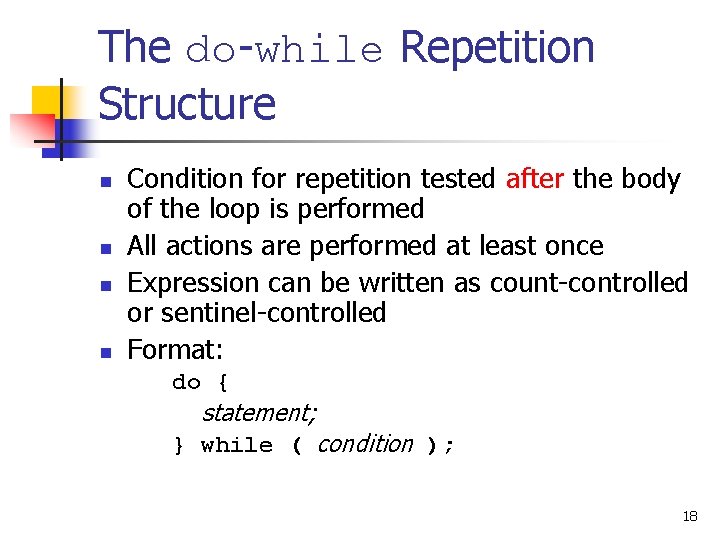
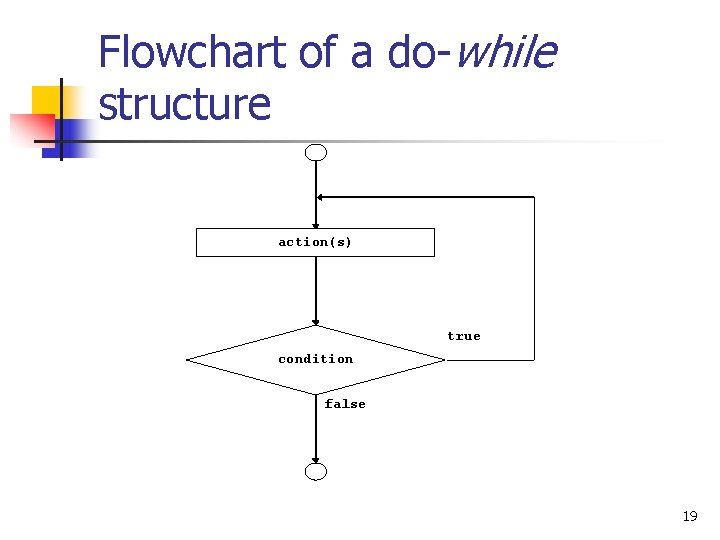
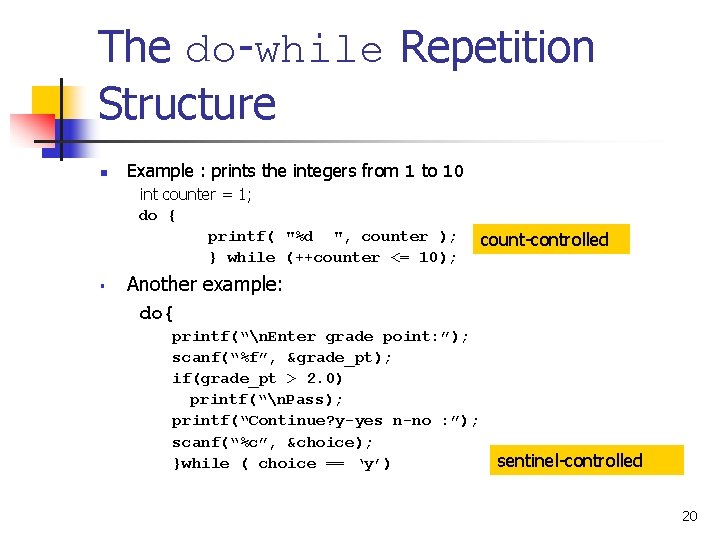
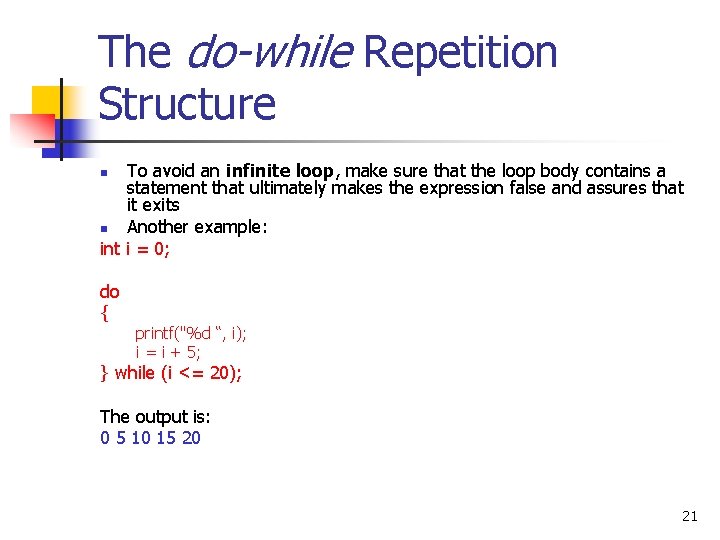
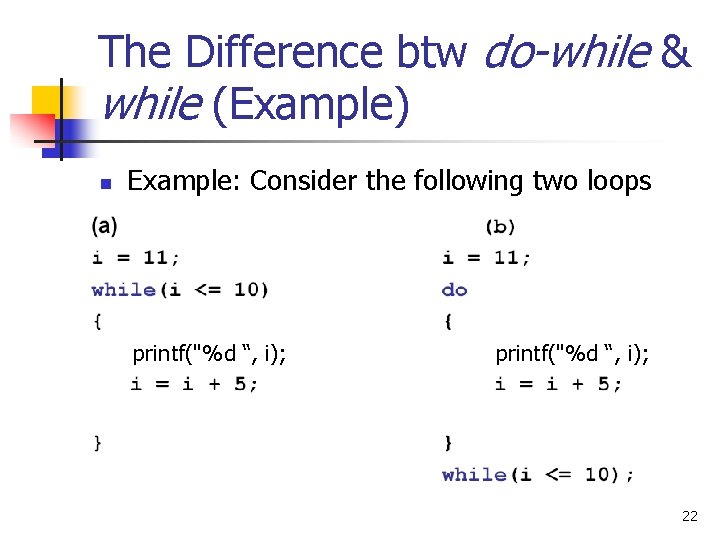
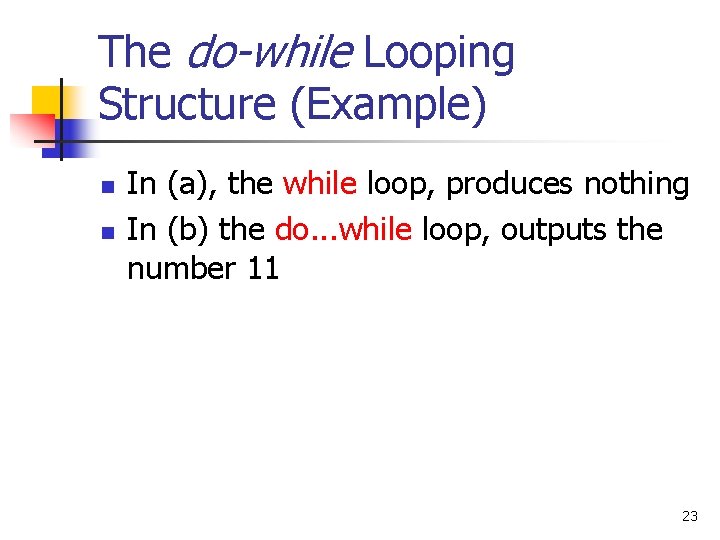
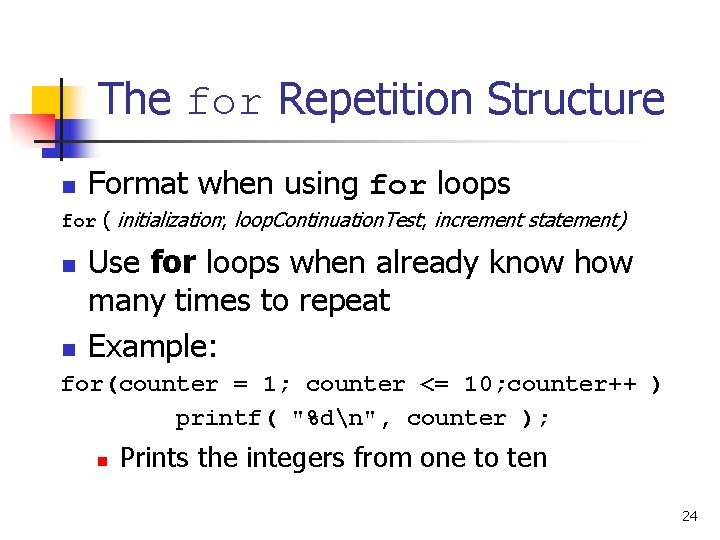
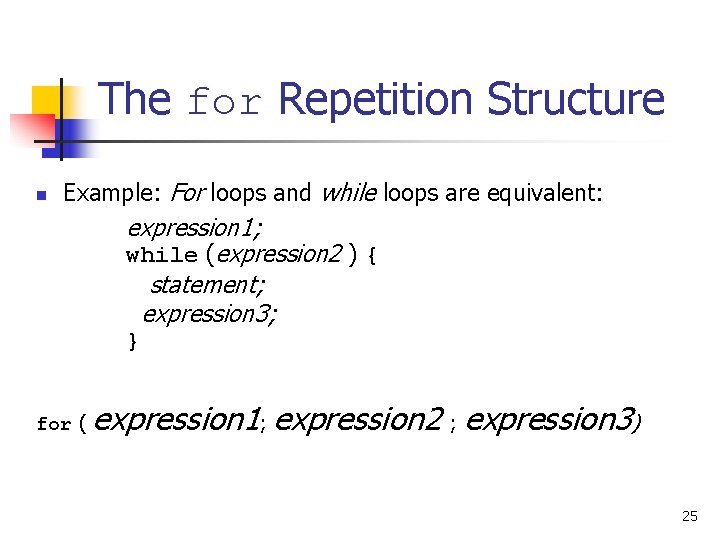
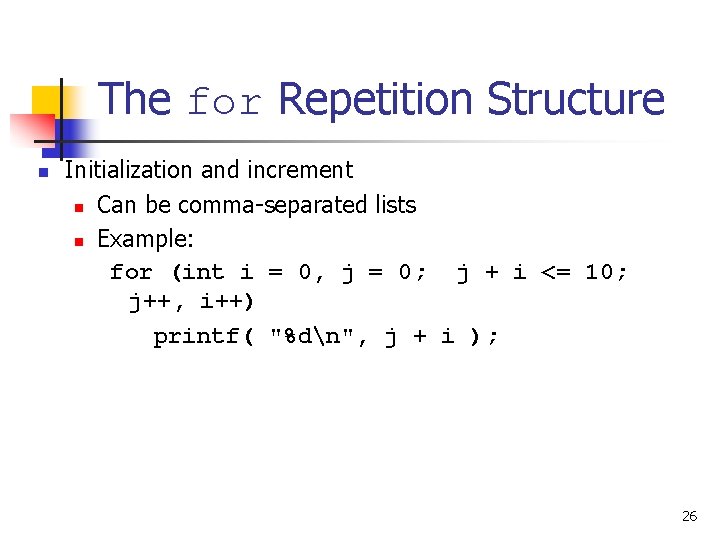
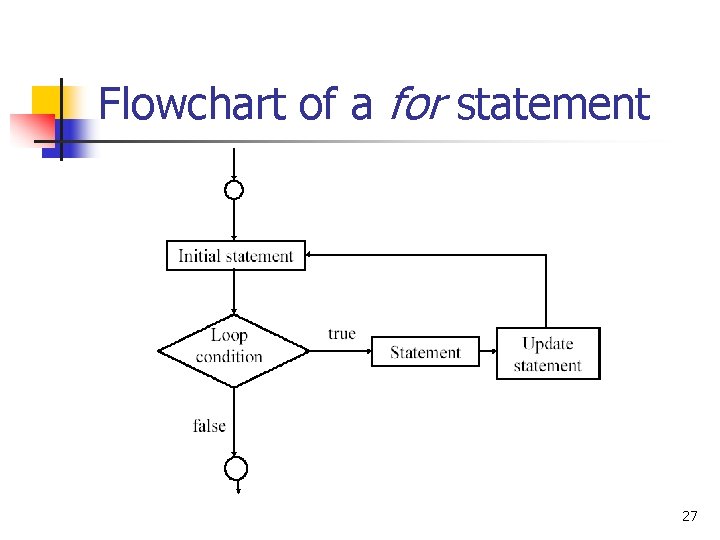
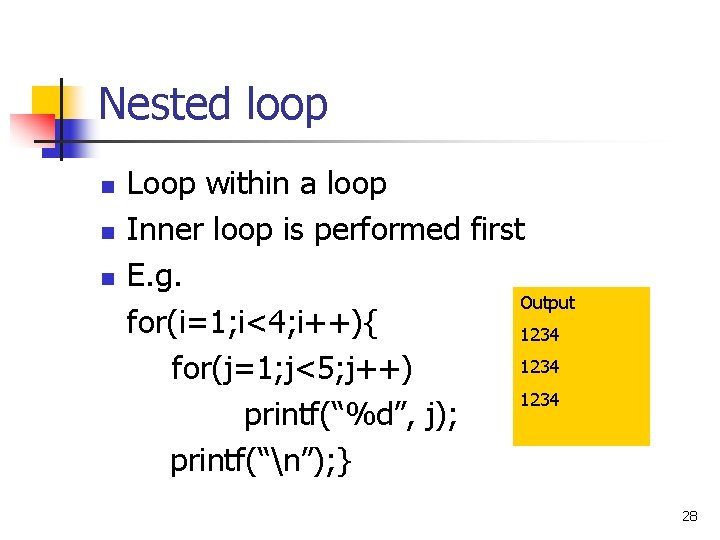
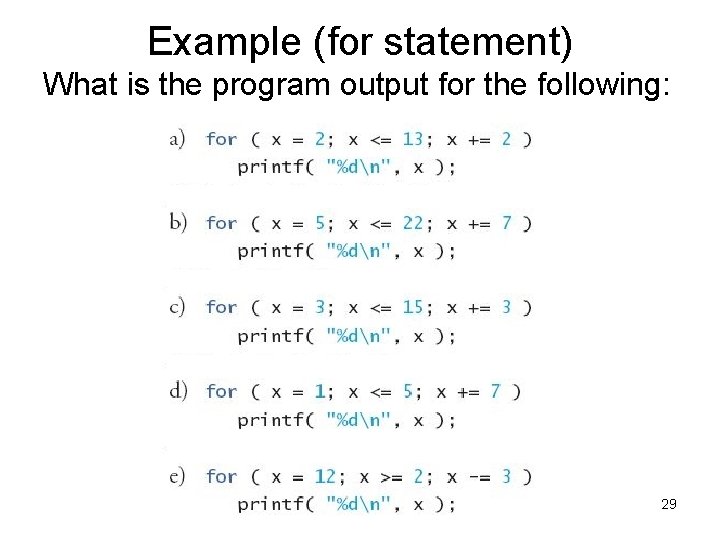
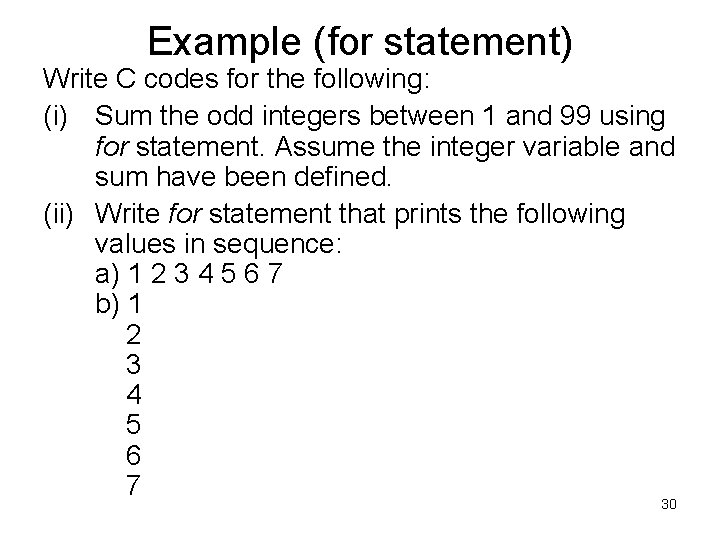
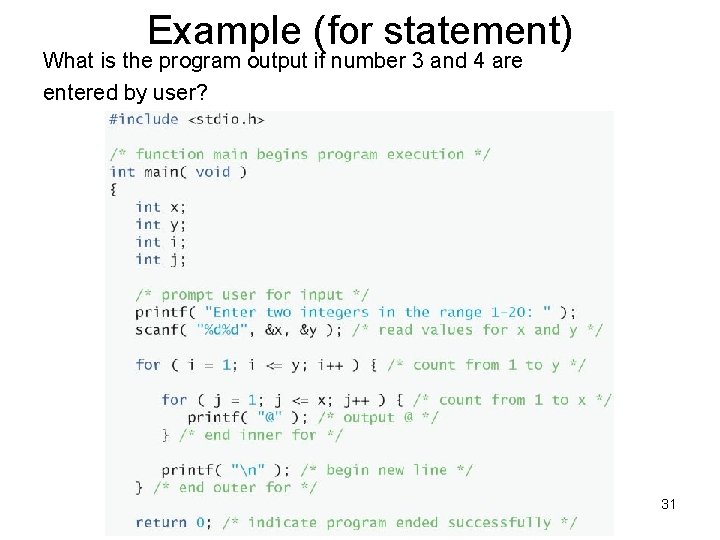
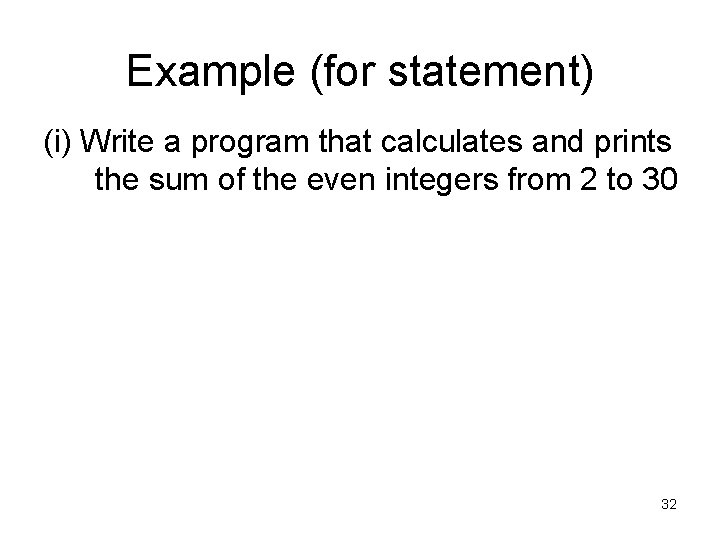
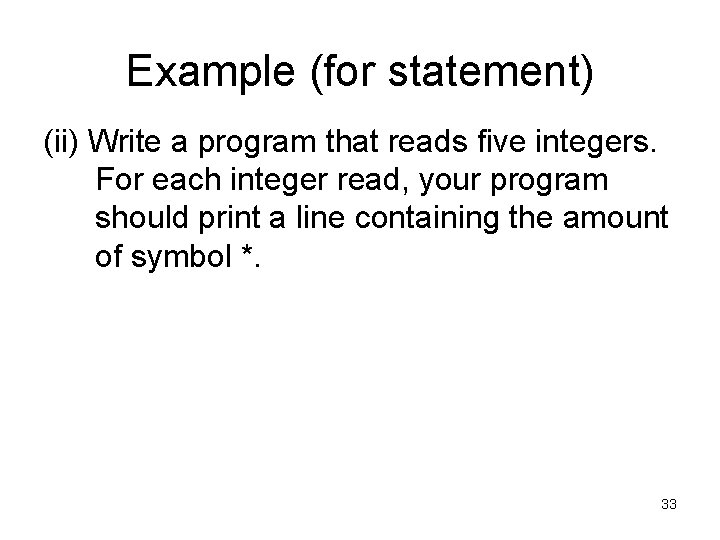
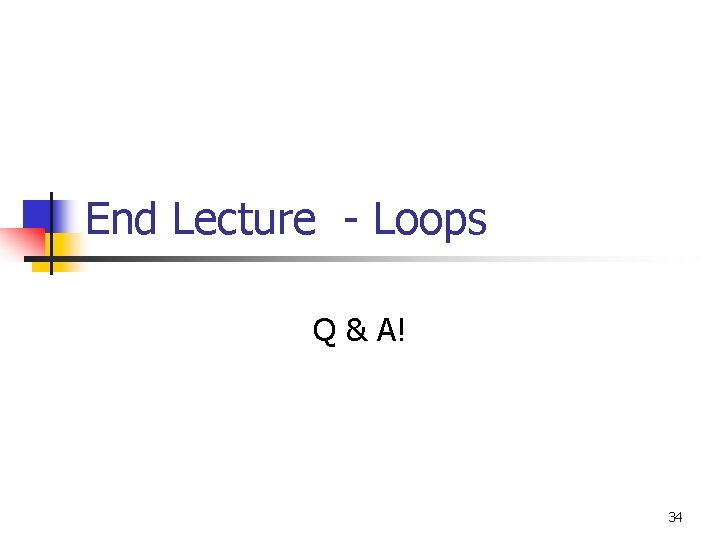
- Slides: 34
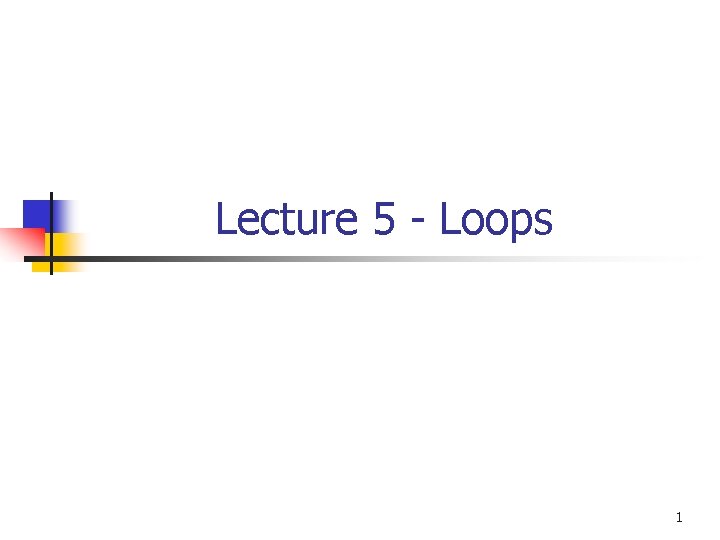
Lecture 5 - Loops 1
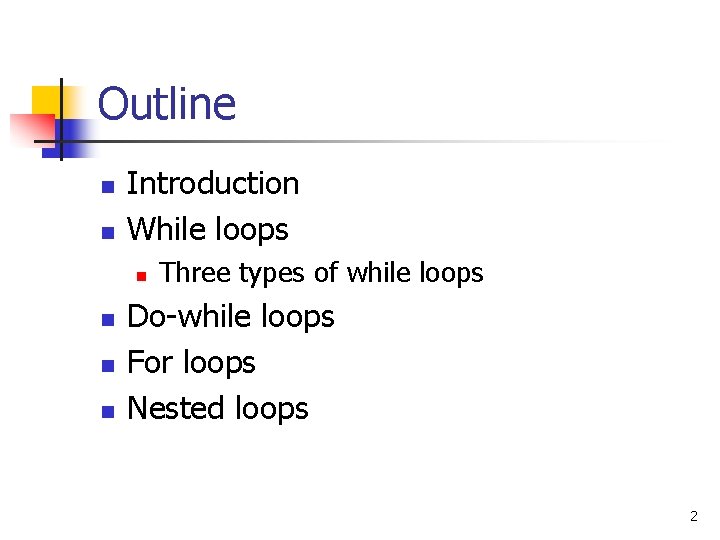
Outline n n Introduction While loops n n Three types of while loops Do-while loops For loops Nested loops 2
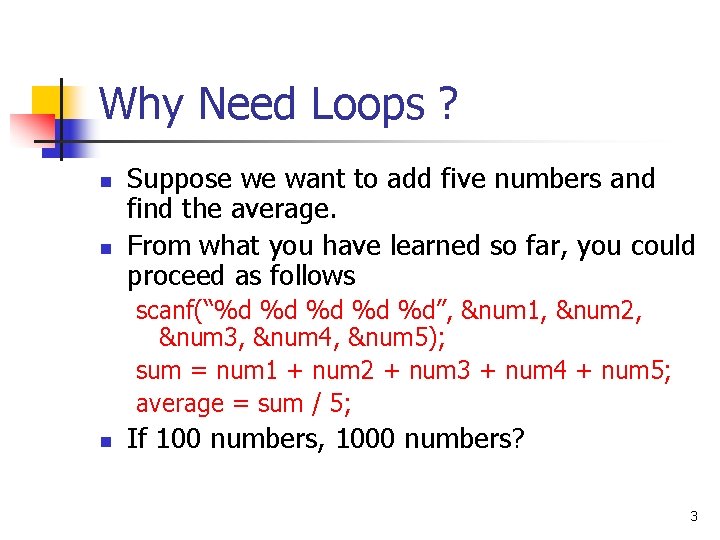
Why Need Loops ? n n Suppose we want to add five numbers and find the average. From what you have learned so far, you could proceed as follows scanf(“%d %d %d”, &num 1, &num 2, &num 3, &num 4, &num 5); sum = num 1 + num 2 + num 3 + num 4 + num 5; average = sum / 5; n If 100 numbers, 1000 numbers? 3
