Exceptions Throwing Catching Defining Outcomes Find the exception
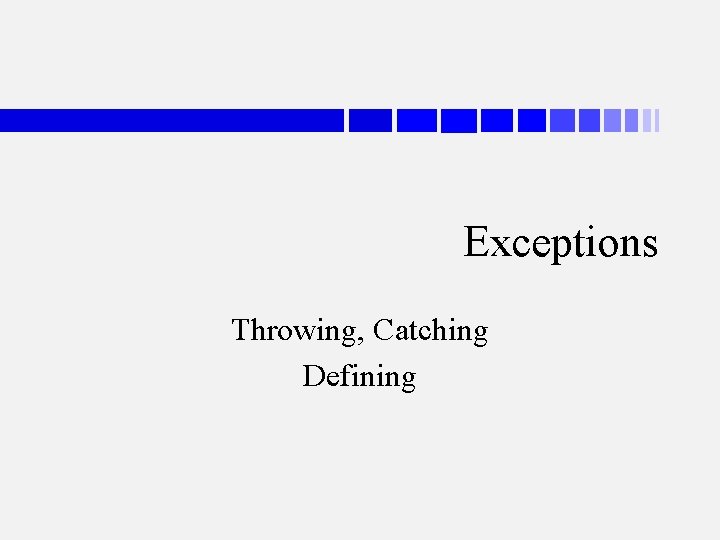
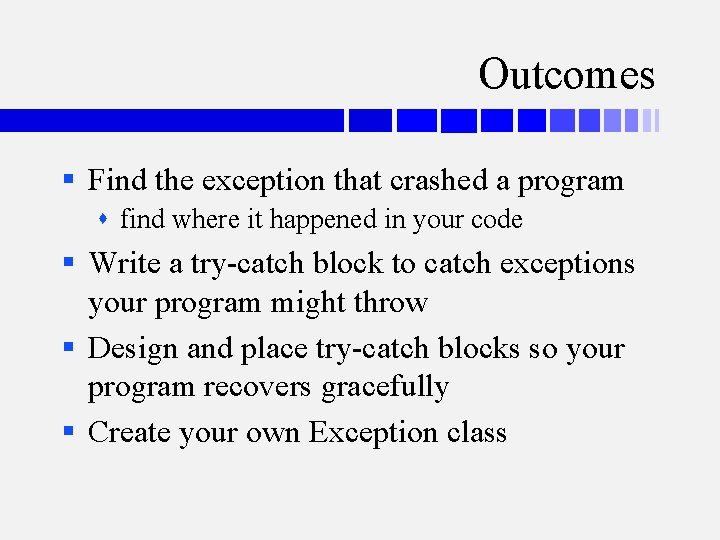
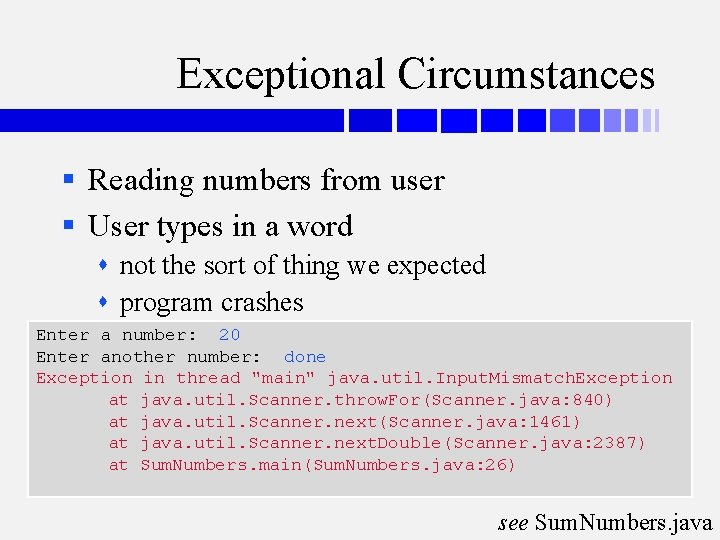
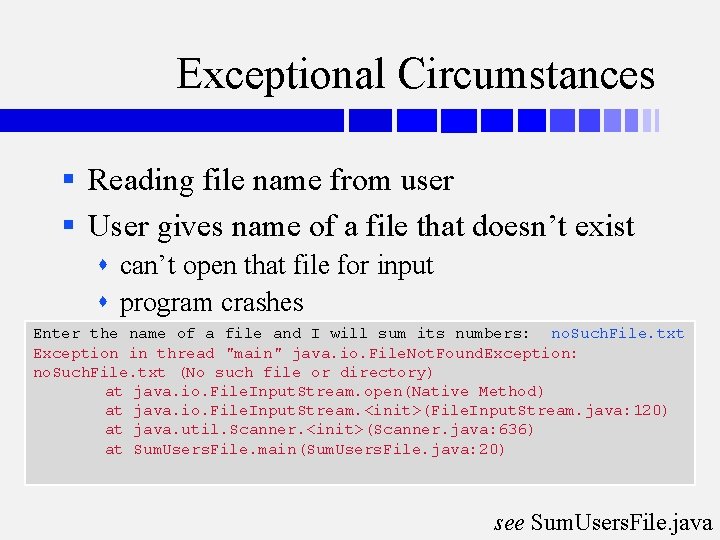
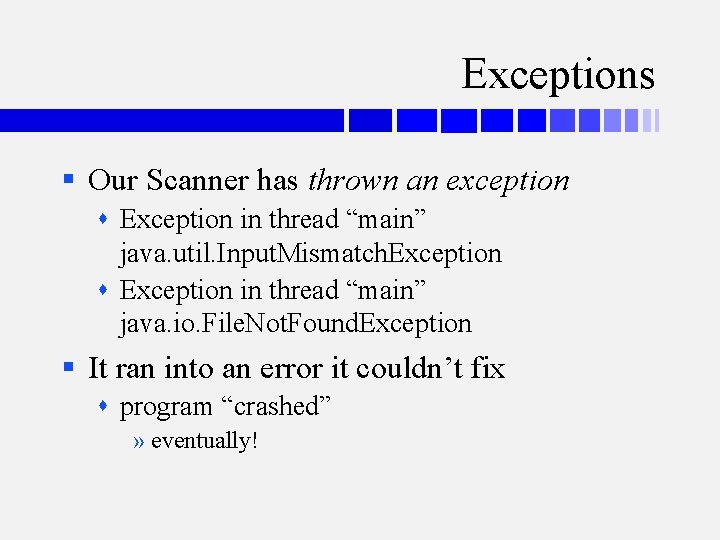
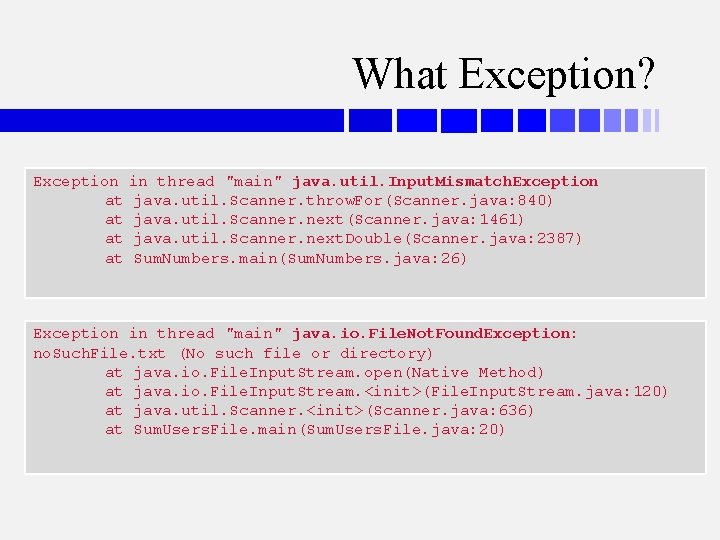
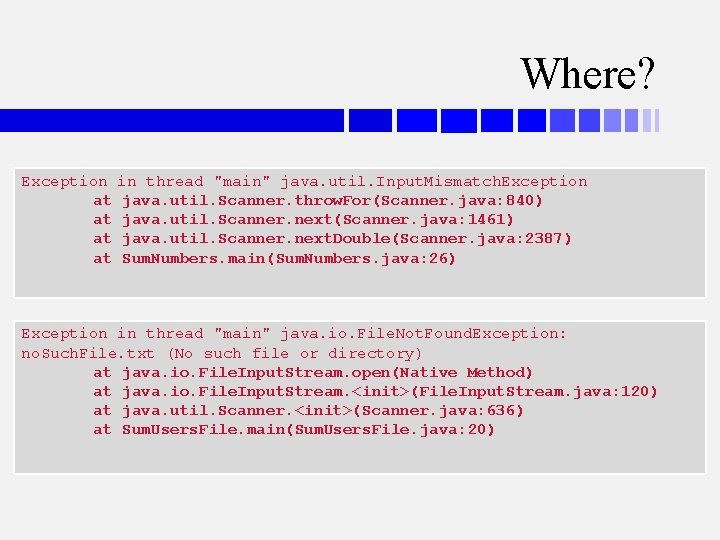
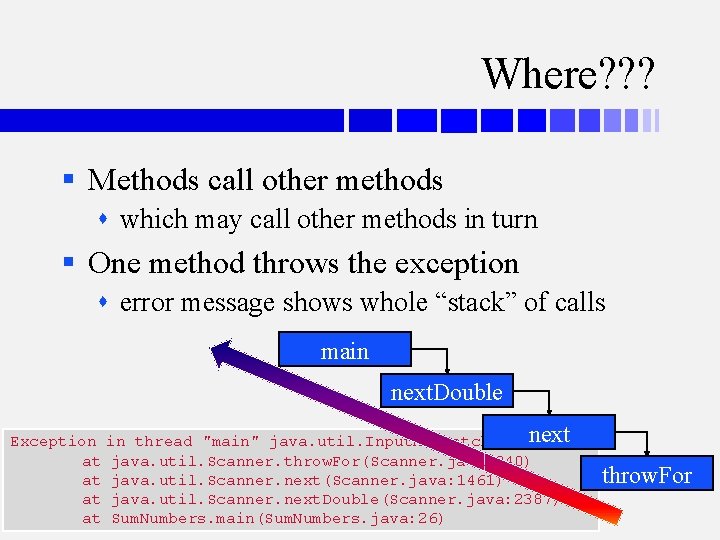
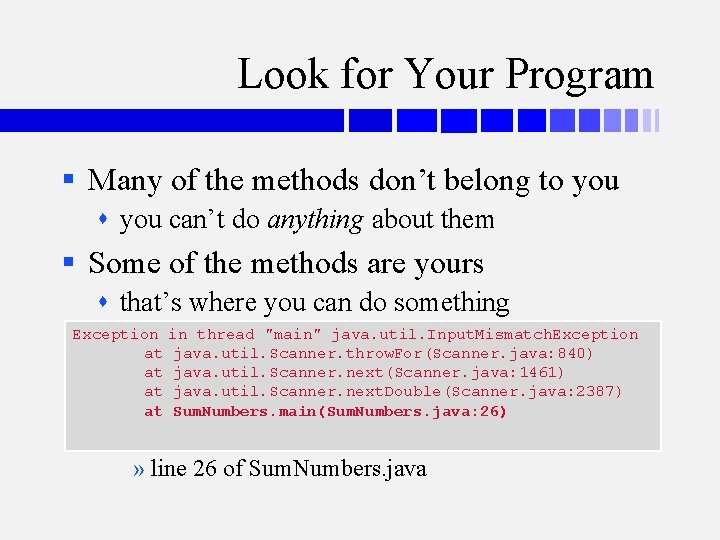
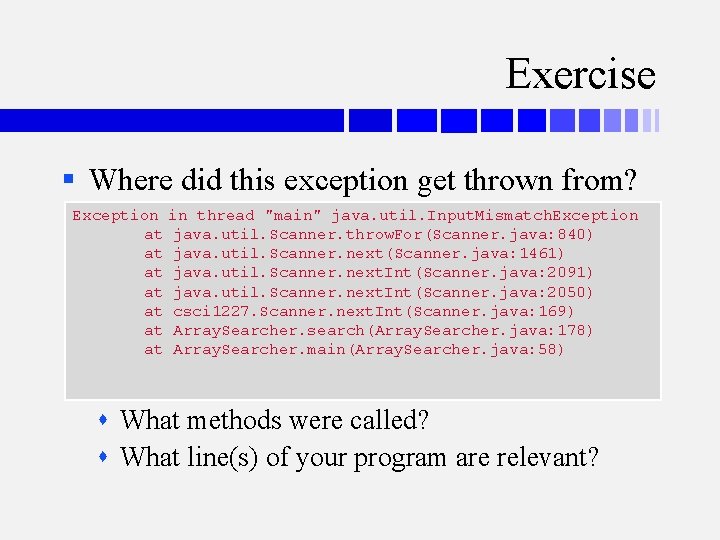
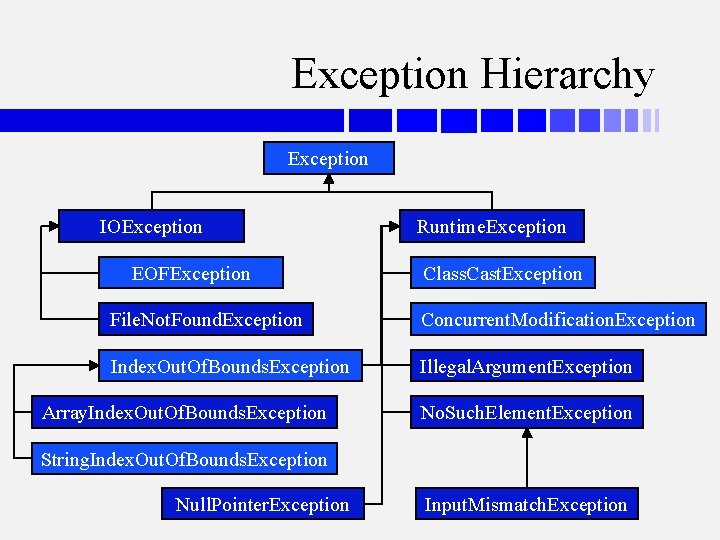
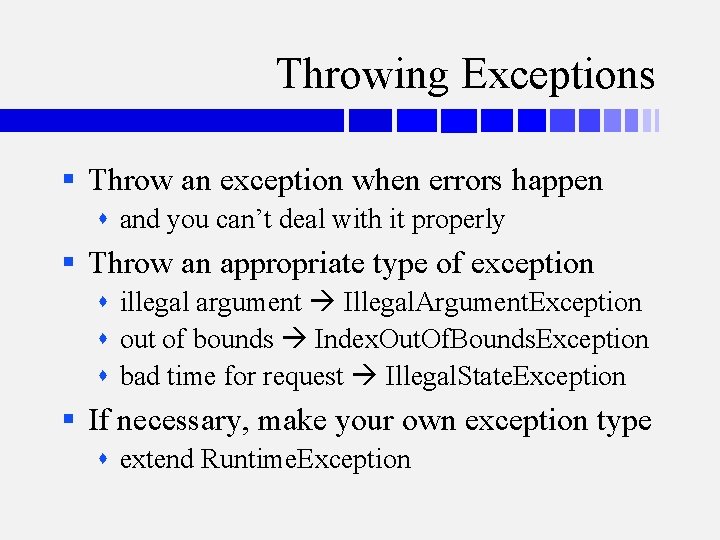
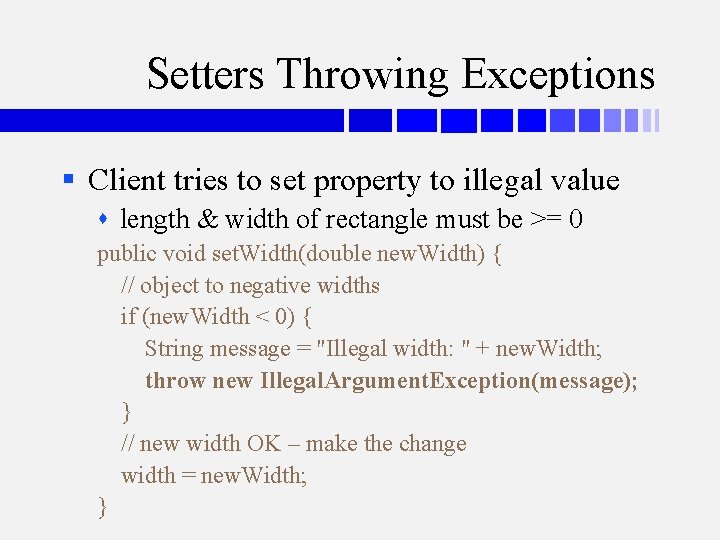
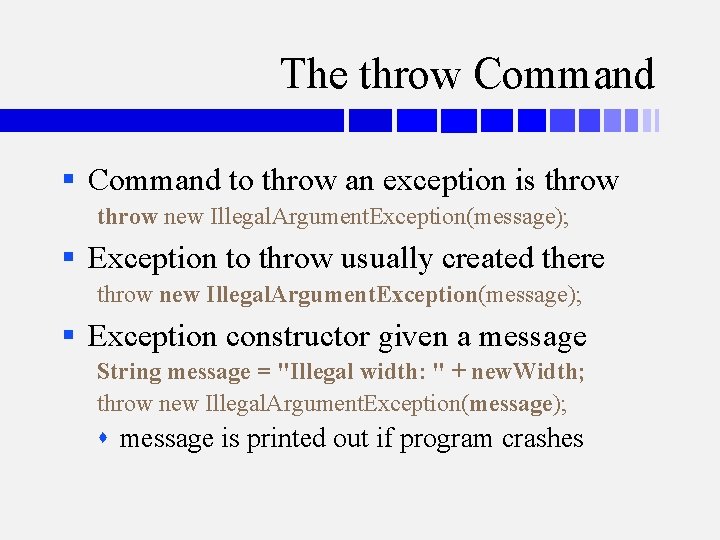
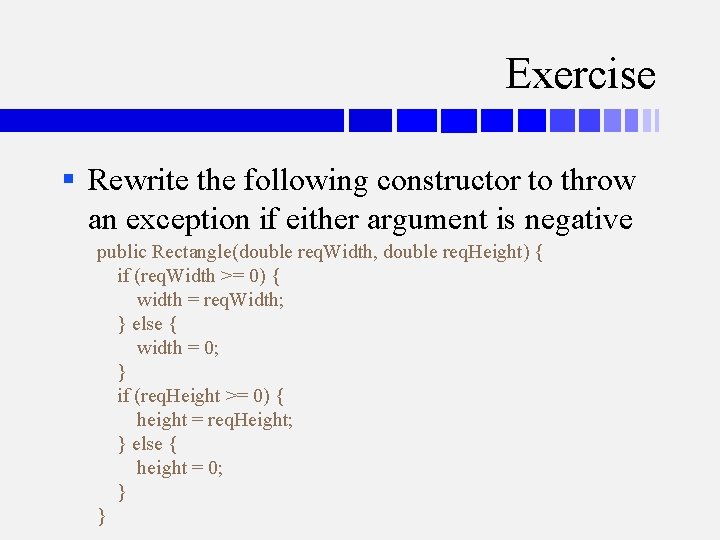
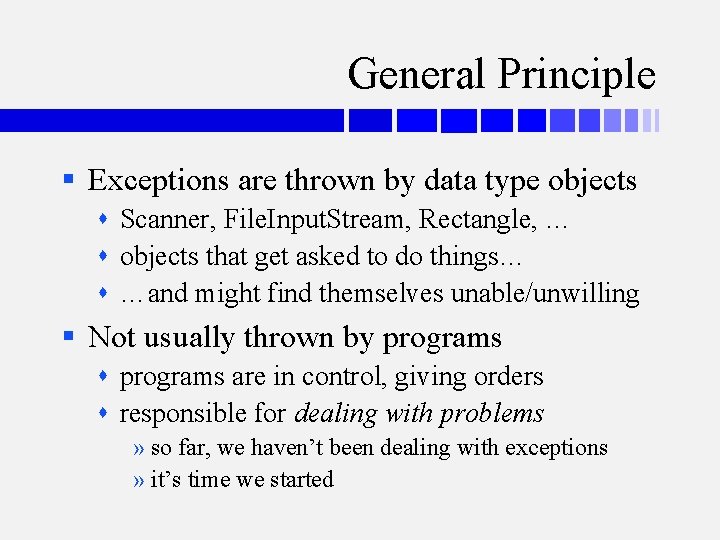
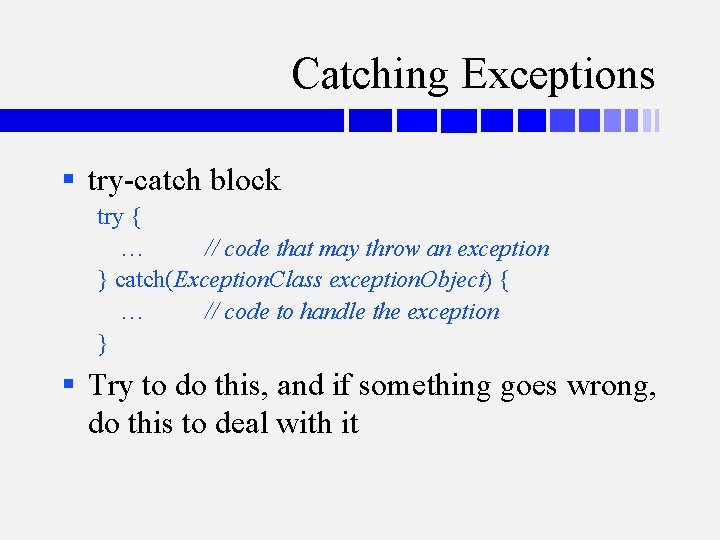
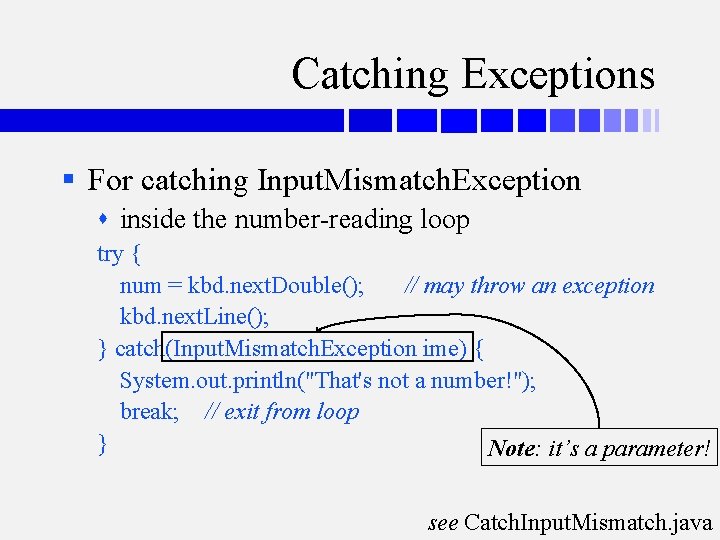
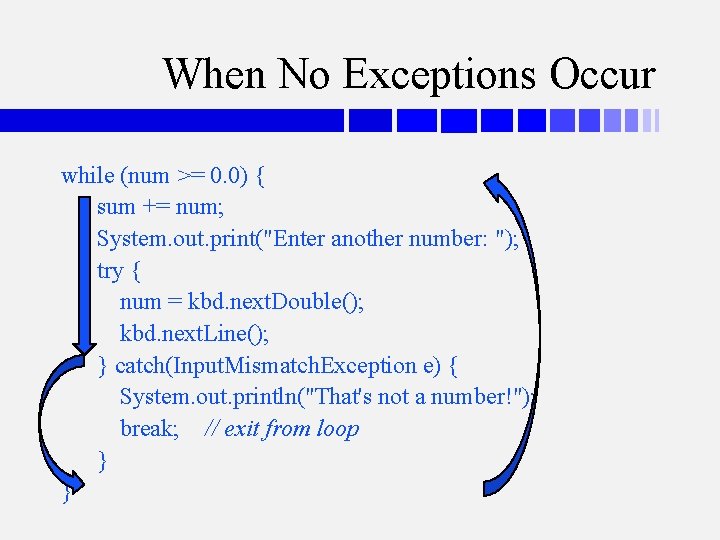
