First Steps in Verilog CPSC 321 Computer Architecture
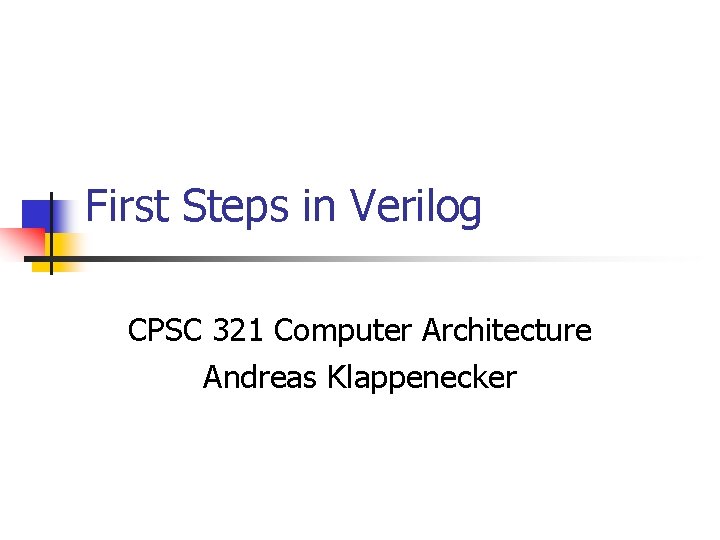
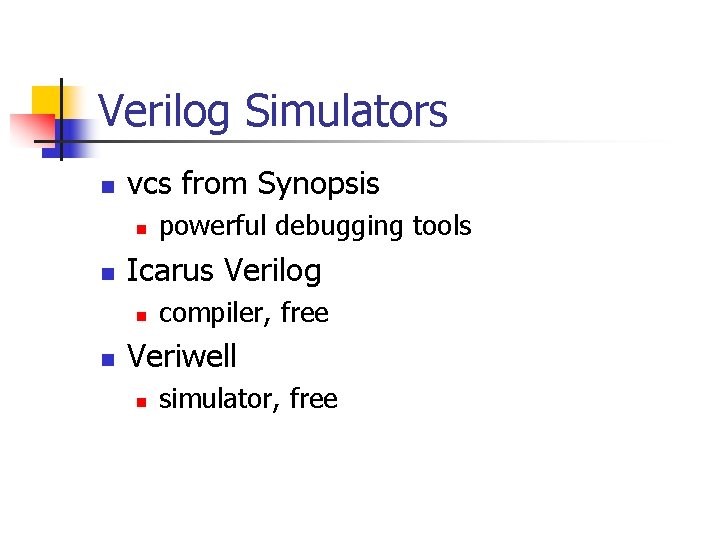
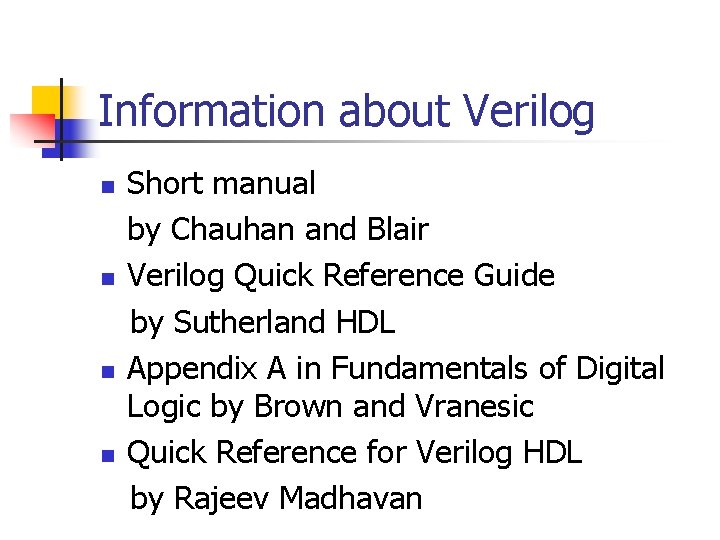
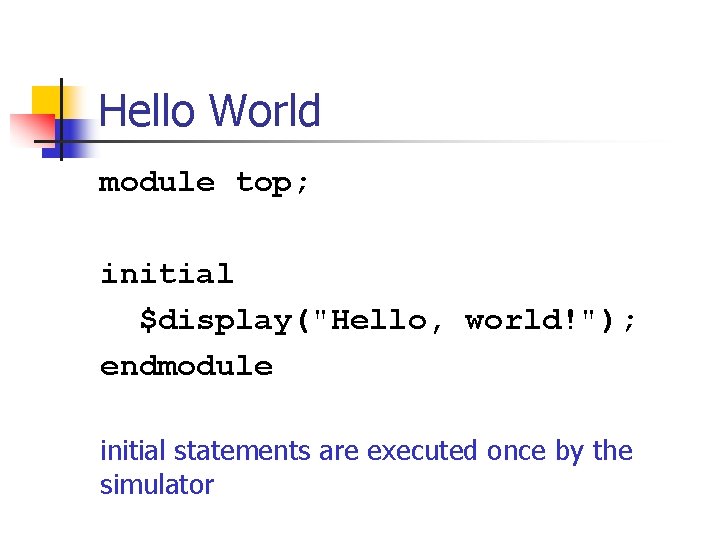
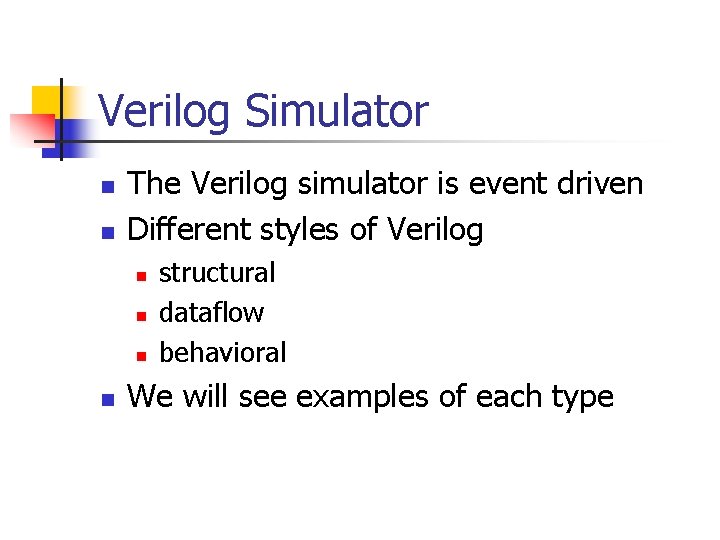
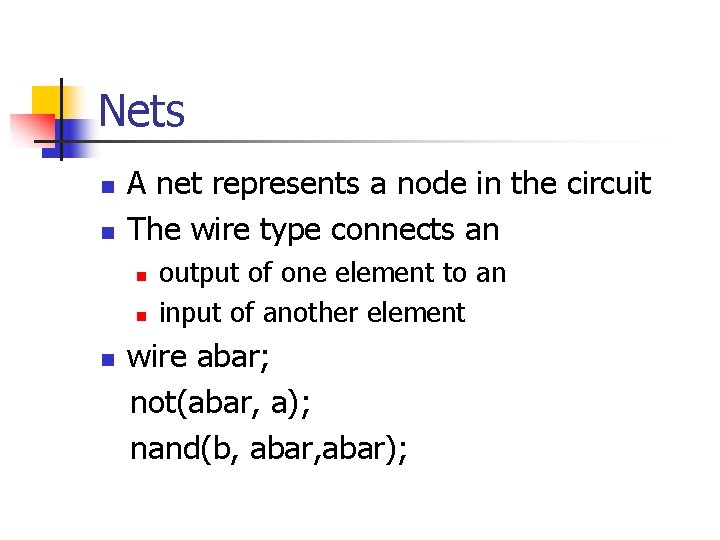
![Vector wires n Range [msb: lsb] wire [3: 0] S; S = 4’b 0011 Vector wires n Range [msb: lsb] wire [3: 0] S; S = 4’b 0011](https://slidetodoc.com/presentation_image_h/0112129b0d47fa0d262449376b133d3c/image-7.jpg)
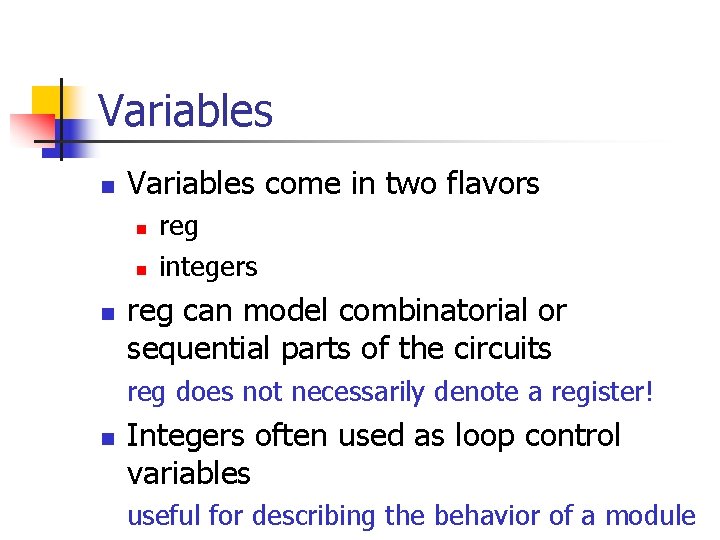
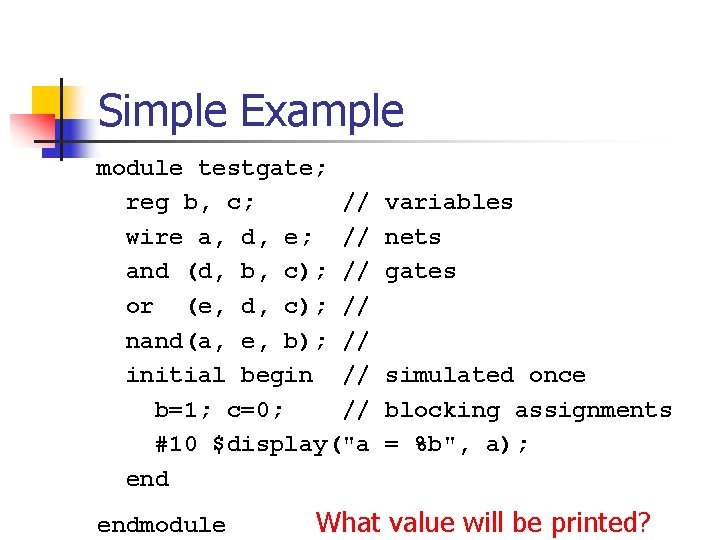
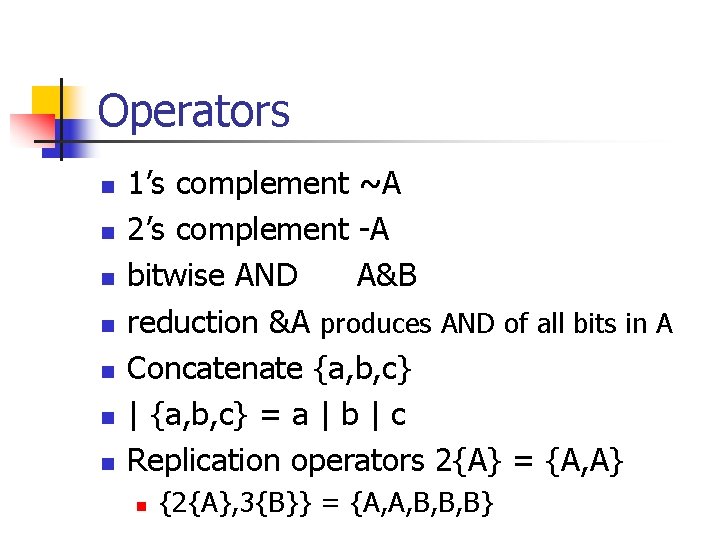
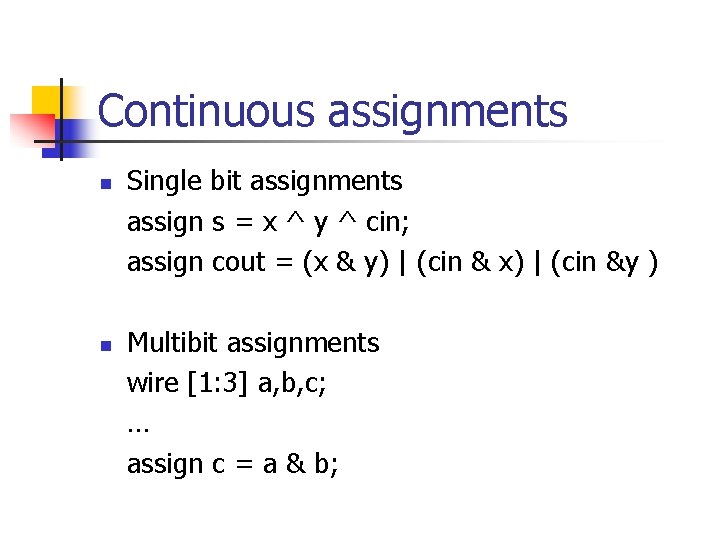
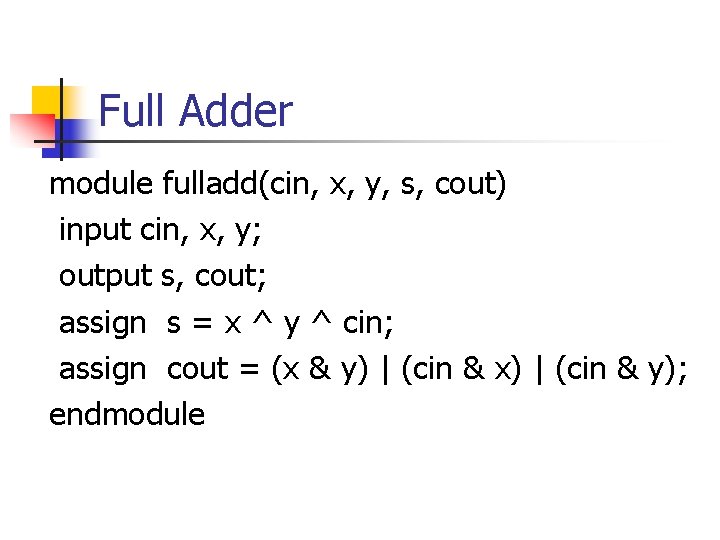
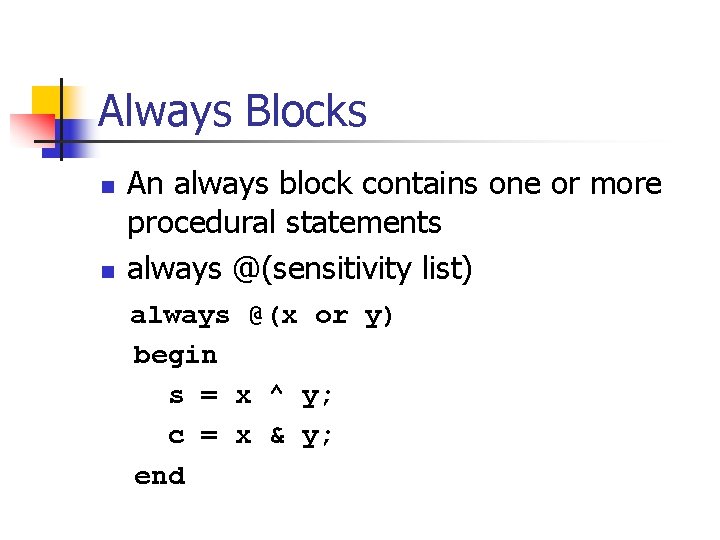
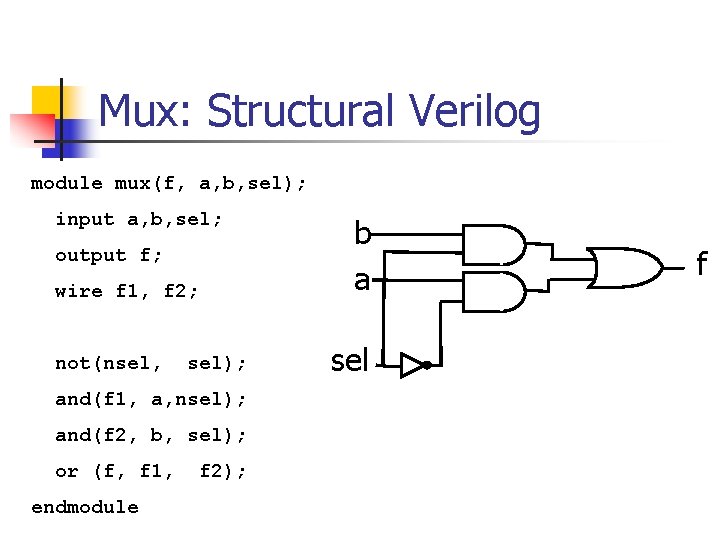
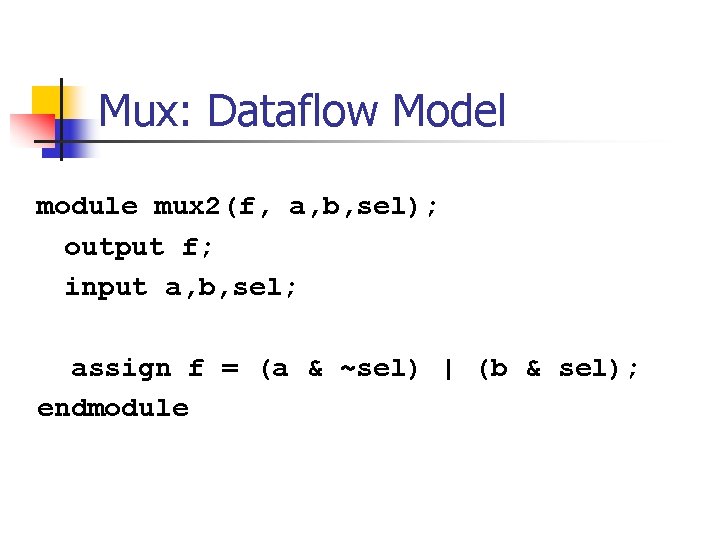
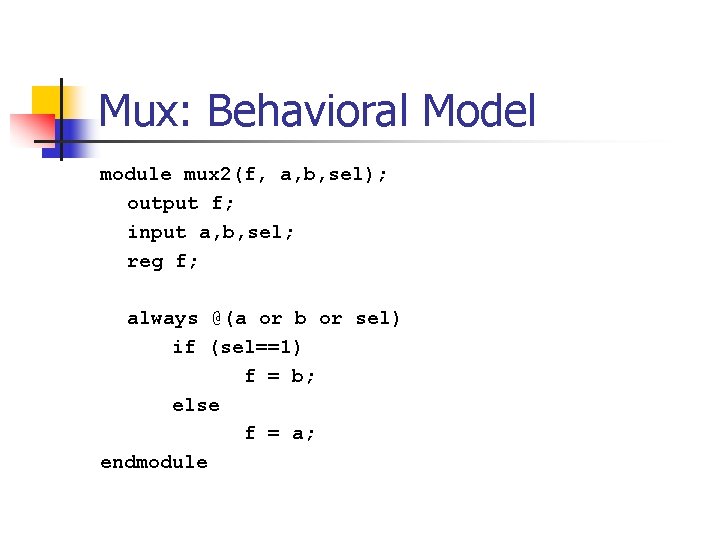
