Data Type how to view it Specification View
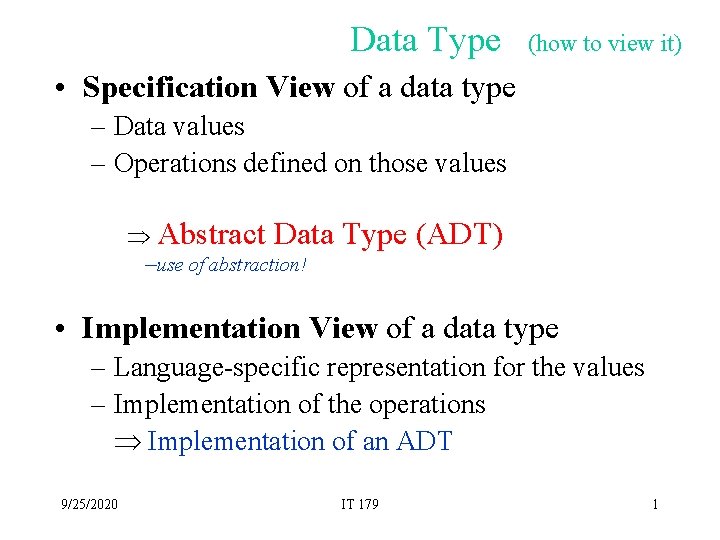
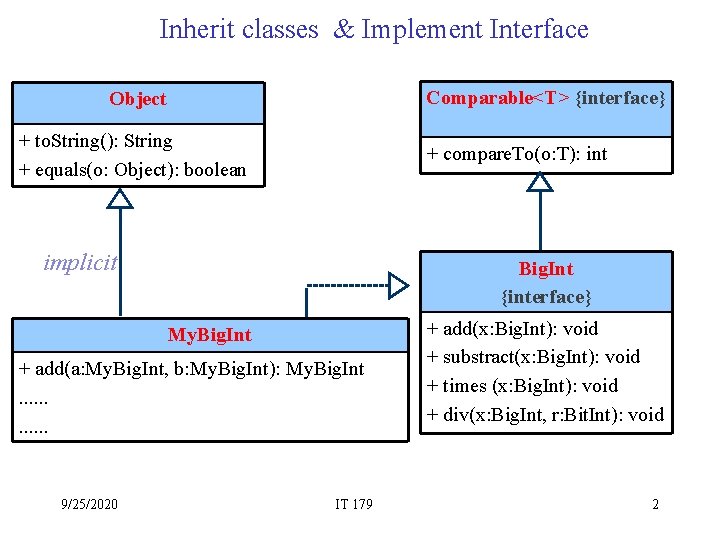
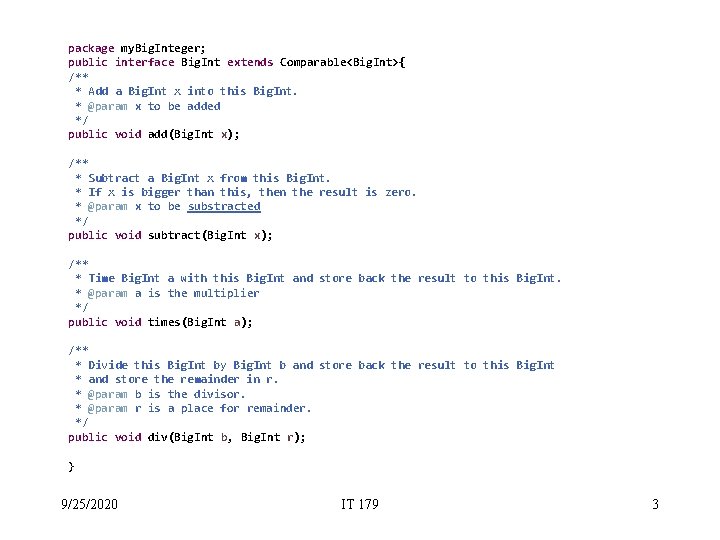
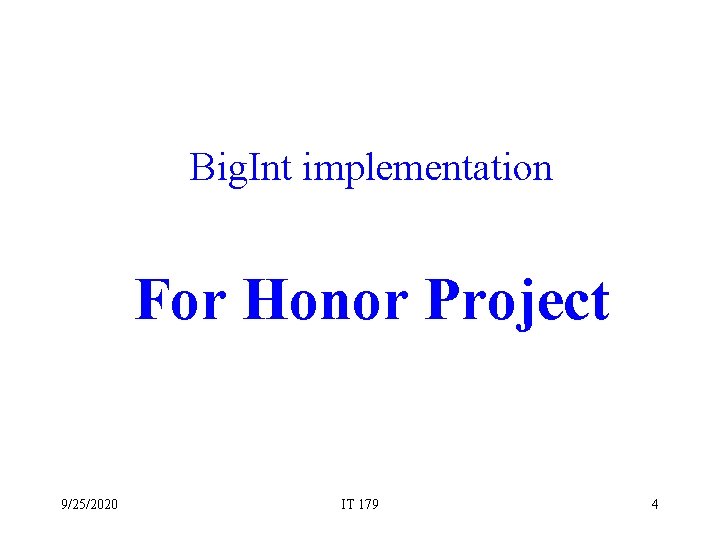
![Inside the Array. List<T> Array. List< > array [0] public int size(); [1] public Inside the Array. List<T> Array. List< > array [0] public int size(); [1] public](https://slidetodoc.com/presentation_image/857bfd8f0cddb17661f42f4394f8a833/image-5.jpg)
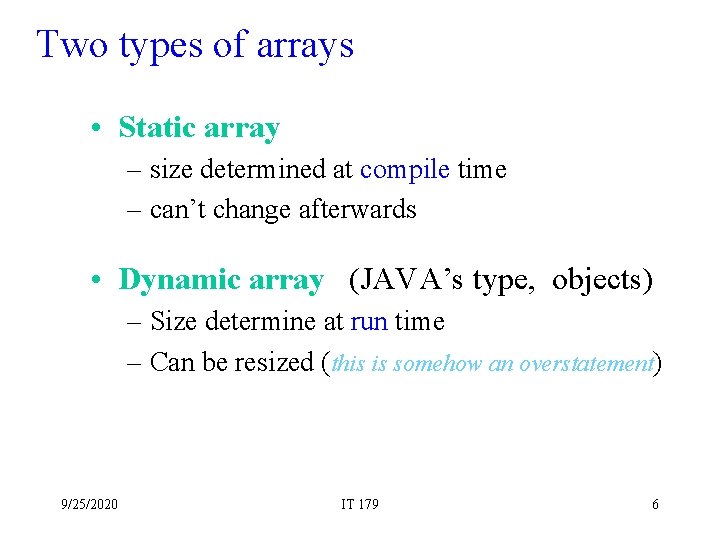
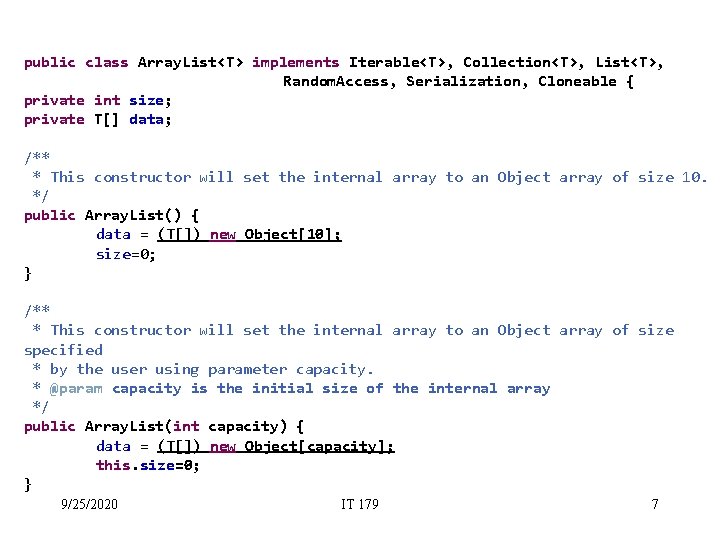
![public int index. Of(Object item) { for (int i=0; i<size; i++) if (data[i]. equals(item)) public int index. Of(Object item) { for (int i=0; i<size; i++) if (data[i]. equals(item))](https://slidetodoc.com/presentation_image/857bfd8f0cddb17661f42f4394f8a833/image-8.jpg)
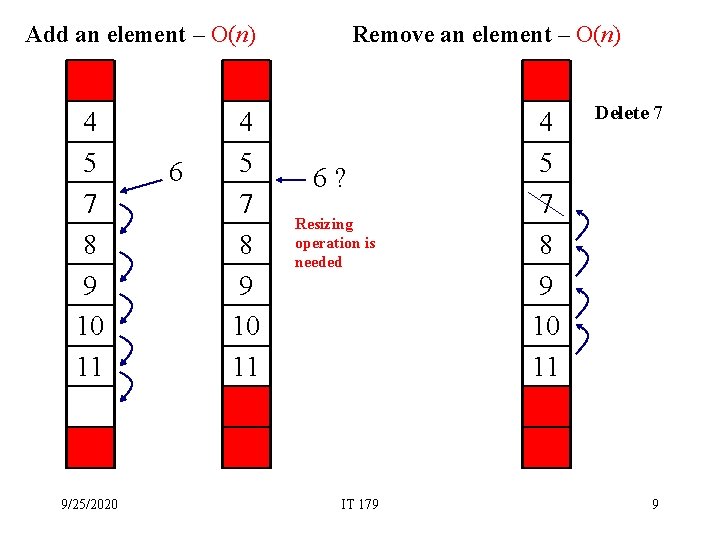
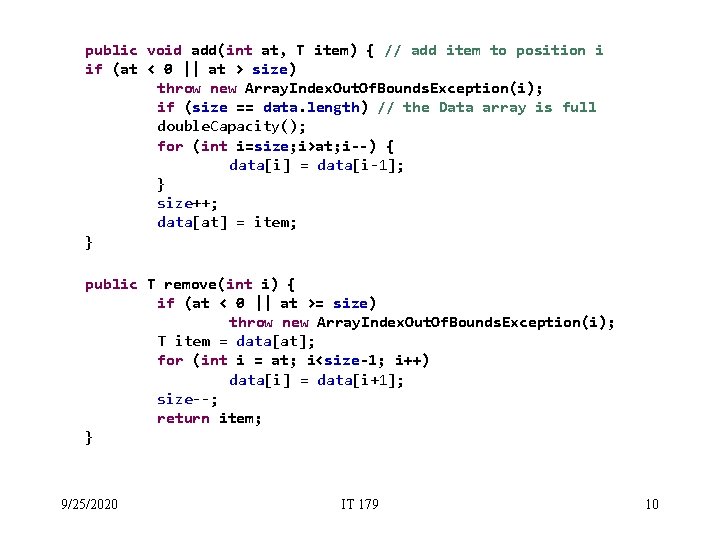
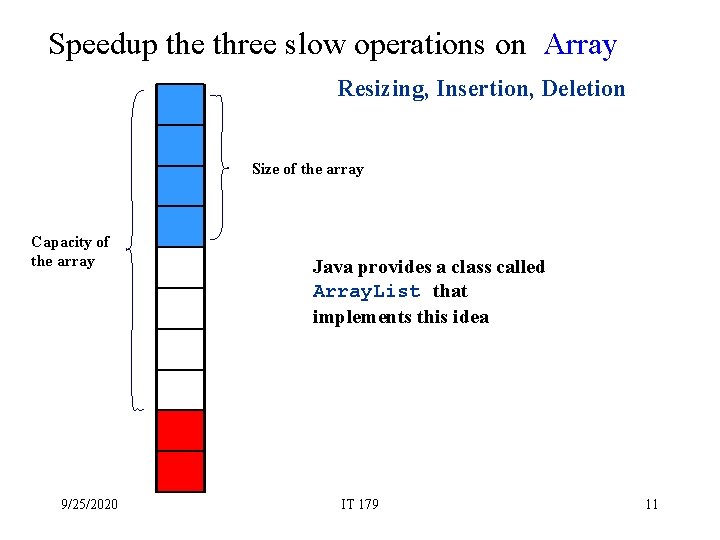
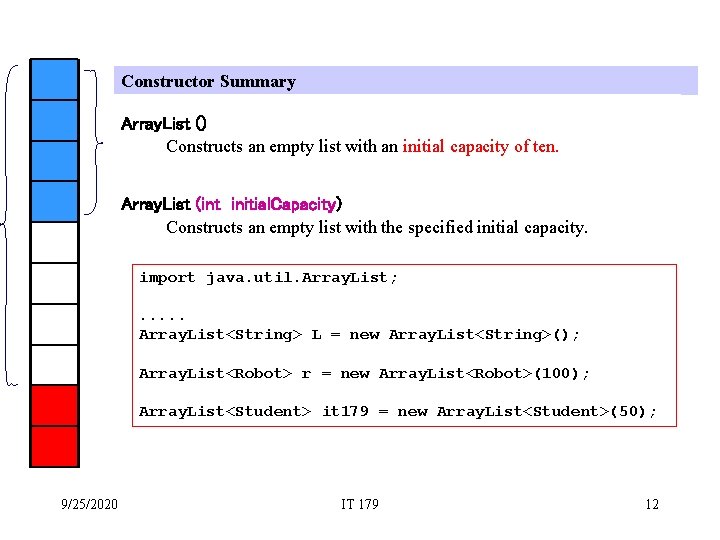
