IPC 144 Introduction to Programming Using C Week
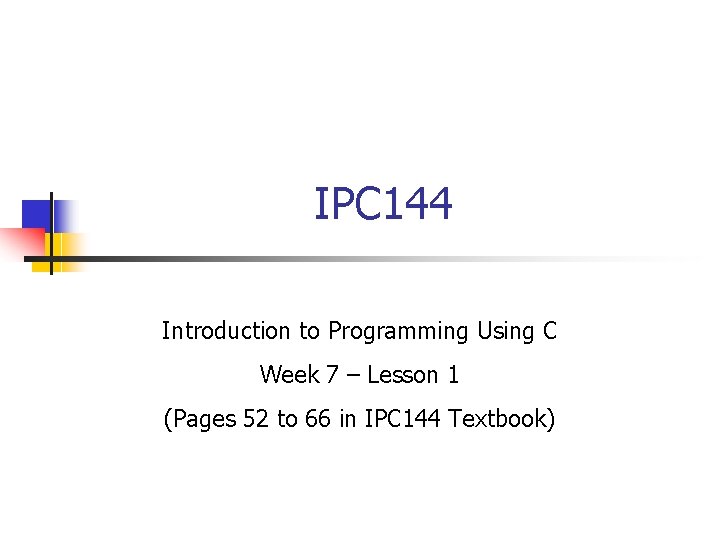
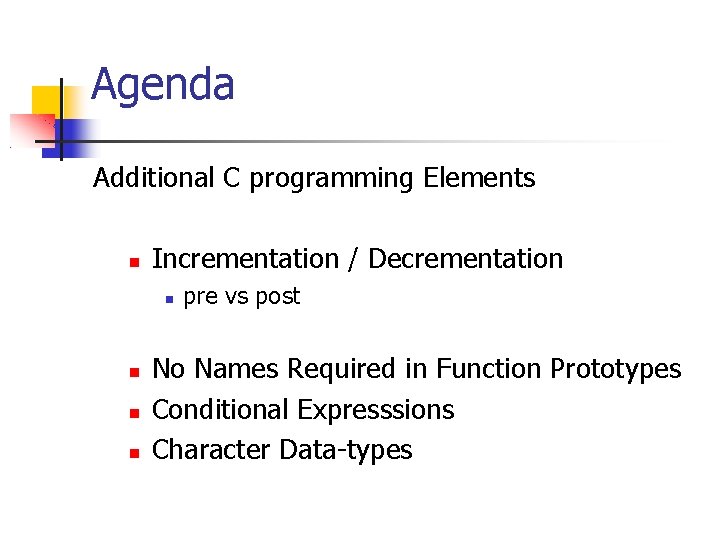
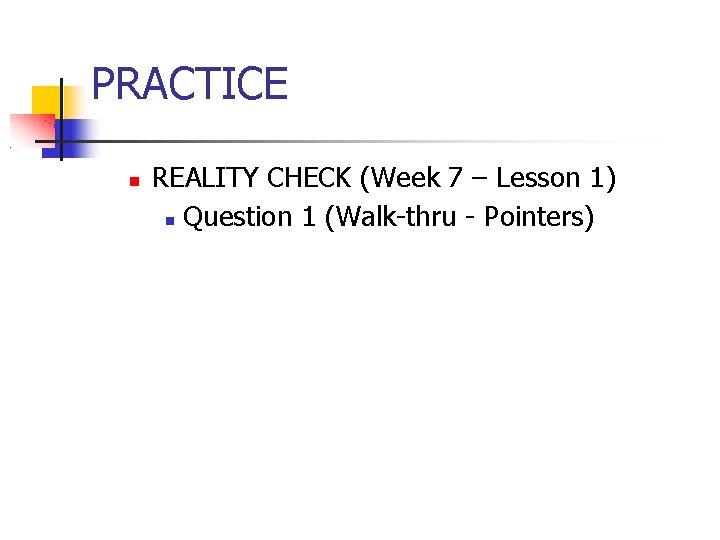
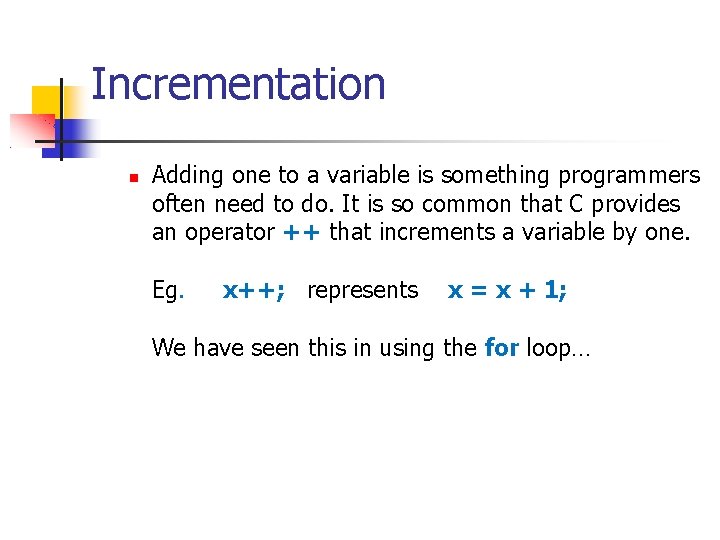
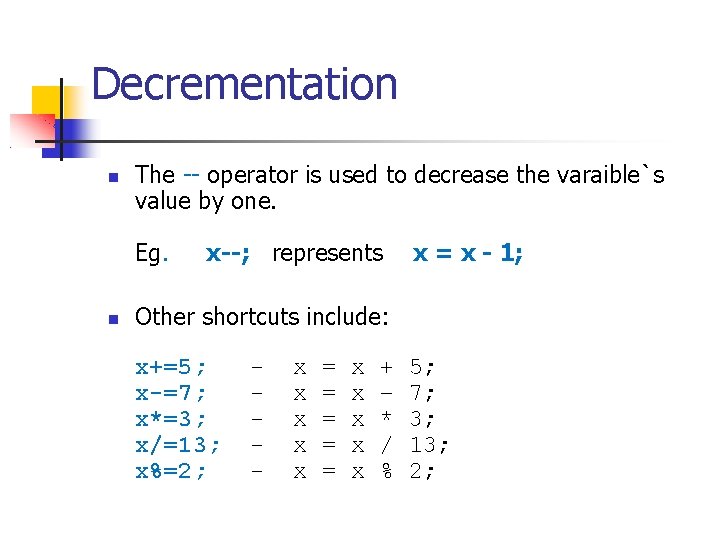
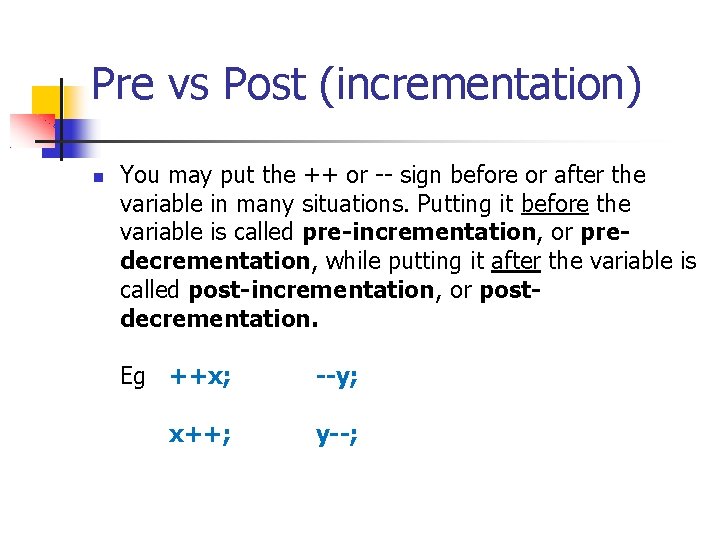
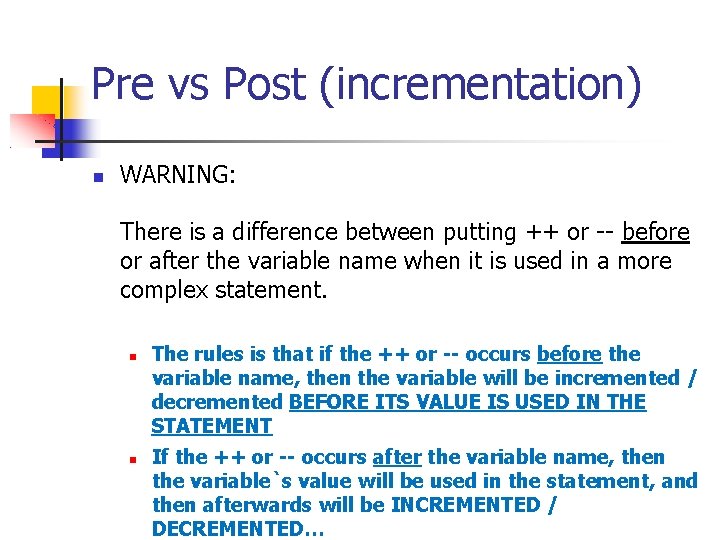
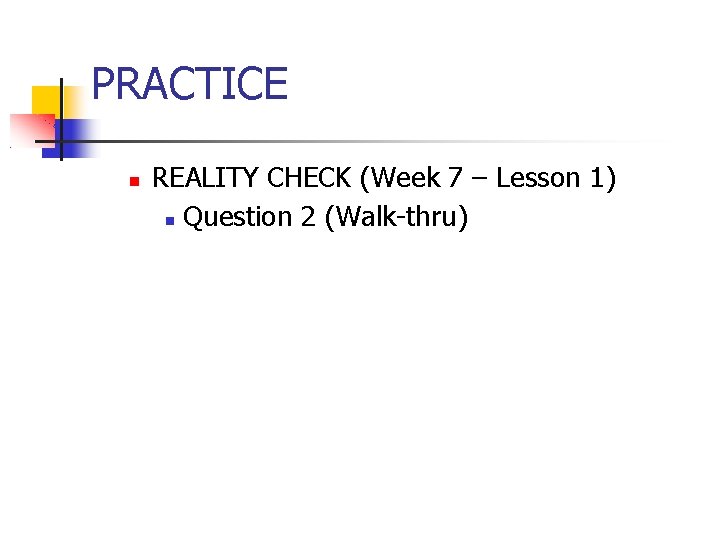
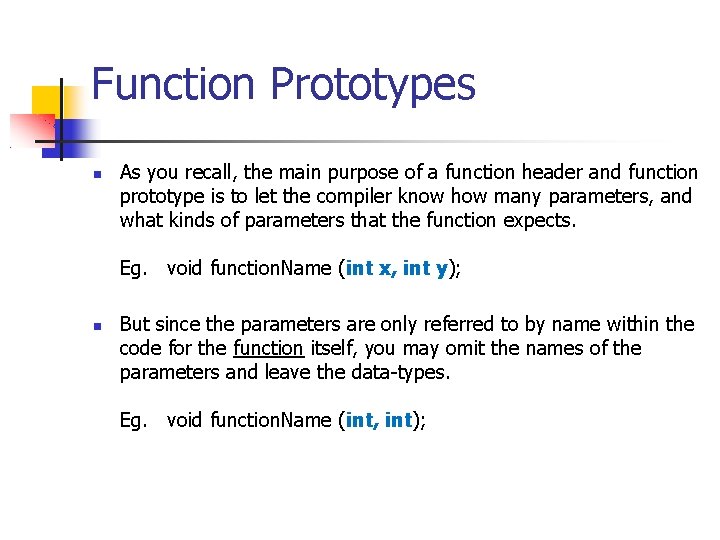
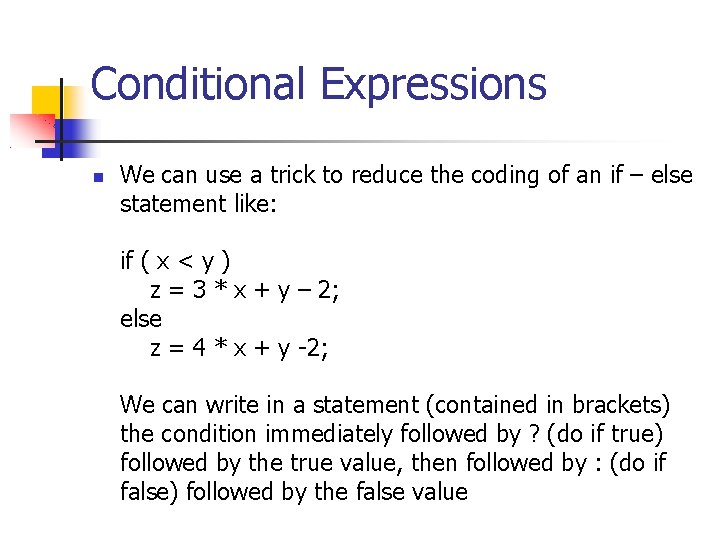
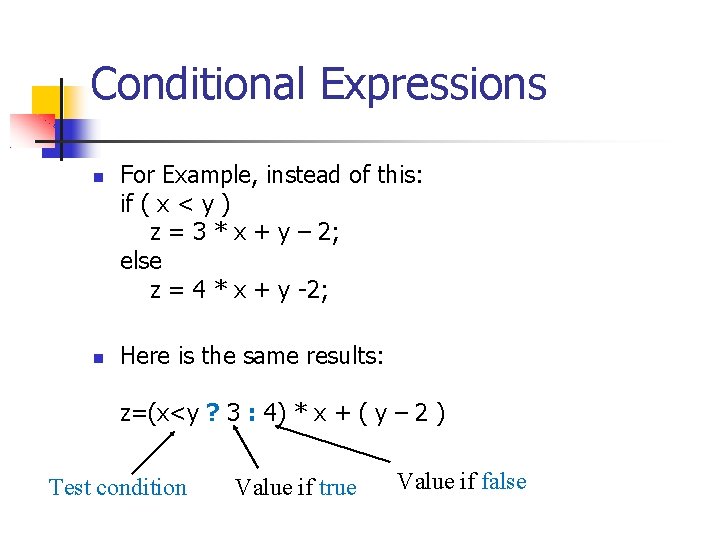
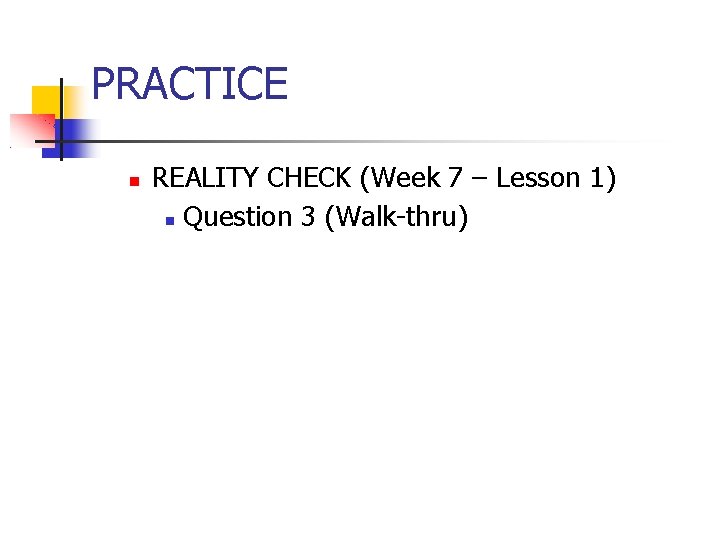
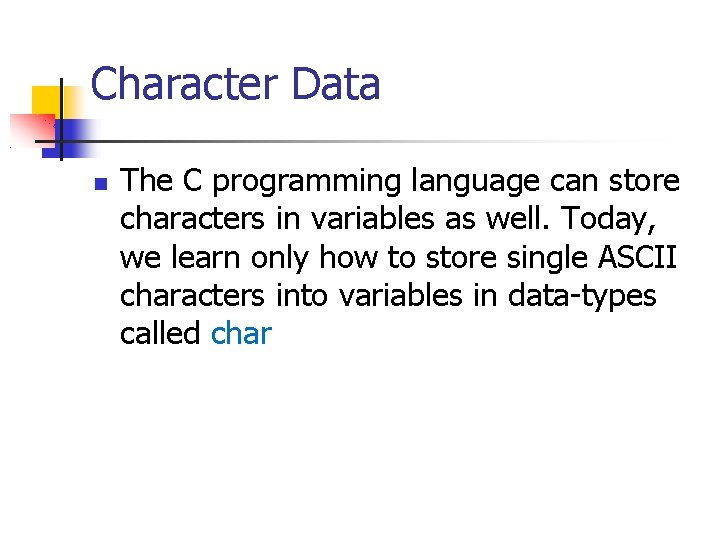
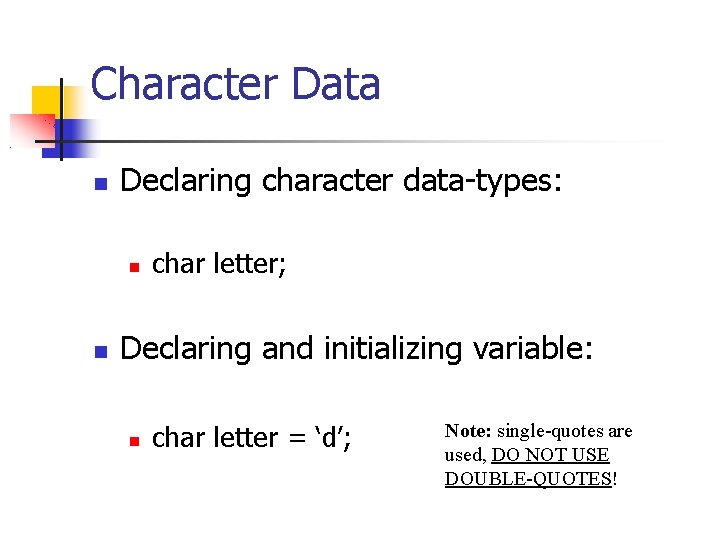
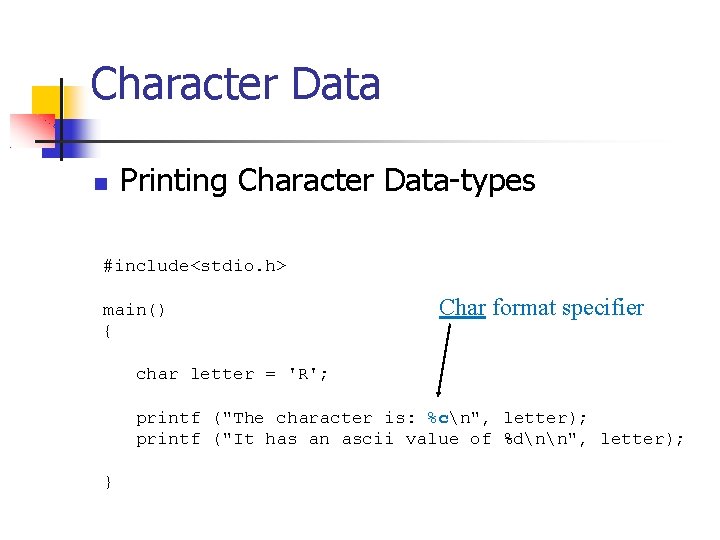
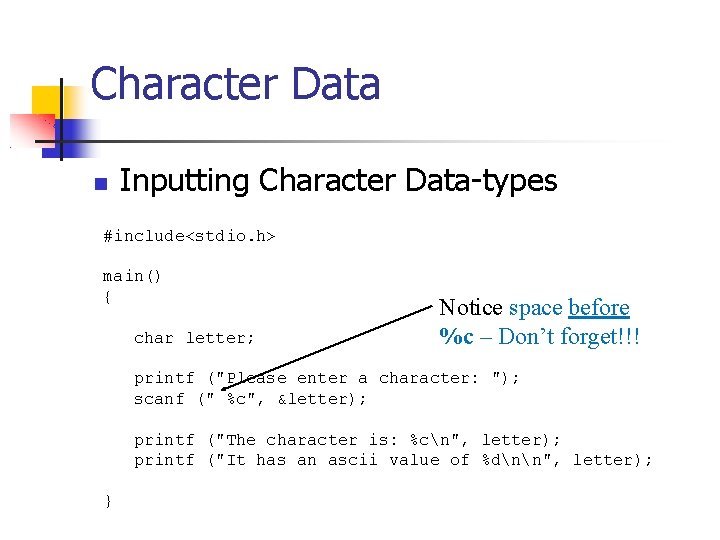
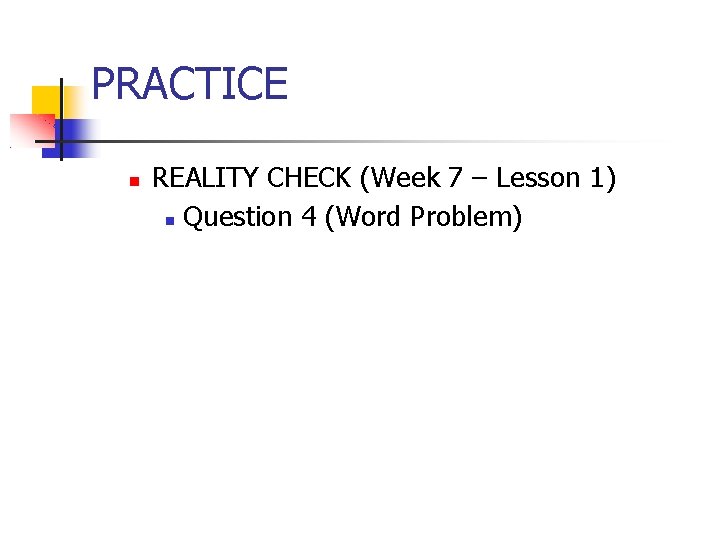
