Functions Return Try Catch Soft Uni Team Technical
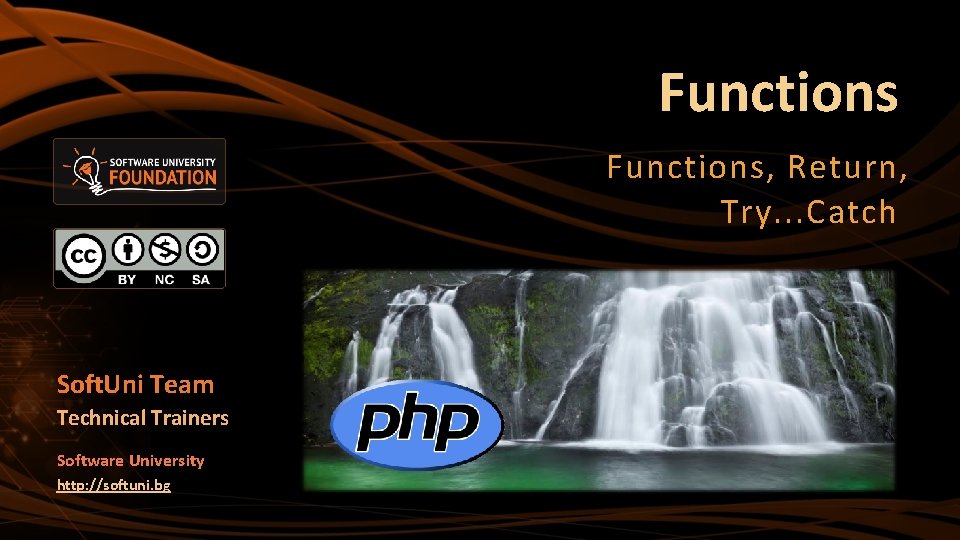
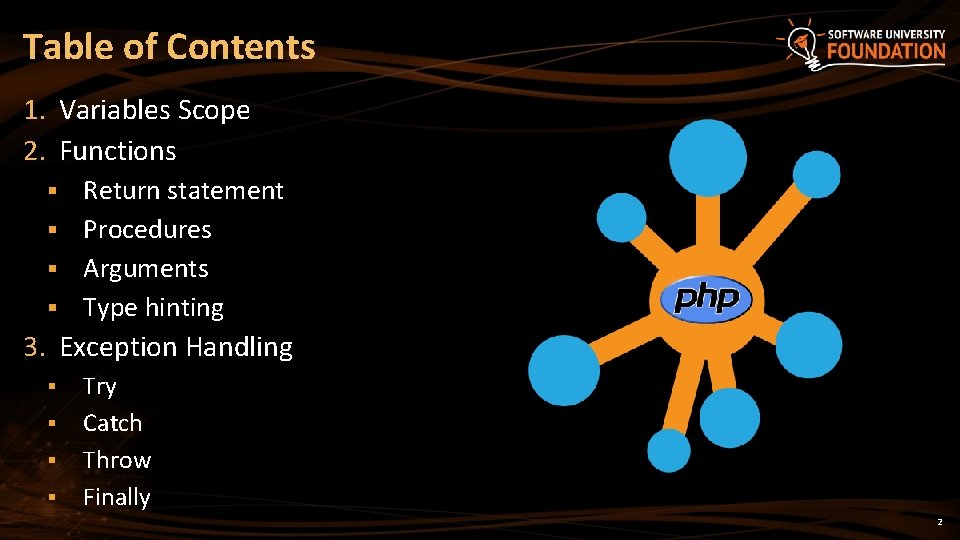
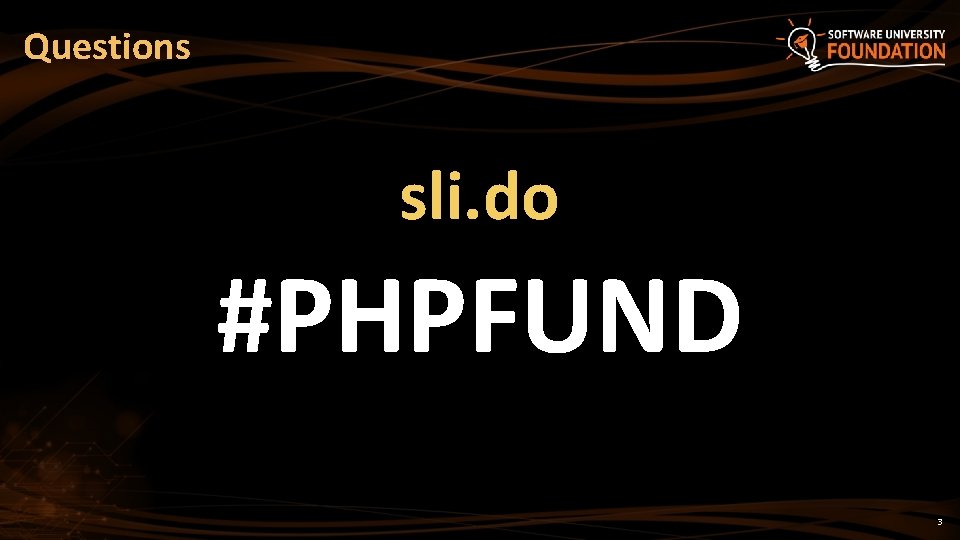
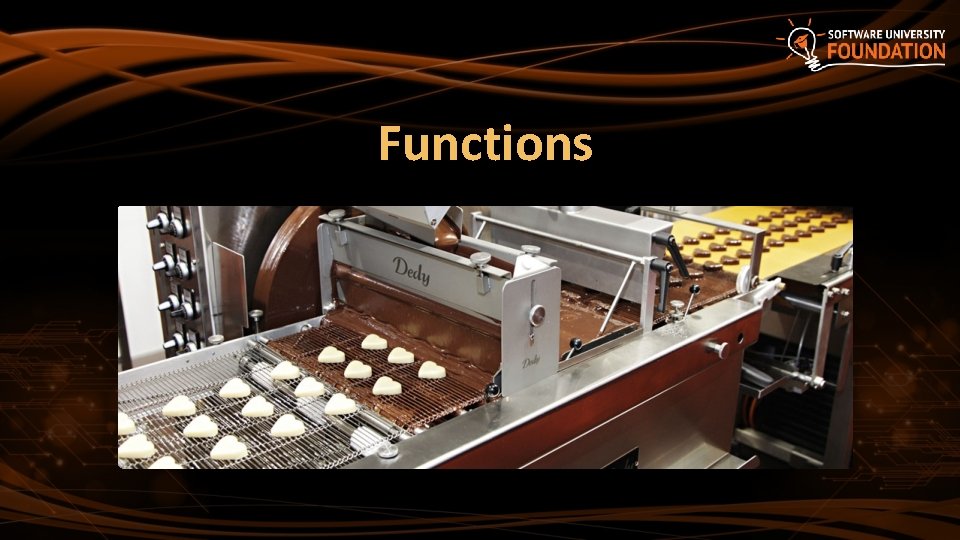
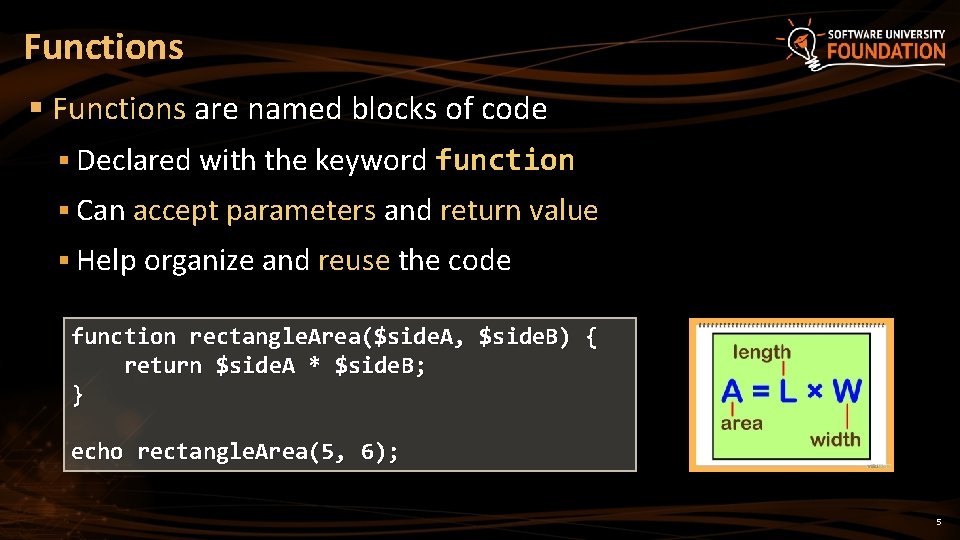
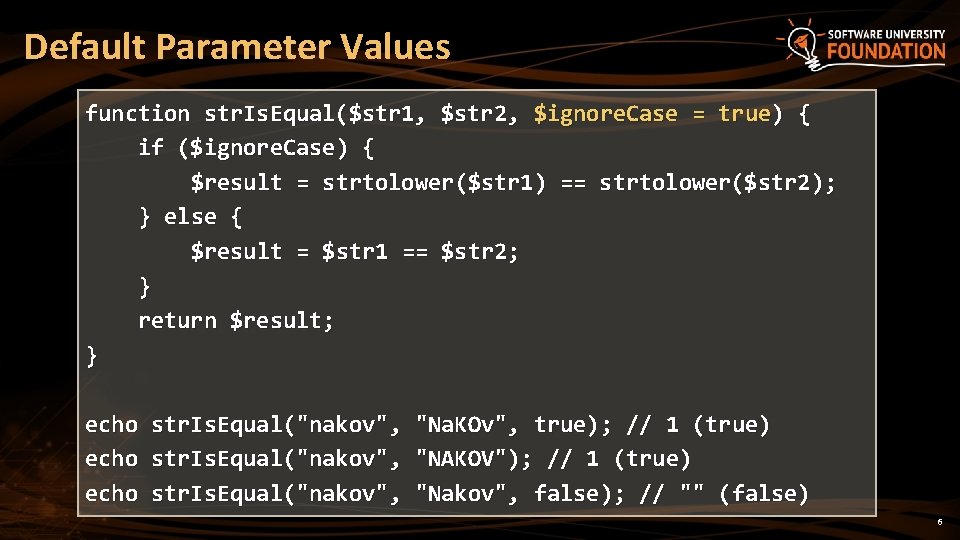
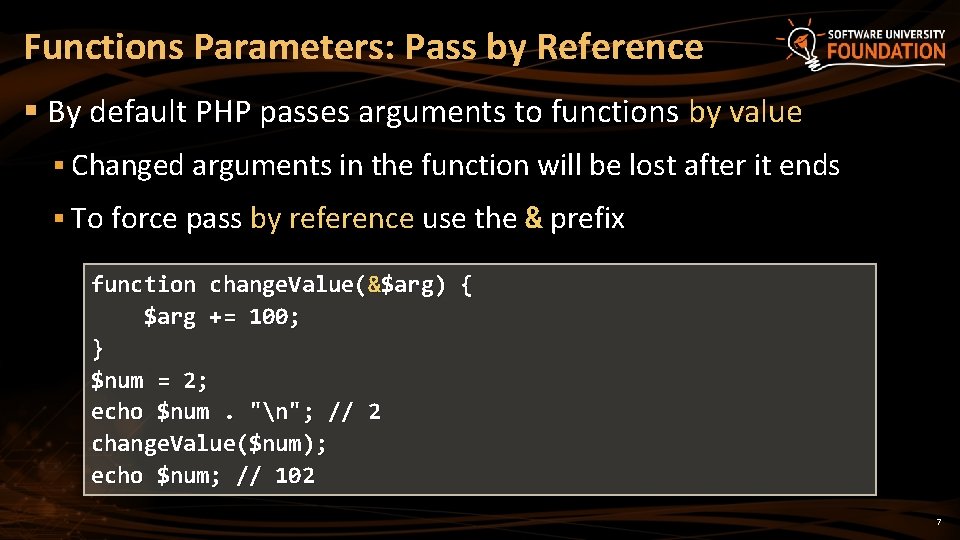
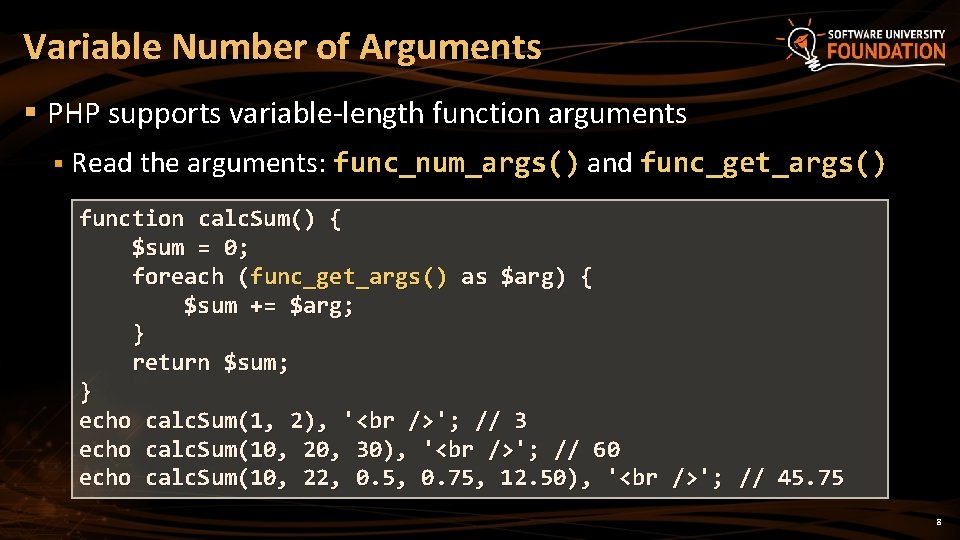
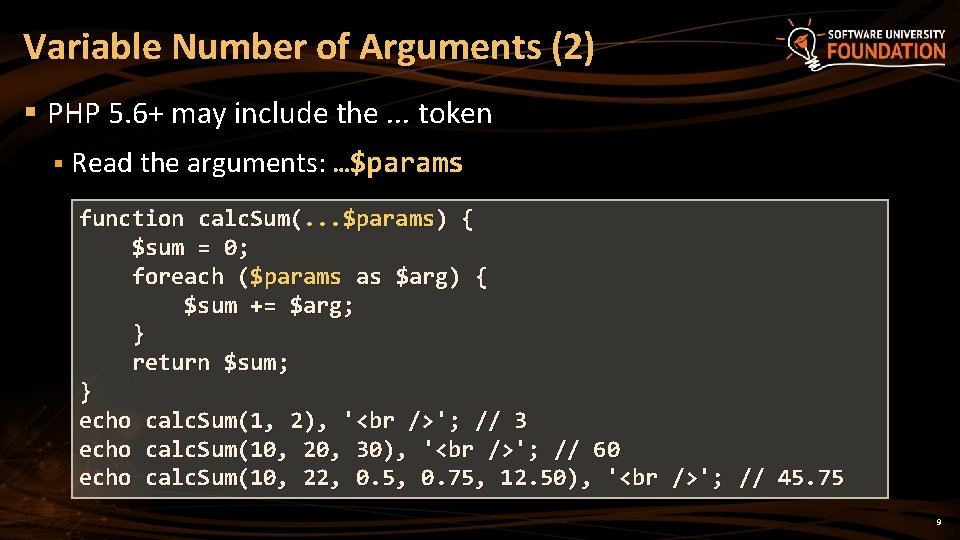
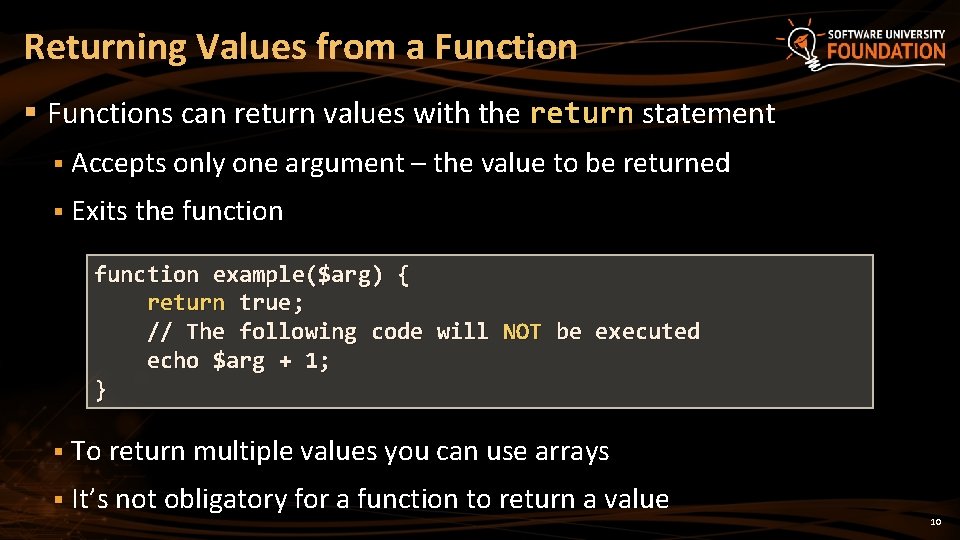
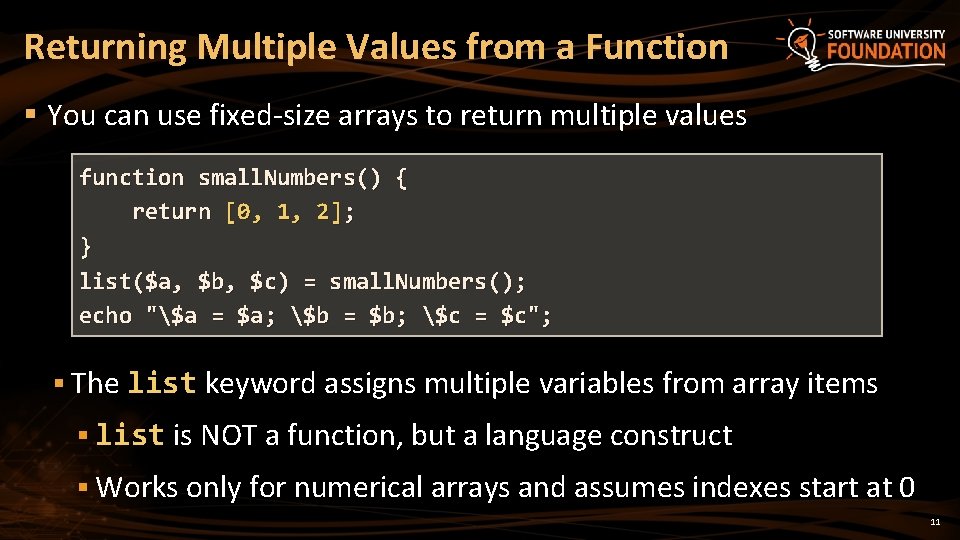
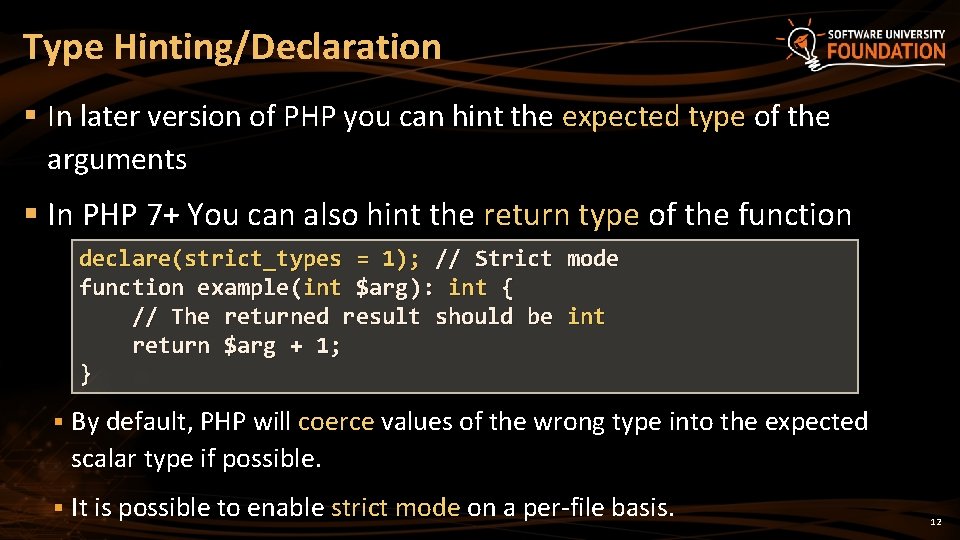
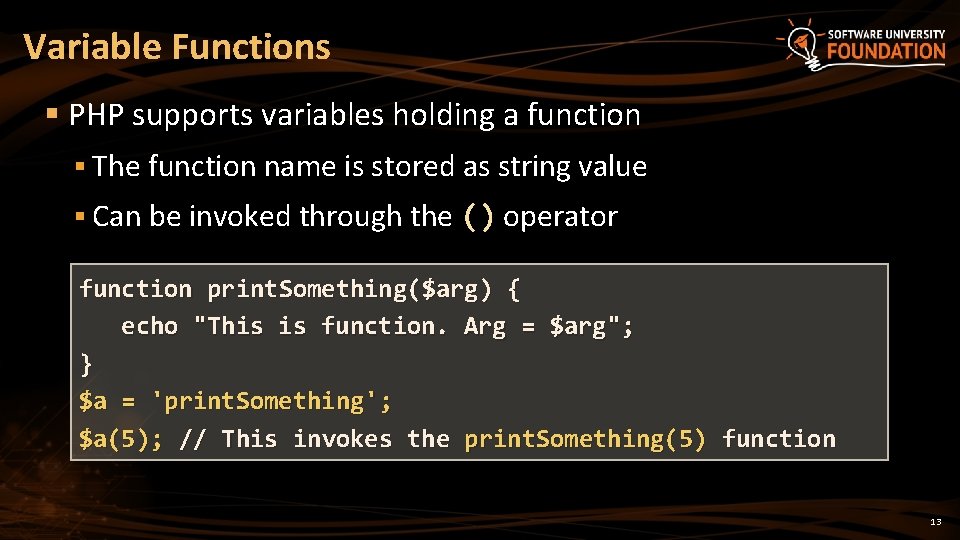
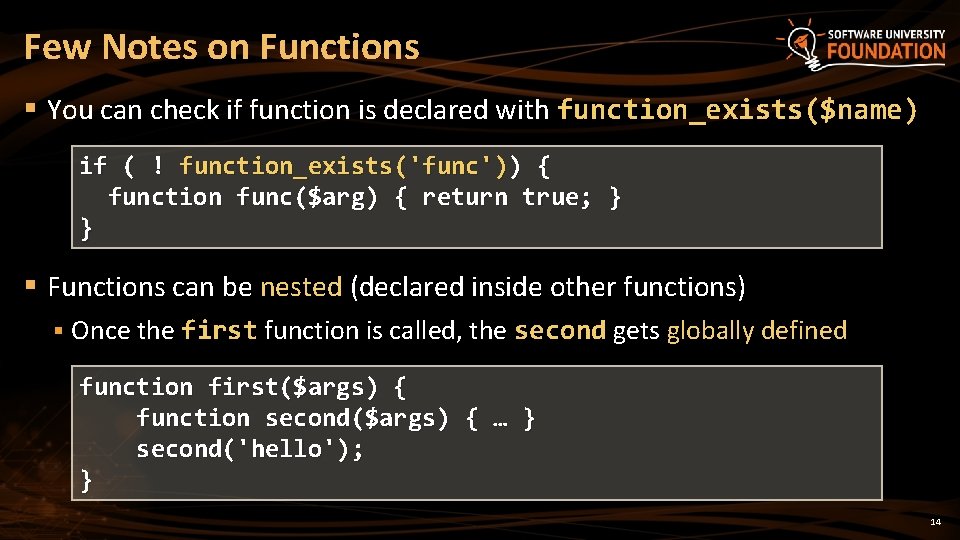
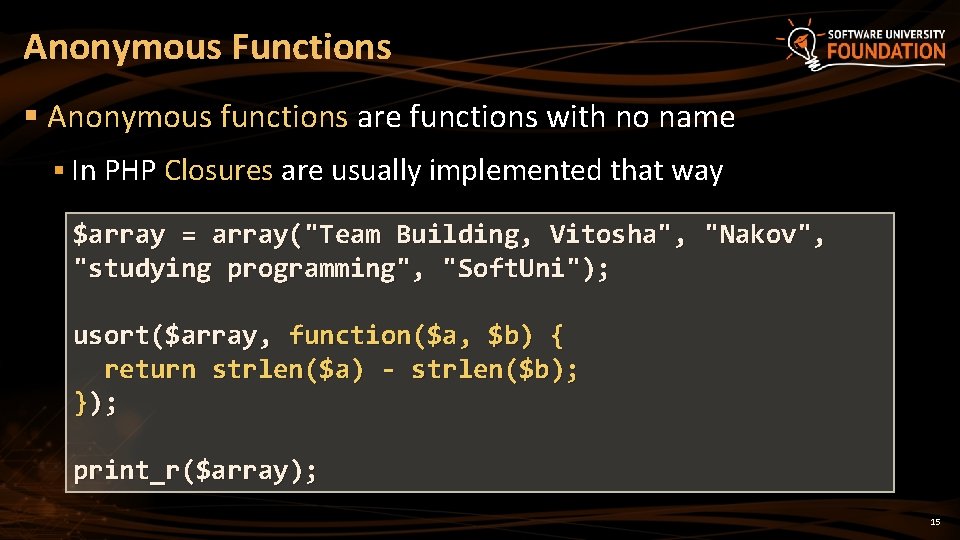
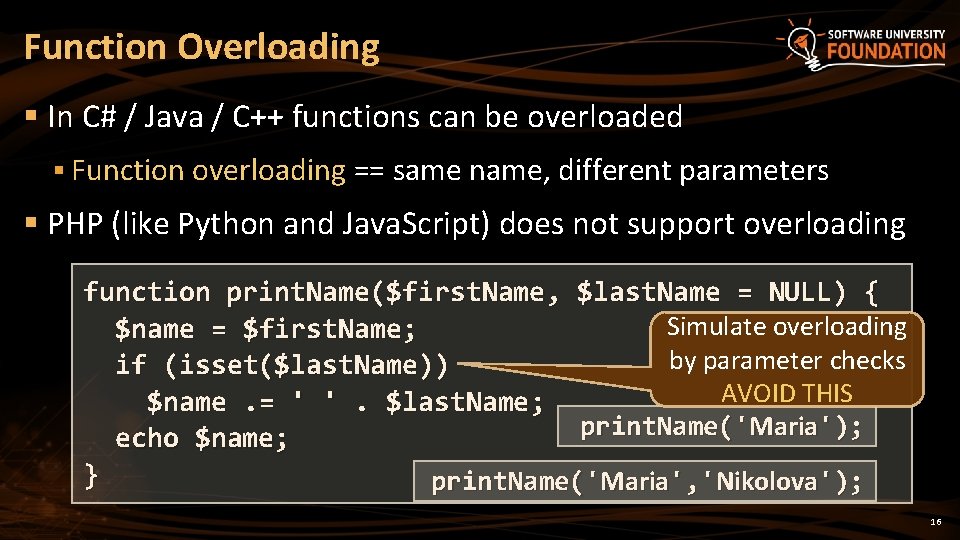
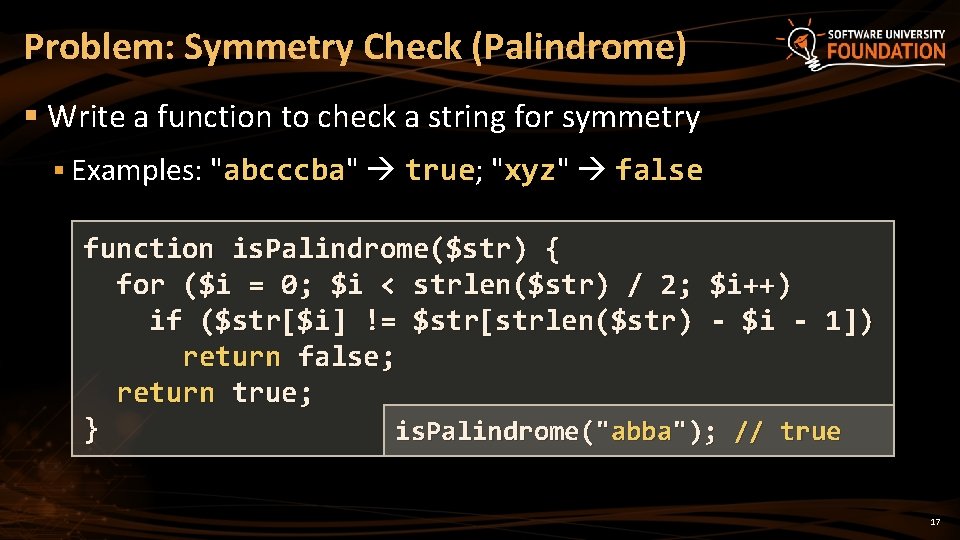
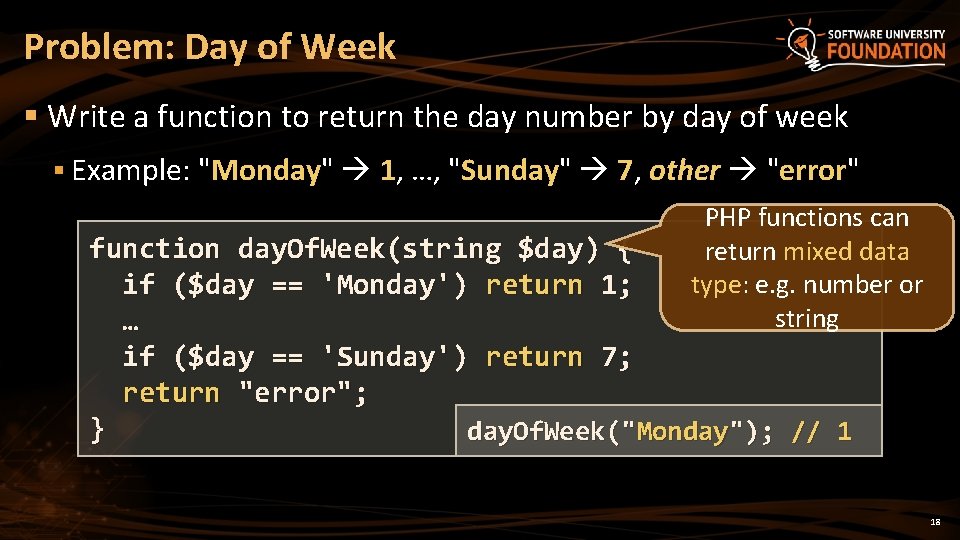
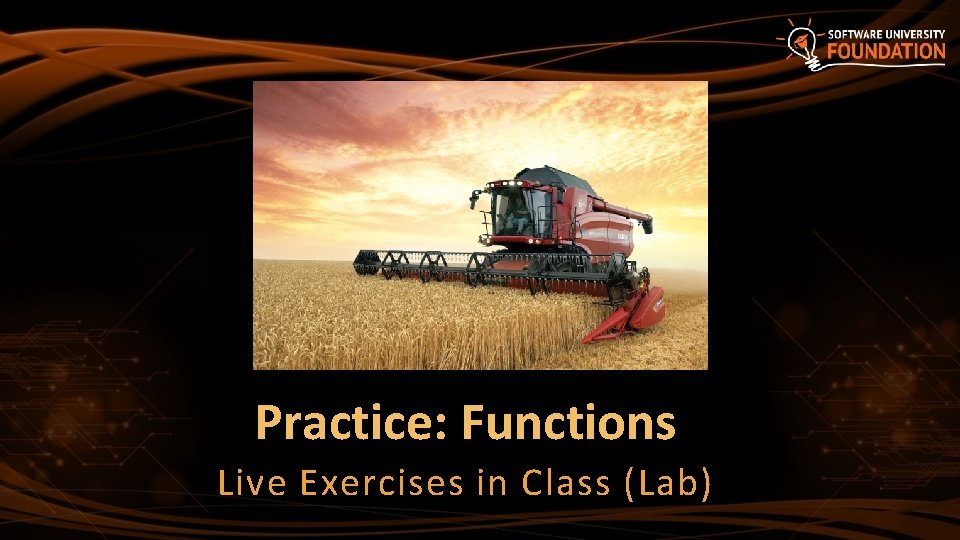
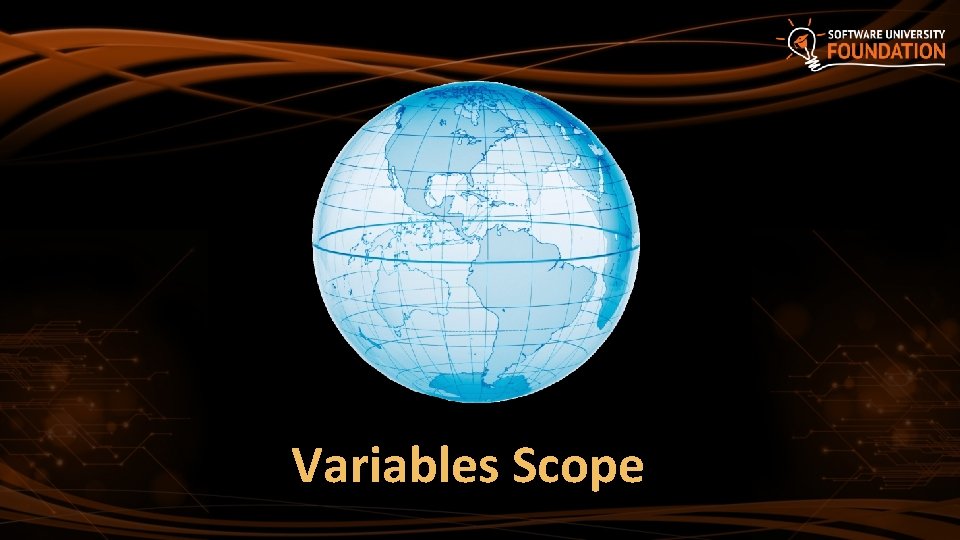
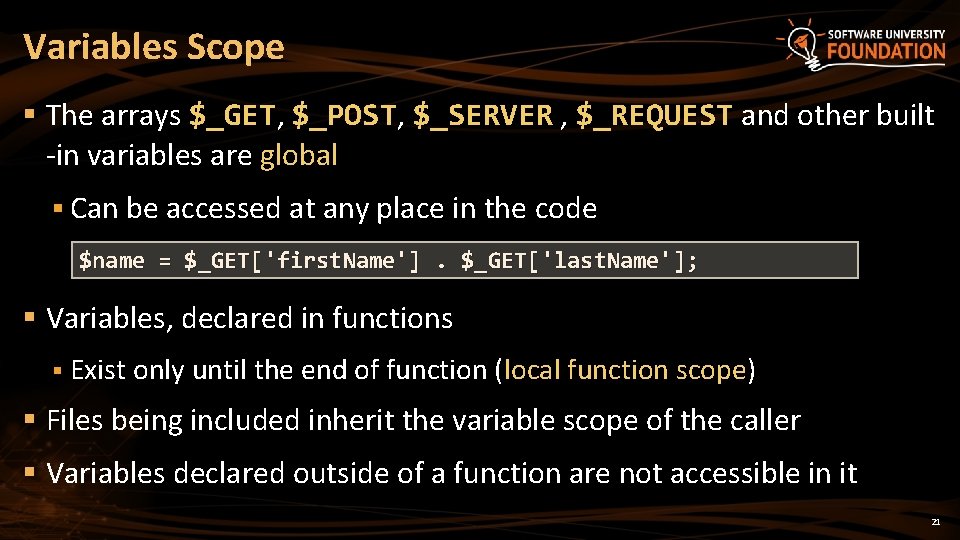
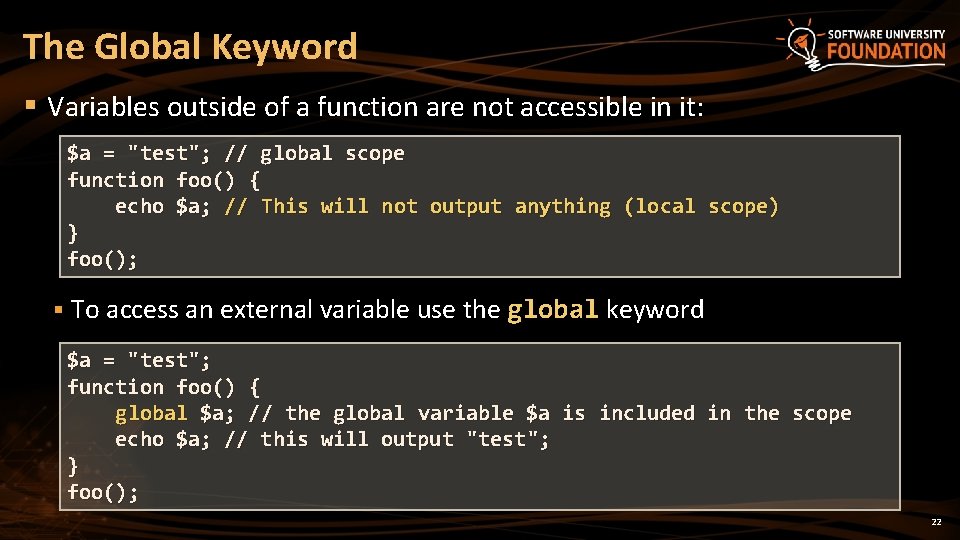
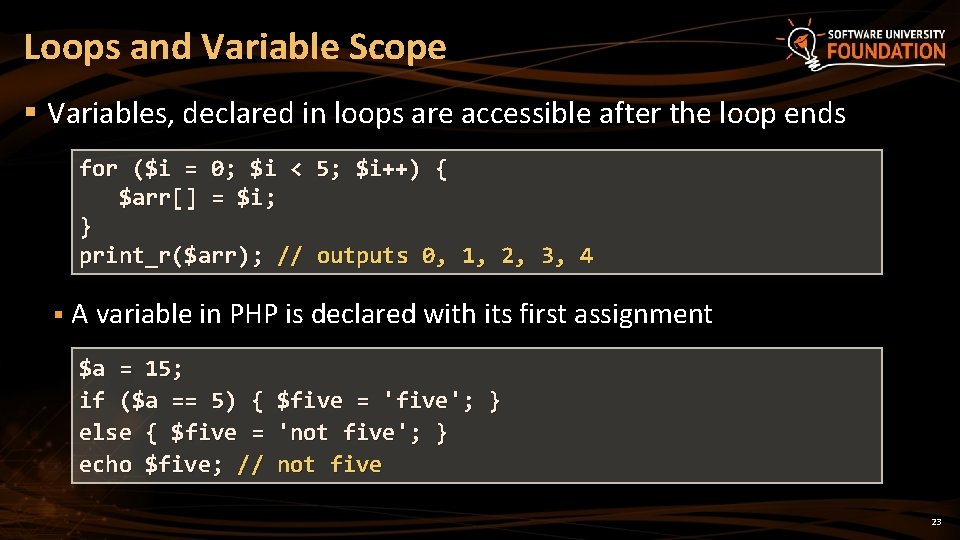
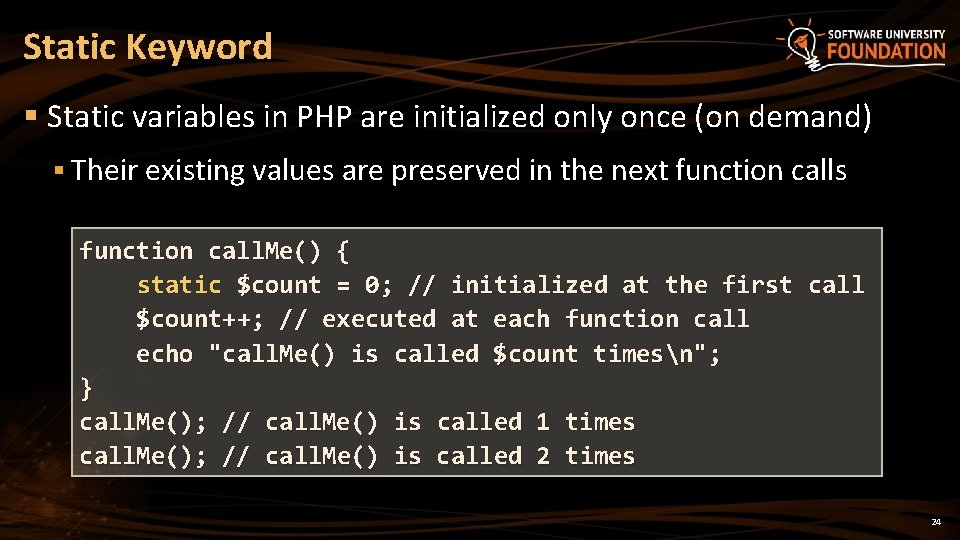
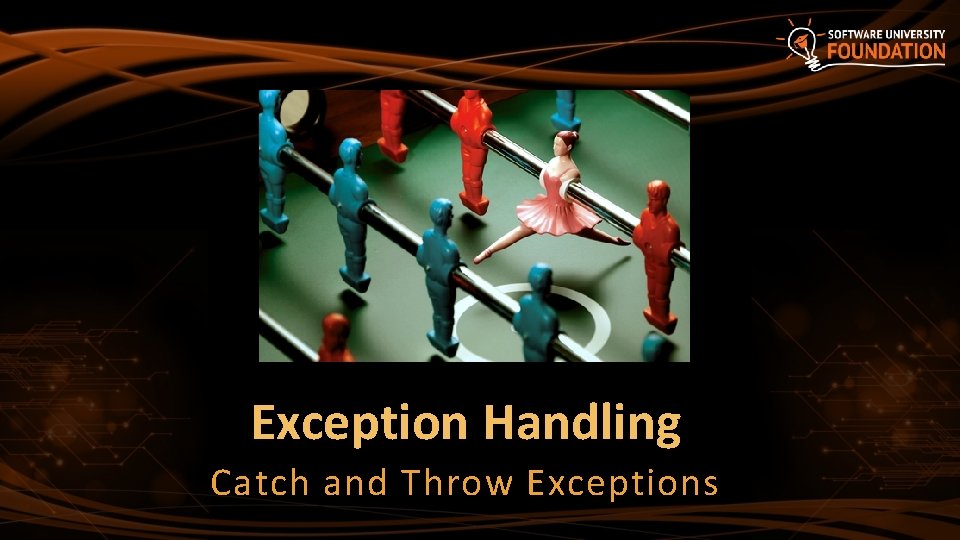
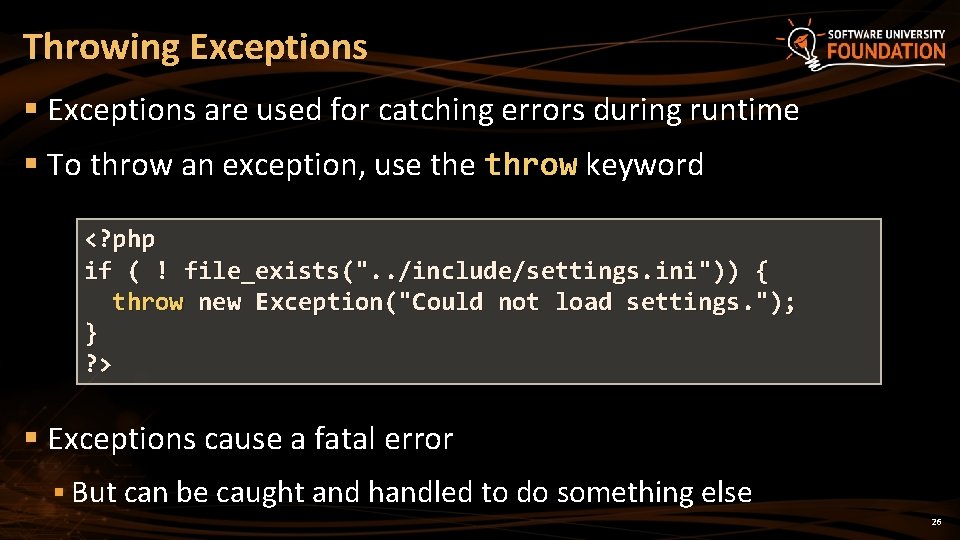
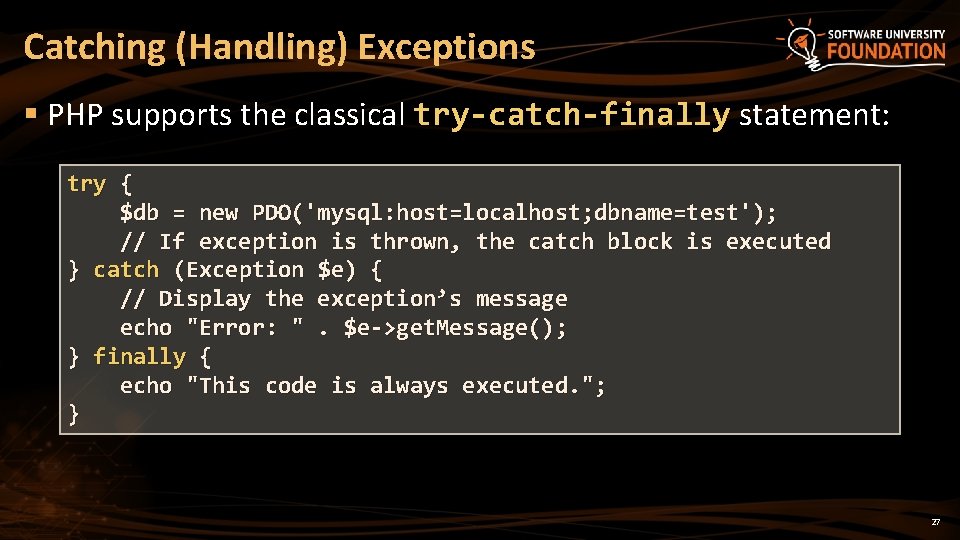
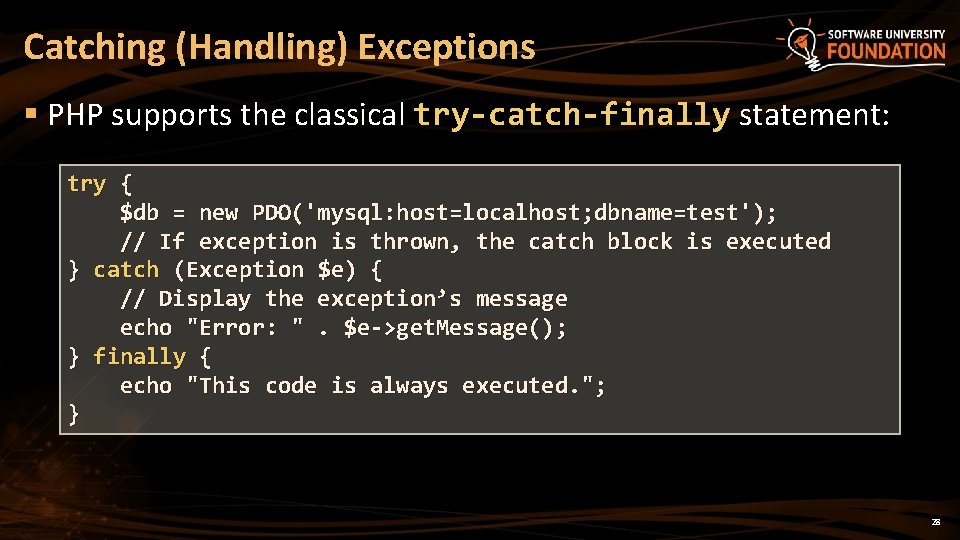
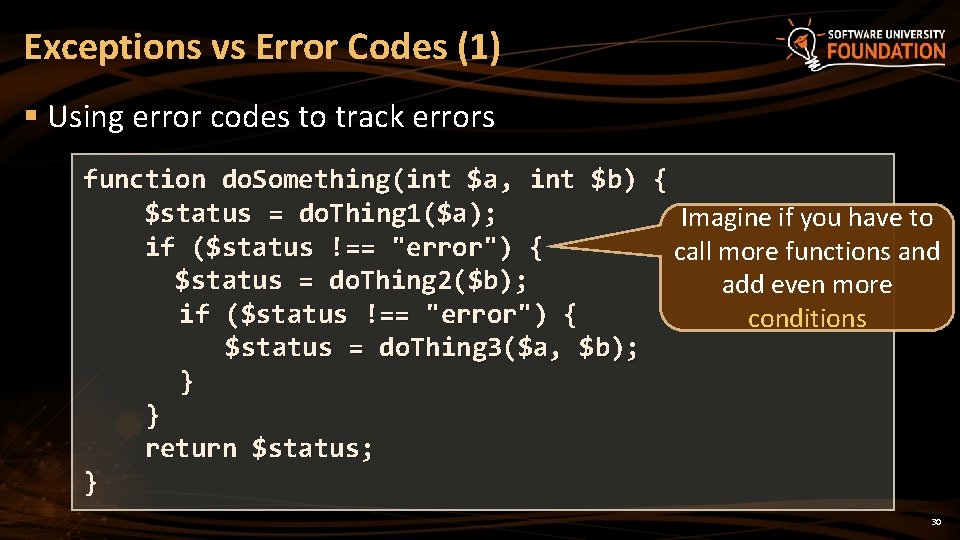
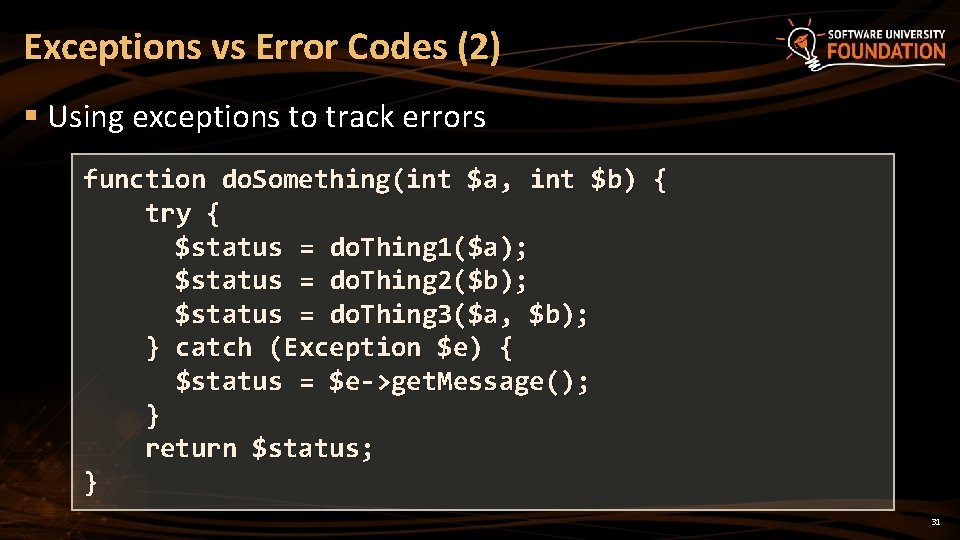
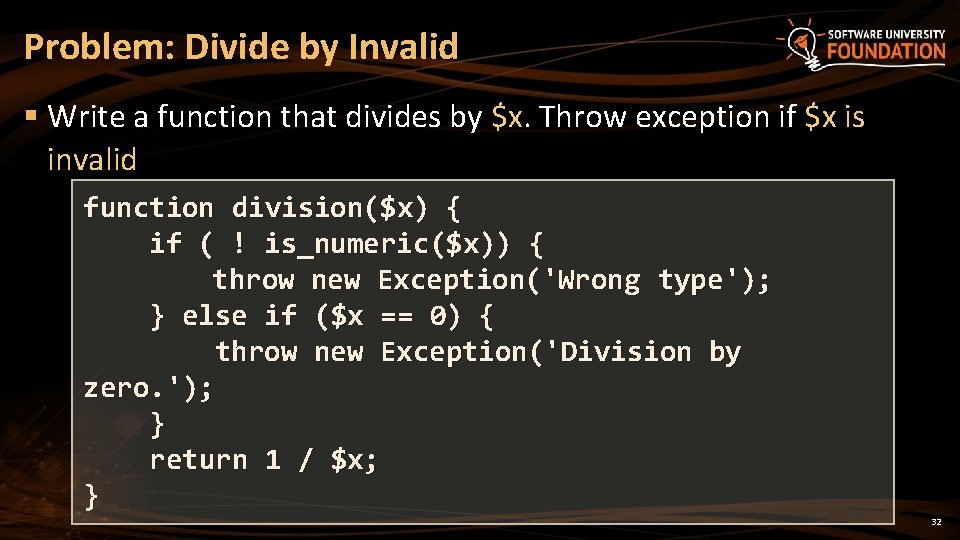
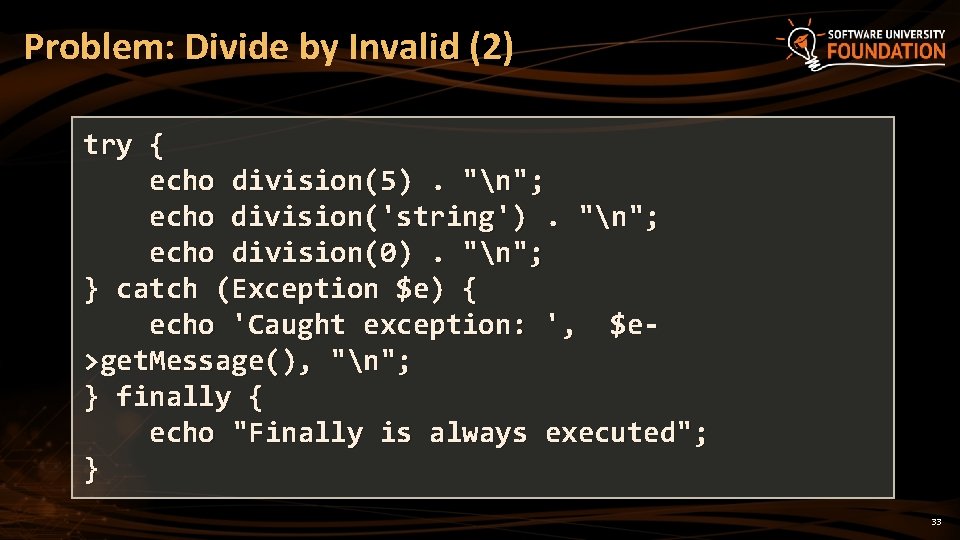
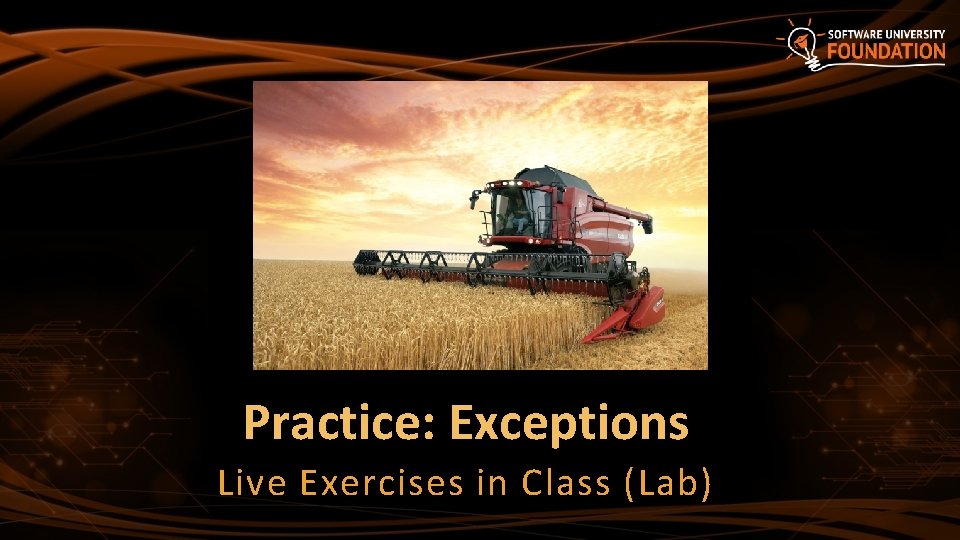
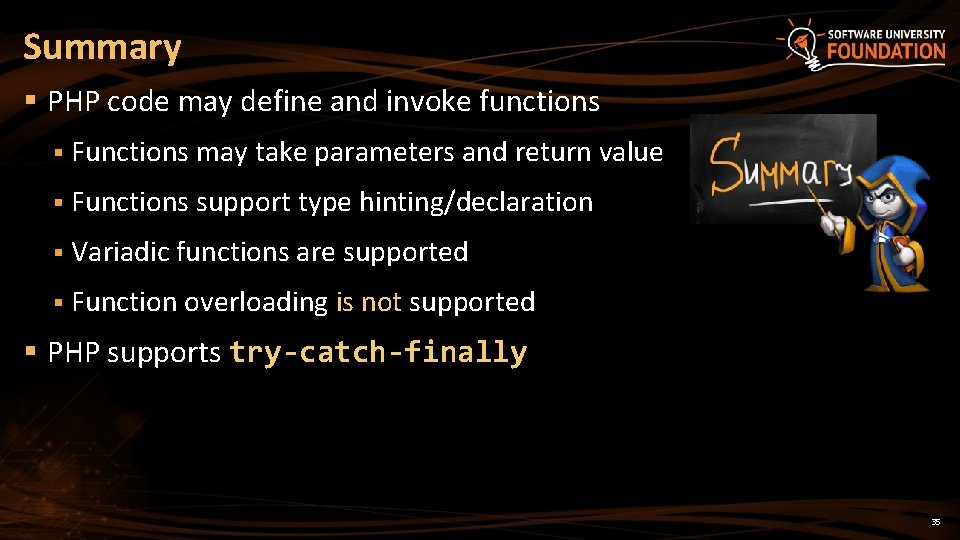
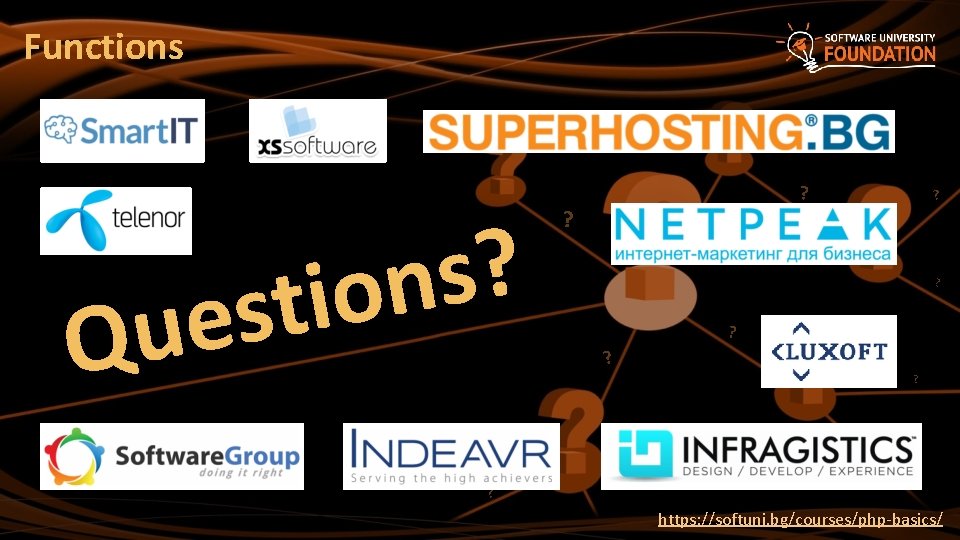
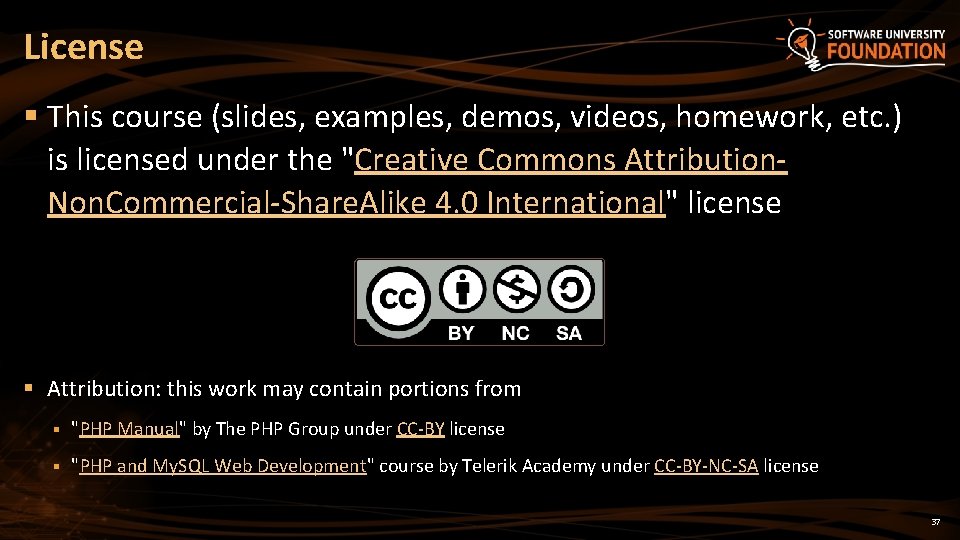
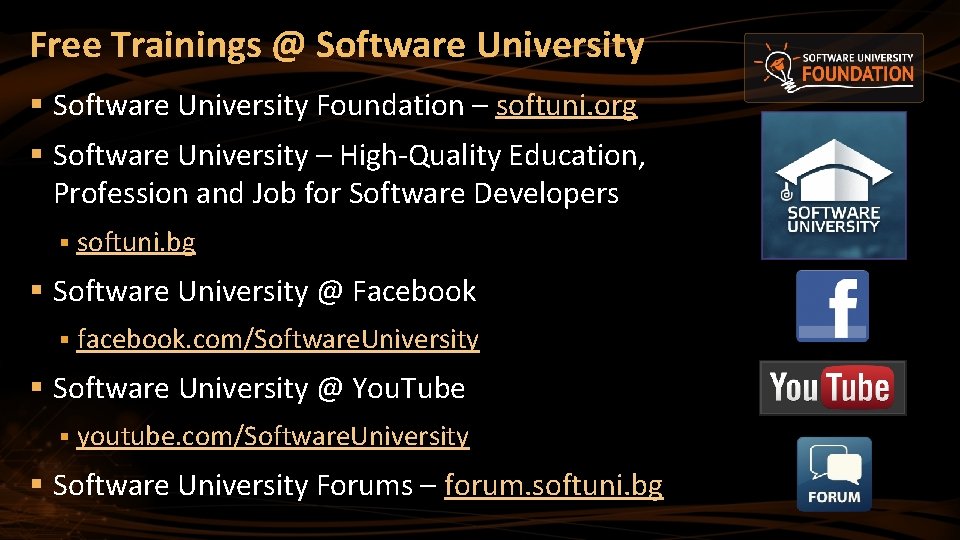
- Slides: 37
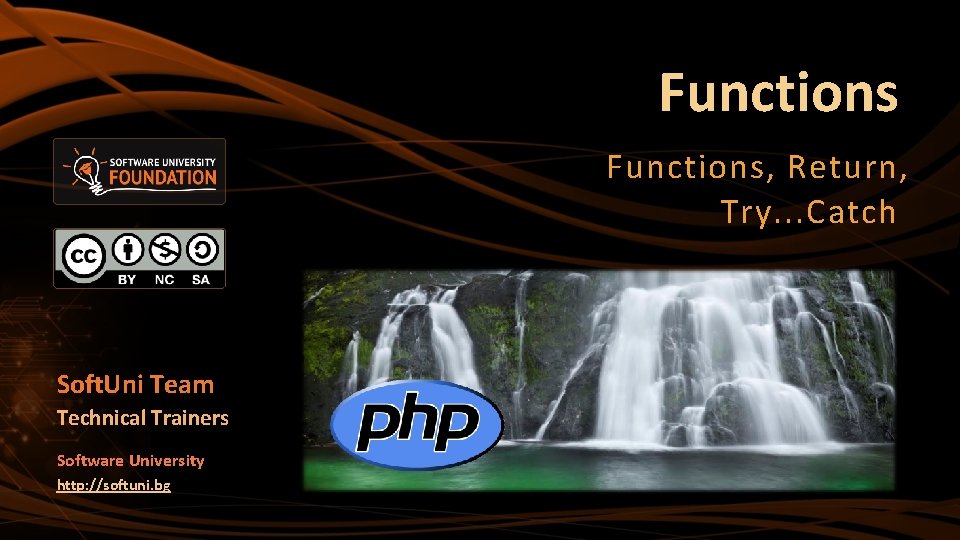
