Introduction to Java Script Coding Ron Charest Charest
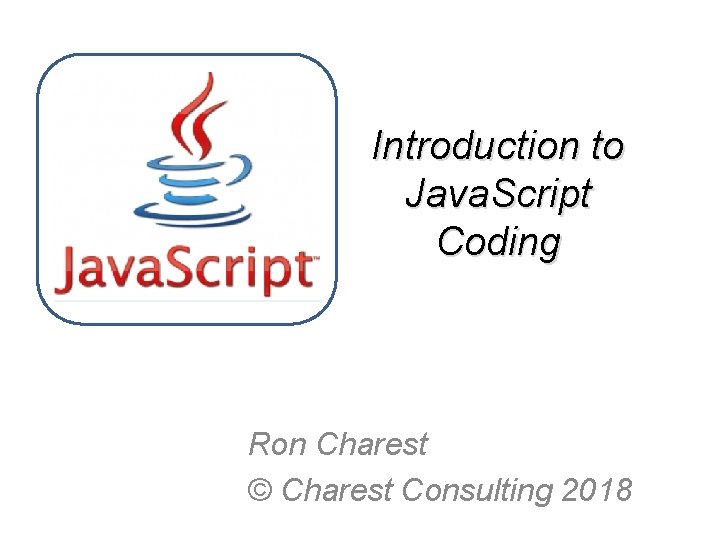
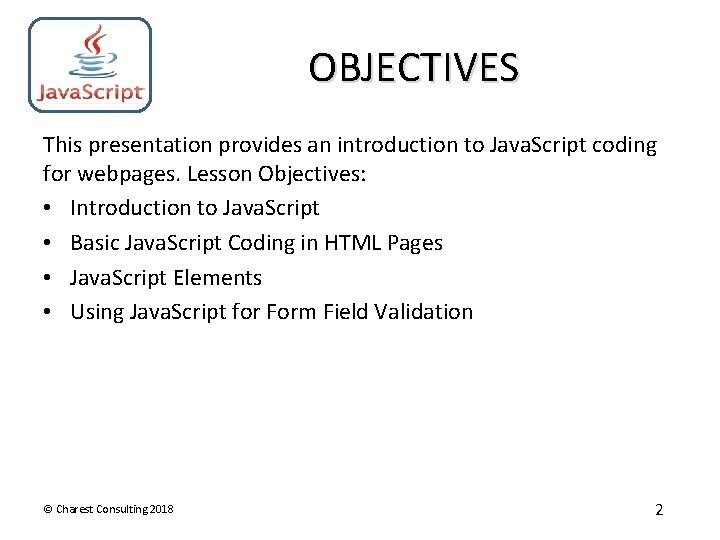
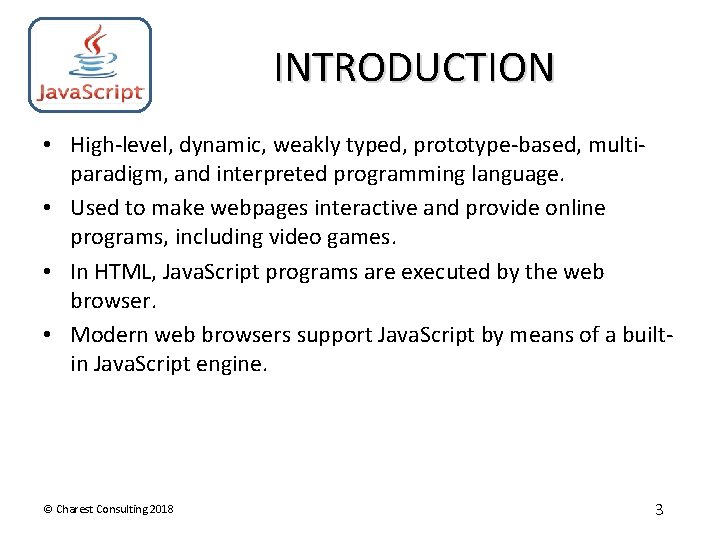
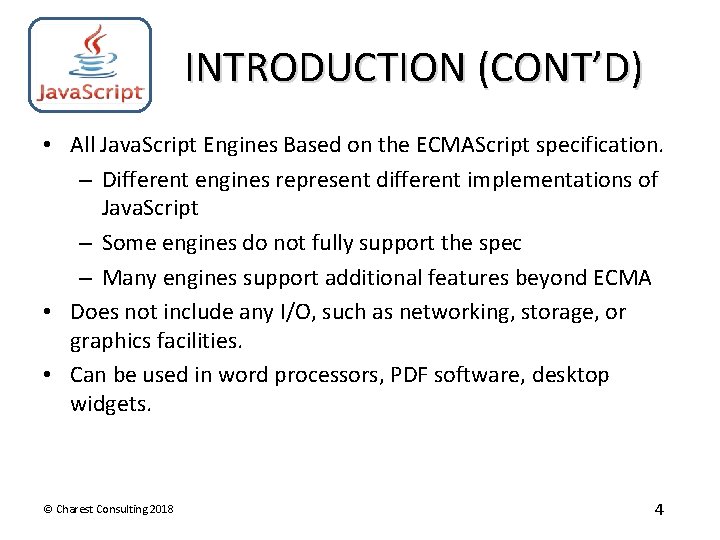
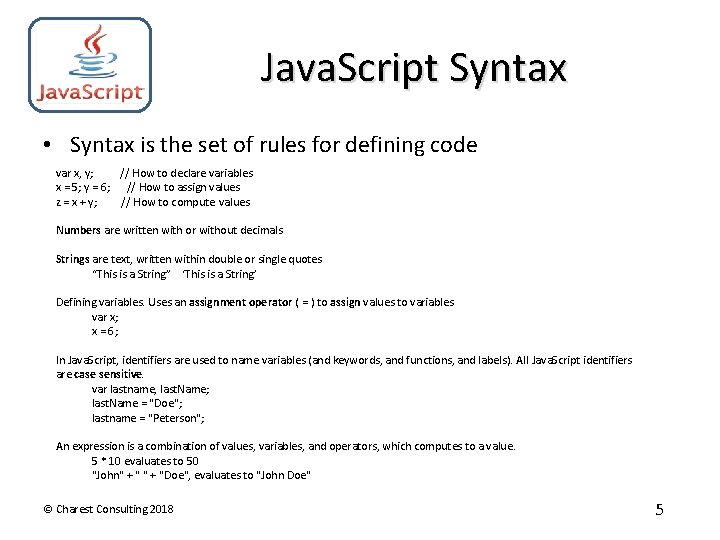
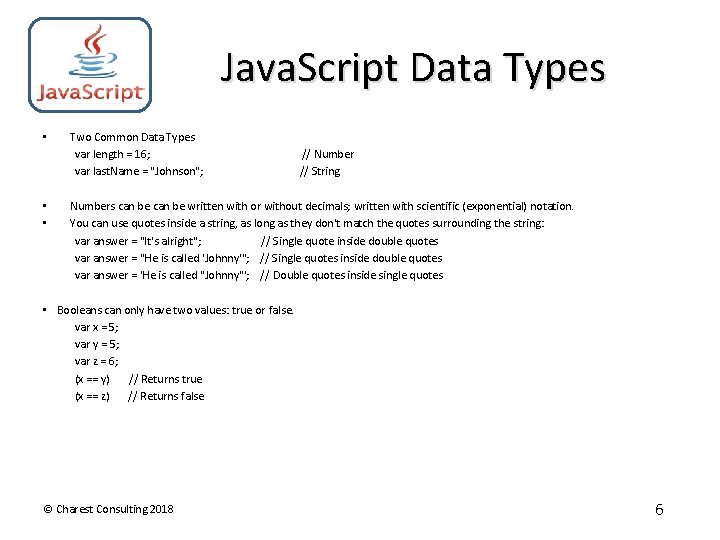
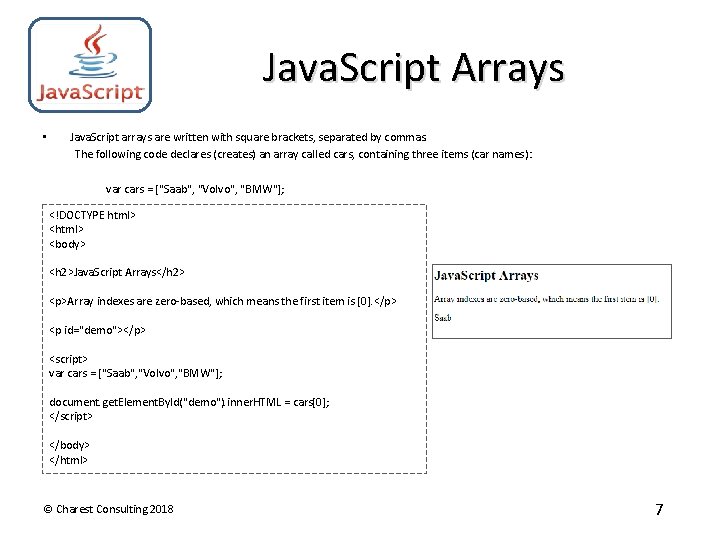
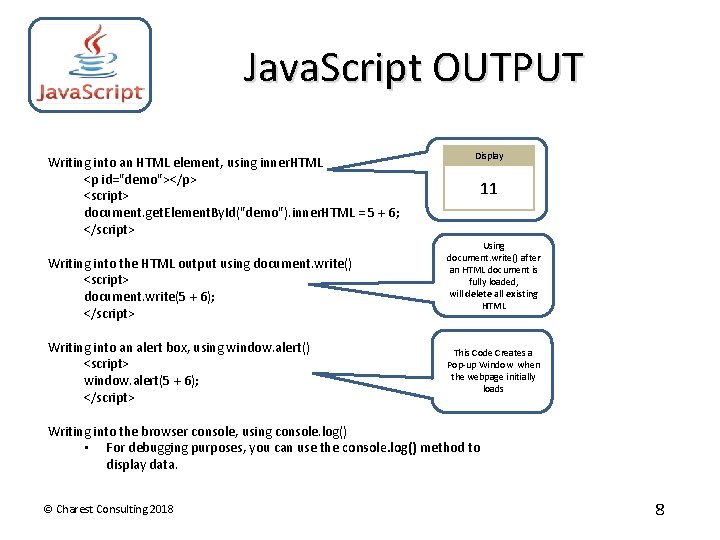
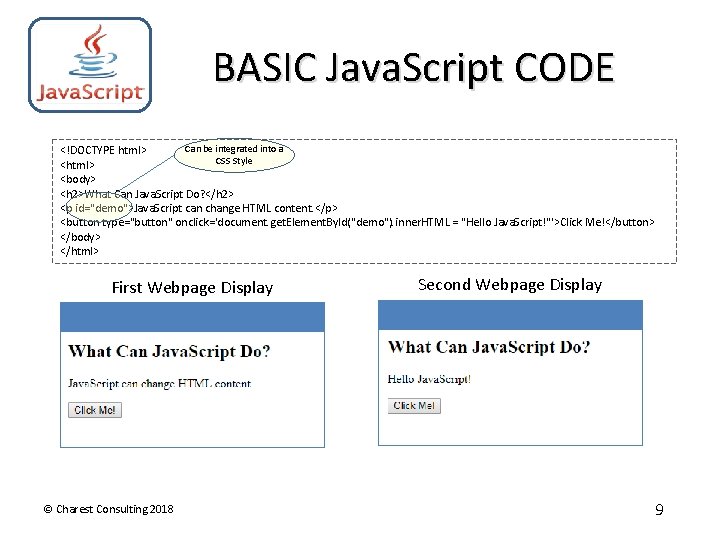
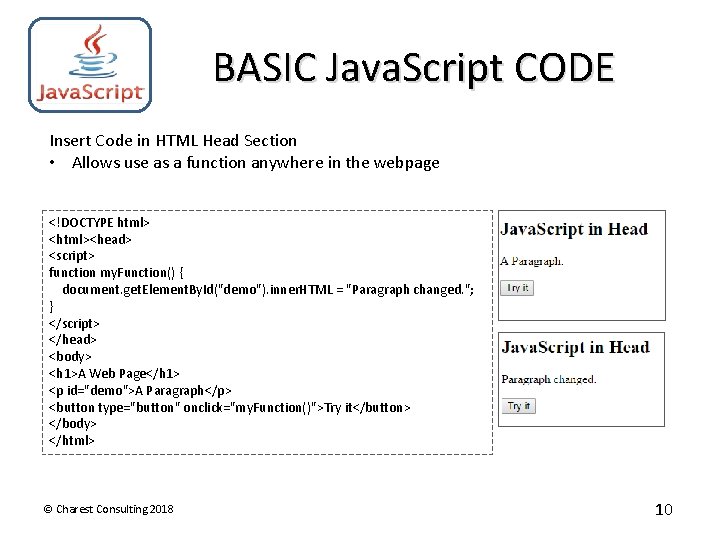
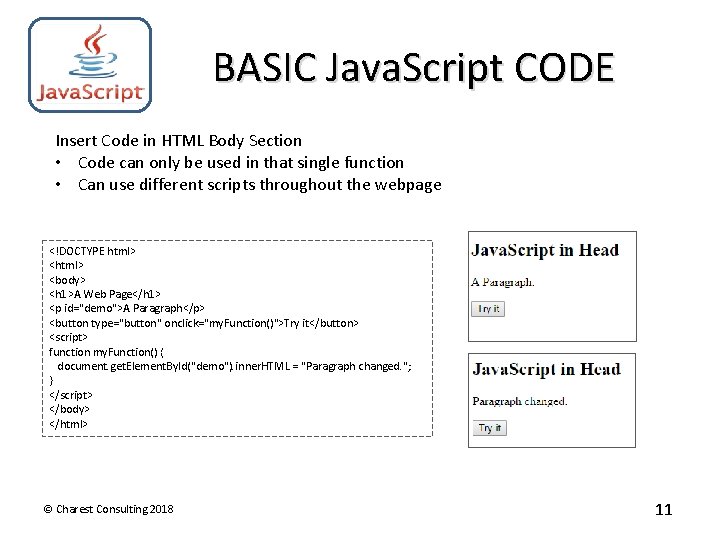
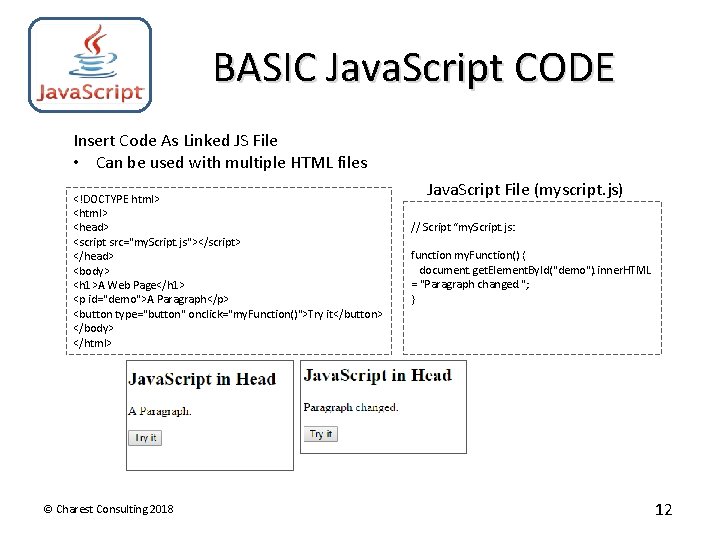
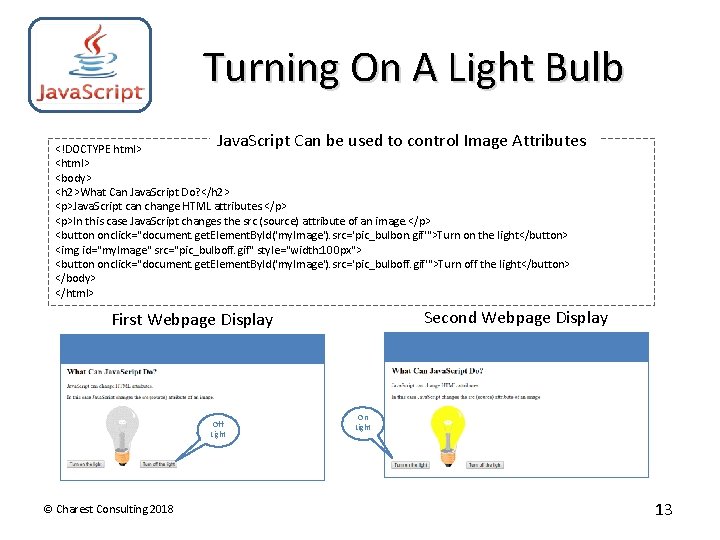
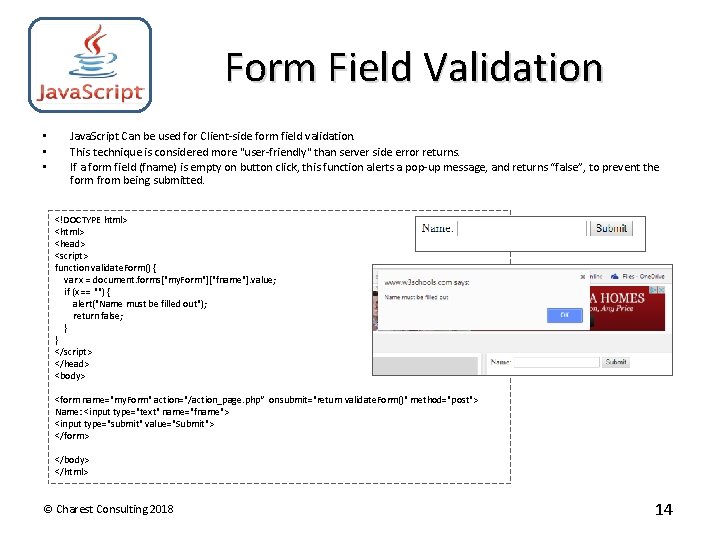
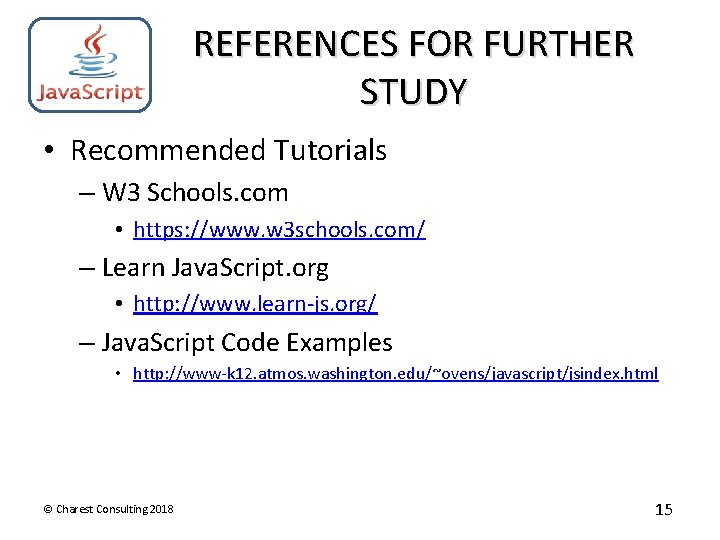
- Slides: 15
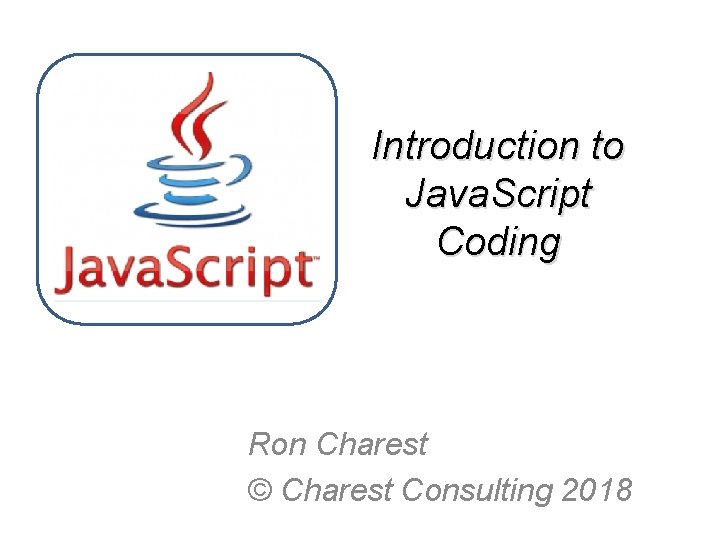
Introduction to Java. Script Coding Ron Charest © Charest Consulting 2018
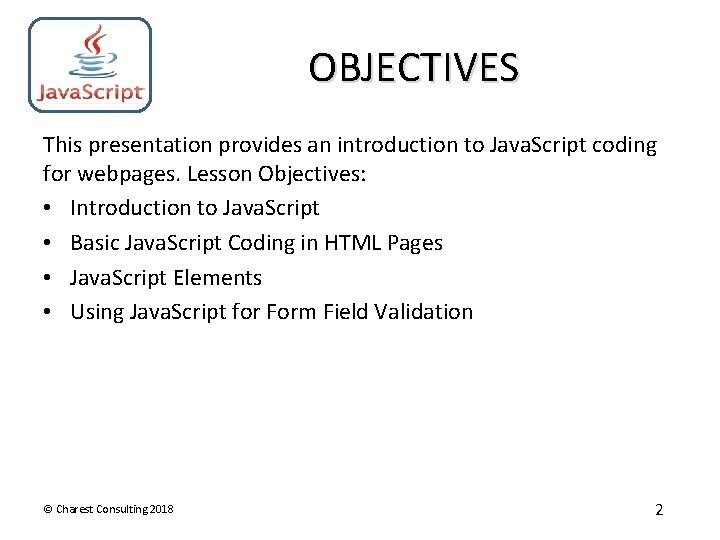
OBJECTIVES This presentation provides an introduction to Java. Script coding for webpages. Lesson Objectives: • Introduction to Java. Script • Basic Java. Script Coding in HTML Pages • Java. Script Elements • Using Java. Script for Form Field Validation © Charest Consulting 2018 2
