Input and Output Using Text Files and Exception
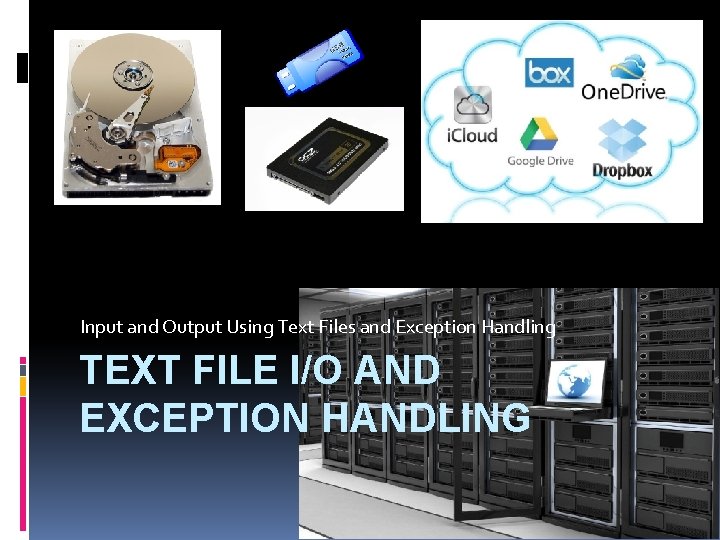
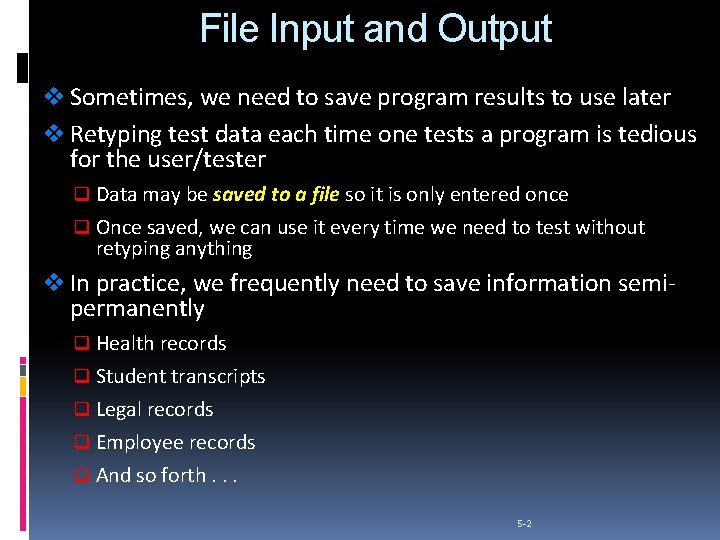
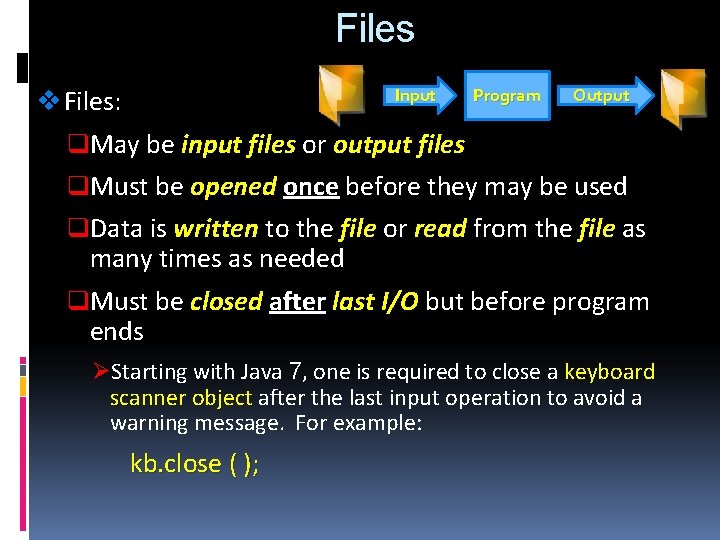
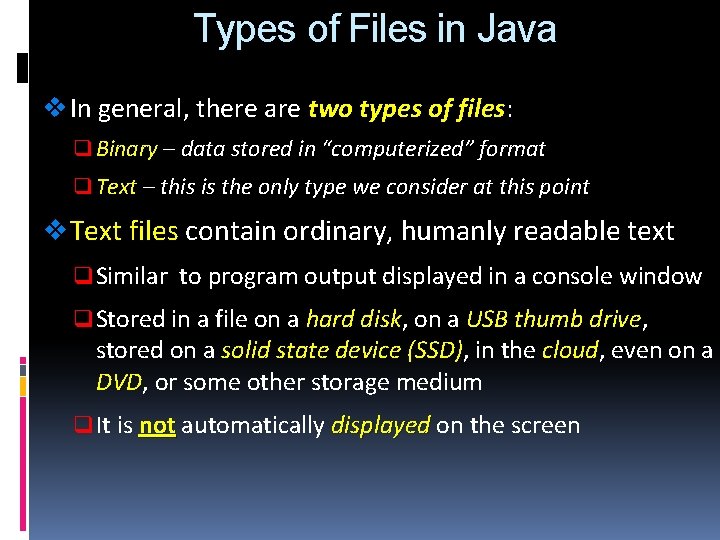
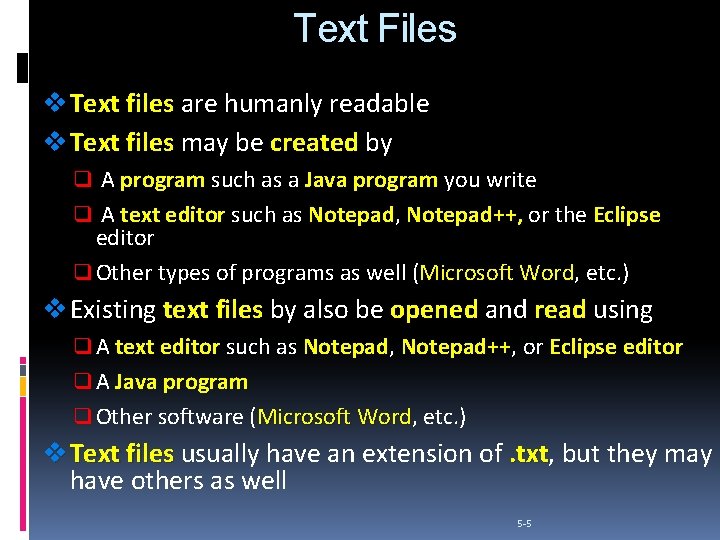
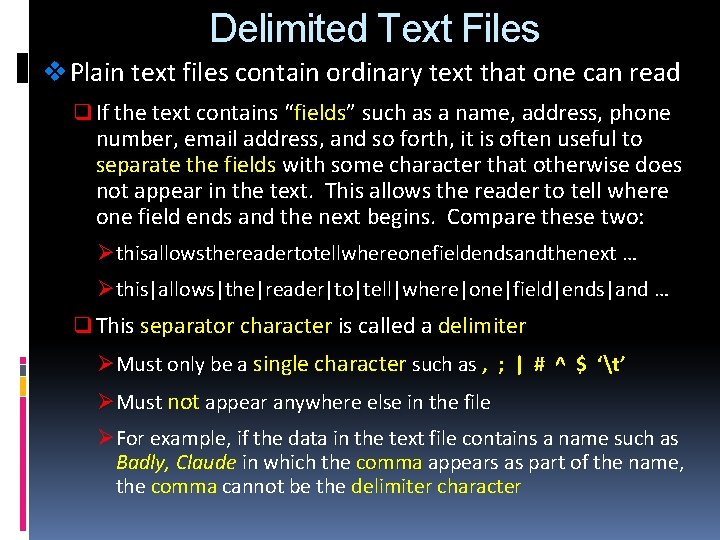
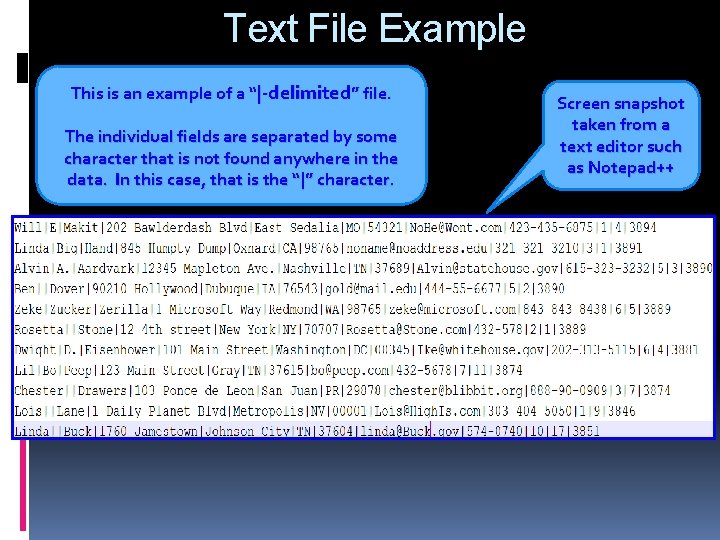
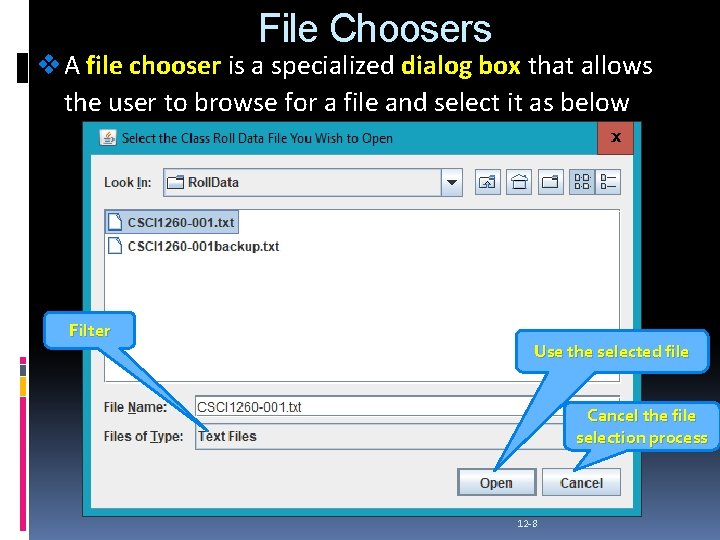
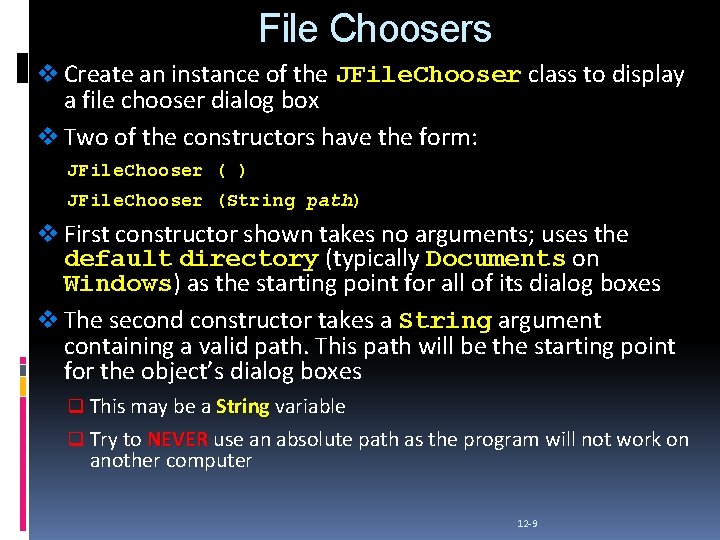
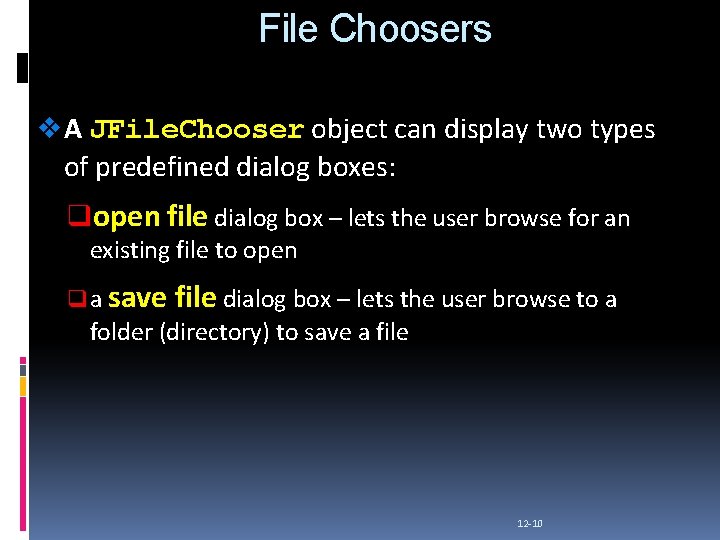
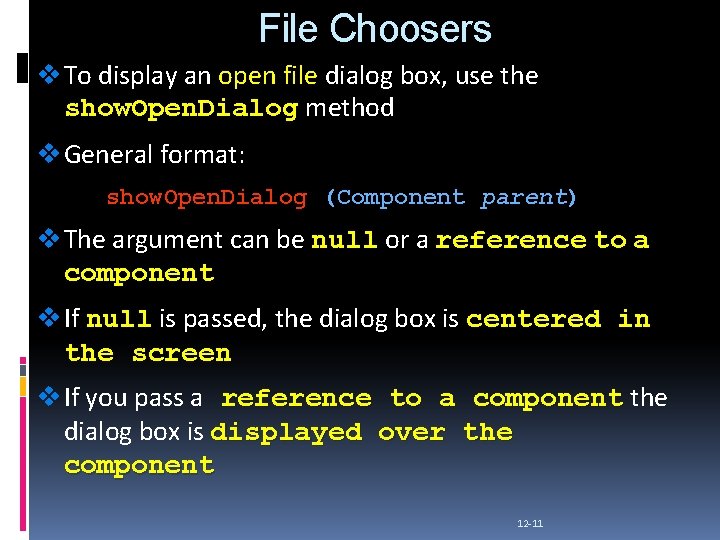
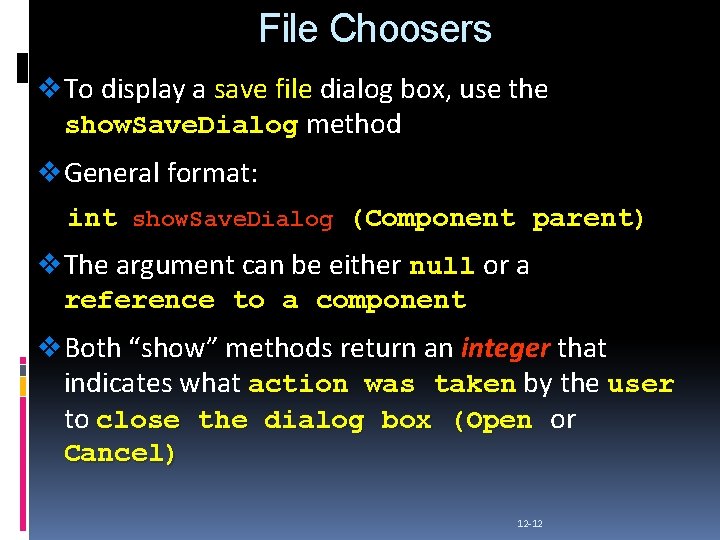
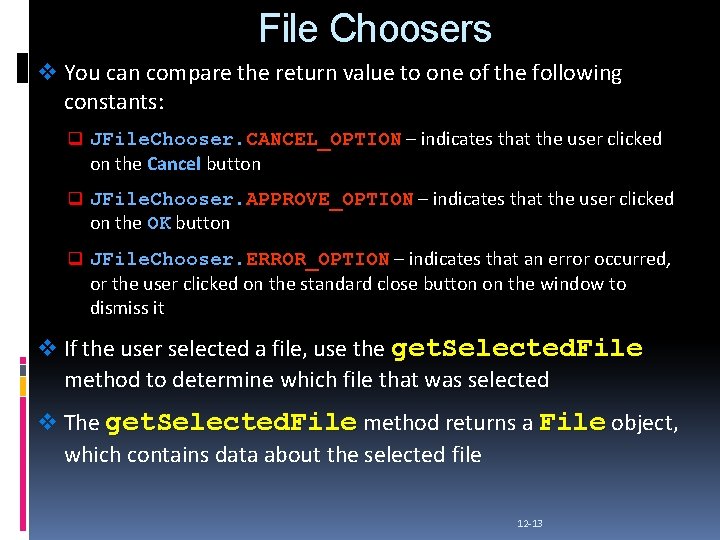
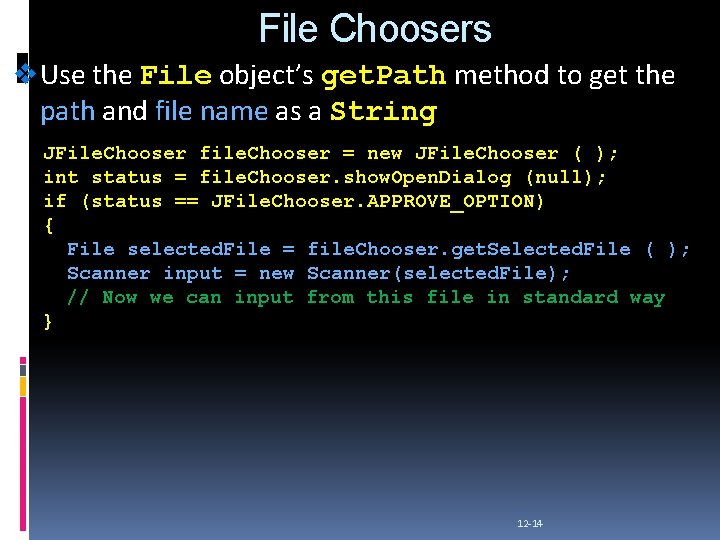
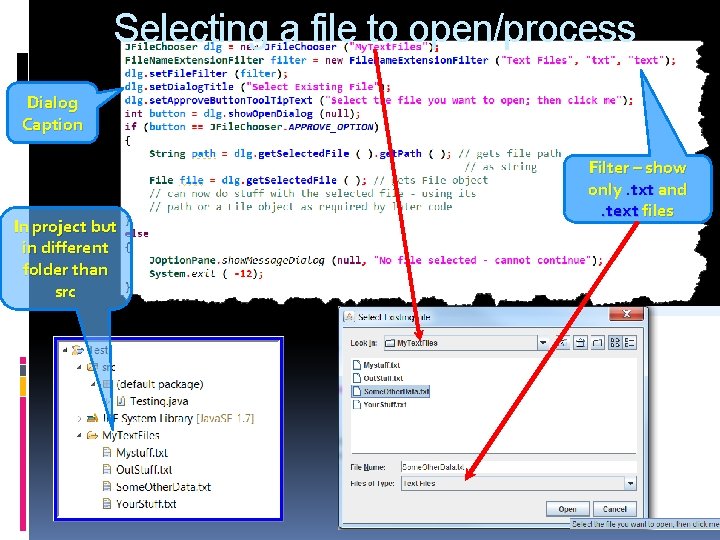
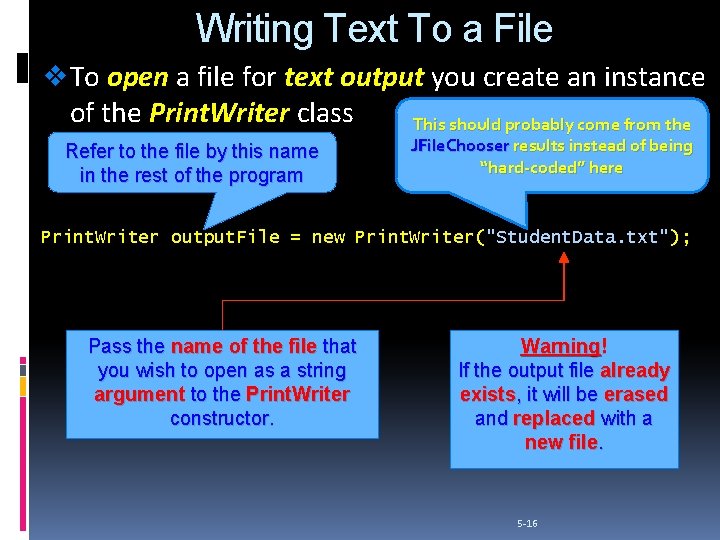
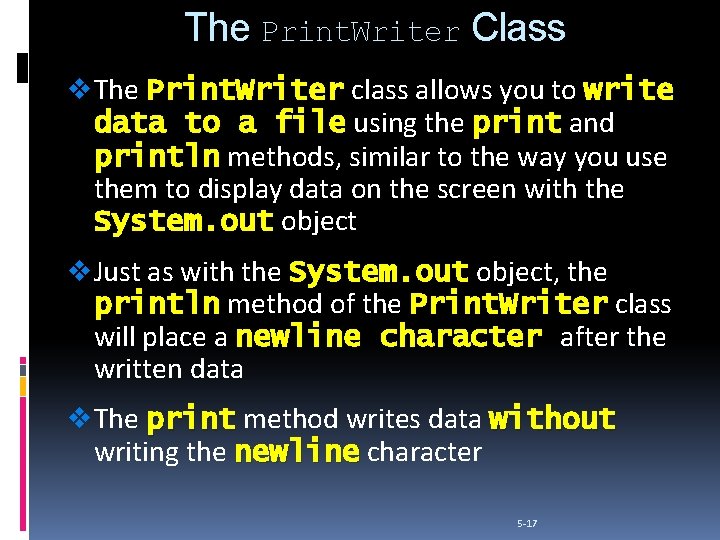
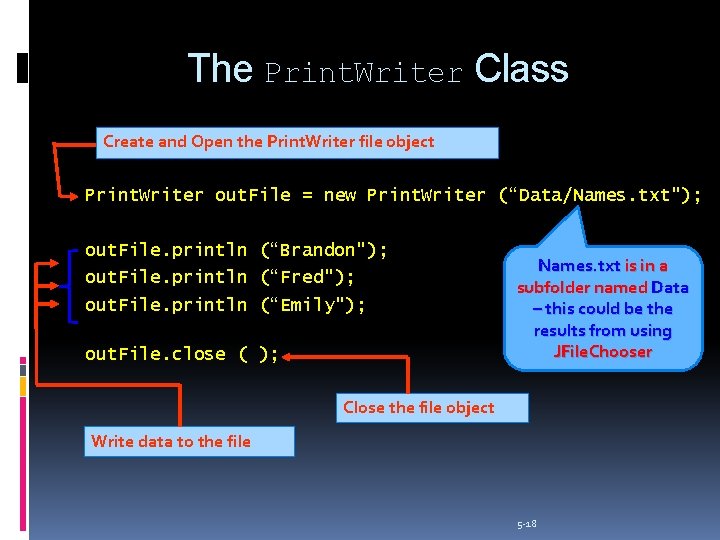
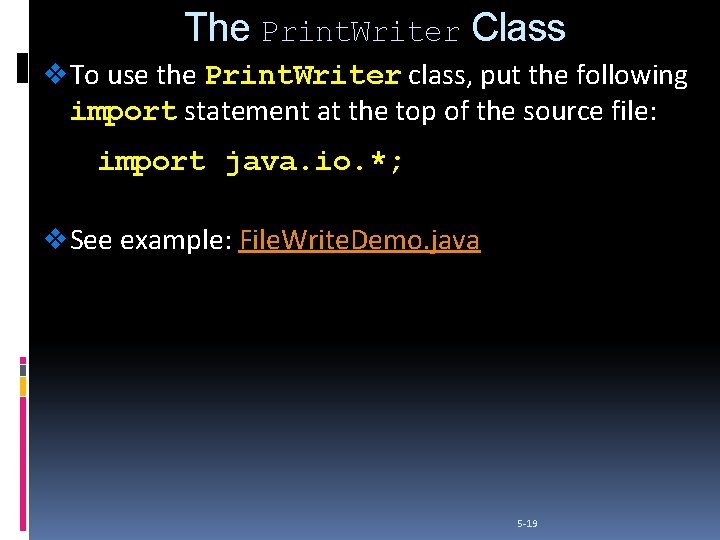
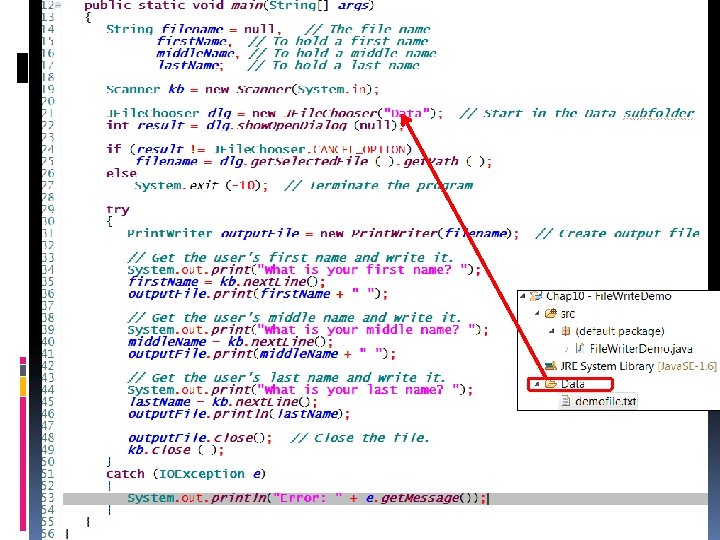
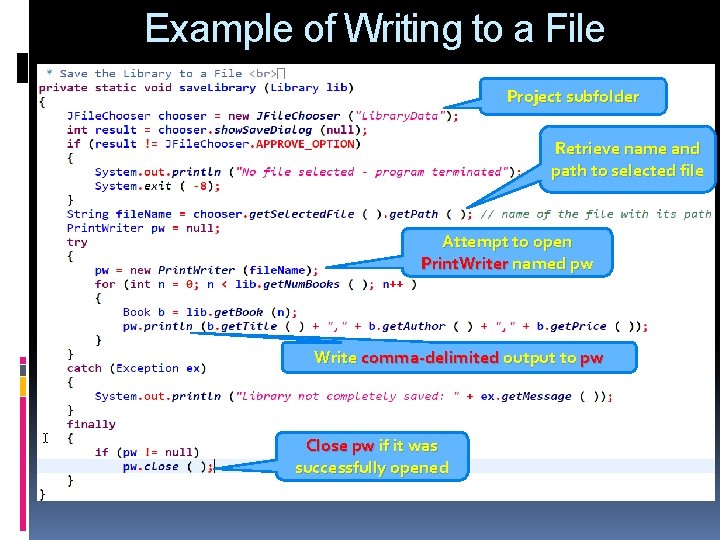
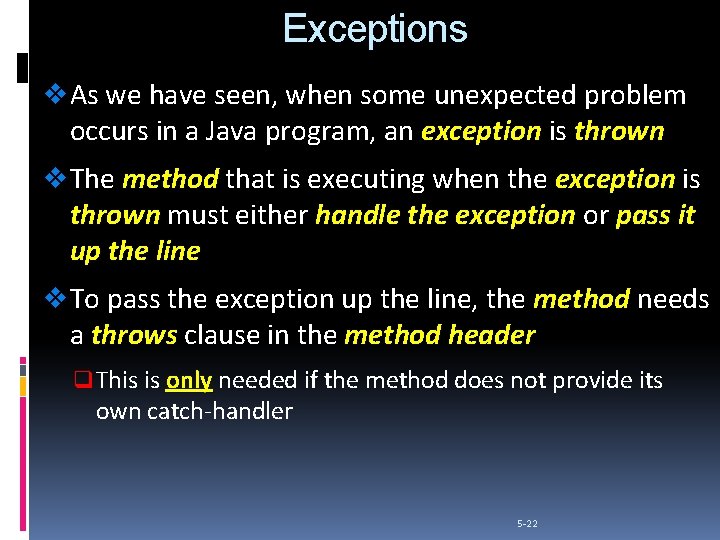
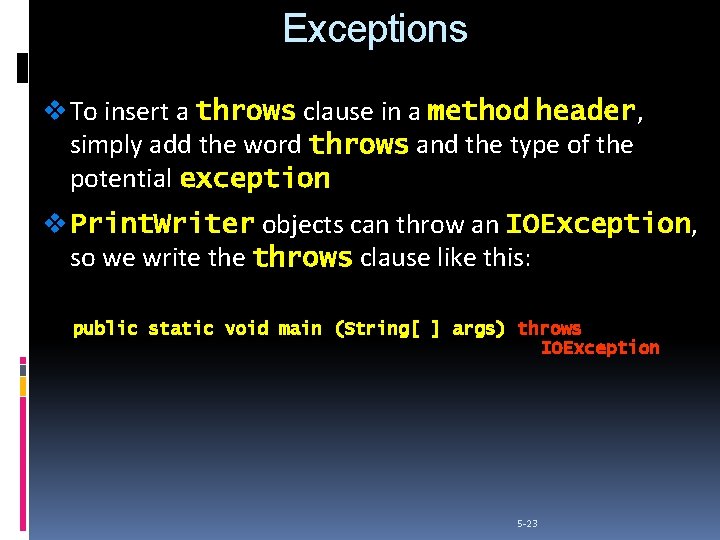
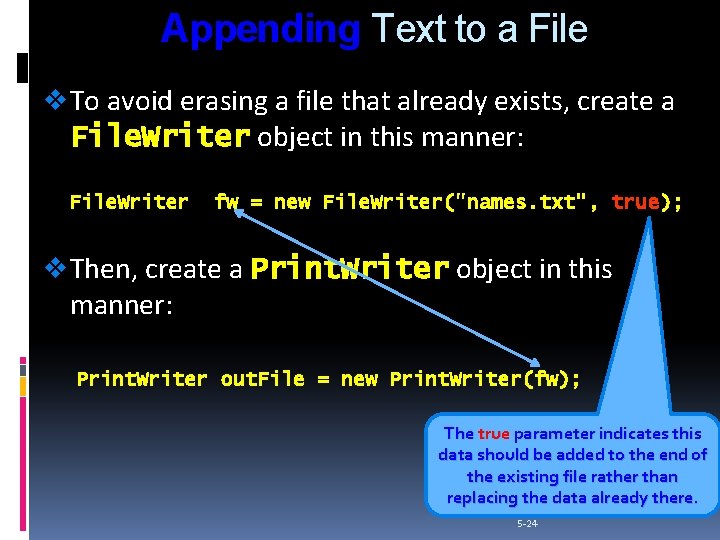
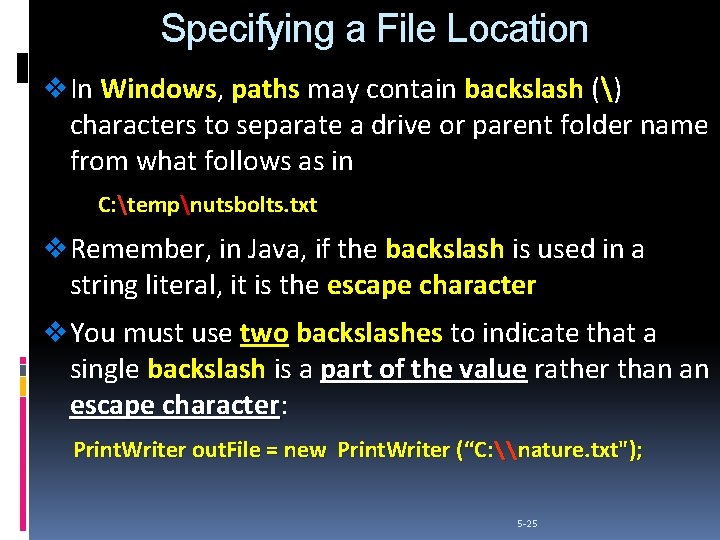
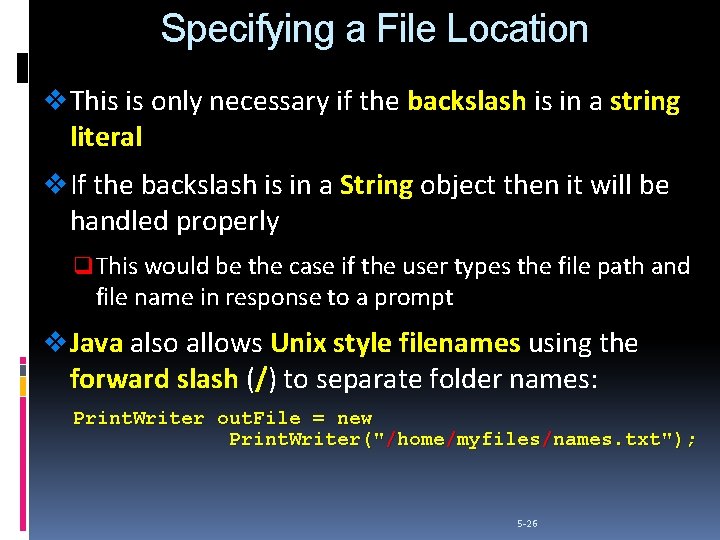
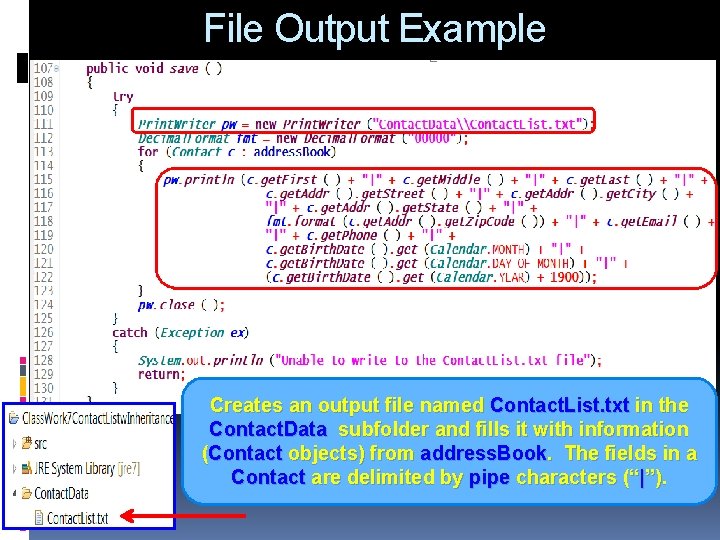
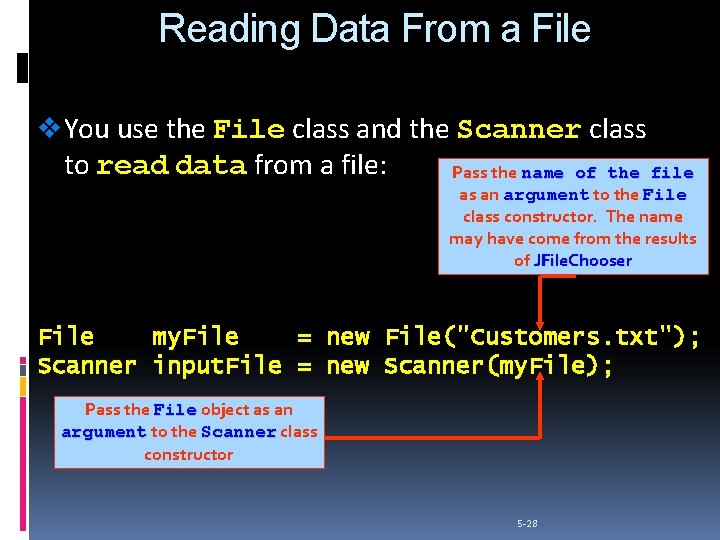
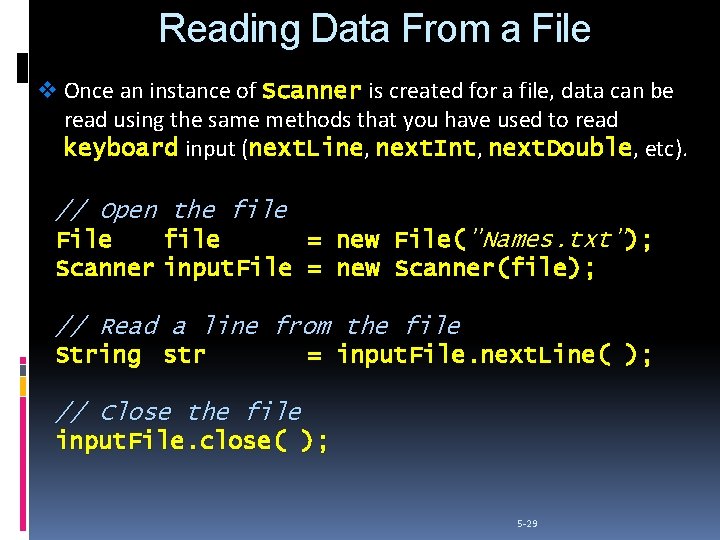
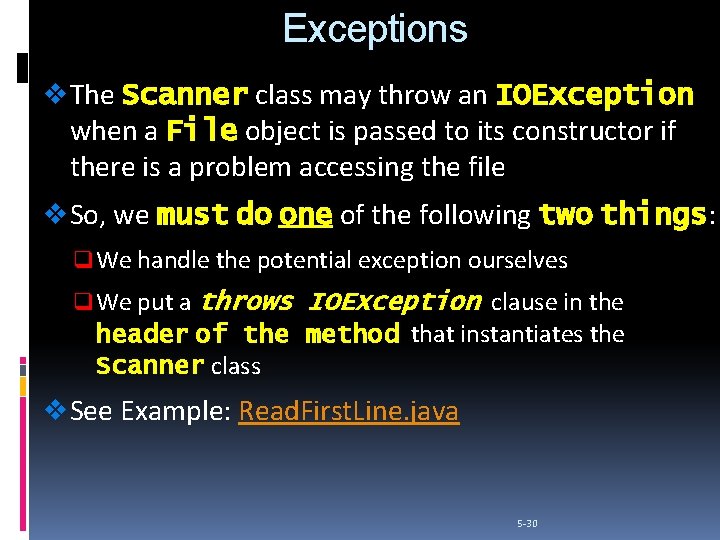
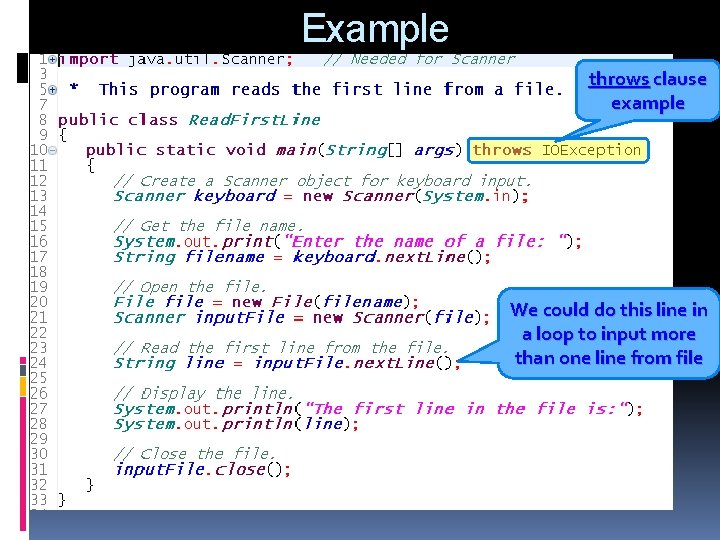
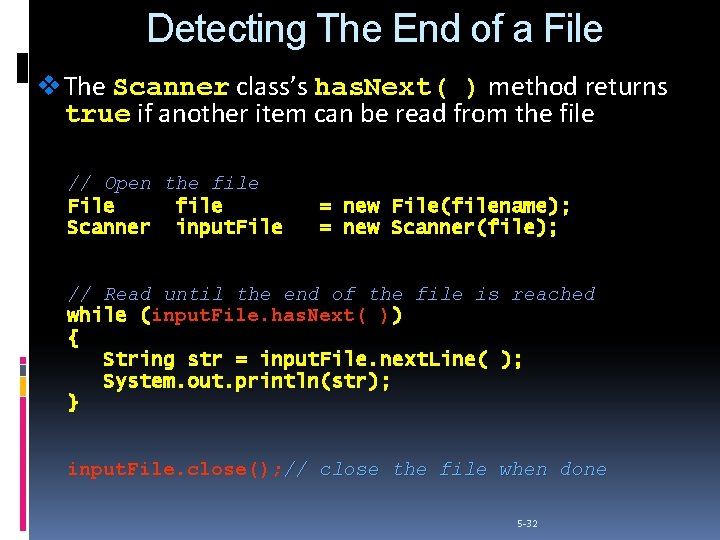
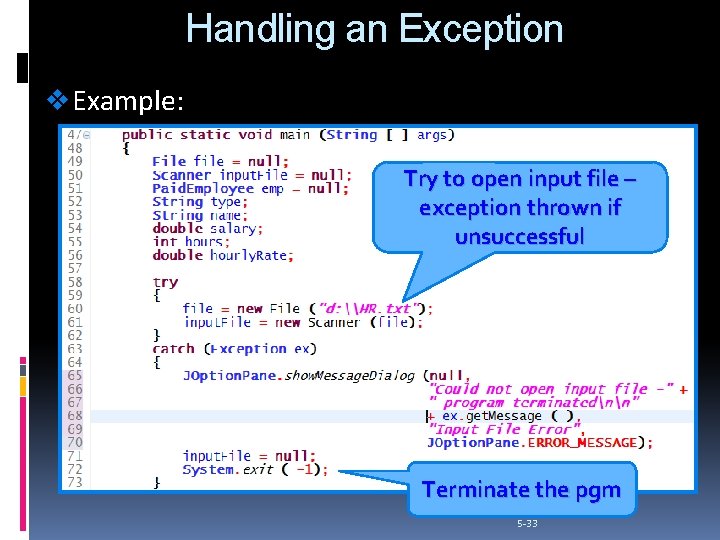
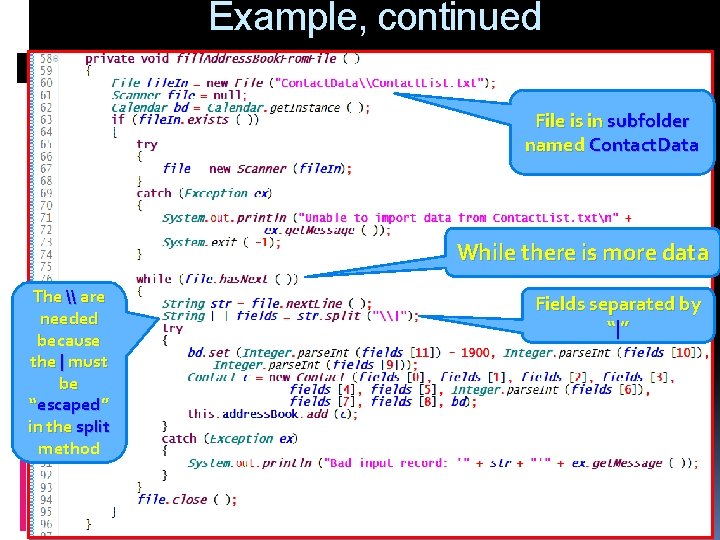
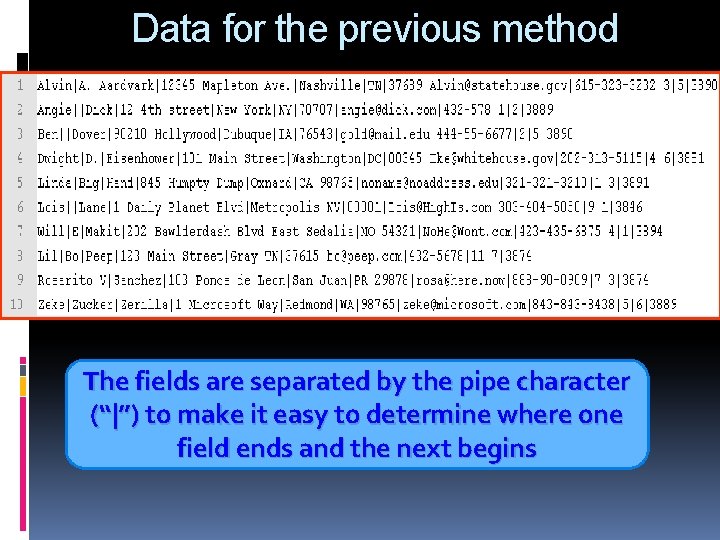
- Slides: 35
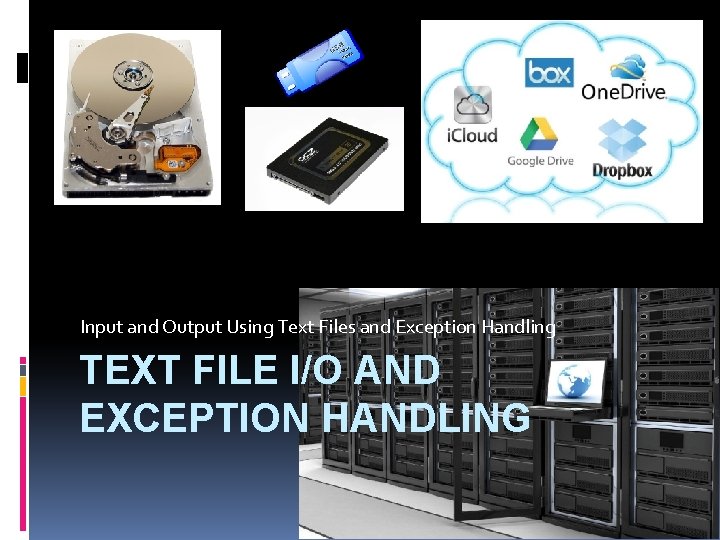
Input and Output Using Text Files and Exception Handling TEXT FILE I/O AND EXCEPTION HANDLING
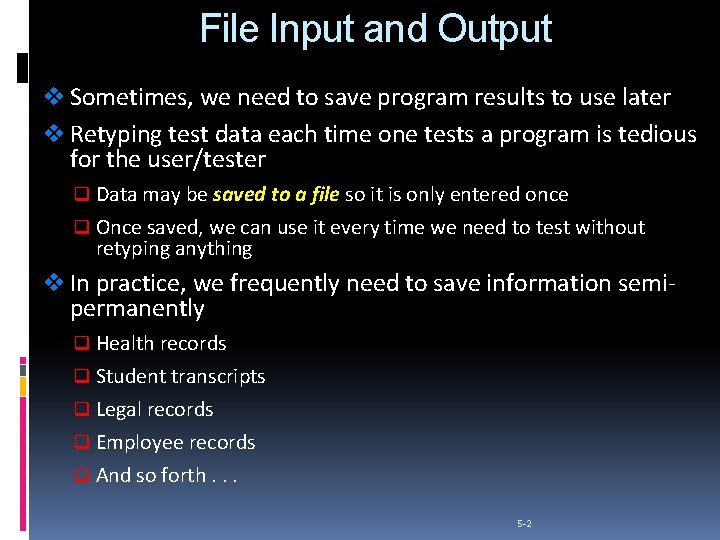