Functions, Return, Try. . . Catch Soft. Uni Team Technical Trainers Software University http: //softuni. bg
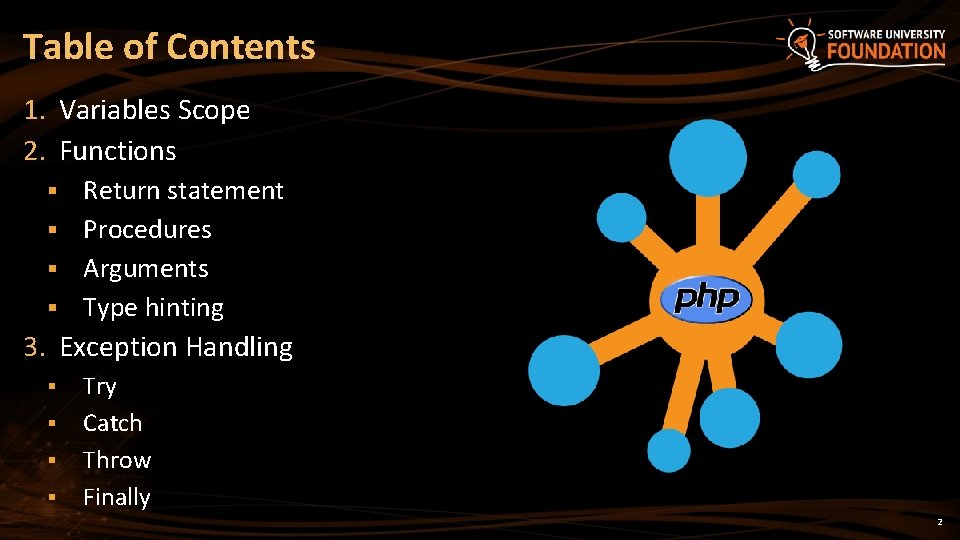
Table of Contents 1. Variables Scope 2. Functions Return statement § Procedures § Arguments § Type hinting § 3. Exception Handling § § Try Catch Throw Finally 2
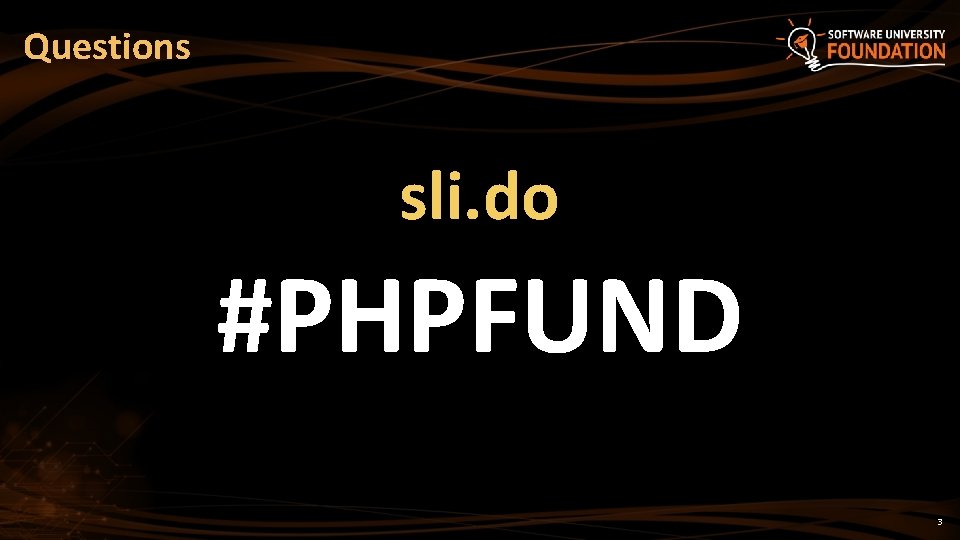
Questions sli. do #PHPFUND 3
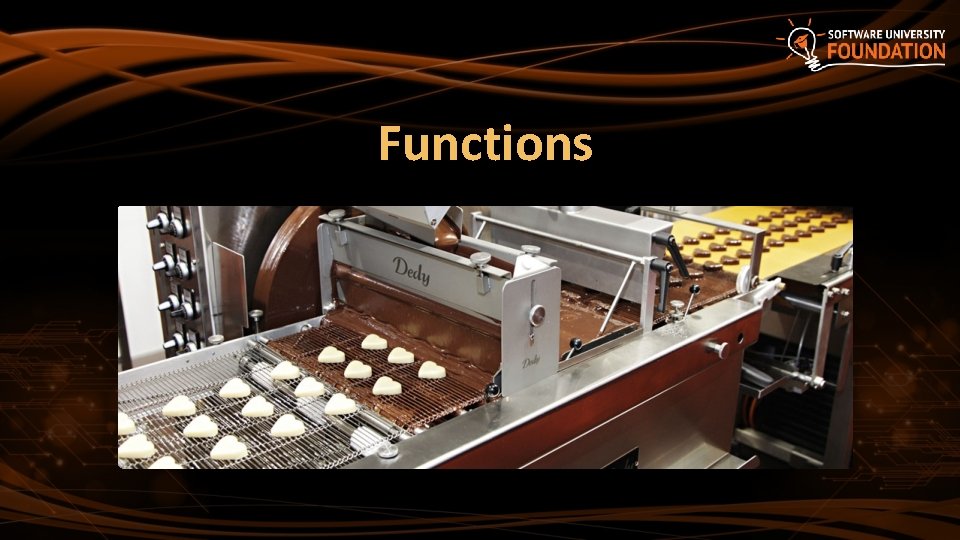
Functions
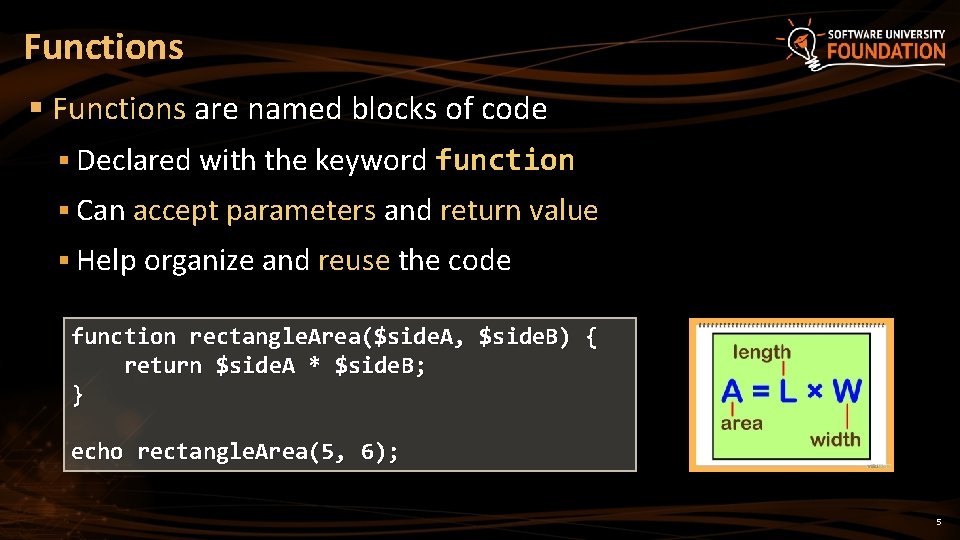
Functions § Functions are named blocks of code § Declared with the keyword function § Can accept parameters and return value § Help organize and reuse the code function rectangle. Area($side. A, $side. B) { return $side. A * $side. B; } echo rectangle. Area(5, 6); 5
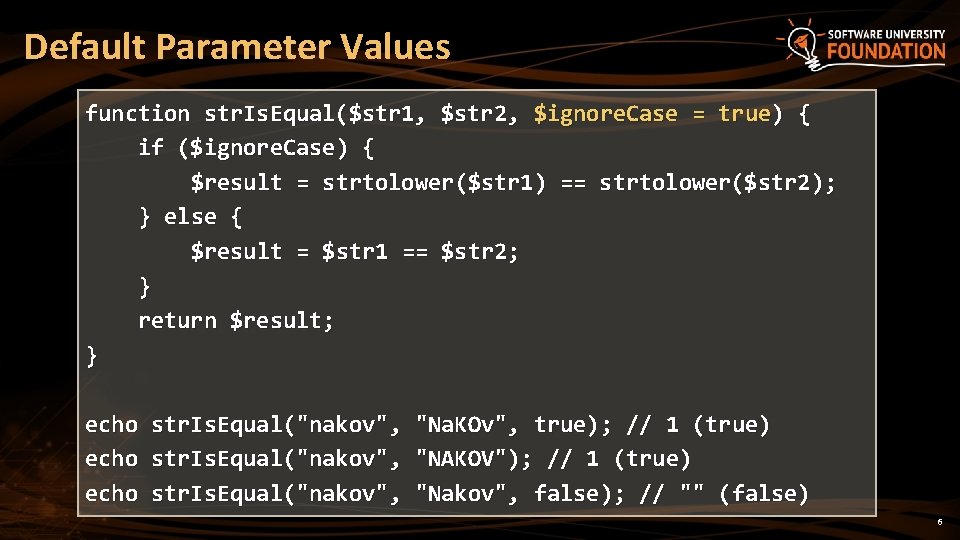
Default Parameter Values function str. Is. Equal($str 1, $str 2, $ignore. Case = true) { if ($ignore. Case) { $result = strtolower($str 1) == strtolower($str 2); } else { $result = $str 1 == $str 2; } return $result; } echo str. Is. Equal("nakov", "Na. KOv", true); // 1 (true) "NAKOV"); // 1 (true) "Nakov", false); // "" (false) 6
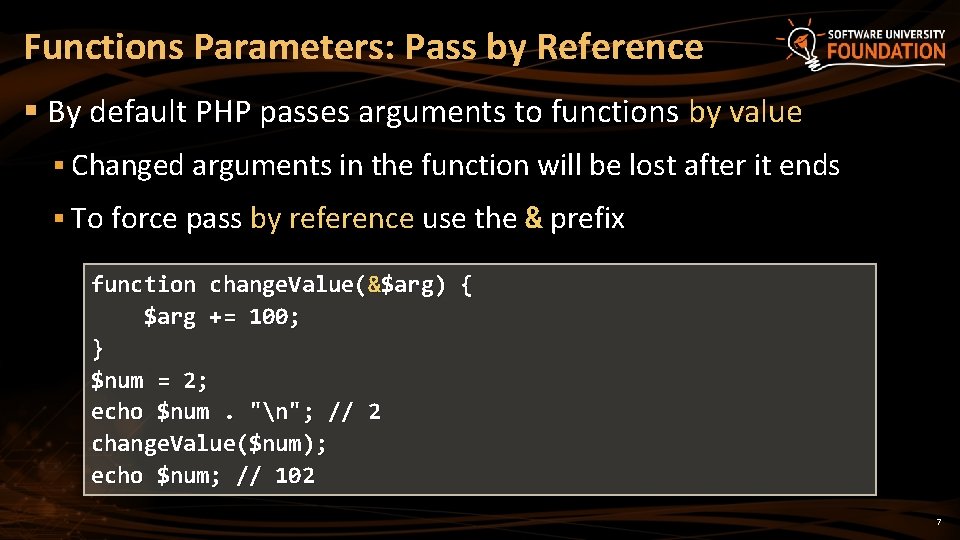
Functions Parameters: Pass by Reference § By default PHP passes arguments to functions by value § Changed arguments in the function will be lost after it ends § To force pass by reference use the & prefix function change. Value(&$arg) { $arg += 100; } $num = 2; echo $num. "n"; // 2 change. Value($num); echo $num; // 102 7
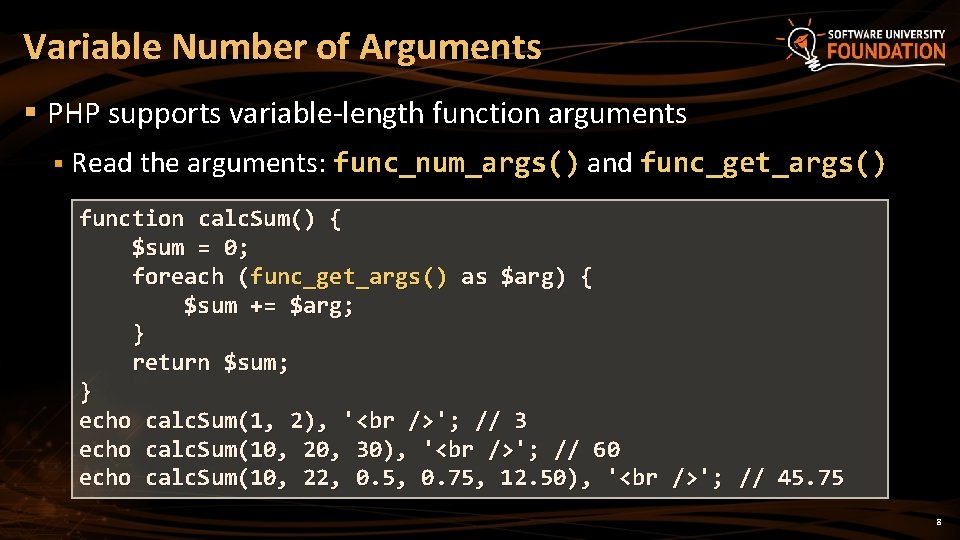