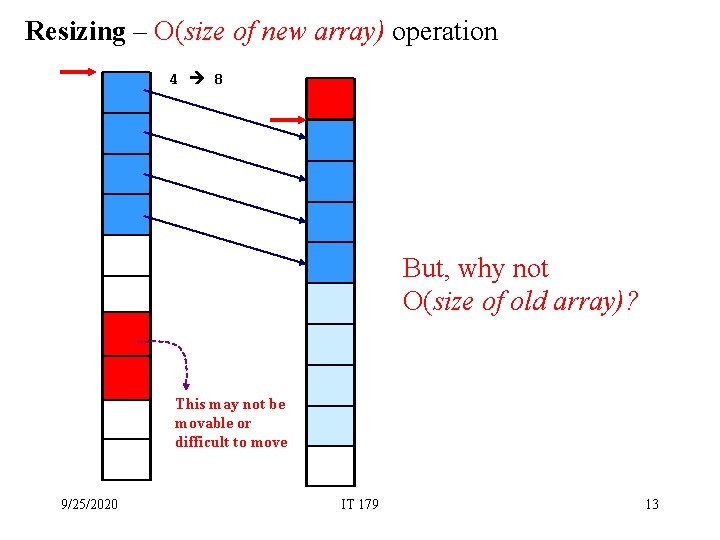
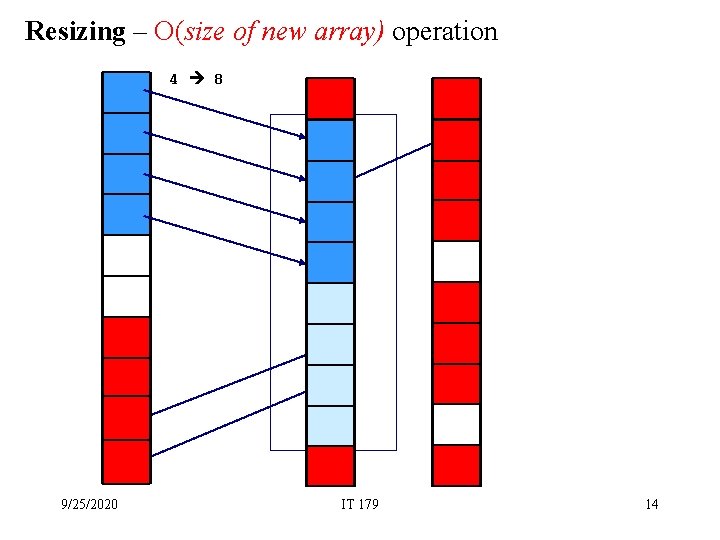
![private void double. Capacity() { int c = data. length*2; T[] new. List = private void double. Capacity() { int c = data. length*2; T[] new. List =](https://slidetodoc.com/presentation_image/857bfd8f0cddb17661f42f4394f8a833/image-15.jpg)
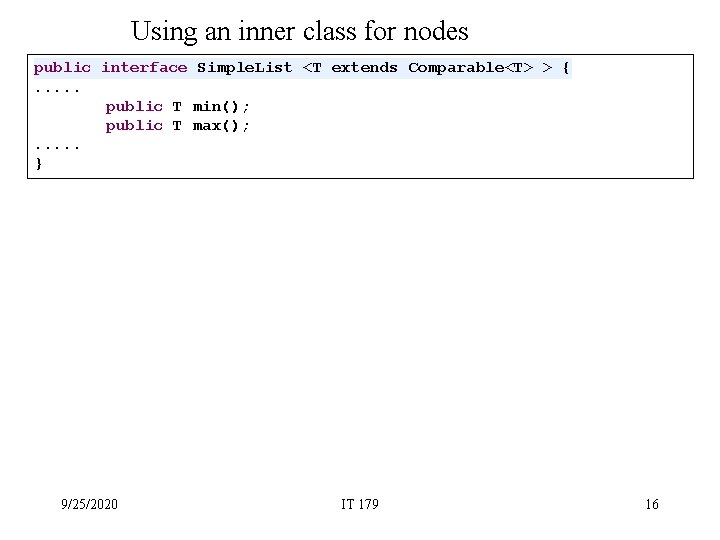
- Slides: 16
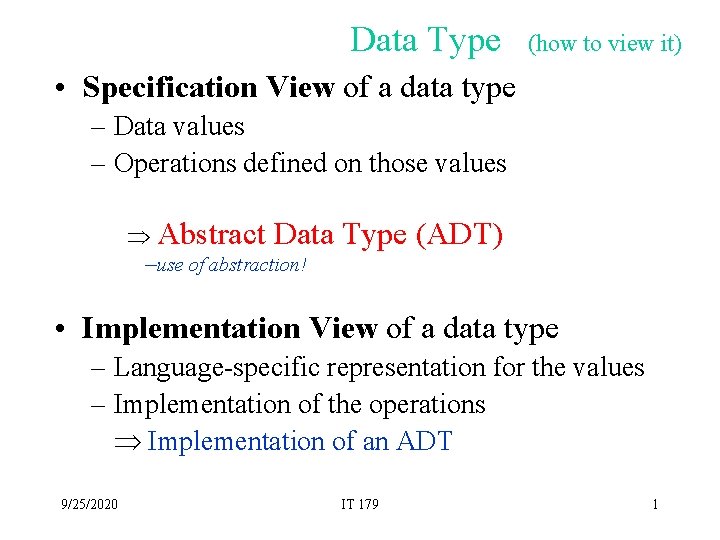
Data Type (how to view it) • Specification View of a data type – Data values – Operations defined on those values Abstract Data Type (ADT) –use of abstraction! • Implementation View of a data type – Language-specific representation for the values – Implementation of the operations Implementation of an ADT 9/25/2020 IT 179 1
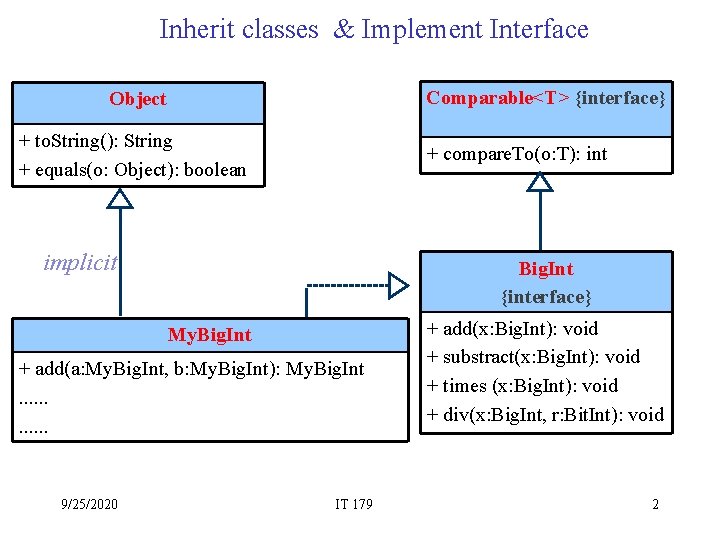
Inherit classes & Implement Interface Comparable<T> {interface} Object + to. String(): String + equals(o: Object): boolean + compare. To(o: T): int implicit Big. Int {interface} My. Big. Int + add(a: My. Big. Int, b: My. Big. Int): My. Big. Int. . . 