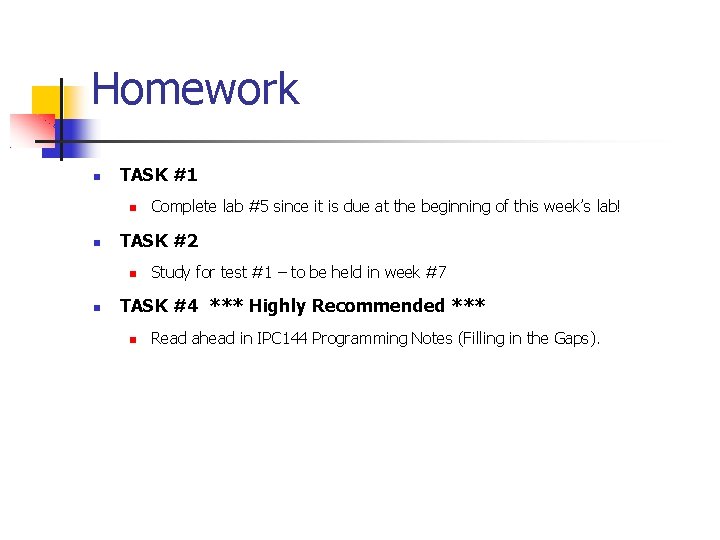
- Slides: 18
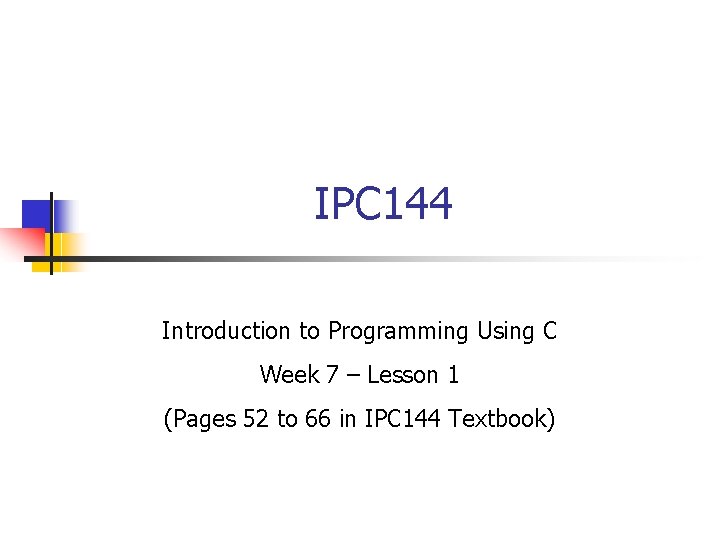
IPC 144 Introduction to Programming Using C Week 7 – Lesson 1 (Pages 52 to 66 in IPC 144 Textbook)
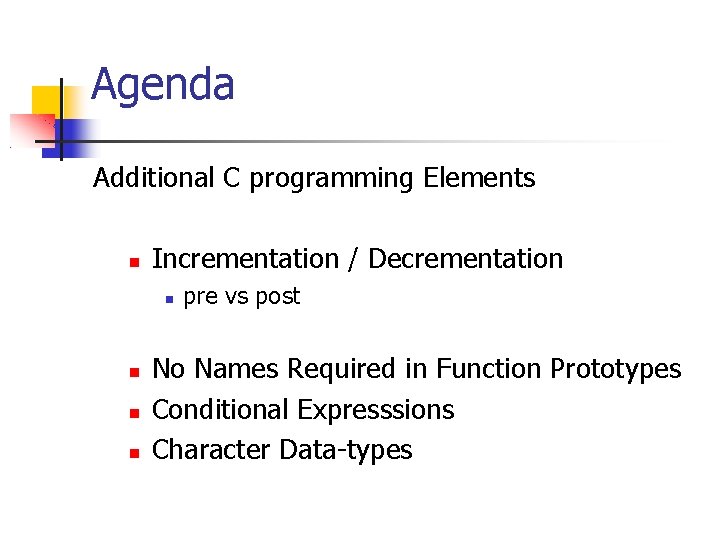
Agenda Additional C programming Elements Incrementation / Decrementation pre vs post No Names Required in Function Prototypes Conditional Expresssions Character Data-types
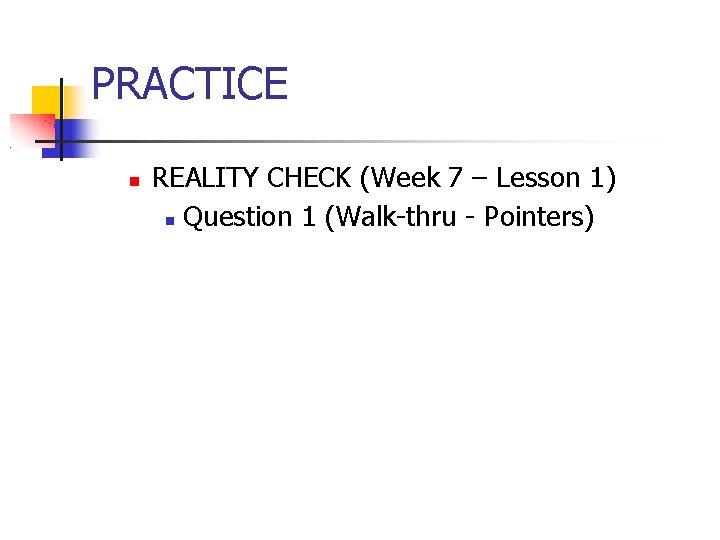
PRACTICE REALITY CHECK (Week 7 – Lesson 1) Question 1 (Walk-thru - Pointers)
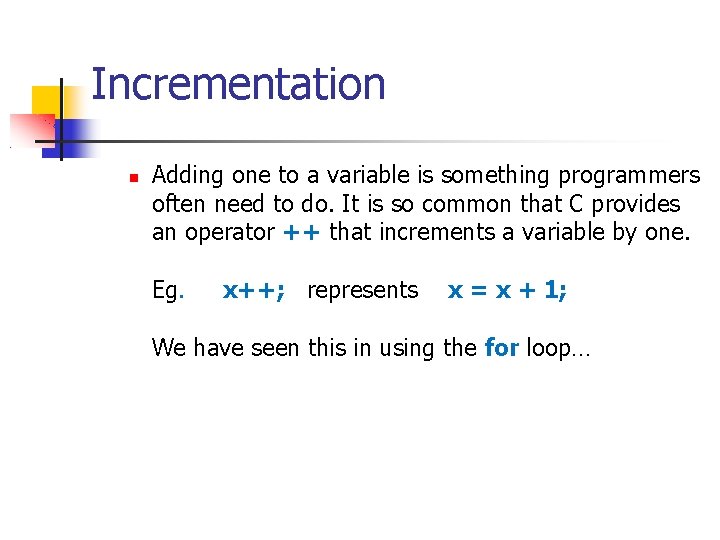
Incrementation Adding one to a variable is something programmers often need to do. It is so common that C provides an operator ++ that increments a variable by one. Eg. x++; represents x = x + 1; We have seen this in using the for loop…