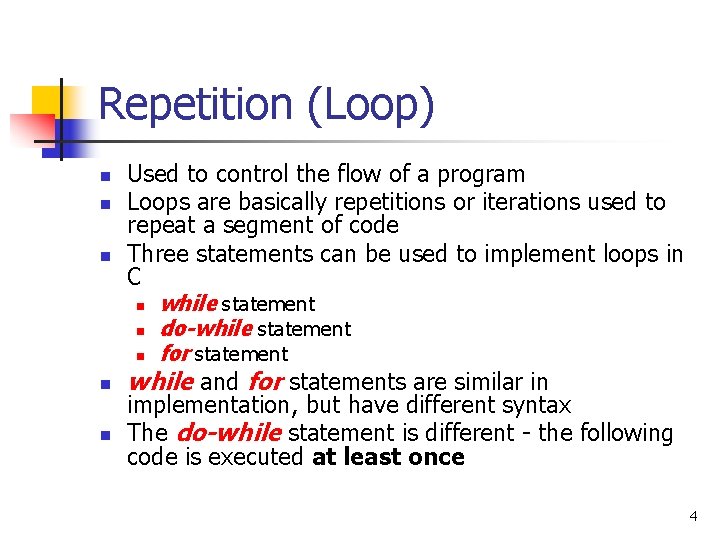
Repetition (Loop) n n n Used to control the flow of a program Loops are basically repetitions or iterations used to repeat a segment of code Three statements can be used to implement loops in C n n n while statement do-while statement for statement while and for statements are similar in implementation, but have different syntax The do-while statement is different - the following code is executed at least once 4
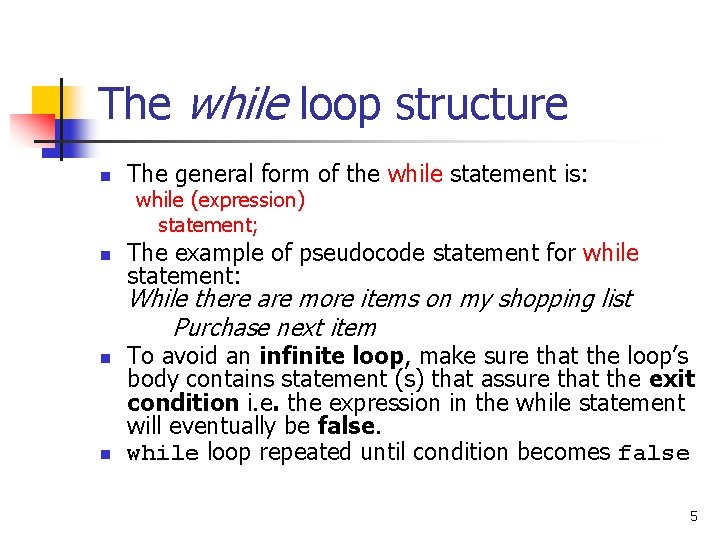
The while loop structure n The general form of the while statement is: while (expression) statement; n The example of pseudocode statement for while statement: While there are more items on my shopping list Purchase next item n n To avoid an infinite loop, make sure that the loop’s body contains statement (s) that assure that the exit condition i. e. the expression in the while statement will eventually be false. while loop repeated until condition becomes false 5
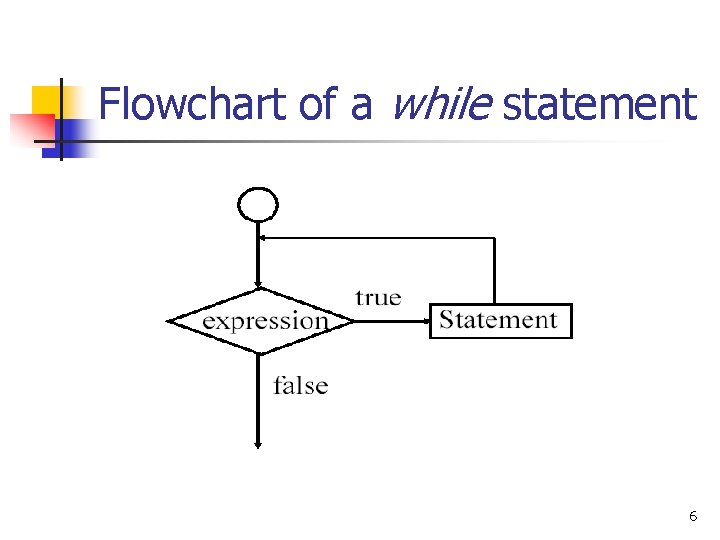
Flowchart of a while statement 6
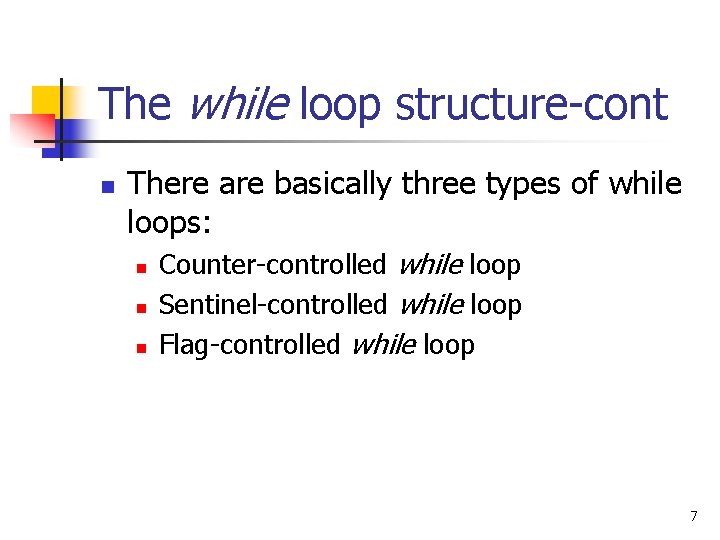