![Sign extension and addition module adder_sign(x, y, s, s 2 s); input [3: 0] Sign extension and addition module adder_sign(x, y, s, s 2 s); input [3: 0]](https://slidetodoc.com/presentation_image_h/0112129b0d47fa0d262449376b133d3c/image-17.jpg)
![Demux Example 2 -to-4 demultiplexer with active low enable a b z[3] z[2] z[1] Demux Example 2 -to-4 demultiplexer with active low enable a b z[3] z[2] z[1]](https://slidetodoc.com/presentation_image_h/0112129b0d47fa0d262449376b133d3c/image-18.jpg)
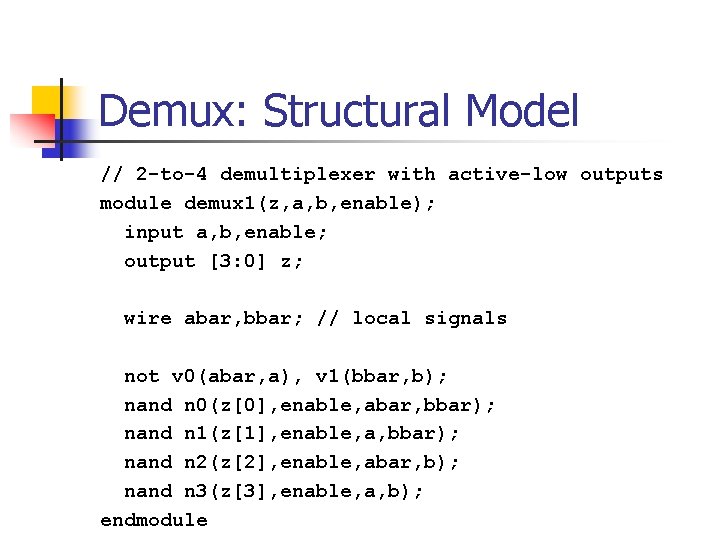
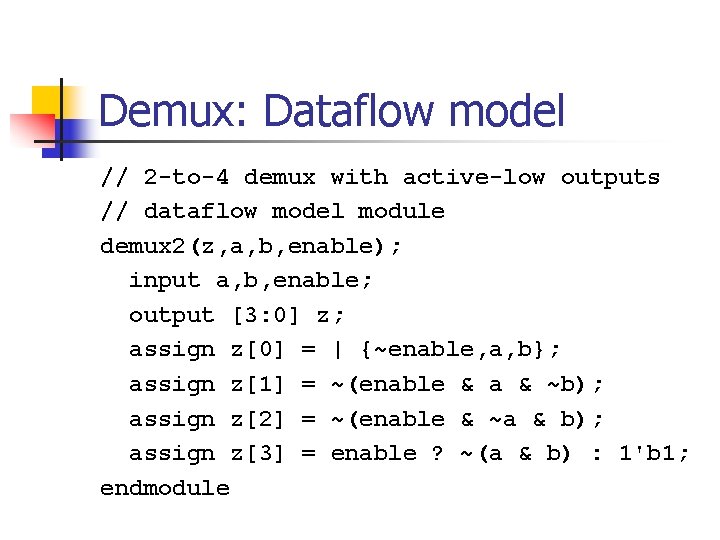
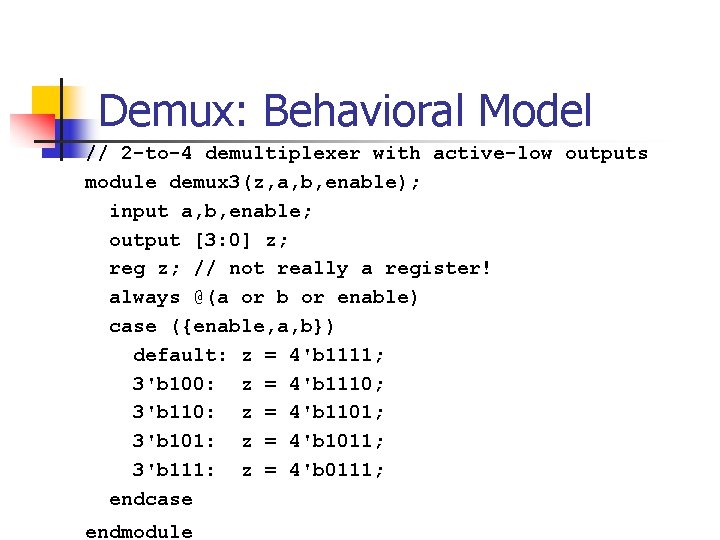
- Slides: 21
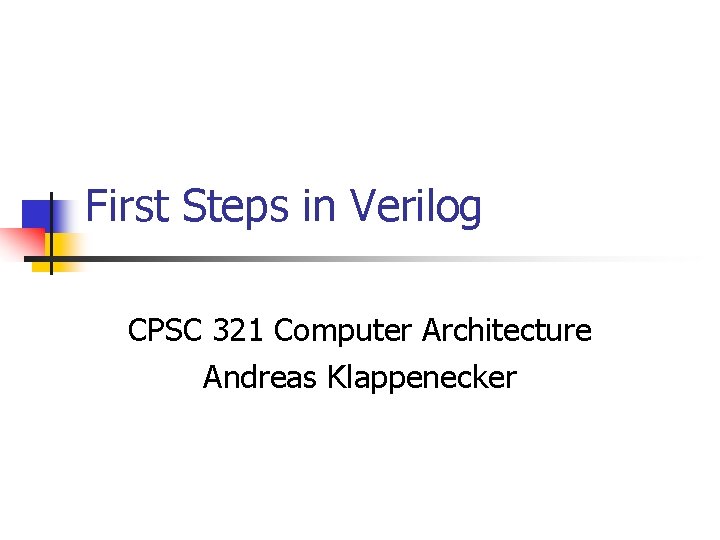
First Steps in Verilog CPSC 321 Computer Architecture Andreas Klappenecker
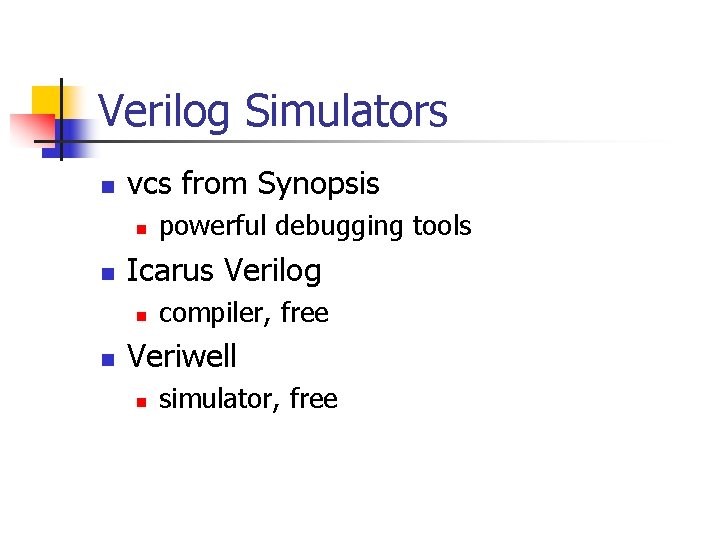
Verilog Simulators n vcs from Synopsis n n Icarus Verilog n n powerful debugging tools compiler, free Veriwell n simulator, free
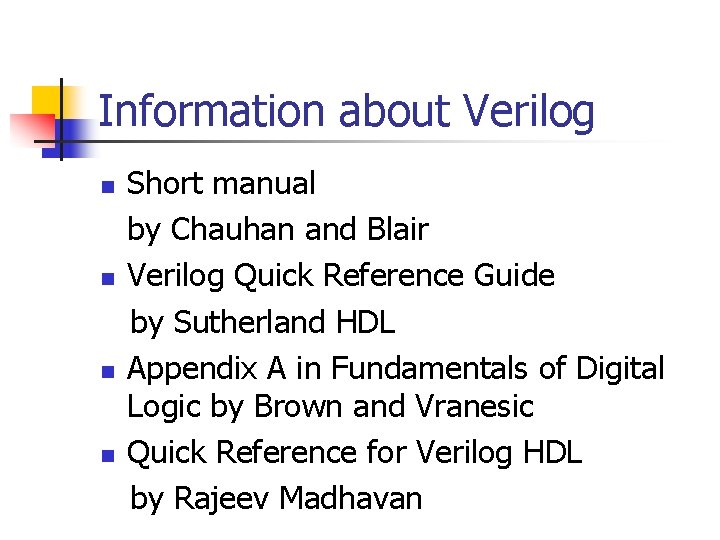