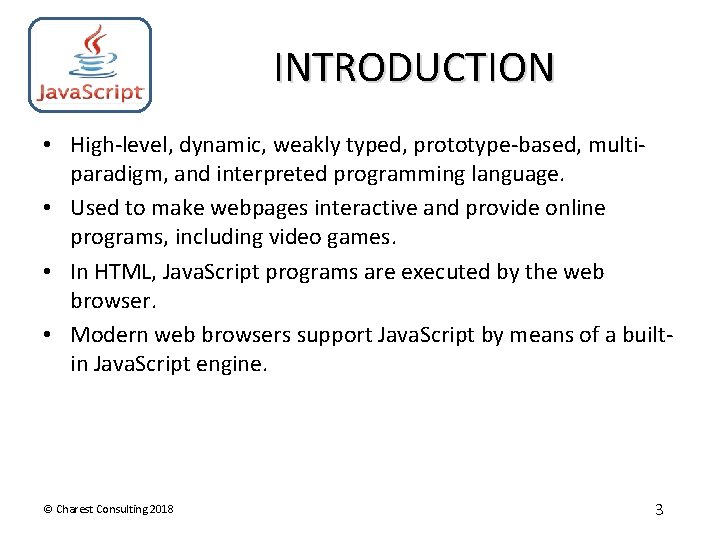
INTRODUCTION • High-level, dynamic, weakly typed, prototype-based, multiparadigm, and interpreted programming language. • Used to make webpages interactive and provide online programs, including video games. • In HTML, Java. Script programs are executed by the web browser. • Modern web browsers support Java. Script by means of a builtin Java. Script engine. © Charest Consulting 2018 3
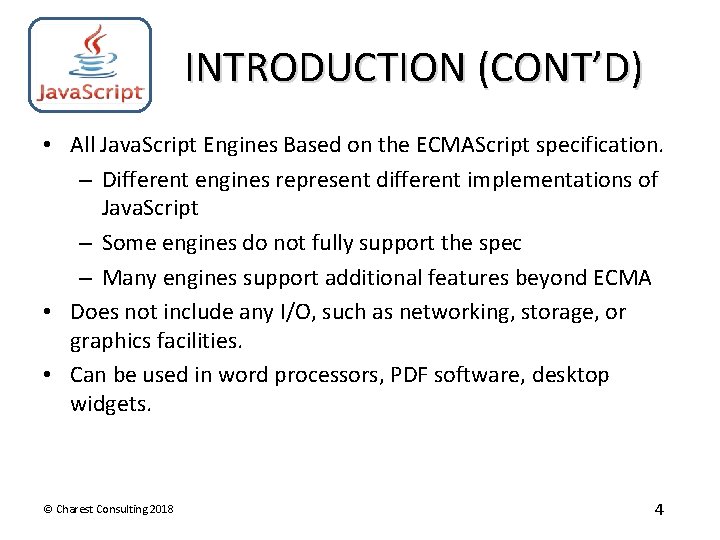
INTRODUCTION (CONT’D) • All Java. Script Engines Based on the ECMAScript specification. – Different engines represent different implementations of Java. Script – Some engines do not fully support the spec – Many engines support additional features beyond ECMA • Does not include any I/O, such as networking, storage, or graphics facilities. • Can be used in word processors, PDF software, desktop widgets. © Charest Consulting 2018 4
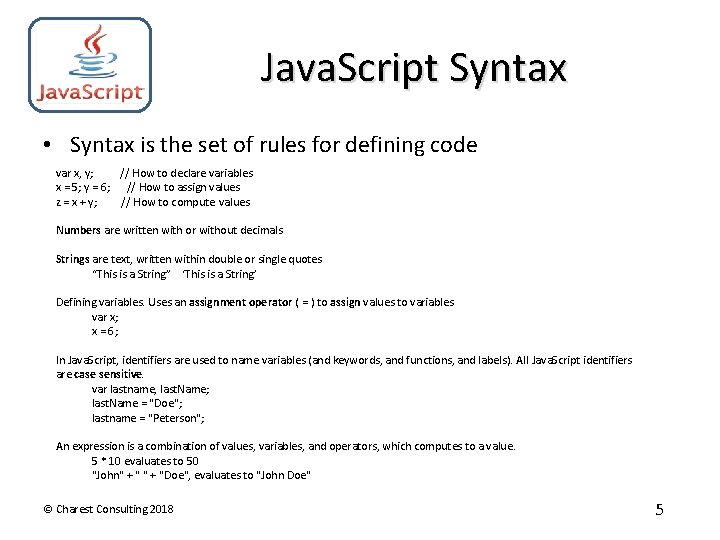
Java. Script Syntax • Syntax is the set of rules for defining code var x, y; // How to declare variables x = 5; y = 6; // How to assign values z = x + y; // How to compute values Numbers are written with or