Java util Arrays class Arrays Useful methods Use
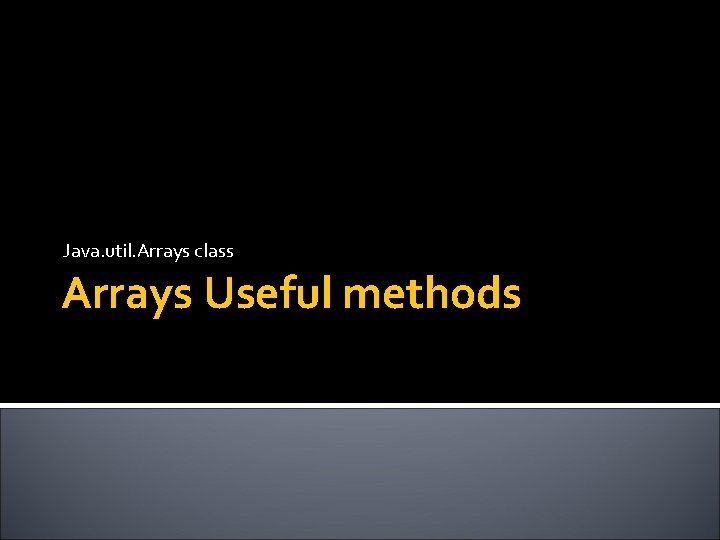
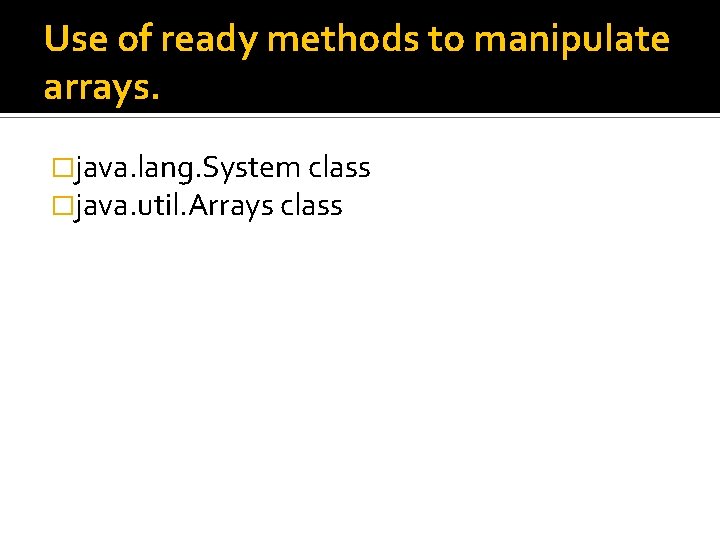
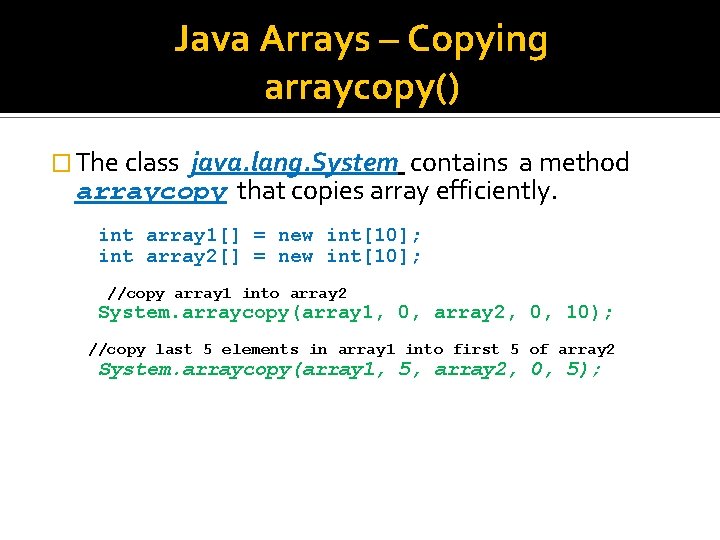
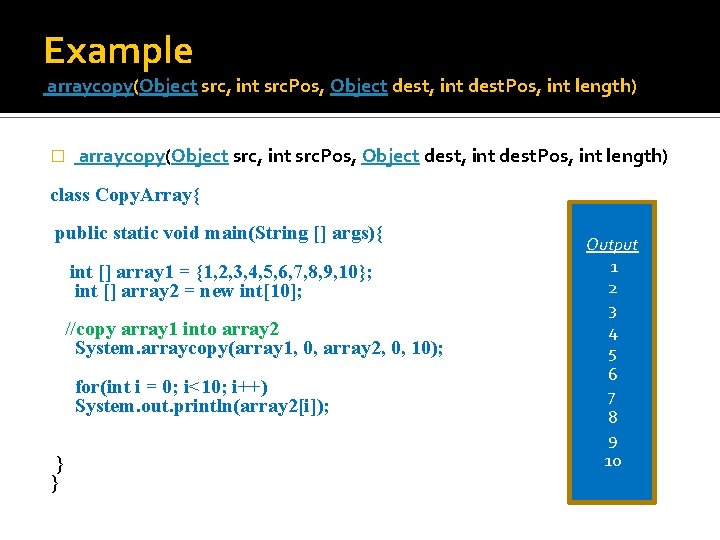
![Example class Copy. Array{ public static void main(String [] args){ int [] array 1 Example class Copy. Array{ public static void main(String [] args){ int [] array 1](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-5.jpg)
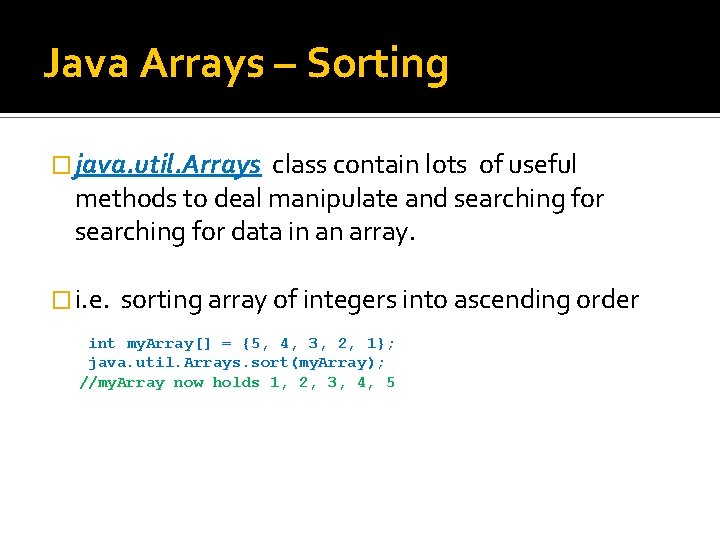
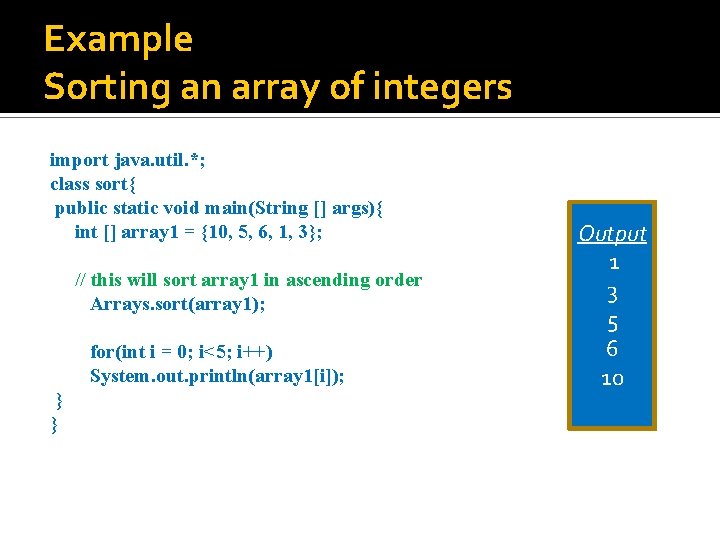
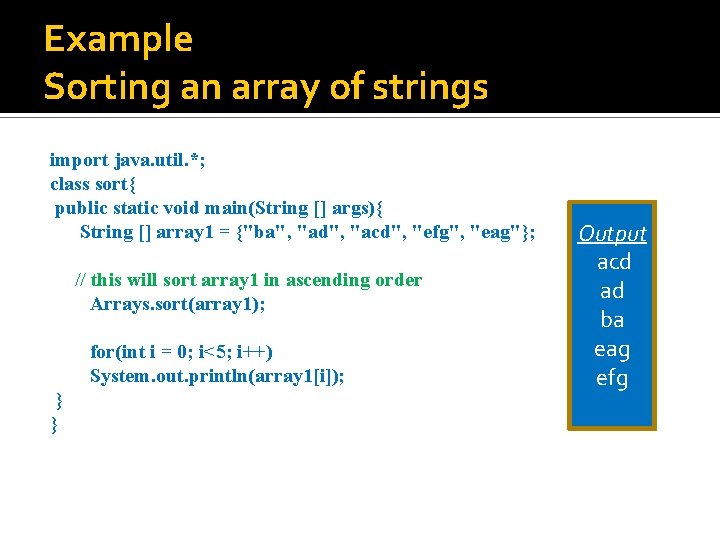
![Java Arrays – fill int my. Array[] = new int[5]; java. util. Arrays. fill(my. Java Arrays – fill int my. Array[] = new int[5]; java. util. Arrays. fill(my.](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-9.jpg)
![Example import java. util. *; class Copy. Array{ public static void main(String [] args){ Example import java. util. *; class Copy. Array{ public static void main(String [] args){](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-10.jpg)
![Example import java. util. *; class Copy. Array{ public static void main(String [] args){ Example import java. util. *; class Copy. Array{ public static void main(String [] args){](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-11.jpg)
![Java Arrays – binary search int my. Array[] = {1, 2, 3, 4, 5, Java Arrays – binary search int my. Array[] = {1, 2, 3, 4, 5,](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-12.jpg)
![Example binary. Search(Object[] a, Object key) import java. util. *; class binarysearch{ public static Example binary. Search(Object[] a, Object key) import java. util. *; class binarysearch{ public static](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-13.jpg)
