Java Programming Language Chapter 2 Primitive Data Type
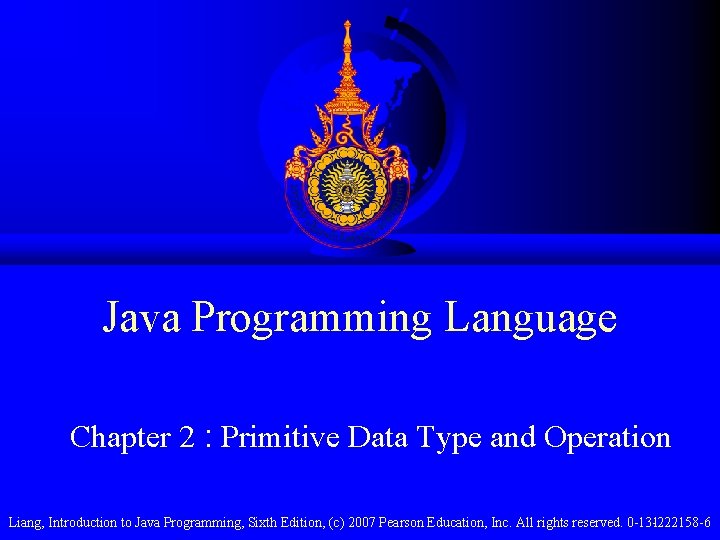
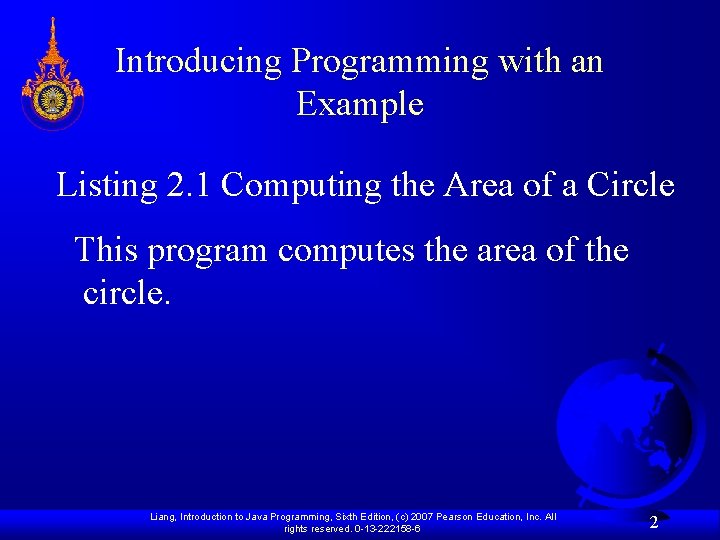
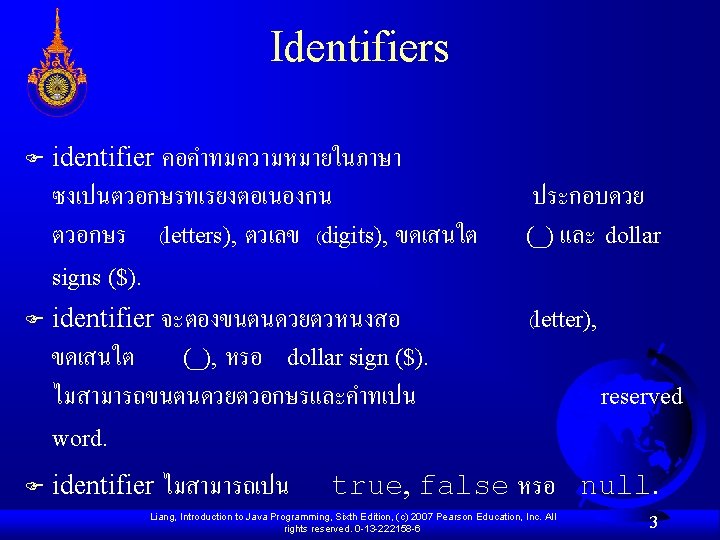
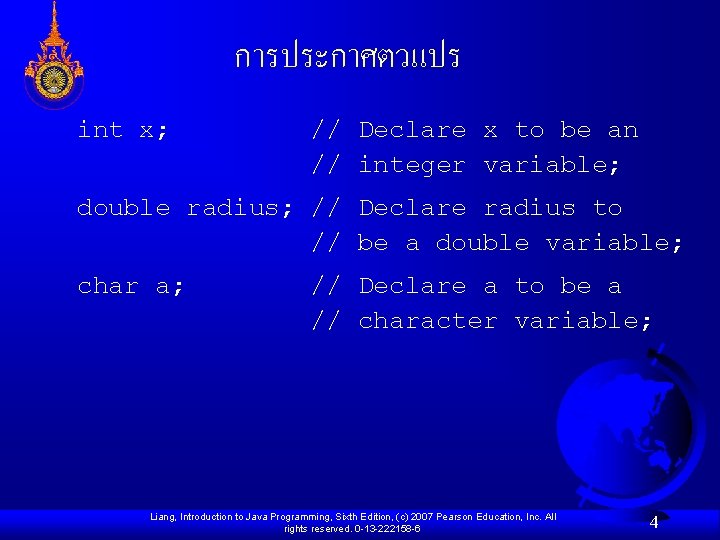
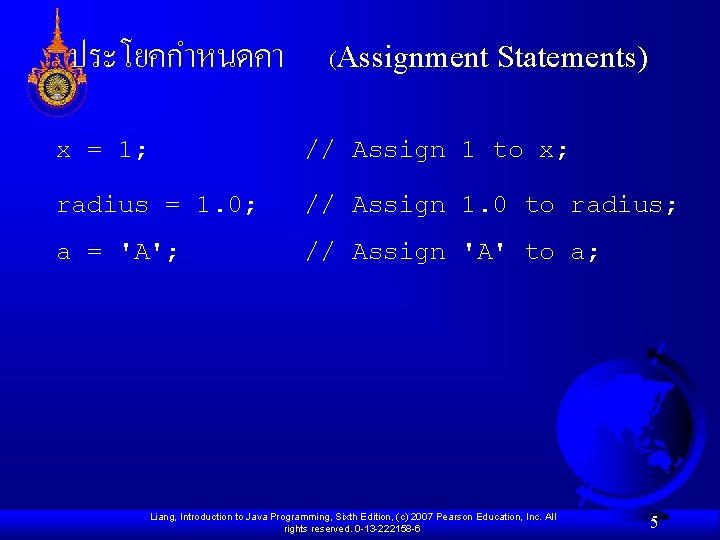
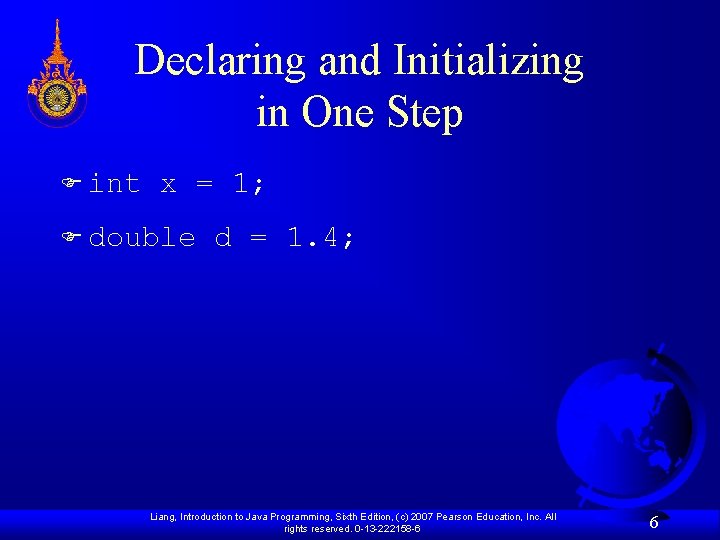
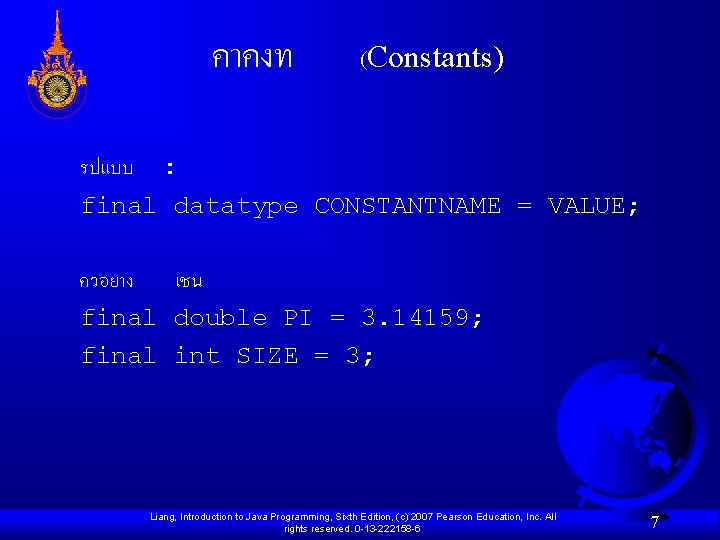
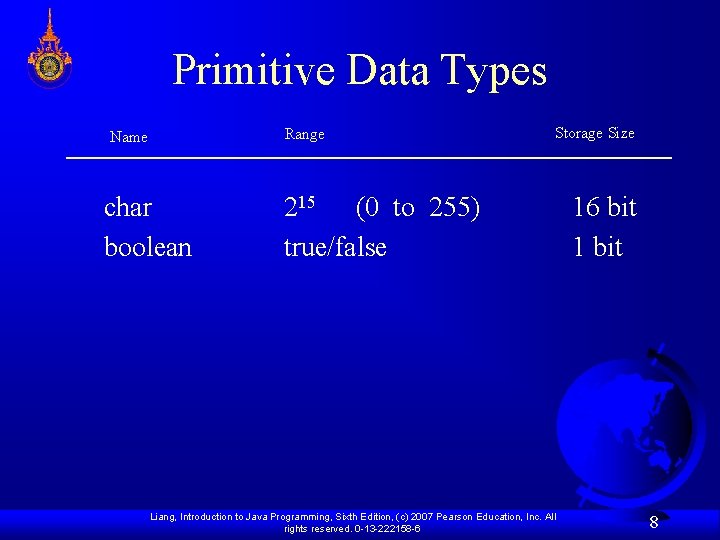
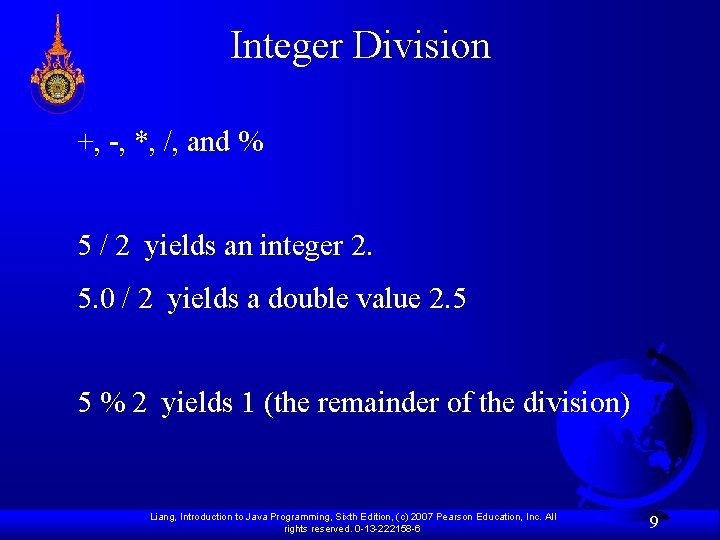
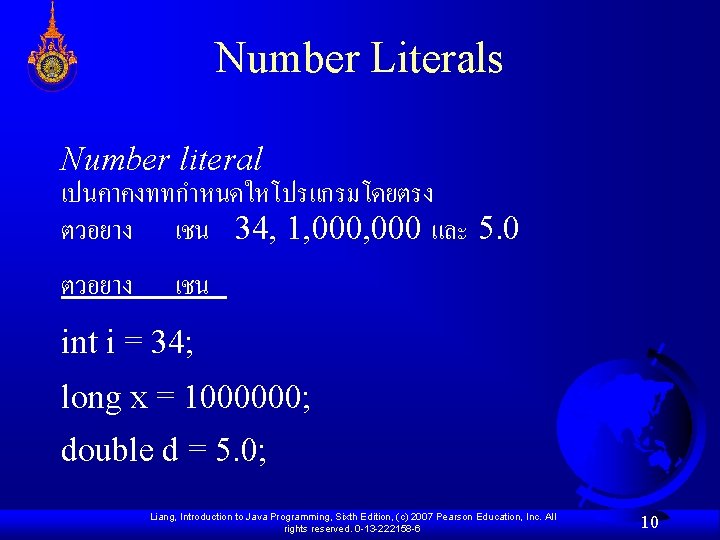
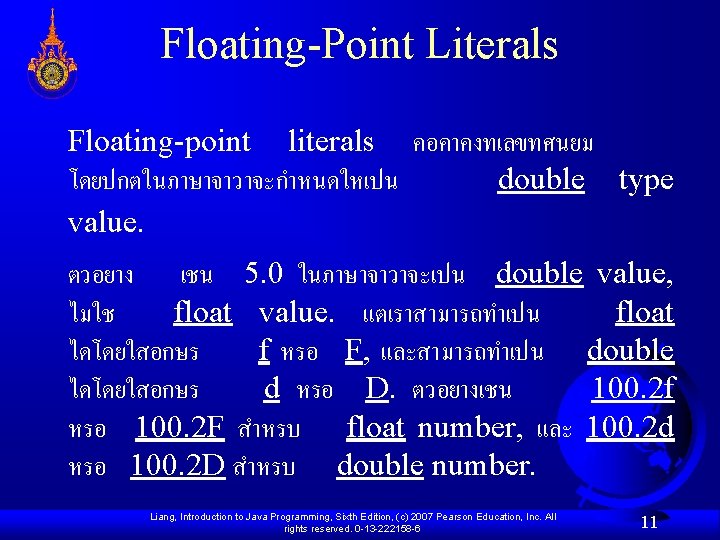
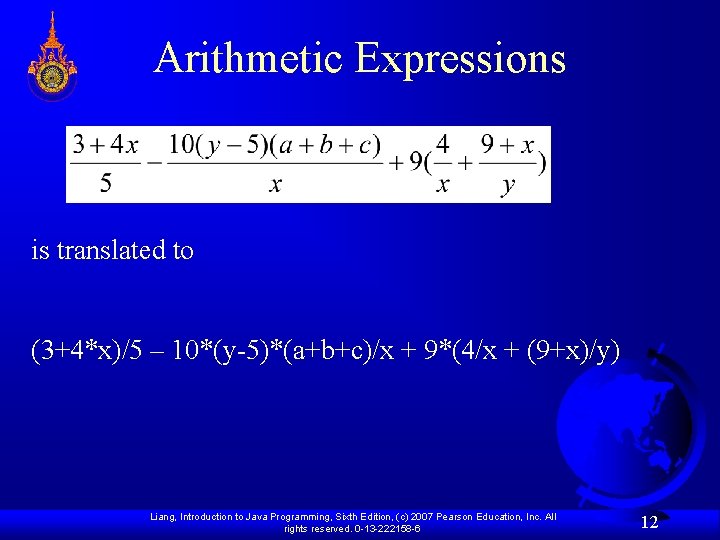
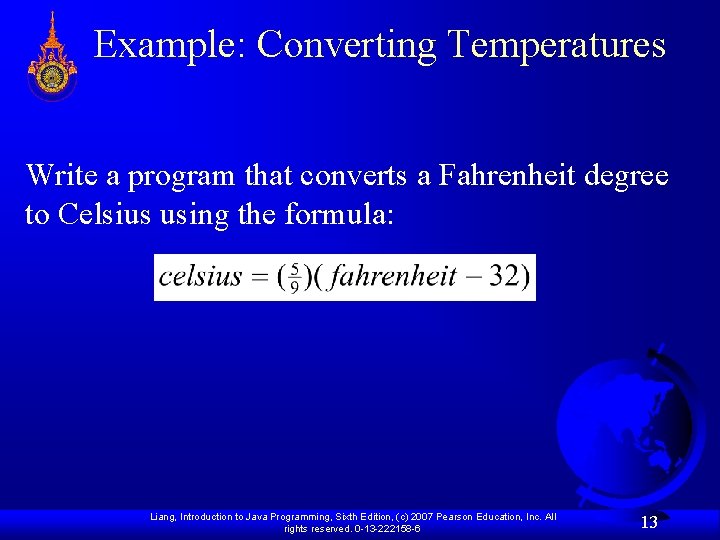
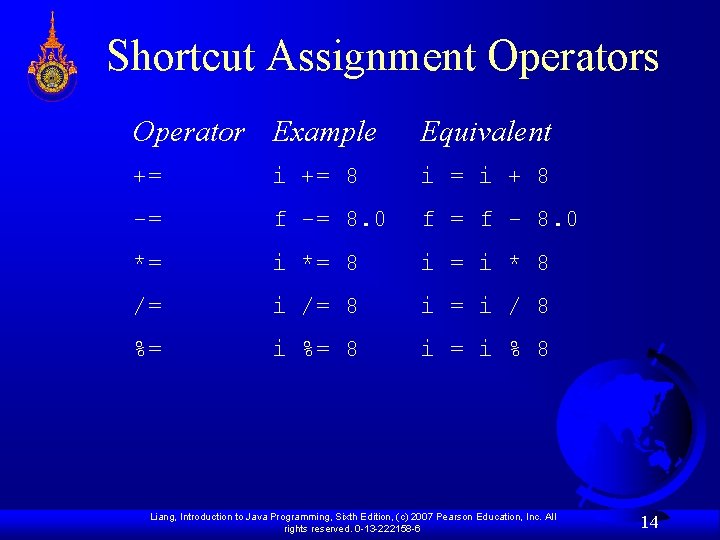
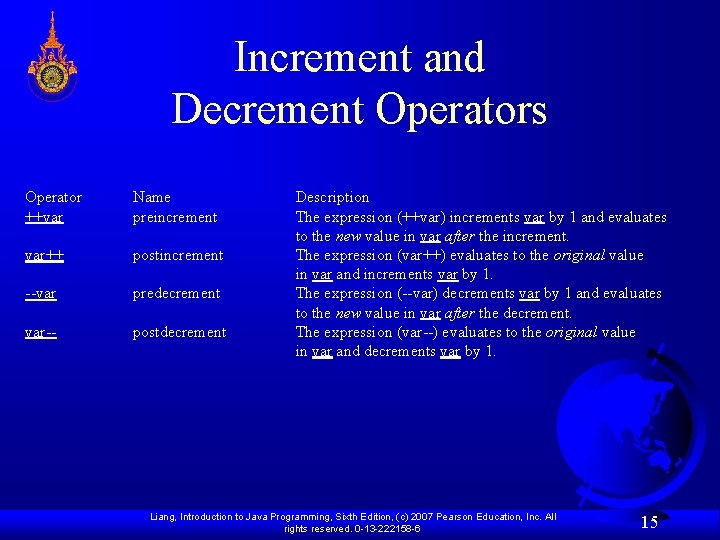
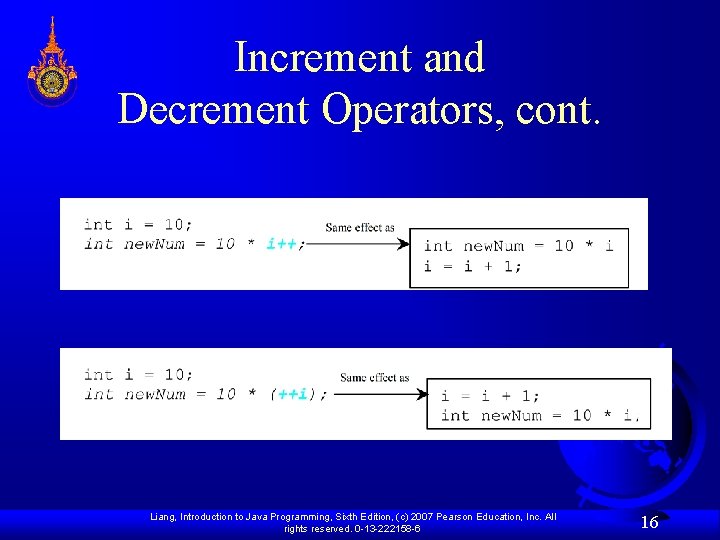
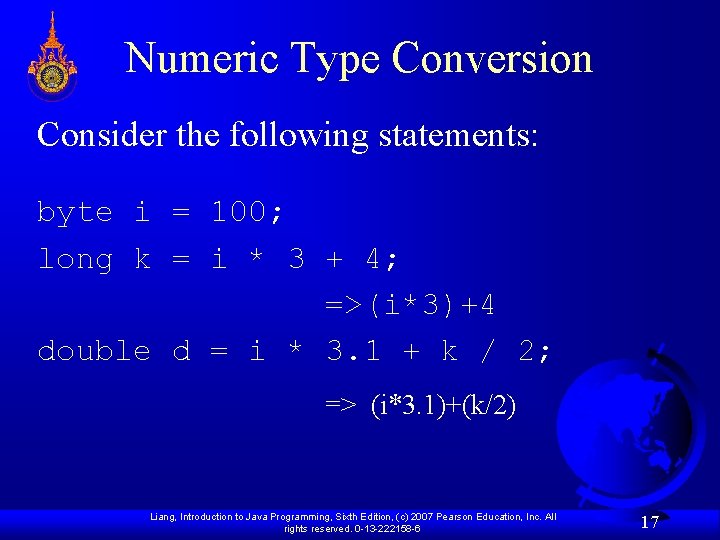
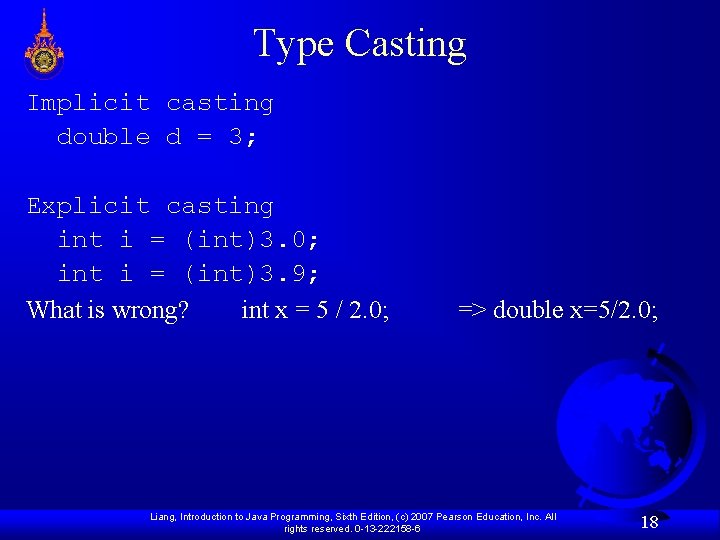
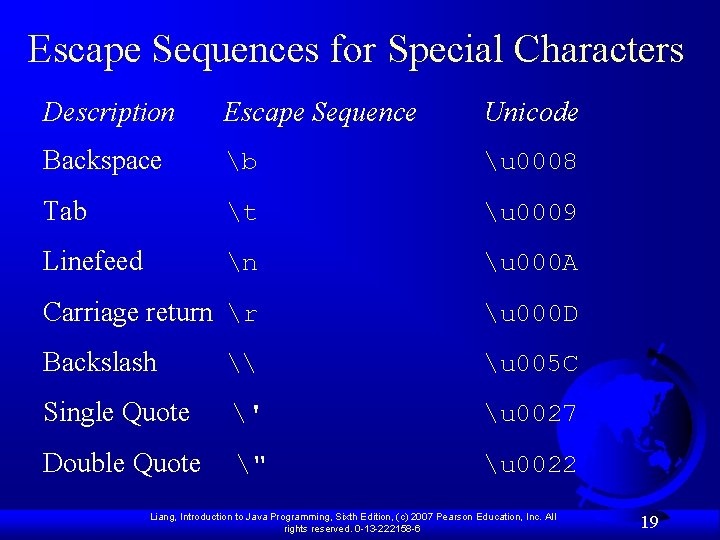
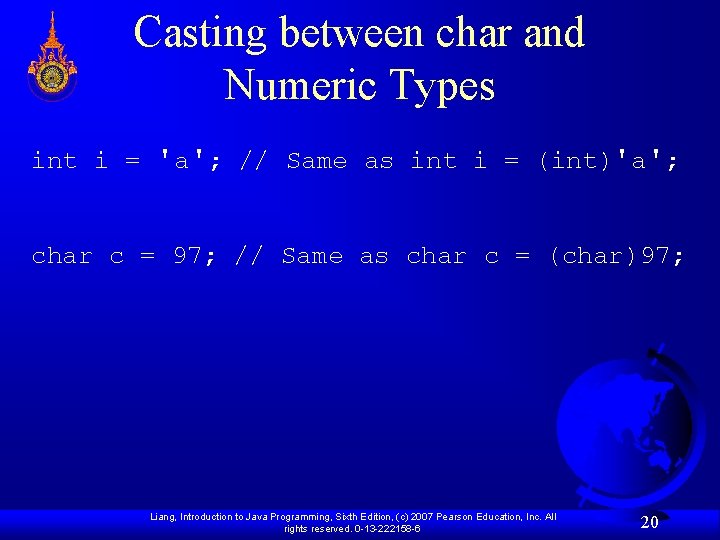
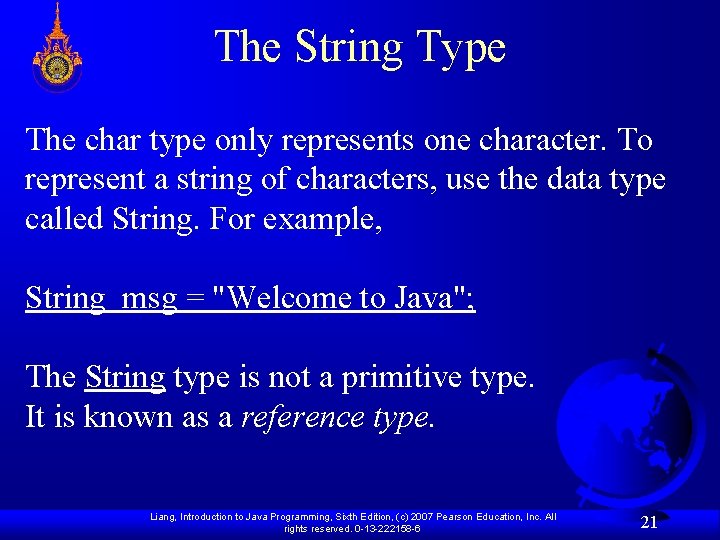
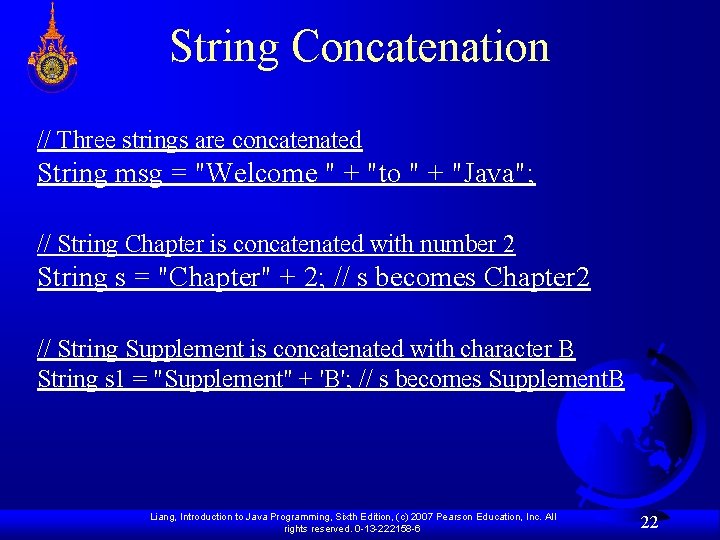
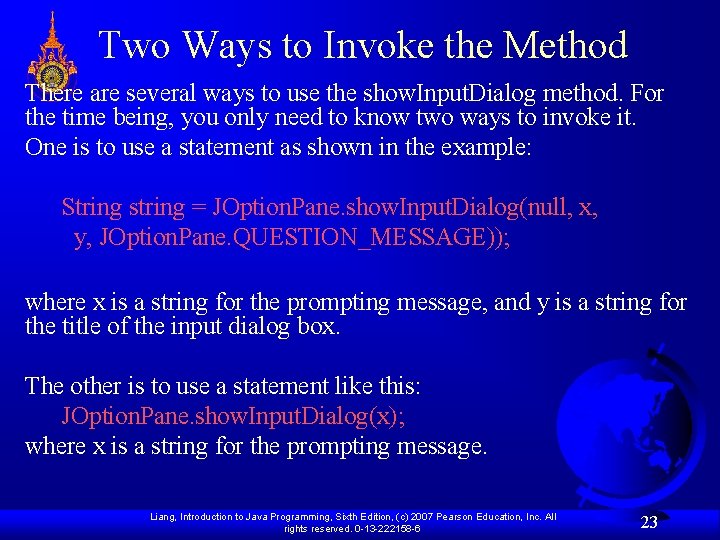
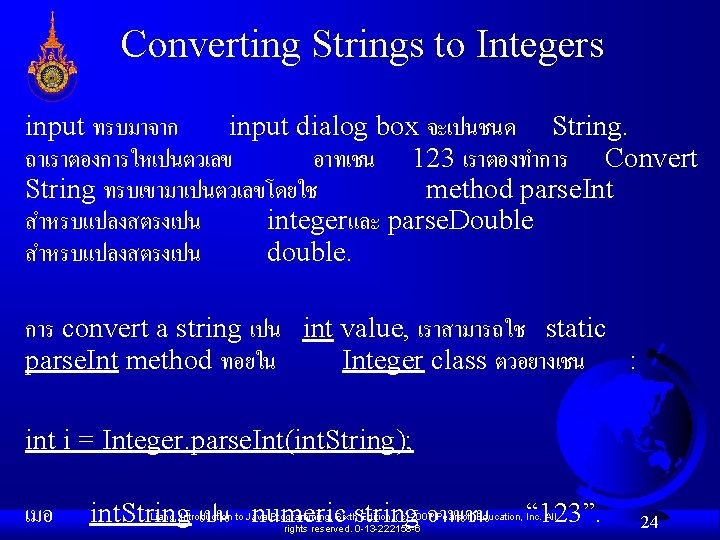
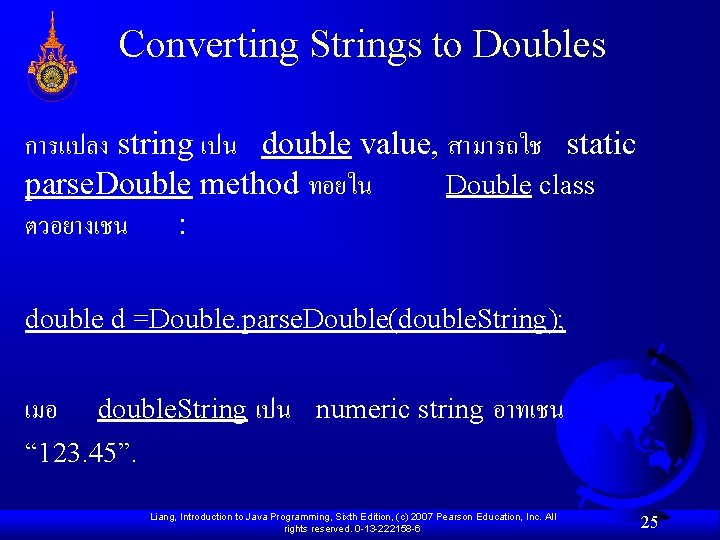
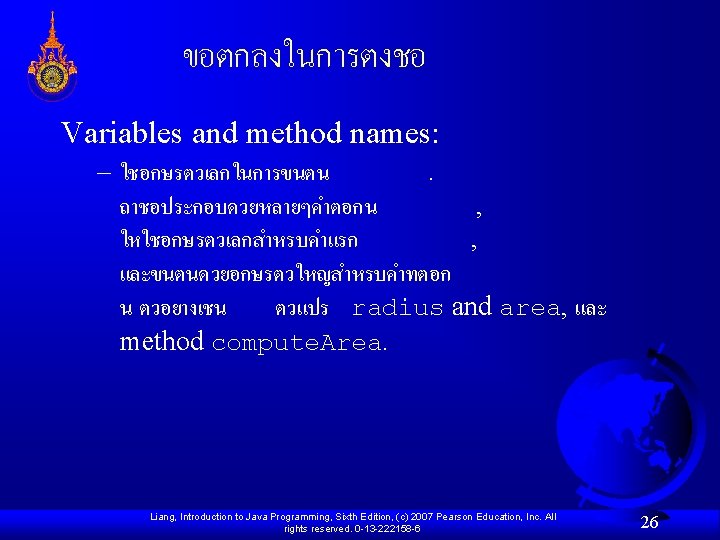
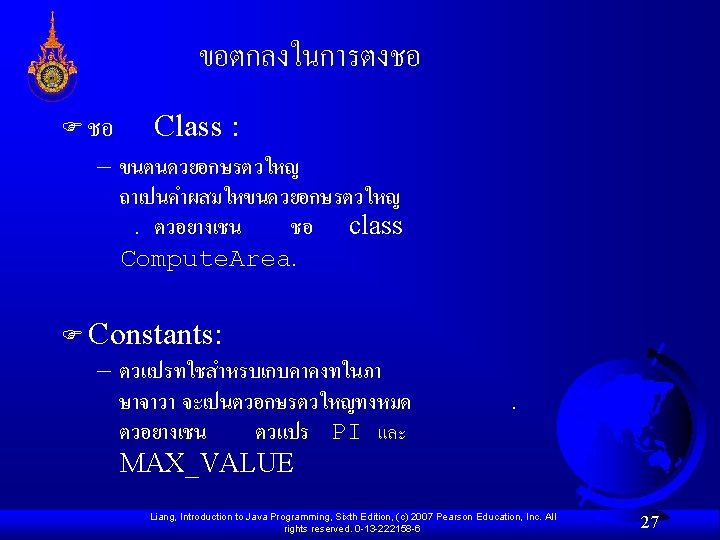
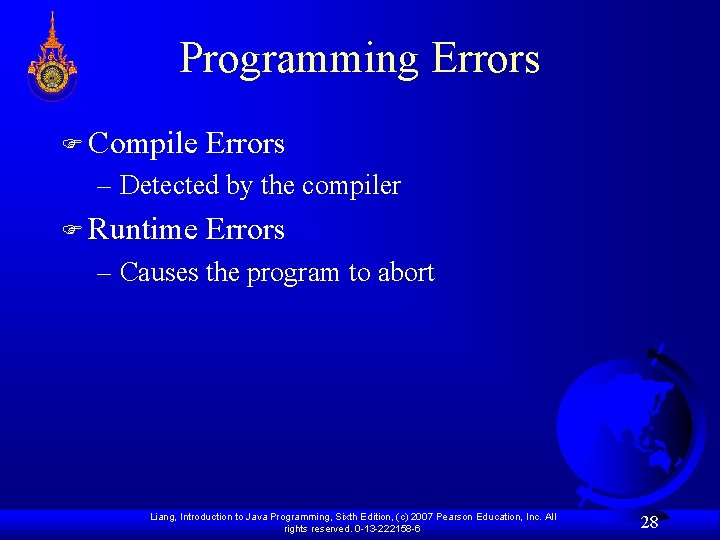