File Input and Output v Sometimes, we need to save program results to use later v Retyping test data each time one tests a program is tedious for the user/tester q Data may be saved to a file so it is only entered once q Once saved, we can use it every time we need to test without retyping anything v In practice, we frequently need to save information semipermanently q Health records q Student transcripts q Legal records q Employee records q And so forth. . . 5 -2
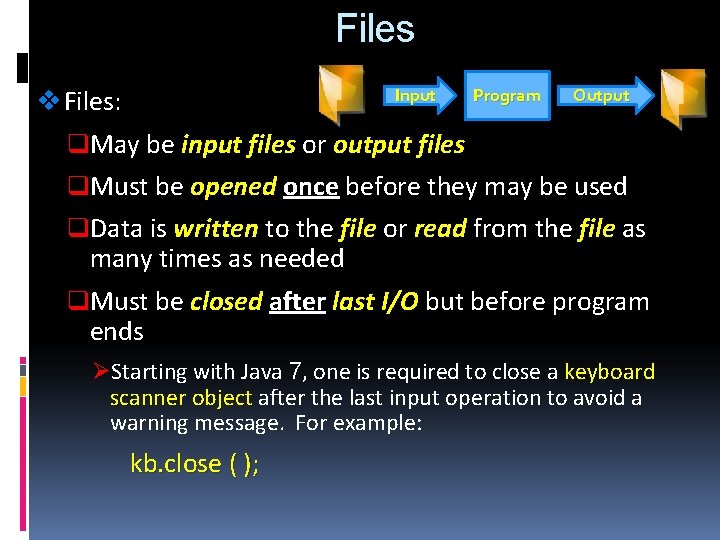
Files v Files: Input Program Output q. May be input files or output files q. Must be opened once before they may be used q. Data is written to the file or read from the file as many times as needed q. Must be closed after last I/O but before program ends ØStarting with Java 7, one is required to close a keyboard scanner object after the last input operation to avoid a warning message. For example: kb. close ( );
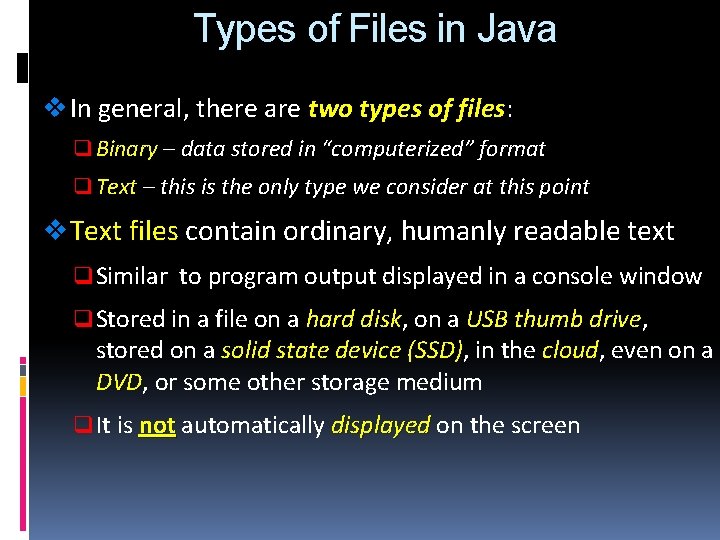
Types of Files in Java v In general, there are two types of files: files q Binary – data stored in “computerized” format q Text – this is the only type we consider at this point v Text files contain ordinary, humanly readable text q Similar to program output displayed in a console window q Stored in a file on a hard disk, disk on a USB thumb drive, drive stored on a solid state device (SSD), (SSD) in the cloud, cloud even on a DVD, DVD or some other storage medium q It is not automatically displayed on the screen
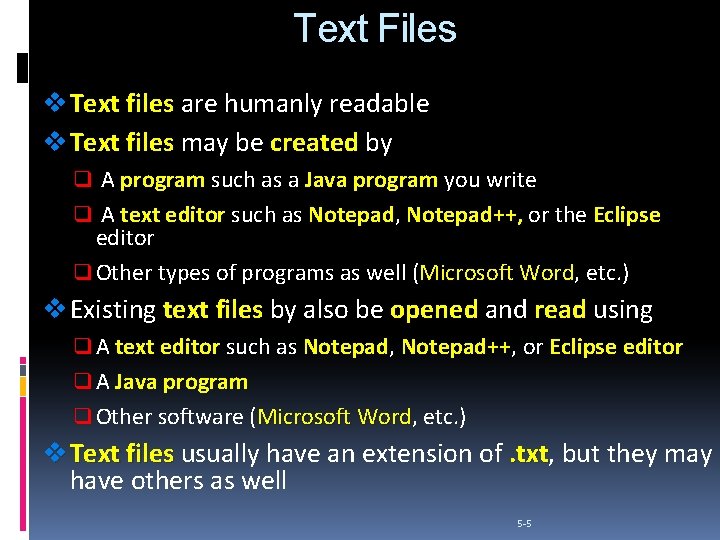
Text Files v Text files are humanly readable v Text files may be created by q A program such as a Java program you write q A text editor such as Notepad, Notepad++, or the Eclipse editor q Other types of programs as well (Microsoft Word, Word etc. ) v Existing text files by also be opened and read using q A text editor such as Notepad, Notepad++, Notepad++ or Eclipse editor q A Java program q Other software (Microsoft Word, Word etc. ) v Text files usually have an extension of. txt, . txt but they may have others as well 5 -5