The while loop structure-cont n There are basically three types of while loops: n n n Counter-controlled while loop Sentinel-controlled while loop Flag-controlled while loop 7
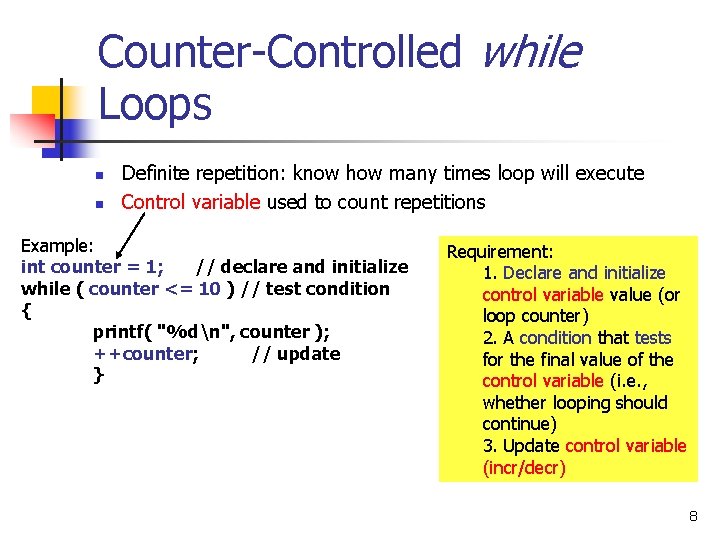
Counter-Controlled while Loops n n Definite repetition: know how many times loop will execute Control variable used to count repetitions Example: int counter = 1; // declare and initialize while ( counter <= 10 ) // test condition { printf( "%dn", counter ); ++counter; // update } Requirement: 1. Declare and initialize control variable value (or loop counter) 2. A condition that tests for the final value of the control variable (i. e. , whether looping should continue) 3. Update control variable (incr/decr) 8
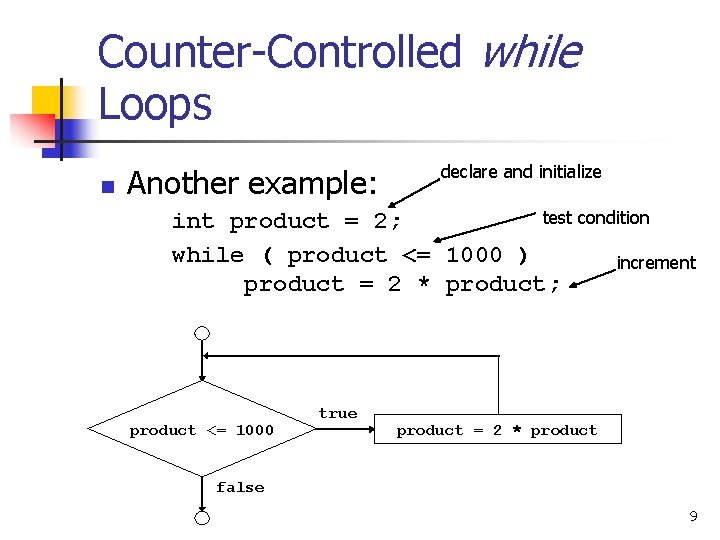
Counter-Controlled while Loops n Another example: declare and initialize test condition int product = 2; while ( product <= 1000 ) increment product = 2 * product; product <= 1000 true product = 2 * product false 9
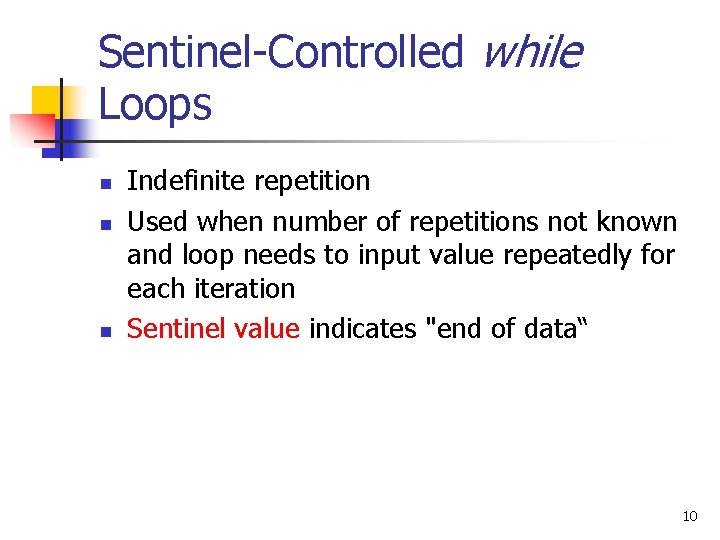
Sentinel-Controlled while Loops n n n Indefinite repetition Used when number of repetitions not known and loop needs to input value repeatedly for each iteration Sentinel value indicates "end of data“ 10
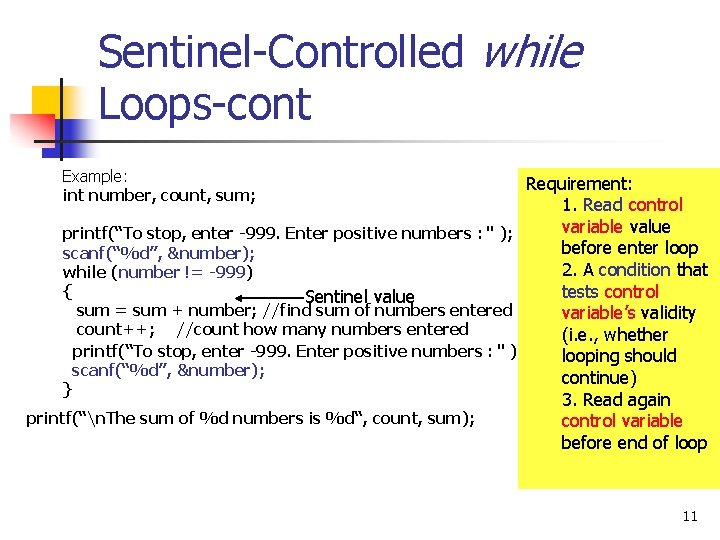