![Compile Errors public class Show. Syntax. Errors { public static void main(String[] args) { Compile Errors public class Show. Syntax. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/4d3e5276d8c2d922d189b91fde04621c/image-29.jpg)
![Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) { Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/4d3e5276d8c2d922d189b91fde04621c/image-30.jpg)
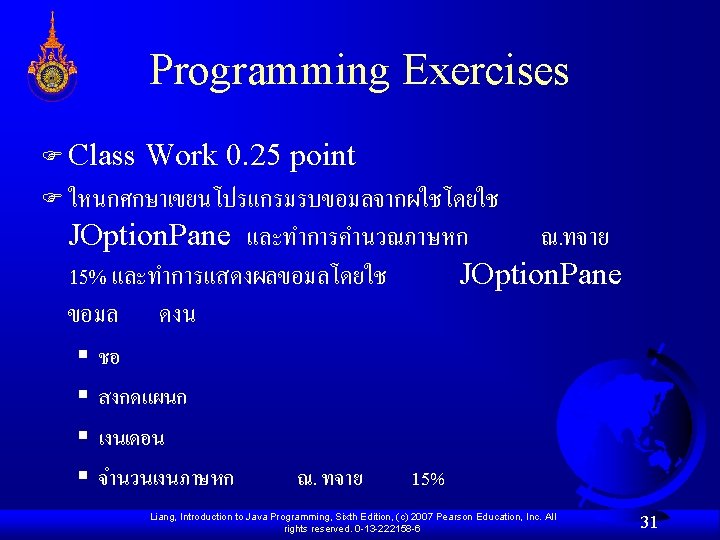
- Slides: 31
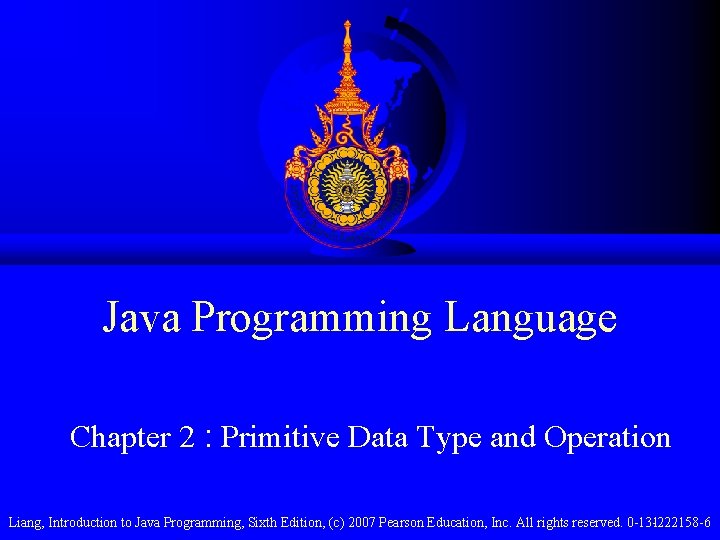
Java Programming Language Chapter 2 : Primitive Data Type and Operation Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 1
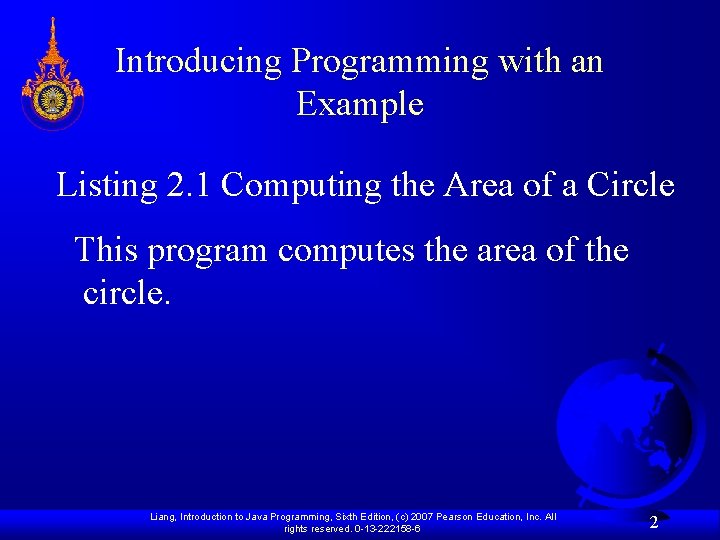
Introducing Programming with an Example Listing 2. 1 Computing the Area of a Circle This program computes the area of the circle. Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 2
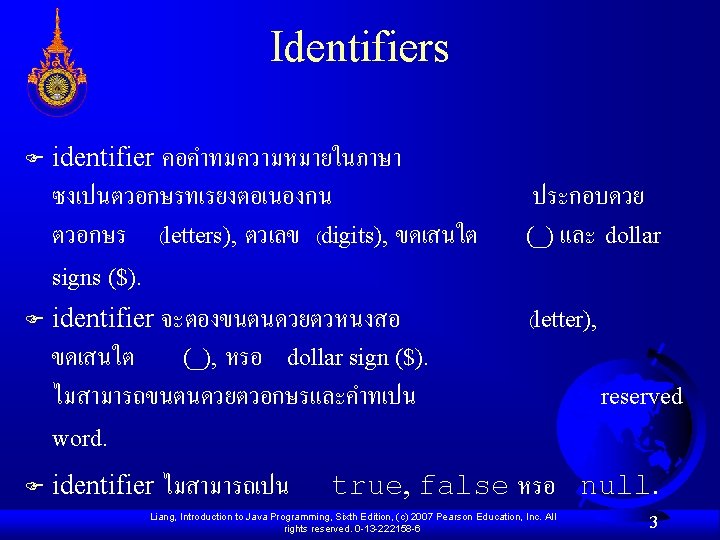
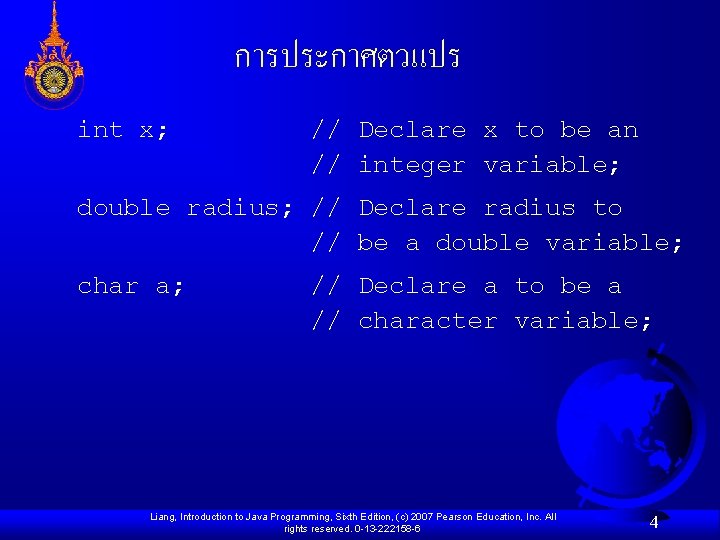
การประกาศตวแปร int x; // Declare x to be an // integer variable; double radius; // Declare radius to // be a double variable; char a; // Declare a to be a // character variable; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 4
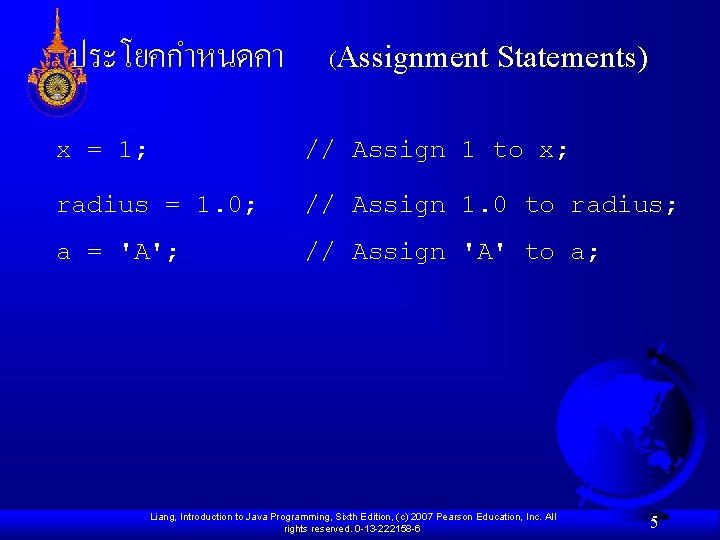