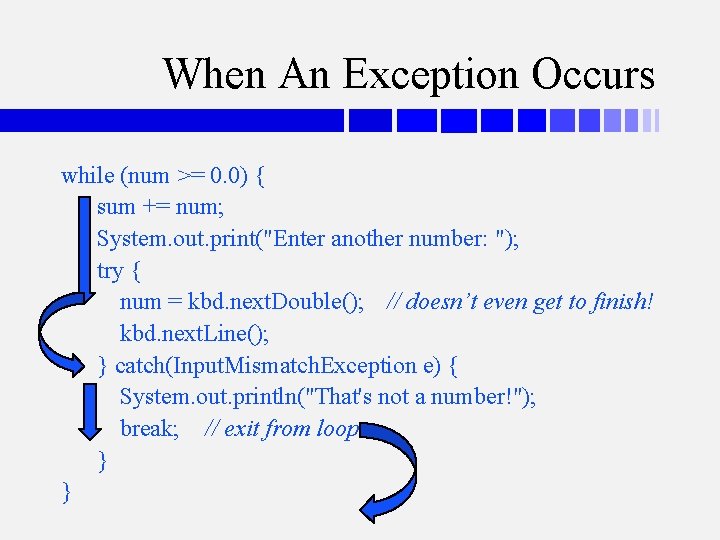
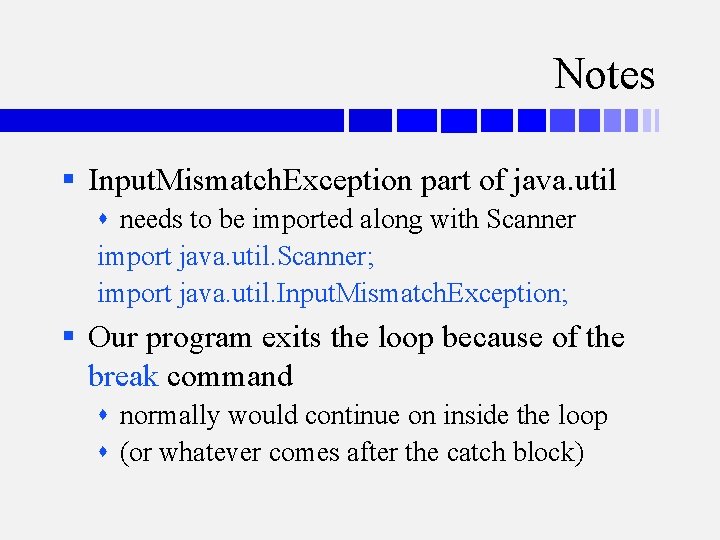
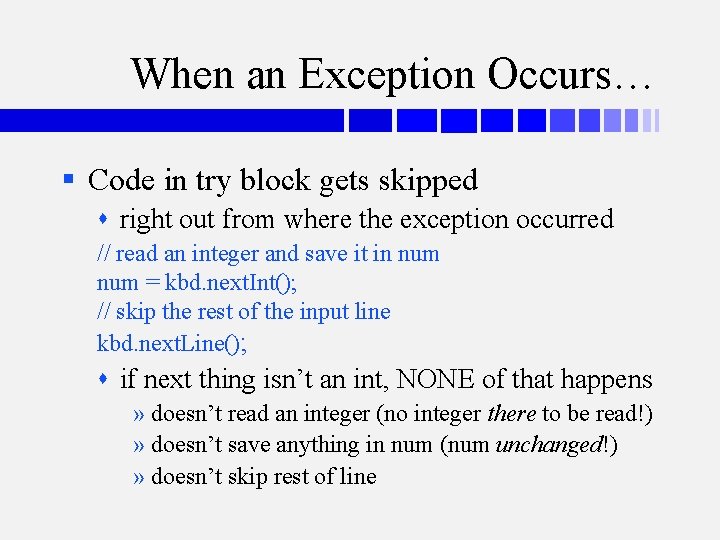
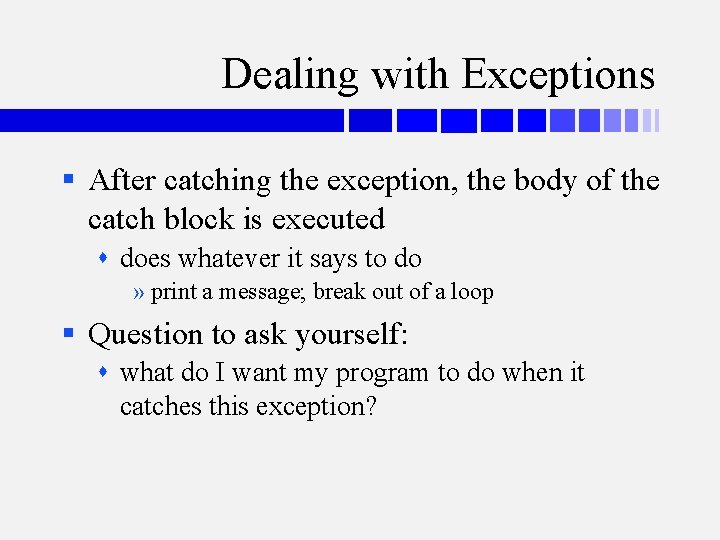
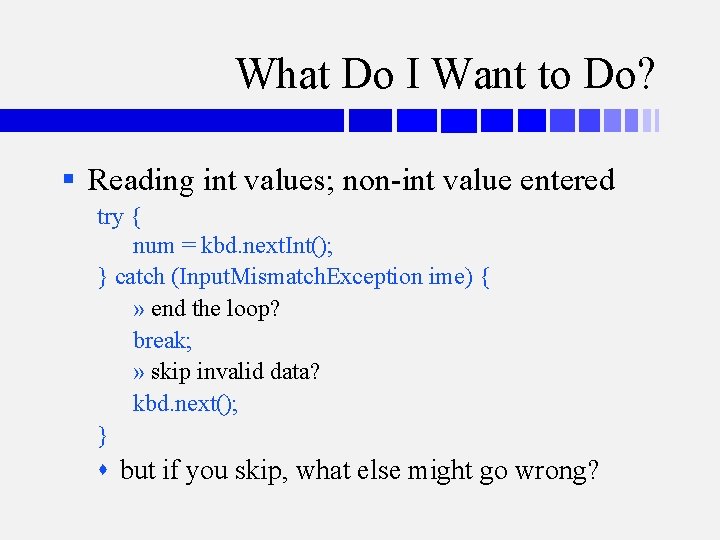
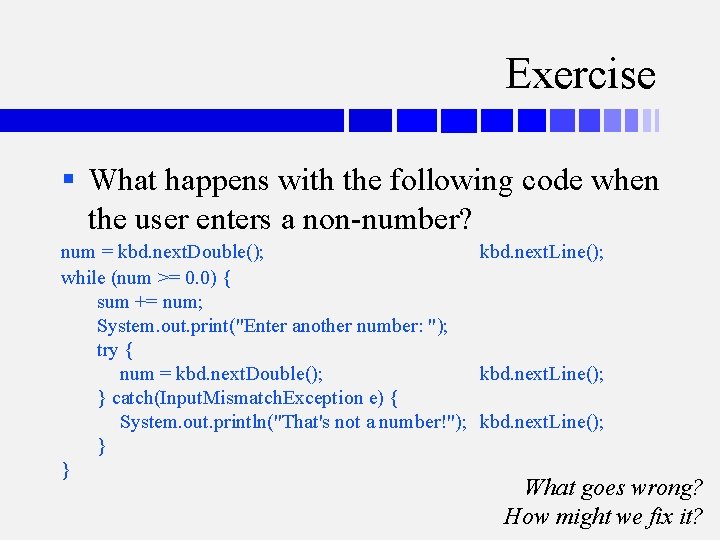
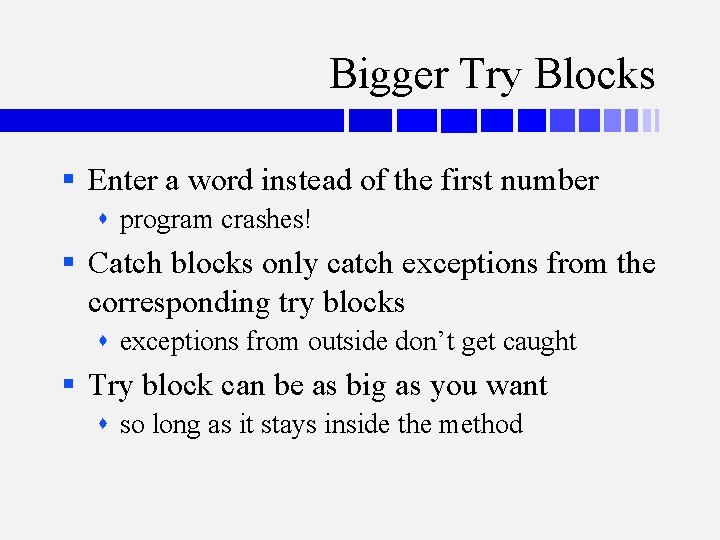
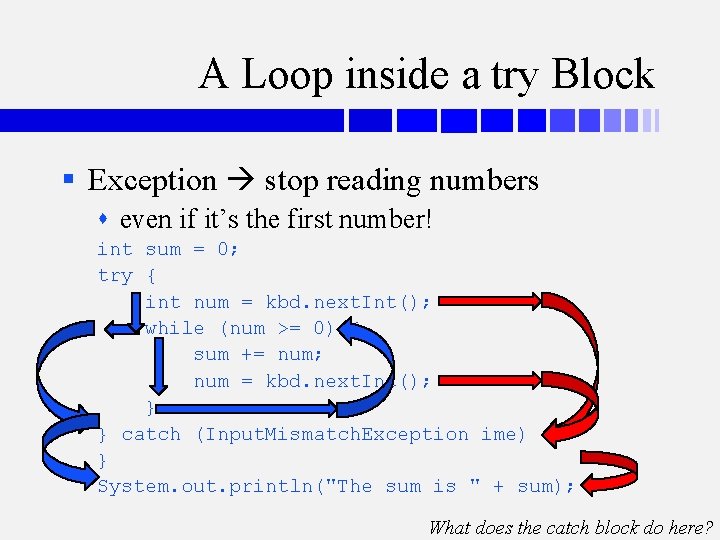
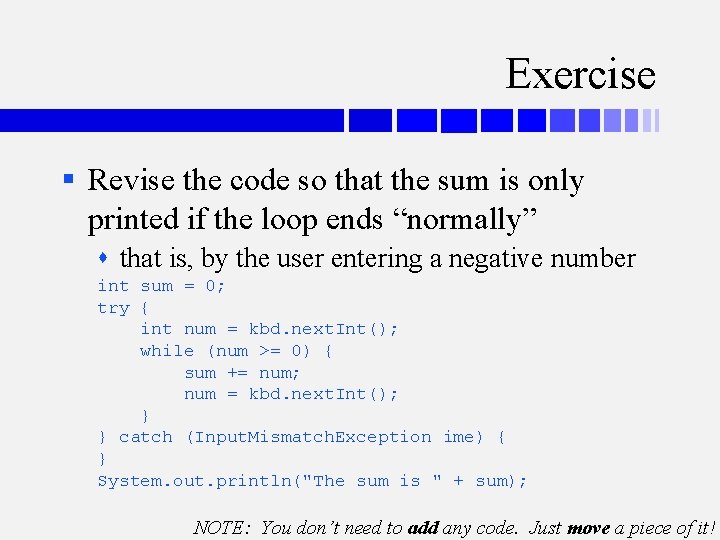
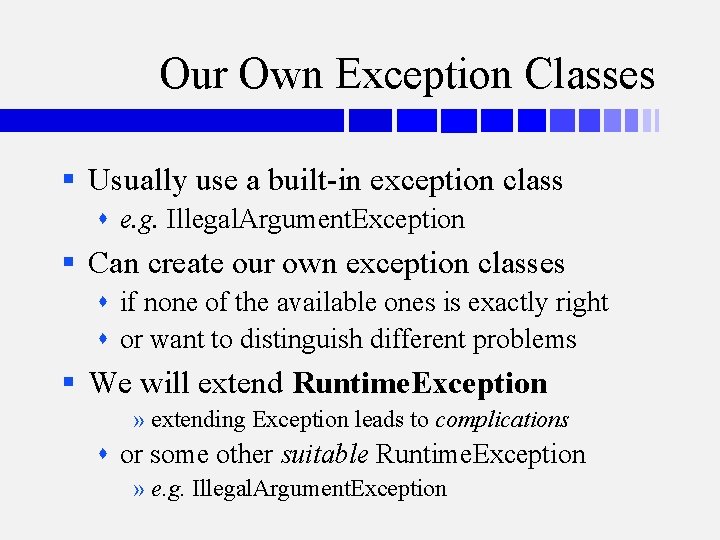
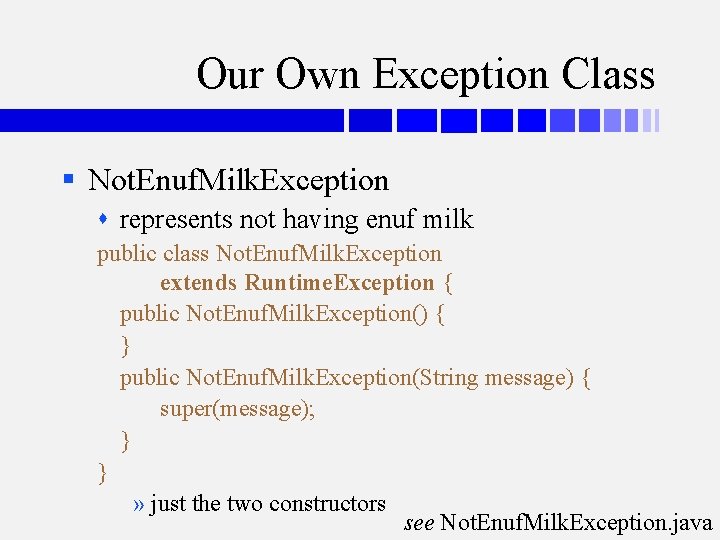
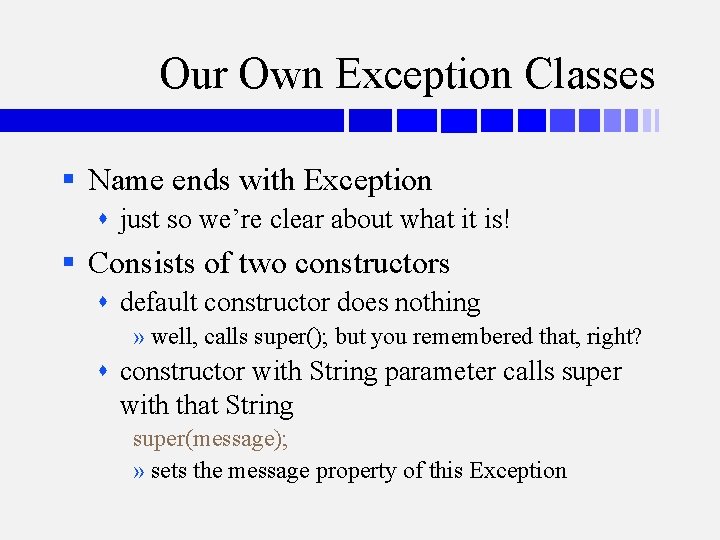
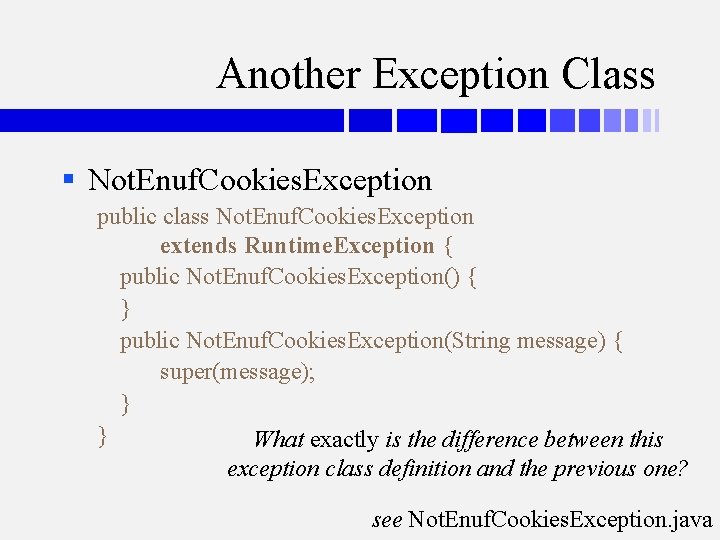
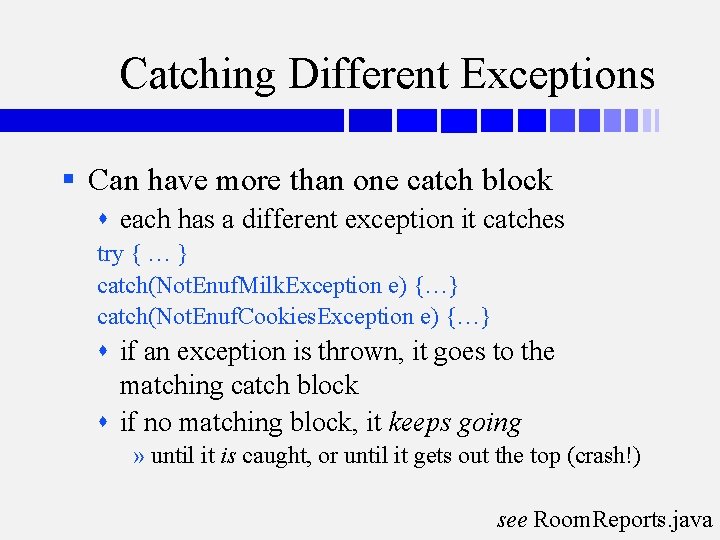
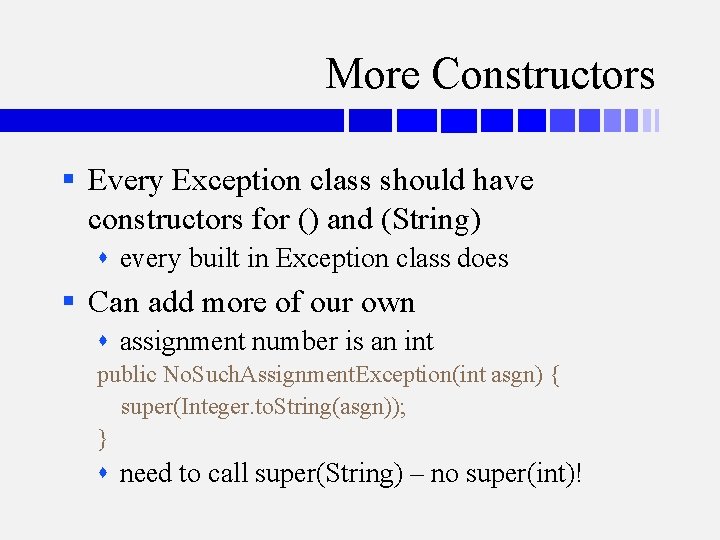
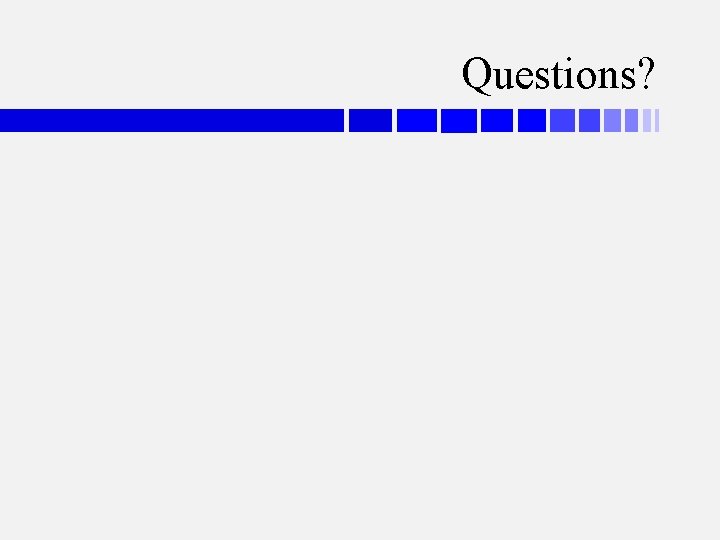
- Slides: 35
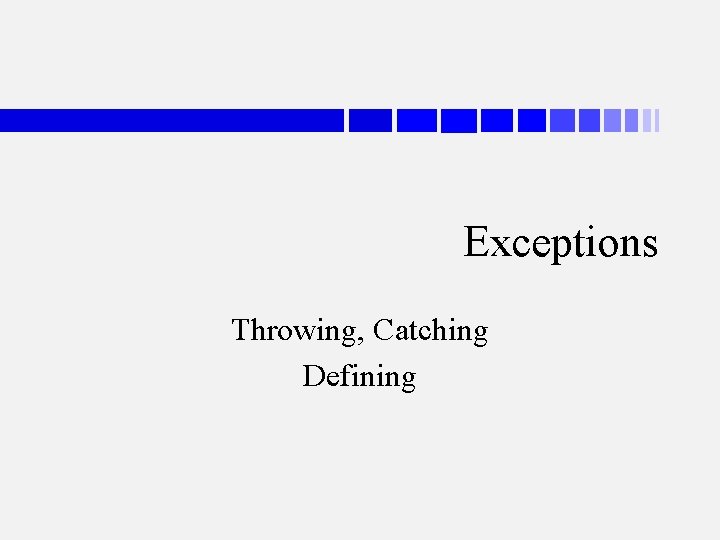
Exceptions Throwing, Catching Defining
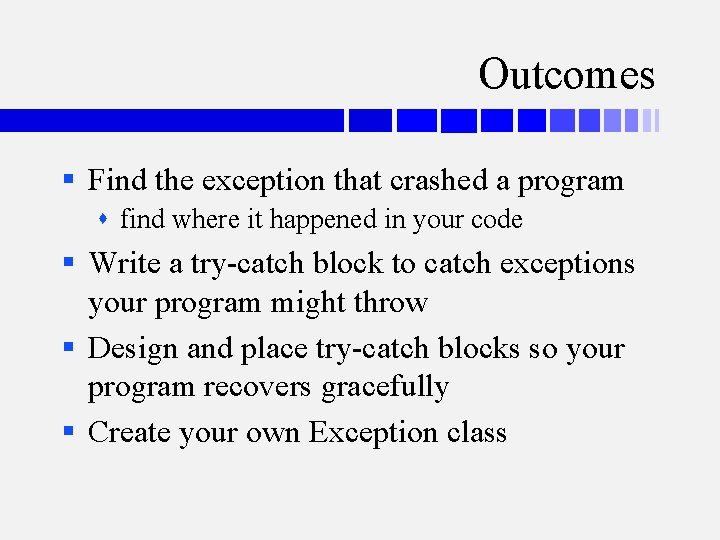