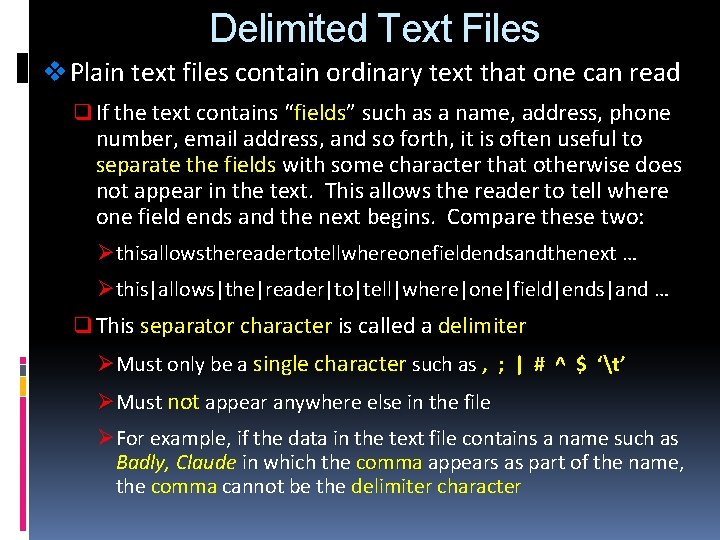
Delimited Text Files v Plain text files contain ordinary text that one can read q If the text contains “fields” fields such as a name, address, phone number, email address, and so forth, it is often useful to separate the fields with some character that otherwise does not appear in the text. This allows the reader to tell where one field ends and the next begins. Compare these two: Øthisallowsthereadertotellwhereonefieldendsandthenext … Øthis|allows|the|reader|to|tell|where|one|field|ends|and … q This separator character is called a delimiter ØMust only be a single character such as , ; | # ^ $ ‘t’ ØMust not appear anywhere else in the file ØFor example, if the data in the text file contains a name such as Badly, Claude in which the comma appears as part of the name, the comma cannot be the delimiter character
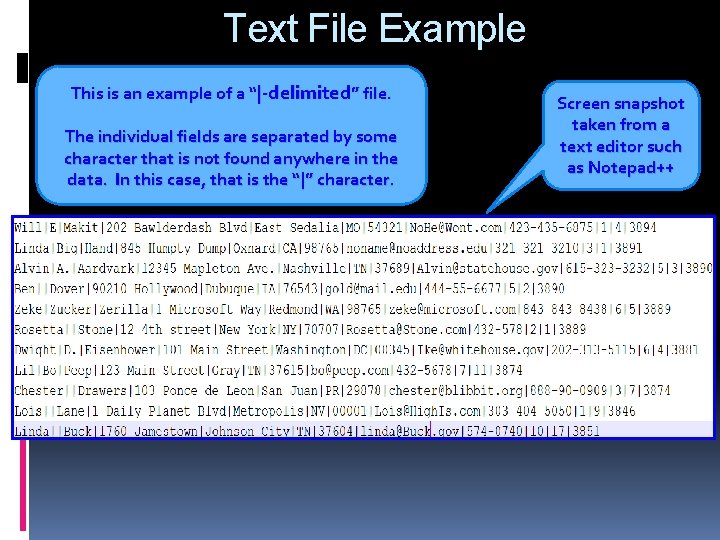
Text File Example This is an example of a “|-delimited” file. The individual fields are separated by some character that is not found anywhere in the data. In this case, that is the “|” character. Screen snapshot taken from a text editor such as Notepad++
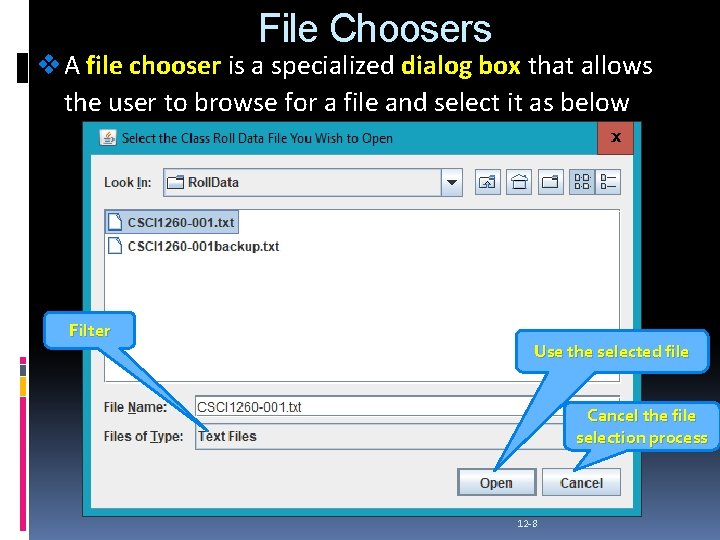
File Choosers v A file chooser is a specialized dialog box that allows the user to browse for a file and select it as below Filter Use the selected file Cancel the file selection process 12 -8
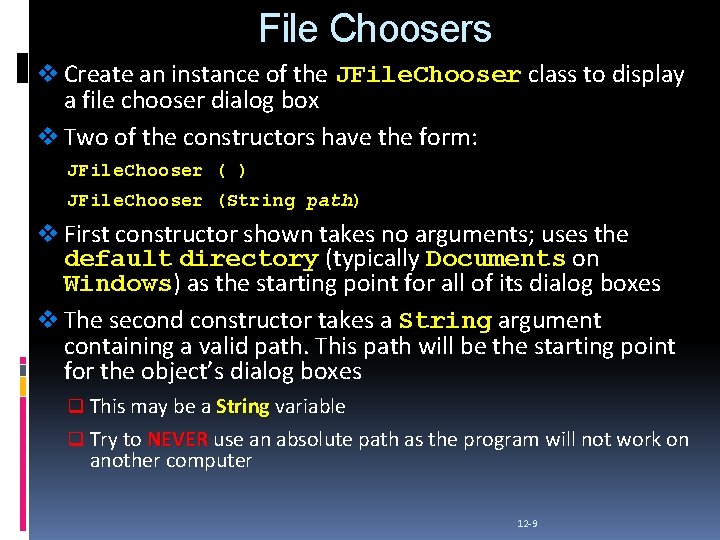