without decimals Strings are text, written within double or single quotes “This is a String” ‘This is a String’ Defining variables. Uses an assignment operator ( = ) to assign values to variables var x; x = 6; In Java. Script, identifiers are used to name variables (and keywords, and functions, and labels). All Java. Script identifiers are case sensitive. var lastname, last. Name; last. Name = "Doe"; lastname = "Peterson"; An expression is a combination of values, variables, and operators, which computes to a value. 5 * 10 evaluates to 50 "John" + "Doe", evaluates to "John Doe" © Charest Consulting 2018 5
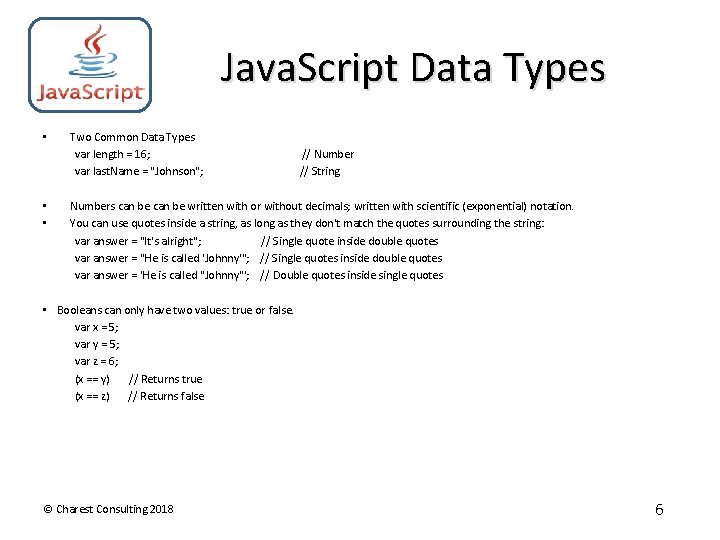
Java. Script Data Types • • • Two Common Data Types var length = 16; var last. Name = "Johnson"; // Number // String Numbers can be written with or without decimals; written with scientific (exponential) notation. You can use quotes inside a string, as long as they don't match the quotes surrounding the string: var answer = "It's alright"; // Single quote inside double quotes var answer = "He is called 'Johnny'"; // Single quotes inside double quotes var answer = 'He is called "Johnny"'; // Double quotes inside single quotes • Booleans can only have two values: true or false. var x = 5; var y = 5; var z = 6; (x == y) // Returns true (x == z) // Returns false © Charest Consulting 2018 6
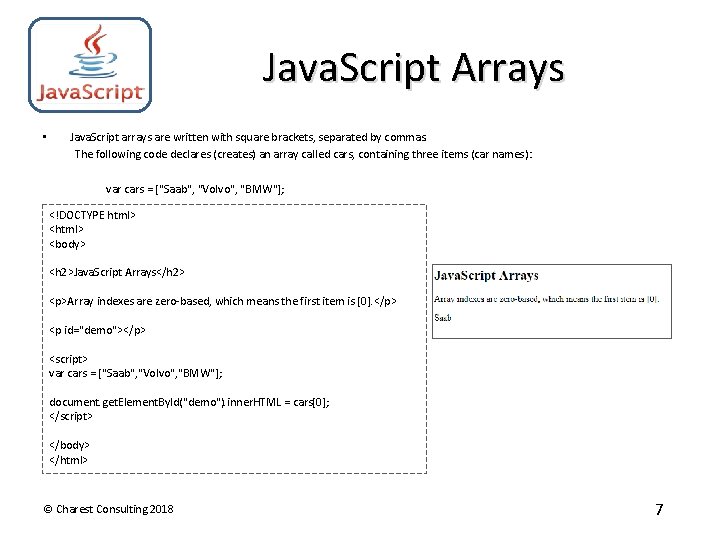
Java. Script Arrays • Java. Script arrays are written with square brackets, separated by commas. The following code declares (creates) an array called cars, containing three items (car names): var cars = ["Saab", "Volvo", "BMW"]; <!DOCTYPE html> <body> <h 2>Java. Script Arrays</h 2> <p>Array indexes are zero-based, which means the first item is [0]. </p> <p id="demo"></p> <script> var cars = ["Saab", "Volvo", "BMW"]; document. get. Element. By. Id("demo"). inner. HTML = cars[0]; </script> </body> </html> © Charest Consulting 2018 7
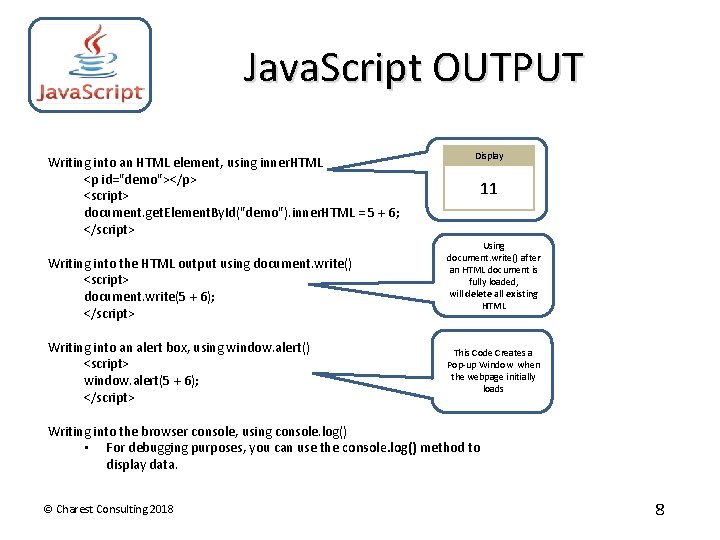
Java. Script OUTPUT Writing into an HTML element, using inner. HTML <p id="demo"></p> <script> document. get. Element. By. Id("demo"). inner. HTML = 5 + 6; </script> Writing into the HTML output using document. write() <script> document. write(5 + 6); </script> Writing into an alert box, using