Outcomes § Find the exception that crashed a program find where it happened in your code § Write a try-catch block to catch exceptions your program might throw § Design and place try-catch blocks so your program recovers gracefully § Create your own Exception class
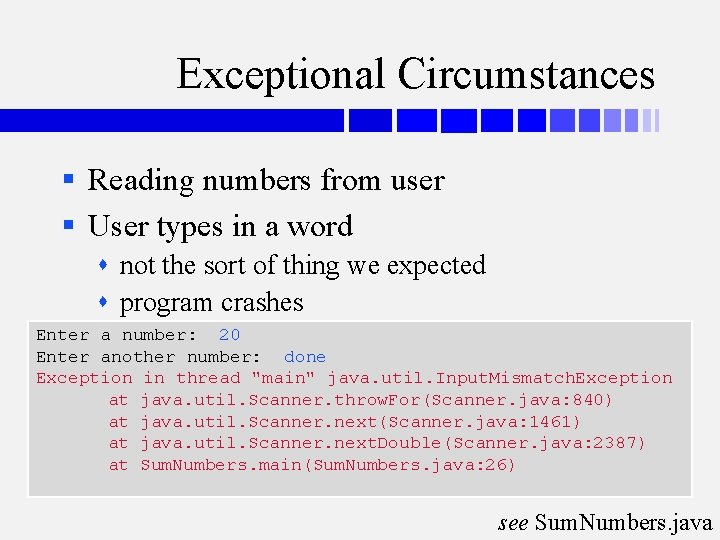
Exceptional Circumstances § Reading numbers from user § User types in a word not the sort of thing we expected program crashes Enter a number: 20 Enter another number: done Exception in thread "main" java. util. Input. Mismatch. Exception at java. util. Scanner. throw. For(Scanner. java: 840) at java. util. Scanner. next(Scanner. java: 1461) at java. util. Scanner. next. Double(Scanner. java: 2387) at Sum. Numbers. main(Sum. Numbers. java: 26) see Sum. Numbers. java
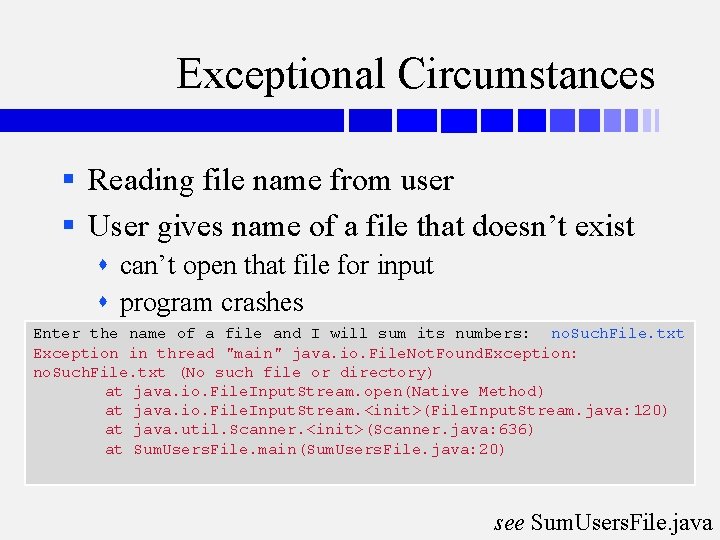
