Repetition Structures Loops 1 Outline Introduction While loops
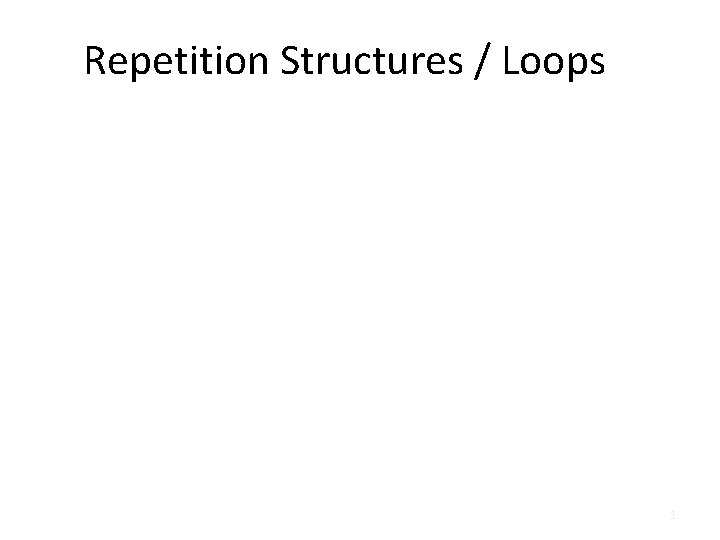
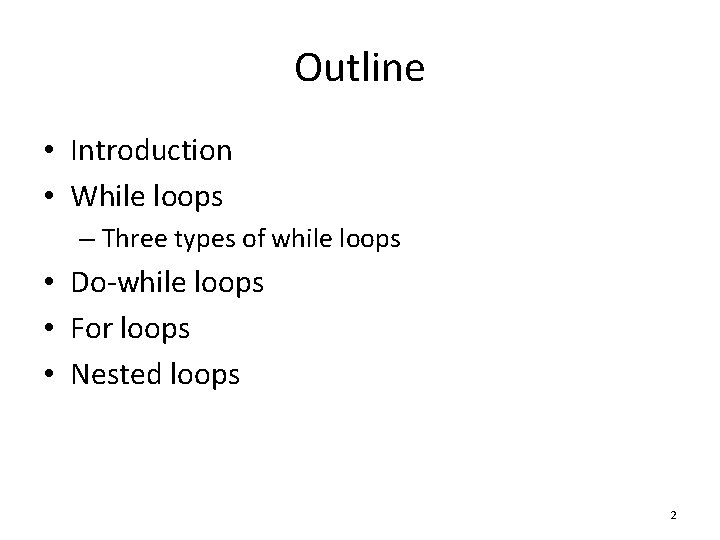
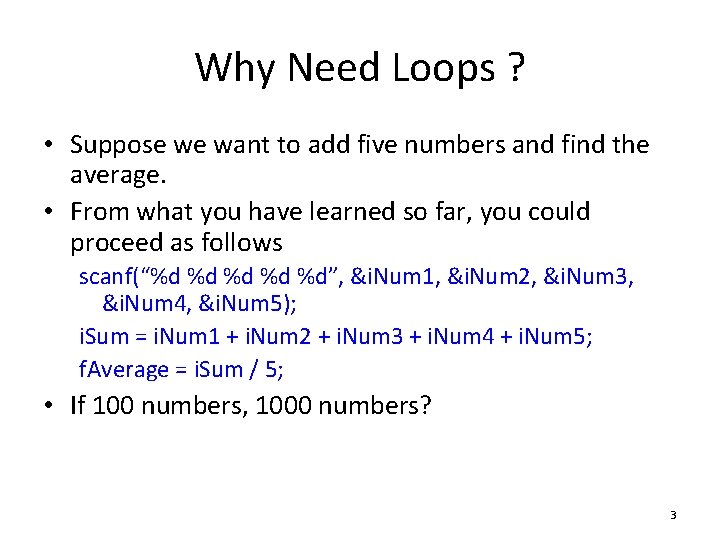
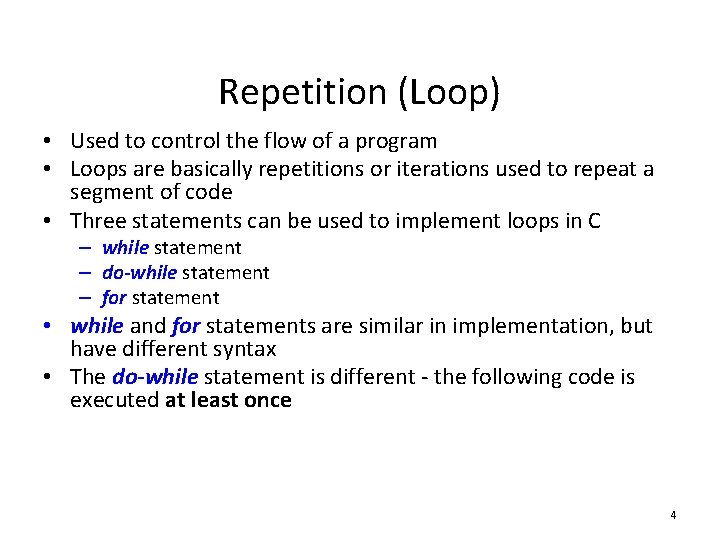
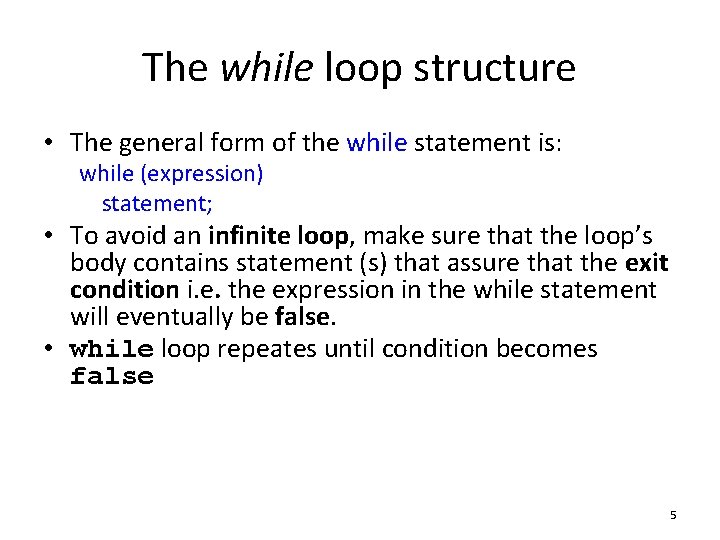
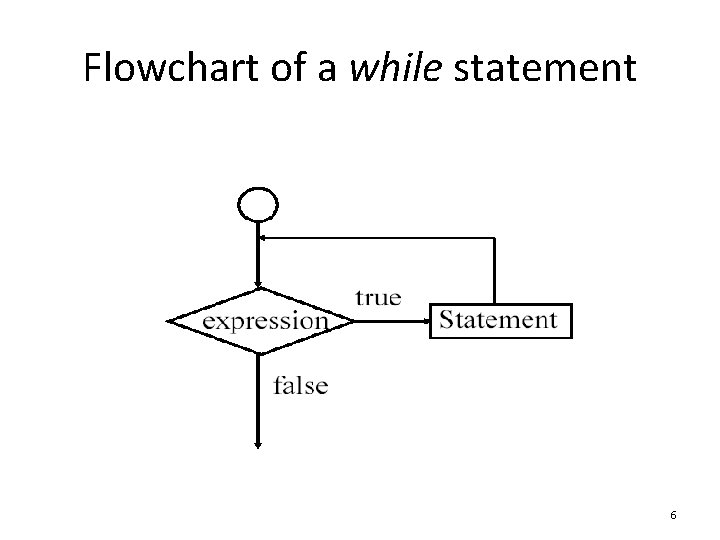
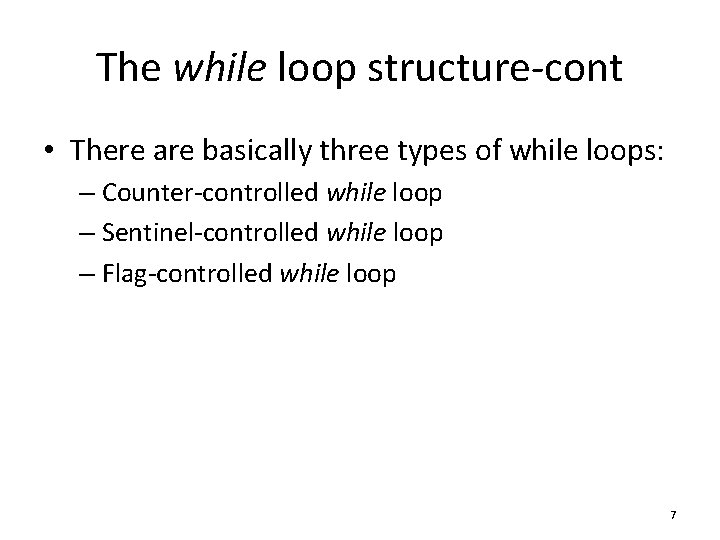
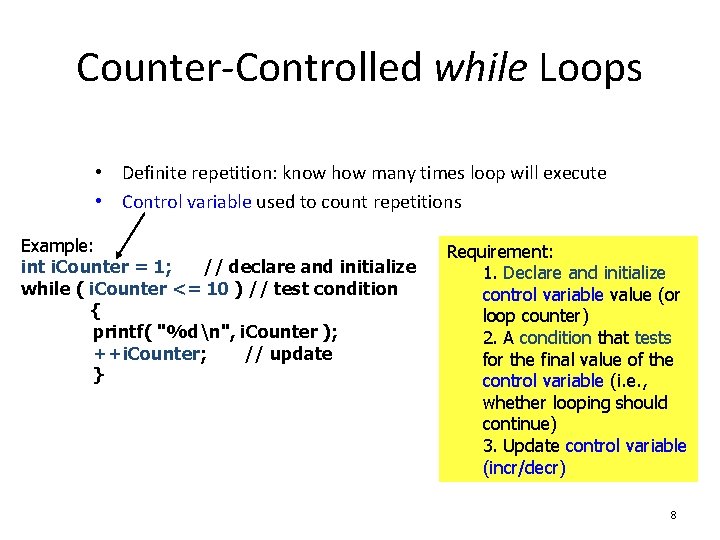
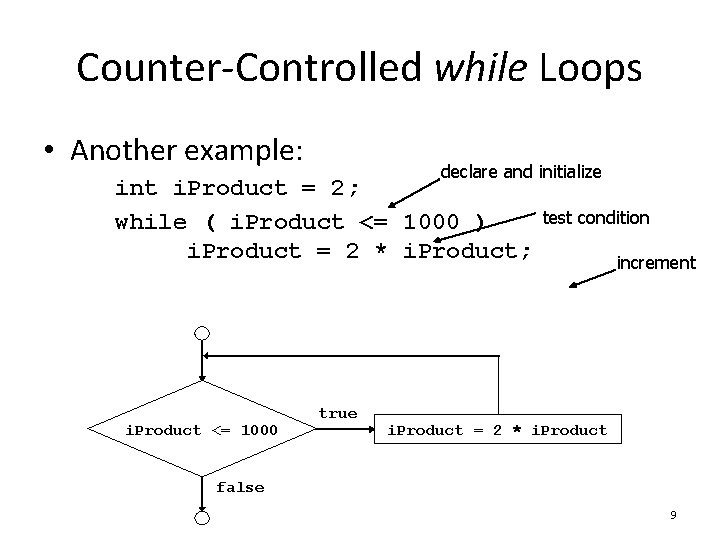
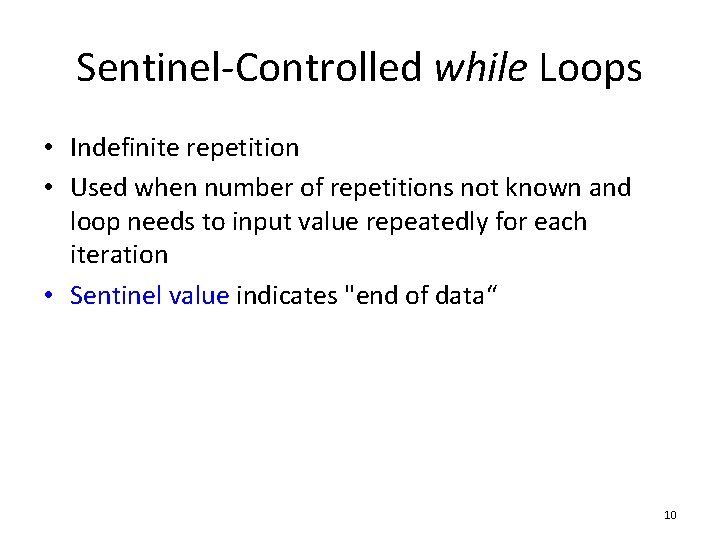
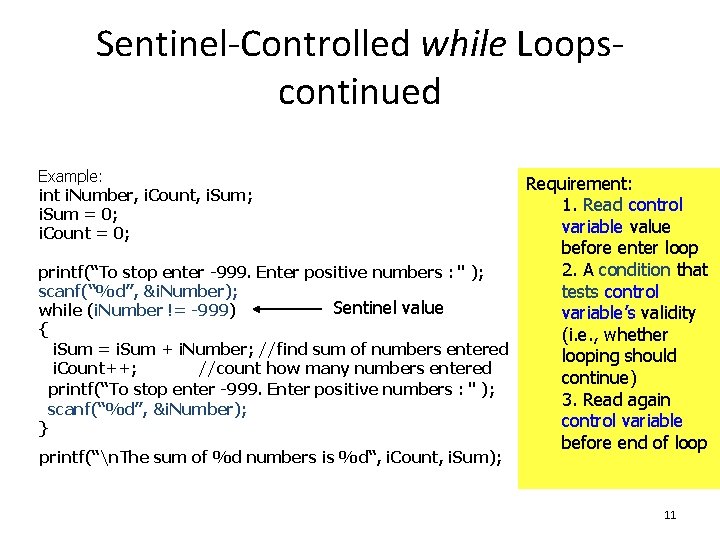
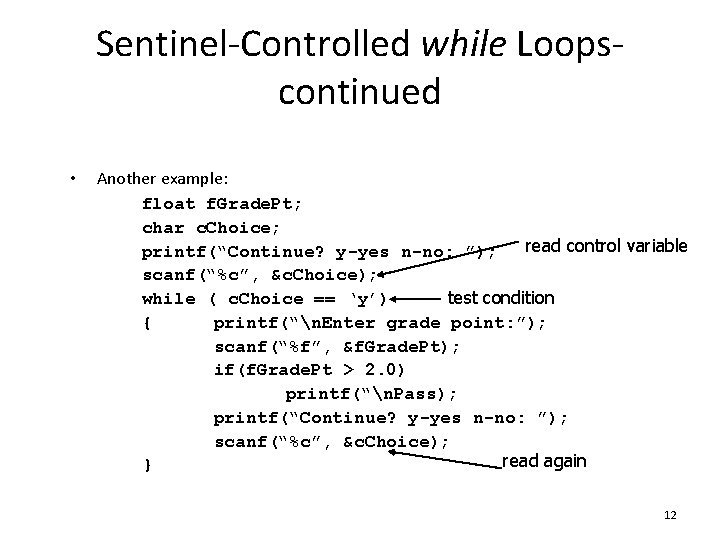
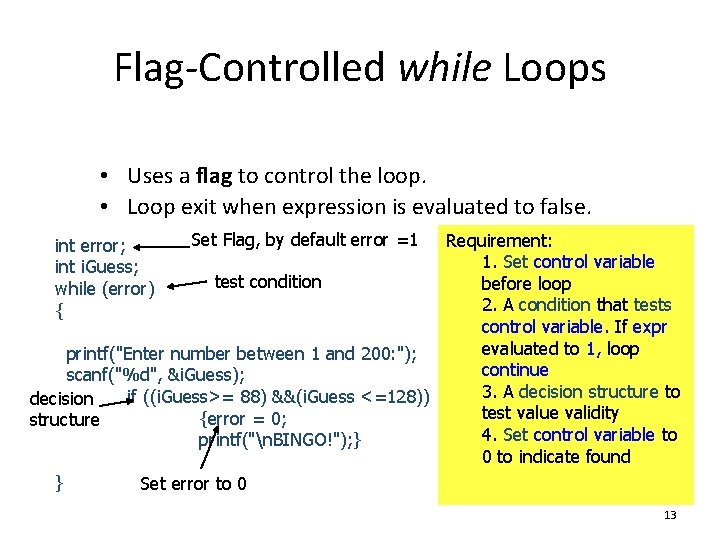
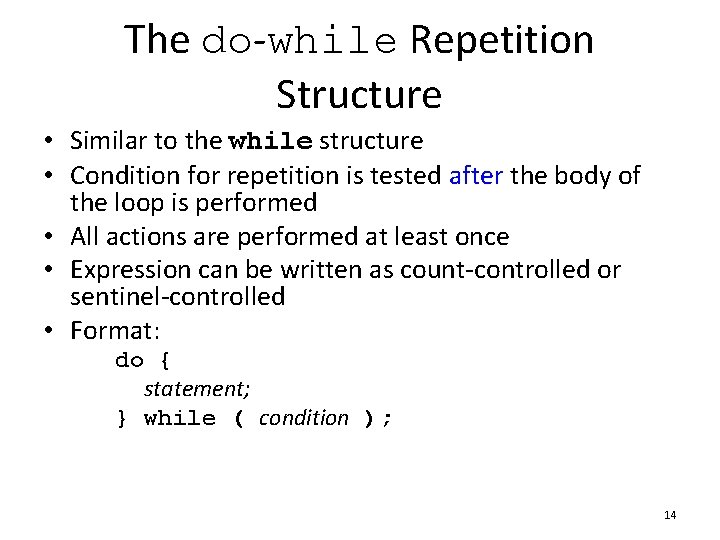
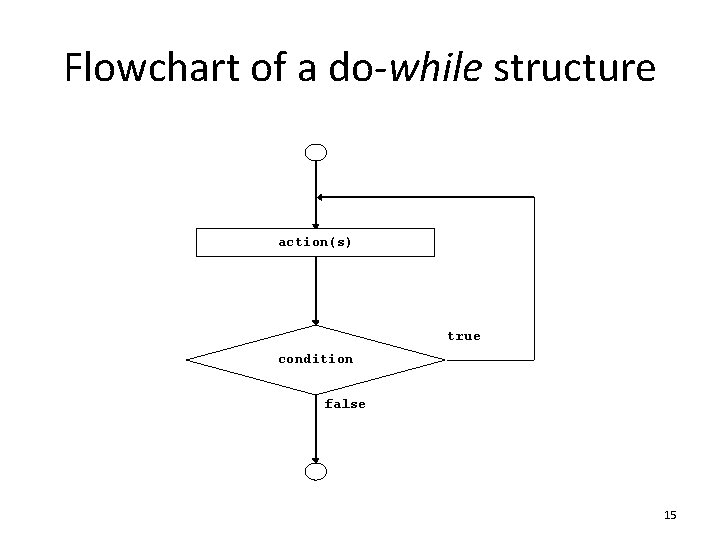
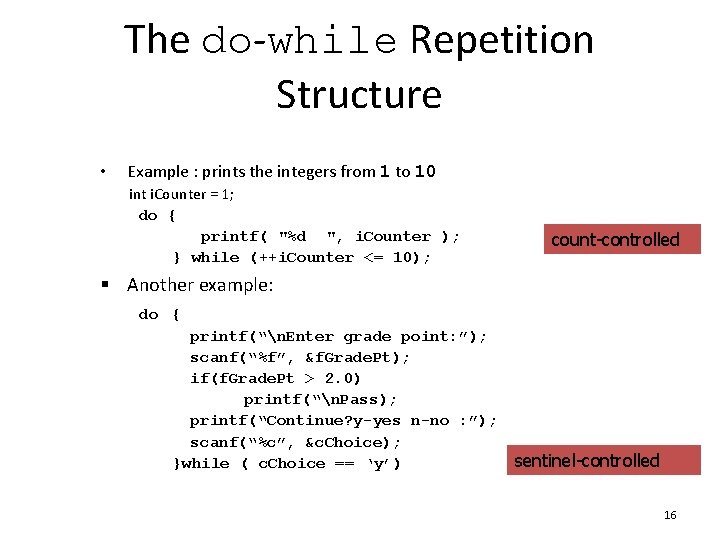
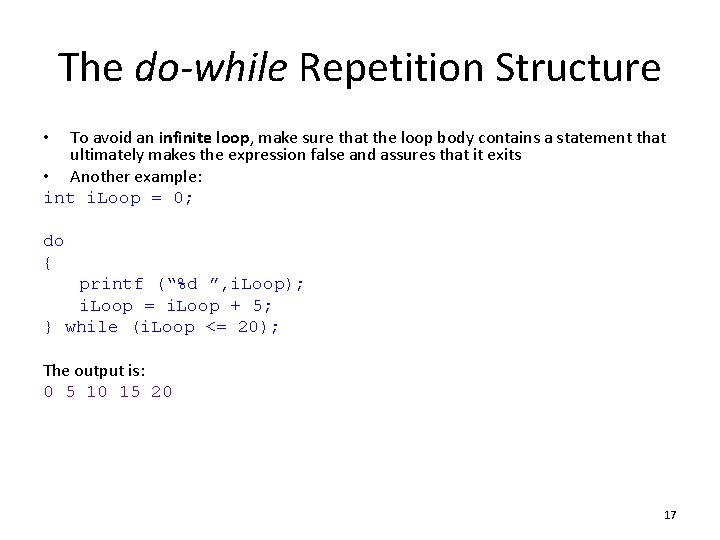
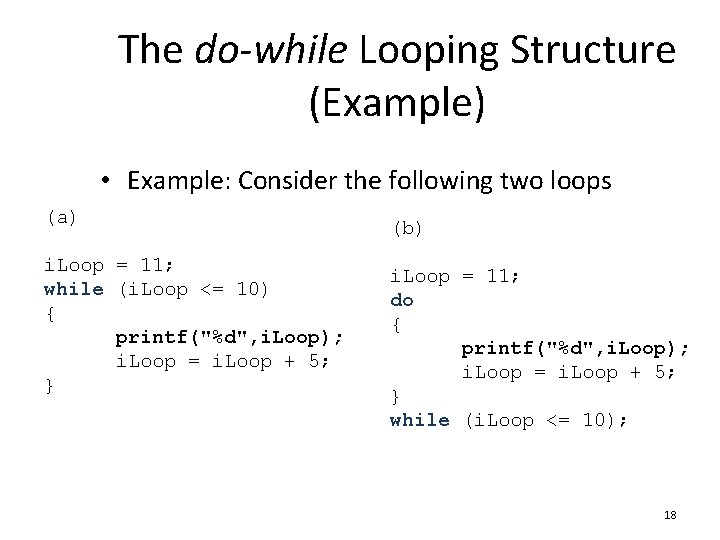
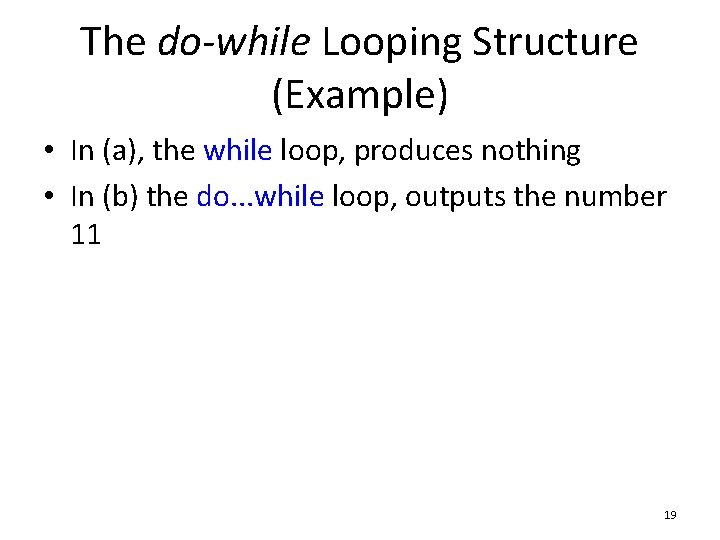
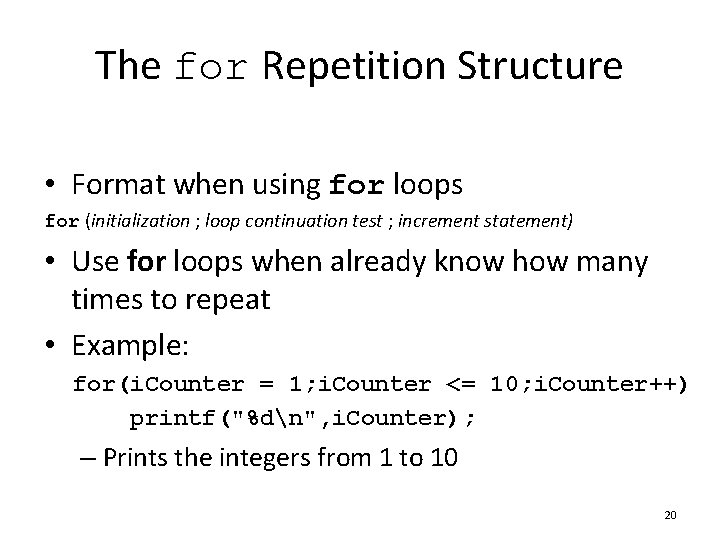
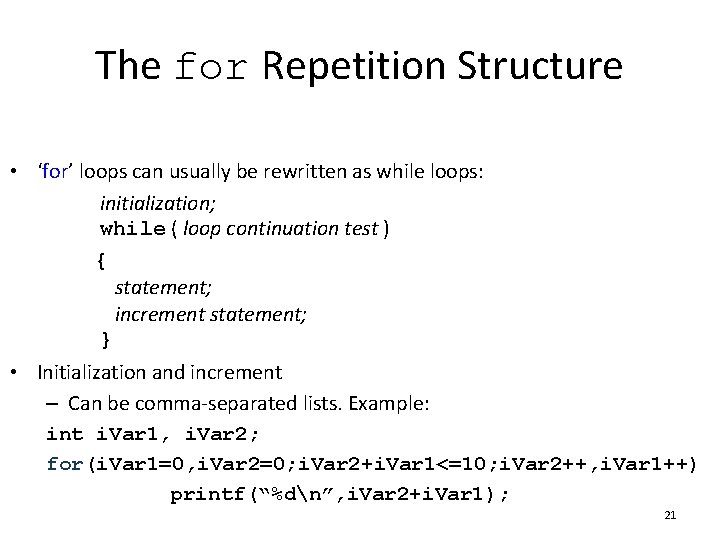
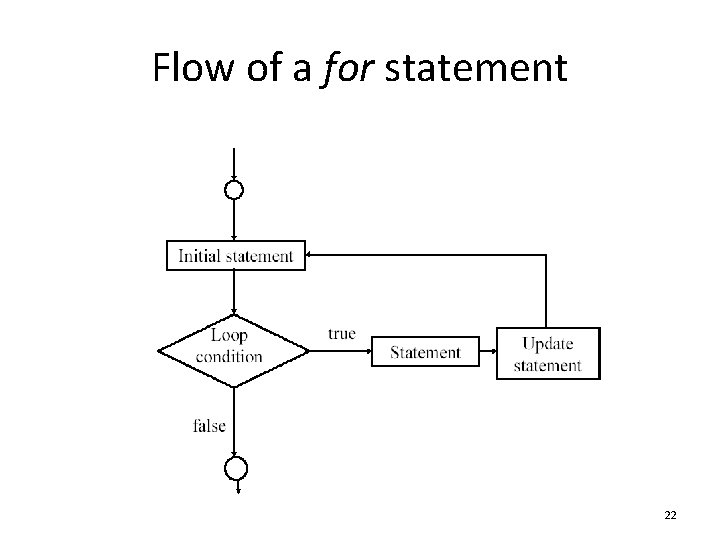
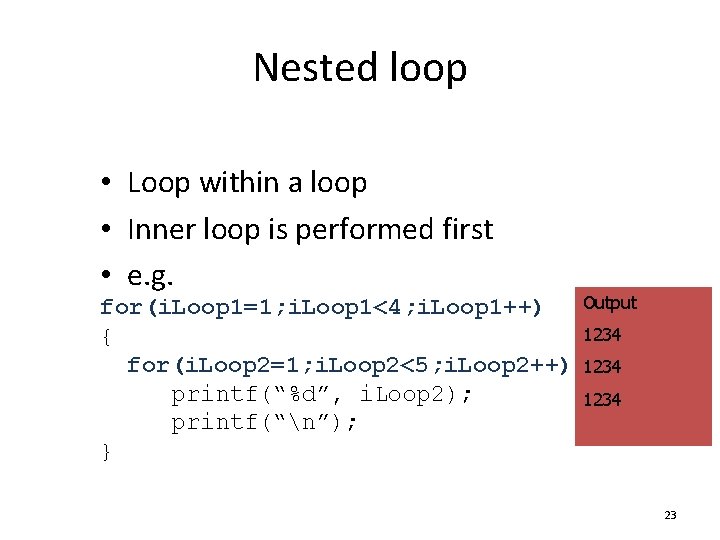
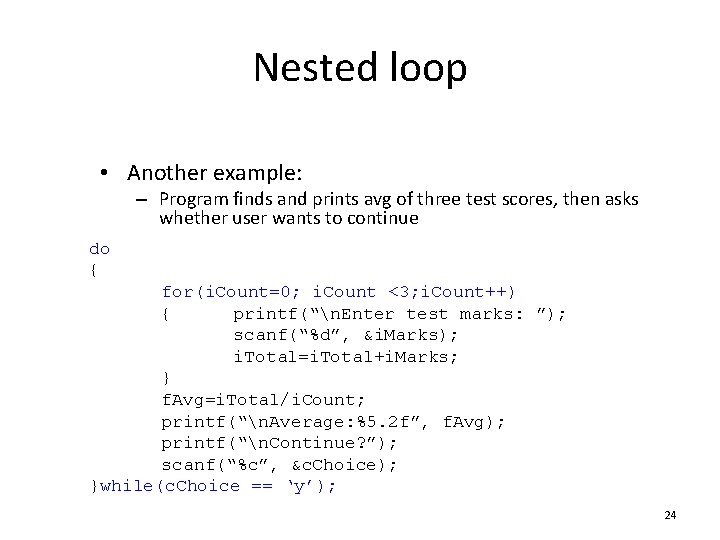
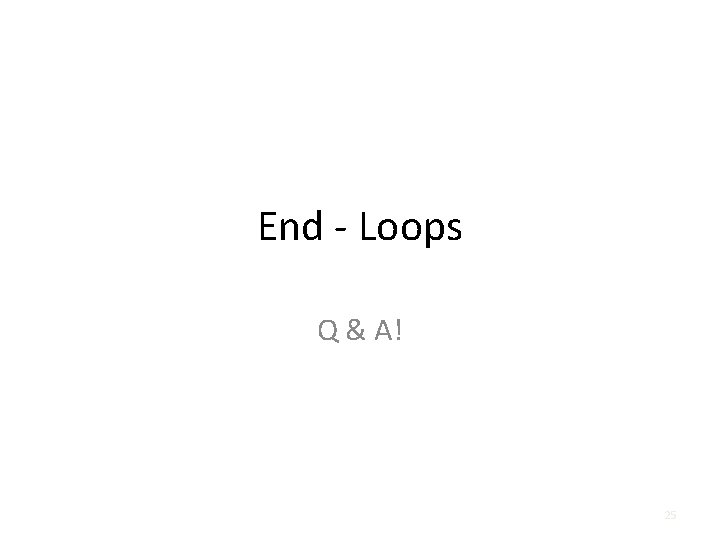
- Slides: 25
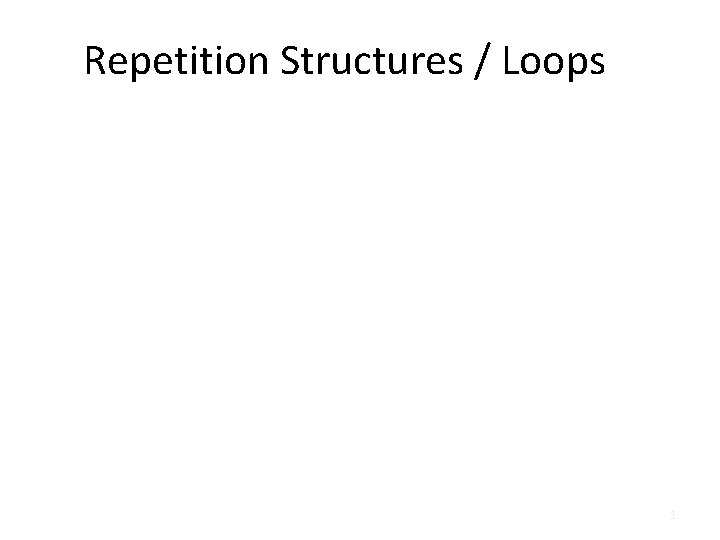
