Chapter 15 Exception Handling 15 1 Throwing Exceptions
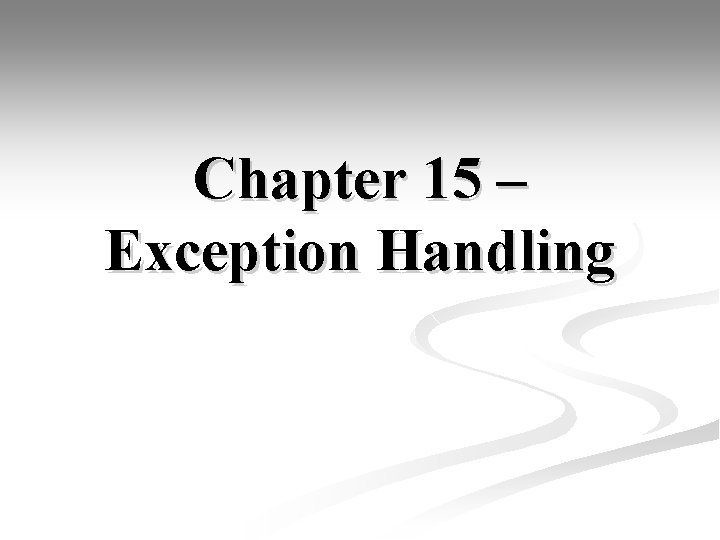
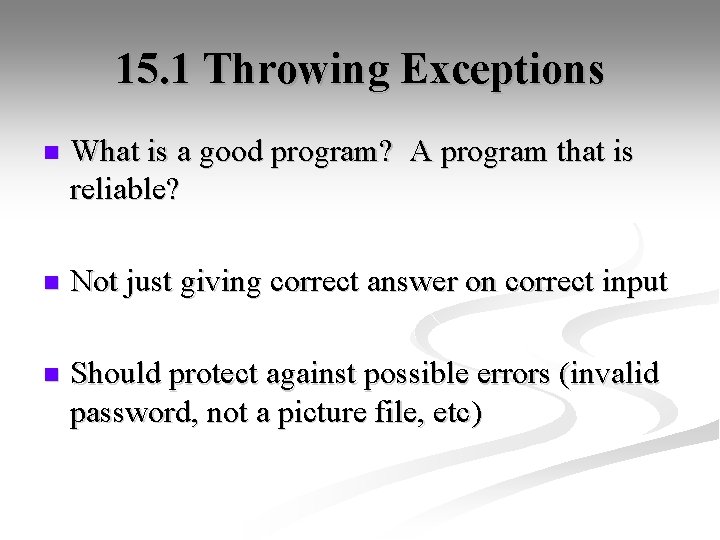
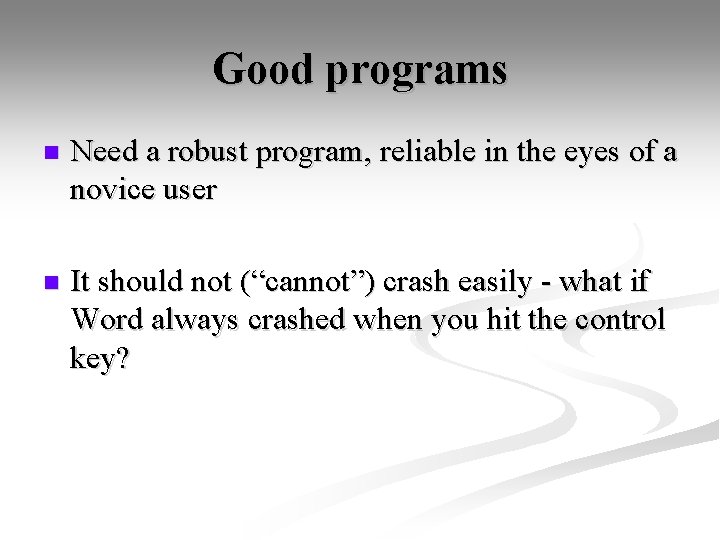
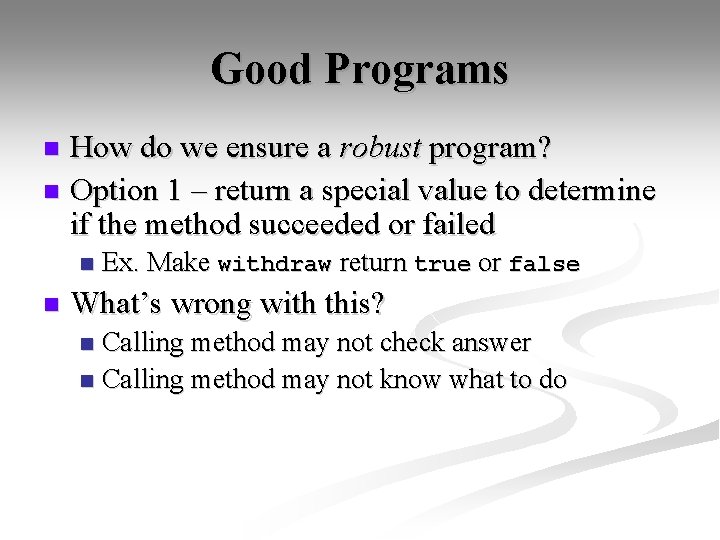
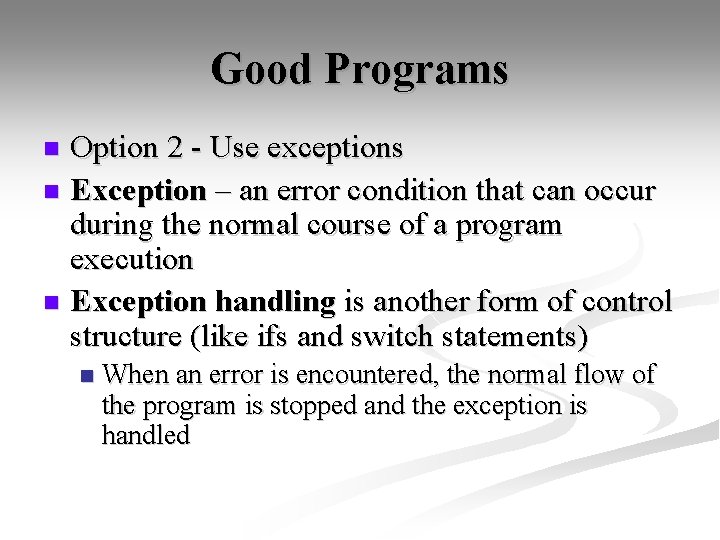
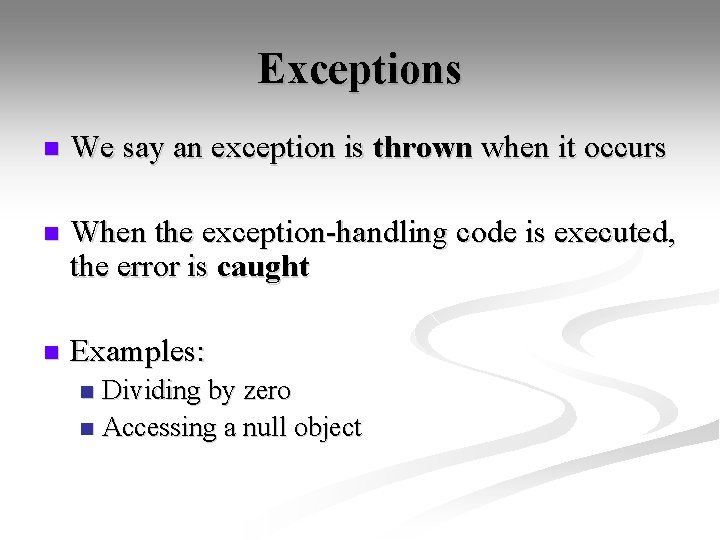
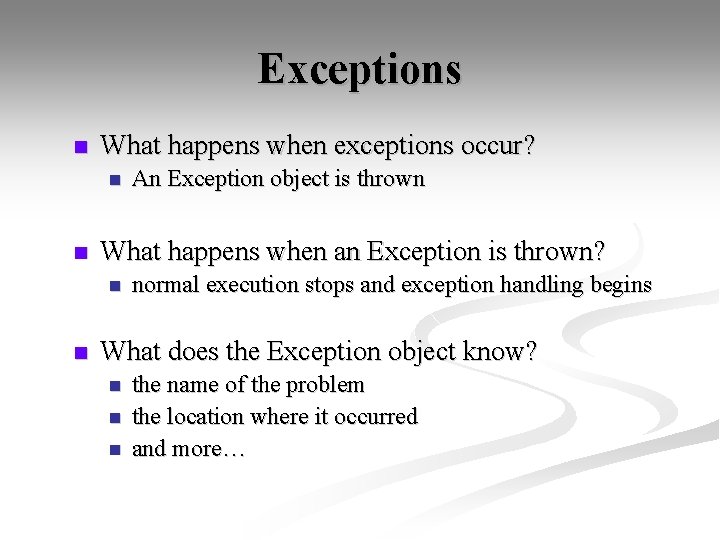
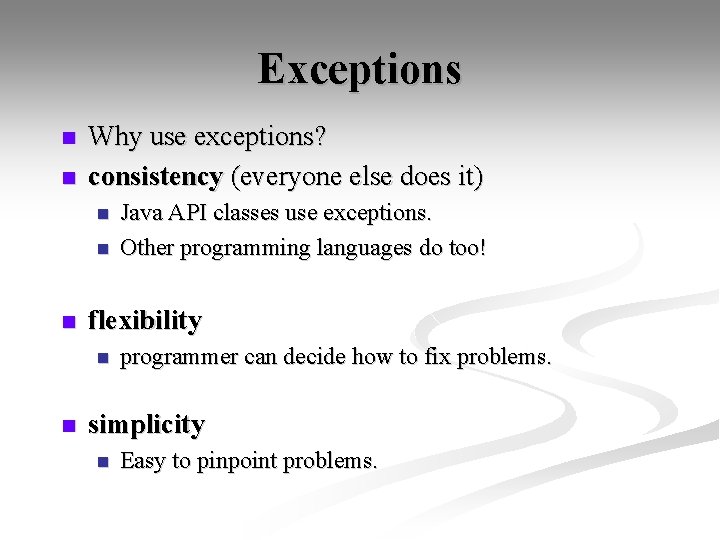
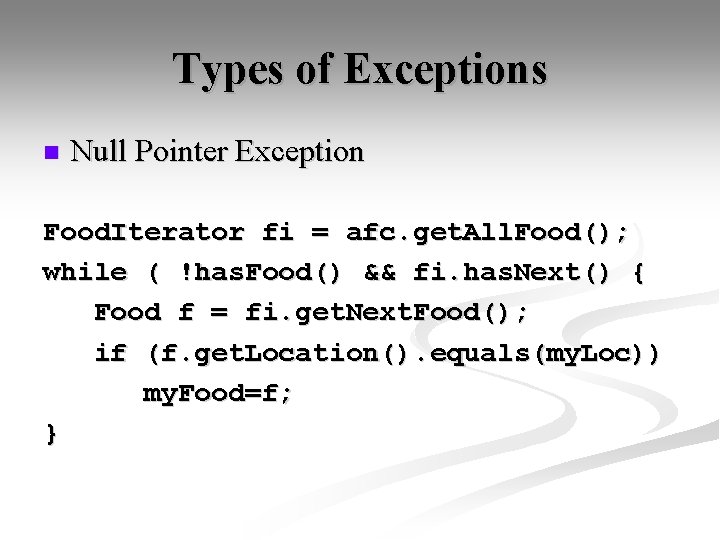
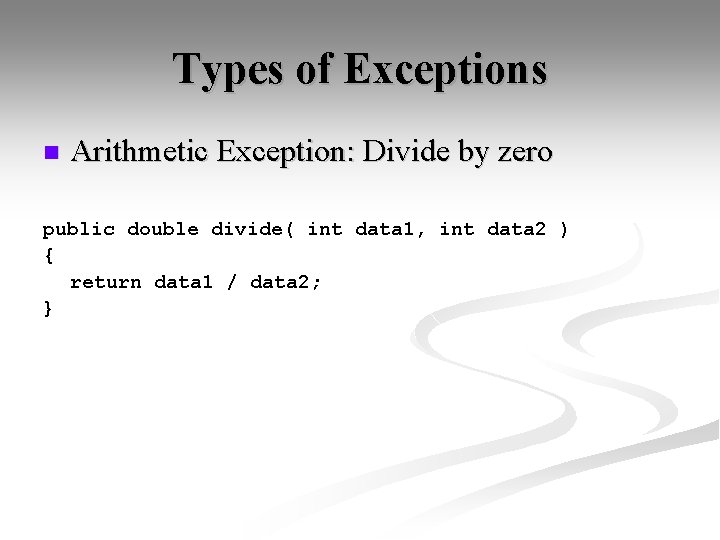
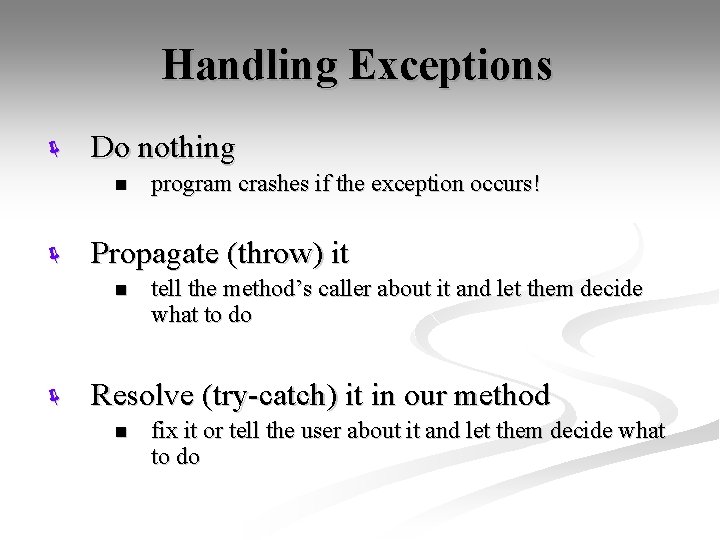
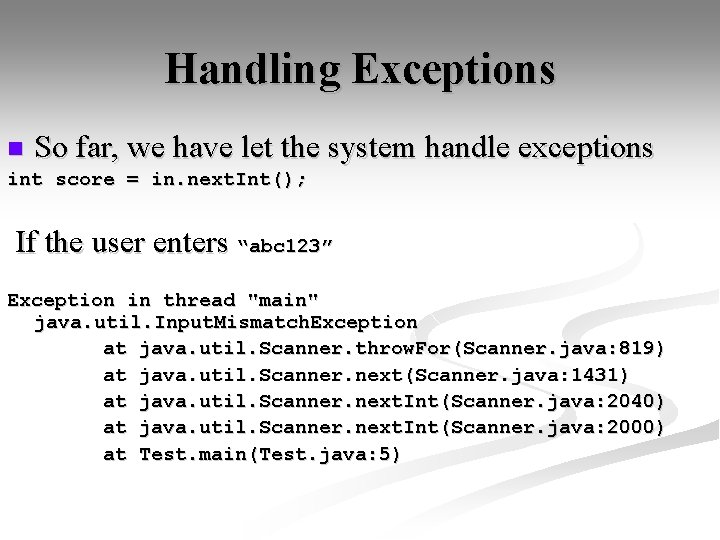
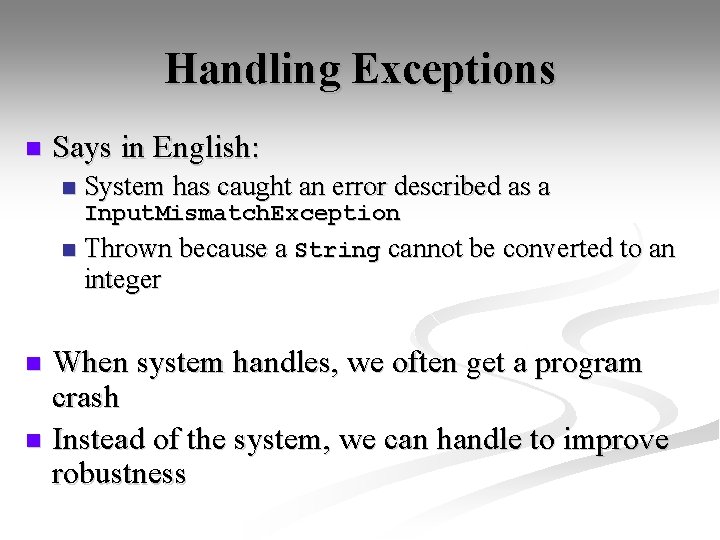
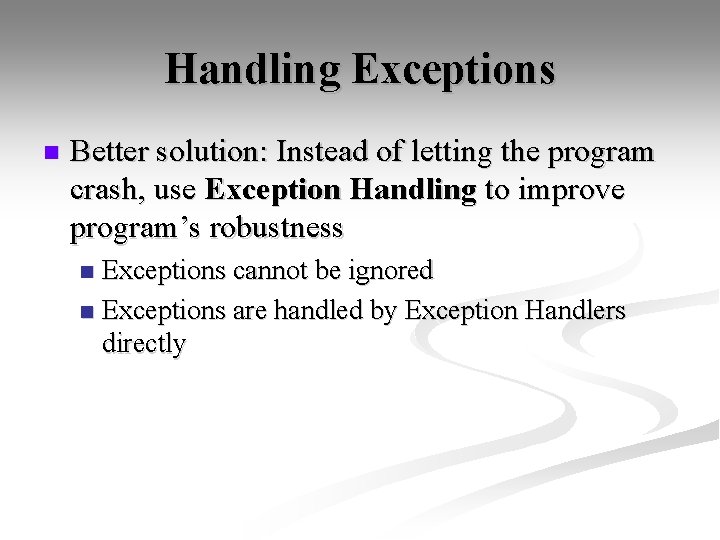
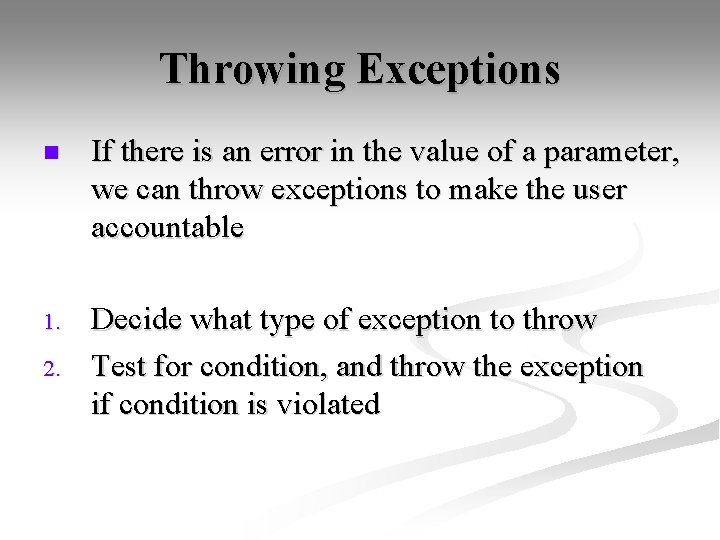
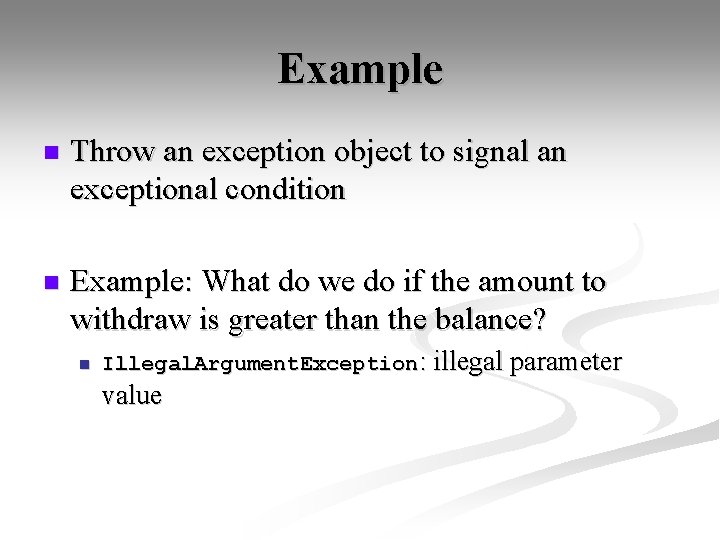
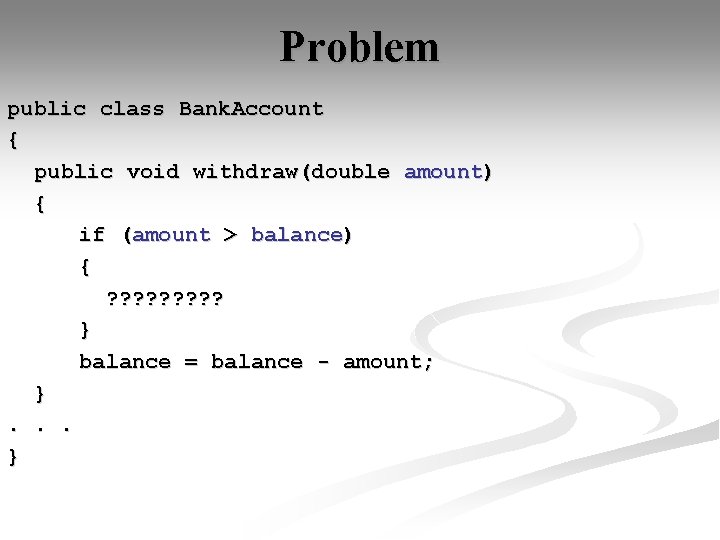
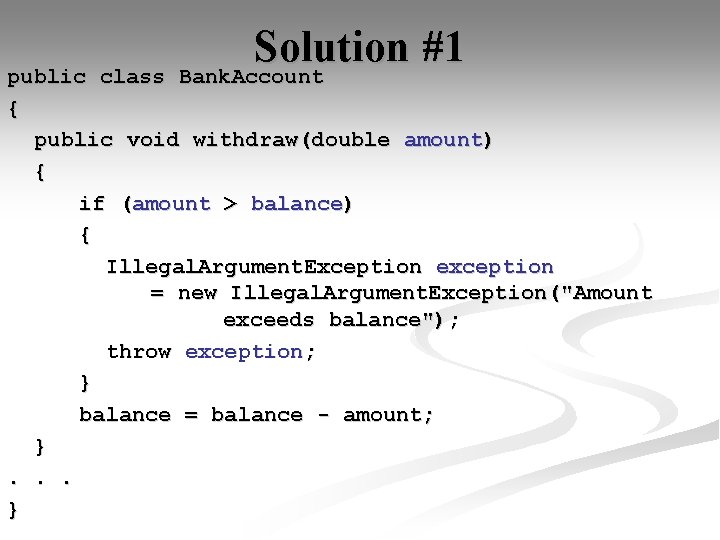
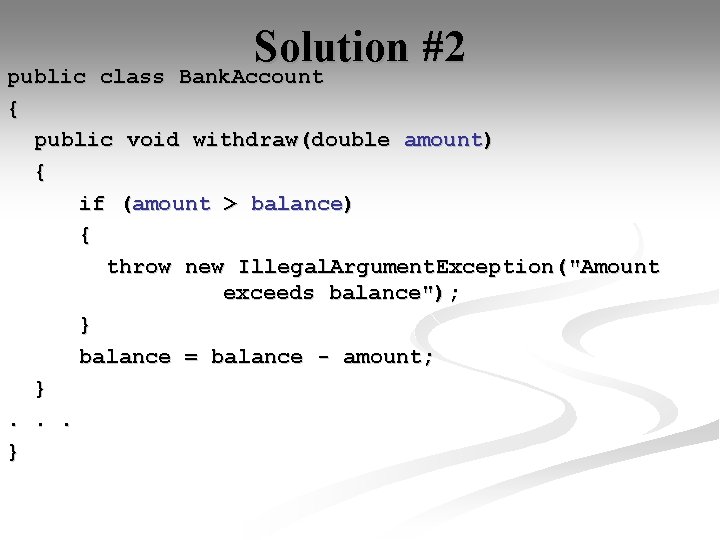
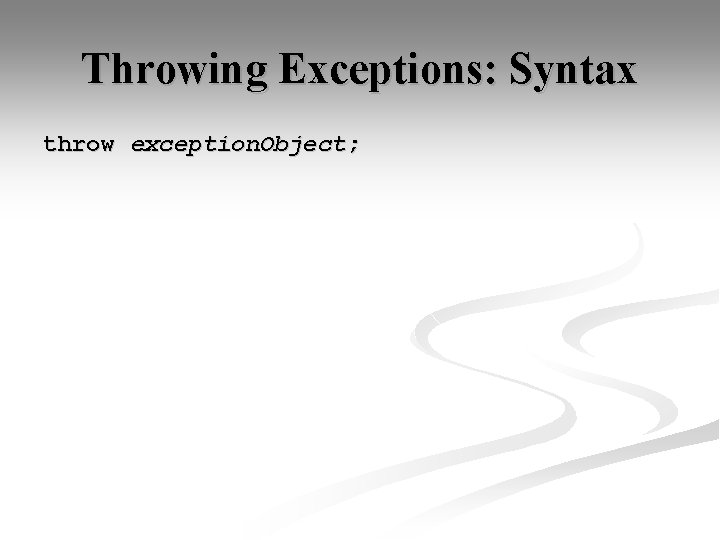
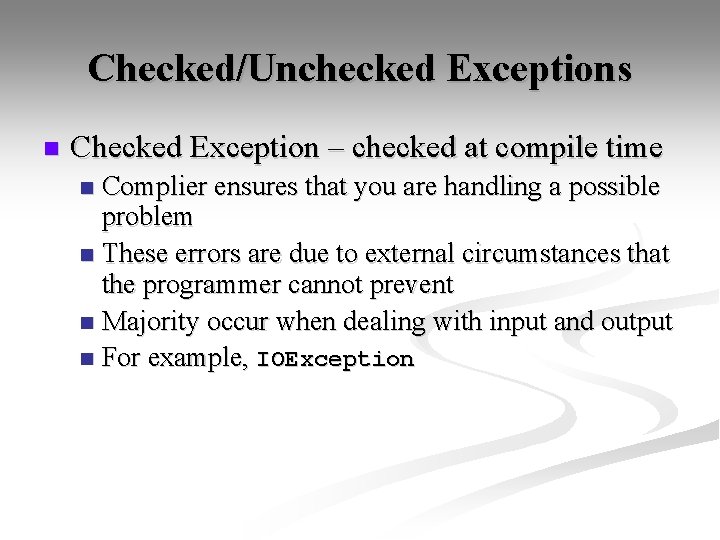