Exceptional Circumstances § Reading file name from user § User gives name of a file that doesn’t exist can’t open that file for input program crashes Enter the name of a file and I will sum its numbers: no. Such. File. txt Exception in thread "main" java. io. File. Not. Found. Exception: no. Such. File. txt (No such file or directory) at java. io. File. Input. Stream. open(Native Method) at java. io. File. Input. Stream. <init>(File. Input. Stream. java: 120) at java. util. Scanner. <init>(Scanner. java: 636) at Sum. Users. File. main(Sum. Users. File. java: 20) see Sum. Users. File. java
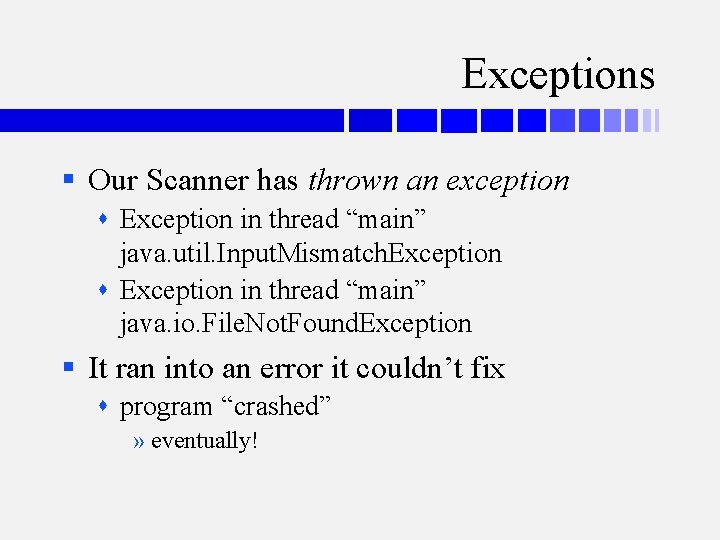
Exceptions § Our Scanner has thrown an exception Exception in thread “main” java. util. Input. Mismatch. Exception in thread “main” java. io. File. Not. Found. Exception § It ran into an error it couldn’t fix program “crashed” » eventually!