Repetition Structures / Loops 1
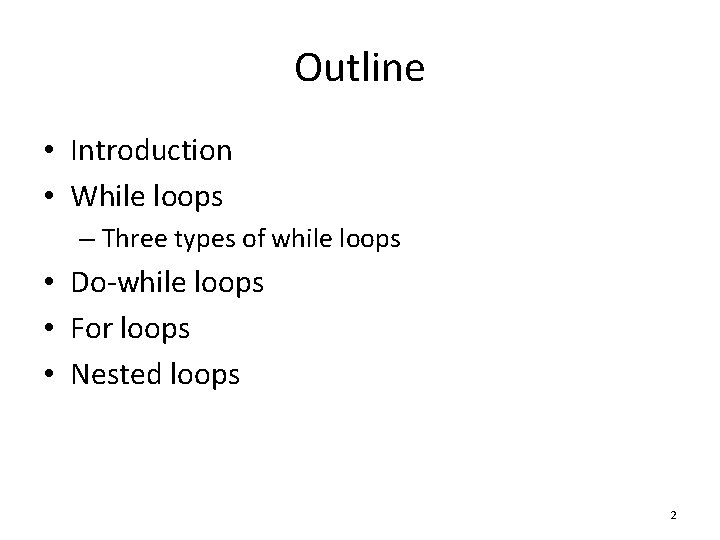
Outline • Introduction • While loops – Three types of while loops • Do-while loops • For loops • Nested loops 2
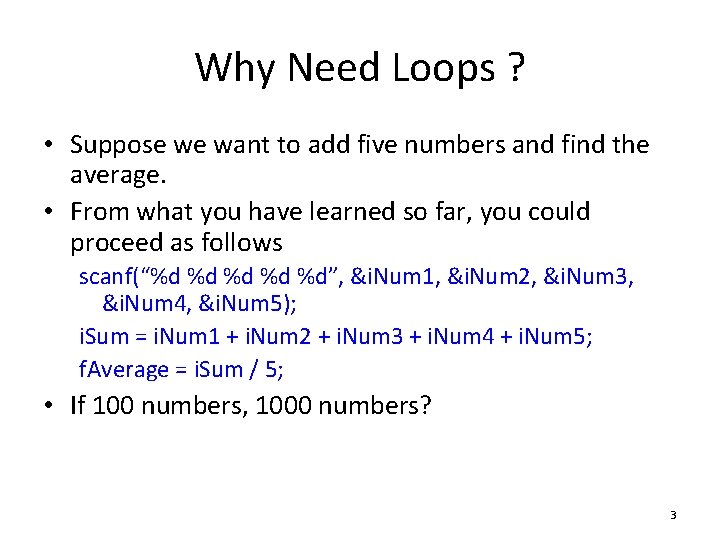
Why Need Loops ? • Suppose we want to add five numbers and find the average. • From what you have learned so far, you could proceed as follows scanf(“%d %d %d”, &i. Num 1, &i. Num 2, &i. Num 3, &i. Num 4, &i. Num 5); i. Sum = i. Num 1 + i. Num 2 + i. Num 3 + i. Num 4 + i. Num 5; f. Average = i. Sum / 5; • If 100 numbers, 1000 numbers? 3
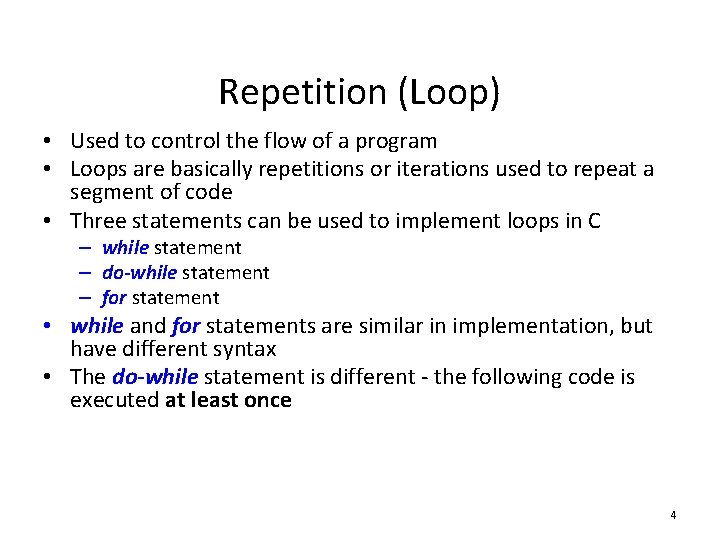
Repetition (Loop) • Used to control the flow of a program • Loops are basically repetitions or iterations used to repeat a segment of code • Three statements can be used to implement loops in C – while statement – do-while statement – for statement • while and for statements are similar in implementation, but have different syntax • The do-while statement is different - the following code is executed at least once 4
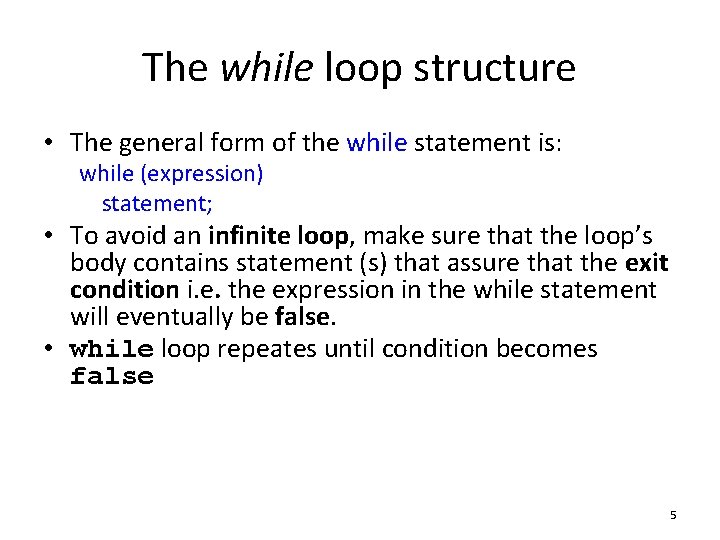
