Iteration Conditional Loops Counted Loops Charting the Flow
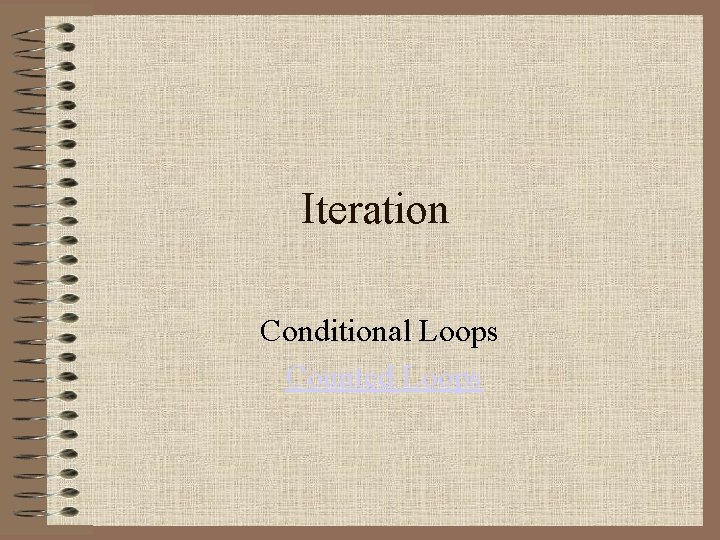
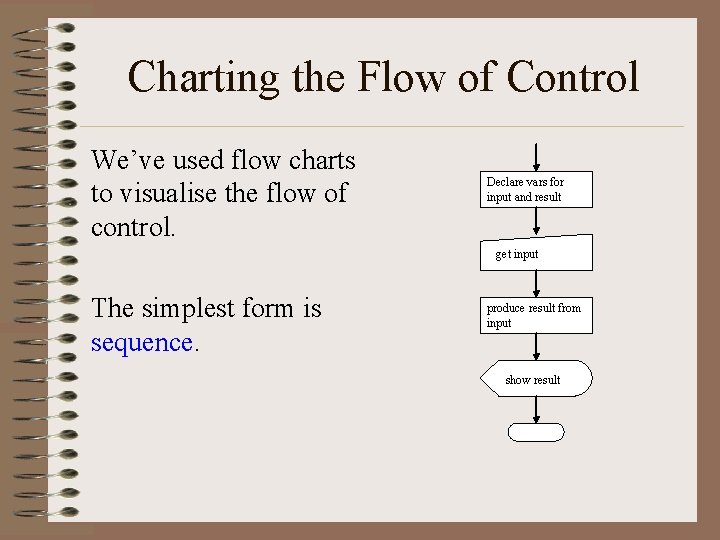
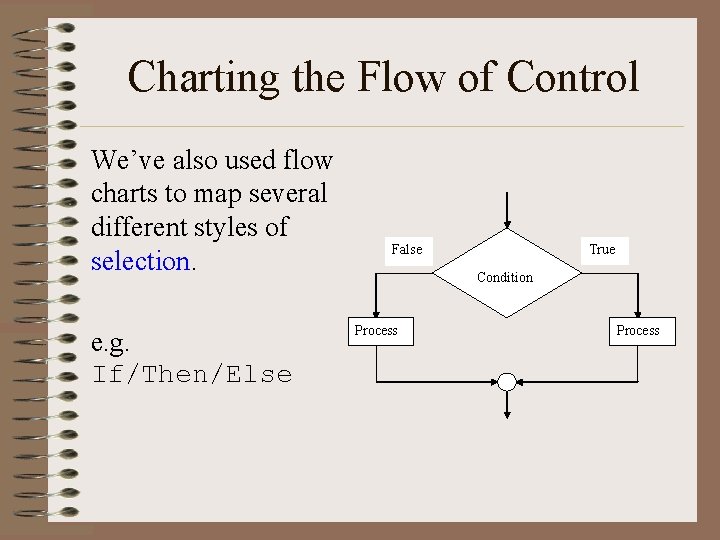
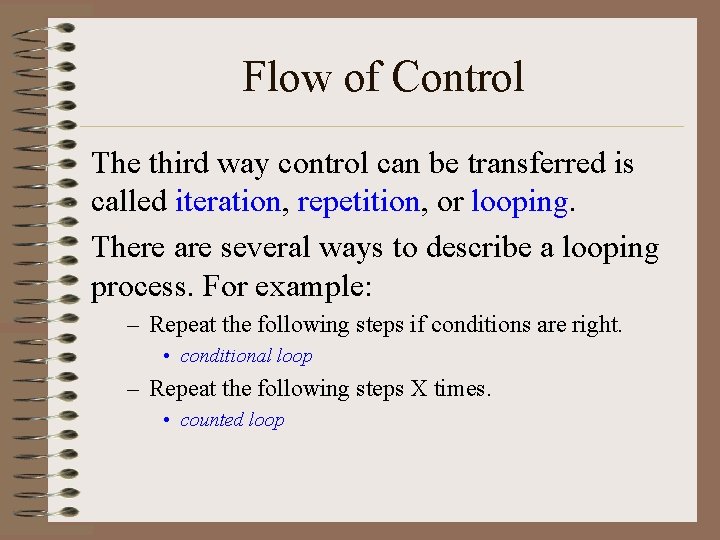
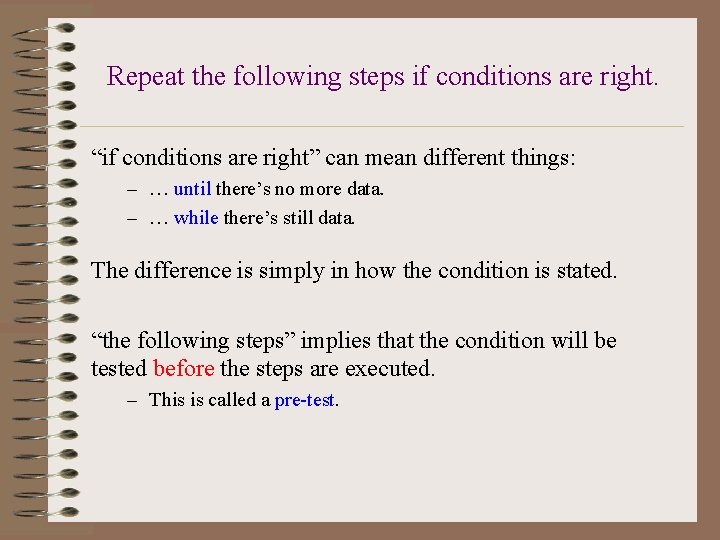
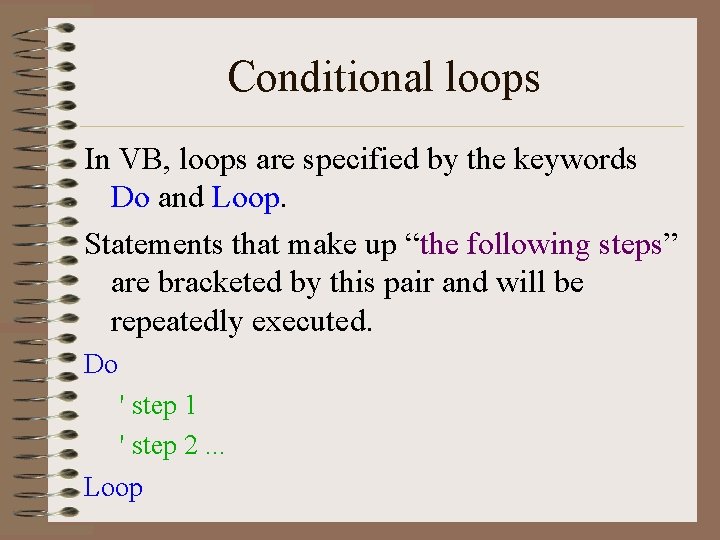
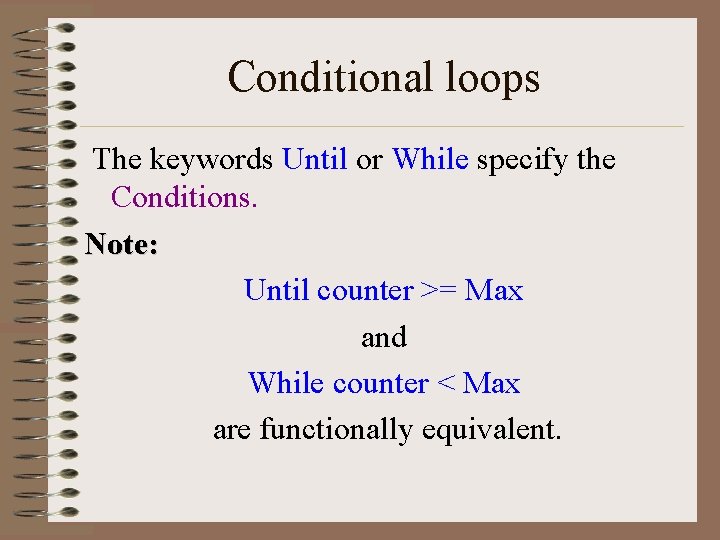
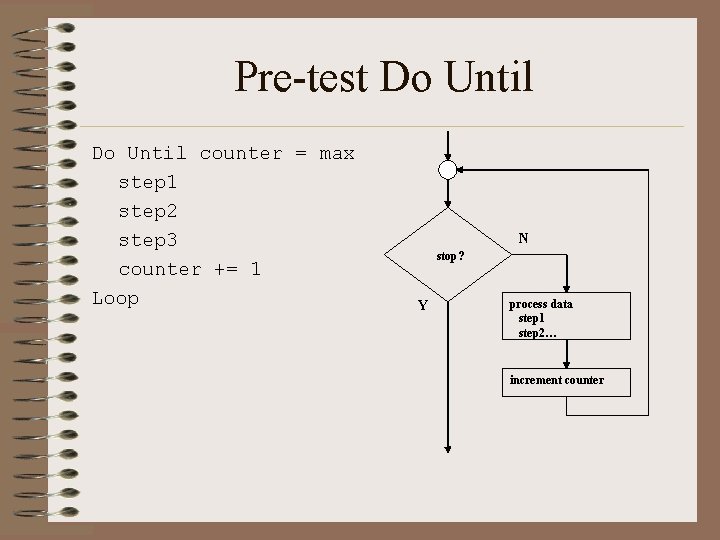
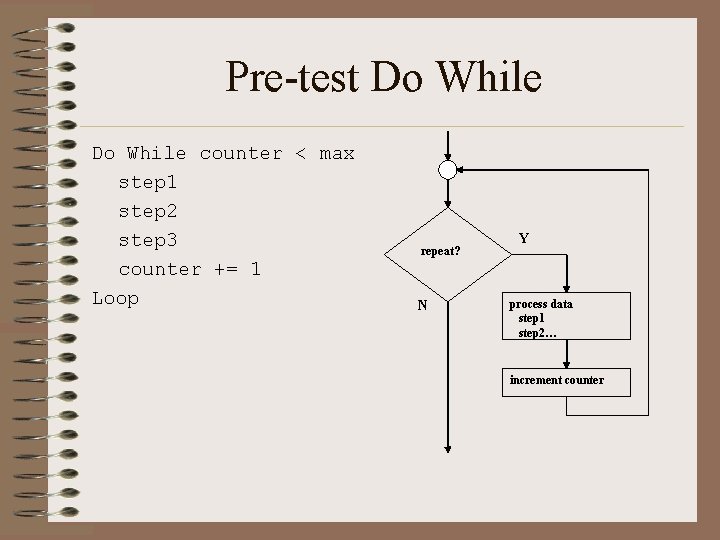
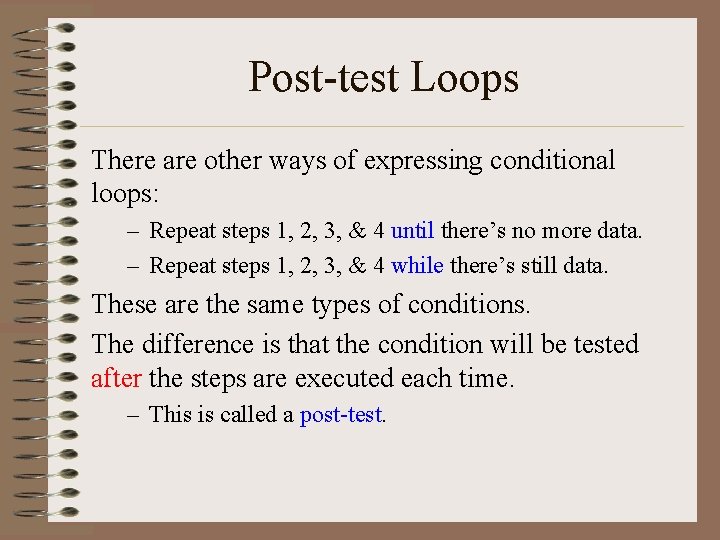
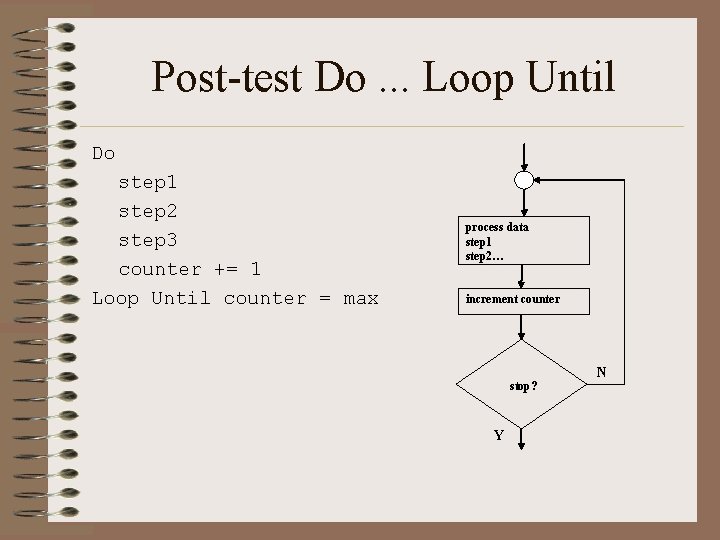
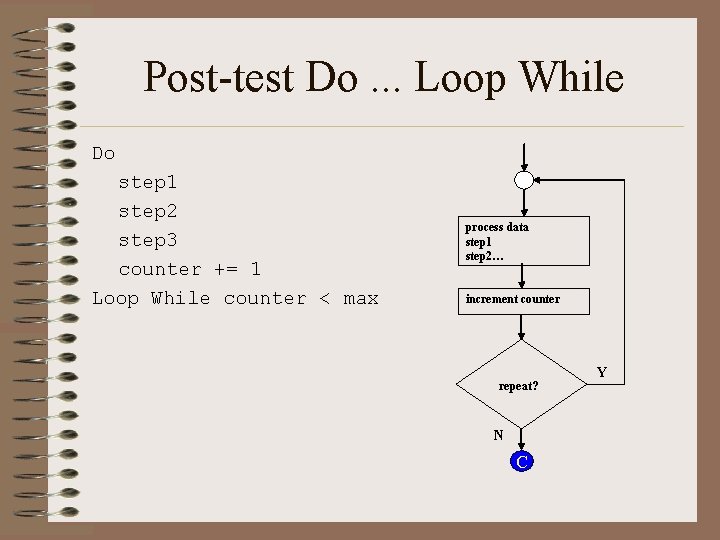
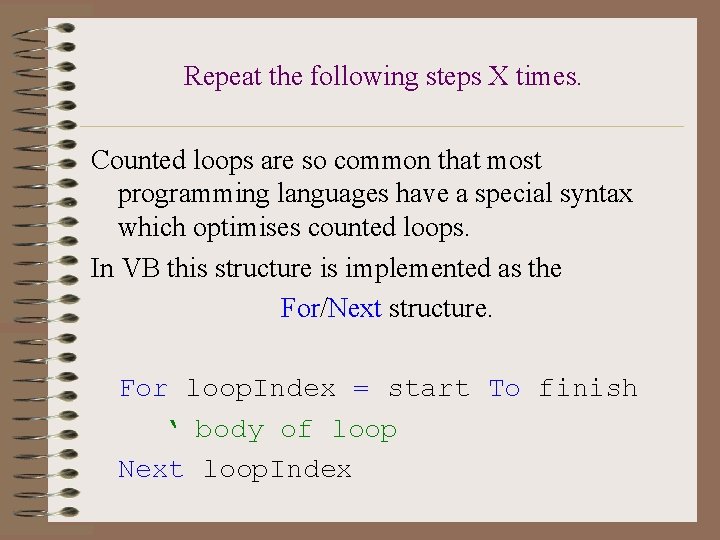
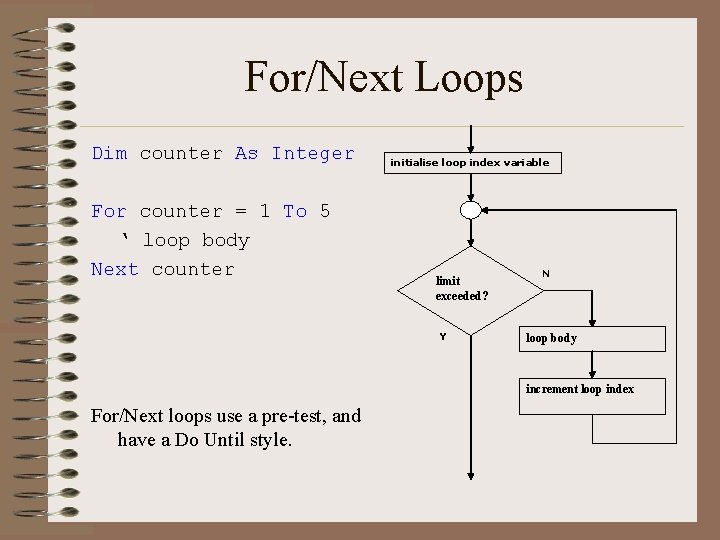
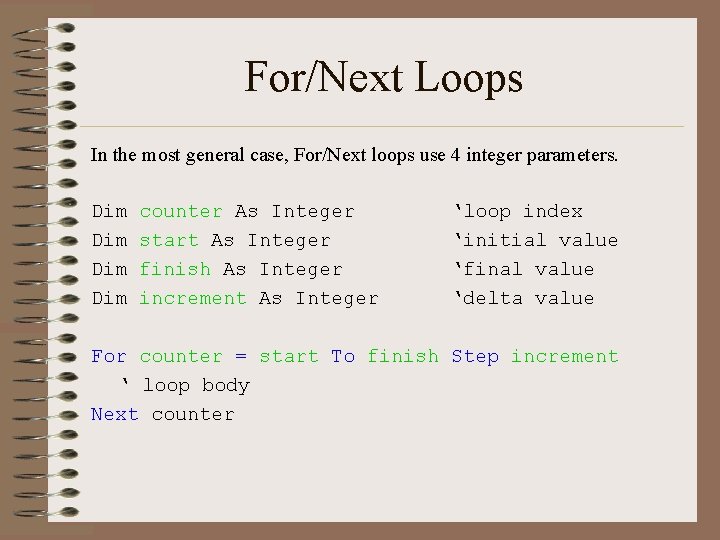
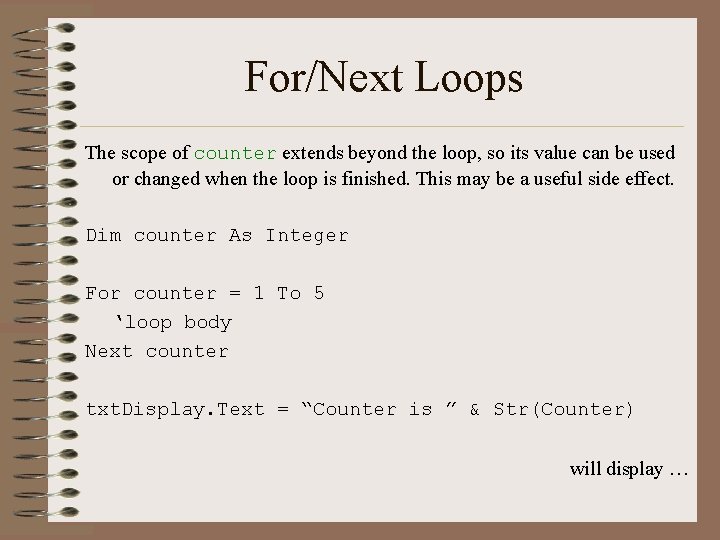
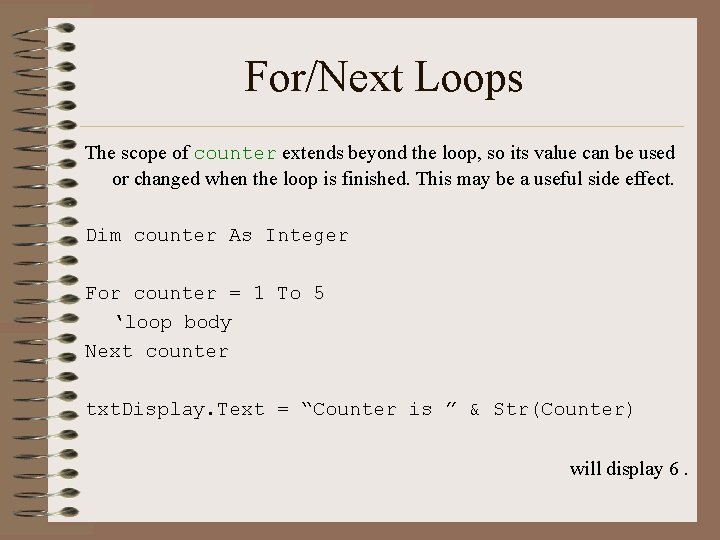
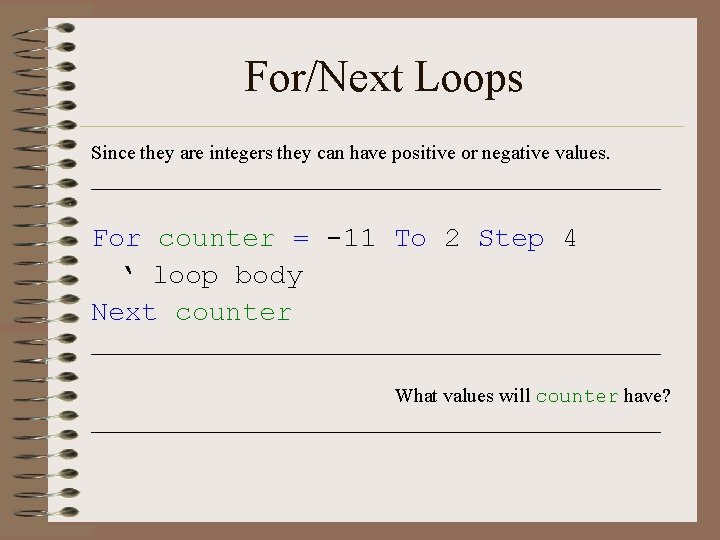