window. alert() <script> window. alert(5 + 6); </script> Display 11 Using document. write() after an HTML document is fully loaded, will delete all existing HTML This Code Creates a Pop-up Window when the webpage initially loads Writing into the browser console, using console. log() • For debugging purposes, you can use the console. log() method to display data. © Charest Consulting 2018 8
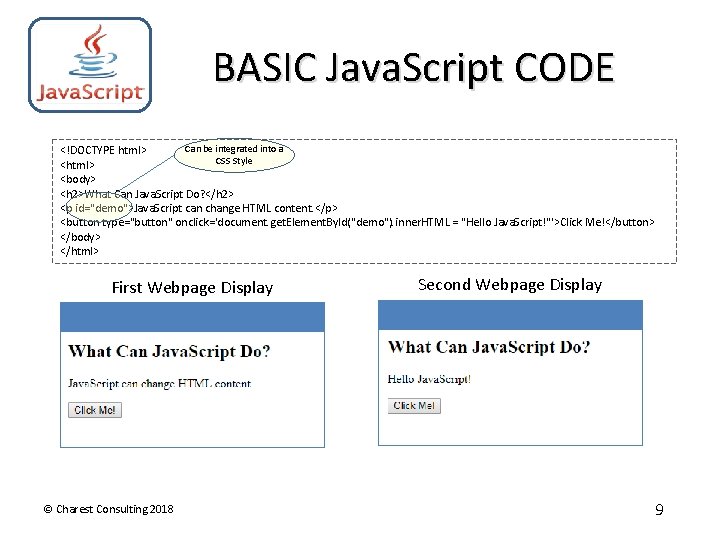
BASIC Java. Script CODE Can be integrated into a <!DOCTYPE html> CSS Style <html> <body> <h 2>What Can Java. Script Do? </h 2> <p id="demo">Java. Script can change HTML content. </p> <button type="button" onclick='document. get. Element. By. Id("demo"). inner. HTML = "Hello Java. Script!"'>Click Me!</button> </body> </html> First Webpage Display © Charest Consulting 2018 Second Webpage Display 9
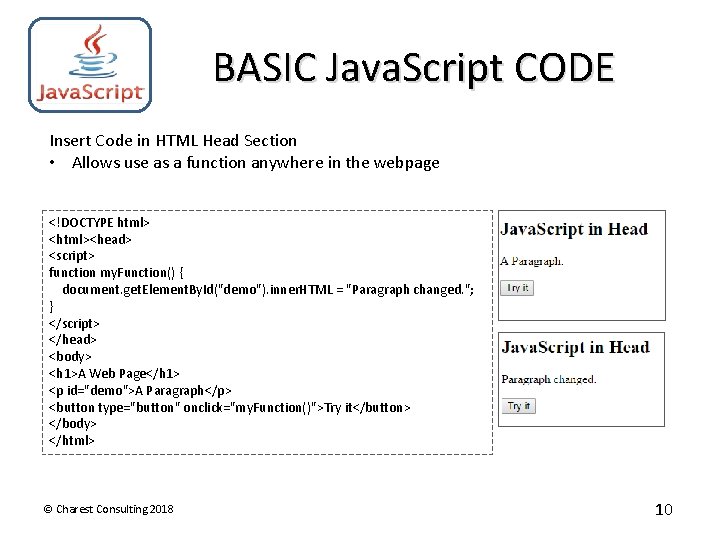
BASIC Java. Script CODE Insert Code in HTML Head Section • Allows use as a function anywhere in the webpage <!DOCTYPE html> <html><head> <script> function my. Function() { document. get. Element. By. Id("demo"). inner. HTML = "Paragraph changed. "; } </script> </head> <body> <h 1>A Web Page</h 1> <p id="demo">A Paragraph</p> <button type="button" onclick="my. Function()">Try it</button> </body> </html> © Charest Consulting 2018 10