The while loop structure • The general form of the while statement is: while (expression) statement; • To avoid an infinite loop, make sure that the loop’s body contains statement (s) that assure that the exit condition i. e. the expression in the while statement will eventually be false. • while loop repeates until condition becomes false 5
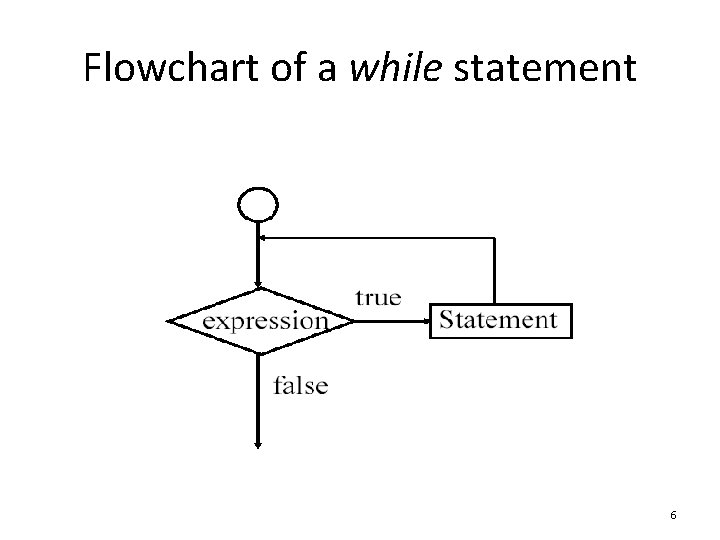
Flowchart of a while statement 6
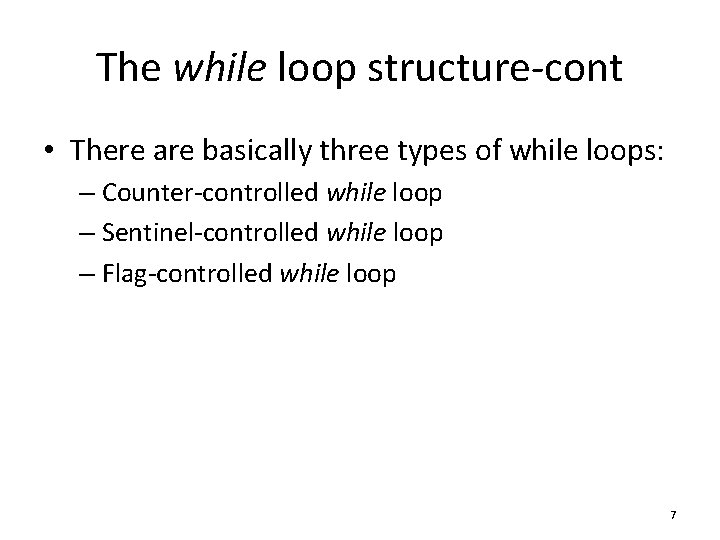
The while loop structure-cont • There are basically three types of while loops: – Counter-controlled while loop – Sentinel-controlled while loop – Flag-controlled while loop 7
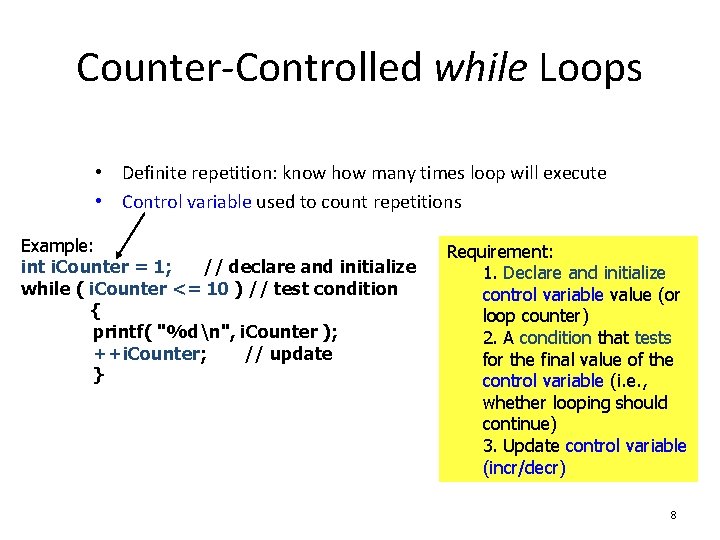
Counter-Controlled while Loops • Definite repetition: know how many times loop will execute • Control variable used to count repetitions Example: int i. Counter = 1; // declare and initialize while ( i. Counter <= 10 ) // test condition { printf( "%dn", i. Counter ); ++i. Counter; // update } Requirement: 1. Declare and initialize control variable value (or loop counter) 2. A condition that tests for the final value of the control variable (i. e. , whether looping should continue) 3. Update control variable (incr/decr) 8