Sentinel-Controlled while Loops-cont Example: int number, count, sum; Requirement: 1. Read control variable value printf(“To stop, enter -999. Enter positive numbers : " ); before enter loop scanf(“%d”, &number); 2. A condition that while (number != -999) { tests control Sentinel value sum = sum + number; //find sum of numbers entered variable’s validity count++; //count how many numbers entered (i. e. , whether printf(“To stop, enter -999. Enter positive numbers : " ); looping should scanf(“%d”, &number); continue) } 3. Read again printf(“n. The sum of %d numbers is %d“, count, sum); control variable before end of loop 11
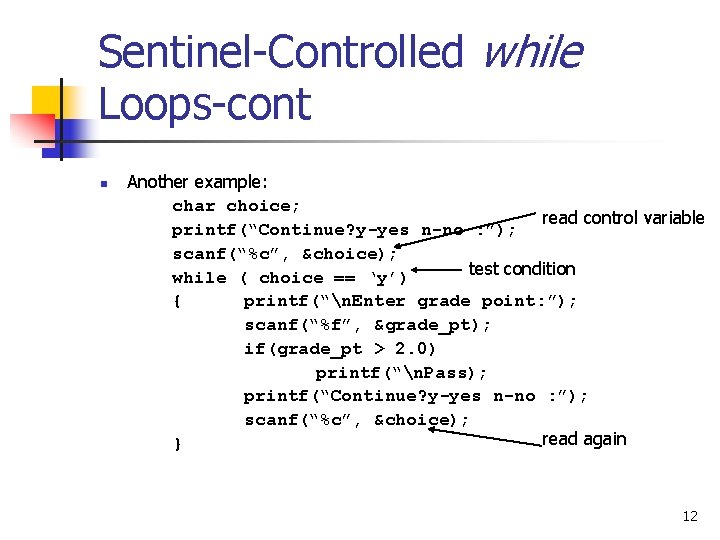
Sentinel-Controlled while Loops-cont n Another example: char choice; read control variable printf(“Continue? y-yes n-no : ”); scanf(“%c”, &choice); test condition while ( choice == ‘y’) { printf(“n. Enter grade point: ”); scanf(“%f”, &grade_pt); if(grade_pt > 2. 0) printf(“n. Pass); printf(“Continue? y-yes n-no : ”); scanf(“%c”, &choice); read again } 12
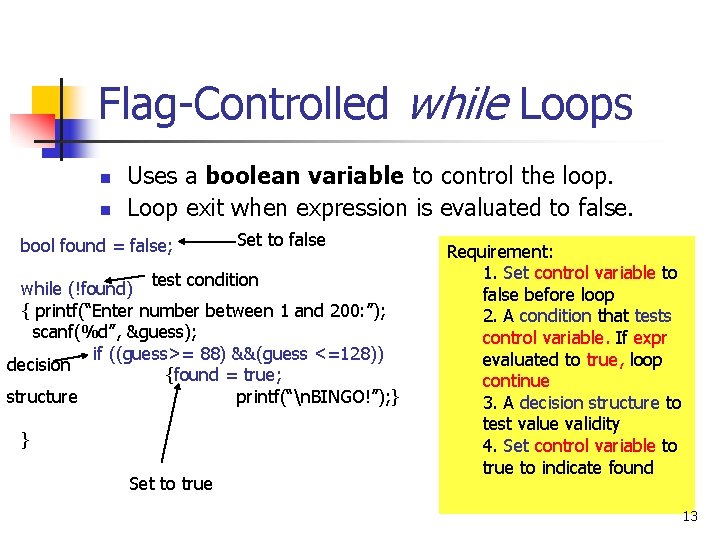