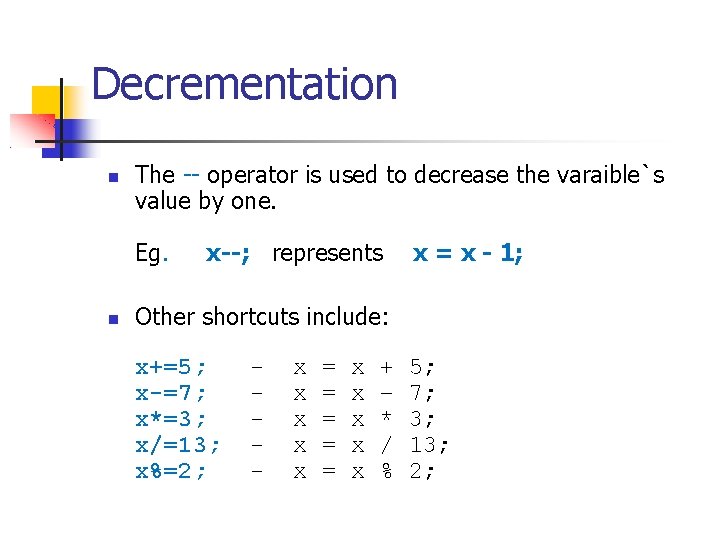
Decrementation The -- operator is used to decrease the varaible`s value by one. Eg. x--; represents x = x - 1; Other shortcuts include: x+=5; x-=7; x*=3; x/=13; x%=2; - x x x = = = x x x + – * / % 5; 7; 3; 13; 2;
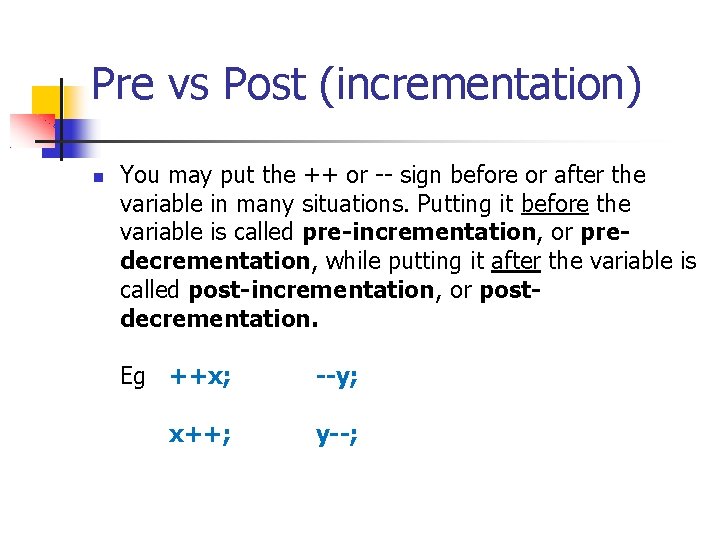
Pre vs Post (incrementation) You may put the ++ or -- sign before or after the variable in many situations. Putting it before the variable is called pre-incrementation, or predecrementation, while putting it after the variable is called post-incrementation, or postdecrementation. Eg ++x; --y; x++; y--;
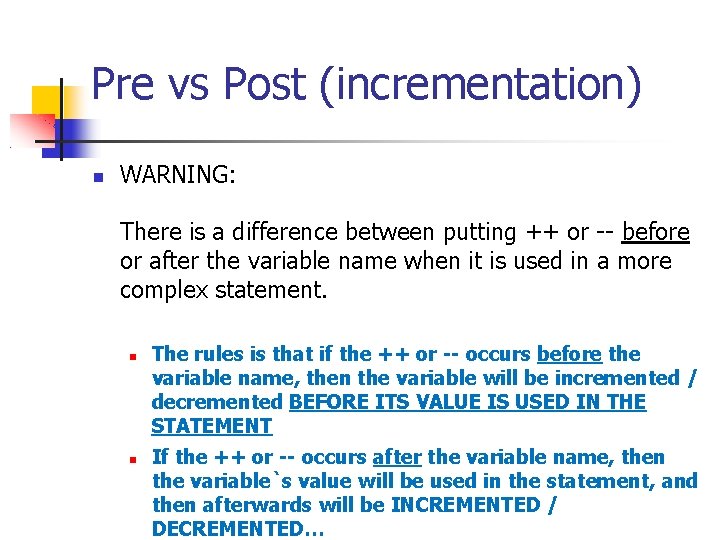