![Example binary. Search(Object[] a, int from. Index, int to. Index, Object key) Import java. Example binary. Search(Object[] a, int from. Index, int to. Index, Object key) Import java.](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-14.jpg)
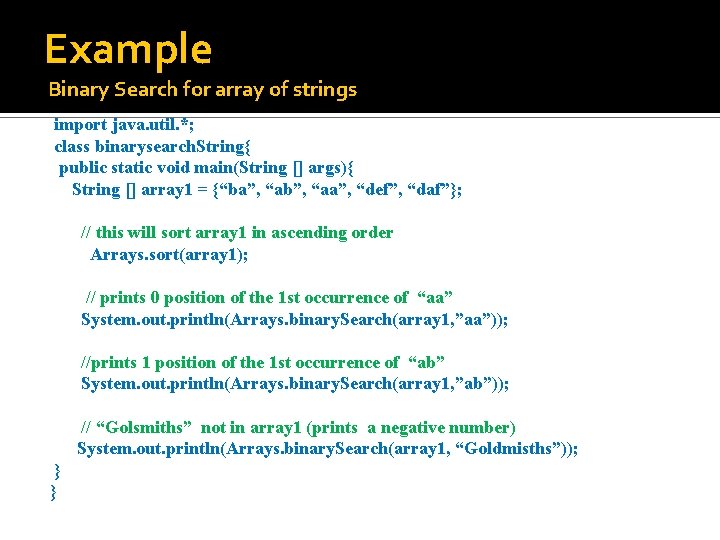
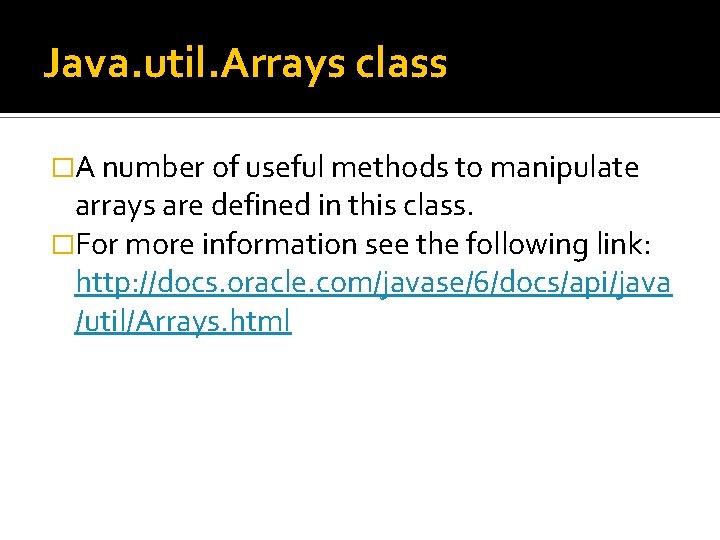
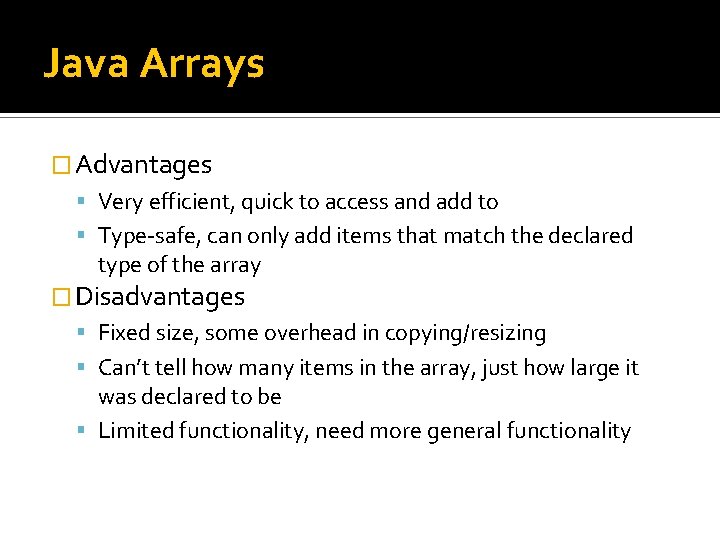
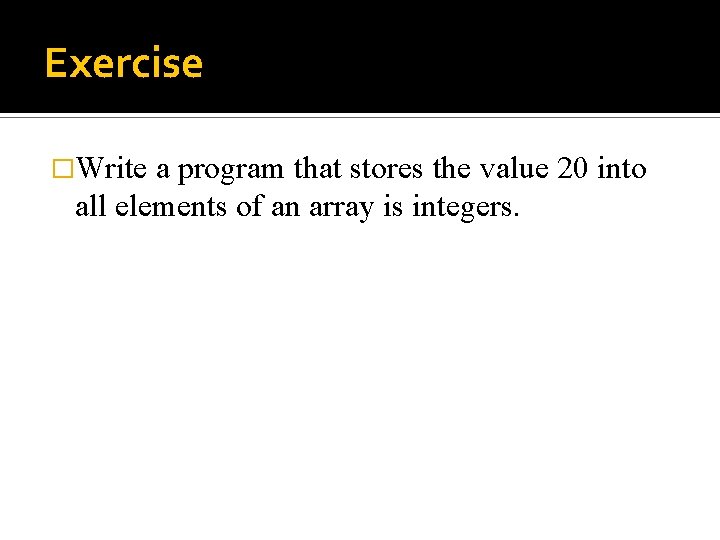
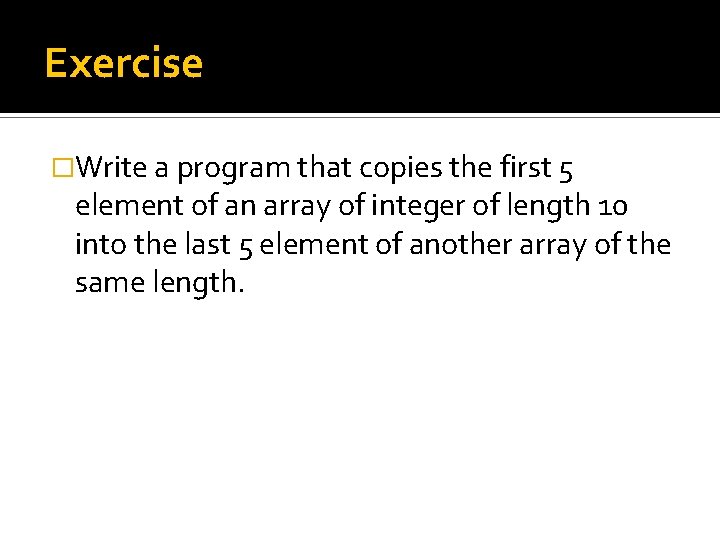
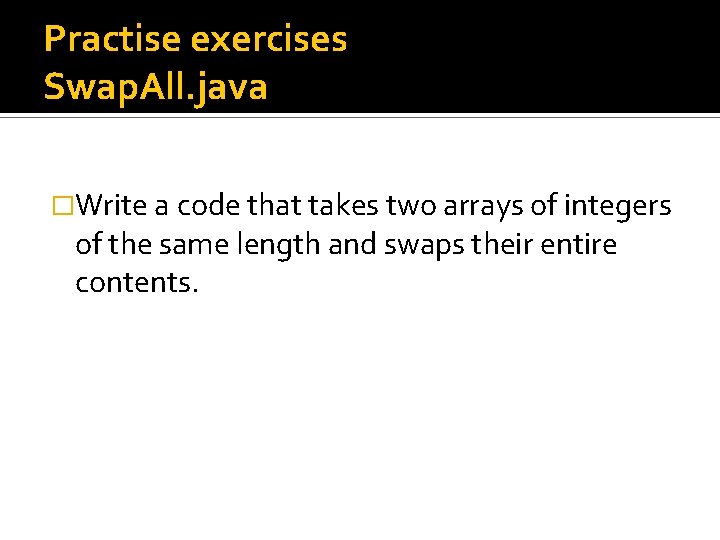
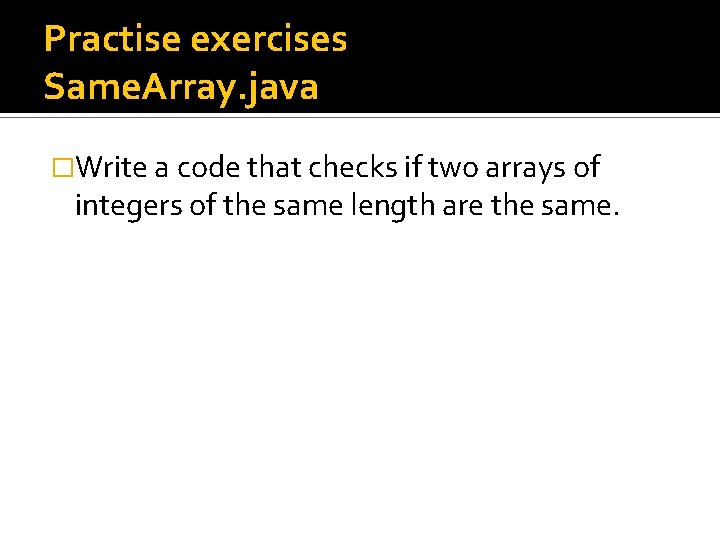
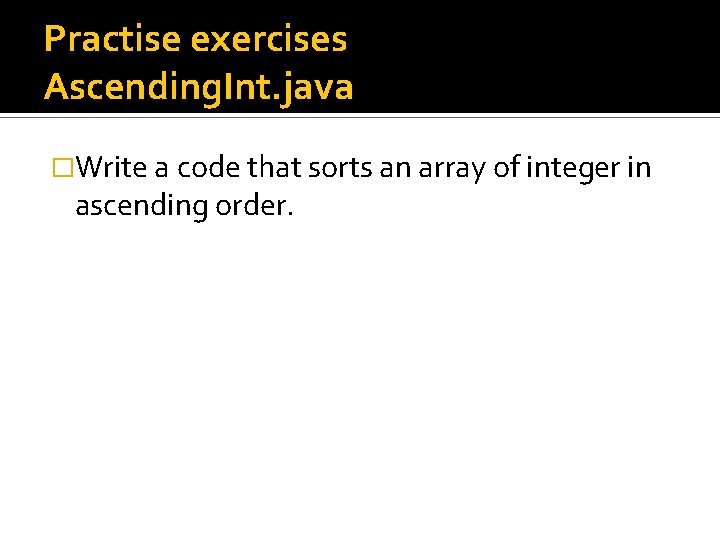
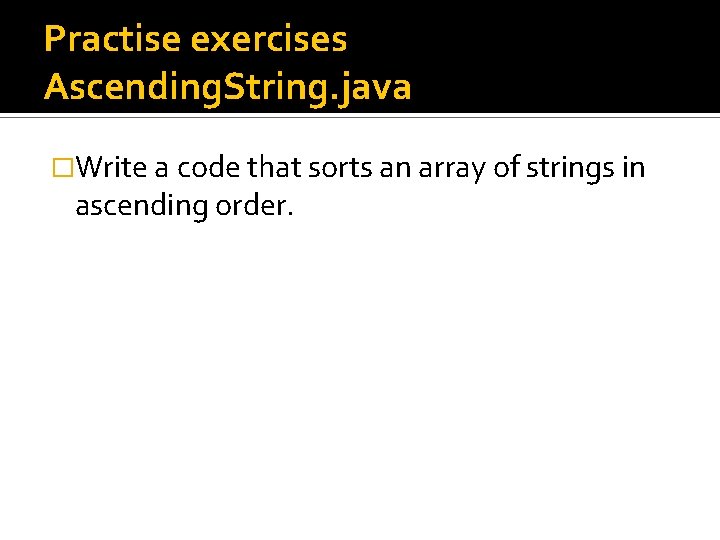
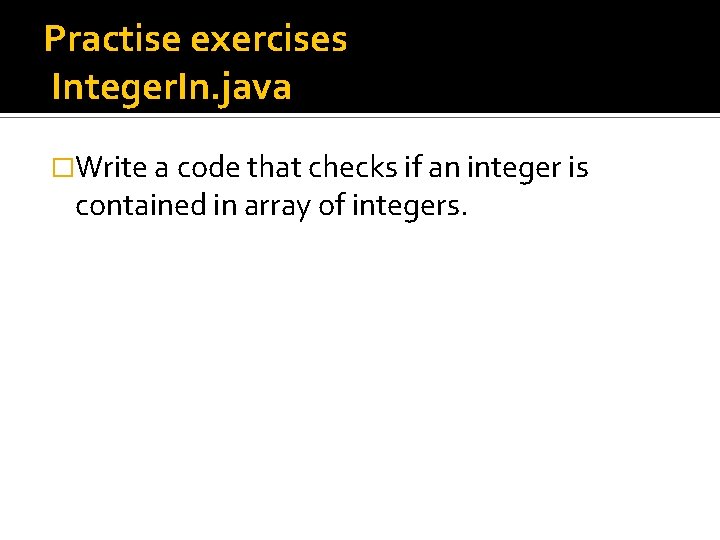
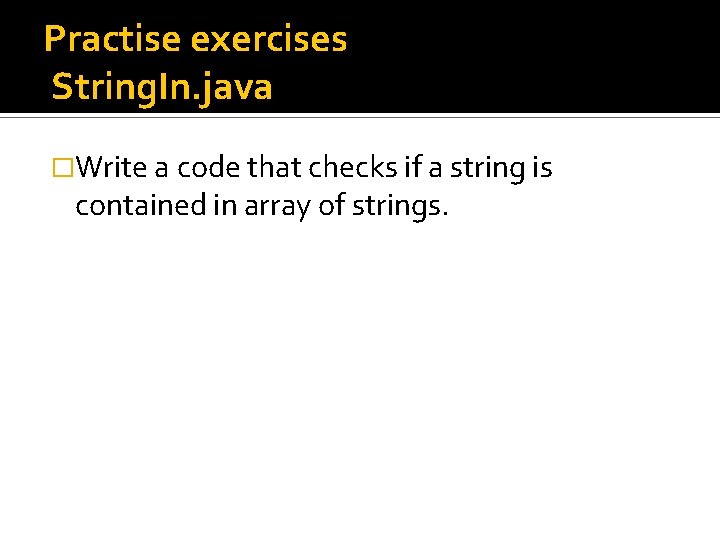
![Search an array of strings �Given the following declaration � String[] cities = {"Washington", Search an array of strings �Given the following declaration � String[] cities = {"Washington",](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-26.jpg)
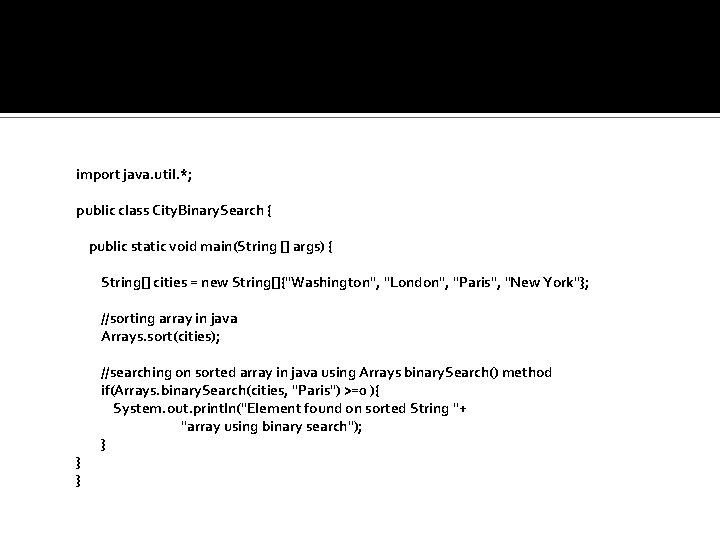
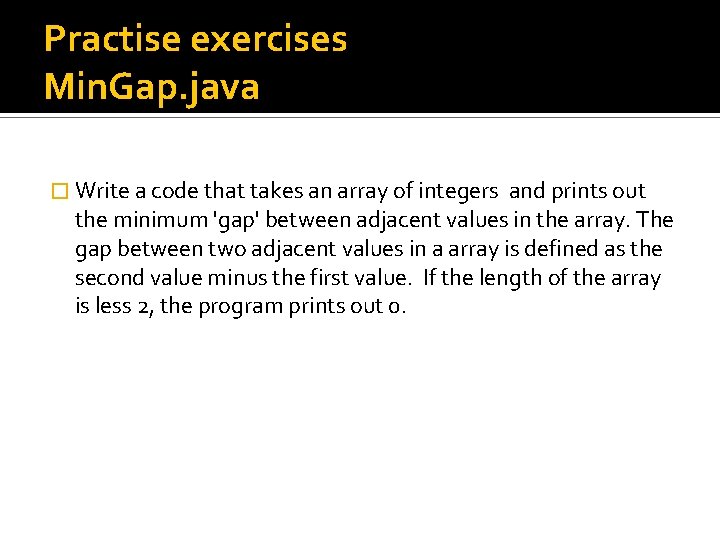
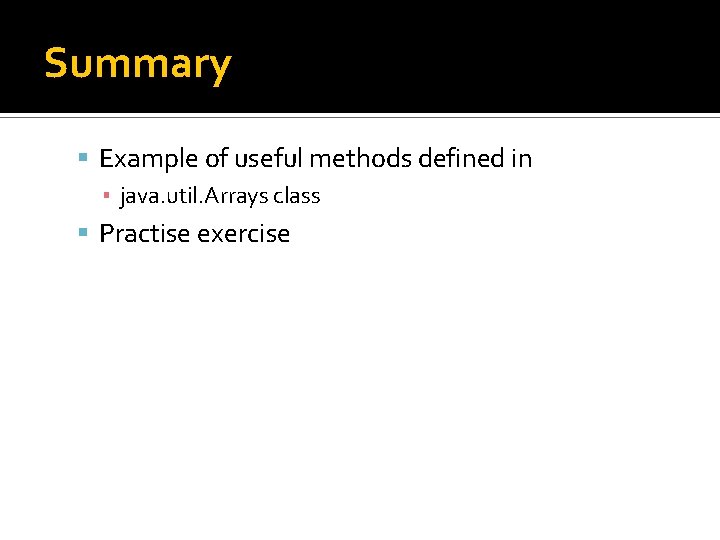
- Slides: 29
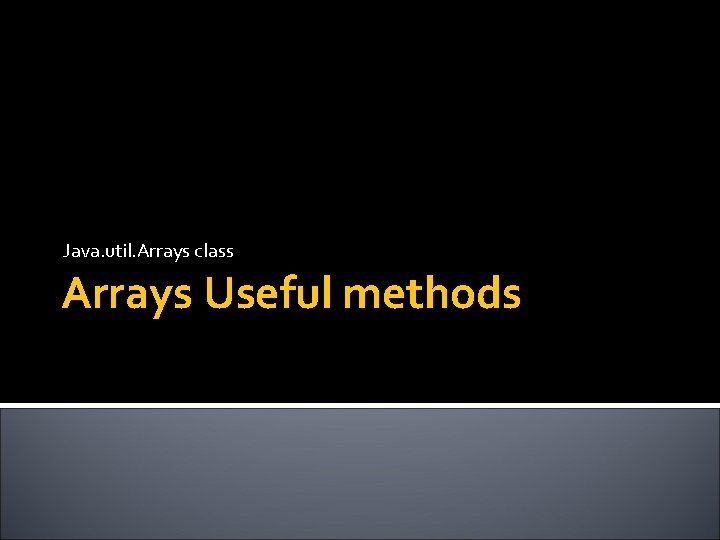
Java. util. Arrays class Arrays Useful methods
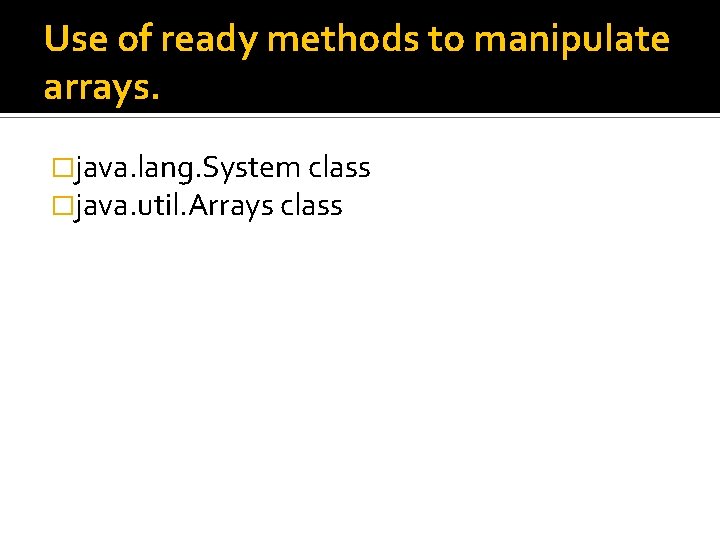
Use of ready methods to manipulate arrays. �java. lang. System class �java. util. Arrays class
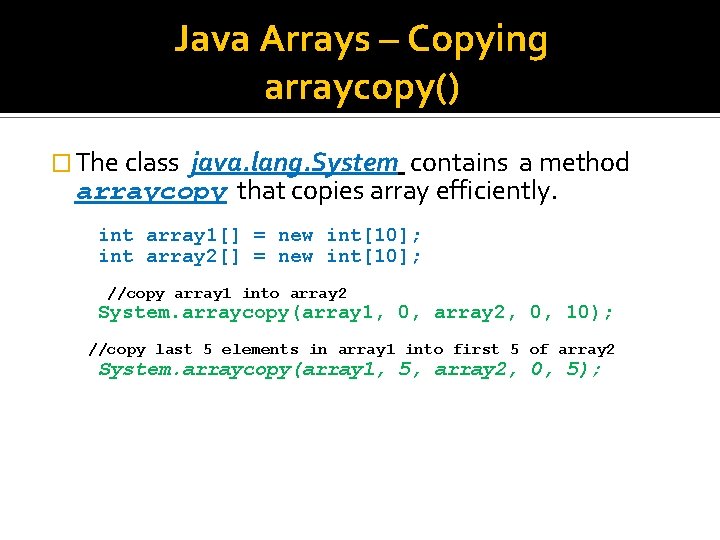
Java Arrays – Copying arraycopy() � The class java. lang. System contains a method arraycopy that copies array efficiently. int array 1[] = new int[10]; int array 2[] = new int[10]; //copy array 1 into array 2 System. arraycopy(array 1, 0, array 2, 0, 10); //copy last 5 elements in array 1 into first 5 of array 2 System. arraycopy(array 1, 5, array 2, 0, 5);
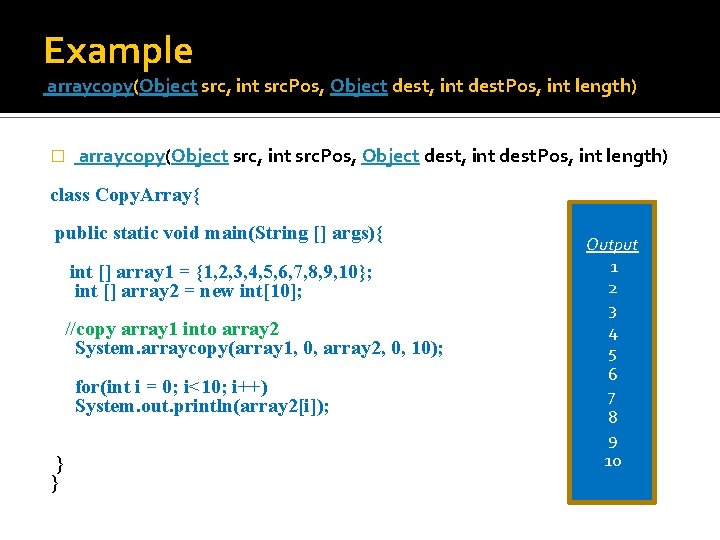