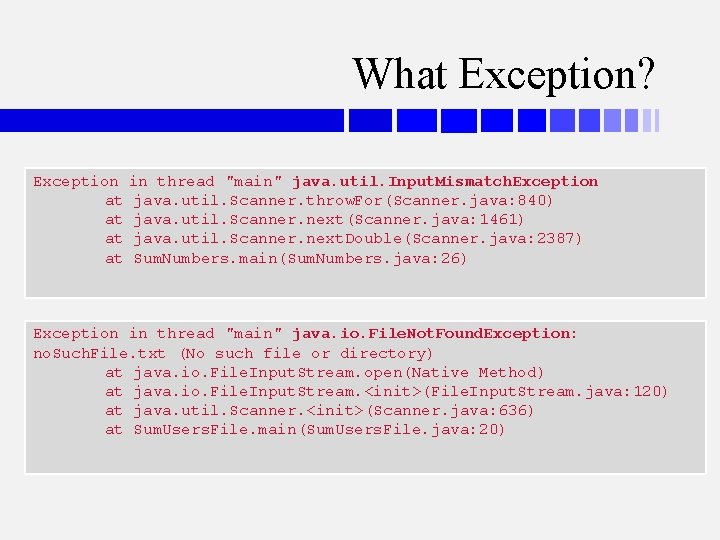
What Exception? Exception at at in thread "main" java. util. Input. Mismatch. Exception java. util. Scanner. throw. For(Scanner. java: 840) java. util. Scanner. next(Scanner. java: 1461) java. util. Scanner. next. Double(Scanner. java: 2387) Sum. Numbers. main(Sum. Numbers. java: 26) Exception in thread "main" java. io. File. Not. Found. Exception: no. Such. File. txt (No such file or directory) at java. io. File. Input. Stream. open(Native Method) at java. io. File. Input. Stream. <init>(File. Input. Stream. java: 120) at java. util. Scanner. <init>(Scanner. java: 636) at Sum. Users. File. main(Sum. Users. File. java: 20)
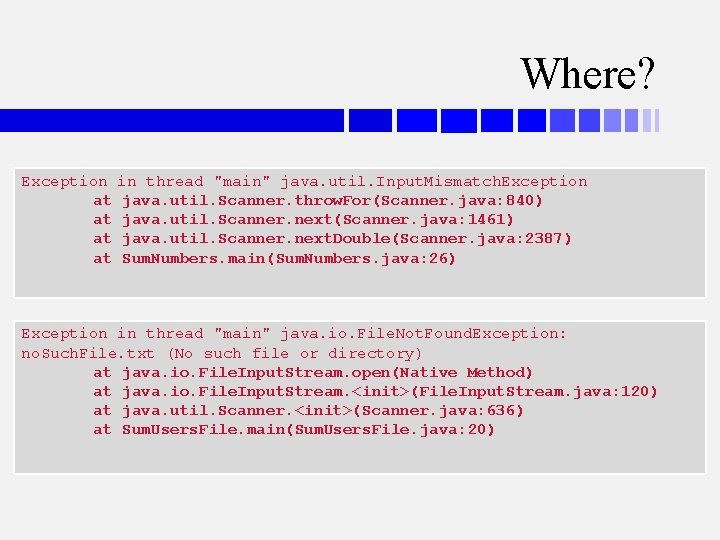
Where? Exception in thread "main" java. util. Input. Mismatch. Exception at java. util. Scanner. throw. For(Scanner. java: 840) at java. util. Scanner. next(Scanner. java: 1461) at java. util. Scanner. next. Double(Scanner. java: 2387) at Sum. Numbers. main(Sum. Numbers. java: 26) Exception in thread "main" java. io. File. Not. Found. Exception: no. Such. File. txt (No such file or directory) at java. io. File. Input. Stream. open(Native Method) at java. io. File. Input. Stream. <init>(File. Input. Stream. java: 120) at java. util. Scanner. <init>(Scanner. java: 636) at Sum. Users. File. main(Sum. Users. File. java: 20)
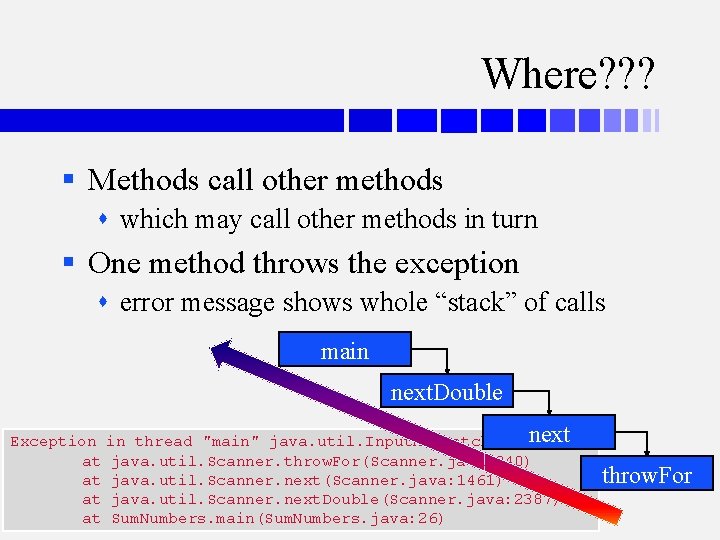
Where? ? ? § Methods call other methods which may call other methods in turn § One method throws the exception error message shows whole “stack” of calls main next. Double Exception at at next in thread "main" java. util. Input. Mismatch. Exception java. util. Scanner. throw. For(Scanner. java: 840) java. util. Scanner. next(Scanner. java: 1461) java. util. Scanner. next. Double(Scanner. java: 2387) Sum. Numbers. main(Sum. Numbers. java: 26) throw. For
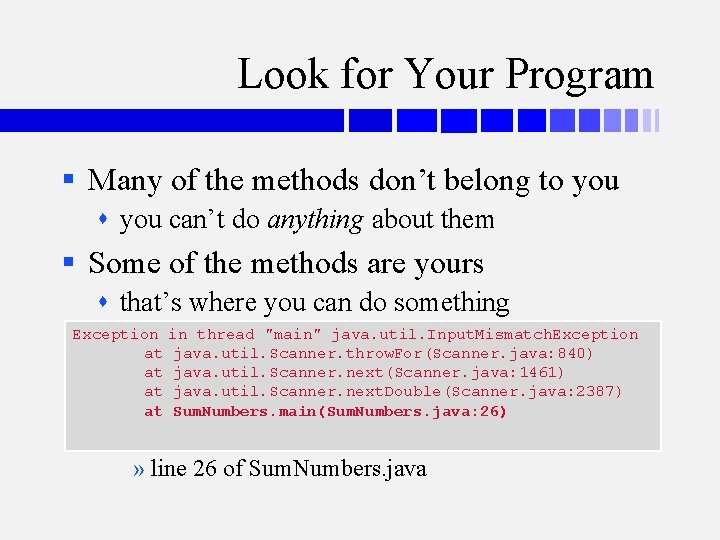
Look for Your Program § Many of the methods don’t belong to you can’t do anything about them § Some of the methods are yours that’s where you can do something Exception at at in thread "main" java. util. Input. Mismatch. Exception java. util. Scanner. throw. For(Scanner. java: 840) java. util. Scanner. next(Scanner. java: 1461) java. util. Scanner. next. Double(Scanner. java: 2387) Sum. Numbers. main(Sum. Numbers. java: 26) » line 26 of Sum. Numbers. java
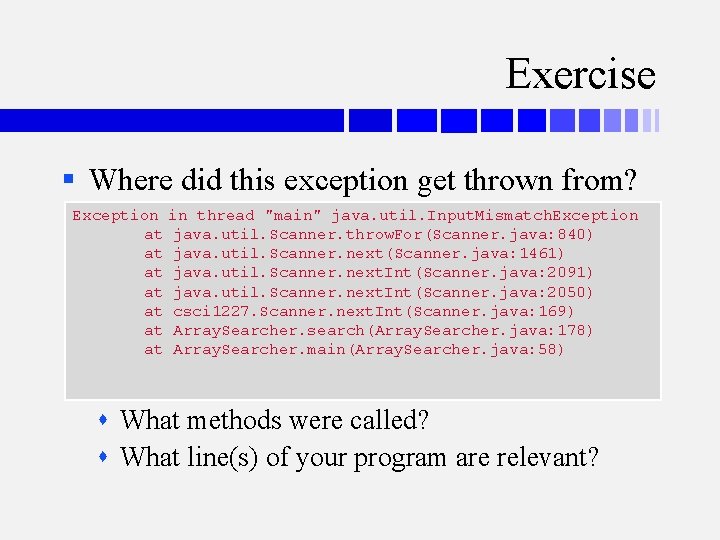
Exercise § Where did this exception get thrown from? Exception at at in thread "main" java. util. Input. Mismatch. Exception java. util. Scanner. throw. For(Scanner. java: 840) java. util. Scanner. next(Scanner. java: 1461) java. util. Scanner. next. Int(Scanner. java: 2091) java. util. Scanner. next. Int(Scanner. java: 2050) csci 1227. Scanner. next. Int(Scanner. java: 169) Array. Searcher. search(Array. Searcher. java: 178) Array. Searcher. main(Array. Searcher. java: 58) What methods were called? What line(s) of your program are relevant?