Pre vs Post (incrementation) WARNING: There is a difference between putting ++ or -- before or after the variable name when it is used in a more complex statement. The rules is that if the ++ or -- occurs before the variable name, then the variable will be incremented / decremented BEFORE ITS VALUE IS USED IN THE STATEMENT If the ++ or -- occurs after the variable name, then the variable`s value will be used in the statement, and then afterwards will be INCREMENTED / DECREMENTED…
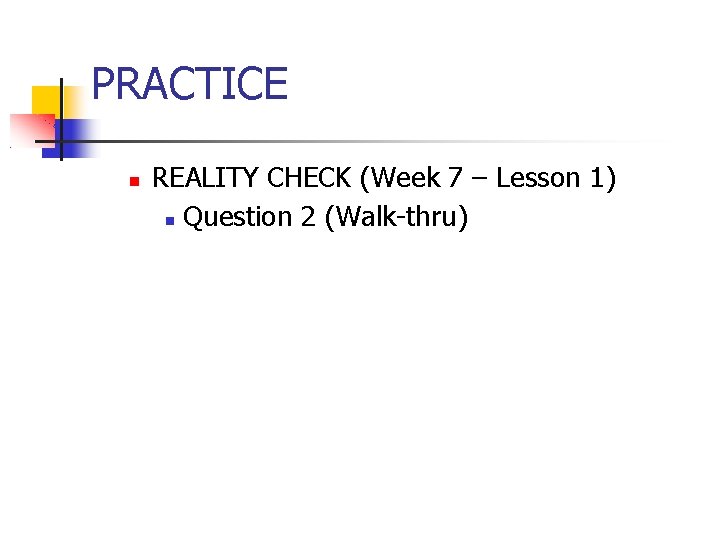
PRACTICE REALITY CHECK (Week 7 – Lesson 1) Question 2 (Walk-thru)
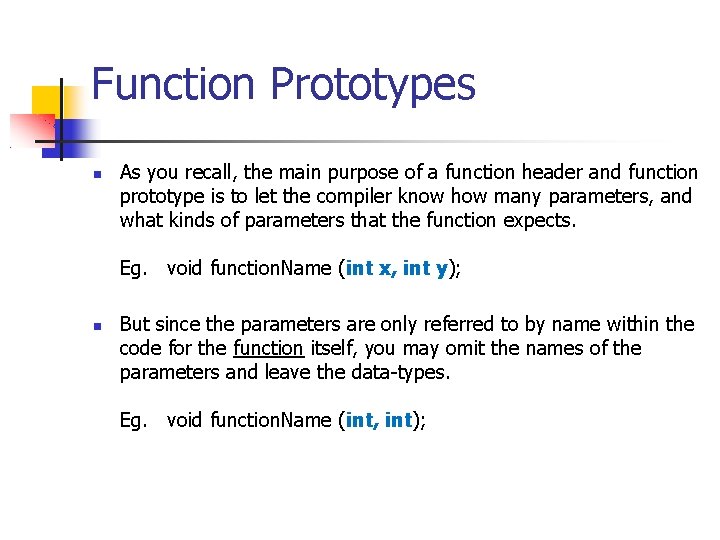
Function Prototypes As you recall, the main purpose of a function header and function prototype is to let the compiler know how many parameters, and what kinds of parameters that