Variable Number of Arguments § PHP supports variable-length function arguments § Read the arguments: func_num_args() and func_get_args() function calc. Sum() { $sum = 0; foreach (func_get_args() as $arg) { $sum += $arg; } return $sum; } echo calc. Sum(1, 2), ' '; // 3 echo calc. Sum(10, 20, 30), ' '; // 60 echo calc. Sum(10, 22, 0. 5, 0. 75, 12. 50), ' '; // 45. 75 8
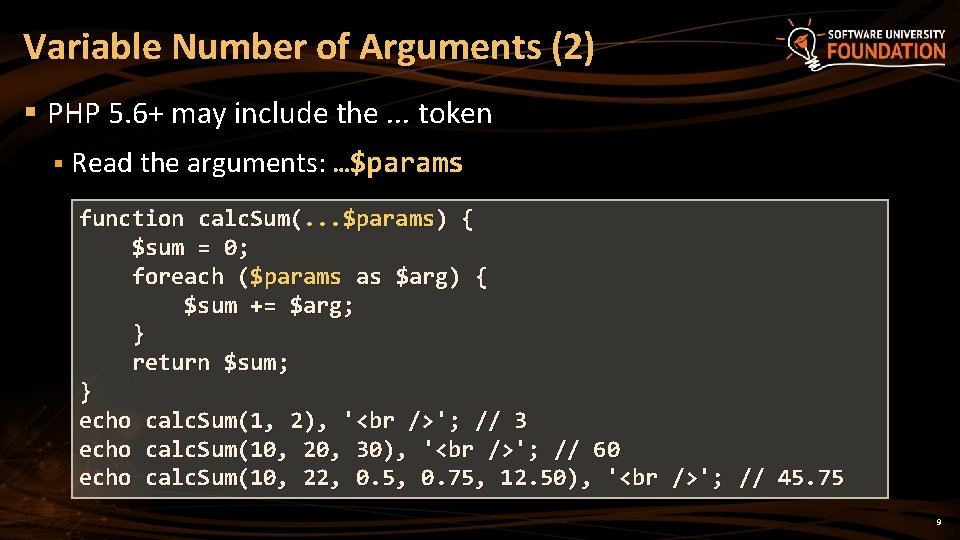
Variable Number of Arguments (2) § PHP 5. 6+ may include the. . . token § Read the arguments: …$params function calc. Sum(. . . $params) { $sum = 0; foreach ($params as $arg) { $sum += $arg; } return $sum; } echo calc. Sum(1, 2), ' '; // 3 echo calc. Sum(10, 20, 30), ' '; // 60 echo calc. Sum(10, 22, 0. 5, 0. 75, 12. 50), ' '; // 45. 75 9
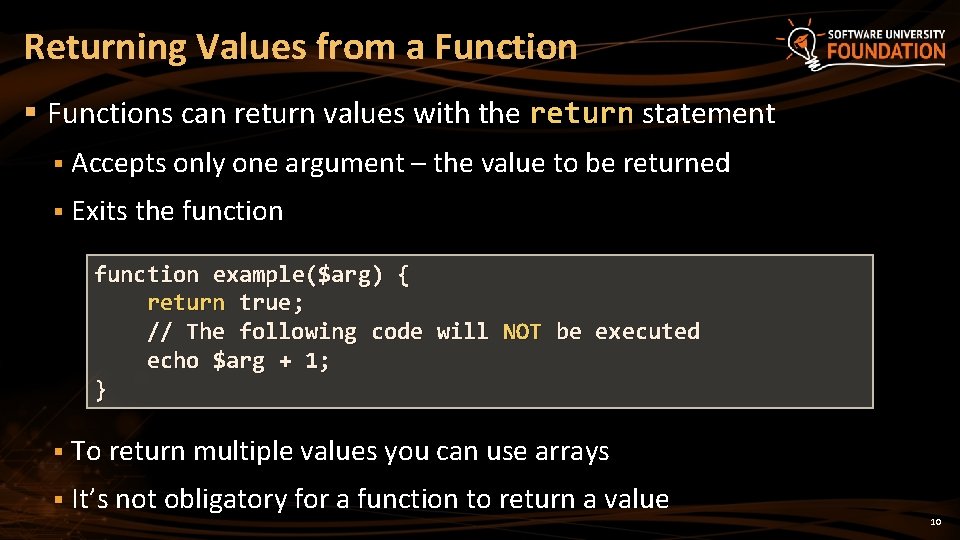
Returning Values from a Function § Functions can return values with the return statement § Accepts only one argument – the value to be returned § Exits the function example($arg) { return true; // The following code will NOT be executed echo $arg + 1; } § To return multiple values you can use arrays § It’s not obligatory for a function to return a value 10
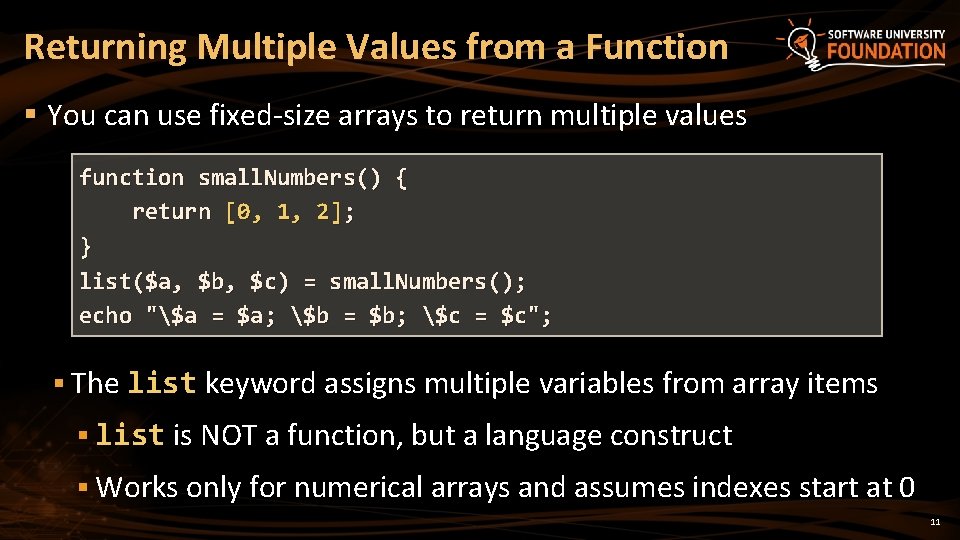
Returning Multiple Values from a Function § You can use fixed-size arrays to return multiple values function small. Numbers() { return [0, 1, 2]; } list($a, $b, $c) = small. Numbers(); echo "$a = $a; $b = $b; $c = $c"; § The list keyword assigns multiple variables from array items § list is NOT a function, but a language construct § Works only for numerical arrays and assumes indexes start at 0 11
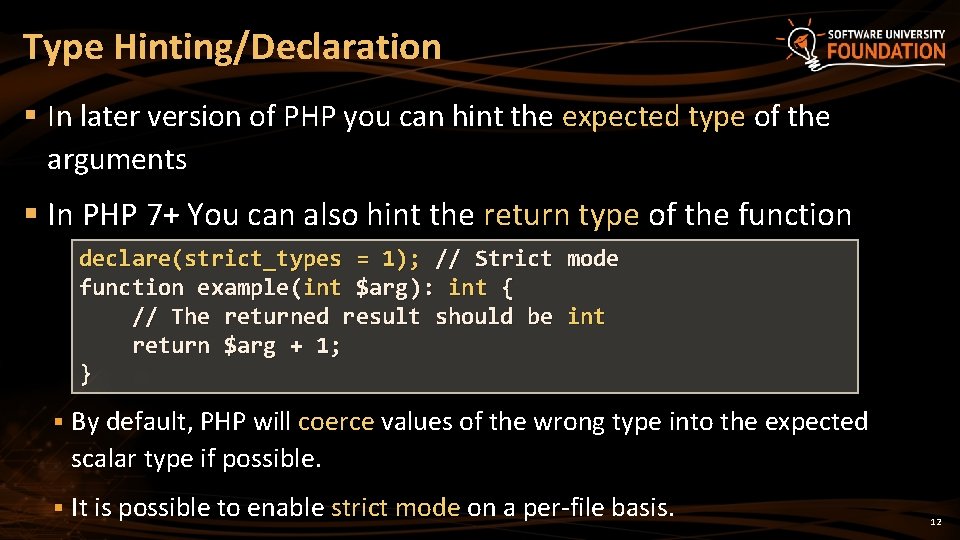