Example arraycopy(Object src, int src. Pos, Object dest, int dest. Pos, int length) � arraycopy(Object src, int src. Pos, Object dest, int dest. Pos, int length) class Copy. Array{ public static void main(String [] args){ int [] array 1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int [] array 2 = new int[10]; //copy array 1 into array 2 System. arraycopy(array 1, 0, array 2, 0, 10); for(int i = 0; i<10; i++) System. out. println(array 2[i]); } } Output 1 2 3 4 5 6 7 8 9 10
![Example class Copy Array public static void mainString args int array 1 Example class Copy. Array{ public static void main(String [] args){ int [] array 1](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-5.jpg)
Example class Copy. Array{ public static void main(String [] args){ int [] array 1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int [] array 2 = new int[10]; //copy array 1 into array 2 System. arraycopy(array 1, 0, array 2, 0, 10); //copy last 5 elements in array 1 into first 5 of array 2 System. arraycopy(array 1, 5, array 2, 0, 5); for(int i = 0; i<10; i++) System. out. println(array 2[i]); } } Output 6 7 8 9 10
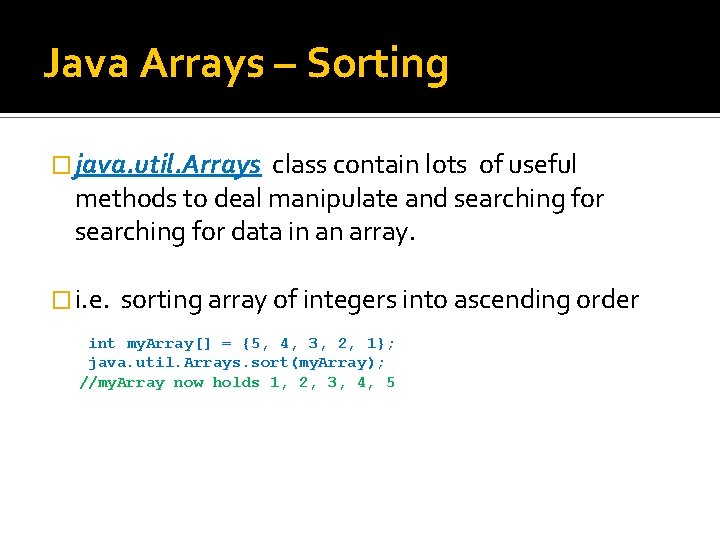
Java Arrays – Sorting � java. util. Arrays class contain lots of useful methods to deal manipulate and searching for data in an array. � i. e. sorting array of integers into ascending order int my. Array[] = {5, 4, 3, 2, 1}; java. util. Arrays. sort(my. Array); //my. Array now holds 1, 2, 3, 4, 5
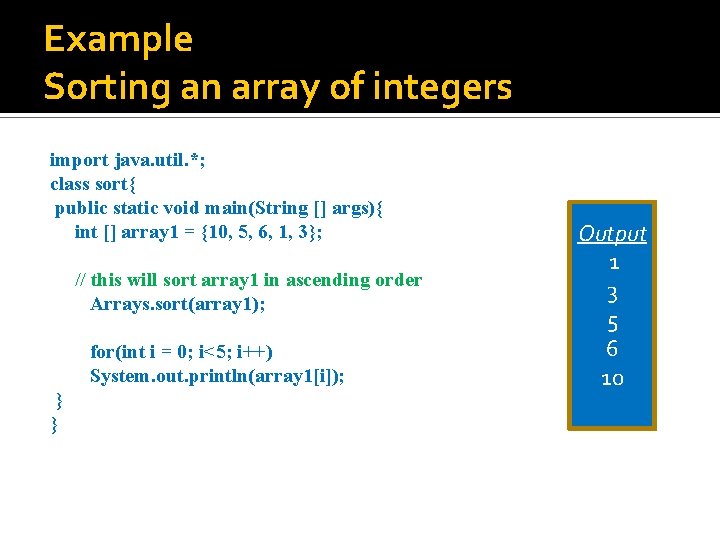
Example Sorting an array of integers import java. util. *; class sort{ public static void main(String [] args){ int [] array 1 = {10, 5, 6, 1, 3}; // this will sort array 1 in ascending order Arrays. sort(array 1); for(int i = 0; i<5; i++) System. out. println(array 1[i]); } } Output 1 3 5 6 10