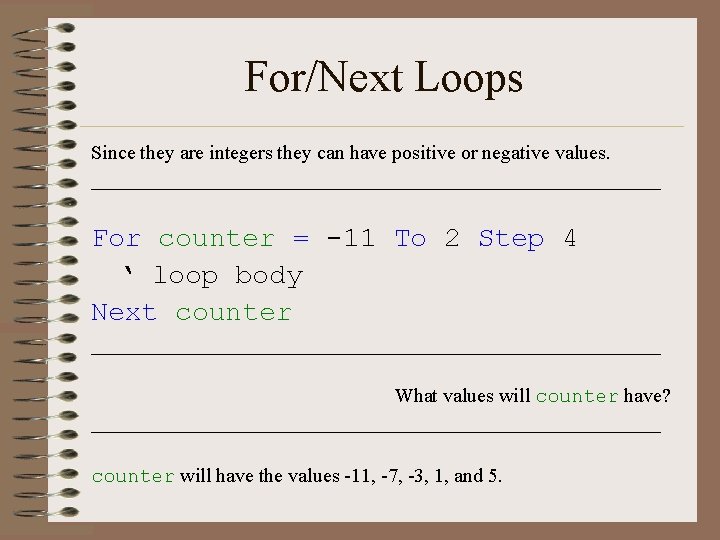
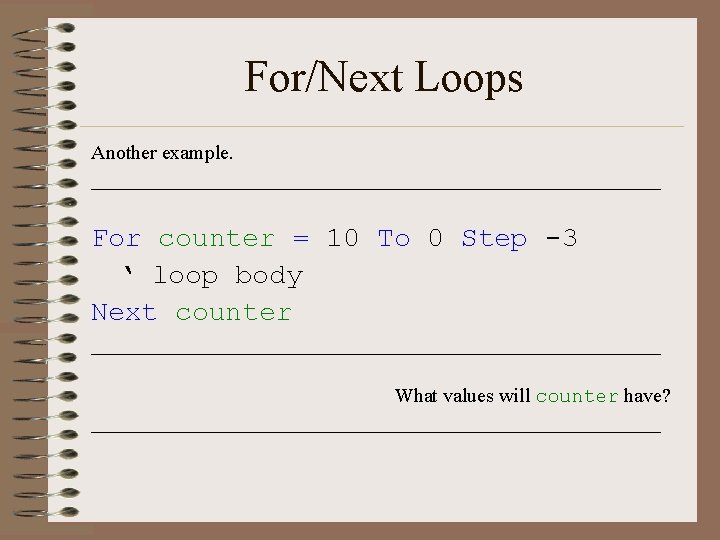
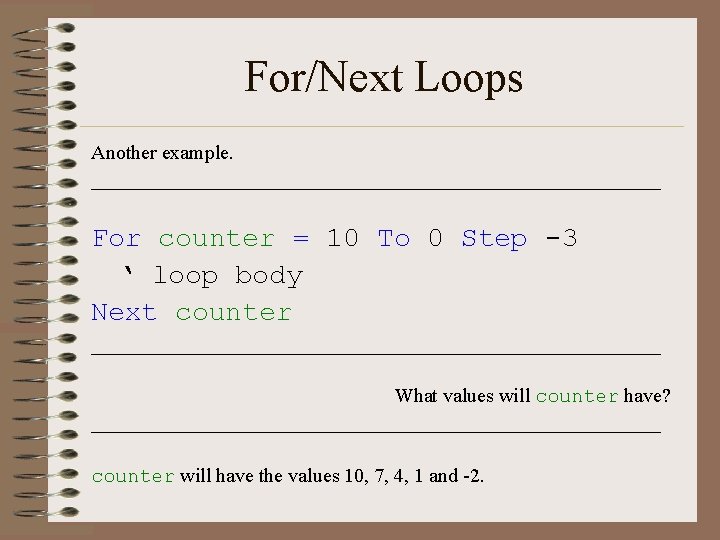
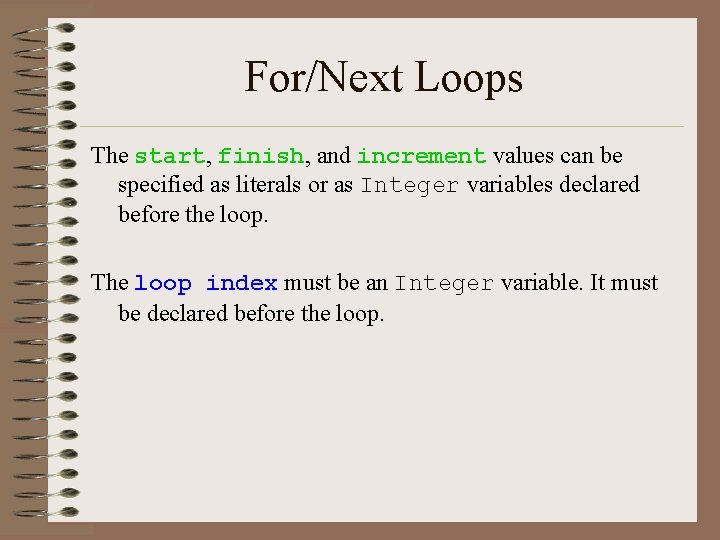
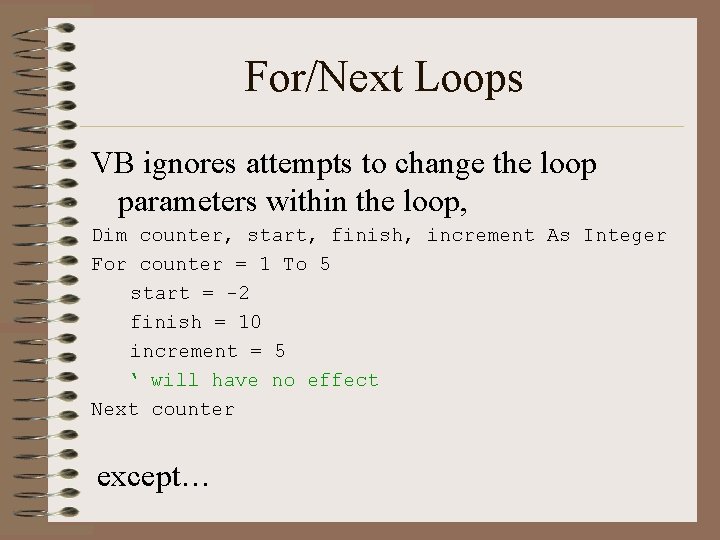
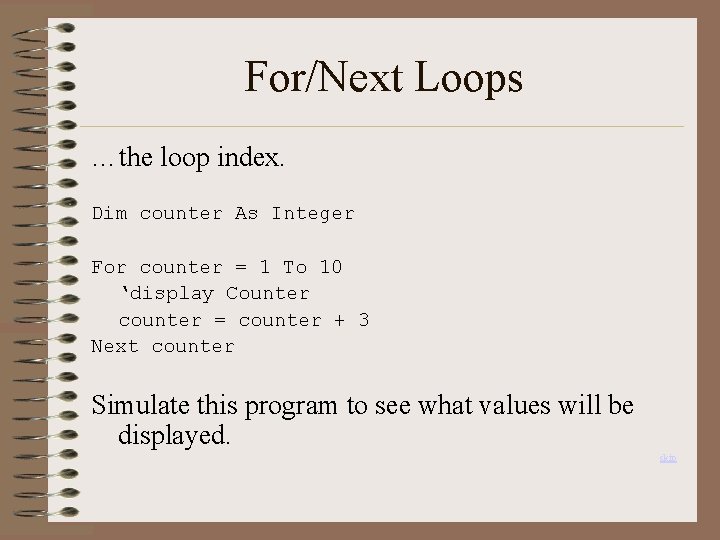
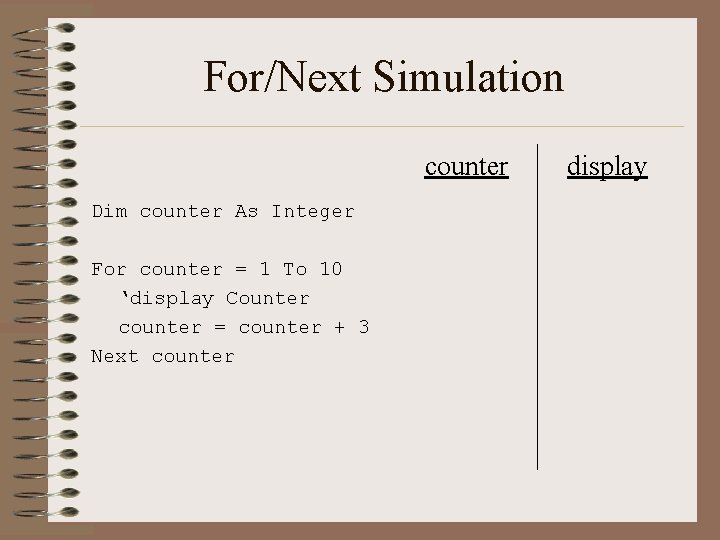
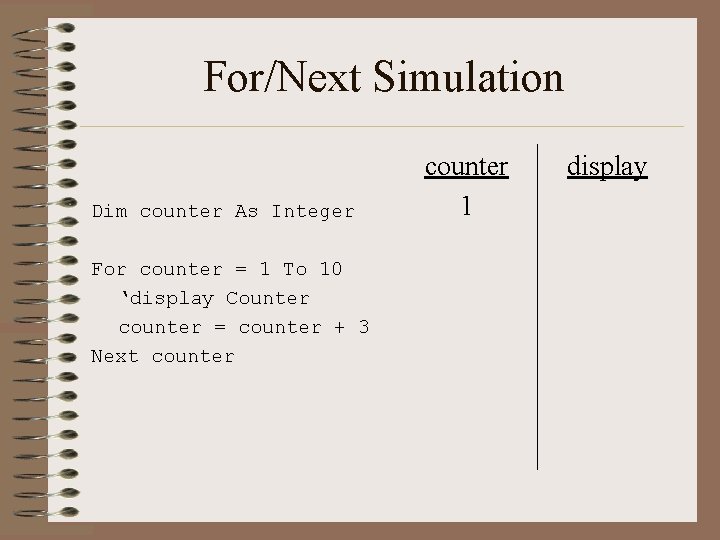
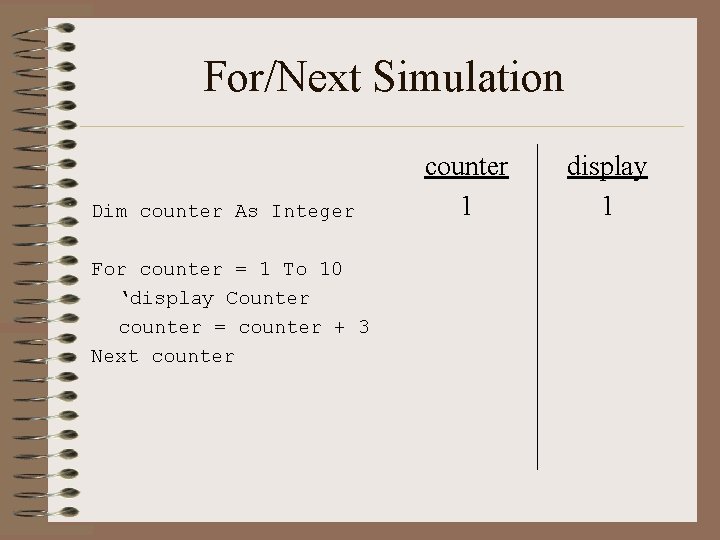
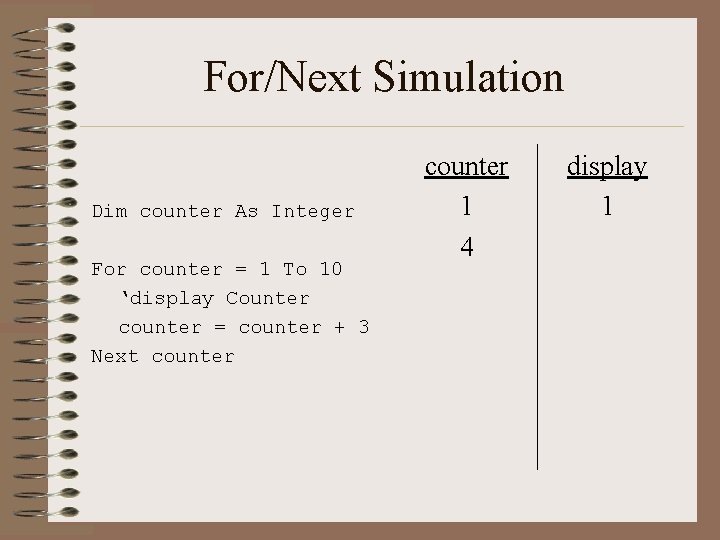
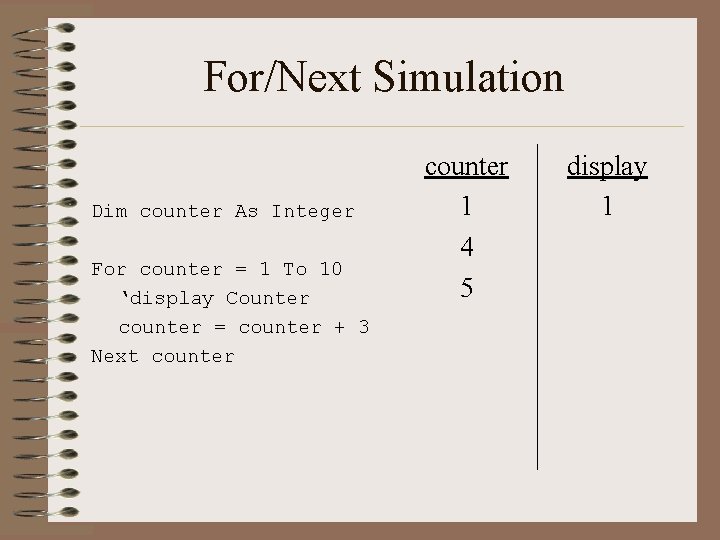
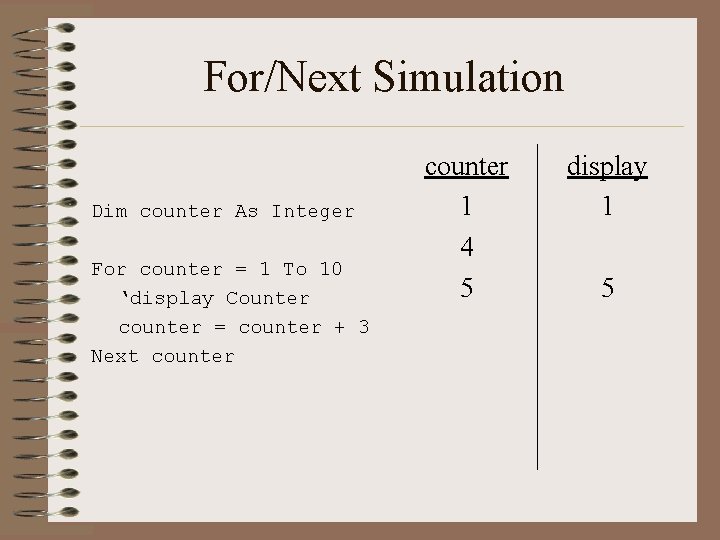
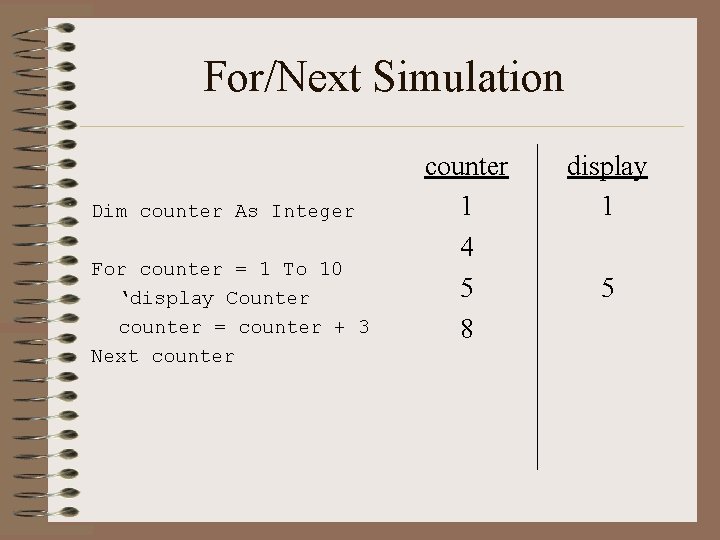
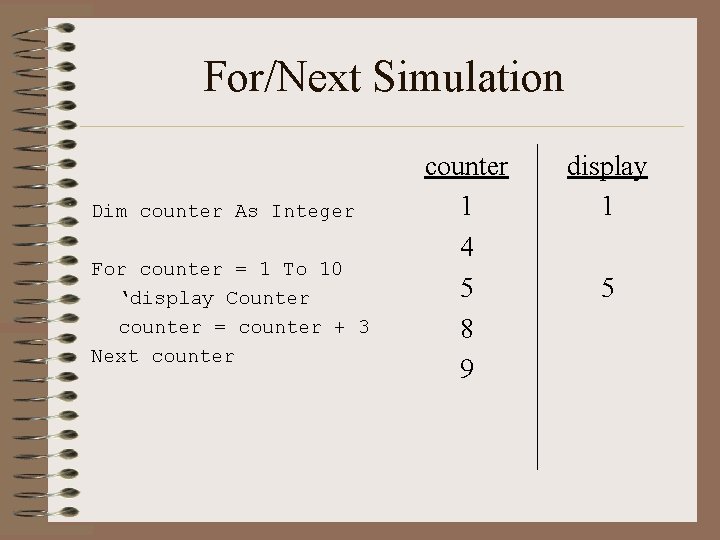
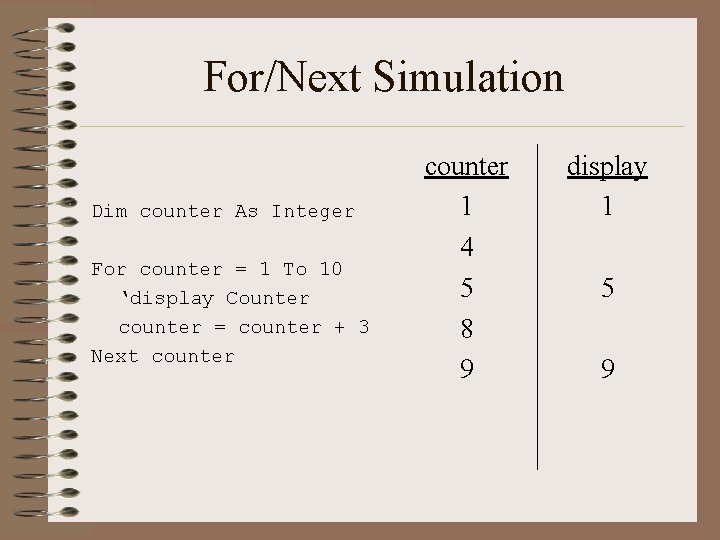
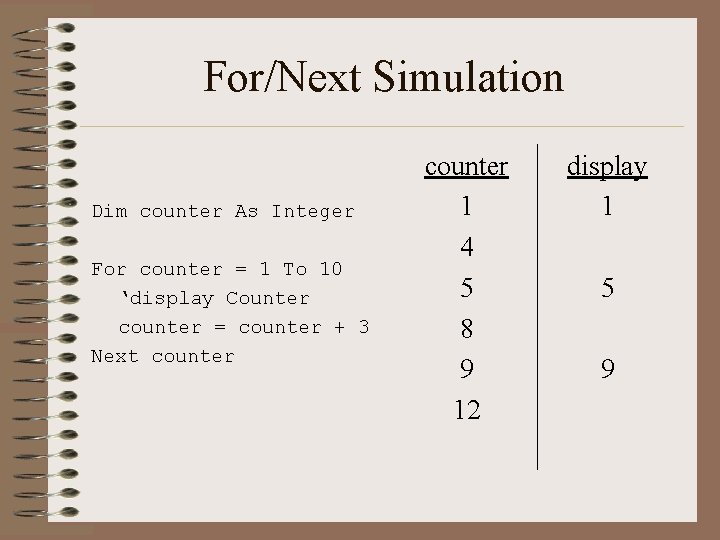
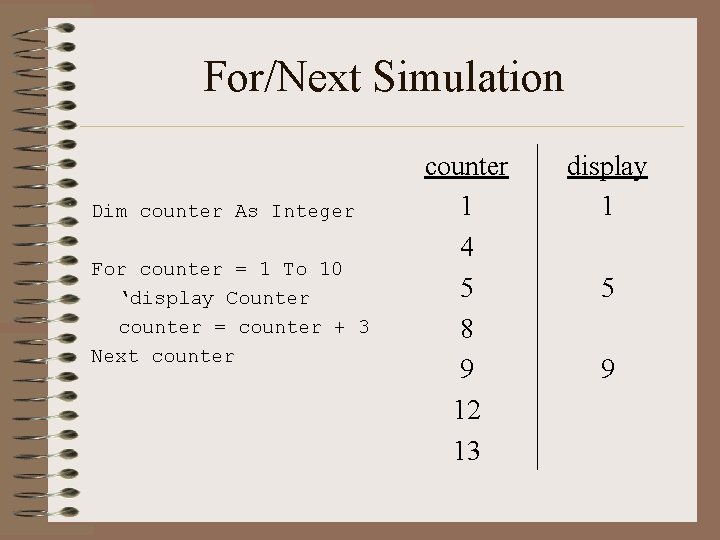
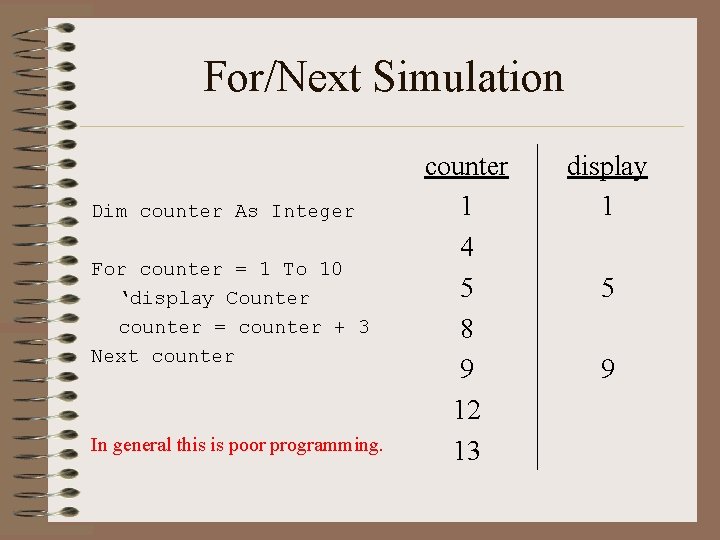
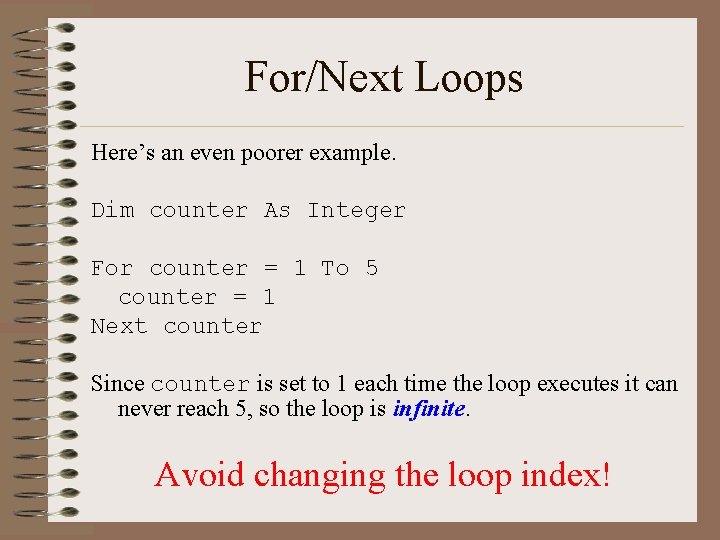