Type Hinting/Declaration § In later version of PHP you can hint the expected type of the arguments § In PHP 7+ You can also hint the return type of the function declare(strict_types = 1); // Strict mode function example(int $arg): int { // The returned result should be int return $arg + 1; } § By default, PHP will coerce values of the wrong type into the expected scalar type if possible. § It is possible to enable strict mode on a per-file basis. 12
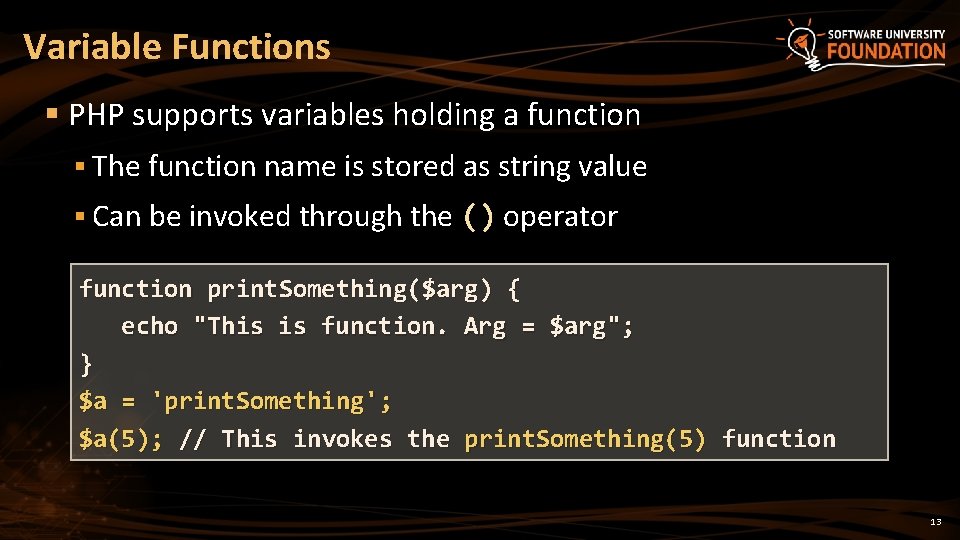
Variable Functions § PHP supports variables holding a function § The function name is stored as string value § Can be invoked through the () operator function print. Something($arg) { echo "This is function. Arg = $arg"; } $a = 'print. Something'; $a(5); // This invokes the print. Something(5) function 13
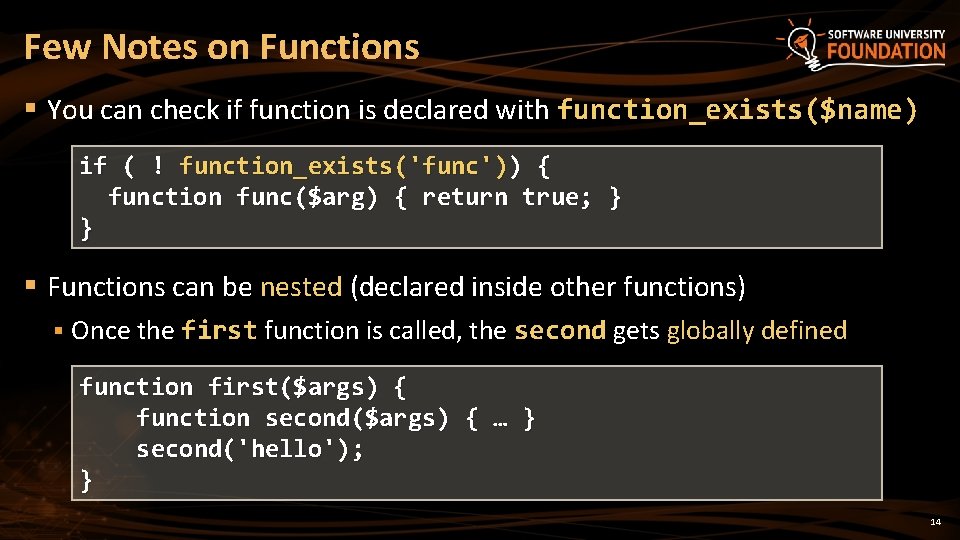
Few Notes on Functions § You can check if function is declared with function_exists($name) if ( ! function_exists('func')) { function func($arg) { return true; } } § Functions can be nested (declared inside other functions) § Once the first function is called, the second gets globally defined function first($args) { function second($args) { … } second('hello'); } 14
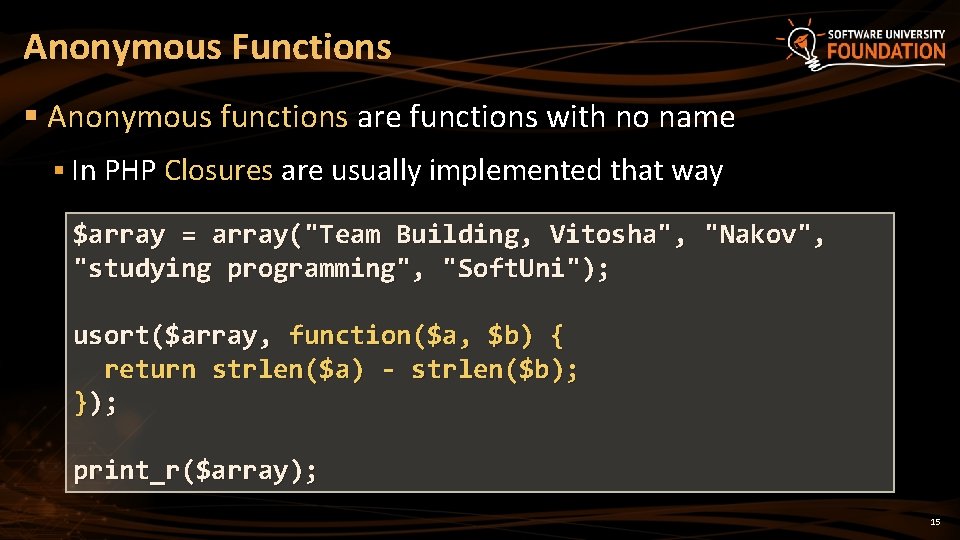
Anonymous Functions § Anonymous functions are functions with no name § In PHP Closures are usually implemented that way $array = array("Team Building, Vitosha", "Nakov", "studying programming", "Soft. Uni"); usort($array, function($a, $b) { return strlen($a) - strlen($b); }); print_r($array); 15
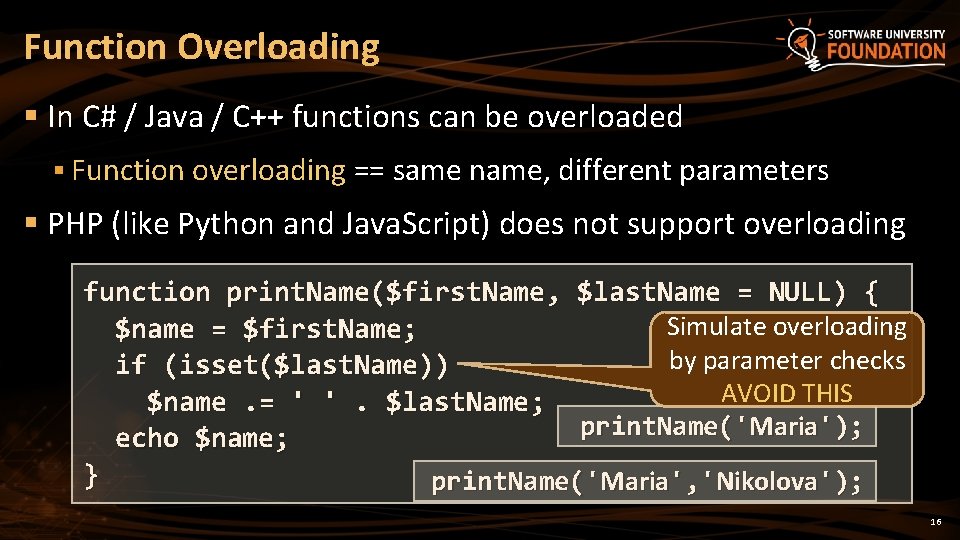
Function Overloading § In C# / Java / C++ functions can be overloaded § Function overloading == same name, different parameters § PHP (like Python and Java. Script) does not support overloading function print. Name($first. Name, $last. Name = NULL) { Simulate overloading $name = $first. Name; by parameter checks if (isset($last. Name)) AVOID THIS $name. = ' '. $last. Name; print. Name('Maria'); echo $name; } print. Name('Maria', 'Nikolova'); 16