9/25/2020 IT 179 + add(x: Big. Int): void + substract(x: Big. Int): void + times (x: Big. Int): void + div(x: Big. Int, r: Bit. Int): void 2
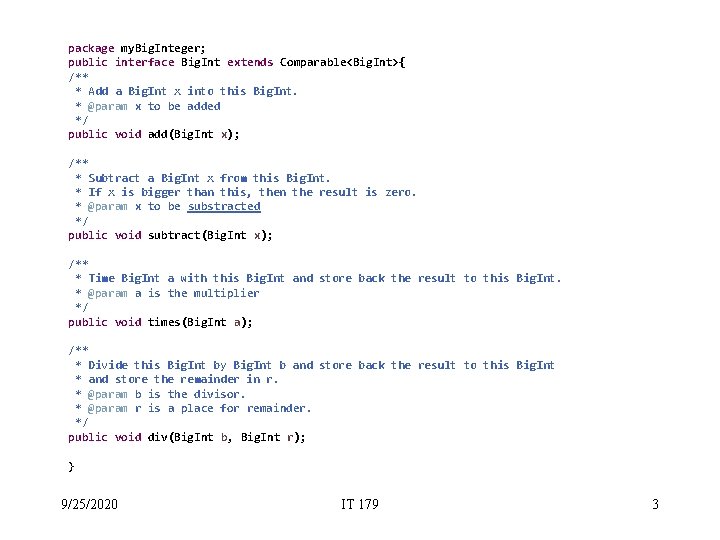
package my. Big. Integer; public interface Big. Int extends Comparable<Big. Int>{ /** * Add a Big. Int x into this Big. Int. * @param x to be added */ public void add(Big. Int x); /** * Subtract a Big. Int x from this Big. Int. * If x is bigger than this, then the result is zero. * @param x to be substracted */ public void subtract(Big. Int x); /** * Time Big. Int a with this Big. Int and store back the result to this Big. Int. * @param a is the multiplier */ public void times(Big. Int a); /** * Divide this Big. Int by Big. Int b and store back the result to this Big. Int * and store the remainder in r. * @param b is the divisor. * @param r is a place for remainder. */ public void div(Big. Int b, Big. Int r); } 9/25/2020 IT 179 3
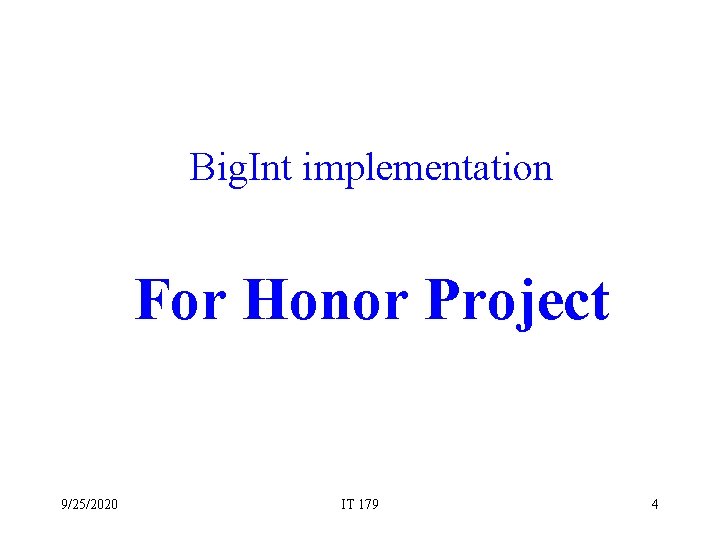