ประโยคกำหนดคา (Assignment Statements) x = 1; // Assign 1 to x; radius = 1. 0; // Assign 1. 0 to radius; a = 'A'; // Assign 'A' to a; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 5
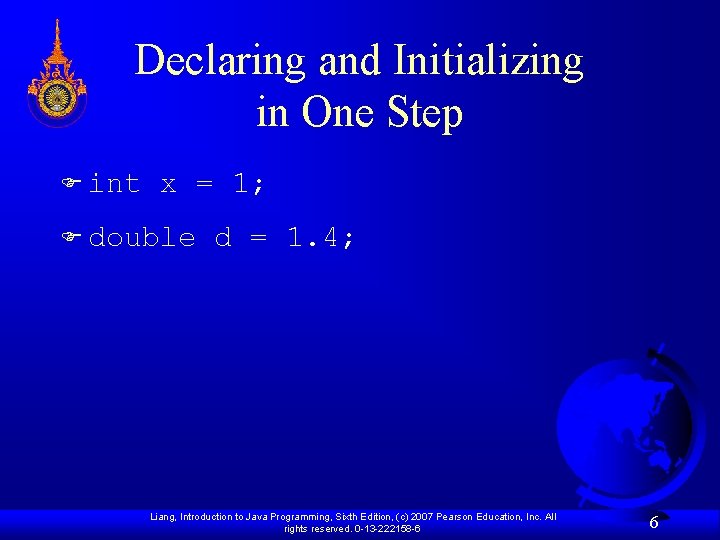
Declaring and Initializing in One Step F int x = 1; F double d = 1. 4; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 6
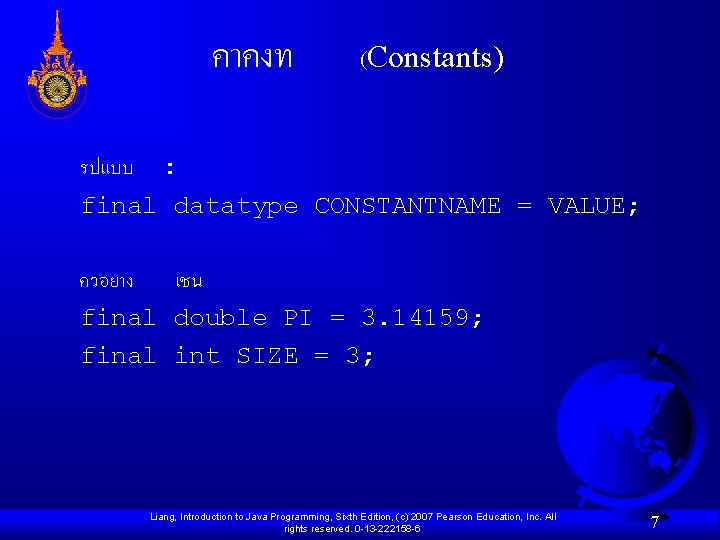
คาคงท (Constants) รปแบบ : final datatype CONSTANTNAME = VALUE; ควอยาง เชน final double PI = 3. 14159; final int SIZE = 3; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 7
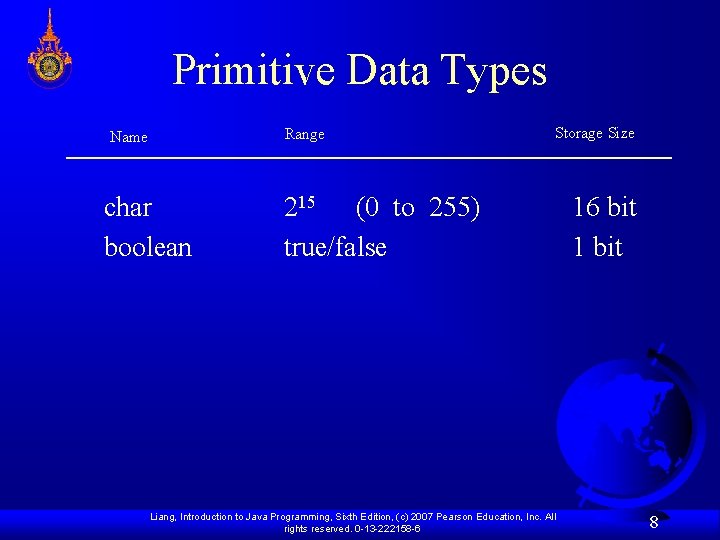
Primitive Data Types Range Name char boolean Storage Size 215 (0 to 255) true/false Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 16 bit 1 bit 8
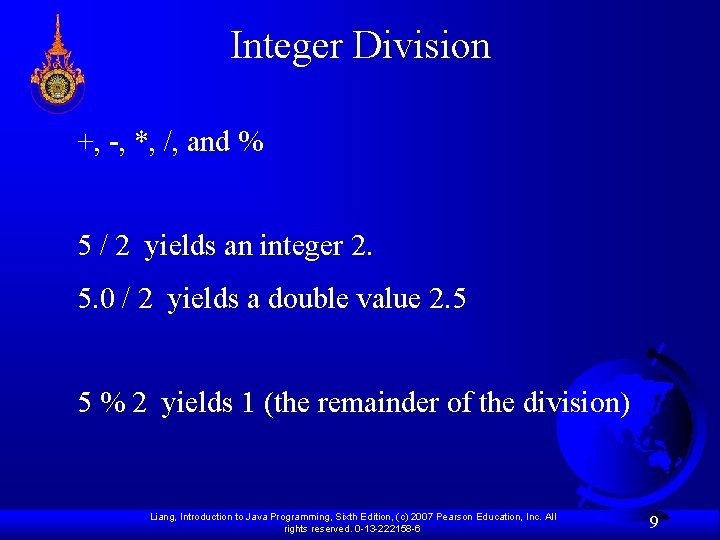
Integer Division +, -, *, /, and % 5 / 2 yields an integer 2. 5. 0 / 2 yields a double value 2. 5 5 % 2 yields 1 (the remainder of the division) Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 9
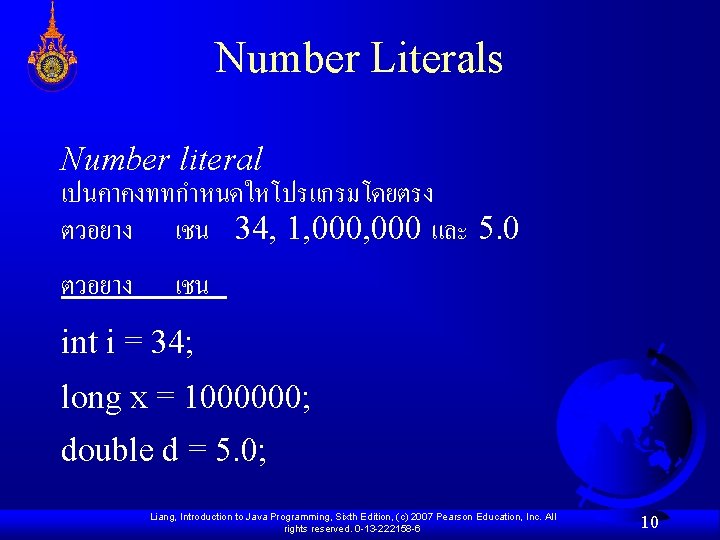
Number Literals Number literal เปนคาคงททกำหนดใหโปรแกรมโดยตรง ตวอยาง เชน 34, 1, 000 และ 5. 0 ตวอยาง เชน int i = 34; long x = 1000000; double d = 5. 0; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 10