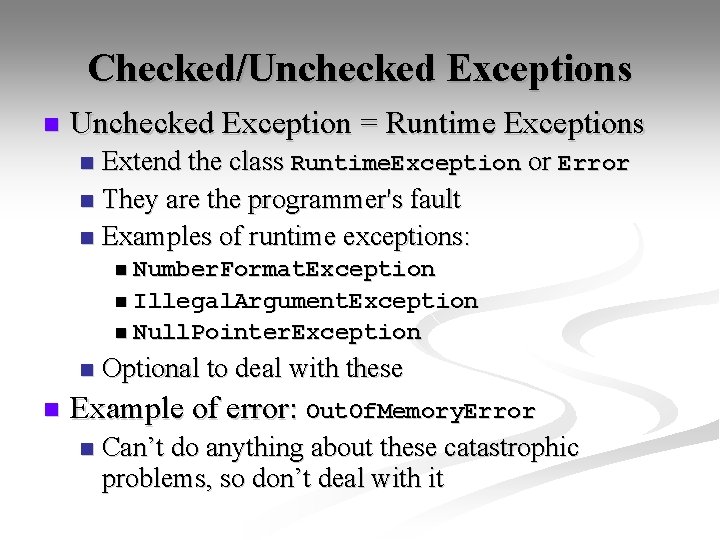
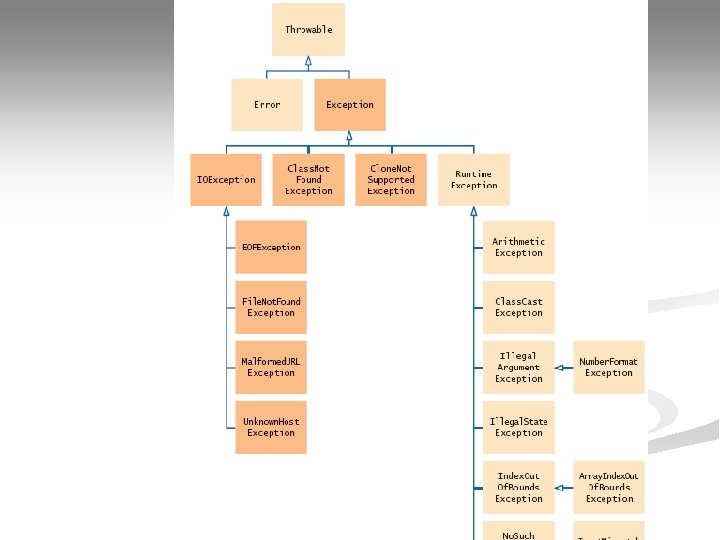
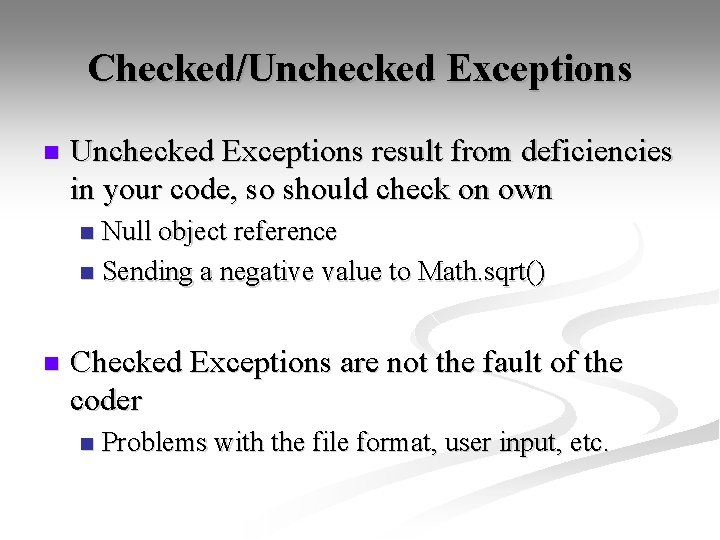
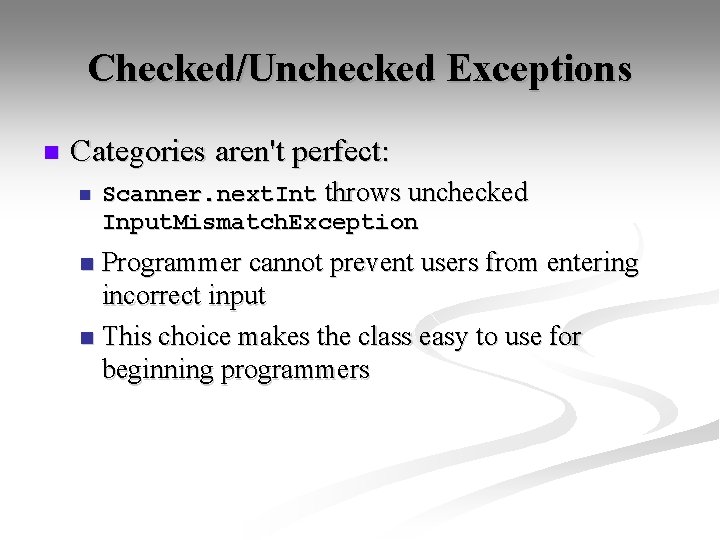
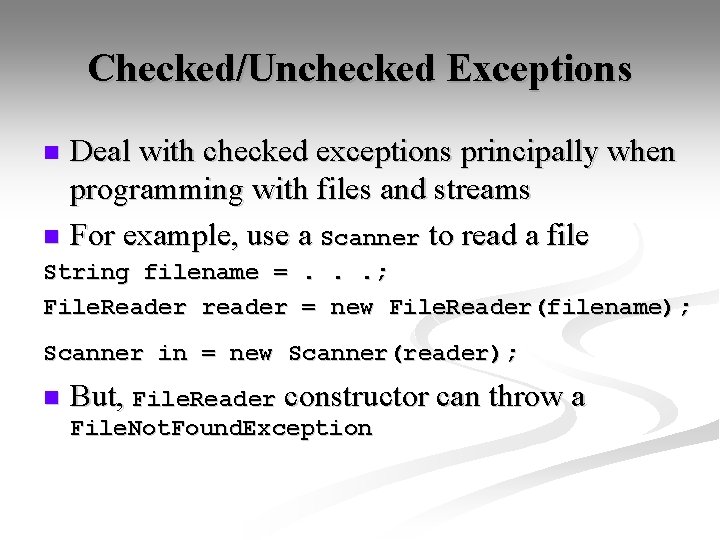
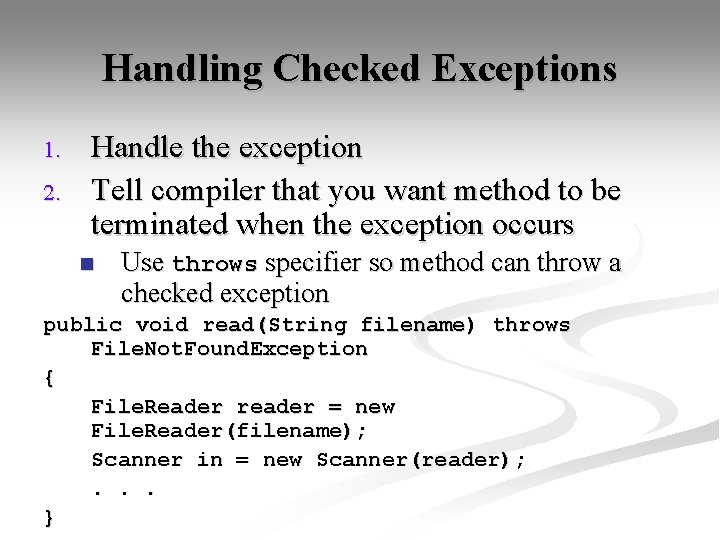
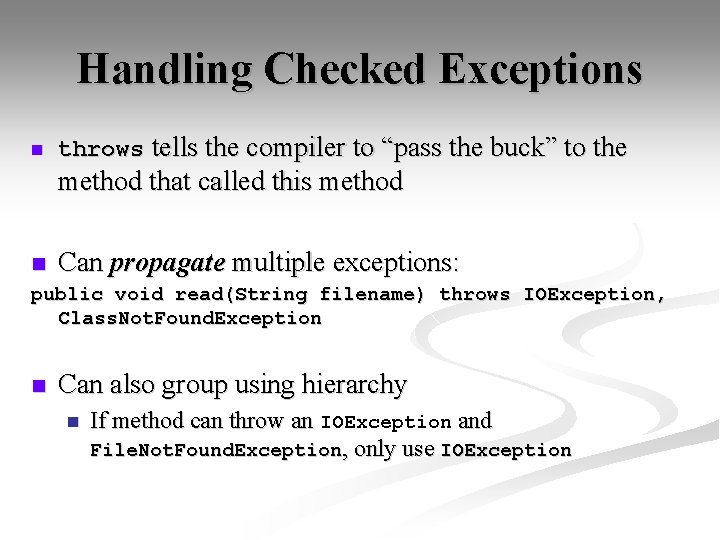
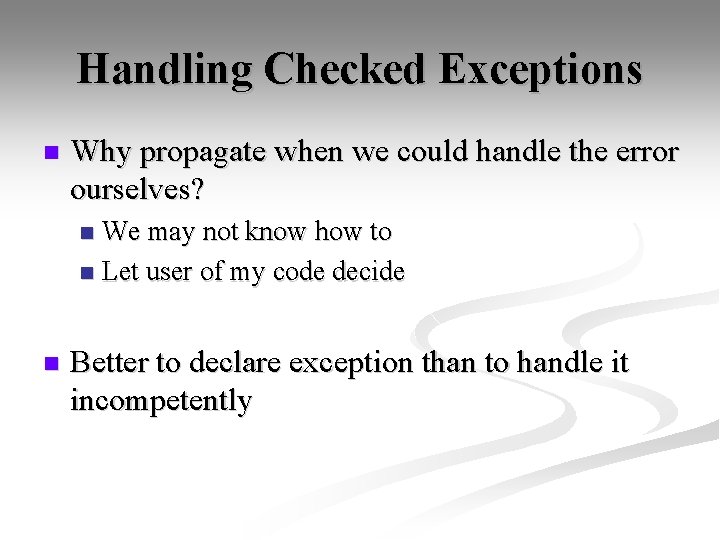
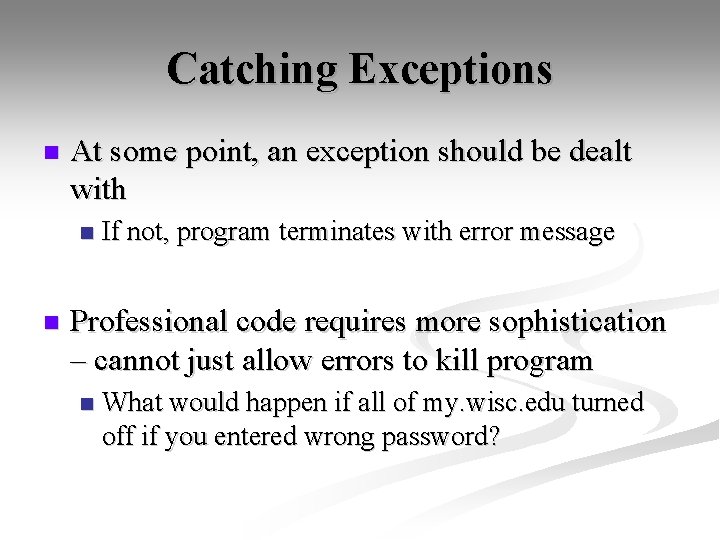
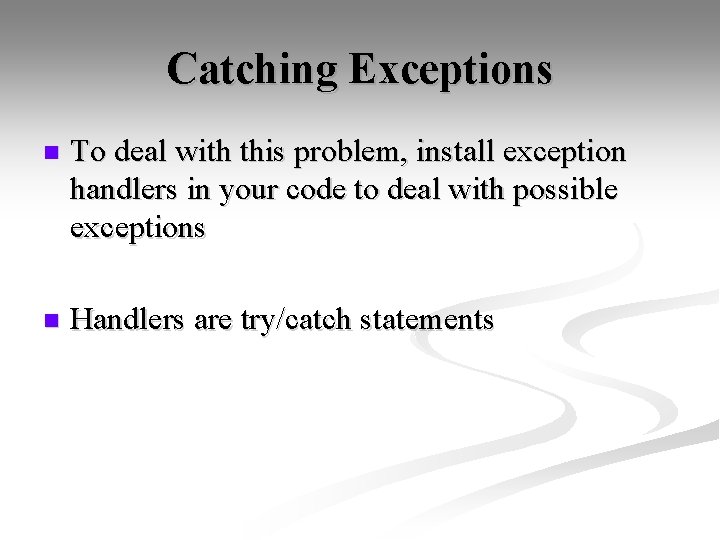
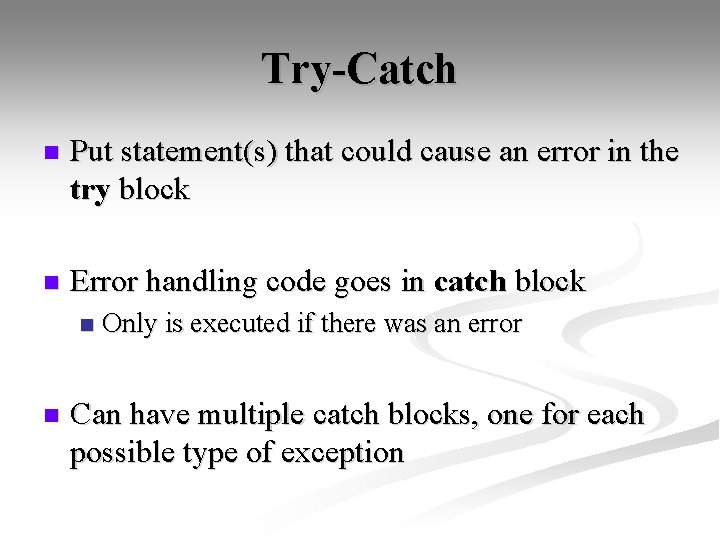
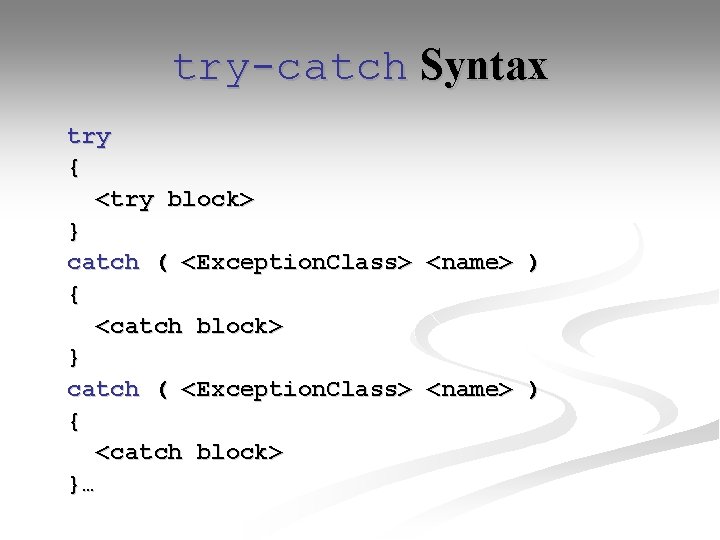
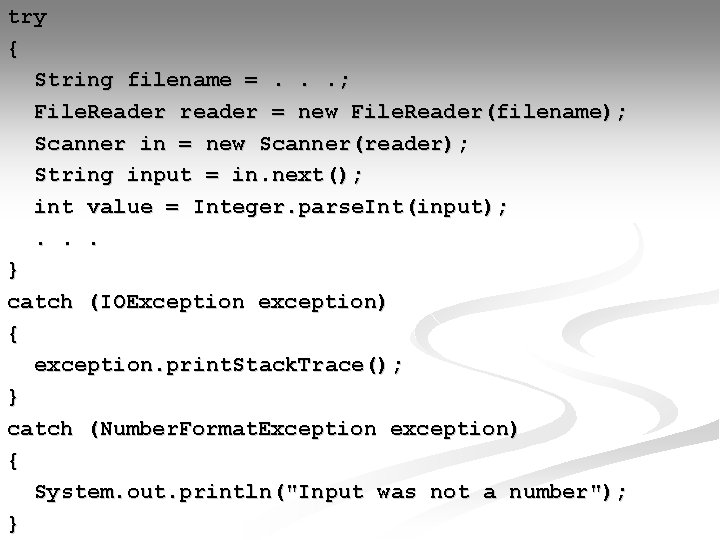
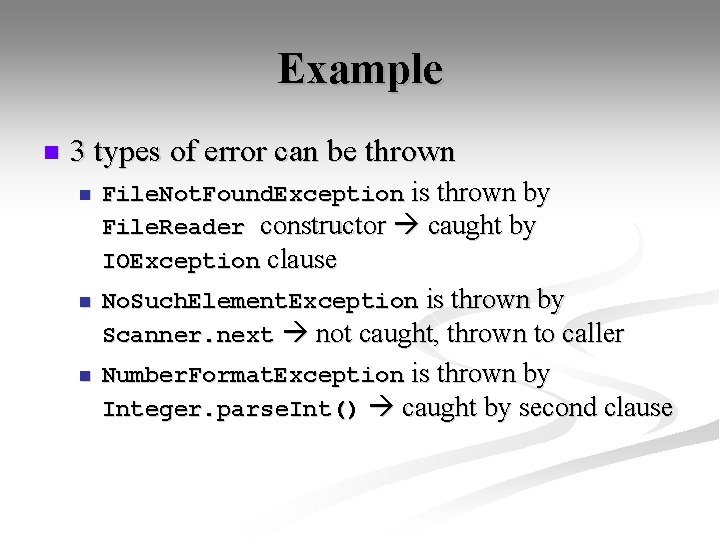
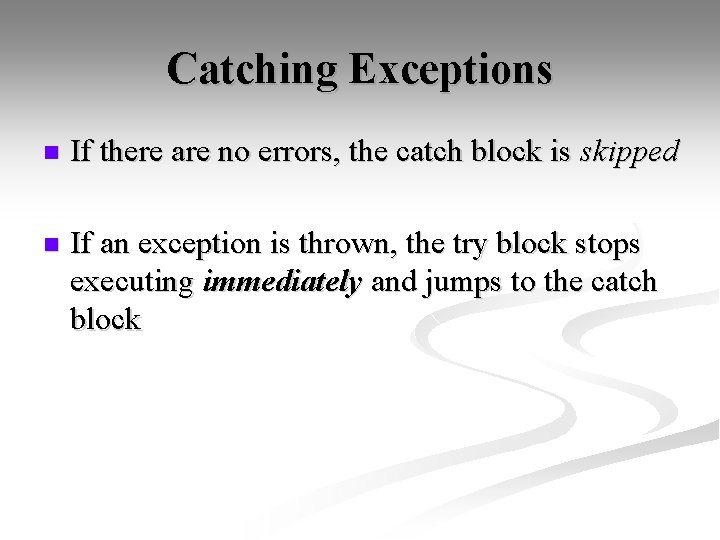
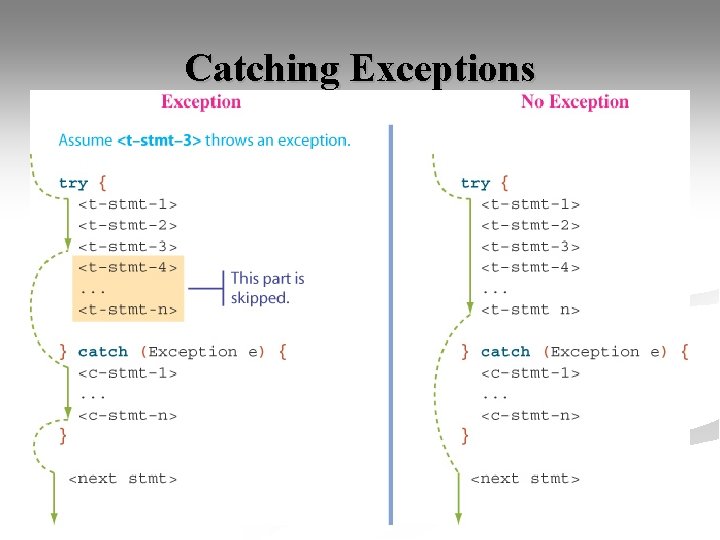
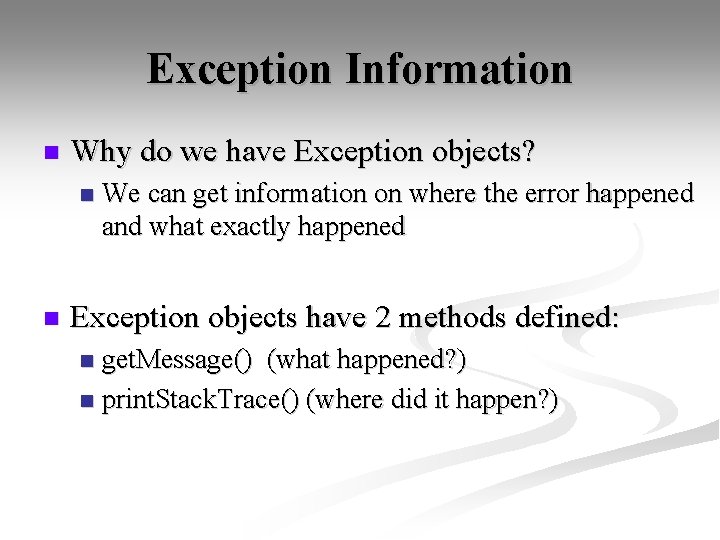
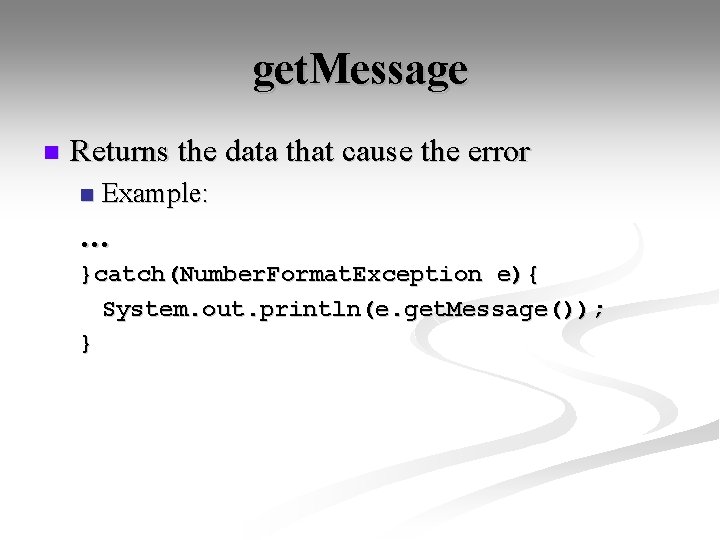
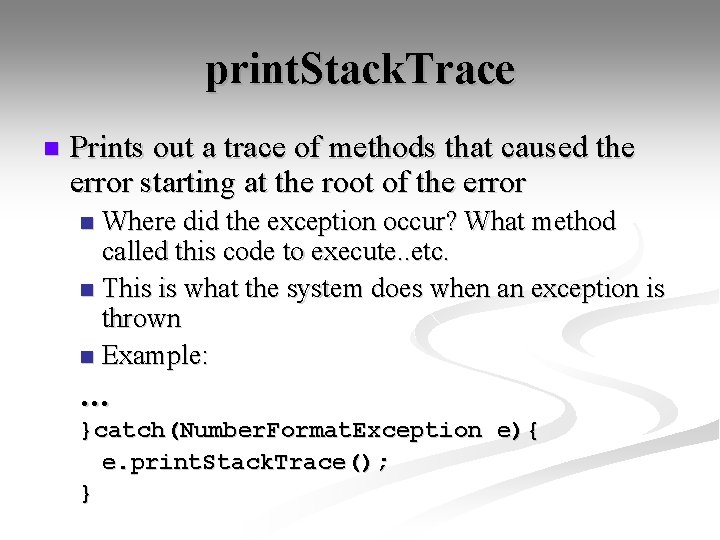
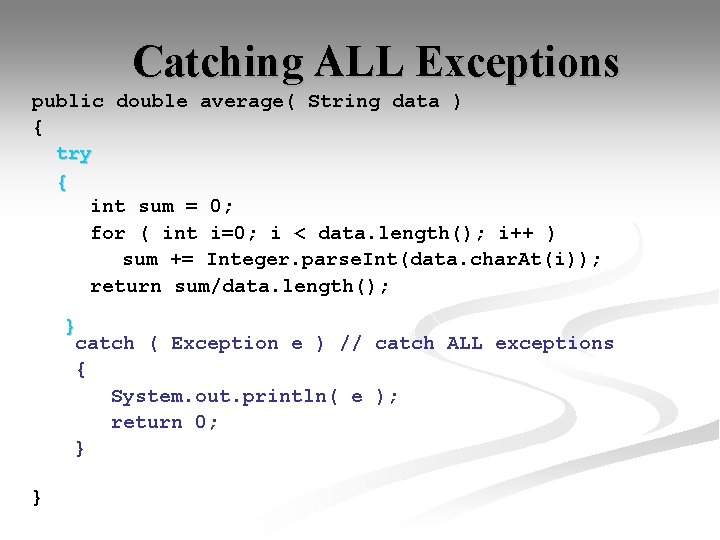
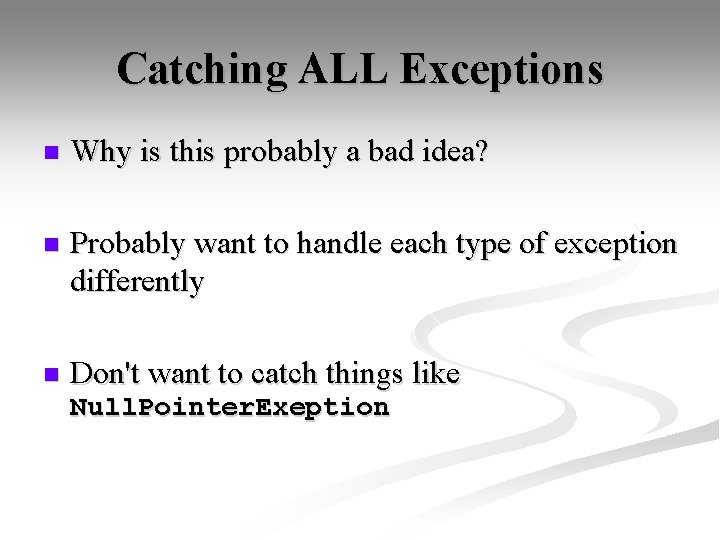
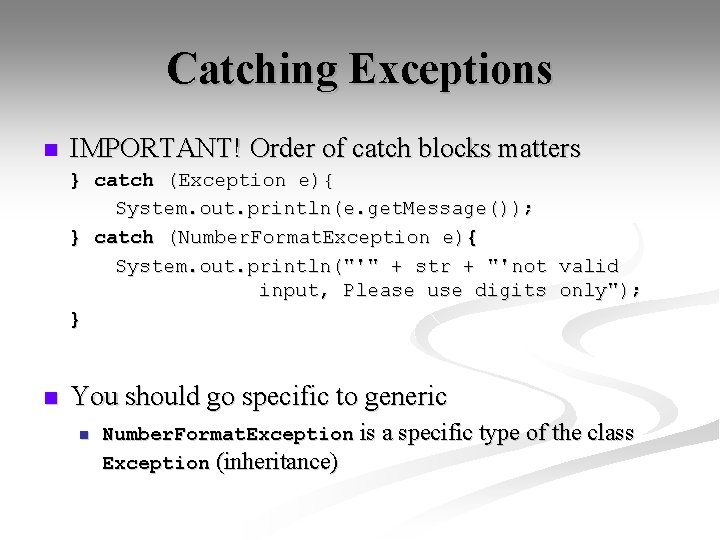