File Choosers v Create an instance of the JFile. Chooser class to display a file chooser dialog box v Two of the constructors have the form: JFile. Chooser ( ) JFile. Chooser (String path) v First constructor shown takes no arguments; uses the default directory (typically Documents on Windows) Windows as the starting point for all of its dialog boxes v The second constructor takes a String argument containing a valid path. This path will be the starting point for the object’s dialog boxes q This may be a String variable q Try to NEVER use an absolute path as the program will not work on another computer 12 -9
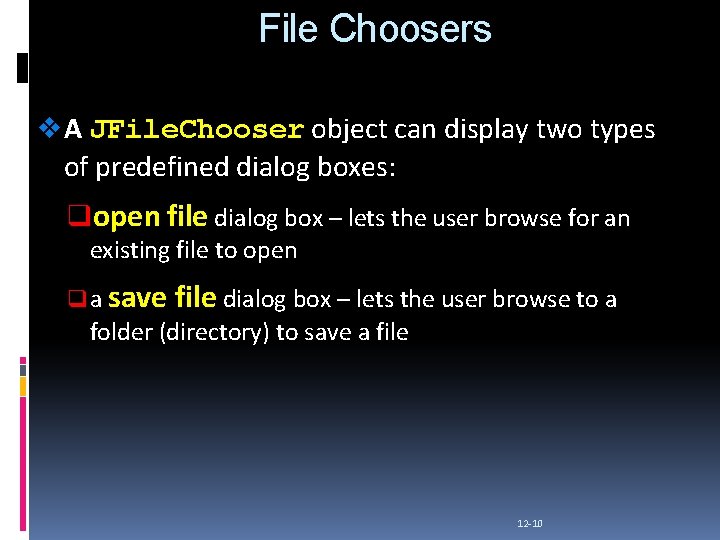
File Choosers v A JFile. Chooser object can display two types of predefined dialog boxes: qopen file dialog box – lets the user browse for an existing file to open q a save file dialog box – lets the user browse to a folder (directory) to save a file 12 -10
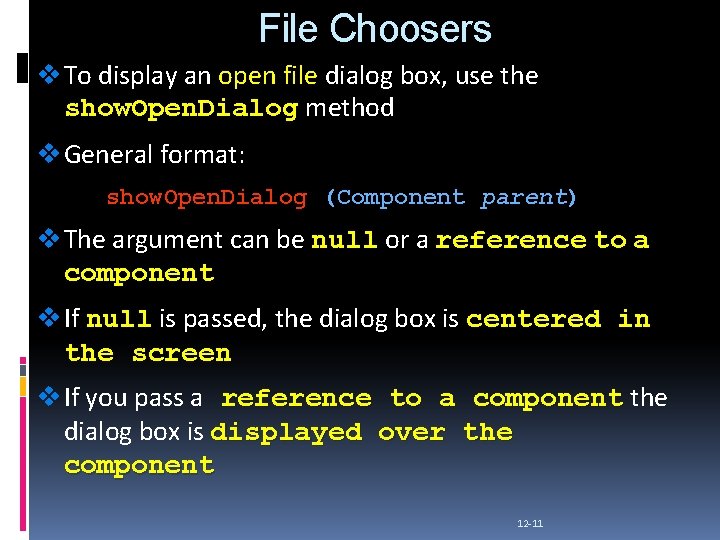
File Choosers v To display an open file dialog box, use the show. Open. Dialog method v General format: show. Open. Dialog (Component parent) v The argument can be null or a reference to a component v If null is passed, the dialog box is centered in the screen v If you pass a reference to a component the dialog box is displayed over the component 12 -11
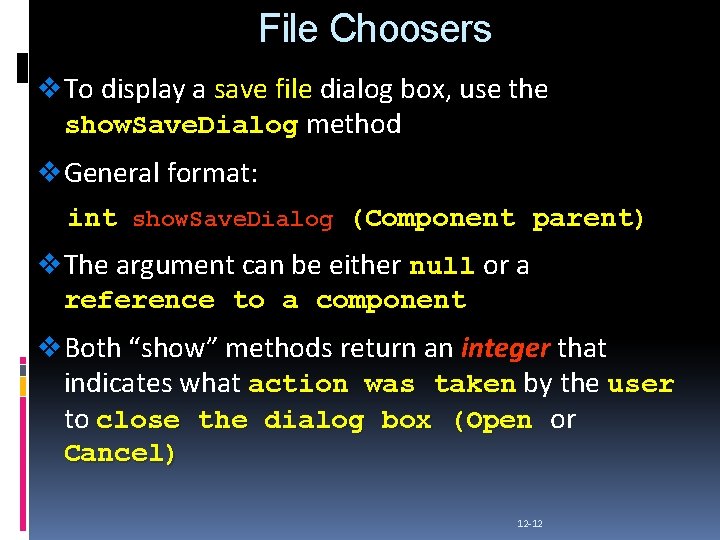
File Choosers v To display a save file dialog box, use the show. Save. Dialog method v General format: int show. Save. Dialog (Component parent) v The argument can be either null or a reference to a component v Both “show” methods return an integer that indicates what action was taken by the user to close the dialog box (Open or Cancel) 12 -12
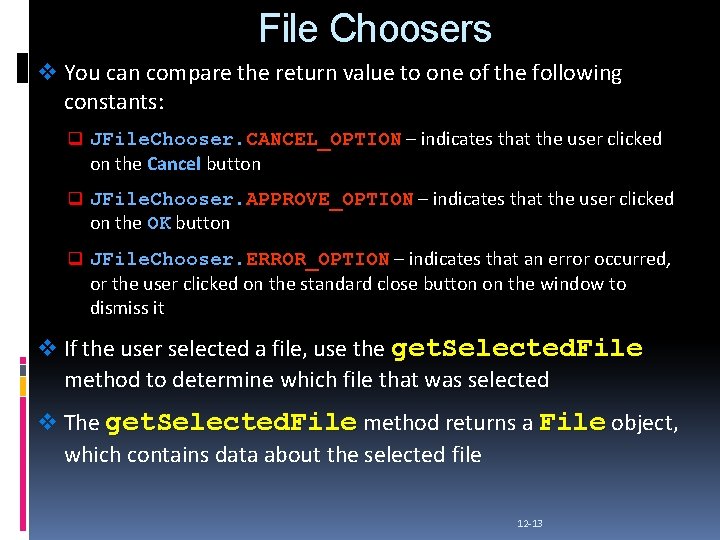
File Choosers v You can compare the return value to one of the following constants: q JFile. Chooser. CANCEL_OPTION – indicates that the user clicked on the Cancel button q JFile. Chooser. APPROVE_OPTION – indicates that the user clicked on the OK button q JFile. Chooser. ERROR_OPTION – indicates that an error