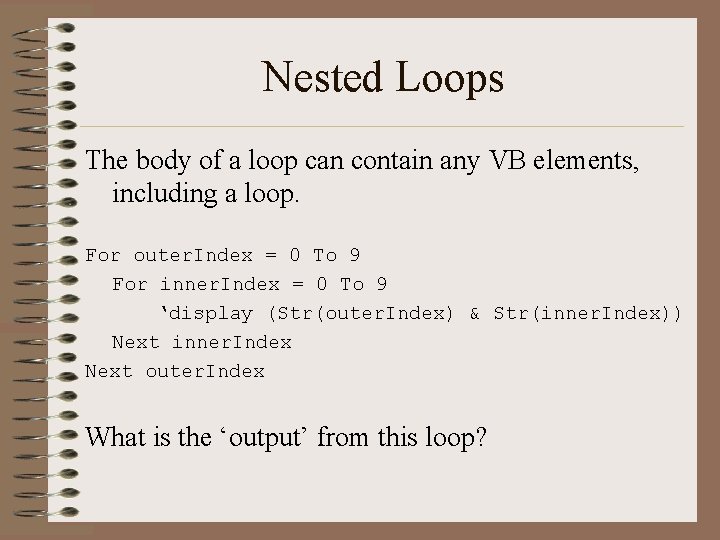
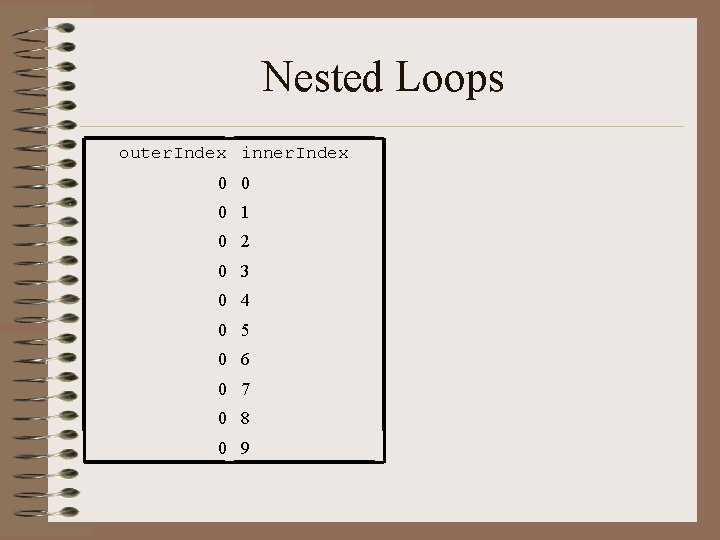
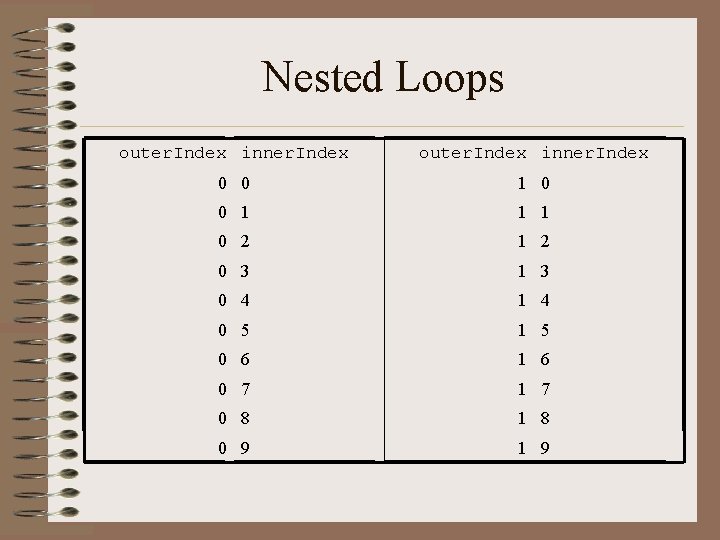
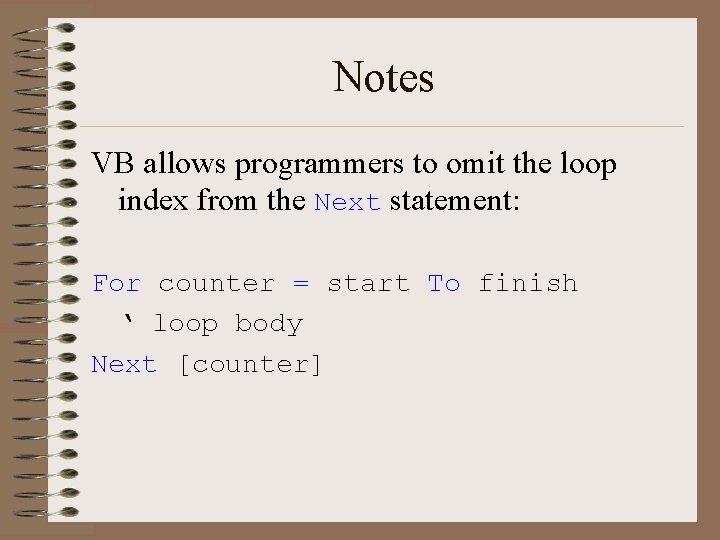
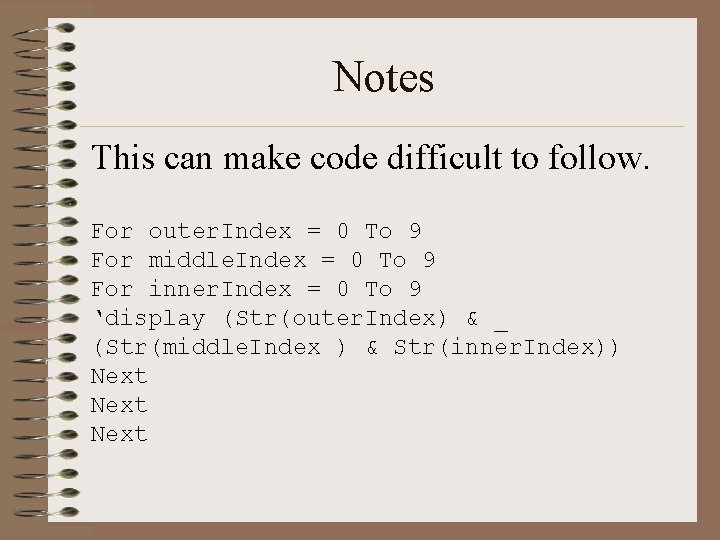
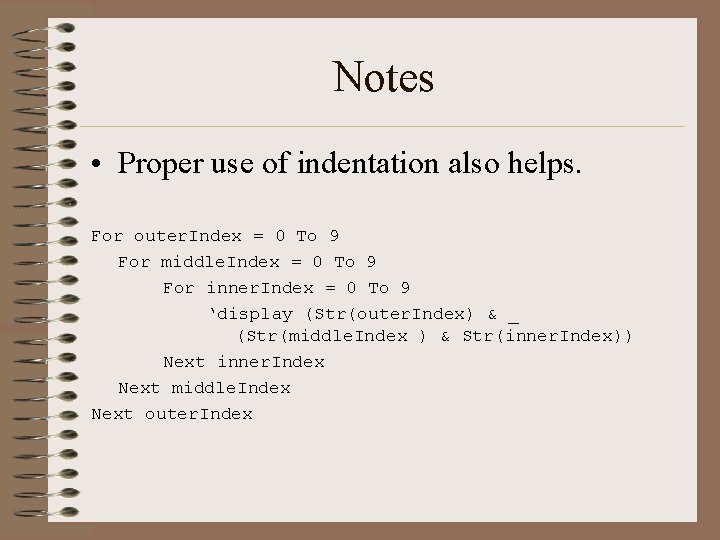
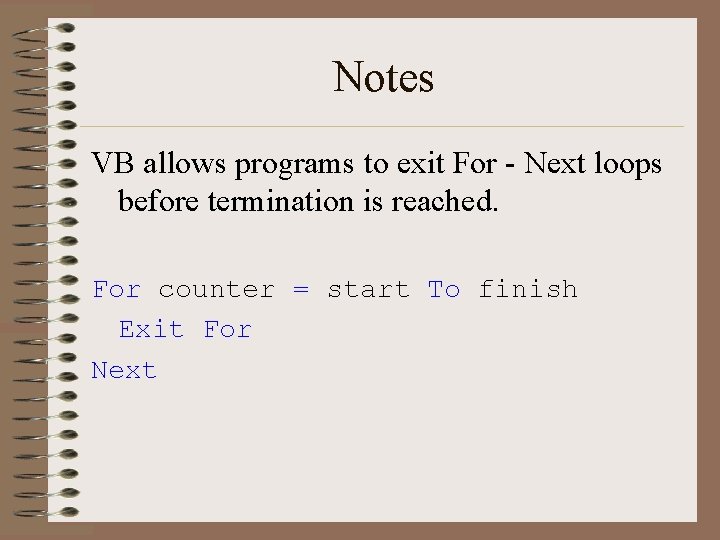
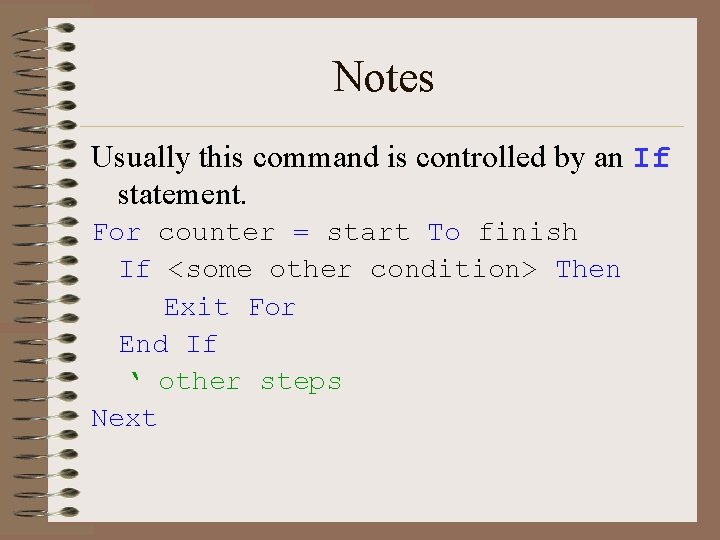
- Slides: 45
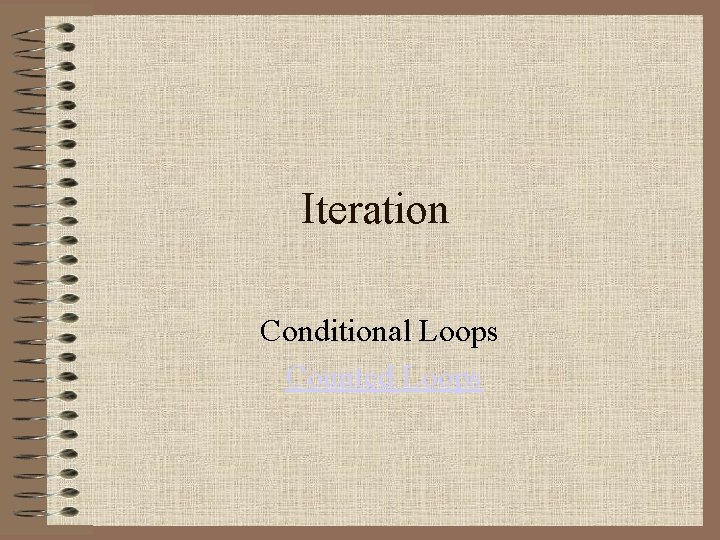
Iteration Conditional Loops Counted Loops
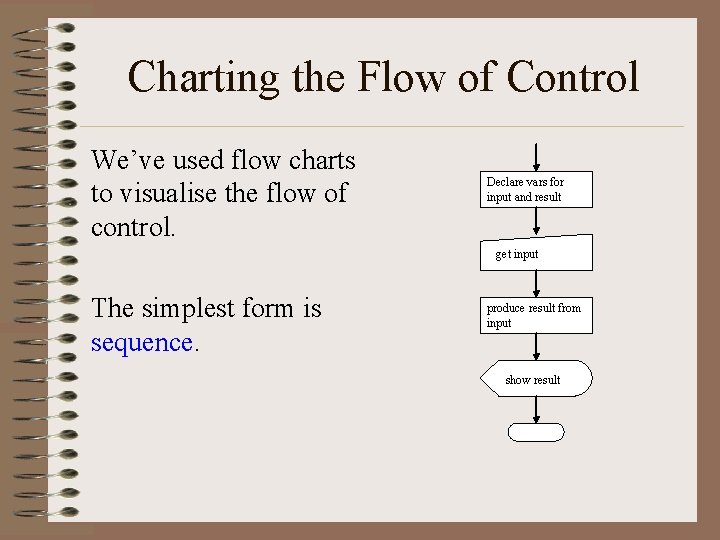
Charting the Flow of Control We’ve used flow charts to visualise the flow of control. Declare vars for input and result get input The simplest form is sequence. produce result from input show result
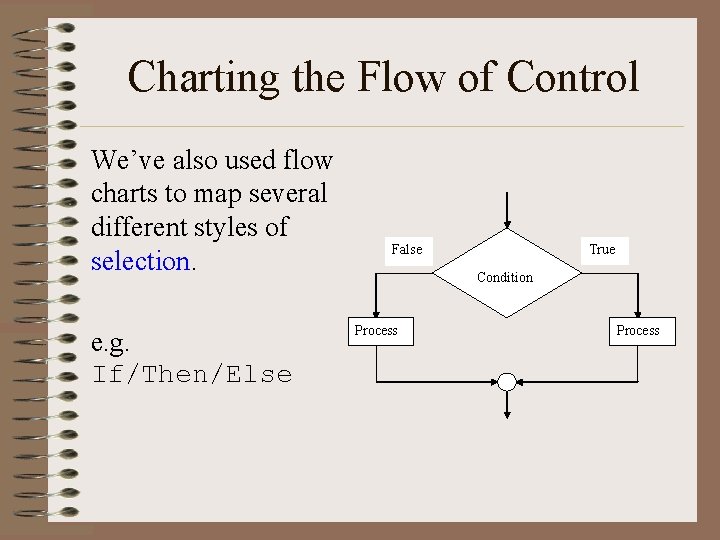
Charting the Flow of Control We’ve also used flow charts to map several different styles of selection. e. g. If/Then/Else False True Condition Process