Information about Verilog n n Short manual by Chauhan and Blair Verilog Quick Reference Guide by Sutherland HDL Appendix A in Fundamentals of Digital Logic by Brown and Vranesic Quick Reference for Verilog HDL by Rajeev Madhavan
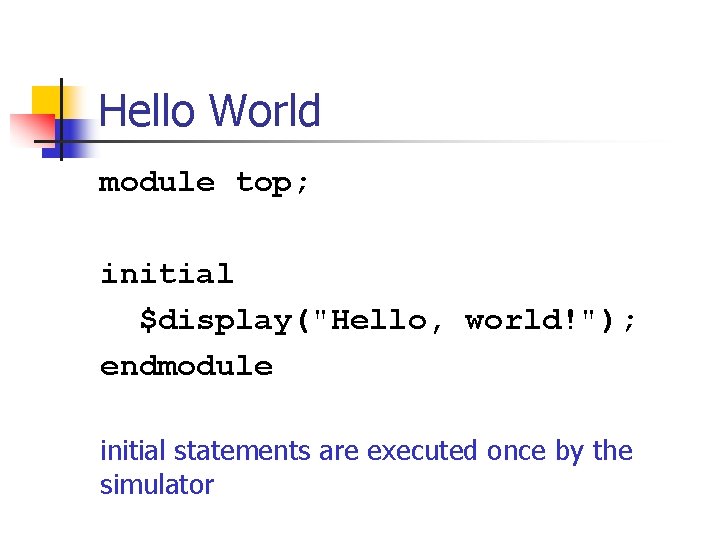
Hello World module top; initial $display("Hello, world!"); endmodule initial statements are executed once by the simulator
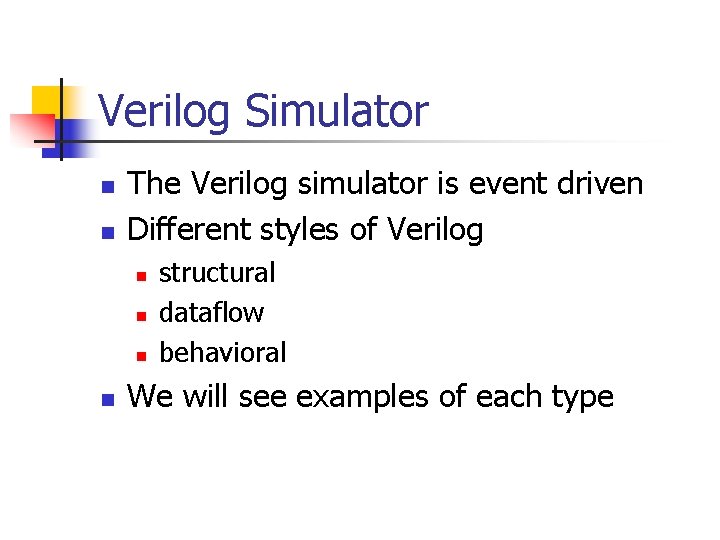
Verilog Simulator n n The Verilog simulator is event driven Different styles of Verilog n n structural dataflow behavioral We will see examples of each type
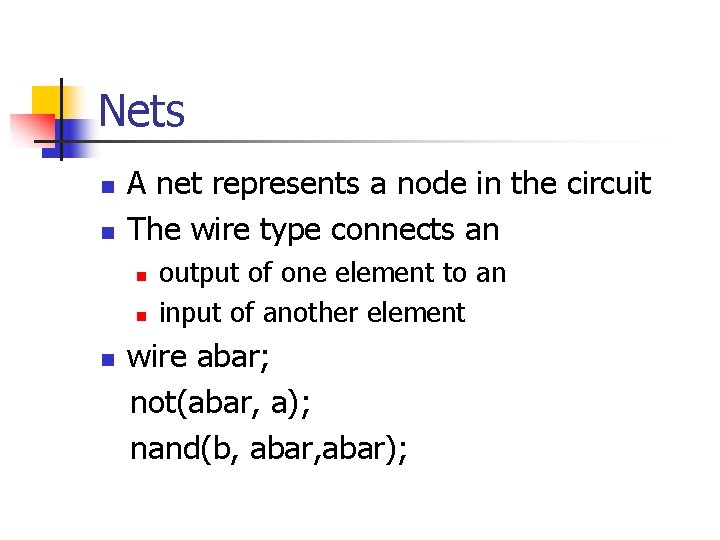
Nets n n A net represents a node in the circuit The wire type connects an n output of one element to an input of another element wire abar; not(abar, a); nand(b, abar);
![Vector wires n Range msb lsb wire 3 0 S S 4b 0011 Vector wires n Range [msb: lsb] wire [3: 0] S; S = 4’b 0011](https://slidetodoc.com/presentation_image_h/0112129b0d47fa0d262449376b133d3c/image-7.jpg)