occurred, or the user clicked on the standard close button on the window to dismiss it v If the user selected a file, use the get. Selected. File method to determine which file that was selected v The get. Selected. File method returns a File object, which contains data about the selected file 12 -13
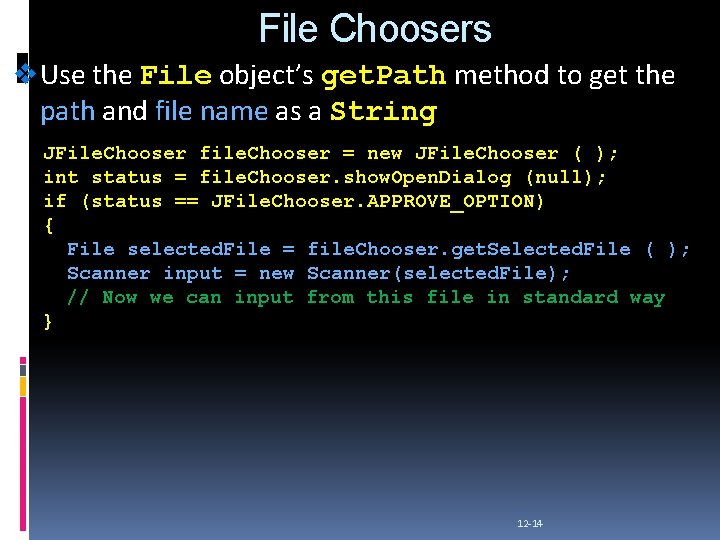
File Choosers v Use the File object’s get. Path method to get the path and file name as a String JFile. Chooser file. Chooser = new JFile. Chooser ( ); int status = file. Chooser. show. Open. Dialog (null); if (status == JFile. Chooser. APPROVE_OPTION) { File selected. File = file. Chooser. get. Selected. File ( ); Scanner input = new Scanner(selected. File); // Now we can input from this file in standard way } 12 -14
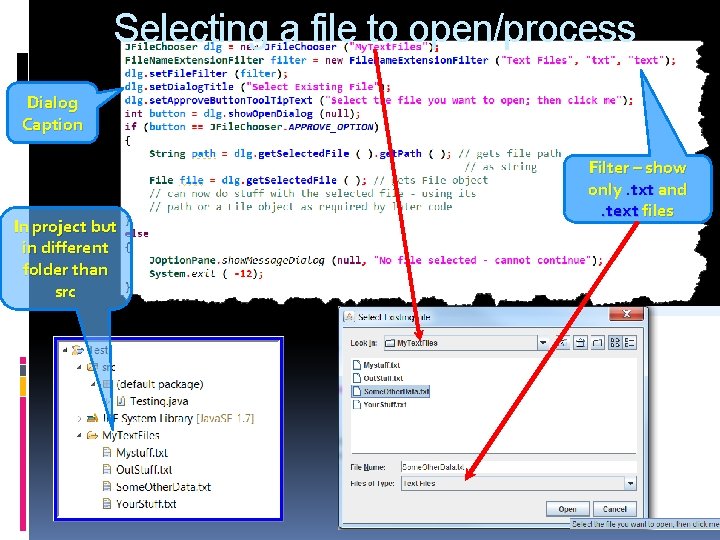
Selecting a file to open/process Dialog Caption In project but in different folder than src Filter – show only. txt and. text files
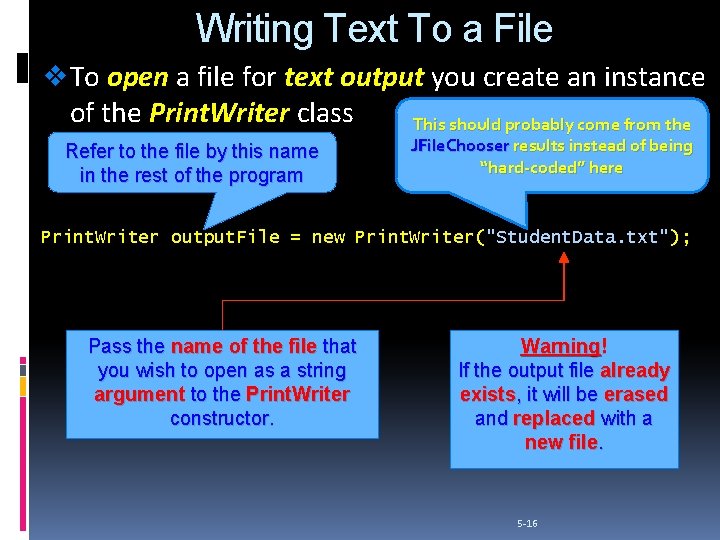
Writing Text To a File v To open a file for text output you create an instance of the Print. Writer class This should probably come from the Refer to the file by this name in the rest of the program JFile. Chooser results instead of being “hard-coded” here Print. Writer output. File = new Print. Writer("Student. Data. txt"); Pass the name of the file that you wish to open as a string argument to the Print. Writer constructor. Warning! If the output file already exists, it will be erased and replaced with a new file. 5 -16
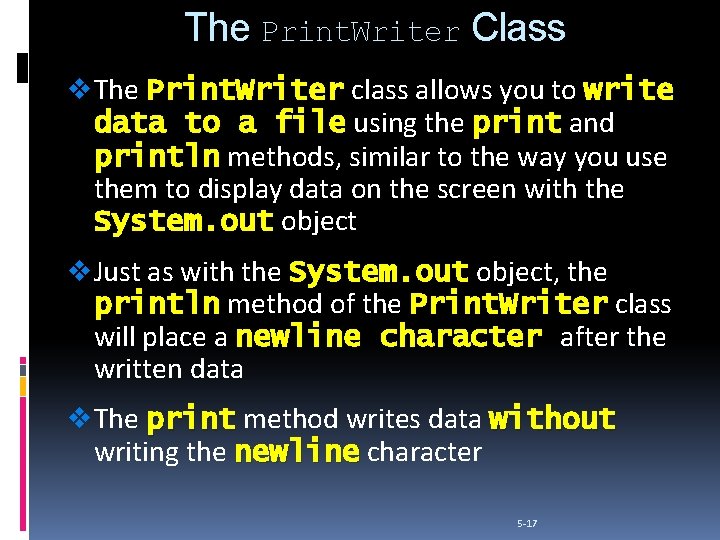
The Print. Writer Class v The Print. Writer class allows you to write data to a file using the print and println methods, similar to the way you use them to display data on the screen with the System. out object v Just as with the System. out object, the println method of the Print. Writer class will place a newline character after the written data v The print method writes data without writing the newline character 5 -17