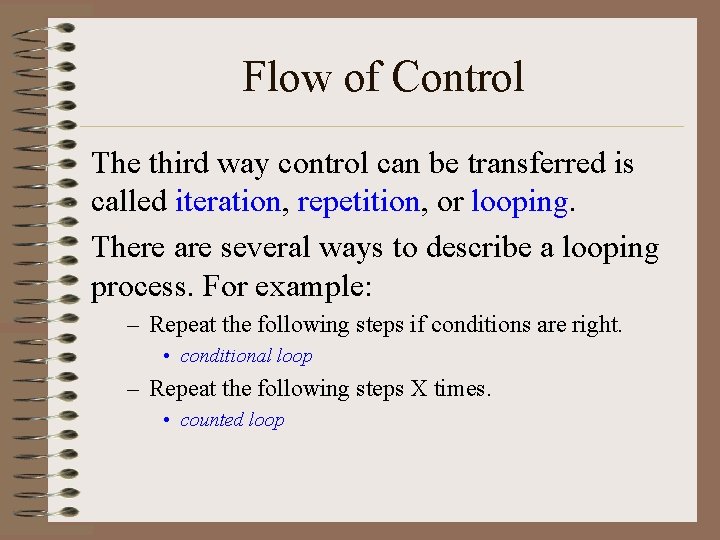
Flow of Control The third way control can be transferred is called iteration, repetition, or looping. There are several ways to describe a looping process. For example: – Repeat the following steps if conditions are right. • conditional loop – Repeat the following steps X times. • counted loop
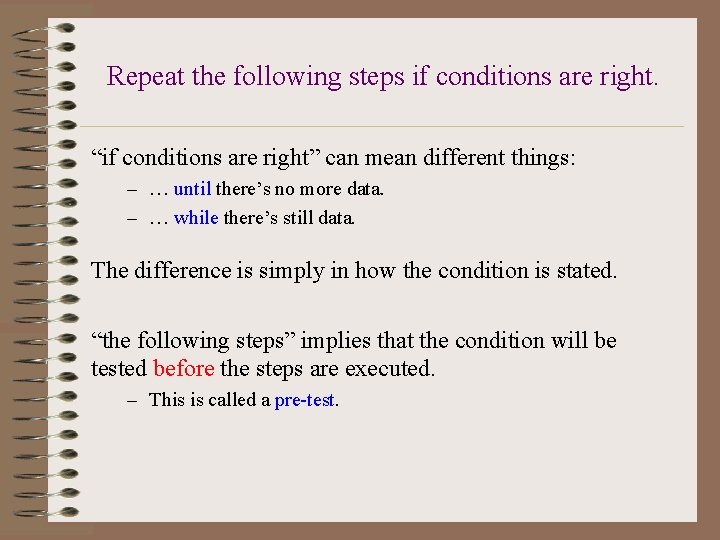
Repeat the following steps if conditions are right. “if conditions are right” can mean different things: – … until there’s no more data. – … while there’s still data. The difference is simply in how the condition is stated. “the following steps” implies that the condition will be tested before the steps are executed. – This is called a pre-test.