![Demo p. s. v. main( String [] args ) { try { String filename Demo p. s. v. main( String [] args ) { try { String filename](https://slidetodoc.com/presentation_image_h/3612230ebfc9ea60189c6cdcd0375289/image-44.jpg)
![String filename=args[0], input; try { scanner = new Scanner(new File(filename)); input = scanner. next. String filename=args[0], input; try { scanner = new Scanner(new File(filename)); input = scanner. next.](https://slidetodoc.com/presentation_image_h/3612230ebfc9ea60189c6cdcd0375289/image-45.jpg)
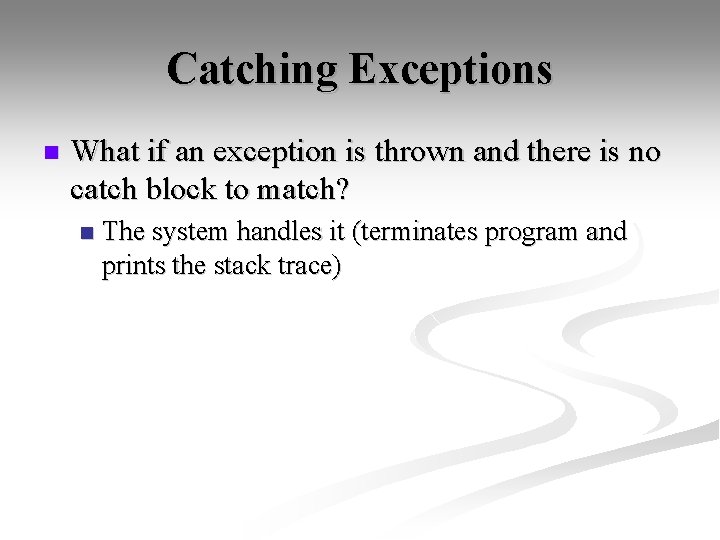
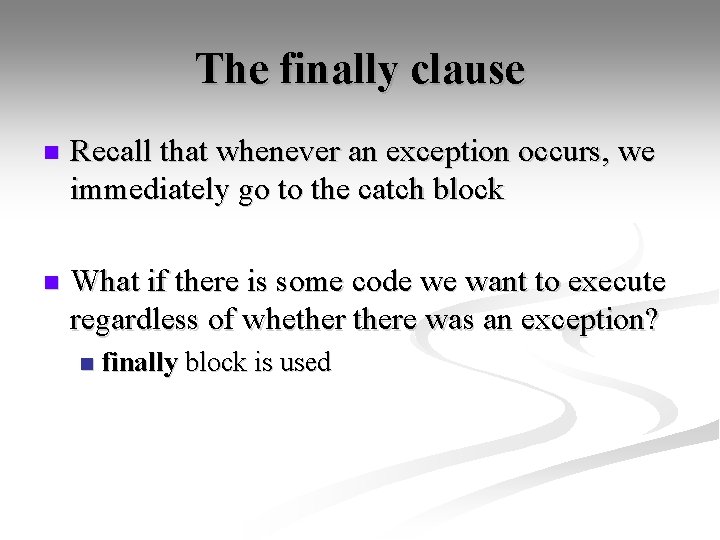
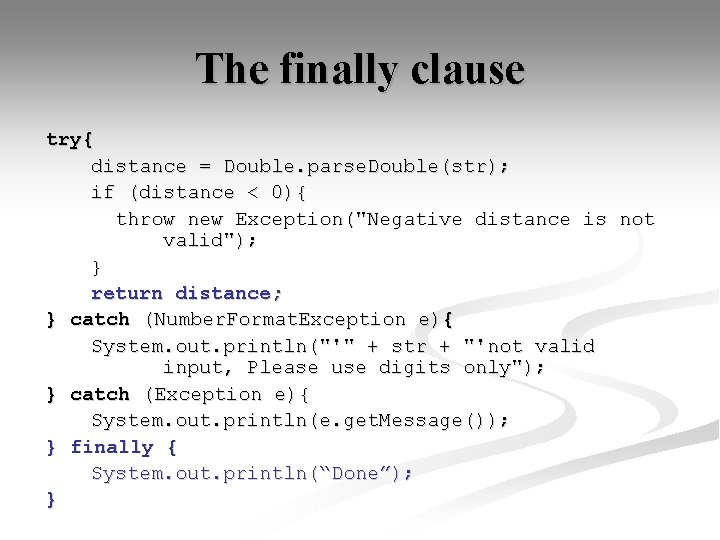
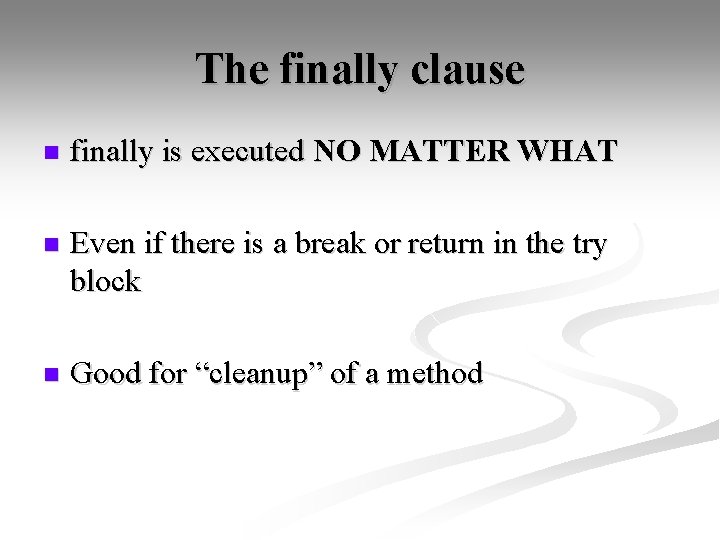
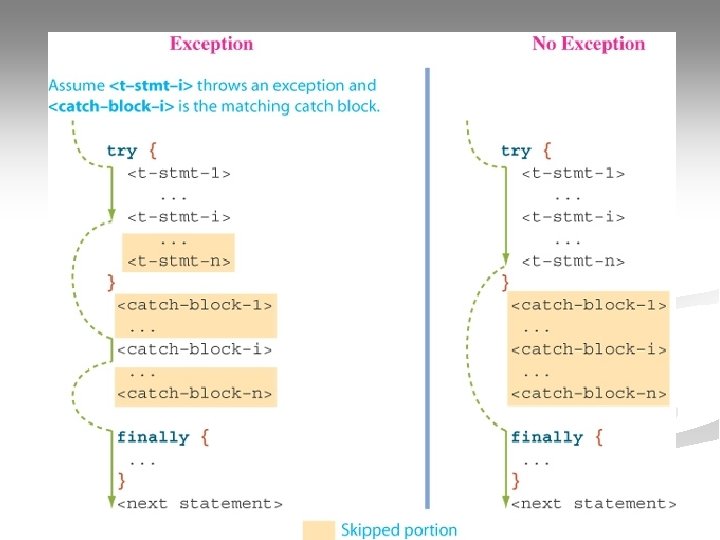
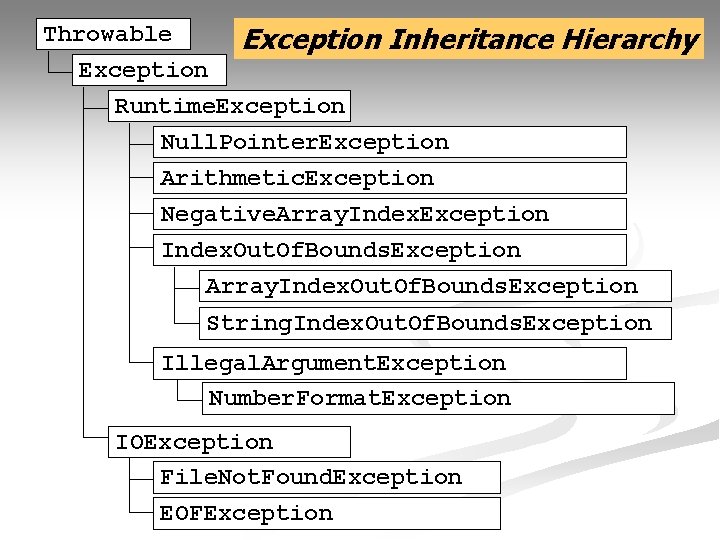
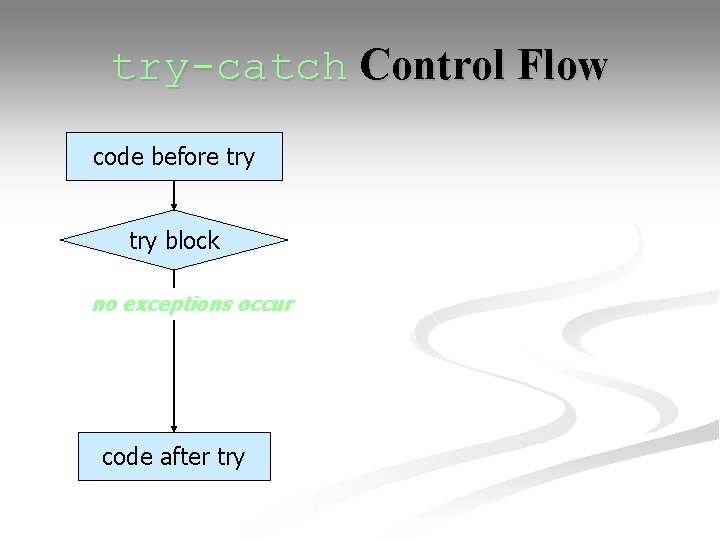
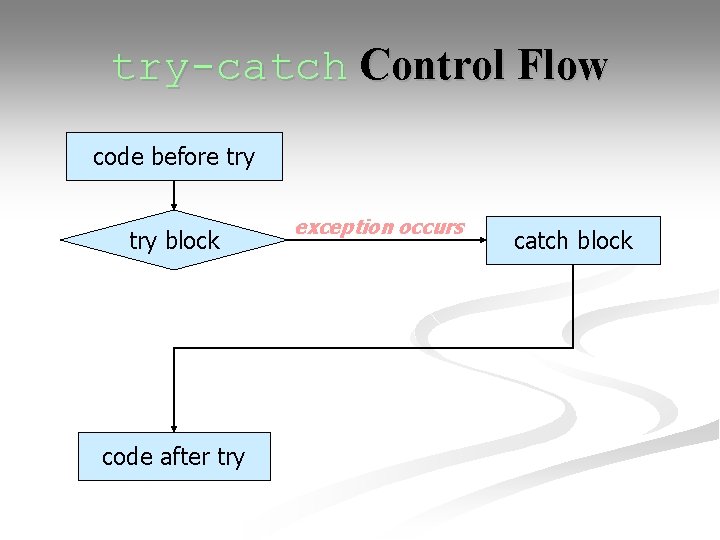
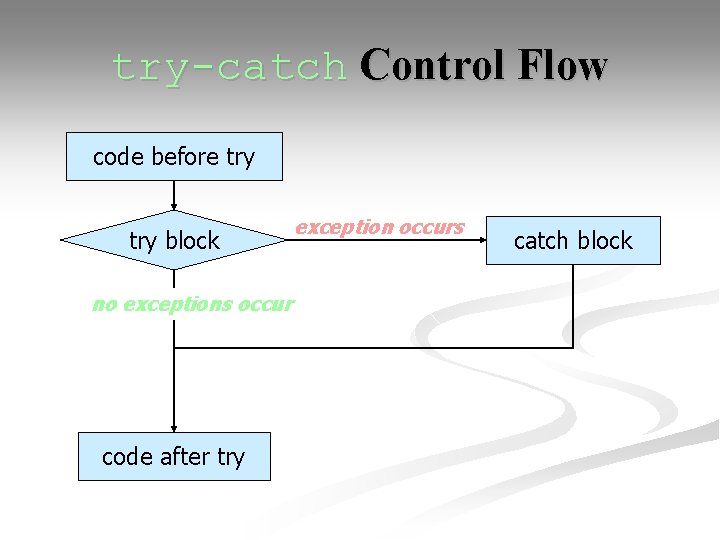
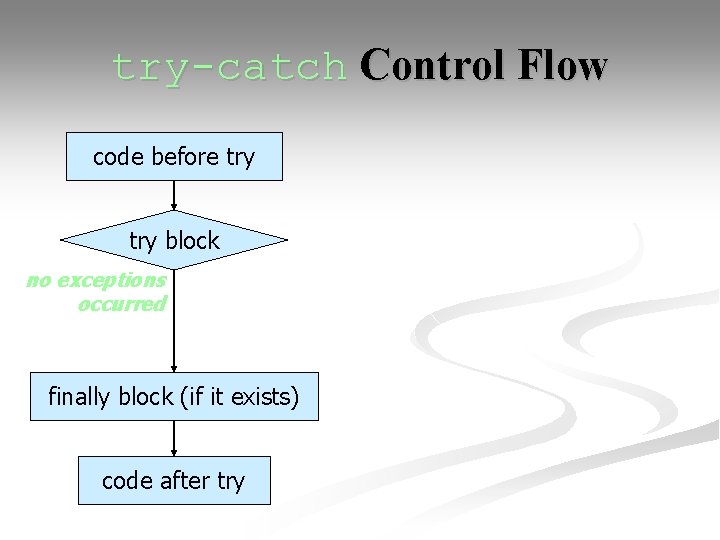
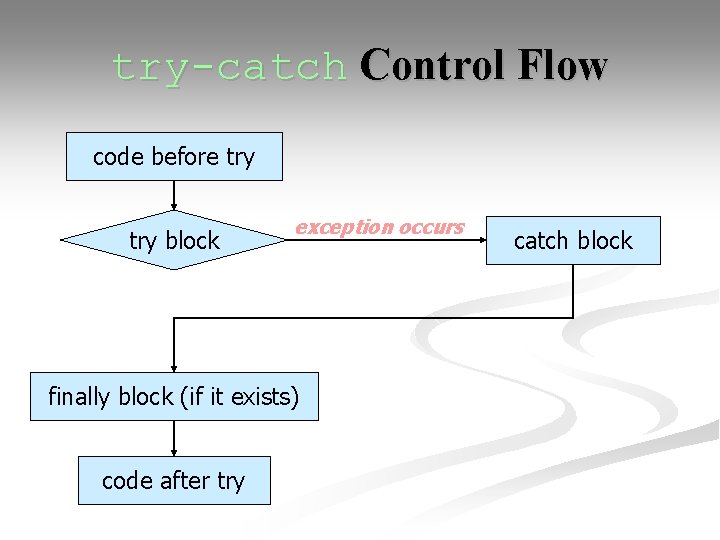