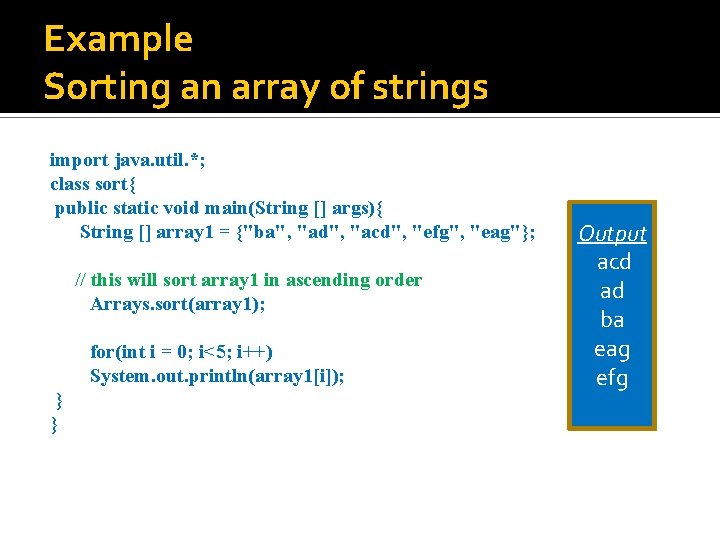
Example Sorting an array of strings import java. util. *; class sort{ public static void main(String [] args){ String [] array 1 = {"ba", "ad", "acd", "efg", "eag"}; // this will sort array 1 in ascending order Arrays. sort(array 1); for(int i = 0; i<5; i++) System. out. println(array 1[i]); } } Output acd ad ba eag efg
![Java Arrays fill int my Array new int5 java util Arrays fillmy Java Arrays – fill int my. Array[] = new int[5]; java. util. Arrays. fill(my.](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-9.jpg)
Java Arrays – fill int my. Array[] = new int[5]; java. util. Arrays. fill(my. Array, 10); //my. Array now holds 10, 10, 10 java. util. Arrays. fill(my. Array, 1, 3, 20); //my. Array now holds 10, 20, 10
![Example import java util class Copy Array public static void mainString args Example import java. util. *; class Copy. Array{ public static void main(String [] args){](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-10.jpg)
Example import java. util. *; class Copy. Array{ public static void main(String [] args){ int [] array 1 = new int[5] //fill array 1 the value 10 Arrays. fill(array 1, 10); for(int i = 0; i<5; i++) System. out. println(array 1[i]); } } Output 10 10 10
![Example import java util class Copy Array public static void mainString args Example import java. util. *; class Copy. Array{ public static void main(String [] args){](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-11.jpg)
Example import java. util. *; class Copy. Array{ public static void main(String [] args){ int [] array 1 = new int[5] //fill array 1 the value 10 Arrays. fill(array 1, 10); //fill array 1 from the position the indetx 1 to 2(3 -1) with the value 10 Arrays. fill(array 1, 1, 3, 20); for(int i = 0; i<5; i++) System. out. println(array 1[i]); } } Output 10 20 20 10 10