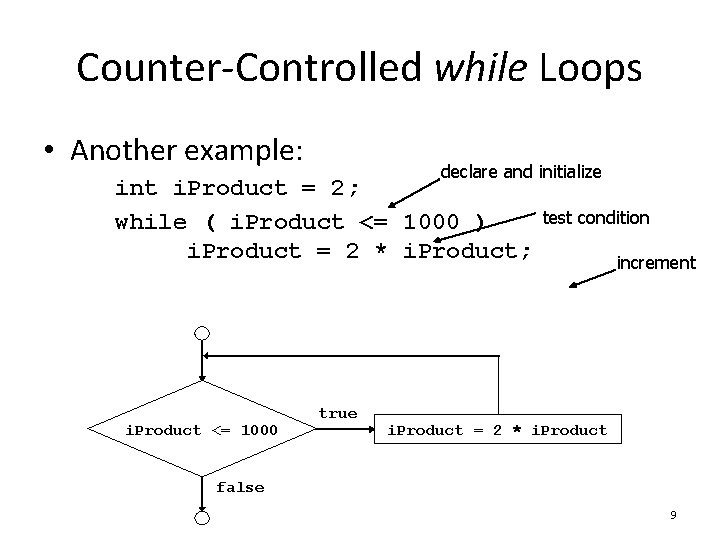
Counter-Controlled while Loops • Another example: declare and initialize int i. Product = 2; while ( i. Product <= 1000 ) i. Product = 2 * i. Product; i. Product <= 1000 true test condition increment i. Product = 2 * i. Product false 9
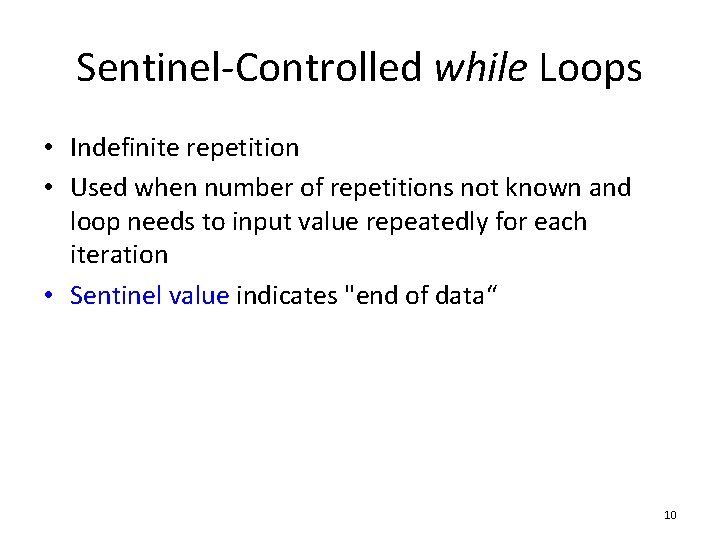
Sentinel-Controlled while Loops • Indefinite repetition • Used when number of repetitions not known and loop needs to input value repeatedly for each iteration • Sentinel value indicates "end of data“ 10
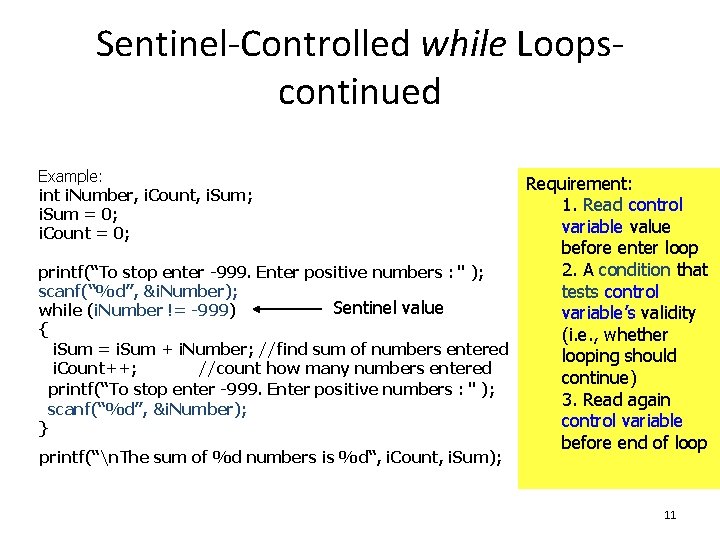
Sentinel-Controlled while Loopscontinued Example: int i. Number, i. Count, i. Sum; i. Sum = 0; i. Count = 0; Requirement: 1. Read control variable value before enter loop 2. A condition that printf(“To stop enter -999. Enter positive numbers : " ); scanf(“%d”, &i. Number); tests control Sentinel value while (i. Number != -999) variable’s validity { (i. e. , whether i. Sum = i. Sum + i. Number; //find sum of numbers entered looping should i. Count++; //count how many numbers entered continue) printf(“To stop enter -999. Enter positive numbers : " ); 3. Read again scanf(“%d”, &i. Number); control variable } before end of loop printf(“n. The sum of %d numbers is %d“, i. Count, i. Sum); 11