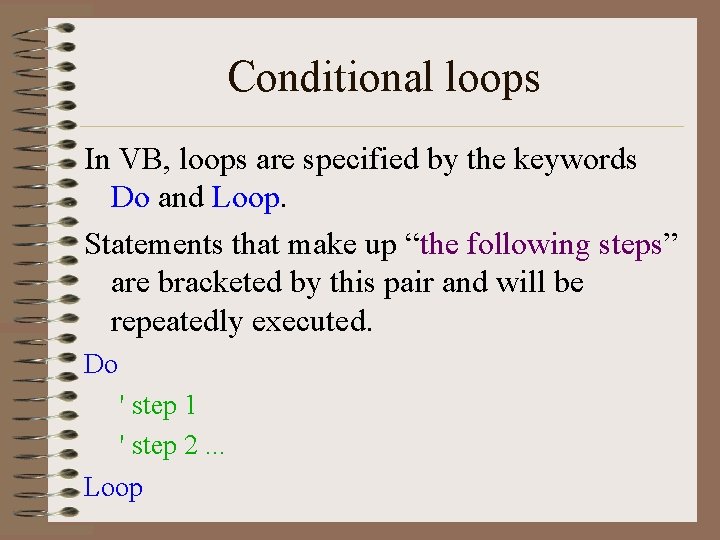
Conditional loops In VB, loops are specified by the keywords Do and Loop. Statements that make up “the following steps” are bracketed by this pair and will be repeatedly executed. Do ' step 1 ' step 2. . . Loop
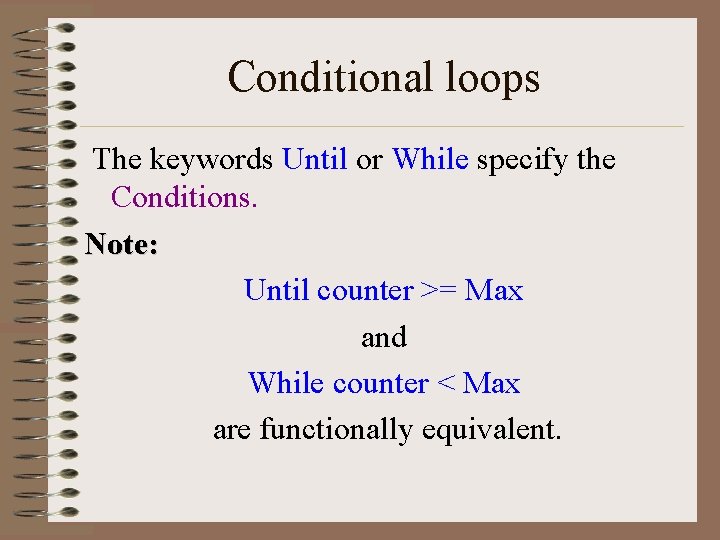
Conditional loops The keywords Until or While specify the Conditions. Note: Until counter >= Max and While counter < Max are functionally equivalent.
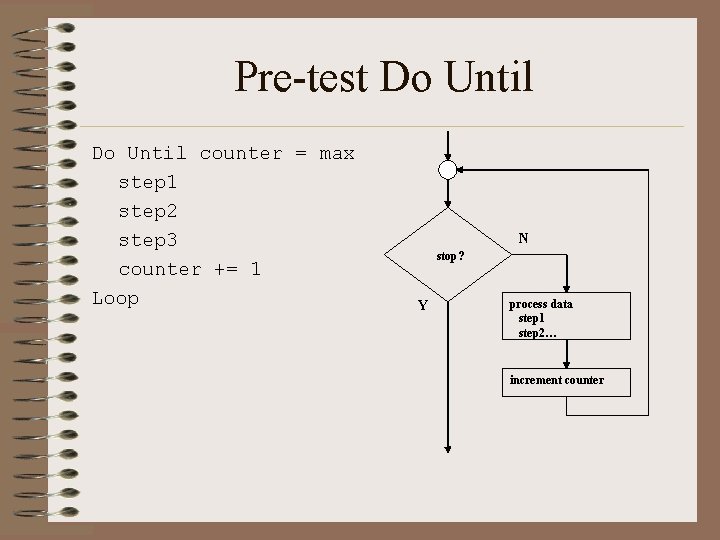
Pre-test Do Until counter = max step 1 step 2 step 3 counter += 1 Loop N stop? Y process data step 1 step 2… increment counter
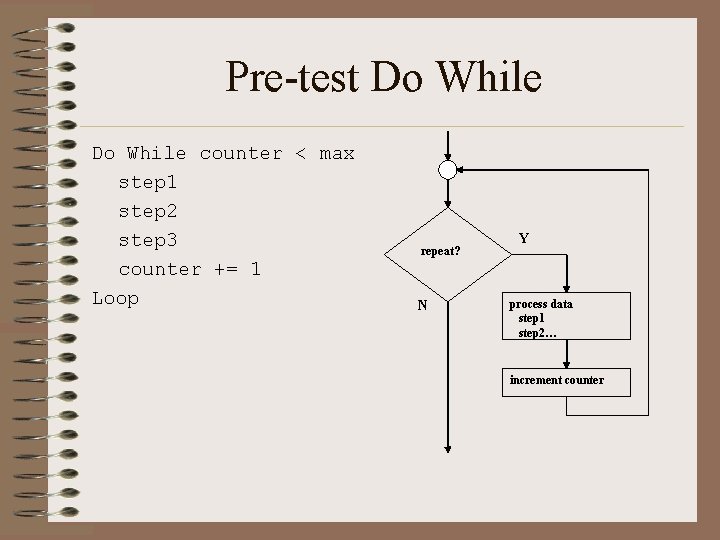
Pre-test Do While counter < max step 1 step 2 step 3 counter += 1 Loop repeat? N Y process data step 1 step 2… increment counter
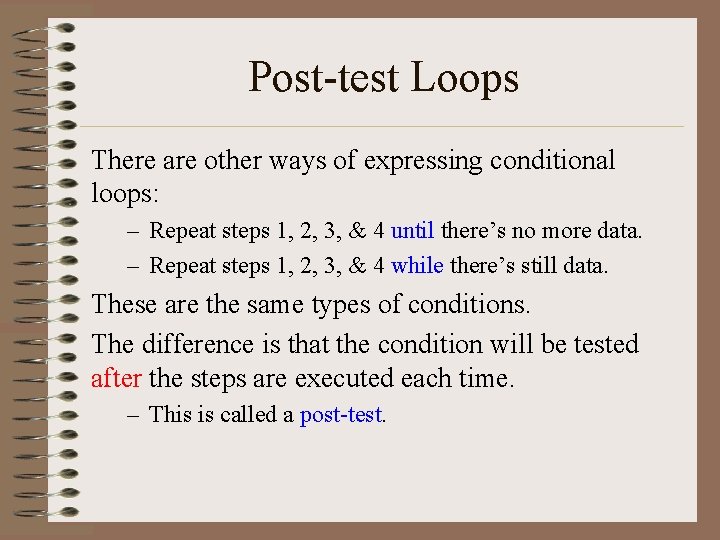
Post-test Loops There are other ways of expressing conditional loops: – Repeat steps 1, 2, 3, & 4 until there’s no more data. – Repeat steps 1, 2, 3, & 4 while there’s still data. These are the same types of conditions. The difference is that the condition will be tested after the steps are executed each time. – This is called a post-test.
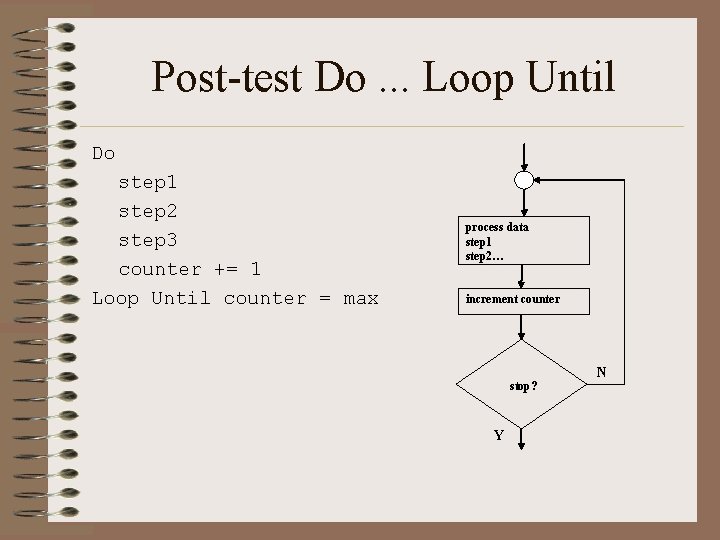
Post-test Do. . . Loop Until Do step 1 step 2 step 3 counter += 1 Loop Until counter = max process data step 1 step 2… increment counter stop? Y N
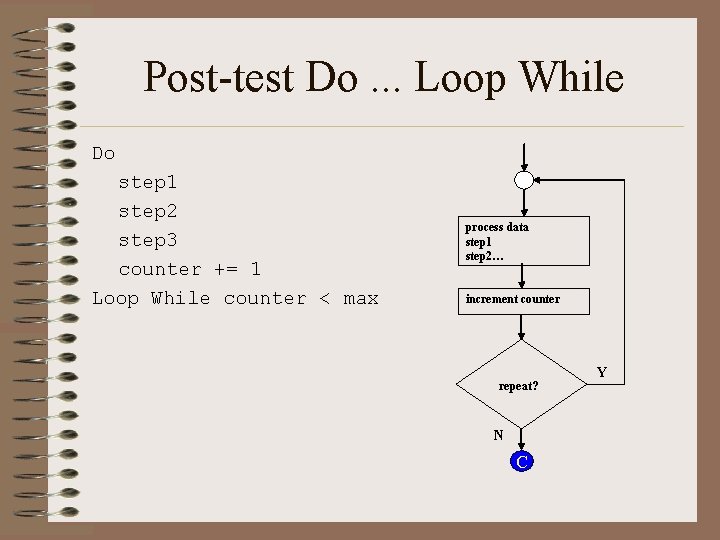
Post-test Do. . . Loop While Do step 1 step 2 step 3 counter += 1 Loop While counter < max process data step 1 step 2… increment counter repeat? N C Y
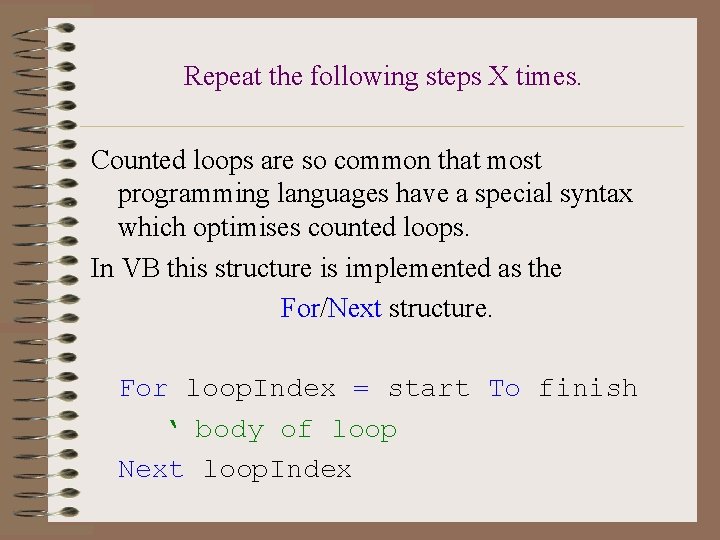
Repeat the following steps X times. Counted loops are so common that most programming languages have a special syntax which optimises counted loops. In VB this structure is implemented as the For/Next structure. For loop. Index = start To finish ‘ body of loop Next loop. Index
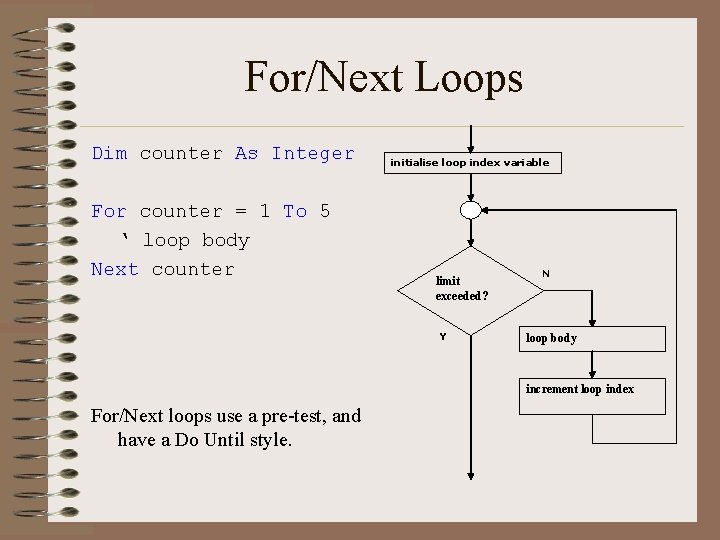
For/Next Loops Dim counter As Integer For counter = 1 To 5 ‘ loop body Next counter initialise loop index variable limit exceeded? Y N loop body increment loop index For/Next loops use a pre-test, and have a Do Until style.