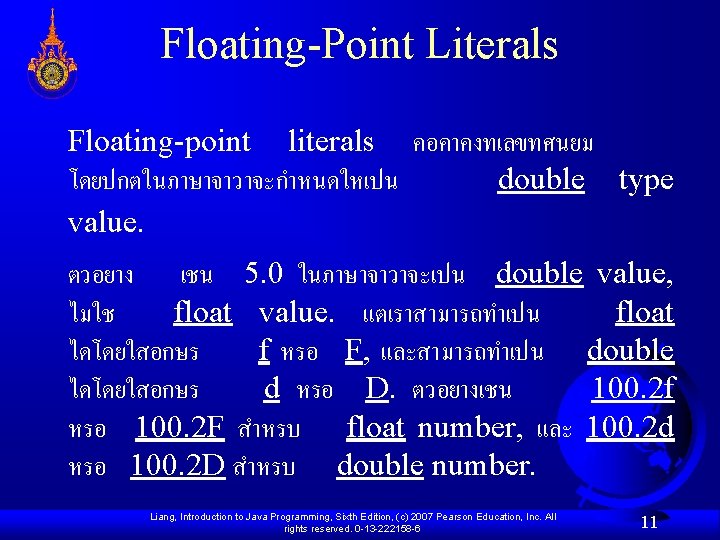
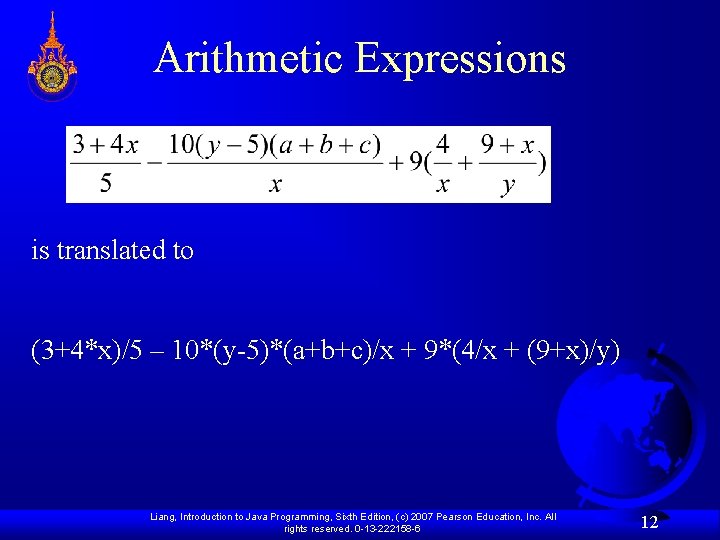
Arithmetic Expressions is translated to (3+4*x)/5 – 10*(y-5)*(a+b+c)/x + 9*(4/x + (9+x)/y) Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 12
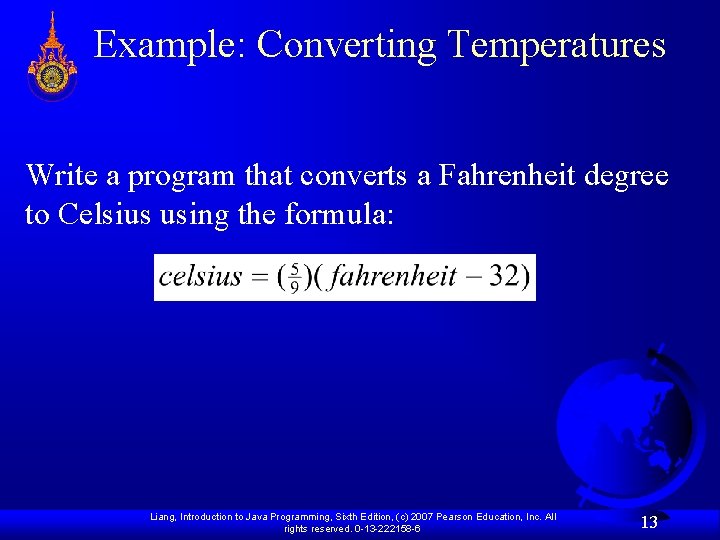
Example: Converting Temperatures Write a program that converts a Fahrenheit degree to Celsius using the formula: Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 13
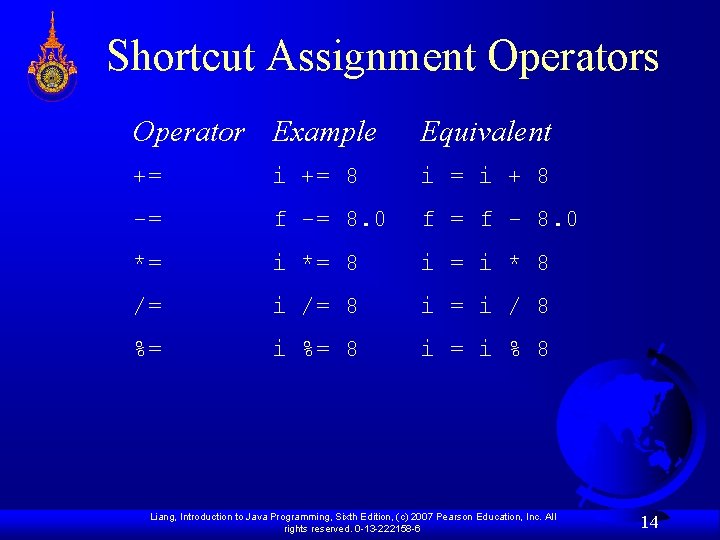
Shortcut Assignment Operators Operator Example Equivalent += i += 8 i = i + 8 -= f -= 8. 0 f = f - 8. 0 *= i *= 8 i = i * 8 /= i /= 8 i = i / 8 %= i %= 8 i = i % 8 Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 14
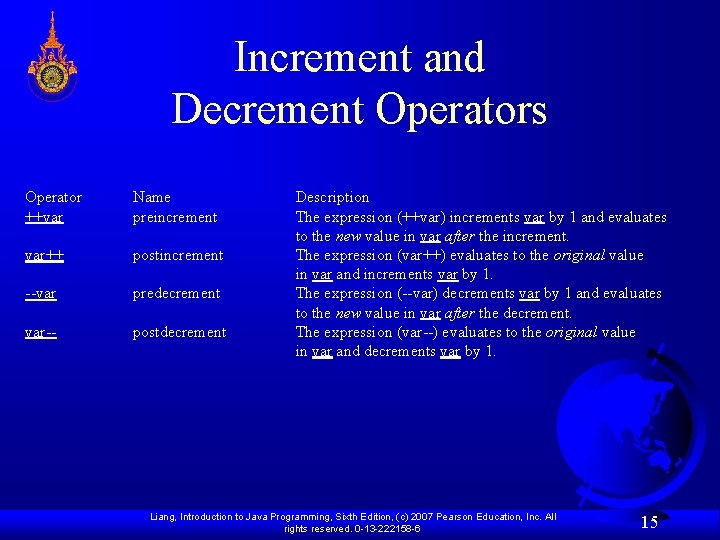
Increment and Decrement Operators Operator ++var Name preincrement var++ postincrement --var predecrement var-- postdecrement Description The expression (++var) increments var by 1 and evaluates to the new value in var after the increment. The expression (var++) evaluates to the original value in var and increments var by 1. The expression (--var) decrements var by 1 and evaluates to the new value in var after the decrement. The expression (var--) evaluates to the original value in var and decrements var by 1. Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 15
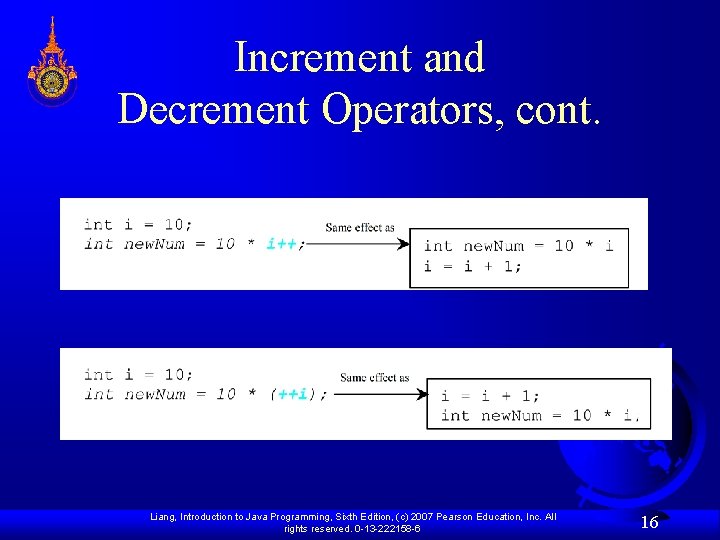
Increment and Decrement Operators, cont. Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 16
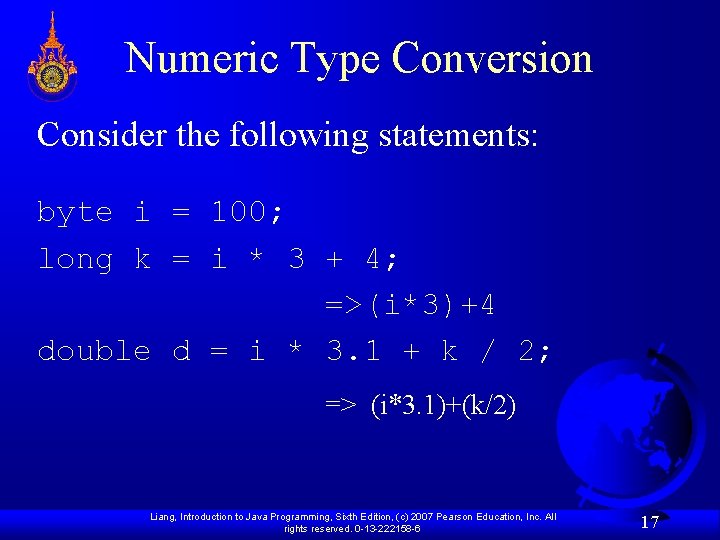
Numeric Type Conversion Consider the following statements: byte i = 100; long k = i * 3 + 4; =>(i*3)+4 double d = i * 3. 1 + k / 2; => (i*3. 1)+(k/2) Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 17
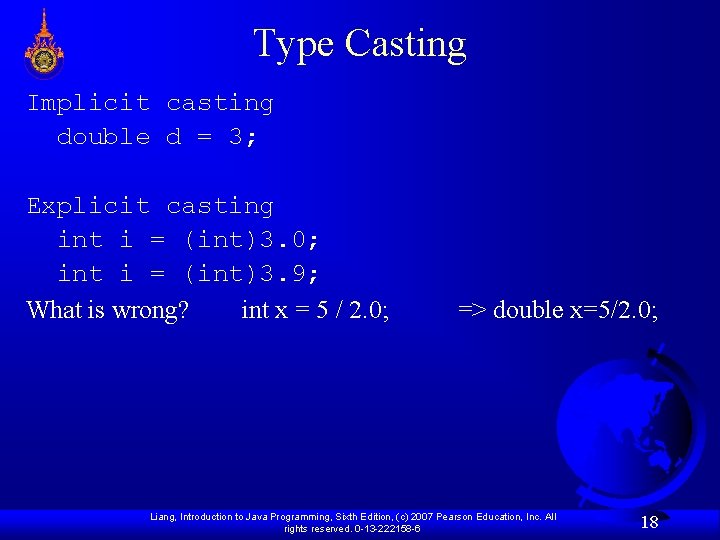
Type Casting Implicit casting double d = 3; Explicit casting int i = (int)3. 0; int i = (int)3. 9; What is wrong? int x = 5 / 2. 0; => double x=5/2. 0; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 18
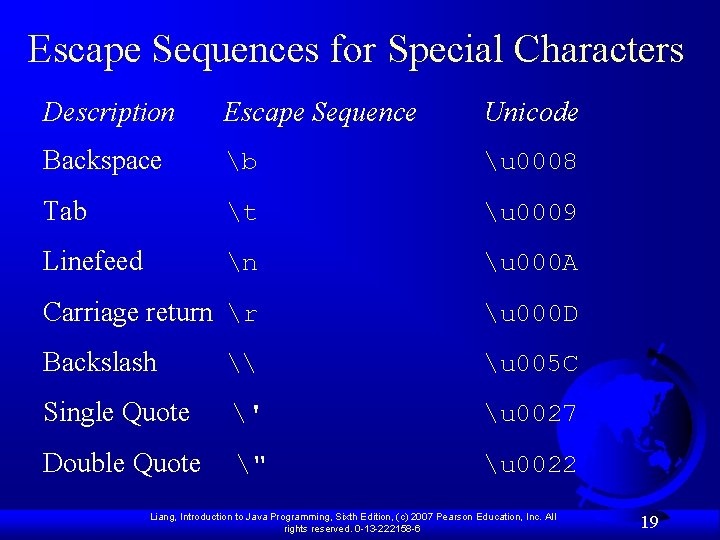
Escape Sequences for Special Characters Description Escape Sequence Unicode Backspace b u 0008 Tab t u 0009 Linefeed n u 000 A Carriage return r u 000 D Backslash \ u 005 C Single Quote ' u 0027 Double Quote " u 0022 Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 19
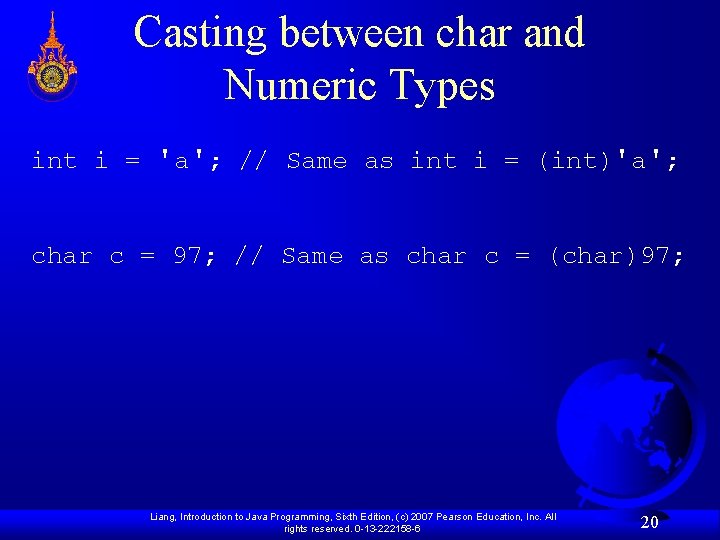