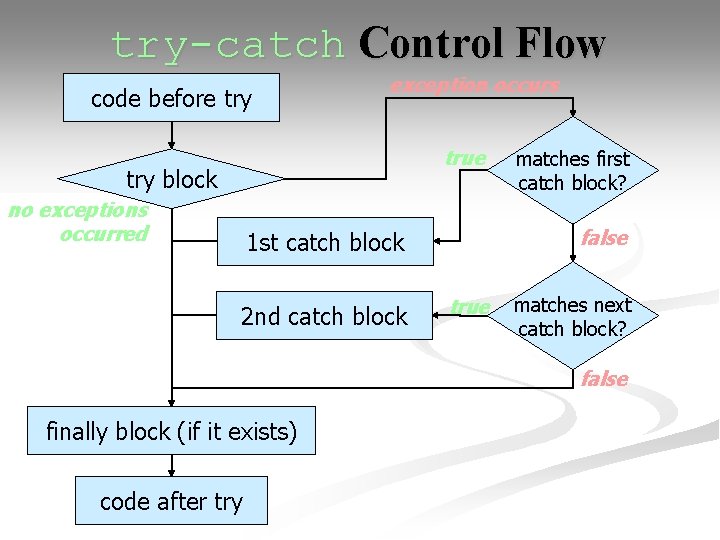
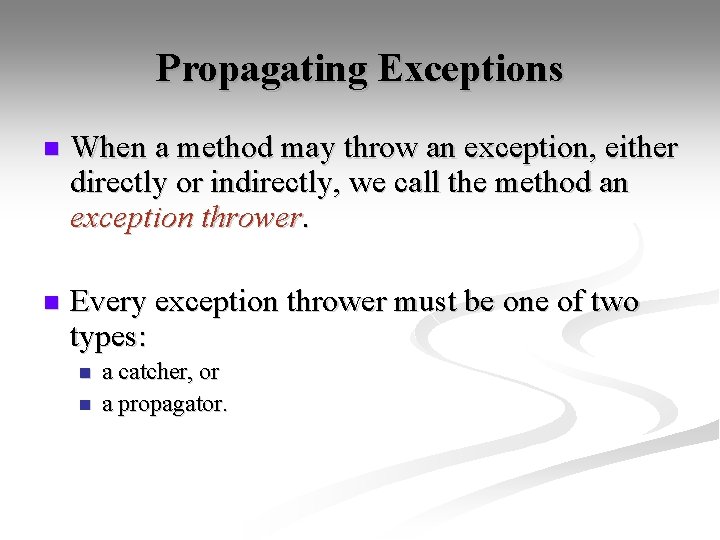
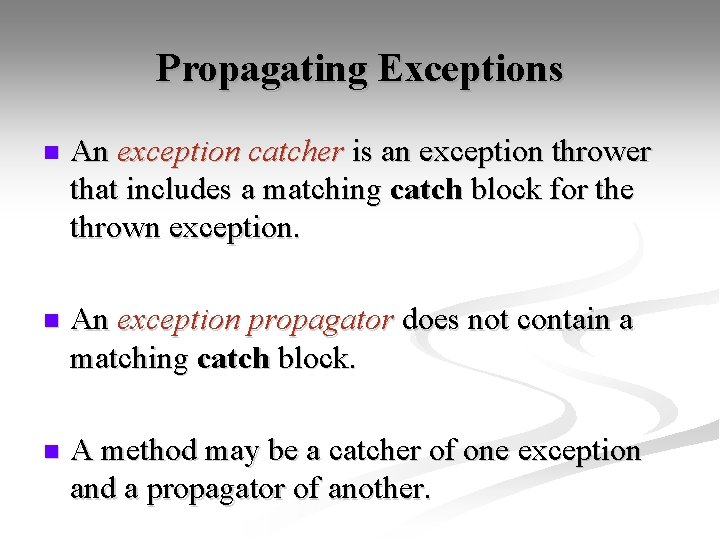
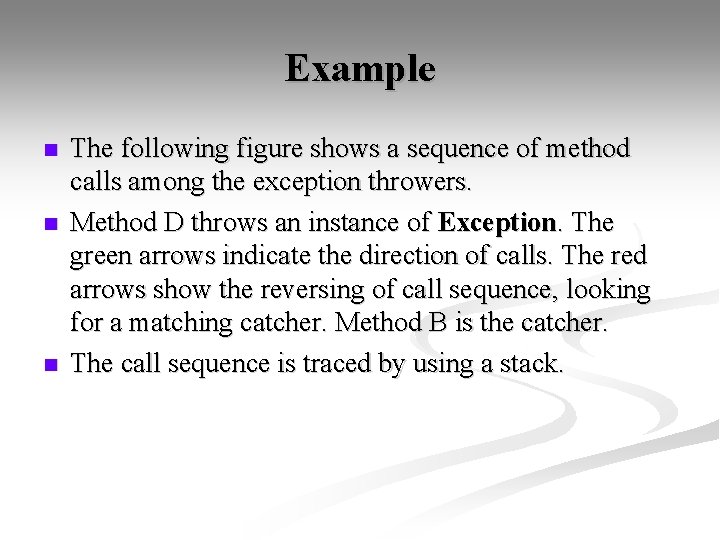
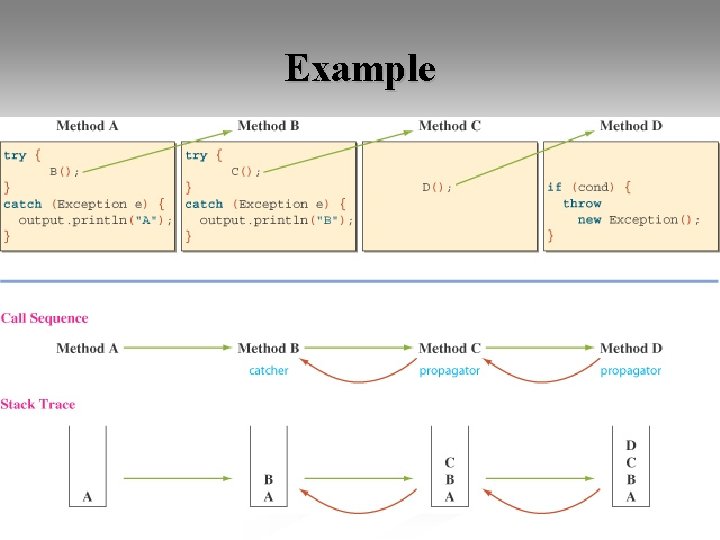
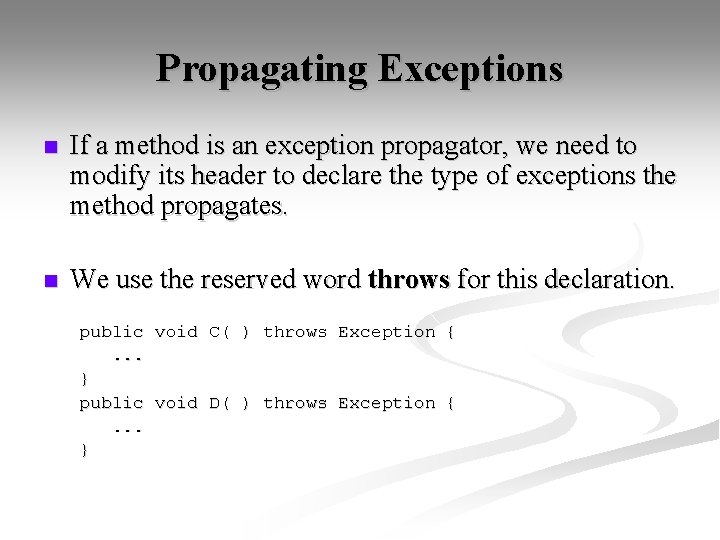
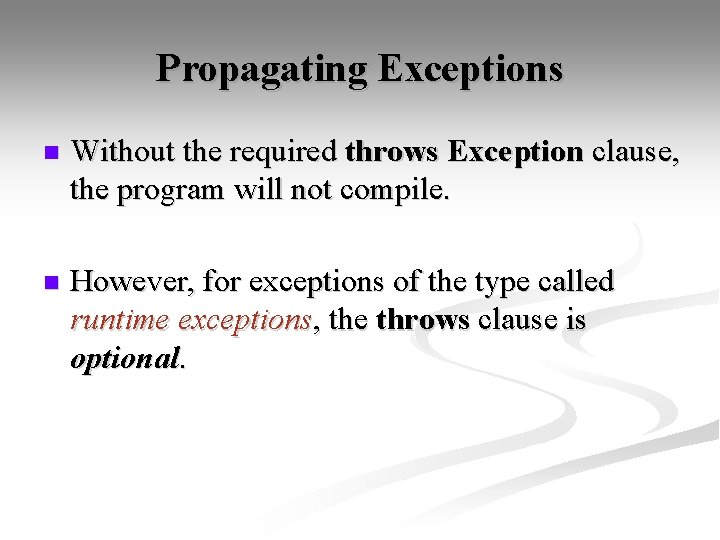
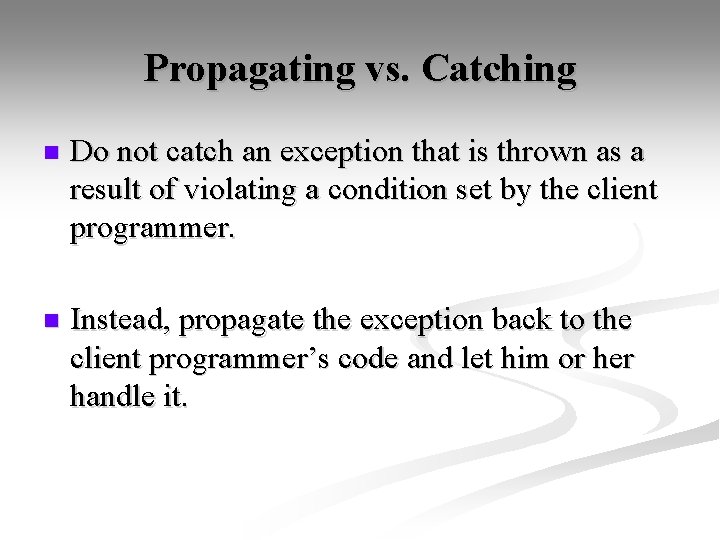
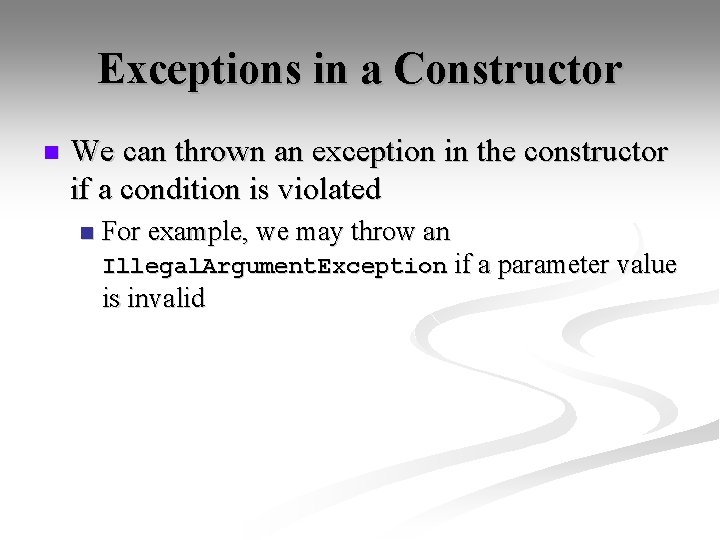
- Slides: 65
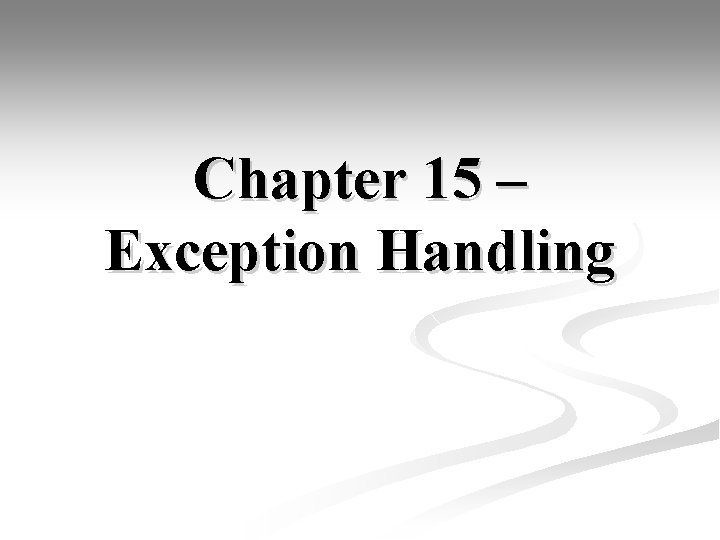
Chapter 15 – Exception Handling
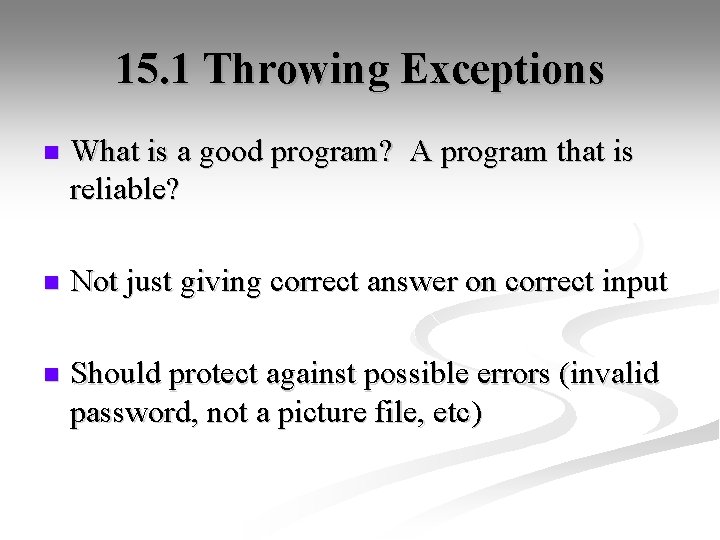
15. 1 Throwing Exceptions n What is a good program? A program that is reliable? n Not just giving correct answer on correct input n Should protect against possible errors (invalid password, not a picture file, etc)
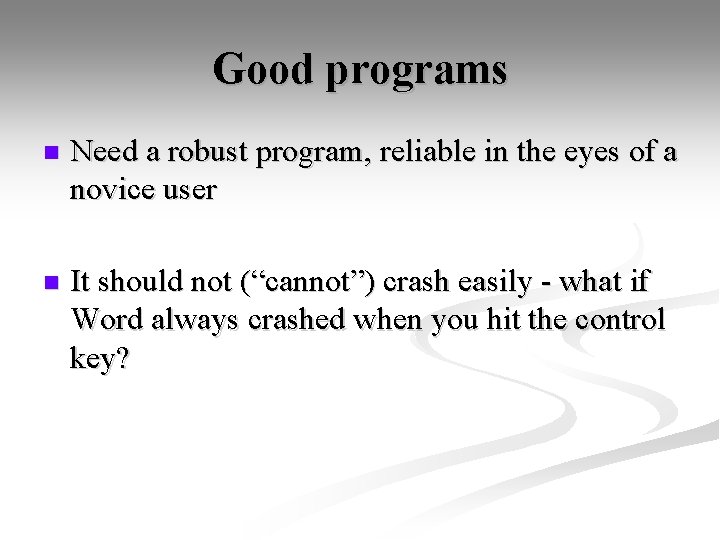
Good programs n Need a robust program, reliable in the eyes of a novice user n It should not (“cannot”) crash easily - what if Word always crashed when you hit the control key?