Vector wires n Range [msb: lsb] wire [3: 0] S; S = 4’b 0011 n The result of this assignment is S[3] = 0, S[2] = 0, S[1] = 1, S[0] = 1 wire [1: 2] A; A = S[2: 1]; means A[1] = S[2], A[2] = S[1]
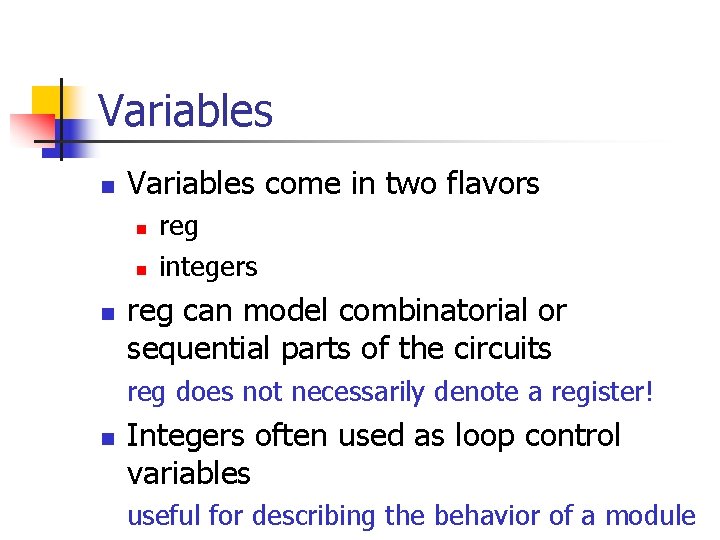
Variables n Variables come in two flavors n n n reg integers reg can model combinatorial or sequential parts of the circuits reg does not necessarily denote a register! n Integers often used as loop control variables useful for describing the behavior of a module
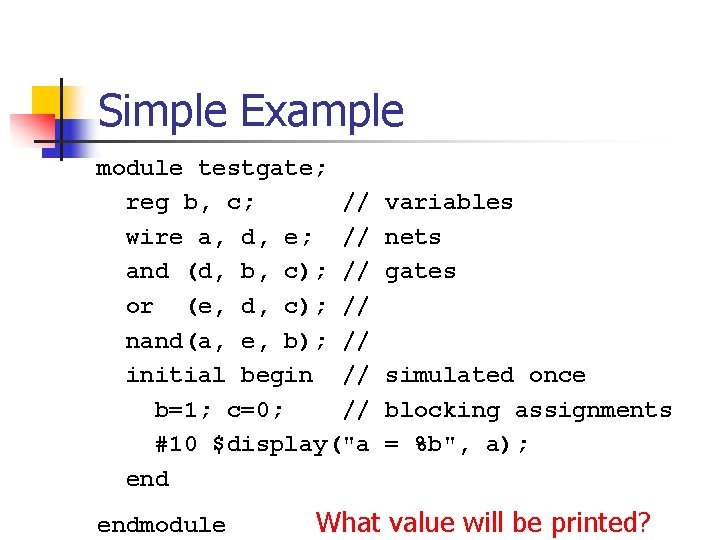
Simple Example module testgate; reg b, c; // wire a, d, e; // and (d, b, c); // or (e, d, c); // nand(a, e, b); // initial begin // b=1; c=0; // #10 $display("a endmodule variables nets gates simulated once blocking assignments = %b", a); What value will be printed?
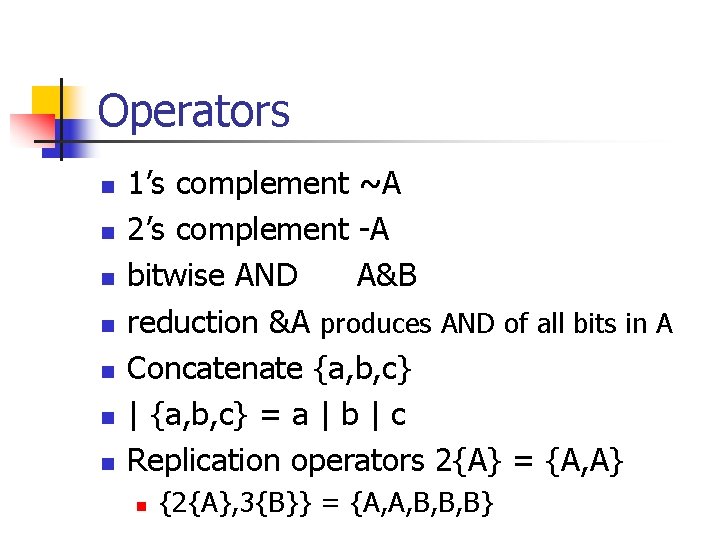