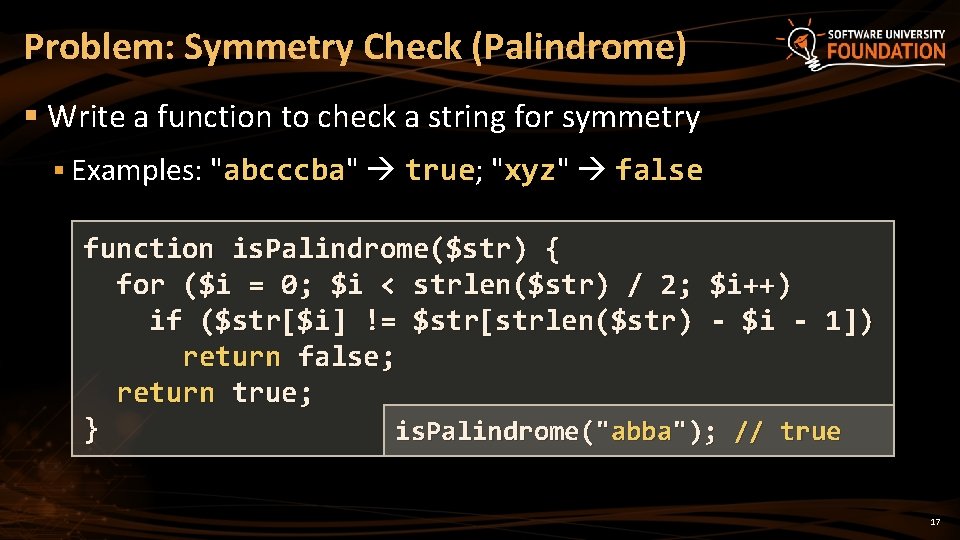
Problem: Symmetry Check (Palindrome) § Write a function to check a string for symmetry § Examples: "abcccba" true; "xyz" false function is. Palindrome($str) { for ($i = 0; $i < strlen($str) / 2; $i++) if ($str[$i] != $str[strlen($str) - $i - 1]) return false; return true; } is. Palindrome("abba"); // true 17
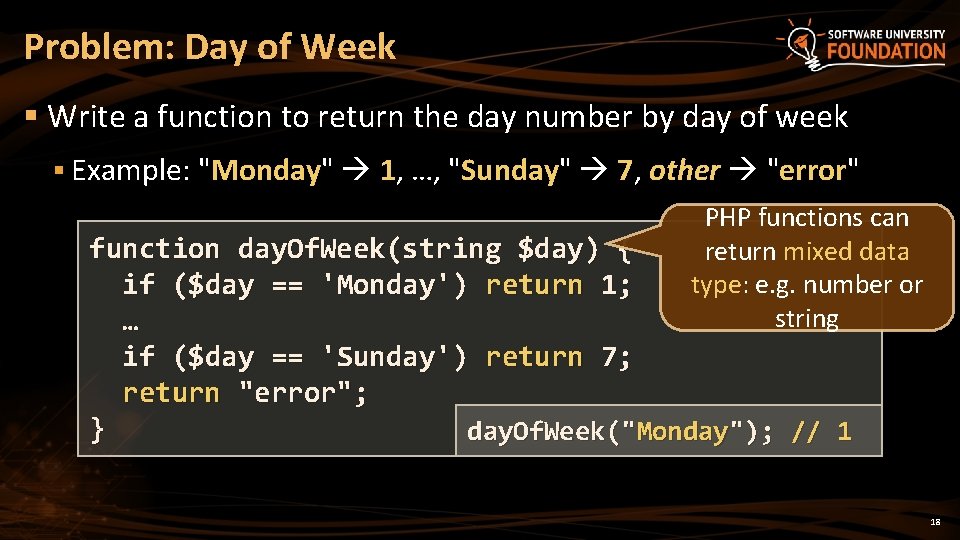
Problem: Day of Week § Write a function to return the day number by day of week § Example: "Monday" 1, …, "Sunday" 7, other "error" PHP functions can return mixed data type: e. g. number or string function day. Of. Week(string $day) { if ($day == 'Monday') return 1; … if ($day == 'Sunday') return 7; return "error"; } day. Of. Week("Monday"); // 1 18
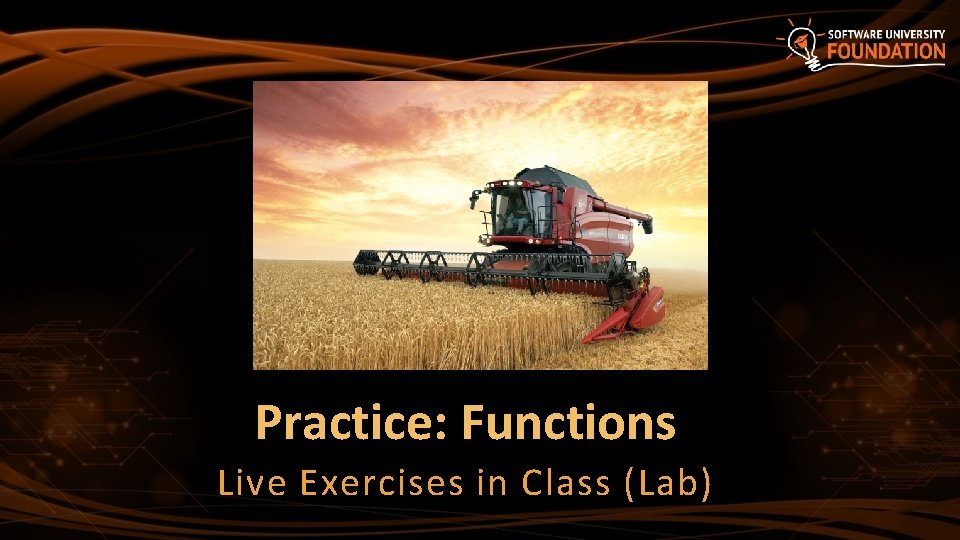
Practice: Functions Live Exercises in Class (Lab)
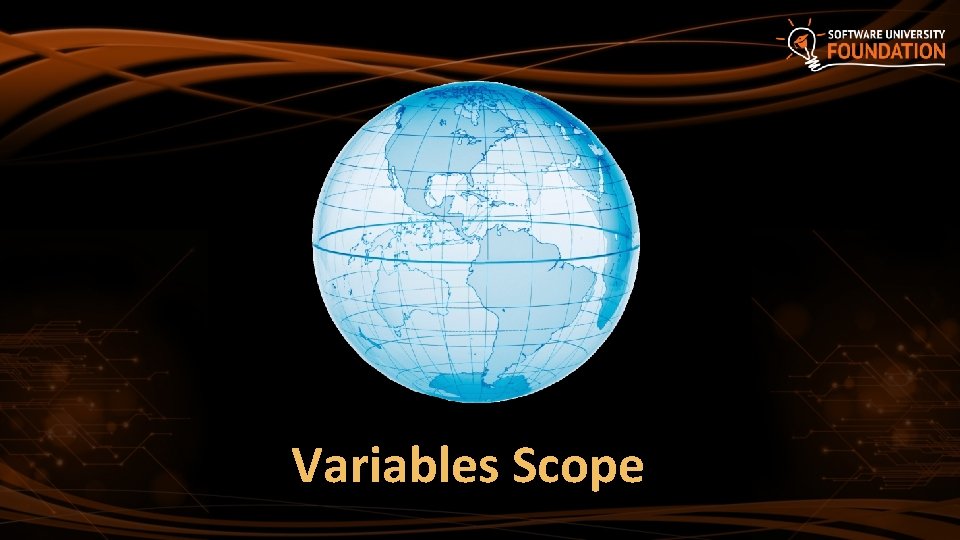
Variables Scope
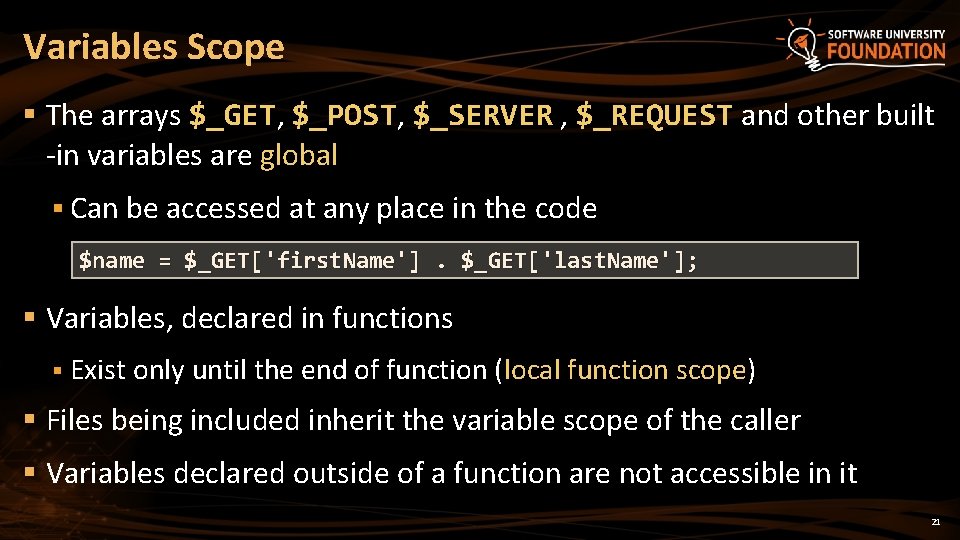
Variables Scope § The arrays $_GET, $_POST, $_SERVER , $_REQUEST and other built -in variables are global § Can be accessed at any place in the code $name = $_GET['first. Name']. $_GET['last. Name']; § Variables, declared in functions § Exist only until the end of function (local function scope) § Files being included inherit the variable scope of the caller § Variables declared outside of a function are not accessible in it 21
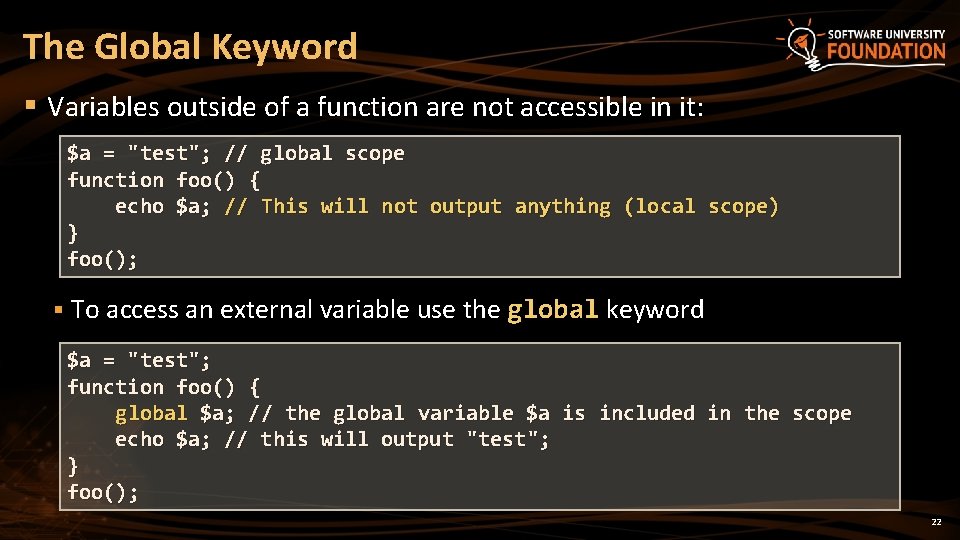