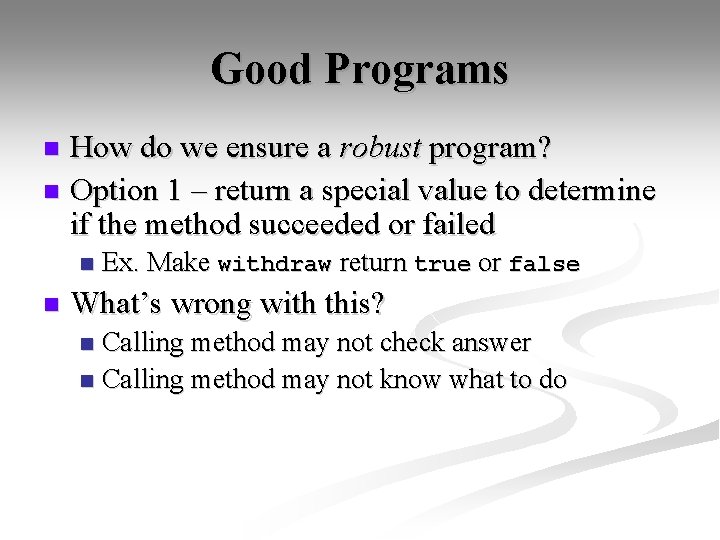
Good Programs How do we ensure a robust program? n Option 1 – return a special value to determine if the method succeeded or failed n n n Ex. Make withdraw return true or false What’s wrong with this? Calling method may not check answer n Calling method may not know what to do n
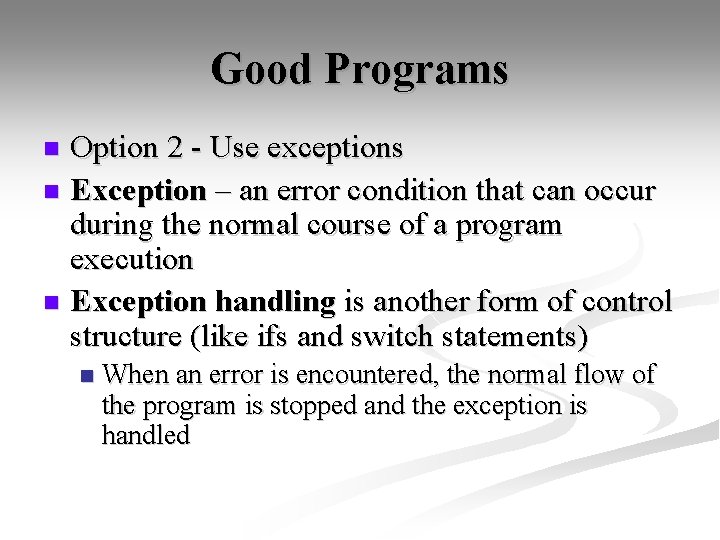
Good Programs Option 2 - Use exceptions n Exception – an error condition that can occur during the normal course of a program execution n Exception handling is another form of control structure (like ifs and switch statements) n n When an error is encountered, the normal flow of the program is stopped and the exception is handled
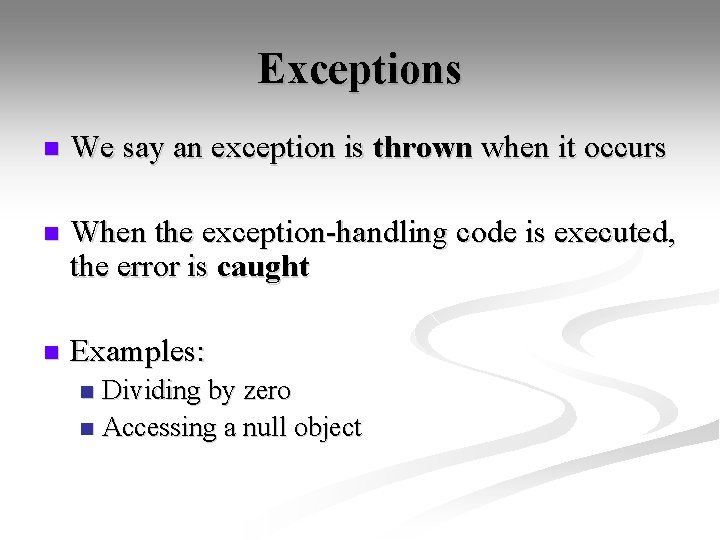
Exceptions n We say an exception is thrown when it occurs n When the exception-handling code is executed, the error is caught n Examples: Dividing by zero n Accessing a null object n
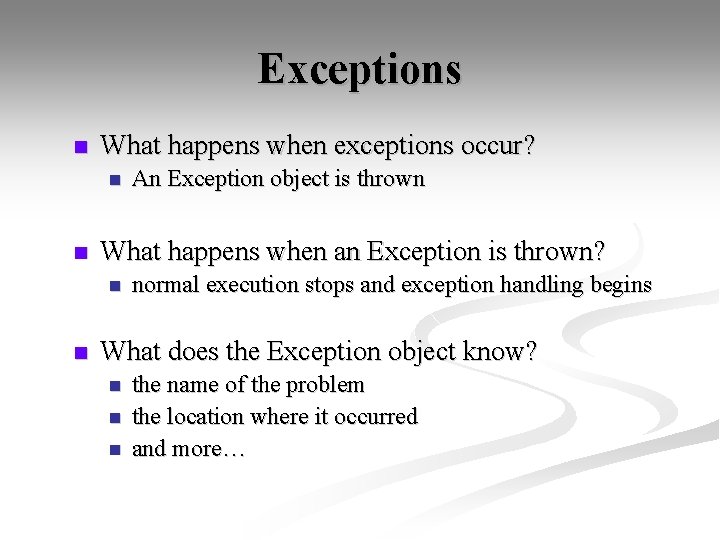
Exceptions n What happens when exceptions occur? n n What happens when an Exception is thrown? n n An Exception object is thrown normal execution stops and exception handling begins What does the Exception object know? n n n the name of the problem the location where it occurred and more…
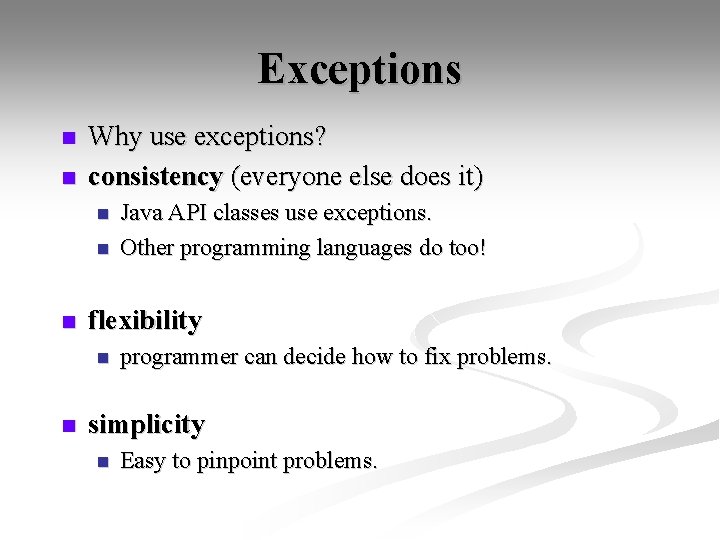
Exceptions n n Why use exceptions? consistency (everyone else does it) n n n flexibility n n Java API classes use exceptions. Other programming languages do too! programmer can decide how to fix problems. simplicity n Easy to pinpoint problems.
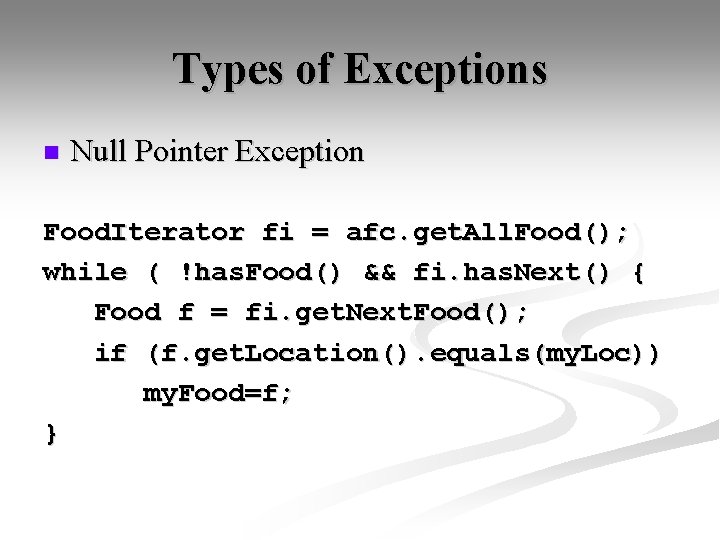
Types of Exceptions n Null Pointer Exception Food. Iterator fi = afc. get. All. Food(); while ( !has. Food() && fi. has. Next() { Food f = fi. get. Next. Food(); if (f. get. Location(). equals(my. Loc)) my. Food=f; }
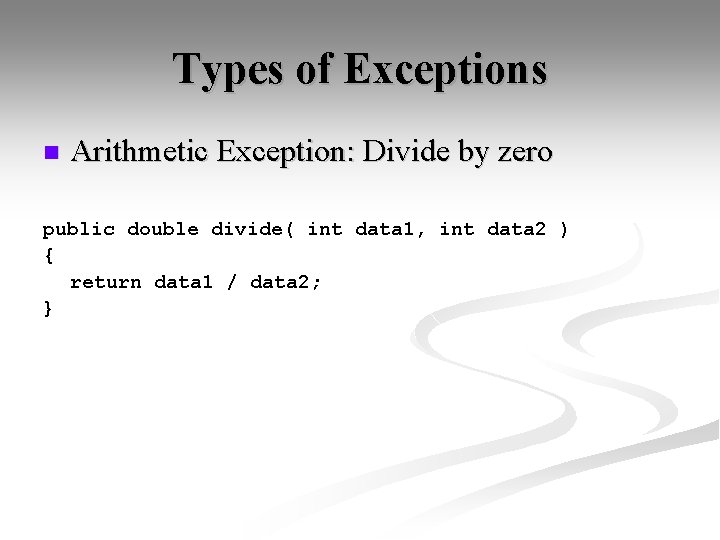
Types of Exceptions n Arithmetic Exception: Divide by zero public double divide( int data 1, int data 2 ) { return data 1 / data 2; }
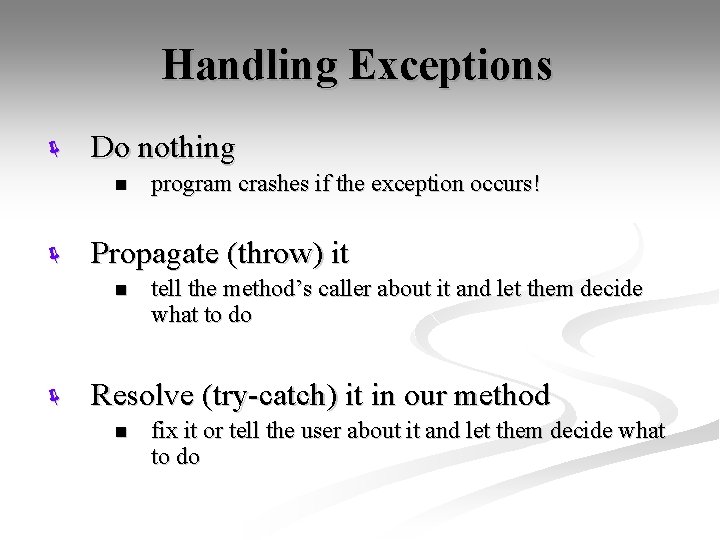
Handling Exceptions ë Do nothing n ë Propagate (throw) it n ë program crashes if the exception occurs! tell the method’s caller about it and let them decide what to do Resolve (try-catch) it in our method n fix it or tell the user about it and let them decide what to do
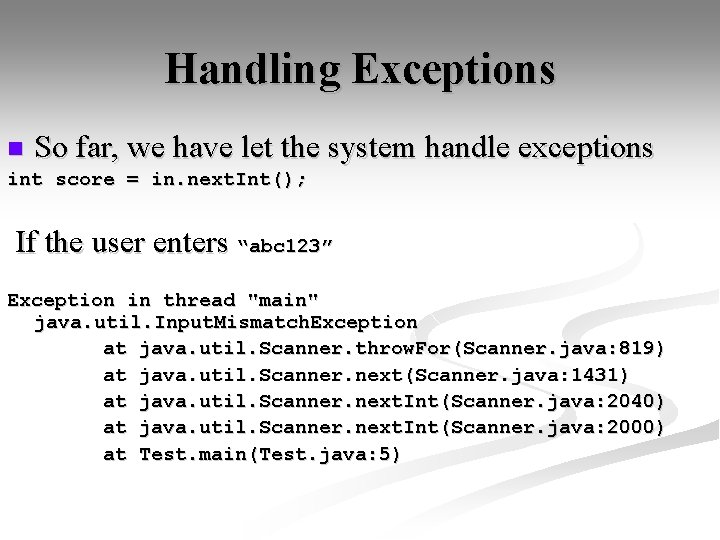
Handling Exceptions n So far, we have let the system handle exceptions int score = in. next. Int(); If the user enters “abc 123” Exception in thread "main" java. util. Input. Mismatch. Exception at java. util. Scanner. throw. For(Scanner. java: 819) at java. util. Scanner. next(Scanner. java: 1431) at java. util. Scanner. next. Int(Scanner. java: 2040) at java. util. Scanner. next. Int(Scanner. java: 2000) at Test. main(Test. java: 5)
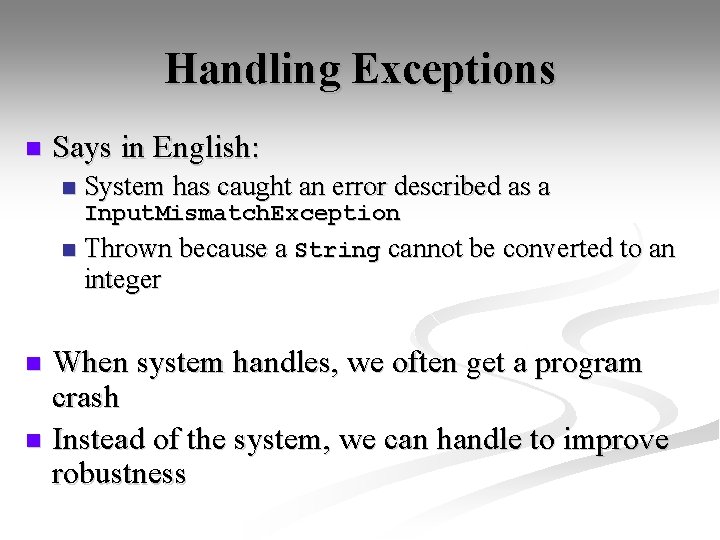
Handling Exceptions n Says in English: n System has caught an error described as a Input. Mismatch. Exception n Thrown because a String cannot be converted to an integer When system handles, we often get a program crash n Instead of the system, we can handle to improve robustness n
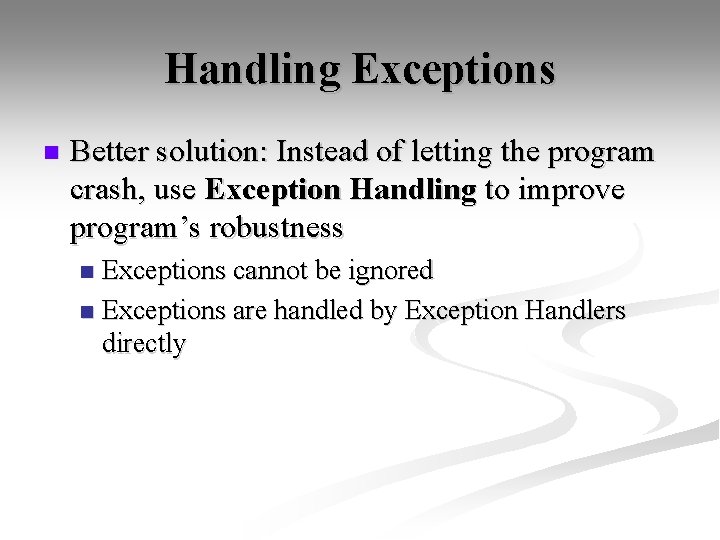
Handling Exceptions n Better solution: Instead of letting the program crash, use Exception Handling to improve program’s robustness Exceptions cannot be ignored n Exceptions are handled by Exception Handlers directly n
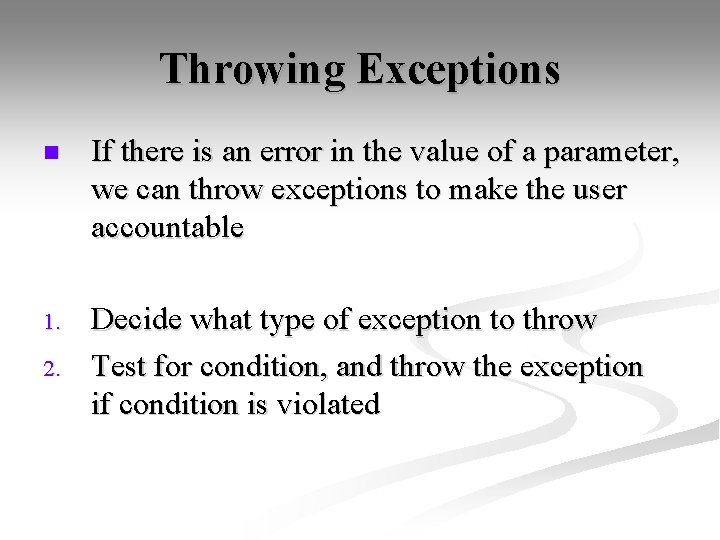
Throwing Exceptions n If there is an error in the value of a parameter, we can throw exceptions to make the user accountable 1. Decide what type of exception to throw Test for condition, and throw the exception if condition is violated 2.