Operators n n n n 1’s complement ~A 2’s complement -A bitwise AND A&B reduction &A produces AND of all bits in A Concatenate {a, b, c} | {a, b, c} = a | b | c Replication operators 2{A} = {A, A} n {2{A}, 3{B}} = {A, A, B, B, B}
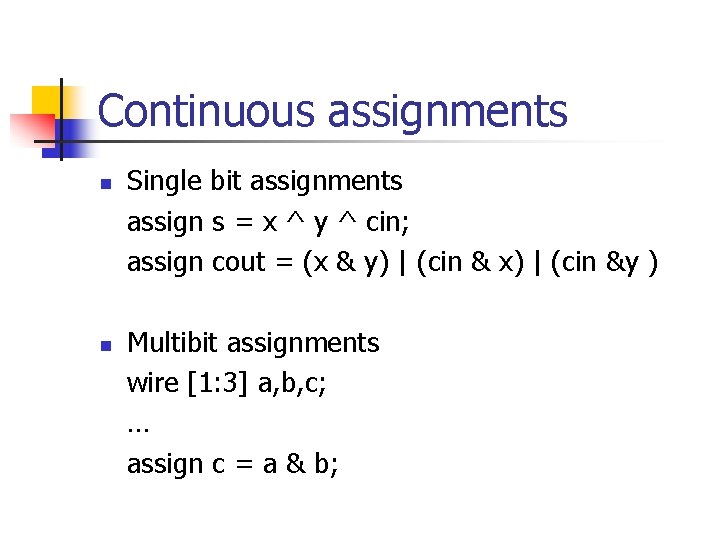
Continuous assignments n n Single bit assignments assign s = x ^ y ^ cin; assign cout = (x & y) | (cin & x) | (cin &y ) Multibit assignments wire [1: 3] a, b, c; … assign c = a & b;
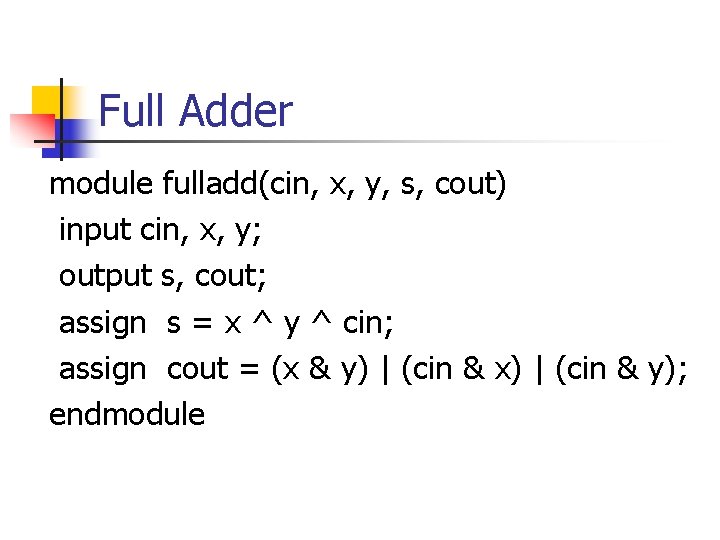
Full Adder module fulladd(cin, x, y, s, cout) input cin, x, y; output s, cout; assign s = x ^ y ^ cin; assign cout = (x & y) | (cin & x) | (cin & y); endmodule
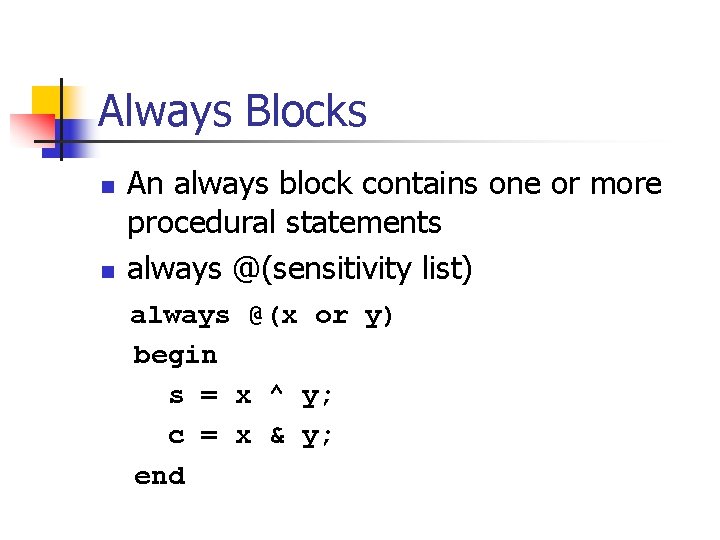
Always Blocks n n An always block contains one or more procedural statements always @(sensitivity list) always @(x or y) begin s = x ^ y; c = x & y; end
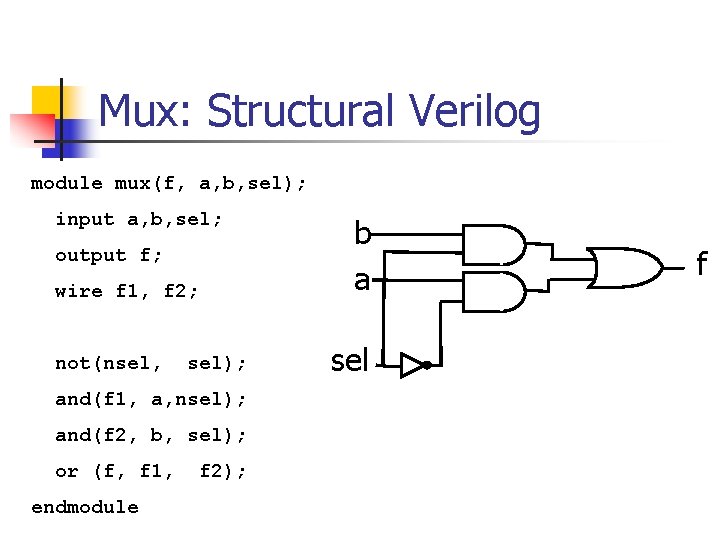
Mux: Structural Verilog module mux(f, a, b, sel); input a, b, sel; output f; wire f 1, f 2; not(nsel, sel); and(f 1, a, nsel); and(f 2, b, sel); or (f, f 1, endmodule f 2); b a sel f