Big. Int implementation For Honor Project 9/25/2020 IT 179 4
![Inside the Array ListT Array List array 0 public int size 1 public Inside the Array. List<T> Array. List< > array [0] public int size(); [1] public](https://slidetodoc.com/presentation_image/857bfd8f0cddb17661f42f4394f8a833/image-5.jpg)
Inside the Array. List<T> Array. List< > array [0] public int size(); [1] public T get(int i); public T set(int i, T item); public int index. Of(T item); C D [4] [5] public void add(int i, T item); [6] public T remove(int i); [7] public Object[] to. Array(); [8] [9] IT 179 B [3] public void add(T item); public <K> K[] to. Array(K[] a); 9/25/2020 [2] A E F G H 5
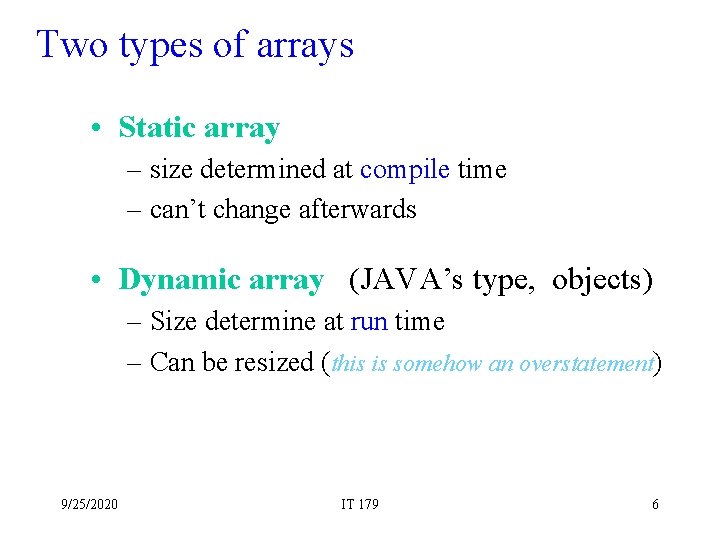
Two types of arrays • Static array – size determined at compile time – can’t change afterwards • Dynamic array (JAVA’s type, objects) – Size determine at run time – Can be resized (this is somehow an overstatement) 9/25/2020 IT 179 6
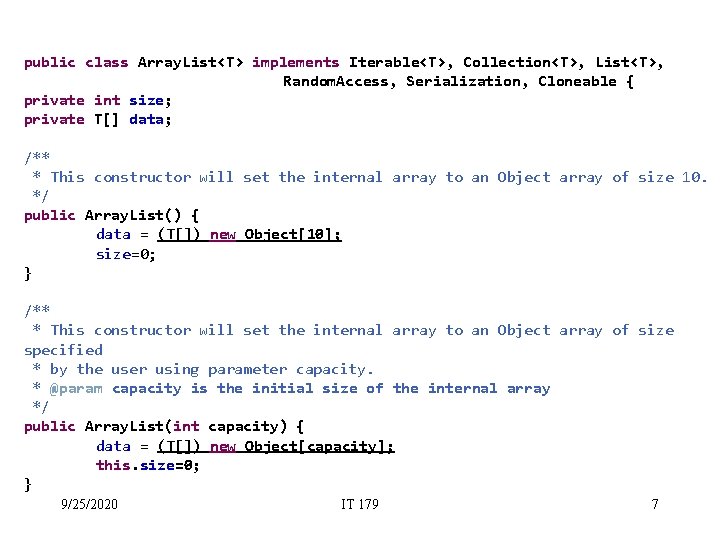