Flag-Controlled while Loops n n Uses a boolean variable to control the loop. Loop exit when expression is evaluated to false. bool found = false; Set to false test condition while (!found) { printf(“Enter number between 1 and 200: ”); scanf(%d”, &guess); if ((guess>= 88) &&(guess <=128)) decision {found = true; printf(“n. BINGO!”); } structure } Set to true Requirement: 1. Set control variable to false before loop 2. A condition that tests control variable. If expr evaluated to true, loop continue 3. A decision structure to test value validity 4. Set control variable to true to indicate found 13
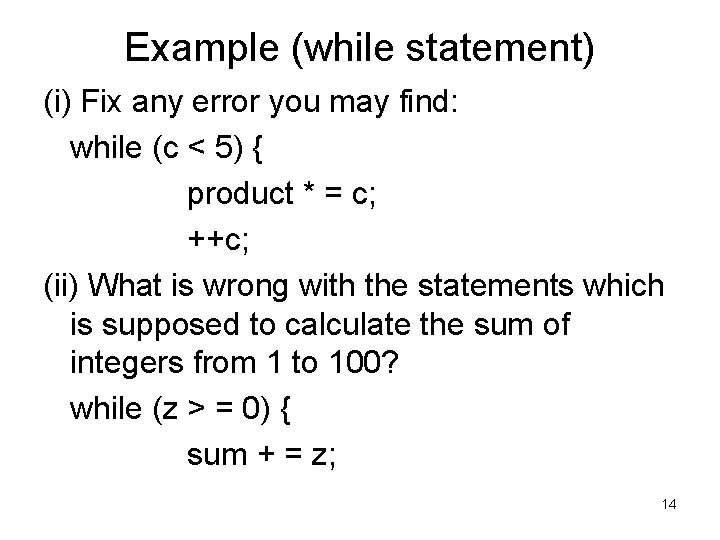
Example (while statement) (i) Fix any error you may find: while (c < 5) { product * = c; ++c; (ii) What is wrong with the statements which is supposed to calculate the sum of integers from 1 to 100? while (z > = 0) { sum + = z; 14
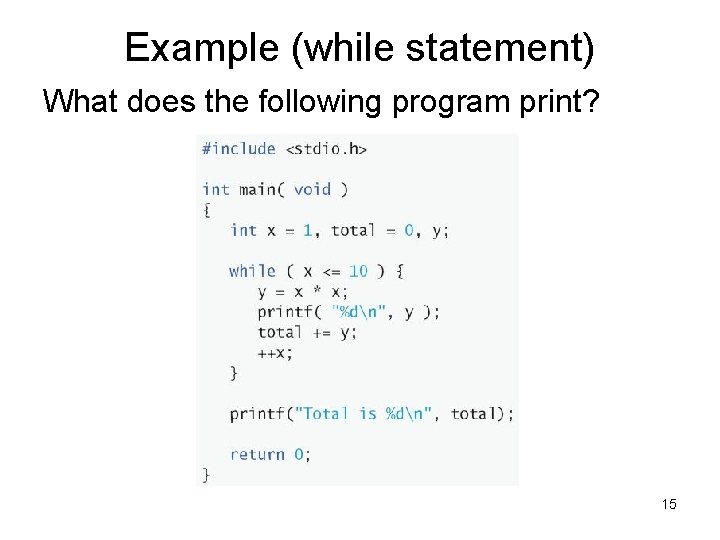
Example (while statement) What does the following program print? 15
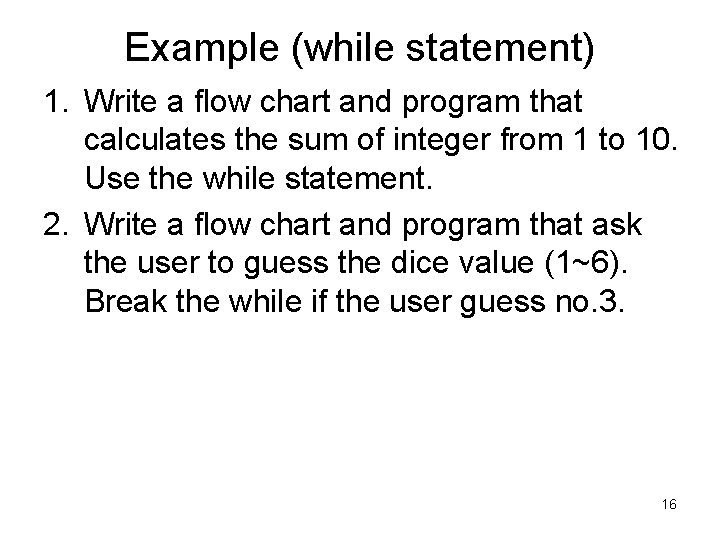
Example (while statement) 1. Write a flow chart and program that calculates the sum of integer from 1 to 10. Use the while statement. 2. Write a flow chart and program that ask the user to guess the dice value (1~6). Break the while if the user guess no. 3. 16
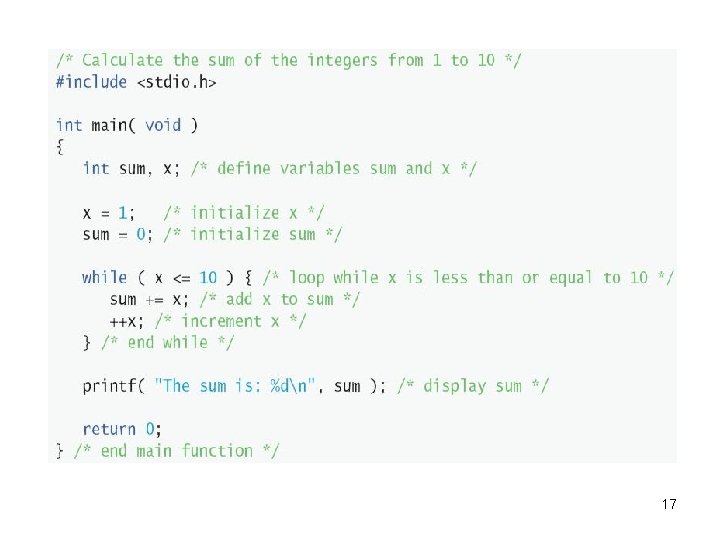
17
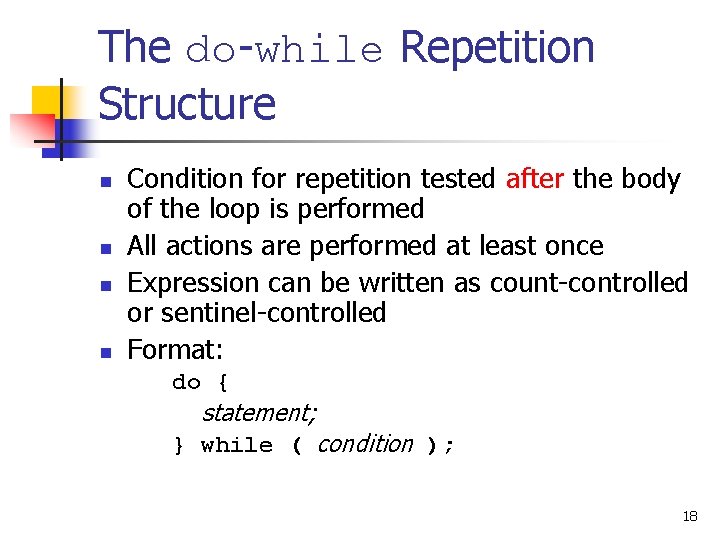