![Java Arrays binary search int my Array 1 2 3 4 5 Java Arrays – binary search int my. Array[] = {1, 2, 3, 4, 5,](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-12.jpg)
Java Arrays – binary search int my. Array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10} java. util. Arrays. binarysearch(my. Array, 10); //my. Array now holds 10, 10, 10
![Example binary SearchObject a Object key import java util class binarysearch public static Example binary. Search(Object[] a, Object key) import java. util. *; class binarysearch{ public static](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-13.jpg)
Example binary. Search(Object[] a, Object key) import java. util. *; class binarysearch{ public static void main(String [] args){ int [] array 1 = {10, 5, 6, 1, 3}; // this will sort array 1 in ascending order Arrays. sort(array 1); // prints 0 position of the 1 st occurrence of 1 System. out. println(Arrays. binary. Search(array 1, 1)); //prints 1 position of the 1 st occurrence of 3 System. out. println(Arrays. binary. Search(array 1, 3)); // 20 does not exists (prints a negative number) System. out. println(Arrays. binary. Search(array 1, 20)); } }
![Example binary SearchObject a int from Index int to Index Object key Import java Example binary. Search(Object[] a, int from. Index, int to. Index, Object key) Import java.](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-14.jpg)
Example binary. Search(Object[] a, int from. Index, int to. Index, Object key) Import java. util. *; class binarysearch{ public static void main(String [] args){ int [] array 1 = {10, 5, 6, 1, 3}; // this will sort array 1 in ascending order Arrays. sort(array 1); // prints 4 position of the 1 st occurrence of 10 System. out. println(Arrays. binary. Search(array 1, 0, 5, 10)); //searching from position 1 to 3 positions System. out. println(Arrays. binary. Search(array 1, 1, 4, 10)); } } Output 4 -4
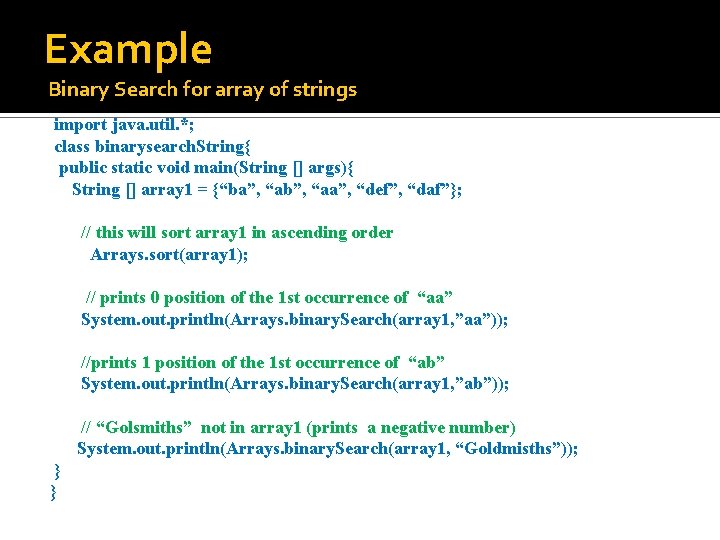
Example Binary Search for array of strings import java. util. *; class binarysearch. String{ public static void main(String [] args){ String [] array 1 = {“ba”, “ab”, “aa”, “def”, “daf”}; // this will sort array 1 in ascending order Arrays. sort(array 1); // prints 0 position of the 1 st occurrence of “aa” System. out. println(Arrays. binary. Search(array 1, ”aa”)); //prints 1 position of the 1 st occurrence of “ab” System. out. println(Arrays. binary. Search(array 1, ”ab”)); // “Golsmiths” not in array 1 (prints a negative number) System. out. println(Arrays. binary. Search(array 1, “Goldmisths”)); } }
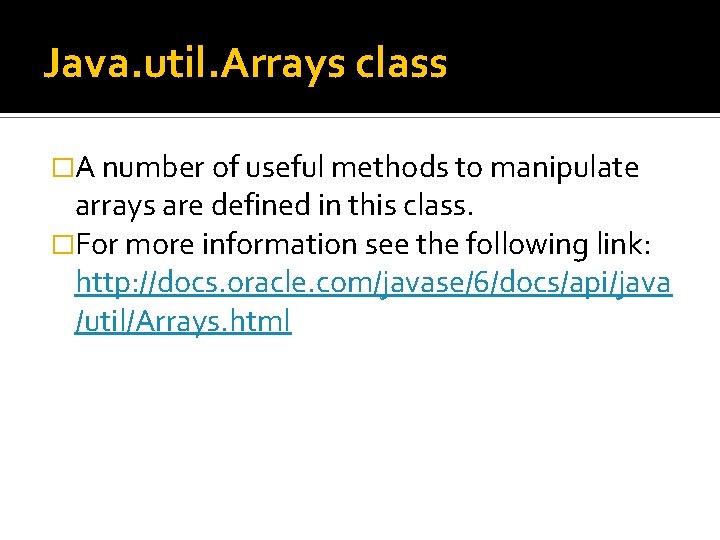
Java. util. Arrays class �A number of useful methods to manipulate arrays are defined in this class. �For more information see the following link: http: //docs. oracle. com/javase/6/docs/api/java /util/Arrays. html
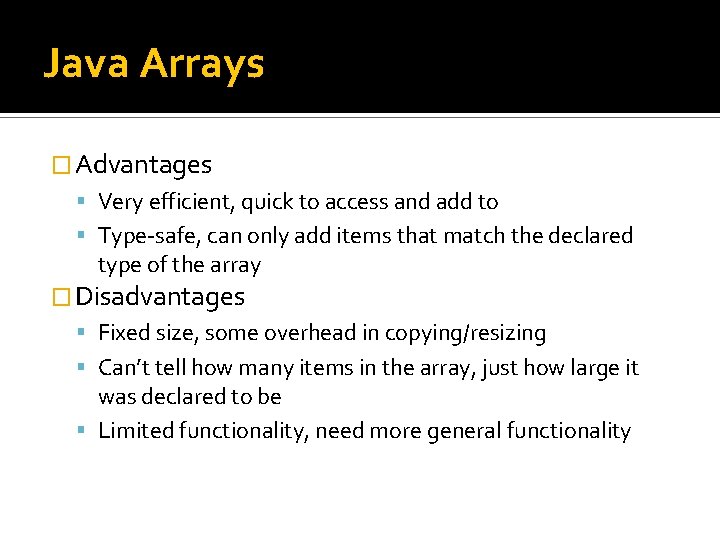
Java Arrays � Advantages Very efficient, quick to access and add to Type-safe, can only add items that match the declared type of the array � Disadvantages Fixed size, some overhead in copying/resizing Can’t tell how many items in the array, just how large it was declared to be Limited functionality, need more general functionality
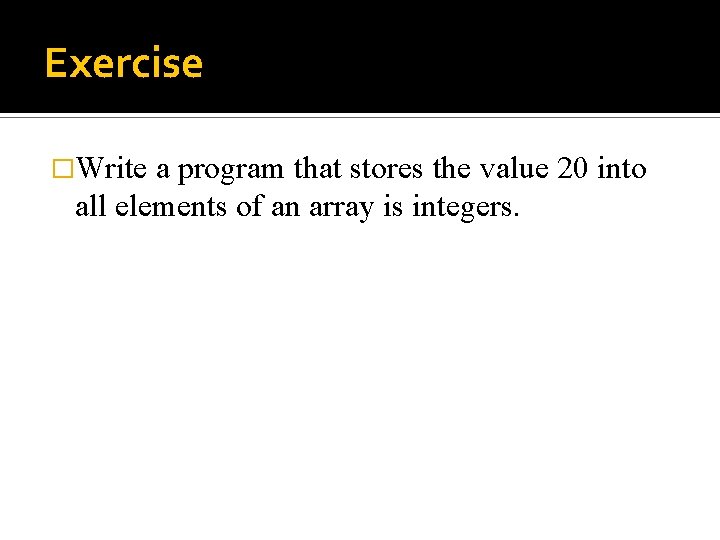
Exercise �Write a program that stores the value 20 into all elements of an array is integers.