the function expects. Eg. void function. Name (int x, int y); But since the parameters are only referred to by name within the code for the function itself, you may omit the names of the parameters and leave the data-types. Eg. void function. Name (int, int);
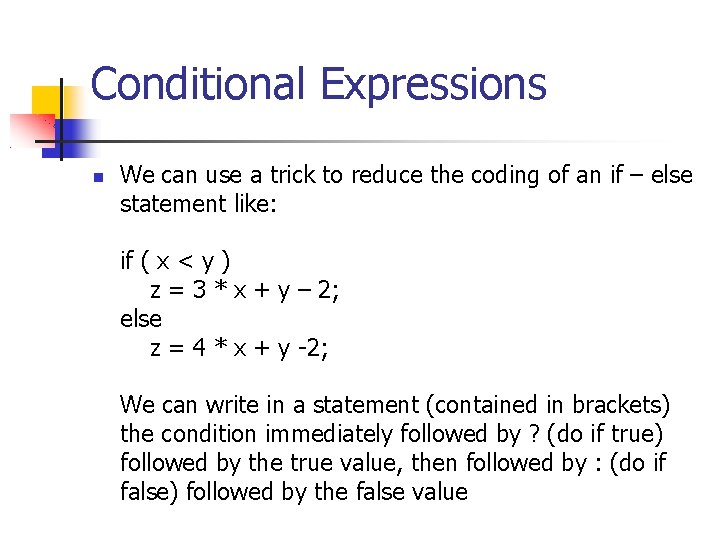
Conditional Expressions We can use a trick to reduce the coding of an if – else statement like: if ( x < y ) z = 3 * x + y – 2; else z = 4 * x + y -2; We can write in a statement (contained in brackets) the condition immediately followed by ? (do if true) followed by the true value, then followed by : (do if false) followed by the false value
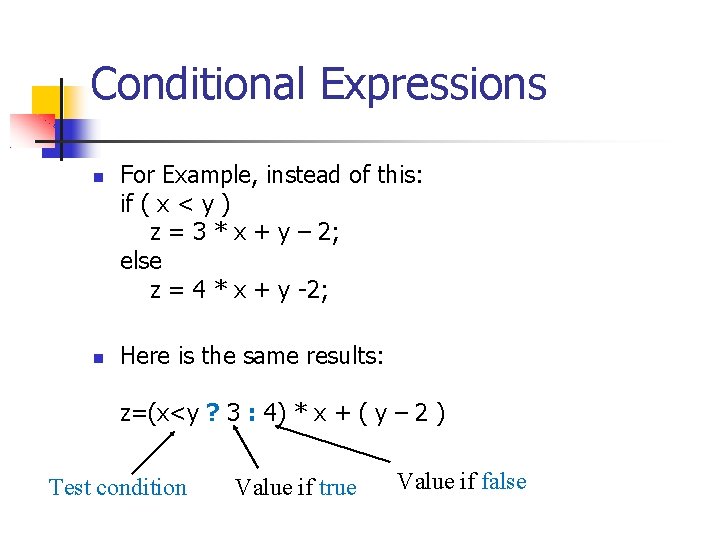