The do-while Repetition Structure n n Condition for repetition tested after the body of the loop is performed All actions are performed at least once Expression can be written as count-controlled or sentinel-controlled Format: do { statement; } while ( condition ); 18
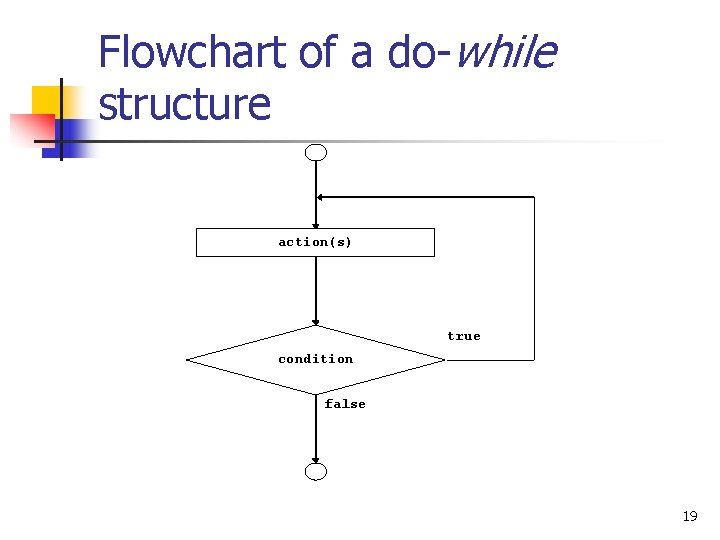
Flowchart of a do-while structure action(s) true condition false 19
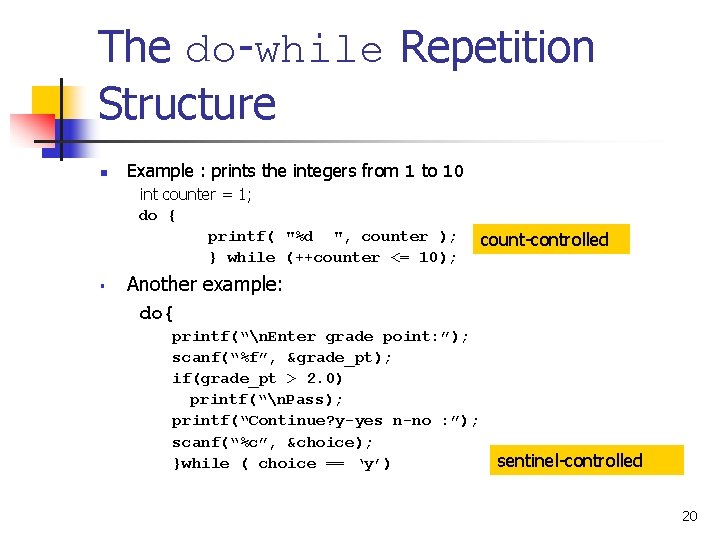
The do-while Repetition Structure n Example : prints the integers from 1 to 10 int counter = 1; do { printf( "%d ", counter ); } while (++counter <= 10); § count-controlled Another example: do{ printf(“n. Enter grade point: ”); scanf(“%f”, &grade_pt); if(grade_pt > 2. 0) printf(“n. Pass); printf(“Continue? y-yes n-no : ”); scanf(“%c”, &choice); }while ( choice == ‘y’) sentinel-controlled 20
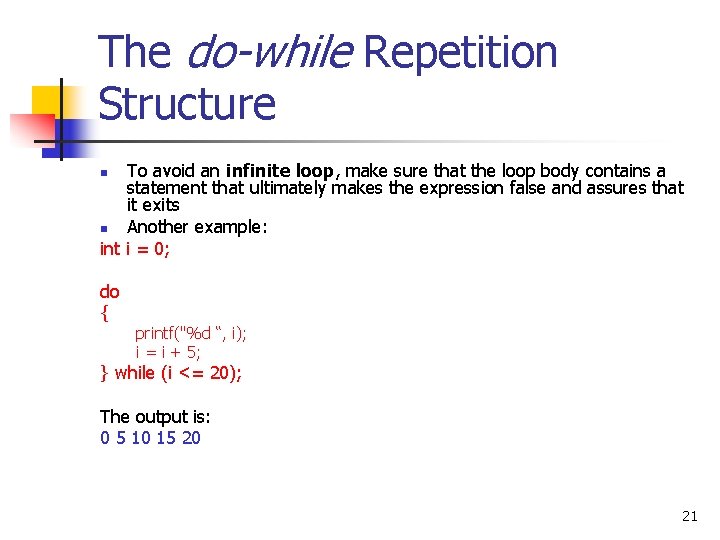
The do-while Repetition Structure To avoid an infinite loop, make sure that the loop body contains a statement that ultimately makes the expression false and assures that it exits n Another example: int i = 0; n do { printf("%d “, i); i = i + 5; } while (i <= 20); The output is: 0 5 10 15 20 21
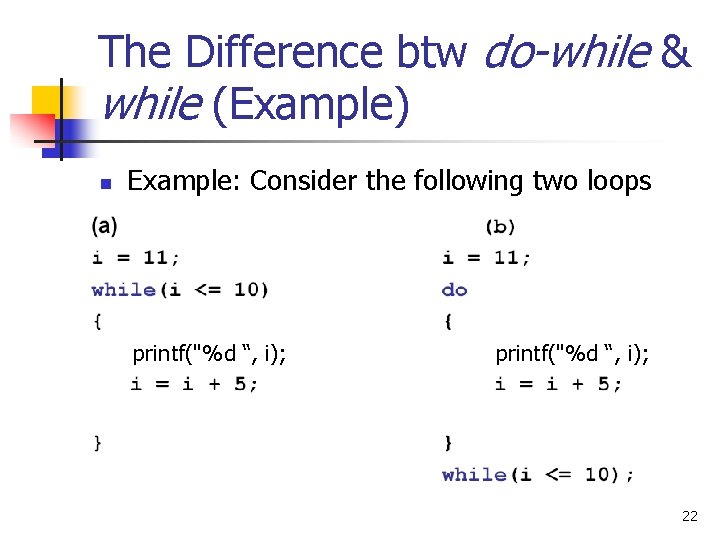