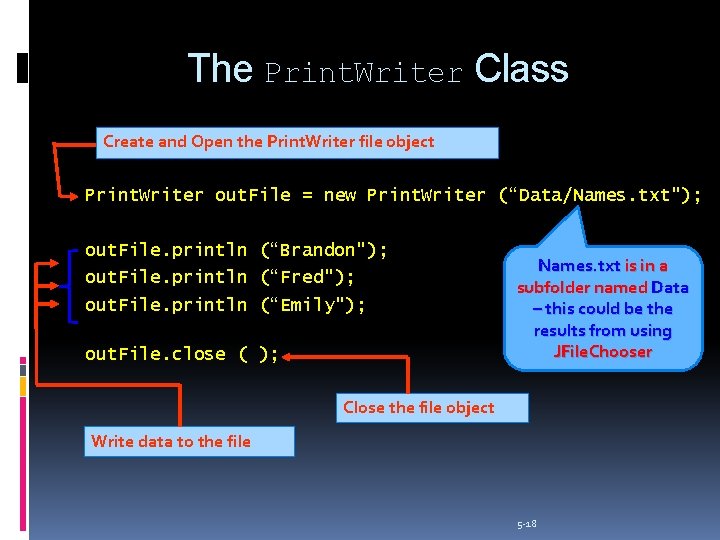
The Print. Writer Class Create and Open the Print. Writer file object Print. Writer out. File = new Print. Writer (“Data/Names. txt"); out. File. println (“Brandon"); (“Fred"); out. File. println (“Emily"); out. File. close ( ); Names. txt is in a subfolder named Data – this could be the results from using JFile. Chooser Close the file object Write data to the file 5 -18
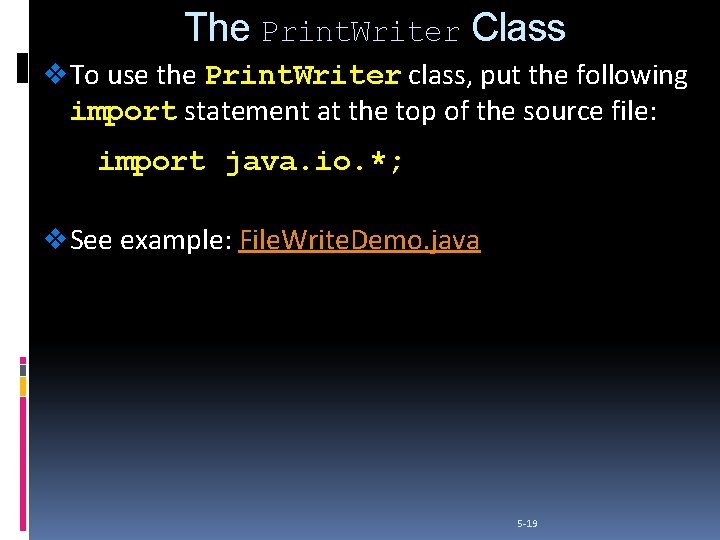
The Print. Writer Class v To use the Print. Writer class, put the following import statement at the top of the source file: import java. io. *; v See example: File. Write. Demo. java 5 -19
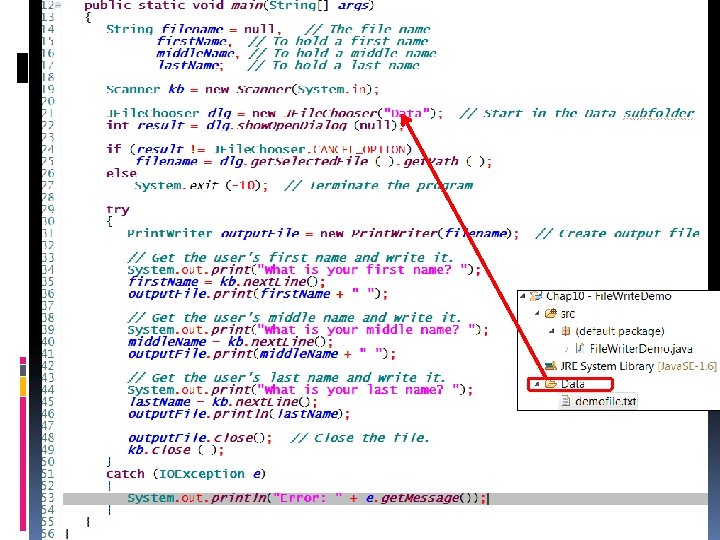
Output File Demo
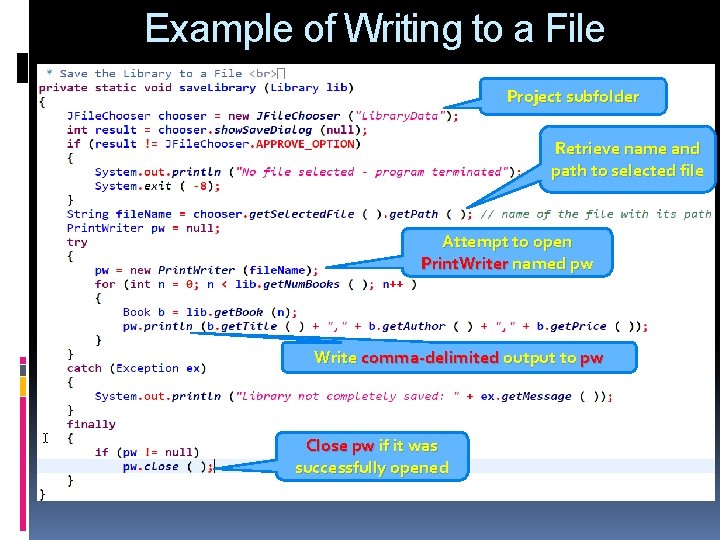
Example of Writing to a File Project subfolder Retrieve name and path to selected file Attempt to open Print. Writer named pw Write comma-delimited output to pw Close pw if it was successfully opened
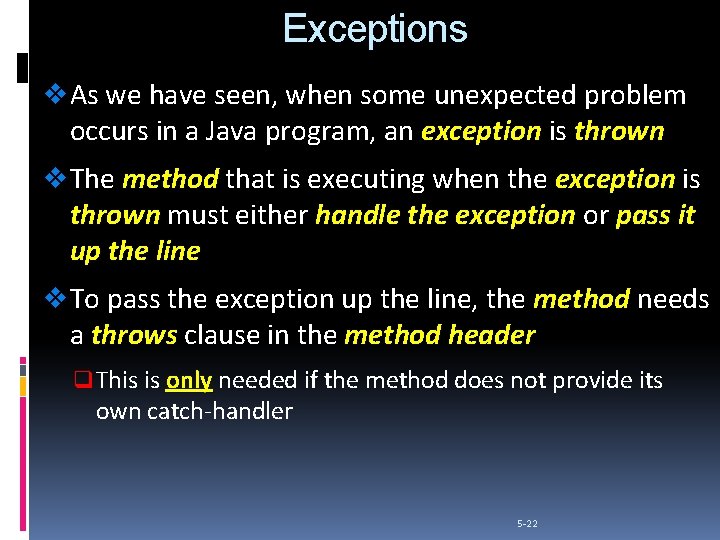
Exceptions v As we have seen, when some unexpected problem occurs in a Java program, an exception is thrown v The method that is executing when the exception is thrown must either handle the exception or pass it up the line v To pass the exception up the line, the method needs a throws clause in the method header q This is only needed if the method does not provide its own catch-handler 5 -22
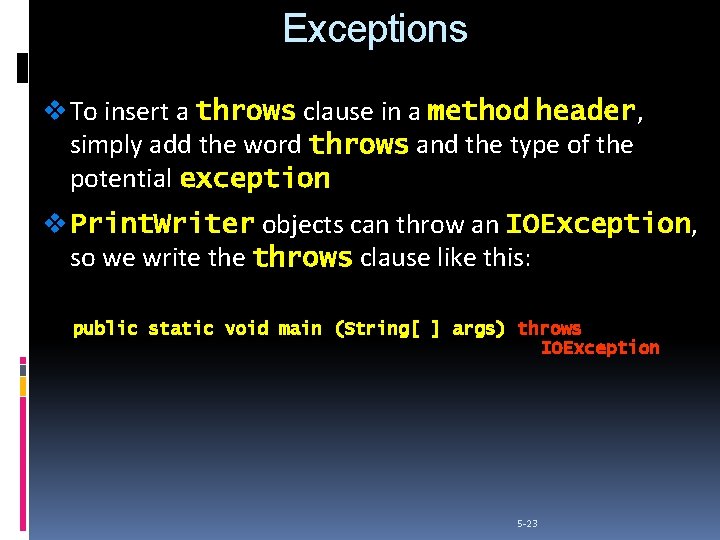
Exceptions v To insert a throws clause in a method header, header simply add the word throws and the type of the potential exception v Print. Writer objects can throw an IOException, IOException so we write throws clause like this: public static void main (String[ ] args) throws IOException 5 -23