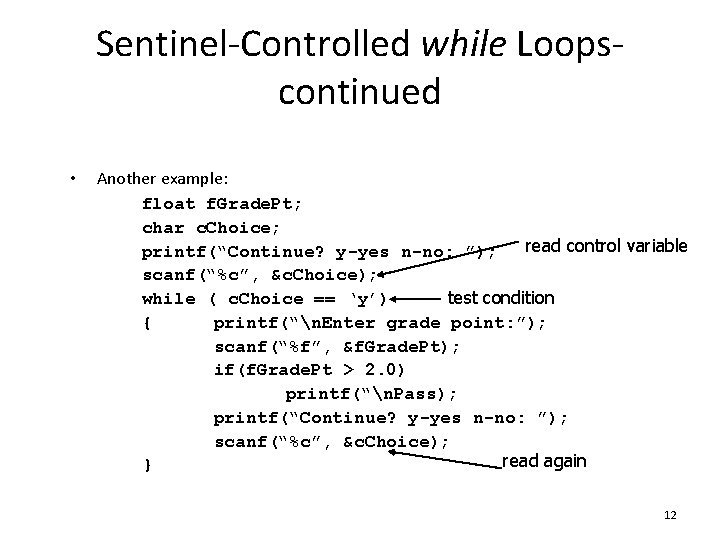
Sentinel-Controlled while Loopscontinued • Another example: float f. Grade. Pt; char c. Choice; read control variable printf(“Continue? y-yes n-no: ”); scanf(“%c”, &c. Choice); test condition while ( c. Choice == ‘y’) { printf(“n. Enter grade point: ”); scanf(“%f”, &f. Grade. Pt); if(f. Grade. Pt > 2. 0) printf(“n. Pass); printf(“Continue? y-yes n-no: ”); scanf(“%c”, &c. Choice); read again } 12
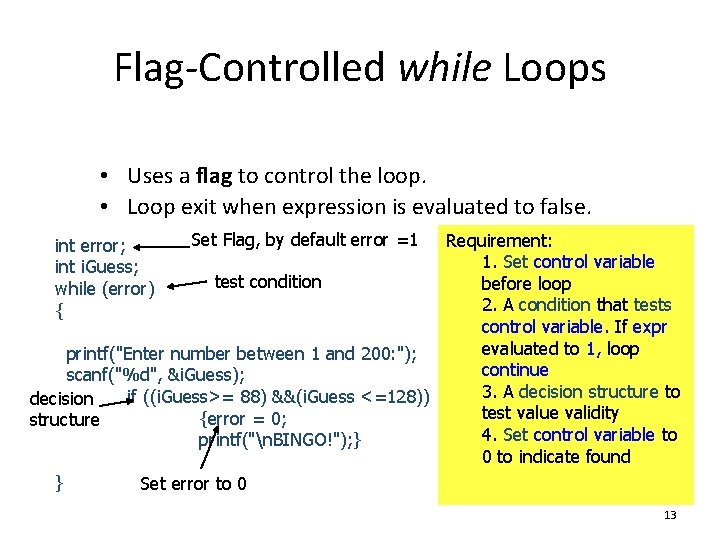
Flag-Controlled while Loops • Uses a flag to control the loop. • Loop exit when expression is evaluated to false. Set Flag, by default error =1 Requirement: 1. Set control variable test condition before loop 2. A condition that tests control variable. If expr evaluated to 1, loop printf("Enter number between 1 and 200: "); continue scanf("%d", &i. Guess); 3. A decision structure to if ((i. Guess>= 88) &&(i. Guess <=128)) decision test value validity {error = 0; structure 4. Set control variable to printf("n. BINGO!"); } 0 to indicate found } Set error to 0 int error; int i. Guess; while (error) { 13