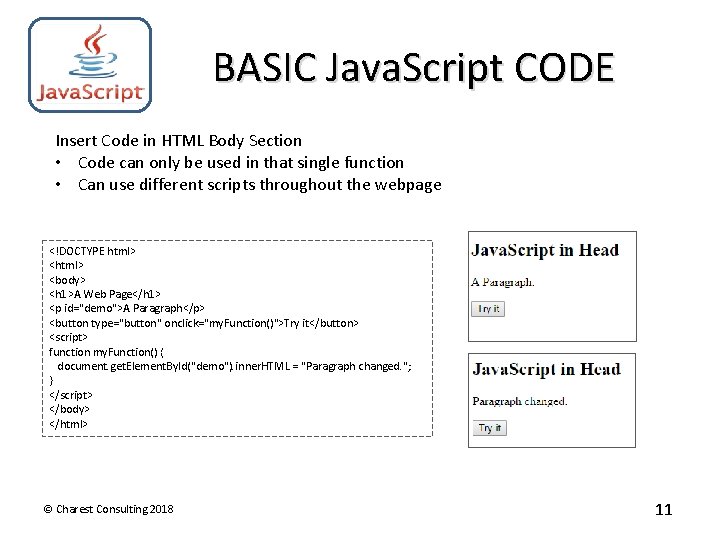
BASIC Java. Script CODE Insert Code in HTML Body Section • Code can only be used in that single function • Can use different scripts throughout the webpage <!DOCTYPE html> <body> <h 1>A Web Page</h 1> <p id="demo">A Paragraph</p> <button type="button" onclick="my. Function()">Try it</button> <script> function my. Function() { document. get. Element. By. Id("demo"). inner. HTML = "Paragraph changed. "; } </script> </body> </html> © Charest Consulting 2018 11
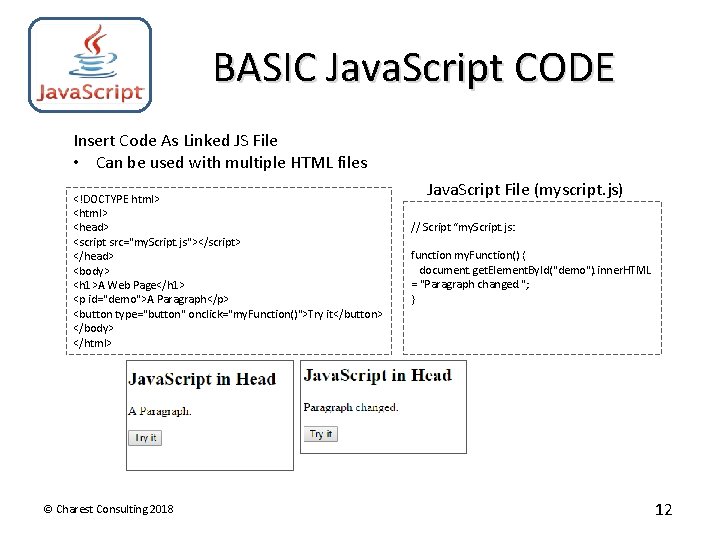
BASIC Java. Script CODE Insert Code As Linked JS File • Can be used with multiple HTML files <!DOCTYPE html> <head> <script src="my. Script. js"></script> </head> <body> <h 1>A Web Page</h 1> <p id="demo">A Paragraph</p> <button type="button" onclick="my. Function()">Try it</button> </body> </html> © Charest Consulting 2018 Java. Script File (myscript. js) // Script “my. Script. js: function my. Function() { document. get. Element. By. Id("demo"). inner. HTML = "Paragraph changed. "; } 12