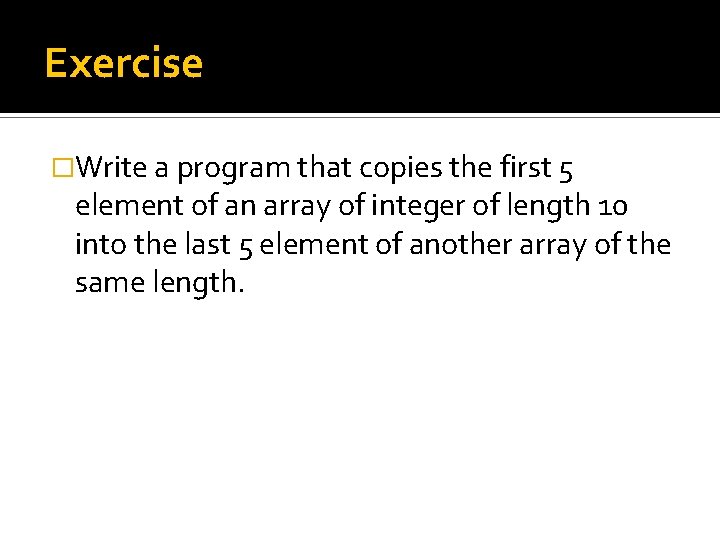
Exercise �Write a program that copies the first 5 element of an array of integer of length 10 into the last 5 element of another array of the same length.
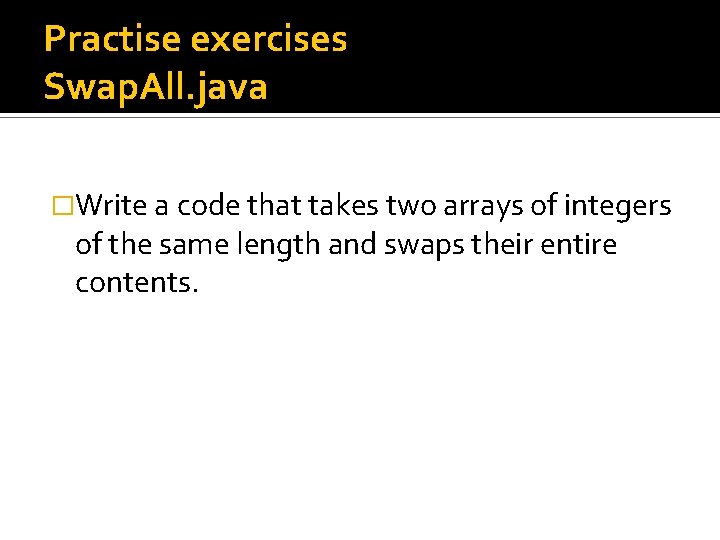
Practise exercises Swap. All. java �Write a code that takes two arrays of integers of the same length and swaps their entire contents.
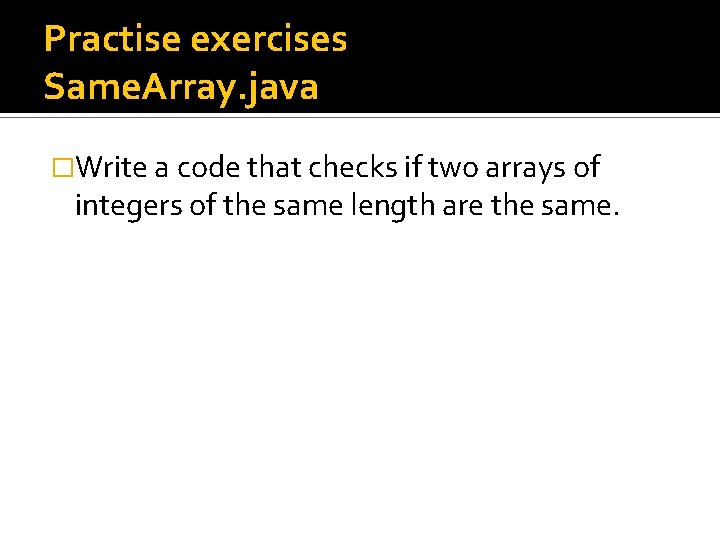
Practise exercises Same. Array. java �Write a code that checks if two arrays of integers of the same length are the same.
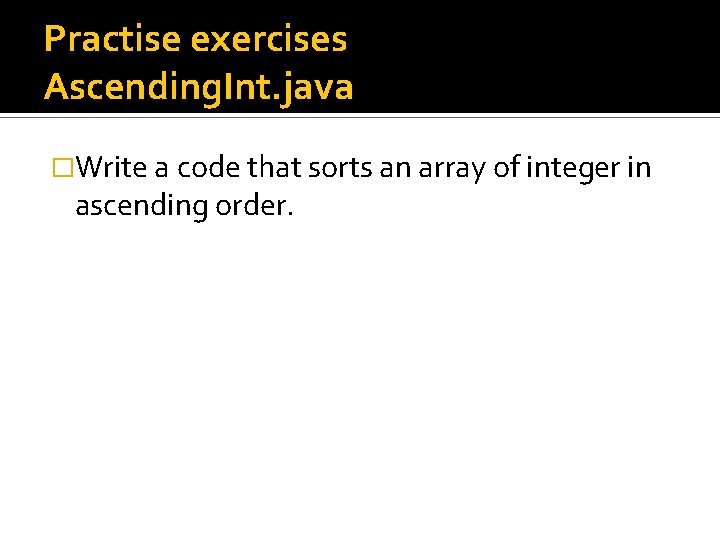
Practise exercises Ascending. Int. java �Write a code that sorts an array of integer in ascending order.
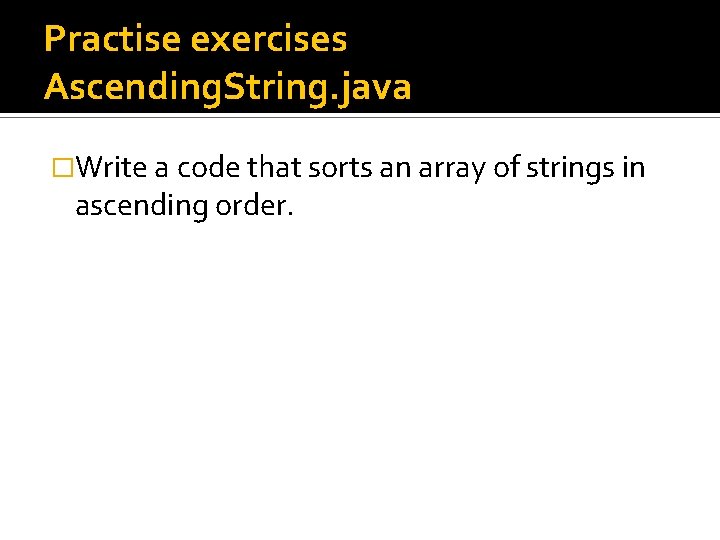
Practise exercises Ascending. String. java �Write a code that sorts an array of strings in ascending order.