Conditional Expressions For Example, instead of this: if ( x < y ) z = 3 * x + y – 2; else z = 4 * x + y -2; Here is the same results: z=(x<y ? 3 : 4) * x + ( y – 2 ) Test condition Value if true Value if false
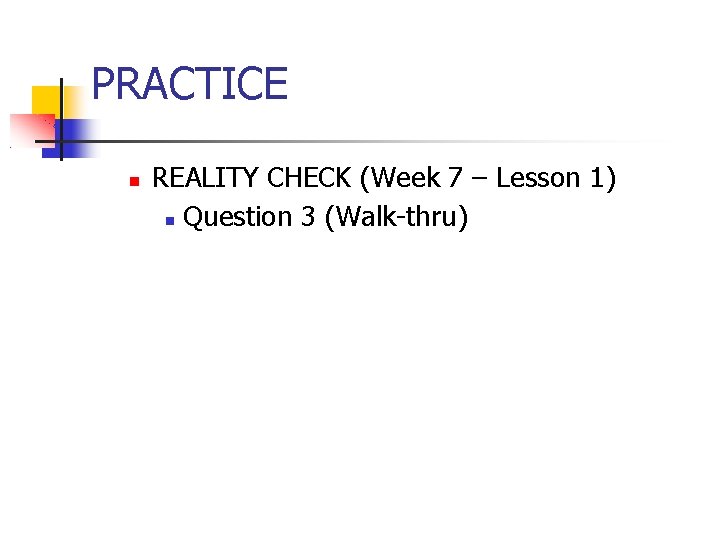
PRACTICE REALITY CHECK (Week 7 – Lesson 1) Question 3 (Walk-thru)
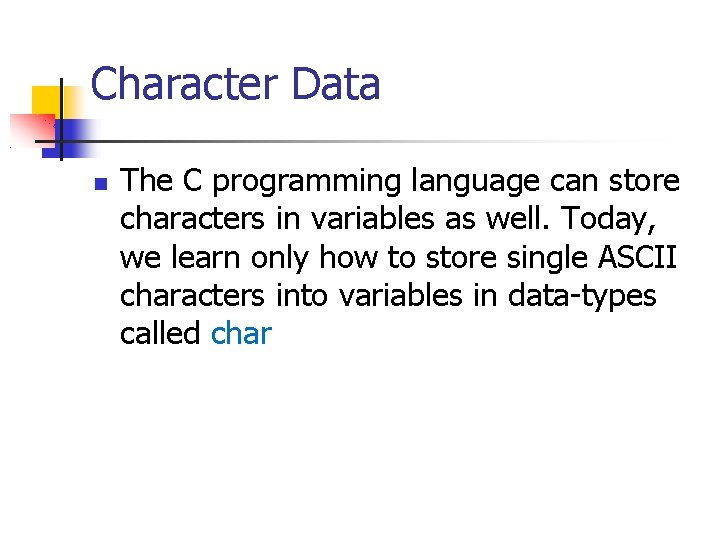
Character Data The C programming language can store characters in variables as well. Today, we learn only how to store single ASCII characters into variables in data-types called char
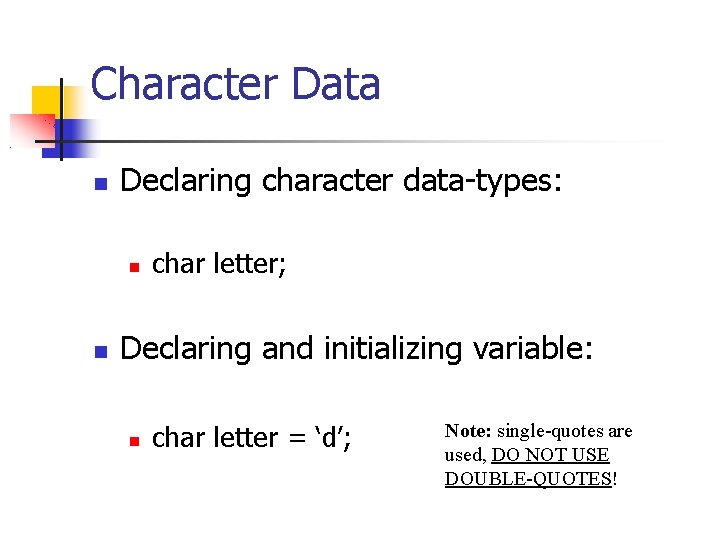
Character Data Declaring character data-types: char letter; Declaring and initializing variable: char letter = ‘d’; Note: single-quotes are used, DO NOT USE DOUBLE-QUOTES! :
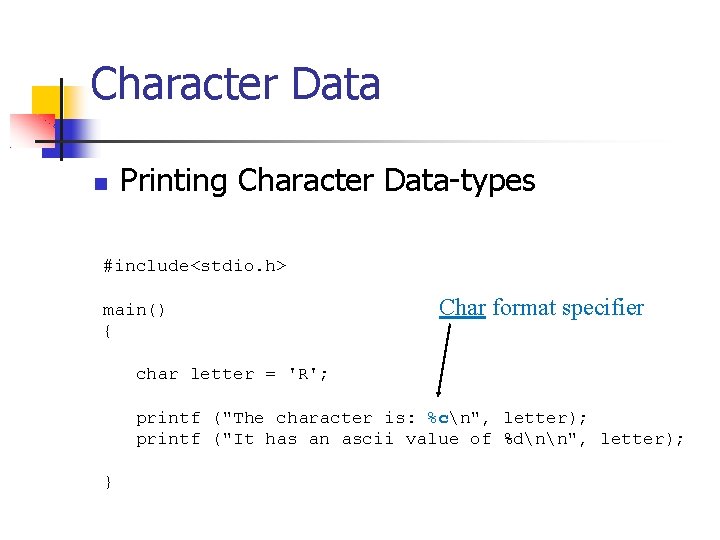