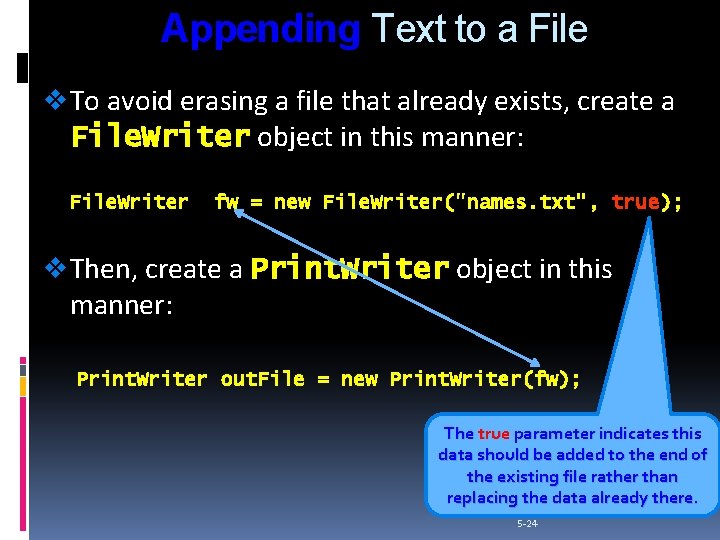
Appending Text to a File v To avoid erasing a file that already exists, create a File. Writer object in this manner: File. Writer fw = new File. Writer("names. txt", true); v Then, create a Print. Writer object in this manner: Print. Writer out. File = new Print. Writer(fw); The true parameter indicates this data should be added to the end of the existing file rather than replacing the data already there. 5 -24
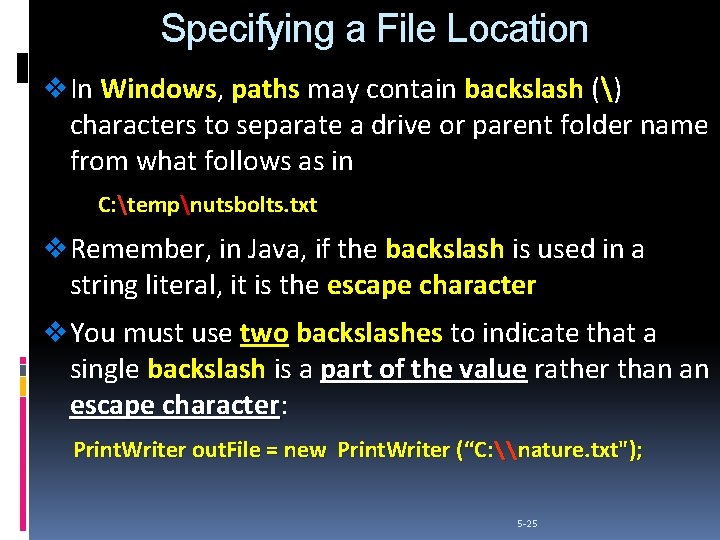
Specifying a File Location v In Windows, Windows paths may contain backslash () characters to separate a drive or parent folder name from what follows as in C: tempnutsbolts. txt v Remember, in Java, if the backslash is used in a string literal, it is the escape character v You must use two backslashes to indicate that a single backslash is a part of the value rather than an escape character: character Print. Writer out. File = new Print. Writer (“C: \nature. txt"); 5 -25
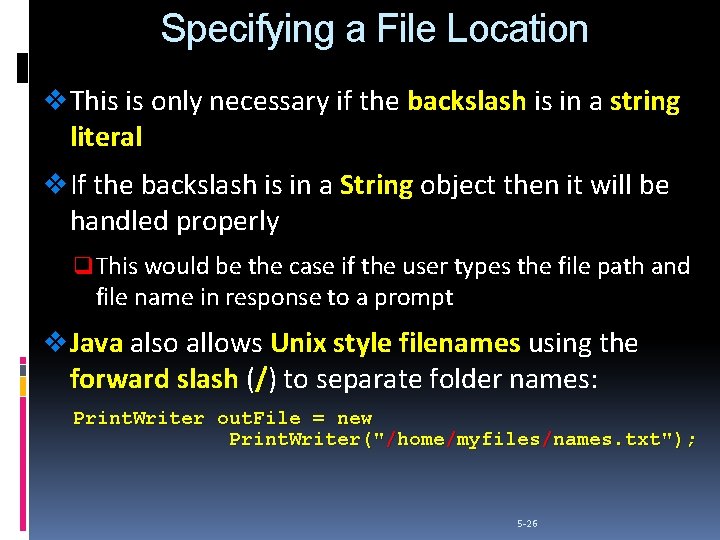
Specifying a File Location v This is only necessary if the backslash is in a string literal v If the backslash is in a String object then it will be handled properly q This would be the case if the user types the file path and file name in response to a prompt v Java also allows Unix style filenames using the forward slash (/) to separate folder names: Print. Writer out. File = new Print. Writer("/home/myfiles/names. txt"); 5 -26
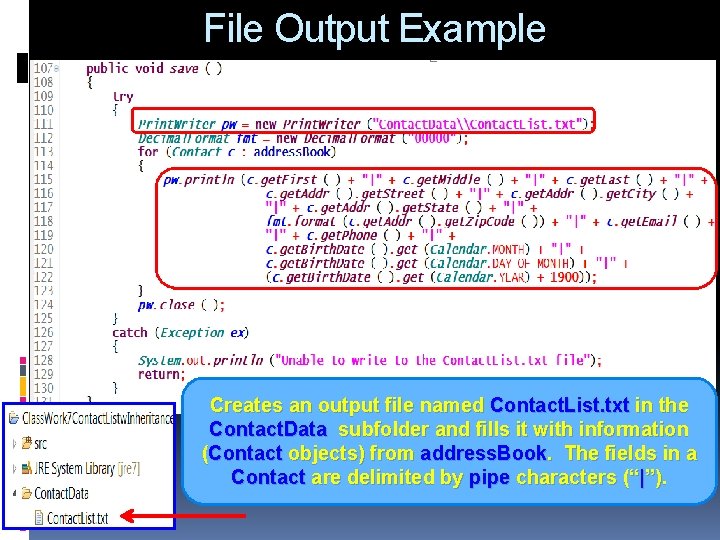
File Output Example Creates an output file named Contact. List. txt in the Contact. Data subfolder and fills it with information (Contact objects) from address. Book. The fields in a Contact are delimited by pipe characters (“|”).