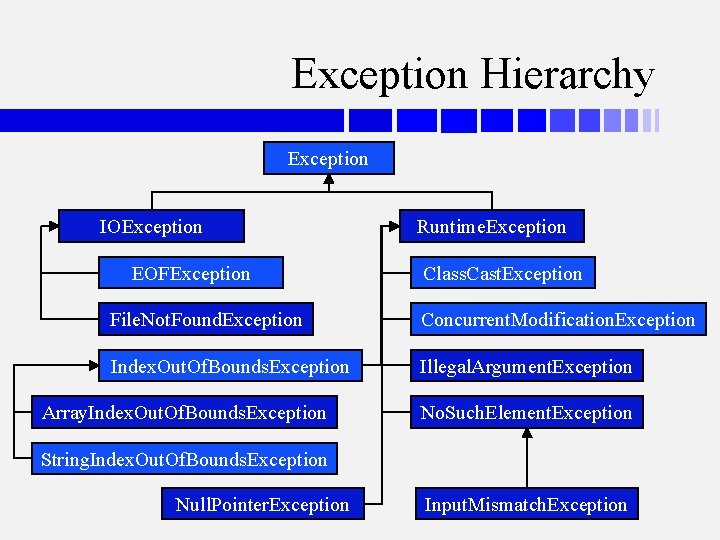
Exception Hierarchy Exception IOException EOFException Runtime. Exception Class. Cast. Exception File. Not. Found. Exception Concurrent. Modification. Exception Index. Out. Of. Bounds. Exception Illegal. Argument. Exception Array. Index. Out. Of. Bounds. Exception No. Such. Element. Exception String. Index. Out. Of. Bounds. Exception Null. Pointer. Exception Input. Mismatch. Exception
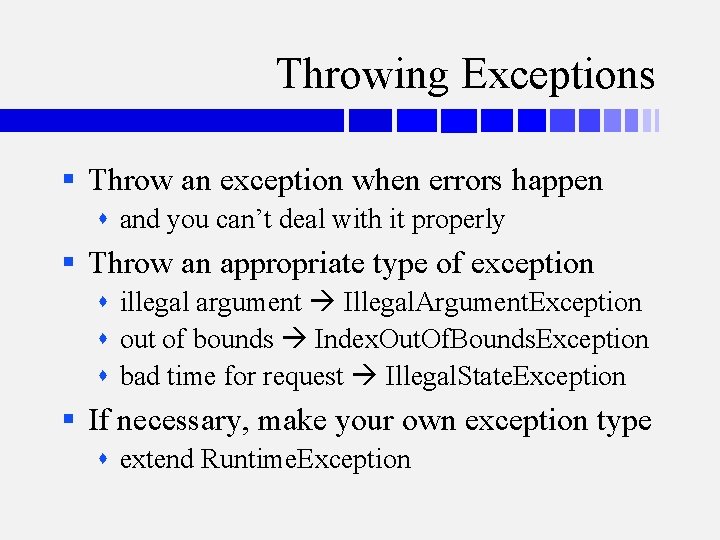
Throwing Exceptions § Throw an exception when errors happen and you can’t deal with it properly § Throw an appropriate type of exception illegal argument Illegal. Argument. Exception out of bounds Index. Out. Of. Bounds. Exception bad time for request Illegal. State. Exception § If necessary, make your own exception type extend Runtime. Exception
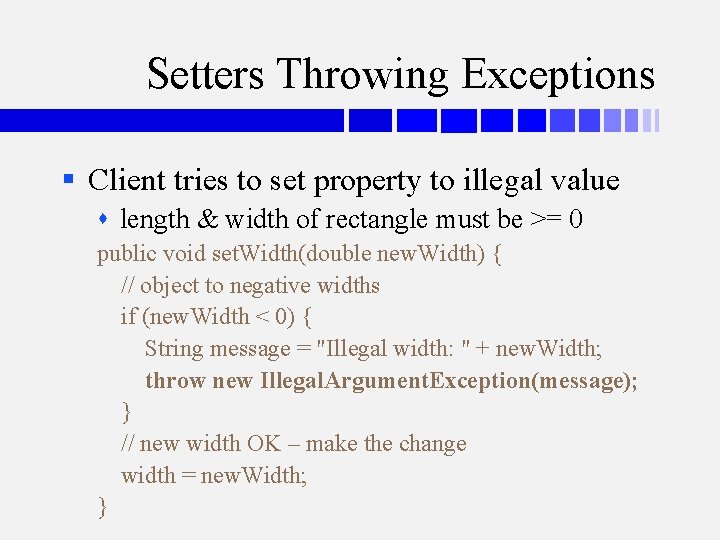
Setters Throwing Exceptions § Client tries to set property to illegal value length & width of rectangle must be >= 0 public void set. Width(double new. Width) { // object to negative widths if (new. Width < 0) { String message = "Illegal width: " + new. Width; throw new Illegal. Argument. Exception(message); } // new width OK – make the change width = new. Width; }
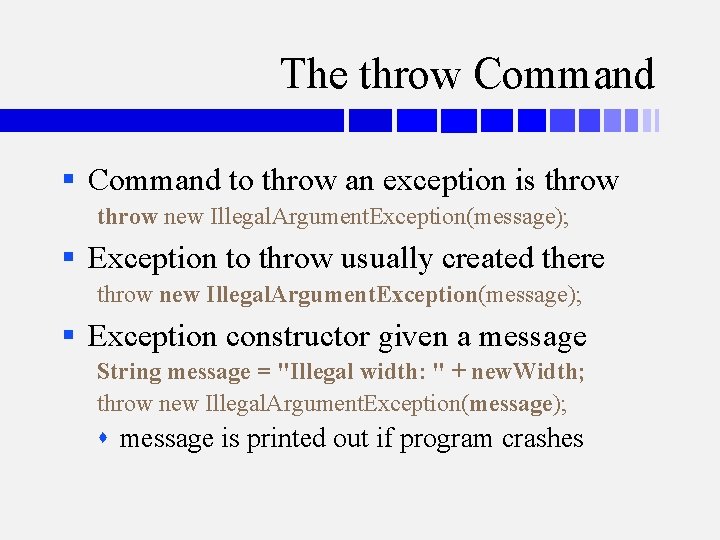
The throw Command § Command to throw an exception is throw new Illegal. Argument. Exception(message); § Exception to throw usually created there throw new Illegal. Argument. Exception(message); § Exception constructor given a message String message = "Illegal width: " + new. Width; throw new Illegal. Argument. Exception(message); message is printed out if program crashes
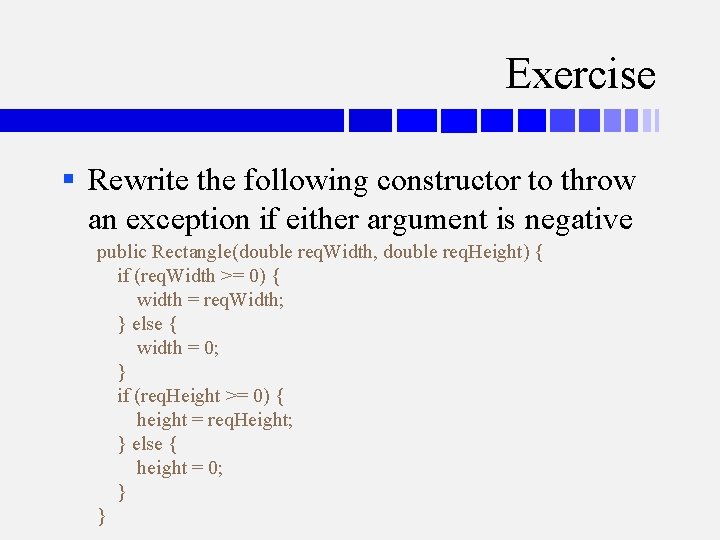
Exercise § Rewrite the following constructor to throw an exception if either argument is negative public Rectangle(double req. Width, double req. Height) { if (req. Width >= 0) { width = req. Width; } else { width = 0; } if (req. Height >= 0) { height = req. Height; } else { height = 0; } }
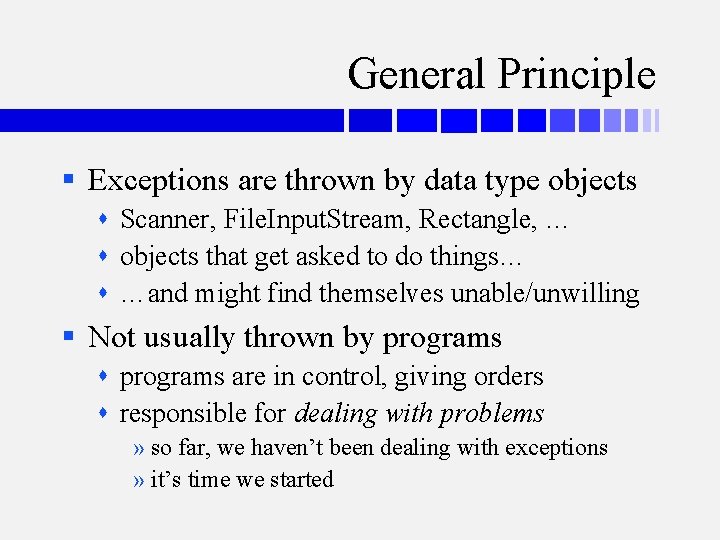
General Principle § Exceptions are thrown by data type objects Scanner, File. Input. Stream, Rectangle, … objects that get asked to do things… …and might find themselves unable/unwilling § Not usually thrown by programs are in control, giving orders responsible for dealing with problems » so far, we haven’t been dealing with exceptions » it’s time we started
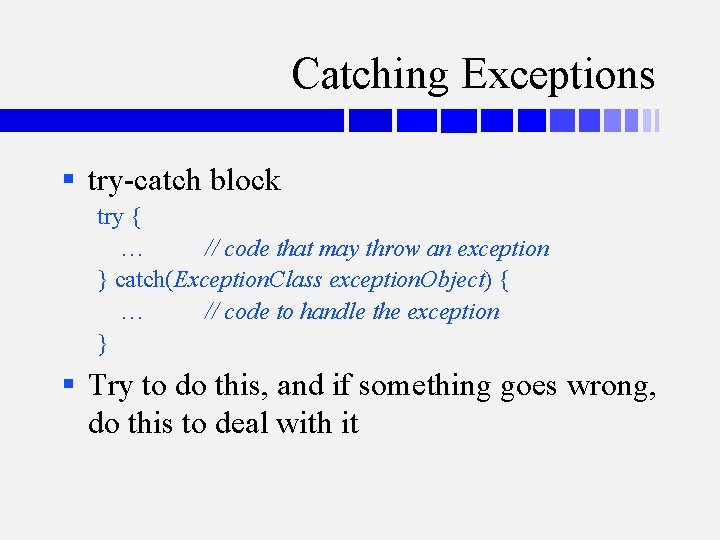
Catching Exceptions § try-catch block try { … // code that may throw an exception } catch(Exception. Class exception. Object) { … // code to handle the exception } § Try to do this, and if something goes wrong, do this to deal with it
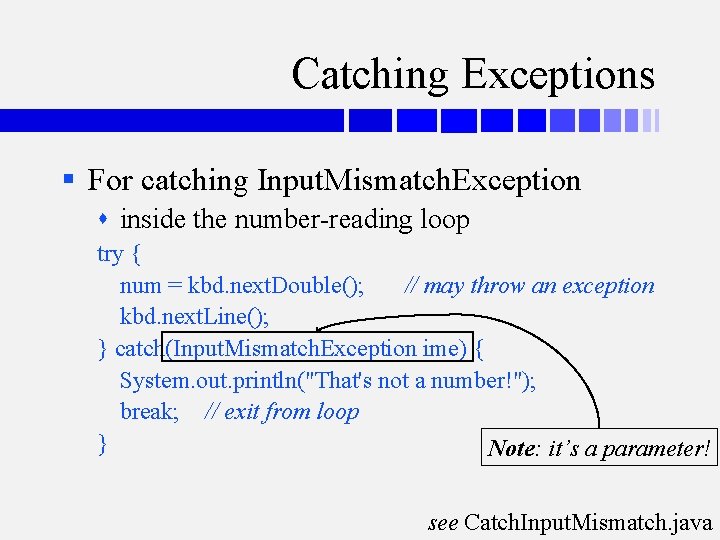
Catching Exceptions § For catching Input. Mismatch. Exception inside the number-reading loop try { num = kbd. next. Double(); // may throw an exception kbd. next. Line(); } catch(Input. Mismatch. Exception ime) { System. out. println("That's not a number!"); break; // exit from loop } Note: it’s a parameter! see Catch. Input. Mismatch. java
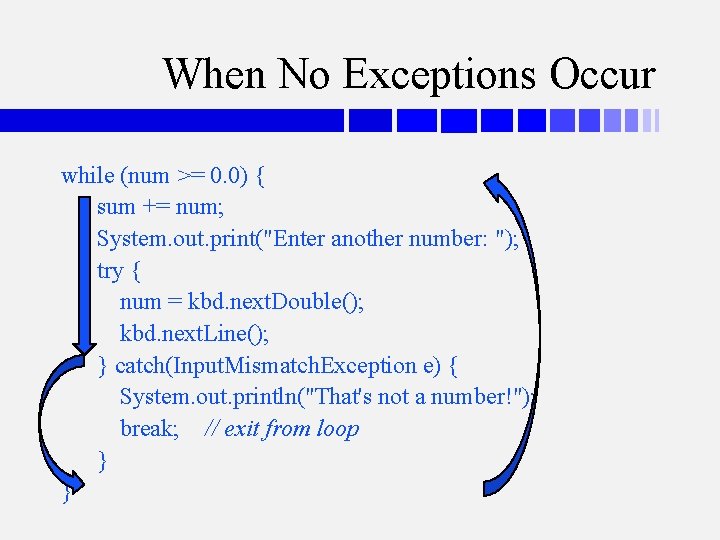