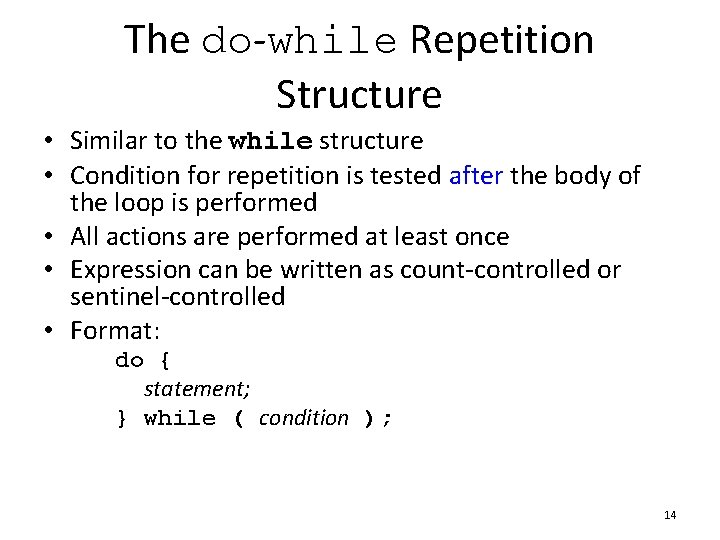
The do-while Repetition Structure • Similar to the while structure • Condition for repetition is tested after the body of the loop is performed • All actions are performed at least once • Expression can be written as count-controlled or sentinel-controlled • Format: do { statement; } while ( condition ); 14
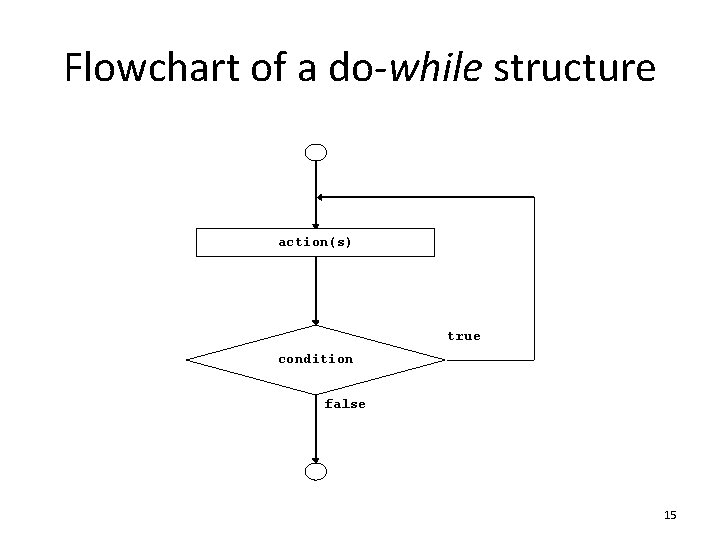
Flowchart of a do-while structure action(s) true condition false 15
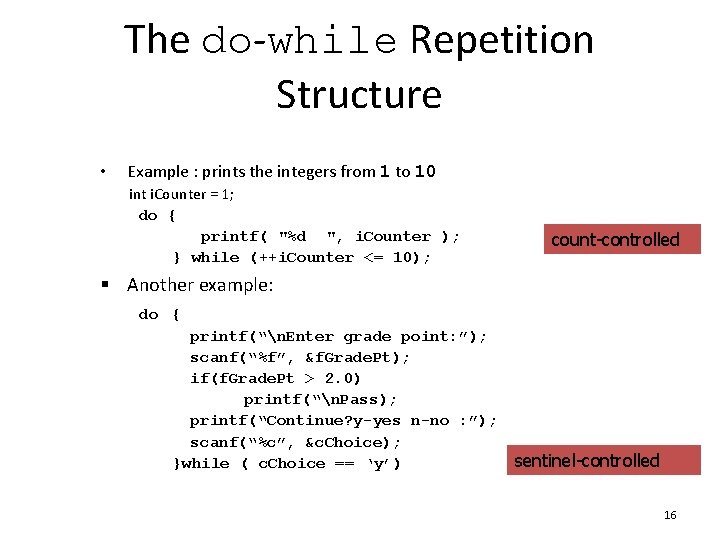
The do-while Repetition Structure • Example : prints the integers from 1 to 10 int i. Counter = 1; do { printf( "%d ", i. Counter ); } while (++i. Counter <= 10); count-controlled § Another example: do { printf(“n. Enter grade point: ”); scanf(“%f”, &f. Grade. Pt); if(f. Grade. Pt > 2. 0) printf(“n. Pass); printf(“Continue? y-yes n-no : ”); scanf(“%c”, &c. Choice); }while ( c. Choice == ‘y’) sentinel-controlled 16
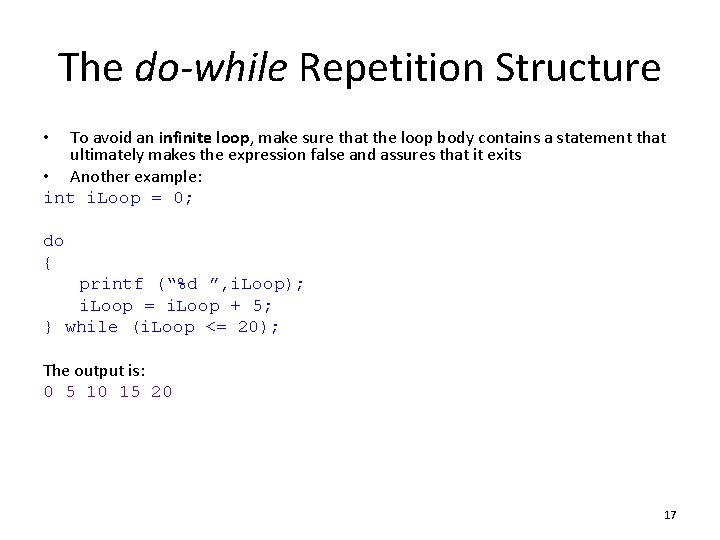