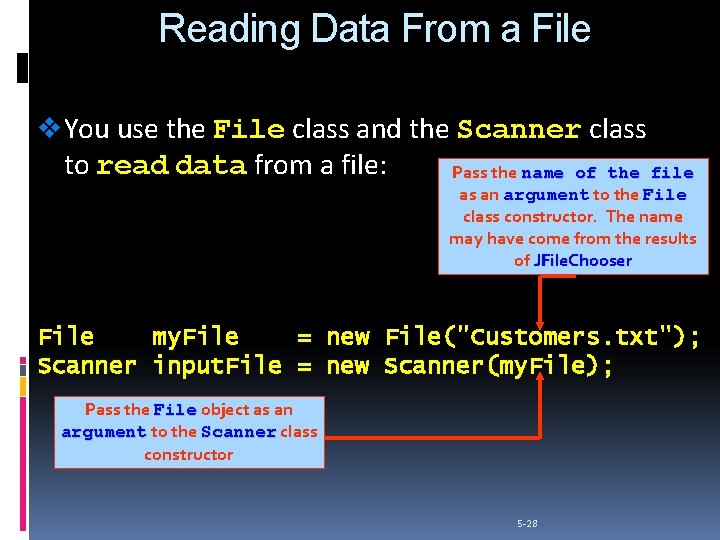
Reading Data From a File v You use the File class and the Scanner class to read data from a file: Pass the name of the file as an argument to the File class constructor. The name may have come from the results of JFile. Chooser File my. File = new File("Customers. txt"); Scanner input. File = new Scanner(my. File); Pass the File object as an argument to the Scanner class constructor 5 -28
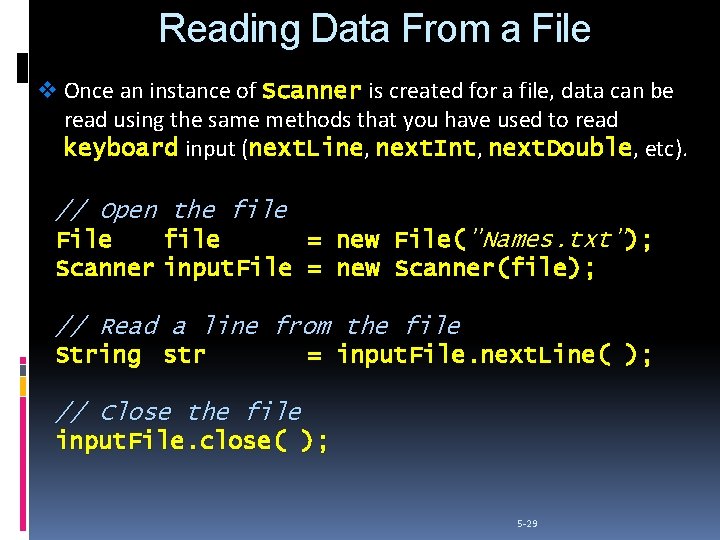
Reading Data From a File v Once an instance of Scanner is created for a file, data can be read using the same methods that you have used to read keyboard input (next. Line, next. Line next. Int, next. Int next. Double, next. Double etc). // Open the file File file = new File("Names. txt"); Scanner input. File = new Scanner(file); // Read a line from the file String str = input. File. next. Line( ); // Close the file input. File. close( ); 5 -29
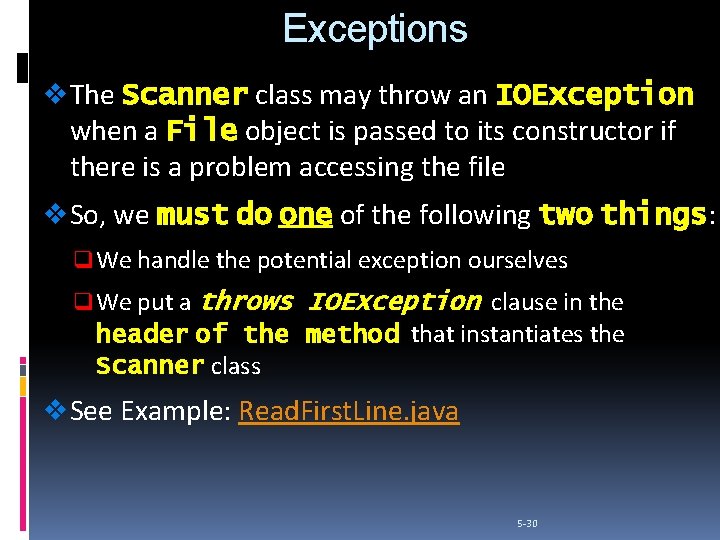
Exceptions v The Scanner class may throw an IOException when a File object is passed to its constructor if there is a problem accessing the file v So, we must do one of the following two things: things q We handle the potential exception ourselves q We put a throws IOException clause in the header of the method that instantiates the Scanner class v See Example: Read. First. Line. java 5 -30
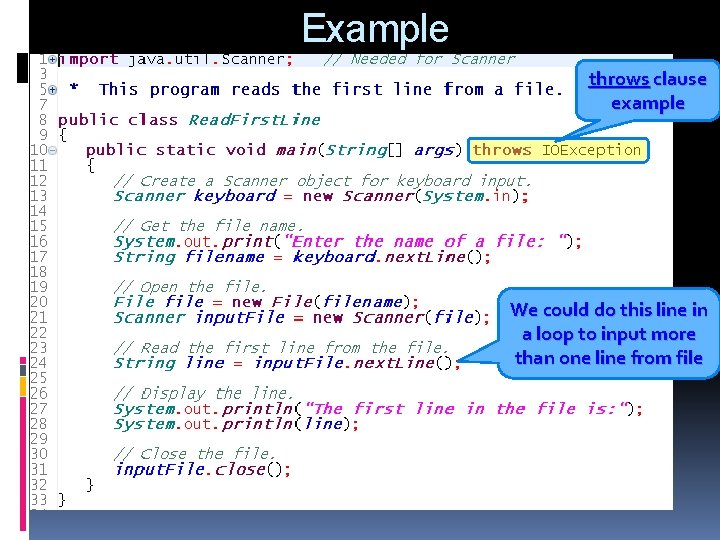
Example throws clause example We could do this line in a loop to input more than one line from file
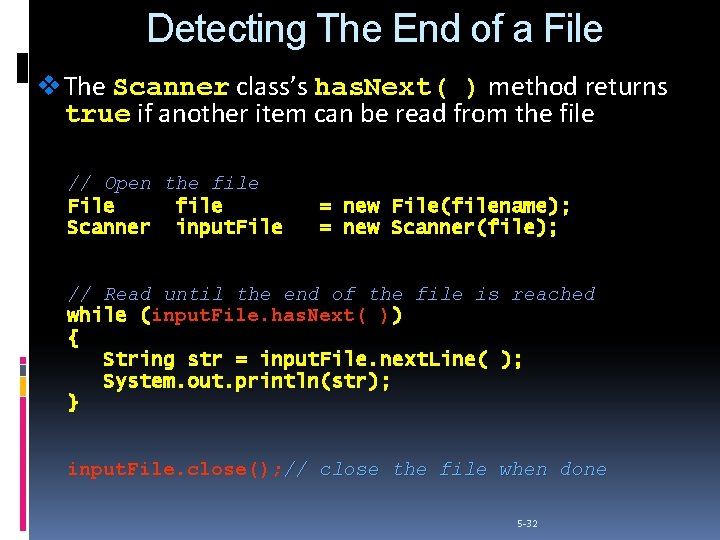
Detecting The End of a File v The Scanner class’s has. Next( ) method returns true if another item can be read from the file // Open the file File file Scanner input. File = new File(filename); = new Scanner(file); // Read until the end of the file is reached while (input. File. has. Next( )) ( { String str = input. File. next. Line( ); System. out. println(str); } input. File. close(); // close the file when done 5 -32
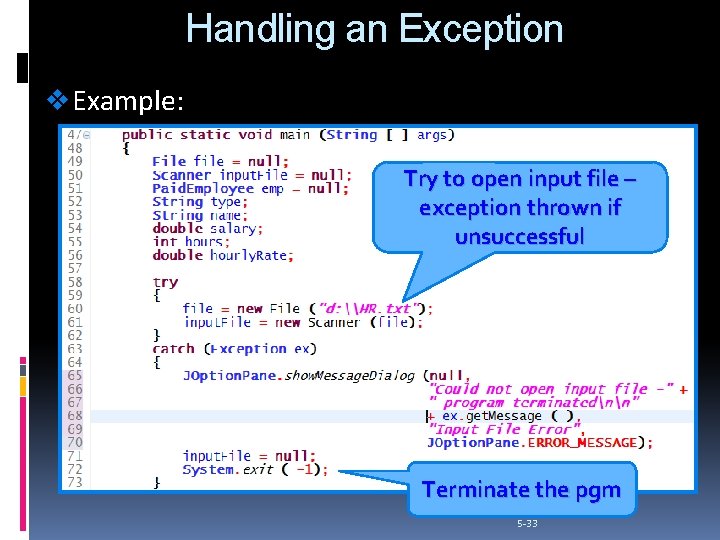
Handling an Exception v Example: Try to open input file – exception thrown if unsuccessful Terminate the pgm 5 -33
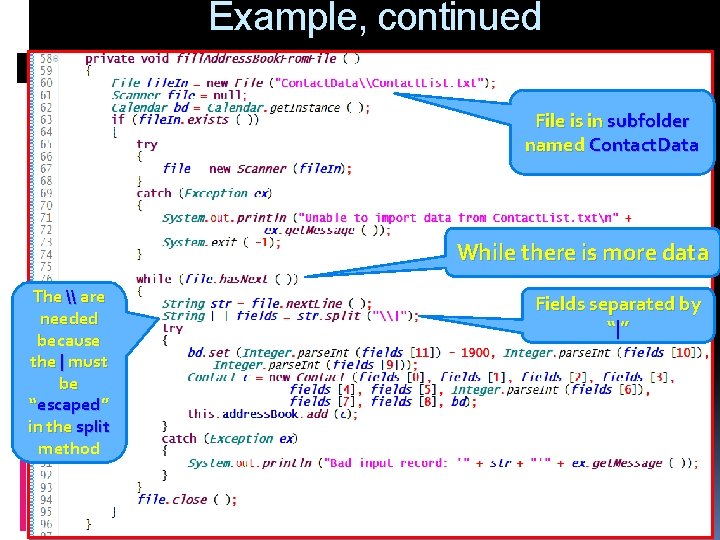
Example, continued File is in subfolder named Contact. Data While there is more data The \ are needed because the | must be “escaped” in the split method Fields separated by “| ”
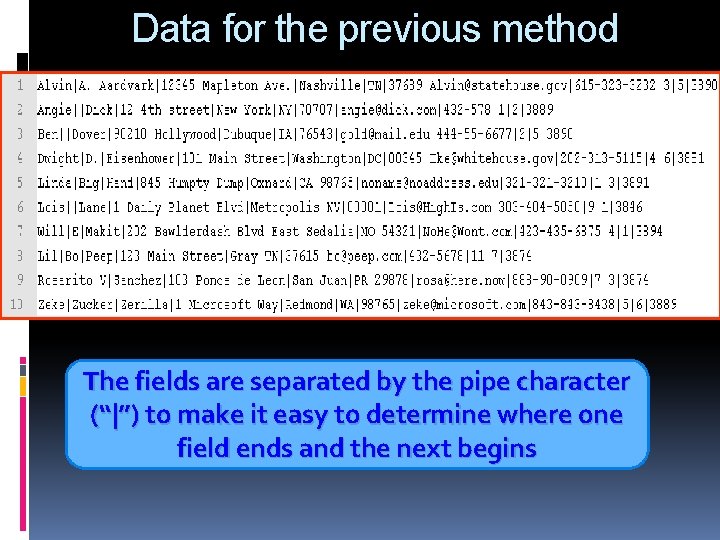
Data for the previous method The fields are separated by the pipe character (“|”) to make it easy to determine where one field ends and the next begins
Cjis security awareness
Ncic hosts restricted files and non-restricted files
Dot powai files are binary files
Text to text text to self text to world
Assume that a firm produces output using one fixed input
Input output form
4 automatic input devices
Vr input
Vat input
Vat input meaning
Vat input
Conclusion for input and output devices of computer
Computer system input
Conclusion of output devices
Modern input devices
Human data entry devices
Input devices
Conclusion of input and output devices
Input and output channels in hci
Computer science input and output
Most abstract input and output in software engineering
Unit input komputer
Disadvantages of output devices
Input of krebs cycle
Vr input and output devices
Citric acid cycle also called
Input transformation output process examples
Input and output devices wiring for plc
Specialized input devices
Keyboard.next java
Domain and range jeopardy
Computer, computer basics, input and output devices
Input and output hardware
Java input and output statements
Algorithm input output
Representing input data and output knowledge