The Global Keyword § Variables outside of a function are not accessible in it: $a = "test"; // global scope function foo() { echo $a; // This will not output anything (local scope) } foo(); § To access an external variable use the global keyword $a = "test"; function foo() { global $a; // the global variable $a is included in the scope echo $a; // this will output "test"; } foo(); 22
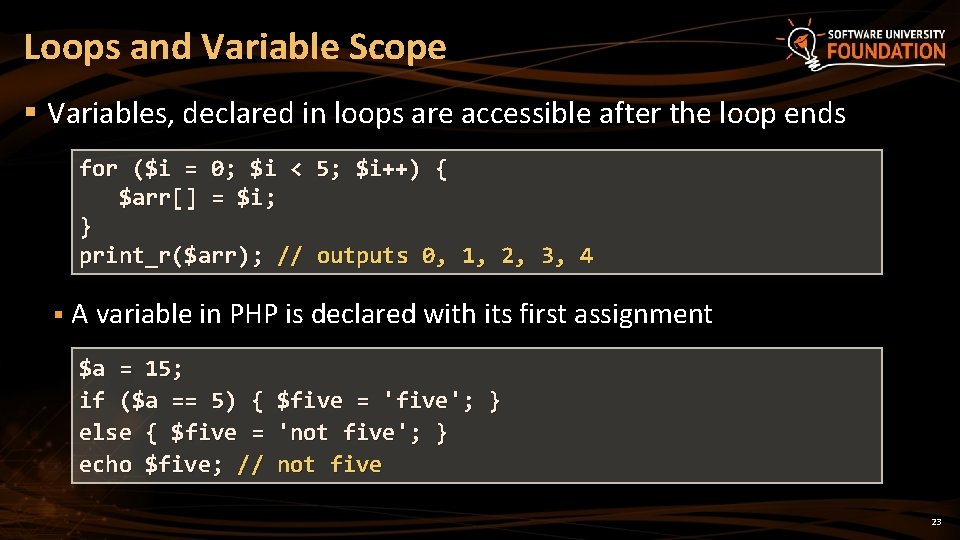
Loops and Variable Scope § Variables, declared in loops are accessible after the loop ends for ($i = 0; $i < 5; $i++) { $arr[] = $i; } print_r($arr); // outputs 0, 1, 2, 3, 4 § A variable in PHP is declared with its first assignment $a = 15; if ($a == 5) { else { $five = echo $five; // $five = 'five'; } 'not five'; } not five 23
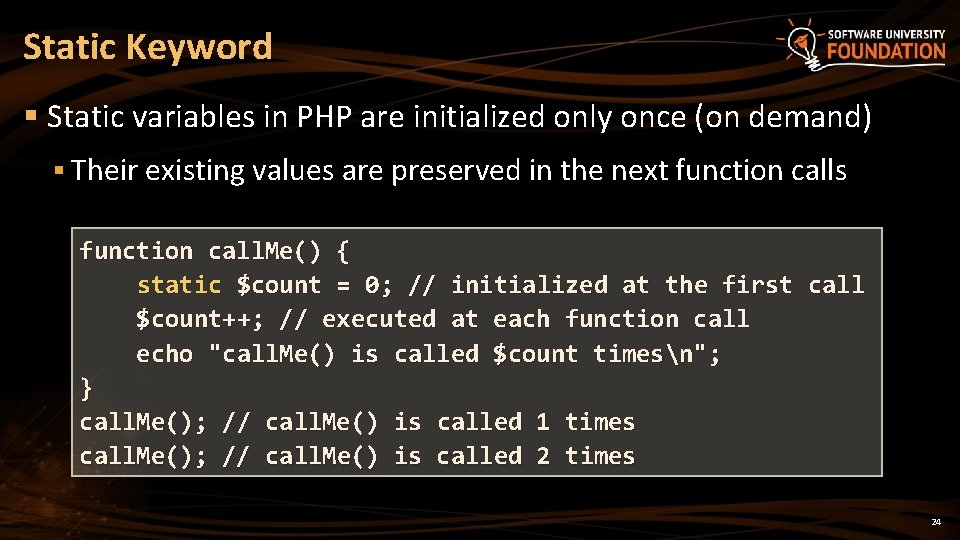
Static Keyword § Static variables in PHP are initialized only once (on demand) § Their existing values are preserved in the next function calls function call. Me() { static $count = 0; // initialized at the first call $count++; // executed at each function call echo "call. Me() is called $count timesn"; } call. Me(); // call. Me() is called 1 times call. Me(); // call. Me() is called 2 times 24
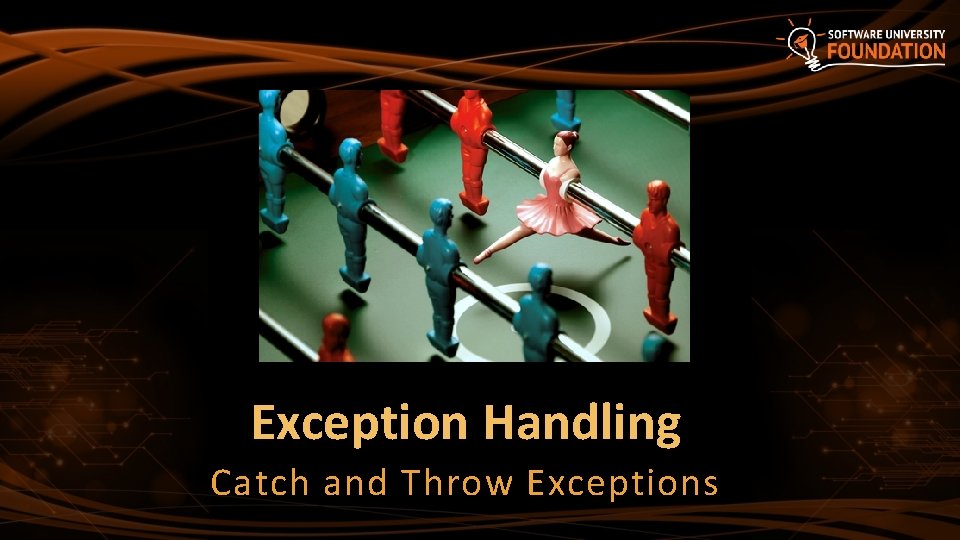
Exception Handling Catch and Throw Exceptions
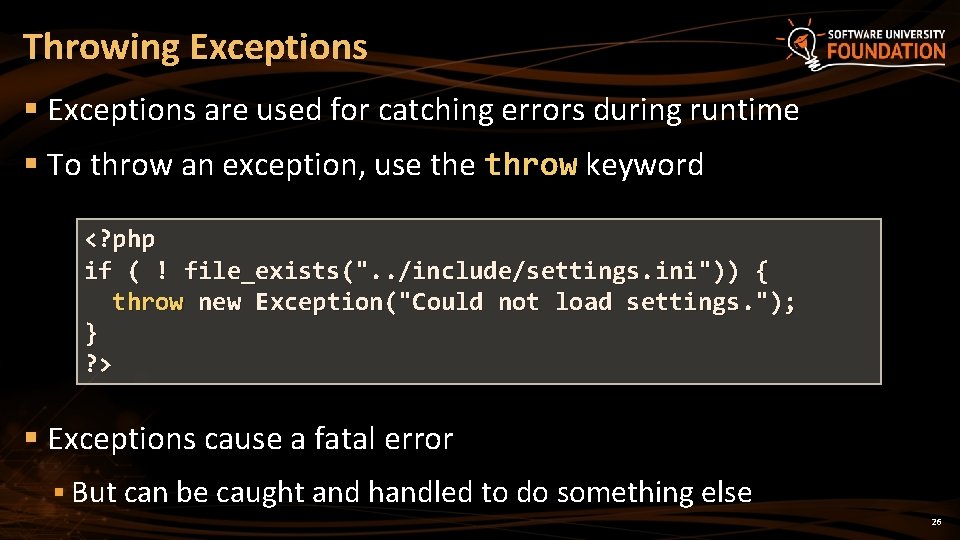
Throwing Exceptions § Exceptions are used for catching errors during runtime § To throw an exception, use throw keyword <? php if ( ! file_exists(". . /include/settings. ini")) { throw new Exception("Could not load settings. "); } ? > § Exceptions cause a fatal error § But can be caught and handled to do something else 26
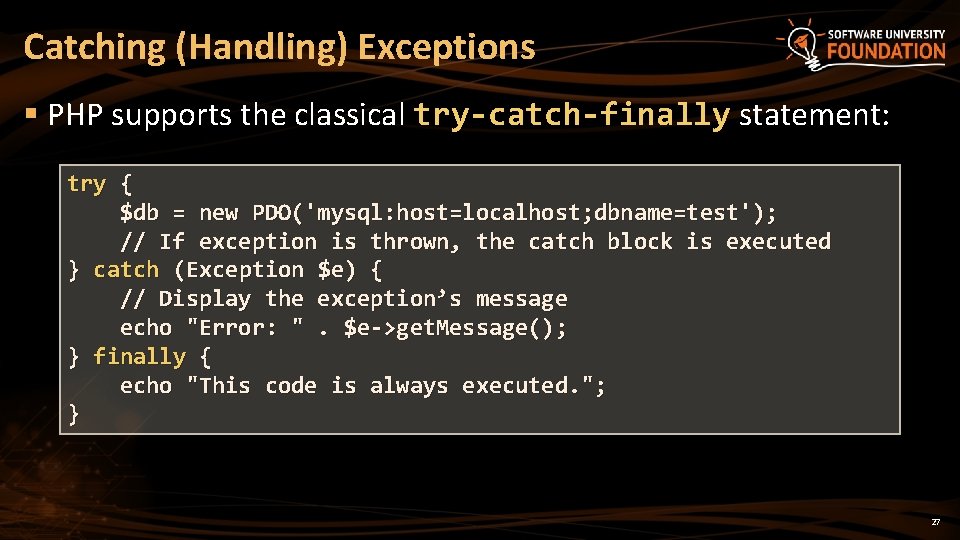