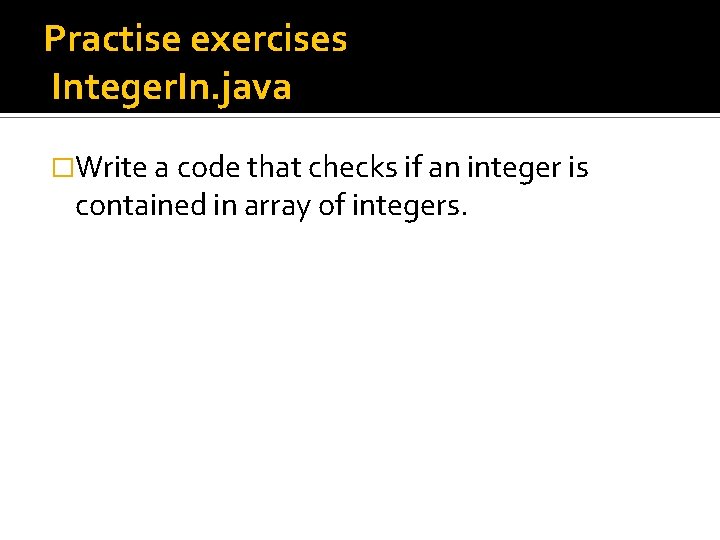
Practise exercises Integer. In. java �Write a code that checks if an integer is contained in array of integers.
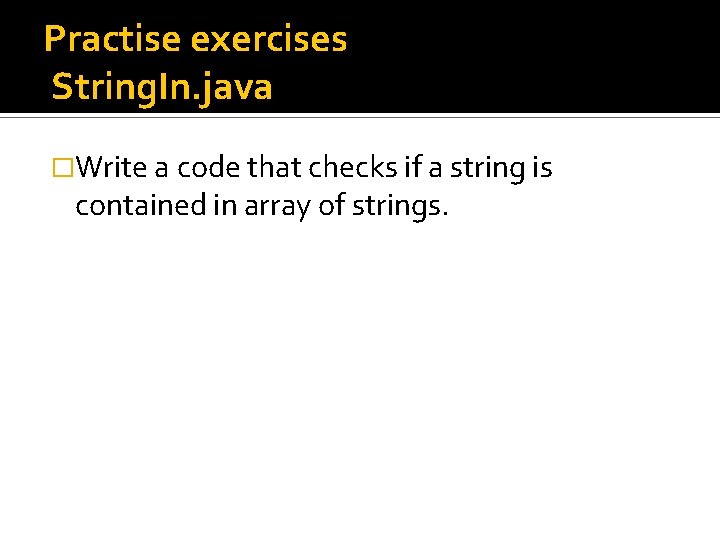
Practise exercises String. In. java �Write a code that checks if a string is contained in array of strings.
![Search an array of strings Given the following declaration String cities Washington Search an array of strings �Given the following declaration � String[] cities = {"Washington",](https://slidetodoc.com/presentation_image_h2/b465282910fe9a94d1c37f56ab3f0153/image-26.jpg)
Search an array of strings �Given the following declaration � String[] cities = {"Washington", "London", "Paris", "New York"}; �Write a program that uses a binary search to search for a particular city is contained in the array cities.
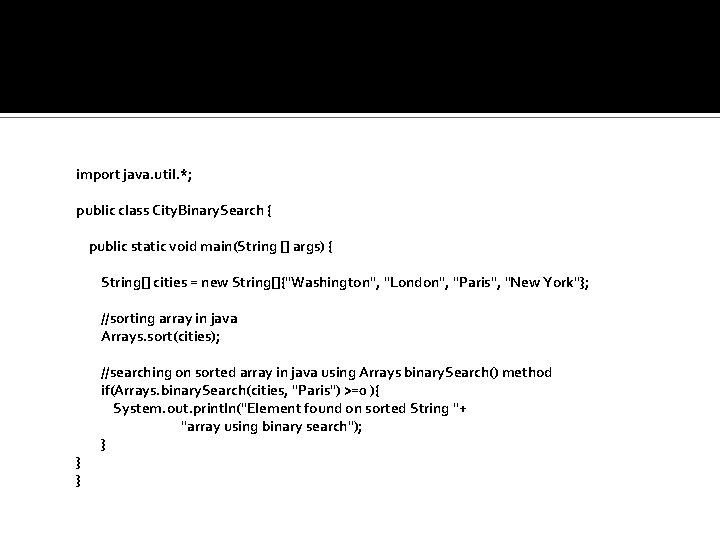
import java. util. *; public class City. Binary. Search { public static void main(String [] args) { String[] cities = new String[]{"Washington", "London", "Paris", "New York"}; //sorting array in java Arrays. sort(cities); } } //searching on sorted array in java using Arrays binary. Search() method if(Arrays. binary. Search(cities, "Paris") >=0 ){ System. out. println("Element found on sorted String "+ "array using binary search"); }
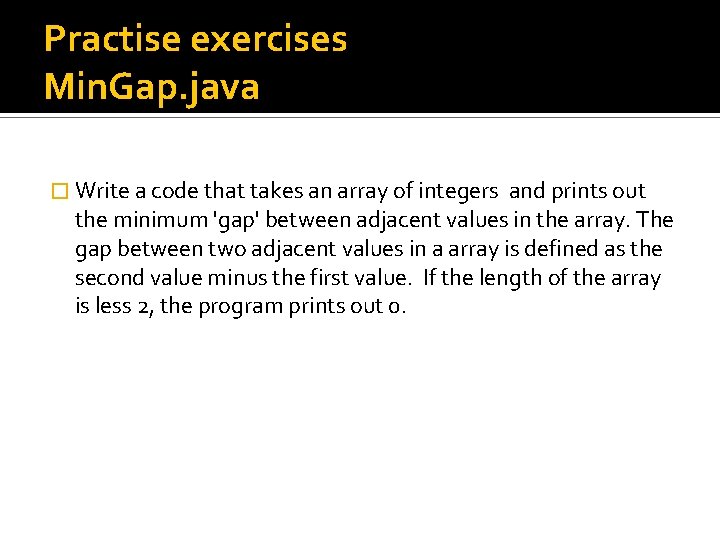
Practise exercises Min. Gap. java � Write a code that takes an array of integers and prints out the minimum 'gap' between adjacent values in the array. The gap between two adjacent values in a array is defined as the second value minus the first value. If the length of the array is less 2, the program prints out 0.
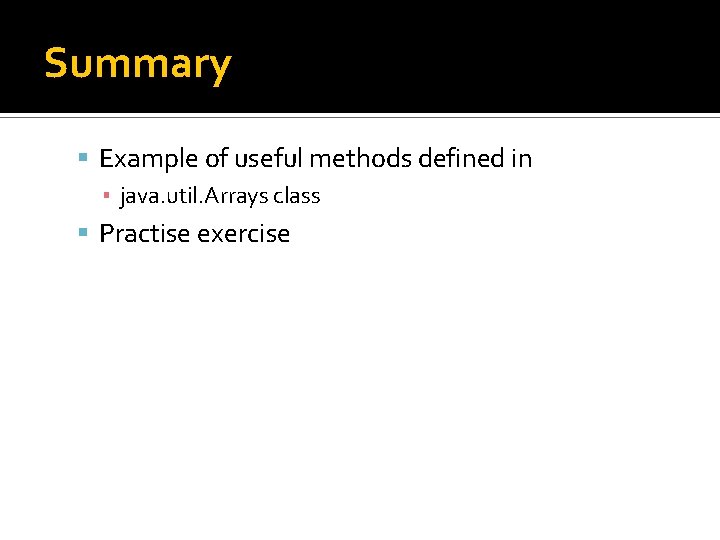
Summary Example of useful methods defined in ▪ java. util. Arrays class Practise exercise
Java import java.util.*
Java import java.util.*
Java import util
Java
Import java.util.*
Java.util.random
Public class
Import java.util.* class test collection 11
Import java.util.* class vector
Parallel arrays python
Variables unidimensionales ejemplos
Arreglos bidimensionales en java
Import java.util.stringtokenizer;
Import java.util.stringtokenizer;
Java.util.io.*
Import java.util."; public
Import java.io.*;
Javajava
Import java.util.random;
Java static import
Import java.util.string
When a material useful
Mole background
Class evidence can have probative value
Wax pattern in dentistry
Mutator and accessor methods in java
Mutator and accessor methods in java
Mutator and accessor methods in java
Java string methods
User-defined methods in java