The do-while Repetition Structure To avoid an infinite loop, make sure that the loop body contains a statement that ultimately makes the expression false and assures that it exits • Another example: int i. Loop = 0; • do { printf (“%d ”, i. Loop); i. Loop = i. Loop + 5; } while (i. Loop <= 20); The output is: 0 5 10 15 20 17
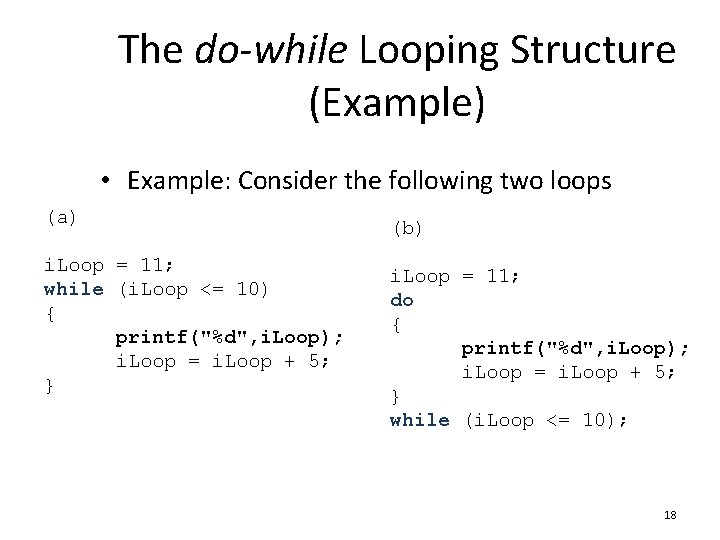
The do-while Looping Structure (Example) • Example: Consider the following two loops (a) i. Loop = 11; while (i. Loop <= 10) { printf("%d", i. Loop); i. Loop = i. Loop + 5; } (b) i. Loop = 11; do { printf("%d", i. Loop); i. Loop = i. Loop + 5; } while (i. Loop <= 10); 18
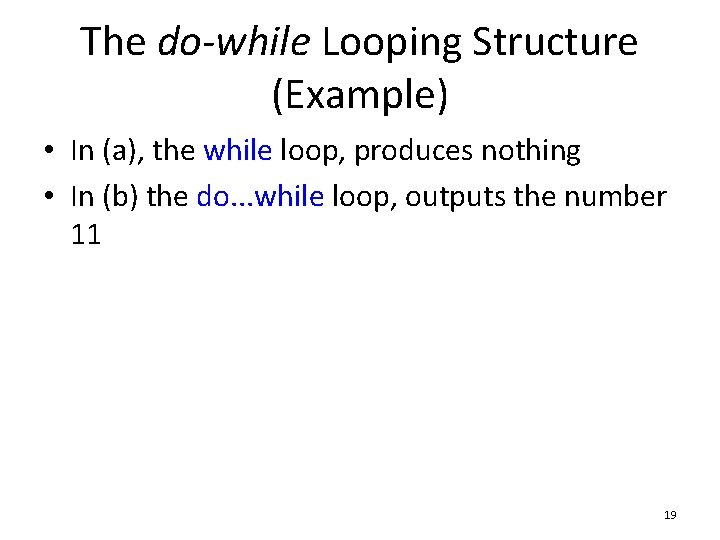
The do-while Looping Structure (Example) • In (a), the while loop, produces nothing • In (b) the do. . . while loop, outputs the number 11 19
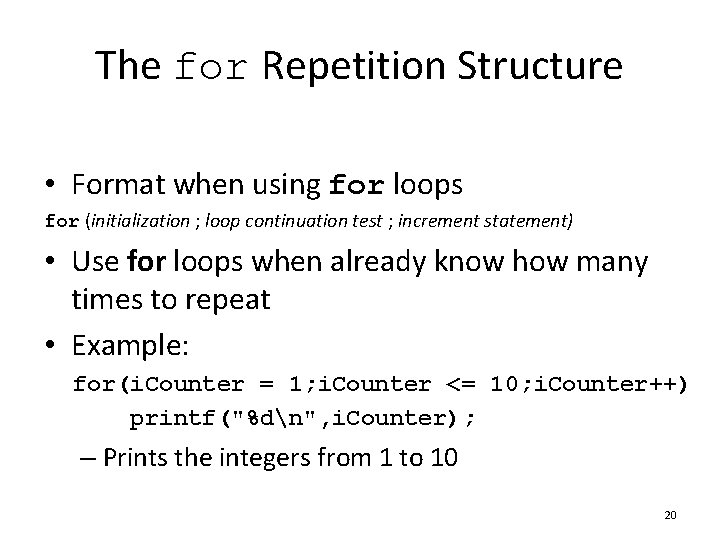
The for Repetition Structure • Format when using for loops for (initialization ; loop continuation test ; increment statement) • Use for loops when already know how many times to repeat • Example: for(i. Counter = 1; i. Counter <= 10; i. Counter++) printf("%dn", i. Counter); – Prints the integers from 1 to 10 20
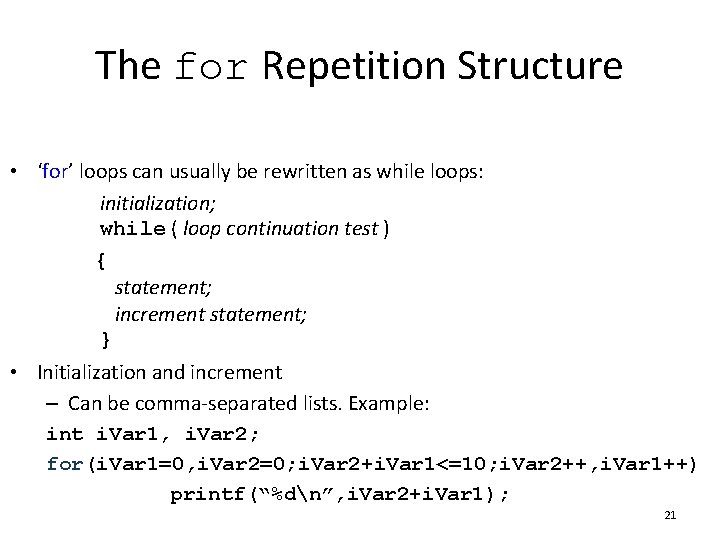