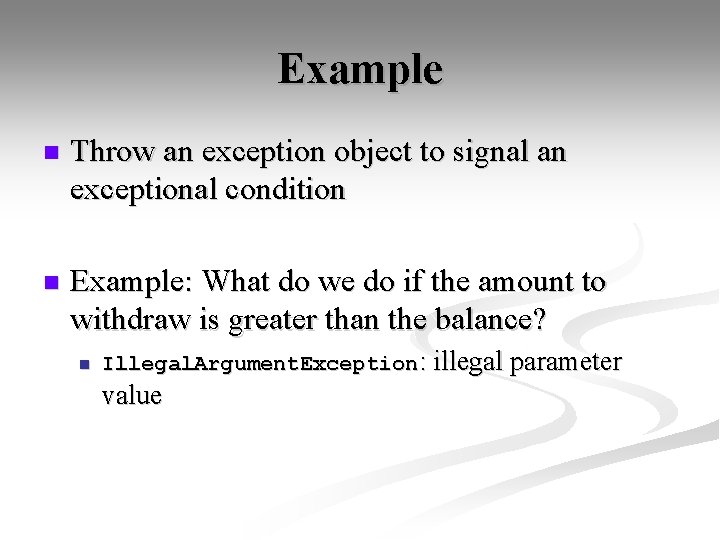
Example n Throw an exception object to signal an exceptional condition n Example: What do we do if the amount to withdraw is greater than the balance? n Illegal. Argument. Exception: illegal parameter value
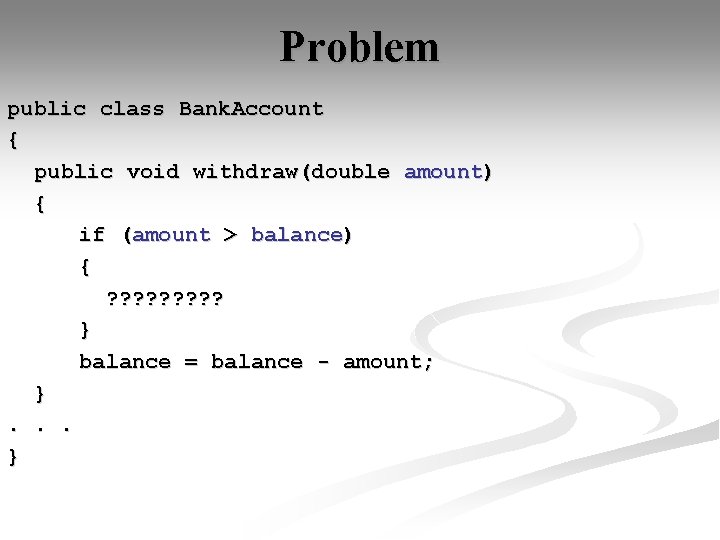
Problem public class Bank. Account { public void withdraw(double amount) { if (amount > balance) { ? ? ? ? ? } balance = balance - amount; }. . . }
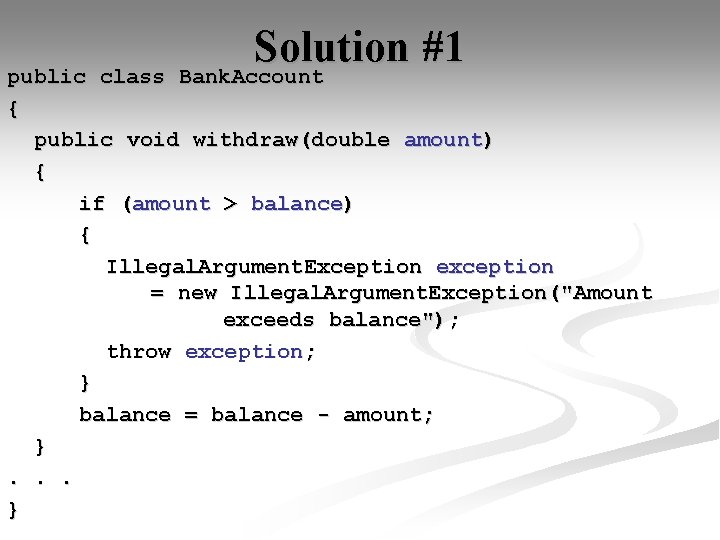
Solution #1 public class Bank. Account { public void withdraw(double amount) { if (amount > balance) { Illegal. Argument. Exception exception = new Illegal. Argument. Exception("Amount exceeds balance"); throw exception; } balance = balance - amount; }. . . }
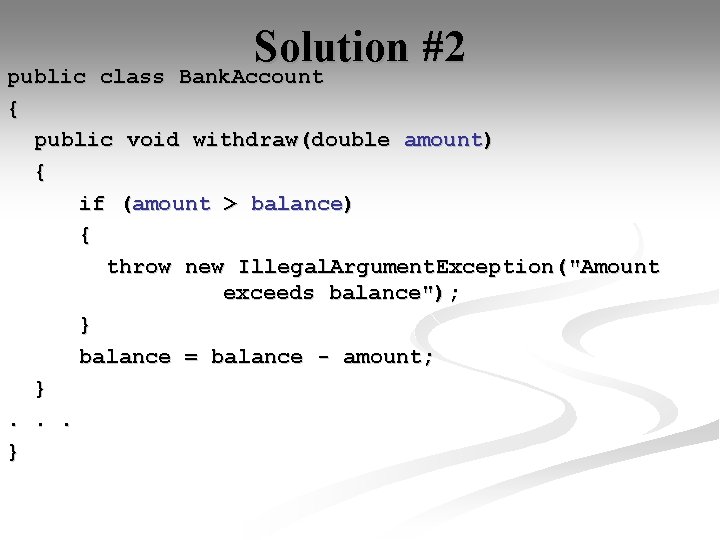
Solution #2 public class Bank. Account { public void withdraw(double amount) { if (amount > balance) { throw new Illegal. Argument. Exception("Amount exceeds balance"); } balance = balance - amount; }. . . }
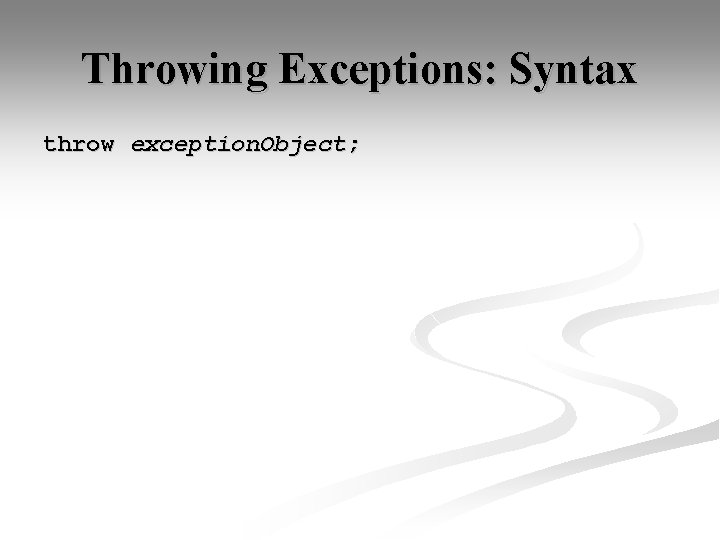
Throwing Exceptions: Syntax throw exception. Object;
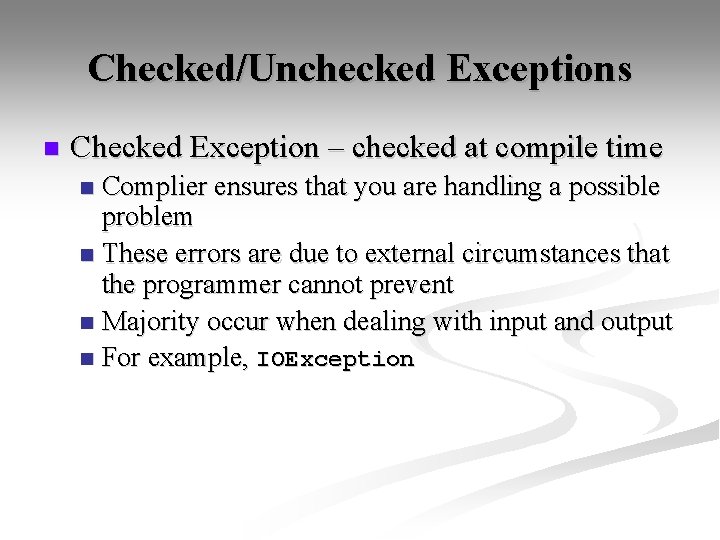
Checked/Unchecked Exceptions n Checked Exception – checked at compile time Complier ensures that you are handling a possible problem n These errors are due to external circumstances that the programmer cannot prevent n Majority occur when dealing with input and output n For example, IOException n
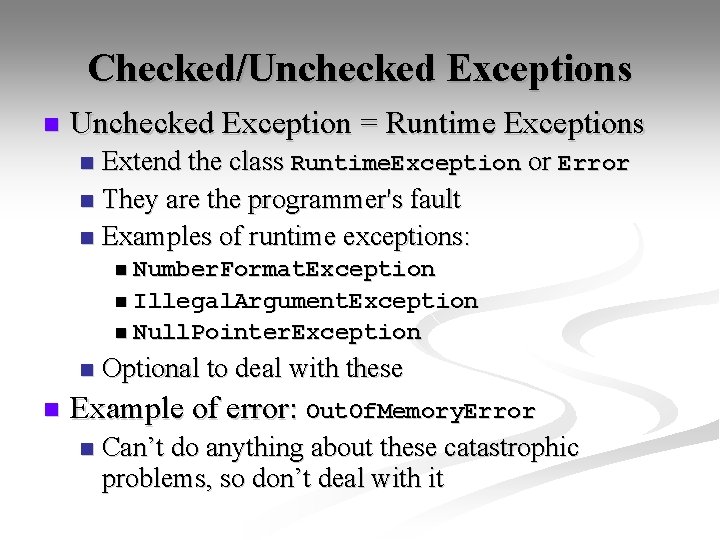
Checked/Unchecked Exceptions n Unchecked Exception = Runtime Exceptions Extend the class Runtime. Exception or Error n They are the programmer's fault n Examples of runtime exceptions: n n Number. Format. Exception n Illegal. Argument. Exception n Null. Pointer. Exception n n Optional to deal with these Example of error: Out. Of. Memory. Error n Can’t do anything about these catastrophic problems, so don’t deal with it
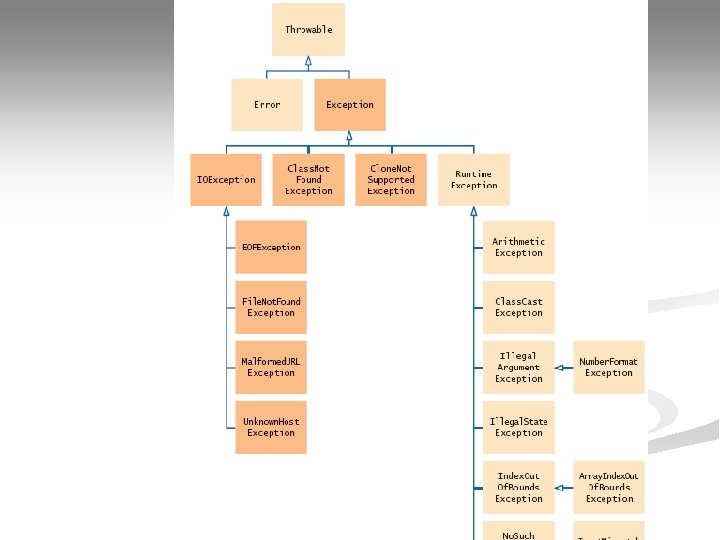
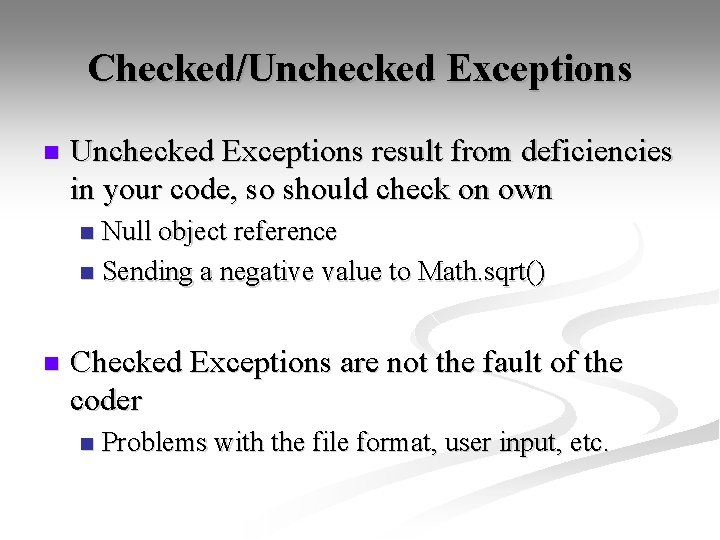
Checked/Unchecked Exceptions n Unchecked Exceptions result from deficiencies in your code, so should check on own Null object reference n Sending a negative value to Math. sqrt() n n Checked Exceptions are not the fault of the coder n Problems with the file format, user input, etc.
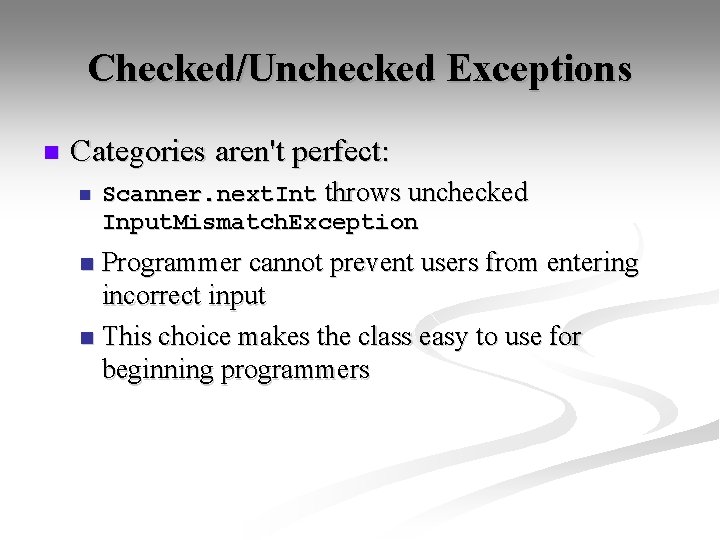
Checked/Unchecked Exceptions n Categories aren't perfect: n Scanner. next. Int throws unchecked Input. Mismatch. Exception Programmer cannot prevent users from entering incorrect input n This choice makes the class easy to use for beginning programmers n
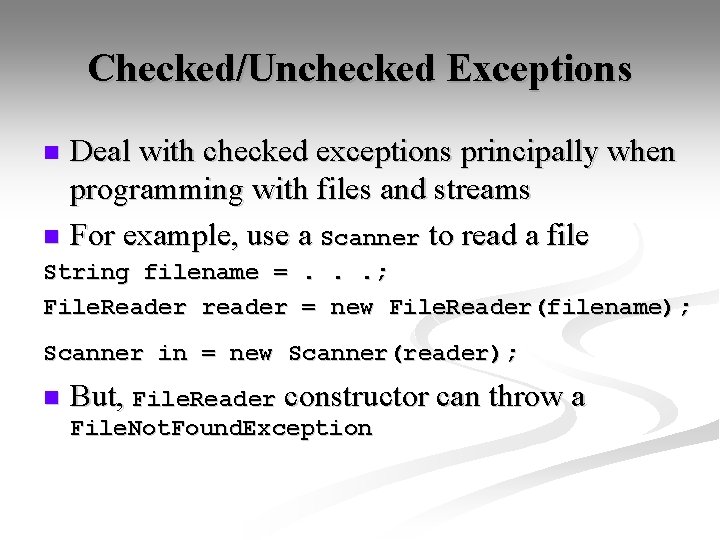
Checked/Unchecked Exceptions Deal with checked exceptions principally when programming with files and streams n For example, use a Scanner to read a file n String filename =. . . ; File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); n But, File. Reader constructor can throw a File. Not. Found. Exception
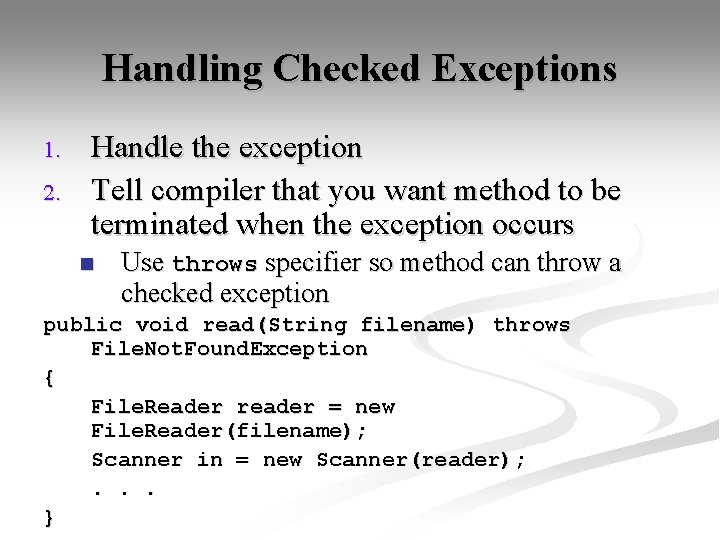
Handling Checked Exceptions 1. 2. Handle the exception Tell compiler that you want method to be terminated when the exception occurs n Use throws specifier so method can throw a checked exception public void read(String filename) throws File. Not. Found. Exception { File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); . . . }
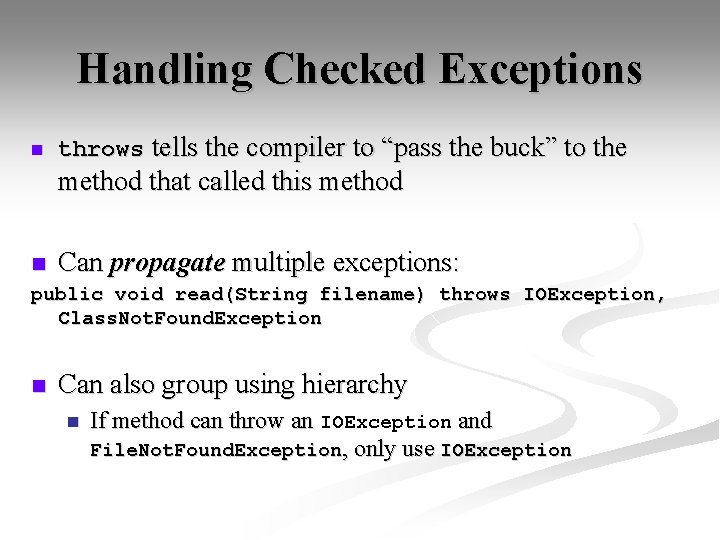
Handling Checked Exceptions n throws tells the compiler to “pass the buck” to the method that called this method n Can propagate multiple exceptions: public void read(String filename) throws IOException, Class. Not. Found. Exception n Can also group using hierarchy n If method can throw an IOException and File. Not. Found. Exception, only use IOException
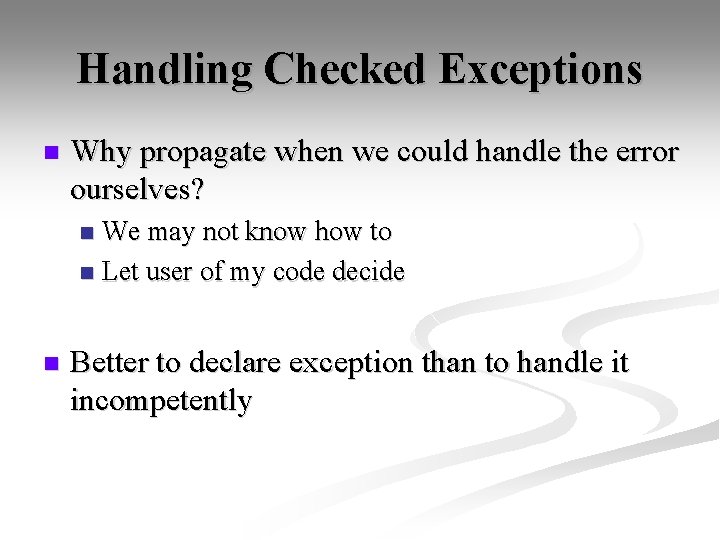
Handling Checked Exceptions n Why propagate when we could handle the error ourselves? We may not know how to n Let user of my code decide n n Better to declare exception than to handle it incompetently
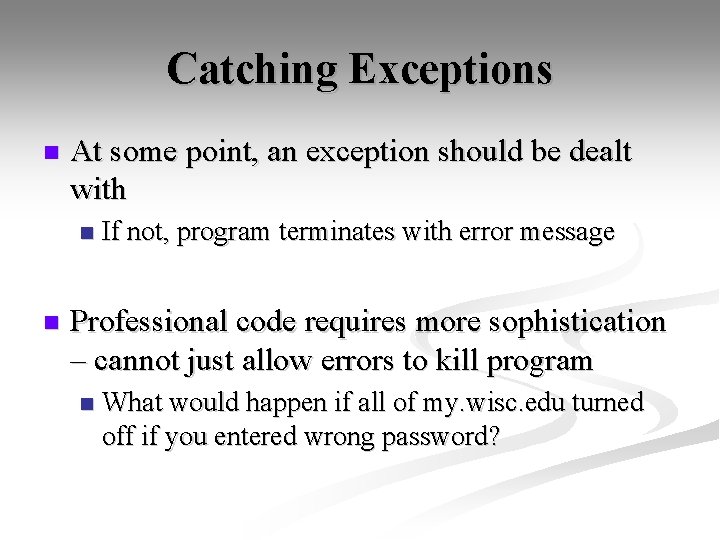
Catching Exceptions n At some point, an exception should be dealt with n n If not, program terminates with error message Professional code requires more sophistication – cannot just allow errors to kill program n What would happen if all of my. wisc. edu turned off if you entered wrong password?