Casting between char and Numeric Types int i = 'a'; // Same as int i = (int)'a'; char c = 97; // Same as char c = (char)97; Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 20
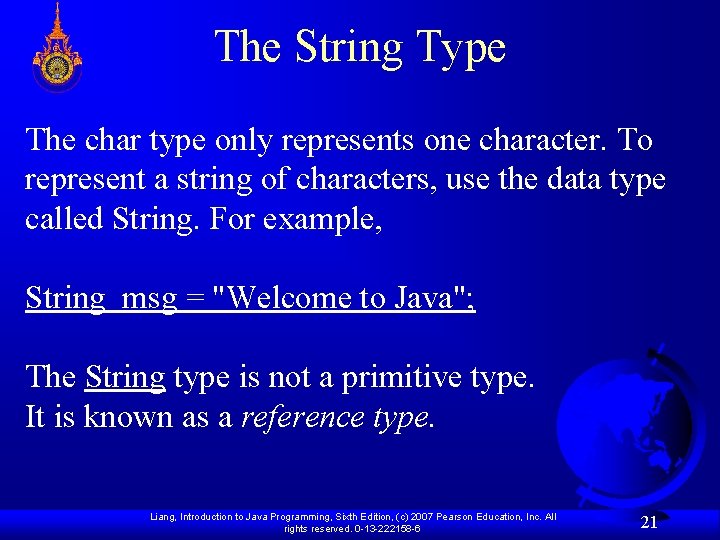
The String Type The char type only represents one character. To represent a string of characters, use the data type called String. For example, String msg = "Welcome to Java"; The String type is not a primitive type. It is known as a reference type. Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 21
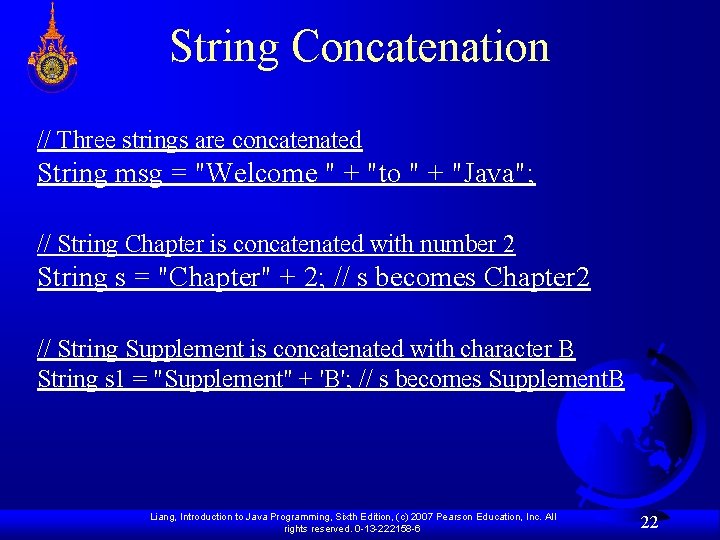
String Concatenation // Three strings are concatenated String msg = "Welcome " + "to " + "Java"; // String Chapter is concatenated with number 2 String s = "Chapter" + 2; // s becomes Chapter 2 // String Supplement is concatenated with character B String s 1 = "Supplement" + 'B'; // s becomes Supplement. B Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 22
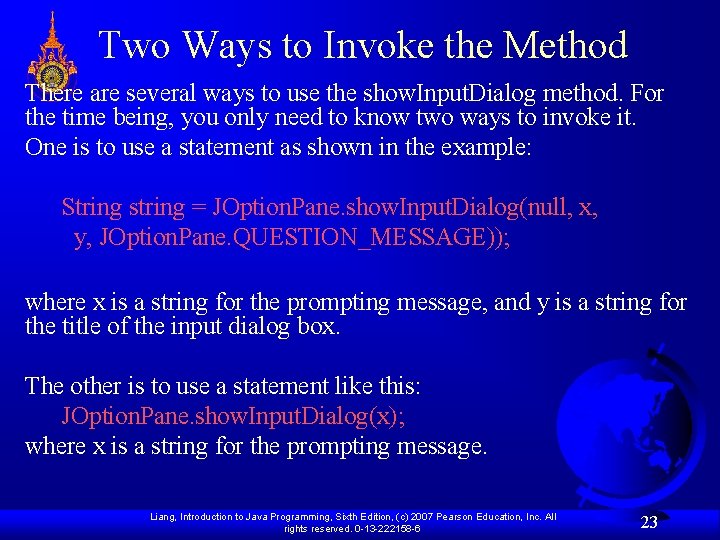
Two Ways to Invoke the Method There are several ways to use the show. Input. Dialog method. For the time being, you only need to know two ways to invoke it. One is to use a statement as shown in the example: String string = JOption. Pane. show. Input. Dialog(null, x, y, JOption. Pane. QUESTION_MESSAGE)); where x is a string for the prompting message, and y is a string for the title of the input dialog box. The other is to use a statement like this: JOption. Pane. show. Input. Dialog(x); where x is a string for the prompting message. Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 23
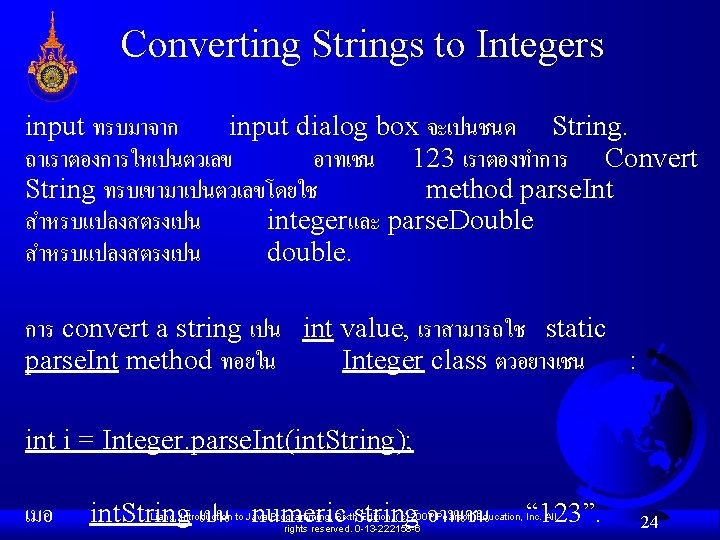
Converting Strings to Integers input ทรบมาจาก input dialog box จะเปนชนด String. ถาเราตองการใหเปนตวเลข อาทเชน 123 เราตองทำการ Convert String ทรบเขามาเปนตวเลขโดยใช method parse. Int สำหรบแปลงสตรงเปน integerและ parse. Double สำหรบแปลงสตรงเปน double. การ convert a string เปน int value, เราสามารถใช static parse. Int method ทอยใน Integer class ตวอยางเชน : int i = Integer. parse. Int(int. String); เมอ int. String เปน numeric string อาทเชน “ 123”. 24 Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6
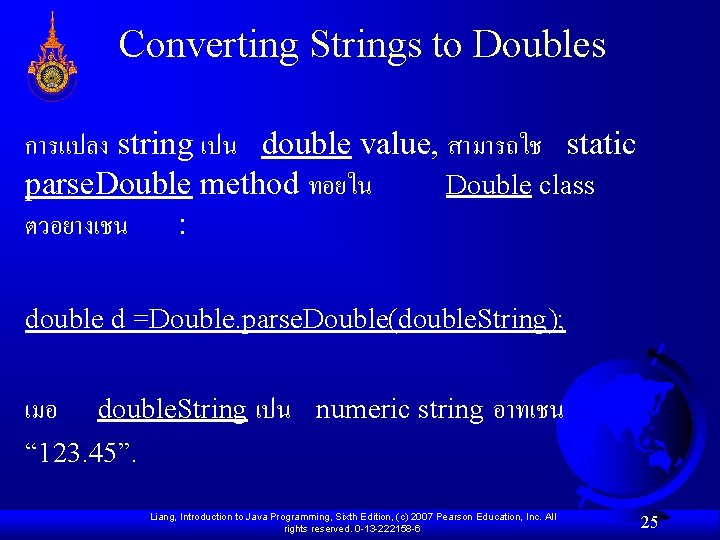
Converting Strings to Doubles การแปลง string เปน double value, สามารถใช static parse. Double method ทอยใน Double class ตวอยางเชน : double d =Double. parse. Double(double. String); เมอ double. String เปน numeric string อาทเชน “ 123. 45”. Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 25