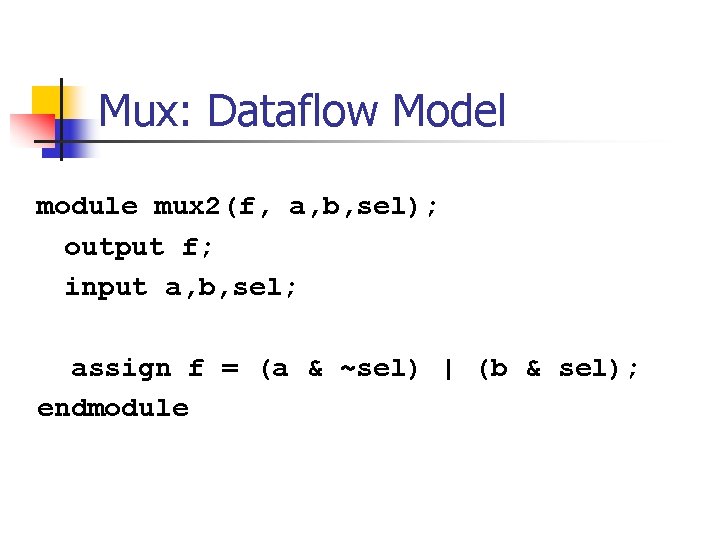
Mux: Dataflow Model module mux 2(f, a, b, sel); output f; input a, b, sel; assign f = (a & ~sel) | (b & sel); endmodule
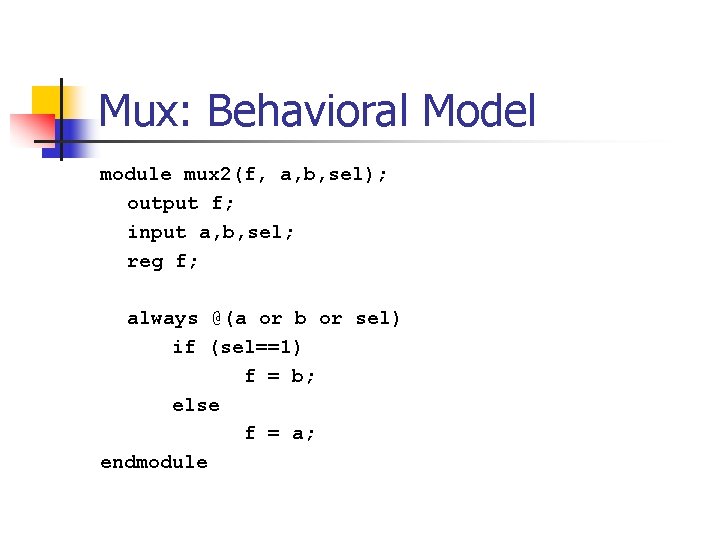
Mux: Behavioral Model module mux 2(f, a, b, sel); output f; input a, b, sel; reg f; always @(a or b or sel) if (sel==1) f = b; else f = a; endmodule
![Sign extension and addition module addersignx y s s 2 s input 3 0 Sign extension and addition module adder_sign(x, y, s, s 2 s); input [3: 0]](https://slidetodoc.com/presentation_image_h/0112129b0d47fa0d262449376b133d3c/image-17.jpg)
Sign extension and addition module adder_sign(x, y, s, s 2 s); input [3: 0] x, y; output [7: 0] s, ss; assign s = x + y, ss = {{4{x[3]}}, x}+{{4{y[3]}, y} endmodule x = 0011, y = 1101 s = 0011 + 1101 = 00010000 ss = 0011 + 1101 = 00000011 + 11111101= = 0000