When No Exceptions Occur while (num >= 0. 0) { sum += num; System. out. print("Enter another number: "); try { num = kbd. next. Double(); kbd. next. Line(); } catch(Input. Mismatch. Exception e) { System. out. println("That's not a number!"); break; // exit from loop } }
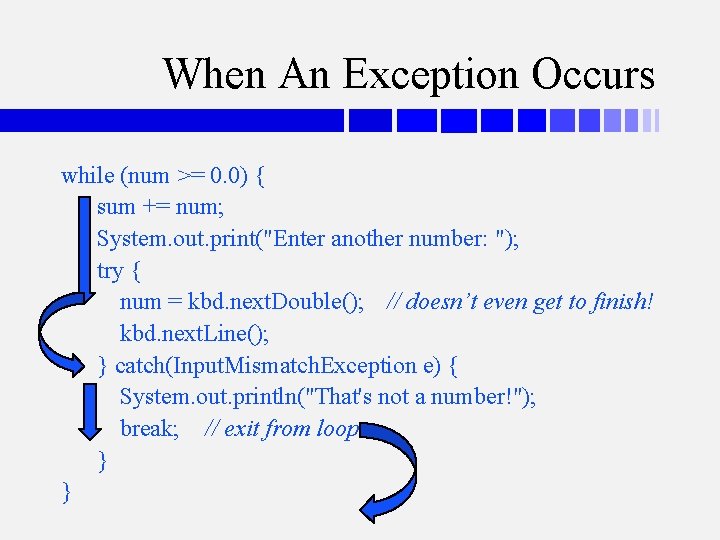
When An Exception Occurs while (num >= 0. 0) { sum += num; System. out. print("Enter another number: "); try { num = kbd. next. Double(); // doesn’t even get to finish! kbd. next. Line(); } catch(Input. Mismatch. Exception e) { System. out. println("That's not a number!"); break; // exit from loop } }
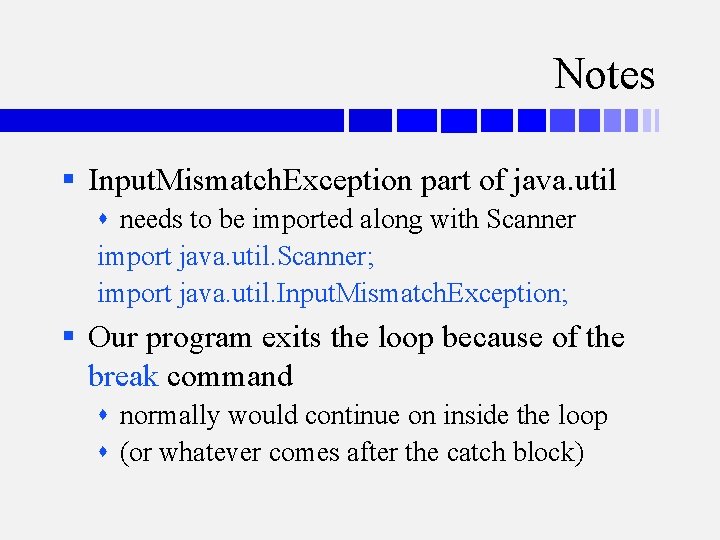
Notes § Input. Mismatch. Exception part of java. util needs to be imported along with Scanner import java. util. Scanner; import java. util. Input. Mismatch. Exception; § Our program exits the loop because of the break command normally would continue on inside the loop (or whatever comes after the catch block)
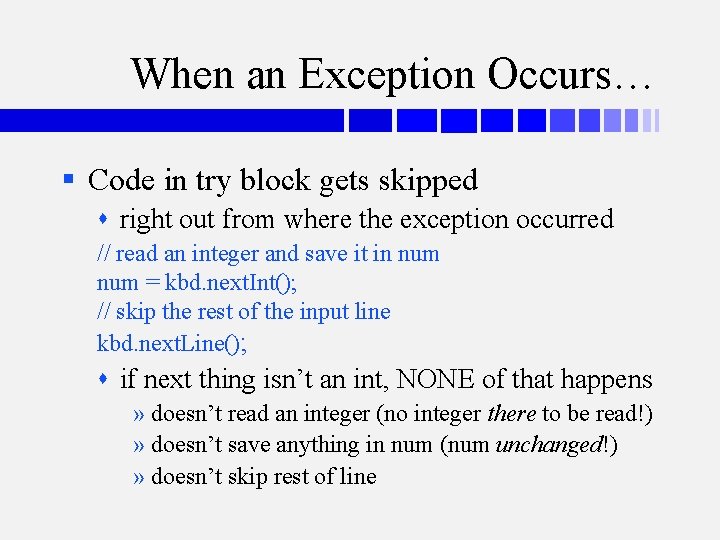
When an Exception Occurs… § Code in try block gets skipped right out from where the exception occurred // read an integer and save it in num = kbd. next. Int(); // skip the rest of the input line kbd. next. Line(); if next thing isn’t an int, NONE of that happens » doesn’t read an integer (no integer there to be read!) » doesn’t save anything in num (num unchanged!) » doesn’t skip rest of line
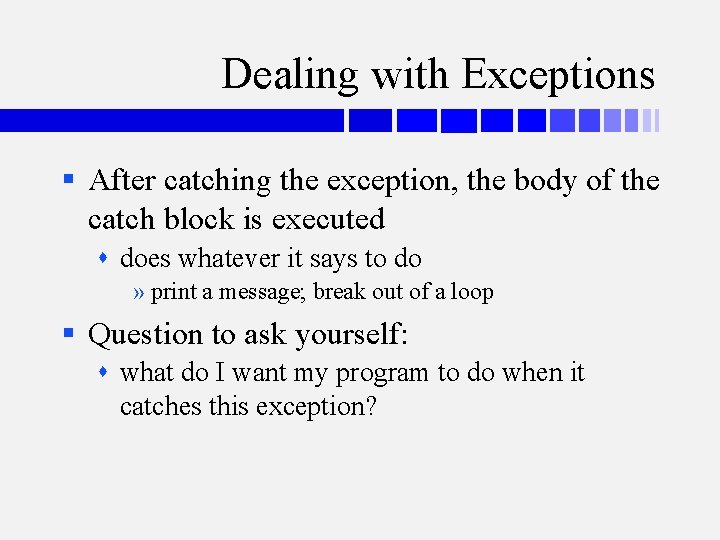
Dealing with Exceptions § After catching the exception, the body of the catch block is executed does whatever it says to do » print a message; break out of a loop § Question to ask yourself: what do I want my program to do when it catches this exception?
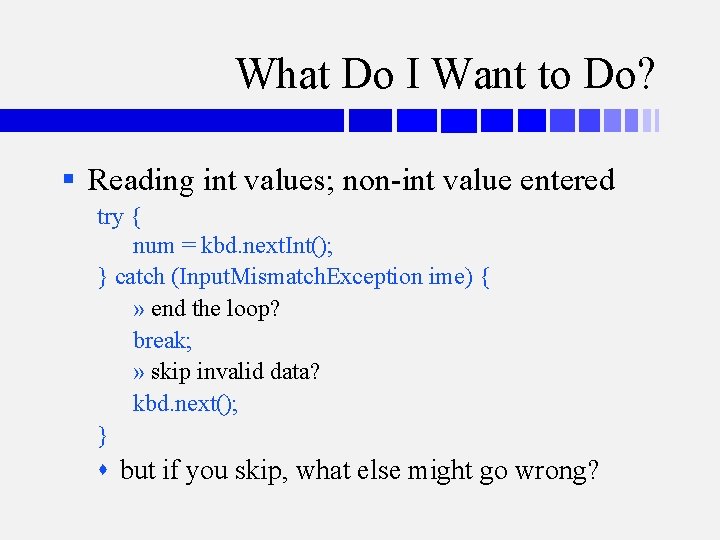
What Do I Want to Do? § Reading int values; non-int value entered try { num = kbd. next. Int(); } catch (Input. Mismatch. Exception ime) { » end the loop? break; » skip invalid data? kbd. next(); } but if you skip, what else might go wrong?
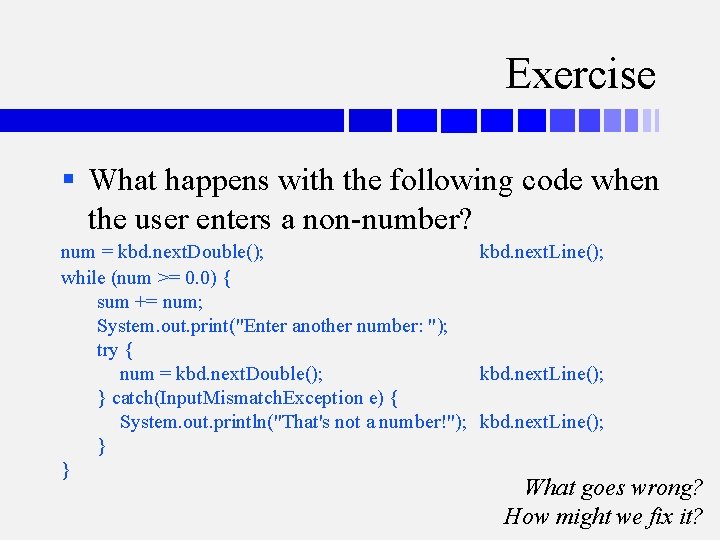
Exercise § What happens with the following code when the user enters a non-number? num = kbd. next. Double(); kbd. next. Line(); while (num >= 0. 0) { sum += num; System. out. print("Enter another number: "); try { num = kbd. next. Double(); kbd. next. Line(); } catch(Input. Mismatch. Exception e) { System. out. println("That's not a number!"); kbd. next. Line(); } } What goes wrong? How might we fix it?
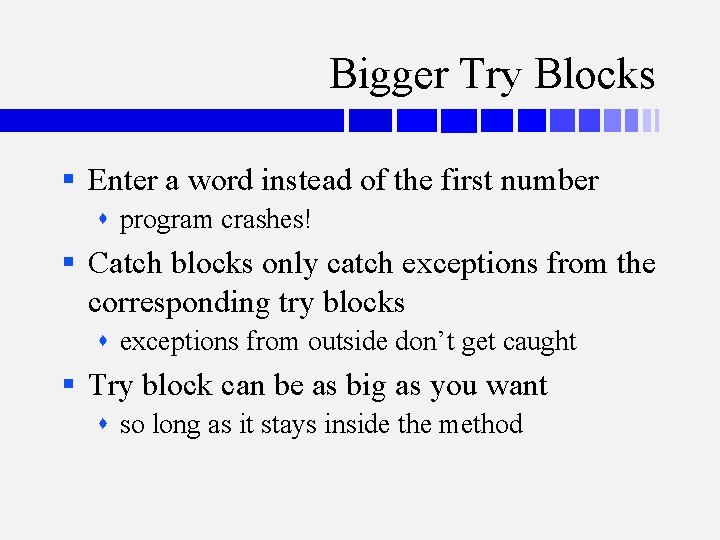
Bigger Try Blocks § Enter a word instead of the first number program crashes! § Catch blocks only catch exceptions from the corresponding try blocks exceptions from outside don’t get caught § Try block can be as big as you want so long as it stays inside the method
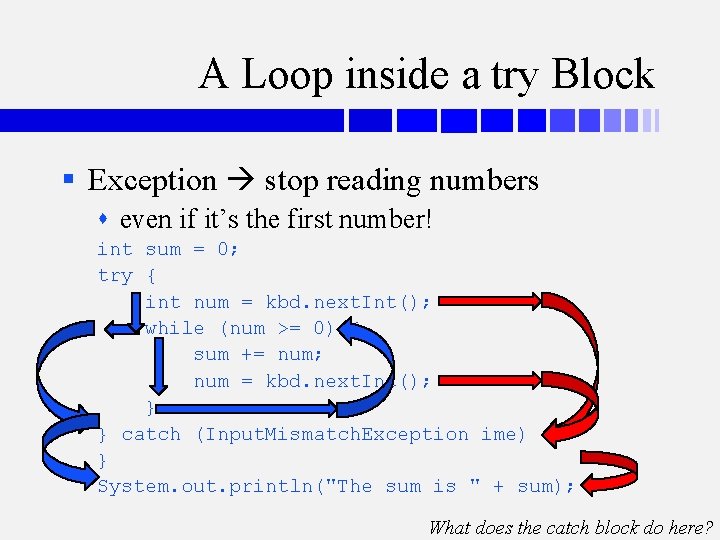
A Loop inside a try Block § Exception stop reading numbers even if it’s the first number! int sum = 0; try { int num = kbd. next. Int(); while (num >= 0) { sum += num; num = kbd. next. Int(); } } catch (Input. Mismatch. Exception ime) { } System. out. println("The sum is " + sum); What does the catch block do here?