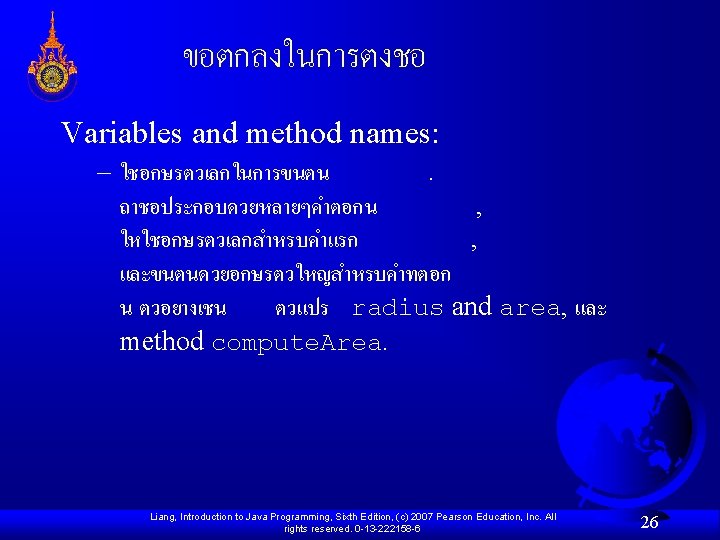
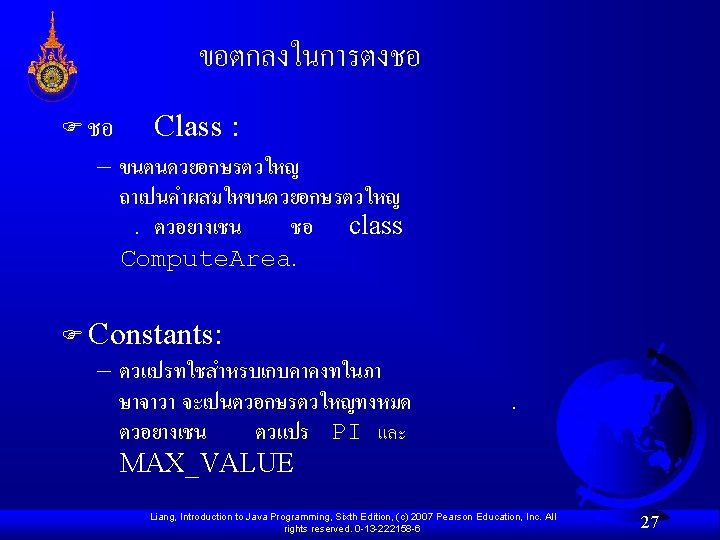
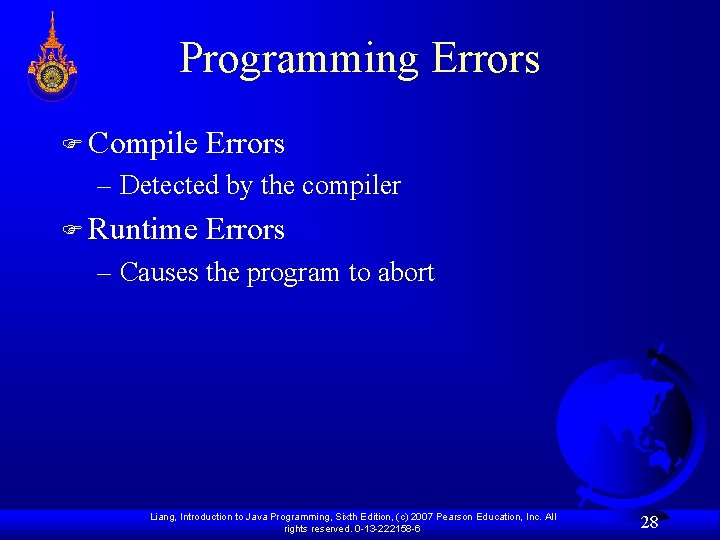
Programming Errors F Compile Errors – Detected by the compiler F Runtime Errors – Causes the program to abort Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 28
![Compile Errors public class Show Syntax Errors public static void mainString args Compile Errors public class Show. Syntax. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/4d3e5276d8c2d922d189b91fde04621c/image-29.jpg)
Compile Errors public class Show. Syntax. Errors { public static void main(String[] args) { i = 30; //variable i is no type System. out. println(i + 4); } } Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 29
![Runtime Errors public class Show Runtime Errors public static void mainString args Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/4d3e5276d8c2d922d189b91fde04621c/image-30.jpg)
Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) { int i = 1 / 0; //devid by zero } } Liang, Introduction to Java Programming, Sixth Edition, (c) 2007 Pearson Education, Inc. All rights reserved. 0 -13 -222158 -6 30
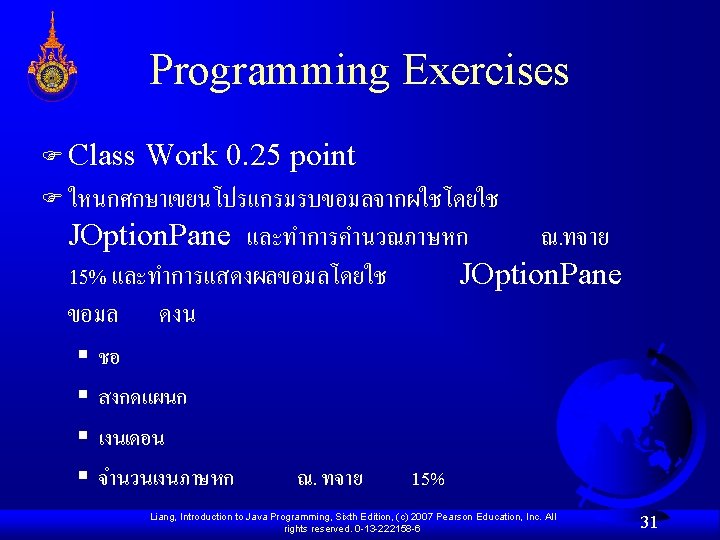