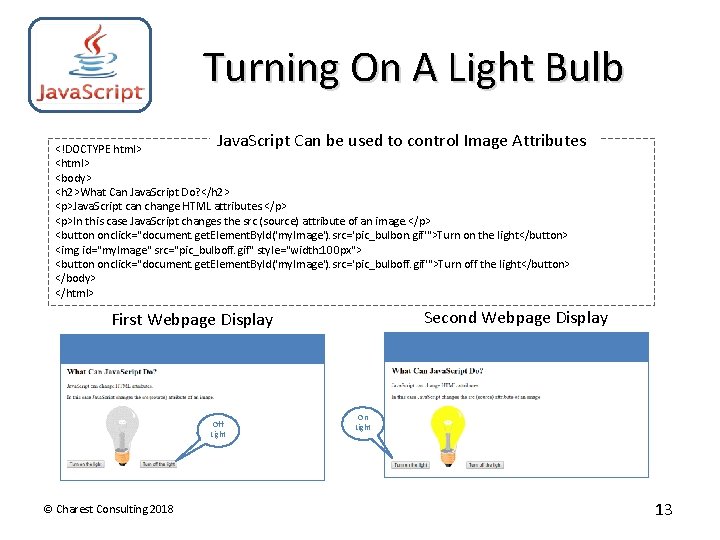
Turning On A Light Bulb Java. Script Can be used to control Image Attributes <!DOCTYPE html> <body> <h 2>What Can Java. Script Do? </h 2> <p>Java. Script can change HTML attributes. </p> <p>In this case Java. Script changes the src (source) attribute of an image. </p> <button onclick="document. get. Element. By. Id('my. Image'). src='pic_bulbon. gif'">Turn on the light</button> <img id="my. Image" src="pic_bulboff. gif" style="width: 100 px"> <button onclick="document. get. Element. By. Id('my. Image'). src='pic_bulboff. gif'">Turn off the light</button> </body> </html> Second Webpage Display First Webpage Display Off Light © Charest Consulting 2018 On Light 13
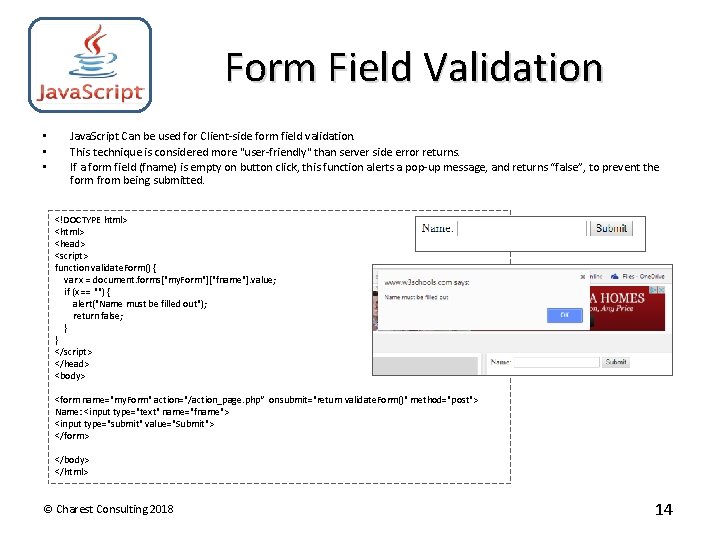
Form Field Validation • • • Java. Script Can be used for Client-side form field validation. This technique is considered more "user-friendly" than server side error returns. If a form field (fname) is empty on button click, this function alerts a pop-up message, and returns “false”, to prevent the form from being