The for Repetition Structure • ‘for’ loops can usually be rewritten as while loops: initialization; while ( loop continuation test ) { statement; increment statement; } • Initialization and increment – Can be comma-separated lists. Example: int i. Var 1, i. Var 2; for(i. Var 1=0, i. Var 2=0; i. Var 2+i. Var 1<=10; i. Var 2++, i. Var 1++) printf(“%dn”, i. Var 2+i. Var 1); 21
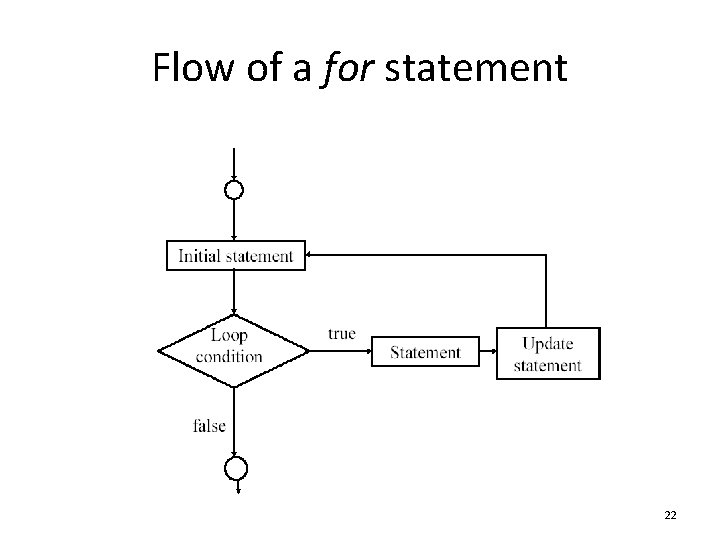
Flow of a for statement 22
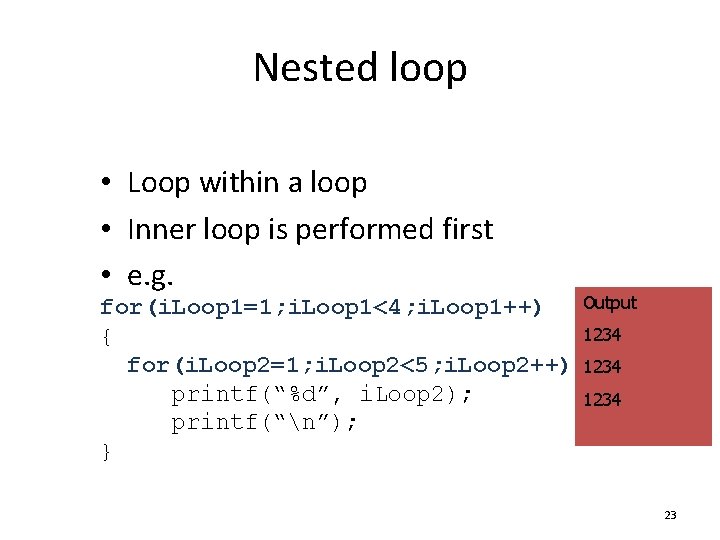
Nested loop • Loop within a loop • Inner loop is performed first • e. g. for(i. Loop 1=1; i. Loop 1<4; i. Loop 1++) { for(i. Loop 2=1; i. Loop 2<5; i. Loop 2++) printf(“%d”, i. Loop 2); printf(“n”); } Output 1234 23
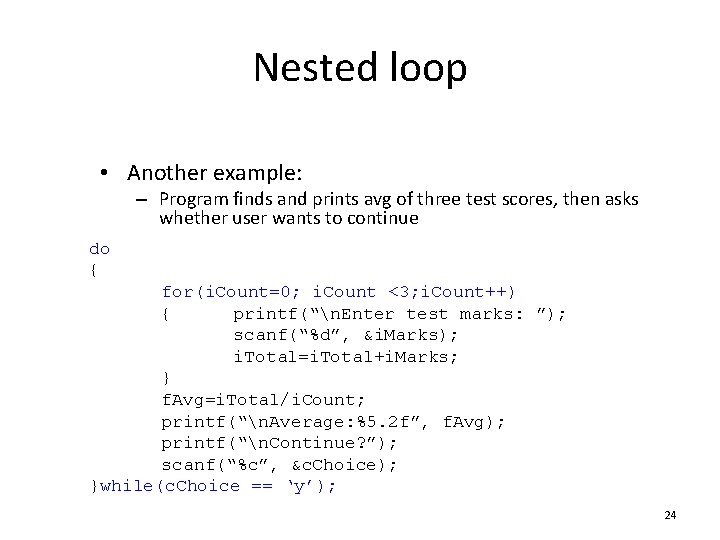
Nested loop • Another example: – Program finds and prints avg of three test scores, then asks whether user wants to continue do { for(i. Count=0; i. Count <3; i. Count++) { printf(“n. Enter test marks: ”); scanf(“%d”, &i. Marks); i. Total=i. Total+i. Marks; } f. Avg=i. Total/i. Count; printf(“n. Average: %5. 2 f”, f. Avg); printf(“n. Continue? ”); scanf(“%c”, &c. Choice); }while(c. Choice == ‘y’); 24
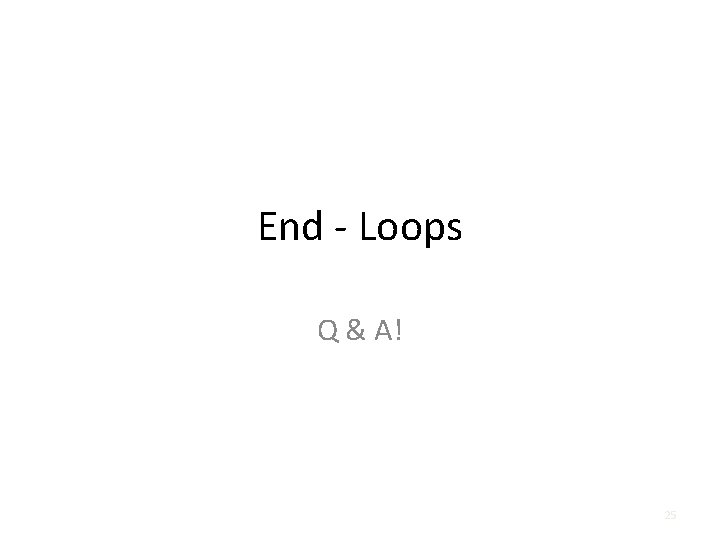
End - Loops Q & A! 25