Catching (Handling) Exceptions § PHP supports the classical try-catch-finally statement: try { $db = new PDO('mysql: host=localhost; dbname=test'); // If exception is thrown, the catch block is executed } catch (Exception $e) { // Display the exception’s message echo "Error: ". $e->get. Message(); } finally { echo "This code is always executed. "; } 27
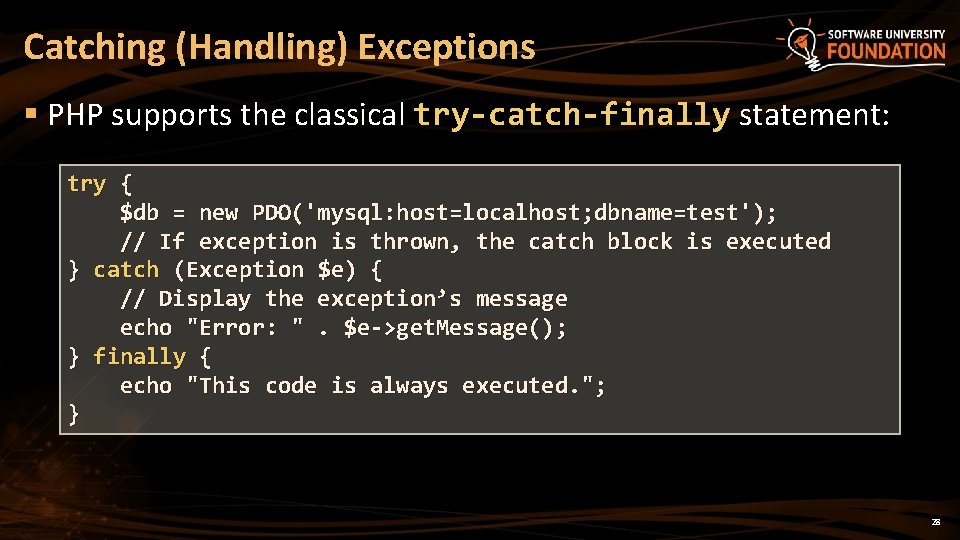
Catching (Handling) Exceptions § PHP supports the classical try-catch-finally statement: try { $db = new PDO('mysql: host=localhost; dbname=test'); // If exception is thrown, the catch block is executed } catch (Exception $e) { // Display the exception’s message echo "Error: ". $e->get. Message(); } finally { echo "This code is always executed. "; } 28
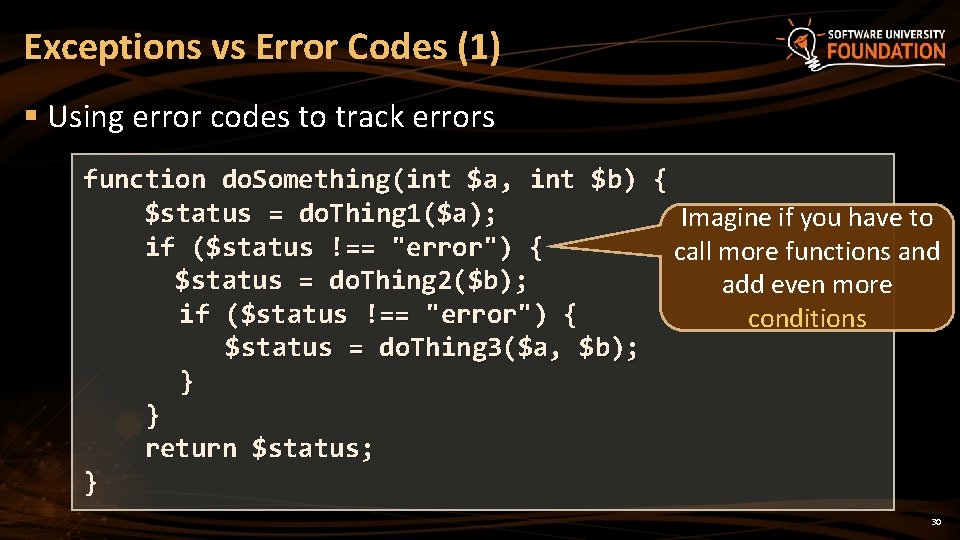
Exceptions vs Error Codes (1) § Using error codes to track errors function do. Something(int $a, int $b) { $status = do. Thing 1($a); Imagine if you have to if ($status !== "error") { call more functions and $status = do. Thing 2($b); add even more if ($status !== "error") { conditions $status = do. Thing 3($a, $b); } } return $status; } 30
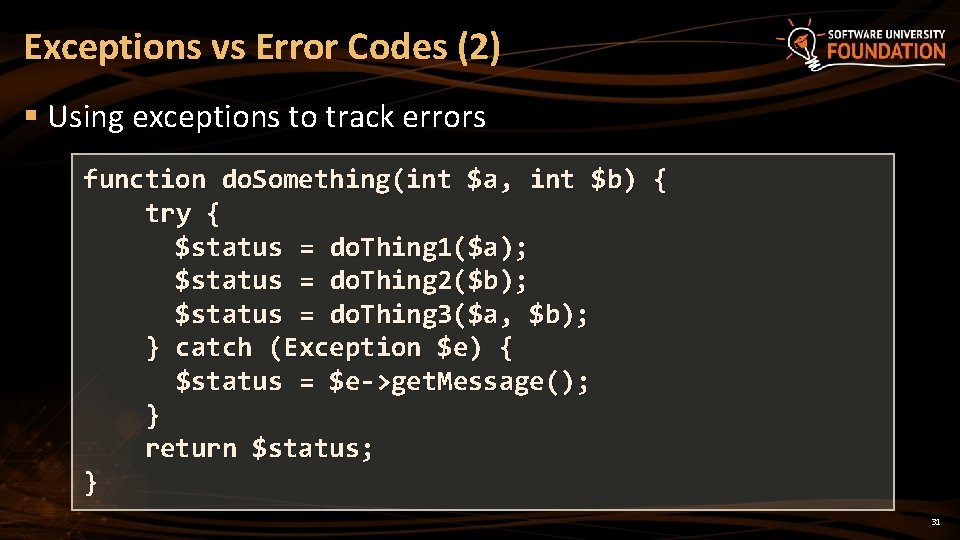
Exceptions vs Error Codes (2) § Using exceptions to track errors function do. Something(int $a, int $b) { try { $status = do. Thing 1($a); $status = do. Thing 2($b); $status = do. Thing 3($a, $b); } catch (Exception $e) { $status = $e->get. Message(); } return $status; } 31
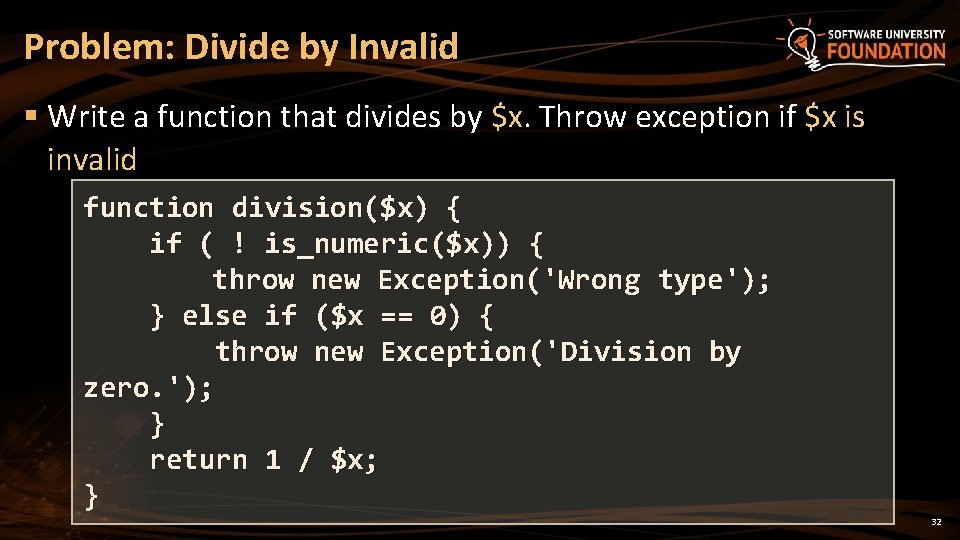
Problem: Divide by Invalid § Write a function that divides by $x. Throw exception if $x is invalid function division($x) { if ( ! is_numeric($x)) { throw new Exception('Wrong type'); } else if ($x == 0) { throw new Exception('Division by zero. '); } return 1 / $x; } 32