The Difference btw do-while & while (Example) n Example: Consider the following two loops printf("%d “, i); 22
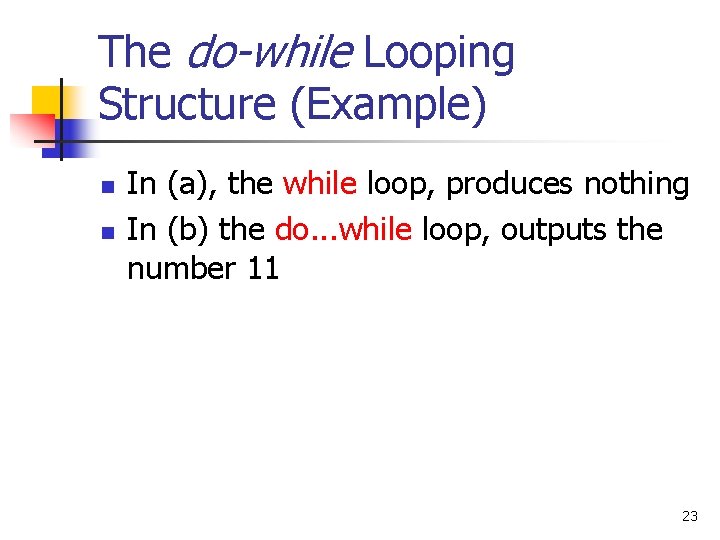
The do-while Looping Structure (Example) n n In (a), the while loop, produces nothing In (b) the do. . . while loop, outputs the number 11 23
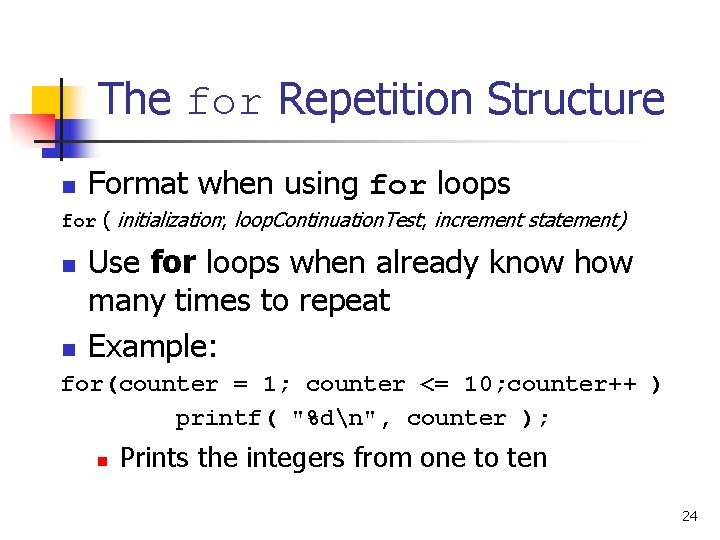
The for Repetition Structure n Format when using for loops for ( initialization; loop. Continuation. Test; increment statement) n n Use for loops when already know how many times to repeat Example: for(counter = 1; counter <= 10; counter++ ) printf( "%dn", counter ); n Prints the integers from one to ten 24
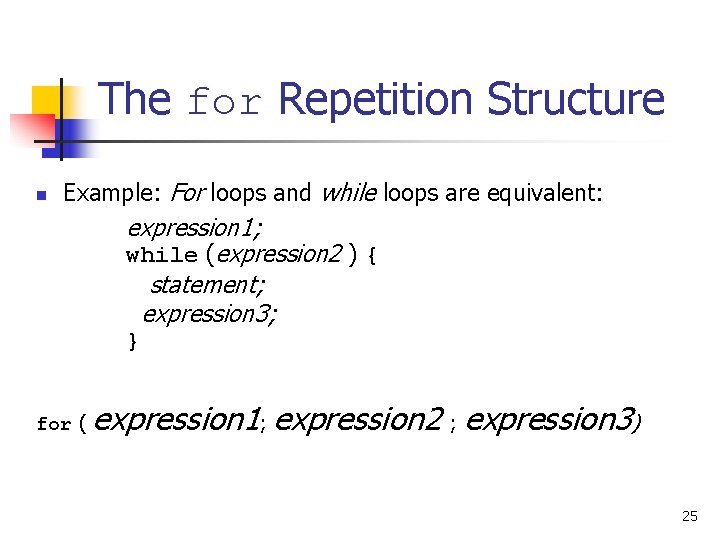
The for Repetition Structure n Example: For loops and while loops are equivalent: expression 1; while (expression 2 ) { statement; expression 3; } for ( expression 1; expression 2 ; expression 3) 25
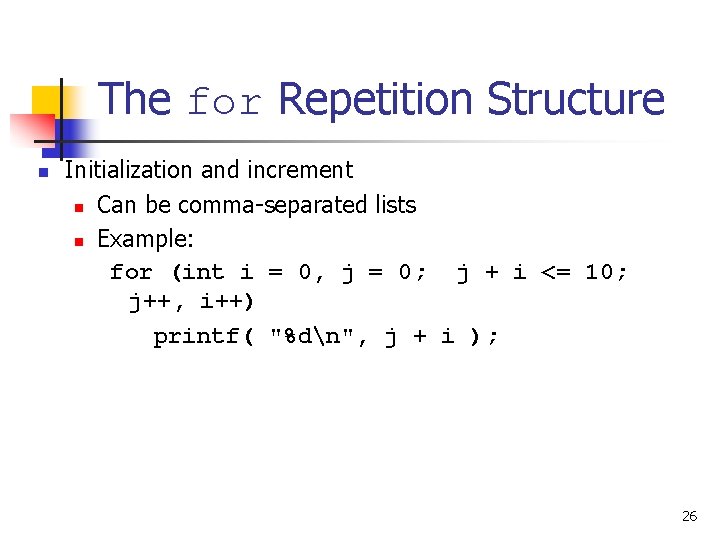
The for Repetition Structure n Initialization and increment n Can be comma-separated lists n Example: for (int i = 0, j = 0; j + i <= 10; j++, i++) printf( "%dn", j + i ); 26
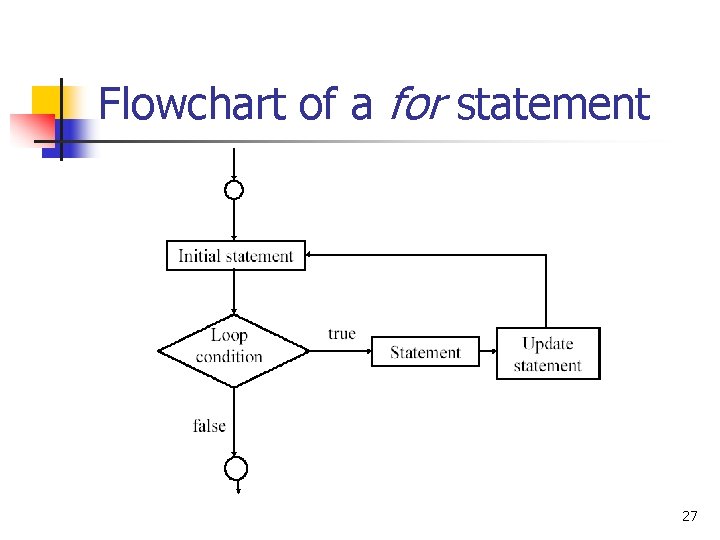
Flowchart of a for statement 27
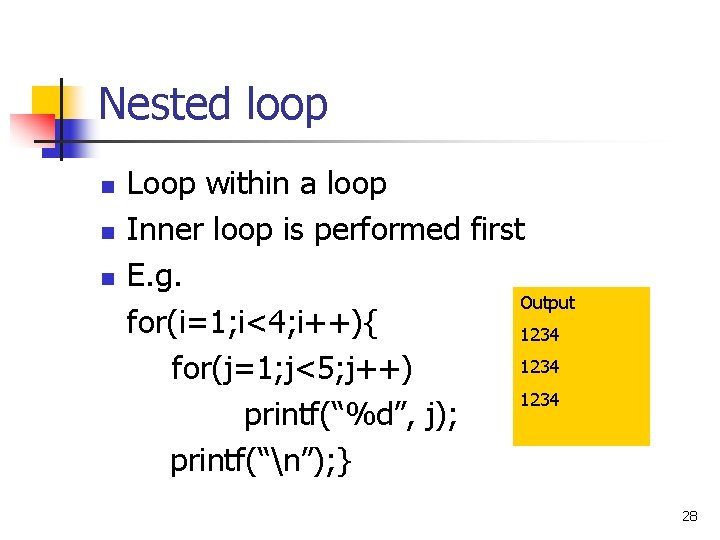