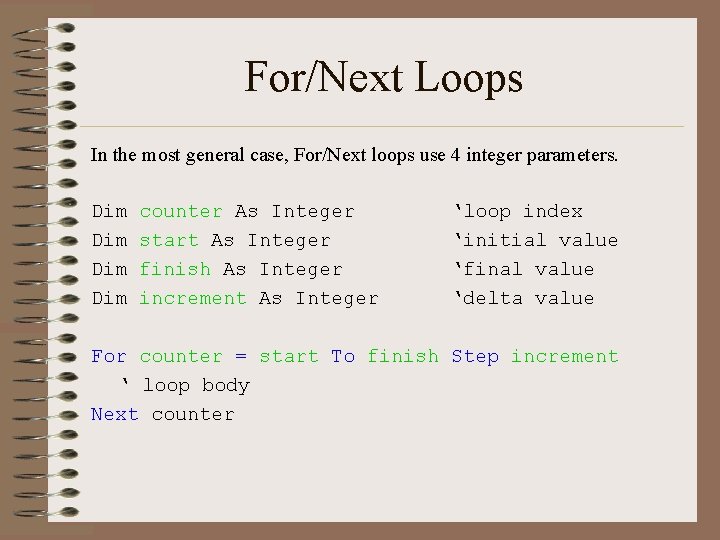
For/Next Loops In the most general case, For/Next loops use 4 integer parameters. Dim Dim counter As Integer start As Integer finish As Integer increment As Integer ‘loop index ‘initial value ‘final value ‘delta value For counter = start To finish Step increment ‘ loop body Next counter
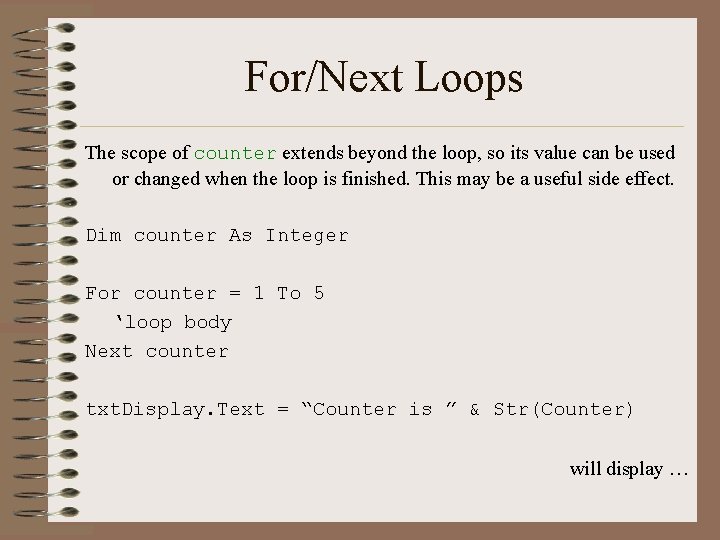
For/Next Loops The scope of counter extends beyond the loop, so its value can be used or changed when the loop is finished. This may be a useful side effect. Dim counter As Integer For counter = 1 To 5 ‘loop body Next counter txt. Display. Text = “Counter is ” & Str(Counter) will display …
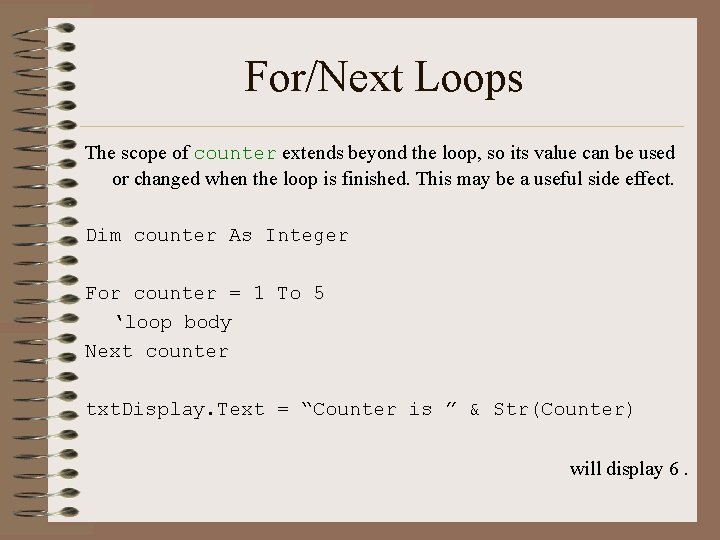
For/Next Loops The scope of counter extends beyond the loop, so its value can be used or changed when the loop is finished. This may be a useful side effect. Dim counter As Integer For counter = 1 To 5 ‘loop body Next counter txt. Display. Text = “Counter is ” & Str(Counter) will display 6.
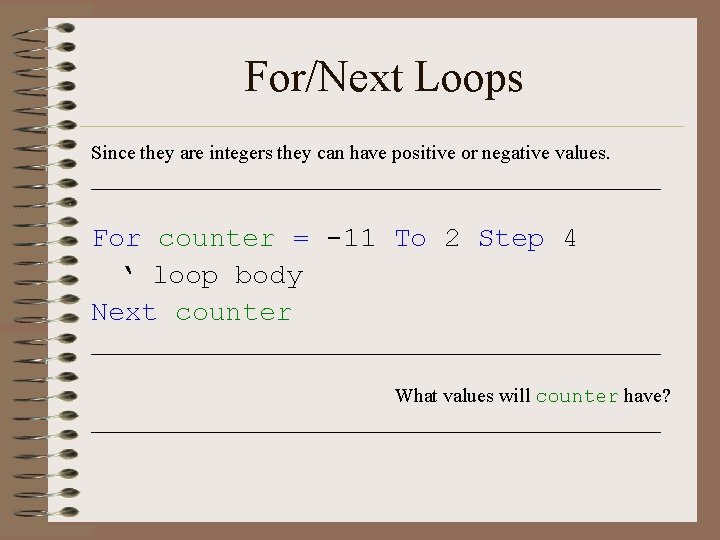
For/Next Loops Since they are integers they can have positive or negative values. _____________________________ For counter = -11 To 2 Step 4 ‘ loop body Next counter _____________________________ What values will counter have? _____________________________
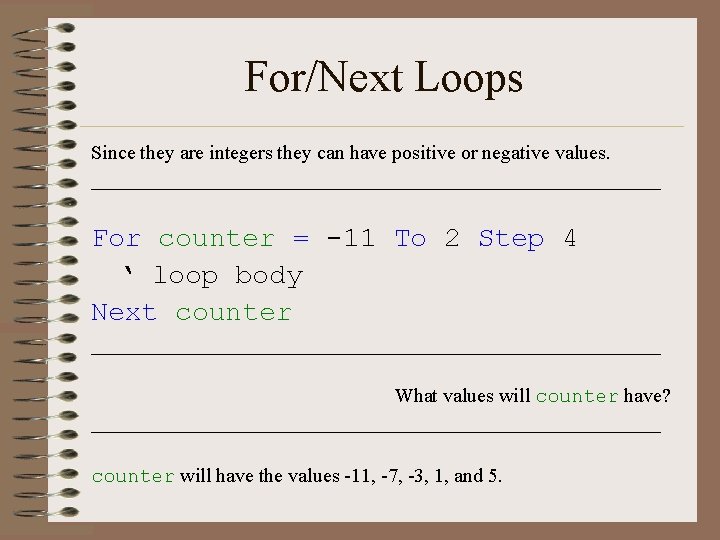
For/Next Loops Since they are integers they can have positive or negative values. _____________________________ For counter = -11 To 2 Step 4 ‘ loop body Next counter _____________________________ What values will counter have? _____________________________ counter will have the values -11, -7, -3, 1, and 5.
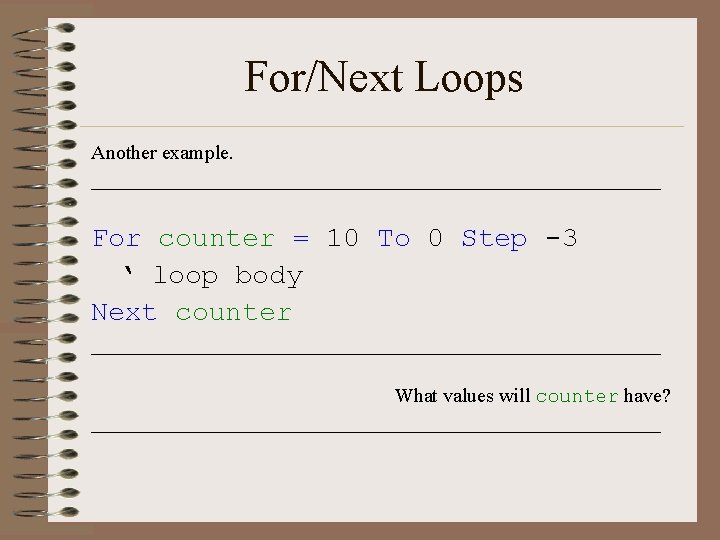
For/Next Loops Another example. _____________________________ For counter = 10 To 0 Step -3 ‘ loop body Next counter _____________________________ What values will counter have? _____________________________
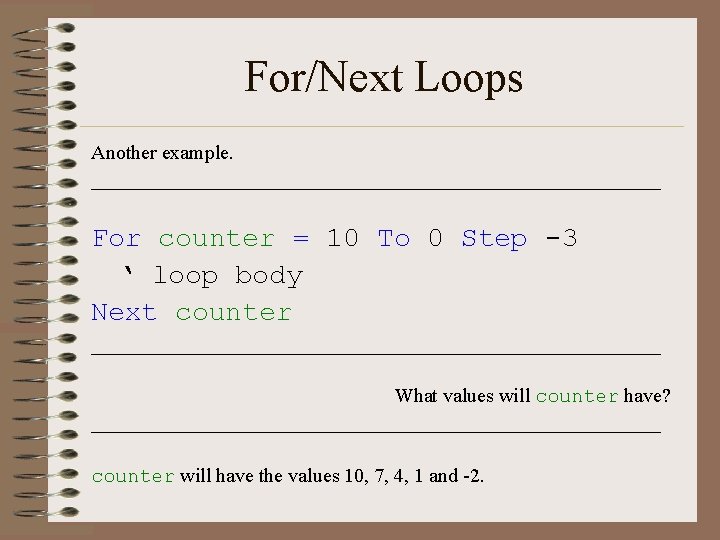
For/Next Loops Another example. _____________________________ For counter = 10 To 0 Step -3 ‘ loop body Next counter _____________________________ What values will counter have? _____________________________ counter will have the values 10, 7, 4, 1 and -2.
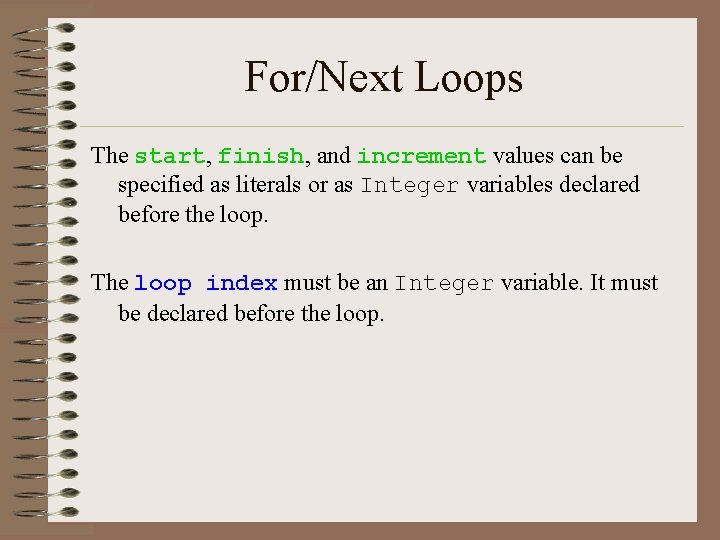
For/Next Loops The start, finish, and increment values can be specified as literals or as Integer variables declared before the loop. The loop index must be an Integer variable. It must be declared before the loop.