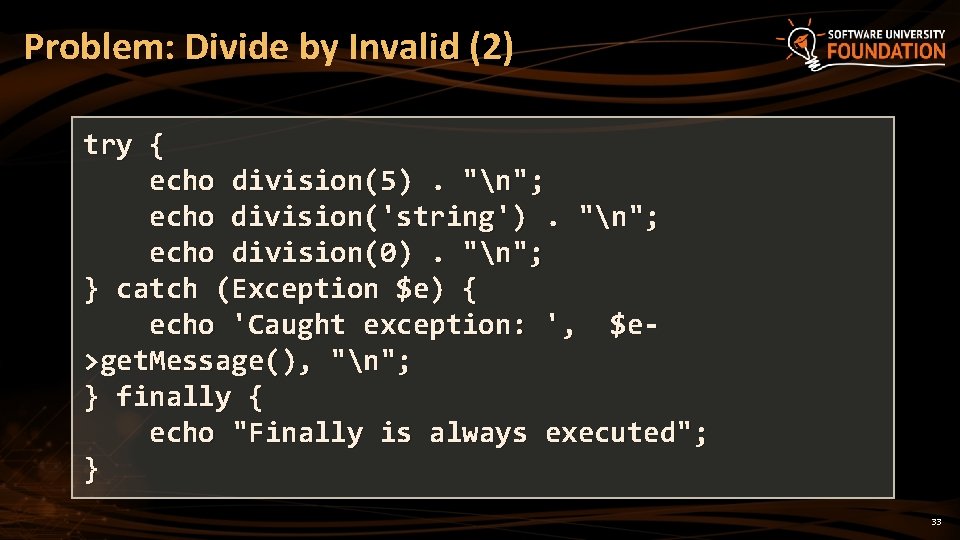
Problem: Divide by Invalid (2) try { echo division(5). "n"; echo division('string'). "n"; echo division(0). "n"; } catch (Exception $e) { echo 'Caught exception: ', $e>get. Message(), "n"; } finally { echo "Finally is always executed"; } 33
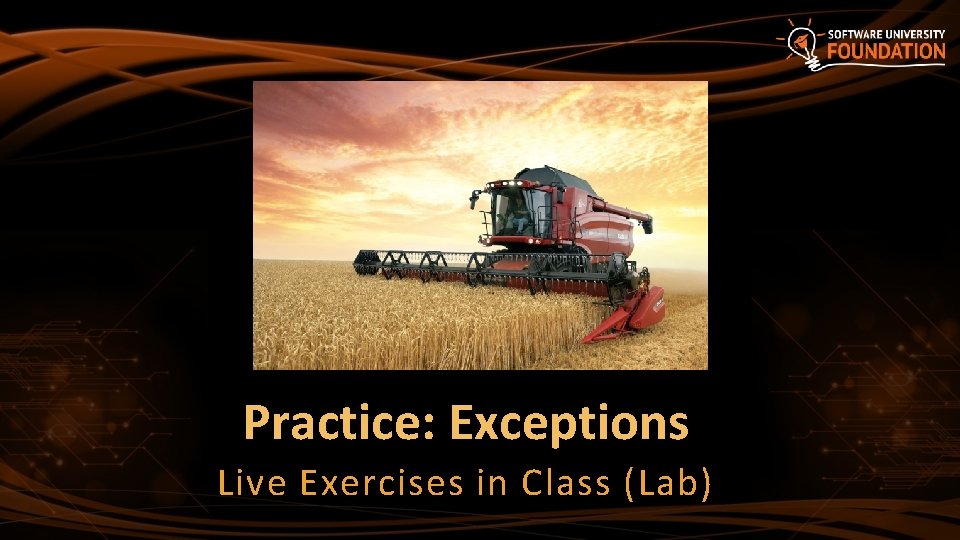
Practice: Exceptions Live Exercises in Class (Lab)
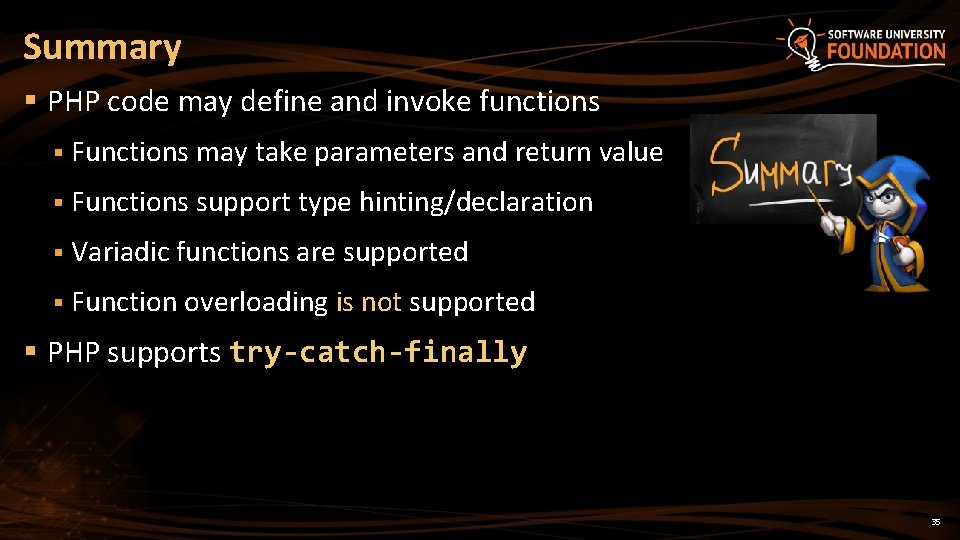
Summary § PHP code may define and invoke functions § Functions may take parameters and return value § Functions support type hinting/declaration § Variadic functions are supported § Function overloading is not supported § PHP supports try-catch-finally 35
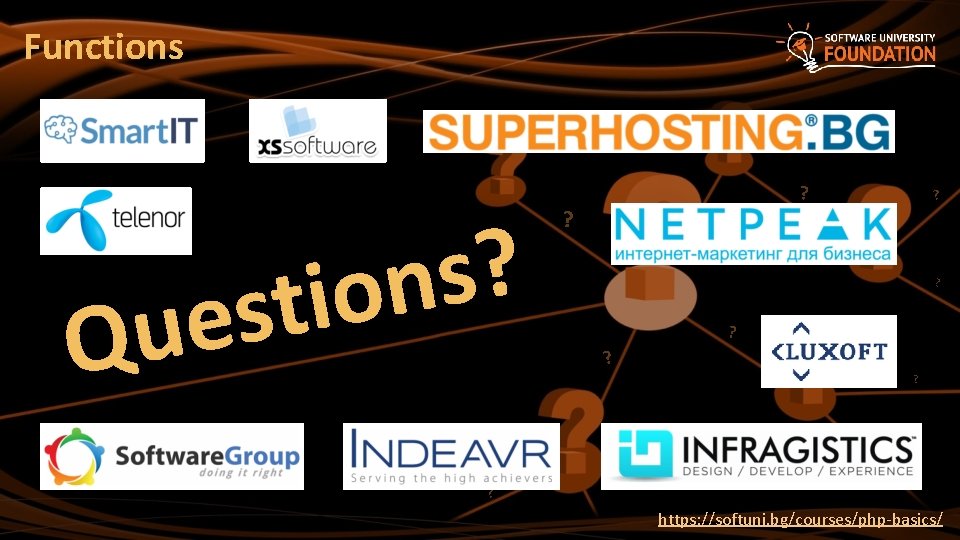
Functions ? s n o i t s e u Q ? ? ? https: //softuni. bg/courses/php-basics/
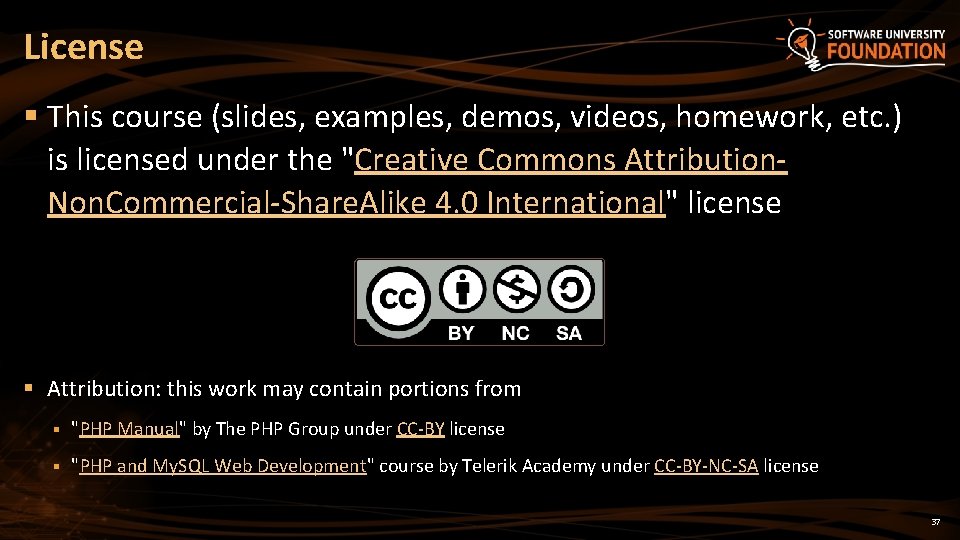
License § This course (slides, examples, demos, videos, homework, etc. ) is licensed under the "Creative Commons Attribution. Non. Commercial-Share. Alike 4. 0 International" license § Attribution: this work may contain portions from § "PHP Manual" by The PHP Group under CC-BY license § "PHP and My. SQL Web Development" course by Telerik Academy under CC-BY-NC-SA license 37
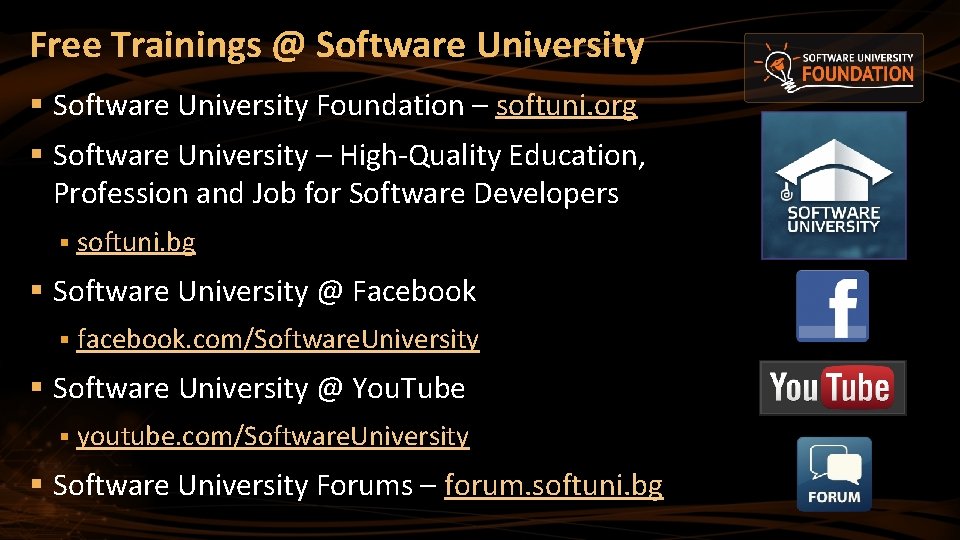
Free Trainings @ Software University § Software University Foundation – softuni. org § Software University – High-Quality Education, Profession and Job for Software Developers § softuni. bg § Software University @ Facebook § facebook. com/Software. University § Software University @ You. Tube § youtube. com/Software. University § Software University Forums – forum. softuni. bg