public class Array. List<T> implements Iterable<T>, Collection<T>, List<T>, Random. Access, Serialization, Cloneable { private int size; private T[] data; /** * This constructor will set the internal array to an Object array of size 10. */ public Array. List() { data = (T[]) new Object[10]; size=0; } /** * This constructor will set the internal array to an Object array of size specified * by the user using parameter capacity. * @param capacity is the initial size of the internal array */ public Array. List(int capacity) { data = (T[]) new Object[capacity]; this. size=0; } 9/25/2020 IT 179 7
![public int index OfObject item for int i0 isize i if datai equalsitem public int index. Of(Object item) { for (int i=0; i<size; i++) if (data[i]. equals(item))](https://slidetodoc.com/presentation_image/857bfd8f0cddb17661f42f4394f8a833/image-8.jpg)
public int index. Of(Object item) { for (int i=0; i<size; i++) if (data[i]. equals(item)) return i; return -1; } public Object[] to. Array() { Object[] t = new Object[size]; for (int i=0; i< size; i++) t[i] = data[i]; return t; } 9/25/2020 IT 179 8
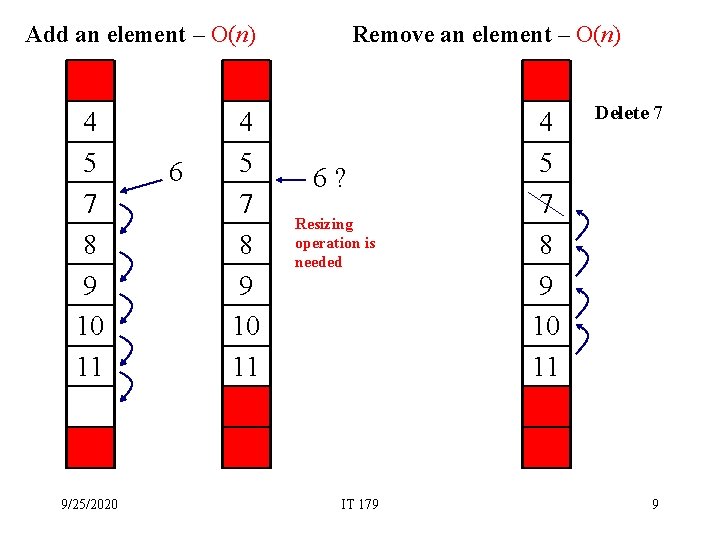
Add an element – O(n) Remove an element – O(n) 4 5 7 8 9 10 11 9/25/2020 6 4 5 7 8 9 10 11 6 ? Resizing operation is needed IT 179 4 5 7 8 9 10 11 Delete 7 9
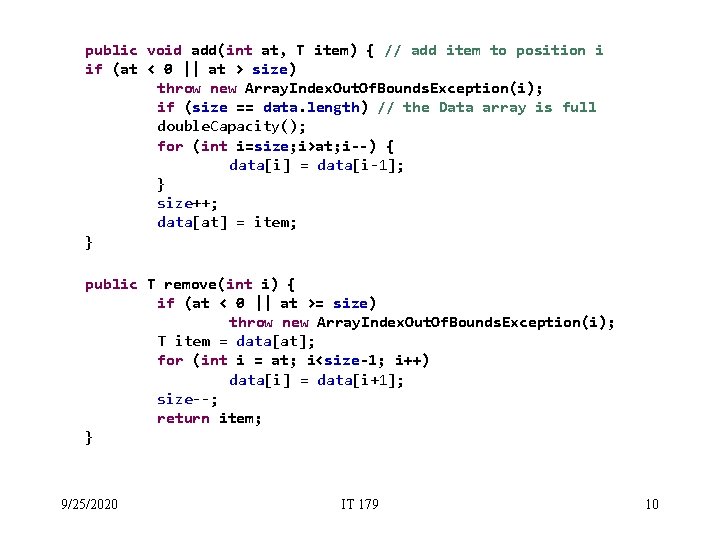
public void add(int at, T item) { // add item to position i if (at < 0 || at > size) throw new Array. Index. Out. Of. Bounds. Exception(i); if (size == data. length) // the Data array is full double. Capacity(); for (int i=size; i>at; i--) { data[i] = data[i-1]; } size++; data[at] = item; } public T remove(int i) { if (at < 0 || at >= size) throw new Array. Index. Out. Of. Bounds. Exception(i); T item = data[at]; for (int i = at; i<size-1; i++) data[i] = data[i+1]; size--; return item; } 9/25/2020 IT 179 10
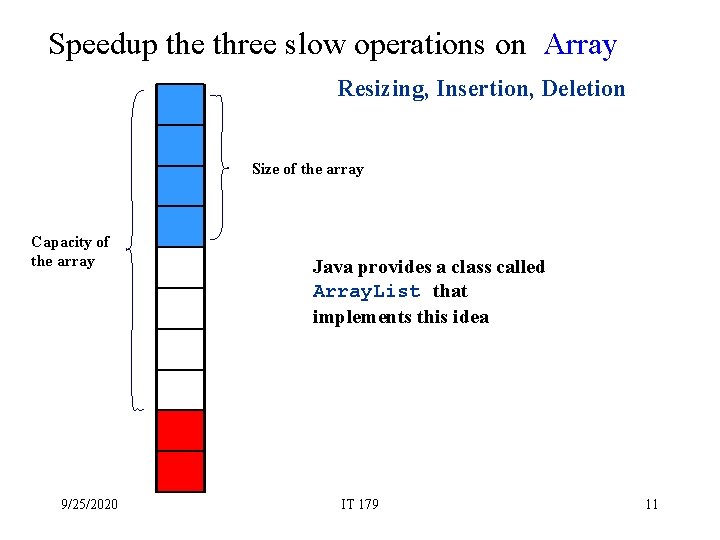
Speedup the three slow operations on Array Resizing, Insertion, Deletion Size of the array Capacity of the array 9/25/2020 Java provides a class called Array. List that implements this idea IT 179 11