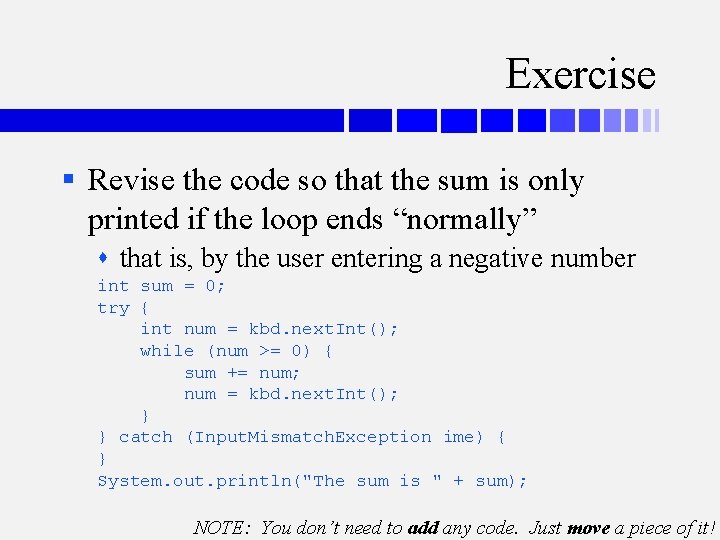
Exercise § Revise the code so that the sum is only printed if the loop ends “normally” that is, by the user entering a negative number int sum = 0; try { int num = kbd. next. Int(); while (num >= 0) { sum += num; num = kbd. next. Int(); } } catch (Input. Mismatch. Exception ime) { } System. out. println("The sum is " + sum); NOTE: You don’t need to add any code. Just move a piece of it!
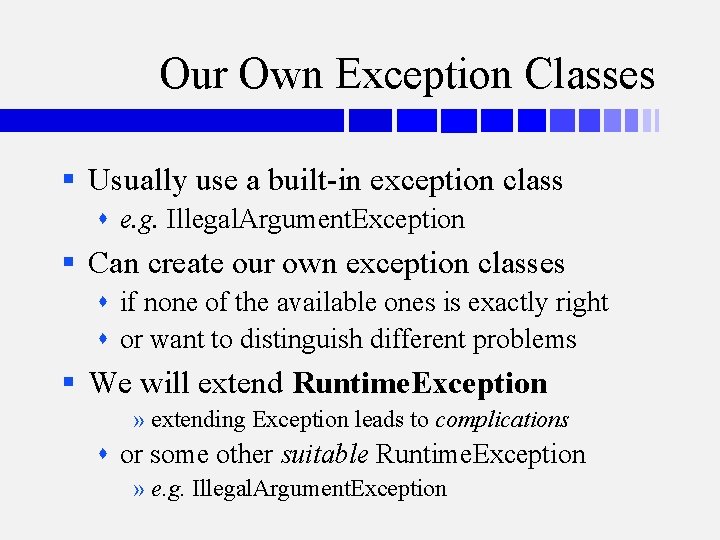
Our Own Exception Classes § Usually use a built-in exception class e. g. Illegal. Argument. Exception § Can create our own exception classes if none of the available ones is exactly right or want to distinguish different problems § We will extend Runtime. Exception » extending Exception leads to complications or some other suitable Runtime. Exception » e. g. Illegal. Argument. Exception
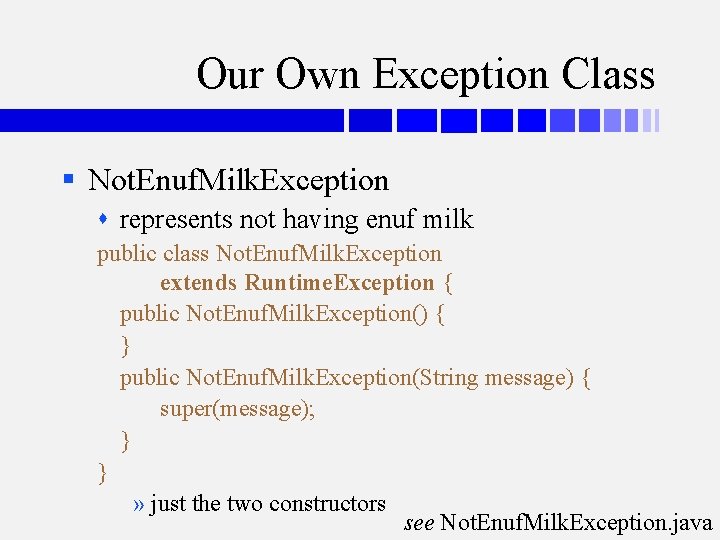
Our Own Exception Class § Not. Enuf. Milk. Exception represents not having enuf milk public class Not. Enuf. Milk. Exception extends Runtime. Exception { public Not. Enuf. Milk. Exception() { } public Not. Enuf. Milk. Exception(String message) { super(message); } } » just the two constructors see Not. Enuf. Milk. Exception. java
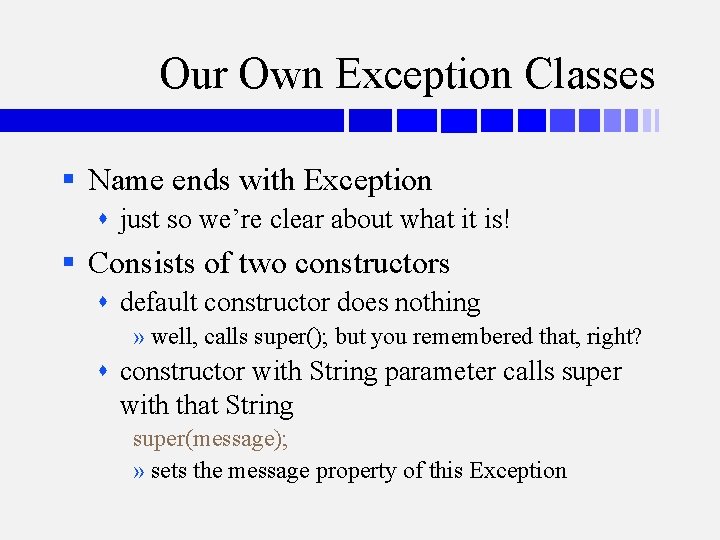
Our Own Exception Classes § Name ends with Exception just so we’re clear about what it is! § Consists of two constructors default constructor does nothing » well, calls super(); but you remembered that, right? constructor with String parameter calls super with that String super(message); » sets the message property of this Exception
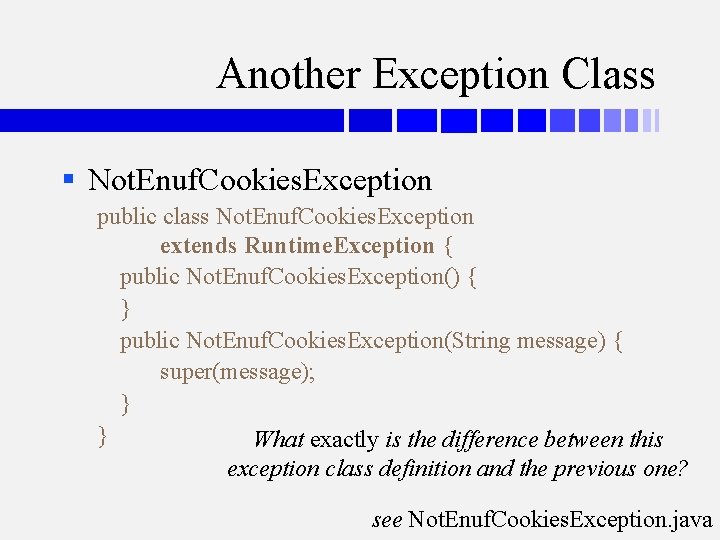
Another Exception Class § Not. Enuf. Cookies. Exception public class Not. Enuf. Cookies. Exception extends Runtime. Exception { public Not. Enuf. Cookies. Exception() { } public Not. Enuf. Cookies. Exception(String message) { super(message); } } What exactly is the difference between this exception class definition and the previous one? see Not. Enuf. Cookies. Exception. java
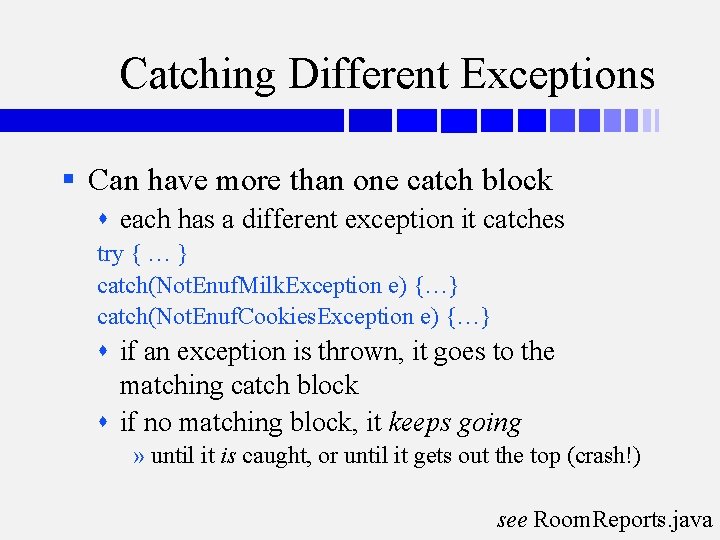
Catching Different Exceptions § Can have more than one catch block each has a different exception it catches try { … } catch(Not. Enuf. Milk. Exception e) {…} catch(Not. Enuf. Cookies. Exception e) {…} if an exception is thrown, it goes to the matching catch block if no matching block, it keeps going » until it is caught, or until it gets out the top (crash!) see Room. Reports. java
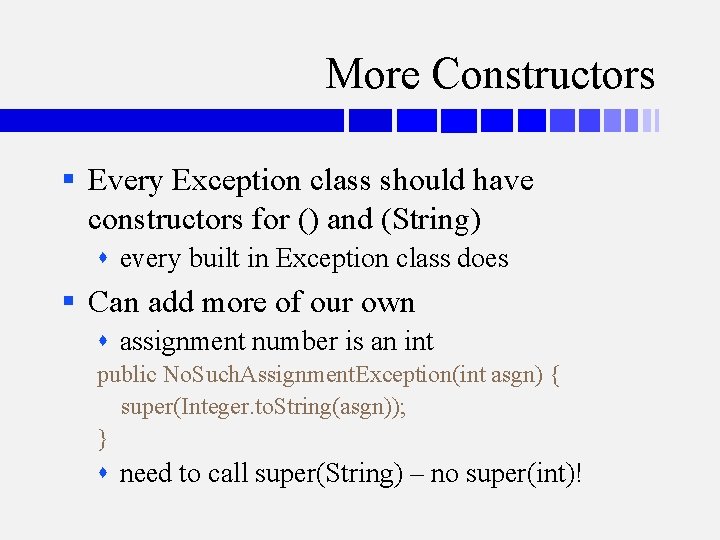
More Constructors § Every Exception class should have constructors for () and (String) every built in Exception class does § Can add more of our own assignment number is an int public No. Such. Assignment. Exception(int asgn) { super(Integer. to. String(asgn)); } need to call super(String) – no super(int)!
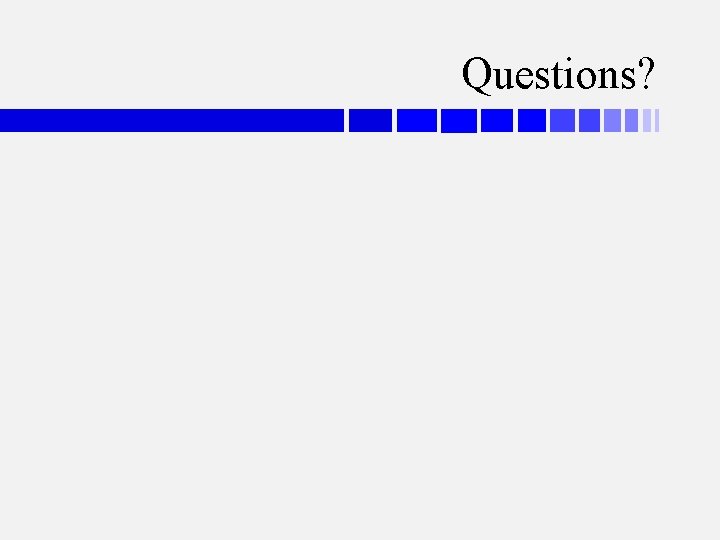
Questions?
Oraciones defining y non defining
Defining relative clause meaning in telugu
Defining/non-defining relative clauses
Defining non defining farkı
Relative clauses defining and non defining
Relative clauses
Dog catching net
Catching killers fire investigation
Your playground voice catching on the wind
Is my attitude worth catching meaning
Teaching proper throwing mechanics
Egyptian throwing stick
Phases of throwing
Night of the scorpion poem by nissim ezekiel
Dice k matsuzaka gyroball
Throwing a ball quadratic equation
Anatomy of throwing a dart
Throwing process of silk
Biomechanics throwing football
Native american throwing stick
Biomechanics of throwing a football
Movement analysis of throwing a frisbee
Throwing off asia
Throwing off asia
Stickman animation ideas
Venn diagram of running jumping and throwing
Custom exceptions in java
Exception of mendel law
Different types of exceptions in c++
State verbs
Non predefined exceptions in oracle
Tallcomparative and superlative
Pad see ew pronunciation
What is microbiology
Present continuous questions
Koch's postulates exceptions