Nested loop n n n Loop within a loop Inner loop is performed first E. g. Output for(i=1; i<4; i++){ 1234 for(j=1; j<5; j++) 1234 printf(“%d”, j); printf(“n”); } 28
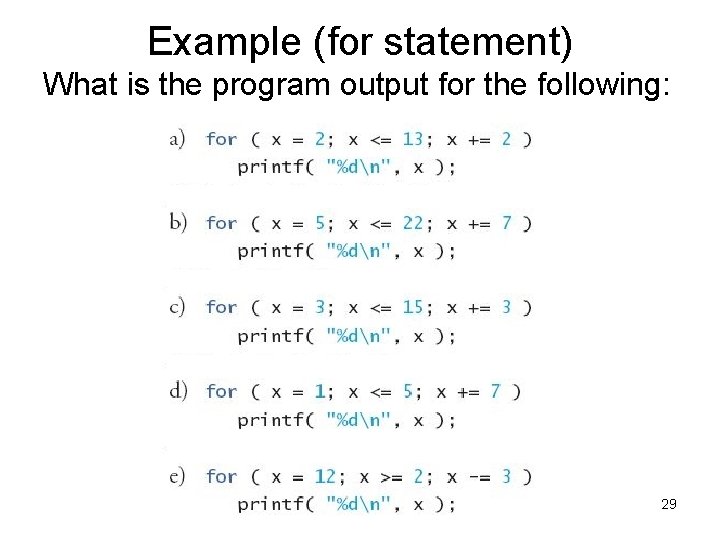
Example (for statement) What is the program output for the following: Uni. MAP ENT 189 Sem I-10/11 29
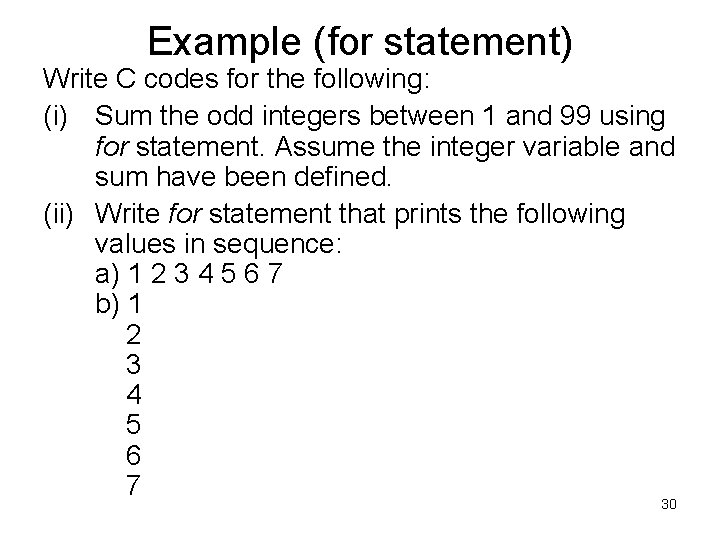
Example (for statement) Write C codes for the following: (i) Sum the odd integers between 1 and 99 using for statement. Assume the integer variable and sum have been defined. (ii) Write for statement that prints the following values in sequence: a) 1 2 3 4 5 6 7 b) 1 2 3 4 5 6 7 30
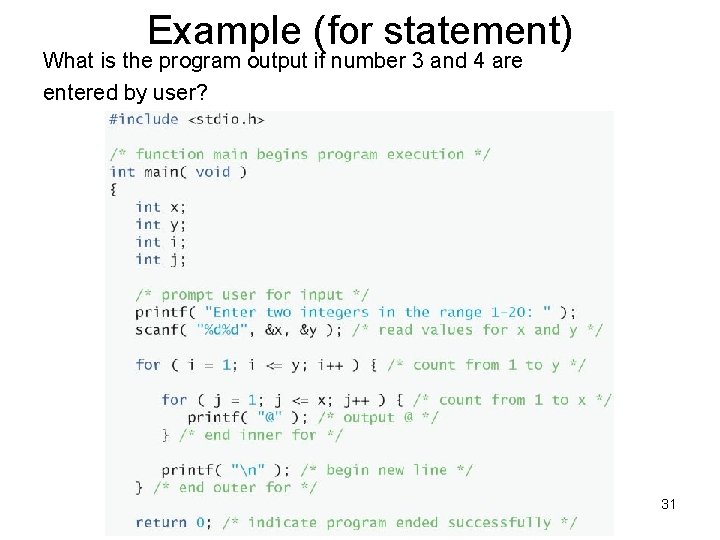
Example (for statement) What is the program output if number 3 and 4 are entered by user? Uni. MAP ENT 189 Sem I-10/11 31
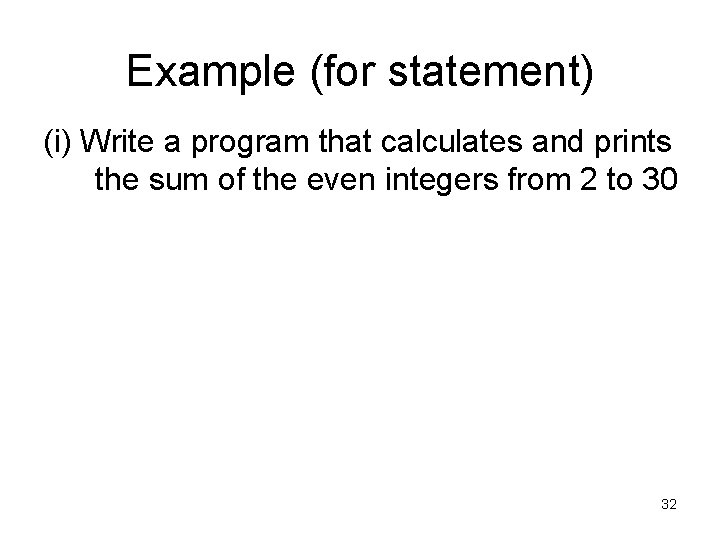
Example (for statement) (i) Write a program that calculates and prints the sum of the even integers from 2 to 30 32
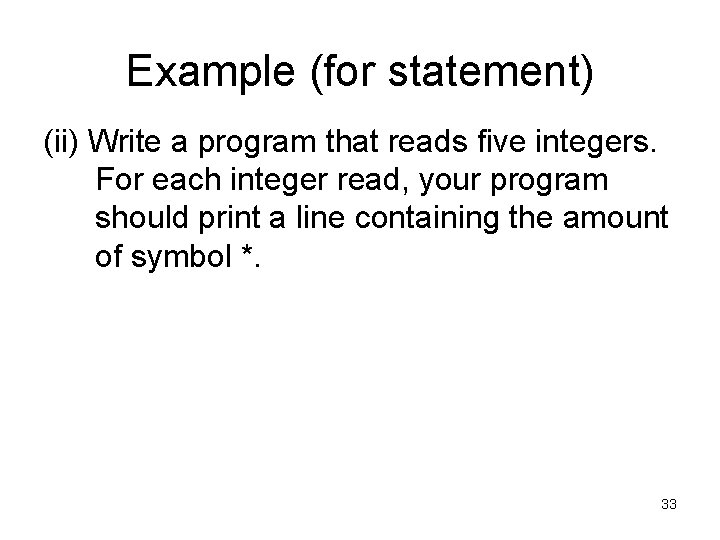
Example (for statement) (ii) Write a program that reads five integers. For each integer read, your program should print a line containing the amount of symbol *. 33
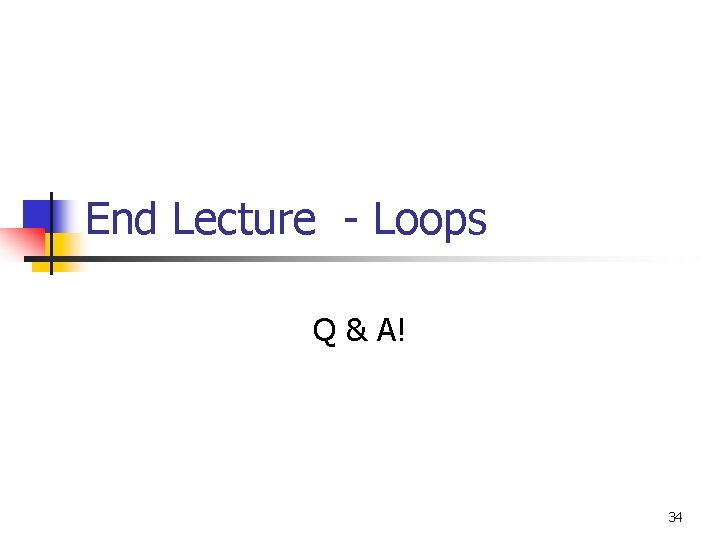
End Lecture - Loops Q & A! 34