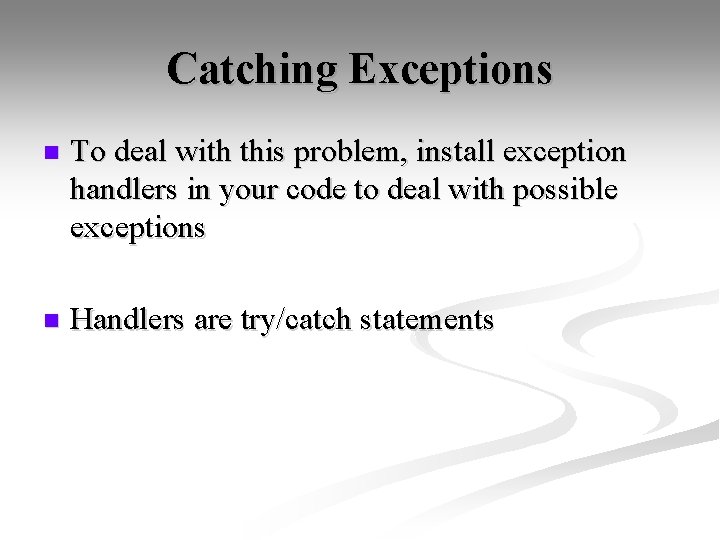
Catching Exceptions n To deal with this problem, install exception handlers in your code to deal with possible exceptions n Handlers are try/catch statements
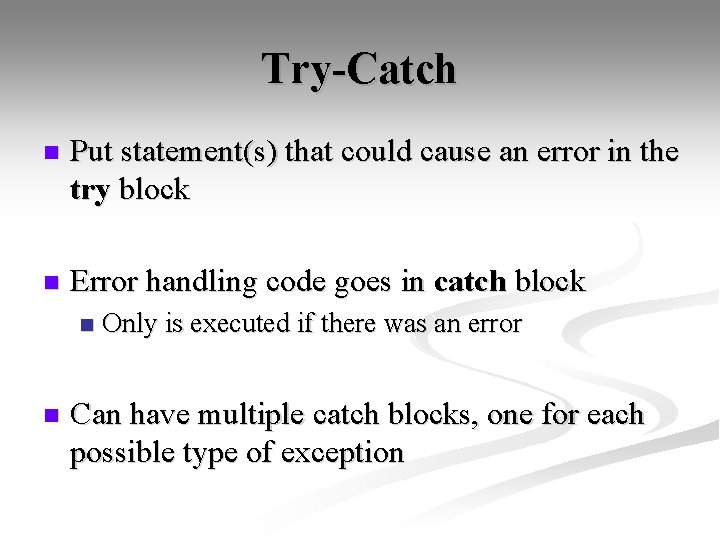
Try-Catch n Put statement(s) that could cause an error in the try block n Error handling code goes in catch block n n Only is executed if there was an error Can have multiple catch blocks, one for each possible type of exception
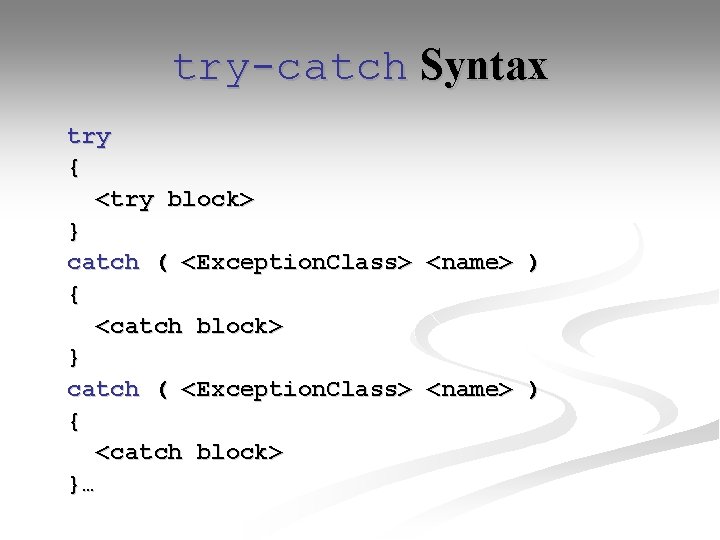
try-catch Syntax try { <try block> } catch ( <Exception. Class> <name> ) { <catch block> }…
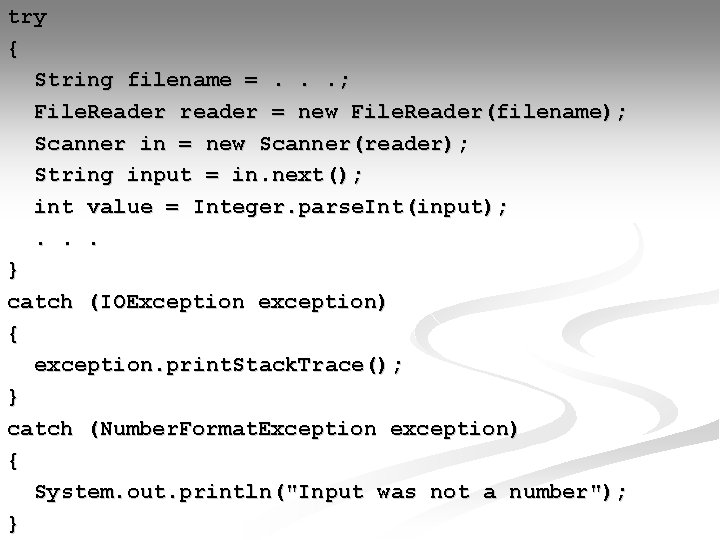
try { String filename =. . . ; File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); String input = in. next(); int value = Integer. parse. Int(input); . . . } catch (IOException exception) { exception. print. Stack. Trace(); } catch (Number. Format. Exception exception) { System. out. println("Input was not a number"); }
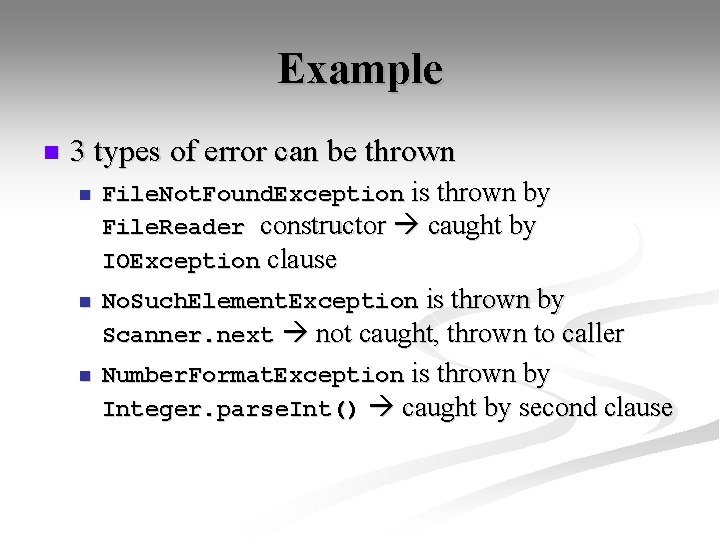
Example n 3 types of error can be thrown n File. Not. Found. Exception is thrown by File. Reader constructor caught by IOException clause No. Such. Element. Exception is thrown by Scanner. next not caught, thrown to caller Number. Format. Exception is thrown by Integer. parse. Int() caught by second clause
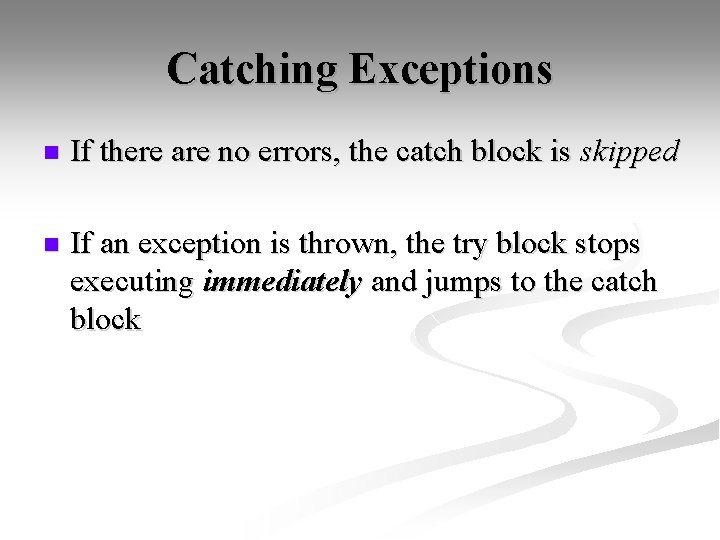
Catching Exceptions n If there are no errors, the catch block is skipped n If an exception is thrown, the try block stops executing immediately and jumps to the catch block
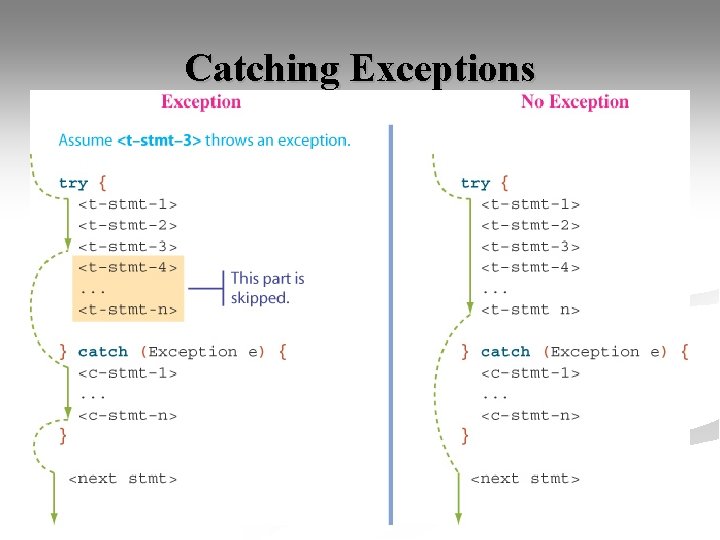
Catching Exceptions
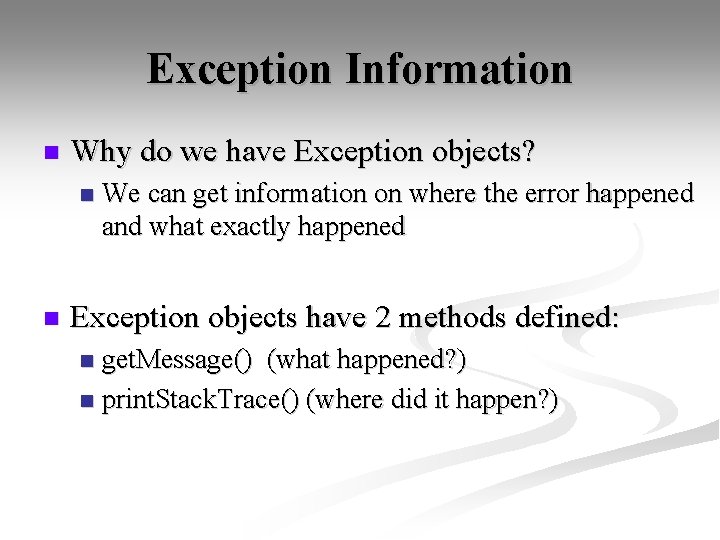
Exception Information n Why do we have Exception objects? n n We can get information on where the error happened and what exactly happened Exception objects have 2 methods defined: get. Message() (what happened? ) n print. Stack. Trace() (where did it happen? ) n
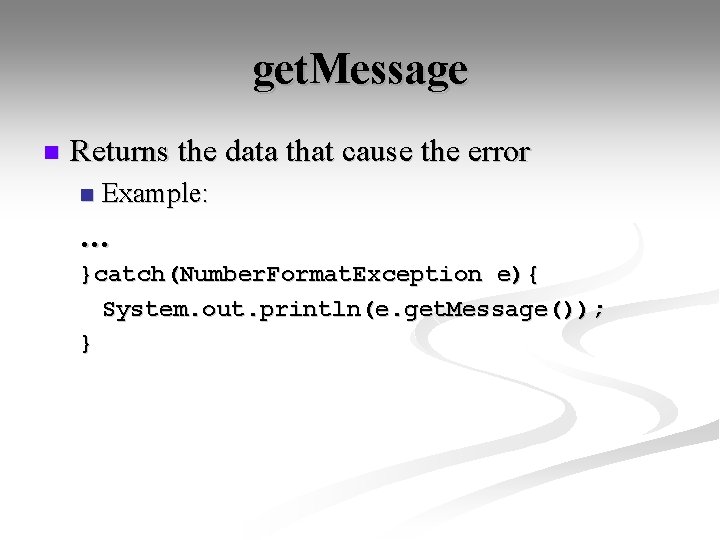
get. Message n Returns the data that cause the error n Example: … }catch(Number. Format. Exception e){ System. out. println(e. get. Message()); }
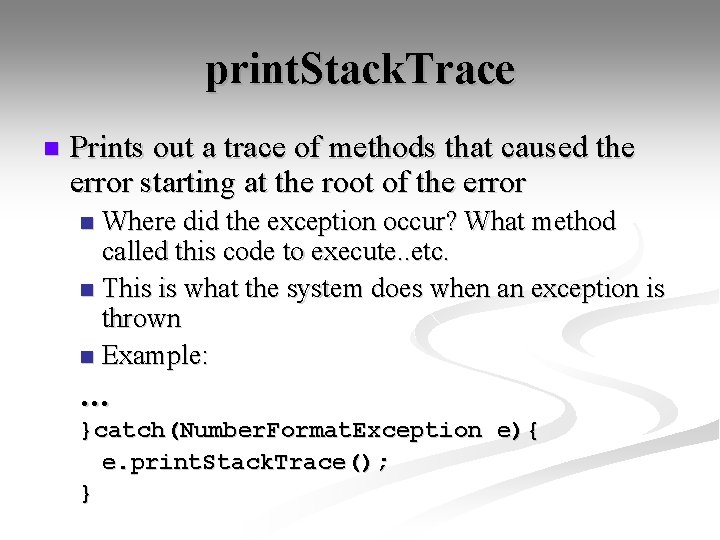
print. Stack. Trace n Prints out a trace of methods that caused the error starting at the root of the error Where did the exception occur? What method called this code to execute. . etc. n This is what the system does when an exception is thrown n Example: n … }catch(Number. Format. Exception e){ e. print. Stack. Trace(); }
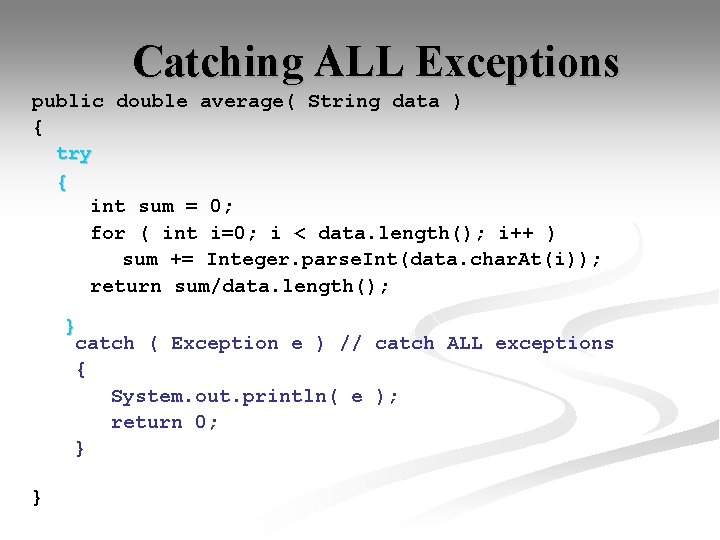
Catching ALL Exceptions public double average( String data ) { try { int sum = 0; for ( int i=0; i < data. length(); i++ ) sum += Integer. parse. Int(data. char. At(i)); return sum/data. length(); } catch ( Exception e ) // catch ALL exceptions { System. out. println( e ); return 0; } }
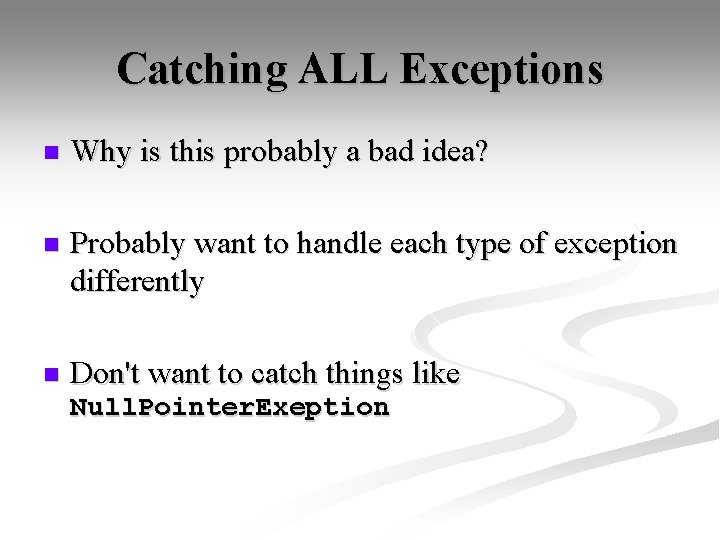
Catching ALL Exceptions n Why is this probably a bad idea? n Probably want to handle each type of exception differently n Don't want to catch things like Null. Pointer. Exeption
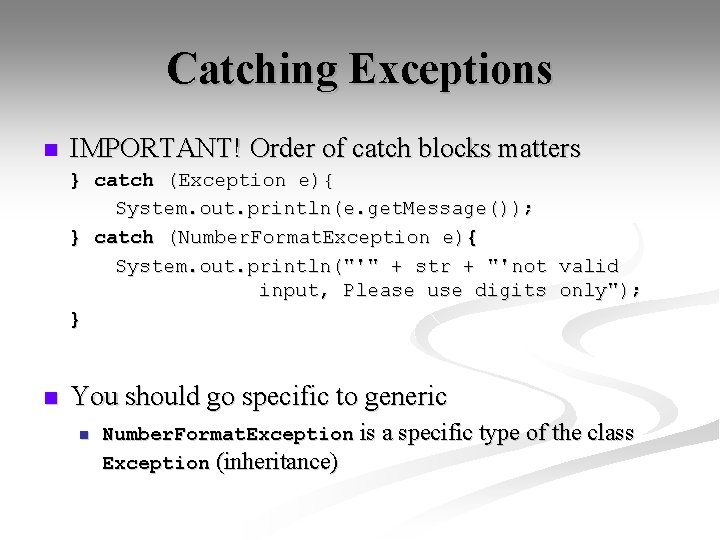
Catching Exceptions n IMPORTANT! Order of catch blocks matters } catch (Exception e){ System. out. println(e. get. Message()); } catch (Number. Format. Exception e){ System. out. println("'" + str + "'not valid input, Please use digits only"); } n You should go specific to generic n Number. Format. Exception is a specific type of the class Exception (inheritance)
![Demo p s v main String args try String filename Demo p. s. v. main( String [] args ) { try { String filename](https://slidetodoc.com/presentation_image_h/3612230ebfc9ea60189c6cdcd0375289/image-44.jpg)
Demo p. s. v. main( String [] args ) { try { String filename = args[0], input; scanner = new Scanner( new File(filename) ); input = scanner. next. Line(); int value = Integer. parse. Int( input ); double reciprocal = 1. 0 / value; System. out. println( "rec=" + rec ); } } catch ( Exception e ) { S. o. pln( "Found Exception: " + e ); } S. o. pln( "Done" );
![String filenameargs0 input try scanner new Scannernew Filefilename input scanner next String filename=args[0], input; try { scanner = new Scanner(new File(filename)); input = scanner. next.](https://slidetodoc.com/presentation_image_h/3612230ebfc9ea60189c6cdcd0375289/image-45.jpg)
String filename=args[0], input; try { scanner = new Scanner(new File(filename)); input = scanner. next. Line(); int value = Integer. parse. Int( input ); double reciprocal = 1. 0 / value; System. out. println( "rec=" + rec ); } catch ( Number. Format. Exception e ) { S. o. pln( input + " is not an integer"); } catch ( Arithmetic. Exception e ) { S. o. pln( "Can't divide by zero" ); } catch ( File. Not. Found. Exception e ) { S. o. pln( filename + " Not Found" ); } finally { S. o. pln( "Done" ); }