![Demux Example 2 to4 demultiplexer with active low enable a b z3 z2 z1 Demux Example 2 -to-4 demultiplexer with active low enable a b z[3] z[2] z[1]](https://slidetodoc.com/presentation_image_h/0112129b0d47fa0d262449376b133d3c/image-18.jpg)
Demux Example 2 -to-4 demultiplexer with active low enable a b z[3] z[2] z[1] z[0] 0 x x 1 1 1 0 0 1 1 1 0 1 1 0 1 1 1
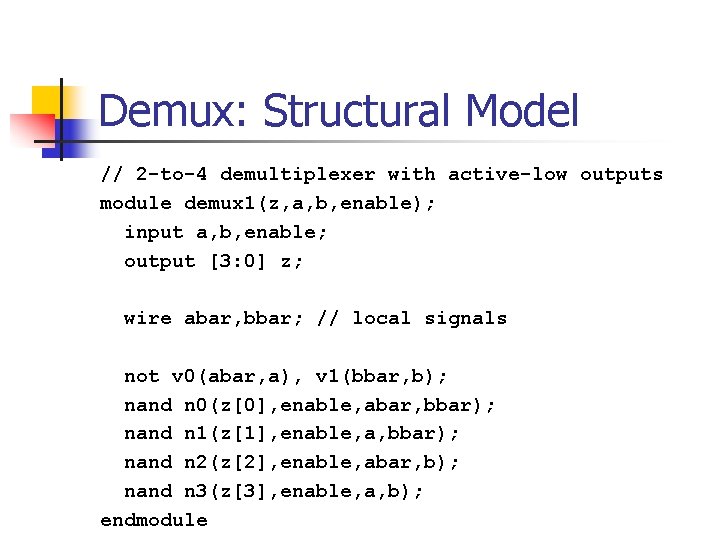
Demux: Structural Model // 2 -to-4 demultiplexer with active-low outputs module demux 1(z, a, b, enable); input a, b, enable; output [3: 0] z; wire abar, bbar; // local signals not v 0(abar, a), v 1(bbar, b); nand n 0(z[0], enable, abar, bbar); nand n 1(z[1], enable, a, bbar); nand n 2(z[2], enable, abar, b); nand n 3(z[3], enable, a, b); endmodule
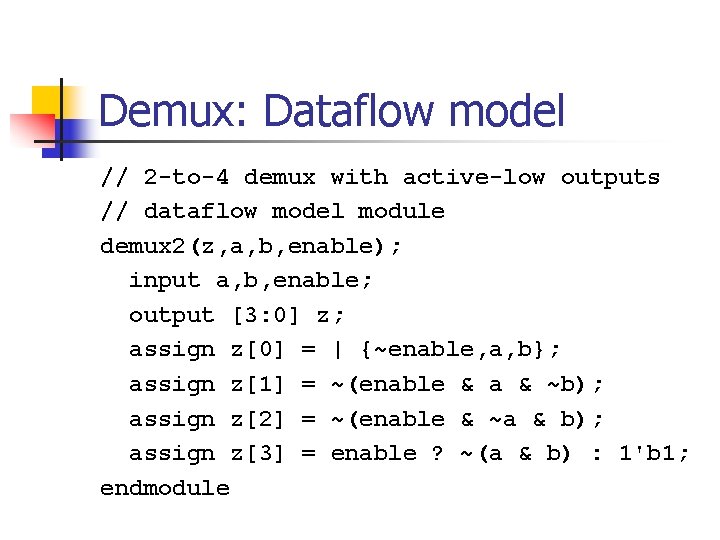
Demux: Dataflow model // 2 -to-4 demux with active-low outputs // dataflow model module demux 2(z, a, b, enable); input a, b, enable; output [3: 0] z; assign