Character Data Printing Character Data-types #include<stdio. h> main() { Char format specifier char letter = 'R'; printf ("The character is: %cn", letter); printf ("It has an ascii value of %dnn", letter); }
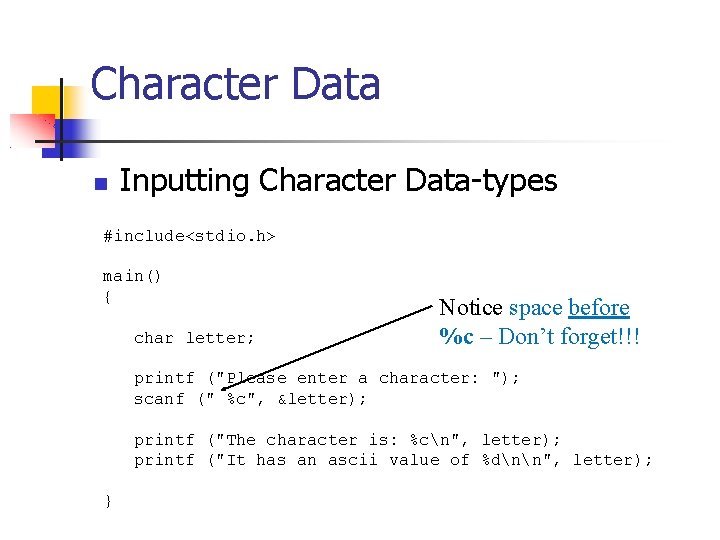
Character Data Inputting Character Data-types #include<stdio. h> main() { char letter; Notice space before %c – Don’t forget!!! printf ("Please enter a character: "); scanf (" %c", &letter); printf ("The character is: %cn", letter); printf ("It has an ascii value of %dnn", letter); }
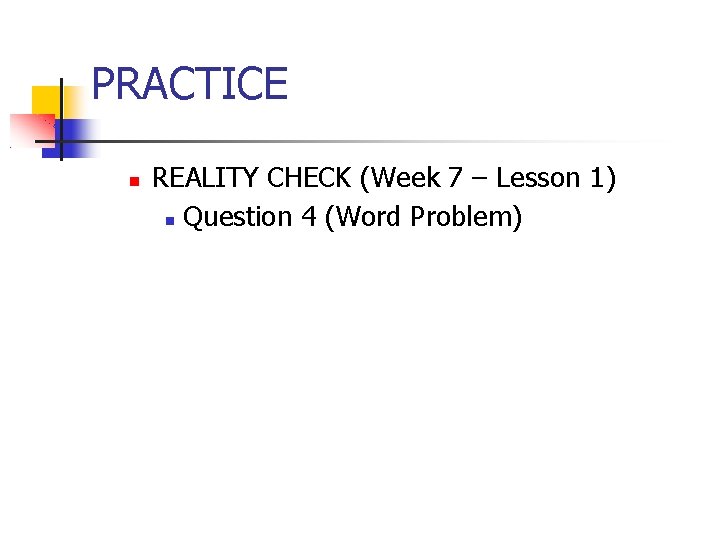
PRACTICE REALITY CHECK (Week 7 – Lesson 1) Question 4 (Word Problem)
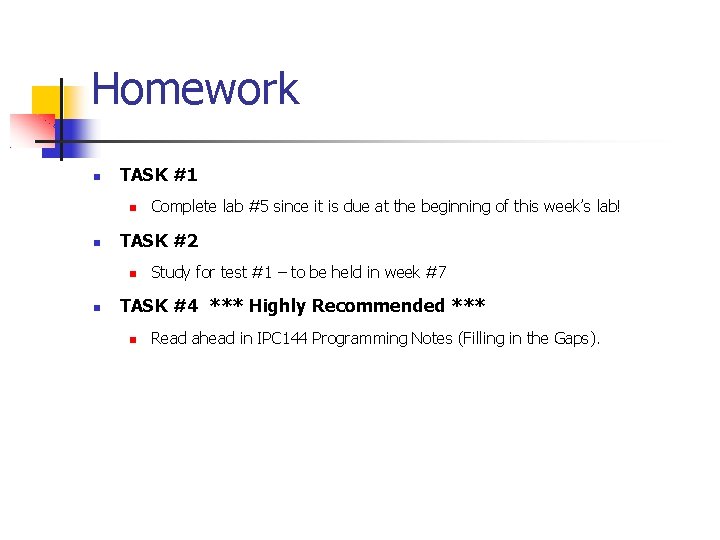
Homework TASK #1 TASK #2 Complete lab #5 since it is due at the beginning of this week’s lab! Study for test #1 – to be held in week #7 TASK #4 *** Highly Recommended *** Read ahead in IPC 144 Programming Notes (Filling in the Gaps).
Ipc144 workshop 6
Ipc java
Solve using the square root property x^2=144
Prime factorization of 450 using exponents
Week by week plans for documenting children's development
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Runtime programming
Integer programming vs linear programming
Definisi integer
Interrupt programming in 8051 examples
Binomial coefficient using dynamic programming
Solving goal programming problems using simplex method
Apprenticeship learning using linear programming
Ipc quality control
Systemv ipc
Acts as a conduit allowing two processes to communicate
Cpc ipc
Ipc-4562