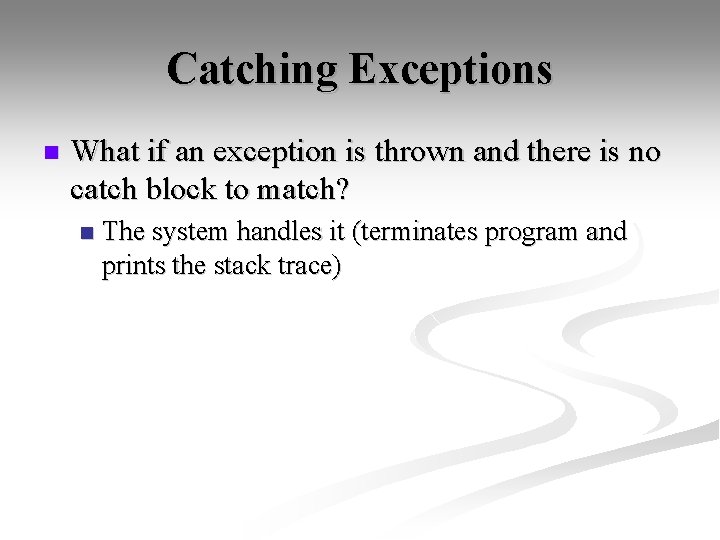
Catching Exceptions n What if an exception is thrown and there is no catch block to match? n The system handles it (terminates program and prints the stack trace)
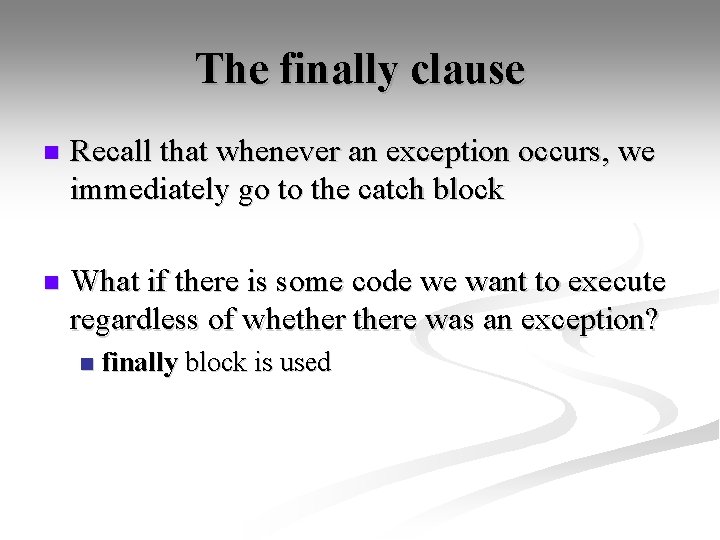
The finally clause n Recall that whenever an exception occurs, we immediately go to the catch block n What if there is some code we want to execute regardless of whethere was an exception? n finally block is used
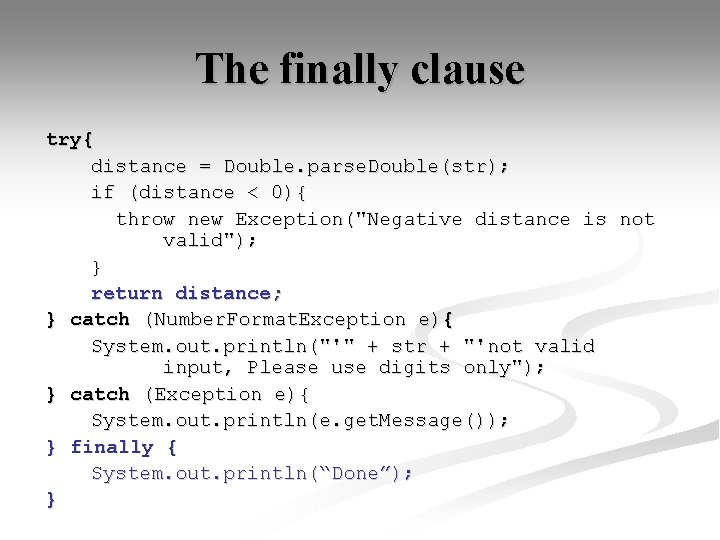
The finally clause try{ distance = Double. parse. Double(str); if (distance < 0){ throw new Exception("Negative distance is not valid"); } return distance; } catch (Number. Format. Exception e){ System. out. println("'" + str + "'not valid input, Please use digits only"); } catch (Exception e){ System. out. println(e. get. Message()); } finally { System. out. println(“Done”); }
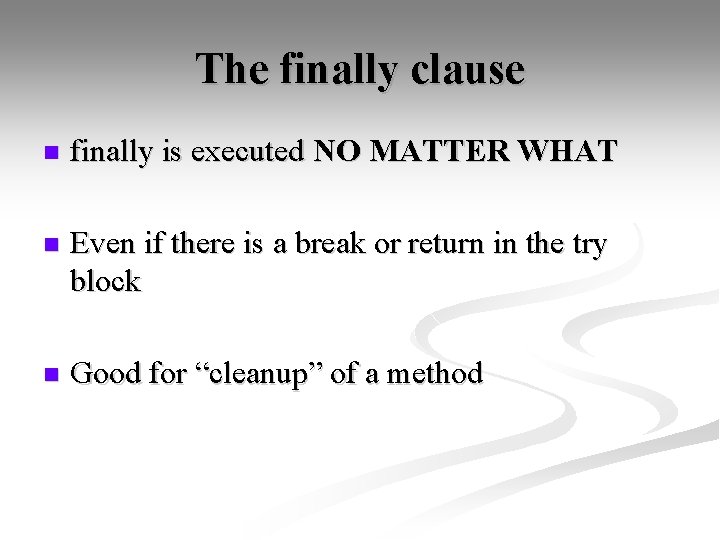
The finally clause n finally is executed NO MATTER WHAT n Even if there is a break or return in the try block n Good for “cleanup” of a method
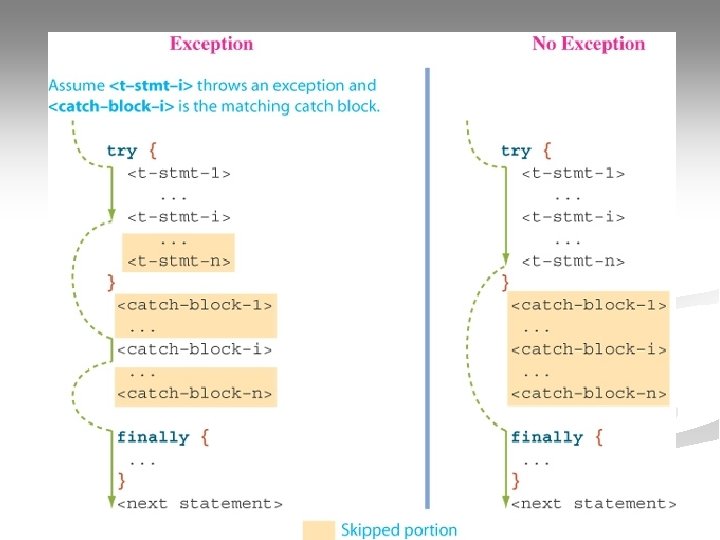
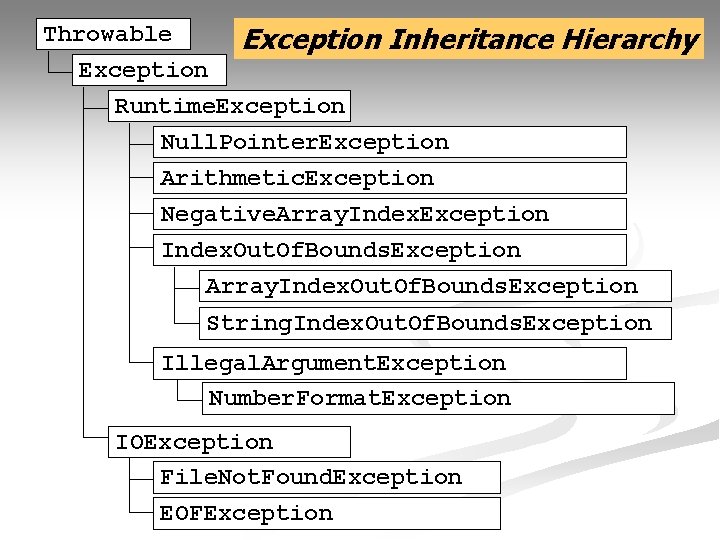
Throwable Exception Inheritance Hierarchy Runtime. Exception Null. Pointer. Exception Arithmetic. Exception Negative. Array. Index. Exception Index. Out. Of. Bounds. Exception Array. Index. Out. Of. Bounds. Exception String. Index. Out. Of. Bounds. Exception Illegal. Argument. Exception Number. Format. Exception IOException File. Not. Found. Exception EOFException
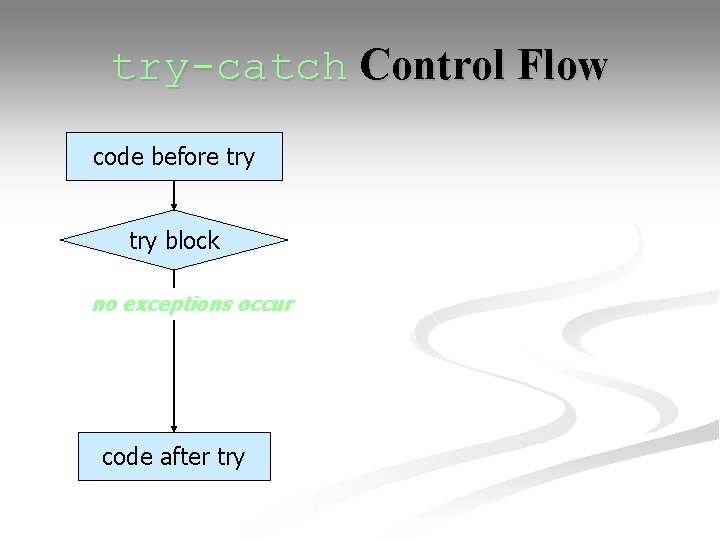
try-catch Control Flow code before try block no exceptions occur code after try
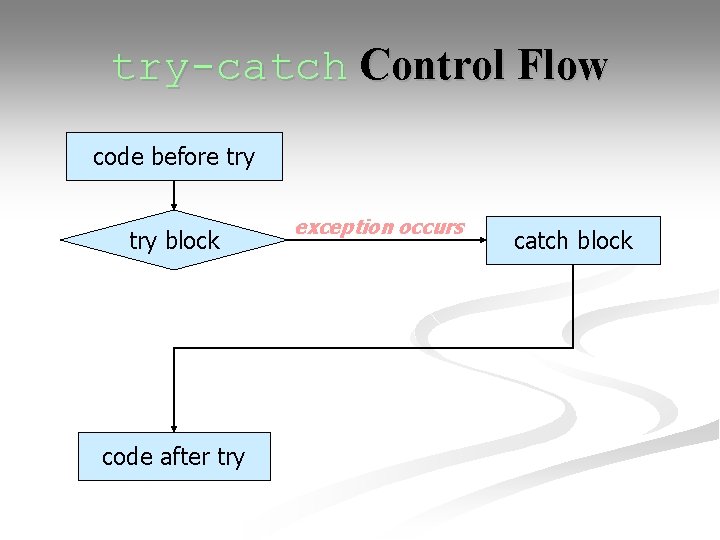
try-catch Control Flow code before try block code after try exception occurs catch block
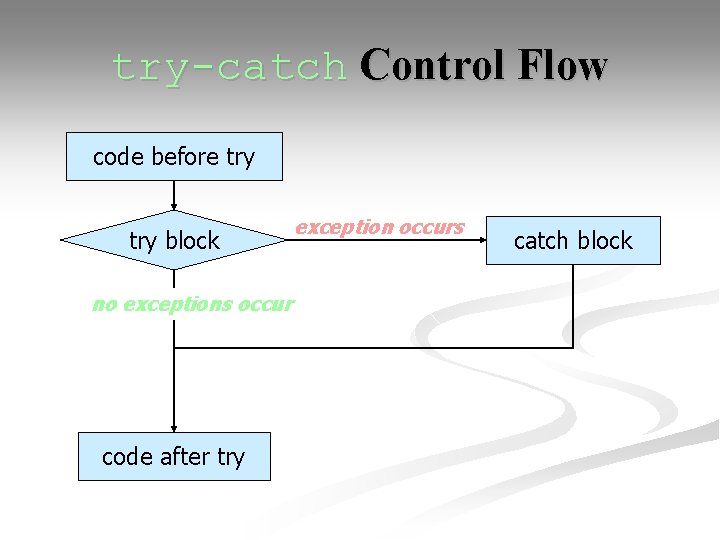
try-catch Control Flow code before try block no exceptions occur code after try exception occurs catch block
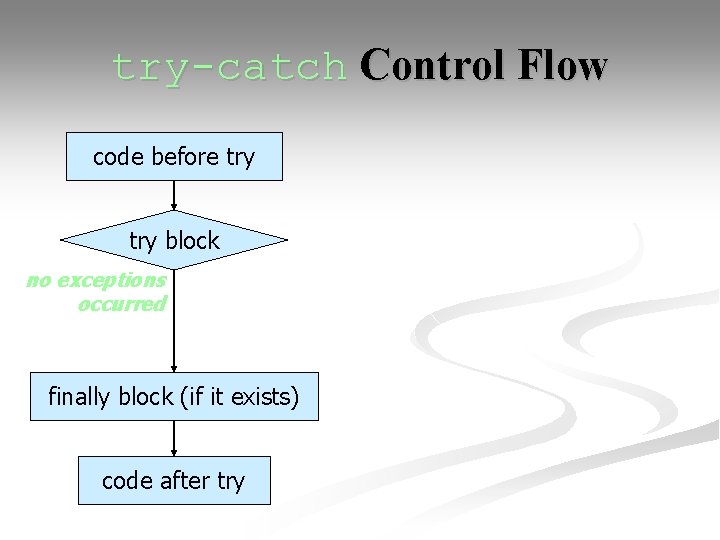
try-catch Control Flow code before try block no exceptions occurred finally block (if it exists) code after try
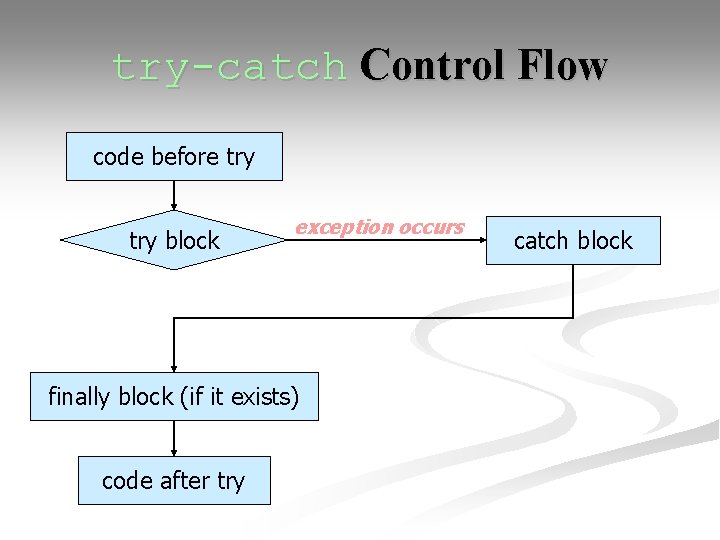
try-catch Control Flow code before try block exception occurs finally block (if it exists) code after try catch block
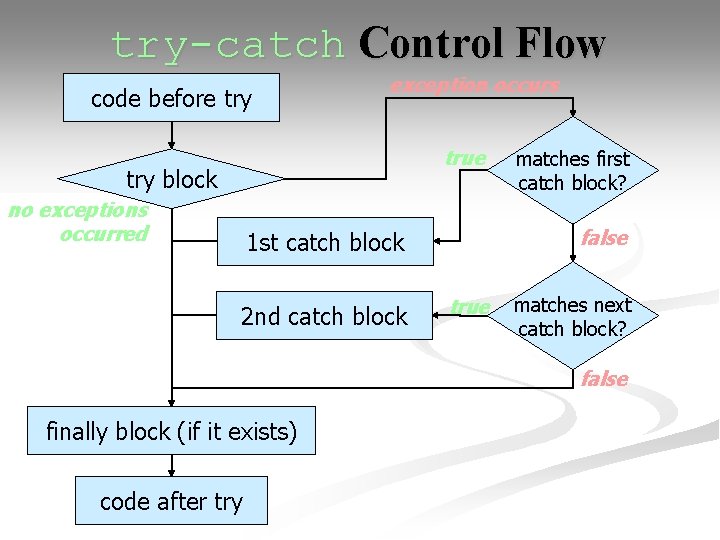
try-catch Control Flow code before try exception occurs true try block no exceptions occurred false 1 st catch block 2 nd catch block matches first catch block? true matches next catch block? false finally block (if it exists) code after try
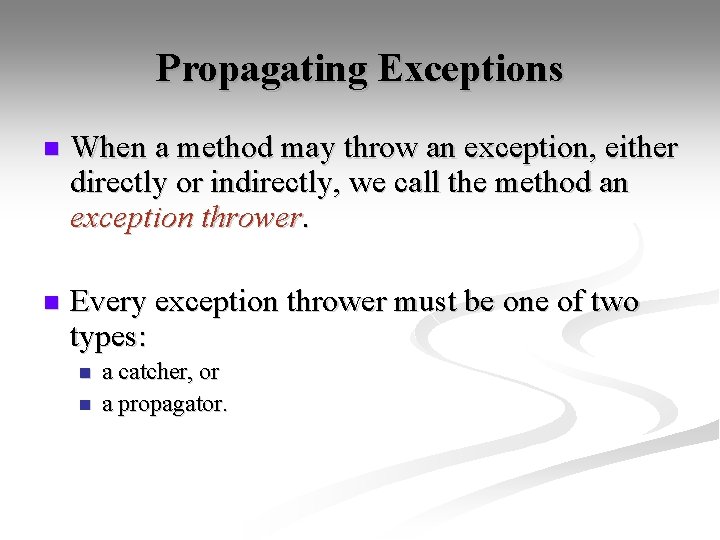
Propagating Exceptions n When a method may throw an exception, either directly or indirectly, we call the method an exception thrower. n Every exception thrower must be one of two types: n n a catcher, or a propagator.
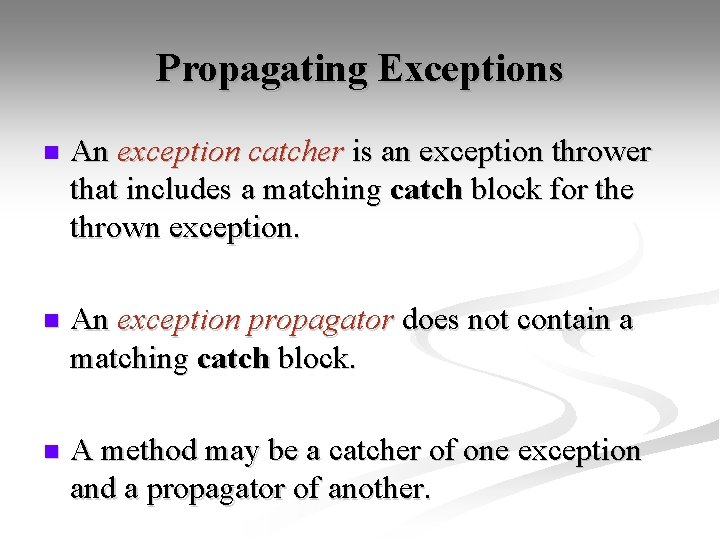
Propagating Exceptions n An exception catcher is an exception thrower that includes a matching catch block for the thrown exception. n An exception propagator does not contain a matching catch block. n A method may be a catcher of one exception and a propagator of another.
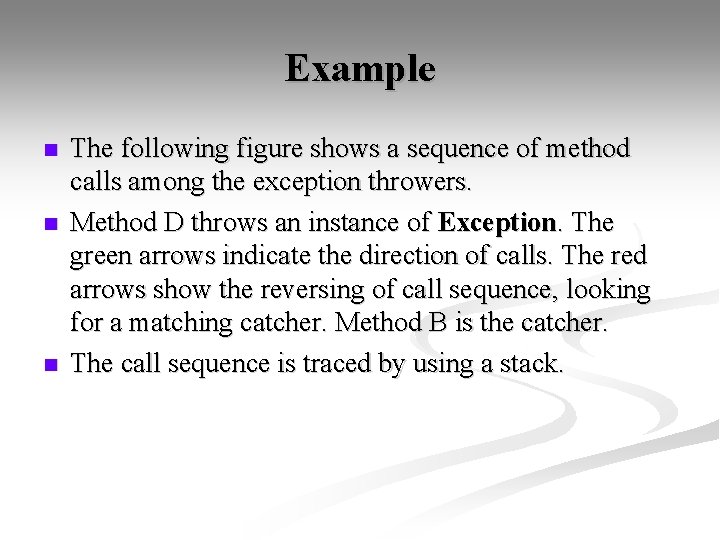
Example n n n The following figure shows a sequence of method calls among the exception throwers. Method D throws an instance of Exception. The green arrows indicate the direction of calls. The red arrows show the reversing of call sequence, looking for a matching catcher. Method B is the catcher. The call sequence is traced by using a stack.
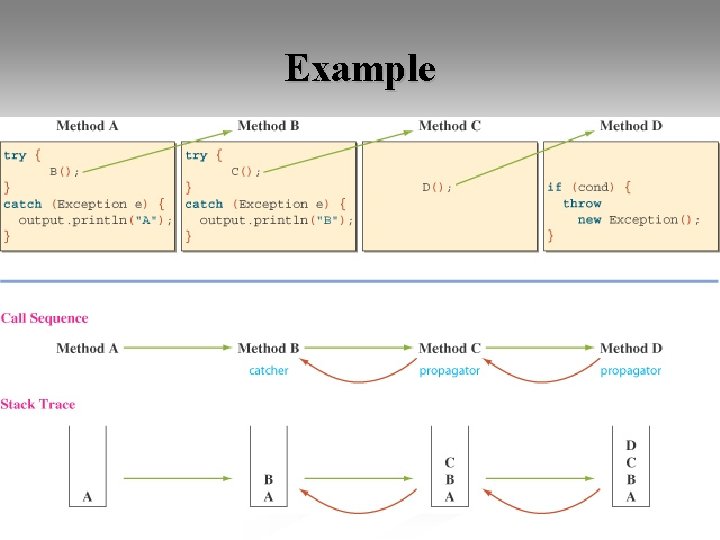
Example
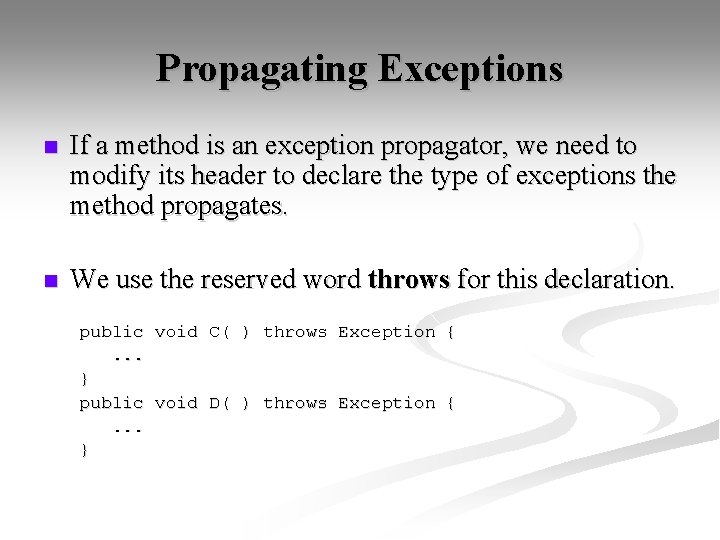
Propagating Exceptions n If a method is an exception propagator, we need to modify its header to declare the type of exceptions the method propagates. n We use the reserved word throws for this declaration. public void C( ) throws Exception {. . . } public void D( ) throws Exception {. . . }
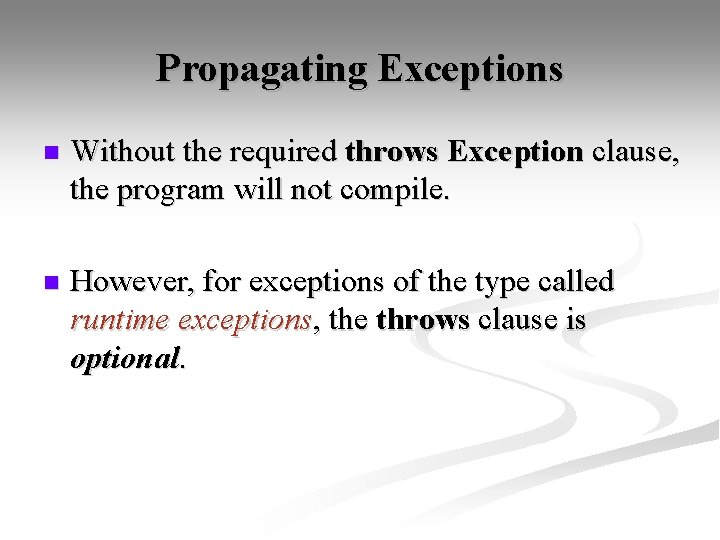
Propagating Exceptions n Without the required throws Exception clause, the program will not compile. n However, for exceptions of the type called runtime exceptions, the throws clause is optional.
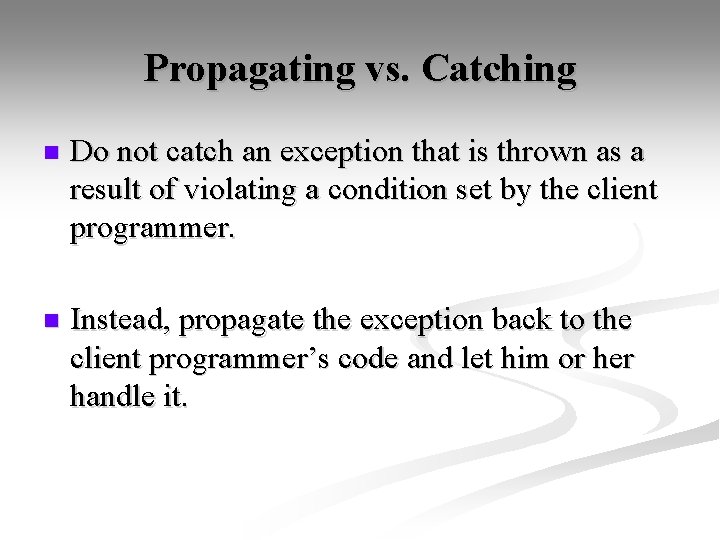
Propagating vs. Catching n Do not catch an exception that is thrown as a result of violating a condition set by the client programmer. n Instead, propagate the exception back to the client programmer’s code and let him or her handle it.
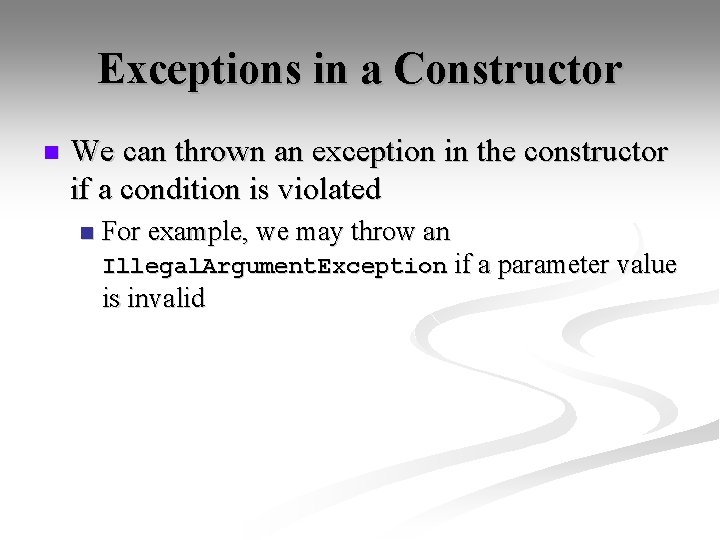
Exceptions in a Constructor n We can thrown an exception in the constructor if a condition is violated n For example, we may throw an Illegal. Argument. Exception if a parameter value is invalid