z[0] = | {~enable, a, b}; assign z[1] = ~(enable & a & ~b); assign z[2] = ~(enable & ~a & b); assign z[3] = enable ? ~(a & b) : 1'b 1; endmodule
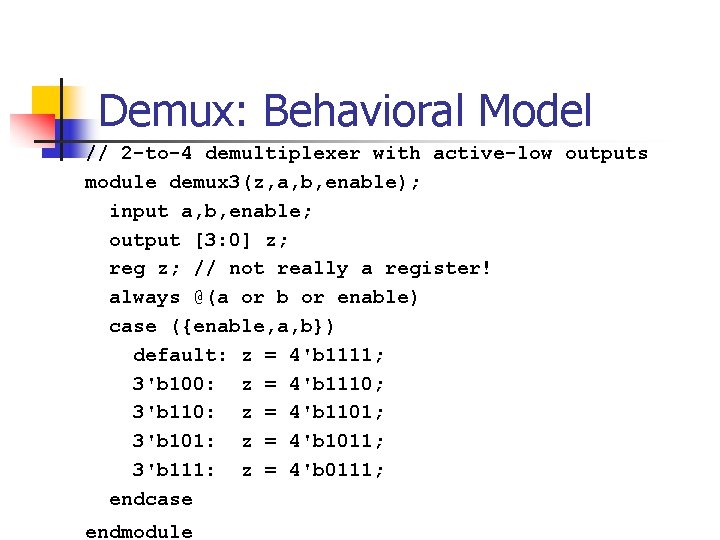
Demux: Behavioral Model // 2 -to-4 demultiplexer with active-low outputs module demux 3(z, a, b, enable); input a, b, enable; output [3: 0] z; reg z; // not really a register! always @(a or b or enable) case ({enable, a, b}) default: z = 4'b 1111; 3'b 100: z = 4'b 1110; 3'b 110: z = 4'b 1101; 3'b 101: z = 4'b 1011; 3'b 111: z = 4'b 0111; endcase endmodule
Cpsc 321
Cpsc 321
3 bus architecture
Diff between computer organization and architecture
Design of basic computer
Edel 321
Responsibilities
Csi 321
132 213
Apokalypsis 321
Oam 321
"raj chaudhury"
Ics-321
Ics 321
Cbp entry type 86
Eccentric leveling lugs
321 feedback
Acuerdo 0321 de 2011
Project scheduling and tracking in software engineering
3-2-1 dough refers to what ratio
Ipx-321
321 riq