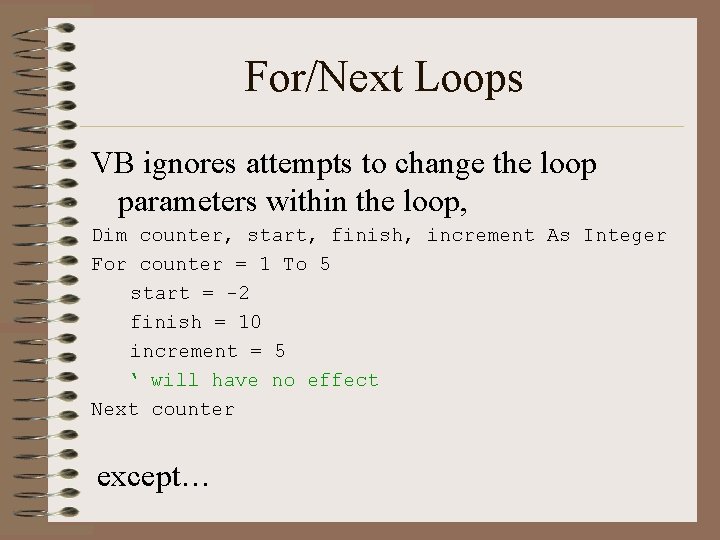
For/Next Loops VB ignores attempts to change the loop parameters within the loop, Dim counter, start, finish, increment As Integer For counter = 1 To 5 start = -2 finish = 10 increment = 5 ‘ will have no effect Next counter except…
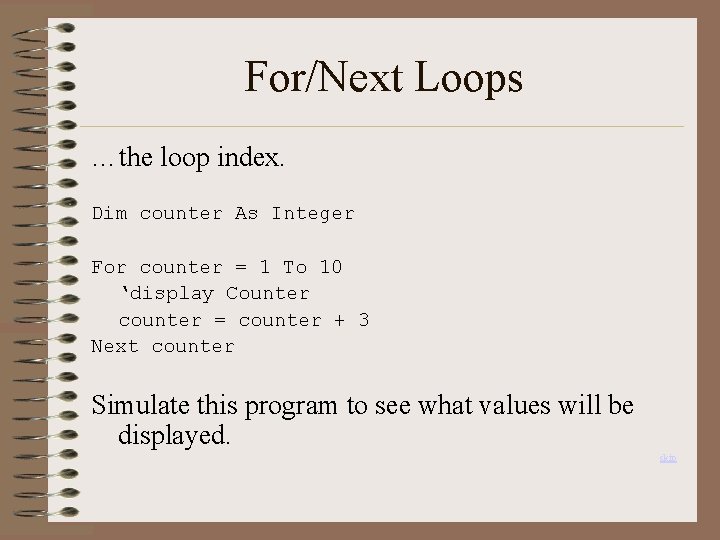
For/Next Loops …the loop index. Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter Simulate this program to see what values will be displayed. skip
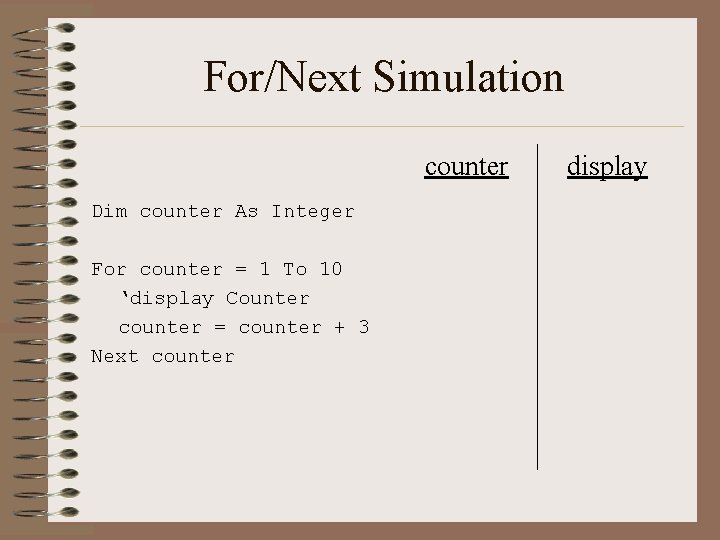
For/Next Simulation counter Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter display
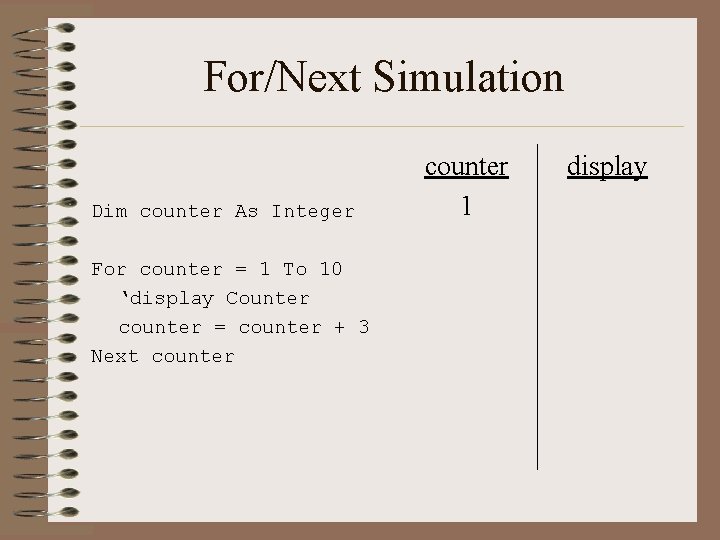
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 display
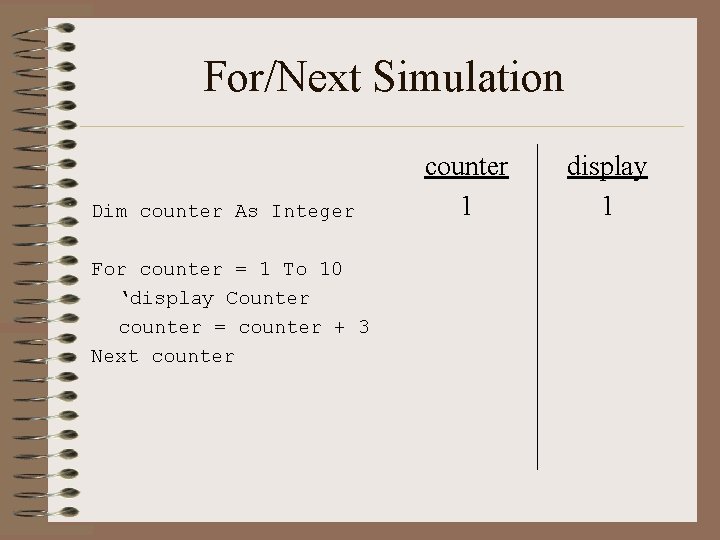
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 display 1
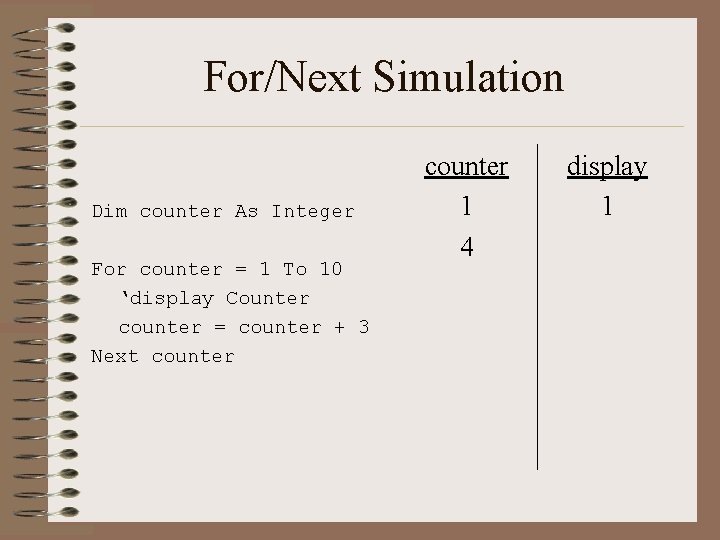
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 display 1
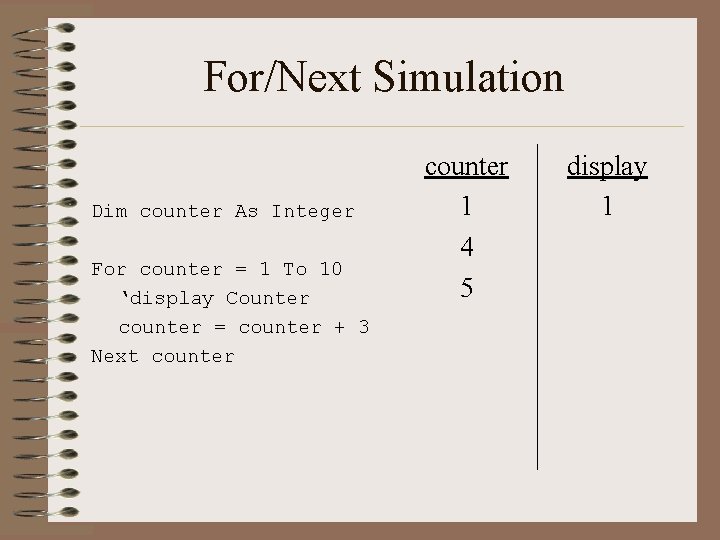
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 display 1
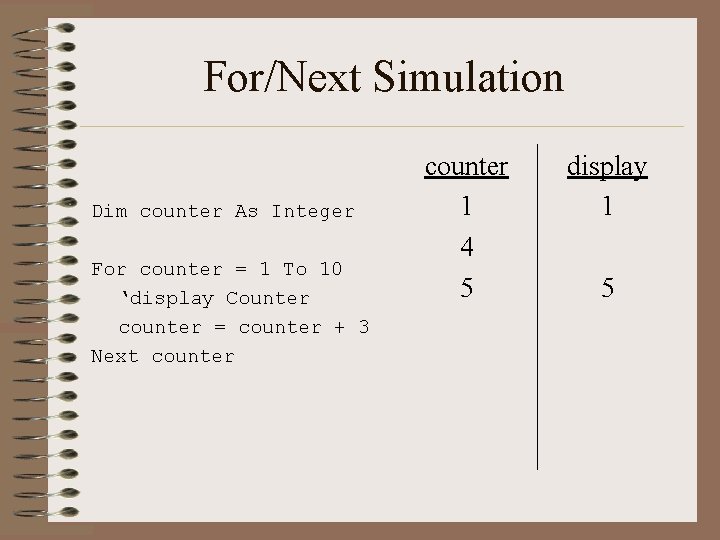
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 display 1 5
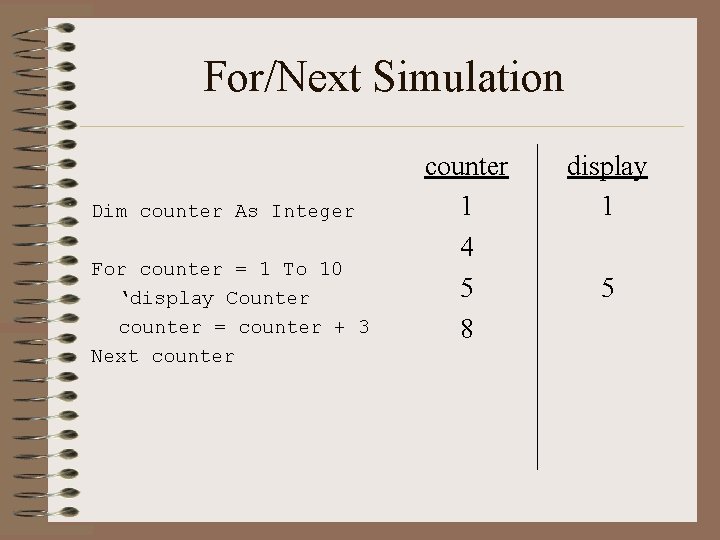
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 8 display 1 5
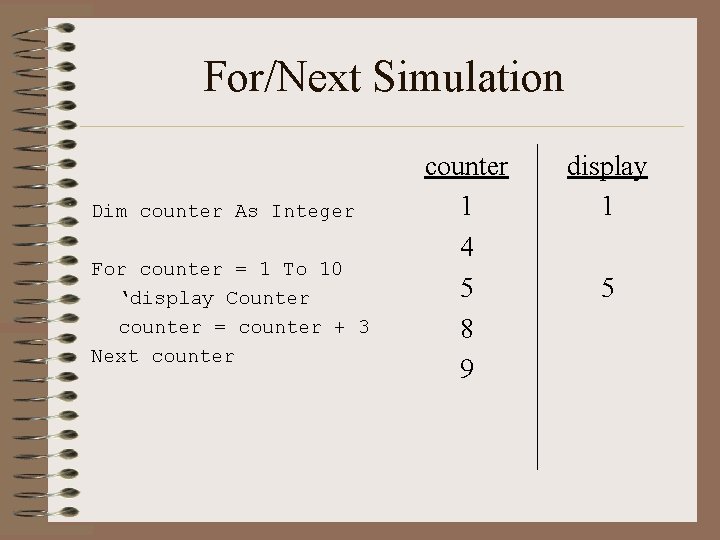
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 8 9 display 1 5
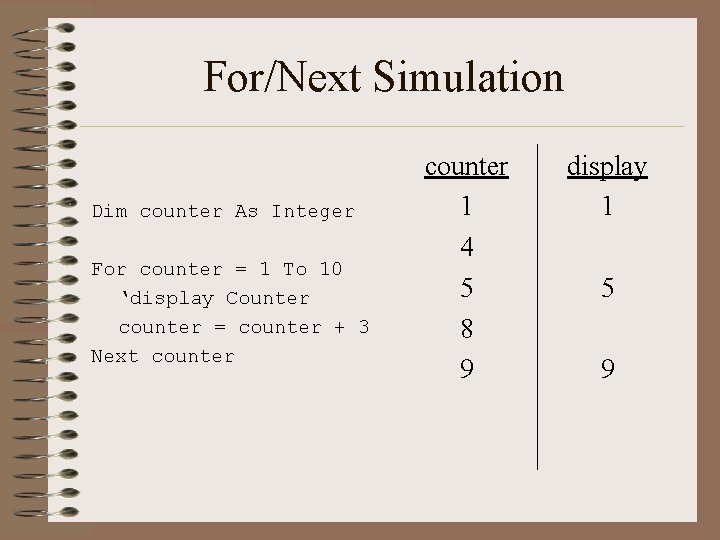
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 8 9 display 1 5 9
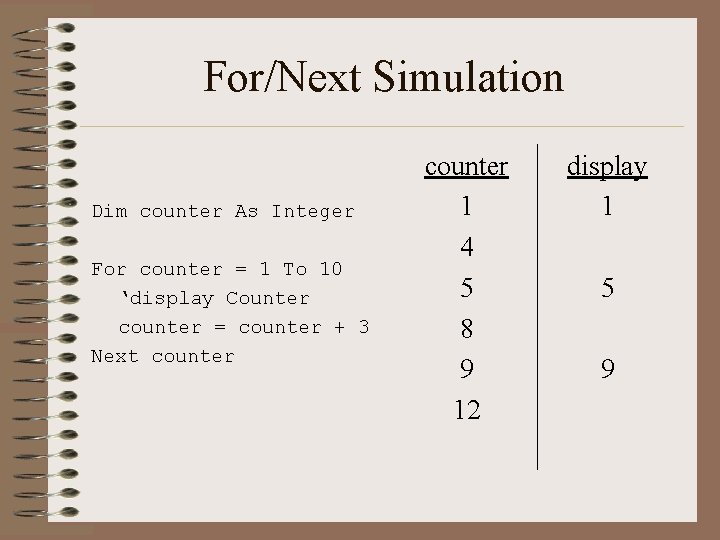
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 8 9 12 display 1 5 9
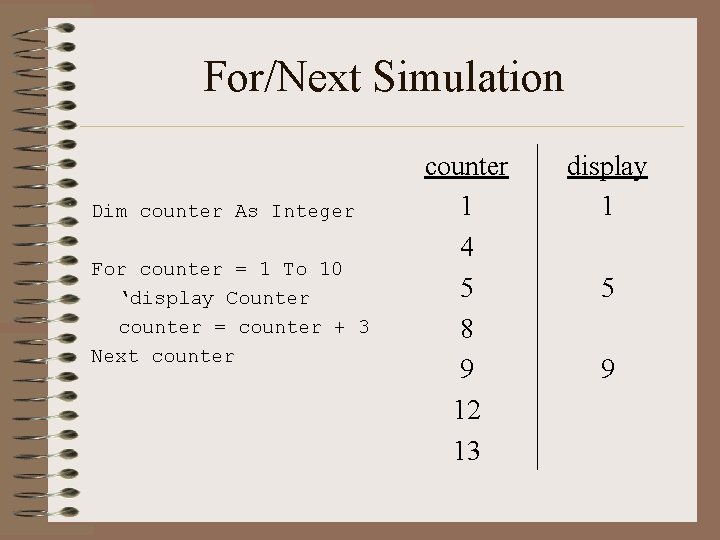
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter 1 4 5 8 9 12 13 display 1 5 9
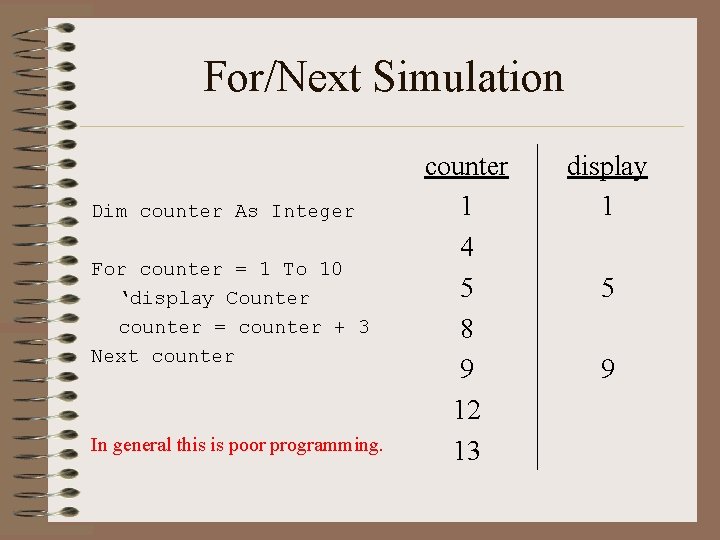
For/Next Simulation Dim counter As Integer For counter = 1 To 10 ‘display Counter counter = counter + 3 Next counter In general this is poor programming. counter 1 4 5 8 9 12 13 display 1 5 9
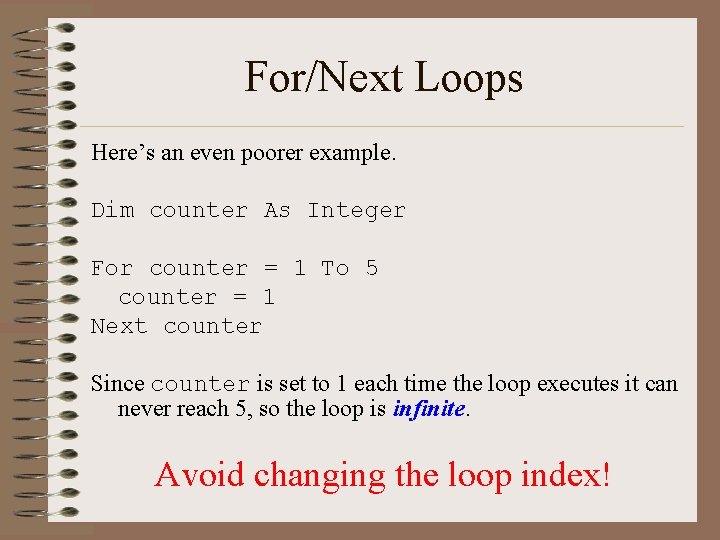
For/Next Loops Here’s an even poorer example. Dim counter As Integer For counter = 1 To 5 counter = 1 Next counter Since counter is set to 1 each time the loop executes it can never reach 5, so the loop is infinite. Avoid changing the loop index!