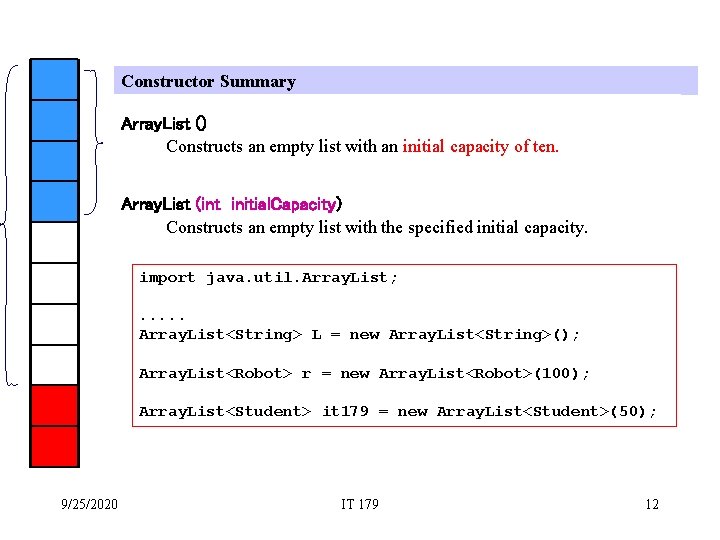
Constructor Summary Array. List () Constructs an empty list with an initial capacity of ten. Array. List (int initial. Capacity) Constructs an empty list with the specified initial capacity. import java. util. Array. List; . . . Array. List<String> L = new Array. List<String>(); Array. List<Robot> r = new Array. List<Robot>(100); Array. List<Student> it 179 = new Array. List<Student>(50); 9/25/2020 IT 179 12
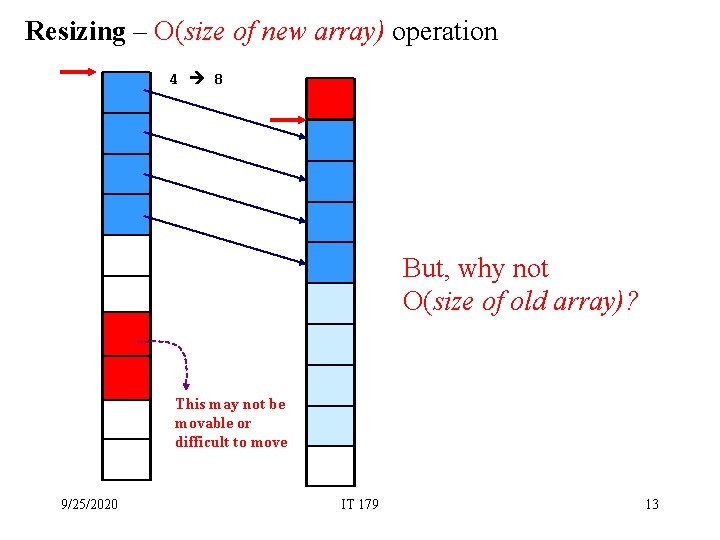
Resizing – O(size of new array) operation 4 8 But, why not O(size of old array)? This may not be movable or difficult to move 9/25/2020 IT 179 13
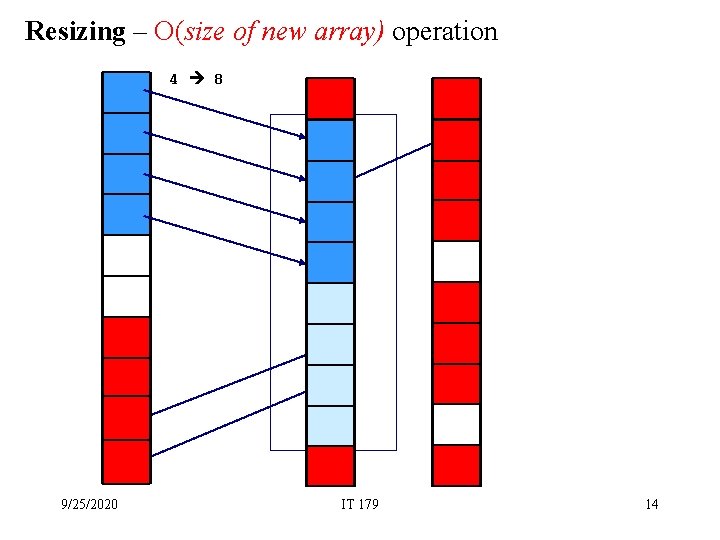
Resizing – O(size of new array) operation 4 8 9/25/2020 IT 179 14
![private void double Capacity int c data length2 T new List private void double. Capacity() { int c = data. length*2; T[] new. List =](https://slidetodoc.com/presentation_image/857bfd8f0cddb17661f42f4394f8a833/image-15.jpg)