submitted. <!DOCTYPE html> <head> <script> function validate. Form() { var x = document. forms["my. Form"]["fname"]. value; if (x == "") { alert("Name must be filled out"); return false; } } </script> </head> <body> <form name="my. Form" action="/action_page. php“ onsubmit="return validate. Form()" method="post"> Name: <input type="text" name="fname"> <input type="submit" value="Submit"> </form> </body> </html> © Charest Consulting 2018 14
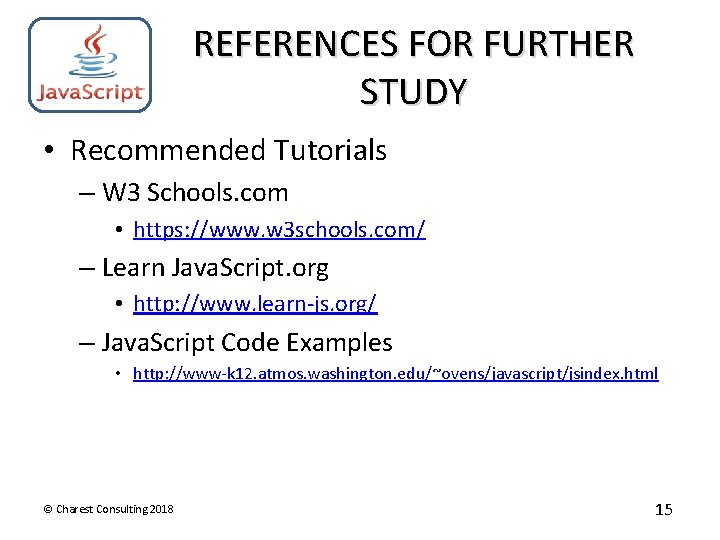
REFERENCES FOR FURTHER STUDY • Recommended Tutorials – W 3 Schools. com • https: //www. w 3 schools. com/ – Learn Java. Script. org • http: //www. learn-js. org/ – Java. Script Code Examples • http: //www-k 12. atmos. washington. edu/~ovens/javascript/jsindex. html © Charest Consulting 2018 15
Open coding axial coding selective coding adalah
Contoh open coding, axial coding selective coding
Axial coding vs open coding
Coding dna and non coding dna
Vanessa charest
Nicole charest
Nicole charest
Nicole charest
Nicole charest
Nicole charest
Nathalie charest
Java secure coding
Java code playground
Java code review checklist pdf
Script de java
Script java