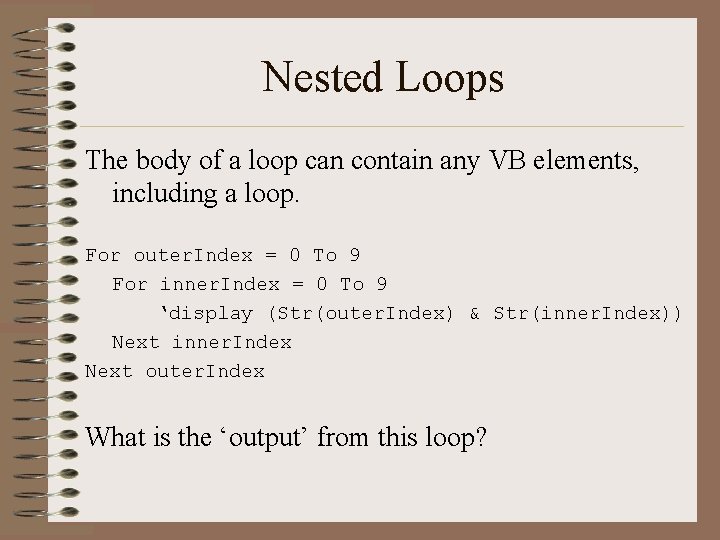
Nested Loops The body of a loop can contain any VB elements, including a loop. For outer. Index = 0 To 9 For inner. Index = 0 To 9 ‘display (Str(outer. Index) & Str(inner. Index)) Next inner. Index Next outer. Index What is the ‘output’ from this loop?
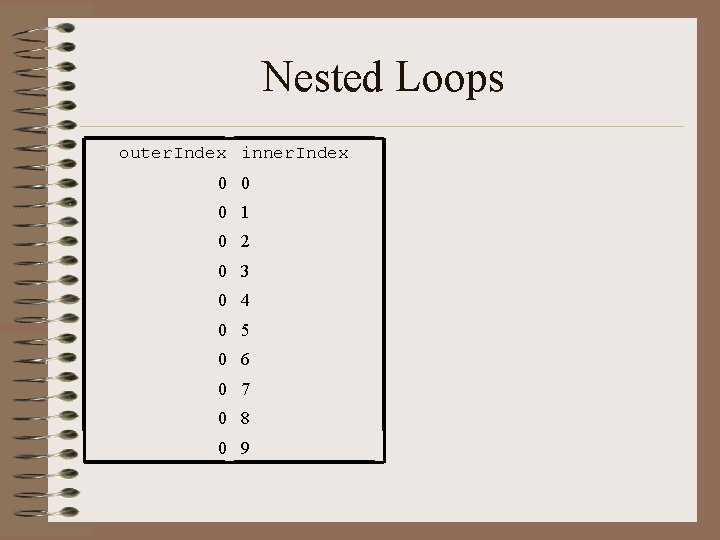
Nested Loops outer. Index inner. Index 0 0 0 1 0 2 0 3 0 4 0 5 0 6 0 7 0 8 0 9
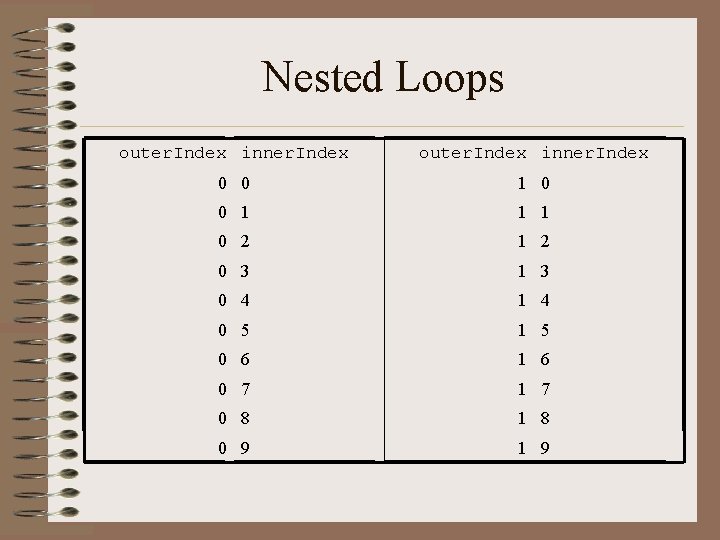
Nested Loops outer. Index inner. Index 0 0 1 1 1 0 2 1 2 0 3 1 3 0 4 1 4 0 5 1 5 0 6 1 6 0 7 1 7 0 8 1 8 0 9 1 9
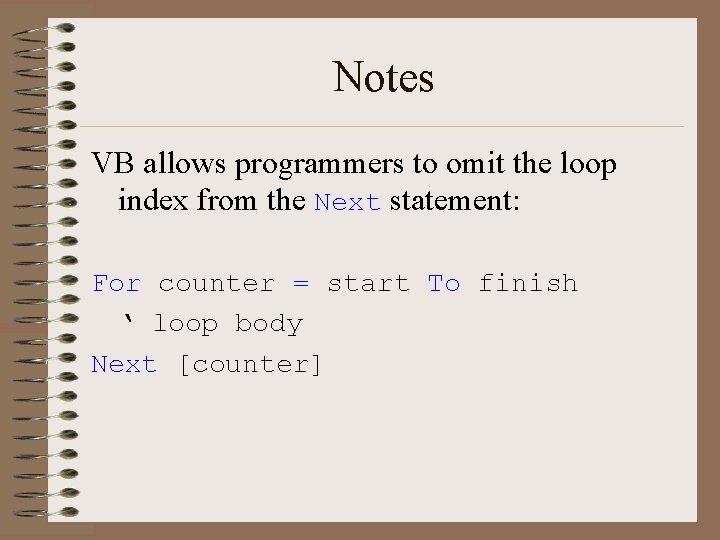
Notes VB allows programmers to omit the loop index from the Next statement: For counter = start To finish ‘ loop body Next [counter]
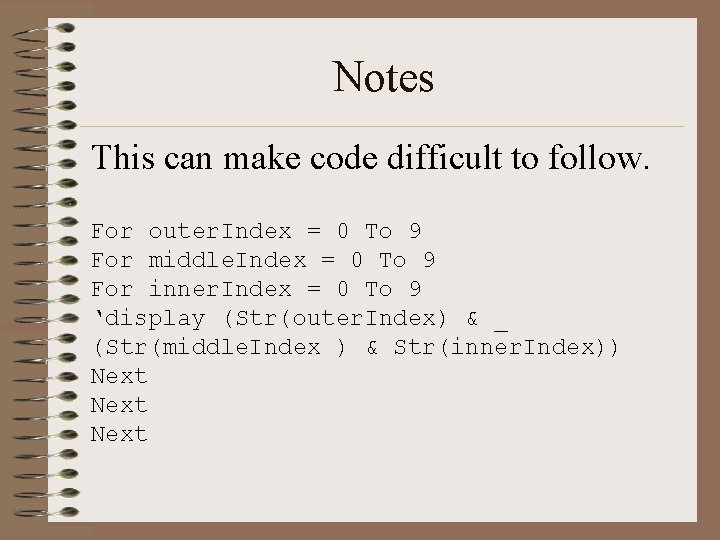
Notes This can make code difficult to follow. For outer. Index = 0 To 9 For middle. Index = 0 To 9 For inner. Index = 0 To 9 ‘display (Str(outer. Index) & _ (Str(middle. Index ) & Str(inner. Index)) Next
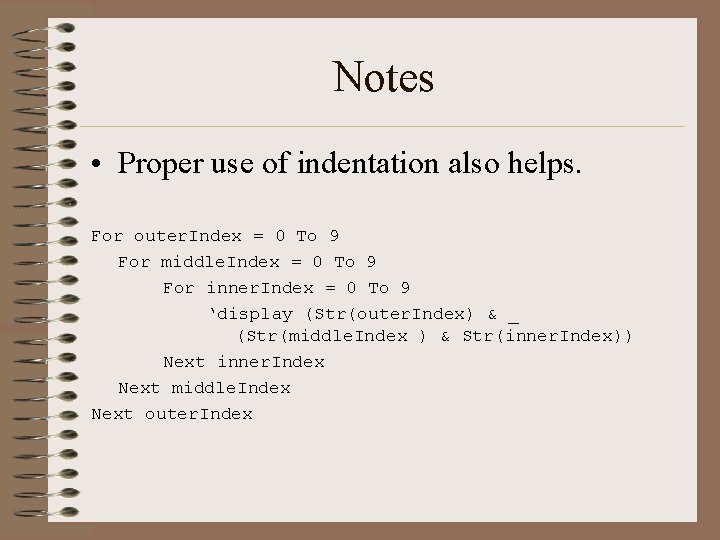
Notes • Proper use of indentation also helps. For outer. Index = 0 To 9 For middle. Index = 0 To 9 For inner. Index = 0 To 9 ‘display (Str(outer. Index) & _ (Str(middle. Index ) & Str(inner. Index)) Next inner. Index Next middle. Index Next outer. Index
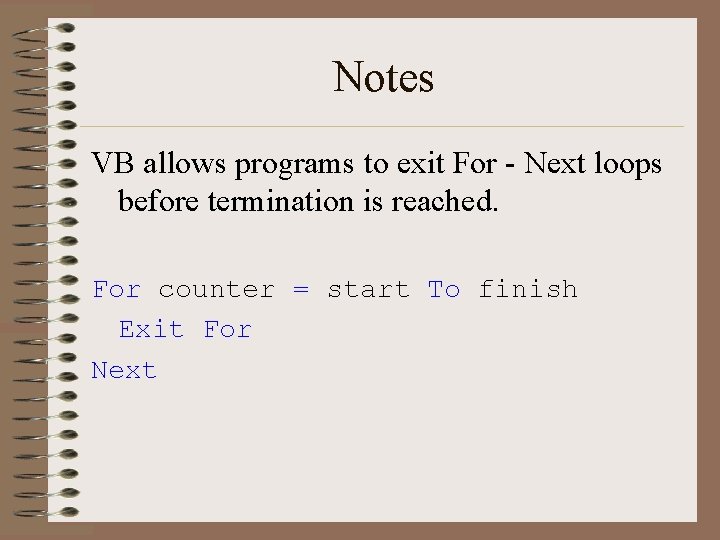
Notes VB allows programs to exit For - Next loops before termination is reached. For counter = start To finish Exit For Next
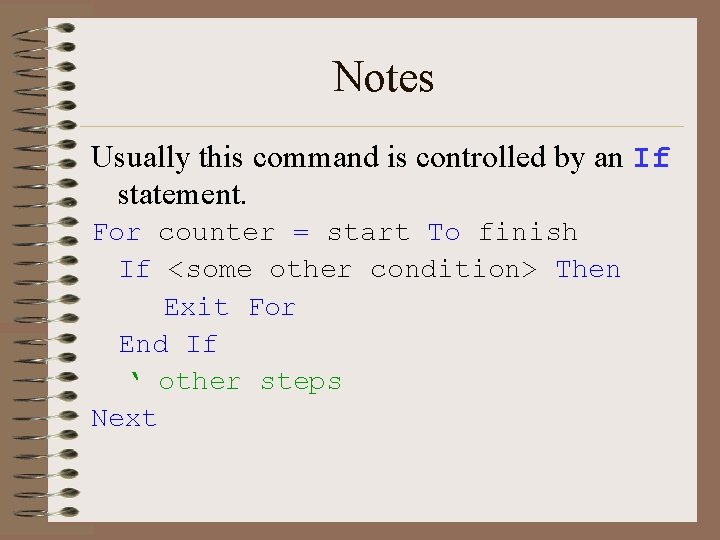
Notes Usually this command is controlled by an If statement. For counter = start To finish If <some other condition> Then Exit For End If ‘ other steps Next