private void double. Capacity() { int c = data. length*2; T[] new. List = (T[]) new Object[c]; for (int i =0; i< data. length; i++) new. List[i] = data[i]; // This is OK? data = new. List; } 9/25/2020 IT 179 15
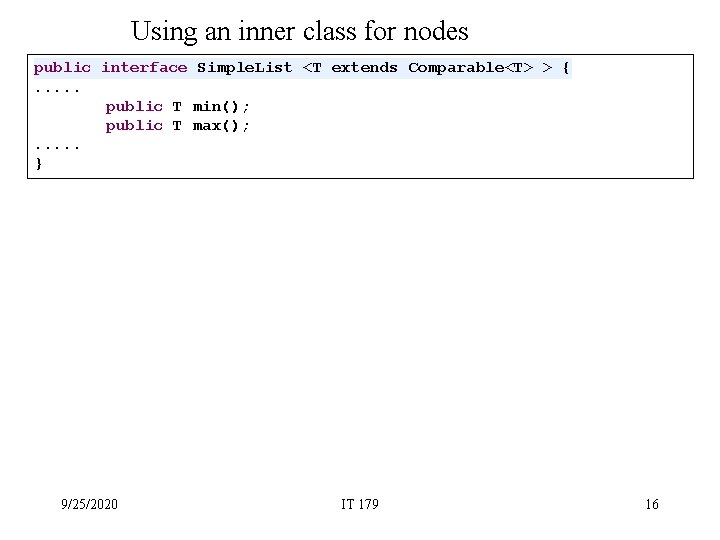
Using an inner class for nodes public interface Simple. List <T extends Comparable<T> > {. . . public T min(); public T max(); . . . } 9/25/2020 IT 179 16
Upper specification limit and lower specification limit
Upper specification limit and lower specification limit
022598 color
Trf7970
Services provided by functional view specification
Umler data specification manual
Inspire data specification
Inspire data specification
Is hyper v type 1 or type 2
Order of recruitment of muscle fiber types
Type 1 error vs type 2 error example
Type 1 error vs type 2 error example
Rock cycle
Type a and type b personality theory
Type a and type b personality theory
Myotonic dystrophy
Deflection type instruments